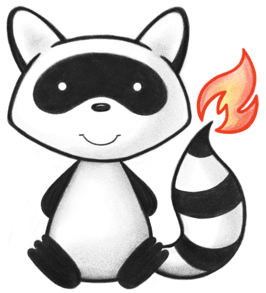
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 050 */ 051@ResourceDef(name="MessageHeader", profile="http://hl7.org/fhir/Profile/MessageHeader") 052public class MessageHeader extends DomainResource { 053 054 public enum ResponseType { 055 /** 056 * The message was accepted and processed without error. 057 */ 058 OK, 059 /** 060 * Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required. 061 */ 062 TRANSIENTERROR, 063 /** 064 * The message was rejected because of a problem with the content. There is no point in re-sending without change. The response narrative SHALL describe the issue. 065 */ 066 FATALERROR, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 public static ResponseType fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("ok".equals(codeString)) 075 return OK; 076 if ("transient-error".equals(codeString)) 077 return TRANSIENTERROR; 078 if ("fatal-error".equals(codeString)) 079 return FATALERROR; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown ResponseType code '"+codeString+"'"); 084 } 085 public String toCode() { 086 switch (this) { 087 case OK: return "ok"; 088 case TRANSIENTERROR: return "transient-error"; 089 case FATALERROR: return "fatal-error"; 090 case NULL: return null; 091 default: return "?"; 092 } 093 } 094 public String getSystem() { 095 switch (this) { 096 case OK: return "http://hl7.org/fhir/response-code"; 097 case TRANSIENTERROR: return "http://hl7.org/fhir/response-code"; 098 case FATALERROR: return "http://hl7.org/fhir/response-code"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDefinition() { 104 switch (this) { 105 case OK: return "The message was accepted and processed without error."; 106 case TRANSIENTERROR: return "Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required."; 107 case FATALERROR: return "The message was rejected because of a problem with the content. There is no point in re-sending without change. The response narrative SHALL describe the issue."; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDisplay() { 113 switch (this) { 114 case OK: return "OK"; 115 case TRANSIENTERROR: return "Transient Error"; 116 case FATALERROR: return "Fatal Error"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 } 122 123 public static class ResponseTypeEnumFactory implements EnumFactory<ResponseType> { 124 public ResponseType fromCode(String codeString) throws IllegalArgumentException { 125 if (codeString == null || "".equals(codeString)) 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("ok".equals(codeString)) 129 return ResponseType.OK; 130 if ("transient-error".equals(codeString)) 131 return ResponseType.TRANSIENTERROR; 132 if ("fatal-error".equals(codeString)) 133 return ResponseType.FATALERROR; 134 throw new IllegalArgumentException("Unknown ResponseType code '"+codeString+"'"); 135 } 136 public Enumeration<ResponseType> fromType(PrimitiveType<?> code) throws FHIRException { 137 if (code == null) 138 return null; 139 if (code.isEmpty()) 140 return new Enumeration<ResponseType>(this); 141 String codeString = code.asStringValue(); 142 if (codeString == null || "".equals(codeString)) 143 return null; 144 if ("ok".equals(codeString)) 145 return new Enumeration<ResponseType>(this, ResponseType.OK); 146 if ("transient-error".equals(codeString)) 147 return new Enumeration<ResponseType>(this, ResponseType.TRANSIENTERROR); 148 if ("fatal-error".equals(codeString)) 149 return new Enumeration<ResponseType>(this, ResponseType.FATALERROR); 150 throw new FHIRException("Unknown ResponseType code '"+codeString+"'"); 151 } 152 public String toCode(ResponseType code) { 153 if (code == ResponseType.NULL) 154 return null; 155 if (code == ResponseType.OK) 156 return "ok"; 157 if (code == ResponseType.TRANSIENTERROR) 158 return "transient-error"; 159 if (code == ResponseType.FATALERROR) 160 return "fatal-error"; 161 return "?"; 162 } 163 public String toSystem(ResponseType code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class MessageDestinationComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * Human-readable name for the target system. 172 */ 173 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 174 @Description(shortDefinition="Name of system", formalDefinition="Human-readable name for the target system." ) 175 protected StringType name; 176 177 /** 178 * Identifies the target end system in situations where the initial message transmission is to an intermediary system. 179 */ 180 @Child(name = "target", type = {Device.class}, order=2, min=0, max=1, modifier=false, summary=true) 181 @Description(shortDefinition="Particular delivery destination within the destination", formalDefinition="Identifies the target end system in situations where the initial message transmission is to an intermediary system." ) 182 protected Reference target; 183 184 /** 185 * The actual object that is the target of the reference (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 186 */ 187 protected Device targetTarget; 188 189 /** 190 * Indicates where the message should be routed to. 191 */ 192 @Child(name = "endpoint", type = {UriType.class}, order=3, min=1, max=1, modifier=false, summary=true) 193 @Description(shortDefinition="Actual destination address or id", formalDefinition="Indicates where the message should be routed to." ) 194 protected UriType endpoint; 195 196 private static final long serialVersionUID = -2097633309L; 197 198 /** 199 * Constructor 200 */ 201 public MessageDestinationComponent() { 202 super(); 203 } 204 205 /** 206 * Constructor 207 */ 208 public MessageDestinationComponent(UriType endpoint) { 209 super(); 210 this.endpoint = endpoint; 211 } 212 213 /** 214 * @return {@link #name} (Human-readable name for the target system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 215 */ 216 public StringType getNameElement() { 217 if (this.name == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create MessageDestinationComponent.name"); 220 else if (Configuration.doAutoCreate()) 221 this.name = new StringType(); // bb 222 return this.name; 223 } 224 225 public boolean hasNameElement() { 226 return this.name != null && !this.name.isEmpty(); 227 } 228 229 public boolean hasName() { 230 return this.name != null && !this.name.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #name} (Human-readable name for the target system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 235 */ 236 public MessageDestinationComponent setNameElement(StringType value) { 237 this.name = value; 238 return this; 239 } 240 241 /** 242 * @return Human-readable name for the target system. 243 */ 244 public String getName() { 245 return this.name == null ? null : this.name.getValue(); 246 } 247 248 /** 249 * @param value Human-readable name for the target system. 250 */ 251 public MessageDestinationComponent setName(String value) { 252 if (Utilities.noString(value)) 253 this.name = null; 254 else { 255 if (this.name == null) 256 this.name = new StringType(); 257 this.name.setValue(value); 258 } 259 return this; 260 } 261 262 /** 263 * @return {@link #target} (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 264 */ 265 public Reference getTarget() { 266 if (this.target == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 269 else if (Configuration.doAutoCreate()) 270 this.target = new Reference(); // cc 271 return this.target; 272 } 273 274 public boolean hasTarget() { 275 return this.target != null && !this.target.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #target} (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 280 */ 281 public MessageDestinationComponent setTarget(Reference value) { 282 this.target = value; 283 return this; 284 } 285 286 /** 287 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 288 */ 289 public Device getTargetTarget() { 290 if (this.targetTarget == null) 291 if (Configuration.errorOnAutoCreate()) 292 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 293 else if (Configuration.doAutoCreate()) 294 this.targetTarget = new Device(); // aa 295 return this.targetTarget; 296 } 297 298 /** 299 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the target end system in situations where the initial message transmission is to an intermediary system.) 300 */ 301 public MessageDestinationComponent setTargetTarget(Device value) { 302 this.targetTarget = value; 303 return this; 304 } 305 306 /** 307 * @return {@link #endpoint} (Indicates where the message should be routed to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 308 */ 309 public UriType getEndpointElement() { 310 if (this.endpoint == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create MessageDestinationComponent.endpoint"); 313 else if (Configuration.doAutoCreate()) 314 this.endpoint = new UriType(); // bb 315 return this.endpoint; 316 } 317 318 public boolean hasEndpointElement() { 319 return this.endpoint != null && !this.endpoint.isEmpty(); 320 } 321 322 public boolean hasEndpoint() { 323 return this.endpoint != null && !this.endpoint.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #endpoint} (Indicates where the message should be routed to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 328 */ 329 public MessageDestinationComponent setEndpointElement(UriType value) { 330 this.endpoint = value; 331 return this; 332 } 333 334 /** 335 * @return Indicates where the message should be routed to. 336 */ 337 public String getEndpoint() { 338 return this.endpoint == null ? null : this.endpoint.getValue(); 339 } 340 341 /** 342 * @param value Indicates where the message should be routed to. 343 */ 344 public MessageDestinationComponent setEndpoint(String value) { 345 if (this.endpoint == null) 346 this.endpoint = new UriType(); 347 this.endpoint.setValue(value); 348 return this; 349 } 350 351 protected void listChildren(List<Property> children) { 352 super.listChildren(children); 353 children.add(new Property("name", "string", "Human-readable name for the target system.", 0, 1, name)); 354 children.add(new Property("target", "Reference(Device)", "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 0, 1, target)); 355 children.add(new Property("endpoint", "uri", "Indicates where the message should be routed to.", 0, 1, endpoint)); 356 } 357 358 @Override 359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 360 switch (_hash) { 361 case 3373707: /*name*/ return new Property("name", "string", "Human-readable name for the target system.", 0, 1, name); 362 case -880905839: /*target*/ return new Property("target", "Reference(Device)", "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 0, 1, target); 363 case 1741102485: /*endpoint*/ return new Property("endpoint", "uri", "Indicates where the message should be routed to.", 0, 1, endpoint); 364 default: return super.getNamedProperty(_hash, _name, _checkValid); 365 } 366 367 } 368 369 @Override 370 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 371 switch (hash) { 372 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 373 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 374 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // UriType 375 default: return super.getProperty(hash, name, checkValid); 376 } 377 378 } 379 380 @Override 381 public Base setProperty(int hash, String name, Base value) throws FHIRException { 382 switch (hash) { 383 case 3373707: // name 384 this.name = castToString(value); // StringType 385 return value; 386 case -880905839: // target 387 this.target = castToReference(value); // Reference 388 return value; 389 case 1741102485: // endpoint 390 this.endpoint = castToUri(value); // UriType 391 return value; 392 default: return super.setProperty(hash, name, value); 393 } 394 395 } 396 397 @Override 398 public Base setProperty(String name, Base value) throws FHIRException { 399 if (name.equals("name")) { 400 this.name = castToString(value); // StringType 401 } else if (name.equals("target")) { 402 this.target = castToReference(value); // Reference 403 } else if (name.equals("endpoint")) { 404 this.endpoint = castToUri(value); // UriType 405 } else 406 return super.setProperty(name, value); 407 return value; 408 } 409 410 @Override 411 public Base makeProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case 3373707: return getNameElement(); 414 case -880905839: return getTarget(); 415 case 1741102485: return getEndpointElement(); 416 default: return super.makeProperty(hash, name); 417 } 418 419 } 420 421 @Override 422 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 423 switch (hash) { 424 case 3373707: /*name*/ return new String[] {"string"}; 425 case -880905839: /*target*/ return new String[] {"Reference"}; 426 case 1741102485: /*endpoint*/ return new String[] {"uri"}; 427 default: return super.getTypesForProperty(hash, name); 428 } 429 430 } 431 432 @Override 433 public Base addChild(String name) throws FHIRException { 434 if (name.equals("name")) { 435 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 436 } 437 else if (name.equals("target")) { 438 this.target = new Reference(); 439 return this.target; 440 } 441 else if (name.equals("endpoint")) { 442 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 443 } 444 else 445 return super.addChild(name); 446 } 447 448 public MessageDestinationComponent copy() { 449 MessageDestinationComponent dst = new MessageDestinationComponent(); 450 copyValues(dst); 451 dst.name = name == null ? null : name.copy(); 452 dst.target = target == null ? null : target.copy(); 453 dst.endpoint = endpoint == null ? null : endpoint.copy(); 454 return dst; 455 } 456 457 @Override 458 public boolean equalsDeep(Base other_) { 459 if (!super.equalsDeep(other_)) 460 return false; 461 if (!(other_ instanceof MessageDestinationComponent)) 462 return false; 463 MessageDestinationComponent o = (MessageDestinationComponent) other_; 464 return compareDeep(name, o.name, true) && compareDeep(target, o.target, true) && compareDeep(endpoint, o.endpoint, true) 465 ; 466 } 467 468 @Override 469 public boolean equalsShallow(Base other_) { 470 if (!super.equalsShallow(other_)) 471 return false; 472 if (!(other_ instanceof MessageDestinationComponent)) 473 return false; 474 MessageDestinationComponent o = (MessageDestinationComponent) other_; 475 return compareValues(name, o.name, true) && compareValues(endpoint, o.endpoint, true); 476 } 477 478 public boolean isEmpty() { 479 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, target, endpoint); 480 } 481 482 public String fhirType() { 483 return "MessageHeader.destination"; 484 485 } 486 487 } 488 489 @Block() 490 public static class MessageSourceComponent extends BackboneElement implements IBaseBackboneElement { 491 /** 492 * Human-readable name for the source system. 493 */ 494 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 495 @Description(shortDefinition="Name of system", formalDefinition="Human-readable name for the source system." ) 496 protected StringType name; 497 498 /** 499 * May include configuration or other information useful in debugging. 500 */ 501 @Child(name = "software", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 502 @Description(shortDefinition="Name of software running the system", formalDefinition="May include configuration or other information useful in debugging." ) 503 protected StringType software; 504 505 /** 506 * Can convey versions of multiple systems in situations where a message passes through multiple hands. 507 */ 508 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 509 @Description(shortDefinition="Version of software running", formalDefinition="Can convey versions of multiple systems in situations where a message passes through multiple hands." ) 510 protected StringType version; 511 512 /** 513 * An e-mail, phone, website or other contact point to use to resolve issues with message communications. 514 */ 515 @Child(name = "contact", type = {ContactPoint.class}, order=4, min=0, max=1, modifier=false, summary=true) 516 @Description(shortDefinition="Human contact for problems", formalDefinition="An e-mail, phone, website or other contact point to use to resolve issues with message communications." ) 517 protected ContactPoint contact; 518 519 /** 520 * Identifies the routing target to send acknowledgements to. 521 */ 522 @Child(name = "endpoint", type = {UriType.class}, order=5, min=1, max=1, modifier=false, summary=true) 523 @Description(shortDefinition="Actual message source address or id", formalDefinition="Identifies the routing target to send acknowledgements to." ) 524 protected UriType endpoint; 525 526 private static final long serialVersionUID = -115878196L; 527 528 /** 529 * Constructor 530 */ 531 public MessageSourceComponent() { 532 super(); 533 } 534 535 /** 536 * Constructor 537 */ 538 public MessageSourceComponent(UriType endpoint) { 539 super(); 540 this.endpoint = endpoint; 541 } 542 543 /** 544 * @return {@link #name} (Human-readable name for the source system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 545 */ 546 public StringType getNameElement() { 547 if (this.name == null) 548 if (Configuration.errorOnAutoCreate()) 549 throw new Error("Attempt to auto-create MessageSourceComponent.name"); 550 else if (Configuration.doAutoCreate()) 551 this.name = new StringType(); // bb 552 return this.name; 553 } 554 555 public boolean hasNameElement() { 556 return this.name != null && !this.name.isEmpty(); 557 } 558 559 public boolean hasName() { 560 return this.name != null && !this.name.isEmpty(); 561 } 562 563 /** 564 * @param value {@link #name} (Human-readable name for the source system.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 565 */ 566 public MessageSourceComponent setNameElement(StringType value) { 567 this.name = value; 568 return this; 569 } 570 571 /** 572 * @return Human-readable name for the source system. 573 */ 574 public String getName() { 575 return this.name == null ? null : this.name.getValue(); 576 } 577 578 /** 579 * @param value Human-readable name for the source system. 580 */ 581 public MessageSourceComponent setName(String value) { 582 if (Utilities.noString(value)) 583 this.name = null; 584 else { 585 if (this.name == null) 586 this.name = new StringType(); 587 this.name.setValue(value); 588 } 589 return this; 590 } 591 592 /** 593 * @return {@link #software} (May include configuration or other information useful in debugging.). This is the underlying object with id, value and extensions. The accessor "getSoftware" gives direct access to the value 594 */ 595 public StringType getSoftwareElement() { 596 if (this.software == null) 597 if (Configuration.errorOnAutoCreate()) 598 throw new Error("Attempt to auto-create MessageSourceComponent.software"); 599 else if (Configuration.doAutoCreate()) 600 this.software = new StringType(); // bb 601 return this.software; 602 } 603 604 public boolean hasSoftwareElement() { 605 return this.software != null && !this.software.isEmpty(); 606 } 607 608 public boolean hasSoftware() { 609 return this.software != null && !this.software.isEmpty(); 610 } 611 612 /** 613 * @param value {@link #software} (May include configuration or other information useful in debugging.). This is the underlying object with id, value and extensions. The accessor "getSoftware" gives direct access to the value 614 */ 615 public MessageSourceComponent setSoftwareElement(StringType value) { 616 this.software = value; 617 return this; 618 } 619 620 /** 621 * @return May include configuration or other information useful in debugging. 622 */ 623 public String getSoftware() { 624 return this.software == null ? null : this.software.getValue(); 625 } 626 627 /** 628 * @param value May include configuration or other information useful in debugging. 629 */ 630 public MessageSourceComponent setSoftware(String value) { 631 if (Utilities.noString(value)) 632 this.software = null; 633 else { 634 if (this.software == null) 635 this.software = new StringType(); 636 this.software.setValue(value); 637 } 638 return this; 639 } 640 641 /** 642 * @return {@link #version} (Can convey versions of multiple systems in situations where a message passes through multiple hands.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 643 */ 644 public StringType getVersionElement() { 645 if (this.version == null) 646 if (Configuration.errorOnAutoCreate()) 647 throw new Error("Attempt to auto-create MessageSourceComponent.version"); 648 else if (Configuration.doAutoCreate()) 649 this.version = new StringType(); // bb 650 return this.version; 651 } 652 653 public boolean hasVersionElement() { 654 return this.version != null && !this.version.isEmpty(); 655 } 656 657 public boolean hasVersion() { 658 return this.version != null && !this.version.isEmpty(); 659 } 660 661 /** 662 * @param value {@link #version} (Can convey versions of multiple systems in situations where a message passes through multiple hands.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 663 */ 664 public MessageSourceComponent setVersionElement(StringType value) { 665 this.version = value; 666 return this; 667 } 668 669 /** 670 * @return Can convey versions of multiple systems in situations where a message passes through multiple hands. 671 */ 672 public String getVersion() { 673 return this.version == null ? null : this.version.getValue(); 674 } 675 676 /** 677 * @param value Can convey versions of multiple systems in situations where a message passes through multiple hands. 678 */ 679 public MessageSourceComponent setVersion(String value) { 680 if (Utilities.noString(value)) 681 this.version = null; 682 else { 683 if (this.version == null) 684 this.version = new StringType(); 685 this.version.setValue(value); 686 } 687 return this; 688 } 689 690 /** 691 * @return {@link #contact} (An e-mail, phone, website or other contact point to use to resolve issues with message communications.) 692 */ 693 public ContactPoint getContact() { 694 if (this.contact == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create MessageSourceComponent.contact"); 697 else if (Configuration.doAutoCreate()) 698 this.contact = new ContactPoint(); // cc 699 return this.contact; 700 } 701 702 public boolean hasContact() { 703 return this.contact != null && !this.contact.isEmpty(); 704 } 705 706 /** 707 * @param value {@link #contact} (An e-mail, phone, website or other contact point to use to resolve issues with message communications.) 708 */ 709 public MessageSourceComponent setContact(ContactPoint value) { 710 this.contact = value; 711 return this; 712 } 713 714 /** 715 * @return {@link #endpoint} (Identifies the routing target to send acknowledgements to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 716 */ 717 public UriType getEndpointElement() { 718 if (this.endpoint == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create MessageSourceComponent.endpoint"); 721 else if (Configuration.doAutoCreate()) 722 this.endpoint = new UriType(); // bb 723 return this.endpoint; 724 } 725 726 public boolean hasEndpointElement() { 727 return this.endpoint != null && !this.endpoint.isEmpty(); 728 } 729 730 public boolean hasEndpoint() { 731 return this.endpoint != null && !this.endpoint.isEmpty(); 732 } 733 734 /** 735 * @param value {@link #endpoint} (Identifies the routing target to send acknowledgements to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 736 */ 737 public MessageSourceComponent setEndpointElement(UriType value) { 738 this.endpoint = value; 739 return this; 740 } 741 742 /** 743 * @return Identifies the routing target to send acknowledgements to. 744 */ 745 public String getEndpoint() { 746 return this.endpoint == null ? null : this.endpoint.getValue(); 747 } 748 749 /** 750 * @param value Identifies the routing target to send acknowledgements to. 751 */ 752 public MessageSourceComponent setEndpoint(String value) { 753 if (this.endpoint == null) 754 this.endpoint = new UriType(); 755 this.endpoint.setValue(value); 756 return this; 757 } 758 759 protected void listChildren(List<Property> children) { 760 super.listChildren(children); 761 children.add(new Property("name", "string", "Human-readable name for the source system.", 0, 1, name)); 762 children.add(new Property("software", "string", "May include configuration or other information useful in debugging.", 0, 1, software)); 763 children.add(new Property("version", "string", "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1, version)); 764 children.add(new Property("contact", "ContactPoint", "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 1, contact)); 765 children.add(new Property("endpoint", "uri", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint)); 766 } 767 768 @Override 769 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 770 switch (_hash) { 771 case 3373707: /*name*/ return new Property("name", "string", "Human-readable name for the source system.", 0, 1, name); 772 case 1319330215: /*software*/ return new Property("software", "string", "May include configuration or other information useful in debugging.", 0, 1, software); 773 case 351608024: /*version*/ return new Property("version", "string", "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1, version); 774 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 1, contact); 775 case 1741102485: /*endpoint*/ return new Property("endpoint", "uri", "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 776 default: return super.getNamedProperty(_hash, _name, _checkValid); 777 } 778 779 } 780 781 @Override 782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 783 switch (hash) { 784 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 785 case 1319330215: /*software*/ return this.software == null ? new Base[0] : new Base[] {this.software}; // StringType 786 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 787 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : new Base[] {this.contact}; // ContactPoint 788 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // UriType 789 default: return super.getProperty(hash, name, checkValid); 790 } 791 792 } 793 794 @Override 795 public Base setProperty(int hash, String name, Base value) throws FHIRException { 796 switch (hash) { 797 case 3373707: // name 798 this.name = castToString(value); // StringType 799 return value; 800 case 1319330215: // software 801 this.software = castToString(value); // StringType 802 return value; 803 case 351608024: // version 804 this.version = castToString(value); // StringType 805 return value; 806 case 951526432: // contact 807 this.contact = castToContactPoint(value); // ContactPoint 808 return value; 809 case 1741102485: // endpoint 810 this.endpoint = castToUri(value); // UriType 811 return value; 812 default: return super.setProperty(hash, name, value); 813 } 814 815 } 816 817 @Override 818 public Base setProperty(String name, Base value) throws FHIRException { 819 if (name.equals("name")) { 820 this.name = castToString(value); // StringType 821 } else if (name.equals("software")) { 822 this.software = castToString(value); // StringType 823 } else if (name.equals("version")) { 824 this.version = castToString(value); // StringType 825 } else if (name.equals("contact")) { 826 this.contact = castToContactPoint(value); // ContactPoint 827 } else if (name.equals("endpoint")) { 828 this.endpoint = castToUri(value); // UriType 829 } else 830 return super.setProperty(name, value); 831 return value; 832 } 833 834 @Override 835 public Base makeProperty(int hash, String name) throws FHIRException { 836 switch (hash) { 837 case 3373707: return getNameElement(); 838 case 1319330215: return getSoftwareElement(); 839 case 351608024: return getVersionElement(); 840 case 951526432: return getContact(); 841 case 1741102485: return getEndpointElement(); 842 default: return super.makeProperty(hash, name); 843 } 844 845 } 846 847 @Override 848 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 849 switch (hash) { 850 case 3373707: /*name*/ return new String[] {"string"}; 851 case 1319330215: /*software*/ return new String[] {"string"}; 852 case 351608024: /*version*/ return new String[] {"string"}; 853 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 854 case 1741102485: /*endpoint*/ return new String[] {"uri"}; 855 default: return super.getTypesForProperty(hash, name); 856 } 857 858 } 859 860 @Override 861 public Base addChild(String name) throws FHIRException { 862 if (name.equals("name")) { 863 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 864 } 865 else if (name.equals("software")) { 866 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.software"); 867 } 868 else if (name.equals("version")) { 869 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.version"); 870 } 871 else if (name.equals("contact")) { 872 this.contact = new ContactPoint(); 873 return this.contact; 874 } 875 else if (name.equals("endpoint")) { 876 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 877 } 878 else 879 return super.addChild(name); 880 } 881 882 public MessageSourceComponent copy() { 883 MessageSourceComponent dst = new MessageSourceComponent(); 884 copyValues(dst); 885 dst.name = name == null ? null : name.copy(); 886 dst.software = software == null ? null : software.copy(); 887 dst.version = version == null ? null : version.copy(); 888 dst.contact = contact == null ? null : contact.copy(); 889 dst.endpoint = endpoint == null ? null : endpoint.copy(); 890 return dst; 891 } 892 893 @Override 894 public boolean equalsDeep(Base other_) { 895 if (!super.equalsDeep(other_)) 896 return false; 897 if (!(other_ instanceof MessageSourceComponent)) 898 return false; 899 MessageSourceComponent o = (MessageSourceComponent) other_; 900 return compareDeep(name, o.name, true) && compareDeep(software, o.software, true) && compareDeep(version, o.version, true) 901 && compareDeep(contact, o.contact, true) && compareDeep(endpoint, o.endpoint, true); 902 } 903 904 @Override 905 public boolean equalsShallow(Base other_) { 906 if (!super.equalsShallow(other_)) 907 return false; 908 if (!(other_ instanceof MessageSourceComponent)) 909 return false; 910 MessageSourceComponent o = (MessageSourceComponent) other_; 911 return compareValues(name, o.name, true) && compareValues(software, o.software, true) && compareValues(version, o.version, true) 912 && compareValues(endpoint, o.endpoint, true); 913 } 914 915 public boolean isEmpty() { 916 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, software, version 917 , contact, endpoint); 918 } 919 920 public String fhirType() { 921 return "MessageHeader.source"; 922 923 } 924 925 } 926 927 @Block() 928 public static class MessageHeaderResponseComponent extends BackboneElement implements IBaseBackboneElement { 929 /** 930 * The MessageHeader.id of the message to which this message is a response. 931 */ 932 @Child(name = "identifier", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 933 @Description(shortDefinition="Id of original message", formalDefinition="The MessageHeader.id of the message to which this message is a response." ) 934 protected IdType identifier; 935 936 /** 937 * Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 938 */ 939 @Child(name = "code", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 940 @Description(shortDefinition="ok | transient-error | fatal-error", formalDefinition="Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/response-code") 942 protected Enumeration<ResponseType> code; 943 944 /** 945 * Full details of any issues found in the message. 946 */ 947 @Child(name = "details", type = {OperationOutcome.class}, order=3, min=0, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="Specific list of hints/warnings/errors", formalDefinition="Full details of any issues found in the message." ) 949 protected Reference details; 950 951 /** 952 * The actual object that is the target of the reference (Full details of any issues found in the message.) 953 */ 954 protected OperationOutcome detailsTarget; 955 956 private static final long serialVersionUID = -1008716838L; 957 958 /** 959 * Constructor 960 */ 961 public MessageHeaderResponseComponent() { 962 super(); 963 } 964 965 /** 966 * Constructor 967 */ 968 public MessageHeaderResponseComponent(IdType identifier, Enumeration<ResponseType> code) { 969 super(); 970 this.identifier = identifier; 971 this.code = code; 972 } 973 974 /** 975 * @return {@link #identifier} (The MessageHeader.id of the message to which this message is a response.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 976 */ 977 public IdType getIdentifierElement() { 978 if (this.identifier == null) 979 if (Configuration.errorOnAutoCreate()) 980 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.identifier"); 981 else if (Configuration.doAutoCreate()) 982 this.identifier = new IdType(); // bb 983 return this.identifier; 984 } 985 986 public boolean hasIdentifierElement() { 987 return this.identifier != null && !this.identifier.isEmpty(); 988 } 989 990 public boolean hasIdentifier() { 991 return this.identifier != null && !this.identifier.isEmpty(); 992 } 993 994 /** 995 * @param value {@link #identifier} (The MessageHeader.id of the message to which this message is a response.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 996 */ 997 public MessageHeaderResponseComponent setIdentifierElement(IdType value) { 998 this.identifier = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return The MessageHeader.id of the message to which this message is a response. 1004 */ 1005 public String getIdentifier() { 1006 return this.identifier == null ? null : this.identifier.getValue(); 1007 } 1008 1009 /** 1010 * @param value The MessageHeader.id of the message to which this message is a response. 1011 */ 1012 public MessageHeaderResponseComponent setIdentifier(String value) { 1013 if (this.identifier == null) 1014 this.identifier = new IdType(); 1015 this.identifier.setValue(value); 1016 return this; 1017 } 1018 1019 /** 1020 * @return {@link #code} (Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1021 */ 1022 public Enumeration<ResponseType> getCodeElement() { 1023 if (this.code == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.code"); 1026 else if (Configuration.doAutoCreate()) 1027 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); // bb 1028 return this.code; 1029 } 1030 1031 public boolean hasCodeElement() { 1032 return this.code != null && !this.code.isEmpty(); 1033 } 1034 1035 public boolean hasCode() { 1036 return this.code != null && !this.code.isEmpty(); 1037 } 1038 1039 /** 1040 * @param value {@link #code} (Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1041 */ 1042 public MessageHeaderResponseComponent setCodeElement(Enumeration<ResponseType> value) { 1043 this.code = value; 1044 return this; 1045 } 1046 1047 /** 1048 * @return Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 1049 */ 1050 public ResponseType getCode() { 1051 return this.code == null ? null : this.code.getValue(); 1052 } 1053 1054 /** 1055 * @param value Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not. 1056 */ 1057 public MessageHeaderResponseComponent setCode(ResponseType value) { 1058 if (this.code == null) 1059 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); 1060 this.code.setValue(value); 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #details} (Full details of any issues found in the message.) 1066 */ 1067 public Reference getDetails() { 1068 if (this.details == null) 1069 if (Configuration.errorOnAutoCreate()) 1070 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 1071 else if (Configuration.doAutoCreate()) 1072 this.details = new Reference(); // cc 1073 return this.details; 1074 } 1075 1076 public boolean hasDetails() { 1077 return this.details != null && !this.details.isEmpty(); 1078 } 1079 1080 /** 1081 * @param value {@link #details} (Full details of any issues found in the message.) 1082 */ 1083 public MessageHeaderResponseComponent setDetails(Reference value) { 1084 this.details = value; 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #details} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Full details of any issues found in the message.) 1090 */ 1091 public OperationOutcome getDetailsTarget() { 1092 if (this.detailsTarget == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 1095 else if (Configuration.doAutoCreate()) 1096 this.detailsTarget = new OperationOutcome(); // aa 1097 return this.detailsTarget; 1098 } 1099 1100 /** 1101 * @param value {@link #details} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Full details of any issues found in the message.) 1102 */ 1103 public MessageHeaderResponseComponent setDetailsTarget(OperationOutcome value) { 1104 this.detailsTarget = value; 1105 return this; 1106 } 1107 1108 protected void listChildren(List<Property> children) { 1109 super.listChildren(children); 1110 children.add(new Property("identifier", "id", "The MessageHeader.id of the message to which this message is a response.", 0, 1, identifier)); 1111 children.add(new Property("code", "code", "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 0, 1, code)); 1112 children.add(new Property("details", "Reference(OperationOutcome)", "Full details of any issues found in the message.", 0, 1, details)); 1113 } 1114 1115 @Override 1116 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1117 switch (_hash) { 1118 case -1618432855: /*identifier*/ return new Property("identifier", "id", "The MessageHeader.id of the message to which this message is a response.", 0, 1, identifier); 1119 case 3059181: /*code*/ return new Property("code", "code", "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 0, 1, code); 1120 case 1557721666: /*details*/ return new Property("details", "Reference(OperationOutcome)", "Full details of any issues found in the message.", 0, 1, details); 1121 default: return super.getNamedProperty(_hash, _name, _checkValid); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1128 switch (hash) { 1129 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // IdType 1130 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<ResponseType> 1131 case 1557721666: /*details*/ return this.details == null ? new Base[0] : new Base[] {this.details}; // Reference 1132 default: return super.getProperty(hash, name, checkValid); 1133 } 1134 1135 } 1136 1137 @Override 1138 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1139 switch (hash) { 1140 case -1618432855: // identifier 1141 this.identifier = castToId(value); // IdType 1142 return value; 1143 case 3059181: // code 1144 value = new ResponseTypeEnumFactory().fromType(castToCode(value)); 1145 this.code = (Enumeration) value; // Enumeration<ResponseType> 1146 return value; 1147 case 1557721666: // details 1148 this.details = castToReference(value); // Reference 1149 return value; 1150 default: return super.setProperty(hash, name, value); 1151 } 1152 1153 } 1154 1155 @Override 1156 public Base setProperty(String name, Base value) throws FHIRException { 1157 if (name.equals("identifier")) { 1158 this.identifier = castToId(value); // IdType 1159 } else if (name.equals("code")) { 1160 value = new ResponseTypeEnumFactory().fromType(castToCode(value)); 1161 this.code = (Enumeration) value; // Enumeration<ResponseType> 1162 } else if (name.equals("details")) { 1163 this.details = castToReference(value); // Reference 1164 } else 1165 return super.setProperty(name, value); 1166 return value; 1167 } 1168 1169 @Override 1170 public Base makeProperty(int hash, String name) throws FHIRException { 1171 switch (hash) { 1172 case -1618432855: return getIdentifierElement(); 1173 case 3059181: return getCodeElement(); 1174 case 1557721666: return getDetails(); 1175 default: return super.makeProperty(hash, name); 1176 } 1177 1178 } 1179 1180 @Override 1181 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1182 switch (hash) { 1183 case -1618432855: /*identifier*/ return new String[] {"id"}; 1184 case 3059181: /*code*/ return new String[] {"code"}; 1185 case 1557721666: /*details*/ return new String[] {"Reference"}; 1186 default: return super.getTypesForProperty(hash, name); 1187 } 1188 1189 } 1190 1191 @Override 1192 public Base addChild(String name) throws FHIRException { 1193 if (name.equals("identifier")) { 1194 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.identifier"); 1195 } 1196 else if (name.equals("code")) { 1197 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.code"); 1198 } 1199 else if (name.equals("details")) { 1200 this.details = new Reference(); 1201 return this.details; 1202 } 1203 else 1204 return super.addChild(name); 1205 } 1206 1207 public MessageHeaderResponseComponent copy() { 1208 MessageHeaderResponseComponent dst = new MessageHeaderResponseComponent(); 1209 copyValues(dst); 1210 dst.identifier = identifier == null ? null : identifier.copy(); 1211 dst.code = code == null ? null : code.copy(); 1212 dst.details = details == null ? null : details.copy(); 1213 return dst; 1214 } 1215 1216 @Override 1217 public boolean equalsDeep(Base other_) { 1218 if (!super.equalsDeep(other_)) 1219 return false; 1220 if (!(other_ instanceof MessageHeaderResponseComponent)) 1221 return false; 1222 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1223 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(details, o.details, true) 1224 ; 1225 } 1226 1227 @Override 1228 public boolean equalsShallow(Base other_) { 1229 if (!super.equalsShallow(other_)) 1230 return false; 1231 if (!(other_ instanceof MessageHeaderResponseComponent)) 1232 return false; 1233 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1234 return compareValues(identifier, o.identifier, true) && compareValues(code, o.code, true); 1235 } 1236 1237 public boolean isEmpty() { 1238 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, details 1239 ); 1240 } 1241 1242 public String fhirType() { 1243 return "MessageHeader.response"; 1244 1245 } 1246 1247 } 1248 1249 /** 1250 * Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events". 1251 */ 1252 @Child(name = "event", type = {Coding.class}, order=0, min=1, max=1, modifier=false, summary=true) 1253 @Description(shortDefinition="Code for the event this message represents", formalDefinition="Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://hl7.org/fhir/message-events\"." ) 1254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-events") 1255 protected Coding event; 1256 1257 /** 1258 * The destination application which the message is intended for. 1259 */ 1260 @Child(name = "destination", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1261 @Description(shortDefinition="Message destination application(s)", formalDefinition="The destination application which the message is intended for." ) 1262 protected List<MessageDestinationComponent> destination; 1263 1264 /** 1265 * Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient. 1266 */ 1267 @Child(name = "receiver", type = {Practitioner.class, Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 1268 @Description(shortDefinition="Intended \"real-world\" recipient for the data", formalDefinition="Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient." ) 1269 protected Reference receiver; 1270 1271 /** 1272 * The actual object that is the target of the reference (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 1273 */ 1274 protected Resource receiverTarget; 1275 1276 /** 1277 * Identifies the sending system to allow the use of a trust relationship. 1278 */ 1279 @Child(name = "sender", type = {Practitioner.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 1280 @Description(shortDefinition="Real world sender of the message", formalDefinition="Identifies the sending system to allow the use of a trust relationship." ) 1281 protected Reference sender; 1282 1283 /** 1284 * The actual object that is the target of the reference (Identifies the sending system to allow the use of a trust relationship.) 1285 */ 1286 protected Resource senderTarget; 1287 1288 /** 1289 * The time that the message was sent. 1290 */ 1291 @Child(name = "timestamp", type = {InstantType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1292 @Description(shortDefinition="Time that the message was sent", formalDefinition="The time that the message was sent." ) 1293 protected InstantType timestamp; 1294 1295 /** 1296 * The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions. 1297 */ 1298 @Child(name = "enterer", type = {Practitioner.class}, order=5, min=0, max=1, modifier=false, summary=true) 1299 @Description(shortDefinition="The source of the data entry", formalDefinition="The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions." ) 1300 protected Reference enterer; 1301 1302 /** 1303 * The actual object that is the target of the reference (The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) 1304 */ 1305 protected Practitioner entererTarget; 1306 1307 /** 1308 * The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions. 1309 */ 1310 @Child(name = "author", type = {Practitioner.class}, order=6, min=0, max=1, modifier=false, summary=true) 1311 @Description(shortDefinition="The source of the decision", formalDefinition="The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions." ) 1312 protected Reference author; 1313 1314 /** 1315 * The actual object that is the target of the reference (The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1316 */ 1317 protected Practitioner authorTarget; 1318 1319 /** 1320 * The source application from which this message originated. 1321 */ 1322 @Child(name = "source", type = {}, order=7, min=1, max=1, modifier=false, summary=true) 1323 @Description(shortDefinition="Message source application", formalDefinition="The source application from which this message originated." ) 1324 protected MessageSourceComponent source; 1325 1326 /** 1327 * The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party. 1328 */ 1329 @Child(name = "responsible", type = {Practitioner.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=true) 1330 @Description(shortDefinition="Final responsibility for event", formalDefinition="The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party." ) 1331 protected Reference responsible; 1332 1333 /** 1334 * The actual object that is the target of the reference (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1335 */ 1336 protected Resource responsibleTarget; 1337 1338 /** 1339 * Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message. 1340 */ 1341 @Child(name = "reason", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 1342 @Description(shortDefinition="Cause of event", formalDefinition="Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message." ) 1343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-reason-encounter") 1344 protected CodeableConcept reason; 1345 1346 /** 1347 * Information about the message that this message is a response to. Only present if this message is a response. 1348 */ 1349 @Child(name = "response", type = {}, order=10, min=0, max=1, modifier=false, summary=true) 1350 @Description(shortDefinition="If this is a reply to prior message", formalDefinition="Information about the message that this message is a response to. Only present if this message is a response." ) 1351 protected MessageHeaderResponseComponent response; 1352 1353 /** 1354 * The actual data of the message - a reference to the root/focus class of the event. 1355 */ 1356 @Child(name = "focus", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1357 @Description(shortDefinition="The actual content of the message", formalDefinition="The actual data of the message - a reference to the root/focus class of the event." ) 1358 protected List<Reference> focus; 1359 /** 1360 * The actual objects that are the target of the reference (The actual data of the message - a reference to the root/focus class of the event.) 1361 */ 1362 protected List<Resource> focusTarget; 1363 1364 1365 private static final long serialVersionUID = 1142547869L; 1366 1367 /** 1368 * Constructor 1369 */ 1370 public MessageHeader() { 1371 super(); 1372 } 1373 1374 /** 1375 * Constructor 1376 */ 1377 public MessageHeader(Coding event, InstantType timestamp, MessageSourceComponent source) { 1378 super(); 1379 this.event = event; 1380 this.timestamp = timestamp; 1381 this.source = source; 1382 } 1383 1384 /** 1385 * @return {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events".) 1386 */ 1387 public Coding getEvent() { 1388 if (this.event == null) 1389 if (Configuration.errorOnAutoCreate()) 1390 throw new Error("Attempt to auto-create MessageHeader.event"); 1391 else if (Configuration.doAutoCreate()) 1392 this.event = new Coding(); // cc 1393 return this.event; 1394 } 1395 1396 public boolean hasEvent() { 1397 return this.event != null && !this.event.isEmpty(); 1398 } 1399 1400 /** 1401 * @param value {@link #event} (Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value "http://hl7.org/fhir/message-events".) 1402 */ 1403 public MessageHeader setEvent(Coding value) { 1404 this.event = value; 1405 return this; 1406 } 1407 1408 /** 1409 * @return {@link #destination} (The destination application which the message is intended for.) 1410 */ 1411 public List<MessageDestinationComponent> getDestination() { 1412 if (this.destination == null) 1413 this.destination = new ArrayList<MessageDestinationComponent>(); 1414 return this.destination; 1415 } 1416 1417 /** 1418 * @return Returns a reference to <code>this</code> for easy method chaining 1419 */ 1420 public MessageHeader setDestination(List<MessageDestinationComponent> theDestination) { 1421 this.destination = theDestination; 1422 return this; 1423 } 1424 1425 public boolean hasDestination() { 1426 if (this.destination == null) 1427 return false; 1428 for (MessageDestinationComponent item : this.destination) 1429 if (!item.isEmpty()) 1430 return true; 1431 return false; 1432 } 1433 1434 public MessageDestinationComponent addDestination() { //3 1435 MessageDestinationComponent t = new MessageDestinationComponent(); 1436 if (this.destination == null) 1437 this.destination = new ArrayList<MessageDestinationComponent>(); 1438 this.destination.add(t); 1439 return t; 1440 } 1441 1442 public MessageHeader addDestination(MessageDestinationComponent t) { //3 1443 if (t == null) 1444 return this; 1445 if (this.destination == null) 1446 this.destination = new ArrayList<MessageDestinationComponent>(); 1447 this.destination.add(t); 1448 return this; 1449 } 1450 1451 /** 1452 * @return The first repetition of repeating field {@link #destination}, creating it if it does not already exist 1453 */ 1454 public MessageDestinationComponent getDestinationFirstRep() { 1455 if (getDestination().isEmpty()) { 1456 addDestination(); 1457 } 1458 return getDestination().get(0); 1459 } 1460 1461 /** 1462 * @return {@link #receiver} (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 1463 */ 1464 public Reference getReceiver() { 1465 if (this.receiver == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create MessageHeader.receiver"); 1468 else if (Configuration.doAutoCreate()) 1469 this.receiver = new Reference(); // cc 1470 return this.receiver; 1471 } 1472 1473 public boolean hasReceiver() { 1474 return this.receiver != null && !this.receiver.isEmpty(); 1475 } 1476 1477 /** 1478 * @param value {@link #receiver} (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 1479 */ 1480 public MessageHeader setReceiver(Reference value) { 1481 this.receiver = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #receiver} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 1487 */ 1488 public Resource getReceiverTarget() { 1489 return this.receiverTarget; 1490 } 1491 1492 /** 1493 * @param value {@link #receiver} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.) 1494 */ 1495 public MessageHeader setReceiverTarget(Resource value) { 1496 this.receiverTarget = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #sender} (Identifies the sending system to allow the use of a trust relationship.) 1502 */ 1503 public Reference getSender() { 1504 if (this.sender == null) 1505 if (Configuration.errorOnAutoCreate()) 1506 throw new Error("Attempt to auto-create MessageHeader.sender"); 1507 else if (Configuration.doAutoCreate()) 1508 this.sender = new Reference(); // cc 1509 return this.sender; 1510 } 1511 1512 public boolean hasSender() { 1513 return this.sender != null && !this.sender.isEmpty(); 1514 } 1515 1516 /** 1517 * @param value {@link #sender} (Identifies the sending system to allow the use of a trust relationship.) 1518 */ 1519 public MessageHeader setSender(Reference value) { 1520 this.sender = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #sender} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the sending system to allow the use of a trust relationship.) 1526 */ 1527 public Resource getSenderTarget() { 1528 return this.senderTarget; 1529 } 1530 1531 /** 1532 * @param value {@link #sender} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the sending system to allow the use of a trust relationship.) 1533 */ 1534 public MessageHeader setSenderTarget(Resource value) { 1535 this.senderTarget = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #timestamp} (The time that the message was sent.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 1541 */ 1542 public InstantType getTimestampElement() { 1543 if (this.timestamp == null) 1544 if (Configuration.errorOnAutoCreate()) 1545 throw new Error("Attempt to auto-create MessageHeader.timestamp"); 1546 else if (Configuration.doAutoCreate()) 1547 this.timestamp = new InstantType(); // bb 1548 return this.timestamp; 1549 } 1550 1551 public boolean hasTimestampElement() { 1552 return this.timestamp != null && !this.timestamp.isEmpty(); 1553 } 1554 1555 public boolean hasTimestamp() { 1556 return this.timestamp != null && !this.timestamp.isEmpty(); 1557 } 1558 1559 /** 1560 * @param value {@link #timestamp} (The time that the message was sent.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 1561 */ 1562 public MessageHeader setTimestampElement(InstantType value) { 1563 this.timestamp = value; 1564 return this; 1565 } 1566 1567 /** 1568 * @return The time that the message was sent. 1569 */ 1570 public Date getTimestamp() { 1571 return this.timestamp == null ? null : this.timestamp.getValue(); 1572 } 1573 1574 /** 1575 * @param value The time that the message was sent. 1576 */ 1577 public MessageHeader setTimestamp(Date value) { 1578 if (this.timestamp == null) 1579 this.timestamp = new InstantType(); 1580 this.timestamp.setValue(value); 1581 return this; 1582 } 1583 1584 /** 1585 * @return {@link #enterer} (The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) 1586 */ 1587 public Reference getEnterer() { 1588 if (this.enterer == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create MessageHeader.enterer"); 1591 else if (Configuration.doAutoCreate()) 1592 this.enterer = new Reference(); // cc 1593 return this.enterer; 1594 } 1595 1596 public boolean hasEnterer() { 1597 return this.enterer != null && !this.enterer.isEmpty(); 1598 } 1599 1600 /** 1601 * @param value {@link #enterer} (The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) 1602 */ 1603 public MessageHeader setEnterer(Reference value) { 1604 this.enterer = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) 1610 */ 1611 public Practitioner getEntererTarget() { 1612 if (this.entererTarget == null) 1613 if (Configuration.errorOnAutoCreate()) 1614 throw new Error("Attempt to auto-create MessageHeader.enterer"); 1615 else if (Configuration.doAutoCreate()) 1616 this.entererTarget = new Practitioner(); // aa 1617 return this.entererTarget; 1618 } 1619 1620 /** 1621 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.) 1622 */ 1623 public MessageHeader setEntererTarget(Practitioner value) { 1624 this.entererTarget = value; 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #author} (The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1630 */ 1631 public Reference getAuthor() { 1632 if (this.author == null) 1633 if (Configuration.errorOnAutoCreate()) 1634 throw new Error("Attempt to auto-create MessageHeader.author"); 1635 else if (Configuration.doAutoCreate()) 1636 this.author = new Reference(); // cc 1637 return this.author; 1638 } 1639 1640 public boolean hasAuthor() { 1641 return this.author != null && !this.author.isEmpty(); 1642 } 1643 1644 /** 1645 * @param value {@link #author} (The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1646 */ 1647 public MessageHeader setAuthor(Reference value) { 1648 this.author = value; 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1654 */ 1655 public Practitioner getAuthorTarget() { 1656 if (this.authorTarget == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error("Attempt to auto-create MessageHeader.author"); 1659 else if (Configuration.doAutoCreate()) 1660 this.authorTarget = new Practitioner(); // aa 1661 return this.authorTarget; 1662 } 1663 1664 /** 1665 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.) 1666 */ 1667 public MessageHeader setAuthorTarget(Practitioner value) { 1668 this.authorTarget = value; 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #source} (The source application from which this message originated.) 1674 */ 1675 public MessageSourceComponent getSource() { 1676 if (this.source == null) 1677 if (Configuration.errorOnAutoCreate()) 1678 throw new Error("Attempt to auto-create MessageHeader.source"); 1679 else if (Configuration.doAutoCreate()) 1680 this.source = new MessageSourceComponent(); // cc 1681 return this.source; 1682 } 1683 1684 public boolean hasSource() { 1685 return this.source != null && !this.source.isEmpty(); 1686 } 1687 1688 /** 1689 * @param value {@link #source} (The source application from which this message originated.) 1690 */ 1691 public MessageHeader setSource(MessageSourceComponent value) { 1692 this.source = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #responsible} (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1698 */ 1699 public Reference getResponsible() { 1700 if (this.responsible == null) 1701 if (Configuration.errorOnAutoCreate()) 1702 throw new Error("Attempt to auto-create MessageHeader.responsible"); 1703 else if (Configuration.doAutoCreate()) 1704 this.responsible = new Reference(); // cc 1705 return this.responsible; 1706 } 1707 1708 public boolean hasResponsible() { 1709 return this.responsible != null && !this.responsible.isEmpty(); 1710 } 1711 1712 /** 1713 * @param value {@link #responsible} (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1714 */ 1715 public MessageHeader setResponsible(Reference value) { 1716 this.responsible = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return {@link #responsible} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1722 */ 1723 public Resource getResponsibleTarget() { 1724 return this.responsibleTarget; 1725 } 1726 1727 /** 1728 * @param value {@link #responsible} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.) 1729 */ 1730 public MessageHeader setResponsibleTarget(Resource value) { 1731 this.responsibleTarget = value; 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #reason} (Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.) 1737 */ 1738 public CodeableConcept getReason() { 1739 if (this.reason == null) 1740 if (Configuration.errorOnAutoCreate()) 1741 throw new Error("Attempt to auto-create MessageHeader.reason"); 1742 else if (Configuration.doAutoCreate()) 1743 this.reason = new CodeableConcept(); // cc 1744 return this.reason; 1745 } 1746 1747 public boolean hasReason() { 1748 return this.reason != null && !this.reason.isEmpty(); 1749 } 1750 1751 /** 1752 * @param value {@link #reason} (Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.) 1753 */ 1754 public MessageHeader setReason(CodeableConcept value) { 1755 this.reason = value; 1756 return this; 1757 } 1758 1759 /** 1760 * @return {@link #response} (Information about the message that this message is a response to. Only present if this message is a response.) 1761 */ 1762 public MessageHeaderResponseComponent getResponse() { 1763 if (this.response == null) 1764 if (Configuration.errorOnAutoCreate()) 1765 throw new Error("Attempt to auto-create MessageHeader.response"); 1766 else if (Configuration.doAutoCreate()) 1767 this.response = new MessageHeaderResponseComponent(); // cc 1768 return this.response; 1769 } 1770 1771 public boolean hasResponse() { 1772 return this.response != null && !this.response.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #response} (Information about the message that this message is a response to. Only present if this message is a response.) 1777 */ 1778 public MessageHeader setResponse(MessageHeaderResponseComponent value) { 1779 this.response = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return {@link #focus} (The actual data of the message - a reference to the root/focus class of the event.) 1785 */ 1786 public List<Reference> getFocus() { 1787 if (this.focus == null) 1788 this.focus = new ArrayList<Reference>(); 1789 return this.focus; 1790 } 1791 1792 /** 1793 * @return Returns a reference to <code>this</code> for easy method chaining 1794 */ 1795 public MessageHeader setFocus(List<Reference> theFocus) { 1796 this.focus = theFocus; 1797 return this; 1798 } 1799 1800 public boolean hasFocus() { 1801 if (this.focus == null) 1802 return false; 1803 for (Reference item : this.focus) 1804 if (!item.isEmpty()) 1805 return true; 1806 return false; 1807 } 1808 1809 public Reference addFocus() { //3 1810 Reference t = new Reference(); 1811 if (this.focus == null) 1812 this.focus = new ArrayList<Reference>(); 1813 this.focus.add(t); 1814 return t; 1815 } 1816 1817 public MessageHeader addFocus(Reference t) { //3 1818 if (t == null) 1819 return this; 1820 if (this.focus == null) 1821 this.focus = new ArrayList<Reference>(); 1822 this.focus.add(t); 1823 return this; 1824 } 1825 1826 /** 1827 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist 1828 */ 1829 public Reference getFocusFirstRep() { 1830 if (getFocus().isEmpty()) { 1831 addFocus(); 1832 } 1833 return getFocus().get(0); 1834 } 1835 1836 /** 1837 * @deprecated Use Reference#setResource(IBaseResource) instead 1838 */ 1839 @Deprecated 1840 public List<Resource> getFocusTarget() { 1841 if (this.focusTarget == null) 1842 this.focusTarget = new ArrayList<Resource>(); 1843 return this.focusTarget; 1844 } 1845 1846 protected void listChildren(List<Property> children) { 1847 super.listChildren(children); 1848 children.add(new Property("event", "Coding", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://hl7.org/fhir/message-events\".", 0, 1, event)); 1849 children.add(new Property("destination", "", "The destination application which the message is intended for.", 0, java.lang.Integer.MAX_VALUE, destination)); 1850 children.add(new Property("receiver", "Reference(Practitioner|Organization)", "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 0, 1, receiver)); 1851 children.add(new Property("sender", "Reference(Practitioner|Organization)", "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender)); 1852 children.add(new Property("timestamp", "instant", "The time that the message was sent.", 0, 1, timestamp)); 1853 children.add(new Property("enterer", "Reference(Practitioner)", "The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.", 0, 1, enterer)); 1854 children.add(new Property("author", "Reference(Practitioner)", "The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 0, 1, author)); 1855 children.add(new Property("source", "", "The source application from which this message originated.", 0, 1, source)); 1856 children.add(new Property("responsible", "Reference(Practitioner|Organization)", "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 0, 1, responsible)); 1857 children.add(new Property("reason", "CodeableConcept", "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 0, 1, reason)); 1858 children.add(new Property("response", "", "Information about the message that this message is a response to. Only present if this message is a response.", 0, 1, response)); 1859 children.add(new Property("focus", "Reference(Any)", "The actual data of the message - a reference to the root/focus class of the event.", 0, java.lang.Integer.MAX_VALUE, focus)); 1860 } 1861 1862 @Override 1863 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1864 switch (_hash) { 1865 case 96891546: /*event*/ return new Property("event", "Coding", "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://hl7.org/fhir/message-events\".", 0, 1, event); 1866 case -1429847026: /*destination*/ return new Property("destination", "", "The destination application which the message is intended for.", 0, java.lang.Integer.MAX_VALUE, destination); 1867 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Practitioner|Organization)", "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 0, 1, receiver); 1868 case -905962955: /*sender*/ return new Property("sender", "Reference(Practitioner|Organization)", "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender); 1869 case 55126294: /*timestamp*/ return new Property("timestamp", "instant", "The time that the message was sent.", 0, 1, timestamp); 1870 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.", 0, 1, enterer); 1871 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner)", "The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 0, 1, author); 1872 case -896505829: /*source*/ return new Property("source", "", "The source application from which this message originated.", 0, 1, source); 1873 case 1847674614: /*responsible*/ return new Property("responsible", "Reference(Practitioner|Organization)", "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 0, 1, responsible); 1874 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 0, 1, reason); 1875 case -340323263: /*response*/ return new Property("response", "", "Information about the message that this message is a response to. Only present if this message is a response.", 0, 1, response); 1876 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual data of the message - a reference to the root/focus class of the event.", 0, java.lang.Integer.MAX_VALUE, focus); 1877 default: return super.getNamedProperty(_hash, _name, _checkValid); 1878 } 1879 1880 } 1881 1882 @Override 1883 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1884 switch (hash) { 1885 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // Coding 1886 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : this.destination.toArray(new Base[this.destination.size()]); // MessageDestinationComponent 1887 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : new Base[] {this.receiver}; // Reference 1888 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 1889 case 55126294: /*timestamp*/ return this.timestamp == null ? new Base[0] : new Base[] {this.timestamp}; // InstantType 1890 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 1891 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1892 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // MessageSourceComponent 1893 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // Reference 1894 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1895 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // MessageHeaderResponseComponent 1896 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 1897 default: return super.getProperty(hash, name, checkValid); 1898 } 1899 1900 } 1901 1902 @Override 1903 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1904 switch (hash) { 1905 case 96891546: // event 1906 this.event = castToCoding(value); // Coding 1907 return value; 1908 case -1429847026: // destination 1909 this.getDestination().add((MessageDestinationComponent) value); // MessageDestinationComponent 1910 return value; 1911 case -808719889: // receiver 1912 this.receiver = castToReference(value); // Reference 1913 return value; 1914 case -905962955: // sender 1915 this.sender = castToReference(value); // Reference 1916 return value; 1917 case 55126294: // timestamp 1918 this.timestamp = castToInstant(value); // InstantType 1919 return value; 1920 case -1591951995: // enterer 1921 this.enterer = castToReference(value); // Reference 1922 return value; 1923 case -1406328437: // author 1924 this.author = castToReference(value); // Reference 1925 return value; 1926 case -896505829: // source 1927 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1928 return value; 1929 case 1847674614: // responsible 1930 this.responsible = castToReference(value); // Reference 1931 return value; 1932 case -934964668: // reason 1933 this.reason = castToCodeableConcept(value); // CodeableConcept 1934 return value; 1935 case -340323263: // response 1936 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1937 return value; 1938 case 97604824: // focus 1939 this.getFocus().add(castToReference(value)); // Reference 1940 return value; 1941 default: return super.setProperty(hash, name, value); 1942 } 1943 1944 } 1945 1946 @Override 1947 public Base setProperty(String name, Base value) throws FHIRException { 1948 if (name.equals("event")) { 1949 this.event = castToCoding(value); // Coding 1950 } else if (name.equals("destination")) { 1951 this.getDestination().add((MessageDestinationComponent) value); 1952 } else if (name.equals("receiver")) { 1953 this.receiver = castToReference(value); // Reference 1954 } else if (name.equals("sender")) { 1955 this.sender = castToReference(value); // Reference 1956 } else if (name.equals("timestamp")) { 1957 this.timestamp = castToInstant(value); // InstantType 1958 } else if (name.equals("enterer")) { 1959 this.enterer = castToReference(value); // Reference 1960 } else if (name.equals("author")) { 1961 this.author = castToReference(value); // Reference 1962 } else if (name.equals("source")) { 1963 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1964 } else if (name.equals("responsible")) { 1965 this.responsible = castToReference(value); // Reference 1966 } else if (name.equals("reason")) { 1967 this.reason = castToCodeableConcept(value); // CodeableConcept 1968 } else if (name.equals("response")) { 1969 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1970 } else if (name.equals("focus")) { 1971 this.getFocus().add(castToReference(value)); 1972 } else 1973 return super.setProperty(name, value); 1974 return value; 1975 } 1976 1977 @Override 1978 public Base makeProperty(int hash, String name) throws FHIRException { 1979 switch (hash) { 1980 case 96891546: return getEvent(); 1981 case -1429847026: return addDestination(); 1982 case -808719889: return getReceiver(); 1983 case -905962955: return getSender(); 1984 case 55126294: return getTimestampElement(); 1985 case -1591951995: return getEnterer(); 1986 case -1406328437: return getAuthor(); 1987 case -896505829: return getSource(); 1988 case 1847674614: return getResponsible(); 1989 case -934964668: return getReason(); 1990 case -340323263: return getResponse(); 1991 case 97604824: return addFocus(); 1992 default: return super.makeProperty(hash, name); 1993 } 1994 1995 } 1996 1997 @Override 1998 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1999 switch (hash) { 2000 case 96891546: /*event*/ return new String[] {"Coding"}; 2001 case -1429847026: /*destination*/ return new String[] {}; 2002 case -808719889: /*receiver*/ return new String[] {"Reference"}; 2003 case -905962955: /*sender*/ return new String[] {"Reference"}; 2004 case 55126294: /*timestamp*/ return new String[] {"instant"}; 2005 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 2006 case -1406328437: /*author*/ return new String[] {"Reference"}; 2007 case -896505829: /*source*/ return new String[] {}; 2008 case 1847674614: /*responsible*/ return new String[] {"Reference"}; 2009 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 2010 case -340323263: /*response*/ return new String[] {}; 2011 case 97604824: /*focus*/ return new String[] {"Reference"}; 2012 default: return super.getTypesForProperty(hash, name); 2013 } 2014 2015 } 2016 2017 @Override 2018 public Base addChild(String name) throws FHIRException { 2019 if (name.equals("event")) { 2020 this.event = new Coding(); 2021 return this.event; 2022 } 2023 else if (name.equals("destination")) { 2024 return addDestination(); 2025 } 2026 else if (name.equals("receiver")) { 2027 this.receiver = new Reference(); 2028 return this.receiver; 2029 } 2030 else if (name.equals("sender")) { 2031 this.sender = new Reference(); 2032 return this.sender; 2033 } 2034 else if (name.equals("timestamp")) { 2035 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.timestamp"); 2036 } 2037 else if (name.equals("enterer")) { 2038 this.enterer = new Reference(); 2039 return this.enterer; 2040 } 2041 else if (name.equals("author")) { 2042 this.author = new Reference(); 2043 return this.author; 2044 } 2045 else if (name.equals("source")) { 2046 this.source = new MessageSourceComponent(); 2047 return this.source; 2048 } 2049 else if (name.equals("responsible")) { 2050 this.responsible = new Reference(); 2051 return this.responsible; 2052 } 2053 else if (name.equals("reason")) { 2054 this.reason = new CodeableConcept(); 2055 return this.reason; 2056 } 2057 else if (name.equals("response")) { 2058 this.response = new MessageHeaderResponseComponent(); 2059 return this.response; 2060 } 2061 else if (name.equals("focus")) { 2062 return addFocus(); 2063 } 2064 else 2065 return super.addChild(name); 2066 } 2067 2068 public String fhirType() { 2069 return "MessageHeader"; 2070 2071 } 2072 2073 public MessageHeader copy() { 2074 MessageHeader dst = new MessageHeader(); 2075 copyValues(dst); 2076 dst.event = event == null ? null : event.copy(); 2077 if (destination != null) { 2078 dst.destination = new ArrayList<MessageDestinationComponent>(); 2079 for (MessageDestinationComponent i : destination) 2080 dst.destination.add(i.copy()); 2081 }; 2082 dst.receiver = receiver == null ? null : receiver.copy(); 2083 dst.sender = sender == null ? null : sender.copy(); 2084 dst.timestamp = timestamp == null ? null : timestamp.copy(); 2085 dst.enterer = enterer == null ? null : enterer.copy(); 2086 dst.author = author == null ? null : author.copy(); 2087 dst.source = source == null ? null : source.copy(); 2088 dst.responsible = responsible == null ? null : responsible.copy(); 2089 dst.reason = reason == null ? null : reason.copy(); 2090 dst.response = response == null ? null : response.copy(); 2091 if (focus != null) { 2092 dst.focus = new ArrayList<Reference>(); 2093 for (Reference i : focus) 2094 dst.focus.add(i.copy()); 2095 }; 2096 return dst; 2097 } 2098 2099 protected MessageHeader typedCopy() { 2100 return copy(); 2101 } 2102 2103 @Override 2104 public boolean equalsDeep(Base other_) { 2105 if (!super.equalsDeep(other_)) 2106 return false; 2107 if (!(other_ instanceof MessageHeader)) 2108 return false; 2109 MessageHeader o = (MessageHeader) other_; 2110 return compareDeep(event, o.event, true) && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) 2111 && compareDeep(sender, o.sender, true) && compareDeep(timestamp, o.timestamp, true) && compareDeep(enterer, o.enterer, true) 2112 && compareDeep(author, o.author, true) && compareDeep(source, o.source, true) && compareDeep(responsible, o.responsible, true) 2113 && compareDeep(reason, o.reason, true) && compareDeep(response, o.response, true) && compareDeep(focus, o.focus, true) 2114 ; 2115 } 2116 2117 @Override 2118 public boolean equalsShallow(Base other_) { 2119 if (!super.equalsShallow(other_)) 2120 return false; 2121 if (!(other_ instanceof MessageHeader)) 2122 return false; 2123 MessageHeader o = (MessageHeader) other_; 2124 return compareValues(timestamp, o.timestamp, true); 2125 } 2126 2127 public boolean isEmpty() { 2128 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, destination, receiver 2129 , sender, timestamp, enterer, author, source, responsible, reason, response 2130 , focus); 2131 } 2132 2133 @Override 2134 public ResourceType getResourceType() { 2135 return ResourceType.MessageHeader; 2136 } 2137 2138 /** 2139 * Search parameter: <b>code</b> 2140 * <p> 2141 * Description: <b>ok | transient-error | fatal-error</b><br> 2142 * Type: <b>token</b><br> 2143 * Path: <b>MessageHeader.response.code</b><br> 2144 * </p> 2145 */ 2146 @SearchParamDefinition(name="code", path="MessageHeader.response.code", description="ok | transient-error | fatal-error", type="token" ) 2147 public static final String SP_CODE = "code"; 2148 /** 2149 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2150 * <p> 2151 * Description: <b>ok | transient-error | fatal-error</b><br> 2152 * Type: <b>token</b><br> 2153 * Path: <b>MessageHeader.response.code</b><br> 2154 * </p> 2155 */ 2156 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2157 2158 /** 2159 * Search parameter: <b>receiver</b> 2160 * <p> 2161 * Description: <b>Intended "real-world" recipient for the data</b><br> 2162 * Type: <b>reference</b><br> 2163 * Path: <b>MessageHeader.receiver</b><br> 2164 * </p> 2165 */ 2166 @SearchParamDefinition(name="receiver", path="MessageHeader.receiver", description="Intended \"real-world\" recipient for the data", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 2167 public static final String SP_RECEIVER = "receiver"; 2168 /** 2169 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 2170 * <p> 2171 * Description: <b>Intended "real-world" recipient for the data</b><br> 2172 * Type: <b>reference</b><br> 2173 * Path: <b>MessageHeader.receiver</b><br> 2174 * </p> 2175 */ 2176 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 2177 2178/** 2179 * Constant for fluent queries to be used to add include statements. Specifies 2180 * the path value of "<b>MessageHeader:receiver</b>". 2181 */ 2182 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("MessageHeader:receiver").toLocked(); 2183 2184 /** 2185 * Search parameter: <b>author</b> 2186 * <p> 2187 * Description: <b>The source of the decision</b><br> 2188 * Type: <b>reference</b><br> 2189 * Path: <b>MessageHeader.author</b><br> 2190 * </p> 2191 */ 2192 @SearchParamDefinition(name="author", path="MessageHeader.author", description="The source of the decision", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2193 public static final String SP_AUTHOR = "author"; 2194 /** 2195 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2196 * <p> 2197 * Description: <b>The source of the decision</b><br> 2198 * Type: <b>reference</b><br> 2199 * Path: <b>MessageHeader.author</b><br> 2200 * </p> 2201 */ 2202 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2203 2204/** 2205 * Constant for fluent queries to be used to add include statements. Specifies 2206 * the path value of "<b>MessageHeader:author</b>". 2207 */ 2208 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("MessageHeader:author").toLocked(); 2209 2210 /** 2211 * Search parameter: <b>destination</b> 2212 * <p> 2213 * Description: <b>Name of system</b><br> 2214 * Type: <b>string</b><br> 2215 * Path: <b>MessageHeader.destination.name</b><br> 2216 * </p> 2217 */ 2218 @SearchParamDefinition(name="destination", path="MessageHeader.destination.name", description="Name of system", type="string" ) 2219 public static final String SP_DESTINATION = "destination"; 2220 /** 2221 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 2222 * <p> 2223 * Description: <b>Name of system</b><br> 2224 * Type: <b>string</b><br> 2225 * Path: <b>MessageHeader.destination.name</b><br> 2226 * </p> 2227 */ 2228 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESTINATION); 2229 2230 /** 2231 * Search parameter: <b>focus</b> 2232 * <p> 2233 * Description: <b>The actual content of the message</b><br> 2234 * Type: <b>reference</b><br> 2235 * Path: <b>MessageHeader.focus</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name="focus", path="MessageHeader.focus", description="The actual content of the message", type="reference" ) 2239 public static final String SP_FOCUS = "focus"; 2240 /** 2241 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2242 * <p> 2243 * Description: <b>The actual content of the message</b><br> 2244 * Type: <b>reference</b><br> 2245 * Path: <b>MessageHeader.focus</b><br> 2246 * </p> 2247 */ 2248 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 2249 2250/** 2251 * Constant for fluent queries to be used to add include statements. Specifies 2252 * the path value of "<b>MessageHeader:focus</b>". 2253 */ 2254 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("MessageHeader:focus").toLocked(); 2255 2256 /** 2257 * Search parameter: <b>source</b> 2258 * <p> 2259 * Description: <b>Name of system</b><br> 2260 * Type: <b>string</b><br> 2261 * Path: <b>MessageHeader.source.name</b><br> 2262 * </p> 2263 */ 2264 @SearchParamDefinition(name="source", path="MessageHeader.source.name", description="Name of system", type="string" ) 2265 public static final String SP_SOURCE = "source"; 2266 /** 2267 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2268 * <p> 2269 * Description: <b>Name of system</b><br> 2270 * Type: <b>string</b><br> 2271 * Path: <b>MessageHeader.source.name</b><br> 2272 * </p> 2273 */ 2274 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOURCE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SOURCE); 2275 2276 /** 2277 * Search parameter: <b>target</b> 2278 * <p> 2279 * Description: <b>Particular delivery destination within the destination</b><br> 2280 * Type: <b>reference</b><br> 2281 * Path: <b>MessageHeader.destination.target</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name="target", path="MessageHeader.destination.target", description="Particular delivery destination within the destination", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 2285 public static final String SP_TARGET = "target"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2288 * <p> 2289 * Description: <b>Particular delivery destination within the destination</b><br> 2290 * Type: <b>reference</b><br> 2291 * Path: <b>MessageHeader.destination.target</b><br> 2292 * </p> 2293 */ 2294 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 2295 2296/** 2297 * Constant for fluent queries to be used to add include statements. Specifies 2298 * the path value of "<b>MessageHeader:target</b>". 2299 */ 2300 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("MessageHeader:target").toLocked(); 2301 2302 /** 2303 * Search parameter: <b>destination-uri</b> 2304 * <p> 2305 * Description: <b>Actual destination address or id</b><br> 2306 * Type: <b>uri</b><br> 2307 * Path: <b>MessageHeader.destination.endpoint</b><br> 2308 * </p> 2309 */ 2310 @SearchParamDefinition(name="destination-uri", path="MessageHeader.destination.endpoint", description="Actual destination address or id", type="uri" ) 2311 public static final String SP_DESTINATION_URI = "destination-uri"; 2312 /** 2313 * <b>Fluent Client</b> search parameter constant for <b>destination-uri</b> 2314 * <p> 2315 * Description: <b>Actual destination address or id</b><br> 2316 * Type: <b>uri</b><br> 2317 * Path: <b>MessageHeader.destination.endpoint</b><br> 2318 * </p> 2319 */ 2320 public static final ca.uhn.fhir.rest.gclient.UriClientParam DESTINATION_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DESTINATION_URI); 2321 2322 /** 2323 * Search parameter: <b>source-uri</b> 2324 * <p> 2325 * Description: <b>Actual message source address or id</b><br> 2326 * Type: <b>uri</b><br> 2327 * Path: <b>MessageHeader.source.endpoint</b><br> 2328 * </p> 2329 */ 2330 @SearchParamDefinition(name="source-uri", path="MessageHeader.source.endpoint", description="Actual message source address or id", type="uri" ) 2331 public static final String SP_SOURCE_URI = "source-uri"; 2332 /** 2333 * <b>Fluent Client</b> search parameter constant for <b>source-uri</b> 2334 * <p> 2335 * Description: <b>Actual message source address or id</b><br> 2336 * Type: <b>uri</b><br> 2337 * Path: <b>MessageHeader.source.endpoint</b><br> 2338 * </p> 2339 */ 2340 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SOURCE_URI); 2341 2342 /** 2343 * Search parameter: <b>sender</b> 2344 * <p> 2345 * Description: <b>Real world sender of the message</b><br> 2346 * Type: <b>reference</b><br> 2347 * Path: <b>MessageHeader.sender</b><br> 2348 * </p> 2349 */ 2350 @SearchParamDefinition(name="sender", path="MessageHeader.sender", description="Real world sender of the message", type="reference", target={Organization.class, Practitioner.class } ) 2351 public static final String SP_SENDER = "sender"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2354 * <p> 2355 * Description: <b>Real world sender of the message</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>MessageHeader.sender</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2361 2362/** 2363 * Constant for fluent queries to be used to add include statements. Specifies 2364 * the path value of "<b>MessageHeader:sender</b>". 2365 */ 2366 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("MessageHeader:sender").toLocked(); 2367 2368 /** 2369 * Search parameter: <b>responsible</b> 2370 * <p> 2371 * Description: <b>Final responsibility for event</b><br> 2372 * Type: <b>reference</b><br> 2373 * Path: <b>MessageHeader.responsible</b><br> 2374 * </p> 2375 */ 2376 @SearchParamDefinition(name="responsible", path="MessageHeader.responsible", description="Final responsibility for event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 2377 public static final String SP_RESPONSIBLE = "responsible"; 2378 /** 2379 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 2380 * <p> 2381 * Description: <b>Final responsibility for event</b><br> 2382 * Type: <b>reference</b><br> 2383 * Path: <b>MessageHeader.responsible</b><br> 2384 * </p> 2385 */ 2386 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSIBLE); 2387 2388/** 2389 * Constant for fluent queries to be used to add include statements. Specifies 2390 * the path value of "<b>MessageHeader:responsible</b>". 2391 */ 2392 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLE = new ca.uhn.fhir.model.api.Include("MessageHeader:responsible").toLocked(); 2393 2394 /** 2395 * Search parameter: <b>enterer</b> 2396 * <p> 2397 * Description: <b>The source of the data entry</b><br> 2398 * Type: <b>reference</b><br> 2399 * Path: <b>MessageHeader.enterer</b><br> 2400 * </p> 2401 */ 2402 @SearchParamDefinition(name="enterer", path="MessageHeader.enterer", description="The source of the data entry", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2403 public static final String SP_ENTERER = "enterer"; 2404 /** 2405 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 2406 * <p> 2407 * Description: <b>The source of the data entry</b><br> 2408 * Type: <b>reference</b><br> 2409 * Path: <b>MessageHeader.enterer</b><br> 2410 * </p> 2411 */ 2412 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 2413 2414/** 2415 * Constant for fluent queries to be used to add include statements. Specifies 2416 * the path value of "<b>MessageHeader:enterer</b>". 2417 */ 2418 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("MessageHeader:enterer").toLocked(); 2419 2420 /** 2421 * Search parameter: <b>response-id</b> 2422 * <p> 2423 * Description: <b>Id of original message</b><br> 2424 * Type: <b>token</b><br> 2425 * Path: <b>MessageHeader.response.identifier</b><br> 2426 * </p> 2427 */ 2428 @SearchParamDefinition(name="response-id", path="MessageHeader.response.identifier", description="Id of original message", type="token" ) 2429 public static final String SP_RESPONSE_ID = "response-id"; 2430 /** 2431 * <b>Fluent Client</b> search parameter constant for <b>response-id</b> 2432 * <p> 2433 * Description: <b>Id of original message</b><br> 2434 * Type: <b>token</b><br> 2435 * Path: <b>MessageHeader.response.identifier</b><br> 2436 * </p> 2437 */ 2438 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESPONSE_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESPONSE_ID); 2439 2440 /** 2441 * Search parameter: <b>event</b> 2442 * <p> 2443 * Description: <b>Code for the event this message represents</b><br> 2444 * Type: <b>token</b><br> 2445 * Path: <b>MessageHeader.event</b><br> 2446 * </p> 2447 */ 2448 @SearchParamDefinition(name="event", path="MessageHeader.event", description="Code for the event this message represents", type="token" ) 2449 public static final String SP_EVENT = "event"; 2450 /** 2451 * <b>Fluent Client</b> search parameter constant for <b>event</b> 2452 * <p> 2453 * Description: <b>Code for the event this message represents</b><br> 2454 * Type: <b>token</b><br> 2455 * Path: <b>MessageHeader.event</b><br> 2456 * </p> 2457 */ 2458 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 2459 2460 /** 2461 * Search parameter: <b>timestamp</b> 2462 * <p> 2463 * Description: <b>Time that the message was sent</b><br> 2464 * Type: <b>date</b><br> 2465 * Path: <b>MessageHeader.timestamp</b><br> 2466 * </p> 2467 */ 2468 @SearchParamDefinition(name="timestamp", path="MessageHeader.timestamp", description="Time that the message was sent", type="date" ) 2469 public static final String SP_TIMESTAMP = "timestamp"; 2470 /** 2471 * <b>Fluent Client</b> search parameter constant for <b>timestamp</b> 2472 * <p> 2473 * Description: <b>Time that the message was sent</b><br> 2474 * Type: <b>date</b><br> 2475 * Path: <b>MessageHeader.timestamp</b><br> 2476 * </p> 2477 */ 2478 public static final ca.uhn.fhir.rest.gclient.DateClientParam TIMESTAMP = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_TIMESTAMP); 2479 2480 2481}