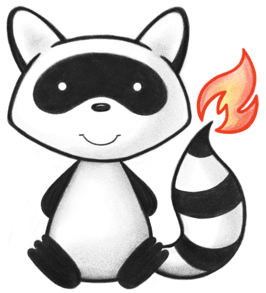
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.instance.model.api.IBaseParameters; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046/** 047 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 048 */ 049@ResourceDef(name="Parameters", profile="http://hl7.org/fhir/Profile/Parameters") 050public class Parameters extends Resource implements IBaseParameters { 051 052 @Block() 053 public static class ParametersParameterComponent extends BackboneElement implements IBaseBackboneElement { 054 /** 055 * The name of the parameter (reference to the operation definition). 056 */ 057 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Name from the definition", formalDefinition="The name of the parameter (reference to the operation definition)." ) 059 protected StringType name; 060 061 /** 062 * If the parameter is a data type. 063 */ 064 @Child(name = "value", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="If parameter is a data type", formalDefinition="If the parameter is a data type." ) 066 protected org.hl7.fhir.dstu3.model.Type value; 067 068 /** 069 * If the parameter is a whole resource. 070 */ 071 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=true) 072 @Description(shortDefinition="If parameter is a whole resource", formalDefinition="If the parameter is a whole resource." ) 073 protected Resource resource; 074 075 /** 076 * A named part of a multi-part parameter. 077 */ 078 @Child(name = "part", type = {ParametersParameterComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 079 @Description(shortDefinition="Named part of a multi-part parameter", formalDefinition="A named part of a multi-part parameter." ) 080 protected List<ParametersParameterComponent> part; 081 082 private static final long serialVersionUID = -839605058L; 083 084 /** 085 * Constructor 086 */ 087 public ParametersParameterComponent() { 088 super(); 089 } 090 091 /** 092 * Constructor 093 */ 094 public ParametersParameterComponent(StringType name) { 095 super(); 096 this.name = name; 097 } 098 099 /** 100 * @return {@link #name} (The name of the parameter (reference to the operation definition).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 101 */ 102 public StringType getNameElement() { 103 if (this.name == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create ParametersParameterComponent.name"); 106 else if (Configuration.doAutoCreate()) 107 this.name = new StringType(); // bb 108 return this.name; 109 } 110 111 public boolean hasNameElement() { 112 return this.name != null && !this.name.isEmpty(); 113 } 114 115 public boolean hasName() { 116 return this.name != null && !this.name.isEmpty(); 117 } 118 119 /** 120 * @param value {@link #name} (The name of the parameter (reference to the operation definition).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 121 */ 122 public ParametersParameterComponent setNameElement(StringType value) { 123 this.name = value; 124 return this; 125 } 126 127 /** 128 * @return The name of the parameter (reference to the operation definition). 129 */ 130 public String getName() { 131 return this.name == null ? null : this.name.getValue(); 132 } 133 134 /** 135 * @param value The name of the parameter (reference to the operation definition). 136 */ 137 public ParametersParameterComponent setName(String value) { 138 if (this.name == null) 139 this.name = new StringType(); 140 this.name.setValue(value); 141 return this; 142 } 143 144 /** 145 * @return {@link #value} (If the parameter is a data type.) 146 */ 147 public org.hl7.fhir.dstu3.model.Type getValue() { 148 return this.value; 149 } 150 151 public boolean hasValue() { 152 return this.value != null && !this.value.isEmpty(); 153 } 154 155 /** 156 * @param value {@link #value} (If the parameter is a data type.) 157 */ 158 public ParametersParameterComponent setValue(org.hl7.fhir.dstu3.model.Type value) { 159 this.value = value; 160 return this; 161 } 162 163 /** 164 * @return {@link #resource} (If the parameter is a whole resource.) 165 */ 166 public Resource getResource() { 167 return this.resource; 168 } 169 170 public boolean hasResource() { 171 return this.resource != null && !this.resource.isEmpty(); 172 } 173 174 /** 175 * @param value {@link #resource} (If the parameter is a whole resource.) 176 */ 177 public ParametersParameterComponent setResource(Resource value) { 178 this.resource = value; 179 return this; 180 } 181 182 /** 183 * @return {@link #part} (A named part of a multi-part parameter.) 184 */ 185 public List<ParametersParameterComponent> getPart() { 186 if (this.part == null) 187 this.part = new ArrayList<ParametersParameterComponent>(); 188 return this.part; 189 } 190 191 /** 192 * @return Returns a reference to <code>this</code> for easy method chaining 193 */ 194 public ParametersParameterComponent setPart(List<ParametersParameterComponent> thePart) { 195 this.part = thePart; 196 return this; 197 } 198 199 public boolean hasPart() { 200 if (this.part == null) 201 return false; 202 for (ParametersParameterComponent item : this.part) 203 if (!item.isEmpty()) 204 return true; 205 return false; 206 } 207 208 public ParametersParameterComponent addPart() { //3 209 ParametersParameterComponent t = new ParametersParameterComponent(); 210 if (this.part == null) 211 this.part = new ArrayList<ParametersParameterComponent>(); 212 this.part.add(t); 213 return t; 214 } 215 216 public ParametersParameterComponent addPart(ParametersParameterComponent t) { //3 217 if (t == null) 218 return this; 219 if (this.part == null) 220 this.part = new ArrayList<ParametersParameterComponent>(); 221 this.part.add(t); 222 return this; 223 } 224 225 /** 226 * @return The first repetition of repeating field {@link #part}, creating it if it does not already exist 227 */ 228 public ParametersParameterComponent getPartFirstRep() { 229 if (getPart().isEmpty()) { 230 addPart(); 231 } 232 return getPart().get(0); 233 } 234 235 protected void listChildren(List<Property> children) { 236 super.listChildren(children); 237 children.add(new Property("name", "string", "The name of the parameter (reference to the operation definition).", 0, 1, name)); 238 children.add(new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value)); 239 children.add(new Property("resource", "Resource", "If the parameter is a whole resource.", 0, 1, resource)); 240 children.add(new Property("part", "@Parameters.parameter", "A named part of a multi-part parameter.", 0, java.lang.Integer.MAX_VALUE, part)); 241 } 242 243 @Override 244 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 245 switch (_hash) { 246 case 3373707: /*name*/ return new Property("name", "string", "The name of the parameter (reference to the operation definition).", 0, 1, name); 247 case -1410166417: /*value[x]*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 248 case 111972721: /*value*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 249 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 250 case 733421943: /*valueBoolean*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 251 case -786218365: /*valueCanonical*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 252 case -766209282: /*valueCode*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 253 case -766192449: /*valueDate*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 254 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 255 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 256 case 231604844: /*valueId*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 257 case -1668687056: /*valueInstant*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 258 case -1668204915: /*valueInteger*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 259 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 260 case -1410178407: /*valueOid*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 261 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 262 case -1424603934: /*valueString*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 263 case -765708322: /*valueTime*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 264 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 265 case -1410172357: /*valueUri*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 266 case -1410172354: /*valueUrl*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 267 case -765667124: /*valueUuid*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 268 case -478981821: /*valueAddress*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 269 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 270 case -475566732: /*valueAttachment*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 271 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 272 case -1887705029: /*valueCoding*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 273 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 274 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 275 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 276 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 277 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 278 case 2030761548: /*valueRange*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 279 case 2030767386: /*valueRatio*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 280 case 1755241690: /*valueReference*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 281 case -962229101: /*valueSampledData*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 282 case -540985785: /*valueSignature*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 283 case -1406282469: /*valueTiming*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 284 case -1858636920: /*valueDosage*/ return new Property("value[x]", "*", "If the parameter is a data type.", 0, 1, value); 285 case -341064690: /*resource*/ return new Property("resource", "Resource", "If the parameter is a whole resource.", 0, 1, resource); 286 case 3433459: /*part*/ return new Property("part", "@Parameters.parameter", "A named part of a multi-part parameter.", 0, java.lang.Integer.MAX_VALUE, part); 287 default: return super.getNamedProperty(_hash, _name, _checkValid); 288 } 289 290 } 291 292 @Override 293 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 294 switch (hash) { 295 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 296 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // org.hl7.fhir.dstu3.model.Type 297 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 298 case 3433459: /*part*/ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // ParametersParameterComponent 299 default: return super.getProperty(hash, name, checkValid); 300 } 301 302 } 303 304 @Override 305 public Base setProperty(int hash, String name, Base value) throws FHIRException { 306 switch (hash) { 307 case 3373707: // name 308 this.name = castToString(value); // StringType 309 return value; 310 case 111972721: // value 311 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 312 return value; 313 case -341064690: // resource 314 this.resource = castToResource(value); // Resource 315 return value; 316 case 3433459: // part 317 this.getPart().add((ParametersParameterComponent) value); // ParametersParameterComponent 318 return value; 319 default: return super.setProperty(hash, name, value); 320 } 321 322 } 323 324 @Override 325 public Base setProperty(String name, Base value) throws FHIRException { 326 if (name.equals("name")) { 327 this.name = castToString(value); // StringType 328 } else if (name.equals("value[x]")) { 329 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 330 } else if (name.equals("resource")) { 331 this.resource = castToResource(value); // Resource 332 } else if (name.equals("part")) { 333 this.getPart().add((ParametersParameterComponent) value); 334 } else 335 return super.setProperty(name, value); 336 return value; 337 } 338 339 @Override 340 public Base makeProperty(int hash, String name) throws FHIRException { 341 switch (hash) { 342 case 3373707: return getNameElement(); 343 case -1410166417: return getValue(); 344 case 111972721: return getValue(); 345 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 346 case 3433459: return addPart(); 347 default: return super.makeProperty(hash, name); 348 } 349 350 } 351 352 @Override 353 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 354 switch (hash) { 355 case 3373707: /*name*/ return new String[] {"string"}; 356 case 111972721: /*value*/ return new String[] {"*"}; 357 case -341064690: /*resource*/ return new String[] {"Resource"}; 358 case 3433459: /*part*/ return new String[] {"@Parameters.parameter"}; 359 default: return super.getTypesForProperty(hash, name); 360 } 361 362 } 363 364 @Override 365 public Base addChild(String name) throws FHIRException { 366 if (name.equals("name")) { 367 throw new FHIRException("Cannot call addChild on a singleton property Parameters.name"); 368 } 369 else if (name.equals("valueBoolean")) { 370 this.value = new BooleanType(); 371 return this.value; 372 } 373 else if (name.equals("valueInteger")) { 374 this.value = new IntegerType(); 375 return this.value; 376 } 377 else if (name.equals("valueDecimal")) { 378 this.value = new DecimalType(); 379 return this.value; 380 } 381 else if (name.equals("valueBase64Binary")) { 382 this.value = new Base64BinaryType(); 383 return this.value; 384 } 385 else if (name.equals("valueInstant")) { 386 this.value = new InstantType(); 387 return this.value; 388 } 389 else if (name.equals("valueString")) { 390 this.value = new StringType(); 391 return this.value; 392 } 393 else if (name.equals("valueUri")) { 394 this.value = new UriType(); 395 return this.value; 396 } 397 else if (name.equals("valueDate")) { 398 this.value = new DateType(); 399 return this.value; 400 } 401 else if (name.equals("valueDateTime")) { 402 this.value = new DateTimeType(); 403 return this.value; 404 } 405 else if (name.equals("valueTime")) { 406 this.value = new TimeType(); 407 return this.value; 408 } 409 else if (name.equals("valueCode")) { 410 this.value = new CodeType(); 411 return this.value; 412 } 413 else if (name.equals("valueOid")) { 414 this.value = new OidType(); 415 return this.value; 416 } 417 else if (name.equals("valueId")) { 418 this.value = new IdType(); 419 return this.value; 420 } 421 else if (name.equals("valueUnsignedInt")) { 422 this.value = new UnsignedIntType(); 423 return this.value; 424 } 425 else if (name.equals("valuePositiveInt")) { 426 this.value = new PositiveIntType(); 427 return this.value; 428 } 429 else if (name.equals("valueMarkdown")) { 430 this.value = new MarkdownType(); 431 return this.value; 432 } 433 else if (name.equals("valueAnnotation")) { 434 this.value = new Annotation(); 435 return this.value; 436 } 437 else if (name.equals("valueAttachment")) { 438 this.value = new Attachment(); 439 return this.value; 440 } 441 else if (name.equals("valueIdentifier")) { 442 this.value = new Identifier(); 443 return this.value; 444 } 445 else if (name.equals("valueCodeableConcept")) { 446 this.value = new CodeableConcept(); 447 return this.value; 448 } 449 else if (name.equals("valueCoding")) { 450 this.value = new Coding(); 451 return this.value; 452 } 453 else if (name.equals("valueQuantity")) { 454 this.value = new Quantity(); 455 return this.value; 456 } 457 else if (name.equals("valueRange")) { 458 this.value = new Range(); 459 return this.value; 460 } 461 else if (name.equals("valuePeriod")) { 462 this.value = new Period(); 463 return this.value; 464 } 465 else if (name.equals("valueRatio")) { 466 this.value = new Ratio(); 467 return this.value; 468 } 469 else if (name.equals("valueSampledData")) { 470 this.value = new SampledData(); 471 return this.value; 472 } 473 else if (name.equals("valueSignature")) { 474 this.value = new Signature(); 475 return this.value; 476 } 477 else if (name.equals("valueHumanName")) { 478 this.value = new HumanName(); 479 return this.value; 480 } 481 else if (name.equals("valueAddress")) { 482 this.value = new Address(); 483 return this.value; 484 } 485 else if (name.equals("valueContactPoint")) { 486 this.value = new ContactPoint(); 487 return this.value; 488 } 489 else if (name.equals("valueTiming")) { 490 this.value = new Timing(); 491 return this.value; 492 } 493 else if (name.equals("valueReference")) { 494 this.value = new Reference(); 495 return this.value; 496 } 497 else if (name.equals("valueMeta")) { 498 this.value = new Meta(); 499 return this.value; 500 } 501 else if (name.equals("resource")) { 502 throw new FHIRException("Cannot call addChild on an abstract type Parameters.resource"); 503 } 504 else if (name.equals("part")) { 505 return addPart(); 506 } 507 else 508 return super.addChild(name); 509 } 510 511 public ParametersParameterComponent copy() { 512 ParametersParameterComponent dst = new ParametersParameterComponent(); 513 copyValues(dst); 514 dst.name = name == null ? null : name.copy(); 515 dst.value = value == null ? null : value.copy(); 516 dst.resource = resource == null ? null : resource.copy(); 517 if (part != null) { 518 dst.part = new ArrayList<ParametersParameterComponent>(); 519 for (ParametersParameterComponent i : part) 520 dst.part.add(i.copy()); 521 }; 522 return dst; 523 } 524 525 @Override 526 public boolean equalsDeep(Base other_) { 527 if (!super.equalsDeep(other_)) 528 return false; 529 if (!(other_ instanceof ParametersParameterComponent)) 530 return false; 531 ParametersParameterComponent o = (ParametersParameterComponent) other_; 532 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true) && compareDeep(resource, o.resource, true) 533 && compareDeep(part, o.part, true); 534 } 535 536 @Override 537 public boolean equalsShallow(Base other_) { 538 if (!super.equalsShallow(other_)) 539 return false; 540 if (!(other_ instanceof ParametersParameterComponent)) 541 return false; 542 ParametersParameterComponent o = (ParametersParameterComponent) other_; 543 return compareValues(name, o.name, true); 544 } 545 546 public boolean isEmpty() { 547 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value, resource, part 548 ); 549 } 550 551 public String fhirType() { 552 return "Parameters.parameter"; 553 554 } 555 556 } 557 558 /** 559 * A parameter passed to or received from the operation. 560 */ 561 @Child(name = "parameter", type = {}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 562 @Description(shortDefinition="Operation Parameter", formalDefinition="A parameter passed to or received from the operation." ) 563 protected List<ParametersParameterComponent> parameter; 564 565 private static final long serialVersionUID = -1495940293L; 566 567 /** 568 * Constructor 569 */ 570 public Parameters() { 571 super(); 572 } 573 574 /** 575 * @return {@link #parameter} (A parameter passed to or received from the operation.) 576 */ 577 public List<ParametersParameterComponent> getParameter() { 578 if (this.parameter == null) 579 this.parameter = new ArrayList<ParametersParameterComponent>(); 580 return this.parameter; 581 } 582 583 /** 584 * @return Returns a reference to <code>this</code> for easy method chaining 585 */ 586 public Parameters setParameter(List<ParametersParameterComponent> theParameter) { 587 this.parameter = theParameter; 588 return this; 589 } 590 591 public boolean hasParameter() { 592 if (this.parameter == null) 593 return false; 594 for (ParametersParameterComponent item : this.parameter) 595 if (!item.isEmpty()) 596 return true; 597 return false; 598 } 599 600 public ParametersParameterComponent addParameter() { //3 601 ParametersParameterComponent t = new ParametersParameterComponent(); 602 if (this.parameter == null) 603 this.parameter = new ArrayList<ParametersParameterComponent>(); 604 this.parameter.add(t); 605 return t; 606 } 607 608 public Parameters addParameter(ParametersParameterComponent t) { //3 609 if (t == null) 610 return this; 611 if (this.parameter == null) 612 this.parameter = new ArrayList<ParametersParameterComponent>(); 613 this.parameter.add(t); 614 return this; 615 } 616 617 /** 618 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist 619 */ 620 public ParametersParameterComponent getParameterFirstRep() { 621 if (getParameter().isEmpty()) { 622 addParameter(); 623 } 624 return getParameter().get(0); 625 } 626 627 protected void listChildren(List<Property> children) { 628 super.listChildren(children); 629 children.add(new Property("parameter", "", "A parameter passed to or received from the operation.", 0, java.lang.Integer.MAX_VALUE, parameter)); 630 } 631 632 @Override 633 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 634 switch (_hash) { 635 case 1954460585: /*parameter*/ return new Property("parameter", "", "A parameter passed to or received from the operation.", 0, java.lang.Integer.MAX_VALUE, parameter); 636 default: return super.getNamedProperty(_hash, _name, _checkValid); 637 } 638 639 } 640 641 @Override 642 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 643 switch (hash) { 644 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ParametersParameterComponent 645 default: return super.getProperty(hash, name, checkValid); 646 } 647 648 } 649 650 @Override 651 public Base setProperty(int hash, String name, Base value) throws FHIRException { 652 switch (hash) { 653 case 1954460585: // parameter 654 this.getParameter().add((ParametersParameterComponent) value); // ParametersParameterComponent 655 return value; 656 default: return super.setProperty(hash, name, value); 657 } 658 659 } 660 661 @Override 662 public Base setProperty(String name, Base value) throws FHIRException { 663 if (name.equals("parameter")) { 664 this.getParameter().add((ParametersParameterComponent) value); 665 } else 666 return super.setProperty(name, value); 667 return value; 668 } 669 670 @Override 671 public Base makeProperty(int hash, String name) throws FHIRException { 672 switch (hash) { 673 case 1954460585: return addParameter(); 674 default: return super.makeProperty(hash, name); 675 } 676 677 } 678 679 @Override 680 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 681 switch (hash) { 682 case 1954460585: /*parameter*/ return new String[] {}; 683 default: return super.getTypesForProperty(hash, name); 684 } 685 686 } 687 688 @Override 689 public Base addChild(String name) throws FHIRException { 690 if (name.equals("parameter")) { 691 return addParameter(); 692 } 693 else 694 return super.addChild(name); 695 } 696 697 public String fhirType() { 698 return "Parameters"; 699 700 } 701 702 public Parameters copy() { 703 Parameters dst = new Parameters(); 704 copyValues(dst); 705 if (parameter != null) { 706 dst.parameter = new ArrayList<ParametersParameterComponent>(); 707 for (ParametersParameterComponent i : parameter) 708 dst.parameter.add(i.copy()); 709 }; 710 return dst; 711 } 712 713 protected Parameters typedCopy() { 714 return copy(); 715 } 716 717 @Override 718 public boolean equalsDeep(Base other_) { 719 if (!super.equalsDeep(other_)) 720 return false; 721 if (!(other_ instanceof Parameters)) 722 return false; 723 Parameters o = (Parameters) other_; 724 return compareDeep(parameter, o.parameter, true); 725 } 726 727 @Override 728 public boolean equalsShallow(Base other_) { 729 if (!super.equalsShallow(other_)) 730 return false; 731 if (!(other_ instanceof Parameters)) 732 return false; 733 Parameters o = (Parameters) other_; 734 return true; 735 } 736 737 public boolean isEmpty() { 738 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameter); 739 } 740 741 @Override 742 public ResourceType getResourceType() { 743 return ResourceType.Parameters; 744 } 745 746 747}