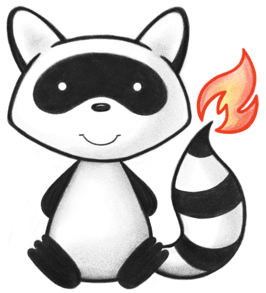
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 053 */ 054@ResourceDef(name="NamingSystem", profile="http://hl7.org/fhir/Profile/NamingSystem") 055@ChildOrder(names={"name", "status", "kind", "date", "publisher", "contact", "responsible", "type", "description", "useContext", "jurisdiction", "usage", "uniqueId", "replacedBy"}) 056public class NamingSystem extends MetadataResource { 057 058 public enum NamingSystemType { 059 /** 060 * The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 061 */ 062 CODESYSTEM, 063 /** 064 * The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.). 065 */ 066 IDENTIFIER, 067 /** 068 * The naming system is used as the root for other identifiers and naming systems. 069 */ 070 ROOT, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static NamingSystemType fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("codesystem".equals(codeString)) 079 return CODESYSTEM; 080 if ("identifier".equals(codeString)) 081 return IDENTIFIER; 082 if ("root".equals(codeString)) 083 return ROOT; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case CODESYSTEM: return "codesystem"; 092 case IDENTIFIER: return "identifier"; 093 case ROOT: return "root"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case CODESYSTEM: return "http://hl7.org/fhir/namingsystem-type"; 101 case IDENTIFIER: return "http://hl7.org/fhir/namingsystem-type"; 102 case ROOT: return "http://hl7.org/fhir/namingsystem-type"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case CODESYSTEM: return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 110 case IDENTIFIER: return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 111 case ROOT: return "The naming system is used as the root for other identifiers and naming systems."; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case CODESYSTEM: return "Code System"; 119 case IDENTIFIER: return "Identifier"; 120 case ROOT: return "Root"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 128 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("codesystem".equals(codeString)) 133 return NamingSystemType.CODESYSTEM; 134 if ("identifier".equals(codeString)) 135 return NamingSystemType.IDENTIFIER; 136 if ("root".equals(codeString)) 137 return NamingSystemType.ROOT; 138 throw new IllegalArgumentException("Unknown NamingSystemType code '"+codeString+"'"); 139 } 140 public Enumeration<NamingSystemType> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<NamingSystemType>(this); 145 String codeString = code.asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("codesystem".equals(codeString)) 149 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM); 150 if ("identifier".equals(codeString)) 151 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER); 152 if ("root".equals(codeString)) 153 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT); 154 throw new FHIRException("Unknown NamingSystemType code '"+codeString+"'"); 155 } 156 public String toCode(NamingSystemType code) { 157 if (code == NamingSystemType.NULL) 158 return null; 159 if (code == NamingSystemType.CODESYSTEM) 160 return "codesystem"; 161 if (code == NamingSystemType.IDENTIFIER) 162 return "identifier"; 163 if (code == NamingSystemType.ROOT) 164 return "root"; 165 return "?"; 166 } 167 public String toSystem(NamingSystemType code) { 168 return code.getSystem(); 169 } 170 } 171 172 public enum NamingSystemIdentifierType { 173 /** 174 * An ISO object identifier; e.g. 1.2.3.4.5. 175 */ 176 OID, 177 /** 178 * A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11. 179 */ 180 UUID, 181 /** 182 * A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org. 183 */ 184 URI, 185 /** 186 * Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC. 187 */ 188 OTHER, 189 /** 190 * added to help the parsers with the generic types 191 */ 192 NULL; 193 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 194 if (codeString == null || "".equals(codeString)) 195 return null; 196 if ("oid".equals(codeString)) 197 return OID; 198 if ("uuid".equals(codeString)) 199 return UUID; 200 if ("uri".equals(codeString)) 201 return URI; 202 if ("other".equals(codeString)) 203 return OTHER; 204 if (Configuration.isAcceptInvalidEnums()) 205 return null; 206 else 207 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 208 } 209 public String toCode() { 210 switch (this) { 211 case OID: return "oid"; 212 case UUID: return "uuid"; 213 case URI: return "uri"; 214 case OTHER: return "other"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getSystem() { 220 switch (this) { 221 case OID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 222 case UUID: return "http://hl7.org/fhir/namingsystem-identifier-type"; 223 case URI: return "http://hl7.org/fhir/namingsystem-identifier-type"; 224 case OTHER: return "http://hl7.org/fhir/namingsystem-identifier-type"; 225 case NULL: return null; 226 default: return "?"; 227 } 228 } 229 public String getDefinition() { 230 switch (this) { 231 case OID: return "An ISO object identifier; e.g. 1.2.3.4.5."; 232 case UUID: return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 233 case URI: return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 234 case OTHER: return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 235 case NULL: return null; 236 default: return "?"; 237 } 238 } 239 public String getDisplay() { 240 switch (this) { 241 case OID: return "OID"; 242 case UUID: return "UUID"; 243 case URI: return "URI"; 244 case OTHER: return "Other"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 } 250 251 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 252 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 253 if (codeString == null || "".equals(codeString)) 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("oid".equals(codeString)) 257 return NamingSystemIdentifierType.OID; 258 if ("uuid".equals(codeString)) 259 return NamingSystemIdentifierType.UUID; 260 if ("uri".equals(codeString)) 261 return NamingSystemIdentifierType.URI; 262 if ("other".equals(codeString)) 263 return NamingSystemIdentifierType.OTHER; 264 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 265 } 266 public Enumeration<NamingSystemIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 267 if (code == null) 268 return null; 269 if (code.isEmpty()) 270 return new Enumeration<NamingSystemIdentifierType>(this); 271 String codeString = code.asStringValue(); 272 if (codeString == null || "".equals(codeString)) 273 return null; 274 if ("oid".equals(codeString)) 275 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID); 276 if ("uuid".equals(codeString)) 277 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID); 278 if ("uri".equals(codeString)) 279 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI); 280 if ("other".equals(codeString)) 281 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER); 282 throw new FHIRException("Unknown NamingSystemIdentifierType code '"+codeString+"'"); 283 } 284 public String toCode(NamingSystemIdentifierType code) { 285 if (code == NamingSystemIdentifierType.NULL) 286 return null; 287 if (code == NamingSystemIdentifierType.OID) 288 return "oid"; 289 if (code == NamingSystemIdentifierType.UUID) 290 return "uuid"; 291 if (code == NamingSystemIdentifierType.URI) 292 return "uri"; 293 if (code == NamingSystemIdentifierType.OTHER) 294 return "other"; 295 return "?"; 296 } 297 public String toSystem(NamingSystemIdentifierType code) { 298 return code.getSystem(); 299 } 300 } 301 302 @Block() 303 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 304 /** 305 * Identifies the unique identifier scheme used for this particular identifier. 306 */ 307 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 308 @Description(shortDefinition="oid | uuid | uri | other", formalDefinition="Identifies the unique identifier scheme used for this particular identifier." ) 309 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-identifier-type") 310 protected Enumeration<NamingSystemIdentifierType> type; 311 312 /** 313 * The string that should be sent over the wire to identify the code system or identifier system. 314 */ 315 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 316 @Description(shortDefinition="The unique identifier", formalDefinition="The string that should be sent over the wire to identify the code system or identifier system." ) 317 protected StringType value; 318 319 /** 320 * Indicates whether this identifier is the "preferred" identifier of this type. 321 */ 322 @Child(name = "preferred", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 323 @Description(shortDefinition="Is this the id that should be used for this type", formalDefinition="Indicates whether this identifier is the \"preferred\" identifier of this type." ) 324 protected BooleanType preferred; 325 326 /** 327 * Notes about the past or intended usage of this identifier. 328 */ 329 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 330 @Description(shortDefinition="Notes about identifier usage", formalDefinition="Notes about the past or intended usage of this identifier." ) 331 protected StringType comment; 332 333 /** 334 * Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic. 335 */ 336 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 337 @Description(shortDefinition="When is identifier valid?", formalDefinition="Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic." ) 338 protected Period period; 339 340 private static final long serialVersionUID = -1458889328L; 341 342 /** 343 * Constructor 344 */ 345 public NamingSystemUniqueIdComponent() { 346 super(); 347 } 348 349 /** 350 * Constructor 351 */ 352 public NamingSystemUniqueIdComponent(Enumeration<NamingSystemIdentifierType> type, StringType value) { 353 super(); 354 this.type = type; 355 this.value = value; 356 } 357 358 /** 359 * @return {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 360 */ 361 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 362 if (this.type == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 365 else if (Configuration.doAutoCreate()) 366 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 367 return this.type; 368 } 369 370 public boolean hasTypeElement() { 371 return this.type != null && !this.type.isEmpty(); 372 } 373 374 public boolean hasType() { 375 return this.type != null && !this.type.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #type} (Identifies the unique identifier scheme used for this particular identifier.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 380 */ 381 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 382 this.type = value; 383 return this; 384 } 385 386 /** 387 * @return Identifies the unique identifier scheme used for this particular identifier. 388 */ 389 public NamingSystemIdentifierType getType() { 390 return this.type == null ? null : this.type.getValue(); 391 } 392 393 /** 394 * @param value Identifies the unique identifier scheme used for this particular identifier. 395 */ 396 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 397 if (this.type == null) 398 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 399 this.type.setValue(value); 400 return this; 401 } 402 403 /** 404 * @return {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 405 */ 406 public StringType getValueElement() { 407 if (this.value == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 410 else if (Configuration.doAutoCreate()) 411 this.value = new StringType(); // bb 412 return this.value; 413 } 414 415 public boolean hasValueElement() { 416 return this.value != null && !this.value.isEmpty(); 417 } 418 419 public boolean hasValue() { 420 return this.value != null && !this.value.isEmpty(); 421 } 422 423 /** 424 * @param value {@link #value} (The string that should be sent over the wire to identify the code system or identifier system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 425 */ 426 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 427 this.value = value; 428 return this; 429 } 430 431 /** 432 * @return The string that should be sent over the wire to identify the code system or identifier system. 433 */ 434 public String getValue() { 435 return this.value == null ? null : this.value.getValue(); 436 } 437 438 /** 439 * @param value The string that should be sent over the wire to identify the code system or identifier system. 440 */ 441 public NamingSystemUniqueIdComponent setValue(String value) { 442 if (this.value == null) 443 this.value = new StringType(); 444 this.value.setValue(value); 445 return this; 446 } 447 448 /** 449 * @return {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 450 */ 451 public BooleanType getPreferredElement() { 452 if (this.preferred == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 455 else if (Configuration.doAutoCreate()) 456 this.preferred = new BooleanType(); // bb 457 return this.preferred; 458 } 459 460 public boolean hasPreferredElement() { 461 return this.preferred != null && !this.preferred.isEmpty(); 462 } 463 464 public boolean hasPreferred() { 465 return this.preferred != null && !this.preferred.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #preferred} (Indicates whether this identifier is the "preferred" identifier of this type.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 470 */ 471 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 472 this.preferred = value; 473 return this; 474 } 475 476 /** 477 * @return Indicates whether this identifier is the "preferred" identifier of this type. 478 */ 479 public boolean getPreferred() { 480 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 481 } 482 483 /** 484 * @param value Indicates whether this identifier is the "preferred" identifier of this type. 485 */ 486 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 487 if (this.preferred == null) 488 this.preferred = new BooleanType(); 489 this.preferred.setValue(value); 490 return this; 491 } 492 493 /** 494 * @return {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 495 */ 496 public StringType getCommentElement() { 497 if (this.comment == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.comment"); 500 else if (Configuration.doAutoCreate()) 501 this.comment = new StringType(); // bb 502 return this.comment; 503 } 504 505 public boolean hasCommentElement() { 506 return this.comment != null && !this.comment.isEmpty(); 507 } 508 509 public boolean hasComment() { 510 return this.comment != null && !this.comment.isEmpty(); 511 } 512 513 /** 514 * @param value {@link #comment} (Notes about the past or intended usage of this identifier.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 515 */ 516 public NamingSystemUniqueIdComponent setCommentElement(StringType value) { 517 this.comment = value; 518 return this; 519 } 520 521 /** 522 * @return Notes about the past or intended usage of this identifier. 523 */ 524 public String getComment() { 525 return this.comment == null ? null : this.comment.getValue(); 526 } 527 528 /** 529 * @param value Notes about the past or intended usage of this identifier. 530 */ 531 public NamingSystemUniqueIdComponent setComment(String value) { 532 if (Utilities.noString(value)) 533 this.comment = null; 534 else { 535 if (this.comment == null) 536 this.comment = new StringType(); 537 this.comment.setValue(value); 538 } 539 return this; 540 } 541 542 /** 543 * @return {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 544 */ 545 public Period getPeriod() { 546 if (this.period == null) 547 if (Configuration.errorOnAutoCreate()) 548 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 549 else if (Configuration.doAutoCreate()) 550 this.period = new Period(); // cc 551 return this.period; 552 } 553 554 public boolean hasPeriod() { 555 return this.period != null && !this.period.isEmpty(); 556 } 557 558 /** 559 * @param value {@link #period} (Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.) 560 */ 561 public NamingSystemUniqueIdComponent setPeriod(Period value) { 562 this.period = value; 563 return this; 564 } 565 566 protected void listChildren(List<Property> children) { 567 super.listChildren(children); 568 children.add(new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type)); 569 children.add(new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value)); 570 children.add(new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred)); 571 children.add(new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment)); 572 children.add(new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period)); 573 } 574 575 @Override 576 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 577 switch (_hash) { 578 case 3575610: /*type*/ return new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type); 579 case 111972721: /*value*/ return new Property("value", "string", "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, value); 580 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred); 581 case 950398559: /*comment*/ return new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, comment); 582 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 0, 1, period); 583 default: return super.getNamedProperty(_hash, _name, _checkValid); 584 } 585 586 } 587 588 @Override 589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 590 switch (hash) { 591 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<NamingSystemIdentifierType> 592 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 593 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 594 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 595 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 596 default: return super.getProperty(hash, name, checkValid); 597 } 598 599 } 600 601 @Override 602 public Base setProperty(int hash, String name, Base value) throws FHIRException { 603 switch (hash) { 604 case 3575610: // type 605 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 606 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 607 return value; 608 case 111972721: // value 609 this.value = castToString(value); // StringType 610 return value; 611 case -1294005119: // preferred 612 this.preferred = castToBoolean(value); // BooleanType 613 return value; 614 case 950398559: // comment 615 this.comment = castToString(value); // StringType 616 return value; 617 case -991726143: // period 618 this.period = castToPeriod(value); // Period 619 return value; 620 default: return super.setProperty(hash, name, value); 621 } 622 623 } 624 625 @Override 626 public Base setProperty(String name, Base value) throws FHIRException { 627 if (name.equals("type")) { 628 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 629 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 630 } else if (name.equals("value")) { 631 this.value = castToString(value); // StringType 632 } else if (name.equals("preferred")) { 633 this.preferred = castToBoolean(value); // BooleanType 634 } else if (name.equals("comment")) { 635 this.comment = castToString(value); // StringType 636 } else if (name.equals("period")) { 637 this.period = castToPeriod(value); // Period 638 } else 639 return super.setProperty(name, value); 640 return value; 641 } 642 643 @Override 644 public Base makeProperty(int hash, String name) throws FHIRException { 645 switch (hash) { 646 case 3575610: return getTypeElement(); 647 case 111972721: return getValueElement(); 648 case -1294005119: return getPreferredElement(); 649 case 950398559: return getCommentElement(); 650 case -991726143: return getPeriod(); 651 default: return super.makeProperty(hash, name); 652 } 653 654 } 655 656 @Override 657 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 658 switch (hash) { 659 case 3575610: /*type*/ return new String[] {"code"}; 660 case 111972721: /*value*/ return new String[] {"string"}; 661 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 662 case 950398559: /*comment*/ return new String[] {"string"}; 663 case -991726143: /*period*/ return new String[] {"Period"}; 664 default: return super.getTypesForProperty(hash, name); 665 } 666 667 } 668 669 @Override 670 public Base addChild(String name) throws FHIRException { 671 if (name.equals("type")) { 672 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.type"); 673 } 674 else if (name.equals("value")) { 675 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.value"); 676 } 677 else if (name.equals("preferred")) { 678 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.preferred"); 679 } 680 else if (name.equals("comment")) { 681 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.comment"); 682 } 683 else if (name.equals("period")) { 684 this.period = new Period(); 685 return this.period; 686 } 687 else 688 return super.addChild(name); 689 } 690 691 public NamingSystemUniqueIdComponent copy() { 692 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 693 copyValues(dst); 694 dst.type = type == null ? null : type.copy(); 695 dst.value = value == null ? null : value.copy(); 696 dst.preferred = preferred == null ? null : preferred.copy(); 697 dst.comment = comment == null ? null : comment.copy(); 698 dst.period = period == null ? null : period.copy(); 699 return dst; 700 } 701 702 @Override 703 public boolean equalsDeep(Base other_) { 704 if (!super.equalsDeep(other_)) 705 return false; 706 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 707 return false; 708 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 709 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(preferred, o.preferred, true) 710 && compareDeep(comment, o.comment, true) && compareDeep(period, o.period, true); 711 } 712 713 @Override 714 public boolean equalsShallow(Base other_) { 715 if (!super.equalsShallow(other_)) 716 return false; 717 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 718 return false; 719 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 720 return compareValues(type, o.type, true) && compareValues(value, o.value, true) && compareValues(preferred, o.preferred, true) 721 && compareValues(comment, o.comment, true); 722 } 723 724 public boolean isEmpty() { 725 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, preferred, comment 726 , period); 727 } 728 729 public String fhirType() { 730 return "NamingSystem.uniqueId"; 731 732 } 733 734 } 735 736 /** 737 * Indicates the purpose for the naming system - what kinds of things does it make unique? 738 */ 739 @Child(name = "kind", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=false) 740 @Description(shortDefinition="codesystem | identifier | root", formalDefinition="Indicates the purpose for the naming system - what kinds of things does it make unique?" ) 741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/namingsystem-type") 742 protected Enumeration<NamingSystemType> kind; 743 744 /** 745 * The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 746 */ 747 @Child(name = "responsible", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 748 @Description(shortDefinition="Who maintains system namespace?", formalDefinition="The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision." ) 749 protected StringType responsible; 750 751 /** 752 * Categorizes a naming system for easier search by grouping related naming systems. 753 */ 754 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 755 @Description(shortDefinition="e.g. driver, provider, patient, bank etc.", formalDefinition="Categorizes a naming system for easier search by grouping related naming systems." ) 756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identifier-type") 757 protected CodeableConcept type; 758 759 /** 760 * Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 761 */ 762 @Child(name = "usage", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 763 @Description(shortDefinition="How/where is it used", formalDefinition="Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc." ) 764 protected StringType usage; 765 766 /** 767 * Indicates how the system may be identified when referenced in electronic exchange. 768 */ 769 @Child(name = "uniqueId", type = {}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 770 @Description(shortDefinition="Unique identifiers used for system", formalDefinition="Indicates how the system may be identified when referenced in electronic exchange." ) 771 protected List<NamingSystemUniqueIdComponent> uniqueId; 772 773 /** 774 * For naming systems that are retired, indicates the naming system that should be used in their place (if any). 775 */ 776 @Child(name = "replacedBy", type = {NamingSystem.class}, order=5, min=0, max=1, modifier=false, summary=false) 777 @Description(shortDefinition="Use this instead", formalDefinition="For naming systems that are retired, indicates the naming system that should be used in their place (if any)." ) 778 protected Reference replacedBy; 779 780 /** 781 * The actual object that is the target of the reference (For naming systems that are retired, indicates the naming system that should be used in their place (if any).) 782 */ 783 protected NamingSystem replacedByTarget; 784 785 private static final long serialVersionUID = -743416513L; 786 787 /** 788 * Constructor 789 */ 790 public NamingSystem() { 791 super(); 792 } 793 794 /** 795 * Constructor 796 */ 797 public NamingSystem(StringType name, Enumeration<PublicationStatus> status, Enumeration<NamingSystemType> kind, DateTimeType date) { 798 super(); 799 this.name = name; 800 this.status = status; 801 this.kind = kind; 802 this.date = date; 803 } 804 805 /** 806 * @return {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 807 */ 808 public StringType getNameElement() { 809 if (this.name == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create NamingSystem.name"); 812 else if (Configuration.doAutoCreate()) 813 this.name = new StringType(); // bb 814 return this.name; 815 } 816 817 public boolean hasNameElement() { 818 return this.name != null && !this.name.isEmpty(); 819 } 820 821 public boolean hasName() { 822 return this.name != null && !this.name.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #name} (A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 827 */ 828 public NamingSystem setNameElement(StringType value) { 829 this.name = value; 830 return this; 831 } 832 833 /** 834 * @return A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 835 */ 836 public String getName() { 837 return this.name == null ? null : this.name.getValue(); 838 } 839 840 /** 841 * @param value A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 842 */ 843 public NamingSystem setName(String value) { 844 if (this.name == null) 845 this.name = new StringType(); 846 this.name.setValue(value); 847 return this; 848 } 849 850 /** 851 * @return {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 852 */ 853 public Enumeration<PublicationStatus> getStatusElement() { 854 if (this.status == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create NamingSystem.status"); 857 else if (Configuration.doAutoCreate()) 858 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 859 return this.status; 860 } 861 862 public boolean hasStatusElement() { 863 return this.status != null && !this.status.isEmpty(); 864 } 865 866 public boolean hasStatus() { 867 return this.status != null && !this.status.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #status} (The status of this naming system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 872 */ 873 public NamingSystem setStatusElement(Enumeration<PublicationStatus> value) { 874 this.status = value; 875 return this; 876 } 877 878 /** 879 * @return The status of this naming system. Enables tracking the life-cycle of the content. 880 */ 881 public PublicationStatus getStatus() { 882 return this.status == null ? null : this.status.getValue(); 883 } 884 885 /** 886 * @param value The status of this naming system. Enables tracking the life-cycle of the content. 887 */ 888 public NamingSystem setStatus(PublicationStatus value) { 889 if (this.status == null) 890 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 891 this.status.setValue(value); 892 return this; 893 } 894 895 /** 896 * @return {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 897 */ 898 public Enumeration<NamingSystemType> getKindElement() { 899 if (this.kind == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create NamingSystem.kind"); 902 else if (Configuration.doAutoCreate()) 903 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 904 return this.kind; 905 } 906 907 public boolean hasKindElement() { 908 return this.kind != null && !this.kind.isEmpty(); 909 } 910 911 public boolean hasKind() { 912 return this.kind != null && !this.kind.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #kind} (Indicates the purpose for the naming system - what kinds of things does it make unique?). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 917 */ 918 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 919 this.kind = value; 920 return this; 921 } 922 923 /** 924 * @return Indicates the purpose for the naming system - what kinds of things does it make unique? 925 */ 926 public NamingSystemType getKind() { 927 return this.kind == null ? null : this.kind.getValue(); 928 } 929 930 /** 931 * @param value Indicates the purpose for the naming system - what kinds of things does it make unique? 932 */ 933 public NamingSystem setKind(NamingSystemType value) { 934 if (this.kind == null) 935 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 936 this.kind.setValue(value); 937 return this; 938 } 939 940 /** 941 * @return {@link #date} (The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 942 */ 943 public DateTimeType getDateElement() { 944 if (this.date == null) 945 if (Configuration.errorOnAutoCreate()) 946 throw new Error("Attempt to auto-create NamingSystem.date"); 947 else if (Configuration.doAutoCreate()) 948 this.date = new DateTimeType(); // bb 949 return this.date; 950 } 951 952 public boolean hasDateElement() { 953 return this.date != null && !this.date.isEmpty(); 954 } 955 956 public boolean hasDate() { 957 return this.date != null && !this.date.isEmpty(); 958 } 959 960 /** 961 * @param value {@link #date} (The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 962 */ 963 public NamingSystem setDateElement(DateTimeType value) { 964 this.date = value; 965 return this; 966 } 967 968 /** 969 * @return The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 970 */ 971 public Date getDate() { 972 return this.date == null ? null : this.date.getValue(); 973 } 974 975 /** 976 * @param value The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes. 977 */ 978 public NamingSystem setDate(Date value) { 979 if (this.date == null) 980 this.date = new DateTimeType(); 981 this.date.setValue(value); 982 return this; 983 } 984 985 /** 986 * @return {@link #publisher} (The name of the individual or organization that published the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 987 */ 988 public StringType getPublisherElement() { 989 if (this.publisher == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create NamingSystem.publisher"); 992 else if (Configuration.doAutoCreate()) 993 this.publisher = new StringType(); // bb 994 return this.publisher; 995 } 996 997 public boolean hasPublisherElement() { 998 return this.publisher != null && !this.publisher.isEmpty(); 999 } 1000 1001 public boolean hasPublisher() { 1002 return this.publisher != null && !this.publisher.isEmpty(); 1003 } 1004 1005 /** 1006 * @param value {@link #publisher} (The name of the individual or organization that published the naming system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1007 */ 1008 public NamingSystem setPublisherElement(StringType value) { 1009 this.publisher = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return The name of the individual or organization that published the naming system. 1015 */ 1016 public String getPublisher() { 1017 return this.publisher == null ? null : this.publisher.getValue(); 1018 } 1019 1020 /** 1021 * @param value The name of the individual or organization that published the naming system. 1022 */ 1023 public NamingSystem setPublisher(String value) { 1024 if (Utilities.noString(value)) 1025 this.publisher = null; 1026 else { 1027 if (this.publisher == null) 1028 this.publisher = new StringType(); 1029 this.publisher.setValue(value); 1030 } 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1036 */ 1037 public List<ContactDetail> getContact() { 1038 if (this.contact == null) 1039 this.contact = new ArrayList<ContactDetail>(); 1040 return this.contact; 1041 } 1042 1043 /** 1044 * @return Returns a reference to <code>this</code> for easy method chaining 1045 */ 1046 public NamingSystem setContact(List<ContactDetail> theContact) { 1047 this.contact = theContact; 1048 return this; 1049 } 1050 1051 public boolean hasContact() { 1052 if (this.contact == null) 1053 return false; 1054 for (ContactDetail item : this.contact) 1055 if (!item.isEmpty()) 1056 return true; 1057 return false; 1058 } 1059 1060 public ContactDetail addContact() { //3 1061 ContactDetail t = new ContactDetail(); 1062 if (this.contact == null) 1063 this.contact = new ArrayList<ContactDetail>(); 1064 this.contact.add(t); 1065 return t; 1066 } 1067 1068 public NamingSystem addContact(ContactDetail t) { //3 1069 if (t == null) 1070 return this; 1071 if (this.contact == null) 1072 this.contact = new ArrayList<ContactDetail>(); 1073 this.contact.add(t); 1074 return this; 1075 } 1076 1077 /** 1078 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1079 */ 1080 public ContactDetail getContactFirstRep() { 1081 if (getContact().isEmpty()) { 1082 addContact(); 1083 } 1084 return getContact().get(0); 1085 } 1086 1087 /** 1088 * @return {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1089 */ 1090 public StringType getResponsibleElement() { 1091 if (this.responsible == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1094 else if (Configuration.doAutoCreate()) 1095 this.responsible = new StringType(); // bb 1096 return this.responsible; 1097 } 1098 1099 public boolean hasResponsibleElement() { 1100 return this.responsible != null && !this.responsible.isEmpty(); 1101 } 1102 1103 public boolean hasResponsible() { 1104 return this.responsible != null && !this.responsible.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #responsible} (The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1109 */ 1110 public NamingSystem setResponsibleElement(StringType value) { 1111 this.responsible = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1117 */ 1118 public String getResponsible() { 1119 return this.responsible == null ? null : this.responsible.getValue(); 1120 } 1121 1122 /** 1123 * @param value The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision. 1124 */ 1125 public NamingSystem setResponsible(String value) { 1126 if (Utilities.noString(value)) 1127 this.responsible = null; 1128 else { 1129 if (this.responsible == null) 1130 this.responsible = new StringType(); 1131 this.responsible.setValue(value); 1132 } 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1138 */ 1139 public CodeableConcept getType() { 1140 if (this.type == null) 1141 if (Configuration.errorOnAutoCreate()) 1142 throw new Error("Attempt to auto-create NamingSystem.type"); 1143 else if (Configuration.doAutoCreate()) 1144 this.type = new CodeableConcept(); // cc 1145 return this.type; 1146 } 1147 1148 public boolean hasType() { 1149 return this.type != null && !this.type.isEmpty(); 1150 } 1151 1152 /** 1153 * @param value {@link #type} (Categorizes a naming system for easier search by grouping related naming systems.) 1154 */ 1155 public NamingSystem setType(CodeableConcept value) { 1156 this.type = value; 1157 return this; 1158 } 1159 1160 /** 1161 * @return {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1162 */ 1163 public MarkdownType getDescriptionElement() { 1164 if (this.description == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create NamingSystem.description"); 1167 else if (Configuration.doAutoCreate()) 1168 this.description = new MarkdownType(); // bb 1169 return this.description; 1170 } 1171 1172 public boolean hasDescriptionElement() { 1173 return this.description != null && !this.description.isEmpty(); 1174 } 1175 1176 public boolean hasDescription() { 1177 return this.description != null && !this.description.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #description} (A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1182 */ 1183 public NamingSystem setDescriptionElement(MarkdownType value) { 1184 this.description = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1190 */ 1191 public String getDescription() { 1192 return this.description == null ? null : this.description.getValue(); 1193 } 1194 1195 /** 1196 * @param value A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc. 1197 */ 1198 public NamingSystem setDescription(String value) { 1199 if (value == null) 1200 this.description = null; 1201 else { 1202 if (this.description == null) 1203 this.description = new MarkdownType(); 1204 this.description.setValue(value); 1205 } 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate naming system instances.) 1211 */ 1212 public List<UsageContext> getUseContext() { 1213 if (this.useContext == null) 1214 this.useContext = new ArrayList<UsageContext>(); 1215 return this.useContext; 1216 } 1217 1218 /** 1219 * @return Returns a reference to <code>this</code> for easy method chaining 1220 */ 1221 public NamingSystem setUseContext(List<UsageContext> theUseContext) { 1222 this.useContext = theUseContext; 1223 return this; 1224 } 1225 1226 public boolean hasUseContext() { 1227 if (this.useContext == null) 1228 return false; 1229 for (UsageContext item : this.useContext) 1230 if (!item.isEmpty()) 1231 return true; 1232 return false; 1233 } 1234 1235 public UsageContext addUseContext() { //3 1236 UsageContext t = new UsageContext(); 1237 if (this.useContext == null) 1238 this.useContext = new ArrayList<UsageContext>(); 1239 this.useContext.add(t); 1240 return t; 1241 } 1242 1243 public NamingSystem addUseContext(UsageContext t) { //3 1244 if (t == null) 1245 return this; 1246 if (this.useContext == null) 1247 this.useContext = new ArrayList<UsageContext>(); 1248 this.useContext.add(t); 1249 return this; 1250 } 1251 1252 /** 1253 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1254 */ 1255 public UsageContext getUseContextFirstRep() { 1256 if (getUseContext().isEmpty()) { 1257 addUseContext(); 1258 } 1259 return getUseContext().get(0); 1260 } 1261 1262 /** 1263 * @return {@link #jurisdiction} (A legal or geographic region in which the naming system is intended to be used.) 1264 */ 1265 public List<CodeableConcept> getJurisdiction() { 1266 if (this.jurisdiction == null) 1267 this.jurisdiction = new ArrayList<CodeableConcept>(); 1268 return this.jurisdiction; 1269 } 1270 1271 /** 1272 * @return Returns a reference to <code>this</code> for easy method chaining 1273 */ 1274 public NamingSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 1275 this.jurisdiction = theJurisdiction; 1276 return this; 1277 } 1278 1279 public boolean hasJurisdiction() { 1280 if (this.jurisdiction == null) 1281 return false; 1282 for (CodeableConcept item : this.jurisdiction) 1283 if (!item.isEmpty()) 1284 return true; 1285 return false; 1286 } 1287 1288 public CodeableConcept addJurisdiction() { //3 1289 CodeableConcept t = new CodeableConcept(); 1290 if (this.jurisdiction == null) 1291 this.jurisdiction = new ArrayList<CodeableConcept>(); 1292 this.jurisdiction.add(t); 1293 return t; 1294 } 1295 1296 public NamingSystem addJurisdiction(CodeableConcept t) { //3 1297 if (t == null) 1298 return this; 1299 if (this.jurisdiction == null) 1300 this.jurisdiction = new ArrayList<CodeableConcept>(); 1301 this.jurisdiction.add(t); 1302 return this; 1303 } 1304 1305 /** 1306 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1307 */ 1308 public CodeableConcept getJurisdictionFirstRep() { 1309 if (getJurisdiction().isEmpty()) { 1310 addJurisdiction(); 1311 } 1312 return getJurisdiction().get(0); 1313 } 1314 1315 /** 1316 * @return {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1317 */ 1318 public StringType getUsageElement() { 1319 if (this.usage == null) 1320 if (Configuration.errorOnAutoCreate()) 1321 throw new Error("Attempt to auto-create NamingSystem.usage"); 1322 else if (Configuration.doAutoCreate()) 1323 this.usage = new StringType(); // bb 1324 return this.usage; 1325 } 1326 1327 public boolean hasUsageElement() { 1328 return this.usage != null && !this.usage.isEmpty(); 1329 } 1330 1331 public boolean hasUsage() { 1332 return this.usage != null && !this.usage.isEmpty(); 1333 } 1334 1335 /** 1336 * @param value {@link #usage} (Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1337 */ 1338 public NamingSystem setUsageElement(StringType value) { 1339 this.usage = value; 1340 return this; 1341 } 1342 1343 /** 1344 * @return Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 1345 */ 1346 public String getUsage() { 1347 return this.usage == null ? null : this.usage.getValue(); 1348 } 1349 1350 /** 1351 * @param value Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc. 1352 */ 1353 public NamingSystem setUsage(String value) { 1354 if (Utilities.noString(value)) 1355 this.usage = null; 1356 else { 1357 if (this.usage == null) 1358 this.usage = new StringType(); 1359 this.usage.setValue(value); 1360 } 1361 return this; 1362 } 1363 1364 /** 1365 * @return {@link #uniqueId} (Indicates how the system may be identified when referenced in electronic exchange.) 1366 */ 1367 public List<NamingSystemUniqueIdComponent> getUniqueId() { 1368 if (this.uniqueId == null) 1369 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1370 return this.uniqueId; 1371 } 1372 1373 /** 1374 * @return Returns a reference to <code>this</code> for easy method chaining 1375 */ 1376 public NamingSystem setUniqueId(List<NamingSystemUniqueIdComponent> theUniqueId) { 1377 this.uniqueId = theUniqueId; 1378 return this; 1379 } 1380 1381 public boolean hasUniqueId() { 1382 if (this.uniqueId == null) 1383 return false; 1384 for (NamingSystemUniqueIdComponent item : this.uniqueId) 1385 if (!item.isEmpty()) 1386 return true; 1387 return false; 1388 } 1389 1390 public NamingSystemUniqueIdComponent addUniqueId() { //3 1391 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 1392 if (this.uniqueId == null) 1393 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1394 this.uniqueId.add(t); 1395 return t; 1396 } 1397 1398 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { //3 1399 if (t == null) 1400 return this; 1401 if (this.uniqueId == null) 1402 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1403 this.uniqueId.add(t); 1404 return this; 1405 } 1406 1407 /** 1408 * @return The first repetition of repeating field {@link #uniqueId}, creating it if it does not already exist 1409 */ 1410 public NamingSystemUniqueIdComponent getUniqueIdFirstRep() { 1411 if (getUniqueId().isEmpty()) { 1412 addUniqueId(); 1413 } 1414 return getUniqueId().get(0); 1415 } 1416 1417 /** 1418 * @return {@link #replacedBy} (For naming systems that are retired, indicates the naming system that should be used in their place (if any).) 1419 */ 1420 public Reference getReplacedBy() { 1421 if (this.replacedBy == null) 1422 if (Configuration.errorOnAutoCreate()) 1423 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1424 else if (Configuration.doAutoCreate()) 1425 this.replacedBy = new Reference(); // cc 1426 return this.replacedBy; 1427 } 1428 1429 public boolean hasReplacedBy() { 1430 return this.replacedBy != null && !this.replacedBy.isEmpty(); 1431 } 1432 1433 /** 1434 * @param value {@link #replacedBy} (For naming systems that are retired, indicates the naming system that should be used in their place (if any).) 1435 */ 1436 public NamingSystem setReplacedBy(Reference value) { 1437 this.replacedBy = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return {@link #replacedBy} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (For naming systems that are retired, indicates the naming system that should be used in their place (if any).) 1443 */ 1444 public NamingSystem getReplacedByTarget() { 1445 if (this.replacedByTarget == null) 1446 if (Configuration.errorOnAutoCreate()) 1447 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1448 else if (Configuration.doAutoCreate()) 1449 this.replacedByTarget = new NamingSystem(); // aa 1450 return this.replacedByTarget; 1451 } 1452 1453 /** 1454 * @param value {@link #replacedBy} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (For naming systems that are retired, indicates the naming system that should be used in their place (if any).) 1455 */ 1456 public NamingSystem setReplacedByTarget(NamingSystem value) { 1457 this.replacedByTarget = value; 1458 return this; 1459 } 1460 1461 protected void listChildren(List<Property> children) { 1462 super.listChildren(children); 1463 children.add(new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1464 children.add(new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status)); 1465 children.add(new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind)); 1466 children.add(new Property("date", "dateTime", "The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date)); 1467 children.add(new Property("publisher", "string", "The name of the individual or organization that published the naming system.", 0, 1, publisher)); 1468 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1469 children.add(new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible)); 1470 children.add(new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type)); 1471 children.add(new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description)); 1472 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1473 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1474 children.add(new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage)); 1475 children.add(new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId)); 1476 children.add(new Property("replacedBy", "Reference(NamingSystem)", "For naming systems that are retired, indicates the naming system that should be used in their place (if any).", 0, 1, replacedBy)); 1477 } 1478 1479 @Override 1480 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1481 switch (_hash) { 1482 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1483 case -892481550: /*status*/ return new Property("status", "code", "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status); 1484 case 3292052: /*kind*/ return new Property("kind", "code", "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind); 1485 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the naming system was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 0, 1, date); 1486 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the naming system.", 0, 1, publisher); 1487 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1488 case 1847674614: /*responsible*/ return new Property("responsible", "string", "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 0, 1, responsible); 1489 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type); 1490 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1, description); 1491 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate naming system instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1492 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the naming system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1493 case 111574433: /*usage*/ return new Property("usage", "string", "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 0, 1, usage); 1494 case -294460212: /*uniqueId*/ return new Property("uniqueId", "", "Indicates how the system may be identified when referenced in electronic exchange.", 0, java.lang.Integer.MAX_VALUE, uniqueId); 1495 case -1233035097: /*replacedBy*/ return new Property("replacedBy", "Reference(NamingSystem)", "For naming systems that are retired, indicates the naming system that should be used in their place (if any).", 0, 1, replacedBy); 1496 default: return super.getNamedProperty(_hash, _name, _checkValid); 1497 } 1498 1499 } 1500 1501 @Override 1502 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1503 switch (hash) { 1504 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1505 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1506 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<NamingSystemType> 1507 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1508 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1509 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1510 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // StringType 1511 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1512 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1513 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1514 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1515 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 1516 case -294460212: /*uniqueId*/ return this.uniqueId == null ? new Base[0] : this.uniqueId.toArray(new Base[this.uniqueId.size()]); // NamingSystemUniqueIdComponent 1517 case -1233035097: /*replacedBy*/ return this.replacedBy == null ? new Base[0] : new Base[] {this.replacedBy}; // Reference 1518 default: return super.getProperty(hash, name, checkValid); 1519 } 1520 1521 } 1522 1523 @Override 1524 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1525 switch (hash) { 1526 case 3373707: // name 1527 this.name = castToString(value); // StringType 1528 return value; 1529 case -892481550: // status 1530 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1531 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1532 return value; 1533 case 3292052: // kind 1534 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1535 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1536 return value; 1537 case 3076014: // date 1538 this.date = castToDateTime(value); // DateTimeType 1539 return value; 1540 case 1447404028: // publisher 1541 this.publisher = castToString(value); // StringType 1542 return value; 1543 case 951526432: // contact 1544 this.getContact().add(castToContactDetail(value)); // ContactDetail 1545 return value; 1546 case 1847674614: // responsible 1547 this.responsible = castToString(value); // StringType 1548 return value; 1549 case 3575610: // type 1550 this.type = castToCodeableConcept(value); // CodeableConcept 1551 return value; 1552 case -1724546052: // description 1553 this.description = castToMarkdown(value); // MarkdownType 1554 return value; 1555 case -669707736: // useContext 1556 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1557 return value; 1558 case -507075711: // jurisdiction 1559 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1560 return value; 1561 case 111574433: // usage 1562 this.usage = castToString(value); // StringType 1563 return value; 1564 case -294460212: // uniqueId 1565 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); // NamingSystemUniqueIdComponent 1566 return value; 1567 case -1233035097: // replacedBy 1568 this.replacedBy = castToReference(value); // Reference 1569 return value; 1570 default: return super.setProperty(hash, name, value); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base setProperty(String name, Base value) throws FHIRException { 1577 if (name.equals("name")) { 1578 this.name = castToString(value); // StringType 1579 } else if (name.equals("status")) { 1580 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1581 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1582 } else if (name.equals("kind")) { 1583 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1584 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1585 } else if (name.equals("date")) { 1586 this.date = castToDateTime(value); // DateTimeType 1587 } else if (name.equals("publisher")) { 1588 this.publisher = castToString(value); // StringType 1589 } else if (name.equals("contact")) { 1590 this.getContact().add(castToContactDetail(value)); 1591 } else if (name.equals("responsible")) { 1592 this.responsible = castToString(value); // StringType 1593 } else if (name.equals("type")) { 1594 this.type = castToCodeableConcept(value); // CodeableConcept 1595 } else if (name.equals("description")) { 1596 this.description = castToMarkdown(value); // MarkdownType 1597 } else if (name.equals("useContext")) { 1598 this.getUseContext().add(castToUsageContext(value)); 1599 } else if (name.equals("jurisdiction")) { 1600 this.getJurisdiction().add(castToCodeableConcept(value)); 1601 } else if (name.equals("usage")) { 1602 this.usage = castToString(value); // StringType 1603 } else if (name.equals("uniqueId")) { 1604 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 1605 } else if (name.equals("replacedBy")) { 1606 this.replacedBy = castToReference(value); // Reference 1607 } else 1608 return super.setProperty(name, value); 1609 return value; 1610 } 1611 1612 @Override 1613 public Base makeProperty(int hash, String name) throws FHIRException { 1614 switch (hash) { 1615 case 3373707: return getNameElement(); 1616 case -892481550: return getStatusElement(); 1617 case 3292052: return getKindElement(); 1618 case 3076014: return getDateElement(); 1619 case 1447404028: return getPublisherElement(); 1620 case 951526432: return addContact(); 1621 case 1847674614: return getResponsibleElement(); 1622 case 3575610: return getType(); 1623 case -1724546052: return getDescriptionElement(); 1624 case -669707736: return addUseContext(); 1625 case -507075711: return addJurisdiction(); 1626 case 111574433: return getUsageElement(); 1627 case -294460212: return addUniqueId(); 1628 case -1233035097: return getReplacedBy(); 1629 default: return super.makeProperty(hash, name); 1630 } 1631 1632 } 1633 1634 @Override 1635 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1636 switch (hash) { 1637 case 3373707: /*name*/ return new String[] {"string"}; 1638 case -892481550: /*status*/ return new String[] {"code"}; 1639 case 3292052: /*kind*/ return new String[] {"code"}; 1640 case 3076014: /*date*/ return new String[] {"dateTime"}; 1641 case 1447404028: /*publisher*/ return new String[] {"string"}; 1642 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1643 case 1847674614: /*responsible*/ return new String[] {"string"}; 1644 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1645 case -1724546052: /*description*/ return new String[] {"markdown"}; 1646 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1647 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1648 case 111574433: /*usage*/ return new String[] {"string"}; 1649 case -294460212: /*uniqueId*/ return new String[] {}; 1650 case -1233035097: /*replacedBy*/ return new String[] {"Reference"}; 1651 default: return super.getTypesForProperty(hash, name); 1652 } 1653 1654 } 1655 1656 @Override 1657 public Base addChild(String name) throws FHIRException { 1658 if (name.equals("name")) { 1659 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 1660 } 1661 else if (name.equals("status")) { 1662 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 1663 } 1664 else if (name.equals("kind")) { 1665 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 1666 } 1667 else if (name.equals("date")) { 1668 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 1669 } 1670 else if (name.equals("publisher")) { 1671 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 1672 } 1673 else if (name.equals("contact")) { 1674 return addContact(); 1675 } 1676 else if (name.equals("responsible")) { 1677 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 1678 } 1679 else if (name.equals("type")) { 1680 this.type = new CodeableConcept(); 1681 return this.type; 1682 } 1683 else if (name.equals("description")) { 1684 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 1685 } 1686 else if (name.equals("useContext")) { 1687 return addUseContext(); 1688 } 1689 else if (name.equals("jurisdiction")) { 1690 return addJurisdiction(); 1691 } 1692 else if (name.equals("usage")) { 1693 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 1694 } 1695 else if (name.equals("uniqueId")) { 1696 return addUniqueId(); 1697 } 1698 else if (name.equals("replacedBy")) { 1699 this.replacedBy = new Reference(); 1700 return this.replacedBy; 1701 } 1702 else 1703 return super.addChild(name); 1704 } 1705 1706 public String fhirType() { 1707 return "NamingSystem"; 1708 1709 } 1710 1711 public NamingSystem copy() { 1712 NamingSystem dst = new NamingSystem(); 1713 copyValues(dst); 1714 dst.name = name == null ? null : name.copy(); 1715 dst.status = status == null ? null : status.copy(); 1716 dst.kind = kind == null ? null : kind.copy(); 1717 dst.date = date == null ? null : date.copy(); 1718 dst.publisher = publisher == null ? null : publisher.copy(); 1719 if (contact != null) { 1720 dst.contact = new ArrayList<ContactDetail>(); 1721 for (ContactDetail i : contact) 1722 dst.contact.add(i.copy()); 1723 }; 1724 dst.responsible = responsible == null ? null : responsible.copy(); 1725 dst.type = type == null ? null : type.copy(); 1726 dst.description = description == null ? null : description.copy(); 1727 if (useContext != null) { 1728 dst.useContext = new ArrayList<UsageContext>(); 1729 for (UsageContext i : useContext) 1730 dst.useContext.add(i.copy()); 1731 }; 1732 if (jurisdiction != null) { 1733 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1734 for (CodeableConcept i : jurisdiction) 1735 dst.jurisdiction.add(i.copy()); 1736 }; 1737 dst.usage = usage == null ? null : usage.copy(); 1738 if (uniqueId != null) { 1739 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1740 for (NamingSystemUniqueIdComponent i : uniqueId) 1741 dst.uniqueId.add(i.copy()); 1742 }; 1743 dst.replacedBy = replacedBy == null ? null : replacedBy.copy(); 1744 return dst; 1745 } 1746 1747 protected NamingSystem typedCopy() { 1748 return copy(); 1749 } 1750 1751 @Override 1752 public boolean equalsDeep(Base other_) { 1753 if (!super.equalsDeep(other_)) 1754 return false; 1755 if (!(other_ instanceof NamingSystem)) 1756 return false; 1757 NamingSystem o = (NamingSystem) other_; 1758 return compareDeep(kind, o.kind, true) && compareDeep(responsible, o.responsible, true) && compareDeep(type, o.type, true) 1759 && compareDeep(usage, o.usage, true) && compareDeep(uniqueId, o.uniqueId, true) && compareDeep(replacedBy, o.replacedBy, true) 1760 ; 1761 } 1762 1763 @Override 1764 public boolean equalsShallow(Base other_) { 1765 if (!super.equalsShallow(other_)) 1766 return false; 1767 if (!(other_ instanceof NamingSystem)) 1768 return false; 1769 NamingSystem o = (NamingSystem) other_; 1770 return compareValues(kind, o.kind, true) && compareValues(responsible, o.responsible, true) && compareValues(usage, o.usage, true) 1771 ; 1772 } 1773 1774 public boolean isEmpty() { 1775 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, responsible, type 1776 , usage, uniqueId, replacedBy); 1777 } 1778 1779 @Override 1780 public ResourceType getResourceType() { 1781 return ResourceType.NamingSystem; 1782 } 1783 1784 /** 1785 * Search parameter: <b>date</b> 1786 * <p> 1787 * Description: <b>The naming system publication date</b><br> 1788 * Type: <b>date</b><br> 1789 * Path: <b>NamingSystem.date</b><br> 1790 * </p> 1791 */ 1792 @SearchParamDefinition(name="date", path="NamingSystem.date", description="The naming system publication date", type="date" ) 1793 public static final String SP_DATE = "date"; 1794 /** 1795 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1796 * <p> 1797 * Description: <b>The naming system publication date</b><br> 1798 * Type: <b>date</b><br> 1799 * Path: <b>NamingSystem.date</b><br> 1800 * </p> 1801 */ 1802 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1803 1804 /** 1805 * Search parameter: <b>period</b> 1806 * <p> 1807 * Description: <b>When is identifier valid?</b><br> 1808 * Type: <b>date</b><br> 1809 * Path: <b>NamingSystem.uniqueId.period</b><br> 1810 * </p> 1811 */ 1812 @SearchParamDefinition(name="period", path="NamingSystem.uniqueId.period", description="When is identifier valid?", type="date" ) 1813 public static final String SP_PERIOD = "period"; 1814 /** 1815 * <b>Fluent Client</b> search parameter constant for <b>period</b> 1816 * <p> 1817 * Description: <b>When is identifier valid?</b><br> 1818 * Type: <b>date</b><br> 1819 * Path: <b>NamingSystem.uniqueId.period</b><br> 1820 * </p> 1821 */ 1822 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 1823 1824 /** 1825 * Search parameter: <b>kind</b> 1826 * <p> 1827 * Description: <b>codesystem | identifier | root</b><br> 1828 * Type: <b>token</b><br> 1829 * Path: <b>NamingSystem.kind</b><br> 1830 * </p> 1831 */ 1832 @SearchParamDefinition(name="kind", path="NamingSystem.kind", description="codesystem | identifier | root", type="token" ) 1833 public static final String SP_KIND = "kind"; 1834 /** 1835 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 1836 * <p> 1837 * Description: <b>codesystem | identifier | root</b><br> 1838 * Type: <b>token</b><br> 1839 * Path: <b>NamingSystem.kind</b><br> 1840 * </p> 1841 */ 1842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 1843 1844 /** 1845 * Search parameter: <b>jurisdiction</b> 1846 * <p> 1847 * Description: <b>Intended jurisdiction for the naming system</b><br> 1848 * Type: <b>token</b><br> 1849 * Path: <b>NamingSystem.jurisdiction</b><br> 1850 * </p> 1851 */ 1852 @SearchParamDefinition(name="jurisdiction", path="NamingSystem.jurisdiction", description="Intended jurisdiction for the naming system", type="token" ) 1853 public static final String SP_JURISDICTION = "jurisdiction"; 1854 /** 1855 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 1856 * <p> 1857 * Description: <b>Intended jurisdiction for the naming system</b><br> 1858 * Type: <b>token</b><br> 1859 * Path: <b>NamingSystem.jurisdiction</b><br> 1860 * </p> 1861 */ 1862 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 1863 1864 /** 1865 * Search parameter: <b>description</b> 1866 * <p> 1867 * Description: <b>The description of the naming system</b><br> 1868 * Type: <b>string</b><br> 1869 * Path: <b>NamingSystem.description</b><br> 1870 * </p> 1871 */ 1872 @SearchParamDefinition(name="description", path="NamingSystem.description", description="The description of the naming system", type="string" ) 1873 public static final String SP_DESCRIPTION = "description"; 1874 /** 1875 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1876 * <p> 1877 * Description: <b>The description of the naming system</b><br> 1878 * Type: <b>string</b><br> 1879 * Path: <b>NamingSystem.description</b><br> 1880 * </p> 1881 */ 1882 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 1883 1884 /** 1885 * Search parameter: <b>type</b> 1886 * <p> 1887 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 1888 * Type: <b>token</b><br> 1889 * Path: <b>NamingSystem.type</b><br> 1890 * </p> 1891 */ 1892 @SearchParamDefinition(name="type", path="NamingSystem.type", description="e.g. driver, provider, patient, bank etc.", type="token" ) 1893 public static final String SP_TYPE = "type"; 1894 /** 1895 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1896 * <p> 1897 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 1898 * Type: <b>token</b><br> 1899 * Path: <b>NamingSystem.type</b><br> 1900 * </p> 1901 */ 1902 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1903 1904 /** 1905 * Search parameter: <b>id-type</b> 1906 * <p> 1907 * Description: <b>oid | uuid | uri | other</b><br> 1908 * Type: <b>token</b><br> 1909 * Path: <b>NamingSystem.uniqueId.type</b><br> 1910 * </p> 1911 */ 1912 @SearchParamDefinition(name="id-type", path="NamingSystem.uniqueId.type", description="oid | uuid | uri | other", type="token" ) 1913 public static final String SP_ID_TYPE = "id-type"; 1914 /** 1915 * <b>Fluent Client</b> search parameter constant for <b>id-type</b> 1916 * <p> 1917 * Description: <b>oid | uuid | uri | other</b><br> 1918 * Type: <b>token</b><br> 1919 * Path: <b>NamingSystem.uniqueId.type</b><br> 1920 * </p> 1921 */ 1922 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ID_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ID_TYPE); 1923 1924 /** 1925 * Search parameter: <b>responsible</b> 1926 * <p> 1927 * Description: <b>Who maintains system namespace?</b><br> 1928 * Type: <b>string</b><br> 1929 * Path: <b>NamingSystem.responsible</b><br> 1930 * </p> 1931 */ 1932 @SearchParamDefinition(name="responsible", path="NamingSystem.responsible", description="Who maintains system namespace?", type="string" ) 1933 public static final String SP_RESPONSIBLE = "responsible"; 1934 /** 1935 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 1936 * <p> 1937 * Description: <b>Who maintains system namespace?</b><br> 1938 * Type: <b>string</b><br> 1939 * Path: <b>NamingSystem.responsible</b><br> 1940 * </p> 1941 */ 1942 public static final ca.uhn.fhir.rest.gclient.StringClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_RESPONSIBLE); 1943 1944 /** 1945 * Search parameter: <b>contact</b> 1946 * <p> 1947 * Description: <b>Name of an individual to contact</b><br> 1948 * Type: <b>string</b><br> 1949 * Path: <b>NamingSystem.contact.name</b><br> 1950 * </p> 1951 */ 1952 @SearchParamDefinition(name="contact", path="NamingSystem.contact.name", description="Name of an individual to contact", type="string" ) 1953 public static final String SP_CONTACT = "contact"; 1954 /** 1955 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 1956 * <p> 1957 * Description: <b>Name of an individual to contact</b><br> 1958 * Type: <b>string</b><br> 1959 * Path: <b>NamingSystem.contact.name</b><br> 1960 * </p> 1961 */ 1962 public static final ca.uhn.fhir.rest.gclient.StringClientParam CONTACT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CONTACT); 1963 1964 /** 1965 * Search parameter: <b>name</b> 1966 * <p> 1967 * Description: <b>Computationally friendly name of the naming system</b><br> 1968 * Type: <b>string</b><br> 1969 * Path: <b>NamingSystem.name</b><br> 1970 * </p> 1971 */ 1972 @SearchParamDefinition(name="name", path="NamingSystem.name", description="Computationally friendly name of the naming system", type="string" ) 1973 public static final String SP_NAME = "name"; 1974 /** 1975 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1976 * <p> 1977 * Description: <b>Computationally friendly name of the naming system</b><br> 1978 * Type: <b>string</b><br> 1979 * Path: <b>NamingSystem.name</b><br> 1980 * </p> 1981 */ 1982 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1983 1984 /** 1985 * Search parameter: <b>publisher</b> 1986 * <p> 1987 * Description: <b>Name of the publisher of the naming system</b><br> 1988 * Type: <b>string</b><br> 1989 * Path: <b>NamingSystem.publisher</b><br> 1990 * </p> 1991 */ 1992 @SearchParamDefinition(name="publisher", path="NamingSystem.publisher", description="Name of the publisher of the naming system", type="string" ) 1993 public static final String SP_PUBLISHER = "publisher"; 1994 /** 1995 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 1996 * <p> 1997 * Description: <b>Name of the publisher of the naming system</b><br> 1998 * Type: <b>string</b><br> 1999 * Path: <b>NamingSystem.publisher</b><br> 2000 * </p> 2001 */ 2002 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2003 2004 /** 2005 * Search parameter: <b>telecom</b> 2006 * <p> 2007 * Description: <b>Contact details for individual or organization</b><br> 2008 * Type: <b>token</b><br> 2009 * Path: <b>NamingSystem.contact.telecom</b><br> 2010 * </p> 2011 */ 2012 @SearchParamDefinition(name="telecom", path="NamingSystem.contact.telecom", description="Contact details for individual or organization", type="token" ) 2013 public static final String SP_TELECOM = "telecom"; 2014 /** 2015 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2016 * <p> 2017 * Description: <b>Contact details for individual or organization</b><br> 2018 * Type: <b>token</b><br> 2019 * Path: <b>NamingSystem.contact.telecom</b><br> 2020 * </p> 2021 */ 2022 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2023 2024 /** 2025 * Search parameter: <b>value</b> 2026 * <p> 2027 * Description: <b>The unique identifier</b><br> 2028 * Type: <b>string</b><br> 2029 * Path: <b>NamingSystem.uniqueId.value</b><br> 2030 * </p> 2031 */ 2032 @SearchParamDefinition(name="value", path="NamingSystem.uniqueId.value", description="The unique identifier", type="string" ) 2033 public static final String SP_VALUE = "value"; 2034 /** 2035 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2036 * <p> 2037 * Description: <b>The unique identifier</b><br> 2038 * Type: <b>string</b><br> 2039 * Path: <b>NamingSystem.uniqueId.value</b><br> 2040 * </p> 2041 */ 2042 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE); 2043 2044 /** 2045 * Search parameter: <b>replaced-by</b> 2046 * <p> 2047 * Description: <b>Use this instead</b><br> 2048 * Type: <b>reference</b><br> 2049 * Path: <b>NamingSystem.replacedBy</b><br> 2050 * </p> 2051 */ 2052 @SearchParamDefinition(name="replaced-by", path="NamingSystem.replacedBy", description="Use this instead", type="reference", target={NamingSystem.class } ) 2053 public static final String SP_REPLACED_BY = "replaced-by"; 2054 /** 2055 * <b>Fluent Client</b> search parameter constant for <b>replaced-by</b> 2056 * <p> 2057 * Description: <b>Use this instead</b><br> 2058 * Type: <b>reference</b><br> 2059 * Path: <b>NamingSystem.replacedBy</b><br> 2060 * </p> 2061 */ 2062 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACED_BY); 2063 2064/** 2065 * Constant for fluent queries to be used to add include statements. Specifies 2066 * the path value of "<b>NamingSystem:replaced-by</b>". 2067 */ 2068 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACED_BY = new ca.uhn.fhir.model.api.Include("NamingSystem:replaced-by").toLocked(); 2069 2070 /** 2071 * Search parameter: <b>status</b> 2072 * <p> 2073 * Description: <b>The current status of the naming system</b><br> 2074 * Type: <b>token</b><br> 2075 * Path: <b>NamingSystem.status</b><br> 2076 * </p> 2077 */ 2078 @SearchParamDefinition(name="status", path="NamingSystem.status", description="The current status of the naming system", type="token" ) 2079 public static final String SP_STATUS = "status"; 2080 /** 2081 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2082 * <p> 2083 * Description: <b>The current status of the naming system</b><br> 2084 * Type: <b>token</b><br> 2085 * Path: <b>NamingSystem.status</b><br> 2086 * </p> 2087 */ 2088 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2089 2090 @Override 2091 protected void checkCanUseUrl() { 2092 throw new Error("URL cannot be used on NamingSystem"); 2093 } 2094 2095 2096}