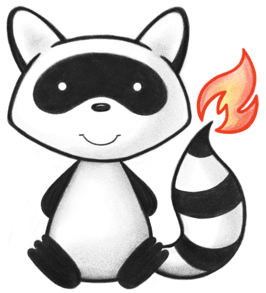
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * Measurements and simple assertions made about a patient, device or other subject. 052 */ 053@ResourceDef(name="Observation", profile="http://hl7.org/fhir/Profile/Observation") 054public class Observation extends DomainResource { 055 056 public enum ObservationStatus { 057 /** 058 * The existence of the observation is registered, but there is no result yet available. 059 */ 060 REGISTERED, 061 /** 062 * This is an initial or interim observation: data may be incomplete or unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * The observation is complete. 067 */ 068 FINAL, 069 /** 070 * Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections. 071 */ 072 AMENDED, 073 /** 074 * Subsequent to being Final, the observation has been modified to correct an error in the test result. 075 */ 076 CORRECTED, 077 /** 078 * The observation is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 079 */ 080 CANCELLED, 081 /** 082 * The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 083 */ 084 ENTEREDINERROR, 085 /** 086 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring system does not know which. 087 */ 088 UNKNOWN, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static ObservationStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("registered".equals(codeString)) 097 return REGISTERED; 098 if ("preliminary".equals(codeString)) 099 return PRELIMINARY; 100 if ("final".equals(codeString)) 101 return FINAL; 102 if ("amended".equals(codeString)) 103 return AMENDED; 104 if ("corrected".equals(codeString)) 105 return CORRECTED; 106 if ("cancelled".equals(codeString)) 107 return CANCELLED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ObservationStatus code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case REGISTERED: return "registered"; 120 case PRELIMINARY: return "preliminary"; 121 case FINAL: return "final"; 122 case AMENDED: return "amended"; 123 case CORRECTED: return "corrected"; 124 case CANCELLED: return "cancelled"; 125 case ENTEREDINERROR: return "entered-in-error"; 126 case UNKNOWN: return "unknown"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case REGISTERED: return "http://hl7.org/fhir/observation-status"; 134 case PRELIMINARY: return "http://hl7.org/fhir/observation-status"; 135 case FINAL: return "http://hl7.org/fhir/observation-status"; 136 case AMENDED: return "http://hl7.org/fhir/observation-status"; 137 case CORRECTED: return "http://hl7.org/fhir/observation-status"; 138 case CANCELLED: return "http://hl7.org/fhir/observation-status"; 139 case ENTEREDINERROR: return "http://hl7.org/fhir/observation-status"; 140 case UNKNOWN: return "http://hl7.org/fhir/observation-status"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case REGISTERED: return "The existence of the observation is registered, but there is no result yet available."; 148 case PRELIMINARY: return "This is an initial or interim observation: data may be incomplete or unverified."; 149 case FINAL: return "The observation is complete."; 150 case AMENDED: return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 151 case CORRECTED: return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 152 case CANCELLED: return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 153 case ENTEREDINERROR: return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 154 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring system does not know which."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case REGISTERED: return "Registered"; 162 case PRELIMINARY: return "Preliminary"; 163 case FINAL: return "Final"; 164 case AMENDED: return "Amended"; 165 case CORRECTED: return "Corrected"; 166 case CANCELLED: return "Cancelled"; 167 case ENTEREDINERROR: return "Entered in Error"; 168 case UNKNOWN: return "Unknown"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class ObservationStatusEnumFactory implements EnumFactory<ObservationStatus> { 176 public ObservationStatus fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("registered".equals(codeString)) 181 return ObservationStatus.REGISTERED; 182 if ("preliminary".equals(codeString)) 183 return ObservationStatus.PRELIMINARY; 184 if ("final".equals(codeString)) 185 return ObservationStatus.FINAL; 186 if ("amended".equals(codeString)) 187 return ObservationStatus.AMENDED; 188 if ("corrected".equals(codeString)) 189 return ObservationStatus.CORRECTED; 190 if ("cancelled".equals(codeString)) 191 return ObservationStatus.CANCELLED; 192 if ("entered-in-error".equals(codeString)) 193 return ObservationStatus.ENTEREDINERROR; 194 if ("unknown".equals(codeString)) 195 return ObservationStatus.UNKNOWN; 196 throw new IllegalArgumentException("Unknown ObservationStatus code '"+codeString+"'"); 197 } 198 public Enumeration<ObservationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<ObservationStatus>(this); 203 String codeString = code.asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return null; 206 if ("registered".equals(codeString)) 207 return new Enumeration<ObservationStatus>(this, ObservationStatus.REGISTERED); 208 if ("preliminary".equals(codeString)) 209 return new Enumeration<ObservationStatus>(this, ObservationStatus.PRELIMINARY); 210 if ("final".equals(codeString)) 211 return new Enumeration<ObservationStatus>(this, ObservationStatus.FINAL); 212 if ("amended".equals(codeString)) 213 return new Enumeration<ObservationStatus>(this, ObservationStatus.AMENDED); 214 if ("corrected".equals(codeString)) 215 return new Enumeration<ObservationStatus>(this, ObservationStatus.CORRECTED); 216 if ("cancelled".equals(codeString)) 217 return new Enumeration<ObservationStatus>(this, ObservationStatus.CANCELLED); 218 if ("entered-in-error".equals(codeString)) 219 return new Enumeration<ObservationStatus>(this, ObservationStatus.ENTEREDINERROR); 220 if ("unknown".equals(codeString)) 221 return new Enumeration<ObservationStatus>(this, ObservationStatus.UNKNOWN); 222 throw new FHIRException("Unknown ObservationStatus code '"+codeString+"'"); 223 } 224 public String toCode(ObservationStatus code) { 225 if (code == ObservationStatus.NULL) 226 return null; 227 if (code == ObservationStatus.REGISTERED) 228 return "registered"; 229 if (code == ObservationStatus.PRELIMINARY) 230 return "preliminary"; 231 if (code == ObservationStatus.FINAL) 232 return "final"; 233 if (code == ObservationStatus.AMENDED) 234 return "amended"; 235 if (code == ObservationStatus.CORRECTED) 236 return "corrected"; 237 if (code == ObservationStatus.CANCELLED) 238 return "cancelled"; 239 if (code == ObservationStatus.ENTEREDINERROR) 240 return "entered-in-error"; 241 if (code == ObservationStatus.UNKNOWN) 242 return "unknown"; 243 return "?"; 244 } 245 public String toSystem(ObservationStatus code) { 246 return code.getSystem(); 247 } 248 } 249 250 public enum ObservationRelationshipType { 251 /** 252 * This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group. 253 */ 254 HASMEMBER, 255 /** 256 * The target resource (Observation or QuestionnaireResponse) is part of the information from which this observation value is derived. (e.g. calculated anion gap, Apgar score) NOTE: "derived-from" is the only logical choice when referencing QuestionnaireResponse. 257 */ 258 DERIVEDFROM, 259 /** 260 * This observation follows the target observation (e.g. timed tests such as Glucose Tolerance Test). 261 */ 262 SEQUELTO, 263 /** 264 * This observation replaces a previous observation (i.e. a revised value). The target observation is now obsolete. 265 */ 266 REPLACES, 267 /** 268 * The value of the target observation qualifies (refines) the semantics of the source observation (e.g. a lipemia measure target from a plasma measure). 269 */ 270 QUALIFIEDBY, 271 /** 272 * The value of the target observation interferes (degrades quality, or prevents valid observation) with the semantics of the source observation (e.g. a hemolysis measure target from a plasma potassium measure, which has no value). 273 */ 274 INTERFEREDBY, 275 /** 276 * added to help the parsers with the generic types 277 */ 278 NULL; 279 public static ObservationRelationshipType fromCode(String codeString) throws FHIRException { 280 if (codeString == null || "".equals(codeString)) 281 return null; 282 if ("has-member".equals(codeString)) 283 return HASMEMBER; 284 if ("derived-from".equals(codeString)) 285 return DERIVEDFROM; 286 if ("sequel-to".equals(codeString)) 287 return SEQUELTO; 288 if ("replaces".equals(codeString)) 289 return REPLACES; 290 if ("qualified-by".equals(codeString)) 291 return QUALIFIEDBY; 292 if ("interfered-by".equals(codeString)) 293 return INTERFEREDBY; 294 if (Configuration.isAcceptInvalidEnums()) 295 return null; 296 else 297 throw new FHIRException("Unknown ObservationRelationshipType code '"+codeString+"'"); 298 } 299 public String toCode() { 300 switch (this) { 301 case HASMEMBER: return "has-member"; 302 case DERIVEDFROM: return "derived-from"; 303 case SEQUELTO: return "sequel-to"; 304 case REPLACES: return "replaces"; 305 case QUALIFIEDBY: return "qualified-by"; 306 case INTERFEREDBY: return "interfered-by"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 public String getSystem() { 312 switch (this) { 313 case HASMEMBER: return "http://hl7.org/fhir/observation-relationshiptypes"; 314 case DERIVEDFROM: return "http://hl7.org/fhir/observation-relationshiptypes"; 315 case SEQUELTO: return "http://hl7.org/fhir/observation-relationshiptypes"; 316 case REPLACES: return "http://hl7.org/fhir/observation-relationshiptypes"; 317 case QUALIFIEDBY: return "http://hl7.org/fhir/observation-relationshiptypes"; 318 case INTERFEREDBY: return "http://hl7.org/fhir/observation-relationshiptypes"; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getDefinition() { 324 switch (this) { 325 case HASMEMBER: return "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group."; 326 case DERIVEDFROM: return "The target resource (Observation or QuestionnaireResponse) is part of the information from which this observation value is derived. (e.g. calculated anion gap, Apgar score) NOTE: \"derived-from\" is the only logical choice when referencing QuestionnaireResponse."; 327 case SEQUELTO: return "This observation follows the target observation (e.g. timed tests such as Glucose Tolerance Test)."; 328 case REPLACES: return "This observation replaces a previous observation (i.e. a revised value). The target observation is now obsolete."; 329 case QUALIFIEDBY: return "The value of the target observation qualifies (refines) the semantics of the source observation (e.g. a lipemia measure target from a plasma measure)."; 330 case INTERFEREDBY: return "The value of the target observation interferes (degrades quality, or prevents valid observation) with the semantics of the source observation (e.g. a hemolysis measure target from a plasma potassium measure, which has no value)."; 331 case NULL: return null; 332 default: return "?"; 333 } 334 } 335 public String getDisplay() { 336 switch (this) { 337 case HASMEMBER: return "Has Member"; 338 case DERIVEDFROM: return "Derived From"; 339 case SEQUELTO: return "Sequel To"; 340 case REPLACES: return "Replaces"; 341 case QUALIFIEDBY: return "Qualified By"; 342 case INTERFEREDBY: return "Interfered By"; 343 case NULL: return null; 344 default: return "?"; 345 } 346 } 347 } 348 349 public static class ObservationRelationshipTypeEnumFactory implements EnumFactory<ObservationRelationshipType> { 350 public ObservationRelationshipType fromCode(String codeString) throws IllegalArgumentException { 351 if (codeString == null || "".equals(codeString)) 352 if (codeString == null || "".equals(codeString)) 353 return null; 354 if ("has-member".equals(codeString)) 355 return ObservationRelationshipType.HASMEMBER; 356 if ("derived-from".equals(codeString)) 357 return ObservationRelationshipType.DERIVEDFROM; 358 if ("sequel-to".equals(codeString)) 359 return ObservationRelationshipType.SEQUELTO; 360 if ("replaces".equals(codeString)) 361 return ObservationRelationshipType.REPLACES; 362 if ("qualified-by".equals(codeString)) 363 return ObservationRelationshipType.QUALIFIEDBY; 364 if ("interfered-by".equals(codeString)) 365 return ObservationRelationshipType.INTERFEREDBY; 366 throw new IllegalArgumentException("Unknown ObservationRelationshipType code '"+codeString+"'"); 367 } 368 public Enumeration<ObservationRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 369 if (code == null) 370 return null; 371 if (code.isEmpty()) 372 return new Enumeration<ObservationRelationshipType>(this); 373 String codeString = code.asStringValue(); 374 if (codeString == null || "".equals(codeString)) 375 return null; 376 if ("has-member".equals(codeString)) 377 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.HASMEMBER); 378 if ("derived-from".equals(codeString)) 379 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.DERIVEDFROM); 380 if ("sequel-to".equals(codeString)) 381 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.SEQUELTO); 382 if ("replaces".equals(codeString)) 383 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.REPLACES); 384 if ("qualified-by".equals(codeString)) 385 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.QUALIFIEDBY); 386 if ("interfered-by".equals(codeString)) 387 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.INTERFEREDBY); 388 throw new FHIRException("Unknown ObservationRelationshipType code '"+codeString+"'"); 389 } 390 public String toCode(ObservationRelationshipType code) { 391 if (code == ObservationRelationshipType.NULL) 392 return null; 393 if (code == ObservationRelationshipType.HASMEMBER) 394 return "has-member"; 395 if (code == ObservationRelationshipType.DERIVEDFROM) 396 return "derived-from"; 397 if (code == ObservationRelationshipType.SEQUELTO) 398 return "sequel-to"; 399 if (code == ObservationRelationshipType.REPLACES) 400 return "replaces"; 401 if (code == ObservationRelationshipType.QUALIFIEDBY) 402 return "qualified-by"; 403 if (code == ObservationRelationshipType.INTERFEREDBY) 404 return "interfered-by"; 405 return "?"; 406 } 407 public String toSystem(ObservationRelationshipType code) { 408 return code.getSystem(); 409 } 410 } 411 412 @Block() 413 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 414 /** 415 * The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3). 416 */ 417 @Child(name = "low", type = {SimpleQuantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 418 @Description(shortDefinition="Low Range, if relevant", formalDefinition="The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3)." ) 419 protected SimpleQuantity low; 420 421 /** 422 * The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3). 423 */ 424 @Child(name = "high", type = {SimpleQuantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 425 @Description(shortDefinition="High Range, if relevant", formalDefinition="The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3)." ) 426 protected SimpleQuantity high; 427 428 /** 429 * Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range. 430 */ 431 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 432 @Description(shortDefinition="Reference range qualifier", formalDefinition="Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range." ) 433 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-meaning") 434 protected CodeableConcept type; 435 436 /** 437 * Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. 438 */ 439 @Child(name = "appliesTo", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 440 @Description(shortDefinition="Reference range population", formalDefinition="Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race." ) 441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referencerange-appliesto") 442 protected List<CodeableConcept> appliesTo; 443 444 /** 445 * The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so. 446 */ 447 @Child(name = "age", type = {Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 448 @Description(shortDefinition="Applicable age range, if relevant", formalDefinition="The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so." ) 449 protected Range age; 450 451 /** 452 * Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of 'normals'. 453 */ 454 @Child(name = "text", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 455 @Description(shortDefinition="Text based reference range in an observation", formalDefinition="Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of 'normals'." ) 456 protected StringType text; 457 458 private static final long serialVersionUID = -955638831L; 459 460 /** 461 * Constructor 462 */ 463 public ObservationReferenceRangeComponent() { 464 super(); 465 } 466 467 /** 468 * @return {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 469 */ 470 public SimpleQuantity getLow() { 471 if (this.low == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 474 else if (Configuration.doAutoCreate()) 475 this.low = new SimpleQuantity(); // cc 476 return this.low; 477 } 478 479 public boolean hasLow() { 480 return this.low != null && !this.low.isEmpty(); 481 } 482 483 /** 484 * @param value {@link #low} (The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).) 485 */ 486 public ObservationReferenceRangeComponent setLow(SimpleQuantity value) { 487 this.low = value; 488 return this; 489 } 490 491 /** 492 * @return {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 493 */ 494 public SimpleQuantity getHigh() { 495 if (this.high == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 498 else if (Configuration.doAutoCreate()) 499 this.high = new SimpleQuantity(); // cc 500 return this.high; 501 } 502 503 public boolean hasHigh() { 504 return this.high != null && !this.high.isEmpty(); 505 } 506 507 /** 508 * @param value {@link #high} (The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).) 509 */ 510 public ObservationReferenceRangeComponent setHigh(SimpleQuantity value) { 511 this.high = value; 512 return this; 513 } 514 515 /** 516 * @return {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 517 */ 518 public CodeableConcept getType() { 519 if (this.type == null) 520 if (Configuration.errorOnAutoCreate()) 521 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.type"); 522 else if (Configuration.doAutoCreate()) 523 this.type = new CodeableConcept(); // cc 524 return this.type; 525 } 526 527 public boolean hasType() { 528 return this.type != null && !this.type.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #type} (Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.) 533 */ 534 public ObservationReferenceRangeComponent setType(CodeableConcept value) { 535 this.type = value; 536 return this; 537 } 538 539 /** 540 * @return {@link #appliesTo} (Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race.) 541 */ 542 public List<CodeableConcept> getAppliesTo() { 543 if (this.appliesTo == null) 544 this.appliesTo = new ArrayList<CodeableConcept>(); 545 return this.appliesTo; 546 } 547 548 /** 549 * @return Returns a reference to <code>this</code> for easy method chaining 550 */ 551 public ObservationReferenceRangeComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 552 this.appliesTo = theAppliesTo; 553 return this; 554 } 555 556 public boolean hasAppliesTo() { 557 if (this.appliesTo == null) 558 return false; 559 for (CodeableConcept item : this.appliesTo) 560 if (!item.isEmpty()) 561 return true; 562 return false; 563 } 564 565 public CodeableConcept addAppliesTo() { //3 566 CodeableConcept t = new CodeableConcept(); 567 if (this.appliesTo == null) 568 this.appliesTo = new ArrayList<CodeableConcept>(); 569 this.appliesTo.add(t); 570 return t; 571 } 572 573 public ObservationReferenceRangeComponent addAppliesTo(CodeableConcept t) { //3 574 if (t == null) 575 return this; 576 if (this.appliesTo == null) 577 this.appliesTo = new ArrayList<CodeableConcept>(); 578 this.appliesTo.add(t); 579 return this; 580 } 581 582 /** 583 * @return The first repetition of repeating field {@link #appliesTo}, creating it if it does not already exist 584 */ 585 public CodeableConcept getAppliesToFirstRep() { 586 if (getAppliesTo().isEmpty()) { 587 addAppliesTo(); 588 } 589 return getAppliesTo().get(0); 590 } 591 592 /** 593 * @return {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 594 */ 595 public Range getAge() { 596 if (this.age == null) 597 if (Configuration.errorOnAutoCreate()) 598 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 599 else if (Configuration.doAutoCreate()) 600 this.age = new Range(); // cc 601 return this.age; 602 } 603 604 public boolean hasAge() { 605 return this.age != null && !this.age.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #age} (The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.) 610 */ 611 public ObservationReferenceRangeComponent setAge(Range value) { 612 this.age = value; 613 return this; 614 } 615 616 /** 617 * @return {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of 'normals'.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 618 */ 619 public StringType getTextElement() { 620 if (this.text == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 623 else if (Configuration.doAutoCreate()) 624 this.text = new StringType(); // bb 625 return this.text; 626 } 627 628 public boolean hasTextElement() { 629 return this.text != null && !this.text.isEmpty(); 630 } 631 632 public boolean hasText() { 633 return this.text != null && !this.text.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #text} (Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of 'normals'.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 638 */ 639 public ObservationReferenceRangeComponent setTextElement(StringType value) { 640 this.text = value; 641 return this; 642 } 643 644 /** 645 * @return Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of 'normals'. 646 */ 647 public String getText() { 648 return this.text == null ? null : this.text.getValue(); 649 } 650 651 /** 652 * @param value Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of "Negative" or a list or table of 'normals'. 653 */ 654 public ObservationReferenceRangeComponent setText(String value) { 655 if (Utilities.noString(value)) 656 this.text = null; 657 else { 658 if (this.text == null) 659 this.text = new StringType(); 660 this.text.setValue(value); 661 } 662 return this; 663 } 664 665 protected void listChildren(List<Property> children) { 666 super.listChildren(children); 667 children.add(new Property("low", "SimpleQuantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low)); 668 children.add(new Property("high", "SimpleQuantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high)); 669 children.add(new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type)); 670 children.add(new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race.", 0, java.lang.Integer.MAX_VALUE, appliesTo)); 671 children.add(new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age)); 672 children.add(new Property("text", "string", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of 'normals'.", 0, 1, text)); 673 } 674 675 @Override 676 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 677 switch (_hash) { 678 case 107348: /*low*/ return new Property("low", "SimpleQuantity", "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 0, 1, low); 679 case 3202466: /*high*/ return new Property("high", "SimpleQuantity", "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 0, 1, high); 680 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 0, 1, type); 681 case -2089924569: /*appliesTo*/ return new Property("appliesTo", "CodeableConcept", "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race.", 0, java.lang.Integer.MAX_VALUE, appliesTo); 682 case 96511: /*age*/ return new Property("age", "Range", "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 0, 1, age); 683 case 3556653: /*text*/ return new Property("text", "string", "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of 'normals'.", 0, 1, text); 684 default: return super.getNamedProperty(_hash, _name, _checkValid); 685 } 686 687 } 688 689 @Override 690 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 691 switch (hash) { 692 case 107348: /*low*/ return this.low == null ? new Base[0] : new Base[] {this.low}; // SimpleQuantity 693 case 3202466: /*high*/ return this.high == null ? new Base[0] : new Base[] {this.high}; // SimpleQuantity 694 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 695 case -2089924569: /*appliesTo*/ return this.appliesTo == null ? new Base[0] : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 696 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Range 697 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 698 default: return super.getProperty(hash, name, checkValid); 699 } 700 701 } 702 703 @Override 704 public Base setProperty(int hash, String name, Base value) throws FHIRException { 705 switch (hash) { 706 case 107348: // low 707 this.low = castToSimpleQuantity(value); // SimpleQuantity 708 return value; 709 case 3202466: // high 710 this.high = castToSimpleQuantity(value); // SimpleQuantity 711 return value; 712 case 3575610: // type 713 this.type = castToCodeableConcept(value); // CodeableConcept 714 return value; 715 case -2089924569: // appliesTo 716 this.getAppliesTo().add(castToCodeableConcept(value)); // CodeableConcept 717 return value; 718 case 96511: // age 719 this.age = castToRange(value); // Range 720 return value; 721 case 3556653: // text 722 this.text = castToString(value); // StringType 723 return value; 724 default: return super.setProperty(hash, name, value); 725 } 726 727 } 728 729 @Override 730 public Base setProperty(String name, Base value) throws FHIRException { 731 if (name.equals("low")) { 732 this.low = castToSimpleQuantity(value); // SimpleQuantity 733 } else if (name.equals("high")) { 734 this.high = castToSimpleQuantity(value); // SimpleQuantity 735 } else if (name.equals("type")) { 736 this.type = castToCodeableConcept(value); // CodeableConcept 737 } else if (name.equals("appliesTo")) { 738 this.getAppliesTo().add(castToCodeableConcept(value)); 739 } else if (name.equals("age")) { 740 this.age = castToRange(value); // Range 741 } else if (name.equals("text")) { 742 this.text = castToString(value); // StringType 743 } else 744 return super.setProperty(name, value); 745 return value; 746 } 747 748 @Override 749 public Base makeProperty(int hash, String name) throws FHIRException { 750 switch (hash) { 751 case 107348: return getLow(); 752 case 3202466: return getHigh(); 753 case 3575610: return getType(); 754 case -2089924569: return addAppliesTo(); 755 case 96511: return getAge(); 756 case 3556653: return getTextElement(); 757 default: return super.makeProperty(hash, name); 758 } 759 760 } 761 762 @Override 763 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 764 switch (hash) { 765 case 107348: /*low*/ return new String[] {"SimpleQuantity"}; 766 case 3202466: /*high*/ return new String[] {"SimpleQuantity"}; 767 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 768 case -2089924569: /*appliesTo*/ return new String[] {"CodeableConcept"}; 769 case 96511: /*age*/ return new String[] {"Range"}; 770 case 3556653: /*text*/ return new String[] {"string"}; 771 default: return super.getTypesForProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public Base addChild(String name) throws FHIRException { 778 if (name.equals("low")) { 779 this.low = new SimpleQuantity(); 780 return this.low; 781 } 782 else if (name.equals("high")) { 783 this.high = new SimpleQuantity(); 784 return this.high; 785 } 786 else if (name.equals("type")) { 787 this.type = new CodeableConcept(); 788 return this.type; 789 } 790 else if (name.equals("appliesTo")) { 791 return addAppliesTo(); 792 } 793 else if (name.equals("age")) { 794 this.age = new Range(); 795 return this.age; 796 } 797 else if (name.equals("text")) { 798 throw new FHIRException("Cannot call addChild on a singleton property Observation.text"); 799 } 800 else 801 return super.addChild(name); 802 } 803 804 public ObservationReferenceRangeComponent copy() { 805 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 806 copyValues(dst); 807 dst.low = low == null ? null : low.copy(); 808 dst.high = high == null ? null : high.copy(); 809 dst.type = type == null ? null : type.copy(); 810 if (appliesTo != null) { 811 dst.appliesTo = new ArrayList<CodeableConcept>(); 812 for (CodeableConcept i : appliesTo) 813 dst.appliesTo.add(i.copy()); 814 }; 815 dst.age = age == null ? null : age.copy(); 816 dst.text = text == null ? null : text.copy(); 817 return dst; 818 } 819 820 @Override 821 public boolean equalsDeep(Base other_) { 822 if (!super.equalsDeep(other_)) 823 return false; 824 if (!(other_ instanceof ObservationReferenceRangeComponent)) 825 return false; 826 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 827 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(type, o.type, true) 828 && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(age, o.age, true) && compareDeep(text, o.text, true) 829 ; 830 } 831 832 @Override 833 public boolean equalsShallow(Base other_) { 834 if (!super.equalsShallow(other_)) 835 return false; 836 if (!(other_ instanceof ObservationReferenceRangeComponent)) 837 return false; 838 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 839 return compareValues(text, o.text, true); 840 } 841 842 public boolean isEmpty() { 843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high, type, appliesTo 844 , age, text); 845 } 846 847 public String fhirType() { 848 return "Observation.referenceRange"; 849 850 } 851 852 } 853 854 @Block() 855 public static class ObservationRelatedComponent extends BackboneElement implements IBaseBackboneElement { 856 /** 857 * A code specifying the kind of relationship that exists with the target resource. 858 */ 859 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 860 @Description(shortDefinition="has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by", formalDefinition="A code specifying the kind of relationship that exists with the target resource." ) 861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-relationshiptypes") 862 protected Enumeration<ObservationRelationshipType> type; 863 864 /** 865 * A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation. 866 */ 867 @Child(name = "target", type = {Observation.class, QuestionnaireResponse.class, Sequence.class}, order=2, min=1, max=1, modifier=false, summary=false) 868 @Description(shortDefinition="Resource that is related to this one", formalDefinition="A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation." ) 869 protected Reference target; 870 871 /** 872 * The actual object that is the target of the reference (A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.) 873 */ 874 protected Resource targetTarget; 875 876 private static final long serialVersionUID = 1541802577L; 877 878 /** 879 * Constructor 880 */ 881 public ObservationRelatedComponent() { 882 super(); 883 } 884 885 /** 886 * Constructor 887 */ 888 public ObservationRelatedComponent(Reference target) { 889 super(); 890 this.target = target; 891 } 892 893 /** 894 * @return {@link #type} (A code specifying the kind of relationship that exists with the target resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 895 */ 896 public Enumeration<ObservationRelationshipType> getTypeElement() { 897 if (this.type == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create ObservationRelatedComponent.type"); 900 else if (Configuration.doAutoCreate()) 901 this.type = new Enumeration<ObservationRelationshipType>(new ObservationRelationshipTypeEnumFactory()); // bb 902 return this.type; 903 } 904 905 public boolean hasTypeElement() { 906 return this.type != null && !this.type.isEmpty(); 907 } 908 909 public boolean hasType() { 910 return this.type != null && !this.type.isEmpty(); 911 } 912 913 /** 914 * @param value {@link #type} (A code specifying the kind of relationship that exists with the target resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 915 */ 916 public ObservationRelatedComponent setTypeElement(Enumeration<ObservationRelationshipType> value) { 917 this.type = value; 918 return this; 919 } 920 921 /** 922 * @return A code specifying the kind of relationship that exists with the target resource. 923 */ 924 public ObservationRelationshipType getType() { 925 return this.type == null ? null : this.type.getValue(); 926 } 927 928 /** 929 * @param value A code specifying the kind of relationship that exists with the target resource. 930 */ 931 public ObservationRelatedComponent setType(ObservationRelationshipType value) { 932 if (value == null) 933 this.type = null; 934 else { 935 if (this.type == null) 936 this.type = new Enumeration<ObservationRelationshipType>(new ObservationRelationshipTypeEnumFactory()); 937 this.type.setValue(value); 938 } 939 return this; 940 } 941 942 /** 943 * @return {@link #target} (A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.) 944 */ 945 public Reference getTarget() { 946 if (this.target == null) 947 if (Configuration.errorOnAutoCreate()) 948 throw new Error("Attempt to auto-create ObservationRelatedComponent.target"); 949 else if (Configuration.doAutoCreate()) 950 this.target = new Reference(); // cc 951 return this.target; 952 } 953 954 public boolean hasTarget() { 955 return this.target != null && !this.target.isEmpty(); 956 } 957 958 /** 959 * @param value {@link #target} (A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.) 960 */ 961 public ObservationRelatedComponent setTarget(Reference value) { 962 this.target = value; 963 return this; 964 } 965 966 /** 967 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.) 968 */ 969 public Resource getTargetTarget() { 970 return this.targetTarget; 971 } 972 973 /** 974 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.) 975 */ 976 public ObservationRelatedComponent setTargetTarget(Resource value) { 977 this.targetTarget = value; 978 return this; 979 } 980 981 protected void listChildren(List<Property> children) { 982 super.listChildren(children); 983 children.add(new Property("type", "code", "A code specifying the kind of relationship that exists with the target resource.", 0, 1, type)); 984 children.add(new Property("target", "Reference(Observation|QuestionnaireResponse|Sequence)", "A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.", 0, 1, target)); 985 } 986 987 @Override 988 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 989 switch (_hash) { 990 case 3575610: /*type*/ return new Property("type", "code", "A code specifying the kind of relationship that exists with the target resource.", 0, 1, type); 991 case -880905839: /*target*/ return new Property("target", "Reference(Observation|QuestionnaireResponse|Sequence)", "A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.", 0, 1, target); 992 default: return super.getNamedProperty(_hash, _name, _checkValid); 993 } 994 995 } 996 997 @Override 998 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 999 switch (hash) { 1000 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ObservationRelationshipType> 1001 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 1002 default: return super.getProperty(hash, name, checkValid); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1009 switch (hash) { 1010 case 3575610: // type 1011 value = new ObservationRelationshipTypeEnumFactory().fromType(castToCode(value)); 1012 this.type = (Enumeration) value; // Enumeration<ObservationRelationshipType> 1013 return value; 1014 case -880905839: // target 1015 this.target = castToReference(value); // Reference 1016 return value; 1017 default: return super.setProperty(hash, name, value); 1018 } 1019 1020 } 1021 1022 @Override 1023 public Base setProperty(String name, Base value) throws FHIRException { 1024 if (name.equals("type")) { 1025 value = new ObservationRelationshipTypeEnumFactory().fromType(castToCode(value)); 1026 this.type = (Enumeration) value; // Enumeration<ObservationRelationshipType> 1027 } else if (name.equals("target")) { 1028 this.target = castToReference(value); // Reference 1029 } else 1030 return super.setProperty(name, value); 1031 return value; 1032 } 1033 1034 @Override 1035 public Base makeProperty(int hash, String name) throws FHIRException { 1036 switch (hash) { 1037 case 3575610: return getTypeElement(); 1038 case -880905839: return getTarget(); 1039 default: return super.makeProperty(hash, name); 1040 } 1041 1042 } 1043 1044 @Override 1045 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1046 switch (hash) { 1047 case 3575610: /*type*/ return new String[] {"code"}; 1048 case -880905839: /*target*/ return new String[] {"Reference"}; 1049 default: return super.getTypesForProperty(hash, name); 1050 } 1051 1052 } 1053 1054 @Override 1055 public Base addChild(String name) throws FHIRException { 1056 if (name.equals("type")) { 1057 throw new FHIRException("Cannot call addChild on a singleton property Observation.type"); 1058 } 1059 else if (name.equals("target")) { 1060 this.target = new Reference(); 1061 return this.target; 1062 } 1063 else 1064 return super.addChild(name); 1065 } 1066 1067 public ObservationRelatedComponent copy() { 1068 ObservationRelatedComponent dst = new ObservationRelatedComponent(); 1069 copyValues(dst); 1070 dst.type = type == null ? null : type.copy(); 1071 dst.target = target == null ? null : target.copy(); 1072 return dst; 1073 } 1074 1075 @Override 1076 public boolean equalsDeep(Base other_) { 1077 if (!super.equalsDeep(other_)) 1078 return false; 1079 if (!(other_ instanceof ObservationRelatedComponent)) 1080 return false; 1081 ObservationRelatedComponent o = (ObservationRelatedComponent) other_; 1082 return compareDeep(type, o.type, true) && compareDeep(target, o.target, true); 1083 } 1084 1085 @Override 1086 public boolean equalsShallow(Base other_) { 1087 if (!super.equalsShallow(other_)) 1088 return false; 1089 if (!(other_ instanceof ObservationRelatedComponent)) 1090 return false; 1091 ObservationRelatedComponent o = (ObservationRelatedComponent) other_; 1092 return compareValues(type, o.type, true); 1093 } 1094 1095 public boolean isEmpty() { 1096 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, target); 1097 } 1098 1099 public String fhirType() { 1100 return "Observation.related"; 1101 1102 } 1103 1104 } 1105 1106 @Block() 1107 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 1108 /** 1109 * Describes what was observed. Sometimes this is called the observation "code". 1110 */ 1111 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1112 @Description(shortDefinition="Type of component observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"code\"." ) 1113 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1114 protected CodeableConcept code; 1115 1116 /** 1117 * The information determined as a result of making the observation, if the information has a simple value. 1118 */ 1119 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, Range.class, Ratio.class, SampledData.class, Attachment.class, TimeType.class, DateTimeType.class, Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1120 @Description(shortDefinition="Actual component result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 1121 protected Type value; 1122 1123 /** 1124 * Provides a reason why the expected value in the element Observation.value[x] is missing. 1125 */ 1126 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1127 @Description(shortDefinition="Why the component result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.value[x] is missing." ) 1128 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-valueabsentreason") 1129 protected CodeableConcept dataAbsentReason; 1130 1131 /** 1132 * The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag. 1133 */ 1134 @Child(name = "interpretation", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1135 @Description(shortDefinition="High, low, normal, etc.", formalDefinition="The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag." ) 1136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1137 protected CodeableConcept interpretation; 1138 1139 /** 1140 * Guidance on how to interpret the value by comparison to a normal or recommended range. 1141 */ 1142 @Child(name = "referenceRange", type = {ObservationReferenceRangeComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1143 @Description(shortDefinition="Provides guide for interpretation of component result", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range." ) 1144 protected List<ObservationReferenceRangeComponent> referenceRange; 1145 1146 private static final long serialVersionUID = -846379911L; 1147 1148 /** 1149 * Constructor 1150 */ 1151 public ObservationComponentComponent() { 1152 super(); 1153 } 1154 1155 /** 1156 * Constructor 1157 */ 1158 public ObservationComponentComponent(CodeableConcept code) { 1159 super(); 1160 this.code = code; 1161 } 1162 1163 /** 1164 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1165 */ 1166 public CodeableConcept getCode() { 1167 if (this.code == null) 1168 if (Configuration.errorOnAutoCreate()) 1169 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 1170 else if (Configuration.doAutoCreate()) 1171 this.code = new CodeableConcept(); // cc 1172 return this.code; 1173 } 1174 1175 public boolean hasCode() { 1176 return this.code != null && !this.code.isEmpty(); 1177 } 1178 1179 /** 1180 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "code".) 1181 */ 1182 public ObservationComponentComponent setCode(CodeableConcept value) { 1183 this.code = value; 1184 return this; 1185 } 1186 1187 /** 1188 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1189 */ 1190 public Type getValue() { 1191 return this.value; 1192 } 1193 1194 /** 1195 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1196 */ 1197 public Quantity getValueQuantity() throws FHIRException { 1198 if (this.value == null) 1199 return null; 1200 if (!(this.value instanceof Quantity)) 1201 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1202 return (Quantity) this.value; 1203 } 1204 1205 public boolean hasValueQuantity() { 1206 return this.value instanceof Quantity; 1207 } 1208 1209 /** 1210 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1211 */ 1212 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1213 if (this.value == null) 1214 return null; 1215 if (!(this.value instanceof CodeableConcept)) 1216 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1217 return (CodeableConcept) this.value; 1218 } 1219 1220 public boolean hasValueCodeableConcept() { 1221 return this.value instanceof CodeableConcept; 1222 } 1223 1224 /** 1225 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1226 */ 1227 public StringType getValueStringType() throws FHIRException { 1228 if (this.value == null) 1229 return null; 1230 if (!(this.value instanceof StringType)) 1231 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1232 return (StringType) this.value; 1233 } 1234 1235 public boolean hasValueStringType() { 1236 return this.value instanceof StringType; 1237 } 1238 1239 /** 1240 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1241 */ 1242 public Range getValueRange() throws FHIRException { 1243 if (this.value == null) 1244 return null; 1245 if (!(this.value instanceof Range)) 1246 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1247 return (Range) this.value; 1248 } 1249 1250 public boolean hasValueRange() { 1251 return this.value instanceof Range; 1252 } 1253 1254 /** 1255 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1256 */ 1257 public Ratio getValueRatio() throws FHIRException { 1258 if (this.value == null) 1259 return null; 1260 if (!(this.value instanceof Ratio)) 1261 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1262 return (Ratio) this.value; 1263 } 1264 1265 public boolean hasValueRatio() { 1266 return this.value instanceof Ratio; 1267 } 1268 1269 /** 1270 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1271 */ 1272 public SampledData getValueSampledData() throws FHIRException { 1273 if (this.value == null) 1274 return null; 1275 if (!(this.value instanceof SampledData)) 1276 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 1277 return (SampledData) this.value; 1278 } 1279 1280 public boolean hasValueSampledData() { 1281 return this.value instanceof SampledData; 1282 } 1283 1284 /** 1285 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1286 */ 1287 public Attachment getValueAttachment() throws FHIRException { 1288 if (this.value == null) 1289 return null; 1290 if (!(this.value instanceof Attachment)) 1291 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1292 return (Attachment) this.value; 1293 } 1294 1295 public boolean hasValueAttachment() { 1296 return this.value instanceof Attachment; 1297 } 1298 1299 /** 1300 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1301 */ 1302 public TimeType getValueTimeType() throws FHIRException { 1303 if (this.value == null) 1304 return null; 1305 if (!(this.value instanceof TimeType)) 1306 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1307 return (TimeType) this.value; 1308 } 1309 1310 public boolean hasValueTimeType() { 1311 return this.value instanceof TimeType; 1312 } 1313 1314 /** 1315 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1316 */ 1317 public DateTimeType getValueDateTimeType() throws FHIRException { 1318 if (this.value == null) 1319 return null; 1320 if (!(this.value instanceof DateTimeType)) 1321 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1322 return (DateTimeType) this.value; 1323 } 1324 1325 public boolean hasValueDateTimeType() { 1326 return this.value instanceof DateTimeType; 1327 } 1328 1329 /** 1330 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1331 */ 1332 public Period getValuePeriod() throws FHIRException { 1333 if (this.value == null) 1334 return null; 1335 if (!(this.value instanceof Period)) 1336 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1337 return (Period) this.value; 1338 } 1339 1340 public boolean hasValuePeriod() { 1341 return this.value instanceof Period; 1342 } 1343 1344 public boolean hasValue() { 1345 return this.value != null && !this.value.isEmpty(); 1346 } 1347 1348 /** 1349 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 1350 */ 1351 public ObservationComponentComponent setValue(Type value) throws FHIRFormatError { 1352 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof Attachment || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period)) 1353 throw new FHIRFormatError("Not the right type for Observation.component.value[x]: "+value.fhirType()); 1354 this.value = value; 1355 return this; 1356 } 1357 1358 /** 1359 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 1360 */ 1361 public CodeableConcept getDataAbsentReason() { 1362 if (this.dataAbsentReason == null) 1363 if (Configuration.errorOnAutoCreate()) 1364 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1365 else if (Configuration.doAutoCreate()) 1366 this.dataAbsentReason = new CodeableConcept(); // cc 1367 return this.dataAbsentReason; 1368 } 1369 1370 public boolean hasDataAbsentReason() { 1371 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1372 } 1373 1374 /** 1375 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 1376 */ 1377 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1378 this.dataAbsentReason = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #interpretation} (The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.) 1384 */ 1385 public CodeableConcept getInterpretation() { 1386 if (this.interpretation == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create ObservationComponentComponent.interpretation"); 1389 else if (Configuration.doAutoCreate()) 1390 this.interpretation = new CodeableConcept(); // cc 1391 return this.interpretation; 1392 } 1393 1394 public boolean hasInterpretation() { 1395 return this.interpretation != null && !this.interpretation.isEmpty(); 1396 } 1397 1398 /** 1399 * @param value {@link #interpretation} (The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.) 1400 */ 1401 public ObservationComponentComponent setInterpretation(CodeableConcept value) { 1402 this.interpretation = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range.) 1408 */ 1409 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1410 if (this.referenceRange == null) 1411 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1412 return this.referenceRange; 1413 } 1414 1415 /** 1416 * @return Returns a reference to <code>this</code> for easy method chaining 1417 */ 1418 public ObservationComponentComponent setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 1419 this.referenceRange = theReferenceRange; 1420 return this; 1421 } 1422 1423 public boolean hasReferenceRange() { 1424 if (this.referenceRange == null) 1425 return false; 1426 for (ObservationReferenceRangeComponent item : this.referenceRange) 1427 if (!item.isEmpty()) 1428 return true; 1429 return false; 1430 } 1431 1432 public ObservationReferenceRangeComponent addReferenceRange() { //3 1433 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1434 if (this.referenceRange == null) 1435 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1436 this.referenceRange.add(t); 1437 return t; 1438 } 1439 1440 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { //3 1441 if (t == null) 1442 return this; 1443 if (this.referenceRange == null) 1444 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1445 this.referenceRange.add(t); 1446 return this; 1447 } 1448 1449 /** 1450 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist 1451 */ 1452 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 1453 if (getReferenceRange().isEmpty()) { 1454 addReferenceRange(); 1455 } 1456 return getReferenceRange().get(0); 1457 } 1458 1459 protected void listChildren(List<Property> children) { 1460 super.listChildren(children); 1461 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code)); 1462 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 1463 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason)); 1464 children.add(new Property("interpretation", "CodeableConcept", "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.", 0, 1, interpretation)); 1465 children.add(new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 1466 } 1467 1468 @Override 1469 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1470 switch (_hash) { 1471 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code); 1472 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1473 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1474 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1475 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1476 case -1424603934: /*valueString*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1477 case 2030761548: /*valueRange*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1478 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1479 case -962229101: /*valueSampledData*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1480 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1481 case -765708322: /*valueTime*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1482 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1483 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 1484 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason); 1485 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.", 0, 1, interpretation); 1486 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "@Observation.referenceRange", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 1487 default: return super.getNamedProperty(_hash, _name, _checkValid); 1488 } 1489 1490 } 1491 1492 @Override 1493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1494 switch (hash) { 1495 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1496 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1497 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 1498 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : new Base[] {this.interpretation}; // CodeableConcept 1499 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 1500 default: return super.getProperty(hash, name, checkValid); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1507 switch (hash) { 1508 case 3059181: // code 1509 this.code = castToCodeableConcept(value); // CodeableConcept 1510 return value; 1511 case 111972721: // value 1512 this.value = castToType(value); // Type 1513 return value; 1514 case 1034315687: // dataAbsentReason 1515 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1516 return value; 1517 case -297950712: // interpretation 1518 this.interpretation = castToCodeableConcept(value); // CodeableConcept 1519 return value; 1520 case -1912545102: // referenceRange 1521 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 1522 return value; 1523 default: return super.setProperty(hash, name, value); 1524 } 1525 1526 } 1527 1528 @Override 1529 public Base setProperty(String name, Base value) throws FHIRException { 1530 if (name.equals("code")) { 1531 this.code = castToCodeableConcept(value); // CodeableConcept 1532 } else if (name.equals("value[x]")) { 1533 this.value = castToType(value); // Type 1534 } else if (name.equals("dataAbsentReason")) { 1535 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1536 } else if (name.equals("interpretation")) { 1537 this.interpretation = castToCodeableConcept(value); // CodeableConcept 1538 } else if (name.equals("referenceRange")) { 1539 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1540 } else 1541 return super.setProperty(name, value); 1542 return value; 1543 } 1544 1545 @Override 1546 public Base makeProperty(int hash, String name) throws FHIRException { 1547 switch (hash) { 1548 case 3059181: return getCode(); 1549 case -1410166417: return getValue(); 1550 case 111972721: return getValue(); 1551 case 1034315687: return getDataAbsentReason(); 1552 case -297950712: return getInterpretation(); 1553 case -1912545102: return addReferenceRange(); 1554 default: return super.makeProperty(hash, name); 1555 } 1556 1557 } 1558 1559 @Override 1560 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1561 switch (hash) { 1562 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1563 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "Range", "Ratio", "SampledData", "Attachment", "time", "dateTime", "Period"}; 1564 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 1565 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 1566 case -1912545102: /*referenceRange*/ return new String[] {"@Observation.referenceRange"}; 1567 default: return super.getTypesForProperty(hash, name); 1568 } 1569 1570 } 1571 1572 @Override 1573 public Base addChild(String name) throws FHIRException { 1574 if (name.equals("code")) { 1575 this.code = new CodeableConcept(); 1576 return this.code; 1577 } 1578 else if (name.equals("valueQuantity")) { 1579 this.value = new Quantity(); 1580 return this.value; 1581 } 1582 else if (name.equals("valueCodeableConcept")) { 1583 this.value = new CodeableConcept(); 1584 return this.value; 1585 } 1586 else if (name.equals("valueString")) { 1587 this.value = new StringType(); 1588 return this.value; 1589 } 1590 else if (name.equals("valueRange")) { 1591 this.value = new Range(); 1592 return this.value; 1593 } 1594 else if (name.equals("valueRatio")) { 1595 this.value = new Ratio(); 1596 return this.value; 1597 } 1598 else if (name.equals("valueSampledData")) { 1599 this.value = new SampledData(); 1600 return this.value; 1601 } 1602 else if (name.equals("valueAttachment")) { 1603 this.value = new Attachment(); 1604 return this.value; 1605 } 1606 else if (name.equals("valueTime")) { 1607 this.value = new TimeType(); 1608 return this.value; 1609 } 1610 else if (name.equals("valueDateTime")) { 1611 this.value = new DateTimeType(); 1612 return this.value; 1613 } 1614 else if (name.equals("valuePeriod")) { 1615 this.value = new Period(); 1616 return this.value; 1617 } 1618 else if (name.equals("dataAbsentReason")) { 1619 this.dataAbsentReason = new CodeableConcept(); 1620 return this.dataAbsentReason; 1621 } 1622 else if (name.equals("interpretation")) { 1623 this.interpretation = new CodeableConcept(); 1624 return this.interpretation; 1625 } 1626 else if (name.equals("referenceRange")) { 1627 return addReferenceRange(); 1628 } 1629 else 1630 return super.addChild(name); 1631 } 1632 1633 public ObservationComponentComponent copy() { 1634 ObservationComponentComponent dst = new ObservationComponentComponent(); 1635 copyValues(dst); 1636 dst.code = code == null ? null : code.copy(); 1637 dst.value = value == null ? null : value.copy(); 1638 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1639 dst.interpretation = interpretation == null ? null : interpretation.copy(); 1640 if (referenceRange != null) { 1641 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1642 for (ObservationReferenceRangeComponent i : referenceRange) 1643 dst.referenceRange.add(i.copy()); 1644 }; 1645 return dst; 1646 } 1647 1648 @Override 1649 public boolean equalsDeep(Base other_) { 1650 if (!super.equalsDeep(other_)) 1651 return false; 1652 if (!(other_ instanceof ObservationComponentComponent)) 1653 return false; 1654 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1655 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1656 && compareDeep(interpretation, o.interpretation, true) && compareDeep(referenceRange, o.referenceRange, true) 1657 ; 1658 } 1659 1660 @Override 1661 public boolean equalsShallow(Base other_) { 1662 if (!super.equalsShallow(other_)) 1663 return false; 1664 if (!(other_ instanceof ObservationComponentComponent)) 1665 return false; 1666 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1667 return true; 1668 } 1669 1670 public boolean isEmpty() { 1671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, dataAbsentReason 1672 , interpretation, referenceRange); 1673 } 1674 1675 public String fhirType() { 1676 return "Observation.component"; 1677 1678 } 1679 1680 } 1681 1682 /** 1683 * A unique identifier assigned to this observation. 1684 */ 1685 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1686 @Description(shortDefinition="Business Identifier for observation", formalDefinition="A unique identifier assigned to this observation." ) 1687 protected List<Identifier> identifier; 1688 1689 /** 1690 * A plan, proposal or order that is fulfilled in whole or in part by this event. 1691 */ 1692 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1693 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 1694 protected List<Reference> basedOn; 1695 /** 1696 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this event.) 1697 */ 1698 protected List<Resource> basedOnTarget; 1699 1700 1701 /** 1702 * The status of the result value. 1703 */ 1704 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1705 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="The status of the result value." ) 1706 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 1707 protected Enumeration<ObservationStatus> status; 1708 1709 /** 1710 * A code that classifies the general type of observation being made. 1711 */ 1712 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1713 @Description(shortDefinition="Classification of type of observation", formalDefinition="A code that classifies the general type of observation being made." ) 1714 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 1715 protected List<CodeableConcept> category; 1716 1717 /** 1718 * Describes what was observed. Sometimes this is called the observation "name". 1719 */ 1720 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 1721 @Description(shortDefinition="Type of observation (code / type)", formalDefinition="Describes what was observed. Sometimes this is called the observation \"name\"." ) 1722 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1723 protected CodeableConcept code; 1724 1725 /** 1726 * The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject. 1727 */ 1728 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class}, order=5, min=0, max=1, modifier=false, summary=true) 1729 @Description(shortDefinition="Who and/or what this is about", formalDefinition="The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject." ) 1730 protected Reference subject; 1731 1732 /** 1733 * The actual object that is the target of the reference (The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.) 1734 */ 1735 protected Resource subjectTarget; 1736 1737 /** 1738 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made. 1739 */ 1740 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=false) 1741 @Description(shortDefinition="Healthcare event during which this observation is made", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made." ) 1742 protected Reference context; 1743 1744 /** 1745 * The actual object that is the target of the reference (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 1746 */ 1747 protected Resource contextTarget; 1748 1749 /** 1750 * The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself. 1751 */ 1752 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 1753 @Description(shortDefinition="Clinically relevant time/time-period for observation", formalDefinition="The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself." ) 1754 protected Type effective; 1755 1756 /** 1757 * The date and time this observation was made available to providers, typically after the results have been reviewed and verified. 1758 */ 1759 @Child(name = "issued", type = {InstantType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1760 @Description(shortDefinition="Date/Time this was made available", formalDefinition="The date and time this observation was made available to providers, typically after the results have been reviewed and verified." ) 1761 protected InstantType issued; 1762 1763 /** 1764 * Who was responsible for asserting the observed value as "true". 1765 */ 1766 @Child(name = "performer", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1767 @Description(shortDefinition="Who is responsible for the observation", formalDefinition="Who was responsible for asserting the observed value as \"true\"." ) 1768 protected List<Reference> performer; 1769 /** 1770 * The actual objects that are the target of the reference (Who was responsible for asserting the observed value as "true".) 1771 */ 1772 protected List<Resource> performerTarget; 1773 1774 1775 /** 1776 * The information determined as a result of making the observation, if the information has a simple value. 1777 */ 1778 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, Range.class, Ratio.class, SampledData.class, Attachment.class, TimeType.class, DateTimeType.class, Period.class}, order=10, min=0, max=1, modifier=false, summary=true) 1779 @Description(shortDefinition="Actual result", formalDefinition="The information determined as a result of making the observation, if the information has a simple value." ) 1780 protected Type value; 1781 1782 /** 1783 * Provides a reason why the expected value in the element Observation.value[x] is missing. 1784 */ 1785 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 1786 @Description(shortDefinition="Why the result is missing", formalDefinition="Provides a reason why the expected value in the element Observation.value[x] is missing." ) 1787 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-valueabsentreason") 1788 protected CodeableConcept dataAbsentReason; 1789 1790 /** 1791 * The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag. 1792 */ 1793 @Child(name = "interpretation", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 1794 @Description(shortDefinition="High, low, normal, etc.", formalDefinition="The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag." ) 1795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-interpretation") 1796 protected CodeableConcept interpretation; 1797 1798 /** 1799 * May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result. 1800 */ 1801 @Child(name = "comment", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 1802 @Description(shortDefinition="Comments about result", formalDefinition="May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result." ) 1803 protected StringType comment; 1804 1805 /** 1806 * Indicates the site on the subject's body where the observation was made (i.e. the target site). 1807 */ 1808 @Child(name = "bodySite", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 1809 @Description(shortDefinition="Observed body part", formalDefinition="Indicates the site on the subject's body where the observation was made (i.e. the target site)." ) 1810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1811 protected CodeableConcept bodySite; 1812 1813 /** 1814 * Indicates the mechanism used to perform the observation. 1815 */ 1816 @Child(name = "method", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 1817 @Description(shortDefinition="How it was done", formalDefinition="Indicates the mechanism used to perform the observation." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-methods") 1819 protected CodeableConcept method; 1820 1821 /** 1822 * The specimen that was used when this observation was made. 1823 */ 1824 @Child(name = "specimen", type = {Specimen.class}, order=16, min=0, max=1, modifier=false, summary=false) 1825 @Description(shortDefinition="Specimen used for this observation", formalDefinition="The specimen that was used when this observation was made." ) 1826 protected Reference specimen; 1827 1828 /** 1829 * The actual object that is the target of the reference (The specimen that was used when this observation was made.) 1830 */ 1831 protected Specimen specimenTarget; 1832 1833 /** 1834 * The device used to generate the observation data. 1835 */ 1836 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=17, min=0, max=1, modifier=false, summary=false) 1837 @Description(shortDefinition="(Measurement) Device", formalDefinition="The device used to generate the observation data." ) 1838 protected Reference device; 1839 1840 /** 1841 * The actual object that is the target of the reference (The device used to generate the observation data.) 1842 */ 1843 protected Resource deviceTarget; 1844 1845 /** 1846 * Guidance on how to interpret the value by comparison to a normal or recommended range. 1847 */ 1848 @Child(name = "referenceRange", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1849 @Description(shortDefinition="Provides guide for interpretation", formalDefinition="Guidance on how to interpret the value by comparison to a normal or recommended range." ) 1850 protected List<ObservationReferenceRangeComponent> referenceRange; 1851 1852 /** 1853 * A reference to another resource (usually another Observation) whose relationship is defined by the relationship type code. 1854 */ 1855 @Child(name = "related", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1856 @Description(shortDefinition="Resource related to this observation", formalDefinition="A reference to another resource (usually another Observation) whose relationship is defined by the relationship type code." ) 1857 protected List<ObservationRelatedComponent> related; 1858 1859 /** 1860 * Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations. 1861 */ 1862 @Child(name = "component", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1863 @Description(shortDefinition="Component results", formalDefinition="Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations." ) 1864 protected List<ObservationComponentComponent> component; 1865 1866 private static final long serialVersionUID = -1771290322L; 1867 1868 /** 1869 * Constructor 1870 */ 1871 public Observation() { 1872 super(); 1873 } 1874 1875 /** 1876 * Constructor 1877 */ 1878 public Observation(Enumeration<ObservationStatus> status, CodeableConcept code) { 1879 super(); 1880 this.status = status; 1881 this.code = code; 1882 } 1883 1884 /** 1885 * @return {@link #identifier} (A unique identifier assigned to this observation.) 1886 */ 1887 public List<Identifier> getIdentifier() { 1888 if (this.identifier == null) 1889 this.identifier = new ArrayList<Identifier>(); 1890 return this.identifier; 1891 } 1892 1893 /** 1894 * @return Returns a reference to <code>this</code> for easy method chaining 1895 */ 1896 public Observation setIdentifier(List<Identifier> theIdentifier) { 1897 this.identifier = theIdentifier; 1898 return this; 1899 } 1900 1901 public boolean hasIdentifier() { 1902 if (this.identifier == null) 1903 return false; 1904 for (Identifier item : this.identifier) 1905 if (!item.isEmpty()) 1906 return true; 1907 return false; 1908 } 1909 1910 public Identifier addIdentifier() { //3 1911 Identifier t = new Identifier(); 1912 if (this.identifier == null) 1913 this.identifier = new ArrayList<Identifier>(); 1914 this.identifier.add(t); 1915 return t; 1916 } 1917 1918 public Observation addIdentifier(Identifier t) { //3 1919 if (t == null) 1920 return this; 1921 if (this.identifier == null) 1922 this.identifier = new ArrayList<Identifier>(); 1923 this.identifier.add(t); 1924 return this; 1925 } 1926 1927 /** 1928 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1929 */ 1930 public Identifier getIdentifierFirstRep() { 1931 if (getIdentifier().isEmpty()) { 1932 addIdentifier(); 1933 } 1934 return getIdentifier().get(0); 1935 } 1936 1937 /** 1938 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 1939 */ 1940 public List<Reference> getBasedOn() { 1941 if (this.basedOn == null) 1942 this.basedOn = new ArrayList<Reference>(); 1943 return this.basedOn; 1944 } 1945 1946 /** 1947 * @return Returns a reference to <code>this</code> for easy method chaining 1948 */ 1949 public Observation setBasedOn(List<Reference> theBasedOn) { 1950 this.basedOn = theBasedOn; 1951 return this; 1952 } 1953 1954 public boolean hasBasedOn() { 1955 if (this.basedOn == null) 1956 return false; 1957 for (Reference item : this.basedOn) 1958 if (!item.isEmpty()) 1959 return true; 1960 return false; 1961 } 1962 1963 public Reference addBasedOn() { //3 1964 Reference t = new Reference(); 1965 if (this.basedOn == null) 1966 this.basedOn = new ArrayList<Reference>(); 1967 this.basedOn.add(t); 1968 return t; 1969 } 1970 1971 public Observation addBasedOn(Reference t) { //3 1972 if (t == null) 1973 return this; 1974 if (this.basedOn == null) 1975 this.basedOn = new ArrayList<Reference>(); 1976 this.basedOn.add(t); 1977 return this; 1978 } 1979 1980 /** 1981 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1982 */ 1983 public Reference getBasedOnFirstRep() { 1984 if (getBasedOn().isEmpty()) { 1985 addBasedOn(); 1986 } 1987 return getBasedOn().get(0); 1988 } 1989 1990 /** 1991 * @deprecated Use Reference#setResource(IBaseResource) instead 1992 */ 1993 @Deprecated 1994 public List<Resource> getBasedOnTarget() { 1995 if (this.basedOnTarget == null) 1996 this.basedOnTarget = new ArrayList<Resource>(); 1997 return this.basedOnTarget; 1998 } 1999 2000 /** 2001 * @return {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2002 */ 2003 public Enumeration<ObservationStatus> getStatusElement() { 2004 if (this.status == null) 2005 if (Configuration.errorOnAutoCreate()) 2006 throw new Error("Attempt to auto-create Observation.status"); 2007 else if (Configuration.doAutoCreate()) 2008 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 2009 return this.status; 2010 } 2011 2012 public boolean hasStatusElement() { 2013 return this.status != null && !this.status.isEmpty(); 2014 } 2015 2016 public boolean hasStatus() { 2017 return this.status != null && !this.status.isEmpty(); 2018 } 2019 2020 /** 2021 * @param value {@link #status} (The status of the result value.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2022 */ 2023 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 2024 this.status = value; 2025 return this; 2026 } 2027 2028 /** 2029 * @return The status of the result value. 2030 */ 2031 public ObservationStatus getStatus() { 2032 return this.status == null ? null : this.status.getValue(); 2033 } 2034 2035 /** 2036 * @param value The status of the result value. 2037 */ 2038 public Observation setStatus(ObservationStatus value) { 2039 if (this.status == null) 2040 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 2041 this.status.setValue(value); 2042 return this; 2043 } 2044 2045 /** 2046 * @return {@link #category} (A code that classifies the general type of observation being made.) 2047 */ 2048 public List<CodeableConcept> getCategory() { 2049 if (this.category == null) 2050 this.category = new ArrayList<CodeableConcept>(); 2051 return this.category; 2052 } 2053 2054 /** 2055 * @return Returns a reference to <code>this</code> for easy method chaining 2056 */ 2057 public Observation setCategory(List<CodeableConcept> theCategory) { 2058 this.category = theCategory; 2059 return this; 2060 } 2061 2062 public boolean hasCategory() { 2063 if (this.category == null) 2064 return false; 2065 for (CodeableConcept item : this.category) 2066 if (!item.isEmpty()) 2067 return true; 2068 return false; 2069 } 2070 2071 public CodeableConcept addCategory() { //3 2072 CodeableConcept t = new CodeableConcept(); 2073 if (this.category == null) 2074 this.category = new ArrayList<CodeableConcept>(); 2075 this.category.add(t); 2076 return t; 2077 } 2078 2079 public Observation addCategory(CodeableConcept t) { //3 2080 if (t == null) 2081 return this; 2082 if (this.category == null) 2083 this.category = new ArrayList<CodeableConcept>(); 2084 this.category.add(t); 2085 return this; 2086 } 2087 2088 /** 2089 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 2090 */ 2091 public CodeableConcept getCategoryFirstRep() { 2092 if (getCategory().isEmpty()) { 2093 addCategory(); 2094 } 2095 return getCategory().get(0); 2096 } 2097 2098 /** 2099 * @return {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2100 */ 2101 public CodeableConcept getCode() { 2102 if (this.code == null) 2103 if (Configuration.errorOnAutoCreate()) 2104 throw new Error("Attempt to auto-create Observation.code"); 2105 else if (Configuration.doAutoCreate()) 2106 this.code = new CodeableConcept(); // cc 2107 return this.code; 2108 } 2109 2110 public boolean hasCode() { 2111 return this.code != null && !this.code.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #code} (Describes what was observed. Sometimes this is called the observation "name".) 2116 */ 2117 public Observation setCode(CodeableConcept value) { 2118 this.code = value; 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #subject} (The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.) 2124 */ 2125 public Reference getSubject() { 2126 if (this.subject == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create Observation.subject"); 2129 else if (Configuration.doAutoCreate()) 2130 this.subject = new Reference(); // cc 2131 return this.subject; 2132 } 2133 2134 public boolean hasSubject() { 2135 return this.subject != null && !this.subject.isEmpty(); 2136 } 2137 2138 /** 2139 * @param value {@link #subject} (The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.) 2140 */ 2141 public Observation setSubject(Reference value) { 2142 this.subject = value; 2143 return this; 2144 } 2145 2146 /** 2147 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.) 2148 */ 2149 public Resource getSubjectTarget() { 2150 return this.subjectTarget; 2151 } 2152 2153 /** 2154 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.) 2155 */ 2156 public Observation setSubjectTarget(Resource value) { 2157 this.subjectTarget = value; 2158 return this; 2159 } 2160 2161 /** 2162 * @return {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2163 */ 2164 public Reference getContext() { 2165 if (this.context == null) 2166 if (Configuration.errorOnAutoCreate()) 2167 throw new Error("Attempt to auto-create Observation.context"); 2168 else if (Configuration.doAutoCreate()) 2169 this.context = new Reference(); // cc 2170 return this.context; 2171 } 2172 2173 public boolean hasContext() { 2174 return this.context != null && !this.context.isEmpty(); 2175 } 2176 2177 /** 2178 * @param value {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2179 */ 2180 public Observation setContext(Reference value) { 2181 this.context = value; 2182 return this; 2183 } 2184 2185 /** 2186 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2187 */ 2188 public Resource getContextTarget() { 2189 return this.contextTarget; 2190 } 2191 2192 /** 2193 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.) 2194 */ 2195 public Observation setContextTarget(Resource value) { 2196 this.contextTarget = value; 2197 return this; 2198 } 2199 2200 /** 2201 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2202 */ 2203 public Type getEffective() { 2204 return this.effective; 2205 } 2206 2207 /** 2208 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2209 */ 2210 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 2211 if (this.effective == null) 2212 return null; 2213 if (!(this.effective instanceof DateTimeType)) 2214 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 2215 return (DateTimeType) this.effective; 2216 } 2217 2218 public boolean hasEffectiveDateTimeType() { 2219 return this.effective instanceof DateTimeType; 2220 } 2221 2222 /** 2223 * @return {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2224 */ 2225 public Period getEffectivePeriod() throws FHIRException { 2226 if (this.effective == null) 2227 return null; 2228 if (!(this.effective instanceof Period)) 2229 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 2230 return (Period) this.effective; 2231 } 2232 2233 public boolean hasEffectivePeriod() { 2234 return this.effective instanceof Period; 2235 } 2236 2237 public boolean hasEffective() { 2238 return this.effective != null && !this.effective.isEmpty(); 2239 } 2240 2241 /** 2242 * @param value {@link #effective} (The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the "physiologically relevant time". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.) 2243 */ 2244 public Observation setEffective(Type value) throws FHIRFormatError { 2245 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 2246 throw new FHIRFormatError("Not the right type for Observation.effective[x]: "+value.fhirType()); 2247 this.effective = value; 2248 return this; 2249 } 2250 2251 /** 2252 * @return {@link #issued} (The date and time this observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2253 */ 2254 public InstantType getIssuedElement() { 2255 if (this.issued == null) 2256 if (Configuration.errorOnAutoCreate()) 2257 throw new Error("Attempt to auto-create Observation.issued"); 2258 else if (Configuration.doAutoCreate()) 2259 this.issued = new InstantType(); // bb 2260 return this.issued; 2261 } 2262 2263 public boolean hasIssuedElement() { 2264 return this.issued != null && !this.issued.isEmpty(); 2265 } 2266 2267 public boolean hasIssued() { 2268 return this.issued != null && !this.issued.isEmpty(); 2269 } 2270 2271 /** 2272 * @param value {@link #issued} (The date and time this observation was made available to providers, typically after the results have been reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2273 */ 2274 public Observation setIssuedElement(InstantType value) { 2275 this.issued = value; 2276 return this; 2277 } 2278 2279 /** 2280 * @return The date and time this observation was made available to providers, typically after the results have been reviewed and verified. 2281 */ 2282 public Date getIssued() { 2283 return this.issued == null ? null : this.issued.getValue(); 2284 } 2285 2286 /** 2287 * @param value The date and time this observation was made available to providers, typically after the results have been reviewed and verified. 2288 */ 2289 public Observation setIssued(Date value) { 2290 if (value == null) 2291 this.issued = null; 2292 else { 2293 if (this.issued == null) 2294 this.issued = new InstantType(); 2295 this.issued.setValue(value); 2296 } 2297 return this; 2298 } 2299 2300 /** 2301 * @return {@link #performer} (Who was responsible for asserting the observed value as "true".) 2302 */ 2303 public List<Reference> getPerformer() { 2304 if (this.performer == null) 2305 this.performer = new ArrayList<Reference>(); 2306 return this.performer; 2307 } 2308 2309 /** 2310 * @return Returns a reference to <code>this</code> for easy method chaining 2311 */ 2312 public Observation setPerformer(List<Reference> thePerformer) { 2313 this.performer = thePerformer; 2314 return this; 2315 } 2316 2317 public boolean hasPerformer() { 2318 if (this.performer == null) 2319 return false; 2320 for (Reference item : this.performer) 2321 if (!item.isEmpty()) 2322 return true; 2323 return false; 2324 } 2325 2326 public Reference addPerformer() { //3 2327 Reference t = new Reference(); 2328 if (this.performer == null) 2329 this.performer = new ArrayList<Reference>(); 2330 this.performer.add(t); 2331 return t; 2332 } 2333 2334 public Observation addPerformer(Reference t) { //3 2335 if (t == null) 2336 return this; 2337 if (this.performer == null) 2338 this.performer = new ArrayList<Reference>(); 2339 this.performer.add(t); 2340 return this; 2341 } 2342 2343 /** 2344 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 2345 */ 2346 public Reference getPerformerFirstRep() { 2347 if (getPerformer().isEmpty()) { 2348 addPerformer(); 2349 } 2350 return getPerformer().get(0); 2351 } 2352 2353 /** 2354 * @deprecated Use Reference#setResource(IBaseResource) instead 2355 */ 2356 @Deprecated 2357 public List<Resource> getPerformerTarget() { 2358 if (this.performerTarget == null) 2359 this.performerTarget = new ArrayList<Resource>(); 2360 return this.performerTarget; 2361 } 2362 2363 /** 2364 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2365 */ 2366 public Type getValue() { 2367 return this.value; 2368 } 2369 2370 /** 2371 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2372 */ 2373 public Quantity getValueQuantity() throws FHIRException { 2374 if (this.value == null) 2375 return null; 2376 if (!(this.value instanceof Quantity)) 2377 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2378 return (Quantity) this.value; 2379 } 2380 2381 public boolean hasValueQuantity() { 2382 return this.value instanceof Quantity; 2383 } 2384 2385 /** 2386 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2387 */ 2388 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2389 if (this.value == null) 2390 return null; 2391 if (!(this.value instanceof CodeableConcept)) 2392 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2393 return (CodeableConcept) this.value; 2394 } 2395 2396 public boolean hasValueCodeableConcept() { 2397 return this.value instanceof CodeableConcept; 2398 } 2399 2400 /** 2401 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2402 */ 2403 public StringType getValueStringType() throws FHIRException { 2404 if (this.value == null) 2405 return null; 2406 if (!(this.value instanceof StringType)) 2407 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2408 return (StringType) this.value; 2409 } 2410 2411 public boolean hasValueStringType() { 2412 return this.value instanceof StringType; 2413 } 2414 2415 /** 2416 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2417 */ 2418 public BooleanType getValueBooleanType() throws FHIRException { 2419 if (this.value == null) 2420 return null; 2421 if (!(this.value instanceof BooleanType)) 2422 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2423 return (BooleanType) this.value; 2424 } 2425 2426 public boolean hasValueBooleanType() { 2427 return this.value instanceof BooleanType; 2428 } 2429 2430 /** 2431 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2432 */ 2433 public Range getValueRange() throws FHIRException { 2434 if (this.value == null) 2435 return null; 2436 if (!(this.value instanceof Range)) 2437 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2438 return (Range) this.value; 2439 } 2440 2441 public boolean hasValueRange() { 2442 return this.value instanceof Range; 2443 } 2444 2445 /** 2446 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2447 */ 2448 public Ratio getValueRatio() throws FHIRException { 2449 if (this.value == null) 2450 return null; 2451 if (!(this.value instanceof Ratio)) 2452 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2453 return (Ratio) this.value; 2454 } 2455 2456 public boolean hasValueRatio() { 2457 return this.value instanceof Ratio; 2458 } 2459 2460 /** 2461 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2462 */ 2463 public SampledData getValueSampledData() throws FHIRException { 2464 if (this.value == null) 2465 return null; 2466 if (!(this.value instanceof SampledData)) 2467 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 2468 return (SampledData) this.value; 2469 } 2470 2471 public boolean hasValueSampledData() { 2472 return this.value instanceof SampledData; 2473 } 2474 2475 /** 2476 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2477 */ 2478 public Attachment getValueAttachment() throws FHIRException { 2479 if (this.value == null) 2480 return null; 2481 if (!(this.value instanceof Attachment)) 2482 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2483 return (Attachment) this.value; 2484 } 2485 2486 public boolean hasValueAttachment() { 2487 return this.value instanceof Attachment; 2488 } 2489 2490 /** 2491 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2492 */ 2493 public TimeType getValueTimeType() throws FHIRException { 2494 if (this.value == null) 2495 return null; 2496 if (!(this.value instanceof TimeType)) 2497 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2498 return (TimeType) this.value; 2499 } 2500 2501 public boolean hasValueTimeType() { 2502 return this.value instanceof TimeType; 2503 } 2504 2505 /** 2506 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2507 */ 2508 public DateTimeType getValueDateTimeType() throws FHIRException { 2509 if (this.value == null) 2510 return null; 2511 if (!(this.value instanceof DateTimeType)) 2512 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2513 return (DateTimeType) this.value; 2514 } 2515 2516 public boolean hasValueDateTimeType() { 2517 return this.value instanceof DateTimeType; 2518 } 2519 2520 /** 2521 * @return {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2522 */ 2523 public Period getValuePeriod() throws FHIRException { 2524 if (this.value == null) 2525 return null; 2526 if (!(this.value instanceof Period)) 2527 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2528 return (Period) this.value; 2529 } 2530 2531 public boolean hasValuePeriod() { 2532 return this.value instanceof Period; 2533 } 2534 2535 public boolean hasValue() { 2536 return this.value != null && !this.value.isEmpty(); 2537 } 2538 2539 /** 2540 * @param value {@link #value} (The information determined as a result of making the observation, if the information has a simple value.) 2541 */ 2542 public Observation setValue(Type value) throws FHIRFormatError { 2543 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof Range || value instanceof Ratio || value instanceof SampledData || value instanceof Attachment || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period)) 2544 throw new FHIRFormatError("Not the right type for Observation.value[x]: "+value.fhirType()); 2545 this.value = value; 2546 return this; 2547 } 2548 2549 /** 2550 * @return {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2551 */ 2552 public CodeableConcept getDataAbsentReason() { 2553 if (this.dataAbsentReason == null) 2554 if (Configuration.errorOnAutoCreate()) 2555 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2556 else if (Configuration.doAutoCreate()) 2557 this.dataAbsentReason = new CodeableConcept(); // cc 2558 return this.dataAbsentReason; 2559 } 2560 2561 public boolean hasDataAbsentReason() { 2562 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2563 } 2564 2565 /** 2566 * @param value {@link #dataAbsentReason} (Provides a reason why the expected value in the element Observation.value[x] is missing.) 2567 */ 2568 public Observation setDataAbsentReason(CodeableConcept value) { 2569 this.dataAbsentReason = value; 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #interpretation} (The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.) 2575 */ 2576 public CodeableConcept getInterpretation() { 2577 if (this.interpretation == null) 2578 if (Configuration.errorOnAutoCreate()) 2579 throw new Error("Attempt to auto-create Observation.interpretation"); 2580 else if (Configuration.doAutoCreate()) 2581 this.interpretation = new CodeableConcept(); // cc 2582 return this.interpretation; 2583 } 2584 2585 public boolean hasInterpretation() { 2586 return this.interpretation != null && !this.interpretation.isEmpty(); 2587 } 2588 2589 /** 2590 * @param value {@link #interpretation} (The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.) 2591 */ 2592 public Observation setInterpretation(CodeableConcept value) { 2593 this.interpretation = value; 2594 return this; 2595 } 2596 2597 /** 2598 * @return {@link #comment} (May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2599 */ 2600 public StringType getCommentElement() { 2601 if (this.comment == null) 2602 if (Configuration.errorOnAutoCreate()) 2603 throw new Error("Attempt to auto-create Observation.comment"); 2604 else if (Configuration.doAutoCreate()) 2605 this.comment = new StringType(); // bb 2606 return this.comment; 2607 } 2608 2609 public boolean hasCommentElement() { 2610 return this.comment != null && !this.comment.isEmpty(); 2611 } 2612 2613 public boolean hasComment() { 2614 return this.comment != null && !this.comment.isEmpty(); 2615 } 2616 2617 /** 2618 * @param value {@link #comment} (May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2619 */ 2620 public Observation setCommentElement(StringType value) { 2621 this.comment = value; 2622 return this; 2623 } 2624 2625 /** 2626 * @return May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result. 2627 */ 2628 public String getComment() { 2629 return this.comment == null ? null : this.comment.getValue(); 2630 } 2631 2632 /** 2633 * @param value May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result. 2634 */ 2635 public Observation setComment(String value) { 2636 if (Utilities.noString(value)) 2637 this.comment = null; 2638 else { 2639 if (this.comment == null) 2640 this.comment = new StringType(); 2641 this.comment.setValue(value); 2642 } 2643 return this; 2644 } 2645 2646 /** 2647 * @return {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2648 */ 2649 public CodeableConcept getBodySite() { 2650 if (this.bodySite == null) 2651 if (Configuration.errorOnAutoCreate()) 2652 throw new Error("Attempt to auto-create Observation.bodySite"); 2653 else if (Configuration.doAutoCreate()) 2654 this.bodySite = new CodeableConcept(); // cc 2655 return this.bodySite; 2656 } 2657 2658 public boolean hasBodySite() { 2659 return this.bodySite != null && !this.bodySite.isEmpty(); 2660 } 2661 2662 /** 2663 * @param value {@link #bodySite} (Indicates the site on the subject's body where the observation was made (i.e. the target site).) 2664 */ 2665 public Observation setBodySite(CodeableConcept value) { 2666 this.bodySite = value; 2667 return this; 2668 } 2669 2670 /** 2671 * @return {@link #method} (Indicates the mechanism used to perform the observation.) 2672 */ 2673 public CodeableConcept getMethod() { 2674 if (this.method == null) 2675 if (Configuration.errorOnAutoCreate()) 2676 throw new Error("Attempt to auto-create Observation.method"); 2677 else if (Configuration.doAutoCreate()) 2678 this.method = new CodeableConcept(); // cc 2679 return this.method; 2680 } 2681 2682 public boolean hasMethod() { 2683 return this.method != null && !this.method.isEmpty(); 2684 } 2685 2686 /** 2687 * @param value {@link #method} (Indicates the mechanism used to perform the observation.) 2688 */ 2689 public Observation setMethod(CodeableConcept value) { 2690 this.method = value; 2691 return this; 2692 } 2693 2694 /** 2695 * @return {@link #specimen} (The specimen that was used when this observation was made.) 2696 */ 2697 public Reference getSpecimen() { 2698 if (this.specimen == null) 2699 if (Configuration.errorOnAutoCreate()) 2700 throw new Error("Attempt to auto-create Observation.specimen"); 2701 else if (Configuration.doAutoCreate()) 2702 this.specimen = new Reference(); // cc 2703 return this.specimen; 2704 } 2705 2706 public boolean hasSpecimen() { 2707 return this.specimen != null && !this.specimen.isEmpty(); 2708 } 2709 2710 /** 2711 * @param value {@link #specimen} (The specimen that was used when this observation was made.) 2712 */ 2713 public Observation setSpecimen(Reference value) { 2714 this.specimen = value; 2715 return this; 2716 } 2717 2718 /** 2719 * @return {@link #specimen} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The specimen that was used when this observation was made.) 2720 */ 2721 public Specimen getSpecimenTarget() { 2722 if (this.specimenTarget == null) 2723 if (Configuration.errorOnAutoCreate()) 2724 throw new Error("Attempt to auto-create Observation.specimen"); 2725 else if (Configuration.doAutoCreate()) 2726 this.specimenTarget = new Specimen(); // aa 2727 return this.specimenTarget; 2728 } 2729 2730 /** 2731 * @param value {@link #specimen} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The specimen that was used when this observation was made.) 2732 */ 2733 public Observation setSpecimenTarget(Specimen value) { 2734 this.specimenTarget = value; 2735 return this; 2736 } 2737 2738 /** 2739 * @return {@link #device} (The device used to generate the observation data.) 2740 */ 2741 public Reference getDevice() { 2742 if (this.device == null) 2743 if (Configuration.errorOnAutoCreate()) 2744 throw new Error("Attempt to auto-create Observation.device"); 2745 else if (Configuration.doAutoCreate()) 2746 this.device = new Reference(); // cc 2747 return this.device; 2748 } 2749 2750 public boolean hasDevice() { 2751 return this.device != null && !this.device.isEmpty(); 2752 } 2753 2754 /** 2755 * @param value {@link #device} (The device used to generate the observation data.) 2756 */ 2757 public Observation setDevice(Reference value) { 2758 this.device = value; 2759 return this; 2760 } 2761 2762 /** 2763 * @return {@link #device} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device used to generate the observation data.) 2764 */ 2765 public Resource getDeviceTarget() { 2766 return this.deviceTarget; 2767 } 2768 2769 /** 2770 * @param value {@link #device} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device used to generate the observation data.) 2771 */ 2772 public Observation setDeviceTarget(Resource value) { 2773 this.deviceTarget = value; 2774 return this; 2775 } 2776 2777 /** 2778 * @return {@link #referenceRange} (Guidance on how to interpret the value by comparison to a normal or recommended range.) 2779 */ 2780 public List<ObservationReferenceRangeComponent> getReferenceRange() { 2781 if (this.referenceRange == null) 2782 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2783 return this.referenceRange; 2784 } 2785 2786 /** 2787 * @return Returns a reference to <code>this</code> for easy method chaining 2788 */ 2789 public Observation setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 2790 this.referenceRange = theReferenceRange; 2791 return this; 2792 } 2793 2794 public boolean hasReferenceRange() { 2795 if (this.referenceRange == null) 2796 return false; 2797 for (ObservationReferenceRangeComponent item : this.referenceRange) 2798 if (!item.isEmpty()) 2799 return true; 2800 return false; 2801 } 2802 2803 public ObservationReferenceRangeComponent addReferenceRange() { //3 2804 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 2805 if (this.referenceRange == null) 2806 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2807 this.referenceRange.add(t); 2808 return t; 2809 } 2810 2811 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { //3 2812 if (t == null) 2813 return this; 2814 if (this.referenceRange == null) 2815 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2816 this.referenceRange.add(t); 2817 return this; 2818 } 2819 2820 /** 2821 * @return The first repetition of repeating field {@link #referenceRange}, creating it if it does not already exist 2822 */ 2823 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 2824 if (getReferenceRange().isEmpty()) { 2825 addReferenceRange(); 2826 } 2827 return getReferenceRange().get(0); 2828 } 2829 2830 /** 2831 * @return {@link #related} (A reference to another resource (usually another Observation) whose relationship is defined by the relationship type code.) 2832 */ 2833 public List<ObservationRelatedComponent> getRelated() { 2834 if (this.related == null) 2835 this.related = new ArrayList<ObservationRelatedComponent>(); 2836 return this.related; 2837 } 2838 2839 /** 2840 * @return Returns a reference to <code>this</code> for easy method chaining 2841 */ 2842 public Observation setRelated(List<ObservationRelatedComponent> theRelated) { 2843 this.related = theRelated; 2844 return this; 2845 } 2846 2847 public boolean hasRelated() { 2848 if (this.related == null) 2849 return false; 2850 for (ObservationRelatedComponent item : this.related) 2851 if (!item.isEmpty()) 2852 return true; 2853 return false; 2854 } 2855 2856 public ObservationRelatedComponent addRelated() { //3 2857 ObservationRelatedComponent t = new ObservationRelatedComponent(); 2858 if (this.related == null) 2859 this.related = new ArrayList<ObservationRelatedComponent>(); 2860 this.related.add(t); 2861 return t; 2862 } 2863 2864 public Observation addRelated(ObservationRelatedComponent t) { //3 2865 if (t == null) 2866 return this; 2867 if (this.related == null) 2868 this.related = new ArrayList<ObservationRelatedComponent>(); 2869 this.related.add(t); 2870 return this; 2871 } 2872 2873 /** 2874 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 2875 */ 2876 public ObservationRelatedComponent getRelatedFirstRep() { 2877 if (getRelated().isEmpty()) { 2878 addRelated(); 2879 } 2880 return getRelated().get(0); 2881 } 2882 2883 /** 2884 * @return {@link #component} (Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.) 2885 */ 2886 public List<ObservationComponentComponent> getComponent() { 2887 if (this.component == null) 2888 this.component = new ArrayList<ObservationComponentComponent>(); 2889 return this.component; 2890 } 2891 2892 /** 2893 * @return Returns a reference to <code>this</code> for easy method chaining 2894 */ 2895 public Observation setComponent(List<ObservationComponentComponent> theComponent) { 2896 this.component = theComponent; 2897 return this; 2898 } 2899 2900 public boolean hasComponent() { 2901 if (this.component == null) 2902 return false; 2903 for (ObservationComponentComponent item : this.component) 2904 if (!item.isEmpty()) 2905 return true; 2906 return false; 2907 } 2908 2909 public ObservationComponentComponent addComponent() { //3 2910 ObservationComponentComponent t = new ObservationComponentComponent(); 2911 if (this.component == null) 2912 this.component = new ArrayList<ObservationComponentComponent>(); 2913 this.component.add(t); 2914 return t; 2915 } 2916 2917 public Observation addComponent(ObservationComponentComponent t) { //3 2918 if (t == null) 2919 return this; 2920 if (this.component == null) 2921 this.component = new ArrayList<ObservationComponentComponent>(); 2922 this.component.add(t); 2923 return this; 2924 } 2925 2926 /** 2927 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist 2928 */ 2929 public ObservationComponentComponent getComponentFirstRep() { 2930 if (getComponent().isEmpty()) { 2931 addComponent(); 2932 } 2933 return getComponent().get(0); 2934 } 2935 2936 protected void listChildren(List<Property> children) { 2937 super.listChildren(children); 2938 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2939 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2940 children.add(new Property("status", "code", "The status of the result value.", 0, 1, status)); 2941 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category)); 2942 children.add(new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 2943 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", "The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.", 0, 1, subject)); 2944 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, context)); 2945 children.add(new Property("effective[x]", "dateTime|Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 2946 children.add(new Property("issued", "instant", "The date and time this observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued)); 2947 children.add(new Property("performer", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer)); 2948 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value)); 2949 children.add(new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason)); 2950 children.add(new Property("interpretation", "CodeableConcept", "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.", 0, 1, interpretation)); 2951 children.add(new Property("comment", "string", "May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.", 0, 1, comment)); 2952 children.add(new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite)); 2953 children.add(new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method)); 2954 children.add(new Property("specimen", "Reference(Specimen)", "The specimen that was used when this observation was made.", 0, 1, specimen)); 2955 children.add(new Property("device", "Reference(Device|DeviceMetric)", "The device used to generate the observation data.", 0, 1, device)); 2956 children.add(new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange)); 2957 children.add(new Property("related", "", "A reference to another resource (usually another Observation) whose relationship is defined by the relationship type code.", 0, java.lang.Integer.MAX_VALUE, related)); 2958 children.add(new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component)); 2959 } 2960 2961 @Override 2962 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2963 switch (_hash) { 2964 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier); 2965 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2966 case -892481550: /*status*/ return new Property("status", "code", "The status of the result value.", 0, 1, status); 2967 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, category); 2968 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code); 2969 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location)", "The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.", 0, 1, subject); 2970 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 0, 1, context); 2971 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 2972 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 2973 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 2974 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 2975 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time this observation was made available to providers, typically after the results have been reviewed and verified.", 0, 1, issued); 2976 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, performer); 2977 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2978 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2979 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2980 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2981 case -1424603934: /*valueString*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2982 case 733421943: /*valueBoolean*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2983 case 2030761548: /*valueRange*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2984 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2985 case -962229101: /*valueSampledData*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2986 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2987 case -765708322: /*valueTime*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2988 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2989 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|Range|Ratio|SampledData|Attachment|time|dateTime|Period", "The information determined as a result of making the observation, if the information has a simple value.", 0, 1, value); 2990 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, dataAbsentReason); 2991 case -297950712: /*interpretation*/ return new Property("interpretation", "CodeableConcept", "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.", 0, 1, interpretation); 2992 case 950398559: /*comment*/ return new Property("comment", "string", "May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.", 0, 1, comment); 2993 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, bodySite); 2994 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 0, 1, method); 2995 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "The specimen that was used when this observation was made.", 0, 1, specimen); 2996 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "The device used to generate the observation data.", 0, 1, device); 2997 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "", "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, java.lang.Integer.MAX_VALUE, referenceRange); 2998 case 1090493483: /*related*/ return new Property("related", "", "A reference to another resource (usually another Observation) whose relationship is defined by the relationship type code.", 0, java.lang.Integer.MAX_VALUE, related); 2999 case -1399907075: /*component*/ return new Property("component", "", "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 0, java.lang.Integer.MAX_VALUE, component); 3000 default: return super.getNamedProperty(_hash, _name, _checkValid); 3001 } 3002 3003 } 3004 3005 @Override 3006 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3007 switch (hash) { 3008 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3009 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3010 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ObservationStatus> 3011 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3012 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3013 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3014 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 3015 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 3016 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 3017 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 3018 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 3019 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 3020 case -297950712: /*interpretation*/ return this.interpretation == null ? new Base[0] : new Base[] {this.interpretation}; // CodeableConcept 3021 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 3022 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3023 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 3024 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 3025 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 3026 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 3027 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // ObservationRelatedComponent 3028 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ObservationComponentComponent 3029 default: return super.getProperty(hash, name, checkValid); 3030 } 3031 3032 } 3033 3034 @Override 3035 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3036 switch (hash) { 3037 case -1618432855: // identifier 3038 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3039 return value; 3040 case -332612366: // basedOn 3041 this.getBasedOn().add(castToReference(value)); // Reference 3042 return value; 3043 case -892481550: // status 3044 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3045 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3046 return value; 3047 case 50511102: // category 3048 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3049 return value; 3050 case 3059181: // code 3051 this.code = castToCodeableConcept(value); // CodeableConcept 3052 return value; 3053 case -1867885268: // subject 3054 this.subject = castToReference(value); // Reference 3055 return value; 3056 case 951530927: // context 3057 this.context = castToReference(value); // Reference 3058 return value; 3059 case -1468651097: // effective 3060 this.effective = castToType(value); // Type 3061 return value; 3062 case -1179159893: // issued 3063 this.issued = castToInstant(value); // InstantType 3064 return value; 3065 case 481140686: // performer 3066 this.getPerformer().add(castToReference(value)); // Reference 3067 return value; 3068 case 111972721: // value 3069 this.value = castToType(value); // Type 3070 return value; 3071 case 1034315687: // dataAbsentReason 3072 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3073 return value; 3074 case -297950712: // interpretation 3075 this.interpretation = castToCodeableConcept(value); // CodeableConcept 3076 return value; 3077 case 950398559: // comment 3078 this.comment = castToString(value); // StringType 3079 return value; 3080 case 1702620169: // bodySite 3081 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3082 return value; 3083 case -1077554975: // method 3084 this.method = castToCodeableConcept(value); // CodeableConcept 3085 return value; 3086 case -2132868344: // specimen 3087 this.specimen = castToReference(value); // Reference 3088 return value; 3089 case -1335157162: // device 3090 this.device = castToReference(value); // Reference 3091 return value; 3092 case -1912545102: // referenceRange 3093 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 3094 return value; 3095 case 1090493483: // related 3096 this.getRelated().add((ObservationRelatedComponent) value); // ObservationRelatedComponent 3097 return value; 3098 case -1399907075: // component 3099 this.getComponent().add((ObservationComponentComponent) value); // ObservationComponentComponent 3100 return value; 3101 default: return super.setProperty(hash, name, value); 3102 } 3103 3104 } 3105 3106 @Override 3107 public Base setProperty(String name, Base value) throws FHIRException { 3108 if (name.equals("identifier")) { 3109 this.getIdentifier().add(castToIdentifier(value)); 3110 } else if (name.equals("basedOn")) { 3111 this.getBasedOn().add(castToReference(value)); 3112 } else if (name.equals("status")) { 3113 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3114 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3115 } else if (name.equals("category")) { 3116 this.getCategory().add(castToCodeableConcept(value)); 3117 } else if (name.equals("code")) { 3118 this.code = castToCodeableConcept(value); // CodeableConcept 3119 } else if (name.equals("subject")) { 3120 this.subject = castToReference(value); // Reference 3121 } else if (name.equals("context")) { 3122 this.context = castToReference(value); // Reference 3123 } else if (name.equals("effective[x]")) { 3124 this.effective = castToType(value); // Type 3125 } else if (name.equals("issued")) { 3126 this.issued = castToInstant(value); // InstantType 3127 } else if (name.equals("performer")) { 3128 this.getPerformer().add(castToReference(value)); 3129 } else if (name.equals("value[x]")) { 3130 this.value = castToType(value); // Type 3131 } else if (name.equals("dataAbsentReason")) { 3132 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3133 } else if (name.equals("interpretation")) { 3134 this.interpretation = castToCodeableConcept(value); // CodeableConcept 3135 } else if (name.equals("comment")) { 3136 this.comment = castToString(value); // StringType 3137 } else if (name.equals("bodySite")) { 3138 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3139 } else if (name.equals("method")) { 3140 this.method = castToCodeableConcept(value); // CodeableConcept 3141 } else if (name.equals("specimen")) { 3142 this.specimen = castToReference(value); // Reference 3143 } else if (name.equals("device")) { 3144 this.device = castToReference(value); // Reference 3145 } else if (name.equals("referenceRange")) { 3146 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 3147 } else if (name.equals("related")) { 3148 this.getRelated().add((ObservationRelatedComponent) value); 3149 } else if (name.equals("component")) { 3150 this.getComponent().add((ObservationComponentComponent) value); 3151 } else 3152 return super.setProperty(name, value); 3153 return value; 3154 } 3155 3156 @Override 3157 public Base makeProperty(int hash, String name) throws FHIRException { 3158 switch (hash) { 3159 case -1618432855: return addIdentifier(); 3160 case -332612366: return addBasedOn(); 3161 case -892481550: return getStatusElement(); 3162 case 50511102: return addCategory(); 3163 case 3059181: return getCode(); 3164 case -1867885268: return getSubject(); 3165 case 951530927: return getContext(); 3166 case 247104889: return getEffective(); 3167 case -1468651097: return getEffective(); 3168 case -1179159893: return getIssuedElement(); 3169 case 481140686: return addPerformer(); 3170 case -1410166417: return getValue(); 3171 case 111972721: return getValue(); 3172 case 1034315687: return getDataAbsentReason(); 3173 case -297950712: return getInterpretation(); 3174 case 950398559: return getCommentElement(); 3175 case 1702620169: return getBodySite(); 3176 case -1077554975: return getMethod(); 3177 case -2132868344: return getSpecimen(); 3178 case -1335157162: return getDevice(); 3179 case -1912545102: return addReferenceRange(); 3180 case 1090493483: return addRelated(); 3181 case -1399907075: return addComponent(); 3182 default: return super.makeProperty(hash, name); 3183 } 3184 3185 } 3186 3187 @Override 3188 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3189 switch (hash) { 3190 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3191 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3192 case -892481550: /*status*/ return new String[] {"code"}; 3193 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3194 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3195 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3196 case 951530927: /*context*/ return new String[] {"Reference"}; 3197 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 3198 case -1179159893: /*issued*/ return new String[] {"instant"}; 3199 case 481140686: /*performer*/ return new String[] {"Reference"}; 3200 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "Range", "Ratio", "SampledData", "Attachment", "time", "dateTime", "Period"}; 3201 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 3202 case -297950712: /*interpretation*/ return new String[] {"CodeableConcept"}; 3203 case 950398559: /*comment*/ return new String[] {"string"}; 3204 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3205 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 3206 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 3207 case -1335157162: /*device*/ return new String[] {"Reference"}; 3208 case -1912545102: /*referenceRange*/ return new String[] {}; 3209 case 1090493483: /*related*/ return new String[] {}; 3210 case -1399907075: /*component*/ return new String[] {}; 3211 default: return super.getTypesForProperty(hash, name); 3212 } 3213 3214 } 3215 3216 @Override 3217 public Base addChild(String name) throws FHIRException { 3218 if (name.equals("identifier")) { 3219 return addIdentifier(); 3220 } 3221 else if (name.equals("basedOn")) { 3222 return addBasedOn(); 3223 } 3224 else if (name.equals("status")) { 3225 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 3226 } 3227 else if (name.equals("category")) { 3228 return addCategory(); 3229 } 3230 else if (name.equals("code")) { 3231 this.code = new CodeableConcept(); 3232 return this.code; 3233 } 3234 else if (name.equals("subject")) { 3235 this.subject = new Reference(); 3236 return this.subject; 3237 } 3238 else if (name.equals("context")) { 3239 this.context = new Reference(); 3240 return this.context; 3241 } 3242 else if (name.equals("effectiveDateTime")) { 3243 this.effective = new DateTimeType(); 3244 return this.effective; 3245 } 3246 else if (name.equals("effectivePeriod")) { 3247 this.effective = new Period(); 3248 return this.effective; 3249 } 3250 else if (name.equals("issued")) { 3251 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 3252 } 3253 else if (name.equals("performer")) { 3254 return addPerformer(); 3255 } 3256 else if (name.equals("valueQuantity")) { 3257 this.value = new Quantity(); 3258 return this.value; 3259 } 3260 else if (name.equals("valueCodeableConcept")) { 3261 this.value = new CodeableConcept(); 3262 return this.value; 3263 } 3264 else if (name.equals("valueString")) { 3265 this.value = new StringType(); 3266 return this.value; 3267 } 3268 else if (name.equals("valueBoolean")) { 3269 this.value = new BooleanType(); 3270 return this.value; 3271 } 3272 else if (name.equals("valueRange")) { 3273 this.value = new Range(); 3274 return this.value; 3275 } 3276 else if (name.equals("valueRatio")) { 3277 this.value = new Ratio(); 3278 return this.value; 3279 } 3280 else if (name.equals("valueSampledData")) { 3281 this.value = new SampledData(); 3282 return this.value; 3283 } 3284 else if (name.equals("valueAttachment")) { 3285 this.value = new Attachment(); 3286 return this.value; 3287 } 3288 else if (name.equals("valueTime")) { 3289 this.value = new TimeType(); 3290 return this.value; 3291 } 3292 else if (name.equals("valueDateTime")) { 3293 this.value = new DateTimeType(); 3294 return this.value; 3295 } 3296 else if (name.equals("valuePeriod")) { 3297 this.value = new Period(); 3298 return this.value; 3299 } 3300 else if (name.equals("dataAbsentReason")) { 3301 this.dataAbsentReason = new CodeableConcept(); 3302 return this.dataAbsentReason; 3303 } 3304 else if (name.equals("interpretation")) { 3305 this.interpretation = new CodeableConcept(); 3306 return this.interpretation; 3307 } 3308 else if (name.equals("comment")) { 3309 throw new FHIRException("Cannot call addChild on a singleton property Observation.comment"); 3310 } 3311 else if (name.equals("bodySite")) { 3312 this.bodySite = new CodeableConcept(); 3313 return this.bodySite; 3314 } 3315 else if (name.equals("method")) { 3316 this.method = new CodeableConcept(); 3317 return this.method; 3318 } 3319 else if (name.equals("specimen")) { 3320 this.specimen = new Reference(); 3321 return this.specimen; 3322 } 3323 else if (name.equals("device")) { 3324 this.device = new Reference(); 3325 return this.device; 3326 } 3327 else if (name.equals("referenceRange")) { 3328 return addReferenceRange(); 3329 } 3330 else if (name.equals("related")) { 3331 return addRelated(); 3332 } 3333 else if (name.equals("component")) { 3334 return addComponent(); 3335 } 3336 else 3337 return super.addChild(name); 3338 } 3339 3340 public String fhirType() { 3341 return "Observation"; 3342 3343 } 3344 3345 public Observation copy() { 3346 Observation dst = new Observation(); 3347 copyValues(dst); 3348 if (identifier != null) { 3349 dst.identifier = new ArrayList<Identifier>(); 3350 for (Identifier i : identifier) 3351 dst.identifier.add(i.copy()); 3352 }; 3353 if (basedOn != null) { 3354 dst.basedOn = new ArrayList<Reference>(); 3355 for (Reference i : basedOn) 3356 dst.basedOn.add(i.copy()); 3357 }; 3358 dst.status = status == null ? null : status.copy(); 3359 if (category != null) { 3360 dst.category = new ArrayList<CodeableConcept>(); 3361 for (CodeableConcept i : category) 3362 dst.category.add(i.copy()); 3363 }; 3364 dst.code = code == null ? null : code.copy(); 3365 dst.subject = subject == null ? null : subject.copy(); 3366 dst.context = context == null ? null : context.copy(); 3367 dst.effective = effective == null ? null : effective.copy(); 3368 dst.issued = issued == null ? null : issued.copy(); 3369 if (performer != null) { 3370 dst.performer = new ArrayList<Reference>(); 3371 for (Reference i : performer) 3372 dst.performer.add(i.copy()); 3373 }; 3374 dst.value = value == null ? null : value.copy(); 3375 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 3376 dst.interpretation = interpretation == null ? null : interpretation.copy(); 3377 dst.comment = comment == null ? null : comment.copy(); 3378 dst.bodySite = bodySite == null ? null : bodySite.copy(); 3379 dst.method = method == null ? null : method.copy(); 3380 dst.specimen = specimen == null ? null : specimen.copy(); 3381 dst.device = device == null ? null : device.copy(); 3382 if (referenceRange != null) { 3383 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3384 for (ObservationReferenceRangeComponent i : referenceRange) 3385 dst.referenceRange.add(i.copy()); 3386 }; 3387 if (related != null) { 3388 dst.related = new ArrayList<ObservationRelatedComponent>(); 3389 for (ObservationRelatedComponent i : related) 3390 dst.related.add(i.copy()); 3391 }; 3392 if (component != null) { 3393 dst.component = new ArrayList<ObservationComponentComponent>(); 3394 for (ObservationComponentComponent i : component) 3395 dst.component.add(i.copy()); 3396 }; 3397 return dst; 3398 } 3399 3400 protected Observation typedCopy() { 3401 return copy(); 3402 } 3403 3404 @Override 3405 public boolean equalsDeep(Base other_) { 3406 if (!super.equalsDeep(other_)) 3407 return false; 3408 if (!(other_ instanceof Observation)) 3409 return false; 3410 Observation o = (Observation) other_; 3411 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 3412 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 3413 && compareDeep(context, o.context, true) && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 3414 && compareDeep(performer, o.performer, true) && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 3415 && compareDeep(interpretation, o.interpretation, true) && compareDeep(comment, o.comment, true) 3416 && compareDeep(bodySite, o.bodySite, true) && compareDeep(method, o.method, true) && compareDeep(specimen, o.specimen, true) 3417 && compareDeep(device, o.device, true) && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(related, o.related, true) 3418 && compareDeep(component, o.component, true); 3419 } 3420 3421 @Override 3422 public boolean equalsShallow(Base other_) { 3423 if (!super.equalsShallow(other_)) 3424 return false; 3425 if (!(other_ instanceof Observation)) 3426 return false; 3427 Observation o = (Observation) other_; 3428 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(comment, o.comment, true) 3429 ; 3430 } 3431 3432 public boolean isEmpty() { 3433 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 3434 , category, code, subject, context, effective, issued, performer, value, dataAbsentReason 3435 , interpretation, comment, bodySite, method, specimen, device, referenceRange 3436 , related, component); 3437 } 3438 3439 @Override 3440 public ResourceType getResourceType() { 3441 return ResourceType.Observation; 3442 } 3443 3444 /** 3445 * Search parameter: <b>date</b> 3446 * <p> 3447 * Description: <b>Obtained date/time. If the obtained element is a period, a date that falls in the period</b><br> 3448 * Type: <b>date</b><br> 3449 * Path: <b>Observation.effective[x]</b><br> 3450 * </p> 3451 */ 3452 @SearchParamDefinition(name="date", path="Observation.effective", description="Obtained date/time. If the obtained element is a period, a date that falls in the period", type="date" ) 3453 public static final String SP_DATE = "date"; 3454 /** 3455 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3456 * <p> 3457 * Description: <b>Obtained date/time. If the obtained element is a period, a date that falls in the period</b><br> 3458 * Type: <b>date</b><br> 3459 * Path: <b>Observation.effective[x]</b><br> 3460 * </p> 3461 */ 3462 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3463 3464 /** 3465 * Search parameter: <b>combo-data-absent-reason</b> 3466 * <p> 3467 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 3468 * Type: <b>token</b><br> 3469 * Path: <b>Observation.dataAbsentReason, Observation.component.dataAbsentReason</b><br> 3470 * </p> 3471 */ 3472 @SearchParamDefinition(name="combo-data-absent-reason", path="Observation.dataAbsentReason | Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.", type="token" ) 3473 public static final String SP_COMBO_DATA_ABSENT_REASON = "combo-data-absent-reason"; 3474 /** 3475 * <b>Fluent Client</b> search parameter constant for <b>combo-data-absent-reason</b> 3476 * <p> 3477 * Description: <b>The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.</b><br> 3478 * Type: <b>token</b><br> 3479 * Path: <b>Observation.dataAbsentReason, Observation.component.dataAbsentReason</b><br> 3480 * </p> 3481 */ 3482 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_DATA_ABSENT_REASON); 3483 3484 /** 3485 * Search parameter: <b>code</b> 3486 * <p> 3487 * Description: <b>The code of the observation type</b><br> 3488 * Type: <b>token</b><br> 3489 * Path: <b>Observation.code</b><br> 3490 * </p> 3491 */ 3492 @SearchParamDefinition(name="code", path="Observation.code", description="The code of the observation type", type="token" ) 3493 public static final String SP_CODE = "code"; 3494 /** 3495 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3496 * <p> 3497 * Description: <b>The code of the observation type</b><br> 3498 * Type: <b>token</b><br> 3499 * Path: <b>Observation.code</b><br> 3500 * </p> 3501 */ 3502 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3503 3504 /** 3505 * Search parameter: <b>combo-code-value-quantity</b> 3506 * <p> 3507 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 3508 * Type: <b>composite</b><br> 3509 * Path: <b></b><br> 3510 * </p> 3511 */ 3512 @SearchParamDefinition(name="combo-code-value-quantity", path="Observation | Observation.component", description="Code and quantity value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-quantity"} ) 3513 public static final String SP_COMBO_CODE_VALUE_QUANTITY = "combo-code-value-quantity"; 3514 /** 3515 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-quantity</b> 3516 * <p> 3517 * Description: <b>Code and quantity value parameter pair, including in components</b><br> 3518 * Type: <b>composite</b><br> 3519 * Path: <b></b><br> 3520 * </p> 3521 */ 3522 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMBO_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMBO_CODE_VALUE_QUANTITY); 3523 3524 /** 3525 * Search parameter: <b>subject</b> 3526 * <p> 3527 * Description: <b>The subject that the observation is about</b><br> 3528 * Type: <b>reference</b><br> 3529 * Path: <b>Observation.subject</b><br> 3530 * </p> 3531 */ 3532 @SearchParamDefinition(name="subject", path="Observation.subject", description="The subject that the observation is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 3533 public static final String SP_SUBJECT = "subject"; 3534 /** 3535 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3536 * <p> 3537 * Description: <b>The subject that the observation is about</b><br> 3538 * Type: <b>reference</b><br> 3539 * Path: <b>Observation.subject</b><br> 3540 * </p> 3541 */ 3542 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3543 3544/** 3545 * Constant for fluent queries to be used to add include statements. Specifies 3546 * the path value of "<b>Observation:subject</b>". 3547 */ 3548 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Observation:subject").toLocked(); 3549 3550 /** 3551 * Search parameter: <b>component-data-absent-reason</b> 3552 * <p> 3553 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 3554 * Type: <b>token</b><br> 3555 * Path: <b>Observation.component.dataAbsentReason</b><br> 3556 * </p> 3557 */ 3558 @SearchParamDefinition(name="component-data-absent-reason", path="Observation.component.dataAbsentReason", description="The reason why the expected value in the element Observation.component.value[x] is missing.", type="token" ) 3559 public static final String SP_COMPONENT_DATA_ABSENT_REASON = "component-data-absent-reason"; 3560 /** 3561 * <b>Fluent Client</b> search parameter constant for <b>component-data-absent-reason</b> 3562 * <p> 3563 * Description: <b>The reason why the expected value in the element Observation.component.value[x] is missing.</b><br> 3564 * Type: <b>token</b><br> 3565 * Path: <b>Observation.component.dataAbsentReason</b><br> 3566 * </p> 3567 */ 3568 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_DATA_ABSENT_REASON); 3569 3570 /** 3571 * Search parameter: <b>value-concept</b> 3572 * <p> 3573 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 3574 * Type: <b>token</b><br> 3575 * Path: <b>Observation.valueCodeableConcept</b><br> 3576 * </p> 3577 */ 3578 @SearchParamDefinition(name="value-concept", path="Observation.value.as(CodeableConcept)", description="The value of the observation, if the value is a CodeableConcept", type="token" ) 3579 public static final String SP_VALUE_CONCEPT = "value-concept"; 3580 /** 3581 * <b>Fluent Client</b> search parameter constant for <b>value-concept</b> 3582 * <p> 3583 * Description: <b>The value of the observation, if the value is a CodeableConcept</b><br> 3584 * Type: <b>token</b><br> 3585 * Path: <b>Observation.valueCodeableConcept</b><br> 3586 * </p> 3587 */ 3588 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VALUE_CONCEPT); 3589 3590 /** 3591 * Search parameter: <b>value-date</b> 3592 * <p> 3593 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 3594 * Type: <b>date</b><br> 3595 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 3596 * </p> 3597 */ 3598 @SearchParamDefinition(name="value-date", path="Observation.value.as(DateTime) | Observation.value.as(Period)", description="The value of the observation, if the value is a date or period of time", type="date" ) 3599 public static final String SP_VALUE_DATE = "value-date"; 3600 /** 3601 * <b>Fluent Client</b> search parameter constant for <b>value-date</b> 3602 * <p> 3603 * Description: <b>The value of the observation, if the value is a date or period of time</b><br> 3604 * Type: <b>date</b><br> 3605 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 3606 * </p> 3607 */ 3608 public static final ca.uhn.fhir.rest.gclient.DateClientParam VALUE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_VALUE_DATE); 3609 3610 /** 3611 * Search parameter: <b>code-value-string</b> 3612 * <p> 3613 * Description: <b>Code and string value parameter pair</b><br> 3614 * Type: <b>composite</b><br> 3615 * Path: <b></b><br> 3616 * </p> 3617 */ 3618 @SearchParamDefinition(name="code-value-string", path="Observation", description="Code and string value parameter pair", type="composite", compositeOf={"code", "value-string"} ) 3619 public static final String SP_CODE_VALUE_STRING = "code-value-string"; 3620 /** 3621 * <b>Fluent Client</b> search parameter constant for <b>code-value-string</b> 3622 * <p> 3623 * Description: <b>Code and string value parameter pair</b><br> 3624 * Type: <b>composite</b><br> 3625 * Path: <b></b><br> 3626 * </p> 3627 */ 3628 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> CODE_VALUE_STRING = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>(SP_CODE_VALUE_STRING); 3629 3630 /** 3631 * Search parameter: <b>component-code-value-quantity</b> 3632 * <p> 3633 * Description: <b>Component code and component quantity value parameter pair</b><br> 3634 * Type: <b>composite</b><br> 3635 * Path: <b></b><br> 3636 * </p> 3637 */ 3638 @SearchParamDefinition(name="component-code-value-quantity", path="Observation.component", description="Component code and component quantity value parameter pair", type="composite", compositeOf={"component-code", "component-value-quantity"} ) 3639 public static final String SP_COMPONENT_CODE_VALUE_QUANTITY = "component-code-value-quantity"; 3640 /** 3641 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-quantity</b> 3642 * <p> 3643 * Description: <b>Component code and component quantity value parameter pair</b><br> 3644 * Type: <b>composite</b><br> 3645 * Path: <b></b><br> 3646 * </p> 3647 */ 3648 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMPONENT_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_COMPONENT_CODE_VALUE_QUANTITY); 3649 3650 /** 3651 * Search parameter: <b>based-on</b> 3652 * <p> 3653 * Description: <b>Reference to the test or procedure request.</b><br> 3654 * Type: <b>reference</b><br> 3655 * Path: <b>Observation.basedOn</b><br> 3656 * </p> 3657 */ 3658 @SearchParamDefinition(name="based-on", path="Observation.basedOn", description="Reference to the test or procedure request.", type="reference", target={CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ProcedureRequest.class, ReferralRequest.class } ) 3659 public static final String SP_BASED_ON = "based-on"; 3660 /** 3661 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3662 * <p> 3663 * Description: <b>Reference to the test or procedure request.</b><br> 3664 * Type: <b>reference</b><br> 3665 * Path: <b>Observation.basedOn</b><br> 3666 * </p> 3667 */ 3668 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3669 3670/** 3671 * Constant for fluent queries to be used to add include statements. Specifies 3672 * the path value of "<b>Observation:based-on</b>". 3673 */ 3674 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Observation:based-on").toLocked(); 3675 3676 /** 3677 * Search parameter: <b>related</b> 3678 * <p> 3679 * Description: <b>Related Observations - search on related-type and related-target together</b><br> 3680 * Type: <b>composite</b><br> 3681 * Path: <b></b><br> 3682 * </p> 3683 */ 3684 @SearchParamDefinition(name="related", path="Observation.related", description="Related Observations - search on related-type and related-target together", type="composite", compositeOf={"related-target", "related-type"} ) 3685 public static final String SP_RELATED = "related"; 3686 /** 3687 * <b>Fluent Client</b> search parameter constant for <b>related</b> 3688 * <p> 3689 * Description: <b>Related Observations - search on related-type and related-target together</b><br> 3690 * Type: <b>composite</b><br> 3691 * Path: <b></b><br> 3692 * </p> 3693 */ 3694 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATED = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_RELATED); 3695 3696 /** 3697 * Search parameter: <b>code-value-date</b> 3698 * <p> 3699 * Description: <b>Code and date/time value parameter pair</b><br> 3700 * Type: <b>composite</b><br> 3701 * Path: <b></b><br> 3702 * </p> 3703 */ 3704 @SearchParamDefinition(name="code-value-date", path="Observation", description="Code and date/time value parameter pair", type="composite", compositeOf={"code", "value-date"} ) 3705 public static final String SP_CODE_VALUE_DATE = "code-value-date"; 3706 /** 3707 * <b>Fluent Client</b> search parameter constant for <b>code-value-date</b> 3708 * <p> 3709 * Description: <b>Code and date/time value parameter pair</b><br> 3710 * Type: <b>composite</b><br> 3711 * Path: <b></b><br> 3712 * </p> 3713 */ 3714 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam> CODE_VALUE_DATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam>(SP_CODE_VALUE_DATE); 3715 3716 /** 3717 * Search parameter: <b>patient</b> 3718 * <p> 3719 * Description: <b>The subject that the observation is about (if patient)</b><br> 3720 * Type: <b>reference</b><br> 3721 * Path: <b>Observation.subject</b><br> 3722 * </p> 3723 */ 3724 @SearchParamDefinition(name="patient", path="Observation.subject", description="The subject that the observation is about (if patient)", type="reference", target={Patient.class } ) 3725 public static final String SP_PATIENT = "patient"; 3726 /** 3727 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3728 * <p> 3729 * Description: <b>The subject that the observation is about (if patient)</b><br> 3730 * Type: <b>reference</b><br> 3731 * Path: <b>Observation.subject</b><br> 3732 * </p> 3733 */ 3734 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3735 3736/** 3737 * Constant for fluent queries to be used to add include statements. Specifies 3738 * the path value of "<b>Observation:patient</b>". 3739 */ 3740 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Observation:patient").toLocked(); 3741 3742 /** 3743 * Search parameter: <b>specimen</b> 3744 * <p> 3745 * Description: <b>Specimen used for this observation</b><br> 3746 * Type: <b>reference</b><br> 3747 * Path: <b>Observation.specimen</b><br> 3748 * </p> 3749 */ 3750 @SearchParamDefinition(name="specimen", path="Observation.specimen", description="Specimen used for this observation", type="reference", target={Specimen.class } ) 3751 public static final String SP_SPECIMEN = "specimen"; 3752 /** 3753 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 3754 * <p> 3755 * Description: <b>Specimen used for this observation</b><br> 3756 * Type: <b>reference</b><br> 3757 * Path: <b>Observation.specimen</b><br> 3758 * </p> 3759 */ 3760 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 3761 3762/** 3763 * Constant for fluent queries to be used to add include statements. Specifies 3764 * the path value of "<b>Observation:specimen</b>". 3765 */ 3766 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("Observation:specimen").toLocked(); 3767 3768 /** 3769 * Search parameter: <b>component-code</b> 3770 * <p> 3771 * Description: <b>The component code of the observation type</b><br> 3772 * Type: <b>token</b><br> 3773 * Path: <b>Observation.component.code</b><br> 3774 * </p> 3775 */ 3776 @SearchParamDefinition(name="component-code", path="Observation.component.code", description="The component code of the observation type", type="token" ) 3777 public static final String SP_COMPONENT_CODE = "component-code"; 3778 /** 3779 * <b>Fluent Client</b> search parameter constant for <b>component-code</b> 3780 * <p> 3781 * Description: <b>The component code of the observation type</b><br> 3782 * Type: <b>token</b><br> 3783 * Path: <b>Observation.component.code</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_CODE); 3787 3788 /** 3789 * Search parameter: <b>code-value-quantity</b> 3790 * <p> 3791 * Description: <b>Code and quantity value parameter pair</b><br> 3792 * Type: <b>composite</b><br> 3793 * Path: <b></b><br> 3794 * </p> 3795 */ 3796 @SearchParamDefinition(name="code-value-quantity", path="Observation", description="Code and quantity value parameter pair", type="composite", compositeOf={"code", "value-quantity"} ) 3797 public static final String SP_CODE_VALUE_QUANTITY = "code-value-quantity"; 3798 /** 3799 * <b>Fluent Client</b> search parameter constant for <b>code-value-quantity</b> 3800 * <p> 3801 * Description: <b>Code and quantity value parameter pair</b><br> 3802 * Type: <b>composite</b><br> 3803 * Path: <b></b><br> 3804 * </p> 3805 */ 3806 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CODE_VALUE_QUANTITY); 3807 3808 /** 3809 * Search parameter: <b>context</b> 3810 * <p> 3811 * Description: <b>Healthcare event (Episode-of-care or Encounter) related to the observation</b><br> 3812 * Type: <b>reference</b><br> 3813 * Path: <b>Observation.context</b><br> 3814 * </p> 3815 */ 3816 @SearchParamDefinition(name="context", path="Observation.context", description="Healthcare event (Episode-of-care or Encounter) related to the observation", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 3817 public static final String SP_CONTEXT = "context"; 3818 /** 3819 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3820 * <p> 3821 * Description: <b>Healthcare event (Episode-of-care or Encounter) related to the observation</b><br> 3822 * Type: <b>reference</b><br> 3823 * Path: <b>Observation.context</b><br> 3824 * </p> 3825 */ 3826 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3827 3828/** 3829 * Constant for fluent queries to be used to add include statements. Specifies 3830 * the path value of "<b>Observation:context</b>". 3831 */ 3832 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Observation:context").toLocked(); 3833 3834 /** 3835 * Search parameter: <b>combo-code-value-concept</b> 3836 * <p> 3837 * Description: <b>Code and coded value parameter pair, including in components</b><br> 3838 * Type: <b>composite</b><br> 3839 * Path: <b></b><br> 3840 * </p> 3841 */ 3842 @SearchParamDefinition(name="combo-code-value-concept", path="Observation | Observation.component", description="Code and coded value parameter pair, including in components", type="composite", compositeOf={"combo-code", "combo-value-concept"} ) 3843 public static final String SP_COMBO_CODE_VALUE_CONCEPT = "combo-code-value-concept"; 3844 /** 3845 * <b>Fluent Client</b> search parameter constant for <b>combo-code-value-concept</b> 3846 * <p> 3847 * Description: <b>Code and coded value parameter pair, including in components</b><br> 3848 * Type: <b>composite</b><br> 3849 * Path: <b></b><br> 3850 * </p> 3851 */ 3852 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMBO_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMBO_CODE_VALUE_CONCEPT); 3853 3854 /** 3855 * Search parameter: <b>value-string</b> 3856 * <p> 3857 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 3858 * Type: <b>string</b><br> 3859 * Path: <b>Observation.valueString</b><br> 3860 * </p> 3861 */ 3862 @SearchParamDefinition(name="value-string", path="Observation.value.as(String)", description="The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type="string" ) 3863 public static final String SP_VALUE_STRING = "value-string"; 3864 /** 3865 * <b>Fluent Client</b> search parameter constant for <b>value-string</b> 3866 * <p> 3867 * Description: <b>The value of the observation, if the value is a string, and also searches in CodeableConcept.text</b><br> 3868 * Type: <b>string</b><br> 3869 * Path: <b>Observation.valueString</b><br> 3870 * </p> 3871 */ 3872 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VALUE_STRING); 3873 3874 /** 3875 * Search parameter: <b>identifier</b> 3876 * <p> 3877 * Description: <b>The unique id for a particular observation</b><br> 3878 * Type: <b>token</b><br> 3879 * Path: <b>Observation.identifier</b><br> 3880 * </p> 3881 */ 3882 @SearchParamDefinition(name="identifier", path="Observation.identifier", description="The unique id for a particular observation", type="token" ) 3883 public static final String SP_IDENTIFIER = "identifier"; 3884 /** 3885 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3886 * <p> 3887 * Description: <b>The unique id for a particular observation</b><br> 3888 * Type: <b>token</b><br> 3889 * Path: <b>Observation.identifier</b><br> 3890 * </p> 3891 */ 3892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3893 3894 /** 3895 * Search parameter: <b>performer</b> 3896 * <p> 3897 * Description: <b>Who performed the observation</b><br> 3898 * Type: <b>reference</b><br> 3899 * Path: <b>Observation.performer</b><br> 3900 * </p> 3901 */ 3902 @SearchParamDefinition(name="performer", path="Observation.performer", description="Who performed the observation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3903 public static final String SP_PERFORMER = "performer"; 3904 /** 3905 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3906 * <p> 3907 * Description: <b>Who performed the observation</b><br> 3908 * Type: <b>reference</b><br> 3909 * Path: <b>Observation.performer</b><br> 3910 * </p> 3911 */ 3912 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3913 3914/** 3915 * Constant for fluent queries to be used to add include statements. Specifies 3916 * the path value of "<b>Observation:performer</b>". 3917 */ 3918 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Observation:performer").toLocked(); 3919 3920 /** 3921 * Search parameter: <b>combo-code</b> 3922 * <p> 3923 * Description: <b>The code of the observation type or component type</b><br> 3924 * Type: <b>token</b><br> 3925 * Path: <b>Observation.code, Observation.component.code</b><br> 3926 * </p> 3927 */ 3928 @SearchParamDefinition(name="combo-code", path="Observation.code | Observation.component.code", description="The code of the observation type or component type", type="token" ) 3929 public static final String SP_COMBO_CODE = "combo-code"; 3930 /** 3931 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 3932 * <p> 3933 * Description: <b>The code of the observation type or component type</b><br> 3934 * Type: <b>token</b><br> 3935 * Path: <b>Observation.code, Observation.component.code</b><br> 3936 * </p> 3937 */ 3938 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_CODE); 3939 3940 /** 3941 * Search parameter: <b>method</b> 3942 * <p> 3943 * Description: <b>The method used for the observation</b><br> 3944 * Type: <b>token</b><br> 3945 * Path: <b>Observation.method</b><br> 3946 * </p> 3947 */ 3948 @SearchParamDefinition(name="method", path="Observation.method", description="The method used for the observation", type="token" ) 3949 public static final String SP_METHOD = "method"; 3950 /** 3951 * <b>Fluent Client</b> search parameter constant for <b>method</b> 3952 * <p> 3953 * Description: <b>The method used for the observation</b><br> 3954 * Type: <b>token</b><br> 3955 * Path: <b>Observation.method</b><br> 3956 * </p> 3957 */ 3958 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 3959 3960 /** 3961 * Search parameter: <b>value-quantity</b> 3962 * <p> 3963 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 3964 * Type: <b>quantity</b><br> 3965 * Path: <b>Observation.valueQuantity</b><br> 3966 * </p> 3967 */ 3968 @SearchParamDefinition(name="value-quantity", path="Observation.value.as(Quantity)", description="The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 3969 public static final String SP_VALUE_QUANTITY = "value-quantity"; 3970 /** 3971 * <b>Fluent Client</b> search parameter constant for <b>value-quantity</b> 3972 * <p> 3973 * Description: <b>The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 3974 * Type: <b>quantity</b><br> 3975 * Path: <b>Observation.valueQuantity</b><br> 3976 * </p> 3977 */ 3978 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_VALUE_QUANTITY); 3979 3980 /** 3981 * Search parameter: <b>component-value-quantity</b> 3982 * <p> 3983 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 3984 * Type: <b>quantity</b><br> 3985 * Path: <b>Observation.component.valueQuantity</b><br> 3986 * </p> 3987 */ 3988 @SearchParamDefinition(name="component-value-quantity", path="Observation.component.value.as(Quantity)", description="The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 3989 public static final String SP_COMPONENT_VALUE_QUANTITY = "component-value-quantity"; 3990 /** 3991 * <b>Fluent Client</b> search parameter constant for <b>component-value-quantity</b> 3992 * <p> 3993 * Description: <b>The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 3994 * Type: <b>quantity</b><br> 3995 * Path: <b>Observation.component.valueQuantity</b><br> 3996 * </p> 3997 */ 3998 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMPONENT_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMPONENT_VALUE_QUANTITY); 3999 4000 /** 4001 * Search parameter: <b>data-absent-reason</b> 4002 * <p> 4003 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4004 * Type: <b>token</b><br> 4005 * Path: <b>Observation.dataAbsentReason</b><br> 4006 * </p> 4007 */ 4008 @SearchParamDefinition(name="data-absent-reason", path="Observation.dataAbsentReason", description="The reason why the expected value in the element Observation.value[x] is missing.", type="token" ) 4009 public static final String SP_DATA_ABSENT_REASON = "data-absent-reason"; 4010 /** 4011 * <b>Fluent Client</b> search parameter constant for <b>data-absent-reason</b> 4012 * <p> 4013 * Description: <b>The reason why the expected value in the element Observation.value[x] is missing.</b><br> 4014 * Type: <b>token</b><br> 4015 * Path: <b>Observation.dataAbsentReason</b><br> 4016 * </p> 4017 */ 4018 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DATA_ABSENT_REASON); 4019 4020 /** 4021 * Search parameter: <b>combo-value-quantity</b> 4022 * <p> 4023 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4024 * Type: <b>quantity</b><br> 4025 * Path: <b>Observation.valueQuantity, Observation.component.valueQuantity</b><br> 4026 * </p> 4027 */ 4028 @SearchParamDefinition(name="combo-value-quantity", path="Observation.value.as(Quantity) | Observation.component.value.as(Quantity)", description="The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type="quantity" ) 4029 public static final String SP_COMBO_VALUE_QUANTITY = "combo-value-quantity"; 4030 /** 4031 * <b>Fluent Client</b> search parameter constant for <b>combo-value-quantity</b> 4032 * <p> 4033 * Description: <b>The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)</b><br> 4034 * Type: <b>quantity</b><br> 4035 * Path: <b>Observation.valueQuantity, Observation.component.valueQuantity</b><br> 4036 * </p> 4037 */ 4038 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMBO_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_COMBO_VALUE_QUANTITY); 4039 4040 /** 4041 * Search parameter: <b>encounter</b> 4042 * <p> 4043 * Description: <b>Encounter related to the observation</b><br> 4044 * Type: <b>reference</b><br> 4045 * Path: <b>Observation.context</b><br> 4046 * </p> 4047 */ 4048 @SearchParamDefinition(name="encounter", path="Observation.context", description="Encounter related to the observation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 4049 public static final String SP_ENCOUNTER = "encounter"; 4050 /** 4051 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4052 * <p> 4053 * Description: <b>Encounter related to the observation</b><br> 4054 * Type: <b>reference</b><br> 4055 * Path: <b>Observation.context</b><br> 4056 * </p> 4057 */ 4058 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4059 4060/** 4061 * Constant for fluent queries to be used to add include statements. Specifies 4062 * the path value of "<b>Observation:encounter</b>". 4063 */ 4064 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Observation:encounter").toLocked(); 4065 4066 /** 4067 * Search parameter: <b>related-type</b> 4068 * <p> 4069 * Description: <b>has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by</b><br> 4070 * Type: <b>token</b><br> 4071 * Path: <b>Observation.related.type</b><br> 4072 * </p> 4073 */ 4074 @SearchParamDefinition(name="related-type", path="Observation.related.type", description="has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by", type="token" ) 4075 public static final String SP_RELATED_TYPE = "related-type"; 4076 /** 4077 * <b>Fluent Client</b> search parameter constant for <b>related-type</b> 4078 * <p> 4079 * Description: <b>has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by</b><br> 4080 * Type: <b>token</b><br> 4081 * Path: <b>Observation.related.type</b><br> 4082 * </p> 4083 */ 4084 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATED_TYPE); 4085 4086 /** 4087 * Search parameter: <b>related-target</b> 4088 * <p> 4089 * Description: <b>Resource that is related to this one</b><br> 4090 * Type: <b>reference</b><br> 4091 * Path: <b>Observation.related.target</b><br> 4092 * </p> 4093 */ 4094 @SearchParamDefinition(name="related-target", path="Observation.related.target", description="Resource that is related to this one", type="reference", target={Observation.class, QuestionnaireResponse.class, Sequence.class } ) 4095 public static final String SP_RELATED_TARGET = "related-target"; 4096 /** 4097 * <b>Fluent Client</b> search parameter constant for <b>related-target</b> 4098 * <p> 4099 * Description: <b>Resource that is related to this one</b><br> 4100 * Type: <b>reference</b><br> 4101 * Path: <b>Observation.related.target</b><br> 4102 * </p> 4103 */ 4104 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATED_TARGET); 4105 4106/** 4107 * Constant for fluent queries to be used to add include statements. Specifies 4108 * the path value of "<b>Observation:related-target</b>". 4109 */ 4110 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_TARGET = new ca.uhn.fhir.model.api.Include("Observation:related-target").toLocked(); 4111 4112 /** 4113 * Search parameter: <b>code-value-concept</b> 4114 * <p> 4115 * Description: <b>Code and coded value parameter pair</b><br> 4116 * Type: <b>composite</b><br> 4117 * Path: <b></b><br> 4118 * </p> 4119 */ 4120 @SearchParamDefinition(name="code-value-concept", path="Observation", description="Code and coded value parameter pair", type="composite", compositeOf={"code", "value-concept"} ) 4121 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 4122 /** 4123 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 4124 * <p> 4125 * Description: <b>Code and coded value parameter pair</b><br> 4126 * Type: <b>composite</b><br> 4127 * Path: <b></b><br> 4128 * </p> 4129 */ 4130 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CODE_VALUE_CONCEPT); 4131 4132 /** 4133 * Search parameter: <b>component-code-value-concept</b> 4134 * <p> 4135 * Description: <b>Component code and component coded value parameter pair</b><br> 4136 * Type: <b>composite</b><br> 4137 * Path: <b></b><br> 4138 * </p> 4139 */ 4140 @SearchParamDefinition(name="component-code-value-concept", path="Observation.component", description="Component code and component coded value parameter pair", type="composite", compositeOf={"component-code", "component-value-concept"} ) 4141 public static final String SP_COMPONENT_CODE_VALUE_CONCEPT = "component-code-value-concept"; 4142 /** 4143 * <b>Fluent Client</b> search parameter constant for <b>component-code-value-concept</b> 4144 * <p> 4145 * Description: <b>Component code and component coded value parameter pair</b><br> 4146 * Type: <b>composite</b><br> 4147 * Path: <b></b><br> 4148 * </p> 4149 */ 4150 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMPONENT_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_COMPONENT_CODE_VALUE_CONCEPT); 4151 4152 /** 4153 * Search parameter: <b>component-value-concept</b> 4154 * <p> 4155 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4156 * Type: <b>token</b><br> 4157 * Path: <b>Observation.component.valueCodeableConcept</b><br> 4158 * </p> 4159 */ 4160 @SearchParamDefinition(name="component-value-concept", path="Observation.component.value.as(CodeableConcept)", description="The value of the component observation, if the value is a CodeableConcept", type="token" ) 4161 public static final String SP_COMPONENT_VALUE_CONCEPT = "component-value-concept"; 4162 /** 4163 * <b>Fluent Client</b> search parameter constant for <b>component-value-concept</b> 4164 * <p> 4165 * Description: <b>The value of the component observation, if the value is a CodeableConcept</b><br> 4166 * Type: <b>token</b><br> 4167 * Path: <b>Observation.component.valueCodeableConcept</b><br> 4168 * </p> 4169 */ 4170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMPONENT_VALUE_CONCEPT); 4171 4172 /** 4173 * Search parameter: <b>category</b> 4174 * <p> 4175 * Description: <b>The classification of the type of observation</b><br> 4176 * Type: <b>token</b><br> 4177 * Path: <b>Observation.category</b><br> 4178 * </p> 4179 */ 4180 @SearchParamDefinition(name="category", path="Observation.category", description="The classification of the type of observation", type="token" ) 4181 public static final String SP_CATEGORY = "category"; 4182 /** 4183 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4184 * <p> 4185 * Description: <b>The classification of the type of observation</b><br> 4186 * Type: <b>token</b><br> 4187 * Path: <b>Observation.category</b><br> 4188 * </p> 4189 */ 4190 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 4191 4192 /** 4193 * Search parameter: <b>device</b> 4194 * <p> 4195 * Description: <b>The Device that generated the observation data.</b><br> 4196 * Type: <b>reference</b><br> 4197 * Path: <b>Observation.device</b><br> 4198 * </p> 4199 */ 4200 @SearchParamDefinition(name="device", path="Observation.device", description="The Device that generated the observation data.", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class, DeviceMetric.class } ) 4201 public static final String SP_DEVICE = "device"; 4202 /** 4203 * <b>Fluent Client</b> search parameter constant for <b>device</b> 4204 * <p> 4205 * Description: <b>The Device that generated the observation data.</b><br> 4206 * Type: <b>reference</b><br> 4207 * Path: <b>Observation.device</b><br> 4208 * </p> 4209 */ 4210 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 4211 4212/** 4213 * Constant for fluent queries to be used to add include statements. Specifies 4214 * the path value of "<b>Observation:device</b>". 4215 */ 4216 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Observation:device").toLocked(); 4217 4218 /** 4219 * Search parameter: <b>combo-value-concept</b> 4220 * <p> 4221 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4222 * Type: <b>token</b><br> 4223 * Path: <b>Observation.valueCodeableConcept, Observation.component.valueCodeableConcept</b><br> 4224 * </p> 4225 */ 4226 @SearchParamDefinition(name="combo-value-concept", path="Observation.value.as(CodeableConcept) | Observation.component.value.as(CodeableConcept)", description="The value or component value of the observation, if the value is a CodeableConcept", type="token" ) 4227 public static final String SP_COMBO_VALUE_CONCEPT = "combo-value-concept"; 4228 /** 4229 * <b>Fluent Client</b> search parameter constant for <b>combo-value-concept</b> 4230 * <p> 4231 * Description: <b>The value or component value of the observation, if the value is a CodeableConcept</b><br> 4232 * Type: <b>token</b><br> 4233 * Path: <b>Observation.valueCodeableConcept, Observation.component.valueCodeableConcept</b><br> 4234 * </p> 4235 */ 4236 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMBO_VALUE_CONCEPT); 4237 4238 /** 4239 * Search parameter: <b>status</b> 4240 * <p> 4241 * Description: <b>The status of the observation</b><br> 4242 * Type: <b>token</b><br> 4243 * Path: <b>Observation.status</b><br> 4244 * </p> 4245 */ 4246 @SearchParamDefinition(name="status", path="Observation.status", description="The status of the observation", type="token" ) 4247 public static final String SP_STATUS = "status"; 4248 /** 4249 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4250 * <p> 4251 * Description: <b>The status of the observation</b><br> 4252 * Type: <b>token</b><br> 4253 * Path: <b>Observation.status</b><br> 4254 * </p> 4255 */ 4256 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4257 4258 4259}