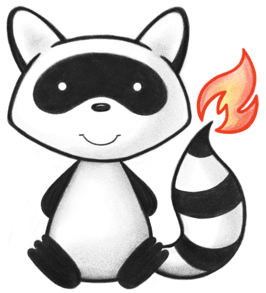
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048/** 049 * A collection of error, warning or information messages that result from a system action. 050 */ 051@ResourceDef(name="OperationOutcome", profile="http://hl7.org/fhir/Profile/OperationOutcome") 052public class OperationOutcome extends DomainResource implements IBaseOperationOutcome { 053 054 public enum IssueSeverity { 055 /** 056 * The issue caused the action to fail, and no further checking could be performed. 057 */ 058 FATAL, 059 /** 060 * The issue is sufficiently important to cause the action to fail. 061 */ 062 ERROR, 063 /** 064 * The issue is not important enough to cause the action to fail, but may cause it to be performed suboptimally or in a way that is not as desired. 065 */ 066 WARNING, 067 /** 068 * The issue has no relation to the degree of success of the action. 069 */ 070 INFORMATION, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static IssueSeverity fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("fatal".equals(codeString)) 079 return FATAL; 080 if ("error".equals(codeString)) 081 return ERROR; 082 if ("warning".equals(codeString)) 083 return WARNING; 084 if ("information".equals(codeString)) 085 return INFORMATION; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown IssueSeverity code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case FATAL: return "fatal"; 094 case ERROR: return "error"; 095 case WARNING: return "warning"; 096 case INFORMATION: return "information"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case FATAL: return "http://hl7.org/fhir/issue-severity"; 104 case ERROR: return "http://hl7.org/fhir/issue-severity"; 105 case WARNING: return "http://hl7.org/fhir/issue-severity"; 106 case INFORMATION: return "http://hl7.org/fhir/issue-severity"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case FATAL: return "The issue caused the action to fail, and no further checking could be performed."; 114 case ERROR: return "The issue is sufficiently important to cause the action to fail."; 115 case WARNING: return "The issue is not important enough to cause the action to fail, but may cause it to be performed suboptimally or in a way that is not as desired."; 116 case INFORMATION: return "The issue has no relation to the degree of success of the action."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case FATAL: return "Fatal"; 124 case ERROR: return "Error"; 125 case WARNING: return "Warning"; 126 case INFORMATION: return "Information"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class IssueSeverityEnumFactory implements EnumFactory<IssueSeverity> { 134 public IssueSeverity fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("fatal".equals(codeString)) 139 return IssueSeverity.FATAL; 140 if ("error".equals(codeString)) 141 return IssueSeverity.ERROR; 142 if ("warning".equals(codeString)) 143 return IssueSeverity.WARNING; 144 if ("information".equals(codeString)) 145 return IssueSeverity.INFORMATION; 146 throw new IllegalArgumentException("Unknown IssueSeverity code '"+codeString+"'"); 147 } 148 public Enumeration<IssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<IssueSeverity>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("fatal".equals(codeString)) 157 return new Enumeration<IssueSeverity>(this, IssueSeverity.FATAL); 158 if ("error".equals(codeString)) 159 return new Enumeration<IssueSeverity>(this, IssueSeverity.ERROR); 160 if ("warning".equals(codeString)) 161 return new Enumeration<IssueSeverity>(this, IssueSeverity.WARNING); 162 if ("information".equals(codeString)) 163 return new Enumeration<IssueSeverity>(this, IssueSeverity.INFORMATION); 164 throw new FHIRException("Unknown IssueSeverity code '"+codeString+"'"); 165 } 166 public String toCode(IssueSeverity code) { 167 if (code == IssueSeverity.NULL) 168 return null; 169 if (code == IssueSeverity.FATAL) 170 return "fatal"; 171 if (code == IssueSeverity.ERROR) 172 return "error"; 173 if (code == IssueSeverity.WARNING) 174 return "warning"; 175 if (code == IssueSeverity.INFORMATION) 176 return "information"; 177 return "?"; 178 } 179 public String toSystem(IssueSeverity code) { 180 return code.getSystem(); 181 } 182 } 183 184 public enum IssueType { 185 /** 186 * Content invalid against the specification or a profile. 187 */ 188 INVALID, 189 /** 190 * A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax. 191 */ 192 STRUCTURE, 193 /** 194 * A required element is missing. 195 */ 196 REQUIRED, 197 /** 198 * An element value is invalid. 199 */ 200 VALUE, 201 /** 202 * A content validation rule failed - e.g. a schematron rule. 203 */ 204 INVARIANT, 205 /** 206 * An authentication/authorization/permissions issue of some kind. 207 */ 208 SECURITY, 209 /** 210 * The client needs to initiate an authentication process. 211 */ 212 LOGIN, 213 /** 214 * The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable). 215 */ 216 UNKNOWN, 217 /** 218 * User session expired; a login may be required. 219 */ 220 EXPIRED, 221 /** 222 * The user does not have the rights to perform this action. 223 */ 224 FORBIDDEN, 225 /** 226 * Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes. 227 */ 228 SUPPRESSED, 229 /** 230 * Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged. 231 */ 232 PROCESSING, 233 /** 234 * The resource or profile is not supported. 235 */ 236 NOTSUPPORTED, 237 /** 238 * An attempt was made to create a duplicate record. 239 */ 240 DUPLICATE, 241 /** 242 * The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture. 243 */ 244 NOTFOUND, 245 /** 246 * Provided content is too long (typically, this is a denial of service protection type of error). 247 */ 248 TOOLONG, 249 /** 250 * The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code. 251 */ 252 CODEINVALID, 253 /** 254 * An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized. 255 */ 256 EXTENSION, 257 /** 258 * The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT. 259 */ 260 TOOCOSTLY, 261 /** 262 * The content/operation failed to pass some business rule, and so could not proceed. 263 */ 264 BUSINESSRULE, 265 /** 266 * Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.) 267 */ 268 CONFLICT, 269 /** 270 * Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete. 271 */ 272 INCOMPLETE, 273 /** 274 * Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved. 275 */ 276 TRANSIENT, 277 /** 278 * A resource/record locking failure (usually in an underlying database). 279 */ 280 LOCKERROR, 281 /** 282 * The persistent store is unavailable; e.g. the database is down for maintenance or similar action. 283 */ 284 NOSTORE, 285 /** 286 * An unexpected internal error has occurred. 287 */ 288 EXCEPTION, 289 /** 290 * An internal timeout has occurred. 291 */ 292 TIMEOUT, 293 /** 294 * The system is not prepared to handle this request due to load management. 295 */ 296 THROTTLED, 297 /** 298 * A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.). 299 */ 300 INFORMATIONAL, 301 /** 302 * added to help the parsers with the generic types 303 */ 304 NULL; 305 public static IssueType fromCode(String codeString) throws FHIRException { 306 if (codeString == null || "".equals(codeString)) 307 return null; 308 if ("invalid".equals(codeString)) 309 return INVALID; 310 if ("structure".equals(codeString)) 311 return STRUCTURE; 312 if ("required".equals(codeString)) 313 return REQUIRED; 314 if ("value".equals(codeString)) 315 return VALUE; 316 if ("invariant".equals(codeString)) 317 return INVARIANT; 318 if ("security".equals(codeString)) 319 return SECURITY; 320 if ("login".equals(codeString)) 321 return LOGIN; 322 if ("unknown".equals(codeString)) 323 return UNKNOWN; 324 if ("expired".equals(codeString)) 325 return EXPIRED; 326 if ("forbidden".equals(codeString)) 327 return FORBIDDEN; 328 if ("suppressed".equals(codeString)) 329 return SUPPRESSED; 330 if ("processing".equals(codeString)) 331 return PROCESSING; 332 if ("not-supported".equals(codeString)) 333 return NOTSUPPORTED; 334 if ("duplicate".equals(codeString)) 335 return DUPLICATE; 336 if ("not-found".equals(codeString)) 337 return NOTFOUND; 338 if ("too-long".equals(codeString)) 339 return TOOLONG; 340 if ("code-invalid".equals(codeString)) 341 return CODEINVALID; 342 if ("extension".equals(codeString)) 343 return EXTENSION; 344 if ("too-costly".equals(codeString)) 345 return TOOCOSTLY; 346 if ("business-rule".equals(codeString)) 347 return BUSINESSRULE; 348 if ("conflict".equals(codeString)) 349 return CONFLICT; 350 if ("incomplete".equals(codeString)) 351 return INCOMPLETE; 352 if ("transient".equals(codeString)) 353 return TRANSIENT; 354 if ("lock-error".equals(codeString)) 355 return LOCKERROR; 356 if ("no-store".equals(codeString)) 357 return NOSTORE; 358 if ("exception".equals(codeString)) 359 return EXCEPTION; 360 if ("timeout".equals(codeString)) 361 return TIMEOUT; 362 if ("throttled".equals(codeString)) 363 return THROTTLED; 364 if ("informational".equals(codeString)) 365 return INFORMATIONAL; 366 if (Configuration.isAcceptInvalidEnums()) 367 return null; 368 else 369 throw new FHIRException("Unknown IssueType code '"+codeString+"'"); 370 } 371 public String toCode() { 372 switch (this) { 373 case INVALID: return "invalid"; 374 case STRUCTURE: return "structure"; 375 case REQUIRED: return "required"; 376 case VALUE: return "value"; 377 case INVARIANT: return "invariant"; 378 case SECURITY: return "security"; 379 case LOGIN: return "login"; 380 case UNKNOWN: return "unknown"; 381 case EXPIRED: return "expired"; 382 case FORBIDDEN: return "forbidden"; 383 case SUPPRESSED: return "suppressed"; 384 case PROCESSING: return "processing"; 385 case NOTSUPPORTED: return "not-supported"; 386 case DUPLICATE: return "duplicate"; 387 case NOTFOUND: return "not-found"; 388 case TOOLONG: return "too-long"; 389 case CODEINVALID: return "code-invalid"; 390 case EXTENSION: return "extension"; 391 case TOOCOSTLY: return "too-costly"; 392 case BUSINESSRULE: return "business-rule"; 393 case CONFLICT: return "conflict"; 394 case INCOMPLETE: return "incomplete"; 395 case TRANSIENT: return "transient"; 396 case LOCKERROR: return "lock-error"; 397 case NOSTORE: return "no-store"; 398 case EXCEPTION: return "exception"; 399 case TIMEOUT: return "timeout"; 400 case THROTTLED: return "throttled"; 401 case INFORMATIONAL: return "informational"; 402 case NULL: return null; 403 default: return "?"; 404 } 405 } 406 public String getSystem() { 407 switch (this) { 408 case INVALID: return "http://hl7.org/fhir/issue-type"; 409 case STRUCTURE: return "http://hl7.org/fhir/issue-type"; 410 case REQUIRED: return "http://hl7.org/fhir/issue-type"; 411 case VALUE: return "http://hl7.org/fhir/issue-type"; 412 case INVARIANT: return "http://hl7.org/fhir/issue-type"; 413 case SECURITY: return "http://hl7.org/fhir/issue-type"; 414 case LOGIN: return "http://hl7.org/fhir/issue-type"; 415 case UNKNOWN: return "http://hl7.org/fhir/issue-type"; 416 case EXPIRED: return "http://hl7.org/fhir/issue-type"; 417 case FORBIDDEN: return "http://hl7.org/fhir/issue-type"; 418 case SUPPRESSED: return "http://hl7.org/fhir/issue-type"; 419 case PROCESSING: return "http://hl7.org/fhir/issue-type"; 420 case NOTSUPPORTED: return "http://hl7.org/fhir/issue-type"; 421 case DUPLICATE: return "http://hl7.org/fhir/issue-type"; 422 case NOTFOUND: return "http://hl7.org/fhir/issue-type"; 423 case TOOLONG: return "http://hl7.org/fhir/issue-type"; 424 case CODEINVALID: return "http://hl7.org/fhir/issue-type"; 425 case EXTENSION: return "http://hl7.org/fhir/issue-type"; 426 case TOOCOSTLY: return "http://hl7.org/fhir/issue-type"; 427 case BUSINESSRULE: return "http://hl7.org/fhir/issue-type"; 428 case CONFLICT: return "http://hl7.org/fhir/issue-type"; 429 case INCOMPLETE: return "http://hl7.org/fhir/issue-type"; 430 case TRANSIENT: return "http://hl7.org/fhir/issue-type"; 431 case LOCKERROR: return "http://hl7.org/fhir/issue-type"; 432 case NOSTORE: return "http://hl7.org/fhir/issue-type"; 433 case EXCEPTION: return "http://hl7.org/fhir/issue-type"; 434 case TIMEOUT: return "http://hl7.org/fhir/issue-type"; 435 case THROTTLED: return "http://hl7.org/fhir/issue-type"; 436 case INFORMATIONAL: return "http://hl7.org/fhir/issue-type"; 437 case NULL: return null; 438 default: return "?"; 439 } 440 } 441 public String getDefinition() { 442 switch (this) { 443 case INVALID: return "Content invalid against the specification or a profile."; 444 case STRUCTURE: return "A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax."; 445 case REQUIRED: return "A required element is missing."; 446 case VALUE: return "An element value is invalid."; 447 case INVARIANT: return "A content validation rule failed - e.g. a schematron rule."; 448 case SECURITY: return "An authentication/authorization/permissions issue of some kind."; 449 case LOGIN: return "The client needs to initiate an authentication process."; 450 case UNKNOWN: return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 451 case EXPIRED: return "User session expired; a login may be required."; 452 case FORBIDDEN: return "The user does not have the rights to perform this action."; 453 case SUPPRESSED: return "Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 454 case PROCESSING: return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 455 case NOTSUPPORTED: return "The resource or profile is not supported."; 456 case DUPLICATE: return "An attempt was made to create a duplicate record."; 457 case NOTFOUND: return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 458 case TOOLONG: return "Provided content is too long (typically, this is a denial of service protection type of error)."; 459 case CODEINVALID: return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 460 case EXTENSION: return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 461 case TOOCOSTLY: return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 462 case BUSINESSRULE: return "The content/operation failed to pass some business rule, and so could not proceed."; 463 case CONFLICT: return "Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.)"; 464 case INCOMPLETE: return "Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete."; 465 case TRANSIENT: return "Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved."; 466 case LOCKERROR: return "A resource/record locking failure (usually in an underlying database)."; 467 case NOSTORE: return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action."; 468 case EXCEPTION: return "An unexpected internal error has occurred."; 469 case TIMEOUT: return "An internal timeout has occurred."; 470 case THROTTLED: return "The system is not prepared to handle this request due to load management."; 471 case INFORMATIONAL: return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 472 case NULL: return null; 473 default: return "?"; 474 } 475 } 476 public String getDisplay() { 477 switch (this) { 478 case INVALID: return "Invalid Content"; 479 case STRUCTURE: return "Structural Issue"; 480 case REQUIRED: return "Required element missing"; 481 case VALUE: return "Element value invalid"; 482 case INVARIANT: return "Validation rule failed"; 483 case SECURITY: return "Security Problem"; 484 case LOGIN: return "Login Required"; 485 case UNKNOWN: return "Unknown User"; 486 case EXPIRED: return "Session Expired"; 487 case FORBIDDEN: return "Forbidden"; 488 case SUPPRESSED: return "Information Suppressed"; 489 case PROCESSING: return "Processing Failure"; 490 case NOTSUPPORTED: return "Content not supported"; 491 case DUPLICATE: return "Duplicate"; 492 case NOTFOUND: return "Not Found"; 493 case TOOLONG: return "Content Too Long"; 494 case CODEINVALID: return "Invalid Code"; 495 case EXTENSION: return "Unacceptable Extension"; 496 case TOOCOSTLY: return "Operation Too Costly"; 497 case BUSINESSRULE: return "Business Rule Violation"; 498 case CONFLICT: return "Edit Version Conflict"; 499 case INCOMPLETE: return "Incomplete Results"; 500 case TRANSIENT: return "Transient Issue"; 501 case LOCKERROR: return "Lock Error"; 502 case NOSTORE: return "No Store Available"; 503 case EXCEPTION: return "Exception"; 504 case TIMEOUT: return "Timeout"; 505 case THROTTLED: return "Throttled"; 506 case INFORMATIONAL: return "Informational Note"; 507 case NULL: return null; 508 default: return "?"; 509 } 510 } 511 } 512 513 public static class IssueTypeEnumFactory implements EnumFactory<IssueType> { 514 public IssueType fromCode(String codeString) throws IllegalArgumentException { 515 if (codeString == null || "".equals(codeString)) 516 if (codeString == null || "".equals(codeString)) 517 return null; 518 if ("invalid".equals(codeString)) 519 return IssueType.INVALID; 520 if ("structure".equals(codeString)) 521 return IssueType.STRUCTURE; 522 if ("required".equals(codeString)) 523 return IssueType.REQUIRED; 524 if ("value".equals(codeString)) 525 return IssueType.VALUE; 526 if ("invariant".equals(codeString)) 527 return IssueType.INVARIANT; 528 if ("security".equals(codeString)) 529 return IssueType.SECURITY; 530 if ("login".equals(codeString)) 531 return IssueType.LOGIN; 532 if ("unknown".equals(codeString)) 533 return IssueType.UNKNOWN; 534 if ("expired".equals(codeString)) 535 return IssueType.EXPIRED; 536 if ("forbidden".equals(codeString)) 537 return IssueType.FORBIDDEN; 538 if ("suppressed".equals(codeString)) 539 return IssueType.SUPPRESSED; 540 if ("processing".equals(codeString)) 541 return IssueType.PROCESSING; 542 if ("not-supported".equals(codeString)) 543 return IssueType.NOTSUPPORTED; 544 if ("duplicate".equals(codeString)) 545 return IssueType.DUPLICATE; 546 if ("not-found".equals(codeString)) 547 return IssueType.NOTFOUND; 548 if ("too-long".equals(codeString)) 549 return IssueType.TOOLONG; 550 if ("code-invalid".equals(codeString)) 551 return IssueType.CODEINVALID; 552 if ("extension".equals(codeString)) 553 return IssueType.EXTENSION; 554 if ("too-costly".equals(codeString)) 555 return IssueType.TOOCOSTLY; 556 if ("business-rule".equals(codeString)) 557 return IssueType.BUSINESSRULE; 558 if ("conflict".equals(codeString)) 559 return IssueType.CONFLICT; 560 if ("incomplete".equals(codeString)) 561 return IssueType.INCOMPLETE; 562 if ("transient".equals(codeString)) 563 return IssueType.TRANSIENT; 564 if ("lock-error".equals(codeString)) 565 return IssueType.LOCKERROR; 566 if ("no-store".equals(codeString)) 567 return IssueType.NOSTORE; 568 if ("exception".equals(codeString)) 569 return IssueType.EXCEPTION; 570 if ("timeout".equals(codeString)) 571 return IssueType.TIMEOUT; 572 if ("throttled".equals(codeString)) 573 return IssueType.THROTTLED; 574 if ("informational".equals(codeString)) 575 return IssueType.INFORMATIONAL; 576 throw new IllegalArgumentException("Unknown IssueType code '"+codeString+"'"); 577 } 578 public Enumeration<IssueType> fromType(PrimitiveType<?> code) throws FHIRException { 579 if (code == null) 580 return null; 581 if (code.isEmpty()) 582 return new Enumeration<IssueType>(this); 583 String codeString = code.asStringValue(); 584 if (codeString == null || "".equals(codeString)) 585 return null; 586 if ("invalid".equals(codeString)) 587 return new Enumeration<IssueType>(this, IssueType.INVALID); 588 if ("structure".equals(codeString)) 589 return new Enumeration<IssueType>(this, IssueType.STRUCTURE); 590 if ("required".equals(codeString)) 591 return new Enumeration<IssueType>(this, IssueType.REQUIRED); 592 if ("value".equals(codeString)) 593 return new Enumeration<IssueType>(this, IssueType.VALUE); 594 if ("invariant".equals(codeString)) 595 return new Enumeration<IssueType>(this, IssueType.INVARIANT); 596 if ("security".equals(codeString)) 597 return new Enumeration<IssueType>(this, IssueType.SECURITY); 598 if ("login".equals(codeString)) 599 return new Enumeration<IssueType>(this, IssueType.LOGIN); 600 if ("unknown".equals(codeString)) 601 return new Enumeration<IssueType>(this, IssueType.UNKNOWN); 602 if ("expired".equals(codeString)) 603 return new Enumeration<IssueType>(this, IssueType.EXPIRED); 604 if ("forbidden".equals(codeString)) 605 return new Enumeration<IssueType>(this, IssueType.FORBIDDEN); 606 if ("suppressed".equals(codeString)) 607 return new Enumeration<IssueType>(this, IssueType.SUPPRESSED); 608 if ("processing".equals(codeString)) 609 return new Enumeration<IssueType>(this, IssueType.PROCESSING); 610 if ("not-supported".equals(codeString)) 611 return new Enumeration<IssueType>(this, IssueType.NOTSUPPORTED); 612 if ("duplicate".equals(codeString)) 613 return new Enumeration<IssueType>(this, IssueType.DUPLICATE); 614 if ("not-found".equals(codeString)) 615 return new Enumeration<IssueType>(this, IssueType.NOTFOUND); 616 if ("too-long".equals(codeString)) 617 return new Enumeration<IssueType>(this, IssueType.TOOLONG); 618 if ("code-invalid".equals(codeString)) 619 return new Enumeration<IssueType>(this, IssueType.CODEINVALID); 620 if ("extension".equals(codeString)) 621 return new Enumeration<IssueType>(this, IssueType.EXTENSION); 622 if ("too-costly".equals(codeString)) 623 return new Enumeration<IssueType>(this, IssueType.TOOCOSTLY); 624 if ("business-rule".equals(codeString)) 625 return new Enumeration<IssueType>(this, IssueType.BUSINESSRULE); 626 if ("conflict".equals(codeString)) 627 return new Enumeration<IssueType>(this, IssueType.CONFLICT); 628 if ("incomplete".equals(codeString)) 629 return new Enumeration<IssueType>(this, IssueType.INCOMPLETE); 630 if ("transient".equals(codeString)) 631 return new Enumeration<IssueType>(this, IssueType.TRANSIENT); 632 if ("lock-error".equals(codeString)) 633 return new Enumeration<IssueType>(this, IssueType.LOCKERROR); 634 if ("no-store".equals(codeString)) 635 return new Enumeration<IssueType>(this, IssueType.NOSTORE); 636 if ("exception".equals(codeString)) 637 return new Enumeration<IssueType>(this, IssueType.EXCEPTION); 638 if ("timeout".equals(codeString)) 639 return new Enumeration<IssueType>(this, IssueType.TIMEOUT); 640 if ("throttled".equals(codeString)) 641 return new Enumeration<IssueType>(this, IssueType.THROTTLED); 642 if ("informational".equals(codeString)) 643 return new Enumeration<IssueType>(this, IssueType.INFORMATIONAL); 644 throw new FHIRException("Unknown IssueType code '"+codeString+"'"); 645 } 646 public String toCode(IssueType code) { 647 if (code == IssueType.NULL) 648 return null; 649 if (code == IssueType.INVALID) 650 return "invalid"; 651 if (code == IssueType.STRUCTURE) 652 return "structure"; 653 if (code == IssueType.REQUIRED) 654 return "required"; 655 if (code == IssueType.VALUE) 656 return "value"; 657 if (code == IssueType.INVARIANT) 658 return "invariant"; 659 if (code == IssueType.SECURITY) 660 return "security"; 661 if (code == IssueType.LOGIN) 662 return "login"; 663 if (code == IssueType.UNKNOWN) 664 return "unknown"; 665 if (code == IssueType.EXPIRED) 666 return "expired"; 667 if (code == IssueType.FORBIDDEN) 668 return "forbidden"; 669 if (code == IssueType.SUPPRESSED) 670 return "suppressed"; 671 if (code == IssueType.PROCESSING) 672 return "processing"; 673 if (code == IssueType.NOTSUPPORTED) 674 return "not-supported"; 675 if (code == IssueType.DUPLICATE) 676 return "duplicate"; 677 if (code == IssueType.NOTFOUND) 678 return "not-found"; 679 if (code == IssueType.TOOLONG) 680 return "too-long"; 681 if (code == IssueType.CODEINVALID) 682 return "code-invalid"; 683 if (code == IssueType.EXTENSION) 684 return "extension"; 685 if (code == IssueType.TOOCOSTLY) 686 return "too-costly"; 687 if (code == IssueType.BUSINESSRULE) 688 return "business-rule"; 689 if (code == IssueType.CONFLICT) 690 return "conflict"; 691 if (code == IssueType.INCOMPLETE) 692 return "incomplete"; 693 if (code == IssueType.TRANSIENT) 694 return "transient"; 695 if (code == IssueType.LOCKERROR) 696 return "lock-error"; 697 if (code == IssueType.NOSTORE) 698 return "no-store"; 699 if (code == IssueType.EXCEPTION) 700 return "exception"; 701 if (code == IssueType.TIMEOUT) 702 return "timeout"; 703 if (code == IssueType.THROTTLED) 704 return "throttled"; 705 if (code == IssueType.INFORMATIONAL) 706 return "informational"; 707 return "?"; 708 } 709 public String toSystem(IssueType code) { 710 return code.getSystem(); 711 } 712 } 713 714 @Block() 715 public static class OperationOutcomeIssueComponent extends BackboneElement implements IBaseBackboneElement { 716 /** 717 * Indicates whether the issue indicates a variation from successful processing. 718 */ 719 @Child(name = "severity", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 720 @Description(shortDefinition="fatal | error | warning | information", formalDefinition="Indicates whether the issue indicates a variation from successful processing." ) 721 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/issue-severity") 722 protected Enumeration<IssueSeverity> severity; 723 724 /** 725 * Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 726 */ 727 @Child(name = "code", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 728 @Description(shortDefinition="Error or warning code", formalDefinition="Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element." ) 729 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/issue-type") 730 protected Enumeration<IssueType> code; 731 732 /** 733 * Additional details about the error. This may be a text description of the error, or a system code that identifies the error. 734 */ 735 @Child(name = "details", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 736 @Description(shortDefinition="Additional details about the error", formalDefinition="Additional details about the error. This may be a text description of the error, or a system code that identifies the error." ) 737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-outcome") 738 protected CodeableConcept details; 739 740 /** 741 * Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue. 742 */ 743 @Child(name = "diagnostics", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 744 @Description(shortDefinition="Additional diagnostic information about the issue", formalDefinition="Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue." ) 745 protected StringType diagnostics; 746 747 /** 748 * For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name. 749 */ 750 @Child(name = "location", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 751 @Description(shortDefinition="Path of element(s) related to issue", formalDefinition="For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name." ) 752 protected List<StringType> location; 753 754 /** 755 * A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. 756 */ 757 @Child(name = "expression", type = {StringType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 758 @Description(shortDefinition="FHIRPath of element(s) related to issue", formalDefinition="A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised." ) 759 protected List<StringType> expression; 760 761 private static final long serialVersionUID = -1681095438L; 762 763 /** 764 * Constructor 765 */ 766 public OperationOutcomeIssueComponent() { 767 super(); 768 } 769 770 /** 771 * Constructor 772 */ 773 public OperationOutcomeIssueComponent(Enumeration<IssueSeverity> severity, Enumeration<IssueType> code) { 774 super(); 775 this.severity = severity; 776 this.code = code; 777 } 778 779 /** 780 * @return {@link #severity} (Indicates whether the issue indicates a variation from successful processing.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 781 */ 782 public Enumeration<IssueSeverity> getSeverityElement() { 783 if (this.severity == null) 784 if (Configuration.errorOnAutoCreate()) 785 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.severity"); 786 else if (Configuration.doAutoCreate()) 787 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); // bb 788 return this.severity; 789 } 790 791 public boolean hasSeverityElement() { 792 return this.severity != null && !this.severity.isEmpty(); 793 } 794 795 public boolean hasSeverity() { 796 return this.severity != null && !this.severity.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #severity} (Indicates whether the issue indicates a variation from successful processing.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 801 */ 802 public OperationOutcomeIssueComponent setSeverityElement(Enumeration<IssueSeverity> value) { 803 this.severity = value; 804 return this; 805 } 806 807 /** 808 * @return Indicates whether the issue indicates a variation from successful processing. 809 */ 810 public IssueSeverity getSeverity() { 811 return this.severity == null ? null : this.severity.getValue(); 812 } 813 814 /** 815 * @param value Indicates whether the issue indicates a variation from successful processing. 816 */ 817 public OperationOutcomeIssueComponent setSeverity(IssueSeverity value) { 818 if (this.severity == null) 819 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); 820 this.severity.setValue(value); 821 return this; 822 } 823 824 /** 825 * @return {@link #code} (Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 826 */ 827 public Enumeration<IssueType> getCodeElement() { 828 if (this.code == null) 829 if (Configuration.errorOnAutoCreate()) 830 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.code"); 831 else if (Configuration.doAutoCreate()) 832 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); // bb 833 return this.code; 834 } 835 836 public boolean hasCodeElement() { 837 return this.code != null && !this.code.isEmpty(); 838 } 839 840 public boolean hasCode() { 841 return this.code != null && !this.code.isEmpty(); 842 } 843 844 /** 845 * @param value {@link #code} (Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 846 */ 847 public OperationOutcomeIssueComponent setCodeElement(Enumeration<IssueType> value) { 848 this.code = value; 849 return this; 850 } 851 852 /** 853 * @return Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 854 */ 855 public IssueType getCode() { 856 return this.code == null ? null : this.code.getValue(); 857 } 858 859 /** 860 * @param value Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element. 861 */ 862 public OperationOutcomeIssueComponent setCode(IssueType value) { 863 if (this.code == null) 864 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); 865 this.code.setValue(value); 866 return this; 867 } 868 869 /** 870 * @return {@link #details} (Additional details about the error. This may be a text description of the error, or a system code that identifies the error.) 871 */ 872 public CodeableConcept getDetails() { 873 if (this.details == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.details"); 876 else if (Configuration.doAutoCreate()) 877 this.details = new CodeableConcept(); // cc 878 return this.details; 879 } 880 881 public boolean hasDetails() { 882 return this.details != null && !this.details.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #details} (Additional details about the error. This may be a text description of the error, or a system code that identifies the error.) 887 */ 888 public OperationOutcomeIssueComponent setDetails(CodeableConcept value) { 889 this.details = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #diagnostics} (Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.). This is the underlying object with id, value and extensions. The accessor "getDiagnostics" gives direct access to the value 895 */ 896 public StringType getDiagnosticsElement() { 897 if (this.diagnostics == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.diagnostics"); 900 else if (Configuration.doAutoCreate()) 901 this.diagnostics = new StringType(); // bb 902 return this.diagnostics; 903 } 904 905 public boolean hasDiagnosticsElement() { 906 return this.diagnostics != null && !this.diagnostics.isEmpty(); 907 } 908 909 public boolean hasDiagnostics() { 910 return this.diagnostics != null && !this.diagnostics.isEmpty(); 911 } 912 913 /** 914 * @param value {@link #diagnostics} (Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.). This is the underlying object with id, value and extensions. The accessor "getDiagnostics" gives direct access to the value 915 */ 916 public OperationOutcomeIssueComponent setDiagnosticsElement(StringType value) { 917 this.diagnostics = value; 918 return this; 919 } 920 921 /** 922 * @return Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue. 923 */ 924 public String getDiagnostics() { 925 return this.diagnostics == null ? null : this.diagnostics.getValue(); 926 } 927 928 /** 929 * @param value Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue. 930 */ 931 public OperationOutcomeIssueComponent setDiagnostics(String value) { 932 if (Utilities.noString(value)) 933 this.diagnostics = null; 934 else { 935 if (this.diagnostics == null) 936 this.diagnostics = new StringType(); 937 this.diagnostics.setValue(value); 938 } 939 return this; 940 } 941 942 /** 943 * @return {@link #location} (For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 944 */ 945 public List<StringType> getLocation() { 946 if (this.location == null) 947 this.location = new ArrayList<StringType>(); 948 return this.location; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public OperationOutcomeIssueComponent setLocation(List<StringType> theLocation) { 955 this.location = theLocation; 956 return this; 957 } 958 959 public boolean hasLocation() { 960 if (this.location == null) 961 return false; 962 for (StringType item : this.location) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 /** 969 * @return {@link #location} (For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 970 */ 971 public StringType addLocationElement() {//2 972 StringType t = new StringType(); 973 if (this.location == null) 974 this.location = new ArrayList<StringType>(); 975 this.location.add(t); 976 return t; 977 } 978 979 /** 980 * @param value {@link #location} (For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 981 */ 982 public OperationOutcomeIssueComponent addLocation(String value) { //1 983 StringType t = new StringType(); 984 t.setValue(value); 985 if (this.location == null) 986 this.location = new ArrayList<StringType>(); 987 this.location.add(t); 988 return this; 989 } 990 991 /** 992 * @param value {@link #location} (For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be "http." + the parameter name.) 993 */ 994 public boolean hasLocation(String value) { 995 if (this.location == null) 996 return false; 997 for (StringType v : this.location) 998 if (v.getValue().equals(value)) // string 999 return true; 1000 return false; 1001 } 1002 1003 /** 1004 * @return {@link #expression} (A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.) 1005 */ 1006 public List<StringType> getExpression() { 1007 if (this.expression == null) 1008 this.expression = new ArrayList<StringType>(); 1009 return this.expression; 1010 } 1011 1012 /** 1013 * @return Returns a reference to <code>this</code> for easy method chaining 1014 */ 1015 public OperationOutcomeIssueComponent setExpression(List<StringType> theExpression) { 1016 this.expression = theExpression; 1017 return this; 1018 } 1019 1020 public boolean hasExpression() { 1021 if (this.expression == null) 1022 return false; 1023 for (StringType item : this.expression) 1024 if (!item.isEmpty()) 1025 return true; 1026 return false; 1027 } 1028 1029 /** 1030 * @return {@link #expression} (A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.) 1031 */ 1032 public StringType addExpressionElement() {//2 1033 StringType t = new StringType(); 1034 if (this.expression == null) 1035 this.expression = new ArrayList<StringType>(); 1036 this.expression.add(t); 1037 return t; 1038 } 1039 1040 /** 1041 * @param value {@link #expression} (A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.) 1042 */ 1043 public OperationOutcomeIssueComponent addExpression(String value) { //1 1044 StringType t = new StringType(); 1045 t.setValue(value); 1046 if (this.expression == null) 1047 this.expression = new ArrayList<StringType>(); 1048 this.expression.add(t); 1049 return this; 1050 } 1051 1052 /** 1053 * @param value {@link #expression} (A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.) 1054 */ 1055 public boolean hasExpression(String value) { 1056 if (this.expression == null) 1057 return false; 1058 for (StringType v : this.expression) 1059 if (v.getValue().equals(value)) // string 1060 return true; 1061 return false; 1062 } 1063 1064 protected void listChildren(List<Property> children) { 1065 super.listChildren(children); 1066 children.add(new Property("severity", "code", "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity)); 1067 children.add(new Property("code", "code", "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 0, 1, code)); 1068 children.add(new Property("details", "CodeableConcept", "Additional details about the error. This may be a text description of the error, or a system code that identifies the error.", 0, 1, details)); 1069 children.add(new Property("diagnostics", "string", "Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.", 0, 1, diagnostics)); 1070 children.add(new Property("location", "string", "For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 0, java.lang.Integer.MAX_VALUE, location)); 1071 children.add(new Property("expression", "string", "A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression)); 1072 } 1073 1074 @Override 1075 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1076 switch (_hash) { 1077 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates whether the issue indicates a variation from successful processing.", 0, 1, severity); 1078 case 3059181: /*code*/ return new Property("code", "code", "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 0, 1, code); 1079 case 1557721666: /*details*/ return new Property("details", "CodeableConcept", "Additional details about the error. This may be a text description of the error, or a system code that identifies the error.", 0, 1, details); 1080 case -740386388: /*diagnostics*/ return new Property("diagnostics", "string", "Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.", 0, 1, diagnostics); 1081 case 1901043637: /*location*/ return new Property("location", "string", "For resource issues, this will be a simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised. For HTTP errors, will be \"http.\" + the parameter name.", 0, java.lang.Integer.MAX_VALUE, location); 1082 case -1795452264: /*expression*/ return new Property("expression", "string", "A simple FHIRPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression); 1083 default: return super.getNamedProperty(_hash, _name, _checkValid); 1084 } 1085 1086 } 1087 1088 @Override 1089 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1090 switch (hash) { 1091 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<IssueSeverity> 1092 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<IssueType> 1093 case 1557721666: /*details*/ return this.details == null ? new Base[0] : new Base[] {this.details}; // CodeableConcept 1094 case -740386388: /*diagnostics*/ return this.diagnostics == null ? new Base[0] : new Base[] {this.diagnostics}; // StringType 1095 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // StringType 1096 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : this.expression.toArray(new Base[this.expression.size()]); // StringType 1097 default: return super.getProperty(hash, name, checkValid); 1098 } 1099 1100 } 1101 1102 @Override 1103 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1104 switch (hash) { 1105 case 1478300413: // severity 1106 value = new IssueSeverityEnumFactory().fromType(castToCode(value)); 1107 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1108 return value; 1109 case 3059181: // code 1110 value = new IssueTypeEnumFactory().fromType(castToCode(value)); 1111 this.code = (Enumeration) value; // Enumeration<IssueType> 1112 return value; 1113 case 1557721666: // details 1114 this.details = castToCodeableConcept(value); // CodeableConcept 1115 return value; 1116 case -740386388: // diagnostics 1117 this.diagnostics = castToString(value); // StringType 1118 return value; 1119 case 1901043637: // location 1120 this.getLocation().add(castToString(value)); // StringType 1121 return value; 1122 case -1795452264: // expression 1123 this.getExpression().add(castToString(value)); // StringType 1124 return value; 1125 default: return super.setProperty(hash, name, value); 1126 } 1127 1128 } 1129 1130 @Override 1131 public Base setProperty(String name, Base value) throws FHIRException { 1132 if (name.equals("severity")) { 1133 value = new IssueSeverityEnumFactory().fromType(castToCode(value)); 1134 this.severity = (Enumeration) value; // Enumeration<IssueSeverity> 1135 } else if (name.equals("code")) { 1136 value = new IssueTypeEnumFactory().fromType(castToCode(value)); 1137 this.code = (Enumeration) value; // Enumeration<IssueType> 1138 } else if (name.equals("details")) { 1139 this.details = castToCodeableConcept(value); // CodeableConcept 1140 } else if (name.equals("diagnostics")) { 1141 this.diagnostics = castToString(value); // StringType 1142 } else if (name.equals("location")) { 1143 this.getLocation().add(castToString(value)); 1144 } else if (name.equals("expression")) { 1145 this.getExpression().add(castToString(value)); 1146 } else 1147 return super.setProperty(name, value); 1148 return value; 1149 } 1150 1151 @Override 1152 public Base makeProperty(int hash, String name) throws FHIRException { 1153 switch (hash) { 1154 case 1478300413: return getSeverityElement(); 1155 case 3059181: return getCodeElement(); 1156 case 1557721666: return getDetails(); 1157 case -740386388: return getDiagnosticsElement(); 1158 case 1901043637: return addLocationElement(); 1159 case -1795452264: return addExpressionElement(); 1160 default: return super.makeProperty(hash, name); 1161 } 1162 1163 } 1164 1165 @Override 1166 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1167 switch (hash) { 1168 case 1478300413: /*severity*/ return new String[] {"code"}; 1169 case 3059181: /*code*/ return new String[] {"code"}; 1170 case 1557721666: /*details*/ return new String[] {"CodeableConcept"}; 1171 case -740386388: /*diagnostics*/ return new String[] {"string"}; 1172 case 1901043637: /*location*/ return new String[] {"string"}; 1173 case -1795452264: /*expression*/ return new String[] {"string"}; 1174 default: return super.getTypesForProperty(hash, name); 1175 } 1176 1177 } 1178 1179 @Override 1180 public Base addChild(String name) throws FHIRException { 1181 if (name.equals("severity")) { 1182 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.severity"); 1183 } 1184 else if (name.equals("code")) { 1185 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.code"); 1186 } 1187 else if (name.equals("details")) { 1188 this.details = new CodeableConcept(); 1189 return this.details; 1190 } 1191 else if (name.equals("diagnostics")) { 1192 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.diagnostics"); 1193 } 1194 else if (name.equals("location")) { 1195 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.location"); 1196 } 1197 else if (name.equals("expression")) { 1198 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.expression"); 1199 } 1200 else 1201 return super.addChild(name); 1202 } 1203 1204 public OperationOutcomeIssueComponent copy() { 1205 OperationOutcomeIssueComponent dst = new OperationOutcomeIssueComponent(); 1206 copyValues(dst); 1207 dst.severity = severity == null ? null : severity.copy(); 1208 dst.code = code == null ? null : code.copy(); 1209 dst.details = details == null ? null : details.copy(); 1210 dst.diagnostics = diagnostics == null ? null : diagnostics.copy(); 1211 if (location != null) { 1212 dst.location = new ArrayList<StringType>(); 1213 for (StringType i : location) 1214 dst.location.add(i.copy()); 1215 }; 1216 if (expression != null) { 1217 dst.expression = new ArrayList<StringType>(); 1218 for (StringType i : expression) 1219 dst.expression.add(i.copy()); 1220 }; 1221 return dst; 1222 } 1223 1224 @Override 1225 public boolean equalsDeep(Base other_) { 1226 if (!super.equalsDeep(other_)) 1227 return false; 1228 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1229 return false; 1230 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1231 return compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) && compareDeep(details, o.details, true) 1232 && compareDeep(diagnostics, o.diagnostics, true) && compareDeep(location, o.location, true) && compareDeep(expression, o.expression, true) 1233 ; 1234 } 1235 1236 @Override 1237 public boolean equalsShallow(Base other_) { 1238 if (!super.equalsShallow(other_)) 1239 return false; 1240 if (!(other_ instanceof OperationOutcomeIssueComponent)) 1241 return false; 1242 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other_; 1243 return compareValues(severity, o.severity, true) && compareValues(code, o.code, true) && compareValues(diagnostics, o.diagnostics, true) 1244 && compareValues(location, o.location, true) && compareValues(expression, o.expression, true); 1245 } 1246 1247 public boolean isEmpty() { 1248 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(severity, code, details 1249 , diagnostics, location, expression); 1250 } 1251 1252 public String fhirType() { 1253 return "OperationOutcome.issue"; 1254 1255 } 1256 1257 } 1258 1259 /** 1260 * An error, warning or information message that results from a system action. 1261 */ 1262 @Child(name = "issue", type = {}, order=0, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1263 @Description(shortDefinition="A single issue associated with the action", formalDefinition="An error, warning or information message that results from a system action." ) 1264 protected List<OperationOutcomeIssueComponent> issue; 1265 1266 private static final long serialVersionUID = -152150052L; 1267 1268 /** 1269 * Constructor 1270 */ 1271 public OperationOutcome() { 1272 super(); 1273 } 1274 1275 /** 1276 * @return {@link #issue} (An error, warning or information message that results from a system action.) 1277 */ 1278 public List<OperationOutcomeIssueComponent> getIssue() { 1279 if (this.issue == null) 1280 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1281 return this.issue; 1282 } 1283 1284 /** 1285 * @return Returns a reference to <code>this</code> for easy method chaining 1286 */ 1287 public OperationOutcome setIssue(List<OperationOutcomeIssueComponent> theIssue) { 1288 this.issue = theIssue; 1289 return this; 1290 } 1291 1292 public boolean hasIssue() { 1293 if (this.issue == null) 1294 return false; 1295 for (OperationOutcomeIssueComponent item : this.issue) 1296 if (!item.isEmpty()) 1297 return true; 1298 return false; 1299 } 1300 1301 public OperationOutcomeIssueComponent addIssue() { //3 1302 OperationOutcomeIssueComponent t = new OperationOutcomeIssueComponent(); 1303 if (this.issue == null) 1304 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1305 this.issue.add(t); 1306 return t; 1307 } 1308 1309 public OperationOutcome addIssue(OperationOutcomeIssueComponent t) { //3 1310 if (t == null) 1311 return this; 1312 if (this.issue == null) 1313 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1314 this.issue.add(t); 1315 return this; 1316 } 1317 1318 /** 1319 * @return The first repetition of repeating field {@link #issue}, creating it if it does not already exist 1320 */ 1321 public OperationOutcomeIssueComponent getIssueFirstRep() { 1322 if (getIssue().isEmpty()) { 1323 addIssue(); 1324 } 1325 return getIssue().get(0); 1326 } 1327 1328 protected void listChildren(List<Property> children) { 1329 super.listChildren(children); 1330 children.add(new Property("issue", "", "An error, warning or information message that results from a system action.", 0, java.lang.Integer.MAX_VALUE, issue)); 1331 } 1332 1333 @Override 1334 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1335 switch (_hash) { 1336 case 100509913: /*issue*/ return new Property("issue", "", "An error, warning or information message that results from a system action.", 0, java.lang.Integer.MAX_VALUE, issue); 1337 default: return super.getNamedProperty(_hash, _name, _checkValid); 1338 } 1339 1340 } 1341 1342 @Override 1343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1344 switch (hash) { 1345 case 100509913: /*issue*/ return this.issue == null ? new Base[0] : this.issue.toArray(new Base[this.issue.size()]); // OperationOutcomeIssueComponent 1346 default: return super.getProperty(hash, name, checkValid); 1347 } 1348 1349 } 1350 1351 @Override 1352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1353 switch (hash) { 1354 case 100509913: // issue 1355 this.getIssue().add((OperationOutcomeIssueComponent) value); // OperationOutcomeIssueComponent 1356 return value; 1357 default: return super.setProperty(hash, name, value); 1358 } 1359 1360 } 1361 1362 @Override 1363 public Base setProperty(String name, Base value) throws FHIRException { 1364 if (name.equals("issue")) { 1365 this.getIssue().add((OperationOutcomeIssueComponent) value); 1366 } else 1367 return super.setProperty(name, value); 1368 return value; 1369 } 1370 1371 @Override 1372 public Base makeProperty(int hash, String name) throws FHIRException { 1373 switch (hash) { 1374 case 100509913: return addIssue(); 1375 default: return super.makeProperty(hash, name); 1376 } 1377 1378 } 1379 1380 @Override 1381 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1382 switch (hash) { 1383 case 100509913: /*issue*/ return new String[] {}; 1384 default: return super.getTypesForProperty(hash, name); 1385 } 1386 1387 } 1388 1389 @Override 1390 public Base addChild(String name) throws FHIRException { 1391 if (name.equals("issue")) { 1392 return addIssue(); 1393 } 1394 else 1395 return super.addChild(name); 1396 } 1397 1398 public String fhirType() { 1399 return "OperationOutcome"; 1400 1401 } 1402 1403 public OperationOutcome copy() { 1404 OperationOutcome dst = new OperationOutcome(); 1405 copyValues(dst); 1406 if (issue != null) { 1407 dst.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1408 for (OperationOutcomeIssueComponent i : issue) 1409 dst.issue.add(i.copy()); 1410 }; 1411 return dst; 1412 } 1413 1414 protected OperationOutcome typedCopy() { 1415 return copy(); 1416 } 1417 1418 @Override 1419 public boolean equalsDeep(Base other_) { 1420 if (!super.equalsDeep(other_)) 1421 return false; 1422 if (!(other_ instanceof OperationOutcome)) 1423 return false; 1424 OperationOutcome o = (OperationOutcome) other_; 1425 return compareDeep(issue, o.issue, true); 1426 } 1427 1428 @Override 1429 public boolean equalsShallow(Base other_) { 1430 if (!super.equalsShallow(other_)) 1431 return false; 1432 if (!(other_ instanceof OperationOutcome)) 1433 return false; 1434 OperationOutcome o = (OperationOutcome) other_; 1435 return true; 1436 } 1437 1438 public boolean isEmpty() { 1439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(issue); 1440 } 1441 1442 @Override 1443 public ResourceType getResourceType() { 1444 return ResourceType.OperationOutcome; 1445 } 1446 1447 1448}