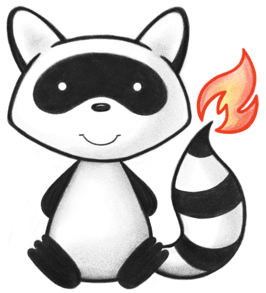
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.INarrative; 039import org.hl7.fhir.utilities.xhtml.XhtmlNode; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * A human-readable formatted text, including images. 046 */ 047@DatatypeDef(name="Narrative") 048public class Narrative extends BaseNarrative implements INarrative { 049 050 public enum NarrativeStatus { 051 /** 052 * The contents of the narrative are entirely generated from the structured data in the content. 053 */ 054 GENERATED, 055 /** 056 * The contents of the narrative are entirely generated from the structured data in the content and some of the content is generated from extensions 057 */ 058 EXTENSIONS, 059 /** 060 * The contents of the narrative may contain additional information not found in the structured data. Note that there is no computable way to determine what the extra information is, other than by human inspection 061 */ 062 ADDITIONAL, 063 /** 064 * The contents of the narrative are some equivalent of "No human-readable text provided in this case" 065 */ 066 EMPTY, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 public static NarrativeStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("generated".equals(codeString)) 075 return GENERATED; 076 if ("extensions".equals(codeString)) 077 return EXTENSIONS; 078 if ("additional".equals(codeString)) 079 return ADDITIONAL; 080 if ("empty".equals(codeString)) 081 return EMPTY; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown NarrativeStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case GENERATED: return "generated"; 090 case EXTENSIONS: return "extensions"; 091 case ADDITIONAL: return "additional"; 092 case EMPTY: return "empty"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getSystem() { 098 switch (this) { 099 case GENERATED: return "http://hl7.org/fhir/narrative-status"; 100 case EXTENSIONS: return "http://hl7.org/fhir/narrative-status"; 101 case ADDITIONAL: return "http://hl7.org/fhir/narrative-status"; 102 case EMPTY: return "http://hl7.org/fhir/narrative-status"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case GENERATED: return "The contents of the narrative are entirely generated from the structured data in the content."; 110 case EXTENSIONS: return "The contents of the narrative are entirely generated from the structured data in the content and some of the content is generated from extensions"; 111 case ADDITIONAL: return "The contents of the narrative may contain additional information not found in the structured data. Note that there is no computable way to determine what the extra information is, other than by human inspection"; 112 case EMPTY: return "The contents of the narrative are some equivalent of \"No human-readable text provided in this case\""; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getDisplay() { 118 switch (this) { 119 case GENERATED: return "Generated"; 120 case EXTENSIONS: return "Extensions"; 121 case ADDITIONAL: return "Additional"; 122 case EMPTY: return "Empty"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 } 128 129 public static class NarrativeStatusEnumFactory implements EnumFactory<NarrativeStatus> { 130 public NarrativeStatus fromCode(String codeString) throws IllegalArgumentException { 131 if (codeString == null || "".equals(codeString)) 132 if (codeString == null || "".equals(codeString)) 133 return null; 134 if ("generated".equals(codeString)) 135 return NarrativeStatus.GENERATED; 136 if ("extensions".equals(codeString)) 137 return NarrativeStatus.EXTENSIONS; 138 if ("additional".equals(codeString)) 139 return NarrativeStatus.ADDITIONAL; 140 if ("empty".equals(codeString)) 141 return NarrativeStatus.EMPTY; 142 throw new IllegalArgumentException("Unknown NarrativeStatus code '"+codeString+"'"); 143 } 144 public Enumeration<NarrativeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 145 if (code == null) 146 return null; 147 if (code.isEmpty()) 148 return new Enumeration<NarrativeStatus>(this); 149 String codeString = code.asStringValue(); 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("generated".equals(codeString)) 153 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.GENERATED); 154 if ("extensions".equals(codeString)) 155 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EXTENSIONS); 156 if ("additional".equals(codeString)) 157 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.ADDITIONAL); 158 if ("empty".equals(codeString)) 159 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EMPTY); 160 throw new FHIRException("Unknown NarrativeStatus code '"+codeString+"'"); 161 } 162 public String toCode(NarrativeStatus code) { 163 if (code == NarrativeStatus.NULL) 164 return null; 165 if (code == NarrativeStatus.GENERATED) 166 return "generated"; 167 if (code == NarrativeStatus.EXTENSIONS) 168 return "extensions"; 169 if (code == NarrativeStatus.ADDITIONAL) 170 return "additional"; 171 if (code == NarrativeStatus.EMPTY) 172 return "empty"; 173 return "?"; 174 } 175 public String toSystem(NarrativeStatus code) { 176 return code.getSystem(); 177 } 178 } 179 180 /** 181 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 182 */ 183 @Child(name = "status", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="generated | extensions | additional | empty", formalDefinition="The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data." ) 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/narrative-status") 186 protected Enumeration<NarrativeStatus> status; 187 188 /** 189 * The actual narrative content, a stripped down version of XHTML. 190 */ 191 @Child(name = "div", type = {}, order=1, min=1, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="Limited xhtml content", formalDefinition="The actual narrative content, a stripped down version of XHTML." ) 193 protected XhtmlNode div; 194 195 private static final long serialVersionUID = 1463852859L; 196 197 /** 198 * Constructor 199 */ 200 public Narrative() { 201 super(); 202 } 203 204 /** 205 * Constructor 206 */ 207 public Narrative(Enumeration<NarrativeStatus> status, XhtmlNode div) { 208 super(); 209 this.status = status; 210 this.div = div; 211 } 212 213 /** 214 * @return {@link #status} (The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 215 */ 216 public Enumeration<NarrativeStatus> getStatusElement() { 217 if (this.status == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create Narrative.status"); 220 else if (Configuration.doAutoCreate()) 221 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); // bb 222 return this.status; 223 } 224 225 public boolean hasStatusElement() { 226 return this.status != null && !this.status.isEmpty(); 227 } 228 229 public boolean hasStatus() { 230 return this.status != null && !this.status.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #status} (The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 235 */ 236 public Narrative setStatusElement(Enumeration<NarrativeStatus> value) { 237 this.status = value; 238 return this; 239 } 240 241 /** 242 * @return The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 243 */ 244 public NarrativeStatus getStatus() { 245 return this.status == null ? null : this.status.getValue(); 246 } 247 248 /** 249 * @param value The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data. 250 */ 251 public Narrative setStatus(NarrativeStatus value) { 252 if (this.status == null) 253 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); 254 this.status.setValue(value); 255 return this; 256 } 257 258 /** 259 * @return {@link #div} (The actual narrative content, a stripped down version of XHTML.) 260 */ 261 public XhtmlNode getDiv() { 262 if (this.div == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create Narrative.div"); 265 else if (Configuration.doAutoCreate()) 266 this.div = new XhtmlNode(); // cc 267 return this.div; 268 } 269 270 public boolean hasDiv() { 271 return this.div != null && !this.div.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #div} (The actual narrative content, a stripped down version of XHTML.) 276 */ 277 public Narrative setDiv(XhtmlNode value) { 278 this.div = value; 279 return this; 280 } 281 282 protected void listChildren(List<Property> children) { 283 super.listChildren(children); 284 children.add(new Property("status", "code", "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 0, 1, status)); 285 } 286 287 @Override 288 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 289 switch (_hash) { 290 case -892481550: /*status*/ return new Property("status", "code", "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 0, 1, status); 291 default: return super.getNamedProperty(_hash, _name, _checkValid); 292 } 293 294 } 295 296 @Override 297 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 298 switch (hash) { 299 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<NarrativeStatus> 300 case 99473: /*div*/ return this.div == null ? new Base[0] : new Base[] {new StringType(new org.hl7.fhir.utilities.xhtml.XhtmlComposer(true).composeEx(this.div))}; // XhtmlNode 301 default: return super.getProperty(hash, name, checkValid); 302 } 303 304 } 305 306 @Override 307 public Base setProperty(int hash, String name, Base value) throws FHIRException { 308 switch (hash) { 309 case -892481550: // status 310 value = new NarrativeStatusEnumFactory().fromType(castToCode(value)); 311 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 312 return value; 313 case 99473: // div 314 this.div = castToXhtml(value); // XhtmlNode 315 return value; 316 default: return super.setProperty(hash, name, value); 317 } 318 319 } 320 321 @Override 322 public Base setProperty(String name, Base value) throws FHIRException { 323 if (name.equals("status")) { 324 value = new NarrativeStatusEnumFactory().fromType(castToCode(value)); 325 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 326 } else if (name.equals("div")) { 327 this.div = castToXhtml(value); // XhtmlNode 328 } else 329 return super.setProperty(name, value); 330 return value; 331 } 332 333 @Override 334 public Base makeProperty(int hash, String name) throws FHIRException { 335 switch (hash) { 336 case -892481550: return getStatusElement(); 337 default: return super.makeProperty(hash, name); 338 } 339 340 } 341 342 @Override 343 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 344 switch (hash) { 345 case -892481550: /*status*/ return new String[] {"code"}; 346 case 99473: /*div*/ return new String[] {"xhtml"}; 347 default: return super.getTypesForProperty(hash, name); 348 } 349 350 } 351 352 @Override 353 public Base addChild(String name) throws FHIRException { 354 if (name.equals("status")) { 355 throw new FHIRException("Cannot call addChild on a singleton property Narrative.status"); 356 } 357 else 358 return super.addChild(name); 359 } 360 361 public String fhirType() { 362 return "Narrative"; 363 364 } 365 366 public Narrative copy() { 367 Narrative dst = new Narrative(); 368 copyValues(dst); 369 dst.status = status == null ? null : status.copy(); 370 dst.div = div == null ? null : div.copy(); 371 return dst; 372 } 373 374 protected Narrative typedCopy() { 375 return copy(); 376 } 377 378 @Override 379 public boolean equalsDeep(Base other_) { 380 if (!super.equalsDeep(other_)) 381 return false; 382 if (!(other_ instanceof Narrative)) 383 return false; 384 Narrative o = (Narrative) other_; 385 return compareDeep(status, o.status, true) && compareDeep(div, o.div, true); 386 } 387 388 @Override 389 public boolean equalsShallow(Base other_) { 390 if (!super.equalsShallow(other_)) 391 return false; 392 if (!(other_ instanceof Narrative)) 393 return false; 394 Narrative o = (Narrative) other_; 395 return compareValues(status, o.status, true); 396 } 397 398 public boolean isEmpty() { 399 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, div); 400 } 401 402 403}