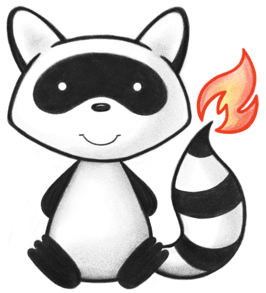
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * Common Ancestor declaration for conformance and knowledge artifact resources. 048 */ 049public abstract class MetadataResource extends DomainResource { 050 051 /** 052 * An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions). 053 */ 054 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 055 @Description(shortDefinition="Logical URI to reference this metadata resource (globally unique)", formalDefinition="An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions)." ) 056 protected UriType url; 057 058 /** 059 * The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 060 */ 061 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Business version of the metadata resource", formalDefinition="The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 063 protected StringType version; 064 065 /** 066 * A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation. 067 */ 068 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Name for this metadata resource (computer friendly)", formalDefinition="A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 070 protected StringType name; 071 072 /** 073 * A short, descriptive, user-friendly title for the metadata resource. 074 */ 075 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="Name for this metadata resource (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the metadata resource." ) 077 protected StringType title; 078 079 /** 080 * The status of this metadata resource. Enables tracking the life-cycle of the content. 081 */ 082 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 083 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this metadata resource. Enables tracking the life-cycle of the content." ) 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 085 protected Enumeration<PublicationStatus> status; 086 087 /** 088 * A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 089 */ 090 @Child(name = "experimental", type = {BooleanType.class}, order=5, min=0, max=1, modifier=true, summary=true) 091 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage." ) 092 protected BooleanType experimental; 093 094 /** 095 * The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes. 096 */ 097 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 098 @Description(shortDefinition="Date this was last changed", formalDefinition="The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes." ) 099 protected DateTimeType date; 100 101 /** 102 * The name of the individual or organization that published the metadata resource. 103 */ 104 @Child(name = "publisher", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 105 @Description(shortDefinition="Name of the publisher (organization or individual)", formalDefinition="The name of the individual or organization that published the metadata resource." ) 106 protected StringType publisher; 107 108 /** 109 * Contact details to assist a user in finding and communicating with the publisher. 110 */ 111 @Child(name = "contact", type = {ContactDetail.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 112 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 113 protected List<ContactDetail> contact; 114 115 /** 116 * The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate metadata resource instances. 117 */ 118 @Child(name = "useContext", type = {UsageContext.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 119 @Description(shortDefinition="Context the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate metadata resource instances." ) 120 protected List<UsageContext> useContext; 121 122 /** 123 * A legal or geographic region in which the metadata resource is intended to be used. 124 */ 125 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 126 @Description(shortDefinition="Intended jurisdiction for metadata resource (if applicable)", formalDefinition="A legal or geographic region in which the metadata resource is intended to be used." ) 127 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 128 protected List<CodeableConcept> jurisdiction; 129 130 /** 131 * A free text natural language description of the metadata resource from a consumer's perspective. 132 */ 133 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 134 @Description(shortDefinition="Natural language description of the metadata resource", formalDefinition="A free text natural language description of the metadata resource from a consumer's perspective." ) 135 protected MarkdownType description; 136 137 private static final long serialVersionUID = 145908634L; 138 139 /** 140 * Constructor 141 */ 142 public MetadataResource() { 143 super(); 144 } 145 146 /** 147 * Constructor 148 */ 149 public MetadataResource(Enumeration<PublicationStatus> status) { 150 super(); 151 this.status = status; 152 } 153 154 /** 155 * @return {@link #url} (An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 156 */ 157 public UriType getUrlElement() { 158 if (this.url == null) 159 if (Configuration.errorOnAutoCreate()) 160 throw new Error("Attempt to auto-create MetadataResource.url"); 161 else if (Configuration.doAutoCreate()) 162 this.url = new UriType(); // bb 163 return this.url; 164 } 165 166 public boolean hasUrlElement() { 167 return this.url != null && !this.url.isEmpty(); 168 } 169 170 public boolean hasUrl() { 171 return this.url != null && !this.url.isEmpty(); 172 } 173 174 /** 175 * @param value {@link #url} (An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 176 */ 177 public MetadataResource setUrlElement(UriType value) { 178 checkCanUseUrl(); 179 this.url = value; 180 return this; 181 } 182 183 protected void checkCanUseUrl() { 184 // it's fine 185 } 186 187 /** 188 * @return An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions). 189 */ 190 public String getUrl() { 191 return this.url == null ? null : this.url.getValue(); 192 } 193 194 /** 195 * @param value An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions). 196 */ 197 public MetadataResource setUrl(String value) { 198 if (Utilities.noString(value)) 199 this.url = null; 200 else { 201 checkCanUseUrl(); 202 if (this.url == null) 203 this.url = new UriType(); 204 this.url.setValue(value); 205 } 206 return this; 207 } 208 209 /** 210 * @return {@link #version} (The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 211 */ 212 public StringType getVersionElement() { 213 if (this.version == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create MetadataResource.version"); 216 else if (Configuration.doAutoCreate()) 217 this.version = new StringType(); // bb 218 return this.version; 219 } 220 221 public boolean hasVersionElement() { 222 return this.version != null && !this.version.isEmpty(); 223 } 224 225 public boolean hasVersion() { 226 return this.version != null && !this.version.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #version} (The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 231 */ 232 public MetadataResource setVersionElement(StringType value) { 233 this.version = value; 234 return this; 235 } 236 237 /** 238 * @return The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 239 */ 240 public String getVersion() { 241 return this.version == null ? null : this.version.getValue(); 242 } 243 244 /** 245 * @param value The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 246 */ 247 public MetadataResource setVersion(String value) { 248 if (Utilities.noString(value)) 249 this.version = null; 250 else { 251 if (this.version == null) 252 this.version = new StringType(); 253 this.version.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #name} (A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 260 */ 261 public StringType getNameElement() { 262 if (this.name == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create MetadataResource.name"); 265 else if (Configuration.doAutoCreate()) 266 this.name = new StringType(); // bb 267 return this.name; 268 } 269 270 public boolean hasNameElement() { 271 return this.name != null && !this.name.isEmpty(); 272 } 273 274 public boolean hasName() { 275 return this.name != null && !this.name.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #name} (A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 280 */ 281 public MetadataResource setNameElement(StringType value) { 282 this.name = value; 283 return this; 284 } 285 286 /** 287 * @return A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation. 288 */ 289 public String getName() { 290 return this.name == null ? null : this.name.getValue(); 291 } 292 293 /** 294 * @param value A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation. 295 */ 296 public MetadataResource setName(String value) { 297 if (Utilities.noString(value)) 298 this.name = null; 299 else { 300 if (this.name == null) 301 this.name = new StringType(); 302 this.name.setValue(value); 303 } 304 return this; 305 } 306 307 /** 308 * @return {@link #title} (A short, descriptive, user-friendly title for the metadata resource.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 309 */ 310 public StringType getTitleElement() { 311 if (this.title == null) 312 if (Configuration.errorOnAutoCreate()) 313 throw new Error("Attempt to auto-create MetadataResource.title"); 314 else if (Configuration.doAutoCreate()) 315 this.title = new StringType(); // bb 316 return this.title; 317 } 318 319 public boolean hasTitleElement() { 320 return this.title != null && !this.title.isEmpty(); 321 } 322 323 public boolean hasTitle() { 324 return this.title != null && !this.title.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #title} (A short, descriptive, user-friendly title for the metadata resource.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 329 */ 330 public MetadataResource setTitleElement(StringType value) { 331 this.title = value; 332 return this; 333 } 334 335 /** 336 * @return A short, descriptive, user-friendly title for the metadata resource. 337 */ 338 public String getTitle() { 339 return this.title == null ? null : this.title.getValue(); 340 } 341 342 /** 343 * @param value A short, descriptive, user-friendly title for the metadata resource. 344 */ 345 public MetadataResource setTitle(String value) { 346 if (Utilities.noString(value)) 347 this.title = null; 348 else { 349 if (this.title == null) 350 this.title = new StringType(); 351 this.title.setValue(value); 352 } 353 return this; 354 } 355 356 /** 357 * @return {@link #status} (The status of this metadata resource. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 358 */ 359 public Enumeration<PublicationStatus> getStatusElement() { 360 if (this.status == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create MetadataResource.status"); 363 else if (Configuration.doAutoCreate()) 364 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 365 return this.status; 366 } 367 368 public boolean hasStatusElement() { 369 return this.status != null && !this.status.isEmpty(); 370 } 371 372 public boolean hasStatus() { 373 return this.status != null && !this.status.isEmpty(); 374 } 375 376 /** 377 * @param value {@link #status} (The status of this metadata resource. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 378 */ 379 public MetadataResource setStatusElement(Enumeration<PublicationStatus> value) { 380 this.status = value; 381 return this; 382 } 383 384 /** 385 * @return The status of this metadata resource. Enables tracking the life-cycle of the content. 386 */ 387 public PublicationStatus getStatus() { 388 return this.status == null ? null : this.status.getValue(); 389 } 390 391 /** 392 * @param value The status of this metadata resource. Enables tracking the life-cycle of the content. 393 */ 394 public MetadataResource setStatus(PublicationStatus value) { 395 if (this.status == null) 396 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 397 this.status.setValue(value); 398 return this; 399 } 400 401 /** 402 * @return {@link #experimental} (A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 403 */ 404 public BooleanType getExperimentalElement() { 405 if (this.experimental == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create MetadataResource.experimental"); 408 else if (Configuration.doAutoCreate()) 409 this.experimental = new BooleanType(); // bb 410 return this.experimental; 411 } 412 413 public boolean hasExperimentalElement() { 414 return this.experimental != null && !this.experimental.isEmpty(); 415 } 416 417 public boolean hasExperimental() { 418 return this.experimental != null && !this.experimental.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #experimental} (A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 423 */ 424 public MetadataResource setExperimentalElement(BooleanType value) { 425 this.experimental = value; 426 return this; 427 } 428 429 /** 430 * @return A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 431 */ 432 public boolean getExperimental() { 433 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 434 } 435 436 /** 437 * @param value A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 438 */ 439 public MetadataResource setExperimental(boolean value) { 440 if (this.experimental == null) 441 this.experimental = new BooleanType(); 442 this.experimental.setValue(value); 443 return this; 444 } 445 446 /** 447 * @return {@link #date} (The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 448 */ 449 public DateTimeType getDateElement() { 450 if (this.date == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create MetadataResource.date"); 453 else if (Configuration.doAutoCreate()) 454 this.date = new DateTimeType(); // bb 455 return this.date; 456 } 457 458 public boolean hasDateElement() { 459 return this.date != null && !this.date.isEmpty(); 460 } 461 462 public boolean hasDate() { 463 return this.date != null && !this.date.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #date} (The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 468 */ 469 public MetadataResource setDateElement(DateTimeType value) { 470 this.date = value; 471 return this; 472 } 473 474 /** 475 * @return The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes. 476 */ 477 public Date getDate() { 478 return this.date == null ? null : this.date.getValue(); 479 } 480 481 /** 482 * @param value The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes. 483 */ 484 public MetadataResource setDate(Date value) { 485 if (value == null) 486 this.date = null; 487 else { 488 if (this.date == null) 489 this.date = new DateTimeType(); 490 this.date.setValue(value); 491 } 492 return this; 493 } 494 495 /** 496 * @return {@link #publisher} (The name of the individual or organization that published the metadata resource.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 497 */ 498 public StringType getPublisherElement() { 499 if (this.publisher == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create MetadataResource.publisher"); 502 else if (Configuration.doAutoCreate()) 503 this.publisher = new StringType(); // bb 504 return this.publisher; 505 } 506 507 public boolean hasPublisherElement() { 508 return this.publisher != null && !this.publisher.isEmpty(); 509 } 510 511 public boolean hasPublisher() { 512 return this.publisher != null && !this.publisher.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #publisher} (The name of the individual or organization that published the metadata resource.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 517 */ 518 public MetadataResource setPublisherElement(StringType value) { 519 this.publisher = value; 520 return this; 521 } 522 523 /** 524 * @return The name of the individual or organization that published the metadata resource. 525 */ 526 public String getPublisher() { 527 return this.publisher == null ? null : this.publisher.getValue(); 528 } 529 530 /** 531 * @param value The name of the individual or organization that published the metadata resource. 532 */ 533 public MetadataResource setPublisher(String value) { 534 if (Utilities.noString(value)) 535 this.publisher = null; 536 else { 537 if (this.publisher == null) 538 this.publisher = new StringType(); 539 this.publisher.setValue(value); 540 } 541 return this; 542 } 543 544 /** 545 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 546 */ 547 public List<ContactDetail> getContact() { 548 if (this.contact == null) 549 this.contact = new ArrayList<ContactDetail>(); 550 return this.contact; 551 } 552 553 /** 554 * @return Returns a reference to <code>this</code> for easy method chaining 555 */ 556 public MetadataResource setContact(List<ContactDetail> theContact) { 557 this.contact = theContact; 558 return this; 559 } 560 561 public boolean hasContact() { 562 if (this.contact == null) 563 return false; 564 for (ContactDetail item : this.contact) 565 if (!item.isEmpty()) 566 return true; 567 return false; 568 } 569 570 public ContactDetail addContact() { //3 571 ContactDetail t = new ContactDetail(); 572 if (this.contact == null) 573 this.contact = new ArrayList<ContactDetail>(); 574 this.contact.add(t); 575 return t; 576 } 577 578 public MetadataResource addContact(ContactDetail t) { //3 579 if (t == null) 580 return this; 581 if (this.contact == null) 582 this.contact = new ArrayList<ContactDetail>(); 583 this.contact.add(t); 584 return this; 585 } 586 587 /** 588 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 589 */ 590 public ContactDetail getContactFirstRep() { 591 if (getContact().isEmpty()) { 592 addContact(); 593 } 594 return getContact().get(0); 595 } 596 597 /** 598 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate metadata resource instances.) 599 */ 600 public List<UsageContext> getUseContext() { 601 if (this.useContext == null) 602 this.useContext = new ArrayList<UsageContext>(); 603 return this.useContext; 604 } 605 606 /** 607 * @return Returns a reference to <code>this</code> for easy method chaining 608 */ 609 public MetadataResource setUseContext(List<UsageContext> theUseContext) { 610 this.useContext = theUseContext; 611 return this; 612 } 613 614 public boolean hasUseContext() { 615 if (this.useContext == null) 616 return false; 617 for (UsageContext item : this.useContext) 618 if (!item.isEmpty()) 619 return true; 620 return false; 621 } 622 623 public UsageContext addUseContext() { //3 624 UsageContext t = new UsageContext(); 625 if (this.useContext == null) 626 this.useContext = new ArrayList<UsageContext>(); 627 this.useContext.add(t); 628 return t; 629 } 630 631 public MetadataResource addUseContext(UsageContext t) { //3 632 if (t == null) 633 return this; 634 if (this.useContext == null) 635 this.useContext = new ArrayList<UsageContext>(); 636 this.useContext.add(t); 637 return this; 638 } 639 640 /** 641 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 642 */ 643 public UsageContext getUseContextFirstRep() { 644 if (getUseContext().isEmpty()) { 645 addUseContext(); 646 } 647 return getUseContext().get(0); 648 } 649 650 /** 651 * @return {@link #jurisdiction} (A legal or geographic region in which the metadata resource is intended to be used.) 652 */ 653 public List<CodeableConcept> getJurisdiction() { 654 if (this.jurisdiction == null) 655 this.jurisdiction = new ArrayList<CodeableConcept>(); 656 return this.jurisdiction; 657 } 658 659 /** 660 * @return Returns a reference to <code>this</code> for easy method chaining 661 */ 662 public MetadataResource setJurisdiction(List<CodeableConcept> theJurisdiction) { 663 this.jurisdiction = theJurisdiction; 664 return this; 665 } 666 667 public boolean hasJurisdiction() { 668 if (this.jurisdiction == null) 669 return false; 670 for (CodeableConcept item : this.jurisdiction) 671 if (!item.isEmpty()) 672 return true; 673 return false; 674 } 675 676 public CodeableConcept addJurisdiction() { //3 677 CodeableConcept t = new CodeableConcept(); 678 if (this.jurisdiction == null) 679 this.jurisdiction = new ArrayList<CodeableConcept>(); 680 this.jurisdiction.add(t); 681 return t; 682 } 683 684 public MetadataResource addJurisdiction(CodeableConcept t) { //3 685 if (t == null) 686 return this; 687 if (this.jurisdiction == null) 688 this.jurisdiction = new ArrayList<CodeableConcept>(); 689 this.jurisdiction.add(t); 690 return this; 691 } 692 693 /** 694 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 695 */ 696 public CodeableConcept getJurisdictionFirstRep() { 697 if (getJurisdiction().isEmpty()) { 698 addJurisdiction(); 699 } 700 return getJurisdiction().get(0); 701 } 702 703 /** 704 * @return {@link #description} (A free text natural language description of the metadata resource from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 705 */ 706 public MarkdownType getDescriptionElement() { 707 if (this.description == null) 708 if (Configuration.errorOnAutoCreate()) 709 throw new Error("Attempt to auto-create MetadataResource.description"); 710 else if (Configuration.doAutoCreate()) 711 this.description = new MarkdownType(); // bb 712 return this.description; 713 } 714 715 public boolean hasDescriptionElement() { 716 return this.description != null && !this.description.isEmpty(); 717 } 718 719 public boolean hasDescription() { 720 return this.description != null && !this.description.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #description} (A free text natural language description of the metadata resource from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 725 */ 726 public MetadataResource setDescriptionElement(MarkdownType value) { 727 this.description = value; 728 return this; 729 } 730 731 /** 732 * @return A free text natural language description of the metadata resource from a consumer's perspective. 733 */ 734 public String getDescription() { 735 return this.description == null ? null : this.description.getValue(); 736 } 737 738 /** 739 * @param value A free text natural language description of the metadata resource from a consumer's perspective. 740 */ 741 public MetadataResource setDescription(String value) { 742 if (value == null) 743 this.description = null; 744 else { 745 if (this.description == null) 746 this.description = new MarkdownType(); 747 this.description.setValue(value); 748 } 749 return this; 750 } 751 752 protected void listChildren(List<Property> children) { 753 children.add(new Property("url", "uri", "An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 754 children.add(new Property("version", "string", "The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 755 children.add(new Property("name", "string", "A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 756 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the metadata resource.", 0, 1, title)); 757 children.add(new Property("status", "code", "The status of this metadata resource. Enables tracking the life-cycle of the content.", 0, 1, status)); 758 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 759 children.add(new Property("date", "dateTime", "The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes.", 0, 1, date)); 760 children.add(new Property("publisher", "string", "The name of the individual or organization that published the metadata resource.", 0, 1, publisher)); 761 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 762 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate metadata resource instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 763 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the metadata resource is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 764 children.add(new Property("description", "markdown", "A free text natural language description of the metadata resource from a consumer's perspective.", 0, 1, description)); 765 } 766 767 @Override 768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 769 switch (_hash) { 770 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this metadata resource is (or will be) published. The URL SHOULD include the major version of the metadata resource. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 771 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 772 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 773 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the metadata resource.", 0, 1, title); 774 case -892481550: /*status*/ return new Property("status", "code", "The status of this metadata resource. Enables tracking the life-cycle of the content.", 0, 1, status); 775 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 776 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the metadata resource was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes.", 0, 1, date); 777 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the metadata resource.", 0, 1, publisher); 778 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 779 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate metadata resource instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 780 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the metadata resource is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 781 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the metadata resource from a consumer's perspective.", 0, 1, description); 782 default: return super.getNamedProperty(_hash, _name, _checkValid); 783 } 784 785 } 786 787 @Override 788 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 789 switch (hash) { 790 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 791 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 792 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 793 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 794 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 795 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 796 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 797 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 798 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 799 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 800 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 801 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 802 default: return super.getProperty(hash, name, checkValid); 803 } 804 805 } 806 807 @Override 808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 809 switch (hash) { 810 case 116079: // url 811 this.url = castToUri(value); // UriType 812 return value; 813 case 351608024: // version 814 this.version = castToString(value); // StringType 815 return value; 816 case 3373707: // name 817 this.name = castToString(value); // StringType 818 return value; 819 case 110371416: // title 820 this.title = castToString(value); // StringType 821 return value; 822 case -892481550: // status 823 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 824 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 825 return value; 826 case -404562712: // experimental 827 this.experimental = castToBoolean(value); // BooleanType 828 return value; 829 case 3076014: // date 830 this.date = castToDateTime(value); // DateTimeType 831 return value; 832 case 1447404028: // publisher 833 this.publisher = castToString(value); // StringType 834 return value; 835 case 951526432: // contact 836 this.getContact().add(castToContactDetail(value)); // ContactDetail 837 return value; 838 case -669707736: // useContext 839 this.getUseContext().add(castToUsageContext(value)); // UsageContext 840 return value; 841 case -507075711: // jurisdiction 842 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 843 return value; 844 case -1724546052: // description 845 this.description = castToMarkdown(value); // MarkdownType 846 return value; 847 default: return super.setProperty(hash, name, value); 848 } 849 850 } 851 852 @Override 853 public Base setProperty(String name, Base value) throws FHIRException { 854 if (name.equals("url")) { 855 this.url = castToUri(value); // UriType 856 } else if (name.equals("version")) { 857 this.version = castToString(value); // StringType 858 } else if (name.equals("name")) { 859 this.name = castToString(value); // StringType 860 } else if (name.equals("title")) { 861 this.title = castToString(value); // StringType 862 } else if (name.equals("status")) { 863 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 864 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 865 } else if (name.equals("experimental")) { 866 this.experimental = castToBoolean(value); // BooleanType 867 } else if (name.equals("date")) { 868 this.date = castToDateTime(value); // DateTimeType 869 } else if (name.equals("publisher")) { 870 this.publisher = castToString(value); // StringType 871 } else if (name.equals("contact")) { 872 this.getContact().add(castToContactDetail(value)); 873 } else if (name.equals("useContext")) { 874 this.getUseContext().add(castToUsageContext(value)); 875 } else if (name.equals("jurisdiction")) { 876 this.getJurisdiction().add(castToCodeableConcept(value)); 877 } else if (name.equals("description")) { 878 this.description = castToMarkdown(value); // MarkdownType 879 } else 880 return super.setProperty(name, value); 881 return value; 882 } 883 884 @Override 885 public Base makeProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case 116079: return getUrlElement(); 888 case 351608024: return getVersionElement(); 889 case 3373707: return getNameElement(); 890 case 110371416: return getTitleElement(); 891 case -892481550: return getStatusElement(); 892 case -404562712: return getExperimentalElement(); 893 case 3076014: return getDateElement(); 894 case 1447404028: return getPublisherElement(); 895 case 951526432: return addContact(); 896 case -669707736: return addUseContext(); 897 case -507075711: return addJurisdiction(); 898 case -1724546052: return getDescriptionElement(); 899 default: return super.makeProperty(hash, name); 900 } 901 902 } 903 904 @Override 905 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 906 switch (hash) { 907 case 116079: /*url*/ return new String[] {"uri"}; 908 case 351608024: /*version*/ return new String[] {"string"}; 909 case 3373707: /*name*/ return new String[] {"string"}; 910 case 110371416: /*title*/ return new String[] {"string"}; 911 case -892481550: /*status*/ return new String[] {"code"}; 912 case -404562712: /*experimental*/ return new String[] {"boolean"}; 913 case 3076014: /*date*/ return new String[] {"dateTime"}; 914 case 1447404028: /*publisher*/ return new String[] {"string"}; 915 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 916 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 917 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 918 case -1724546052: /*description*/ return new String[] {"markdown"}; 919 default: return super.getTypesForProperty(hash, name); 920 } 921 922 } 923 924 @Override 925 public Base addChild(String name) throws FHIRException { 926 if (name.equals("url")) { 927 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.url"); 928 } 929 else if (name.equals("version")) { 930 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.version"); 931 } 932 else if (name.equals("name")) { 933 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.name"); 934 } 935 else if (name.equals("title")) { 936 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.title"); 937 } 938 else if (name.equals("status")) { 939 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.status"); 940 } 941 else if (name.equals("experimental")) { 942 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.experimental"); 943 } 944 else if (name.equals("date")) { 945 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.date"); 946 } 947 else if (name.equals("publisher")) { 948 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.publisher"); 949 } 950 else if (name.equals("contact")) { 951 return addContact(); 952 } 953 else if (name.equals("useContext")) { 954 return addUseContext(); 955 } 956 else if (name.equals("jurisdiction")) { 957 return addJurisdiction(); 958 } 959 else if (name.equals("description")) { 960 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.description"); 961 } 962 else 963 return super.addChild(name); 964 } 965 966 public String fhirType() { 967 return "MetadataResource"; 968 969 } 970 971 public abstract MetadataResource copy(); 972 973 public void copyValues(MetadataResource dst) { 974 super.copyValues(dst); 975 dst.url = url == null ? null : url.copy(); 976 dst.version = version == null ? null : version.copy(); 977 dst.name = name == null ? null : name.copy(); 978 dst.title = title == null ? null : title.copy(); 979 dst.status = status == null ? null : status.copy(); 980 dst.experimental = experimental == null ? null : experimental.copy(); 981 dst.date = date == null ? null : date.copy(); 982 dst.publisher = publisher == null ? null : publisher.copy(); 983 if (contact != null) { 984 dst.contact = new ArrayList<ContactDetail>(); 985 for (ContactDetail i : contact) 986 dst.contact.add(i.copy()); 987 }; 988 if (useContext != null) { 989 dst.useContext = new ArrayList<UsageContext>(); 990 for (UsageContext i : useContext) 991 dst.useContext.add(i.copy()); 992 }; 993 if (jurisdiction != null) { 994 dst.jurisdiction = new ArrayList<CodeableConcept>(); 995 for (CodeableConcept i : jurisdiction) 996 dst.jurisdiction.add(i.copy()); 997 }; 998 dst.description = description == null ? null : description.copy(); 999 } 1000 1001 @Override 1002 public boolean equalsDeep(Base other_) { 1003 if (!super.equalsDeep(other_)) 1004 return false; 1005 if (!(other_ instanceof MetadataResource)) 1006 return false; 1007 MetadataResource o = (MetadataResource) other_; 1008 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 1009 && compareDeep(title, o.title, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 1010 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1011 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 1012 && compareDeep(description, o.description, true); 1013 } 1014 1015 @Override 1016 public boolean equalsShallow(Base other_) { 1017 if (!super.equalsShallow(other_)) 1018 return false; 1019 if (!(other_ instanceof MetadataResource)) 1020 return false; 1021 MetadataResource o = (MetadataResource) other_; 1022 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 1023 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 1024 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 1025 ; 1026 } 1027 1028 public boolean isEmpty() { 1029 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, version, name, title 1030 , status, experimental, date, publisher, contact, useContext, jurisdiction, description 1031 ); 1032 } 1033 1034// added from java-adornments.txt: 1035 @Override 1036 public String toString() { 1037 return fhirType()+"["+getUrl()+"]"; 1038 } 1039 1040 1041// end addition 1042 1043}