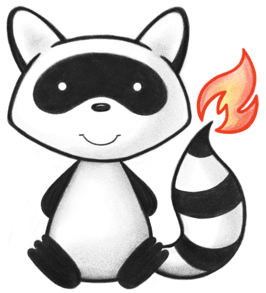
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.IBaseMetaType; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047/** 048 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 049 */ 050@DatatypeDef(name="Meta") 051public class Meta extends Type implements IBaseMetaType { 052 053 /** 054 * The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted. 055 */ 056 @Child(name = "versionId", type = {IdType.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="Version specific identifier", formalDefinition="The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted." ) 058 protected IdType versionId; 059 060 /** 061 * When the resource last changed - e.g. when the version changed. 062 */ 063 @Child(name = "lastUpdated", type = {InstantType.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="When the resource version last changed", formalDefinition="When the resource last changed - e.g. when the version changed." ) 065 protected InstantType lastUpdated; 066 067 /** 068 * A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]]. 069 */ 070 @Child(name = "profile", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 071 @Description(shortDefinition="Profiles this resource claims to conform to", formalDefinition="A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]]." ) 072 protected List<UriType> profile; 073 074 /** 075 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure. 076 */ 077 @Child(name = "security", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 078 @Description(shortDefinition="Security Labels applied to this resource", formalDefinition="Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure." ) 079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-labels") 080 protected List<Coding> security; 081 082 /** 083 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource. 084 */ 085 @Child(name = "tag", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 086 @Description(shortDefinition="Tags applied to this resource", formalDefinition="Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource." ) 087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/common-tags") 088 protected List<Coding> tag; 089 090 private static final long serialVersionUID = 867134915L; 091 092 /** 093 * Constructor 094 */ 095 public Meta() { 096 super(); 097 } 098 099 /** 100 * @return {@link #versionId} (The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.). This is the underlying object with id, value and extensions. The accessor "getVersionId" gives direct access to the value 101 */ 102 public IdType getVersionIdElement() { 103 if (this.versionId == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create Meta.versionId"); 106 else if (Configuration.doAutoCreate()) 107 this.versionId = new IdType(); // bb 108 return this.versionId; 109 } 110 111 public boolean hasVersionIdElement() { 112 return this.versionId != null && !this.versionId.isEmpty(); 113 } 114 115 public boolean hasVersionId() { 116 return this.versionId != null && !this.versionId.isEmpty(); 117 } 118 119 /** 120 * @param value {@link #versionId} (The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.). This is the underlying object with id, value and extensions. The accessor "getVersionId" gives direct access to the value 121 */ 122 public Meta setVersionIdElement(IdType value) { 123 this.versionId = value; 124 return this; 125 } 126 127 /** 128 * @return The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted. 129 */ 130 public String getVersionId() { 131 return this.versionId == null ? null : this.versionId.getValue(); 132 } 133 134 /** 135 * @param value The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted. 136 */ 137 public Meta setVersionId(String value) { 138 if (Utilities.noString(value)) 139 this.versionId = null; 140 else { 141 if (this.versionId == null) 142 this.versionId = new IdType(); 143 this.versionId.setValue(value); 144 } 145 return this; 146 } 147 148 /** 149 * @return {@link #lastUpdated} (When the resource last changed - e.g. when the version changed.). This is the underlying object with id, value and extensions. The accessor "getLastUpdated" gives direct access to the value 150 */ 151 public InstantType getLastUpdatedElement() { 152 if (this.lastUpdated == null) 153 if (Configuration.errorOnAutoCreate()) 154 throw new Error("Attempt to auto-create Meta.lastUpdated"); 155 else if (Configuration.doAutoCreate()) 156 this.lastUpdated = new InstantType(); // bb 157 return this.lastUpdated; 158 } 159 160 public boolean hasLastUpdatedElement() { 161 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 162 } 163 164 public boolean hasLastUpdated() { 165 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #lastUpdated} (When the resource last changed - e.g. when the version changed.). This is the underlying object with id, value and extensions. The accessor "getLastUpdated" gives direct access to the value 170 */ 171 public Meta setLastUpdatedElement(InstantType value) { 172 this.lastUpdated = value; 173 return this; 174 } 175 176 /** 177 * @return When the resource last changed - e.g. when the version changed. 178 */ 179 public Date getLastUpdated() { 180 return this.lastUpdated == null ? null : this.lastUpdated.getValue(); 181 } 182 183 /** 184 * @param value When the resource last changed - e.g. when the version changed. 185 */ 186 public Meta setLastUpdated(Date value) { 187 if (value == null) 188 this.lastUpdated = null; 189 else { 190 if (this.lastUpdated == null) 191 this.lastUpdated = new InstantType(); 192 this.lastUpdated.setValue(value); 193 } 194 return this; 195 } 196 197 /** 198 * @return {@link #profile} (A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].) 199 */ 200 public List<UriType> getProfile() { 201 if (this.profile == null) 202 this.profile = new ArrayList<UriType>(); 203 return this.profile; 204 } 205 206 /** 207 * @return Returns a reference to <code>this</code> for easy method chaining 208 */ 209 public Meta setProfile(List<UriType> theProfile) { 210 this.profile = theProfile; 211 return this; 212 } 213 214 public boolean hasProfile() { 215 if (this.profile == null) 216 return false; 217 for (UriType item : this.profile) 218 if (!item.isEmpty()) 219 return true; 220 return false; 221 } 222 223 /** 224 * @return {@link #profile} (A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].) 225 */ 226 public UriType addProfileElement() {//2 227 UriType t = new UriType(); 228 if (this.profile == null) 229 this.profile = new ArrayList<UriType>(); 230 this.profile.add(t); 231 return t; 232 } 233 234 /** 235 * @param value {@link #profile} (A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].) 236 */ 237 public Meta addProfile(String value) { //1 238 UriType t = new UriType(); 239 t.setValue(value); 240 if (this.profile == null) 241 this.profile = new ArrayList<UriType>(); 242 this.profile.add(t); 243 return this; 244 } 245 246 /** 247 * @param value {@link #profile} (A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].) 248 */ 249 public boolean hasProfile(String value) { 250 if (this.profile == null) 251 return false; 252 for (UriType v : this.profile) 253 if (v.getValue().equals(value)) // uri 254 return true; 255 return false; 256 } 257 258 /** 259 * @return {@link #security} (Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.) 260 */ 261 public List<Coding> getSecurity() { 262 if (this.security == null) 263 this.security = new ArrayList<Coding>(); 264 return this.security; 265 } 266 267 /** 268 * @return Returns a reference to <code>this</code> for easy method chaining 269 */ 270 public Meta setSecurity(List<Coding> theSecurity) { 271 this.security = theSecurity; 272 return this; 273 } 274 275 public boolean hasSecurity() { 276 if (this.security == null) 277 return false; 278 for (Coding item : this.security) 279 if (!item.isEmpty()) 280 return true; 281 return false; 282 } 283 284 public Coding addSecurity() { //3 285 Coding t = new Coding(); 286 if (this.security == null) 287 this.security = new ArrayList<Coding>(); 288 this.security.add(t); 289 return t; 290 } 291 292 public Meta addSecurity(Coding t) { //3 293 if (t == null) 294 return this; 295 if (this.security == null) 296 this.security = new ArrayList<Coding>(); 297 this.security.add(t); 298 return this; 299 } 300 301 /** 302 * @return The first repetition of repeating field {@link #security}, creating it if it does not already exist 303 */ 304 public Coding getSecurityFirstRep() { 305 if (getSecurity().isEmpty()) { 306 addSecurity(); 307 } 308 return getSecurity().get(0); 309 } 310 311 /** 312 * @return {@link #tag} (Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.) 313 */ 314 public List<Coding> getTag() { 315 if (this.tag == null) 316 this.tag = new ArrayList<Coding>(); 317 return this.tag; 318 } 319 320 /** 321 * @return Returns a reference to <code>this</code> for easy method chaining 322 */ 323 public Meta setTag(List<Coding> theTag) { 324 this.tag = theTag; 325 return this; 326 } 327 328 public boolean hasTag() { 329 if (this.tag == null) 330 return false; 331 for (Coding item : this.tag) 332 if (!item.isEmpty()) 333 return true; 334 return false; 335 } 336 337 public Coding addTag() { //3 338 Coding t = new Coding(); 339 if (this.tag == null) 340 this.tag = new ArrayList<Coding>(); 341 this.tag.add(t); 342 return t; 343 } 344 345 public Meta addTag(Coding t) { //3 346 if (t == null) 347 return this; 348 if (this.tag == null) 349 this.tag = new ArrayList<Coding>(); 350 this.tag.add(t); 351 return this; 352 } 353 354 /** 355 * @return The first repetition of repeating field {@link #tag}, creating it if it does not already exist 356 */ 357 public Coding getTagFirstRep() { 358 if (getTag().isEmpty()) { 359 addTag(); 360 } 361 return getTag().get(0); 362 } 363 364 /** 365 * Convenience method which adds a tag 366 * 367 * @param theSystem The code system 368 * @param theCode The code 369 * @param theDisplay The display name 370 * @return Returns a reference to <code>this</code> for easy chaining 371 */ 372 public Meta addTag(String theSystem, String theCode, String theDisplay) { 373 addTag().setSystem(theSystem).setCode(theCode).setDisplay(theDisplay); 374 return this; 375 } 376 /** 377 * Convenience method which adds a security tag 378 * 379 * @param theSystem The code system 380 * @param theCode The code 381 * @param theDisplay The display name 382 * @return Returns a reference to <code>this</code> for easy chaining 383 */ 384 public Meta addSecurity(String theSystem, String theCode, String theDisplay) { 385 addSecurity().setSystem(theSystem).setCode(theCode).setDisplay(theDisplay); 386 return this; 387 } 388 /** 389 * Returns the first tag (if any) that has the given system and code, or returns 390 * <code>null</code> if none 391 */ 392 public Coding getTag(String theSystem, String theCode) { 393 for (Coding next : getTag()) { 394 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 395 return next; 396 } 397 } 398 return null; 399 } 400 401 /** 402 * Returns the first security label (if any) that has the given system and code, or returns 403 * <code>null</code> if none 404 */ 405 public Coding getSecurity(String theSystem, String theCode) { 406 for (Coding next : getSecurity()) { 407 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 408 return next; 409 } 410 } 411 return null; 412 } 413 protected void listChildren(List<Property> children) { 414 super.listChildren(children); 415 children.add(new Property("versionId", "id", "The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.", 0, 1, versionId)); 416 children.add(new Property("lastUpdated", "instant", "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated)); 417 children.add(new Property("profile", "uri", "A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].", 0, java.lang.Integer.MAX_VALUE, profile)); 418 children.add(new Property("security", "Coding", "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 0, java.lang.Integer.MAX_VALUE, security)); 419 children.add(new Property("tag", "Coding", "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 0, java.lang.Integer.MAX_VALUE, tag)); 420 } 421 422 @Override 423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 424 switch (_hash) { 425 case -1407102957: /*versionId*/ return new Property("versionId", "id", "The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.", 0, 1, versionId); 426 case 1649733957: /*lastUpdated*/ return new Property("lastUpdated", "instant", "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated); 427 case -309425751: /*profile*/ return new Property("profile", "uri", "A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].", 0, java.lang.Integer.MAX_VALUE, profile); 428 case 949122880: /*security*/ return new Property("security", "Coding", "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 0, java.lang.Integer.MAX_VALUE, security); 429 case 114586: /*tag*/ return new Property("tag", "Coding", "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 0, java.lang.Integer.MAX_VALUE, tag); 430 default: return super.getNamedProperty(_hash, _name, _checkValid); 431 } 432 433 } 434 435 @Override 436 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 437 switch (hash) { 438 case -1407102957: /*versionId*/ return this.versionId == null ? new Base[0] : new Base[] {this.versionId}; // IdType 439 case 1649733957: /*lastUpdated*/ return this.lastUpdated == null ? new Base[0] : new Base[] {this.lastUpdated}; // InstantType 440 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // UriType 441 case 949122880: /*security*/ return this.security == null ? new Base[0] : this.security.toArray(new Base[this.security.size()]); // Coding 442 case 114586: /*tag*/ return this.tag == null ? new Base[0] : this.tag.toArray(new Base[this.tag.size()]); // Coding 443 default: return super.getProperty(hash, name, checkValid); 444 } 445 446 } 447 448 @Override 449 public Base setProperty(int hash, String name, Base value) throws FHIRException { 450 switch (hash) { 451 case -1407102957: // versionId 452 this.versionId = castToId(value); // IdType 453 return value; 454 case 1649733957: // lastUpdated 455 this.lastUpdated = castToInstant(value); // InstantType 456 return value; 457 case -309425751: // profile 458 this.getProfile().add(castToUri(value)); // UriType 459 return value; 460 case 949122880: // security 461 this.getSecurity().add(castToCoding(value)); // Coding 462 return value; 463 case 114586: // tag 464 this.getTag().add(castToCoding(value)); // Coding 465 return value; 466 default: return super.setProperty(hash, name, value); 467 } 468 469 } 470 471 @Override 472 public Base setProperty(String name, Base value) throws FHIRException { 473 if (name.equals("versionId")) { 474 this.versionId = castToId(value); // IdType 475 } else if (name.equals("lastUpdated")) { 476 this.lastUpdated = castToInstant(value); // InstantType 477 } else if (name.equals("profile")) { 478 this.getProfile().add(castToUri(value)); 479 } else if (name.equals("security")) { 480 this.getSecurity().add(castToCoding(value)); 481 } else if (name.equals("tag")) { 482 this.getTag().add(castToCoding(value)); 483 } else 484 return super.setProperty(name, value); 485 return value; 486 } 487 488 @Override 489 public Base makeProperty(int hash, String name) throws FHIRException { 490 switch (hash) { 491 case -1407102957: return getVersionIdElement(); 492 case 1649733957: return getLastUpdatedElement(); 493 case -309425751: return addProfileElement(); 494 case 949122880: return addSecurity(); 495 case 114586: return addTag(); 496 default: return super.makeProperty(hash, name); 497 } 498 499 } 500 501 @Override 502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case -1407102957: /*versionId*/ return new String[] {"id"}; 505 case 1649733957: /*lastUpdated*/ return new String[] {"instant"}; 506 case -309425751: /*profile*/ return new String[] {"uri"}; 507 case 949122880: /*security*/ return new String[] {"Coding"}; 508 case 114586: /*tag*/ return new String[] {"Coding"}; 509 default: return super.getTypesForProperty(hash, name); 510 } 511 512 } 513 514 @Override 515 public Base addChild(String name) throws FHIRException { 516 if (name.equals("versionId")) { 517 throw new FHIRException("Cannot call addChild on a singleton property Meta.versionId"); 518 } 519 else if (name.equals("lastUpdated")) { 520 throw new FHIRException("Cannot call addChild on a singleton property Meta.lastUpdated"); 521 } 522 else if (name.equals("profile")) { 523 throw new FHIRException("Cannot call addChild on a singleton property Meta.profile"); 524 } 525 else if (name.equals("security")) { 526 return addSecurity(); 527 } 528 else if (name.equals("tag")) { 529 return addTag(); 530 } 531 else 532 return super.addChild(name); 533 } 534 535 public String fhirType() { 536 return "Meta"; 537 538 } 539 540 public Meta copy() { 541 Meta dst = new Meta(); 542 copyValues(dst); 543 dst.versionId = versionId == null ? null : versionId.copy(); 544 dst.lastUpdated = lastUpdated == null ? null : lastUpdated.copy(); 545 if (profile != null) { 546 dst.profile = new ArrayList<UriType>(); 547 for (UriType i : profile) 548 dst.profile.add(i.copy()); 549 }; 550 if (security != null) { 551 dst.security = new ArrayList<Coding>(); 552 for (Coding i : security) 553 dst.security.add(i.copy()); 554 }; 555 if (tag != null) { 556 dst.tag = new ArrayList<Coding>(); 557 for (Coding i : tag) 558 dst.tag.add(i.copy()); 559 }; 560 return dst; 561 } 562 563 protected Meta typedCopy() { 564 return copy(); 565 } 566 567 @Override 568 public boolean equalsDeep(Base other_) { 569 if (!super.equalsDeep(other_)) 570 return false; 571 if (!(other_ instanceof Meta)) 572 return false; 573 Meta o = (Meta) other_; 574 return compareDeep(versionId, o.versionId, true) && compareDeep(lastUpdated, o.lastUpdated, true) 575 && compareDeep(profile, o.profile, true) && compareDeep(security, o.security, true) && compareDeep(tag, o.tag, true) 576 ; 577 } 578 579 @Override 580 public boolean equalsShallow(Base other_) { 581 if (!super.equalsShallow(other_)) 582 return false; 583 if (!(other_ instanceof Meta)) 584 return false; 585 Meta o = (Meta) other_; 586 return compareValues(versionId, o.versionId, true) && compareValues(lastUpdated, o.lastUpdated, true) 587 && compareValues(profile, o.profile, true); 588 } 589 590 public boolean isEmpty() { 591 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(versionId, lastUpdated, profile 592 , security, tag); 593 } 594 595 596}