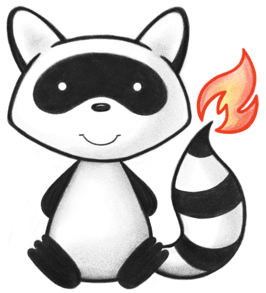
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.BindingStrength; 040import org.hl7.fhir.dstu3.model.Enumerations.BindingStrengthEnumFactory; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.dstu3.model.Enumerations.SearchParamType; 044import org.hl7.fhir.dstu3.model.Enumerations.SearchParamTypeEnumFactory; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.exceptions.FHIRFormatError; 047import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 048import org.hl7.fhir.utilities.Utilities; 049 050import ca.uhn.fhir.model.api.annotation.Block; 051import ca.uhn.fhir.model.api.annotation.Child; 052import ca.uhn.fhir.model.api.annotation.ChildOrder; 053import ca.uhn.fhir.model.api.annotation.Description; 054import ca.uhn.fhir.model.api.annotation.ResourceDef; 055import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 056/** 057 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 058 */ 059@ResourceDef(name="OperationDefinition", profile="http://hl7.org/fhir/Profile/OperationDefinition") 060@ChildOrder(names={"url", "version", "name", "status", "kind", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "idempotent", "code", "comment", "base", "resource", "system", "type", "instance", "parameter", "overload"}) 061public class OperationDefinition extends MetadataResource { 062 063 public enum OperationKind { 064 /** 065 * This operation is invoked as an operation. 066 */ 067 OPERATION, 068 /** 069 * This operation is a named query, invoked using the search mechanism. 070 */ 071 QUERY, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static OperationKind fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("operation".equals(codeString)) 080 return OPERATION; 081 if ("query".equals(codeString)) 082 return QUERY; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown OperationKind code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case OPERATION: return "operation"; 091 case QUERY: return "query"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case OPERATION: return "http://hl7.org/fhir/operation-kind"; 099 case QUERY: return "http://hl7.org/fhir/operation-kind"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getDefinition() { 105 switch (this) { 106 case OPERATION: return "This operation is invoked as an operation."; 107 case QUERY: return "This operation is a named query, invoked using the search mechanism."; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDisplay() { 113 switch (this) { 114 case OPERATION: return "Operation"; 115 case QUERY: return "Query"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 } 121 122 public static class OperationKindEnumFactory implements EnumFactory<OperationKind> { 123 public OperationKind fromCode(String codeString) throws IllegalArgumentException { 124 if (codeString == null || "".equals(codeString)) 125 if (codeString == null || "".equals(codeString)) 126 return null; 127 if ("operation".equals(codeString)) 128 return OperationKind.OPERATION; 129 if ("query".equals(codeString)) 130 return OperationKind.QUERY; 131 throw new IllegalArgumentException("Unknown OperationKind code '"+codeString+"'"); 132 } 133 public Enumeration<OperationKind> fromType(PrimitiveType<?> code) throws FHIRException { 134 if (code == null) 135 return null; 136 if (code.isEmpty()) 137 return new Enumeration<OperationKind>(this); 138 String codeString = code.asStringValue(); 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("operation".equals(codeString)) 142 return new Enumeration<OperationKind>(this, OperationKind.OPERATION); 143 if ("query".equals(codeString)) 144 return new Enumeration<OperationKind>(this, OperationKind.QUERY); 145 throw new FHIRException("Unknown OperationKind code '"+codeString+"'"); 146 } 147 public String toCode(OperationKind code) { 148 if (code == OperationKind.NULL) 149 return null; 150 if (code == OperationKind.OPERATION) 151 return "operation"; 152 if (code == OperationKind.QUERY) 153 return "query"; 154 return "?"; 155 } 156 public String toSystem(OperationKind code) { 157 return code.getSystem(); 158 } 159 } 160 161 public enum OperationParameterUse { 162 /** 163 * This is an input parameter. 164 */ 165 IN, 166 /** 167 * This is an output parameter. 168 */ 169 OUT, 170 /** 171 * added to help the parsers with the generic types 172 */ 173 NULL; 174 public static OperationParameterUse fromCode(String codeString) throws FHIRException { 175 if (codeString == null || "".equals(codeString)) 176 return null; 177 if ("in".equals(codeString)) 178 return IN; 179 if ("out".equals(codeString)) 180 return OUT; 181 if (Configuration.isAcceptInvalidEnums()) 182 return null; 183 else 184 throw new FHIRException("Unknown OperationParameterUse code '"+codeString+"'"); 185 } 186 public String toCode() { 187 switch (this) { 188 case IN: return "in"; 189 case OUT: return "out"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case IN: return "http://hl7.org/fhir/operation-parameter-use"; 197 case OUT: return "http://hl7.org/fhir/operation-parameter-use"; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDefinition() { 203 switch (this) { 204 case IN: return "This is an input parameter."; 205 case OUT: return "This is an output parameter."; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getDisplay() { 211 switch (this) { 212 case IN: return "In"; 213 case OUT: return "Out"; 214 case NULL: return null; 215 default: return "?"; 216 } 217 } 218 } 219 220 public static class OperationParameterUseEnumFactory implements EnumFactory<OperationParameterUse> { 221 public OperationParameterUse fromCode(String codeString) throws IllegalArgumentException { 222 if (codeString == null || "".equals(codeString)) 223 if (codeString == null || "".equals(codeString)) 224 return null; 225 if ("in".equals(codeString)) 226 return OperationParameterUse.IN; 227 if ("out".equals(codeString)) 228 return OperationParameterUse.OUT; 229 throw new IllegalArgumentException("Unknown OperationParameterUse code '"+codeString+"'"); 230 } 231 public Enumeration<OperationParameterUse> fromType(PrimitiveType<?> code) throws FHIRException { 232 if (code == null) 233 return null; 234 if (code.isEmpty()) 235 return new Enumeration<OperationParameterUse>(this); 236 String codeString = code.asStringValue(); 237 if (codeString == null || "".equals(codeString)) 238 return null; 239 if ("in".equals(codeString)) 240 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.IN); 241 if ("out".equals(codeString)) 242 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.OUT); 243 throw new FHIRException("Unknown OperationParameterUse code '"+codeString+"'"); 244 } 245 public String toCode(OperationParameterUse code) { 246 if (code == OperationParameterUse.NULL) 247 return null; 248 if (code == OperationParameterUse.IN) 249 return "in"; 250 if (code == OperationParameterUse.OUT) 251 return "out"; 252 return "?"; 253 } 254 public String toSystem(OperationParameterUse code) { 255 return code.getSystem(); 256 } 257 } 258 259 @Block() 260 public static class OperationDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 261 /** 262 * The name of used to identify the parameter. 263 */ 264 @Child(name = "name", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 265 @Description(shortDefinition="Name in Parameters.parameter.name or in URL", formalDefinition="The name of used to identify the parameter." ) 266 protected CodeType name; 267 268 /** 269 * Whether this is an input or an output parameter. 270 */ 271 @Child(name = "use", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 272 @Description(shortDefinition="in | out", formalDefinition="Whether this is an input or an output parameter." ) 273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-parameter-use") 274 protected Enumeration<OperationParameterUse> use; 275 276 /** 277 * The minimum number of times this parameter SHALL appear in the request or response. 278 */ 279 @Child(name = "min", type = {IntegerType.class}, order=3, min=1, max=1, modifier=false, summary=false) 280 @Description(shortDefinition="Minimum Cardinality", formalDefinition="The minimum number of times this parameter SHALL appear in the request or response." ) 281 protected IntegerType min; 282 283 /** 284 * The maximum number of times this element is permitted to appear in the request or response. 285 */ 286 @Child(name = "max", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 287 @Description(shortDefinition="Maximum Cardinality (a number or *)", formalDefinition="The maximum number of times this element is permitted to appear in the request or response." ) 288 protected StringType max; 289 290 /** 291 * Describes the meaning or use of this parameter. 292 */ 293 @Child(name = "documentation", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 294 @Description(shortDefinition="Description of meaning/use", formalDefinition="Describes the meaning or use of this parameter." ) 295 protected StringType documentation; 296 297 /** 298 * The type for this parameter. 299 */ 300 @Child(name = "type", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 301 @Description(shortDefinition="What type this parameter has", formalDefinition="The type for this parameter." ) 302 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-types") 303 protected CodeType type; 304 305 /** 306 * How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'. 307 */ 308 @Child(name = "searchType", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 309 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri", formalDefinition="How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'." ) 310 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 311 protected Enumeration<SearchParamType> searchType; 312 313 /** 314 * A profile the specifies the rules that this parameter must conform to. 315 */ 316 @Child(name = "profile", type = {StructureDefinition.class}, order=8, min=0, max=1, modifier=false, summary=false) 317 @Description(shortDefinition="Profile on the type", formalDefinition="A profile the specifies the rules that this parameter must conform to." ) 318 protected Reference profile; 319 320 /** 321 * The actual object that is the target of the reference (A profile the specifies the rules that this parameter must conform to.) 322 */ 323 protected StructureDefinition profileTarget; 324 325 /** 326 * Binds to a value set if this parameter is coded (code, Coding, CodeableConcept). 327 */ 328 @Child(name = "binding", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 329 @Description(shortDefinition="ValueSet details if this is coded", formalDefinition="Binds to a value set if this parameter is coded (code, Coding, CodeableConcept)." ) 330 protected OperationDefinitionParameterBindingComponent binding; 331 332 /** 333 * The parts of a nested Parameter. 334 */ 335 @Child(name = "part", type = {OperationDefinitionParameterComponent.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 336 @Description(shortDefinition="Parts of a nested Parameter", formalDefinition="The parts of a nested Parameter." ) 337 protected List<OperationDefinitionParameterComponent> part; 338 339 private static final long serialVersionUID = -885506257L; 340 341 /** 342 * Constructor 343 */ 344 public OperationDefinitionParameterComponent() { 345 super(); 346 } 347 348 /** 349 * Constructor 350 */ 351 public OperationDefinitionParameterComponent(CodeType name, Enumeration<OperationParameterUse> use, IntegerType min, StringType max) { 352 super(); 353 this.name = name; 354 this.use = use; 355 this.min = min; 356 this.max = max; 357 } 358 359 /** 360 * @return {@link #name} (The name of used to identify the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 361 */ 362 public CodeType getNameElement() { 363 if (this.name == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.name"); 366 else if (Configuration.doAutoCreate()) 367 this.name = new CodeType(); // bb 368 return this.name; 369 } 370 371 public boolean hasNameElement() { 372 return this.name != null && !this.name.isEmpty(); 373 } 374 375 public boolean hasName() { 376 return this.name != null && !this.name.isEmpty(); 377 } 378 379 /** 380 * @param value {@link #name} (The name of used to identify the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 381 */ 382 public OperationDefinitionParameterComponent setNameElement(CodeType value) { 383 this.name = value; 384 return this; 385 } 386 387 /** 388 * @return The name of used to identify the parameter. 389 */ 390 public String getName() { 391 return this.name == null ? null : this.name.getValue(); 392 } 393 394 /** 395 * @param value The name of used to identify the parameter. 396 */ 397 public OperationDefinitionParameterComponent setName(String value) { 398 if (this.name == null) 399 this.name = new CodeType(); 400 this.name.setValue(value); 401 return this; 402 } 403 404 /** 405 * @return {@link #use} (Whether this is an input or an output parameter.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 406 */ 407 public Enumeration<OperationParameterUse> getUseElement() { 408 if (this.use == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.use"); 411 else if (Configuration.doAutoCreate()) 412 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 413 return this.use; 414 } 415 416 public boolean hasUseElement() { 417 return this.use != null && !this.use.isEmpty(); 418 } 419 420 public boolean hasUse() { 421 return this.use != null && !this.use.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #use} (Whether this is an input or an output parameter.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 426 */ 427 public OperationDefinitionParameterComponent setUseElement(Enumeration<OperationParameterUse> value) { 428 this.use = value; 429 return this; 430 } 431 432 /** 433 * @return Whether this is an input or an output parameter. 434 */ 435 public OperationParameterUse getUse() { 436 return this.use == null ? null : this.use.getValue(); 437 } 438 439 /** 440 * @param value Whether this is an input or an output parameter. 441 */ 442 public OperationDefinitionParameterComponent setUse(OperationParameterUse value) { 443 if (this.use == null) 444 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 445 this.use.setValue(value); 446 return this; 447 } 448 449 /** 450 * @return {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 451 */ 452 public IntegerType getMinElement() { 453 if (this.min == null) 454 if (Configuration.errorOnAutoCreate()) 455 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.min"); 456 else if (Configuration.doAutoCreate()) 457 this.min = new IntegerType(); // bb 458 return this.min; 459 } 460 461 public boolean hasMinElement() { 462 return this.min != null && !this.min.isEmpty(); 463 } 464 465 public boolean hasMin() { 466 return this.min != null && !this.min.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 471 */ 472 public OperationDefinitionParameterComponent setMinElement(IntegerType value) { 473 this.min = value; 474 return this; 475 } 476 477 /** 478 * @return The minimum number of times this parameter SHALL appear in the request or response. 479 */ 480 public int getMin() { 481 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 482 } 483 484 /** 485 * @param value The minimum number of times this parameter SHALL appear in the request or response. 486 */ 487 public OperationDefinitionParameterComponent setMin(int value) { 488 if (this.min == null) 489 this.min = new IntegerType(); 490 this.min.setValue(value); 491 return this; 492 } 493 494 /** 495 * @return {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 496 */ 497 public StringType getMaxElement() { 498 if (this.max == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.max"); 501 else if (Configuration.doAutoCreate()) 502 this.max = new StringType(); // bb 503 return this.max; 504 } 505 506 public boolean hasMaxElement() { 507 return this.max != null && !this.max.isEmpty(); 508 } 509 510 public boolean hasMax() { 511 return this.max != null && !this.max.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 516 */ 517 public OperationDefinitionParameterComponent setMaxElement(StringType value) { 518 this.max = value; 519 return this; 520 } 521 522 /** 523 * @return The maximum number of times this element is permitted to appear in the request or response. 524 */ 525 public String getMax() { 526 return this.max == null ? null : this.max.getValue(); 527 } 528 529 /** 530 * @param value The maximum number of times this element is permitted to appear in the request or response. 531 */ 532 public OperationDefinitionParameterComponent setMax(String value) { 533 if (this.max == null) 534 this.max = new StringType(); 535 this.max.setValue(value); 536 return this; 537 } 538 539 /** 540 * @return {@link #documentation} (Describes the meaning or use of this parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 541 */ 542 public StringType getDocumentationElement() { 543 if (this.documentation == null) 544 if (Configuration.errorOnAutoCreate()) 545 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.documentation"); 546 else if (Configuration.doAutoCreate()) 547 this.documentation = new StringType(); // bb 548 return this.documentation; 549 } 550 551 public boolean hasDocumentationElement() { 552 return this.documentation != null && !this.documentation.isEmpty(); 553 } 554 555 public boolean hasDocumentation() { 556 return this.documentation != null && !this.documentation.isEmpty(); 557 } 558 559 /** 560 * @param value {@link #documentation} (Describes the meaning or use of this parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 561 */ 562 public OperationDefinitionParameterComponent setDocumentationElement(StringType value) { 563 this.documentation = value; 564 return this; 565 } 566 567 /** 568 * @return Describes the meaning or use of this parameter. 569 */ 570 public String getDocumentation() { 571 return this.documentation == null ? null : this.documentation.getValue(); 572 } 573 574 /** 575 * @param value Describes the meaning or use of this parameter. 576 */ 577 public OperationDefinitionParameterComponent setDocumentation(String value) { 578 if (Utilities.noString(value)) 579 this.documentation = null; 580 else { 581 if (this.documentation == null) 582 this.documentation = new StringType(); 583 this.documentation.setValue(value); 584 } 585 return this; 586 } 587 588 /** 589 * @return {@link #type} (The type for this parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 590 */ 591 public CodeType getTypeElement() { 592 if (this.type == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.type"); 595 else if (Configuration.doAutoCreate()) 596 this.type = new CodeType(); // bb 597 return this.type; 598 } 599 600 public boolean hasTypeElement() { 601 return this.type != null && !this.type.isEmpty(); 602 } 603 604 public boolean hasType() { 605 return this.type != null && !this.type.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #type} (The type for this parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 610 */ 611 public OperationDefinitionParameterComponent setTypeElement(CodeType value) { 612 this.type = value; 613 return this; 614 } 615 616 /** 617 * @return The type for this parameter. 618 */ 619 public String getType() { 620 return this.type == null ? null : this.type.getValue(); 621 } 622 623 /** 624 * @param value The type for this parameter. 625 */ 626 public OperationDefinitionParameterComponent setType(String value) { 627 if (Utilities.noString(value)) 628 this.type = null; 629 else { 630 if (this.type == null) 631 this.type = new CodeType(); 632 this.type.setValue(value); 633 } 634 return this; 635 } 636 637 /** 638 * @return {@link #searchType} (How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.). This is the underlying object with id, value and extensions. The accessor "getSearchType" gives direct access to the value 639 */ 640 public Enumeration<SearchParamType> getSearchTypeElement() { 641 if (this.searchType == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.searchType"); 644 else if (Configuration.doAutoCreate()) 645 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 646 return this.searchType; 647 } 648 649 public boolean hasSearchTypeElement() { 650 return this.searchType != null && !this.searchType.isEmpty(); 651 } 652 653 public boolean hasSearchType() { 654 return this.searchType != null && !this.searchType.isEmpty(); 655 } 656 657 /** 658 * @param value {@link #searchType} (How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.). This is the underlying object with id, value and extensions. The accessor "getSearchType" gives direct access to the value 659 */ 660 public OperationDefinitionParameterComponent setSearchTypeElement(Enumeration<SearchParamType> value) { 661 this.searchType = value; 662 return this; 663 } 664 665 /** 666 * @return How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'. 667 */ 668 public SearchParamType getSearchType() { 669 return this.searchType == null ? null : this.searchType.getValue(); 670 } 671 672 /** 673 * @param value How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'. 674 */ 675 public OperationDefinitionParameterComponent setSearchType(SearchParamType value) { 676 if (value == null) 677 this.searchType = null; 678 else { 679 if (this.searchType == null) 680 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 681 this.searchType.setValue(value); 682 } 683 return this; 684 } 685 686 /** 687 * @return {@link #profile} (A profile the specifies the rules that this parameter must conform to.) 688 */ 689 public Reference getProfile() { 690 if (this.profile == null) 691 if (Configuration.errorOnAutoCreate()) 692 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.profile"); 693 else if (Configuration.doAutoCreate()) 694 this.profile = new Reference(); // cc 695 return this.profile; 696 } 697 698 public boolean hasProfile() { 699 return this.profile != null && !this.profile.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #profile} (A profile the specifies the rules that this parameter must conform to.) 704 */ 705 public OperationDefinitionParameterComponent setProfile(Reference value) { 706 this.profile = value; 707 return this; 708 } 709 710 /** 711 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A profile the specifies the rules that this parameter must conform to.) 712 */ 713 public StructureDefinition getProfileTarget() { 714 if (this.profileTarget == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.profile"); 717 else if (Configuration.doAutoCreate()) 718 this.profileTarget = new StructureDefinition(); // aa 719 return this.profileTarget; 720 } 721 722 /** 723 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A profile the specifies the rules that this parameter must conform to.) 724 */ 725 public OperationDefinitionParameterComponent setProfileTarget(StructureDefinition value) { 726 this.profileTarget = value; 727 return this; 728 } 729 730 /** 731 * @return {@link #binding} (Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).) 732 */ 733 public OperationDefinitionParameterBindingComponent getBinding() { 734 if (this.binding == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.binding"); 737 else if (Configuration.doAutoCreate()) 738 this.binding = new OperationDefinitionParameterBindingComponent(); // cc 739 return this.binding; 740 } 741 742 public boolean hasBinding() { 743 return this.binding != null && !this.binding.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #binding} (Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).) 748 */ 749 public OperationDefinitionParameterComponent setBinding(OperationDefinitionParameterBindingComponent value) { 750 this.binding = value; 751 return this; 752 } 753 754 /** 755 * @return {@link #part} (The parts of a nested Parameter.) 756 */ 757 public List<OperationDefinitionParameterComponent> getPart() { 758 if (this.part == null) 759 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 760 return this.part; 761 } 762 763 /** 764 * @return Returns a reference to <code>this</code> for easy method chaining 765 */ 766 public OperationDefinitionParameterComponent setPart(List<OperationDefinitionParameterComponent> thePart) { 767 this.part = thePart; 768 return this; 769 } 770 771 public boolean hasPart() { 772 if (this.part == null) 773 return false; 774 for (OperationDefinitionParameterComponent item : this.part) 775 if (!item.isEmpty()) 776 return true; 777 return false; 778 } 779 780 public OperationDefinitionParameterComponent addPart() { //3 781 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 782 if (this.part == null) 783 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 784 this.part.add(t); 785 return t; 786 } 787 788 public OperationDefinitionParameterComponent addPart(OperationDefinitionParameterComponent t) { //3 789 if (t == null) 790 return this; 791 if (this.part == null) 792 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 793 this.part.add(t); 794 return this; 795 } 796 797 /** 798 * @return The first repetition of repeating field {@link #part}, creating it if it does not already exist 799 */ 800 public OperationDefinitionParameterComponent getPartFirstRep() { 801 if (getPart().isEmpty()) { 802 addPart(); 803 } 804 return getPart().get(0); 805 } 806 807 protected void listChildren(List<Property> children) { 808 super.listChildren(children); 809 children.add(new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name)); 810 children.add(new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use)); 811 children.add(new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 812 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 813 children.add(new Property("documentation", "string", "Describes the meaning or use of this parameter.", 0, 1, documentation)); 814 children.add(new Property("type", "code", "The type for this parameter.", 0, 1, type)); 815 children.add(new Property("searchType", "code", "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 0, 1, searchType)); 816 children.add(new Property("profile", "Reference(StructureDefinition)", "A profile the specifies the rules that this parameter must conform to.", 0, 1, profile)); 817 children.add(new Property("binding", "", "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding)); 818 children.add(new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, java.lang.Integer.MAX_VALUE, part)); 819 } 820 821 @Override 822 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 823 switch (_hash) { 824 case 3373707: /*name*/ return new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name); 825 case 116103: /*use*/ return new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use); 826 case 108114: /*min*/ return new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 827 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 828 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Describes the meaning or use of this parameter.", 0, 1, documentation); 829 case 3575610: /*type*/ return new Property("type", "code", "The type for this parameter.", 0, 1, type); 830 case -710454014: /*searchType*/ return new Property("searchType", "code", "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 0, 1, searchType); 831 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A profile the specifies the rules that this parameter must conform to.", 0, 1, profile); 832 case -108220795: /*binding*/ return new Property("binding", "", "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding); 833 case 3433459: /*part*/ return new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, java.lang.Integer.MAX_VALUE, part); 834 default: return super.getNamedProperty(_hash, _name, _checkValid); 835 } 836 837 } 838 839 @Override 840 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 841 switch (hash) { 842 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 843 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<OperationParameterUse> 844 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 845 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 846 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 847 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 848 case -710454014: /*searchType*/ return this.searchType == null ? new Base[0] : new Base[] {this.searchType}; // Enumeration<SearchParamType> 849 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 850 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // OperationDefinitionParameterBindingComponent 851 case 3433459: /*part*/ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // OperationDefinitionParameterComponent 852 default: return super.getProperty(hash, name, checkValid); 853 } 854 855 } 856 857 @Override 858 public Base setProperty(int hash, String name, Base value) throws FHIRException { 859 switch (hash) { 860 case 3373707: // name 861 this.name = castToCode(value); // CodeType 862 return value; 863 case 116103: // use 864 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 865 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 866 return value; 867 case 108114: // min 868 this.min = castToInteger(value); // IntegerType 869 return value; 870 case 107876: // max 871 this.max = castToString(value); // StringType 872 return value; 873 case 1587405498: // documentation 874 this.documentation = castToString(value); // StringType 875 return value; 876 case 3575610: // type 877 this.type = castToCode(value); // CodeType 878 return value; 879 case -710454014: // searchType 880 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 881 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 882 return value; 883 case -309425751: // profile 884 this.profile = castToReference(value); // Reference 885 return value; 886 case -108220795: // binding 887 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 888 return value; 889 case 3433459: // part 890 this.getPart().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 891 return value; 892 default: return super.setProperty(hash, name, value); 893 } 894 895 } 896 897 @Override 898 public Base setProperty(String name, Base value) throws FHIRException { 899 if (name.equals("name")) { 900 this.name = castToCode(value); // CodeType 901 } else if (name.equals("use")) { 902 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 903 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 904 } else if (name.equals("min")) { 905 this.min = castToInteger(value); // IntegerType 906 } else if (name.equals("max")) { 907 this.max = castToString(value); // StringType 908 } else if (name.equals("documentation")) { 909 this.documentation = castToString(value); // StringType 910 } else if (name.equals("type")) { 911 this.type = castToCode(value); // CodeType 912 } else if (name.equals("searchType")) { 913 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 914 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 915 } else if (name.equals("profile")) { 916 this.profile = castToReference(value); // Reference 917 } else if (name.equals("binding")) { 918 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 919 } else if (name.equals("part")) { 920 this.getPart().add((OperationDefinitionParameterComponent) value); 921 } else 922 return super.setProperty(name, value); 923 return value; 924 } 925 926 @Override 927 public Base makeProperty(int hash, String name) throws FHIRException { 928 switch (hash) { 929 case 3373707: return getNameElement(); 930 case 116103: return getUseElement(); 931 case 108114: return getMinElement(); 932 case 107876: return getMaxElement(); 933 case 1587405498: return getDocumentationElement(); 934 case 3575610: return getTypeElement(); 935 case -710454014: return getSearchTypeElement(); 936 case -309425751: return getProfile(); 937 case -108220795: return getBinding(); 938 case 3433459: return addPart(); 939 default: return super.makeProperty(hash, name); 940 } 941 942 } 943 944 @Override 945 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 946 switch (hash) { 947 case 3373707: /*name*/ return new String[] {"code"}; 948 case 116103: /*use*/ return new String[] {"code"}; 949 case 108114: /*min*/ return new String[] {"integer"}; 950 case 107876: /*max*/ return new String[] {"string"}; 951 case 1587405498: /*documentation*/ return new String[] {"string"}; 952 case 3575610: /*type*/ return new String[] {"code"}; 953 case -710454014: /*searchType*/ return new String[] {"code"}; 954 case -309425751: /*profile*/ return new String[] {"Reference"}; 955 case -108220795: /*binding*/ return new String[] {}; 956 case 3433459: /*part*/ return new String[] {"@OperationDefinition.parameter"}; 957 default: return super.getTypesForProperty(hash, name); 958 } 959 960 } 961 962 @Override 963 public Base addChild(String name) throws FHIRException { 964 if (name.equals("name")) { 965 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 966 } 967 else if (name.equals("use")) { 968 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.use"); 969 } 970 else if (name.equals("min")) { 971 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.min"); 972 } 973 else if (name.equals("max")) { 974 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.max"); 975 } 976 else if (name.equals("documentation")) { 977 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.documentation"); 978 } 979 else if (name.equals("type")) { 980 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 981 } 982 else if (name.equals("searchType")) { 983 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.searchType"); 984 } 985 else if (name.equals("profile")) { 986 this.profile = new Reference(); 987 return this.profile; 988 } 989 else if (name.equals("binding")) { 990 this.binding = new OperationDefinitionParameterBindingComponent(); 991 return this.binding; 992 } 993 else if (name.equals("part")) { 994 return addPart(); 995 } 996 else 997 return super.addChild(name); 998 } 999 1000 public OperationDefinitionParameterComponent copy() { 1001 OperationDefinitionParameterComponent dst = new OperationDefinitionParameterComponent(); 1002 copyValues(dst); 1003 dst.name = name == null ? null : name.copy(); 1004 dst.use = use == null ? null : use.copy(); 1005 dst.min = min == null ? null : min.copy(); 1006 dst.max = max == null ? null : max.copy(); 1007 dst.documentation = documentation == null ? null : documentation.copy(); 1008 dst.type = type == null ? null : type.copy(); 1009 dst.searchType = searchType == null ? null : searchType.copy(); 1010 dst.profile = profile == null ? null : profile.copy(); 1011 dst.binding = binding == null ? null : binding.copy(); 1012 if (part != null) { 1013 dst.part = new ArrayList<OperationDefinitionParameterComponent>(); 1014 for (OperationDefinitionParameterComponent i : part) 1015 dst.part.add(i.copy()); 1016 }; 1017 return dst; 1018 } 1019 1020 @Override 1021 public boolean equalsDeep(Base other_) { 1022 if (!super.equalsDeep(other_)) 1023 return false; 1024 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1025 return false; 1026 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1027 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 1028 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) && compareDeep(type, o.type, true) 1029 && compareDeep(searchType, o.searchType, true) && compareDeep(profile, o.profile, true) && compareDeep(binding, o.binding, true) 1030 && compareDeep(part, o.part, true); 1031 } 1032 1033 @Override 1034 public boolean equalsShallow(Base other_) { 1035 if (!super.equalsShallow(other_)) 1036 return false; 1037 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1038 return false; 1039 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1040 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 1041 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) && compareValues(type, o.type, true) 1042 && compareValues(searchType, o.searchType, true); 1043 } 1044 1045 public boolean isEmpty() { 1046 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation 1047 , type, searchType, profile, binding, part); 1048 } 1049 1050 public String fhirType() { 1051 return "OperationDefinition.parameter"; 1052 1053 } 1054 1055 } 1056 1057 @Block() 1058 public static class OperationDefinitionParameterBindingComponent extends BackboneElement implements IBaseBackboneElement { 1059 /** 1060 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1061 */ 1062 @Child(name = "strength", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1063 @Description(shortDefinition="required | extensible | preferred | example", formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances." ) 1064 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/binding-strength") 1065 protected Enumeration<BindingStrength> strength; 1066 1067 /** 1068 * Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. 1069 */ 1070 @Child(name = "valueSet", type = {UriType.class, ValueSet.class}, order=2, min=1, max=1, modifier=false, summary=false) 1071 @Description(shortDefinition="Source of value set", formalDefinition="Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used." ) 1072 protected Type valueSet; 1073 1074 private static final long serialVersionUID = 857140521L; 1075 1076 /** 1077 * Constructor 1078 */ 1079 public OperationDefinitionParameterBindingComponent() { 1080 super(); 1081 } 1082 1083 /** 1084 * Constructor 1085 */ 1086 public OperationDefinitionParameterBindingComponent(Enumeration<BindingStrength> strength, Type valueSet) { 1087 super(); 1088 this.strength = strength; 1089 this.valueSet = valueSet; 1090 } 1091 1092 /** 1093 * @return {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 1094 */ 1095 public Enumeration<BindingStrength> getStrengthElement() { 1096 if (this.strength == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.strength"); 1099 else if (Configuration.doAutoCreate()) 1100 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 1101 return this.strength; 1102 } 1103 1104 public boolean hasStrengthElement() { 1105 return this.strength != null && !this.strength.isEmpty(); 1106 } 1107 1108 public boolean hasStrength() { 1109 return this.strength != null && !this.strength.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 1114 */ 1115 public OperationDefinitionParameterBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 1116 this.strength = value; 1117 return this; 1118 } 1119 1120 /** 1121 * @return Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1122 */ 1123 public BindingStrength getStrength() { 1124 return this.strength == null ? null : this.strength.getValue(); 1125 } 1126 1127 /** 1128 * @param value Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 1129 */ 1130 public OperationDefinitionParameterBindingComponent setStrength(BindingStrength value) { 1131 if (this.strength == null) 1132 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 1133 this.strength.setValue(value); 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.) 1139 */ 1140 public Type getValueSet() { 1141 return this.valueSet; 1142 } 1143 1144 /** 1145 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.) 1146 */ 1147 public UriType getValueSetUriType() throws FHIRException { 1148 if (this.valueSet == null) 1149 return null; 1150 if (!(this.valueSet instanceof UriType)) 1151 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 1152 return (UriType) this.valueSet; 1153 } 1154 1155 public boolean hasValueSetUriType() { 1156 return this != null && this.valueSet instanceof UriType; 1157 } 1158 1159 /** 1160 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.) 1161 */ 1162 public Reference getValueSetReference() throws FHIRException { 1163 if (this.valueSet == null) 1164 return null; 1165 if (!(this.valueSet instanceof Reference)) 1166 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 1167 return (Reference) this.valueSet; 1168 } 1169 1170 public boolean hasValueSetReference() { 1171 return this != null && this.valueSet instanceof Reference; 1172 } 1173 1174 public boolean hasValueSet() { 1175 return this.valueSet != null && !this.valueSet.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.) 1180 */ 1181 public OperationDefinitionParameterBindingComponent setValueSet(Type value) throws FHIRFormatError { 1182 if (value != null && !(value instanceof UriType || value instanceof Reference)) 1183 throw new FHIRFormatError("Not the right type for OperationDefinition.parameter.binding.valueSet[x]: "+value.fhirType()); 1184 this.valueSet = value; 1185 return this; 1186 } 1187 1188 protected void listChildren(List<Property> children) { 1189 super.listChildren(children); 1190 children.add(new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength)); 1191 children.add(new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet)); 1192 } 1193 1194 @Override 1195 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1196 switch (_hash) { 1197 case 1791316033: /*strength*/ return new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength); 1198 case -1438410321: /*valueSet[x]*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet); 1199 case -1410174671: /*valueSet*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet); 1200 case -1438416261: /*valueSetUri*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet); 1201 case 295220506: /*valueSetReference*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 0, 1, valueSet); 1202 default: return super.getNamedProperty(_hash, _name, _checkValid); 1203 } 1204 1205 } 1206 1207 @Override 1208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1209 switch (hash) { 1210 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // Enumeration<BindingStrength> 1211 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // Type 1212 default: return super.getProperty(hash, name, checkValid); 1213 } 1214 1215 } 1216 1217 @Override 1218 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1219 switch (hash) { 1220 case 1791316033: // strength 1221 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1222 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1223 return value; 1224 case -1410174671: // valueSet 1225 this.valueSet = castToType(value); // Type 1226 return value; 1227 default: return super.setProperty(hash, name, value); 1228 } 1229 1230 } 1231 1232 @Override 1233 public Base setProperty(String name, Base value) throws FHIRException { 1234 if (name.equals("strength")) { 1235 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1236 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1237 } else if (name.equals("valueSet[x]")) { 1238 this.valueSet = castToType(value); // Type 1239 } else 1240 return super.setProperty(name, value); 1241 return value; 1242 } 1243 1244 @Override 1245 public Base makeProperty(int hash, String name) throws FHIRException { 1246 switch (hash) { 1247 case 1791316033: return getStrengthElement(); 1248 case -1438410321: return getValueSet(); 1249 case -1410174671: return getValueSet(); 1250 default: return super.makeProperty(hash, name); 1251 } 1252 1253 } 1254 1255 @Override 1256 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1257 switch (hash) { 1258 case 1791316033: /*strength*/ return new String[] {"code"}; 1259 case -1410174671: /*valueSet*/ return new String[] {"uri", "Reference"}; 1260 default: return super.getTypesForProperty(hash, name); 1261 } 1262 1263 } 1264 1265 @Override 1266 public Base addChild(String name) throws FHIRException { 1267 if (name.equals("strength")) { 1268 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.strength"); 1269 } 1270 else if (name.equals("valueSetUri")) { 1271 this.valueSet = new UriType(); 1272 return this.valueSet; 1273 } 1274 else if (name.equals("valueSetReference")) { 1275 this.valueSet = new Reference(); 1276 return this.valueSet; 1277 } 1278 else 1279 return super.addChild(name); 1280 } 1281 1282 public OperationDefinitionParameterBindingComponent copy() { 1283 OperationDefinitionParameterBindingComponent dst = new OperationDefinitionParameterBindingComponent(); 1284 copyValues(dst); 1285 dst.strength = strength == null ? null : strength.copy(); 1286 dst.valueSet = valueSet == null ? null : valueSet.copy(); 1287 return dst; 1288 } 1289 1290 @Override 1291 public boolean equalsDeep(Base other_) { 1292 if (!super.equalsDeep(other_)) 1293 return false; 1294 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1295 return false; 1296 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1297 return compareDeep(strength, o.strength, true) && compareDeep(valueSet, o.valueSet, true); 1298 } 1299 1300 @Override 1301 public boolean equalsShallow(Base other_) { 1302 if (!super.equalsShallow(other_)) 1303 return false; 1304 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1305 return false; 1306 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1307 return compareValues(strength, o.strength, true); 1308 } 1309 1310 public boolean isEmpty() { 1311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, valueSet); 1312 } 1313 1314 public String fhirType() { 1315 return "OperationDefinition.parameter.binding"; 1316 1317 } 1318 1319 } 1320 1321 @Block() 1322 public static class OperationDefinitionOverloadComponent extends BackboneElement implements IBaseBackboneElement { 1323 /** 1324 * Name of parameter to include in overload. 1325 */ 1326 @Child(name = "parameterName", type = {StringType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1327 @Description(shortDefinition="Name of parameter to include in overload", formalDefinition="Name of parameter to include in overload." ) 1328 protected List<StringType> parameterName; 1329 1330 /** 1331 * Comments to go on overload. 1332 */ 1333 @Child(name = "comment", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1334 @Description(shortDefinition="Comments to go on overload", formalDefinition="Comments to go on overload." ) 1335 protected StringType comment; 1336 1337 private static final long serialVersionUID = -907948545L; 1338 1339 /** 1340 * Constructor 1341 */ 1342 public OperationDefinitionOverloadComponent() { 1343 super(); 1344 } 1345 1346 /** 1347 * @return {@link #parameterName} (Name of parameter to include in overload.) 1348 */ 1349 public List<StringType> getParameterName() { 1350 if (this.parameterName == null) 1351 this.parameterName = new ArrayList<StringType>(); 1352 return this.parameterName; 1353 } 1354 1355 /** 1356 * @return Returns a reference to <code>this</code> for easy method chaining 1357 */ 1358 public OperationDefinitionOverloadComponent setParameterName(List<StringType> theParameterName) { 1359 this.parameterName = theParameterName; 1360 return this; 1361 } 1362 1363 public boolean hasParameterName() { 1364 if (this.parameterName == null) 1365 return false; 1366 for (StringType item : this.parameterName) 1367 if (!item.isEmpty()) 1368 return true; 1369 return false; 1370 } 1371 1372 /** 1373 * @return {@link #parameterName} (Name of parameter to include in overload.) 1374 */ 1375 public StringType addParameterNameElement() {//2 1376 StringType t = new StringType(); 1377 if (this.parameterName == null) 1378 this.parameterName = new ArrayList<StringType>(); 1379 this.parameterName.add(t); 1380 return t; 1381 } 1382 1383 /** 1384 * @param value {@link #parameterName} (Name of parameter to include in overload.) 1385 */ 1386 public OperationDefinitionOverloadComponent addParameterName(String value) { //1 1387 StringType t = new StringType(); 1388 t.setValue(value); 1389 if (this.parameterName == null) 1390 this.parameterName = new ArrayList<StringType>(); 1391 this.parameterName.add(t); 1392 return this; 1393 } 1394 1395 /** 1396 * @param value {@link #parameterName} (Name of parameter to include in overload.) 1397 */ 1398 public boolean hasParameterName(String value) { 1399 if (this.parameterName == null) 1400 return false; 1401 for (StringType v : this.parameterName) 1402 if (v.getValue().equals(value)) // string 1403 return true; 1404 return false; 1405 } 1406 1407 /** 1408 * @return {@link #comment} (Comments to go on overload.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1409 */ 1410 public StringType getCommentElement() { 1411 if (this.comment == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create OperationDefinitionOverloadComponent.comment"); 1414 else if (Configuration.doAutoCreate()) 1415 this.comment = new StringType(); // bb 1416 return this.comment; 1417 } 1418 1419 public boolean hasCommentElement() { 1420 return this.comment != null && !this.comment.isEmpty(); 1421 } 1422 1423 public boolean hasComment() { 1424 return this.comment != null && !this.comment.isEmpty(); 1425 } 1426 1427 /** 1428 * @param value {@link #comment} (Comments to go on overload.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1429 */ 1430 public OperationDefinitionOverloadComponent setCommentElement(StringType value) { 1431 this.comment = value; 1432 return this; 1433 } 1434 1435 /** 1436 * @return Comments to go on overload. 1437 */ 1438 public String getComment() { 1439 return this.comment == null ? null : this.comment.getValue(); 1440 } 1441 1442 /** 1443 * @param value Comments to go on overload. 1444 */ 1445 public OperationDefinitionOverloadComponent setComment(String value) { 1446 if (Utilities.noString(value)) 1447 this.comment = null; 1448 else { 1449 if (this.comment == null) 1450 this.comment = new StringType(); 1451 this.comment.setValue(value); 1452 } 1453 return this; 1454 } 1455 1456 protected void listChildren(List<Property> children) { 1457 super.listChildren(children); 1458 children.add(new Property("parameterName", "string", "Name of parameter to include in overload.", 0, java.lang.Integer.MAX_VALUE, parameterName)); 1459 children.add(new Property("comment", "string", "Comments to go on overload.", 0, 1, comment)); 1460 } 1461 1462 @Override 1463 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1464 switch (_hash) { 1465 case -379607596: /*parameterName*/ return new Property("parameterName", "string", "Name of parameter to include in overload.", 0, java.lang.Integer.MAX_VALUE, parameterName); 1466 case 950398559: /*comment*/ return new Property("comment", "string", "Comments to go on overload.", 0, 1, comment); 1467 default: return super.getNamedProperty(_hash, _name, _checkValid); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1474 switch (hash) { 1475 case -379607596: /*parameterName*/ return this.parameterName == null ? new Base[0] : this.parameterName.toArray(new Base[this.parameterName.size()]); // StringType 1476 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 1477 default: return super.getProperty(hash, name, checkValid); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1484 switch (hash) { 1485 case -379607596: // parameterName 1486 this.getParameterName().add(castToString(value)); // StringType 1487 return value; 1488 case 950398559: // comment 1489 this.comment = castToString(value); // StringType 1490 return value; 1491 default: return super.setProperty(hash, name, value); 1492 } 1493 1494 } 1495 1496 @Override 1497 public Base setProperty(String name, Base value) throws FHIRException { 1498 if (name.equals("parameterName")) { 1499 this.getParameterName().add(castToString(value)); 1500 } else if (name.equals("comment")) { 1501 this.comment = castToString(value); // StringType 1502 } else 1503 return super.setProperty(name, value); 1504 return value; 1505 } 1506 1507 @Override 1508 public Base makeProperty(int hash, String name) throws FHIRException { 1509 switch (hash) { 1510 case -379607596: return addParameterNameElement(); 1511 case 950398559: return getCommentElement(); 1512 default: return super.makeProperty(hash, name); 1513 } 1514 1515 } 1516 1517 @Override 1518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1519 switch (hash) { 1520 case -379607596: /*parameterName*/ return new String[] {"string"}; 1521 case 950398559: /*comment*/ return new String[] {"string"}; 1522 default: return super.getTypesForProperty(hash, name); 1523 } 1524 1525 } 1526 1527 @Override 1528 public Base addChild(String name) throws FHIRException { 1529 if (name.equals("parameterName")) { 1530 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameterName"); 1531 } 1532 else if (name.equals("comment")) { 1533 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 1534 } 1535 else 1536 return super.addChild(name); 1537 } 1538 1539 public OperationDefinitionOverloadComponent copy() { 1540 OperationDefinitionOverloadComponent dst = new OperationDefinitionOverloadComponent(); 1541 copyValues(dst); 1542 if (parameterName != null) { 1543 dst.parameterName = new ArrayList<StringType>(); 1544 for (StringType i : parameterName) 1545 dst.parameterName.add(i.copy()); 1546 }; 1547 dst.comment = comment == null ? null : comment.copy(); 1548 return dst; 1549 } 1550 1551 @Override 1552 public boolean equalsDeep(Base other_) { 1553 if (!super.equalsDeep(other_)) 1554 return false; 1555 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 1556 return false; 1557 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 1558 return compareDeep(parameterName, o.parameterName, true) && compareDeep(comment, o.comment, true) 1559 ; 1560 } 1561 1562 @Override 1563 public boolean equalsShallow(Base other_) { 1564 if (!super.equalsShallow(other_)) 1565 return false; 1566 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 1567 return false; 1568 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 1569 return compareValues(parameterName, o.parameterName, true) && compareValues(comment, o.comment, true) 1570 ; 1571 } 1572 1573 public boolean isEmpty() { 1574 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameterName, comment); 1575 } 1576 1577 public String fhirType() { 1578 return "OperationDefinition.overload"; 1579 1580 } 1581 1582 } 1583 1584 /** 1585 * Whether this is an operation or a named query. 1586 */ 1587 @Child(name = "kind", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=false) 1588 @Description(shortDefinition="operation | query", formalDefinition="Whether this is an operation or a named query." ) 1589 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-kind") 1590 protected Enumeration<OperationKind> kind; 1591 1592 /** 1593 * Explaination of why this operation definition is needed and why it has been designed as it has. 1594 */ 1595 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1596 @Description(shortDefinition="Why this operation definition is defined", formalDefinition="Explaination of why this operation definition is needed and why it has been designed as it has." ) 1597 protected MarkdownType purpose; 1598 1599 /** 1600 * Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST. 1601 */ 1602 @Child(name = "idempotent", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1603 @Description(shortDefinition="Whether content is unchanged by the operation", formalDefinition="Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST." ) 1604 protected BooleanType idempotent; 1605 1606 /** 1607 * The name used to invoke the operation. 1608 */ 1609 @Child(name = "code", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1610 @Description(shortDefinition="Name used to invoke the operation", formalDefinition="The name used to invoke the operation." ) 1611 protected CodeType code; 1612 1613 /** 1614 * Additional information about how to use this operation or named query. 1615 */ 1616 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1617 @Description(shortDefinition="Additional information about use", formalDefinition="Additional information about how to use this operation or named query." ) 1618 protected StringType comment; 1619 1620 /** 1621 * Indicates that this operation definition is a constraining profile on the base. 1622 */ 1623 @Child(name = "base", type = {OperationDefinition.class}, order=5, min=0, max=1, modifier=false, summary=true) 1624 @Description(shortDefinition="Marks this as a profile of the base", formalDefinition="Indicates that this operation definition is a constraining profile on the base." ) 1625 protected Reference base; 1626 1627 /** 1628 * The actual object that is the target of the reference (Indicates that this operation definition is a constraining profile on the base.) 1629 */ 1630 protected OperationDefinition baseTarget; 1631 1632 /** 1633 * The types on which this operation can be executed. 1634 */ 1635 @Child(name = "resource", type = {CodeType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1636 @Description(shortDefinition="Types this operation applies to", formalDefinition="The types on which this operation can be executed." ) 1637 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 1638 protected List<CodeType> resource; 1639 1640 /** 1641 * Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 1642 */ 1643 @Child(name = "system", type = {BooleanType.class}, order=7, min=1, max=1, modifier=false, summary=true) 1644 @Description(shortDefinition="Invoke at the system level?", formalDefinition="Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context)." ) 1645 protected BooleanType system; 1646 1647 /** 1648 * Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 1649 */ 1650 @Child(name = "type", type = {BooleanType.class}, order=8, min=1, max=1, modifier=false, summary=true) 1651 @Description(shortDefinition="Invole at the type level?", formalDefinition="Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context)." ) 1652 protected BooleanType type; 1653 1654 /** 1655 * Indicates whether this operation can be invoked on a particular instance of one of the given types. 1656 */ 1657 @Child(name = "instance", type = {BooleanType.class}, order=9, min=1, max=1, modifier=false, summary=true) 1658 @Description(shortDefinition="Invoke on an instance?", formalDefinition="Indicates whether this operation can be invoked on a particular instance of one of the given types." ) 1659 protected BooleanType instance; 1660 1661 /** 1662 * The parameters for the operation/query. 1663 */ 1664 @Child(name = "parameter", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1665 @Description(shortDefinition="Parameters for the operation/query", formalDefinition="The parameters for the operation/query." ) 1666 protected List<OperationDefinitionParameterComponent> parameter; 1667 1668 /** 1669 * Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation. 1670 */ 1671 @Child(name = "overload", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1672 @Description(shortDefinition="Define overloaded variants for when generating code", formalDefinition="Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation." ) 1673 protected List<OperationDefinitionOverloadComponent> overload; 1674 1675 private static final long serialVersionUID = 1292377899L; 1676 1677 /** 1678 * Constructor 1679 */ 1680 public OperationDefinition() { 1681 super(); 1682 } 1683 1684 /** 1685 * Constructor 1686 */ 1687 public OperationDefinition(StringType name, Enumeration<PublicationStatus> status, Enumeration<OperationKind> kind, CodeType code, BooleanType system, BooleanType type, BooleanType instance) { 1688 super(); 1689 this.name = name; 1690 this.status = status; 1691 this.kind = kind; 1692 this.code = code; 1693 this.system = system; 1694 this.type = type; 1695 this.instance = instance; 1696 } 1697 1698 /** 1699 * @return {@link #url} (An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1700 */ 1701 public UriType getUrlElement() { 1702 if (this.url == null) 1703 if (Configuration.errorOnAutoCreate()) 1704 throw new Error("Attempt to auto-create OperationDefinition.url"); 1705 else if (Configuration.doAutoCreate()) 1706 this.url = new UriType(); // bb 1707 return this.url; 1708 } 1709 1710 public boolean hasUrlElement() { 1711 return this.url != null && !this.url.isEmpty(); 1712 } 1713 1714 public boolean hasUrl() { 1715 return this.url != null && !this.url.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #url} (An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1720 */ 1721 public OperationDefinition setUrlElement(UriType value) { 1722 this.url = value; 1723 return this; 1724 } 1725 1726 /** 1727 * @return An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions). 1728 */ 1729 public String getUrl() { 1730 return this.url == null ? null : this.url.getValue(); 1731 } 1732 1733 /** 1734 * @param value An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions). 1735 */ 1736 public OperationDefinition setUrl(String value) { 1737 if (Utilities.noString(value)) 1738 this.url = null; 1739 else { 1740 if (this.url == null) 1741 this.url = new UriType(); 1742 this.url.setValue(value); 1743 } 1744 return this; 1745 } 1746 1747 /** 1748 * @return {@link #version} (The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1749 */ 1750 public StringType getVersionElement() { 1751 if (this.version == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create OperationDefinition.version"); 1754 else if (Configuration.doAutoCreate()) 1755 this.version = new StringType(); // bb 1756 return this.version; 1757 } 1758 1759 public boolean hasVersionElement() { 1760 return this.version != null && !this.version.isEmpty(); 1761 } 1762 1763 public boolean hasVersion() { 1764 return this.version != null && !this.version.isEmpty(); 1765 } 1766 1767 /** 1768 * @param value {@link #version} (The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1769 */ 1770 public OperationDefinition setVersionElement(StringType value) { 1771 this.version = value; 1772 return this; 1773 } 1774 1775 /** 1776 * @return The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1777 */ 1778 public String getVersion() { 1779 return this.version == null ? null : this.version.getValue(); 1780 } 1781 1782 /** 1783 * @param value The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1784 */ 1785 public OperationDefinition setVersion(String value) { 1786 if (Utilities.noString(value)) 1787 this.version = null; 1788 else { 1789 if (this.version == null) 1790 this.version = new StringType(); 1791 this.version.setValue(value); 1792 } 1793 return this; 1794 } 1795 1796 /** 1797 * @return {@link #name} (A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1798 */ 1799 public StringType getNameElement() { 1800 if (this.name == null) 1801 if (Configuration.errorOnAutoCreate()) 1802 throw new Error("Attempt to auto-create OperationDefinition.name"); 1803 else if (Configuration.doAutoCreate()) 1804 this.name = new StringType(); // bb 1805 return this.name; 1806 } 1807 1808 public boolean hasNameElement() { 1809 return this.name != null && !this.name.isEmpty(); 1810 } 1811 1812 public boolean hasName() { 1813 return this.name != null && !this.name.isEmpty(); 1814 } 1815 1816 /** 1817 * @param value {@link #name} (A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1818 */ 1819 public OperationDefinition setNameElement(StringType value) { 1820 this.name = value; 1821 return this; 1822 } 1823 1824 /** 1825 * @return A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1826 */ 1827 public String getName() { 1828 return this.name == null ? null : this.name.getValue(); 1829 } 1830 1831 /** 1832 * @param value A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1833 */ 1834 public OperationDefinition setName(String value) { 1835 if (this.name == null) 1836 this.name = new StringType(); 1837 this.name.setValue(value); 1838 return this; 1839 } 1840 1841 /** 1842 * @return {@link #status} (The status of this operation definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1843 */ 1844 public Enumeration<PublicationStatus> getStatusElement() { 1845 if (this.status == null) 1846 if (Configuration.errorOnAutoCreate()) 1847 throw new Error("Attempt to auto-create OperationDefinition.status"); 1848 else if (Configuration.doAutoCreate()) 1849 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1850 return this.status; 1851 } 1852 1853 public boolean hasStatusElement() { 1854 return this.status != null && !this.status.isEmpty(); 1855 } 1856 1857 public boolean hasStatus() { 1858 return this.status != null && !this.status.isEmpty(); 1859 } 1860 1861 /** 1862 * @param value {@link #status} (The status of this operation definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1863 */ 1864 public OperationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1865 this.status = value; 1866 return this; 1867 } 1868 1869 /** 1870 * @return The status of this operation definition. Enables tracking the life-cycle of the content. 1871 */ 1872 public PublicationStatus getStatus() { 1873 return this.status == null ? null : this.status.getValue(); 1874 } 1875 1876 /** 1877 * @param value The status of this operation definition. Enables tracking the life-cycle of the content. 1878 */ 1879 public OperationDefinition setStatus(PublicationStatus value) { 1880 if (this.status == null) 1881 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1882 this.status.setValue(value); 1883 return this; 1884 } 1885 1886 /** 1887 * @return {@link #kind} (Whether this is an operation or a named query.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1888 */ 1889 public Enumeration<OperationKind> getKindElement() { 1890 if (this.kind == null) 1891 if (Configuration.errorOnAutoCreate()) 1892 throw new Error("Attempt to auto-create OperationDefinition.kind"); 1893 else if (Configuration.doAutoCreate()) 1894 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); // bb 1895 return this.kind; 1896 } 1897 1898 public boolean hasKindElement() { 1899 return this.kind != null && !this.kind.isEmpty(); 1900 } 1901 1902 public boolean hasKind() { 1903 return this.kind != null && !this.kind.isEmpty(); 1904 } 1905 1906 /** 1907 * @param value {@link #kind} (Whether this is an operation or a named query.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1908 */ 1909 public OperationDefinition setKindElement(Enumeration<OperationKind> value) { 1910 this.kind = value; 1911 return this; 1912 } 1913 1914 /** 1915 * @return Whether this is an operation or a named query. 1916 */ 1917 public OperationKind getKind() { 1918 return this.kind == null ? null : this.kind.getValue(); 1919 } 1920 1921 /** 1922 * @param value Whether this is an operation or a named query. 1923 */ 1924 public OperationDefinition setKind(OperationKind value) { 1925 if (this.kind == null) 1926 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); 1927 this.kind.setValue(value); 1928 return this; 1929 } 1930 1931 /** 1932 * @return {@link #experimental} (A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1933 */ 1934 public BooleanType getExperimentalElement() { 1935 if (this.experimental == null) 1936 if (Configuration.errorOnAutoCreate()) 1937 throw new Error("Attempt to auto-create OperationDefinition.experimental"); 1938 else if (Configuration.doAutoCreate()) 1939 this.experimental = new BooleanType(); // bb 1940 return this.experimental; 1941 } 1942 1943 public boolean hasExperimentalElement() { 1944 return this.experimental != null && !this.experimental.isEmpty(); 1945 } 1946 1947 public boolean hasExperimental() { 1948 return this.experimental != null && !this.experimental.isEmpty(); 1949 } 1950 1951 /** 1952 * @param value {@link #experimental} (A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1953 */ 1954 public OperationDefinition setExperimentalElement(BooleanType value) { 1955 this.experimental = value; 1956 return this; 1957 } 1958 1959 /** 1960 * @return A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1961 */ 1962 public boolean getExperimental() { 1963 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1964 } 1965 1966 /** 1967 * @param value A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1968 */ 1969 public OperationDefinition setExperimental(boolean value) { 1970 if (this.experimental == null) 1971 this.experimental = new BooleanType(); 1972 this.experimental.setValue(value); 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #date} (The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1978 */ 1979 public DateTimeType getDateElement() { 1980 if (this.date == null) 1981 if (Configuration.errorOnAutoCreate()) 1982 throw new Error("Attempt to auto-create OperationDefinition.date"); 1983 else if (Configuration.doAutoCreate()) 1984 this.date = new DateTimeType(); // bb 1985 return this.date; 1986 } 1987 1988 public boolean hasDateElement() { 1989 return this.date != null && !this.date.isEmpty(); 1990 } 1991 1992 public boolean hasDate() { 1993 return this.date != null && !this.date.isEmpty(); 1994 } 1995 1996 /** 1997 * @param value {@link #date} (The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1998 */ 1999 public OperationDefinition setDateElement(DateTimeType value) { 2000 this.date = value; 2001 return this; 2002 } 2003 2004 /** 2005 * @return The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes. 2006 */ 2007 public Date getDate() { 2008 return this.date == null ? null : this.date.getValue(); 2009 } 2010 2011 /** 2012 * @param value The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes. 2013 */ 2014 public OperationDefinition setDate(Date value) { 2015 if (value == null) 2016 this.date = null; 2017 else { 2018 if (this.date == null) 2019 this.date = new DateTimeType(); 2020 this.date.setValue(value); 2021 } 2022 return this; 2023 } 2024 2025 /** 2026 * @return {@link #publisher} (The name of the individual or organization that published the operation definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2027 */ 2028 public StringType getPublisherElement() { 2029 if (this.publisher == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create OperationDefinition.publisher"); 2032 else if (Configuration.doAutoCreate()) 2033 this.publisher = new StringType(); // bb 2034 return this.publisher; 2035 } 2036 2037 public boolean hasPublisherElement() { 2038 return this.publisher != null && !this.publisher.isEmpty(); 2039 } 2040 2041 public boolean hasPublisher() { 2042 return this.publisher != null && !this.publisher.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #publisher} (The name of the individual or organization that published the operation definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2047 */ 2048 public OperationDefinition setPublisherElement(StringType value) { 2049 this.publisher = value; 2050 return this; 2051 } 2052 2053 /** 2054 * @return The name of the individual or organization that published the operation definition. 2055 */ 2056 public String getPublisher() { 2057 return this.publisher == null ? null : this.publisher.getValue(); 2058 } 2059 2060 /** 2061 * @param value The name of the individual or organization that published the operation definition. 2062 */ 2063 public OperationDefinition setPublisher(String value) { 2064 if (Utilities.noString(value)) 2065 this.publisher = null; 2066 else { 2067 if (this.publisher == null) 2068 this.publisher = new StringType(); 2069 this.publisher.setValue(value); 2070 } 2071 return this; 2072 } 2073 2074 /** 2075 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2076 */ 2077 public List<ContactDetail> getContact() { 2078 if (this.contact == null) 2079 this.contact = new ArrayList<ContactDetail>(); 2080 return this.contact; 2081 } 2082 2083 /** 2084 * @return Returns a reference to <code>this</code> for easy method chaining 2085 */ 2086 public OperationDefinition setContact(List<ContactDetail> theContact) { 2087 this.contact = theContact; 2088 return this; 2089 } 2090 2091 public boolean hasContact() { 2092 if (this.contact == null) 2093 return false; 2094 for (ContactDetail item : this.contact) 2095 if (!item.isEmpty()) 2096 return true; 2097 return false; 2098 } 2099 2100 public ContactDetail addContact() { //3 2101 ContactDetail t = new ContactDetail(); 2102 if (this.contact == null) 2103 this.contact = new ArrayList<ContactDetail>(); 2104 this.contact.add(t); 2105 return t; 2106 } 2107 2108 public OperationDefinition addContact(ContactDetail t) { //3 2109 if (t == null) 2110 return this; 2111 if (this.contact == null) 2112 this.contact = new ArrayList<ContactDetail>(); 2113 this.contact.add(t); 2114 return this; 2115 } 2116 2117 /** 2118 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2119 */ 2120 public ContactDetail getContactFirstRep() { 2121 if (getContact().isEmpty()) { 2122 addContact(); 2123 } 2124 return getContact().get(0); 2125 } 2126 2127 /** 2128 * @return {@link #description} (A free text natural language description of the operation definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2129 */ 2130 public MarkdownType getDescriptionElement() { 2131 if (this.description == null) 2132 if (Configuration.errorOnAutoCreate()) 2133 throw new Error("Attempt to auto-create OperationDefinition.description"); 2134 else if (Configuration.doAutoCreate()) 2135 this.description = new MarkdownType(); // bb 2136 return this.description; 2137 } 2138 2139 public boolean hasDescriptionElement() { 2140 return this.description != null && !this.description.isEmpty(); 2141 } 2142 2143 public boolean hasDescription() { 2144 return this.description != null && !this.description.isEmpty(); 2145 } 2146 2147 /** 2148 * @param value {@link #description} (A free text natural language description of the operation definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2149 */ 2150 public OperationDefinition setDescriptionElement(MarkdownType value) { 2151 this.description = value; 2152 return this; 2153 } 2154 2155 /** 2156 * @return A free text natural language description of the operation definition from a consumer's perspective. 2157 */ 2158 public String getDescription() { 2159 return this.description == null ? null : this.description.getValue(); 2160 } 2161 2162 /** 2163 * @param value A free text natural language description of the operation definition from a consumer's perspective. 2164 */ 2165 public OperationDefinition setDescription(String value) { 2166 if (value == null) 2167 this.description = null; 2168 else { 2169 if (this.description == null) 2170 this.description = new MarkdownType(); 2171 this.description.setValue(value); 2172 } 2173 return this; 2174 } 2175 2176 /** 2177 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate operation definition instances.) 2178 */ 2179 public List<UsageContext> getUseContext() { 2180 if (this.useContext == null) 2181 this.useContext = new ArrayList<UsageContext>(); 2182 return this.useContext; 2183 } 2184 2185 /** 2186 * @return Returns a reference to <code>this</code> for easy method chaining 2187 */ 2188 public OperationDefinition setUseContext(List<UsageContext> theUseContext) { 2189 this.useContext = theUseContext; 2190 return this; 2191 } 2192 2193 public boolean hasUseContext() { 2194 if (this.useContext == null) 2195 return false; 2196 for (UsageContext item : this.useContext) 2197 if (!item.isEmpty()) 2198 return true; 2199 return false; 2200 } 2201 2202 public UsageContext addUseContext() { //3 2203 UsageContext t = new UsageContext(); 2204 if (this.useContext == null) 2205 this.useContext = new ArrayList<UsageContext>(); 2206 this.useContext.add(t); 2207 return t; 2208 } 2209 2210 public OperationDefinition addUseContext(UsageContext t) { //3 2211 if (t == null) 2212 return this; 2213 if (this.useContext == null) 2214 this.useContext = new ArrayList<UsageContext>(); 2215 this.useContext.add(t); 2216 return this; 2217 } 2218 2219 /** 2220 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2221 */ 2222 public UsageContext getUseContextFirstRep() { 2223 if (getUseContext().isEmpty()) { 2224 addUseContext(); 2225 } 2226 return getUseContext().get(0); 2227 } 2228 2229 /** 2230 * @return {@link #jurisdiction} (A legal or geographic region in which the operation definition is intended to be used.) 2231 */ 2232 public List<CodeableConcept> getJurisdiction() { 2233 if (this.jurisdiction == null) 2234 this.jurisdiction = new ArrayList<CodeableConcept>(); 2235 return this.jurisdiction; 2236 } 2237 2238 /** 2239 * @return Returns a reference to <code>this</code> for easy method chaining 2240 */ 2241 public OperationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2242 this.jurisdiction = theJurisdiction; 2243 return this; 2244 } 2245 2246 public boolean hasJurisdiction() { 2247 if (this.jurisdiction == null) 2248 return false; 2249 for (CodeableConcept item : this.jurisdiction) 2250 if (!item.isEmpty()) 2251 return true; 2252 return false; 2253 } 2254 2255 public CodeableConcept addJurisdiction() { //3 2256 CodeableConcept t = new CodeableConcept(); 2257 if (this.jurisdiction == null) 2258 this.jurisdiction = new ArrayList<CodeableConcept>(); 2259 this.jurisdiction.add(t); 2260 return t; 2261 } 2262 2263 public OperationDefinition addJurisdiction(CodeableConcept t) { //3 2264 if (t == null) 2265 return this; 2266 if (this.jurisdiction == null) 2267 this.jurisdiction = new ArrayList<CodeableConcept>(); 2268 this.jurisdiction.add(t); 2269 return this; 2270 } 2271 2272 /** 2273 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2274 */ 2275 public CodeableConcept getJurisdictionFirstRep() { 2276 if (getJurisdiction().isEmpty()) { 2277 addJurisdiction(); 2278 } 2279 return getJurisdiction().get(0); 2280 } 2281 2282 /** 2283 * @return {@link #purpose} (Explaination of why this operation definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2284 */ 2285 public MarkdownType getPurposeElement() { 2286 if (this.purpose == null) 2287 if (Configuration.errorOnAutoCreate()) 2288 throw new Error("Attempt to auto-create OperationDefinition.purpose"); 2289 else if (Configuration.doAutoCreate()) 2290 this.purpose = new MarkdownType(); // bb 2291 return this.purpose; 2292 } 2293 2294 public boolean hasPurposeElement() { 2295 return this.purpose != null && !this.purpose.isEmpty(); 2296 } 2297 2298 public boolean hasPurpose() { 2299 return this.purpose != null && !this.purpose.isEmpty(); 2300 } 2301 2302 /** 2303 * @param value {@link #purpose} (Explaination of why this operation definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2304 */ 2305 public OperationDefinition setPurposeElement(MarkdownType value) { 2306 this.purpose = value; 2307 return this; 2308 } 2309 2310 /** 2311 * @return Explaination of why this operation definition is needed and why it has been designed as it has. 2312 */ 2313 public String getPurpose() { 2314 return this.purpose == null ? null : this.purpose.getValue(); 2315 } 2316 2317 /** 2318 * @param value Explaination of why this operation definition is needed and why it has been designed as it has. 2319 */ 2320 public OperationDefinition setPurpose(String value) { 2321 if (value == null) 2322 this.purpose = null; 2323 else { 2324 if (this.purpose == null) 2325 this.purpose = new MarkdownType(); 2326 this.purpose.setValue(value); 2327 } 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #idempotent} (Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.). This is the underlying object with id, value and extensions. The accessor "getIdempotent" gives direct access to the value 2333 */ 2334 public BooleanType getIdempotentElement() { 2335 if (this.idempotent == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create OperationDefinition.idempotent"); 2338 else if (Configuration.doAutoCreate()) 2339 this.idempotent = new BooleanType(); // bb 2340 return this.idempotent; 2341 } 2342 2343 public boolean hasIdempotentElement() { 2344 return this.idempotent != null && !this.idempotent.isEmpty(); 2345 } 2346 2347 public boolean hasIdempotent() { 2348 return this.idempotent != null && !this.idempotent.isEmpty(); 2349 } 2350 2351 /** 2352 * @param value {@link #idempotent} (Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.). This is the underlying object with id, value and extensions. The accessor "getIdempotent" gives direct access to the value 2353 */ 2354 public OperationDefinition setIdempotentElement(BooleanType value) { 2355 this.idempotent = value; 2356 return this; 2357 } 2358 2359 /** 2360 * @return Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST. 2361 */ 2362 public boolean getIdempotent() { 2363 return this.idempotent == null || this.idempotent.isEmpty() ? false : this.idempotent.getValue(); 2364 } 2365 2366 /** 2367 * @param value Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST. 2368 */ 2369 public OperationDefinition setIdempotent(boolean value) { 2370 if (this.idempotent == null) 2371 this.idempotent = new BooleanType(); 2372 this.idempotent.setValue(value); 2373 return this; 2374 } 2375 2376 /** 2377 * @return {@link #code} (The name used to invoke the operation.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2378 */ 2379 public CodeType getCodeElement() { 2380 if (this.code == null) 2381 if (Configuration.errorOnAutoCreate()) 2382 throw new Error("Attempt to auto-create OperationDefinition.code"); 2383 else if (Configuration.doAutoCreate()) 2384 this.code = new CodeType(); // bb 2385 return this.code; 2386 } 2387 2388 public boolean hasCodeElement() { 2389 return this.code != null && !this.code.isEmpty(); 2390 } 2391 2392 public boolean hasCode() { 2393 return this.code != null && !this.code.isEmpty(); 2394 } 2395 2396 /** 2397 * @param value {@link #code} (The name used to invoke the operation.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2398 */ 2399 public OperationDefinition setCodeElement(CodeType value) { 2400 this.code = value; 2401 return this; 2402 } 2403 2404 /** 2405 * @return The name used to invoke the operation. 2406 */ 2407 public String getCode() { 2408 return this.code == null ? null : this.code.getValue(); 2409 } 2410 2411 /** 2412 * @param value The name used to invoke the operation. 2413 */ 2414 public OperationDefinition setCode(String value) { 2415 if (this.code == null) 2416 this.code = new CodeType(); 2417 this.code.setValue(value); 2418 return this; 2419 } 2420 2421 /** 2422 * @return {@link #comment} (Additional information about how to use this operation or named query.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2423 */ 2424 public StringType getCommentElement() { 2425 if (this.comment == null) 2426 if (Configuration.errorOnAutoCreate()) 2427 throw new Error("Attempt to auto-create OperationDefinition.comment"); 2428 else if (Configuration.doAutoCreate()) 2429 this.comment = new StringType(); // bb 2430 return this.comment; 2431 } 2432 2433 public boolean hasCommentElement() { 2434 return this.comment != null && !this.comment.isEmpty(); 2435 } 2436 2437 public boolean hasComment() { 2438 return this.comment != null && !this.comment.isEmpty(); 2439 } 2440 2441 /** 2442 * @param value {@link #comment} (Additional information about how to use this operation or named query.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2443 */ 2444 public OperationDefinition setCommentElement(StringType value) { 2445 this.comment = value; 2446 return this; 2447 } 2448 2449 /** 2450 * @return Additional information about how to use this operation or named query. 2451 */ 2452 public String getComment() { 2453 return this.comment == null ? null : this.comment.getValue(); 2454 } 2455 2456 /** 2457 * @param value Additional information about how to use this operation or named query. 2458 */ 2459 public OperationDefinition setComment(String value) { 2460 if (Utilities.noString(value)) 2461 this.comment = null; 2462 else { 2463 if (this.comment == null) 2464 this.comment = new StringType(); 2465 this.comment.setValue(value); 2466 } 2467 return this; 2468 } 2469 2470 /** 2471 * @return {@link #base} (Indicates that this operation definition is a constraining profile on the base.) 2472 */ 2473 public Reference getBase() { 2474 if (this.base == null) 2475 if (Configuration.errorOnAutoCreate()) 2476 throw new Error("Attempt to auto-create OperationDefinition.base"); 2477 else if (Configuration.doAutoCreate()) 2478 this.base = new Reference(); // cc 2479 return this.base; 2480 } 2481 2482 public boolean hasBase() { 2483 return this.base != null && !this.base.isEmpty(); 2484 } 2485 2486 /** 2487 * @param value {@link #base} (Indicates that this operation definition is a constraining profile on the base.) 2488 */ 2489 public OperationDefinition setBase(Reference value) { 2490 this.base = value; 2491 return this; 2492 } 2493 2494 /** 2495 * @return {@link #base} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates that this operation definition is a constraining profile on the base.) 2496 */ 2497 public OperationDefinition getBaseTarget() { 2498 if (this.baseTarget == null) 2499 if (Configuration.errorOnAutoCreate()) 2500 throw new Error("Attempt to auto-create OperationDefinition.base"); 2501 else if (Configuration.doAutoCreate()) 2502 this.baseTarget = new OperationDefinition(); // aa 2503 return this.baseTarget; 2504 } 2505 2506 /** 2507 * @param value {@link #base} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates that this operation definition is a constraining profile on the base.) 2508 */ 2509 public OperationDefinition setBaseTarget(OperationDefinition value) { 2510 this.baseTarget = value; 2511 return this; 2512 } 2513 2514 /** 2515 * @return {@link #resource} (The types on which this operation can be executed.) 2516 */ 2517 public List<CodeType> getResource() { 2518 if (this.resource == null) 2519 this.resource = new ArrayList<CodeType>(); 2520 return this.resource; 2521 } 2522 2523 /** 2524 * @return Returns a reference to <code>this</code> for easy method chaining 2525 */ 2526 public OperationDefinition setResource(List<CodeType> theResource) { 2527 this.resource = theResource; 2528 return this; 2529 } 2530 2531 public boolean hasResource() { 2532 if (this.resource == null) 2533 return false; 2534 for (CodeType item : this.resource) 2535 if (!item.isEmpty()) 2536 return true; 2537 return false; 2538 } 2539 2540 /** 2541 * @return {@link #resource} (The types on which this operation can be executed.) 2542 */ 2543 public CodeType addResourceElement() {//2 2544 CodeType t = new CodeType(); 2545 if (this.resource == null) 2546 this.resource = new ArrayList<CodeType>(); 2547 this.resource.add(t); 2548 return t; 2549 } 2550 2551 /** 2552 * @param value {@link #resource} (The types on which this operation can be executed.) 2553 */ 2554 public OperationDefinition addResource(String value) { //1 2555 CodeType t = new CodeType(); 2556 t.setValue(value); 2557 if (this.resource == null) 2558 this.resource = new ArrayList<CodeType>(); 2559 this.resource.add(t); 2560 return this; 2561 } 2562 2563 /** 2564 * @param value {@link #resource} (The types on which this operation can be executed.) 2565 */ 2566 public boolean hasResource(String value) { 2567 if (this.resource == null) 2568 return false; 2569 for (CodeType v : this.resource) 2570 if (v.getValue().equals(value)) // code 2571 return true; 2572 return false; 2573 } 2574 2575 /** 2576 * @return {@link #system} (Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 2577 */ 2578 public BooleanType getSystemElement() { 2579 if (this.system == null) 2580 if (Configuration.errorOnAutoCreate()) 2581 throw new Error("Attempt to auto-create OperationDefinition.system"); 2582 else if (Configuration.doAutoCreate()) 2583 this.system = new BooleanType(); // bb 2584 return this.system; 2585 } 2586 2587 public boolean hasSystemElement() { 2588 return this.system != null && !this.system.isEmpty(); 2589 } 2590 2591 public boolean hasSystem() { 2592 return this.system != null && !this.system.isEmpty(); 2593 } 2594 2595 /** 2596 * @param value {@link #system} (Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 2597 */ 2598 public OperationDefinition setSystemElement(BooleanType value) { 2599 this.system = value; 2600 return this; 2601 } 2602 2603 /** 2604 * @return Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 2605 */ 2606 public boolean getSystem() { 2607 return this.system == null || this.system.isEmpty() ? false : this.system.getValue(); 2608 } 2609 2610 /** 2611 * @param value Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context). 2612 */ 2613 public OperationDefinition setSystem(boolean value) { 2614 if (this.system == null) 2615 this.system = new BooleanType(); 2616 this.system.setValue(value); 2617 return this; 2618 } 2619 2620 /** 2621 * @return {@link #type} (Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2622 */ 2623 public BooleanType getTypeElement() { 2624 if (this.type == null) 2625 if (Configuration.errorOnAutoCreate()) 2626 throw new Error("Attempt to auto-create OperationDefinition.type"); 2627 else if (Configuration.doAutoCreate()) 2628 this.type = new BooleanType(); // bb 2629 return this.type; 2630 } 2631 2632 public boolean hasTypeElement() { 2633 return this.type != null && !this.type.isEmpty(); 2634 } 2635 2636 public boolean hasType() { 2637 return this.type != null && !this.type.isEmpty(); 2638 } 2639 2640 /** 2641 * @param value {@link #type} (Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2642 */ 2643 public OperationDefinition setTypeElement(BooleanType value) { 2644 this.type = value; 2645 return this; 2646 } 2647 2648 /** 2649 * @return Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 2650 */ 2651 public boolean getType() { 2652 return this.type == null || this.type.isEmpty() ? false : this.type.getValue(); 2653 } 2654 2655 /** 2656 * @param value Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context). 2657 */ 2658 public OperationDefinition setType(boolean value) { 2659 if (this.type == null) 2660 this.type = new BooleanType(); 2661 this.type.setValue(value); 2662 return this; 2663 } 2664 2665 /** 2666 * @return {@link #instance} (Indicates whether this operation can be invoked on a particular instance of one of the given types.). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 2667 */ 2668 public BooleanType getInstanceElement() { 2669 if (this.instance == null) 2670 if (Configuration.errorOnAutoCreate()) 2671 throw new Error("Attempt to auto-create OperationDefinition.instance"); 2672 else if (Configuration.doAutoCreate()) 2673 this.instance = new BooleanType(); // bb 2674 return this.instance; 2675 } 2676 2677 public boolean hasInstanceElement() { 2678 return this.instance != null && !this.instance.isEmpty(); 2679 } 2680 2681 public boolean hasInstance() { 2682 return this.instance != null && !this.instance.isEmpty(); 2683 } 2684 2685 /** 2686 * @param value {@link #instance} (Indicates whether this operation can be invoked on a particular instance of one of the given types.). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 2687 */ 2688 public OperationDefinition setInstanceElement(BooleanType value) { 2689 this.instance = value; 2690 return this; 2691 } 2692 2693 /** 2694 * @return Indicates whether this operation can be invoked on a particular instance of one of the given types. 2695 */ 2696 public boolean getInstance() { 2697 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 2698 } 2699 2700 /** 2701 * @param value Indicates whether this operation can be invoked on a particular instance of one of the given types. 2702 */ 2703 public OperationDefinition setInstance(boolean value) { 2704 if (this.instance == null) 2705 this.instance = new BooleanType(); 2706 this.instance.setValue(value); 2707 return this; 2708 } 2709 2710 /** 2711 * @return {@link #parameter} (The parameters for the operation/query.) 2712 */ 2713 public List<OperationDefinitionParameterComponent> getParameter() { 2714 if (this.parameter == null) 2715 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2716 return this.parameter; 2717 } 2718 2719 /** 2720 * @return Returns a reference to <code>this</code> for easy method chaining 2721 */ 2722 public OperationDefinition setParameter(List<OperationDefinitionParameterComponent> theParameter) { 2723 this.parameter = theParameter; 2724 return this; 2725 } 2726 2727 public boolean hasParameter() { 2728 if (this.parameter == null) 2729 return false; 2730 for (OperationDefinitionParameterComponent item : this.parameter) 2731 if (!item.isEmpty()) 2732 return true; 2733 return false; 2734 } 2735 2736 public OperationDefinitionParameterComponent addParameter() { //3 2737 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 2738 if (this.parameter == null) 2739 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2740 this.parameter.add(t); 2741 return t; 2742 } 2743 2744 public OperationDefinition addParameter(OperationDefinitionParameterComponent t) { //3 2745 if (t == null) 2746 return this; 2747 if (this.parameter == null) 2748 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2749 this.parameter.add(t); 2750 return this; 2751 } 2752 2753 /** 2754 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist 2755 */ 2756 public OperationDefinitionParameterComponent getParameterFirstRep() { 2757 if (getParameter().isEmpty()) { 2758 addParameter(); 2759 } 2760 return getParameter().get(0); 2761 } 2762 2763 /** 2764 * @return {@link #overload} (Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.) 2765 */ 2766 public List<OperationDefinitionOverloadComponent> getOverload() { 2767 if (this.overload == null) 2768 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 2769 return this.overload; 2770 } 2771 2772 /** 2773 * @return Returns a reference to <code>this</code> for easy method chaining 2774 */ 2775 public OperationDefinition setOverload(List<OperationDefinitionOverloadComponent> theOverload) { 2776 this.overload = theOverload; 2777 return this; 2778 } 2779 2780 public boolean hasOverload() { 2781 if (this.overload == null) 2782 return false; 2783 for (OperationDefinitionOverloadComponent item : this.overload) 2784 if (!item.isEmpty()) 2785 return true; 2786 return false; 2787 } 2788 2789 public OperationDefinitionOverloadComponent addOverload() { //3 2790 OperationDefinitionOverloadComponent t = new OperationDefinitionOverloadComponent(); 2791 if (this.overload == null) 2792 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 2793 this.overload.add(t); 2794 return t; 2795 } 2796 2797 public OperationDefinition addOverload(OperationDefinitionOverloadComponent t) { //3 2798 if (t == null) 2799 return this; 2800 if (this.overload == null) 2801 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 2802 this.overload.add(t); 2803 return this; 2804 } 2805 2806 /** 2807 * @return The first repetition of repeating field {@link #overload}, creating it if it does not already exist 2808 */ 2809 public OperationDefinitionOverloadComponent getOverloadFirstRep() { 2810 if (getOverload().isEmpty()) { 2811 addOverload(); 2812 } 2813 return getOverload().get(0); 2814 } 2815 2816 protected void listChildren(List<Property> children) { 2817 super.listChildren(children); 2818 children.add(new Property("url", "uri", "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2819 children.add(new Property("version", "string", "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2820 children.add(new Property("name", "string", "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2821 children.add(new Property("status", "code", "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2822 children.add(new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind)); 2823 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2824 children.add(new Property("date", "dateTime", "The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 0, 1, date)); 2825 children.add(new Property("publisher", "string", "The name of the individual or organization that published the operation definition.", 0, 1, publisher)); 2826 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2827 children.add(new Property("description", "markdown", "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, description)); 2828 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate operation definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2829 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the operation definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2830 children.add(new Property("purpose", "markdown", "Explaination of why this operation definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2831 children.add(new Property("idempotent", "boolean", "Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.", 0, 1, idempotent)); 2832 children.add(new Property("code", "code", "The name used to invoke the operation.", 0, 1, code)); 2833 children.add(new Property("comment", "string", "Additional information about how to use this operation or named query.", 0, 1, comment)); 2834 children.add(new Property("base", "Reference(OperationDefinition)", "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base)); 2835 children.add(new Property("resource", "code", "The types on which this operation can be executed.", 0, java.lang.Integer.MAX_VALUE, resource)); 2836 children.add(new Property("system", "boolean", "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 0, 1, system)); 2837 children.add(new Property("type", "boolean", "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 0, 1, type)); 2838 children.add(new Property("instance", "boolean", "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, instance)); 2839 children.add(new Property("parameter", "", "The parameters for the operation/query.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2840 children.add(new Property("overload", "", "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 0, java.lang.Integer.MAX_VALUE, overload)); 2841 } 2842 2843 @Override 2844 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2845 switch (_hash) { 2846 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published. The URL SHOULD include the major version of the operation definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2847 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2848 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2849 case -892481550: /*status*/ return new Property("status", "code", "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2850 case 3292052: /*kind*/ return new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind); 2851 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2852 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the operation definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 0, 1, date); 2853 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the operation definition.", 0, 1, publisher); 2854 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2855 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, description); 2856 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate operation definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2857 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the operation definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2858 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this operation definition is needed and why it has been designed as it has.", 0, 1, purpose); 2859 case 1680468793: /*idempotent*/ return new Property("idempotent", "boolean", "Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.", 0, 1, idempotent); 2860 case 3059181: /*code*/ return new Property("code", "code", "The name used to invoke the operation.", 0, 1, code); 2861 case 950398559: /*comment*/ return new Property("comment", "string", "Additional information about how to use this operation or named query.", 0, 1, comment); 2862 case 3016401: /*base*/ return new Property("base", "Reference(OperationDefinition)", "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base); 2863 case -341064690: /*resource*/ return new Property("resource", "code", "The types on which this operation can be executed.", 0, java.lang.Integer.MAX_VALUE, resource); 2864 case -887328209: /*system*/ return new Property("system", "boolean", "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 0, 1, system); 2865 case 3575610: /*type*/ return new Property("type", "boolean", "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 0, 1, type); 2866 case 555127957: /*instance*/ return new Property("instance", "boolean", "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, instance); 2867 case 1954460585: /*parameter*/ return new Property("parameter", "", "The parameters for the operation/query.", 0, java.lang.Integer.MAX_VALUE, parameter); 2868 case 529823674: /*overload*/ return new Property("overload", "", "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 0, java.lang.Integer.MAX_VALUE, overload); 2869 default: return super.getNamedProperty(_hash, _name, _checkValid); 2870 } 2871 2872 } 2873 2874 @Override 2875 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2876 switch (hash) { 2877 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2878 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2879 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2880 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2881 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<OperationKind> 2882 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2883 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2884 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2885 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2886 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2887 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2888 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2889 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2890 case 1680468793: /*idempotent*/ return this.idempotent == null ? new Base[0] : new Base[] {this.idempotent}; // BooleanType 2891 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2892 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 2893 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // Reference 2894 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CodeType 2895 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // BooleanType 2896 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // BooleanType 2897 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // BooleanType 2898 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // OperationDefinitionParameterComponent 2899 case 529823674: /*overload*/ return this.overload == null ? new Base[0] : this.overload.toArray(new Base[this.overload.size()]); // OperationDefinitionOverloadComponent 2900 default: return super.getProperty(hash, name, checkValid); 2901 } 2902 2903 } 2904 2905 @Override 2906 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2907 switch (hash) { 2908 case 116079: // url 2909 this.url = castToUri(value); // UriType 2910 return value; 2911 case 351608024: // version 2912 this.version = castToString(value); // StringType 2913 return value; 2914 case 3373707: // name 2915 this.name = castToString(value); // StringType 2916 return value; 2917 case -892481550: // status 2918 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2919 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2920 return value; 2921 case 3292052: // kind 2922 value = new OperationKindEnumFactory().fromType(castToCode(value)); 2923 this.kind = (Enumeration) value; // Enumeration<OperationKind> 2924 return value; 2925 case -404562712: // experimental 2926 this.experimental = castToBoolean(value); // BooleanType 2927 return value; 2928 case 3076014: // date 2929 this.date = castToDateTime(value); // DateTimeType 2930 return value; 2931 case 1447404028: // publisher 2932 this.publisher = castToString(value); // StringType 2933 return value; 2934 case 951526432: // contact 2935 this.getContact().add(castToContactDetail(value)); // ContactDetail 2936 return value; 2937 case -1724546052: // description 2938 this.description = castToMarkdown(value); // MarkdownType 2939 return value; 2940 case -669707736: // useContext 2941 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2942 return value; 2943 case -507075711: // jurisdiction 2944 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2945 return value; 2946 case -220463842: // purpose 2947 this.purpose = castToMarkdown(value); // MarkdownType 2948 return value; 2949 case 1680468793: // idempotent 2950 this.idempotent = castToBoolean(value); // BooleanType 2951 return value; 2952 case 3059181: // code 2953 this.code = castToCode(value); // CodeType 2954 return value; 2955 case 950398559: // comment 2956 this.comment = castToString(value); // StringType 2957 return value; 2958 case 3016401: // base 2959 this.base = castToReference(value); // Reference 2960 return value; 2961 case -341064690: // resource 2962 this.getResource().add(castToCode(value)); // CodeType 2963 return value; 2964 case -887328209: // system 2965 this.system = castToBoolean(value); // BooleanType 2966 return value; 2967 case 3575610: // type 2968 this.type = castToBoolean(value); // BooleanType 2969 return value; 2970 case 555127957: // instance 2971 this.instance = castToBoolean(value); // BooleanType 2972 return value; 2973 case 1954460585: // parameter 2974 this.getParameter().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 2975 return value; 2976 case 529823674: // overload 2977 this.getOverload().add((OperationDefinitionOverloadComponent) value); // OperationDefinitionOverloadComponent 2978 return value; 2979 default: return super.setProperty(hash, name, value); 2980 } 2981 2982 } 2983 2984 @Override 2985 public Base setProperty(String name, Base value) throws FHIRException { 2986 if (name.equals("url")) { 2987 this.url = castToUri(value); // UriType 2988 } else if (name.equals("version")) { 2989 this.version = castToString(value); // StringType 2990 } else if (name.equals("name")) { 2991 this.name = castToString(value); // StringType 2992 } else if (name.equals("status")) { 2993 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2994 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2995 } else if (name.equals("kind")) { 2996 value = new OperationKindEnumFactory().fromType(castToCode(value)); 2997 this.kind = (Enumeration) value; // Enumeration<OperationKind> 2998 } else if (name.equals("experimental")) { 2999 this.experimental = castToBoolean(value); // BooleanType 3000 } else if (name.equals("date")) { 3001 this.date = castToDateTime(value); // DateTimeType 3002 } else if (name.equals("publisher")) { 3003 this.publisher = castToString(value); // StringType 3004 } else if (name.equals("contact")) { 3005 this.getContact().add(castToContactDetail(value)); 3006 } else if (name.equals("description")) { 3007 this.description = castToMarkdown(value); // MarkdownType 3008 } else if (name.equals("useContext")) { 3009 this.getUseContext().add(castToUsageContext(value)); 3010 } else if (name.equals("jurisdiction")) { 3011 this.getJurisdiction().add(castToCodeableConcept(value)); 3012 } else if (name.equals("purpose")) { 3013 this.purpose = castToMarkdown(value); // MarkdownType 3014 } else if (name.equals("idempotent")) { 3015 this.idempotent = castToBoolean(value); // BooleanType 3016 } else if (name.equals("code")) { 3017 this.code = castToCode(value); // CodeType 3018 } else if (name.equals("comment")) { 3019 this.comment = castToString(value); // StringType 3020 } else if (name.equals("base")) { 3021 this.base = castToReference(value); // Reference 3022 } else if (name.equals("resource")) { 3023 this.getResource().add(castToCode(value)); 3024 } else if (name.equals("system")) { 3025 this.system = castToBoolean(value); // BooleanType 3026 } else if (name.equals("type")) { 3027 this.type = castToBoolean(value); // BooleanType 3028 } else if (name.equals("instance")) { 3029 this.instance = castToBoolean(value); // BooleanType 3030 } else if (name.equals("parameter")) { 3031 this.getParameter().add((OperationDefinitionParameterComponent) value); 3032 } else if (name.equals("overload")) { 3033 this.getOverload().add((OperationDefinitionOverloadComponent) value); 3034 } else 3035 return super.setProperty(name, value); 3036 return value; 3037 } 3038 3039 @Override 3040 public Base makeProperty(int hash, String name) throws FHIRException { 3041 switch (hash) { 3042 case 116079: return getUrlElement(); 3043 case 351608024: return getVersionElement(); 3044 case 3373707: return getNameElement(); 3045 case -892481550: return getStatusElement(); 3046 case 3292052: return getKindElement(); 3047 case -404562712: return getExperimentalElement(); 3048 case 3076014: return getDateElement(); 3049 case 1447404028: return getPublisherElement(); 3050 case 951526432: return addContact(); 3051 case -1724546052: return getDescriptionElement(); 3052 case -669707736: return addUseContext(); 3053 case -507075711: return addJurisdiction(); 3054 case -220463842: return getPurposeElement(); 3055 case 1680468793: return getIdempotentElement(); 3056 case 3059181: return getCodeElement(); 3057 case 950398559: return getCommentElement(); 3058 case 3016401: return getBase(); 3059 case -341064690: return addResourceElement(); 3060 case -887328209: return getSystemElement(); 3061 case 3575610: return getTypeElement(); 3062 case 555127957: return getInstanceElement(); 3063 case 1954460585: return addParameter(); 3064 case 529823674: return addOverload(); 3065 default: return super.makeProperty(hash, name); 3066 } 3067 3068 } 3069 3070 @Override 3071 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3072 switch (hash) { 3073 case 116079: /*url*/ return new String[] {"uri"}; 3074 case 351608024: /*version*/ return new String[] {"string"}; 3075 case 3373707: /*name*/ return new String[] {"string"}; 3076 case -892481550: /*status*/ return new String[] {"code"}; 3077 case 3292052: /*kind*/ return new String[] {"code"}; 3078 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3079 case 3076014: /*date*/ return new String[] {"dateTime"}; 3080 case 1447404028: /*publisher*/ return new String[] {"string"}; 3081 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3082 case -1724546052: /*description*/ return new String[] {"markdown"}; 3083 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3084 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3085 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3086 case 1680468793: /*idempotent*/ return new String[] {"boolean"}; 3087 case 3059181: /*code*/ return new String[] {"code"}; 3088 case 950398559: /*comment*/ return new String[] {"string"}; 3089 case 3016401: /*base*/ return new String[] {"Reference"}; 3090 case -341064690: /*resource*/ return new String[] {"code"}; 3091 case -887328209: /*system*/ return new String[] {"boolean"}; 3092 case 3575610: /*type*/ return new String[] {"boolean"}; 3093 case 555127957: /*instance*/ return new String[] {"boolean"}; 3094 case 1954460585: /*parameter*/ return new String[] {}; 3095 case 529823674: /*overload*/ return new String[] {}; 3096 default: return super.getTypesForProperty(hash, name); 3097 } 3098 3099 } 3100 3101 @Override 3102 public Base addChild(String name) throws FHIRException { 3103 if (name.equals("url")) { 3104 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.url"); 3105 } 3106 else if (name.equals("version")) { 3107 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.version"); 3108 } 3109 else if (name.equals("name")) { 3110 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 3111 } 3112 else if (name.equals("status")) { 3113 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.status"); 3114 } 3115 else if (name.equals("kind")) { 3116 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.kind"); 3117 } 3118 else if (name.equals("experimental")) { 3119 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.experimental"); 3120 } 3121 else if (name.equals("date")) { 3122 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.date"); 3123 } 3124 else if (name.equals("publisher")) { 3125 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.publisher"); 3126 } 3127 else if (name.equals("contact")) { 3128 return addContact(); 3129 } 3130 else if (name.equals("description")) { 3131 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.description"); 3132 } 3133 else if (name.equals("useContext")) { 3134 return addUseContext(); 3135 } 3136 else if (name.equals("jurisdiction")) { 3137 return addJurisdiction(); 3138 } 3139 else if (name.equals("purpose")) { 3140 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.purpose"); 3141 } 3142 else if (name.equals("idempotent")) { 3143 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.idempotent"); 3144 } 3145 else if (name.equals("code")) { 3146 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.code"); 3147 } 3148 else if (name.equals("comment")) { 3149 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 3150 } 3151 else if (name.equals("base")) { 3152 this.base = new Reference(); 3153 return this.base; 3154 } 3155 else if (name.equals("resource")) { 3156 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.resource"); 3157 } 3158 else if (name.equals("system")) { 3159 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.system"); 3160 } 3161 else if (name.equals("type")) { 3162 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 3163 } 3164 else if (name.equals("instance")) { 3165 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.instance"); 3166 } 3167 else if (name.equals("parameter")) { 3168 return addParameter(); 3169 } 3170 else if (name.equals("overload")) { 3171 return addOverload(); 3172 } 3173 else 3174 return super.addChild(name); 3175 } 3176 3177 public String fhirType() { 3178 return "OperationDefinition"; 3179 3180 } 3181 3182 public OperationDefinition copy() { 3183 OperationDefinition dst = new OperationDefinition(); 3184 copyValues(dst); 3185 dst.url = url == null ? null : url.copy(); 3186 dst.version = version == null ? null : version.copy(); 3187 dst.name = name == null ? null : name.copy(); 3188 dst.status = status == null ? null : status.copy(); 3189 dst.kind = kind == null ? null : kind.copy(); 3190 dst.experimental = experimental == null ? null : experimental.copy(); 3191 dst.date = date == null ? null : date.copy(); 3192 dst.publisher = publisher == null ? null : publisher.copy(); 3193 if (contact != null) { 3194 dst.contact = new ArrayList<ContactDetail>(); 3195 for (ContactDetail i : contact) 3196 dst.contact.add(i.copy()); 3197 }; 3198 dst.description = description == null ? null : description.copy(); 3199 if (useContext != null) { 3200 dst.useContext = new ArrayList<UsageContext>(); 3201 for (UsageContext i : useContext) 3202 dst.useContext.add(i.copy()); 3203 }; 3204 if (jurisdiction != null) { 3205 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3206 for (CodeableConcept i : jurisdiction) 3207 dst.jurisdiction.add(i.copy()); 3208 }; 3209 dst.purpose = purpose == null ? null : purpose.copy(); 3210 dst.idempotent = idempotent == null ? null : idempotent.copy(); 3211 dst.code = code == null ? null : code.copy(); 3212 dst.comment = comment == null ? null : comment.copy(); 3213 dst.base = base == null ? null : base.copy(); 3214 if (resource != null) { 3215 dst.resource = new ArrayList<CodeType>(); 3216 for (CodeType i : resource) 3217 dst.resource.add(i.copy()); 3218 }; 3219 dst.system = system == null ? null : system.copy(); 3220 dst.type = type == null ? null : type.copy(); 3221 dst.instance = instance == null ? null : instance.copy(); 3222 if (parameter != null) { 3223 dst.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3224 for (OperationDefinitionParameterComponent i : parameter) 3225 dst.parameter.add(i.copy()); 3226 }; 3227 if (overload != null) { 3228 dst.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3229 for (OperationDefinitionOverloadComponent i : overload) 3230 dst.overload.add(i.copy()); 3231 }; 3232 return dst; 3233 } 3234 3235 protected OperationDefinition typedCopy() { 3236 return copy(); 3237 } 3238 3239 @Override 3240 public boolean equalsDeep(Base other_) { 3241 if (!super.equalsDeep(other_)) 3242 return false; 3243 if (!(other_ instanceof OperationDefinition)) 3244 return false; 3245 OperationDefinition o = (OperationDefinition) other_; 3246 return compareDeep(kind, o.kind, true) && compareDeep(purpose, o.purpose, true) && compareDeep(idempotent, o.idempotent, true) 3247 && compareDeep(code, o.code, true) && compareDeep(comment, o.comment, true) && compareDeep(base, o.base, true) 3248 && compareDeep(resource, o.resource, true) && compareDeep(system, o.system, true) && compareDeep(type, o.type, true) 3249 && compareDeep(instance, o.instance, true) && compareDeep(parameter, o.parameter, true) && compareDeep(overload, o.overload, true) 3250 ; 3251 } 3252 3253 @Override 3254 public boolean equalsShallow(Base other_) { 3255 if (!super.equalsShallow(other_)) 3256 return false; 3257 if (!(other_ instanceof OperationDefinition)) 3258 return false; 3259 OperationDefinition o = (OperationDefinition) other_; 3260 return compareValues(kind, o.kind, true) && compareValues(purpose, o.purpose, true) && compareValues(idempotent, o.idempotent, true) 3261 && compareValues(code, o.code, true) && compareValues(comment, o.comment, true) && compareValues(resource, o.resource, true) 3262 && compareValues(system, o.system, true) && compareValues(type, o.type, true) && compareValues(instance, o.instance, true) 3263 ; 3264 } 3265 3266 public boolean isEmpty() { 3267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, purpose, idempotent 3268 , code, comment, base, resource, system, type, instance, parameter, overload 3269 ); 3270 } 3271 3272 @Override 3273 public ResourceType getResourceType() { 3274 return ResourceType.OperationDefinition; 3275 } 3276 3277 /** 3278 * Search parameter: <b>date</b> 3279 * <p> 3280 * Description: <b>The operation definition publication date</b><br> 3281 * Type: <b>date</b><br> 3282 * Path: <b>OperationDefinition.date</b><br> 3283 * </p> 3284 */ 3285 @SearchParamDefinition(name="date", path="OperationDefinition.date", description="The operation definition publication date", type="date" ) 3286 public static final String SP_DATE = "date"; 3287 /** 3288 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3289 * <p> 3290 * Description: <b>The operation definition publication date</b><br> 3291 * Type: <b>date</b><br> 3292 * Path: <b>OperationDefinition.date</b><br> 3293 * </p> 3294 */ 3295 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3296 3297 /** 3298 * Search parameter: <b>code</b> 3299 * <p> 3300 * Description: <b>Name used to invoke the operation</b><br> 3301 * Type: <b>token</b><br> 3302 * Path: <b>OperationDefinition.code</b><br> 3303 * </p> 3304 */ 3305 @SearchParamDefinition(name="code", path="OperationDefinition.code", description="Name used to invoke the operation", type="token" ) 3306 public static final String SP_CODE = "code"; 3307 /** 3308 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3309 * <p> 3310 * Description: <b>Name used to invoke the operation</b><br> 3311 * Type: <b>token</b><br> 3312 * Path: <b>OperationDefinition.code</b><br> 3313 * </p> 3314 */ 3315 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3316 3317 /** 3318 * Search parameter: <b>instance</b> 3319 * <p> 3320 * Description: <b>Invoke on an instance?</b><br> 3321 * Type: <b>token</b><br> 3322 * Path: <b>OperationDefinition.instance</b><br> 3323 * </p> 3324 */ 3325 @SearchParamDefinition(name="instance", path="OperationDefinition.instance", description="Invoke on an instance?", type="token" ) 3326 public static final String SP_INSTANCE = "instance"; 3327 /** 3328 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 3329 * <p> 3330 * Description: <b>Invoke on an instance?</b><br> 3331 * Type: <b>token</b><br> 3332 * Path: <b>OperationDefinition.instance</b><br> 3333 * </p> 3334 */ 3335 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INSTANCE); 3336 3337 /** 3338 * Search parameter: <b>kind</b> 3339 * <p> 3340 * Description: <b>operation | query</b><br> 3341 * Type: <b>token</b><br> 3342 * Path: <b>OperationDefinition.kind</b><br> 3343 * </p> 3344 */ 3345 @SearchParamDefinition(name="kind", path="OperationDefinition.kind", description="operation | query", type="token" ) 3346 public static final String SP_KIND = "kind"; 3347 /** 3348 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 3349 * <p> 3350 * Description: <b>operation | query</b><br> 3351 * Type: <b>token</b><br> 3352 * Path: <b>OperationDefinition.kind</b><br> 3353 * </p> 3354 */ 3355 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 3356 3357 /** 3358 * Search parameter: <b>jurisdiction</b> 3359 * <p> 3360 * Description: <b>Intended jurisdiction for the operation definition</b><br> 3361 * Type: <b>token</b><br> 3362 * Path: <b>OperationDefinition.jurisdiction</b><br> 3363 * </p> 3364 */ 3365 @SearchParamDefinition(name="jurisdiction", path="OperationDefinition.jurisdiction", description="Intended jurisdiction for the operation definition", type="token" ) 3366 public static final String SP_JURISDICTION = "jurisdiction"; 3367 /** 3368 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3369 * <p> 3370 * Description: <b>Intended jurisdiction for the operation definition</b><br> 3371 * Type: <b>token</b><br> 3372 * Path: <b>OperationDefinition.jurisdiction</b><br> 3373 * </p> 3374 */ 3375 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3376 3377 /** 3378 * Search parameter: <b>description</b> 3379 * <p> 3380 * Description: <b>The description of the operation definition</b><br> 3381 * Type: <b>string</b><br> 3382 * Path: <b>OperationDefinition.description</b><br> 3383 * </p> 3384 */ 3385 @SearchParamDefinition(name="description", path="OperationDefinition.description", description="The description of the operation definition", type="string" ) 3386 public static final String SP_DESCRIPTION = "description"; 3387 /** 3388 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3389 * <p> 3390 * Description: <b>The description of the operation definition</b><br> 3391 * Type: <b>string</b><br> 3392 * Path: <b>OperationDefinition.description</b><br> 3393 * </p> 3394 */ 3395 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3396 3397 /** 3398 * Search parameter: <b>type</b> 3399 * <p> 3400 * Description: <b>Invole at the type level?</b><br> 3401 * Type: <b>token</b><br> 3402 * Path: <b>OperationDefinition.type</b><br> 3403 * </p> 3404 */ 3405 @SearchParamDefinition(name="type", path="OperationDefinition.type", description="Invole at the type level?", type="token" ) 3406 public static final String SP_TYPE = "type"; 3407 /** 3408 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3409 * <p> 3410 * Description: <b>Invole at the type level?</b><br> 3411 * Type: <b>token</b><br> 3412 * Path: <b>OperationDefinition.type</b><br> 3413 * </p> 3414 */ 3415 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3416 3417 /** 3418 * Search parameter: <b>version</b> 3419 * <p> 3420 * Description: <b>The business version of the operation definition</b><br> 3421 * Type: <b>token</b><br> 3422 * Path: <b>OperationDefinition.version</b><br> 3423 * </p> 3424 */ 3425 @SearchParamDefinition(name="version", path="OperationDefinition.version", description="The business version of the operation definition", type="token" ) 3426 public static final String SP_VERSION = "version"; 3427 /** 3428 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3429 * <p> 3430 * Description: <b>The business version of the operation definition</b><br> 3431 * Type: <b>token</b><br> 3432 * Path: <b>OperationDefinition.version</b><br> 3433 * </p> 3434 */ 3435 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3436 3437 /** 3438 * Search parameter: <b>url</b> 3439 * <p> 3440 * Description: <b>The uri that identifies the operation definition</b><br> 3441 * Type: <b>uri</b><br> 3442 * Path: <b>OperationDefinition.url</b><br> 3443 * </p> 3444 */ 3445 @SearchParamDefinition(name="url", path="OperationDefinition.url", description="The uri that identifies the operation definition", type="uri" ) 3446 public static final String SP_URL = "url"; 3447 /** 3448 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3449 * <p> 3450 * Description: <b>The uri that identifies the operation definition</b><br> 3451 * Type: <b>uri</b><br> 3452 * Path: <b>OperationDefinition.url</b><br> 3453 * </p> 3454 */ 3455 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3456 3457 /** 3458 * Search parameter: <b>system</b> 3459 * <p> 3460 * Description: <b>Invoke at the system level?</b><br> 3461 * Type: <b>token</b><br> 3462 * Path: <b>OperationDefinition.system</b><br> 3463 * </p> 3464 */ 3465 @SearchParamDefinition(name="system", path="OperationDefinition.system", description="Invoke at the system level?", type="token" ) 3466 public static final String SP_SYSTEM = "system"; 3467 /** 3468 * <b>Fluent Client</b> search parameter constant for <b>system</b> 3469 * <p> 3470 * Description: <b>Invoke at the system level?</b><br> 3471 * Type: <b>token</b><br> 3472 * Path: <b>OperationDefinition.system</b><br> 3473 * </p> 3474 */ 3475 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SYSTEM); 3476 3477 /** 3478 * Search parameter: <b>name</b> 3479 * <p> 3480 * Description: <b>Computationally friendly name of the operation definition</b><br> 3481 * Type: <b>string</b><br> 3482 * Path: <b>OperationDefinition.name</b><br> 3483 * </p> 3484 */ 3485 @SearchParamDefinition(name="name", path="OperationDefinition.name", description="Computationally friendly name of the operation definition", type="string" ) 3486 public static final String SP_NAME = "name"; 3487 /** 3488 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3489 * <p> 3490 * Description: <b>Computationally friendly name of the operation definition</b><br> 3491 * Type: <b>string</b><br> 3492 * Path: <b>OperationDefinition.name</b><br> 3493 * </p> 3494 */ 3495 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3496 3497 /** 3498 * Search parameter: <b>publisher</b> 3499 * <p> 3500 * Description: <b>Name of the publisher of the operation definition</b><br> 3501 * Type: <b>string</b><br> 3502 * Path: <b>OperationDefinition.publisher</b><br> 3503 * </p> 3504 */ 3505 @SearchParamDefinition(name="publisher", path="OperationDefinition.publisher", description="Name of the publisher of the operation definition", type="string" ) 3506 public static final String SP_PUBLISHER = "publisher"; 3507 /** 3508 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3509 * <p> 3510 * Description: <b>Name of the publisher of the operation definition</b><br> 3511 * Type: <b>string</b><br> 3512 * Path: <b>OperationDefinition.publisher</b><br> 3513 * </p> 3514 */ 3515 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3516 3517 /** 3518 * Search parameter: <b>param-profile</b> 3519 * <p> 3520 * Description: <b>Profile on the type</b><br> 3521 * Type: <b>reference</b><br> 3522 * Path: <b>OperationDefinition.parameter.profile</b><br> 3523 * </p> 3524 */ 3525 @SearchParamDefinition(name="param-profile", path="OperationDefinition.parameter.profile", description="Profile on the type", type="reference", target={StructureDefinition.class } ) 3526 public static final String SP_PARAM_PROFILE = "param-profile"; 3527 /** 3528 * <b>Fluent Client</b> search parameter constant for <b>param-profile</b> 3529 * <p> 3530 * Description: <b>Profile on the type</b><br> 3531 * Type: <b>reference</b><br> 3532 * Path: <b>OperationDefinition.parameter.profile</b><br> 3533 * </p> 3534 */ 3535 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARAM_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARAM_PROFILE); 3536 3537/** 3538 * Constant for fluent queries to be used to add include statements. Specifies 3539 * the path value of "<b>OperationDefinition:param-profile</b>". 3540 */ 3541 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARAM_PROFILE = new ca.uhn.fhir.model.api.Include("OperationDefinition:param-profile").toLocked(); 3542 3543 /** 3544 * Search parameter: <b>status</b> 3545 * <p> 3546 * Description: <b>The current status of the operation definition</b><br> 3547 * Type: <b>token</b><br> 3548 * Path: <b>OperationDefinition.status</b><br> 3549 * </p> 3550 */ 3551 @SearchParamDefinition(name="status", path="OperationDefinition.status", description="The current status of the operation definition", type="token" ) 3552 public static final String SP_STATUS = "status"; 3553 /** 3554 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3555 * <p> 3556 * Description: <b>The current status of the operation definition</b><br> 3557 * Type: <b>token</b><br> 3558 * Path: <b>OperationDefinition.status</b><br> 3559 * </p> 3560 */ 3561 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3562 3563 /** 3564 * Search parameter: <b>base</b> 3565 * <p> 3566 * Description: <b>Marks this as a profile of the base</b><br> 3567 * Type: <b>reference</b><br> 3568 * Path: <b>OperationDefinition.base</b><br> 3569 * </p> 3570 */ 3571 @SearchParamDefinition(name="base", path="OperationDefinition.base", description="Marks this as a profile of the base", type="reference", target={OperationDefinition.class } ) 3572 public static final String SP_BASE = "base"; 3573 /** 3574 * <b>Fluent Client</b> search parameter constant for <b>base</b> 3575 * <p> 3576 * Description: <b>Marks this as a profile of the base</b><br> 3577 * Type: <b>reference</b><br> 3578 * Path: <b>OperationDefinition.base</b><br> 3579 * </p> 3580 */ 3581 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASE); 3582 3583/** 3584 * Constant for fluent queries to be used to add include statements. Specifies 3585 * the path value of "<b>OperationDefinition:base</b>". 3586 */ 3587 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include("OperationDefinition:base").toLocked(); 3588 3589 3590}