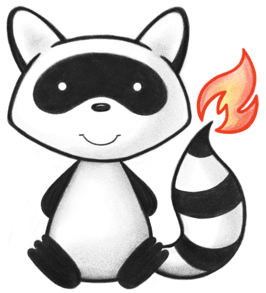
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 050 */ 051@ResourceDef(name="Organization", profile="http://hl7.org/fhir/Profile/Organization") 052public class Organization extends DomainResource { 053 054 @Block() 055 public static class OrganizationContactComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Indicates a purpose for which the contact can be reached. 058 */ 059 @Child(name = "purpose", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 060 @Description(shortDefinition="The type of contact", formalDefinition="Indicates a purpose for which the contact can be reached." ) 061 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contactentity-type") 062 protected CodeableConcept purpose; 063 064 /** 065 * A name associated with the contact. 066 */ 067 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=1, modifier=false, summary=false) 068 @Description(shortDefinition="A name associated with the contact", formalDefinition="A name associated with the contact." ) 069 protected HumanName name; 070 071 /** 072 * A contact detail (e.g. a telephone number or an email address) by which the party may be contacted. 073 */ 074 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 075 @Description(shortDefinition="Contact details (telephone, email, etc.) for a contact", formalDefinition="A contact detail (e.g. a telephone number or an email address) by which the party may be contacted." ) 076 protected List<ContactPoint> telecom; 077 078 /** 079 * Visiting or postal addresses for the contact. 080 */ 081 @Child(name = "address", type = {Address.class}, order=4, min=0, max=1, modifier=false, summary=false) 082 @Description(shortDefinition="Visiting or postal addresses for the contact", formalDefinition="Visiting or postal addresses for the contact." ) 083 protected Address address; 084 085 private static final long serialVersionUID = 1831121305L; 086 087 /** 088 * Constructor 089 */ 090 public OrganizationContactComponent() { 091 super(); 092 } 093 094 /** 095 * @return {@link #purpose} (Indicates a purpose for which the contact can be reached.) 096 */ 097 public CodeableConcept getPurpose() { 098 if (this.purpose == null) 099 if (Configuration.errorOnAutoCreate()) 100 throw new Error("Attempt to auto-create OrganizationContactComponent.purpose"); 101 else if (Configuration.doAutoCreate()) 102 this.purpose = new CodeableConcept(); // cc 103 return this.purpose; 104 } 105 106 public boolean hasPurpose() { 107 return this.purpose != null && !this.purpose.isEmpty(); 108 } 109 110 /** 111 * @param value {@link #purpose} (Indicates a purpose for which the contact can be reached.) 112 */ 113 public OrganizationContactComponent setPurpose(CodeableConcept value) { 114 this.purpose = value; 115 return this; 116 } 117 118 /** 119 * @return {@link #name} (A name associated with the contact.) 120 */ 121 public HumanName getName() { 122 if (this.name == null) 123 if (Configuration.errorOnAutoCreate()) 124 throw new Error("Attempt to auto-create OrganizationContactComponent.name"); 125 else if (Configuration.doAutoCreate()) 126 this.name = new HumanName(); // cc 127 return this.name; 128 } 129 130 public boolean hasName() { 131 return this.name != null && !this.name.isEmpty(); 132 } 133 134 /** 135 * @param value {@link #name} (A name associated with the contact.) 136 */ 137 public OrganizationContactComponent setName(HumanName value) { 138 this.name = value; 139 return this; 140 } 141 142 /** 143 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.) 144 */ 145 public List<ContactPoint> getTelecom() { 146 if (this.telecom == null) 147 this.telecom = new ArrayList<ContactPoint>(); 148 return this.telecom; 149 } 150 151 /** 152 * @return Returns a reference to <code>this</code> for easy method chaining 153 */ 154 public OrganizationContactComponent setTelecom(List<ContactPoint> theTelecom) { 155 this.telecom = theTelecom; 156 return this; 157 } 158 159 public boolean hasTelecom() { 160 if (this.telecom == null) 161 return false; 162 for (ContactPoint item : this.telecom) 163 if (!item.isEmpty()) 164 return true; 165 return false; 166 } 167 168 public ContactPoint addTelecom() { //3 169 ContactPoint t = new ContactPoint(); 170 if (this.telecom == null) 171 this.telecom = new ArrayList<ContactPoint>(); 172 this.telecom.add(t); 173 return t; 174 } 175 176 public OrganizationContactComponent addTelecom(ContactPoint t) { //3 177 if (t == null) 178 return this; 179 if (this.telecom == null) 180 this.telecom = new ArrayList<ContactPoint>(); 181 this.telecom.add(t); 182 return this; 183 } 184 185 /** 186 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 187 */ 188 public ContactPoint getTelecomFirstRep() { 189 if (getTelecom().isEmpty()) { 190 addTelecom(); 191 } 192 return getTelecom().get(0); 193 } 194 195 /** 196 * @return {@link #address} (Visiting or postal addresses for the contact.) 197 */ 198 public Address getAddress() { 199 if (this.address == null) 200 if (Configuration.errorOnAutoCreate()) 201 throw new Error("Attempt to auto-create OrganizationContactComponent.address"); 202 else if (Configuration.doAutoCreate()) 203 this.address = new Address(); // cc 204 return this.address; 205 } 206 207 public boolean hasAddress() { 208 return this.address != null && !this.address.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #address} (Visiting or postal addresses for the contact.) 213 */ 214 public OrganizationContactComponent setAddress(Address value) { 215 this.address = value; 216 return this; 217 } 218 219 protected void listChildren(List<Property> children) { 220 super.listChildren(children); 221 children.add(new Property("purpose", "CodeableConcept", "Indicates a purpose for which the contact can be reached.", 0, 1, purpose)); 222 children.add(new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name)); 223 children.add(new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom)); 224 children.add(new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, address)); 225 } 226 227 @Override 228 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 229 switch (_hash) { 230 case -220463842: /*purpose*/ return new Property("purpose", "CodeableConcept", "Indicates a purpose for which the contact can be reached.", 0, 1, purpose); 231 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name); 232 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom); 233 case -1147692044: /*address*/ return new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, address); 234 default: return super.getNamedProperty(_hash, _name, _checkValid); 235 } 236 237 } 238 239 @Override 240 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 241 switch (hash) { 242 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // CodeableConcept 243 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // HumanName 244 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 245 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 246 default: return super.getProperty(hash, name, checkValid); 247 } 248 249 } 250 251 @Override 252 public Base setProperty(int hash, String name, Base value) throws FHIRException { 253 switch (hash) { 254 case -220463842: // purpose 255 this.purpose = castToCodeableConcept(value); // CodeableConcept 256 return value; 257 case 3373707: // name 258 this.name = castToHumanName(value); // HumanName 259 return value; 260 case -1429363305: // telecom 261 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 262 return value; 263 case -1147692044: // address 264 this.address = castToAddress(value); // Address 265 return value; 266 default: return super.setProperty(hash, name, value); 267 } 268 269 } 270 271 @Override 272 public Base setProperty(String name, Base value) throws FHIRException { 273 if (name.equals("purpose")) { 274 this.purpose = castToCodeableConcept(value); // CodeableConcept 275 } else if (name.equals("name")) { 276 this.name = castToHumanName(value); // HumanName 277 } else if (name.equals("telecom")) { 278 this.getTelecom().add(castToContactPoint(value)); 279 } else if (name.equals("address")) { 280 this.address = castToAddress(value); // Address 281 } else 282 return super.setProperty(name, value); 283 return value; 284 } 285 286 @Override 287 public Base makeProperty(int hash, String name) throws FHIRException { 288 switch (hash) { 289 case -220463842: return getPurpose(); 290 case 3373707: return getName(); 291 case -1429363305: return addTelecom(); 292 case -1147692044: return getAddress(); 293 default: return super.makeProperty(hash, name); 294 } 295 296 } 297 298 @Override 299 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 300 switch (hash) { 301 case -220463842: /*purpose*/ return new String[] {"CodeableConcept"}; 302 case 3373707: /*name*/ return new String[] {"HumanName"}; 303 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 304 case -1147692044: /*address*/ return new String[] {"Address"}; 305 default: return super.getTypesForProperty(hash, name); 306 } 307 308 } 309 310 @Override 311 public Base addChild(String name) throws FHIRException { 312 if (name.equals("purpose")) { 313 this.purpose = new CodeableConcept(); 314 return this.purpose; 315 } 316 else if (name.equals("name")) { 317 this.name = new HumanName(); 318 return this.name; 319 } 320 else if (name.equals("telecom")) { 321 return addTelecom(); 322 } 323 else if (name.equals("address")) { 324 this.address = new Address(); 325 return this.address; 326 } 327 else 328 return super.addChild(name); 329 } 330 331 public OrganizationContactComponent copy() { 332 OrganizationContactComponent dst = new OrganizationContactComponent(); 333 copyValues(dst); 334 dst.purpose = purpose == null ? null : purpose.copy(); 335 dst.name = name == null ? null : name.copy(); 336 if (telecom != null) { 337 dst.telecom = new ArrayList<ContactPoint>(); 338 for (ContactPoint i : telecom) 339 dst.telecom.add(i.copy()); 340 }; 341 dst.address = address == null ? null : address.copy(); 342 return dst; 343 } 344 345 @Override 346 public boolean equalsDeep(Base other_) { 347 if (!super.equalsDeep(other_)) 348 return false; 349 if (!(other_ instanceof OrganizationContactComponent)) 350 return false; 351 OrganizationContactComponent o = (OrganizationContactComponent) other_; 352 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 353 && compareDeep(address, o.address, true); 354 } 355 356 @Override 357 public boolean equalsShallow(Base other_) { 358 if (!super.equalsShallow(other_)) 359 return false; 360 if (!(other_ instanceof OrganizationContactComponent)) 361 return false; 362 OrganizationContactComponent o = (OrganizationContactComponent) other_; 363 return true; 364 } 365 366 public boolean isEmpty() { 367 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, name, telecom, address 368 ); 369 } 370 371 public String fhirType() { 372 return "Organization.contact"; 373 374 } 375 376 } 377 378 /** 379 * Identifier for the organization that is used to identify the organization across multiple disparate systems. 380 */ 381 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 382 @Description(shortDefinition="Identifies this organization across multiple systems", formalDefinition="Identifier for the organization that is used to identify the organization across multiple disparate systems." ) 383 protected List<Identifier> identifier; 384 385 /** 386 * Whether the organization's record is still in active use. 387 */ 388 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 389 @Description(shortDefinition="Whether the organization's record is still in active use", formalDefinition="Whether the organization's record is still in active use." ) 390 protected BooleanType active; 391 392 /** 393 * The kind(s) of organization that this is. 394 */ 395 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 396 @Description(shortDefinition="Kind of organization", formalDefinition="The kind(s) of organization that this is." ) 397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/organization-type") 398 protected List<CodeableConcept> type; 399 400 /** 401 * A name associated with the organization. 402 */ 403 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 404 @Description(shortDefinition="Name used for the organization", formalDefinition="A name associated with the organization." ) 405 protected StringType name; 406 407 /** 408 * A list of alternate names that the organization is known as, or was known as in the past. 409 */ 410 @Child(name = "alias", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 411 @Description(shortDefinition="A list of alternate names that the organization is known as, or was known as in the past", formalDefinition="A list of alternate names that the organization is known as, or was known as in the past." ) 412 protected List<StringType> alias; 413 414 /** 415 * A contact detail for the organization. 416 */ 417 @Child(name = "telecom", type = {ContactPoint.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 418 @Description(shortDefinition="A contact detail for the organization", formalDefinition="A contact detail for the organization." ) 419 protected List<ContactPoint> telecom; 420 421 /** 422 * An address for the organization. 423 */ 424 @Child(name = "address", type = {Address.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 425 @Description(shortDefinition="An address for the organization", formalDefinition="An address for the organization." ) 426 protected List<Address> address; 427 428 /** 429 * The organization of which this organization forms a part. 430 */ 431 @Child(name = "partOf", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=true) 432 @Description(shortDefinition="The organization of which this organization forms a part", formalDefinition="The organization of which this organization forms a part." ) 433 protected Reference partOf; 434 435 /** 436 * The actual object that is the target of the reference (The organization of which this organization forms a part.) 437 */ 438 protected Organization partOfTarget; 439 440 /** 441 * Contact for the organization for a certain purpose. 442 */ 443 @Child(name = "contact", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 444 @Description(shortDefinition="Contact for the organization for a certain purpose", formalDefinition="Contact for the organization for a certain purpose." ) 445 protected List<OrganizationContactComponent> contact; 446 447 /** 448 * Technical endpoints providing access to services operated for the organization. 449 */ 450 @Child(name = "endpoint", type = {Endpoint.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 451 @Description(shortDefinition="Technical endpoints providing access to services operated for the organization", formalDefinition="Technical endpoints providing access to services operated for the organization." ) 452 protected List<Reference> endpoint; 453 /** 454 * The actual objects that are the target of the reference (Technical endpoints providing access to services operated for the organization.) 455 */ 456 protected List<Endpoint> endpointTarget; 457 458 459 private static final long serialVersionUID = -2113244111L; 460 461 /** 462 * Constructor 463 */ 464 public Organization() { 465 super(); 466 } 467 468 /** 469 * @return {@link #identifier} (Identifier for the organization that is used to identify the organization across multiple disparate systems.) 470 */ 471 public List<Identifier> getIdentifier() { 472 if (this.identifier == null) 473 this.identifier = new ArrayList<Identifier>(); 474 return this.identifier; 475 } 476 477 /** 478 * @return Returns a reference to <code>this</code> for easy method chaining 479 */ 480 public Organization setIdentifier(List<Identifier> theIdentifier) { 481 this.identifier = theIdentifier; 482 return this; 483 } 484 485 public boolean hasIdentifier() { 486 if (this.identifier == null) 487 return false; 488 for (Identifier item : this.identifier) 489 if (!item.isEmpty()) 490 return true; 491 return false; 492 } 493 494 public Identifier addIdentifier() { //3 495 Identifier t = new Identifier(); 496 if (this.identifier == null) 497 this.identifier = new ArrayList<Identifier>(); 498 this.identifier.add(t); 499 return t; 500 } 501 502 public Organization addIdentifier(Identifier t) { //3 503 if (t == null) 504 return this; 505 if (this.identifier == null) 506 this.identifier = new ArrayList<Identifier>(); 507 this.identifier.add(t); 508 return this; 509 } 510 511 /** 512 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 513 */ 514 public Identifier getIdentifierFirstRep() { 515 if (getIdentifier().isEmpty()) { 516 addIdentifier(); 517 } 518 return getIdentifier().get(0); 519 } 520 521 /** 522 * @return {@link #active} (Whether the organization's record is still in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 523 */ 524 public BooleanType getActiveElement() { 525 if (this.active == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create Organization.active"); 528 else if (Configuration.doAutoCreate()) 529 this.active = new BooleanType(); // bb 530 return this.active; 531 } 532 533 public boolean hasActiveElement() { 534 return this.active != null && !this.active.isEmpty(); 535 } 536 537 public boolean hasActive() { 538 return this.active != null && !this.active.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #active} (Whether the organization's record is still in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 543 */ 544 public Organization setActiveElement(BooleanType value) { 545 this.active = value; 546 return this; 547 } 548 549 /** 550 * @return Whether the organization's record is still in active use. 551 */ 552 public boolean getActive() { 553 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 554 } 555 556 /** 557 * @param value Whether the organization's record is still in active use. 558 */ 559 public Organization setActive(boolean value) { 560 if (this.active == null) 561 this.active = new BooleanType(); 562 this.active.setValue(value); 563 return this; 564 } 565 566 /** 567 * @return {@link #type} (The kind(s) of organization that this is.) 568 */ 569 public List<CodeableConcept> getType() { 570 if (this.type == null) 571 this.type = new ArrayList<CodeableConcept>(); 572 return this.type; 573 } 574 575 /** 576 * @return Returns a reference to <code>this</code> for easy method chaining 577 */ 578 public Organization setType(List<CodeableConcept> theType) { 579 this.type = theType; 580 return this; 581 } 582 583 public boolean hasType() { 584 if (this.type == null) 585 return false; 586 for (CodeableConcept item : this.type) 587 if (!item.isEmpty()) 588 return true; 589 return false; 590 } 591 592 public CodeableConcept addType() { //3 593 CodeableConcept t = new CodeableConcept(); 594 if (this.type == null) 595 this.type = new ArrayList<CodeableConcept>(); 596 this.type.add(t); 597 return t; 598 } 599 600 public Organization addType(CodeableConcept t) { //3 601 if (t == null) 602 return this; 603 if (this.type == null) 604 this.type = new ArrayList<CodeableConcept>(); 605 this.type.add(t); 606 return this; 607 } 608 609 /** 610 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 611 */ 612 public CodeableConcept getTypeFirstRep() { 613 if (getType().isEmpty()) { 614 addType(); 615 } 616 return getType().get(0); 617 } 618 619 /** 620 * @return {@link #name} (A name associated with the organization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 621 */ 622 public StringType getNameElement() { 623 if (this.name == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create Organization.name"); 626 else if (Configuration.doAutoCreate()) 627 this.name = new StringType(); // bb 628 return this.name; 629 } 630 631 public boolean hasNameElement() { 632 return this.name != null && !this.name.isEmpty(); 633 } 634 635 public boolean hasName() { 636 return this.name != null && !this.name.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #name} (A name associated with the organization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 641 */ 642 public Organization setNameElement(StringType value) { 643 this.name = value; 644 return this; 645 } 646 647 /** 648 * @return A name associated with the organization. 649 */ 650 public String getName() { 651 return this.name == null ? null : this.name.getValue(); 652 } 653 654 /** 655 * @param value A name associated with the organization. 656 */ 657 public Organization setName(String value) { 658 if (Utilities.noString(value)) 659 this.name = null; 660 else { 661 if (this.name == null) 662 this.name = new StringType(); 663 this.name.setValue(value); 664 } 665 return this; 666 } 667 668 /** 669 * @return {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 670 */ 671 public List<StringType> getAlias() { 672 if (this.alias == null) 673 this.alias = new ArrayList<StringType>(); 674 return this.alias; 675 } 676 677 /** 678 * @return Returns a reference to <code>this</code> for easy method chaining 679 */ 680 public Organization setAlias(List<StringType> theAlias) { 681 this.alias = theAlias; 682 return this; 683 } 684 685 public boolean hasAlias() { 686 if (this.alias == null) 687 return false; 688 for (StringType item : this.alias) 689 if (!item.isEmpty()) 690 return true; 691 return false; 692 } 693 694 /** 695 * @return {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 696 */ 697 public StringType addAliasElement() {//2 698 StringType t = new StringType(); 699 if (this.alias == null) 700 this.alias = new ArrayList<StringType>(); 701 this.alias.add(t); 702 return t; 703 } 704 705 /** 706 * @param value {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 707 */ 708 public Organization addAlias(String value) { //1 709 StringType t = new StringType(); 710 t.setValue(value); 711 if (this.alias == null) 712 this.alias = new ArrayList<StringType>(); 713 this.alias.add(t); 714 return this; 715 } 716 717 /** 718 * @param value {@link #alias} (A list of alternate names that the organization is known as, or was known as in the past.) 719 */ 720 public boolean hasAlias(String value) { 721 if (this.alias == null) 722 return false; 723 for (StringType v : this.alias) 724 if (v.getValue().equals(value)) // string 725 return true; 726 return false; 727 } 728 729 /** 730 * @return {@link #telecom} (A contact detail for the organization.) 731 */ 732 public List<ContactPoint> getTelecom() { 733 if (this.telecom == null) 734 this.telecom = new ArrayList<ContactPoint>(); 735 return this.telecom; 736 } 737 738 /** 739 * @return Returns a reference to <code>this</code> for easy method chaining 740 */ 741 public Organization setTelecom(List<ContactPoint> theTelecom) { 742 this.telecom = theTelecom; 743 return this; 744 } 745 746 public boolean hasTelecom() { 747 if (this.telecom == null) 748 return false; 749 for (ContactPoint item : this.telecom) 750 if (!item.isEmpty()) 751 return true; 752 return false; 753 } 754 755 public ContactPoint addTelecom() { //3 756 ContactPoint t = new ContactPoint(); 757 if (this.telecom == null) 758 this.telecom = new ArrayList<ContactPoint>(); 759 this.telecom.add(t); 760 return t; 761 } 762 763 public Organization addTelecom(ContactPoint t) { //3 764 if (t == null) 765 return this; 766 if (this.telecom == null) 767 this.telecom = new ArrayList<ContactPoint>(); 768 this.telecom.add(t); 769 return this; 770 } 771 772 /** 773 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 774 */ 775 public ContactPoint getTelecomFirstRep() { 776 if (getTelecom().isEmpty()) { 777 addTelecom(); 778 } 779 return getTelecom().get(0); 780 } 781 782 /** 783 * @return {@link #address} (An address for the organization.) 784 */ 785 public List<Address> getAddress() { 786 if (this.address == null) 787 this.address = new ArrayList<Address>(); 788 return this.address; 789 } 790 791 /** 792 * @return Returns a reference to <code>this</code> for easy method chaining 793 */ 794 public Organization setAddress(List<Address> theAddress) { 795 this.address = theAddress; 796 return this; 797 } 798 799 public boolean hasAddress() { 800 if (this.address == null) 801 return false; 802 for (Address item : this.address) 803 if (!item.isEmpty()) 804 return true; 805 return false; 806 } 807 808 public Address addAddress() { //3 809 Address t = new Address(); 810 if (this.address == null) 811 this.address = new ArrayList<Address>(); 812 this.address.add(t); 813 return t; 814 } 815 816 public Organization addAddress(Address t) { //3 817 if (t == null) 818 return this; 819 if (this.address == null) 820 this.address = new ArrayList<Address>(); 821 this.address.add(t); 822 return this; 823 } 824 825 /** 826 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist 827 */ 828 public Address getAddressFirstRep() { 829 if (getAddress().isEmpty()) { 830 addAddress(); 831 } 832 return getAddress().get(0); 833 } 834 835 /** 836 * @return {@link #partOf} (The organization of which this organization forms a part.) 837 */ 838 public Reference getPartOf() { 839 if (this.partOf == null) 840 if (Configuration.errorOnAutoCreate()) 841 throw new Error("Attempt to auto-create Organization.partOf"); 842 else if (Configuration.doAutoCreate()) 843 this.partOf = new Reference(); // cc 844 return this.partOf; 845 } 846 847 public boolean hasPartOf() { 848 return this.partOf != null && !this.partOf.isEmpty(); 849 } 850 851 /** 852 * @param value {@link #partOf} (The organization of which this organization forms a part.) 853 */ 854 public Organization setPartOf(Reference value) { 855 this.partOf = value; 856 return this; 857 } 858 859 /** 860 * @return {@link #partOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization of which this organization forms a part.) 861 */ 862 public Organization getPartOfTarget() { 863 if (this.partOfTarget == null) 864 if (Configuration.errorOnAutoCreate()) 865 throw new Error("Attempt to auto-create Organization.partOf"); 866 else if (Configuration.doAutoCreate()) 867 this.partOfTarget = new Organization(); // aa 868 return this.partOfTarget; 869 } 870 871 /** 872 * @param value {@link #partOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization of which this organization forms a part.) 873 */ 874 public Organization setPartOfTarget(Organization value) { 875 this.partOfTarget = value; 876 return this; 877 } 878 879 /** 880 * @return {@link #contact} (Contact for the organization for a certain purpose.) 881 */ 882 public List<OrganizationContactComponent> getContact() { 883 if (this.contact == null) 884 this.contact = new ArrayList<OrganizationContactComponent>(); 885 return this.contact; 886 } 887 888 /** 889 * @return Returns a reference to <code>this</code> for easy method chaining 890 */ 891 public Organization setContact(List<OrganizationContactComponent> theContact) { 892 this.contact = theContact; 893 return this; 894 } 895 896 public boolean hasContact() { 897 if (this.contact == null) 898 return false; 899 for (OrganizationContactComponent item : this.contact) 900 if (!item.isEmpty()) 901 return true; 902 return false; 903 } 904 905 public OrganizationContactComponent addContact() { //3 906 OrganizationContactComponent t = new OrganizationContactComponent(); 907 if (this.contact == null) 908 this.contact = new ArrayList<OrganizationContactComponent>(); 909 this.contact.add(t); 910 return t; 911 } 912 913 public Organization addContact(OrganizationContactComponent t) { //3 914 if (t == null) 915 return this; 916 if (this.contact == null) 917 this.contact = new ArrayList<OrganizationContactComponent>(); 918 this.contact.add(t); 919 return this; 920 } 921 922 /** 923 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 924 */ 925 public OrganizationContactComponent getContactFirstRep() { 926 if (getContact().isEmpty()) { 927 addContact(); 928 } 929 return getContact().get(0); 930 } 931 932 /** 933 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the organization.) 934 */ 935 public List<Reference> getEndpoint() { 936 if (this.endpoint == null) 937 this.endpoint = new ArrayList<Reference>(); 938 return this.endpoint; 939 } 940 941 /** 942 * @return Returns a reference to <code>this</code> for easy method chaining 943 */ 944 public Organization setEndpoint(List<Reference> theEndpoint) { 945 this.endpoint = theEndpoint; 946 return this; 947 } 948 949 public boolean hasEndpoint() { 950 if (this.endpoint == null) 951 return false; 952 for (Reference item : this.endpoint) 953 if (!item.isEmpty()) 954 return true; 955 return false; 956 } 957 958 public Reference addEndpoint() { //3 959 Reference t = new Reference(); 960 if (this.endpoint == null) 961 this.endpoint = new ArrayList<Reference>(); 962 this.endpoint.add(t); 963 return t; 964 } 965 966 public Organization addEndpoint(Reference t) { //3 967 if (t == null) 968 return this; 969 if (this.endpoint == null) 970 this.endpoint = new ArrayList<Reference>(); 971 this.endpoint.add(t); 972 return this; 973 } 974 975 /** 976 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 977 */ 978 public Reference getEndpointFirstRep() { 979 if (getEndpoint().isEmpty()) { 980 addEndpoint(); 981 } 982 return getEndpoint().get(0); 983 } 984 985 /** 986 * @deprecated Use Reference#setResource(IBaseResource) instead 987 */ 988 @Deprecated 989 public List<Endpoint> getEndpointTarget() { 990 if (this.endpointTarget == null) 991 this.endpointTarget = new ArrayList<Endpoint>(); 992 return this.endpointTarget; 993 } 994 995 /** 996 * @deprecated Use Reference#setResource(IBaseResource) instead 997 */ 998 @Deprecated 999 public Endpoint addEndpointTarget() { 1000 Endpoint r = new Endpoint(); 1001 if (this.endpointTarget == null) 1002 this.endpointTarget = new ArrayList<Endpoint>(); 1003 this.endpointTarget.add(r); 1004 return r; 1005 } 1006 1007 protected void listChildren(List<Property> children) { 1008 super.listChildren(children); 1009 children.add(new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1010 children.add(new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 1, active)); 1011 children.add(new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, java.lang.Integer.MAX_VALUE, type)); 1012 children.add(new Property("name", "string", "A name associated with the organization.", 0, 1, name)); 1013 children.add(new Property("alias", "string", "A list of alternate names that the organization is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias)); 1014 children.add(new Property("telecom", "ContactPoint", "A contact detail for the organization.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1015 children.add(new Property("address", "Address", "An address for the organization.", 0, java.lang.Integer.MAX_VALUE, address)); 1016 children.add(new Property("partOf", "Reference(Organization)", "The organization of which this organization forms a part.", 0, 1, partOf)); 1017 children.add(new Property("contact", "", "Contact for the organization for a certain purpose.", 0, java.lang.Integer.MAX_VALUE, contact)); 1018 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the organization.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1019 } 1020 1021 @Override 1022 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1023 switch (_hash) { 1024 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1025 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 1, active); 1026 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind(s) of organization that this is.", 0, java.lang.Integer.MAX_VALUE, type); 1027 case 3373707: /*name*/ return new Property("name", "string", "A name associated with the organization.", 0, 1, name); 1028 case 92902992: /*alias*/ return new Property("alias", "string", "A list of alternate names that the organization is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias); 1029 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the organization.", 0, java.lang.Integer.MAX_VALUE, telecom); 1030 case -1147692044: /*address*/ return new Property("address", "Address", "An address for the organization.", 0, java.lang.Integer.MAX_VALUE, address); 1031 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Organization)", "The organization of which this organization forms a part.", 0, 1, partOf); 1032 case 951526432: /*contact*/ return new Property("contact", "", "Contact for the organization for a certain purpose.", 0, java.lang.Integer.MAX_VALUE, contact); 1033 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the organization.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1034 default: return super.getNamedProperty(_hash, _name, _checkValid); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1041 switch (hash) { 1042 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1043 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1044 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1045 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1046 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 1047 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1048 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1049 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 1050 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // OrganizationContactComponent 1051 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1052 default: return super.getProperty(hash, name, checkValid); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1059 switch (hash) { 1060 case -1618432855: // identifier 1061 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1062 return value; 1063 case -1422950650: // active 1064 this.active = castToBoolean(value); // BooleanType 1065 return value; 1066 case 3575610: // type 1067 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1068 return value; 1069 case 3373707: // name 1070 this.name = castToString(value); // StringType 1071 return value; 1072 case 92902992: // alias 1073 this.getAlias().add(castToString(value)); // StringType 1074 return value; 1075 case -1429363305: // telecom 1076 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1077 return value; 1078 case -1147692044: // address 1079 this.getAddress().add(castToAddress(value)); // Address 1080 return value; 1081 case -995410646: // partOf 1082 this.partOf = castToReference(value); // Reference 1083 return value; 1084 case 951526432: // contact 1085 this.getContact().add((OrganizationContactComponent) value); // OrganizationContactComponent 1086 return value; 1087 case 1741102485: // endpoint 1088 this.getEndpoint().add(castToReference(value)); // Reference 1089 return value; 1090 default: return super.setProperty(hash, name, value); 1091 } 1092 1093 } 1094 1095 @Override 1096 public Base setProperty(String name, Base value) throws FHIRException { 1097 if (name.equals("identifier")) { 1098 this.getIdentifier().add(castToIdentifier(value)); 1099 } else if (name.equals("active")) { 1100 this.active = castToBoolean(value); // BooleanType 1101 } else if (name.equals("type")) { 1102 this.getType().add(castToCodeableConcept(value)); 1103 } else if (name.equals("name")) { 1104 this.name = castToString(value); // StringType 1105 } else if (name.equals("alias")) { 1106 this.getAlias().add(castToString(value)); 1107 } else if (name.equals("telecom")) { 1108 this.getTelecom().add(castToContactPoint(value)); 1109 } else if (name.equals("address")) { 1110 this.getAddress().add(castToAddress(value)); 1111 } else if (name.equals("partOf")) { 1112 this.partOf = castToReference(value); // Reference 1113 } else if (name.equals("contact")) { 1114 this.getContact().add((OrganizationContactComponent) value); 1115 } else if (name.equals("endpoint")) { 1116 this.getEndpoint().add(castToReference(value)); 1117 } else 1118 return super.setProperty(name, value); 1119 return value; 1120 } 1121 1122 @Override 1123 public Base makeProperty(int hash, String name) throws FHIRException { 1124 switch (hash) { 1125 case -1618432855: return addIdentifier(); 1126 case -1422950650: return getActiveElement(); 1127 case 3575610: return addType(); 1128 case 3373707: return getNameElement(); 1129 case 92902992: return addAliasElement(); 1130 case -1429363305: return addTelecom(); 1131 case -1147692044: return addAddress(); 1132 case -995410646: return getPartOf(); 1133 case 951526432: return addContact(); 1134 case 1741102485: return addEndpoint(); 1135 default: return super.makeProperty(hash, name); 1136 } 1137 1138 } 1139 1140 @Override 1141 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1142 switch (hash) { 1143 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1144 case -1422950650: /*active*/ return new String[] {"boolean"}; 1145 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1146 case 3373707: /*name*/ return new String[] {"string"}; 1147 case 92902992: /*alias*/ return new String[] {"string"}; 1148 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1149 case -1147692044: /*address*/ return new String[] {"Address"}; 1150 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1151 case 951526432: /*contact*/ return new String[] {}; 1152 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1153 default: return super.getTypesForProperty(hash, name); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base addChild(String name) throws FHIRException { 1160 if (name.equals("identifier")) { 1161 return addIdentifier(); 1162 } 1163 else if (name.equals("active")) { 1164 throw new FHIRException("Cannot call addChild on a singleton property Organization.active"); 1165 } 1166 else if (name.equals("type")) { 1167 return addType(); 1168 } 1169 else if (name.equals("name")) { 1170 throw new FHIRException("Cannot call addChild on a singleton property Organization.name"); 1171 } 1172 else if (name.equals("alias")) { 1173 throw new FHIRException("Cannot call addChild on a singleton property Organization.alias"); 1174 } 1175 else if (name.equals("telecom")) { 1176 return addTelecom(); 1177 } 1178 else if (name.equals("address")) { 1179 return addAddress(); 1180 } 1181 else if (name.equals("partOf")) { 1182 this.partOf = new Reference(); 1183 return this.partOf; 1184 } 1185 else if (name.equals("contact")) { 1186 return addContact(); 1187 } 1188 else if (name.equals("endpoint")) { 1189 return addEndpoint(); 1190 } 1191 else 1192 return super.addChild(name); 1193 } 1194 1195 public String fhirType() { 1196 return "Organization"; 1197 1198 } 1199 1200 public Organization copy() { 1201 Organization dst = new Organization(); 1202 copyValues(dst); 1203 if (identifier != null) { 1204 dst.identifier = new ArrayList<Identifier>(); 1205 for (Identifier i : identifier) 1206 dst.identifier.add(i.copy()); 1207 }; 1208 dst.active = active == null ? null : active.copy(); 1209 if (type != null) { 1210 dst.type = new ArrayList<CodeableConcept>(); 1211 for (CodeableConcept i : type) 1212 dst.type.add(i.copy()); 1213 }; 1214 dst.name = name == null ? null : name.copy(); 1215 if (alias != null) { 1216 dst.alias = new ArrayList<StringType>(); 1217 for (StringType i : alias) 1218 dst.alias.add(i.copy()); 1219 }; 1220 if (telecom != null) { 1221 dst.telecom = new ArrayList<ContactPoint>(); 1222 for (ContactPoint i : telecom) 1223 dst.telecom.add(i.copy()); 1224 }; 1225 if (address != null) { 1226 dst.address = new ArrayList<Address>(); 1227 for (Address i : address) 1228 dst.address.add(i.copy()); 1229 }; 1230 dst.partOf = partOf == null ? null : partOf.copy(); 1231 if (contact != null) { 1232 dst.contact = new ArrayList<OrganizationContactComponent>(); 1233 for (OrganizationContactComponent i : contact) 1234 dst.contact.add(i.copy()); 1235 }; 1236 if (endpoint != null) { 1237 dst.endpoint = new ArrayList<Reference>(); 1238 for (Reference i : endpoint) 1239 dst.endpoint.add(i.copy()); 1240 }; 1241 return dst; 1242 } 1243 1244 protected Organization typedCopy() { 1245 return copy(); 1246 } 1247 1248 @Override 1249 public boolean equalsDeep(Base other_) { 1250 if (!super.equalsDeep(other_)) 1251 return false; 1252 if (!(other_ instanceof Organization)) 1253 return false; 1254 Organization o = (Organization) other_; 1255 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(type, o.type, true) 1256 && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) && compareDeep(telecom, o.telecom, true) 1257 && compareDeep(address, o.address, true) && compareDeep(partOf, o.partOf, true) && compareDeep(contact, o.contact, true) 1258 && compareDeep(endpoint, o.endpoint, true); 1259 } 1260 1261 @Override 1262 public boolean equalsShallow(Base other_) { 1263 if (!super.equalsShallow(other_)) 1264 return false; 1265 if (!(other_ instanceof Organization)) 1266 return false; 1267 Organization o = (Organization) other_; 1268 return compareValues(active, o.active, true) && compareValues(name, o.name, true) && compareValues(alias, o.alias, true) 1269 ; 1270 } 1271 1272 public boolean isEmpty() { 1273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type 1274 , name, alias, telecom, address, partOf, contact, endpoint); 1275 } 1276 1277 @Override 1278 public ResourceType getResourceType() { 1279 return ResourceType.Organization; 1280 } 1281 1282 /** 1283 * Search parameter: <b>identifier</b> 1284 * <p> 1285 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 1286 * Type: <b>token</b><br> 1287 * Path: <b>Organization.identifier</b><br> 1288 * </p> 1289 */ 1290 @SearchParamDefinition(name="identifier", path="Organization.identifier", description="Any identifier for the organization (not the accreditation issuer's identifier)", type="token" ) 1291 public static final String SP_IDENTIFIER = "identifier"; 1292 /** 1293 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1294 * <p> 1295 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 1296 * Type: <b>token</b><br> 1297 * Path: <b>Organization.identifier</b><br> 1298 * </p> 1299 */ 1300 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1301 1302 /** 1303 * Search parameter: <b>partof</b> 1304 * <p> 1305 * Description: <b>An organization of which this organization forms a part</b><br> 1306 * Type: <b>reference</b><br> 1307 * Path: <b>Organization.partOf</b><br> 1308 * </p> 1309 */ 1310 @SearchParamDefinition(name="partof", path="Organization.partOf", description="An organization of which this organization forms a part", type="reference", target={Organization.class } ) 1311 public static final String SP_PARTOF = "partof"; 1312 /** 1313 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 1314 * <p> 1315 * Description: <b>An organization of which this organization forms a part</b><br> 1316 * Type: <b>reference</b><br> 1317 * Path: <b>Organization.partOf</b><br> 1318 * </p> 1319 */ 1320 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTOF); 1321 1322/** 1323 * Constant for fluent queries to be used to add include statements. Specifies 1324 * the path value of "<b>Organization:partof</b>". 1325 */ 1326 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include("Organization:partof").toLocked(); 1327 1328 /** 1329 * Search parameter: <b>address</b> 1330 * <p> 1331 * Description: <b>A (part of the) address of the organization</b><br> 1332 * Type: <b>string</b><br> 1333 * Path: <b>Organization.address</b><br> 1334 * </p> 1335 */ 1336 @SearchParamDefinition(name="address", path="Organization.address", description="A (part of the) address of the organization", type="string" ) 1337 public static final String SP_ADDRESS = "address"; 1338 /** 1339 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1340 * <p> 1341 * Description: <b>A (part of the) address of the organization</b><br> 1342 * Type: <b>string</b><br> 1343 * Path: <b>Organization.address</b><br> 1344 * </p> 1345 */ 1346 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1347 1348 /** 1349 * Search parameter: <b>address-state</b> 1350 * <p> 1351 * Description: <b>A state specified in an address</b><br> 1352 * Type: <b>string</b><br> 1353 * Path: <b>Organization.address.state</b><br> 1354 * </p> 1355 */ 1356 @SearchParamDefinition(name="address-state", path="Organization.address.state", description="A state specified in an address", type="string" ) 1357 public static final String SP_ADDRESS_STATE = "address-state"; 1358 /** 1359 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1360 * <p> 1361 * Description: <b>A state specified in an address</b><br> 1362 * Type: <b>string</b><br> 1363 * Path: <b>Organization.address.state</b><br> 1364 * </p> 1365 */ 1366 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1367 1368 /** 1369 * Search parameter: <b>active</b> 1370 * <p> 1371 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1372 * Type: <b>token</b><br> 1373 * Path: <b>Organization.active</b><br> 1374 * </p> 1375 */ 1376 @SearchParamDefinition(name="active", path="Organization.active", description="A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text", type="token" ) 1377 public static final String SP_ACTIVE = "active"; 1378 /** 1379 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1380 * <p> 1381 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1382 * Type: <b>token</b><br> 1383 * Path: <b>Organization.active</b><br> 1384 * </p> 1385 */ 1386 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1387 1388 /** 1389 * Search parameter: <b>type</b> 1390 * <p> 1391 * Description: <b>A code for the type of organization</b><br> 1392 * Type: <b>token</b><br> 1393 * Path: <b>Organization.type</b><br> 1394 * </p> 1395 */ 1396 @SearchParamDefinition(name="type", path="Organization.type", description="A code for the type of organization", type="token" ) 1397 public static final String SP_TYPE = "type"; 1398 /** 1399 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1400 * <p> 1401 * Description: <b>A code for the type of organization</b><br> 1402 * Type: <b>token</b><br> 1403 * Path: <b>Organization.type</b><br> 1404 * </p> 1405 */ 1406 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1407 1408 /** 1409 * Search parameter: <b>address-postalcode</b> 1410 * <p> 1411 * Description: <b>A postal code specified in an address</b><br> 1412 * Type: <b>string</b><br> 1413 * Path: <b>Organization.address.postalCode</b><br> 1414 * </p> 1415 */ 1416 @SearchParamDefinition(name="address-postalcode", path="Organization.address.postalCode", description="A postal code specified in an address", type="string" ) 1417 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1418 /** 1419 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1420 * <p> 1421 * Description: <b>A postal code specified in an address</b><br> 1422 * Type: <b>string</b><br> 1423 * Path: <b>Organization.address.postalCode</b><br> 1424 * </p> 1425 */ 1426 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1427 1428 /** 1429 * Search parameter: <b>address-country</b> 1430 * <p> 1431 * Description: <b>A country specified in an address</b><br> 1432 * Type: <b>string</b><br> 1433 * Path: <b>Organization.address.country</b><br> 1434 * </p> 1435 */ 1436 @SearchParamDefinition(name="address-country", path="Organization.address.country", description="A country specified in an address", type="string" ) 1437 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1438 /** 1439 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1440 * <p> 1441 * Description: <b>A country specified in an address</b><br> 1442 * Type: <b>string</b><br> 1443 * Path: <b>Organization.address.country</b><br> 1444 * </p> 1445 */ 1446 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1447 1448 /** 1449 * Search parameter: <b>endpoint</b> 1450 * <p> 1451 * Description: <b>Technical endpoints providing access to services operated for the organization</b><br> 1452 * Type: <b>reference</b><br> 1453 * Path: <b>Organization.endpoint</b><br> 1454 * </p> 1455 */ 1456 @SearchParamDefinition(name="endpoint", path="Organization.endpoint", description="Technical endpoints providing access to services operated for the organization", type="reference", target={Endpoint.class } ) 1457 public static final String SP_ENDPOINT = "endpoint"; 1458 /** 1459 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1460 * <p> 1461 * Description: <b>Technical endpoints providing access to services operated for the organization</b><br> 1462 * Type: <b>reference</b><br> 1463 * Path: <b>Organization.endpoint</b><br> 1464 * </p> 1465 */ 1466 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1467 1468/** 1469 * Constant for fluent queries to be used to add include statements. Specifies 1470 * the path value of "<b>Organization:endpoint</b>". 1471 */ 1472 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("Organization:endpoint").toLocked(); 1473 1474 /** 1475 * Search parameter: <b>phonetic</b> 1476 * <p> 1477 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 1478 * Type: <b>string</b><br> 1479 * Path: <b>Organization.name</b><br> 1480 * </p> 1481 */ 1482 @SearchParamDefinition(name="phonetic", path="Organization.name", description="A portion of the organization's name using some kind of phonetic matching algorithm", type="string" ) 1483 public static final String SP_PHONETIC = "phonetic"; 1484 /** 1485 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1486 * <p> 1487 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 1488 * Type: <b>string</b><br> 1489 * Path: <b>Organization.name</b><br> 1490 * </p> 1491 */ 1492 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 1493 1494 /** 1495 * Search parameter: <b>name</b> 1496 * <p> 1497 * Description: <b>A portion of the organization's name or alias</b><br> 1498 * Type: <b>string</b><br> 1499 * Path: <b>Organization.name, Organization.alias</b><br> 1500 * </p> 1501 */ 1502 @SearchParamDefinition(name="name", path="Organization.name | Organization.alias", description="A portion of the organization's name or alias", type="string" ) 1503 public static final String SP_NAME = "name"; 1504 /** 1505 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1506 * <p> 1507 * Description: <b>A portion of the organization's name or alias</b><br> 1508 * Type: <b>string</b><br> 1509 * Path: <b>Organization.name, Organization.alias</b><br> 1510 * </p> 1511 */ 1512 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1513 1514 /** 1515 * Search parameter: <b>address-use</b> 1516 * <p> 1517 * Description: <b>A use code specified in an address</b><br> 1518 * Type: <b>token</b><br> 1519 * Path: <b>Organization.address.use</b><br> 1520 * </p> 1521 */ 1522 @SearchParamDefinition(name="address-use", path="Organization.address.use", description="A use code specified in an address", type="token" ) 1523 public static final String SP_ADDRESS_USE = "address-use"; 1524 /** 1525 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1526 * <p> 1527 * Description: <b>A use code specified in an address</b><br> 1528 * Type: <b>token</b><br> 1529 * Path: <b>Organization.address.use</b><br> 1530 * </p> 1531 */ 1532 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1533 1534 /** 1535 * Search parameter: <b>address-city</b> 1536 * <p> 1537 * Description: <b>A city specified in an address</b><br> 1538 * Type: <b>string</b><br> 1539 * Path: <b>Organization.address.city</b><br> 1540 * </p> 1541 */ 1542 @SearchParamDefinition(name="address-city", path="Organization.address.city", description="A city specified in an address", type="string" ) 1543 public static final String SP_ADDRESS_CITY = "address-city"; 1544 /** 1545 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1546 * <p> 1547 * Description: <b>A city specified in an address</b><br> 1548 * Type: <b>string</b><br> 1549 * Path: <b>Organization.address.city</b><br> 1550 * </p> 1551 */ 1552 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1553 1554 1555}