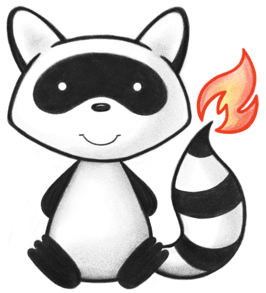
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 051 */ 052@ResourceDef(name="NutritionOrder", profile="http://hl7.org/fhir/Profile/NutritionOrder") 053public class NutritionOrder extends DomainResource { 054 055 public enum NutritionOrderStatus { 056 /** 057 * The request has been proposed. 058 */ 059 PROPOSED, 060 /** 061 * The request is in preliminary form prior to being sent. 062 */ 063 DRAFT, 064 /** 065 * The request has been planned. 066 */ 067 PLANNED, 068 /** 069 * The request has been placed. 070 */ 071 REQUESTED, 072 /** 073 * The request is 'actionable', but not all actions that are implied by it have occurred yet. 074 */ 075 ACTIVE, 076 /** 077 * Actions implied by the request have been temporarily halted, but are expected to continue later. May also be called "suspended". 078 */ 079 ONHOLD, 080 /** 081 * All actions that are implied by the order have occurred and no continuation is planned (this will rarely be made explicit). 082 */ 083 COMPLETED, 084 /** 085 * The request has been withdrawn and is no longer actionable. 086 */ 087 CANCELLED, 088 /** 089 * The request was entered in error and voided. 090 */ 091 ENTEREDINERROR, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 public static NutritionOrderStatus fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("proposed".equals(codeString)) 100 return PROPOSED; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("planned".equals(codeString)) 104 return PLANNED; 105 if ("requested".equals(codeString)) 106 return REQUESTED; 107 if ("active".equals(codeString)) 108 return ACTIVE; 109 if ("on-hold".equals(codeString)) 110 return ONHOLD; 111 if ("completed".equals(codeString)) 112 return COMPLETED; 113 if ("cancelled".equals(codeString)) 114 return CANCELLED; 115 if ("entered-in-error".equals(codeString)) 116 return ENTEREDINERROR; 117 if (Configuration.isAcceptInvalidEnums()) 118 return null; 119 else 120 throw new FHIRException("Unknown NutritionOrderStatus code '"+codeString+"'"); 121 } 122 public String toCode() { 123 switch (this) { 124 case PROPOSED: return "proposed"; 125 case DRAFT: return "draft"; 126 case PLANNED: return "planned"; 127 case REQUESTED: return "requested"; 128 case ACTIVE: return "active"; 129 case ONHOLD: return "on-hold"; 130 case COMPLETED: return "completed"; 131 case CANCELLED: return "cancelled"; 132 case ENTEREDINERROR: return "entered-in-error"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getSystem() { 138 switch (this) { 139 case PROPOSED: return "http://hl7.org/fhir/nutrition-request-status"; 140 case DRAFT: return "http://hl7.org/fhir/nutrition-request-status"; 141 case PLANNED: return "http://hl7.org/fhir/nutrition-request-status"; 142 case REQUESTED: return "http://hl7.org/fhir/nutrition-request-status"; 143 case ACTIVE: return "http://hl7.org/fhir/nutrition-request-status"; 144 case ONHOLD: return "http://hl7.org/fhir/nutrition-request-status"; 145 case COMPLETED: return "http://hl7.org/fhir/nutrition-request-status"; 146 case CANCELLED: return "http://hl7.org/fhir/nutrition-request-status"; 147 case ENTEREDINERROR: return "http://hl7.org/fhir/nutrition-request-status"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getDefinition() { 153 switch (this) { 154 case PROPOSED: return "The request has been proposed."; 155 case DRAFT: return "The request is in preliminary form prior to being sent."; 156 case PLANNED: return "The request has been planned."; 157 case REQUESTED: return "The request has been placed."; 158 case ACTIVE: return "The request is 'actionable', but not all actions that are implied by it have occurred yet."; 159 case ONHOLD: return "Actions implied by the request have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 160 case COMPLETED: return "All actions that are implied by the order have occurred and no continuation is planned (this will rarely be made explicit)."; 161 case CANCELLED: return "The request has been withdrawn and is no longer actionable."; 162 case ENTEREDINERROR: return "The request was entered in error and voided."; 163 case NULL: return null; 164 default: return "?"; 165 } 166 } 167 public String getDisplay() { 168 switch (this) { 169 case PROPOSED: return "Proposed"; 170 case DRAFT: return "Draft"; 171 case PLANNED: return "Planned"; 172 case REQUESTED: return "Requested"; 173 case ACTIVE: return "Active"; 174 case ONHOLD: return "On-Hold"; 175 case COMPLETED: return "Completed"; 176 case CANCELLED: return "Cancelled"; 177 case ENTEREDINERROR: return "Entered in Error"; 178 case NULL: return null; 179 default: return "?"; 180 } 181 } 182 } 183 184 public static class NutritionOrderStatusEnumFactory implements EnumFactory<NutritionOrderStatus> { 185 public NutritionOrderStatus fromCode(String codeString) throws IllegalArgumentException { 186 if (codeString == null || "".equals(codeString)) 187 if (codeString == null || "".equals(codeString)) 188 return null; 189 if ("proposed".equals(codeString)) 190 return NutritionOrderStatus.PROPOSED; 191 if ("draft".equals(codeString)) 192 return NutritionOrderStatus.DRAFT; 193 if ("planned".equals(codeString)) 194 return NutritionOrderStatus.PLANNED; 195 if ("requested".equals(codeString)) 196 return NutritionOrderStatus.REQUESTED; 197 if ("active".equals(codeString)) 198 return NutritionOrderStatus.ACTIVE; 199 if ("on-hold".equals(codeString)) 200 return NutritionOrderStatus.ONHOLD; 201 if ("completed".equals(codeString)) 202 return NutritionOrderStatus.COMPLETED; 203 if ("cancelled".equals(codeString)) 204 return NutritionOrderStatus.CANCELLED; 205 if ("entered-in-error".equals(codeString)) 206 return NutritionOrderStatus.ENTEREDINERROR; 207 throw new IllegalArgumentException("Unknown NutritionOrderStatus code '"+codeString+"'"); 208 } 209 public Enumeration<NutritionOrderStatus> fromType(PrimitiveType<?> code) throws FHIRException { 210 if (code == null) 211 return null; 212 if (code.isEmpty()) 213 return new Enumeration<NutritionOrderStatus>(this); 214 String codeString = code.asStringValue(); 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("proposed".equals(codeString)) 218 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PROPOSED); 219 if ("draft".equals(codeString)) 220 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.DRAFT); 221 if ("planned".equals(codeString)) 222 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PLANNED); 223 if ("requested".equals(codeString)) 224 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.REQUESTED); 225 if ("active".equals(codeString)) 226 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ACTIVE); 227 if ("on-hold".equals(codeString)) 228 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ONHOLD); 229 if ("completed".equals(codeString)) 230 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.COMPLETED); 231 if ("cancelled".equals(codeString)) 232 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.CANCELLED); 233 if ("entered-in-error".equals(codeString)) 234 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ENTEREDINERROR); 235 throw new FHIRException("Unknown NutritionOrderStatus code '"+codeString+"'"); 236 } 237 public String toCode(NutritionOrderStatus code) { 238 if (code == NutritionOrderStatus.NULL) 239 return null; 240 if (code == NutritionOrderStatus.PROPOSED) 241 return "proposed"; 242 if (code == NutritionOrderStatus.DRAFT) 243 return "draft"; 244 if (code == NutritionOrderStatus.PLANNED) 245 return "planned"; 246 if (code == NutritionOrderStatus.REQUESTED) 247 return "requested"; 248 if (code == NutritionOrderStatus.ACTIVE) 249 return "active"; 250 if (code == NutritionOrderStatus.ONHOLD) 251 return "on-hold"; 252 if (code == NutritionOrderStatus.COMPLETED) 253 return "completed"; 254 if (code == NutritionOrderStatus.CANCELLED) 255 return "cancelled"; 256 if (code == NutritionOrderStatus.ENTEREDINERROR) 257 return "entered-in-error"; 258 return "?"; 259 } 260 public String toSystem(NutritionOrderStatus code) { 261 return code.getSystem(); 262 } 263 } 264 265 @Block() 266 public static class NutritionOrderOralDietComponent extends BackboneElement implements IBaseBackboneElement { 267 /** 268 * The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet. 269 */ 270 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 271 @Description(shortDefinition="Type of oral diet or diet restrictions that describe what can be consumed orally", formalDefinition="The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet." ) 272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diet-type") 273 protected List<CodeableConcept> type; 274 275 /** 276 * The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present. 277 */ 278 @Child(name = "schedule", type = {Timing.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 279 @Description(shortDefinition="Scheduled frequency of diet", formalDefinition="The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present." ) 280 protected List<Timing> schedule; 281 282 /** 283 * Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet. 284 */ 285 @Child(name = "nutrient", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 286 @Description(shortDefinition="Required nutrient modifications", formalDefinition="Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet." ) 287 protected List<NutritionOrderOralDietNutrientComponent> nutrient; 288 289 /** 290 * Class that describes any texture modifications required for the patient to safely consume various types of solid foods. 291 */ 292 @Child(name = "texture", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 293 @Description(shortDefinition="Required texture modifications", formalDefinition="Class that describes any texture modifications required for the patient to safely consume various types of solid foods." ) 294 protected List<NutritionOrderOralDietTextureComponent> texture; 295 296 /** 297 * The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient. 298 */ 299 @Child(name = "fluidConsistencyType", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 300 @Description(shortDefinition="The required consistency of fluids and liquids provided to the patient", formalDefinition="The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient." ) 301 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consistency-type") 302 protected List<CodeableConcept> fluidConsistencyType; 303 304 /** 305 * Free text or additional instructions or information pertaining to the oral diet. 306 */ 307 @Child(name = "instruction", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 308 @Description(shortDefinition="Instructions or additional information about the oral diet", formalDefinition="Free text or additional instructions or information pertaining to the oral diet." ) 309 protected StringType instruction; 310 311 private static final long serialVersionUID = 973058412L; 312 313 /** 314 * Constructor 315 */ 316 public NutritionOrderOralDietComponent() { 317 super(); 318 } 319 320 /** 321 * @return {@link #type} (The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.) 322 */ 323 public List<CodeableConcept> getType() { 324 if (this.type == null) 325 this.type = new ArrayList<CodeableConcept>(); 326 return this.type; 327 } 328 329 /** 330 * @return Returns a reference to <code>this</code> for easy method chaining 331 */ 332 public NutritionOrderOralDietComponent setType(List<CodeableConcept> theType) { 333 this.type = theType; 334 return this; 335 } 336 337 public boolean hasType() { 338 if (this.type == null) 339 return false; 340 for (CodeableConcept item : this.type) 341 if (!item.isEmpty()) 342 return true; 343 return false; 344 } 345 346 public CodeableConcept addType() { //3 347 CodeableConcept t = new CodeableConcept(); 348 if (this.type == null) 349 this.type = new ArrayList<CodeableConcept>(); 350 this.type.add(t); 351 return t; 352 } 353 354 public NutritionOrderOralDietComponent addType(CodeableConcept t) { //3 355 if (t == null) 356 return this; 357 if (this.type == null) 358 this.type = new ArrayList<CodeableConcept>(); 359 this.type.add(t); 360 return this; 361 } 362 363 /** 364 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 365 */ 366 public CodeableConcept getTypeFirstRep() { 367 if (getType().isEmpty()) { 368 addType(); 369 } 370 return getType().get(0); 371 } 372 373 /** 374 * @return {@link #schedule} (The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.) 375 */ 376 public List<Timing> getSchedule() { 377 if (this.schedule == null) 378 this.schedule = new ArrayList<Timing>(); 379 return this.schedule; 380 } 381 382 /** 383 * @return Returns a reference to <code>this</code> for easy method chaining 384 */ 385 public NutritionOrderOralDietComponent setSchedule(List<Timing> theSchedule) { 386 this.schedule = theSchedule; 387 return this; 388 } 389 390 public boolean hasSchedule() { 391 if (this.schedule == null) 392 return false; 393 for (Timing item : this.schedule) 394 if (!item.isEmpty()) 395 return true; 396 return false; 397 } 398 399 public Timing addSchedule() { //3 400 Timing t = new Timing(); 401 if (this.schedule == null) 402 this.schedule = new ArrayList<Timing>(); 403 this.schedule.add(t); 404 return t; 405 } 406 407 public NutritionOrderOralDietComponent addSchedule(Timing t) { //3 408 if (t == null) 409 return this; 410 if (this.schedule == null) 411 this.schedule = new ArrayList<Timing>(); 412 this.schedule.add(t); 413 return this; 414 } 415 416 /** 417 * @return The first repetition of repeating field {@link #schedule}, creating it if it does not already exist 418 */ 419 public Timing getScheduleFirstRep() { 420 if (getSchedule().isEmpty()) { 421 addSchedule(); 422 } 423 return getSchedule().get(0); 424 } 425 426 /** 427 * @return {@link #nutrient} (Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.) 428 */ 429 public List<NutritionOrderOralDietNutrientComponent> getNutrient() { 430 if (this.nutrient == null) 431 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 432 return this.nutrient; 433 } 434 435 /** 436 * @return Returns a reference to <code>this</code> for easy method chaining 437 */ 438 public NutritionOrderOralDietComponent setNutrient(List<NutritionOrderOralDietNutrientComponent> theNutrient) { 439 this.nutrient = theNutrient; 440 return this; 441 } 442 443 public boolean hasNutrient() { 444 if (this.nutrient == null) 445 return false; 446 for (NutritionOrderOralDietNutrientComponent item : this.nutrient) 447 if (!item.isEmpty()) 448 return true; 449 return false; 450 } 451 452 public NutritionOrderOralDietNutrientComponent addNutrient() { //3 453 NutritionOrderOralDietNutrientComponent t = new NutritionOrderOralDietNutrientComponent(); 454 if (this.nutrient == null) 455 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 456 this.nutrient.add(t); 457 return t; 458 } 459 460 public NutritionOrderOralDietComponent addNutrient(NutritionOrderOralDietNutrientComponent t) { //3 461 if (t == null) 462 return this; 463 if (this.nutrient == null) 464 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 465 this.nutrient.add(t); 466 return this; 467 } 468 469 /** 470 * @return The first repetition of repeating field {@link #nutrient}, creating it if it does not already exist 471 */ 472 public NutritionOrderOralDietNutrientComponent getNutrientFirstRep() { 473 if (getNutrient().isEmpty()) { 474 addNutrient(); 475 } 476 return getNutrient().get(0); 477 } 478 479 /** 480 * @return {@link #texture} (Class that describes any texture modifications required for the patient to safely consume various types of solid foods.) 481 */ 482 public List<NutritionOrderOralDietTextureComponent> getTexture() { 483 if (this.texture == null) 484 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 485 return this.texture; 486 } 487 488 /** 489 * @return Returns a reference to <code>this</code> for easy method chaining 490 */ 491 public NutritionOrderOralDietComponent setTexture(List<NutritionOrderOralDietTextureComponent> theTexture) { 492 this.texture = theTexture; 493 return this; 494 } 495 496 public boolean hasTexture() { 497 if (this.texture == null) 498 return false; 499 for (NutritionOrderOralDietTextureComponent item : this.texture) 500 if (!item.isEmpty()) 501 return true; 502 return false; 503 } 504 505 public NutritionOrderOralDietTextureComponent addTexture() { //3 506 NutritionOrderOralDietTextureComponent t = new NutritionOrderOralDietTextureComponent(); 507 if (this.texture == null) 508 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 509 this.texture.add(t); 510 return t; 511 } 512 513 public NutritionOrderOralDietComponent addTexture(NutritionOrderOralDietTextureComponent t) { //3 514 if (t == null) 515 return this; 516 if (this.texture == null) 517 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 518 this.texture.add(t); 519 return this; 520 } 521 522 /** 523 * @return The first repetition of repeating field {@link #texture}, creating it if it does not already exist 524 */ 525 public NutritionOrderOralDietTextureComponent getTextureFirstRep() { 526 if (getTexture().isEmpty()) { 527 addTexture(); 528 } 529 return getTexture().get(0); 530 } 531 532 /** 533 * @return {@link #fluidConsistencyType} (The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.) 534 */ 535 public List<CodeableConcept> getFluidConsistencyType() { 536 if (this.fluidConsistencyType == null) 537 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 538 return this.fluidConsistencyType; 539 } 540 541 /** 542 * @return Returns a reference to <code>this</code> for easy method chaining 543 */ 544 public NutritionOrderOralDietComponent setFluidConsistencyType(List<CodeableConcept> theFluidConsistencyType) { 545 this.fluidConsistencyType = theFluidConsistencyType; 546 return this; 547 } 548 549 public boolean hasFluidConsistencyType() { 550 if (this.fluidConsistencyType == null) 551 return false; 552 for (CodeableConcept item : this.fluidConsistencyType) 553 if (!item.isEmpty()) 554 return true; 555 return false; 556 } 557 558 public CodeableConcept addFluidConsistencyType() { //3 559 CodeableConcept t = new CodeableConcept(); 560 if (this.fluidConsistencyType == null) 561 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 562 this.fluidConsistencyType.add(t); 563 return t; 564 } 565 566 public NutritionOrderOralDietComponent addFluidConsistencyType(CodeableConcept t) { //3 567 if (t == null) 568 return this; 569 if (this.fluidConsistencyType == null) 570 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 571 this.fluidConsistencyType.add(t); 572 return this; 573 } 574 575 /** 576 * @return The first repetition of repeating field {@link #fluidConsistencyType}, creating it if it does not already exist 577 */ 578 public CodeableConcept getFluidConsistencyTypeFirstRep() { 579 if (getFluidConsistencyType().isEmpty()) { 580 addFluidConsistencyType(); 581 } 582 return getFluidConsistencyType().get(0); 583 } 584 585 /** 586 * @return {@link #instruction} (Free text or additional instructions or information pertaining to the oral diet.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 587 */ 588 public StringType getInstructionElement() { 589 if (this.instruction == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.instruction"); 592 else if (Configuration.doAutoCreate()) 593 this.instruction = new StringType(); // bb 594 return this.instruction; 595 } 596 597 public boolean hasInstructionElement() { 598 return this.instruction != null && !this.instruction.isEmpty(); 599 } 600 601 public boolean hasInstruction() { 602 return this.instruction != null && !this.instruction.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #instruction} (Free text or additional instructions or information pertaining to the oral diet.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 607 */ 608 public NutritionOrderOralDietComponent setInstructionElement(StringType value) { 609 this.instruction = value; 610 return this; 611 } 612 613 /** 614 * @return Free text or additional instructions or information pertaining to the oral diet. 615 */ 616 public String getInstruction() { 617 return this.instruction == null ? null : this.instruction.getValue(); 618 } 619 620 /** 621 * @param value Free text or additional instructions or information pertaining to the oral diet. 622 */ 623 public NutritionOrderOralDietComponent setInstruction(String value) { 624 if (Utilities.noString(value)) 625 this.instruction = null; 626 else { 627 if (this.instruction == null) 628 this.instruction = new StringType(); 629 this.instruction.setValue(value); 630 } 631 return this; 632 } 633 634 protected void listChildren(List<Property> children) { 635 super.listChildren(children); 636 children.add(new Property("type", "CodeableConcept", "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, java.lang.Integer.MAX_VALUE, type)); 637 children.add(new Property("schedule", "Timing", "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, schedule)); 638 children.add(new Property("nutrient", "", "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 0, java.lang.Integer.MAX_VALUE, nutrient)); 639 children.add(new Property("texture", "", "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 0, java.lang.Integer.MAX_VALUE, texture)); 640 children.add(new Property("fluidConsistencyType", "CodeableConcept", "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType)); 641 children.add(new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction)); 642 } 643 644 @Override 645 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 646 switch (_hash) { 647 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, java.lang.Integer.MAX_VALUE, type); 648 case -697920873: /*schedule*/ return new Property("schedule", "Timing", "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, schedule); 649 case -1671151641: /*nutrient*/ return new Property("nutrient", "", "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 0, java.lang.Integer.MAX_VALUE, nutrient); 650 case -1417816805: /*texture*/ return new Property("texture", "", "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 0, java.lang.Integer.MAX_VALUE, texture); 651 case -525105592: /*fluidConsistencyType*/ return new Property("fluidConsistencyType", "CodeableConcept", "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType); 652 case 301526158: /*instruction*/ return new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction); 653 default: return super.getNamedProperty(_hash, _name, _checkValid); 654 } 655 656 } 657 658 @Override 659 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 660 switch (hash) { 661 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 662 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : this.schedule.toArray(new Base[this.schedule.size()]); // Timing 663 case -1671151641: /*nutrient*/ return this.nutrient == null ? new Base[0] : this.nutrient.toArray(new Base[this.nutrient.size()]); // NutritionOrderOralDietNutrientComponent 664 case -1417816805: /*texture*/ return this.texture == null ? new Base[0] : this.texture.toArray(new Base[this.texture.size()]); // NutritionOrderOralDietTextureComponent 665 case -525105592: /*fluidConsistencyType*/ return this.fluidConsistencyType == null ? new Base[0] : this.fluidConsistencyType.toArray(new Base[this.fluidConsistencyType.size()]); // CodeableConcept 666 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // StringType 667 default: return super.getProperty(hash, name, checkValid); 668 } 669 670 } 671 672 @Override 673 public Base setProperty(int hash, String name, Base value) throws FHIRException { 674 switch (hash) { 675 case 3575610: // type 676 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 677 return value; 678 case -697920873: // schedule 679 this.getSchedule().add(castToTiming(value)); // Timing 680 return value; 681 case -1671151641: // nutrient 682 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); // NutritionOrderOralDietNutrientComponent 683 return value; 684 case -1417816805: // texture 685 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); // NutritionOrderOralDietTextureComponent 686 return value; 687 case -525105592: // fluidConsistencyType 688 this.getFluidConsistencyType().add(castToCodeableConcept(value)); // CodeableConcept 689 return value; 690 case 301526158: // instruction 691 this.instruction = castToString(value); // StringType 692 return value; 693 default: return super.setProperty(hash, name, value); 694 } 695 696 } 697 698 @Override 699 public Base setProperty(String name, Base value) throws FHIRException { 700 if (name.equals("type")) { 701 this.getType().add(castToCodeableConcept(value)); 702 } else if (name.equals("schedule")) { 703 this.getSchedule().add(castToTiming(value)); 704 } else if (name.equals("nutrient")) { 705 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); 706 } else if (name.equals("texture")) { 707 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); 708 } else if (name.equals("fluidConsistencyType")) { 709 this.getFluidConsistencyType().add(castToCodeableConcept(value)); 710 } else if (name.equals("instruction")) { 711 this.instruction = castToString(value); // StringType 712 } else 713 return super.setProperty(name, value); 714 return value; 715 } 716 717 @Override 718 public Base makeProperty(int hash, String name) throws FHIRException { 719 switch (hash) { 720 case 3575610: return addType(); 721 case -697920873: return addSchedule(); 722 case -1671151641: return addNutrient(); 723 case -1417816805: return addTexture(); 724 case -525105592: return addFluidConsistencyType(); 725 case 301526158: return getInstructionElement(); 726 default: return super.makeProperty(hash, name); 727 } 728 729 } 730 731 @Override 732 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 733 switch (hash) { 734 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 735 case -697920873: /*schedule*/ return new String[] {"Timing"}; 736 case -1671151641: /*nutrient*/ return new String[] {}; 737 case -1417816805: /*texture*/ return new String[] {}; 738 case -525105592: /*fluidConsistencyType*/ return new String[] {"CodeableConcept"}; 739 case 301526158: /*instruction*/ return new String[] {"string"}; 740 default: return super.getTypesForProperty(hash, name); 741 } 742 743 } 744 745 @Override 746 public Base addChild(String name) throws FHIRException { 747 if (name.equals("type")) { 748 return addType(); 749 } 750 else if (name.equals("schedule")) { 751 return addSchedule(); 752 } 753 else if (name.equals("nutrient")) { 754 return addNutrient(); 755 } 756 else if (name.equals("texture")) { 757 return addTexture(); 758 } 759 else if (name.equals("fluidConsistencyType")) { 760 return addFluidConsistencyType(); 761 } 762 else if (name.equals("instruction")) { 763 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 764 } 765 else 766 return super.addChild(name); 767 } 768 769 public NutritionOrderOralDietComponent copy() { 770 NutritionOrderOralDietComponent dst = new NutritionOrderOralDietComponent(); 771 copyValues(dst); 772 if (type != null) { 773 dst.type = new ArrayList<CodeableConcept>(); 774 for (CodeableConcept i : type) 775 dst.type.add(i.copy()); 776 }; 777 if (schedule != null) { 778 dst.schedule = new ArrayList<Timing>(); 779 for (Timing i : schedule) 780 dst.schedule.add(i.copy()); 781 }; 782 if (nutrient != null) { 783 dst.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 784 for (NutritionOrderOralDietNutrientComponent i : nutrient) 785 dst.nutrient.add(i.copy()); 786 }; 787 if (texture != null) { 788 dst.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 789 for (NutritionOrderOralDietTextureComponent i : texture) 790 dst.texture.add(i.copy()); 791 }; 792 if (fluidConsistencyType != null) { 793 dst.fluidConsistencyType = new ArrayList<CodeableConcept>(); 794 for (CodeableConcept i : fluidConsistencyType) 795 dst.fluidConsistencyType.add(i.copy()); 796 }; 797 dst.instruction = instruction == null ? null : instruction.copy(); 798 return dst; 799 } 800 801 @Override 802 public boolean equalsDeep(Base other_) { 803 if (!super.equalsDeep(other_)) 804 return false; 805 if (!(other_ instanceof NutritionOrderOralDietComponent)) 806 return false; 807 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 808 return compareDeep(type, o.type, true) && compareDeep(schedule, o.schedule, true) && compareDeep(nutrient, o.nutrient, true) 809 && compareDeep(texture, o.texture, true) && compareDeep(fluidConsistencyType, o.fluidConsistencyType, true) 810 && compareDeep(instruction, o.instruction, true); 811 } 812 813 @Override 814 public boolean equalsShallow(Base other_) { 815 if (!super.equalsShallow(other_)) 816 return false; 817 if (!(other_ instanceof NutritionOrderOralDietComponent)) 818 return false; 819 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 820 return compareValues(instruction, o.instruction, true); 821 } 822 823 public boolean isEmpty() { 824 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, schedule, nutrient 825 , texture, fluidConsistencyType, instruction); 826 } 827 828 public String fhirType() { 829 return "NutritionOrder.oralDiet"; 830 831 } 832 833 } 834 835 @Block() 836 public static class NutritionOrderOralDietNutrientComponent extends BackboneElement implements IBaseBackboneElement { 837 /** 838 * The nutrient that is being modified such as carbohydrate or sodium. 839 */ 840 @Child(name = "modifier", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 841 @Description(shortDefinition="Type of nutrient that is being modified", formalDefinition="The nutrient that is being modified such as carbohydrate or sodium." ) 842 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrient-code") 843 protected CodeableConcept modifier; 844 845 /** 846 * The quantity of the specified nutrient to include in diet. 847 */ 848 @Child(name = "amount", type = {SimpleQuantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 849 @Description(shortDefinition="Quantity of the specified nutrient", formalDefinition="The quantity of the specified nutrient to include in diet." ) 850 protected SimpleQuantity amount; 851 852 private static final long serialVersionUID = 465107295L; 853 854 /** 855 * Constructor 856 */ 857 public NutritionOrderOralDietNutrientComponent() { 858 super(); 859 } 860 861 /** 862 * @return {@link #modifier} (The nutrient that is being modified such as carbohydrate or sodium.) 863 */ 864 public CodeableConcept getModifier() { 865 if (this.modifier == null) 866 if (Configuration.errorOnAutoCreate()) 867 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.modifier"); 868 else if (Configuration.doAutoCreate()) 869 this.modifier = new CodeableConcept(); // cc 870 return this.modifier; 871 } 872 873 public boolean hasModifier() { 874 return this.modifier != null && !this.modifier.isEmpty(); 875 } 876 877 /** 878 * @param value {@link #modifier} (The nutrient that is being modified such as carbohydrate or sodium.) 879 */ 880 public NutritionOrderOralDietNutrientComponent setModifier(CodeableConcept value) { 881 this.modifier = value; 882 return this; 883 } 884 885 /** 886 * @return {@link #amount} (The quantity of the specified nutrient to include in diet.) 887 */ 888 public SimpleQuantity getAmount() { 889 if (this.amount == null) 890 if (Configuration.errorOnAutoCreate()) 891 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.amount"); 892 else if (Configuration.doAutoCreate()) 893 this.amount = new SimpleQuantity(); // cc 894 return this.amount; 895 } 896 897 public boolean hasAmount() { 898 return this.amount != null && !this.amount.isEmpty(); 899 } 900 901 /** 902 * @param value {@link #amount} (The quantity of the specified nutrient to include in diet.) 903 */ 904 public NutritionOrderOralDietNutrientComponent setAmount(SimpleQuantity value) { 905 this.amount = value; 906 return this; 907 } 908 909 protected void listChildren(List<Property> children) { 910 super.listChildren(children); 911 children.add(new Property("modifier", "CodeableConcept", "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier)); 912 children.add(new Property("amount", "SimpleQuantity", "The quantity of the specified nutrient to include in diet.", 0, 1, amount)); 913 } 914 915 @Override 916 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 917 switch (_hash) { 918 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier); 919 case -1413853096: /*amount*/ return new Property("amount", "SimpleQuantity", "The quantity of the specified nutrient to include in diet.", 0, 1, amount); 920 default: return super.getNamedProperty(_hash, _name, _checkValid); 921 } 922 923 } 924 925 @Override 926 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 927 switch (hash) { 928 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : new Base[] {this.modifier}; // CodeableConcept 929 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // SimpleQuantity 930 default: return super.getProperty(hash, name, checkValid); 931 } 932 933 } 934 935 @Override 936 public Base setProperty(int hash, String name, Base value) throws FHIRException { 937 switch (hash) { 938 case -615513385: // modifier 939 this.modifier = castToCodeableConcept(value); // CodeableConcept 940 return value; 941 case -1413853096: // amount 942 this.amount = castToSimpleQuantity(value); // SimpleQuantity 943 return value; 944 default: return super.setProperty(hash, name, value); 945 } 946 947 } 948 949 @Override 950 public Base setProperty(String name, Base value) throws FHIRException { 951 if (name.equals("modifier")) { 952 this.modifier = castToCodeableConcept(value); // CodeableConcept 953 } else if (name.equals("amount")) { 954 this.amount = castToSimpleQuantity(value); // SimpleQuantity 955 } else 956 return super.setProperty(name, value); 957 return value; 958 } 959 960 @Override 961 public Base makeProperty(int hash, String name) throws FHIRException { 962 switch (hash) { 963 case -615513385: return getModifier(); 964 case -1413853096: return getAmount(); 965 default: return super.makeProperty(hash, name); 966 } 967 968 } 969 970 @Override 971 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 972 switch (hash) { 973 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 974 case -1413853096: /*amount*/ return new String[] {"SimpleQuantity"}; 975 default: return super.getTypesForProperty(hash, name); 976 } 977 978 } 979 980 @Override 981 public Base addChild(String name) throws FHIRException { 982 if (name.equals("modifier")) { 983 this.modifier = new CodeableConcept(); 984 return this.modifier; 985 } 986 else if (name.equals("amount")) { 987 this.amount = new SimpleQuantity(); 988 return this.amount; 989 } 990 else 991 return super.addChild(name); 992 } 993 994 public NutritionOrderOralDietNutrientComponent copy() { 995 NutritionOrderOralDietNutrientComponent dst = new NutritionOrderOralDietNutrientComponent(); 996 copyValues(dst); 997 dst.modifier = modifier == null ? null : modifier.copy(); 998 dst.amount = amount == null ? null : amount.copy(); 999 return dst; 1000 } 1001 1002 @Override 1003 public boolean equalsDeep(Base other_) { 1004 if (!super.equalsDeep(other_)) 1005 return false; 1006 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1007 return false; 1008 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1009 return compareDeep(modifier, o.modifier, true) && compareDeep(amount, o.amount, true); 1010 } 1011 1012 @Override 1013 public boolean equalsShallow(Base other_) { 1014 if (!super.equalsShallow(other_)) 1015 return false; 1016 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1017 return false; 1018 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1019 return true; 1020 } 1021 1022 public boolean isEmpty() { 1023 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, amount); 1024 } 1025 1026 public String fhirType() { 1027 return "NutritionOrder.oralDiet.nutrient"; 1028 1029 } 1030 1031 } 1032 1033 @Block() 1034 public static class NutritionOrderOralDietTextureComponent extends BackboneElement implements IBaseBackboneElement { 1035 /** 1036 * Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed. 1037 */ 1038 @Child(name = "modifier", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1039 @Description(shortDefinition="Code to indicate how to alter the texture of the foods, e.g. pureed", formalDefinition="Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed." ) 1040 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/texture-code") 1041 protected CodeableConcept modifier; 1042 1043 /** 1044 * The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types. 1045 */ 1046 @Child(name = "foodType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1047 @Description(shortDefinition="Concepts that are used to identify an entity that is ingested for nutritional purposes", formalDefinition="The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types." ) 1048 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/modified-foodtype") 1049 protected CodeableConcept foodType; 1050 1051 private static final long serialVersionUID = -56402817L; 1052 1053 /** 1054 * Constructor 1055 */ 1056 public NutritionOrderOralDietTextureComponent() { 1057 super(); 1058 } 1059 1060 /** 1061 * @return {@link #modifier} (Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.) 1062 */ 1063 public CodeableConcept getModifier() { 1064 if (this.modifier == null) 1065 if (Configuration.errorOnAutoCreate()) 1066 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.modifier"); 1067 else if (Configuration.doAutoCreate()) 1068 this.modifier = new CodeableConcept(); // cc 1069 return this.modifier; 1070 } 1071 1072 public boolean hasModifier() { 1073 return this.modifier != null && !this.modifier.isEmpty(); 1074 } 1075 1076 /** 1077 * @param value {@link #modifier} (Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.) 1078 */ 1079 public NutritionOrderOralDietTextureComponent setModifier(CodeableConcept value) { 1080 this.modifier = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #foodType} (The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.) 1086 */ 1087 public CodeableConcept getFoodType() { 1088 if (this.foodType == null) 1089 if (Configuration.errorOnAutoCreate()) 1090 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.foodType"); 1091 else if (Configuration.doAutoCreate()) 1092 this.foodType = new CodeableConcept(); // cc 1093 return this.foodType; 1094 } 1095 1096 public boolean hasFoodType() { 1097 return this.foodType != null && !this.foodType.isEmpty(); 1098 } 1099 1100 /** 1101 * @param value {@link #foodType} (The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.) 1102 */ 1103 public NutritionOrderOralDietTextureComponent setFoodType(CodeableConcept value) { 1104 this.foodType = value; 1105 return this; 1106 } 1107 1108 protected void listChildren(List<Property> children) { 1109 super.listChildren(children); 1110 children.add(new Property("modifier", "CodeableConcept", "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 0, 1, modifier)); 1111 children.add(new Property("foodType", "CodeableConcept", "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 0, 1, foodType)); 1112 } 1113 1114 @Override 1115 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1116 switch (_hash) { 1117 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 0, 1, modifier); 1118 case 379498680: /*foodType*/ return new Property("foodType", "CodeableConcept", "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 0, 1, foodType); 1119 default: return super.getNamedProperty(_hash, _name, _checkValid); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1126 switch (hash) { 1127 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : new Base[] {this.modifier}; // CodeableConcept 1128 case 379498680: /*foodType*/ return this.foodType == null ? new Base[0] : new Base[] {this.foodType}; // CodeableConcept 1129 default: return super.getProperty(hash, name, checkValid); 1130 } 1131 1132 } 1133 1134 @Override 1135 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1136 switch (hash) { 1137 case -615513385: // modifier 1138 this.modifier = castToCodeableConcept(value); // CodeableConcept 1139 return value; 1140 case 379498680: // foodType 1141 this.foodType = castToCodeableConcept(value); // CodeableConcept 1142 return value; 1143 default: return super.setProperty(hash, name, value); 1144 } 1145 1146 } 1147 1148 @Override 1149 public Base setProperty(String name, Base value) throws FHIRException { 1150 if (name.equals("modifier")) { 1151 this.modifier = castToCodeableConcept(value); // CodeableConcept 1152 } else if (name.equals("foodType")) { 1153 this.foodType = castToCodeableConcept(value); // CodeableConcept 1154 } else 1155 return super.setProperty(name, value); 1156 return value; 1157 } 1158 1159 @Override 1160 public Base makeProperty(int hash, String name) throws FHIRException { 1161 switch (hash) { 1162 case -615513385: return getModifier(); 1163 case 379498680: return getFoodType(); 1164 default: return super.makeProperty(hash, name); 1165 } 1166 1167 } 1168 1169 @Override 1170 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1171 switch (hash) { 1172 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 1173 case 379498680: /*foodType*/ return new String[] {"CodeableConcept"}; 1174 default: return super.getTypesForProperty(hash, name); 1175 } 1176 1177 } 1178 1179 @Override 1180 public Base addChild(String name) throws FHIRException { 1181 if (name.equals("modifier")) { 1182 this.modifier = new CodeableConcept(); 1183 return this.modifier; 1184 } 1185 else if (name.equals("foodType")) { 1186 this.foodType = new CodeableConcept(); 1187 return this.foodType; 1188 } 1189 else 1190 return super.addChild(name); 1191 } 1192 1193 public NutritionOrderOralDietTextureComponent copy() { 1194 NutritionOrderOralDietTextureComponent dst = new NutritionOrderOralDietTextureComponent(); 1195 copyValues(dst); 1196 dst.modifier = modifier == null ? null : modifier.copy(); 1197 dst.foodType = foodType == null ? null : foodType.copy(); 1198 return dst; 1199 } 1200 1201 @Override 1202 public boolean equalsDeep(Base other_) { 1203 if (!super.equalsDeep(other_)) 1204 return false; 1205 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1206 return false; 1207 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1208 return compareDeep(modifier, o.modifier, true) && compareDeep(foodType, o.foodType, true); 1209 } 1210 1211 @Override 1212 public boolean equalsShallow(Base other_) { 1213 if (!super.equalsShallow(other_)) 1214 return false; 1215 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1216 return false; 1217 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1218 return true; 1219 } 1220 1221 public boolean isEmpty() { 1222 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, foodType); 1223 } 1224 1225 public String fhirType() { 1226 return "NutritionOrder.oralDiet.texture"; 1227 1228 } 1229 1230 } 1231 1232 @Block() 1233 public static class NutritionOrderSupplementComponent extends BackboneElement implements IBaseBackboneElement { 1234 /** 1235 * The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement. 1236 */ 1237 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1238 @Description(shortDefinition="Type of supplement product requested", formalDefinition="The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement." ) 1239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplement-type") 1240 protected CodeableConcept type; 1241 1242 /** 1243 * The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1244 */ 1245 @Child(name = "productName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1246 @Description(shortDefinition="Product or brand name of the nutritional supplement", formalDefinition="The product or brand name of the nutritional supplement such as \"Acme Protein Shake\"." ) 1247 protected StringType productName; 1248 1249 /** 1250 * The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present. 1251 */ 1252 @Child(name = "schedule", type = {Timing.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1253 @Description(shortDefinition="Scheduled frequency of supplement", formalDefinition="The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present." ) 1254 protected List<Timing> schedule; 1255 1256 /** 1257 * The amount of the nutritional supplement to be given. 1258 */ 1259 @Child(name = "quantity", type = {SimpleQuantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 1260 @Description(shortDefinition="Amount of the nutritional supplement", formalDefinition="The amount of the nutritional supplement to be given." ) 1261 protected SimpleQuantity quantity; 1262 1263 /** 1264 * Free text or additional instructions or information pertaining to the oral supplement. 1265 */ 1266 @Child(name = "instruction", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1267 @Description(shortDefinition="Instructions or additional information about the oral supplement", formalDefinition="Free text or additional instructions or information pertaining to the oral supplement." ) 1268 protected StringType instruction; 1269 1270 private static final long serialVersionUID = 297545236L; 1271 1272 /** 1273 * Constructor 1274 */ 1275 public NutritionOrderSupplementComponent() { 1276 super(); 1277 } 1278 1279 /** 1280 * @return {@link #type} (The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.) 1281 */ 1282 public CodeableConcept getType() { 1283 if (this.type == null) 1284 if (Configuration.errorOnAutoCreate()) 1285 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.type"); 1286 else if (Configuration.doAutoCreate()) 1287 this.type = new CodeableConcept(); // cc 1288 return this.type; 1289 } 1290 1291 public boolean hasType() { 1292 return this.type != null && !this.type.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #type} (The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.) 1297 */ 1298 public NutritionOrderSupplementComponent setType(CodeableConcept value) { 1299 this.type = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #productName} (The product or brand name of the nutritional supplement such as "Acme Protein Shake".). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 1305 */ 1306 public StringType getProductNameElement() { 1307 if (this.productName == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.productName"); 1310 else if (Configuration.doAutoCreate()) 1311 this.productName = new StringType(); // bb 1312 return this.productName; 1313 } 1314 1315 public boolean hasProductNameElement() { 1316 return this.productName != null && !this.productName.isEmpty(); 1317 } 1318 1319 public boolean hasProductName() { 1320 return this.productName != null && !this.productName.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #productName} (The product or brand name of the nutritional supplement such as "Acme Protein Shake".). This is the underlying object with id, value and extensions. The accessor "getProductName" gives direct access to the value 1325 */ 1326 public NutritionOrderSupplementComponent setProductNameElement(StringType value) { 1327 this.productName = value; 1328 return this; 1329 } 1330 1331 /** 1332 * @return The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1333 */ 1334 public String getProductName() { 1335 return this.productName == null ? null : this.productName.getValue(); 1336 } 1337 1338 /** 1339 * @param value The product or brand name of the nutritional supplement such as "Acme Protein Shake". 1340 */ 1341 public NutritionOrderSupplementComponent setProductName(String value) { 1342 if (Utilities.noString(value)) 1343 this.productName = null; 1344 else { 1345 if (this.productName == null) 1346 this.productName = new StringType(); 1347 this.productName.setValue(value); 1348 } 1349 return this; 1350 } 1351 1352 /** 1353 * @return {@link #schedule} (The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.) 1354 */ 1355 public List<Timing> getSchedule() { 1356 if (this.schedule == null) 1357 this.schedule = new ArrayList<Timing>(); 1358 return this.schedule; 1359 } 1360 1361 /** 1362 * @return Returns a reference to <code>this</code> for easy method chaining 1363 */ 1364 public NutritionOrderSupplementComponent setSchedule(List<Timing> theSchedule) { 1365 this.schedule = theSchedule; 1366 return this; 1367 } 1368 1369 public boolean hasSchedule() { 1370 if (this.schedule == null) 1371 return false; 1372 for (Timing item : this.schedule) 1373 if (!item.isEmpty()) 1374 return true; 1375 return false; 1376 } 1377 1378 public Timing addSchedule() { //3 1379 Timing t = new Timing(); 1380 if (this.schedule == null) 1381 this.schedule = new ArrayList<Timing>(); 1382 this.schedule.add(t); 1383 return t; 1384 } 1385 1386 public NutritionOrderSupplementComponent addSchedule(Timing t) { //3 1387 if (t == null) 1388 return this; 1389 if (this.schedule == null) 1390 this.schedule = new ArrayList<Timing>(); 1391 this.schedule.add(t); 1392 return this; 1393 } 1394 1395 /** 1396 * @return The first repetition of repeating field {@link #schedule}, creating it if it does not already exist 1397 */ 1398 public Timing getScheduleFirstRep() { 1399 if (getSchedule().isEmpty()) { 1400 addSchedule(); 1401 } 1402 return getSchedule().get(0); 1403 } 1404 1405 /** 1406 * @return {@link #quantity} (The amount of the nutritional supplement to be given.) 1407 */ 1408 public SimpleQuantity getQuantity() { 1409 if (this.quantity == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.quantity"); 1412 else if (Configuration.doAutoCreate()) 1413 this.quantity = new SimpleQuantity(); // cc 1414 return this.quantity; 1415 } 1416 1417 public boolean hasQuantity() { 1418 return this.quantity != null && !this.quantity.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #quantity} (The amount of the nutritional supplement to be given.) 1423 */ 1424 public NutritionOrderSupplementComponent setQuantity(SimpleQuantity value) { 1425 this.quantity = value; 1426 return this; 1427 } 1428 1429 /** 1430 * @return {@link #instruction} (Free text or additional instructions or information pertaining to the oral supplement.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1431 */ 1432 public StringType getInstructionElement() { 1433 if (this.instruction == null) 1434 if (Configuration.errorOnAutoCreate()) 1435 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.instruction"); 1436 else if (Configuration.doAutoCreate()) 1437 this.instruction = new StringType(); // bb 1438 return this.instruction; 1439 } 1440 1441 public boolean hasInstructionElement() { 1442 return this.instruction != null && !this.instruction.isEmpty(); 1443 } 1444 1445 public boolean hasInstruction() { 1446 return this.instruction != null && !this.instruction.isEmpty(); 1447 } 1448 1449 /** 1450 * @param value {@link #instruction} (Free text or additional instructions or information pertaining to the oral supplement.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1451 */ 1452 public NutritionOrderSupplementComponent setInstructionElement(StringType value) { 1453 this.instruction = value; 1454 return this; 1455 } 1456 1457 /** 1458 * @return Free text or additional instructions or information pertaining to the oral supplement. 1459 */ 1460 public String getInstruction() { 1461 return this.instruction == null ? null : this.instruction.getValue(); 1462 } 1463 1464 /** 1465 * @param value Free text or additional instructions or information pertaining to the oral supplement. 1466 */ 1467 public NutritionOrderSupplementComponent setInstruction(String value) { 1468 if (Utilities.noString(value)) 1469 this.instruction = null; 1470 else { 1471 if (this.instruction == null) 1472 this.instruction = new StringType(); 1473 this.instruction.setValue(value); 1474 } 1475 return this; 1476 } 1477 1478 protected void listChildren(List<Property> children) { 1479 super.listChildren(children); 1480 children.add(new Property("type", "CodeableConcept", "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 0, 1, type)); 1481 children.add(new Property("productName", "string", "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, productName)); 1482 children.add(new Property("schedule", "Timing", "The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, schedule)); 1483 children.add(new Property("quantity", "SimpleQuantity", "The amount of the nutritional supplement to be given.", 0, 1, quantity)); 1484 children.add(new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, instruction)); 1485 } 1486 1487 @Override 1488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1489 switch (_hash) { 1490 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 0, 1, type); 1491 case -1491817446: /*productName*/ return new Property("productName", "string", "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, productName); 1492 case -697920873: /*schedule*/ return new Property("schedule", "Timing", "The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 0, java.lang.Integer.MAX_VALUE, schedule); 1493 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount of the nutritional supplement to be given.", 0, 1, quantity); 1494 case 301526158: /*instruction*/ return new Property("instruction", "string", "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, instruction); 1495 default: return super.getNamedProperty(_hash, _name, _checkValid); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1502 switch (hash) { 1503 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1504 case -1491817446: /*productName*/ return this.productName == null ? new Base[0] : new Base[] {this.productName}; // StringType 1505 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : this.schedule.toArray(new Base[this.schedule.size()]); // Timing 1506 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 1507 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // StringType 1508 default: return super.getProperty(hash, name, checkValid); 1509 } 1510 1511 } 1512 1513 @Override 1514 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1515 switch (hash) { 1516 case 3575610: // type 1517 this.type = castToCodeableConcept(value); // CodeableConcept 1518 return value; 1519 case -1491817446: // productName 1520 this.productName = castToString(value); // StringType 1521 return value; 1522 case -697920873: // schedule 1523 this.getSchedule().add(castToTiming(value)); // Timing 1524 return value; 1525 case -1285004149: // quantity 1526 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1527 return value; 1528 case 301526158: // instruction 1529 this.instruction = castToString(value); // StringType 1530 return value; 1531 default: return super.setProperty(hash, name, value); 1532 } 1533 1534 } 1535 1536 @Override 1537 public Base setProperty(String name, Base value) throws FHIRException { 1538 if (name.equals("type")) { 1539 this.type = castToCodeableConcept(value); // CodeableConcept 1540 } else if (name.equals("productName")) { 1541 this.productName = castToString(value); // StringType 1542 } else if (name.equals("schedule")) { 1543 this.getSchedule().add(castToTiming(value)); 1544 } else if (name.equals("quantity")) { 1545 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1546 } else if (name.equals("instruction")) { 1547 this.instruction = castToString(value); // StringType 1548 } else 1549 return super.setProperty(name, value); 1550 return value; 1551 } 1552 1553 @Override 1554 public Base makeProperty(int hash, String name) throws FHIRException { 1555 switch (hash) { 1556 case 3575610: return getType(); 1557 case -1491817446: return getProductNameElement(); 1558 case -697920873: return addSchedule(); 1559 case -1285004149: return getQuantity(); 1560 case 301526158: return getInstructionElement(); 1561 default: return super.makeProperty(hash, name); 1562 } 1563 1564 } 1565 1566 @Override 1567 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1568 switch (hash) { 1569 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1570 case -1491817446: /*productName*/ return new String[] {"string"}; 1571 case -697920873: /*schedule*/ return new String[] {"Timing"}; 1572 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 1573 case 301526158: /*instruction*/ return new String[] {"string"}; 1574 default: return super.getTypesForProperty(hash, name); 1575 } 1576 1577 } 1578 1579 @Override 1580 public Base addChild(String name) throws FHIRException { 1581 if (name.equals("type")) { 1582 this.type = new CodeableConcept(); 1583 return this.type; 1584 } 1585 else if (name.equals("productName")) { 1586 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.productName"); 1587 } 1588 else if (name.equals("schedule")) { 1589 return addSchedule(); 1590 } 1591 else if (name.equals("quantity")) { 1592 this.quantity = new SimpleQuantity(); 1593 return this.quantity; 1594 } 1595 else if (name.equals("instruction")) { 1596 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 1597 } 1598 else 1599 return super.addChild(name); 1600 } 1601 1602 public NutritionOrderSupplementComponent copy() { 1603 NutritionOrderSupplementComponent dst = new NutritionOrderSupplementComponent(); 1604 copyValues(dst); 1605 dst.type = type == null ? null : type.copy(); 1606 dst.productName = productName == null ? null : productName.copy(); 1607 if (schedule != null) { 1608 dst.schedule = new ArrayList<Timing>(); 1609 for (Timing i : schedule) 1610 dst.schedule.add(i.copy()); 1611 }; 1612 dst.quantity = quantity == null ? null : quantity.copy(); 1613 dst.instruction = instruction == null ? null : instruction.copy(); 1614 return dst; 1615 } 1616 1617 @Override 1618 public boolean equalsDeep(Base other_) { 1619 if (!super.equalsDeep(other_)) 1620 return false; 1621 if (!(other_ instanceof NutritionOrderSupplementComponent)) 1622 return false; 1623 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 1624 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) && compareDeep(schedule, o.schedule, true) 1625 && compareDeep(quantity, o.quantity, true) && compareDeep(instruction, o.instruction, true); 1626 } 1627 1628 @Override 1629 public boolean equalsShallow(Base other_) { 1630 if (!super.equalsShallow(other_)) 1631 return false; 1632 if (!(other_ instanceof NutritionOrderSupplementComponent)) 1633 return false; 1634 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 1635 return compareValues(productName, o.productName, true) && compareValues(instruction, o.instruction, true) 1636 ; 1637 } 1638 1639 public boolean isEmpty() { 1640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, productName, schedule 1641 , quantity, instruction); 1642 } 1643 1644 public String fhirType() { 1645 return "NutritionOrder.supplement"; 1646 1647 } 1648 1649 } 1650 1651 @Block() 1652 public static class NutritionOrderEnteralFormulaComponent extends BackboneElement implements IBaseBackboneElement { 1653 /** 1654 * The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula. 1655 */ 1656 @Child(name = "baseFormulaType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1657 @Description(shortDefinition="Type of enteral or infant formula", formalDefinition="The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula." ) 1658 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/entformula-type") 1659 protected CodeableConcept baseFormulaType; 1660 1661 /** 1662 * The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 1663 */ 1664 @Child(name = "baseFormulaProductName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1665 @Description(shortDefinition="Product or brand name of the enteral or infant formula", formalDefinition="The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\"." ) 1666 protected StringType baseFormulaProductName; 1667 1668 /** 1669 * Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula. 1670 */ 1671 @Child(name = "additiveType", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1672 @Description(shortDefinition="Type of modular component to add to the feeding", formalDefinition="Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula." ) 1673 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/entformula-additive") 1674 protected CodeableConcept additiveType; 1675 1676 /** 1677 * The product or brand name of the type of modular component to be added to the formula. 1678 */ 1679 @Child(name = "additiveProductName", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1680 @Description(shortDefinition="Product or brand name of the modular additive", formalDefinition="The product or brand name of the type of modular component to be added to the formula." ) 1681 protected StringType additiveProductName; 1682 1683 /** 1684 * The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL. 1685 */ 1686 @Child(name = "caloricDensity", type = {SimpleQuantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 1687 @Description(shortDefinition="Amount of energy per specified volume that is required", formalDefinition="The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL." ) 1688 protected SimpleQuantity caloricDensity; 1689 1690 /** 1691 * The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube. 1692 */ 1693 @Child(name = "routeofAdministration", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1694 @Description(shortDefinition="How the formula should enter the patient's gastrointestinal tract", formalDefinition="The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube." ) 1695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/enteral-route") 1696 protected CodeableConcept routeofAdministration; 1697 1698 /** 1699 * Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours. 1700 */ 1701 @Child(name = "administration", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1702 @Description(shortDefinition="Formula feeding instruction as structured data", formalDefinition="Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours." ) 1703 protected List<NutritionOrderEnteralFormulaAdministrationComponent> administration; 1704 1705 /** 1706 * The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours. 1707 */ 1708 @Child(name = "maxVolumeToDeliver", type = {SimpleQuantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 1709 @Description(shortDefinition="Upper limit on formula volume per unit of time", formalDefinition="The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours." ) 1710 protected SimpleQuantity maxVolumeToDeliver; 1711 1712 /** 1713 * Free text formula administration, feeding instructions or additional instructions or information. 1714 */ 1715 @Child(name = "administrationInstruction", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1716 @Description(shortDefinition="Formula feeding instructions expressed as text", formalDefinition="Free text formula administration, feeding instructions or additional instructions or information." ) 1717 protected StringType administrationInstruction; 1718 1719 private static final long serialVersionUID = 292116061L; 1720 1721 /** 1722 * Constructor 1723 */ 1724 public NutritionOrderEnteralFormulaComponent() { 1725 super(); 1726 } 1727 1728 /** 1729 * @return {@link #baseFormulaType} (The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.) 1730 */ 1731 public CodeableConcept getBaseFormulaType() { 1732 if (this.baseFormulaType == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaType"); 1735 else if (Configuration.doAutoCreate()) 1736 this.baseFormulaType = new CodeableConcept(); // cc 1737 return this.baseFormulaType; 1738 } 1739 1740 public boolean hasBaseFormulaType() { 1741 return this.baseFormulaType != null && !this.baseFormulaType.isEmpty(); 1742 } 1743 1744 /** 1745 * @param value {@link #baseFormulaType} (The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.) 1746 */ 1747 public NutritionOrderEnteralFormulaComponent setBaseFormulaType(CodeableConcept value) { 1748 this.baseFormulaType = value; 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #baseFormulaProductName} (The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula".). This is the underlying object with id, value and extensions. The accessor "getBaseFormulaProductName" gives direct access to the value 1754 */ 1755 public StringType getBaseFormulaProductNameElement() { 1756 if (this.baseFormulaProductName == null) 1757 if (Configuration.errorOnAutoCreate()) 1758 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaProductName"); 1759 else if (Configuration.doAutoCreate()) 1760 this.baseFormulaProductName = new StringType(); // bb 1761 return this.baseFormulaProductName; 1762 } 1763 1764 public boolean hasBaseFormulaProductNameElement() { 1765 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1766 } 1767 1768 public boolean hasBaseFormulaProductName() { 1769 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1770 } 1771 1772 /** 1773 * @param value {@link #baseFormulaProductName} (The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula".). This is the underlying object with id, value and extensions. The accessor "getBaseFormulaProductName" gives direct access to the value 1774 */ 1775 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductNameElement(StringType value) { 1776 this.baseFormulaProductName = value; 1777 return this; 1778 } 1779 1780 /** 1781 * @return The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 1782 */ 1783 public String getBaseFormulaProductName() { 1784 return this.baseFormulaProductName == null ? null : this.baseFormulaProductName.getValue(); 1785 } 1786 1787 /** 1788 * @param value The product or brand name of the enteral or infant formula product such as "ACME Adult Standard Formula". 1789 */ 1790 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductName(String value) { 1791 if (Utilities.noString(value)) 1792 this.baseFormulaProductName = null; 1793 else { 1794 if (this.baseFormulaProductName == null) 1795 this.baseFormulaProductName = new StringType(); 1796 this.baseFormulaProductName.setValue(value); 1797 } 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #additiveType} (Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.) 1803 */ 1804 public CodeableConcept getAdditiveType() { 1805 if (this.additiveType == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveType"); 1808 else if (Configuration.doAutoCreate()) 1809 this.additiveType = new CodeableConcept(); // cc 1810 return this.additiveType; 1811 } 1812 1813 public boolean hasAdditiveType() { 1814 return this.additiveType != null && !this.additiveType.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #additiveType} (Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.) 1819 */ 1820 public NutritionOrderEnteralFormulaComponent setAdditiveType(CodeableConcept value) { 1821 this.additiveType = value; 1822 return this; 1823 } 1824 1825 /** 1826 * @return {@link #additiveProductName} (The product or brand name of the type of modular component to be added to the formula.). This is the underlying object with id, value and extensions. The accessor "getAdditiveProductName" gives direct access to the value 1827 */ 1828 public StringType getAdditiveProductNameElement() { 1829 if (this.additiveProductName == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveProductName"); 1832 else if (Configuration.doAutoCreate()) 1833 this.additiveProductName = new StringType(); // bb 1834 return this.additiveProductName; 1835 } 1836 1837 public boolean hasAdditiveProductNameElement() { 1838 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1839 } 1840 1841 public boolean hasAdditiveProductName() { 1842 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1843 } 1844 1845 /** 1846 * @param value {@link #additiveProductName} (The product or brand name of the type of modular component to be added to the formula.). This is the underlying object with id, value and extensions. The accessor "getAdditiveProductName" gives direct access to the value 1847 */ 1848 public NutritionOrderEnteralFormulaComponent setAdditiveProductNameElement(StringType value) { 1849 this.additiveProductName = value; 1850 return this; 1851 } 1852 1853 /** 1854 * @return The product or brand name of the type of modular component to be added to the formula. 1855 */ 1856 public String getAdditiveProductName() { 1857 return this.additiveProductName == null ? null : this.additiveProductName.getValue(); 1858 } 1859 1860 /** 1861 * @param value The product or brand name of the type of modular component to be added to the formula. 1862 */ 1863 public NutritionOrderEnteralFormulaComponent setAdditiveProductName(String value) { 1864 if (Utilities.noString(value)) 1865 this.additiveProductName = null; 1866 else { 1867 if (this.additiveProductName == null) 1868 this.additiveProductName = new StringType(); 1869 this.additiveProductName.setValue(value); 1870 } 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #caloricDensity} (The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.) 1876 */ 1877 public SimpleQuantity getCaloricDensity() { 1878 if (this.caloricDensity == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.caloricDensity"); 1881 else if (Configuration.doAutoCreate()) 1882 this.caloricDensity = new SimpleQuantity(); // cc 1883 return this.caloricDensity; 1884 } 1885 1886 public boolean hasCaloricDensity() { 1887 return this.caloricDensity != null && !this.caloricDensity.isEmpty(); 1888 } 1889 1890 /** 1891 * @param value {@link #caloricDensity} (The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.) 1892 */ 1893 public NutritionOrderEnteralFormulaComponent setCaloricDensity(SimpleQuantity value) { 1894 this.caloricDensity = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #routeofAdministration} (The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.) 1900 */ 1901 public CodeableConcept getRouteofAdministration() { 1902 if (this.routeofAdministration == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.routeofAdministration"); 1905 else if (Configuration.doAutoCreate()) 1906 this.routeofAdministration = new CodeableConcept(); // cc 1907 return this.routeofAdministration; 1908 } 1909 1910 public boolean hasRouteofAdministration() { 1911 return this.routeofAdministration != null && !this.routeofAdministration.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #routeofAdministration} (The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.) 1916 */ 1917 public NutritionOrderEnteralFormulaComponent setRouteofAdministration(CodeableConcept value) { 1918 this.routeofAdministration = value; 1919 return this; 1920 } 1921 1922 /** 1923 * @return {@link #administration} (Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.) 1924 */ 1925 public List<NutritionOrderEnteralFormulaAdministrationComponent> getAdministration() { 1926 if (this.administration == null) 1927 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1928 return this.administration; 1929 } 1930 1931 /** 1932 * @return Returns a reference to <code>this</code> for easy method chaining 1933 */ 1934 public NutritionOrderEnteralFormulaComponent setAdministration(List<NutritionOrderEnteralFormulaAdministrationComponent> theAdministration) { 1935 this.administration = theAdministration; 1936 return this; 1937 } 1938 1939 public boolean hasAdministration() { 1940 if (this.administration == null) 1941 return false; 1942 for (NutritionOrderEnteralFormulaAdministrationComponent item : this.administration) 1943 if (!item.isEmpty()) 1944 return true; 1945 return false; 1946 } 1947 1948 public NutritionOrderEnteralFormulaAdministrationComponent addAdministration() { //3 1949 NutritionOrderEnteralFormulaAdministrationComponent t = new NutritionOrderEnteralFormulaAdministrationComponent(); 1950 if (this.administration == null) 1951 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1952 this.administration.add(t); 1953 return t; 1954 } 1955 1956 public NutritionOrderEnteralFormulaComponent addAdministration(NutritionOrderEnteralFormulaAdministrationComponent t) { //3 1957 if (t == null) 1958 return this; 1959 if (this.administration == null) 1960 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1961 this.administration.add(t); 1962 return this; 1963 } 1964 1965 /** 1966 * @return The first repetition of repeating field {@link #administration}, creating it if it does not already exist 1967 */ 1968 public NutritionOrderEnteralFormulaAdministrationComponent getAdministrationFirstRep() { 1969 if (getAdministration().isEmpty()) { 1970 addAdministration(); 1971 } 1972 return getAdministration().get(0); 1973 } 1974 1975 /** 1976 * @return {@link #maxVolumeToDeliver} (The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.) 1977 */ 1978 public SimpleQuantity getMaxVolumeToDeliver() { 1979 if (this.maxVolumeToDeliver == null) 1980 if (Configuration.errorOnAutoCreate()) 1981 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.maxVolumeToDeliver"); 1982 else if (Configuration.doAutoCreate()) 1983 this.maxVolumeToDeliver = new SimpleQuantity(); // cc 1984 return this.maxVolumeToDeliver; 1985 } 1986 1987 public boolean hasMaxVolumeToDeliver() { 1988 return this.maxVolumeToDeliver != null && !this.maxVolumeToDeliver.isEmpty(); 1989 } 1990 1991 /** 1992 * @param value {@link #maxVolumeToDeliver} (The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.) 1993 */ 1994 public NutritionOrderEnteralFormulaComponent setMaxVolumeToDeliver(SimpleQuantity value) { 1995 this.maxVolumeToDeliver = value; 1996 return this; 1997 } 1998 1999 /** 2000 * @return {@link #administrationInstruction} (Free text formula administration, feeding instructions or additional instructions or information.). This is the underlying object with id, value and extensions. The accessor "getAdministrationInstruction" gives direct access to the value 2001 */ 2002 public StringType getAdministrationInstructionElement() { 2003 if (this.administrationInstruction == null) 2004 if (Configuration.errorOnAutoCreate()) 2005 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.administrationInstruction"); 2006 else if (Configuration.doAutoCreate()) 2007 this.administrationInstruction = new StringType(); // bb 2008 return this.administrationInstruction; 2009 } 2010 2011 public boolean hasAdministrationInstructionElement() { 2012 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2013 } 2014 2015 public boolean hasAdministrationInstruction() { 2016 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2017 } 2018 2019 /** 2020 * @param value {@link #administrationInstruction} (Free text formula administration, feeding instructions or additional instructions or information.). This is the underlying object with id, value and extensions. The accessor "getAdministrationInstruction" gives direct access to the value 2021 */ 2022 public NutritionOrderEnteralFormulaComponent setAdministrationInstructionElement(StringType value) { 2023 this.administrationInstruction = value; 2024 return this; 2025 } 2026 2027 /** 2028 * @return Free text formula administration, feeding instructions or additional instructions or information. 2029 */ 2030 public String getAdministrationInstruction() { 2031 return this.administrationInstruction == null ? null : this.administrationInstruction.getValue(); 2032 } 2033 2034 /** 2035 * @param value Free text formula administration, feeding instructions or additional instructions or information. 2036 */ 2037 public NutritionOrderEnteralFormulaComponent setAdministrationInstruction(String value) { 2038 if (Utilities.noString(value)) 2039 this.administrationInstruction = null; 2040 else { 2041 if (this.administrationInstruction == null) 2042 this.administrationInstruction = new StringType(); 2043 this.administrationInstruction.setValue(value); 2044 } 2045 return this; 2046 } 2047 2048 protected void listChildren(List<Property> children) { 2049 super.listChildren(children); 2050 children.add(new Property("baseFormulaType", "CodeableConcept", "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 0, 1, baseFormulaType)); 2051 children.add(new Property("baseFormulaProductName", "string", "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 0, 1, baseFormulaProductName)); 2052 children.add(new Property("additiveType", "CodeableConcept", "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 0, 1, additiveType)); 2053 children.add(new Property("additiveProductName", "string", "The product or brand name of the type of modular component to be added to the formula.", 0, 1, additiveProductName)); 2054 children.add(new Property("caloricDensity", "SimpleQuantity", "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 0, 1, caloricDensity)); 2055 children.add(new Property("routeofAdministration", "CodeableConcept", "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 0, 1, routeofAdministration)); 2056 children.add(new Property("administration", "", "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 0, java.lang.Integer.MAX_VALUE, administration)); 2057 children.add(new Property("maxVolumeToDeliver", "SimpleQuantity", "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 0, 1, maxVolumeToDeliver)); 2058 children.add(new Property("administrationInstruction", "string", "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, administrationInstruction)); 2059 } 2060 2061 @Override 2062 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2063 switch (_hash) { 2064 case -138930641: /*baseFormulaType*/ return new Property("baseFormulaType", "CodeableConcept", "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 0, 1, baseFormulaType); 2065 case -1267705979: /*baseFormulaProductName*/ return new Property("baseFormulaProductName", "string", "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 0, 1, baseFormulaProductName); 2066 case -470746842: /*additiveType*/ return new Property("additiveType", "CodeableConcept", "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 0, 1, additiveType); 2067 case 488079534: /*additiveProductName*/ return new Property("additiveProductName", "string", "The product or brand name of the type of modular component to be added to the formula.", 0, 1, additiveProductName); 2068 case 186983261: /*caloricDensity*/ return new Property("caloricDensity", "SimpleQuantity", "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 0, 1, caloricDensity); 2069 case -1710107042: /*routeofAdministration*/ return new Property("routeofAdministration", "CodeableConcept", "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 0, 1, routeofAdministration); 2070 case 1255702622: /*administration*/ return new Property("administration", "", "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 0, java.lang.Integer.MAX_VALUE, administration); 2071 case 2017924652: /*maxVolumeToDeliver*/ return new Property("maxVolumeToDeliver", "SimpleQuantity", "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 0, 1, maxVolumeToDeliver); 2072 case 427085136: /*administrationInstruction*/ return new Property("administrationInstruction", "string", "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, administrationInstruction); 2073 default: return super.getNamedProperty(_hash, _name, _checkValid); 2074 } 2075 2076 } 2077 2078 @Override 2079 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2080 switch (hash) { 2081 case -138930641: /*baseFormulaType*/ return this.baseFormulaType == null ? new Base[0] : new Base[] {this.baseFormulaType}; // CodeableConcept 2082 case -1267705979: /*baseFormulaProductName*/ return this.baseFormulaProductName == null ? new Base[0] : new Base[] {this.baseFormulaProductName}; // StringType 2083 case -470746842: /*additiveType*/ return this.additiveType == null ? new Base[0] : new Base[] {this.additiveType}; // CodeableConcept 2084 case 488079534: /*additiveProductName*/ return this.additiveProductName == null ? new Base[0] : new Base[] {this.additiveProductName}; // StringType 2085 case 186983261: /*caloricDensity*/ return this.caloricDensity == null ? new Base[0] : new Base[] {this.caloricDensity}; // SimpleQuantity 2086 case -1710107042: /*routeofAdministration*/ return this.routeofAdministration == null ? new Base[0] : new Base[] {this.routeofAdministration}; // CodeableConcept 2087 case 1255702622: /*administration*/ return this.administration == null ? new Base[0] : this.administration.toArray(new Base[this.administration.size()]); // NutritionOrderEnteralFormulaAdministrationComponent 2088 case 2017924652: /*maxVolumeToDeliver*/ return this.maxVolumeToDeliver == null ? new Base[0] : new Base[] {this.maxVolumeToDeliver}; // SimpleQuantity 2089 case 427085136: /*administrationInstruction*/ return this.administrationInstruction == null ? new Base[0] : new Base[] {this.administrationInstruction}; // StringType 2090 default: return super.getProperty(hash, name, checkValid); 2091 } 2092 2093 } 2094 2095 @Override 2096 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2097 switch (hash) { 2098 case -138930641: // baseFormulaType 2099 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 2100 return value; 2101 case -1267705979: // baseFormulaProductName 2102 this.baseFormulaProductName = castToString(value); // StringType 2103 return value; 2104 case -470746842: // additiveType 2105 this.additiveType = castToCodeableConcept(value); // CodeableConcept 2106 return value; 2107 case 488079534: // additiveProductName 2108 this.additiveProductName = castToString(value); // StringType 2109 return value; 2110 case 186983261: // caloricDensity 2111 this.caloricDensity = castToSimpleQuantity(value); // SimpleQuantity 2112 return value; 2113 case -1710107042: // routeofAdministration 2114 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 2115 return value; 2116 case 1255702622: // administration 2117 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); // NutritionOrderEnteralFormulaAdministrationComponent 2118 return value; 2119 case 2017924652: // maxVolumeToDeliver 2120 this.maxVolumeToDeliver = castToSimpleQuantity(value); // SimpleQuantity 2121 return value; 2122 case 427085136: // administrationInstruction 2123 this.administrationInstruction = castToString(value); // StringType 2124 return value; 2125 default: return super.setProperty(hash, name, value); 2126 } 2127 2128 } 2129 2130 @Override 2131 public Base setProperty(String name, Base value) throws FHIRException { 2132 if (name.equals("baseFormulaType")) { 2133 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 2134 } else if (name.equals("baseFormulaProductName")) { 2135 this.baseFormulaProductName = castToString(value); // StringType 2136 } else if (name.equals("additiveType")) { 2137 this.additiveType = castToCodeableConcept(value); // CodeableConcept 2138 } else if (name.equals("additiveProductName")) { 2139 this.additiveProductName = castToString(value); // StringType 2140 } else if (name.equals("caloricDensity")) { 2141 this.caloricDensity = castToSimpleQuantity(value); // SimpleQuantity 2142 } else if (name.equals("routeofAdministration")) { 2143 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 2144 } else if (name.equals("administration")) { 2145 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); 2146 } else if (name.equals("maxVolumeToDeliver")) { 2147 this.maxVolumeToDeliver = castToSimpleQuantity(value); // SimpleQuantity 2148 } else if (name.equals("administrationInstruction")) { 2149 this.administrationInstruction = castToString(value); // StringType 2150 } else 2151 return super.setProperty(name, value); 2152 return value; 2153 } 2154 2155 @Override 2156 public Base makeProperty(int hash, String name) throws FHIRException { 2157 switch (hash) { 2158 case -138930641: return getBaseFormulaType(); 2159 case -1267705979: return getBaseFormulaProductNameElement(); 2160 case -470746842: return getAdditiveType(); 2161 case 488079534: return getAdditiveProductNameElement(); 2162 case 186983261: return getCaloricDensity(); 2163 case -1710107042: return getRouteofAdministration(); 2164 case 1255702622: return addAdministration(); 2165 case 2017924652: return getMaxVolumeToDeliver(); 2166 case 427085136: return getAdministrationInstructionElement(); 2167 default: return super.makeProperty(hash, name); 2168 } 2169 2170 } 2171 2172 @Override 2173 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2174 switch (hash) { 2175 case -138930641: /*baseFormulaType*/ return new String[] {"CodeableConcept"}; 2176 case -1267705979: /*baseFormulaProductName*/ return new String[] {"string"}; 2177 case -470746842: /*additiveType*/ return new String[] {"CodeableConcept"}; 2178 case 488079534: /*additiveProductName*/ return new String[] {"string"}; 2179 case 186983261: /*caloricDensity*/ return new String[] {"SimpleQuantity"}; 2180 case -1710107042: /*routeofAdministration*/ return new String[] {"CodeableConcept"}; 2181 case 1255702622: /*administration*/ return new String[] {}; 2182 case 2017924652: /*maxVolumeToDeliver*/ return new String[] {"SimpleQuantity"}; 2183 case 427085136: /*administrationInstruction*/ return new String[] {"string"}; 2184 default: return super.getTypesForProperty(hash, name); 2185 } 2186 2187 } 2188 2189 @Override 2190 public Base addChild(String name) throws FHIRException { 2191 if (name.equals("baseFormulaType")) { 2192 this.baseFormulaType = new CodeableConcept(); 2193 return this.baseFormulaType; 2194 } 2195 else if (name.equals("baseFormulaProductName")) { 2196 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.baseFormulaProductName"); 2197 } 2198 else if (name.equals("additiveType")) { 2199 this.additiveType = new CodeableConcept(); 2200 return this.additiveType; 2201 } 2202 else if (name.equals("additiveProductName")) { 2203 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.additiveProductName"); 2204 } 2205 else if (name.equals("caloricDensity")) { 2206 this.caloricDensity = new SimpleQuantity(); 2207 return this.caloricDensity; 2208 } 2209 else if (name.equals("routeofAdministration")) { 2210 this.routeofAdministration = new CodeableConcept(); 2211 return this.routeofAdministration; 2212 } 2213 else if (name.equals("administration")) { 2214 return addAdministration(); 2215 } 2216 else if (name.equals("maxVolumeToDeliver")) { 2217 this.maxVolumeToDeliver = new SimpleQuantity(); 2218 return this.maxVolumeToDeliver; 2219 } 2220 else if (name.equals("administrationInstruction")) { 2221 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.administrationInstruction"); 2222 } 2223 else 2224 return super.addChild(name); 2225 } 2226 2227 public NutritionOrderEnteralFormulaComponent copy() { 2228 NutritionOrderEnteralFormulaComponent dst = new NutritionOrderEnteralFormulaComponent(); 2229 copyValues(dst); 2230 dst.baseFormulaType = baseFormulaType == null ? null : baseFormulaType.copy(); 2231 dst.baseFormulaProductName = baseFormulaProductName == null ? null : baseFormulaProductName.copy(); 2232 dst.additiveType = additiveType == null ? null : additiveType.copy(); 2233 dst.additiveProductName = additiveProductName == null ? null : additiveProductName.copy(); 2234 dst.caloricDensity = caloricDensity == null ? null : caloricDensity.copy(); 2235 dst.routeofAdministration = routeofAdministration == null ? null : routeofAdministration.copy(); 2236 if (administration != null) { 2237 dst.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2238 for (NutritionOrderEnteralFormulaAdministrationComponent i : administration) 2239 dst.administration.add(i.copy()); 2240 }; 2241 dst.maxVolumeToDeliver = maxVolumeToDeliver == null ? null : maxVolumeToDeliver.copy(); 2242 dst.administrationInstruction = administrationInstruction == null ? null : administrationInstruction.copy(); 2243 return dst; 2244 } 2245 2246 @Override 2247 public boolean equalsDeep(Base other_) { 2248 if (!super.equalsDeep(other_)) 2249 return false; 2250 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 2251 return false; 2252 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 2253 return compareDeep(baseFormulaType, o.baseFormulaType, true) && compareDeep(baseFormulaProductName, o.baseFormulaProductName, true) 2254 && compareDeep(additiveType, o.additiveType, true) && compareDeep(additiveProductName, o.additiveProductName, true) 2255 && compareDeep(caloricDensity, o.caloricDensity, true) && compareDeep(routeofAdministration, o.routeofAdministration, true) 2256 && compareDeep(administration, o.administration, true) && compareDeep(maxVolumeToDeliver, o.maxVolumeToDeliver, true) 2257 && compareDeep(administrationInstruction, o.administrationInstruction, true); 2258 } 2259 2260 @Override 2261 public boolean equalsShallow(Base other_) { 2262 if (!super.equalsShallow(other_)) 2263 return false; 2264 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 2265 return false; 2266 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 2267 return compareValues(baseFormulaProductName, o.baseFormulaProductName, true) && compareValues(additiveProductName, o.additiveProductName, true) 2268 && compareValues(administrationInstruction, o.administrationInstruction, true); 2269 } 2270 2271 public boolean isEmpty() { 2272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(baseFormulaType, baseFormulaProductName 2273 , additiveType, additiveProductName, caloricDensity, routeofAdministration, administration 2274 , maxVolumeToDeliver, administrationInstruction); 2275 } 2276 2277 public String fhirType() { 2278 return "NutritionOrder.enteralFormula"; 2279 2280 } 2281 2282 } 2283 2284 @Block() 2285 public static class NutritionOrderEnteralFormulaAdministrationComponent extends BackboneElement implements IBaseBackboneElement { 2286 /** 2287 * The time period and frequency at which the enteral formula should be delivered to the patient. 2288 */ 2289 @Child(name = "schedule", type = {Timing.class}, order=1, min=0, max=1, modifier=false, summary=false) 2290 @Description(shortDefinition="Scheduled frequency of enteral feeding", formalDefinition="The time period and frequency at which the enteral formula should be delivered to the patient." ) 2291 protected Timing schedule; 2292 2293 /** 2294 * The volume of formula to provide to the patient per the specified administration schedule. 2295 */ 2296 @Child(name = "quantity", type = {SimpleQuantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 2297 @Description(shortDefinition="The volume of formula to provide", formalDefinition="The volume of formula to provide to the patient per the specified administration schedule." ) 2298 protected SimpleQuantity quantity; 2299 2300 /** 2301 * The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule. 2302 */ 2303 @Child(name = "rate", type = {SimpleQuantity.class, Ratio.class}, order=3, min=0, max=1, modifier=false, summary=false) 2304 @Description(shortDefinition="Speed with which the formula is provided per period of time", formalDefinition="The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule." ) 2305 protected Type rate; 2306 2307 private static final long serialVersionUID = 1895031997L; 2308 2309 /** 2310 * Constructor 2311 */ 2312 public NutritionOrderEnteralFormulaAdministrationComponent() { 2313 super(); 2314 } 2315 2316 /** 2317 * @return {@link #schedule} (The time period and frequency at which the enteral formula should be delivered to the patient.) 2318 */ 2319 public Timing getSchedule() { 2320 if (this.schedule == null) 2321 if (Configuration.errorOnAutoCreate()) 2322 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.schedule"); 2323 else if (Configuration.doAutoCreate()) 2324 this.schedule = new Timing(); // cc 2325 return this.schedule; 2326 } 2327 2328 public boolean hasSchedule() { 2329 return this.schedule != null && !this.schedule.isEmpty(); 2330 } 2331 2332 /** 2333 * @param value {@link #schedule} (The time period and frequency at which the enteral formula should be delivered to the patient.) 2334 */ 2335 public NutritionOrderEnteralFormulaAdministrationComponent setSchedule(Timing value) { 2336 this.schedule = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #quantity} (The volume of formula to provide to the patient per the specified administration schedule.) 2342 */ 2343 public SimpleQuantity getQuantity() { 2344 if (this.quantity == null) 2345 if (Configuration.errorOnAutoCreate()) 2346 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.quantity"); 2347 else if (Configuration.doAutoCreate()) 2348 this.quantity = new SimpleQuantity(); // cc 2349 return this.quantity; 2350 } 2351 2352 public boolean hasQuantity() { 2353 return this.quantity != null && !this.quantity.isEmpty(); 2354 } 2355 2356 /** 2357 * @param value {@link #quantity} (The volume of formula to provide to the patient per the specified administration schedule.) 2358 */ 2359 public NutritionOrderEnteralFormulaAdministrationComponent setQuantity(SimpleQuantity value) { 2360 this.quantity = value; 2361 return this; 2362 } 2363 2364 /** 2365 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 2366 */ 2367 public Type getRate() { 2368 return this.rate; 2369 } 2370 2371 /** 2372 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 2373 */ 2374 public SimpleQuantity getRateSimpleQuantity() throws FHIRException { 2375 if (this.rate == null) 2376 return null; 2377 if (!(this.rate instanceof SimpleQuantity)) 2378 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 2379 return (SimpleQuantity) this.rate; 2380 } 2381 2382 public boolean hasRateSimpleQuantity() { 2383 return this.rate instanceof SimpleQuantity; 2384 } 2385 2386 /** 2387 * @return {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 2388 */ 2389 public Ratio getRateRatio() throws FHIRException { 2390 if (this.rate == null) 2391 return null; 2392 if (!(this.rate instanceof Ratio)) 2393 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 2394 return (Ratio) this.rate; 2395 } 2396 2397 public boolean hasRateRatio() { 2398 return this.rate instanceof Ratio; 2399 } 2400 2401 public boolean hasRate() { 2402 return this.rate != null && !this.rate.isEmpty(); 2403 } 2404 2405 /** 2406 * @param value {@link #rate} (The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.) 2407 */ 2408 public NutritionOrderEnteralFormulaAdministrationComponent setRate(Type value) throws FHIRFormatError { 2409 if (value != null && !(value instanceof Quantity || value instanceof Ratio)) 2410 throw new FHIRFormatError("Not the right type for NutritionOrder.enteralFormula.administration.rate[x]: "+value.fhirType()); 2411 this.rate = value; 2412 return this; 2413 } 2414 2415 protected void listChildren(List<Property> children) { 2416 super.listChildren(children); 2417 children.add(new Property("schedule", "Timing", "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 1, schedule)); 2418 children.add(new Property("quantity", "SimpleQuantity", "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, quantity)); 2419 children.add(new Property("rate[x]", "SimpleQuantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate)); 2420 } 2421 2422 @Override 2423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2424 switch (_hash) { 2425 case -697920873: /*schedule*/ return new Property("schedule", "Timing", "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 1, schedule); 2426 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, quantity); 2427 case 983460768: /*rate[x]*/ return new Property("rate[x]", "SimpleQuantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 2428 case 3493088: /*rate*/ return new Property("rate[x]", "SimpleQuantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 2429 case -2121057955: /*rateSimpleQuantity*/ return new Property("rate[x]", "SimpleQuantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 2430 case 204021515: /*rateRatio*/ return new Property("rate[x]", "SimpleQuantity|Ratio", "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 0, 1, rate); 2431 default: return super.getNamedProperty(_hash, _name, _checkValid); 2432 } 2433 2434 } 2435 2436 @Override 2437 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2438 switch (hash) { 2439 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // Timing 2440 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 2441 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // Type 2442 default: return super.getProperty(hash, name, checkValid); 2443 } 2444 2445 } 2446 2447 @Override 2448 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2449 switch (hash) { 2450 case -697920873: // schedule 2451 this.schedule = castToTiming(value); // Timing 2452 return value; 2453 case -1285004149: // quantity 2454 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2455 return value; 2456 case 3493088: // rate 2457 this.rate = castToType(value); // Type 2458 return value; 2459 default: return super.setProperty(hash, name, value); 2460 } 2461 2462 } 2463 2464 @Override 2465 public Base setProperty(String name, Base value) throws FHIRException { 2466 if (name.equals("schedule")) { 2467 this.schedule = castToTiming(value); // Timing 2468 } else if (name.equals("quantity")) { 2469 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2470 } else if (name.equals("rate[x]")) { 2471 this.rate = castToType(value); // Type 2472 } else 2473 return super.setProperty(name, value); 2474 return value; 2475 } 2476 2477 @Override 2478 public Base makeProperty(int hash, String name) throws FHIRException { 2479 switch (hash) { 2480 case -697920873: return getSchedule(); 2481 case -1285004149: return getQuantity(); 2482 case 983460768: return getRate(); 2483 case 3493088: return getRate(); 2484 default: return super.makeProperty(hash, name); 2485 } 2486 2487 } 2488 2489 @Override 2490 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2491 switch (hash) { 2492 case -697920873: /*schedule*/ return new String[] {"Timing"}; 2493 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 2494 case 3493088: /*rate*/ return new String[] {"SimpleQuantity", "Ratio"}; 2495 default: return super.getTypesForProperty(hash, name); 2496 } 2497 2498 } 2499 2500 @Override 2501 public Base addChild(String name) throws FHIRException { 2502 if (name.equals("schedule")) { 2503 this.schedule = new Timing(); 2504 return this.schedule; 2505 } 2506 else if (name.equals("quantity")) { 2507 this.quantity = new SimpleQuantity(); 2508 return this.quantity; 2509 } 2510 else if (name.equals("rateSimpleQuantity")) { 2511 this.rate = new SimpleQuantity(); 2512 return this.rate; 2513 } 2514 else if (name.equals("rateRatio")) { 2515 this.rate = new Ratio(); 2516 return this.rate; 2517 } 2518 else 2519 return super.addChild(name); 2520 } 2521 2522 public NutritionOrderEnteralFormulaAdministrationComponent copy() { 2523 NutritionOrderEnteralFormulaAdministrationComponent dst = new NutritionOrderEnteralFormulaAdministrationComponent(); 2524 copyValues(dst); 2525 dst.schedule = schedule == null ? null : schedule.copy(); 2526 dst.quantity = quantity == null ? null : quantity.copy(); 2527 dst.rate = rate == null ? null : rate.copy(); 2528 return dst; 2529 } 2530 2531 @Override 2532 public boolean equalsDeep(Base other_) { 2533 if (!super.equalsDeep(other_)) 2534 return false; 2535 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2536 return false; 2537 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 2538 return compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) && compareDeep(rate, o.rate, true) 2539 ; 2540 } 2541 2542 @Override 2543 public boolean equalsShallow(Base other_) { 2544 if (!super.equalsShallow(other_)) 2545 return false; 2546 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2547 return false; 2548 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 2549 return true; 2550 } 2551 2552 public boolean isEmpty() { 2553 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(schedule, quantity, rate 2554 ); 2555 } 2556 2557 public String fhirType() { 2558 return "NutritionOrder.enteralFormula.administration"; 2559 2560 } 2561 2562 } 2563 2564 /** 2565 * Identifiers assigned to this order by the order sender or by the order receiver. 2566 */ 2567 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2568 @Description(shortDefinition="Identifiers assigned to this order", formalDefinition="Identifiers assigned to this order by the order sender or by the order receiver." ) 2569 protected List<Identifier> identifier; 2570 2571 /** 2572 * The workflow status of the nutrition order/request. 2573 */ 2574 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 2575 @Description(shortDefinition="proposed | draft | planned | requested | active | on-hold | completed | cancelled | entered-in-error", formalDefinition="The workflow status of the nutrition order/request." ) 2576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/nutrition-request-status") 2577 protected Enumeration<NutritionOrderStatus> status; 2578 2579 /** 2580 * The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding. 2581 */ 2582 @Child(name = "patient", type = {Patient.class}, order=2, min=1, max=1, modifier=false, summary=true) 2583 @Description(shortDefinition="The person who requires the diet, formula or nutritional supplement", formalDefinition="The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding." ) 2584 protected Reference patient; 2585 2586 /** 2587 * The actual object that is the target of the reference (The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 2588 */ 2589 protected Patient patientTarget; 2590 2591 /** 2592 * An encounter that provides additional information about the healthcare context in which this request is made. 2593 */ 2594 @Child(name = "encounter", type = {Encounter.class}, order=3, min=0, max=1, modifier=false, summary=false) 2595 @Description(shortDefinition="The encounter associated with this nutrition order", formalDefinition="An encounter that provides additional information about the healthcare context in which this request is made." ) 2596 protected Reference encounter; 2597 2598 /** 2599 * The actual object that is the target of the reference (An encounter that provides additional information about the healthcare context in which this request is made.) 2600 */ 2601 protected Encounter encounterTarget; 2602 2603 /** 2604 * The date and time that this nutrition order was requested. 2605 */ 2606 @Child(name = "dateTime", type = {DateTimeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 2607 @Description(shortDefinition="Date and time the nutrition order was requested", formalDefinition="The date and time that this nutrition order was requested." ) 2608 protected DateTimeType dateTime; 2609 2610 /** 2611 * The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings. 2612 */ 2613 @Child(name = "orderer", type = {Practitioner.class}, order=5, min=0, max=1, modifier=false, summary=true) 2614 @Description(shortDefinition="Who ordered the diet, formula or nutritional supplement", formalDefinition="The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings." ) 2615 protected Reference orderer; 2616 2617 /** 2618 * The actual object that is the target of the reference (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 2619 */ 2620 protected Practitioner ordererTarget; 2621 2622 /** 2623 * A link to a record of allergies or intolerances which should be included in the nutrition order. 2624 */ 2625 @Child(name = "allergyIntolerance", type = {AllergyIntolerance.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2626 @Description(shortDefinition="List of the patient's food and nutrition-related allergies and intolerances", formalDefinition="A link to a record of allergies or intolerances which should be included in the nutrition order." ) 2627 protected List<Reference> allergyIntolerance; 2628 /** 2629 * The actual objects that are the target of the reference (A link to a record of allergies or intolerances which should be included in the nutrition order.) 2630 */ 2631 protected List<AllergyIntolerance> allergyIntoleranceTarget; 2632 2633 2634 /** 2635 * This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings. 2636 */ 2637 @Child(name = "foodPreferenceModifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2638 @Description(shortDefinition="Order-specific modifier about the type of food that should be given", formalDefinition="This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings." ) 2639 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diet") 2640 protected List<CodeableConcept> foodPreferenceModifier; 2641 2642 /** 2643 * This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings. 2644 */ 2645 @Child(name = "excludeFoodModifier", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2646 @Description(shortDefinition="Order-specific modifier about the type of food that should not be given", formalDefinition="This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings." ) 2647 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/food-type") 2648 protected List<CodeableConcept> excludeFoodModifier; 2649 2650 /** 2651 * Diet given orally in contrast to enteral (tube) feeding. 2652 */ 2653 @Child(name = "oralDiet", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 2654 @Description(shortDefinition="Oral diet components", formalDefinition="Diet given orally in contrast to enteral (tube) feeding." ) 2655 protected NutritionOrderOralDietComponent oralDiet; 2656 2657 /** 2658 * Oral nutritional products given in order to add further nutritional value to the patient's diet. 2659 */ 2660 @Child(name = "supplement", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2661 @Description(shortDefinition="Supplement components", formalDefinition="Oral nutritional products given in order to add further nutritional value to the patient's diet." ) 2662 protected List<NutritionOrderSupplementComponent> supplement; 2663 2664 /** 2665 * Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity. 2666 */ 2667 @Child(name = "enteralFormula", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 2668 @Description(shortDefinition="Enteral formula components", formalDefinition="Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity." ) 2669 protected NutritionOrderEnteralFormulaComponent enteralFormula; 2670 2671 private static final long serialVersionUID = 1429947433L; 2672 2673 /** 2674 * Constructor 2675 */ 2676 public NutritionOrder() { 2677 super(); 2678 } 2679 2680 /** 2681 * Constructor 2682 */ 2683 public NutritionOrder(Reference patient, DateTimeType dateTime) { 2684 super(); 2685 this.patient = patient; 2686 this.dateTime = dateTime; 2687 } 2688 2689 /** 2690 * @return {@link #identifier} (Identifiers assigned to this order by the order sender or by the order receiver.) 2691 */ 2692 public List<Identifier> getIdentifier() { 2693 if (this.identifier == null) 2694 this.identifier = new ArrayList<Identifier>(); 2695 return this.identifier; 2696 } 2697 2698 /** 2699 * @return Returns a reference to <code>this</code> for easy method chaining 2700 */ 2701 public NutritionOrder setIdentifier(List<Identifier> theIdentifier) { 2702 this.identifier = theIdentifier; 2703 return this; 2704 } 2705 2706 public boolean hasIdentifier() { 2707 if (this.identifier == null) 2708 return false; 2709 for (Identifier item : this.identifier) 2710 if (!item.isEmpty()) 2711 return true; 2712 return false; 2713 } 2714 2715 public Identifier addIdentifier() { //3 2716 Identifier t = new Identifier(); 2717 if (this.identifier == null) 2718 this.identifier = new ArrayList<Identifier>(); 2719 this.identifier.add(t); 2720 return t; 2721 } 2722 2723 public NutritionOrder addIdentifier(Identifier t) { //3 2724 if (t == null) 2725 return this; 2726 if (this.identifier == null) 2727 this.identifier = new ArrayList<Identifier>(); 2728 this.identifier.add(t); 2729 return this; 2730 } 2731 2732 /** 2733 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 2734 */ 2735 public Identifier getIdentifierFirstRep() { 2736 if (getIdentifier().isEmpty()) { 2737 addIdentifier(); 2738 } 2739 return getIdentifier().get(0); 2740 } 2741 2742 /** 2743 * @return {@link #status} (The workflow status of the nutrition order/request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2744 */ 2745 public Enumeration<NutritionOrderStatus> getStatusElement() { 2746 if (this.status == null) 2747 if (Configuration.errorOnAutoCreate()) 2748 throw new Error("Attempt to auto-create NutritionOrder.status"); 2749 else if (Configuration.doAutoCreate()) 2750 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); // bb 2751 return this.status; 2752 } 2753 2754 public boolean hasStatusElement() { 2755 return this.status != null && !this.status.isEmpty(); 2756 } 2757 2758 public boolean hasStatus() { 2759 return this.status != null && !this.status.isEmpty(); 2760 } 2761 2762 /** 2763 * @param value {@link #status} (The workflow status of the nutrition order/request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2764 */ 2765 public NutritionOrder setStatusElement(Enumeration<NutritionOrderStatus> value) { 2766 this.status = value; 2767 return this; 2768 } 2769 2770 /** 2771 * @return The workflow status of the nutrition order/request. 2772 */ 2773 public NutritionOrderStatus getStatus() { 2774 return this.status == null ? null : this.status.getValue(); 2775 } 2776 2777 /** 2778 * @param value The workflow status of the nutrition order/request. 2779 */ 2780 public NutritionOrder setStatus(NutritionOrderStatus value) { 2781 if (value == null) 2782 this.status = null; 2783 else { 2784 if (this.status == null) 2785 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); 2786 this.status.setValue(value); 2787 } 2788 return this; 2789 } 2790 2791 /** 2792 * @return {@link #patient} (The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 2793 */ 2794 public Reference getPatient() { 2795 if (this.patient == null) 2796 if (Configuration.errorOnAutoCreate()) 2797 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2798 else if (Configuration.doAutoCreate()) 2799 this.patient = new Reference(); // cc 2800 return this.patient; 2801 } 2802 2803 public boolean hasPatient() { 2804 return this.patient != null && !this.patient.isEmpty(); 2805 } 2806 2807 /** 2808 * @param value {@link #patient} (The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 2809 */ 2810 public NutritionOrder setPatient(Reference value) { 2811 this.patient = value; 2812 return this; 2813 } 2814 2815 /** 2816 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 2817 */ 2818 public Patient getPatientTarget() { 2819 if (this.patientTarget == null) 2820 if (Configuration.errorOnAutoCreate()) 2821 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2822 else if (Configuration.doAutoCreate()) 2823 this.patientTarget = new Patient(); // aa 2824 return this.patientTarget; 2825 } 2826 2827 /** 2828 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.) 2829 */ 2830 public NutritionOrder setPatientTarget(Patient value) { 2831 this.patientTarget = value; 2832 return this; 2833 } 2834 2835 /** 2836 * @return {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 2837 */ 2838 public Reference getEncounter() { 2839 if (this.encounter == null) 2840 if (Configuration.errorOnAutoCreate()) 2841 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2842 else if (Configuration.doAutoCreate()) 2843 this.encounter = new Reference(); // cc 2844 return this.encounter; 2845 } 2846 2847 public boolean hasEncounter() { 2848 return this.encounter != null && !this.encounter.isEmpty(); 2849 } 2850 2851 /** 2852 * @param value {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 2853 */ 2854 public NutritionOrder setEncounter(Reference value) { 2855 this.encounter = value; 2856 return this; 2857 } 2858 2859 /** 2860 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An encounter that provides additional information about the healthcare context in which this request is made.) 2861 */ 2862 public Encounter getEncounterTarget() { 2863 if (this.encounterTarget == null) 2864 if (Configuration.errorOnAutoCreate()) 2865 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2866 else if (Configuration.doAutoCreate()) 2867 this.encounterTarget = new Encounter(); // aa 2868 return this.encounterTarget; 2869 } 2870 2871 /** 2872 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An encounter that provides additional information about the healthcare context in which this request is made.) 2873 */ 2874 public NutritionOrder setEncounterTarget(Encounter value) { 2875 this.encounterTarget = value; 2876 return this; 2877 } 2878 2879 /** 2880 * @return {@link #dateTime} (The date and time that this nutrition order was requested.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2881 */ 2882 public DateTimeType getDateTimeElement() { 2883 if (this.dateTime == null) 2884 if (Configuration.errorOnAutoCreate()) 2885 throw new Error("Attempt to auto-create NutritionOrder.dateTime"); 2886 else if (Configuration.doAutoCreate()) 2887 this.dateTime = new DateTimeType(); // bb 2888 return this.dateTime; 2889 } 2890 2891 public boolean hasDateTimeElement() { 2892 return this.dateTime != null && !this.dateTime.isEmpty(); 2893 } 2894 2895 public boolean hasDateTime() { 2896 return this.dateTime != null && !this.dateTime.isEmpty(); 2897 } 2898 2899 /** 2900 * @param value {@link #dateTime} (The date and time that this nutrition order was requested.). This is the underlying object with id, value and extensions. The accessor "getDateTime" gives direct access to the value 2901 */ 2902 public NutritionOrder setDateTimeElement(DateTimeType value) { 2903 this.dateTime = value; 2904 return this; 2905 } 2906 2907 /** 2908 * @return The date and time that this nutrition order was requested. 2909 */ 2910 public Date getDateTime() { 2911 return this.dateTime == null ? null : this.dateTime.getValue(); 2912 } 2913 2914 /** 2915 * @param value The date and time that this nutrition order was requested. 2916 */ 2917 public NutritionOrder setDateTime(Date value) { 2918 if (this.dateTime == null) 2919 this.dateTime = new DateTimeType(); 2920 this.dateTime.setValue(value); 2921 return this; 2922 } 2923 2924 /** 2925 * @return {@link #orderer} (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 2926 */ 2927 public Reference getOrderer() { 2928 if (this.orderer == null) 2929 if (Configuration.errorOnAutoCreate()) 2930 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2931 else if (Configuration.doAutoCreate()) 2932 this.orderer = new Reference(); // cc 2933 return this.orderer; 2934 } 2935 2936 public boolean hasOrderer() { 2937 return this.orderer != null && !this.orderer.isEmpty(); 2938 } 2939 2940 /** 2941 * @param value {@link #orderer} (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 2942 */ 2943 public NutritionOrder setOrderer(Reference value) { 2944 this.orderer = value; 2945 return this; 2946 } 2947 2948 /** 2949 * @return {@link #orderer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 2950 */ 2951 public Practitioner getOrdererTarget() { 2952 if (this.ordererTarget == null) 2953 if (Configuration.errorOnAutoCreate()) 2954 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2955 else if (Configuration.doAutoCreate()) 2956 this.ordererTarget = new Practitioner(); // aa 2957 return this.ordererTarget; 2958 } 2959 2960 /** 2961 * @param value {@link #orderer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.) 2962 */ 2963 public NutritionOrder setOrdererTarget(Practitioner value) { 2964 this.ordererTarget = value; 2965 return this; 2966 } 2967 2968 /** 2969 * @return {@link #allergyIntolerance} (A link to a record of allergies or intolerances which should be included in the nutrition order.) 2970 */ 2971 public List<Reference> getAllergyIntolerance() { 2972 if (this.allergyIntolerance == null) 2973 this.allergyIntolerance = new ArrayList<Reference>(); 2974 return this.allergyIntolerance; 2975 } 2976 2977 /** 2978 * @return Returns a reference to <code>this</code> for easy method chaining 2979 */ 2980 public NutritionOrder setAllergyIntolerance(List<Reference> theAllergyIntolerance) { 2981 this.allergyIntolerance = theAllergyIntolerance; 2982 return this; 2983 } 2984 2985 public boolean hasAllergyIntolerance() { 2986 if (this.allergyIntolerance == null) 2987 return false; 2988 for (Reference item : this.allergyIntolerance) 2989 if (!item.isEmpty()) 2990 return true; 2991 return false; 2992 } 2993 2994 public Reference addAllergyIntolerance() { //3 2995 Reference t = new Reference(); 2996 if (this.allergyIntolerance == null) 2997 this.allergyIntolerance = new ArrayList<Reference>(); 2998 this.allergyIntolerance.add(t); 2999 return t; 3000 } 3001 3002 public NutritionOrder addAllergyIntolerance(Reference t) { //3 3003 if (t == null) 3004 return this; 3005 if (this.allergyIntolerance == null) 3006 this.allergyIntolerance = new ArrayList<Reference>(); 3007 this.allergyIntolerance.add(t); 3008 return this; 3009 } 3010 3011 /** 3012 * @return The first repetition of repeating field {@link #allergyIntolerance}, creating it if it does not already exist 3013 */ 3014 public Reference getAllergyIntoleranceFirstRep() { 3015 if (getAllergyIntolerance().isEmpty()) { 3016 addAllergyIntolerance(); 3017 } 3018 return getAllergyIntolerance().get(0); 3019 } 3020 3021 /** 3022 * @deprecated Use Reference#setResource(IBaseResource) instead 3023 */ 3024 @Deprecated 3025 public List<AllergyIntolerance> getAllergyIntoleranceTarget() { 3026 if (this.allergyIntoleranceTarget == null) 3027 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 3028 return this.allergyIntoleranceTarget; 3029 } 3030 3031 /** 3032 * @deprecated Use Reference#setResource(IBaseResource) instead 3033 */ 3034 @Deprecated 3035 public AllergyIntolerance addAllergyIntoleranceTarget() { 3036 AllergyIntolerance r = new AllergyIntolerance(); 3037 if (this.allergyIntoleranceTarget == null) 3038 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 3039 this.allergyIntoleranceTarget.add(r); 3040 return r; 3041 } 3042 3043 /** 3044 * @return {@link #foodPreferenceModifier} (This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.) 3045 */ 3046 public List<CodeableConcept> getFoodPreferenceModifier() { 3047 if (this.foodPreferenceModifier == null) 3048 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3049 return this.foodPreferenceModifier; 3050 } 3051 3052 /** 3053 * @return Returns a reference to <code>this</code> for easy method chaining 3054 */ 3055 public NutritionOrder setFoodPreferenceModifier(List<CodeableConcept> theFoodPreferenceModifier) { 3056 this.foodPreferenceModifier = theFoodPreferenceModifier; 3057 return this; 3058 } 3059 3060 public boolean hasFoodPreferenceModifier() { 3061 if (this.foodPreferenceModifier == null) 3062 return false; 3063 for (CodeableConcept item : this.foodPreferenceModifier) 3064 if (!item.isEmpty()) 3065 return true; 3066 return false; 3067 } 3068 3069 public CodeableConcept addFoodPreferenceModifier() { //3 3070 CodeableConcept t = new CodeableConcept(); 3071 if (this.foodPreferenceModifier == null) 3072 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3073 this.foodPreferenceModifier.add(t); 3074 return t; 3075 } 3076 3077 public NutritionOrder addFoodPreferenceModifier(CodeableConcept t) { //3 3078 if (t == null) 3079 return this; 3080 if (this.foodPreferenceModifier == null) 3081 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3082 this.foodPreferenceModifier.add(t); 3083 return this; 3084 } 3085 3086 /** 3087 * @return The first repetition of repeating field {@link #foodPreferenceModifier}, creating it if it does not already exist 3088 */ 3089 public CodeableConcept getFoodPreferenceModifierFirstRep() { 3090 if (getFoodPreferenceModifier().isEmpty()) { 3091 addFoodPreferenceModifier(); 3092 } 3093 return getFoodPreferenceModifier().get(0); 3094 } 3095 3096 /** 3097 * @return {@link #excludeFoodModifier} (This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.) 3098 */ 3099 public List<CodeableConcept> getExcludeFoodModifier() { 3100 if (this.excludeFoodModifier == null) 3101 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3102 return this.excludeFoodModifier; 3103 } 3104 3105 /** 3106 * @return Returns a reference to <code>this</code> for easy method chaining 3107 */ 3108 public NutritionOrder setExcludeFoodModifier(List<CodeableConcept> theExcludeFoodModifier) { 3109 this.excludeFoodModifier = theExcludeFoodModifier; 3110 return this; 3111 } 3112 3113 public boolean hasExcludeFoodModifier() { 3114 if (this.excludeFoodModifier == null) 3115 return false; 3116 for (CodeableConcept item : this.excludeFoodModifier) 3117 if (!item.isEmpty()) 3118 return true; 3119 return false; 3120 } 3121 3122 public CodeableConcept addExcludeFoodModifier() { //3 3123 CodeableConcept t = new CodeableConcept(); 3124 if (this.excludeFoodModifier == null) 3125 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3126 this.excludeFoodModifier.add(t); 3127 return t; 3128 } 3129 3130 public NutritionOrder addExcludeFoodModifier(CodeableConcept t) { //3 3131 if (t == null) 3132 return this; 3133 if (this.excludeFoodModifier == null) 3134 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3135 this.excludeFoodModifier.add(t); 3136 return this; 3137 } 3138 3139 /** 3140 * @return The first repetition of repeating field {@link #excludeFoodModifier}, creating it if it does not already exist 3141 */ 3142 public CodeableConcept getExcludeFoodModifierFirstRep() { 3143 if (getExcludeFoodModifier().isEmpty()) { 3144 addExcludeFoodModifier(); 3145 } 3146 return getExcludeFoodModifier().get(0); 3147 } 3148 3149 /** 3150 * @return {@link #oralDiet} (Diet given orally in contrast to enteral (tube) feeding.) 3151 */ 3152 public NutritionOrderOralDietComponent getOralDiet() { 3153 if (this.oralDiet == null) 3154 if (Configuration.errorOnAutoCreate()) 3155 throw new Error("Attempt to auto-create NutritionOrder.oralDiet"); 3156 else if (Configuration.doAutoCreate()) 3157 this.oralDiet = new NutritionOrderOralDietComponent(); // cc 3158 return this.oralDiet; 3159 } 3160 3161 public boolean hasOralDiet() { 3162 return this.oralDiet != null && !this.oralDiet.isEmpty(); 3163 } 3164 3165 /** 3166 * @param value {@link #oralDiet} (Diet given orally in contrast to enteral (tube) feeding.) 3167 */ 3168 public NutritionOrder setOralDiet(NutritionOrderOralDietComponent value) { 3169 this.oralDiet = value; 3170 return this; 3171 } 3172 3173 /** 3174 * @return {@link #supplement} (Oral nutritional products given in order to add further nutritional value to the patient's diet.) 3175 */ 3176 public List<NutritionOrderSupplementComponent> getSupplement() { 3177 if (this.supplement == null) 3178 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3179 return this.supplement; 3180 } 3181 3182 /** 3183 * @return Returns a reference to <code>this</code> for easy method chaining 3184 */ 3185 public NutritionOrder setSupplement(List<NutritionOrderSupplementComponent> theSupplement) { 3186 this.supplement = theSupplement; 3187 return this; 3188 } 3189 3190 public boolean hasSupplement() { 3191 if (this.supplement == null) 3192 return false; 3193 for (NutritionOrderSupplementComponent item : this.supplement) 3194 if (!item.isEmpty()) 3195 return true; 3196 return false; 3197 } 3198 3199 public NutritionOrderSupplementComponent addSupplement() { //3 3200 NutritionOrderSupplementComponent t = new NutritionOrderSupplementComponent(); 3201 if (this.supplement == null) 3202 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3203 this.supplement.add(t); 3204 return t; 3205 } 3206 3207 public NutritionOrder addSupplement(NutritionOrderSupplementComponent t) { //3 3208 if (t == null) 3209 return this; 3210 if (this.supplement == null) 3211 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3212 this.supplement.add(t); 3213 return this; 3214 } 3215 3216 /** 3217 * @return The first repetition of repeating field {@link #supplement}, creating it if it does not already exist 3218 */ 3219 public NutritionOrderSupplementComponent getSupplementFirstRep() { 3220 if (getSupplement().isEmpty()) { 3221 addSupplement(); 3222 } 3223 return getSupplement().get(0); 3224 } 3225 3226 /** 3227 * @return {@link #enteralFormula} (Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.) 3228 */ 3229 public NutritionOrderEnteralFormulaComponent getEnteralFormula() { 3230 if (this.enteralFormula == null) 3231 if (Configuration.errorOnAutoCreate()) 3232 throw new Error("Attempt to auto-create NutritionOrder.enteralFormula"); 3233 else if (Configuration.doAutoCreate()) 3234 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); // cc 3235 return this.enteralFormula; 3236 } 3237 3238 public boolean hasEnteralFormula() { 3239 return this.enteralFormula != null && !this.enteralFormula.isEmpty(); 3240 } 3241 3242 /** 3243 * @param value {@link #enteralFormula} (Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.) 3244 */ 3245 public NutritionOrder setEnteralFormula(NutritionOrderEnteralFormulaComponent value) { 3246 this.enteralFormula = value; 3247 return this; 3248 } 3249 3250 protected void listChildren(List<Property> children) { 3251 super.listChildren(children); 3252 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order by the order sender or by the order receiver.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3253 children.add(new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, status)); 3254 children.add(new Property("patient", "Reference(Patient)", "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 0, 1, patient)); 3255 children.add(new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter)); 3256 children.add(new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 0, 1, dateTime)); 3257 children.add(new Property("orderer", "Reference(Practitioner)", "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 0, 1, orderer)); 3258 children.add(new Property("allergyIntolerance", "Reference(AllergyIntolerance)", "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, java.lang.Integer.MAX_VALUE, allergyIntolerance)); 3259 children.add(new Property("foodPreferenceModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier)); 3260 children.add(new Property("excludeFoodModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier)); 3261 children.add(new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 1, oralDiet)); 3262 children.add(new Property("supplement", "", "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, java.lang.Integer.MAX_VALUE, supplement)); 3263 children.add(new Property("enteralFormula", "", "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 0, 1, enteralFormula)); 3264 } 3265 3266 @Override 3267 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3268 switch (_hash) { 3269 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order by the order sender or by the order receiver.", 0, java.lang.Integer.MAX_VALUE, identifier); 3270 case -892481550: /*status*/ return new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, status); 3271 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 0, 1, patient); 3272 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter); 3273 case 1792749467: /*dateTime*/ return new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 0, 1, dateTime); 3274 case -1207109509: /*orderer*/ return new Property("orderer", "Reference(Practitioner)", "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 0, 1, orderer); 3275 case -120164120: /*allergyIntolerance*/ return new Property("allergyIntolerance", "Reference(AllergyIntolerance)", "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, java.lang.Integer.MAX_VALUE, allergyIntolerance); 3276 case 659473872: /*foodPreferenceModifier*/ return new Property("foodPreferenceModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier); 3277 case 1760260175: /*excludeFoodModifier*/ return new Property("excludeFoodModifier", "CodeableConcept", "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier); 3278 case 1153521250: /*oralDiet*/ return new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 1, oralDiet); 3279 case -711993159: /*supplement*/ return new Property("supplement", "", "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, java.lang.Integer.MAX_VALUE, supplement); 3280 case -671083805: /*enteralFormula*/ return new Property("enteralFormula", "", "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 0, 1, enteralFormula); 3281 default: return super.getNamedProperty(_hash, _name, _checkValid); 3282 } 3283 3284 } 3285 3286 @Override 3287 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3288 switch (hash) { 3289 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3290 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<NutritionOrderStatus> 3291 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 3292 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3293 case 1792749467: /*dateTime*/ return this.dateTime == null ? new Base[0] : new Base[] {this.dateTime}; // DateTimeType 3294 case -1207109509: /*orderer*/ return this.orderer == null ? new Base[0] : new Base[] {this.orderer}; // Reference 3295 case -120164120: /*allergyIntolerance*/ return this.allergyIntolerance == null ? new Base[0] : this.allergyIntolerance.toArray(new Base[this.allergyIntolerance.size()]); // Reference 3296 case 659473872: /*foodPreferenceModifier*/ return this.foodPreferenceModifier == null ? new Base[0] : this.foodPreferenceModifier.toArray(new Base[this.foodPreferenceModifier.size()]); // CodeableConcept 3297 case 1760260175: /*excludeFoodModifier*/ return this.excludeFoodModifier == null ? new Base[0] : this.excludeFoodModifier.toArray(new Base[this.excludeFoodModifier.size()]); // CodeableConcept 3298 case 1153521250: /*oralDiet*/ return this.oralDiet == null ? new Base[0] : new Base[] {this.oralDiet}; // NutritionOrderOralDietComponent 3299 case -711993159: /*supplement*/ return this.supplement == null ? new Base[0] : this.supplement.toArray(new Base[this.supplement.size()]); // NutritionOrderSupplementComponent 3300 case -671083805: /*enteralFormula*/ return this.enteralFormula == null ? new Base[0] : new Base[] {this.enteralFormula}; // NutritionOrderEnteralFormulaComponent 3301 default: return super.getProperty(hash, name, checkValid); 3302 } 3303 3304 } 3305 3306 @Override 3307 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3308 switch (hash) { 3309 case -1618432855: // identifier 3310 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3311 return value; 3312 case -892481550: // status 3313 value = new NutritionOrderStatusEnumFactory().fromType(castToCode(value)); 3314 this.status = (Enumeration) value; // Enumeration<NutritionOrderStatus> 3315 return value; 3316 case -791418107: // patient 3317 this.patient = castToReference(value); // Reference 3318 return value; 3319 case 1524132147: // encounter 3320 this.encounter = castToReference(value); // Reference 3321 return value; 3322 case 1792749467: // dateTime 3323 this.dateTime = castToDateTime(value); // DateTimeType 3324 return value; 3325 case -1207109509: // orderer 3326 this.orderer = castToReference(value); // Reference 3327 return value; 3328 case -120164120: // allergyIntolerance 3329 this.getAllergyIntolerance().add(castToReference(value)); // Reference 3330 return value; 3331 case 659473872: // foodPreferenceModifier 3332 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); // CodeableConcept 3333 return value; 3334 case 1760260175: // excludeFoodModifier 3335 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); // CodeableConcept 3336 return value; 3337 case 1153521250: // oralDiet 3338 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 3339 return value; 3340 case -711993159: // supplement 3341 this.getSupplement().add((NutritionOrderSupplementComponent) value); // NutritionOrderSupplementComponent 3342 return value; 3343 case -671083805: // enteralFormula 3344 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 3345 return value; 3346 default: return super.setProperty(hash, name, value); 3347 } 3348 3349 } 3350 3351 @Override 3352 public Base setProperty(String name, Base value) throws FHIRException { 3353 if (name.equals("identifier")) { 3354 this.getIdentifier().add(castToIdentifier(value)); 3355 } else if (name.equals("status")) { 3356 value = new NutritionOrderStatusEnumFactory().fromType(castToCode(value)); 3357 this.status = (Enumeration) value; // Enumeration<NutritionOrderStatus> 3358 } else if (name.equals("patient")) { 3359 this.patient = castToReference(value); // Reference 3360 } else if (name.equals("encounter")) { 3361 this.encounter = castToReference(value); // Reference 3362 } else if (name.equals("dateTime")) { 3363 this.dateTime = castToDateTime(value); // DateTimeType 3364 } else if (name.equals("orderer")) { 3365 this.orderer = castToReference(value); // Reference 3366 } else if (name.equals("allergyIntolerance")) { 3367 this.getAllergyIntolerance().add(castToReference(value)); 3368 } else if (name.equals("foodPreferenceModifier")) { 3369 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); 3370 } else if (name.equals("excludeFoodModifier")) { 3371 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); 3372 } else if (name.equals("oralDiet")) { 3373 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 3374 } else if (name.equals("supplement")) { 3375 this.getSupplement().add((NutritionOrderSupplementComponent) value); 3376 } else if (name.equals("enteralFormula")) { 3377 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 3378 } else 3379 return super.setProperty(name, value); 3380 return value; 3381 } 3382 3383 @Override 3384 public Base makeProperty(int hash, String name) throws FHIRException { 3385 switch (hash) { 3386 case -1618432855: return addIdentifier(); 3387 case -892481550: return getStatusElement(); 3388 case -791418107: return getPatient(); 3389 case 1524132147: return getEncounter(); 3390 case 1792749467: return getDateTimeElement(); 3391 case -1207109509: return getOrderer(); 3392 case -120164120: return addAllergyIntolerance(); 3393 case 659473872: return addFoodPreferenceModifier(); 3394 case 1760260175: return addExcludeFoodModifier(); 3395 case 1153521250: return getOralDiet(); 3396 case -711993159: return addSupplement(); 3397 case -671083805: return getEnteralFormula(); 3398 default: return super.makeProperty(hash, name); 3399 } 3400 3401 } 3402 3403 @Override 3404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3405 switch (hash) { 3406 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3407 case -892481550: /*status*/ return new String[] {"code"}; 3408 case -791418107: /*patient*/ return new String[] {"Reference"}; 3409 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3410 case 1792749467: /*dateTime*/ return new String[] {"dateTime"}; 3411 case -1207109509: /*orderer*/ return new String[] {"Reference"}; 3412 case -120164120: /*allergyIntolerance*/ return new String[] {"Reference"}; 3413 case 659473872: /*foodPreferenceModifier*/ return new String[] {"CodeableConcept"}; 3414 case 1760260175: /*excludeFoodModifier*/ return new String[] {"CodeableConcept"}; 3415 case 1153521250: /*oralDiet*/ return new String[] {}; 3416 case -711993159: /*supplement*/ return new String[] {}; 3417 case -671083805: /*enteralFormula*/ return new String[] {}; 3418 default: return super.getTypesForProperty(hash, name); 3419 } 3420 3421 } 3422 3423 @Override 3424 public Base addChild(String name) throws FHIRException { 3425 if (name.equals("identifier")) { 3426 return addIdentifier(); 3427 } 3428 else if (name.equals("status")) { 3429 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.status"); 3430 } 3431 else if (name.equals("patient")) { 3432 this.patient = new Reference(); 3433 return this.patient; 3434 } 3435 else if (name.equals("encounter")) { 3436 this.encounter = new Reference(); 3437 return this.encounter; 3438 } 3439 else if (name.equals("dateTime")) { 3440 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.dateTime"); 3441 } 3442 else if (name.equals("orderer")) { 3443 this.orderer = new Reference(); 3444 return this.orderer; 3445 } 3446 else if (name.equals("allergyIntolerance")) { 3447 return addAllergyIntolerance(); 3448 } 3449 else if (name.equals("foodPreferenceModifier")) { 3450 return addFoodPreferenceModifier(); 3451 } 3452 else if (name.equals("excludeFoodModifier")) { 3453 return addExcludeFoodModifier(); 3454 } 3455 else if (name.equals("oralDiet")) { 3456 this.oralDiet = new NutritionOrderOralDietComponent(); 3457 return this.oralDiet; 3458 } 3459 else if (name.equals("supplement")) { 3460 return addSupplement(); 3461 } 3462 else if (name.equals("enteralFormula")) { 3463 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); 3464 return this.enteralFormula; 3465 } 3466 else 3467 return super.addChild(name); 3468 } 3469 3470 public String fhirType() { 3471 return "NutritionOrder"; 3472 3473 } 3474 3475 public NutritionOrder copy() { 3476 NutritionOrder dst = new NutritionOrder(); 3477 copyValues(dst); 3478 if (identifier != null) { 3479 dst.identifier = new ArrayList<Identifier>(); 3480 for (Identifier i : identifier) 3481 dst.identifier.add(i.copy()); 3482 }; 3483 dst.status = status == null ? null : status.copy(); 3484 dst.patient = patient == null ? null : patient.copy(); 3485 dst.encounter = encounter == null ? null : encounter.copy(); 3486 dst.dateTime = dateTime == null ? null : dateTime.copy(); 3487 dst.orderer = orderer == null ? null : orderer.copy(); 3488 if (allergyIntolerance != null) { 3489 dst.allergyIntolerance = new ArrayList<Reference>(); 3490 for (Reference i : allergyIntolerance) 3491 dst.allergyIntolerance.add(i.copy()); 3492 }; 3493 if (foodPreferenceModifier != null) { 3494 dst.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3495 for (CodeableConcept i : foodPreferenceModifier) 3496 dst.foodPreferenceModifier.add(i.copy()); 3497 }; 3498 if (excludeFoodModifier != null) { 3499 dst.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3500 for (CodeableConcept i : excludeFoodModifier) 3501 dst.excludeFoodModifier.add(i.copy()); 3502 }; 3503 dst.oralDiet = oralDiet == null ? null : oralDiet.copy(); 3504 if (supplement != null) { 3505 dst.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3506 for (NutritionOrderSupplementComponent i : supplement) 3507 dst.supplement.add(i.copy()); 3508 }; 3509 dst.enteralFormula = enteralFormula == null ? null : enteralFormula.copy(); 3510 return dst; 3511 } 3512 3513 protected NutritionOrder typedCopy() { 3514 return copy(); 3515 } 3516 3517 @Override 3518 public boolean equalsDeep(Base other_) { 3519 if (!super.equalsDeep(other_)) 3520 return false; 3521 if (!(other_ instanceof NutritionOrder)) 3522 return false; 3523 NutritionOrder o = (NutritionOrder) other_; 3524 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) 3525 && compareDeep(encounter, o.encounter, true) && compareDeep(dateTime, o.dateTime, true) && compareDeep(orderer, o.orderer, true) 3526 && compareDeep(allergyIntolerance, o.allergyIntolerance, true) && compareDeep(foodPreferenceModifier, o.foodPreferenceModifier, true) 3527 && compareDeep(excludeFoodModifier, o.excludeFoodModifier, true) && compareDeep(oralDiet, o.oralDiet, true) 3528 && compareDeep(supplement, o.supplement, true) && compareDeep(enteralFormula, o.enteralFormula, true) 3529 ; 3530 } 3531 3532 @Override 3533 public boolean equalsShallow(Base other_) { 3534 if (!super.equalsShallow(other_)) 3535 return false; 3536 if (!(other_ instanceof NutritionOrder)) 3537 return false; 3538 NutritionOrder o = (NutritionOrder) other_; 3539 return compareValues(status, o.status, true) && compareValues(dateTime, o.dateTime, true); 3540 } 3541 3542 public boolean isEmpty() { 3543 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, patient 3544 , encounter, dateTime, orderer, allergyIntolerance, foodPreferenceModifier, excludeFoodModifier 3545 , oralDiet, supplement, enteralFormula); 3546 } 3547 3548 @Override 3549 public ResourceType getResourceType() { 3550 return ResourceType.NutritionOrder; 3551 } 3552 3553 /** 3554 * Search parameter: <b>identifier</b> 3555 * <p> 3556 * Description: <b>Return nutrition orders with this external identifier</b><br> 3557 * Type: <b>token</b><br> 3558 * Path: <b>NutritionOrder.identifier</b><br> 3559 * </p> 3560 */ 3561 @SearchParamDefinition(name="identifier", path="NutritionOrder.identifier", description="Return nutrition orders with this external identifier", type="token" ) 3562 public static final String SP_IDENTIFIER = "identifier"; 3563 /** 3564 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3565 * <p> 3566 * Description: <b>Return nutrition orders with this external identifier</b><br> 3567 * Type: <b>token</b><br> 3568 * Path: <b>NutritionOrder.identifier</b><br> 3569 * </p> 3570 */ 3571 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3572 3573 /** 3574 * Search parameter: <b>datetime</b> 3575 * <p> 3576 * Description: <b>Return nutrition orders requested on this date</b><br> 3577 * Type: <b>date</b><br> 3578 * Path: <b>NutritionOrder.dateTime</b><br> 3579 * </p> 3580 */ 3581 @SearchParamDefinition(name="datetime", path="NutritionOrder.dateTime", description="Return nutrition orders requested on this date", type="date" ) 3582 public static final String SP_DATETIME = "datetime"; 3583 /** 3584 * <b>Fluent Client</b> search parameter constant for <b>datetime</b> 3585 * <p> 3586 * Description: <b>Return nutrition orders requested on this date</b><br> 3587 * Type: <b>date</b><br> 3588 * Path: <b>NutritionOrder.dateTime</b><br> 3589 * </p> 3590 */ 3591 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATETIME = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATETIME); 3592 3593 /** 3594 * Search parameter: <b>provider</b> 3595 * <p> 3596 * Description: <b>The identify of the provider who placed the nutrition order</b><br> 3597 * Type: <b>reference</b><br> 3598 * Path: <b>NutritionOrder.orderer</b><br> 3599 * </p> 3600 */ 3601 @SearchParamDefinition(name="provider", path="NutritionOrder.orderer", description="The identify of the provider who placed the nutrition order", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 3602 public static final String SP_PROVIDER = "provider"; 3603 /** 3604 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 3605 * <p> 3606 * Description: <b>The identify of the provider who placed the nutrition order</b><br> 3607 * Type: <b>reference</b><br> 3608 * Path: <b>NutritionOrder.orderer</b><br> 3609 * </p> 3610 */ 3611 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 3612 3613/** 3614 * Constant for fluent queries to be used to add include statements. Specifies 3615 * the path value of "<b>NutritionOrder:provider</b>". 3616 */ 3617 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("NutritionOrder:provider").toLocked(); 3618 3619 /** 3620 * Search parameter: <b>patient</b> 3621 * <p> 3622 * Description: <b>The identity of the person who requires the diet, formula or nutritional supplement</b><br> 3623 * Type: <b>reference</b><br> 3624 * Path: <b>NutritionOrder.patient</b><br> 3625 * </p> 3626 */ 3627 @SearchParamDefinition(name="patient", path="NutritionOrder.patient", description="The identity of the person who requires the diet, formula or nutritional supplement", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3628 public static final String SP_PATIENT = "patient"; 3629 /** 3630 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3631 * <p> 3632 * Description: <b>The identity of the person who requires the diet, formula or nutritional supplement</b><br> 3633 * Type: <b>reference</b><br> 3634 * Path: <b>NutritionOrder.patient</b><br> 3635 * </p> 3636 */ 3637 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3638 3639/** 3640 * Constant for fluent queries to be used to add include statements. Specifies 3641 * the path value of "<b>NutritionOrder:patient</b>". 3642 */ 3643 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("NutritionOrder:patient").toLocked(); 3644 3645 /** 3646 * Search parameter: <b>supplement</b> 3647 * <p> 3648 * Description: <b>Type of supplement product requested</b><br> 3649 * Type: <b>token</b><br> 3650 * Path: <b>NutritionOrder.supplement.type</b><br> 3651 * </p> 3652 */ 3653 @SearchParamDefinition(name="supplement", path="NutritionOrder.supplement.type", description="Type of supplement product requested", type="token" ) 3654 public static final String SP_SUPPLEMENT = "supplement"; 3655 /** 3656 * <b>Fluent Client</b> search parameter constant for <b>supplement</b> 3657 * <p> 3658 * Description: <b>Type of supplement product requested</b><br> 3659 * Type: <b>token</b><br> 3660 * Path: <b>NutritionOrder.supplement.type</b><br> 3661 * </p> 3662 */ 3663 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUPPLEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUPPLEMENT); 3664 3665 /** 3666 * Search parameter: <b>formula</b> 3667 * <p> 3668 * Description: <b>Type of enteral or infant formula</b><br> 3669 * Type: <b>token</b><br> 3670 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType</b><br> 3671 * </p> 3672 */ 3673 @SearchParamDefinition(name="formula", path="NutritionOrder.enteralFormula.baseFormulaType", description="Type of enteral or infant formula", type="token" ) 3674 public static final String SP_FORMULA = "formula"; 3675 /** 3676 * <b>Fluent Client</b> search parameter constant for <b>formula</b> 3677 * <p> 3678 * Description: <b>Type of enteral or infant formula</b><br> 3679 * Type: <b>token</b><br> 3680 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType</b><br> 3681 * </p> 3682 */ 3683 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMULA = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMULA); 3684 3685 /** 3686 * Search parameter: <b>encounter</b> 3687 * <p> 3688 * Description: <b>Return nutrition orders with this encounter identifier</b><br> 3689 * Type: <b>reference</b><br> 3690 * Path: <b>NutritionOrder.encounter</b><br> 3691 * </p> 3692 */ 3693 @SearchParamDefinition(name="encounter", path="NutritionOrder.encounter", description="Return nutrition orders with this encounter identifier", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 3694 public static final String SP_ENCOUNTER = "encounter"; 3695 /** 3696 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3697 * <p> 3698 * Description: <b>Return nutrition orders with this encounter identifier</b><br> 3699 * Type: <b>reference</b><br> 3700 * Path: <b>NutritionOrder.encounter</b><br> 3701 * </p> 3702 */ 3703 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3704 3705/** 3706 * Constant for fluent queries to be used to add include statements. Specifies 3707 * the path value of "<b>NutritionOrder:encounter</b>". 3708 */ 3709 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("NutritionOrder:encounter").toLocked(); 3710 3711 /** 3712 * Search parameter: <b>oraldiet</b> 3713 * <p> 3714 * Description: <b>Type of diet that can be consumed orally (i.e., take via the mouth).</b><br> 3715 * Type: <b>token</b><br> 3716 * Path: <b>NutritionOrder.oralDiet.type</b><br> 3717 * </p> 3718 */ 3719 @SearchParamDefinition(name="oraldiet", path="NutritionOrder.oralDiet.type", description="Type of diet that can be consumed orally (i.e., take via the mouth).", type="token" ) 3720 public static final String SP_ORALDIET = "oraldiet"; 3721 /** 3722 * <b>Fluent Client</b> search parameter constant for <b>oraldiet</b> 3723 * <p> 3724 * Description: <b>Type of diet that can be consumed orally (i.e., take via the mouth).</b><br> 3725 * Type: <b>token</b><br> 3726 * Path: <b>NutritionOrder.oralDiet.type</b><br> 3727 * </p> 3728 */ 3729 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ORALDIET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ORALDIET); 3730 3731 /** 3732 * Search parameter: <b>status</b> 3733 * <p> 3734 * Description: <b>Status of the nutrition order.</b><br> 3735 * Type: <b>token</b><br> 3736 * Path: <b>NutritionOrder.status</b><br> 3737 * </p> 3738 */ 3739 @SearchParamDefinition(name="status", path="NutritionOrder.status", description="Status of the nutrition order.", type="token" ) 3740 public static final String SP_STATUS = "status"; 3741 /** 3742 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3743 * <p> 3744 * Description: <b>Status of the nutrition order.</b><br> 3745 * Type: <b>token</b><br> 3746 * Path: <b>NutritionOrder.status</b><br> 3747 * </p> 3748 */ 3749 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3750 3751 /** 3752 * Search parameter: <b>additive</b> 3753 * <p> 3754 * Description: <b>Type of module component to add to the feeding</b><br> 3755 * Type: <b>token</b><br> 3756 * Path: <b>NutritionOrder.enteralFormula.additiveType</b><br> 3757 * </p> 3758 */ 3759 @SearchParamDefinition(name="additive", path="NutritionOrder.enteralFormula.additiveType", description="Type of module component to add to the feeding", type="token" ) 3760 public static final String SP_ADDITIVE = "additive"; 3761 /** 3762 * <b>Fluent Client</b> search parameter constant for <b>additive</b> 3763 * <p> 3764 * Description: <b>Type of module component to add to the feeding</b><br> 3765 * Type: <b>token</b><br> 3766 * Path: <b>NutritionOrder.enteralFormula.additiveType</b><br> 3767 * </p> 3768 */ 3769 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDITIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDITIVE); 3770 3771 3772}