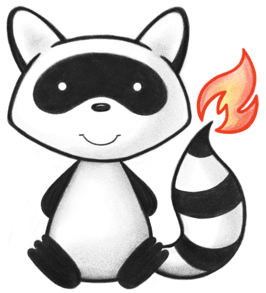
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 046 */ 047@DatatypeDef(name="ParameterDefinition") 048public class ParameterDefinition extends Type implements ICompositeType { 049 050 public enum ParameterUse { 051 /** 052 * This is an input parameter. 053 */ 054 IN, 055 /** 056 * This is an output parameter. 057 */ 058 OUT, 059 /** 060 * added to help the parsers with the generic types 061 */ 062 NULL; 063 public static ParameterUse fromCode(String codeString) throws FHIRException { 064 if (codeString == null || "".equals(codeString)) 065 return null; 066 if ("in".equals(codeString)) 067 return IN; 068 if ("out".equals(codeString)) 069 return OUT; 070 if (Configuration.isAcceptInvalidEnums()) 071 return null; 072 else 073 throw new FHIRException("Unknown ParameterUse code '"+codeString+"'"); 074 } 075 public String toCode() { 076 switch (this) { 077 case IN: return "in"; 078 case OUT: return "out"; 079 case NULL: return null; 080 default: return "?"; 081 } 082 } 083 public String getSystem() { 084 switch (this) { 085 case IN: return "http://hl7.org/fhir/operation-parameter-use"; 086 case OUT: return "http://hl7.org/fhir/operation-parameter-use"; 087 case NULL: return null; 088 default: return "?"; 089 } 090 } 091 public String getDefinition() { 092 switch (this) { 093 case IN: return "This is an input parameter."; 094 case OUT: return "This is an output parameter."; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getDisplay() { 100 switch (this) { 101 case IN: return "In"; 102 case OUT: return "Out"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 } 108 109 public static class ParameterUseEnumFactory implements EnumFactory<ParameterUse> { 110 public ParameterUse fromCode(String codeString) throws IllegalArgumentException { 111 if (codeString == null || "".equals(codeString)) 112 if (codeString == null || "".equals(codeString)) 113 return null; 114 if ("in".equals(codeString)) 115 return ParameterUse.IN; 116 if ("out".equals(codeString)) 117 return ParameterUse.OUT; 118 throw new IllegalArgumentException("Unknown ParameterUse code '"+codeString+"'"); 119 } 120 public Enumeration<ParameterUse> fromType(PrimitiveType<?> code) throws FHIRException { 121 if (code == null) 122 return null; 123 if (code.isEmpty()) 124 return new Enumeration<ParameterUse>(this); 125 String codeString = code.asStringValue(); 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("in".equals(codeString)) 129 return new Enumeration<ParameterUse>(this, ParameterUse.IN); 130 if ("out".equals(codeString)) 131 return new Enumeration<ParameterUse>(this, ParameterUse.OUT); 132 throw new FHIRException("Unknown ParameterUse code '"+codeString+"'"); 133 } 134 public String toCode(ParameterUse code) { 135 if (code == ParameterUse.NULL) 136 return null; 137 if (code == ParameterUse.IN) 138 return "in"; 139 if (code == ParameterUse.OUT) 140 return "out"; 141 return "?"; 142 } 143 public String toSystem(ParameterUse code) { 144 return code.getSystem(); 145 } 146 } 147 148 /** 149 * The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 150 */ 151 @Child(name = "name", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 152 @Description(shortDefinition="Name used to access the parameter value", formalDefinition="The name of the parameter used to allow access to the value of the parameter in evaluation contexts." ) 153 protected CodeType name; 154 155 /** 156 * Whether the parameter is input or output for the module. 157 */ 158 @Child(name = "use", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 159 @Description(shortDefinition="in | out", formalDefinition="Whether the parameter is input or output for the module." ) 160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/operation-parameter-use") 161 protected Enumeration<ParameterUse> use; 162 163 /** 164 * The minimum number of times this parameter SHALL appear in the request or response. 165 */ 166 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 167 @Description(shortDefinition="Minimum cardinality", formalDefinition="The minimum number of times this parameter SHALL appear in the request or response." ) 168 protected IntegerType min; 169 170 /** 171 * The maximum number of times this element is permitted to appear in the request or response. 172 */ 173 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 174 @Description(shortDefinition="Maximum cardinality (a number of *)", formalDefinition="The maximum number of times this element is permitted to appear in the request or response." ) 175 protected StringType max; 176 177 /** 178 * A brief discussion of what the parameter is for and how it is used by the module. 179 */ 180 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 181 @Description(shortDefinition="A brief description of the parameter", formalDefinition="A brief discussion of what the parameter is for and how it is used by the module." ) 182 protected StringType documentation; 183 184 /** 185 * The type of the parameter. 186 */ 187 @Child(name = "type", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 188 @Description(shortDefinition="What type of value", formalDefinition="The type of the parameter." ) 189 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-types") 190 protected CodeType type; 191 192 /** 193 * If specified, this indicates a profile that the input data must conform to, or that the output data will conform to. 194 */ 195 @Child(name = "profile", type = {StructureDefinition.class}, order=6, min=0, max=1, modifier=false, summary=true) 196 @Description(shortDefinition="What profile the value is expected to be", formalDefinition="If specified, this indicates a profile that the input data must conform to, or that the output data will conform to." ) 197 protected Reference profile; 198 199 /** 200 * The actual object that is the target of the reference (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.) 201 */ 202 protected StructureDefinition profileTarget; 203 204 private static final long serialVersionUID = 660888127L; 205 206 /** 207 * Constructor 208 */ 209 public ParameterDefinition() { 210 super(); 211 } 212 213 /** 214 * Constructor 215 */ 216 public ParameterDefinition(Enumeration<ParameterUse> use, CodeType type) { 217 super(); 218 this.use = use; 219 this.type = type; 220 } 221 222 /** 223 * @return {@link #name} (The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 224 */ 225 public CodeType getNameElement() { 226 if (this.name == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create ParameterDefinition.name"); 229 else if (Configuration.doAutoCreate()) 230 this.name = new CodeType(); // bb 231 return this.name; 232 } 233 234 public boolean hasNameElement() { 235 return this.name != null && !this.name.isEmpty(); 236 } 237 238 public boolean hasName() { 239 return this.name != null && !this.name.isEmpty(); 240 } 241 242 /** 243 * @param value {@link #name} (The name of the parameter used to allow access to the value of the parameter in evaluation contexts.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 244 */ 245 public ParameterDefinition setNameElement(CodeType value) { 246 this.name = value; 247 return this; 248 } 249 250 /** 251 * @return The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 252 */ 253 public String getName() { 254 return this.name == null ? null : this.name.getValue(); 255 } 256 257 /** 258 * @param value The name of the parameter used to allow access to the value of the parameter in evaluation contexts. 259 */ 260 public ParameterDefinition setName(String value) { 261 if (Utilities.noString(value)) 262 this.name = null; 263 else { 264 if (this.name == null) 265 this.name = new CodeType(); 266 this.name.setValue(value); 267 } 268 return this; 269 } 270 271 /** 272 * @return {@link #use} (Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 273 */ 274 public Enumeration<ParameterUse> getUseElement() { 275 if (this.use == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create ParameterDefinition.use"); 278 else if (Configuration.doAutoCreate()) 279 this.use = new Enumeration<ParameterUse>(new ParameterUseEnumFactory()); // bb 280 return this.use; 281 } 282 283 public boolean hasUseElement() { 284 return this.use != null && !this.use.isEmpty(); 285 } 286 287 public boolean hasUse() { 288 return this.use != null && !this.use.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #use} (Whether the parameter is input or output for the module.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 293 */ 294 public ParameterDefinition setUseElement(Enumeration<ParameterUse> value) { 295 this.use = value; 296 return this; 297 } 298 299 /** 300 * @return Whether the parameter is input or output for the module. 301 */ 302 public ParameterUse getUse() { 303 return this.use == null ? null : this.use.getValue(); 304 } 305 306 /** 307 * @param value Whether the parameter is input or output for the module. 308 */ 309 public ParameterDefinition setUse(ParameterUse value) { 310 if (this.use == null) 311 this.use = new Enumeration<ParameterUse>(new ParameterUseEnumFactory()); 312 this.use.setValue(value); 313 return this; 314 } 315 316 /** 317 * @return {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 318 */ 319 public IntegerType getMinElement() { 320 if (this.min == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create ParameterDefinition.min"); 323 else if (Configuration.doAutoCreate()) 324 this.min = new IntegerType(); // bb 325 return this.min; 326 } 327 328 public boolean hasMinElement() { 329 return this.min != null && !this.min.isEmpty(); 330 } 331 332 public boolean hasMin() { 333 return this.min != null && !this.min.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #min} (The minimum number of times this parameter SHALL appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 338 */ 339 public ParameterDefinition setMinElement(IntegerType value) { 340 this.min = value; 341 return this; 342 } 343 344 /** 345 * @return The minimum number of times this parameter SHALL appear in the request or response. 346 */ 347 public int getMin() { 348 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 349 } 350 351 /** 352 * @param value The minimum number of times this parameter SHALL appear in the request or response. 353 */ 354 public ParameterDefinition setMin(int value) { 355 if (this.min == null) 356 this.min = new IntegerType(); 357 this.min.setValue(value); 358 return this; 359 } 360 361 /** 362 * @return {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 363 */ 364 public StringType getMaxElement() { 365 if (this.max == null) 366 if (Configuration.errorOnAutoCreate()) 367 throw new Error("Attempt to auto-create ParameterDefinition.max"); 368 else if (Configuration.doAutoCreate()) 369 this.max = new StringType(); // bb 370 return this.max; 371 } 372 373 public boolean hasMaxElement() { 374 return this.max != null && !this.max.isEmpty(); 375 } 376 377 public boolean hasMax() { 378 return this.max != null && !this.max.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the request or response.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 383 */ 384 public ParameterDefinition setMaxElement(StringType value) { 385 this.max = value; 386 return this; 387 } 388 389 /** 390 * @return The maximum number of times this element is permitted to appear in the request or response. 391 */ 392 public String getMax() { 393 return this.max == null ? null : this.max.getValue(); 394 } 395 396 /** 397 * @param value The maximum number of times this element is permitted to appear in the request or response. 398 */ 399 public ParameterDefinition setMax(String value) { 400 if (Utilities.noString(value)) 401 this.max = null; 402 else { 403 if (this.max == null) 404 this.max = new StringType(); 405 this.max.setValue(value); 406 } 407 return this; 408 } 409 410 /** 411 * @return {@link #documentation} (A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 412 */ 413 public StringType getDocumentationElement() { 414 if (this.documentation == null) 415 if (Configuration.errorOnAutoCreate()) 416 throw new Error("Attempt to auto-create ParameterDefinition.documentation"); 417 else if (Configuration.doAutoCreate()) 418 this.documentation = new StringType(); // bb 419 return this.documentation; 420 } 421 422 public boolean hasDocumentationElement() { 423 return this.documentation != null && !this.documentation.isEmpty(); 424 } 425 426 public boolean hasDocumentation() { 427 return this.documentation != null && !this.documentation.isEmpty(); 428 } 429 430 /** 431 * @param value {@link #documentation} (A brief discussion of what the parameter is for and how it is used by the module.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 432 */ 433 public ParameterDefinition setDocumentationElement(StringType value) { 434 this.documentation = value; 435 return this; 436 } 437 438 /** 439 * @return A brief discussion of what the parameter is for and how it is used by the module. 440 */ 441 public String getDocumentation() { 442 return this.documentation == null ? null : this.documentation.getValue(); 443 } 444 445 /** 446 * @param value A brief discussion of what the parameter is for and how it is used by the module. 447 */ 448 public ParameterDefinition setDocumentation(String value) { 449 if (Utilities.noString(value)) 450 this.documentation = null; 451 else { 452 if (this.documentation == null) 453 this.documentation = new StringType(); 454 this.documentation.setValue(value); 455 } 456 return this; 457 } 458 459 /** 460 * @return {@link #type} (The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 461 */ 462 public CodeType getTypeElement() { 463 if (this.type == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create ParameterDefinition.type"); 466 else if (Configuration.doAutoCreate()) 467 this.type = new CodeType(); // bb 468 return this.type; 469 } 470 471 public boolean hasTypeElement() { 472 return this.type != null && !this.type.isEmpty(); 473 } 474 475 public boolean hasType() { 476 return this.type != null && !this.type.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #type} (The type of the parameter.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 481 */ 482 public ParameterDefinition setTypeElement(CodeType value) { 483 this.type = value; 484 return this; 485 } 486 487 /** 488 * @return The type of the parameter. 489 */ 490 public String getType() { 491 return this.type == null ? null : this.type.getValue(); 492 } 493 494 /** 495 * @param value The type of the parameter. 496 */ 497 public ParameterDefinition setType(String value) { 498 if (this.type == null) 499 this.type = new CodeType(); 500 this.type.setValue(value); 501 return this; 502 } 503 504 /** 505 * @return {@link #profile} (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.) 506 */ 507 public Reference getProfile() { 508 if (this.profile == null) 509 if (Configuration.errorOnAutoCreate()) 510 throw new Error("Attempt to auto-create ParameterDefinition.profile"); 511 else if (Configuration.doAutoCreate()) 512 this.profile = new Reference(); // cc 513 return this.profile; 514 } 515 516 public boolean hasProfile() { 517 return this.profile != null && !this.profile.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #profile} (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.) 522 */ 523 public ParameterDefinition setProfile(Reference value) { 524 this.profile = value; 525 return this; 526 } 527 528 /** 529 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.) 530 */ 531 public StructureDefinition getProfileTarget() { 532 if (this.profileTarget == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create ParameterDefinition.profile"); 535 else if (Configuration.doAutoCreate()) 536 this.profileTarget = new StructureDefinition(); // aa 537 return this.profileTarget; 538 } 539 540 /** 541 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.) 542 */ 543 public ParameterDefinition setProfileTarget(StructureDefinition value) { 544 this.profileTarget = value; 545 return this; 546 } 547 548 protected void listChildren(List<Property> children) { 549 super.listChildren(children); 550 children.add(new Property("name", "code", "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, name)); 551 children.add(new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, use)); 552 children.add(new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 553 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 554 children.add(new Property("documentation", "string", "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation)); 555 children.add(new Property("type", "code", "The type of the parameter.", 0, 1, type)); 556 children.add(new Property("profile", "Reference(StructureDefinition)", "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 0, 1, profile)); 557 } 558 559 @Override 560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 561 switch (_hash) { 562 case 3373707: /*name*/ return new Property("name", "code", "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, name); 563 case 116103: /*use*/ return new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, use); 564 case 108114: /*min*/ return new Property("min", "integer", "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 565 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 566 case 1587405498: /*documentation*/ return new Property("documentation", "string", "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation); 567 case 3575610: /*type*/ return new Property("type", "code", "The type of the parameter.", 0, 1, type); 568 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 0, 1, profile); 569 default: return super.getNamedProperty(_hash, _name, _checkValid); 570 } 571 572 } 573 574 @Override 575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 576 switch (hash) { 577 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 578 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<ParameterUse> 579 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 580 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 581 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 582 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 583 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 584 default: return super.getProperty(hash, name, checkValid); 585 } 586 587 } 588 589 @Override 590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 591 switch (hash) { 592 case 3373707: // name 593 this.name = castToCode(value); // CodeType 594 return value; 595 case 116103: // use 596 value = new ParameterUseEnumFactory().fromType(castToCode(value)); 597 this.use = (Enumeration) value; // Enumeration<ParameterUse> 598 return value; 599 case 108114: // min 600 this.min = castToInteger(value); // IntegerType 601 return value; 602 case 107876: // max 603 this.max = castToString(value); // StringType 604 return value; 605 case 1587405498: // documentation 606 this.documentation = castToString(value); // StringType 607 return value; 608 case 3575610: // type 609 this.type = castToCode(value); // CodeType 610 return value; 611 case -309425751: // profile 612 this.profile = castToReference(value); // Reference 613 return value; 614 default: return super.setProperty(hash, name, value); 615 } 616 617 } 618 619 @Override 620 public Base setProperty(String name, Base value) throws FHIRException { 621 if (name.equals("name")) { 622 this.name = castToCode(value); // CodeType 623 } else if (name.equals("use")) { 624 value = new ParameterUseEnumFactory().fromType(castToCode(value)); 625 this.use = (Enumeration) value; // Enumeration<ParameterUse> 626 } else if (name.equals("min")) { 627 this.min = castToInteger(value); // IntegerType 628 } else if (name.equals("max")) { 629 this.max = castToString(value); // StringType 630 } else if (name.equals("documentation")) { 631 this.documentation = castToString(value); // StringType 632 } else if (name.equals("type")) { 633 this.type = castToCode(value); // CodeType 634 } else if (name.equals("profile")) { 635 this.profile = castToReference(value); // Reference 636 } else 637 return super.setProperty(name, value); 638 return value; 639 } 640 641 @Override 642 public Base makeProperty(int hash, String name) throws FHIRException { 643 switch (hash) { 644 case 3373707: return getNameElement(); 645 case 116103: return getUseElement(); 646 case 108114: return getMinElement(); 647 case 107876: return getMaxElement(); 648 case 1587405498: return getDocumentationElement(); 649 case 3575610: return getTypeElement(); 650 case -309425751: return getProfile(); 651 default: return super.makeProperty(hash, name); 652 } 653 654 } 655 656 @Override 657 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 658 switch (hash) { 659 case 3373707: /*name*/ return new String[] {"code"}; 660 case 116103: /*use*/ return new String[] {"code"}; 661 case 108114: /*min*/ return new String[] {"integer"}; 662 case 107876: /*max*/ return new String[] {"string"}; 663 case 1587405498: /*documentation*/ return new String[] {"string"}; 664 case 3575610: /*type*/ return new String[] {"code"}; 665 case -309425751: /*profile*/ return new String[] {"Reference"}; 666 default: return super.getTypesForProperty(hash, name); 667 } 668 669 } 670 671 @Override 672 public Base addChild(String name) throws FHIRException { 673 if (name.equals("name")) { 674 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.name"); 675 } 676 else if (name.equals("use")) { 677 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.use"); 678 } 679 else if (name.equals("min")) { 680 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.min"); 681 } 682 else if (name.equals("max")) { 683 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.max"); 684 } 685 else if (name.equals("documentation")) { 686 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.documentation"); 687 } 688 else if (name.equals("type")) { 689 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.type"); 690 } 691 else if (name.equals("profile")) { 692 this.profile = new Reference(); 693 return this.profile; 694 } 695 else 696 return super.addChild(name); 697 } 698 699 public String fhirType() { 700 return "ParameterDefinition"; 701 702 } 703 704 public ParameterDefinition copy() { 705 ParameterDefinition dst = new ParameterDefinition(); 706 copyValues(dst); 707 dst.name = name == null ? null : name.copy(); 708 dst.use = use == null ? null : use.copy(); 709 dst.min = min == null ? null : min.copy(); 710 dst.max = max == null ? null : max.copy(); 711 dst.documentation = documentation == null ? null : documentation.copy(); 712 dst.type = type == null ? null : type.copy(); 713 dst.profile = profile == null ? null : profile.copy(); 714 return dst; 715 } 716 717 protected ParameterDefinition typedCopy() { 718 return copy(); 719 } 720 721 @Override 722 public boolean equalsDeep(Base other_) { 723 if (!super.equalsDeep(other_)) 724 return false; 725 if (!(other_ instanceof ParameterDefinition)) 726 return false; 727 ParameterDefinition o = (ParameterDefinition) other_; 728 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 729 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) && compareDeep(type, o.type, true) 730 && compareDeep(profile, o.profile, true); 731 } 732 733 @Override 734 public boolean equalsShallow(Base other_) { 735 if (!super.equalsShallow(other_)) 736 return false; 737 if (!(other_ instanceof ParameterDefinition)) 738 return false; 739 ParameterDefinition o = (ParameterDefinition) other_; 740 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 741 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) && compareValues(type, o.type, true) 742 ; 743 } 744 745 public boolean isEmpty() { 746 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation 747 , type, profile); 748 } 749 750 751}