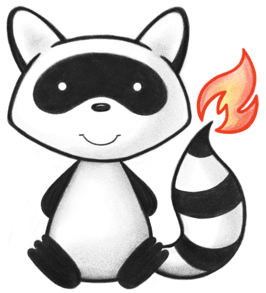
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 052 */ 053@ResourceDef(name="Patient", profile="http://hl7.org/fhir/Profile/Patient") 054public class Patient extends DomainResource { 055 056 public enum LinkType { 057 /** 058 * The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link. 059 */ 060 REPLACEDBY, 061 /** 062 * The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information. 063 */ 064 REPLACES, 065 /** 066 * The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information. 067 */ 068 REFER, 069 /** 070 * The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid. 071 */ 072 SEEALSO, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static LinkType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("replaced-by".equals(codeString)) 081 return REPLACEDBY; 082 if ("replaces".equals(codeString)) 083 return REPLACES; 084 if ("refer".equals(codeString)) 085 return REFER; 086 if ("seealso".equals(codeString)) 087 return SEEALSO; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown LinkType code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case REPLACEDBY: return "replaced-by"; 096 case REPLACES: return "replaces"; 097 case REFER: return "refer"; 098 case SEEALSO: return "seealso"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case REPLACEDBY: return "http://hl7.org/fhir/link-type"; 106 case REPLACES: return "http://hl7.org/fhir/link-type"; 107 case REFER: return "http://hl7.org/fhir/link-type"; 108 case SEEALSO: return "http://hl7.org/fhir/link-type"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case REPLACEDBY: return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 116 case REPLACES: return "The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information."; 117 case REFER: return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 118 case SEEALSO: return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case REPLACEDBY: return "Replaced-by"; 126 case REPLACES: return "Replaces"; 127 case REFER: return "Refer"; 128 case SEEALSO: return "See also"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 136 public LinkType fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("replaced-by".equals(codeString)) 141 return LinkType.REPLACEDBY; 142 if ("replaces".equals(codeString)) 143 return LinkType.REPLACES; 144 if ("refer".equals(codeString)) 145 return LinkType.REFER; 146 if ("seealso".equals(codeString)) 147 return LinkType.SEEALSO; 148 throw new IllegalArgumentException("Unknown LinkType code '"+codeString+"'"); 149 } 150 public Enumeration<LinkType> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<LinkType>(this); 155 String codeString = code.asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("replaced-by".equals(codeString)) 159 return new Enumeration<LinkType>(this, LinkType.REPLACEDBY); 160 if ("replaces".equals(codeString)) 161 return new Enumeration<LinkType>(this, LinkType.REPLACES); 162 if ("refer".equals(codeString)) 163 return new Enumeration<LinkType>(this, LinkType.REFER); 164 if ("seealso".equals(codeString)) 165 return new Enumeration<LinkType>(this, LinkType.SEEALSO); 166 throw new FHIRException("Unknown LinkType code '"+codeString+"'"); 167 } 168 public String toCode(LinkType code) { 169 if (code == LinkType.NULL) 170 return null; 171 if (code == LinkType.REPLACEDBY) 172 return "replaced-by"; 173 if (code == LinkType.REPLACES) 174 return "replaces"; 175 if (code == LinkType.REFER) 176 return "refer"; 177 if (code == LinkType.SEEALSO) 178 return "seealso"; 179 return "?"; 180 } 181 public String toSystem(LinkType code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * The nature of the relationship between the patient and the contact person. 190 */ 191 @Child(name = "relationship", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 192 @Description(shortDefinition="The kind of relationship", formalDefinition="The nature of the relationship between the patient and the contact person." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0131") 194 protected List<CodeableConcept> relationship; 195 196 /** 197 * A name associated with the contact person. 198 */ 199 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="A name associated with the contact person", formalDefinition="A name associated with the contact person." ) 201 protected HumanName name; 202 203 /** 204 * A contact detail for the person, e.g. a telephone number or an email address. 205 */ 206 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 207 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 208 protected List<ContactPoint> telecom; 209 210 /** 211 * Address for the contact person. 212 */ 213 @Child(name = "address", type = {Address.class}, order=4, min=0, max=1, modifier=false, summary=false) 214 @Description(shortDefinition="Address for the contact person", formalDefinition="Address for the contact person." ) 215 protected Address address; 216 217 /** 218 * Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 219 */ 220 @Child(name = "gender", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 221 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes." ) 222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 223 protected Enumeration<AdministrativeGender> gender; 224 225 /** 226 * Organization on behalf of which the contact is acting or for which the contact is working. 227 */ 228 @Child(name = "organization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="Organization that is associated with the contact", formalDefinition="Organization on behalf of which the contact is acting or for which the contact is working." ) 230 protected Reference organization; 231 232 /** 233 * The actual object that is the target of the reference (Organization on behalf of which the contact is acting or for which the contact is working.) 234 */ 235 protected Organization organizationTarget; 236 237 /** 238 * The period during which this contact person or organization is valid to be contacted relating to this patient. 239 */ 240 @Child(name = "period", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=false) 241 @Description(shortDefinition="The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition="The period during which this contact person or organization is valid to be contacted relating to this patient." ) 242 protected Period period; 243 244 private static final long serialVersionUID = 364269017L; 245 246 /** 247 * Constructor 248 */ 249 public ContactComponent() { 250 super(); 251 } 252 253 /** 254 * @return {@link #relationship} (The nature of the relationship between the patient and the contact person.) 255 */ 256 public List<CodeableConcept> getRelationship() { 257 if (this.relationship == null) 258 this.relationship = new ArrayList<CodeableConcept>(); 259 return this.relationship; 260 } 261 262 /** 263 * @return Returns a reference to <code>this</code> for easy method chaining 264 */ 265 public ContactComponent setRelationship(List<CodeableConcept> theRelationship) { 266 this.relationship = theRelationship; 267 return this; 268 } 269 270 public boolean hasRelationship() { 271 if (this.relationship == null) 272 return false; 273 for (CodeableConcept item : this.relationship) 274 if (!item.isEmpty()) 275 return true; 276 return false; 277 } 278 279 public CodeableConcept addRelationship() { //3 280 CodeableConcept t = new CodeableConcept(); 281 if (this.relationship == null) 282 this.relationship = new ArrayList<CodeableConcept>(); 283 this.relationship.add(t); 284 return t; 285 } 286 287 public ContactComponent addRelationship(CodeableConcept t) { //3 288 if (t == null) 289 return this; 290 if (this.relationship == null) 291 this.relationship = new ArrayList<CodeableConcept>(); 292 this.relationship.add(t); 293 return this; 294 } 295 296 /** 297 * @return The first repetition of repeating field {@link #relationship}, creating it if it does not already exist 298 */ 299 public CodeableConcept getRelationshipFirstRep() { 300 if (getRelationship().isEmpty()) { 301 addRelationship(); 302 } 303 return getRelationship().get(0); 304 } 305 306 /** 307 * @return {@link #name} (A name associated with the contact person.) 308 */ 309 public HumanName getName() { 310 if (this.name == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create ContactComponent.name"); 313 else if (Configuration.doAutoCreate()) 314 this.name = new HumanName(); // cc 315 return this.name; 316 } 317 318 public boolean hasName() { 319 return this.name != null && !this.name.isEmpty(); 320 } 321 322 /** 323 * @param value {@link #name} (A name associated with the contact person.) 324 */ 325 public ContactComponent setName(HumanName value) { 326 this.name = value; 327 return this; 328 } 329 330 /** 331 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 332 */ 333 public List<ContactPoint> getTelecom() { 334 if (this.telecom == null) 335 this.telecom = new ArrayList<ContactPoint>(); 336 return this.telecom; 337 } 338 339 /** 340 * @return Returns a reference to <code>this</code> for easy method chaining 341 */ 342 public ContactComponent setTelecom(List<ContactPoint> theTelecom) { 343 this.telecom = theTelecom; 344 return this; 345 } 346 347 public boolean hasTelecom() { 348 if (this.telecom == null) 349 return false; 350 for (ContactPoint item : this.telecom) 351 if (!item.isEmpty()) 352 return true; 353 return false; 354 } 355 356 public ContactPoint addTelecom() { //3 357 ContactPoint t = new ContactPoint(); 358 if (this.telecom == null) 359 this.telecom = new ArrayList<ContactPoint>(); 360 this.telecom.add(t); 361 return t; 362 } 363 364 public ContactComponent addTelecom(ContactPoint t) { //3 365 if (t == null) 366 return this; 367 if (this.telecom == null) 368 this.telecom = new ArrayList<ContactPoint>(); 369 this.telecom.add(t); 370 return this; 371 } 372 373 /** 374 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 375 */ 376 public ContactPoint getTelecomFirstRep() { 377 if (getTelecom().isEmpty()) { 378 addTelecom(); 379 } 380 return getTelecom().get(0); 381 } 382 383 /** 384 * @return {@link #address} (Address for the contact person.) 385 */ 386 public Address getAddress() { 387 if (this.address == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create ContactComponent.address"); 390 else if (Configuration.doAutoCreate()) 391 this.address = new Address(); // cc 392 return this.address; 393 } 394 395 public boolean hasAddress() { 396 return this.address != null && !this.address.isEmpty(); 397 } 398 399 /** 400 * @param value {@link #address} (Address for the contact person.) 401 */ 402 public ContactComponent setAddress(Address value) { 403 this.address = value; 404 return this; 405 } 406 407 /** 408 * @return {@link #gender} (Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 409 */ 410 public Enumeration<AdministrativeGender> getGenderElement() { 411 if (this.gender == null) 412 if (Configuration.errorOnAutoCreate()) 413 throw new Error("Attempt to auto-create ContactComponent.gender"); 414 else if (Configuration.doAutoCreate()) 415 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 416 return this.gender; 417 } 418 419 public boolean hasGenderElement() { 420 return this.gender != null && !this.gender.isEmpty(); 421 } 422 423 public boolean hasGender() { 424 return this.gender != null && !this.gender.isEmpty(); 425 } 426 427 /** 428 * @param value {@link #gender} (Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 429 */ 430 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 431 this.gender = value; 432 return this; 433 } 434 435 /** 436 * @return Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 437 */ 438 public AdministrativeGender getGender() { 439 return this.gender == null ? null : this.gender.getValue(); 440 } 441 442 /** 443 * @param value Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes. 444 */ 445 public ContactComponent setGender(AdministrativeGender value) { 446 if (value == null) 447 this.gender = null; 448 else { 449 if (this.gender == null) 450 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 451 this.gender.setValue(value); 452 } 453 return this; 454 } 455 456 /** 457 * @return {@link #organization} (Organization on behalf of which the contact is acting or for which the contact is working.) 458 */ 459 public Reference getOrganization() { 460 if (this.organization == null) 461 if (Configuration.errorOnAutoCreate()) 462 throw new Error("Attempt to auto-create ContactComponent.organization"); 463 else if (Configuration.doAutoCreate()) 464 this.organization = new Reference(); // cc 465 return this.organization; 466 } 467 468 public boolean hasOrganization() { 469 return this.organization != null && !this.organization.isEmpty(); 470 } 471 472 /** 473 * @param value {@link #organization} (Organization on behalf of which the contact is acting or for which the contact is working.) 474 */ 475 public ContactComponent setOrganization(Reference value) { 476 this.organization = value; 477 return this; 478 } 479 480 /** 481 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Organization on behalf of which the contact is acting or for which the contact is working.) 482 */ 483 public Organization getOrganizationTarget() { 484 if (this.organizationTarget == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create ContactComponent.organization"); 487 else if (Configuration.doAutoCreate()) 488 this.organizationTarget = new Organization(); // aa 489 return this.organizationTarget; 490 } 491 492 /** 493 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Organization on behalf of which the contact is acting or for which the contact is working.) 494 */ 495 public ContactComponent setOrganizationTarget(Organization value) { 496 this.organizationTarget = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #period} (The period during which this contact person or organization is valid to be contacted relating to this patient.) 502 */ 503 public Period getPeriod() { 504 if (this.period == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create ContactComponent.period"); 507 else if (Configuration.doAutoCreate()) 508 this.period = new Period(); // cc 509 return this.period; 510 } 511 512 public boolean hasPeriod() { 513 return this.period != null && !this.period.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #period} (The period during which this contact person or organization is valid to be contacted relating to this patient.) 518 */ 519 public ContactComponent setPeriod(Period value) { 520 this.period = value; 521 return this; 522 } 523 524 protected void listChildren(List<Property> children) { 525 super.listChildren(children); 526 children.add(new Property("relationship", "CodeableConcept", "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, relationship)); 527 children.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name)); 528 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 529 children.add(new Property("address", "Address", "Address for the contact person.", 0, 1, address)); 530 children.add(new Property("gender", "code", "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 531 children.add(new Property("organization", "Reference(Organization)", "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, organization)); 532 children.add(new Property("period", "Period", "The period during which this contact person or organization is valid to be contacted relating to this patient.", 0, 1, period)); 533 } 534 535 @Override 536 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 537 switch (_hash) { 538 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, relationship); 539 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name); 540 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 541 case -1147692044: /*address*/ return new Property("address", "Address", "Address for the contact person.", 0, 1, address); 542 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 0, 1, gender); 543 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, organization); 544 case -991726143: /*period*/ return new Property("period", "Period", "The period during which this contact person or organization is valid to be contacted relating to this patient.", 0, 1, period); 545 default: return super.getNamedProperty(_hash, _name, _checkValid); 546 } 547 548 } 549 550 @Override 551 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 552 switch (hash) { 553 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 554 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // HumanName 555 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 556 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 557 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 558 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 559 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 560 default: return super.getProperty(hash, name, checkValid); 561 } 562 563 } 564 565 @Override 566 public Base setProperty(int hash, String name, Base value) throws FHIRException { 567 switch (hash) { 568 case -261851592: // relationship 569 this.getRelationship().add(castToCodeableConcept(value)); // CodeableConcept 570 return value; 571 case 3373707: // name 572 this.name = castToHumanName(value); // HumanName 573 return value; 574 case -1429363305: // telecom 575 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 576 return value; 577 case -1147692044: // address 578 this.address = castToAddress(value); // Address 579 return value; 580 case -1249512767: // gender 581 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 582 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 583 return value; 584 case 1178922291: // organization 585 this.organization = castToReference(value); // Reference 586 return value; 587 case -991726143: // period 588 this.period = castToPeriod(value); // Period 589 return value; 590 default: return super.setProperty(hash, name, value); 591 } 592 593 } 594 595 @Override 596 public Base setProperty(String name, Base value) throws FHIRException { 597 if (name.equals("relationship")) { 598 this.getRelationship().add(castToCodeableConcept(value)); 599 } else if (name.equals("name")) { 600 this.name = castToHumanName(value); // HumanName 601 } else if (name.equals("telecom")) { 602 this.getTelecom().add(castToContactPoint(value)); 603 } else if (name.equals("address")) { 604 this.address = castToAddress(value); // Address 605 } else if (name.equals("gender")) { 606 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 607 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 608 } else if (name.equals("organization")) { 609 this.organization = castToReference(value); // Reference 610 } else if (name.equals("period")) { 611 this.period = castToPeriod(value); // Period 612 } else 613 return super.setProperty(name, value); 614 return value; 615 } 616 617 @Override 618 public Base makeProperty(int hash, String name) throws FHIRException { 619 switch (hash) { 620 case -261851592: return addRelationship(); 621 case 3373707: return getName(); 622 case -1429363305: return addTelecom(); 623 case -1147692044: return getAddress(); 624 case -1249512767: return getGenderElement(); 625 case 1178922291: return getOrganization(); 626 case -991726143: return getPeriod(); 627 default: return super.makeProperty(hash, name); 628 } 629 630 } 631 632 @Override 633 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 634 switch (hash) { 635 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 636 case 3373707: /*name*/ return new String[] {"HumanName"}; 637 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 638 case -1147692044: /*address*/ return new String[] {"Address"}; 639 case -1249512767: /*gender*/ return new String[] {"code"}; 640 case 1178922291: /*organization*/ return new String[] {"Reference"}; 641 case -991726143: /*period*/ return new String[] {"Period"}; 642 default: return super.getTypesForProperty(hash, name); 643 } 644 645 } 646 647 @Override 648 public Base addChild(String name) throws FHIRException { 649 if (name.equals("relationship")) { 650 return addRelationship(); 651 } 652 else if (name.equals("name")) { 653 this.name = new HumanName(); 654 return this.name; 655 } 656 else if (name.equals("telecom")) { 657 return addTelecom(); 658 } 659 else if (name.equals("address")) { 660 this.address = new Address(); 661 return this.address; 662 } 663 else if (name.equals("gender")) { 664 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 665 } 666 else if (name.equals("organization")) { 667 this.organization = new Reference(); 668 return this.organization; 669 } 670 else if (name.equals("period")) { 671 this.period = new Period(); 672 return this.period; 673 } 674 else 675 return super.addChild(name); 676 } 677 678 public ContactComponent copy() { 679 ContactComponent dst = new ContactComponent(); 680 copyValues(dst); 681 if (relationship != null) { 682 dst.relationship = new ArrayList<CodeableConcept>(); 683 for (CodeableConcept i : relationship) 684 dst.relationship.add(i.copy()); 685 }; 686 dst.name = name == null ? null : name.copy(); 687 if (telecom != null) { 688 dst.telecom = new ArrayList<ContactPoint>(); 689 for (ContactPoint i : telecom) 690 dst.telecom.add(i.copy()); 691 }; 692 dst.address = address == null ? null : address.copy(); 693 dst.gender = gender == null ? null : gender.copy(); 694 dst.organization = organization == null ? null : organization.copy(); 695 dst.period = period == null ? null : period.copy(); 696 return dst; 697 } 698 699 @Override 700 public boolean equalsDeep(Base other_) { 701 if (!super.equalsDeep(other_)) 702 return false; 703 if (!(other_ instanceof ContactComponent)) 704 return false; 705 ContactComponent o = (ContactComponent) other_; 706 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 707 && compareDeep(address, o.address, true) && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 708 && compareDeep(period, o.period, true); 709 } 710 711 @Override 712 public boolean equalsShallow(Base other_) { 713 if (!super.equalsShallow(other_)) 714 return false; 715 if (!(other_ instanceof ContactComponent)) 716 return false; 717 ContactComponent o = (ContactComponent) other_; 718 return compareValues(gender, o.gender, true); 719 } 720 721 public boolean isEmpty() { 722 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, name, telecom 723 , address, gender, organization, period); 724 } 725 726 public String fhirType() { 727 return "Patient.contact"; 728 729 } 730 731 } 732 733 @Block() 734 public static class AnimalComponent extends BackboneElement implements IBaseBackboneElement { 735 /** 736 * Identifies the high level taxonomic categorization of the kind of animal. 737 */ 738 @Child(name = "species", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 739 @Description(shortDefinition="E.g. Dog, Cow", formalDefinition="Identifies the high level taxonomic categorization of the kind of animal." ) 740 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/animal-species") 741 protected CodeableConcept species; 742 743 /** 744 * Identifies the detailed categorization of the kind of animal. 745 */ 746 @Child(name = "breed", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 747 @Description(shortDefinition="E.g. Poodle, Angus", formalDefinition="Identifies the detailed categorization of the kind of animal." ) 748 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/animal-breeds") 749 protected CodeableConcept breed; 750 751 /** 752 * Indicates the current state of the animal's reproductive organs. 753 */ 754 @Child(name = "genderStatus", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 755 @Description(shortDefinition="E.g. Neutered, Intact", formalDefinition="Indicates the current state of the animal's reproductive organs." ) 756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/animal-genderstatus") 757 protected CodeableConcept genderStatus; 758 759 private static final long serialVersionUID = -549738382L; 760 761 /** 762 * Constructor 763 */ 764 public AnimalComponent() { 765 super(); 766 } 767 768 /** 769 * Constructor 770 */ 771 public AnimalComponent(CodeableConcept species) { 772 super(); 773 this.species = species; 774 } 775 776 /** 777 * @return {@link #species} (Identifies the high level taxonomic categorization of the kind of animal.) 778 */ 779 public CodeableConcept getSpecies() { 780 if (this.species == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create AnimalComponent.species"); 783 else if (Configuration.doAutoCreate()) 784 this.species = new CodeableConcept(); // cc 785 return this.species; 786 } 787 788 public boolean hasSpecies() { 789 return this.species != null && !this.species.isEmpty(); 790 } 791 792 /** 793 * @param value {@link #species} (Identifies the high level taxonomic categorization of the kind of animal.) 794 */ 795 public AnimalComponent setSpecies(CodeableConcept value) { 796 this.species = value; 797 return this; 798 } 799 800 /** 801 * @return {@link #breed} (Identifies the detailed categorization of the kind of animal.) 802 */ 803 public CodeableConcept getBreed() { 804 if (this.breed == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create AnimalComponent.breed"); 807 else if (Configuration.doAutoCreate()) 808 this.breed = new CodeableConcept(); // cc 809 return this.breed; 810 } 811 812 public boolean hasBreed() { 813 return this.breed != null && !this.breed.isEmpty(); 814 } 815 816 /** 817 * @param value {@link #breed} (Identifies the detailed categorization of the kind of animal.) 818 */ 819 public AnimalComponent setBreed(CodeableConcept value) { 820 this.breed = value; 821 return this; 822 } 823 824 /** 825 * @return {@link #genderStatus} (Indicates the current state of the animal's reproductive organs.) 826 */ 827 public CodeableConcept getGenderStatus() { 828 if (this.genderStatus == null) 829 if (Configuration.errorOnAutoCreate()) 830 throw new Error("Attempt to auto-create AnimalComponent.genderStatus"); 831 else if (Configuration.doAutoCreate()) 832 this.genderStatus = new CodeableConcept(); // cc 833 return this.genderStatus; 834 } 835 836 public boolean hasGenderStatus() { 837 return this.genderStatus != null && !this.genderStatus.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #genderStatus} (Indicates the current state of the animal's reproductive organs.) 842 */ 843 public AnimalComponent setGenderStatus(CodeableConcept value) { 844 this.genderStatus = value; 845 return this; 846 } 847 848 protected void listChildren(List<Property> children) { 849 super.listChildren(children); 850 children.add(new Property("species", "CodeableConcept", "Identifies the high level taxonomic categorization of the kind of animal.", 0, 1, species)); 851 children.add(new Property("breed", "CodeableConcept", "Identifies the detailed categorization of the kind of animal.", 0, 1, breed)); 852 children.add(new Property("genderStatus", "CodeableConcept", "Indicates the current state of the animal's reproductive organs.", 0, 1, genderStatus)); 853 } 854 855 @Override 856 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 857 switch (_hash) { 858 case -2008465092: /*species*/ return new Property("species", "CodeableConcept", "Identifies the high level taxonomic categorization of the kind of animal.", 0, 1, species); 859 case 94001524: /*breed*/ return new Property("breed", "CodeableConcept", "Identifies the detailed categorization of the kind of animal.", 0, 1, breed); 860 case -678569453: /*genderStatus*/ return new Property("genderStatus", "CodeableConcept", "Indicates the current state of the animal's reproductive organs.", 0, 1, genderStatus); 861 default: return super.getNamedProperty(_hash, _name, _checkValid); 862 } 863 864 } 865 866 @Override 867 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 868 switch (hash) { 869 case -2008465092: /*species*/ return this.species == null ? new Base[0] : new Base[] {this.species}; // CodeableConcept 870 case 94001524: /*breed*/ return this.breed == null ? new Base[0] : new Base[] {this.breed}; // CodeableConcept 871 case -678569453: /*genderStatus*/ return this.genderStatus == null ? new Base[0] : new Base[] {this.genderStatus}; // CodeableConcept 872 default: return super.getProperty(hash, name, checkValid); 873 } 874 875 } 876 877 @Override 878 public Base setProperty(int hash, String name, Base value) throws FHIRException { 879 switch (hash) { 880 case -2008465092: // species 881 this.species = castToCodeableConcept(value); // CodeableConcept 882 return value; 883 case 94001524: // breed 884 this.breed = castToCodeableConcept(value); // CodeableConcept 885 return value; 886 case -678569453: // genderStatus 887 this.genderStatus = castToCodeableConcept(value); // CodeableConcept 888 return value; 889 default: return super.setProperty(hash, name, value); 890 } 891 892 } 893 894 @Override 895 public Base setProperty(String name, Base value) throws FHIRException { 896 if (name.equals("species")) { 897 this.species = castToCodeableConcept(value); // CodeableConcept 898 } else if (name.equals("breed")) { 899 this.breed = castToCodeableConcept(value); // CodeableConcept 900 } else if (name.equals("genderStatus")) { 901 this.genderStatus = castToCodeableConcept(value); // CodeableConcept 902 } else 903 return super.setProperty(name, value); 904 return value; 905 } 906 907 @Override 908 public Base makeProperty(int hash, String name) throws FHIRException { 909 switch (hash) { 910 case -2008465092: return getSpecies(); 911 case 94001524: return getBreed(); 912 case -678569453: return getGenderStatus(); 913 default: return super.makeProperty(hash, name); 914 } 915 916 } 917 918 @Override 919 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 920 switch (hash) { 921 case -2008465092: /*species*/ return new String[] {"CodeableConcept"}; 922 case 94001524: /*breed*/ return new String[] {"CodeableConcept"}; 923 case -678569453: /*genderStatus*/ return new String[] {"CodeableConcept"}; 924 default: return super.getTypesForProperty(hash, name); 925 } 926 927 } 928 929 @Override 930 public Base addChild(String name) throws FHIRException { 931 if (name.equals("species")) { 932 this.species = new CodeableConcept(); 933 return this.species; 934 } 935 else if (name.equals("breed")) { 936 this.breed = new CodeableConcept(); 937 return this.breed; 938 } 939 else if (name.equals("genderStatus")) { 940 this.genderStatus = new CodeableConcept(); 941 return this.genderStatus; 942 } 943 else 944 return super.addChild(name); 945 } 946 947 public AnimalComponent copy() { 948 AnimalComponent dst = new AnimalComponent(); 949 copyValues(dst); 950 dst.species = species == null ? null : species.copy(); 951 dst.breed = breed == null ? null : breed.copy(); 952 dst.genderStatus = genderStatus == null ? null : genderStatus.copy(); 953 return dst; 954 } 955 956 @Override 957 public boolean equalsDeep(Base other_) { 958 if (!super.equalsDeep(other_)) 959 return false; 960 if (!(other_ instanceof AnimalComponent)) 961 return false; 962 AnimalComponent o = (AnimalComponent) other_; 963 return compareDeep(species, o.species, true) && compareDeep(breed, o.breed, true) && compareDeep(genderStatus, o.genderStatus, true) 964 ; 965 } 966 967 @Override 968 public boolean equalsShallow(Base other_) { 969 if (!super.equalsShallow(other_)) 970 return false; 971 if (!(other_ instanceof AnimalComponent)) 972 return false; 973 AnimalComponent o = (AnimalComponent) other_; 974 return true; 975 } 976 977 public boolean isEmpty() { 978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(species, breed, genderStatus 979 ); 980 } 981 982 public String fhirType() { 983 return "Patient.animal"; 984 985 } 986 987 } 988 989 @Block() 990 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 991 /** 992 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English. 993 */ 994 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 995 @Description(shortDefinition="The language which can be used to communicate with the patient about his or her health", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English." ) 996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 997 protected CodeableConcept language; 998 999 /** 1000 * Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 1001 */ 1002 @Child(name = "preferred", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1003 @Description(shortDefinition="Language preference indicator", formalDefinition="Indicates whether or not the patient prefers this language (over other languages he masters up a certain level)." ) 1004 protected BooleanType preferred; 1005 1006 private static final long serialVersionUID = 633792918L; 1007 1008 /** 1009 * Constructor 1010 */ 1011 public PatientCommunicationComponent() { 1012 super(); 1013 } 1014 1015 /** 1016 * Constructor 1017 */ 1018 public PatientCommunicationComponent(CodeableConcept language) { 1019 super(); 1020 this.language = language; 1021 } 1022 1023 /** 1024 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 1025 */ 1026 public CodeableConcept getLanguage() { 1027 if (this.language == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 1030 else if (Configuration.doAutoCreate()) 1031 this.language = new CodeableConcept(); // cc 1032 return this.language; 1033 } 1034 1035 public boolean hasLanguage() { 1036 return this.language != null && !this.language.isEmpty(); 1037 } 1038 1039 /** 1040 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 1041 */ 1042 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 1043 this.language = value; 1044 return this; 1045 } 1046 1047 /** 1048 * @return {@link #preferred} (Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 1049 */ 1050 public BooleanType getPreferredElement() { 1051 if (this.preferred == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 1054 else if (Configuration.doAutoCreate()) 1055 this.preferred = new BooleanType(); // bb 1056 return this.preferred; 1057 } 1058 1059 public boolean hasPreferredElement() { 1060 return this.preferred != null && !this.preferred.isEmpty(); 1061 } 1062 1063 public boolean hasPreferred() { 1064 return this.preferred != null && !this.preferred.isEmpty(); 1065 } 1066 1067 /** 1068 * @param value {@link #preferred} (Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 1069 */ 1070 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 1071 this.preferred = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 1077 */ 1078 public boolean getPreferred() { 1079 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 1080 } 1081 1082 /** 1083 * @param value Indicates whether or not the patient prefers this language (over other languages he masters up a certain level). 1084 */ 1085 public PatientCommunicationComponent setPreferred(boolean value) { 1086 if (this.preferred == null) 1087 this.preferred = new BooleanType(); 1088 this.preferred.setValue(value); 1089 return this; 1090 } 1091 1092 protected void listChildren(List<Property> children) { 1093 super.listChildren(children); 1094 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language)); 1095 children.add(new Property("preferred", "boolean", "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 0, 1, preferred)); 1096 } 1097 1098 @Override 1099 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1100 switch (_hash) { 1101 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language); 1102 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 0, 1, preferred); 1103 default: return super.getNamedProperty(_hash, _name, _checkValid); 1104 } 1105 1106 } 1107 1108 @Override 1109 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1110 switch (hash) { 1111 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 1112 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 1113 default: return super.getProperty(hash, name, checkValid); 1114 } 1115 1116 } 1117 1118 @Override 1119 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1120 switch (hash) { 1121 case -1613589672: // language 1122 this.language = castToCodeableConcept(value); // CodeableConcept 1123 return value; 1124 case -1294005119: // preferred 1125 this.preferred = castToBoolean(value); // BooleanType 1126 return value; 1127 default: return super.setProperty(hash, name, value); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base setProperty(String name, Base value) throws FHIRException { 1134 if (name.equals("language")) { 1135 this.language = castToCodeableConcept(value); // CodeableConcept 1136 } else if (name.equals("preferred")) { 1137 this.preferred = castToBoolean(value); // BooleanType 1138 } else 1139 return super.setProperty(name, value); 1140 return value; 1141 } 1142 1143 @Override 1144 public Base makeProperty(int hash, String name) throws FHIRException { 1145 switch (hash) { 1146 case -1613589672: return getLanguage(); 1147 case -1294005119: return getPreferredElement(); 1148 default: return super.makeProperty(hash, name); 1149 } 1150 1151 } 1152 1153 @Override 1154 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1155 switch (hash) { 1156 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 1157 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 1158 default: return super.getTypesForProperty(hash, name); 1159 } 1160 1161 } 1162 1163 @Override 1164 public Base addChild(String name) throws FHIRException { 1165 if (name.equals("language")) { 1166 this.language = new CodeableConcept(); 1167 return this.language; 1168 } 1169 else if (name.equals("preferred")) { 1170 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1171 } 1172 else 1173 return super.addChild(name); 1174 } 1175 1176 public PatientCommunicationComponent copy() { 1177 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1178 copyValues(dst); 1179 dst.language = language == null ? null : language.copy(); 1180 dst.preferred = preferred == null ? null : preferred.copy(); 1181 return dst; 1182 } 1183 1184 @Override 1185 public boolean equalsDeep(Base other_) { 1186 if (!super.equalsDeep(other_)) 1187 return false; 1188 if (!(other_ instanceof PatientCommunicationComponent)) 1189 return false; 1190 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1191 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1192 } 1193 1194 @Override 1195 public boolean equalsShallow(Base other_) { 1196 if (!super.equalsShallow(other_)) 1197 return false; 1198 if (!(other_ instanceof PatientCommunicationComponent)) 1199 return false; 1200 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1201 return compareValues(preferred, o.preferred, true); 1202 } 1203 1204 public boolean isEmpty() { 1205 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 1206 } 1207 1208 public String fhirType() { 1209 return "Patient.communication"; 1210 1211 } 1212 1213 } 1214 1215 @Block() 1216 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1217 /** 1218 * The other patient resource that the link refers to. 1219 */ 1220 @Child(name = "other", type = {Patient.class, RelatedPerson.class}, order=1, min=1, max=1, modifier=false, summary=true) 1221 @Description(shortDefinition="The other patient or related person resource that the link refers to", formalDefinition="The other patient resource that the link refers to." ) 1222 protected Reference other; 1223 1224 /** 1225 * The actual object that is the target of the reference (The other patient resource that the link refers to.) 1226 */ 1227 protected Resource otherTarget; 1228 1229 /** 1230 * The type of link between this patient resource and another patient resource. 1231 */ 1232 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1233 @Description(shortDefinition="replaced-by | replaces | refer | seealso - type of link", formalDefinition="The type of link between this patient resource and another patient resource." ) 1234 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/link-type") 1235 protected Enumeration<LinkType> type; 1236 1237 private static final long serialVersionUID = 1083576633L; 1238 1239 /** 1240 * Constructor 1241 */ 1242 public PatientLinkComponent() { 1243 super(); 1244 } 1245 1246 /** 1247 * Constructor 1248 */ 1249 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1250 super(); 1251 this.other = other; 1252 this.type = type; 1253 } 1254 1255 /** 1256 * @return {@link #other} (The other patient resource that the link refers to.) 1257 */ 1258 public Reference getOther() { 1259 if (this.other == null) 1260 if (Configuration.errorOnAutoCreate()) 1261 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1262 else if (Configuration.doAutoCreate()) 1263 this.other = new Reference(); // cc 1264 return this.other; 1265 } 1266 1267 public boolean hasOther() { 1268 return this.other != null && !this.other.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #other} (The other patient resource that the link refers to.) 1273 */ 1274 public PatientLinkComponent setOther(Reference value) { 1275 this.other = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #other} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The other patient resource that the link refers to.) 1281 */ 1282 public Resource getOtherTarget() { 1283 return this.otherTarget; 1284 } 1285 1286 /** 1287 * @param value {@link #other} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The other patient resource that the link refers to.) 1288 */ 1289 public PatientLinkComponent setOtherTarget(Resource value) { 1290 this.otherTarget = value; 1291 return this; 1292 } 1293 1294 /** 1295 * @return {@link #type} (The type of link between this patient resource and another patient resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1296 */ 1297 public Enumeration<LinkType> getTypeElement() { 1298 if (this.type == null) 1299 if (Configuration.errorOnAutoCreate()) 1300 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1301 else if (Configuration.doAutoCreate()) 1302 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1303 return this.type; 1304 } 1305 1306 public boolean hasTypeElement() { 1307 return this.type != null && !this.type.isEmpty(); 1308 } 1309 1310 public boolean hasType() { 1311 return this.type != null && !this.type.isEmpty(); 1312 } 1313 1314 /** 1315 * @param value {@link #type} (The type of link between this patient resource and another patient resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1316 */ 1317 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1318 this.type = value; 1319 return this; 1320 } 1321 1322 /** 1323 * @return The type of link between this patient resource and another patient resource. 1324 */ 1325 public LinkType getType() { 1326 return this.type == null ? null : this.type.getValue(); 1327 } 1328 1329 /** 1330 * @param value The type of link between this patient resource and another patient resource. 1331 */ 1332 public PatientLinkComponent setType(LinkType value) { 1333 if (this.type == null) 1334 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1335 this.type.setValue(value); 1336 return this; 1337 } 1338 1339 protected void listChildren(List<Property> children) { 1340 super.listChildren(children); 1341 children.add(new Property("other", "Reference(Patient|RelatedPerson)", "The other patient resource that the link refers to.", 0, 1, other)); 1342 children.add(new Property("type", "code", "The type of link between this patient resource and another patient resource.", 0, 1, type)); 1343 } 1344 1345 @Override 1346 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1347 switch (_hash) { 1348 case 106069776: /*other*/ return new Property("other", "Reference(Patient|RelatedPerson)", "The other patient resource that the link refers to.", 0, 1, other); 1349 case 3575610: /*type*/ return new Property("type", "code", "The type of link between this patient resource and another patient resource.", 0, 1, type); 1350 default: return super.getNamedProperty(_hash, _name, _checkValid); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1357 switch (hash) { 1358 case 106069776: /*other*/ return this.other == null ? new Base[0] : new Base[] {this.other}; // Reference 1359 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<LinkType> 1360 default: return super.getProperty(hash, name, checkValid); 1361 } 1362 1363 } 1364 1365 @Override 1366 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1367 switch (hash) { 1368 case 106069776: // other 1369 this.other = castToReference(value); // Reference 1370 return value; 1371 case 3575610: // type 1372 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1373 this.type = (Enumeration) value; // Enumeration<LinkType> 1374 return value; 1375 default: return super.setProperty(hash, name, value); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(String name, Base value) throws FHIRException { 1382 if (name.equals("other")) { 1383 this.other = castToReference(value); // Reference 1384 } else if (name.equals("type")) { 1385 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1386 this.type = (Enumeration) value; // Enumeration<LinkType> 1387 } else 1388 return super.setProperty(name, value); 1389 return value; 1390 } 1391 1392 @Override 1393 public Base makeProperty(int hash, String name) throws FHIRException { 1394 switch (hash) { 1395 case 106069776: return getOther(); 1396 case 3575610: return getTypeElement(); 1397 default: return super.makeProperty(hash, name); 1398 } 1399 1400 } 1401 1402 @Override 1403 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1404 switch (hash) { 1405 case 106069776: /*other*/ return new String[] {"Reference"}; 1406 case 3575610: /*type*/ return new String[] {"code"}; 1407 default: return super.getTypesForProperty(hash, name); 1408 } 1409 1410 } 1411 1412 @Override 1413 public Base addChild(String name) throws FHIRException { 1414 if (name.equals("other")) { 1415 this.other = new Reference(); 1416 return this.other; 1417 } 1418 else if (name.equals("type")) { 1419 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1420 } 1421 else 1422 return super.addChild(name); 1423 } 1424 1425 public PatientLinkComponent copy() { 1426 PatientLinkComponent dst = new PatientLinkComponent(); 1427 copyValues(dst); 1428 dst.other = other == null ? null : other.copy(); 1429 dst.type = type == null ? null : type.copy(); 1430 return dst; 1431 } 1432 1433 @Override 1434 public boolean equalsDeep(Base other_) { 1435 if (!super.equalsDeep(other_)) 1436 return false; 1437 if (!(other_ instanceof PatientLinkComponent)) 1438 return false; 1439 PatientLinkComponent o = (PatientLinkComponent) other_; 1440 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1441 } 1442 1443 @Override 1444 public boolean equalsShallow(Base other_) { 1445 if (!super.equalsShallow(other_)) 1446 return false; 1447 if (!(other_ instanceof PatientLinkComponent)) 1448 return false; 1449 PatientLinkComponent o = (PatientLinkComponent) other_; 1450 return compareValues(type, o.type, true); 1451 } 1452 1453 public boolean isEmpty() { 1454 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(other, type); 1455 } 1456 1457 public String fhirType() { 1458 return "Patient.link"; 1459 1460 } 1461 1462 } 1463 1464 /** 1465 * An identifier for this patient. 1466 */ 1467 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1468 @Description(shortDefinition="An identifier for this patient", formalDefinition="An identifier for this patient." ) 1469 protected List<Identifier> identifier; 1470 1471 /** 1472 * Whether this patient record is in active use. 1473 */ 1474 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1475 @Description(shortDefinition="Whether this patient's record is in active use", formalDefinition="Whether this patient record is in active use." ) 1476 protected BooleanType active; 1477 1478 /** 1479 * A name associated with the individual. 1480 */ 1481 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1482 @Description(shortDefinition="A name associated with the patient", formalDefinition="A name associated with the individual." ) 1483 protected List<HumanName> name; 1484 1485 /** 1486 * A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted. 1487 */ 1488 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1489 @Description(shortDefinition="A contact detail for the individual", formalDefinition="A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted." ) 1490 protected List<ContactPoint> telecom; 1491 1492 /** 1493 * Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1494 */ 1495 @Child(name = "gender", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1496 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes." ) 1497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 1498 protected Enumeration<AdministrativeGender> gender; 1499 1500 /** 1501 * The date of birth for the individual. 1502 */ 1503 @Child(name = "birthDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1504 @Description(shortDefinition="The date of birth for the individual", formalDefinition="The date of birth for the individual." ) 1505 protected DateType birthDate; 1506 1507 /** 1508 * Indicates if the individual is deceased or not. 1509 */ 1510 @Child(name = "deceased", type = {BooleanType.class, DateTimeType.class}, order=6, min=0, max=1, modifier=true, summary=true) 1511 @Description(shortDefinition="Indicates if the individual is deceased or not", formalDefinition="Indicates if the individual is deceased or not." ) 1512 protected Type deceased; 1513 1514 /** 1515 * Addresses for the individual. 1516 */ 1517 @Child(name = "address", type = {Address.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1518 @Description(shortDefinition="Addresses for the individual", formalDefinition="Addresses for the individual." ) 1519 protected List<Address> address; 1520 1521 /** 1522 * This field contains a patient's most recent marital (civil) status. 1523 */ 1524 @Child(name = "maritalStatus", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1525 @Description(shortDefinition="Marital (civil) status of a patient", formalDefinition="This field contains a patient's most recent marital (civil) status." ) 1526 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/marital-status") 1527 protected CodeableConcept maritalStatus; 1528 1529 /** 1530 * Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer). 1531 */ 1532 @Child(name = "multipleBirth", type = {BooleanType.class, IntegerType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1533 @Description(shortDefinition="Whether patient is part of a multiple birth", formalDefinition="Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer)." ) 1534 protected Type multipleBirth; 1535 1536 /** 1537 * Image of the patient. 1538 */ 1539 @Child(name = "photo", type = {Attachment.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1540 @Description(shortDefinition="Image of the patient", formalDefinition="Image of the patient." ) 1541 protected List<Attachment> photo; 1542 1543 /** 1544 * A contact party (e.g. guardian, partner, friend) for the patient. 1545 */ 1546 @Child(name = "contact", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1547 @Description(shortDefinition="A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition="A contact party (e.g. guardian, partner, friend) for the patient." ) 1548 protected List<ContactComponent> contact; 1549 1550 /** 1551 * This patient is known to be an animal. 1552 */ 1553 @Child(name = "animal", type = {}, order=12, min=0, max=1, modifier=true, summary=true) 1554 @Description(shortDefinition="This patient is known to be an animal (non-human)", formalDefinition="This patient is known to be an animal." ) 1555 protected AnimalComponent animal; 1556 1557 /** 1558 * Languages which may be used to communicate with the patient about his or her health. 1559 */ 1560 @Child(name = "communication", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1561 @Description(shortDefinition="A list of Languages which may be used to communicate with the patient about his or her health", formalDefinition="Languages which may be used to communicate with the patient about his or her health." ) 1562 protected List<PatientCommunicationComponent> communication; 1563 1564 /** 1565 * Patient's nominated care provider. 1566 */ 1567 @Child(name = "generalPractitioner", type = {Organization.class, Practitioner.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1568 @Description(shortDefinition="Patient's nominated primary care provider", formalDefinition="Patient's nominated care provider." ) 1569 protected List<Reference> generalPractitioner; 1570 /** 1571 * The actual objects that are the target of the reference (Patient's nominated care provider.) 1572 */ 1573 protected List<Resource> generalPractitionerTarget; 1574 1575 1576 /** 1577 * Organization that is the custodian of the patient record. 1578 */ 1579 @Child(name = "managingOrganization", type = {Organization.class}, order=15, min=0, max=1, modifier=false, summary=true) 1580 @Description(shortDefinition="Organization that is the custodian of the patient record", formalDefinition="Organization that is the custodian of the patient record." ) 1581 protected Reference managingOrganization; 1582 1583 /** 1584 * The actual object that is the target of the reference (Organization that is the custodian of the patient record.) 1585 */ 1586 protected Organization managingOrganizationTarget; 1587 1588 /** 1589 * Link to another patient resource that concerns the same actual patient. 1590 */ 1591 @Child(name = "link", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 1592 @Description(shortDefinition="Link to another patient resource that concerns the same actual person", formalDefinition="Link to another patient resource that concerns the same actual patient." ) 1593 protected List<PatientLinkComponent> link; 1594 1595 private static final long serialVersionUID = -1985061666L; 1596 1597 /** 1598 * Constructor 1599 */ 1600 public Patient() { 1601 super(); 1602 } 1603 1604 /** 1605 * @return {@link #identifier} (An identifier for this patient.) 1606 */ 1607 public List<Identifier> getIdentifier() { 1608 if (this.identifier == null) 1609 this.identifier = new ArrayList<Identifier>(); 1610 return this.identifier; 1611 } 1612 1613 /** 1614 * @return Returns a reference to <code>this</code> for easy method chaining 1615 */ 1616 public Patient setIdentifier(List<Identifier> theIdentifier) { 1617 this.identifier = theIdentifier; 1618 return this; 1619 } 1620 1621 public boolean hasIdentifier() { 1622 if (this.identifier == null) 1623 return false; 1624 for (Identifier item : this.identifier) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 public Identifier addIdentifier() { //3 1631 Identifier t = new Identifier(); 1632 if (this.identifier == null) 1633 this.identifier = new ArrayList<Identifier>(); 1634 this.identifier.add(t); 1635 return t; 1636 } 1637 1638 public Patient addIdentifier(Identifier t) { //3 1639 if (t == null) 1640 return this; 1641 if (this.identifier == null) 1642 this.identifier = new ArrayList<Identifier>(); 1643 this.identifier.add(t); 1644 return this; 1645 } 1646 1647 /** 1648 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1649 */ 1650 public Identifier getIdentifierFirstRep() { 1651 if (getIdentifier().isEmpty()) { 1652 addIdentifier(); 1653 } 1654 return getIdentifier().get(0); 1655 } 1656 1657 /** 1658 * @return {@link #active} (Whether this patient record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1659 */ 1660 public BooleanType getActiveElement() { 1661 if (this.active == null) 1662 if (Configuration.errorOnAutoCreate()) 1663 throw new Error("Attempt to auto-create Patient.active"); 1664 else if (Configuration.doAutoCreate()) 1665 this.active = new BooleanType(); // bb 1666 return this.active; 1667 } 1668 1669 public boolean hasActiveElement() { 1670 return this.active != null && !this.active.isEmpty(); 1671 } 1672 1673 public boolean hasActive() { 1674 return this.active != null && !this.active.isEmpty(); 1675 } 1676 1677 /** 1678 * @param value {@link #active} (Whether this patient record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1679 */ 1680 public Patient setActiveElement(BooleanType value) { 1681 this.active = value; 1682 return this; 1683 } 1684 1685 /** 1686 * @return Whether this patient record is in active use. 1687 */ 1688 public boolean getActive() { 1689 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1690 } 1691 1692 /** 1693 * @param value Whether this patient record is in active use. 1694 */ 1695 public Patient setActive(boolean value) { 1696 if (this.active == null) 1697 this.active = new BooleanType(); 1698 this.active.setValue(value); 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #name} (A name associated with the individual.) 1704 */ 1705 public List<HumanName> getName() { 1706 if (this.name == null) 1707 this.name = new ArrayList<HumanName>(); 1708 return this.name; 1709 } 1710 1711 /** 1712 * @return Returns a reference to <code>this</code> for easy method chaining 1713 */ 1714 public Patient setName(List<HumanName> theName) { 1715 this.name = theName; 1716 return this; 1717 } 1718 1719 public boolean hasName() { 1720 if (this.name == null) 1721 return false; 1722 for (HumanName item : this.name) 1723 if (!item.isEmpty()) 1724 return true; 1725 return false; 1726 } 1727 1728 public HumanName addName() { //3 1729 HumanName t = new HumanName(); 1730 if (this.name == null) 1731 this.name = new ArrayList<HumanName>(); 1732 this.name.add(t); 1733 return t; 1734 } 1735 1736 public Patient addName(HumanName t) { //3 1737 if (t == null) 1738 return this; 1739 if (this.name == null) 1740 this.name = new ArrayList<HumanName>(); 1741 this.name.add(t); 1742 return this; 1743 } 1744 1745 /** 1746 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist 1747 */ 1748 public HumanName getNameFirstRep() { 1749 if (getName().isEmpty()) { 1750 addName(); 1751 } 1752 return getName().get(0); 1753 } 1754 1755 /** 1756 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.) 1757 */ 1758 public List<ContactPoint> getTelecom() { 1759 if (this.telecom == null) 1760 this.telecom = new ArrayList<ContactPoint>(); 1761 return this.telecom; 1762 } 1763 1764 /** 1765 * @return Returns a reference to <code>this</code> for easy method chaining 1766 */ 1767 public Patient setTelecom(List<ContactPoint> theTelecom) { 1768 this.telecom = theTelecom; 1769 return this; 1770 } 1771 1772 public boolean hasTelecom() { 1773 if (this.telecom == null) 1774 return false; 1775 for (ContactPoint item : this.telecom) 1776 if (!item.isEmpty()) 1777 return true; 1778 return false; 1779 } 1780 1781 public ContactPoint addTelecom() { //3 1782 ContactPoint t = new ContactPoint(); 1783 if (this.telecom == null) 1784 this.telecom = new ArrayList<ContactPoint>(); 1785 this.telecom.add(t); 1786 return t; 1787 } 1788 1789 public Patient addTelecom(ContactPoint t) { //3 1790 if (t == null) 1791 return this; 1792 if (this.telecom == null) 1793 this.telecom = new ArrayList<ContactPoint>(); 1794 this.telecom.add(t); 1795 return this; 1796 } 1797 1798 /** 1799 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 1800 */ 1801 public ContactPoint getTelecomFirstRep() { 1802 if (getTelecom().isEmpty()) { 1803 addTelecom(); 1804 } 1805 return getTelecom().get(0); 1806 } 1807 1808 /** 1809 * @return {@link #gender} (Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1810 */ 1811 public Enumeration<AdministrativeGender> getGenderElement() { 1812 if (this.gender == null) 1813 if (Configuration.errorOnAutoCreate()) 1814 throw new Error("Attempt to auto-create Patient.gender"); 1815 else if (Configuration.doAutoCreate()) 1816 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1817 return this.gender; 1818 } 1819 1820 public boolean hasGenderElement() { 1821 return this.gender != null && !this.gender.isEmpty(); 1822 } 1823 1824 public boolean hasGender() { 1825 return this.gender != null && !this.gender.isEmpty(); 1826 } 1827 1828 /** 1829 * @param value {@link #gender} (Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1830 */ 1831 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1832 this.gender = value; 1833 return this; 1834 } 1835 1836 /** 1837 * @return Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1838 */ 1839 public AdministrativeGender getGender() { 1840 return this.gender == null ? null : this.gender.getValue(); 1841 } 1842 1843 /** 1844 * @param value Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes. 1845 */ 1846 public Patient setGender(AdministrativeGender value) { 1847 if (value == null) 1848 this.gender = null; 1849 else { 1850 if (this.gender == null) 1851 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1852 this.gender.setValue(value); 1853 } 1854 return this; 1855 } 1856 1857 /** 1858 * @return {@link #birthDate} (The date of birth for the individual.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1859 */ 1860 public DateType getBirthDateElement() { 1861 if (this.birthDate == null) 1862 if (Configuration.errorOnAutoCreate()) 1863 throw new Error("Attempt to auto-create Patient.birthDate"); 1864 else if (Configuration.doAutoCreate()) 1865 this.birthDate = new DateType(); // bb 1866 return this.birthDate; 1867 } 1868 1869 public boolean hasBirthDateElement() { 1870 return this.birthDate != null && !this.birthDate.isEmpty(); 1871 } 1872 1873 public boolean hasBirthDate() { 1874 return this.birthDate != null && !this.birthDate.isEmpty(); 1875 } 1876 1877 /** 1878 * @param value {@link #birthDate} (The date of birth for the individual.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 1879 */ 1880 public Patient setBirthDateElement(DateType value) { 1881 this.birthDate = value; 1882 return this; 1883 } 1884 1885 /** 1886 * @return The date of birth for the individual. 1887 */ 1888 public Date getBirthDate() { 1889 return this.birthDate == null ? null : this.birthDate.getValue(); 1890 } 1891 1892 /** 1893 * @param value The date of birth for the individual. 1894 */ 1895 public Patient setBirthDate(Date value) { 1896 if (value == null) 1897 this.birthDate = null; 1898 else { 1899 if (this.birthDate == null) 1900 this.birthDate = new DateType(); 1901 this.birthDate.setValue(value); 1902 } 1903 return this; 1904 } 1905 1906 /** 1907 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1908 */ 1909 public Type getDeceased() { 1910 return this.deceased; 1911 } 1912 1913 /** 1914 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1915 */ 1916 public BooleanType getDeceasedBooleanType() throws FHIRException { 1917 if (this.deceased == null) 1918 return null; 1919 if (!(this.deceased instanceof BooleanType)) 1920 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1921 return (BooleanType) this.deceased; 1922 } 1923 1924 public boolean hasDeceasedBooleanType() { 1925 return this != null && this.deceased instanceof BooleanType; 1926 } 1927 1928 /** 1929 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1930 */ 1931 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1932 if (this.deceased == null) 1933 return null; 1934 if (!(this.deceased instanceof DateTimeType)) 1935 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1936 return (DateTimeType) this.deceased; 1937 } 1938 1939 public boolean hasDeceasedDateTimeType() { 1940 return this != null && this.deceased instanceof DateTimeType; 1941 } 1942 1943 public boolean hasDeceased() { 1944 return this.deceased != null && !this.deceased.isEmpty(); 1945 } 1946 1947 /** 1948 * @param value {@link #deceased} (Indicates if the individual is deceased or not.) 1949 */ 1950 public Patient setDeceased(Type value) throws FHIRFormatError { 1951 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 1952 throw new FHIRFormatError("Not the right type for Patient.deceased[x]: "+value.fhirType()); 1953 this.deceased = value; 1954 return this; 1955 } 1956 1957 /** 1958 * @return {@link #address} (Addresses for the individual.) 1959 */ 1960 public List<Address> getAddress() { 1961 if (this.address == null) 1962 this.address = new ArrayList<Address>(); 1963 return this.address; 1964 } 1965 1966 /** 1967 * @return Returns a reference to <code>this</code> for easy method chaining 1968 */ 1969 public Patient setAddress(List<Address> theAddress) { 1970 this.address = theAddress; 1971 return this; 1972 } 1973 1974 public boolean hasAddress() { 1975 if (this.address == null) 1976 return false; 1977 for (Address item : this.address) 1978 if (!item.isEmpty()) 1979 return true; 1980 return false; 1981 } 1982 1983 public Address addAddress() { //3 1984 Address t = new Address(); 1985 if (this.address == null) 1986 this.address = new ArrayList<Address>(); 1987 this.address.add(t); 1988 return t; 1989 } 1990 1991 public Patient addAddress(Address t) { //3 1992 if (t == null) 1993 return this; 1994 if (this.address == null) 1995 this.address = new ArrayList<Address>(); 1996 this.address.add(t); 1997 return this; 1998 } 1999 2000 /** 2001 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist 2002 */ 2003 public Address getAddressFirstRep() { 2004 if (getAddress().isEmpty()) { 2005 addAddress(); 2006 } 2007 return getAddress().get(0); 2008 } 2009 2010 /** 2011 * @return {@link #maritalStatus} (This field contains a patient's most recent marital (civil) status.) 2012 */ 2013 public CodeableConcept getMaritalStatus() { 2014 if (this.maritalStatus == null) 2015 if (Configuration.errorOnAutoCreate()) 2016 throw new Error("Attempt to auto-create Patient.maritalStatus"); 2017 else if (Configuration.doAutoCreate()) 2018 this.maritalStatus = new CodeableConcept(); // cc 2019 return this.maritalStatus; 2020 } 2021 2022 public boolean hasMaritalStatus() { 2023 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #maritalStatus} (This field contains a patient's most recent marital (civil) status.) 2028 */ 2029 public Patient setMaritalStatus(CodeableConcept value) { 2030 this.maritalStatus = value; 2031 return this; 2032 } 2033 2034 /** 2035 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).) 2036 */ 2037 public Type getMultipleBirth() { 2038 return this.multipleBirth; 2039 } 2040 2041 /** 2042 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).) 2043 */ 2044 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 2045 if (this.multipleBirth == null) 2046 return null; 2047 if (!(this.multipleBirth instanceof BooleanType)) 2048 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.multipleBirth.getClass().getName()+" was encountered"); 2049 return (BooleanType) this.multipleBirth; 2050 } 2051 2052 public boolean hasMultipleBirthBooleanType() { 2053 return this != null && this.multipleBirth instanceof BooleanType; 2054 } 2055 2056 /** 2057 * @return {@link #multipleBirth} (Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).) 2058 */ 2059 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 2060 if (this.multipleBirth == null) 2061 return null; 2062 if (!(this.multipleBirth instanceof IntegerType)) 2063 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.multipleBirth.getClass().getName()+" was encountered"); 2064 return (IntegerType) this.multipleBirth; 2065 } 2066 2067 public boolean hasMultipleBirthIntegerType() { 2068 return this != null && this.multipleBirth instanceof IntegerType; 2069 } 2070 2071 public boolean hasMultipleBirth() { 2072 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 2073 } 2074 2075 /** 2076 * @param value {@link #multipleBirth} (Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).) 2077 */ 2078 public Patient setMultipleBirth(Type value) throws FHIRFormatError { 2079 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType)) 2080 throw new FHIRFormatError("Not the right type for Patient.multipleBirth[x]: "+value.fhirType()); 2081 this.multipleBirth = value; 2082 return this; 2083 } 2084 2085 /** 2086 * @return {@link #photo} (Image of the patient.) 2087 */ 2088 public List<Attachment> getPhoto() { 2089 if (this.photo == null) 2090 this.photo = new ArrayList<Attachment>(); 2091 return this.photo; 2092 } 2093 2094 /** 2095 * @return Returns a reference to <code>this</code> for easy method chaining 2096 */ 2097 public Patient setPhoto(List<Attachment> thePhoto) { 2098 this.photo = thePhoto; 2099 return this; 2100 } 2101 2102 public boolean hasPhoto() { 2103 if (this.photo == null) 2104 return false; 2105 for (Attachment item : this.photo) 2106 if (!item.isEmpty()) 2107 return true; 2108 return false; 2109 } 2110 2111 public Attachment addPhoto() { //3 2112 Attachment t = new Attachment(); 2113 if (this.photo == null) 2114 this.photo = new ArrayList<Attachment>(); 2115 this.photo.add(t); 2116 return t; 2117 } 2118 2119 public Patient addPhoto(Attachment t) { //3 2120 if (t == null) 2121 return this; 2122 if (this.photo == null) 2123 this.photo = new ArrayList<Attachment>(); 2124 this.photo.add(t); 2125 return this; 2126 } 2127 2128 /** 2129 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist 2130 */ 2131 public Attachment getPhotoFirstRep() { 2132 if (getPhoto().isEmpty()) { 2133 addPhoto(); 2134 } 2135 return getPhoto().get(0); 2136 } 2137 2138 /** 2139 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) for the patient.) 2140 */ 2141 public List<ContactComponent> getContact() { 2142 if (this.contact == null) 2143 this.contact = new ArrayList<ContactComponent>(); 2144 return this.contact; 2145 } 2146 2147 /** 2148 * @return Returns a reference to <code>this</code> for easy method chaining 2149 */ 2150 public Patient setContact(List<ContactComponent> theContact) { 2151 this.contact = theContact; 2152 return this; 2153 } 2154 2155 public boolean hasContact() { 2156 if (this.contact == null) 2157 return false; 2158 for (ContactComponent item : this.contact) 2159 if (!item.isEmpty()) 2160 return true; 2161 return false; 2162 } 2163 2164 public ContactComponent addContact() { //3 2165 ContactComponent t = new ContactComponent(); 2166 if (this.contact == null) 2167 this.contact = new ArrayList<ContactComponent>(); 2168 this.contact.add(t); 2169 return t; 2170 } 2171 2172 public Patient addContact(ContactComponent t) { //3 2173 if (t == null) 2174 return this; 2175 if (this.contact == null) 2176 this.contact = new ArrayList<ContactComponent>(); 2177 this.contact.add(t); 2178 return this; 2179 } 2180 2181 /** 2182 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2183 */ 2184 public ContactComponent getContactFirstRep() { 2185 if (getContact().isEmpty()) { 2186 addContact(); 2187 } 2188 return getContact().get(0); 2189 } 2190 2191 /** 2192 * @return {@link #animal} (This patient is known to be an animal.) 2193 */ 2194 public AnimalComponent getAnimal() { 2195 if (this.animal == null) 2196 if (Configuration.errorOnAutoCreate()) 2197 throw new Error("Attempt to auto-create Patient.animal"); 2198 else if (Configuration.doAutoCreate()) 2199 this.animal = new AnimalComponent(); // cc 2200 return this.animal; 2201 } 2202 2203 public boolean hasAnimal() { 2204 return this.animal != null && !this.animal.isEmpty(); 2205 } 2206 2207 /** 2208 * @param value {@link #animal} (This patient is known to be an animal.) 2209 */ 2210 public Patient setAnimal(AnimalComponent value) { 2211 this.animal = value; 2212 return this; 2213 } 2214 2215 /** 2216 * @return {@link #communication} (Languages which may be used to communicate with the patient about his or her health.) 2217 */ 2218 public List<PatientCommunicationComponent> getCommunication() { 2219 if (this.communication == null) 2220 this.communication = new ArrayList<PatientCommunicationComponent>(); 2221 return this.communication; 2222 } 2223 2224 /** 2225 * @return Returns a reference to <code>this</code> for easy method chaining 2226 */ 2227 public Patient setCommunication(List<PatientCommunicationComponent> theCommunication) { 2228 this.communication = theCommunication; 2229 return this; 2230 } 2231 2232 public boolean hasCommunication() { 2233 if (this.communication == null) 2234 return false; 2235 for (PatientCommunicationComponent item : this.communication) 2236 if (!item.isEmpty()) 2237 return true; 2238 return false; 2239 } 2240 2241 public PatientCommunicationComponent addCommunication() { //3 2242 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2243 if (this.communication == null) 2244 this.communication = new ArrayList<PatientCommunicationComponent>(); 2245 this.communication.add(t); 2246 return t; 2247 } 2248 2249 public Patient addCommunication(PatientCommunicationComponent t) { //3 2250 if (t == null) 2251 return this; 2252 if (this.communication == null) 2253 this.communication = new ArrayList<PatientCommunicationComponent>(); 2254 this.communication.add(t); 2255 return this; 2256 } 2257 2258 /** 2259 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist 2260 */ 2261 public PatientCommunicationComponent getCommunicationFirstRep() { 2262 if (getCommunication().isEmpty()) { 2263 addCommunication(); 2264 } 2265 return getCommunication().get(0); 2266 } 2267 2268 /** 2269 * @return {@link #generalPractitioner} (Patient's nominated care provider.) 2270 */ 2271 public List<Reference> getGeneralPractitioner() { 2272 if (this.generalPractitioner == null) 2273 this.generalPractitioner = new ArrayList<Reference>(); 2274 return this.generalPractitioner; 2275 } 2276 2277 /** 2278 * @return Returns a reference to <code>this</code> for easy method chaining 2279 */ 2280 public Patient setGeneralPractitioner(List<Reference> theGeneralPractitioner) { 2281 this.generalPractitioner = theGeneralPractitioner; 2282 return this; 2283 } 2284 2285 public boolean hasGeneralPractitioner() { 2286 if (this.generalPractitioner == null) 2287 return false; 2288 for (Reference item : this.generalPractitioner) 2289 if (!item.isEmpty()) 2290 return true; 2291 return false; 2292 } 2293 2294 public Reference addGeneralPractitioner() { //3 2295 Reference t = new Reference(); 2296 if (this.generalPractitioner == null) 2297 this.generalPractitioner = new ArrayList<Reference>(); 2298 this.generalPractitioner.add(t); 2299 return t; 2300 } 2301 2302 public Patient addGeneralPractitioner(Reference t) { //3 2303 if (t == null) 2304 return this; 2305 if (this.generalPractitioner == null) 2306 this.generalPractitioner = new ArrayList<Reference>(); 2307 this.generalPractitioner.add(t); 2308 return this; 2309 } 2310 2311 /** 2312 * @return The first repetition of repeating field {@link #generalPractitioner}, creating it if it does not already exist 2313 */ 2314 public Reference getGeneralPractitionerFirstRep() { 2315 if (getGeneralPractitioner().isEmpty()) { 2316 addGeneralPractitioner(); 2317 } 2318 return getGeneralPractitioner().get(0); 2319 } 2320 2321 /** 2322 * @deprecated Use Reference#setResource(IBaseResource) instead 2323 */ 2324 @Deprecated 2325 public List<Resource> getGeneralPractitionerTarget() { 2326 if (this.generalPractitionerTarget == null) 2327 this.generalPractitionerTarget = new ArrayList<Resource>(); 2328 return this.generalPractitionerTarget; 2329 } 2330 2331 /** 2332 * @return {@link #managingOrganization} (Organization that is the custodian of the patient record.) 2333 */ 2334 public Reference getManagingOrganization() { 2335 if (this.managingOrganization == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2338 else if (Configuration.doAutoCreate()) 2339 this.managingOrganization = new Reference(); // cc 2340 return this.managingOrganization; 2341 } 2342 2343 public boolean hasManagingOrganization() { 2344 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2345 } 2346 2347 /** 2348 * @param value {@link #managingOrganization} (Organization that is the custodian of the patient record.) 2349 */ 2350 public Patient setManagingOrganization(Reference value) { 2351 this.managingOrganization = value; 2352 return this; 2353 } 2354 2355 /** 2356 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Organization that is the custodian of the patient record.) 2357 */ 2358 public Organization getManagingOrganizationTarget() { 2359 if (this.managingOrganizationTarget == null) 2360 if (Configuration.errorOnAutoCreate()) 2361 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2362 else if (Configuration.doAutoCreate()) 2363 this.managingOrganizationTarget = new Organization(); // aa 2364 return this.managingOrganizationTarget; 2365 } 2366 2367 /** 2368 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Organization that is the custodian of the patient record.) 2369 */ 2370 public Patient setManagingOrganizationTarget(Organization value) { 2371 this.managingOrganizationTarget = value; 2372 return this; 2373 } 2374 2375 /** 2376 * @return {@link #link} (Link to another patient resource that concerns the same actual patient.) 2377 */ 2378 public List<PatientLinkComponent> getLink() { 2379 if (this.link == null) 2380 this.link = new ArrayList<PatientLinkComponent>(); 2381 return this.link; 2382 } 2383 2384 /** 2385 * @return Returns a reference to <code>this</code> for easy method chaining 2386 */ 2387 public Patient setLink(List<PatientLinkComponent> theLink) { 2388 this.link = theLink; 2389 return this; 2390 } 2391 2392 public boolean hasLink() { 2393 if (this.link == null) 2394 return false; 2395 for (PatientLinkComponent item : this.link) 2396 if (!item.isEmpty()) 2397 return true; 2398 return false; 2399 } 2400 2401 public PatientLinkComponent addLink() { //3 2402 PatientLinkComponent t = new PatientLinkComponent(); 2403 if (this.link == null) 2404 this.link = new ArrayList<PatientLinkComponent>(); 2405 this.link.add(t); 2406 return t; 2407 } 2408 2409 public Patient addLink(PatientLinkComponent t) { //3 2410 if (t == null) 2411 return this; 2412 if (this.link == null) 2413 this.link = new ArrayList<PatientLinkComponent>(); 2414 this.link.add(t); 2415 return this; 2416 } 2417 2418 /** 2419 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 2420 */ 2421 public PatientLinkComponent getLinkFirstRep() { 2422 if (getLink().isEmpty()) { 2423 addLink(); 2424 } 2425 return getLink().get(0); 2426 } 2427 2428 protected void listChildren(List<Property> children) { 2429 super.listChildren(children); 2430 children.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2431 children.add(new Property("active", "boolean", "Whether this patient record is in active use.", 0, 1, active)); 2432 children.add(new Property("name", "HumanName", "A name associated with the individual.", 0, java.lang.Integer.MAX_VALUE, name)); 2433 children.add(new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2434 children.add(new Property("gender", "code", "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 0, 1, gender)); 2435 children.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate)); 2436 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased)); 2437 children.add(new Property("address", "Address", "Addresses for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2438 children.add(new Property("maritalStatus", "CodeableConcept", "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus)); 2439 children.add(new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).", 0, 1, multipleBirth)); 2440 children.add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2441 children.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact)); 2442 children.add(new Property("animal", "", "This patient is known to be an animal.", 0, 1, animal)); 2443 children.add(new Property("communication", "", "Languages which may be used to communicate with the patient about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication)); 2444 children.add(new Property("generalPractitioner", "Reference(Organization|Practitioner)", "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner)); 2445 children.add(new Property("managingOrganization", "Reference(Organization)", "Organization that is the custodian of the patient record.", 0, 1, managingOrganization)); 2446 children.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 0, java.lang.Integer.MAX_VALUE, link)); 2447 } 2448 2449 @Override 2450 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2451 switch (_hash) { 2452 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for this patient.", 0, java.lang.Integer.MAX_VALUE, identifier); 2453 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this patient record is in active use.", 0, 1, active); 2454 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the individual.", 0, java.lang.Integer.MAX_VALUE, name); 2455 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, java.lang.Integer.MAX_VALUE, telecom); 2456 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 0, 1, gender); 2457 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate); 2458 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2459 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2460 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2461 case -1971804369: /*deceasedDateTime*/ return new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 1, deceased); 2462 case -1147692044: /*address*/ return new Property("address", "Address", "Addresses for the individual.", 0, java.lang.Integer.MAX_VALUE, address); 2463 case 1756919302: /*maritalStatus*/ return new Property("maritalStatus", "CodeableConcept", "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus); 2464 case -1764672111: /*multipleBirth[x]*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2465 case -677369713: /*multipleBirth*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2466 case -247534439: /*multipleBirthBoolean*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2467 case 1645805999: /*multipleBirthInteger*/ return new Property("multipleBirth[x]", "boolean|integer", "Indicates whether the patient is part of a multiple (bool) or indicates the actual birth order (integer).", 0, 1, multipleBirth); 2468 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo); 2469 case 951526432: /*contact*/ return new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact); 2470 case -1413116420: /*animal*/ return new Property("animal", "", "This patient is known to be an animal.", 0, 1, animal); 2471 case -1035284522: /*communication*/ return new Property("communication", "", "Languages which may be used to communicate with the patient about his or her health.", 0, java.lang.Integer.MAX_VALUE, communication); 2472 case 1488292898: /*generalPractitioner*/ return new Property("generalPractitioner", "Reference(Organization|Practitioner)", "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner); 2473 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "Organization that is the custodian of the patient record.", 0, 1, managingOrganization); 2474 case 3321850: /*link*/ return new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 0, java.lang.Integer.MAX_VALUE, link); 2475 default: return super.getNamedProperty(_hash, _name, _checkValid); 2476 } 2477 2478 } 2479 2480 @Override 2481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2482 switch (hash) { 2483 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2484 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 2485 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 2486 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2487 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 2488 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 2489 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // Type 2490 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 2491 case 1756919302: /*maritalStatus*/ return this.maritalStatus == null ? new Base[0] : new Base[] {this.maritalStatus}; // CodeableConcept 2492 case -677369713: /*multipleBirth*/ return this.multipleBirth == null ? new Base[0] : new Base[] {this.multipleBirth}; // Type 2493 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 2494 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactComponent 2495 case -1413116420: /*animal*/ return this.animal == null ? new Base[0] : new Base[] {this.animal}; // AnimalComponent 2496 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // PatientCommunicationComponent 2497 case 1488292898: /*generalPractitioner*/ return this.generalPractitioner == null ? new Base[0] : this.generalPractitioner.toArray(new Base[this.generalPractitioner.size()]); // Reference 2498 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 2499 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PatientLinkComponent 2500 default: return super.getProperty(hash, name, checkValid); 2501 } 2502 2503 } 2504 2505 @Override 2506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2507 switch (hash) { 2508 case -1618432855: // identifier 2509 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2510 return value; 2511 case -1422950650: // active 2512 this.active = castToBoolean(value); // BooleanType 2513 return value; 2514 case 3373707: // name 2515 this.getName().add(castToHumanName(value)); // HumanName 2516 return value; 2517 case -1429363305: // telecom 2518 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2519 return value; 2520 case -1249512767: // gender 2521 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2522 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2523 return value; 2524 case -1210031859: // birthDate 2525 this.birthDate = castToDate(value); // DateType 2526 return value; 2527 case 561497972: // deceased 2528 this.deceased = castToType(value); // Type 2529 return value; 2530 case -1147692044: // address 2531 this.getAddress().add(castToAddress(value)); // Address 2532 return value; 2533 case 1756919302: // maritalStatus 2534 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2535 return value; 2536 case -677369713: // multipleBirth 2537 this.multipleBirth = castToType(value); // Type 2538 return value; 2539 case 106642994: // photo 2540 this.getPhoto().add(castToAttachment(value)); // Attachment 2541 return value; 2542 case 951526432: // contact 2543 this.getContact().add((ContactComponent) value); // ContactComponent 2544 return value; 2545 case -1413116420: // animal 2546 this.animal = (AnimalComponent) value; // AnimalComponent 2547 return value; 2548 case -1035284522: // communication 2549 this.getCommunication().add((PatientCommunicationComponent) value); // PatientCommunicationComponent 2550 return value; 2551 case 1488292898: // generalPractitioner 2552 this.getGeneralPractitioner().add(castToReference(value)); // Reference 2553 return value; 2554 case -2058947787: // managingOrganization 2555 this.managingOrganization = castToReference(value); // Reference 2556 return value; 2557 case 3321850: // link 2558 this.getLink().add((PatientLinkComponent) value); // PatientLinkComponent 2559 return value; 2560 default: return super.setProperty(hash, name, value); 2561 } 2562 2563 } 2564 2565 @Override 2566 public Base setProperty(String name, Base value) throws FHIRException { 2567 if (name.equals("identifier")) { 2568 this.getIdentifier().add(castToIdentifier(value)); 2569 } else if (name.equals("active")) { 2570 this.active = castToBoolean(value); // BooleanType 2571 } else if (name.equals("name")) { 2572 this.getName().add(castToHumanName(value)); 2573 } else if (name.equals("telecom")) { 2574 this.getTelecom().add(castToContactPoint(value)); 2575 } else if (name.equals("gender")) { 2576 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2577 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2578 } else if (name.equals("birthDate")) { 2579 this.birthDate = castToDate(value); // DateType 2580 } else if (name.equals("deceased[x]")) { 2581 this.deceased = castToType(value); // Type 2582 } else if (name.equals("address")) { 2583 this.getAddress().add(castToAddress(value)); 2584 } else if (name.equals("maritalStatus")) { 2585 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2586 } else if (name.equals("multipleBirth[x]")) { 2587 this.multipleBirth = castToType(value); // Type 2588 } else if (name.equals("photo")) { 2589 this.getPhoto().add(castToAttachment(value)); 2590 } else if (name.equals("contact")) { 2591 this.getContact().add((ContactComponent) value); 2592 } else if (name.equals("animal")) { 2593 this.animal = (AnimalComponent) value; // AnimalComponent 2594 } else if (name.equals("communication")) { 2595 this.getCommunication().add((PatientCommunicationComponent) value); 2596 } else if (name.equals("generalPractitioner")) { 2597 this.getGeneralPractitioner().add(castToReference(value)); 2598 } else if (name.equals("managingOrganization")) { 2599 this.managingOrganization = castToReference(value); // Reference 2600 } else if (name.equals("link")) { 2601 this.getLink().add((PatientLinkComponent) value); 2602 } else 2603 return super.setProperty(name, value); 2604 return value; 2605 } 2606 2607 @Override 2608 public Base makeProperty(int hash, String name) throws FHIRException { 2609 switch (hash) { 2610 case -1618432855: return addIdentifier(); 2611 case -1422950650: return getActiveElement(); 2612 case 3373707: return addName(); 2613 case -1429363305: return addTelecom(); 2614 case -1249512767: return getGenderElement(); 2615 case -1210031859: return getBirthDateElement(); 2616 case -1311442804: return getDeceased(); 2617 case 561497972: return getDeceased(); 2618 case -1147692044: return addAddress(); 2619 case 1756919302: return getMaritalStatus(); 2620 case -1764672111: return getMultipleBirth(); 2621 case -677369713: return getMultipleBirth(); 2622 case 106642994: return addPhoto(); 2623 case 951526432: return addContact(); 2624 case -1413116420: return getAnimal(); 2625 case -1035284522: return addCommunication(); 2626 case 1488292898: return addGeneralPractitioner(); 2627 case -2058947787: return getManagingOrganization(); 2628 case 3321850: return addLink(); 2629 default: return super.makeProperty(hash, name); 2630 } 2631 2632 } 2633 2634 @Override 2635 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2636 switch (hash) { 2637 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2638 case -1422950650: /*active*/ return new String[] {"boolean"}; 2639 case 3373707: /*name*/ return new String[] {"HumanName"}; 2640 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 2641 case -1249512767: /*gender*/ return new String[] {"code"}; 2642 case -1210031859: /*birthDate*/ return new String[] {"date"}; 2643 case 561497972: /*deceased*/ return new String[] {"boolean", "dateTime"}; 2644 case -1147692044: /*address*/ return new String[] {"Address"}; 2645 case 1756919302: /*maritalStatus*/ return new String[] {"CodeableConcept"}; 2646 case -677369713: /*multipleBirth*/ return new String[] {"boolean", "integer"}; 2647 case 106642994: /*photo*/ return new String[] {"Attachment"}; 2648 case 951526432: /*contact*/ return new String[] {}; 2649 case -1413116420: /*animal*/ return new String[] {}; 2650 case -1035284522: /*communication*/ return new String[] {}; 2651 case 1488292898: /*generalPractitioner*/ return new String[] {"Reference"}; 2652 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 2653 case 3321850: /*link*/ return new String[] {}; 2654 default: return super.getTypesForProperty(hash, name); 2655 } 2656 2657 } 2658 2659 @Override 2660 public Base addChild(String name) throws FHIRException { 2661 if (name.equals("identifier")) { 2662 return addIdentifier(); 2663 } 2664 else if (name.equals("active")) { 2665 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2666 } 2667 else if (name.equals("name")) { 2668 return addName(); 2669 } 2670 else if (name.equals("telecom")) { 2671 return addTelecom(); 2672 } 2673 else if (name.equals("gender")) { 2674 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2675 } 2676 else if (name.equals("birthDate")) { 2677 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2678 } 2679 else if (name.equals("deceasedBoolean")) { 2680 this.deceased = new BooleanType(); 2681 return this.deceased; 2682 } 2683 else if (name.equals("deceasedDateTime")) { 2684 this.deceased = new DateTimeType(); 2685 return this.deceased; 2686 } 2687 else if (name.equals("address")) { 2688 return addAddress(); 2689 } 2690 else if (name.equals("maritalStatus")) { 2691 this.maritalStatus = new CodeableConcept(); 2692 return this.maritalStatus; 2693 } 2694 else if (name.equals("multipleBirthBoolean")) { 2695 this.multipleBirth = new BooleanType(); 2696 return this.multipleBirth; 2697 } 2698 else if (name.equals("multipleBirthInteger")) { 2699 this.multipleBirth = new IntegerType(); 2700 return this.multipleBirth; 2701 } 2702 else if (name.equals("photo")) { 2703 return addPhoto(); 2704 } 2705 else if (name.equals("contact")) { 2706 return addContact(); 2707 } 2708 else if (name.equals("animal")) { 2709 this.animal = new AnimalComponent(); 2710 return this.animal; 2711 } 2712 else if (name.equals("communication")) { 2713 return addCommunication(); 2714 } 2715 else if (name.equals("generalPractitioner")) { 2716 return addGeneralPractitioner(); 2717 } 2718 else if (name.equals("managingOrganization")) { 2719 this.managingOrganization = new Reference(); 2720 return this.managingOrganization; 2721 } 2722 else if (name.equals("link")) { 2723 return addLink(); 2724 } 2725 else 2726 return super.addChild(name); 2727 } 2728 2729 public String fhirType() { 2730 return "Patient"; 2731 2732 } 2733 2734 public Patient copy() { 2735 Patient dst = new Patient(); 2736 copyValues(dst); 2737 if (identifier != null) { 2738 dst.identifier = new ArrayList<Identifier>(); 2739 for (Identifier i : identifier) 2740 dst.identifier.add(i.copy()); 2741 }; 2742 dst.active = active == null ? null : active.copy(); 2743 if (name != null) { 2744 dst.name = new ArrayList<HumanName>(); 2745 for (HumanName i : name) 2746 dst.name.add(i.copy()); 2747 }; 2748 if (telecom != null) { 2749 dst.telecom = new ArrayList<ContactPoint>(); 2750 for (ContactPoint i : telecom) 2751 dst.telecom.add(i.copy()); 2752 }; 2753 dst.gender = gender == null ? null : gender.copy(); 2754 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2755 dst.deceased = deceased == null ? null : deceased.copy(); 2756 if (address != null) { 2757 dst.address = new ArrayList<Address>(); 2758 for (Address i : address) 2759 dst.address.add(i.copy()); 2760 }; 2761 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2762 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2763 if (photo != null) { 2764 dst.photo = new ArrayList<Attachment>(); 2765 for (Attachment i : photo) 2766 dst.photo.add(i.copy()); 2767 }; 2768 if (contact != null) { 2769 dst.contact = new ArrayList<ContactComponent>(); 2770 for (ContactComponent i : contact) 2771 dst.contact.add(i.copy()); 2772 }; 2773 dst.animal = animal == null ? null : animal.copy(); 2774 if (communication != null) { 2775 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2776 for (PatientCommunicationComponent i : communication) 2777 dst.communication.add(i.copy()); 2778 }; 2779 if (generalPractitioner != null) { 2780 dst.generalPractitioner = new ArrayList<Reference>(); 2781 for (Reference i : generalPractitioner) 2782 dst.generalPractitioner.add(i.copy()); 2783 }; 2784 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2785 if (link != null) { 2786 dst.link = new ArrayList<PatientLinkComponent>(); 2787 for (PatientLinkComponent i : link) 2788 dst.link.add(i.copy()); 2789 }; 2790 return dst; 2791 } 2792 2793 protected Patient typedCopy() { 2794 return copy(); 2795 } 2796 2797 @Override 2798 public boolean equalsDeep(Base other_) { 2799 if (!super.equalsDeep(other_)) 2800 return false; 2801 if (!(other_ instanceof Patient)) 2802 return false; 2803 Patient o = (Patient) other_; 2804 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(name, o.name, true) 2805 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 2806 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) && compareDeep(maritalStatus, o.maritalStatus, true) 2807 && compareDeep(multipleBirth, o.multipleBirth, true) && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 2808 && compareDeep(animal, o.animal, true) && compareDeep(communication, o.communication, true) && compareDeep(generalPractitioner, o.generalPractitioner, true) 2809 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true) 2810 ; 2811 } 2812 2813 @Override 2814 public boolean equalsShallow(Base other_) { 2815 if (!super.equalsShallow(other_)) 2816 return false; 2817 if (!(other_ instanceof Patient)) 2818 return false; 2819 Patient o = (Patient) other_; 2820 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 2821 ; 2822 } 2823 2824 public boolean isEmpty() { 2825 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name 2826 , telecom, gender, birthDate, deceased, address, maritalStatus, multipleBirth 2827 , photo, contact, animal, communication, generalPractitioner, managingOrganization 2828 , link); 2829 } 2830 2831 @Override 2832 public ResourceType getResourceType() { 2833 return ResourceType.Patient; 2834 } 2835 2836 /** 2837 * Search parameter: <b>birthdate</b> 2838 * <p> 2839 * Description: <b>The patient's date of birth</b><br> 2840 * Type: <b>date</b><br> 2841 * Path: <b>Patient.birthDate</b><br> 2842 * </p> 2843 */ 2844 @SearchParamDefinition(name="birthdate", path="Patient.birthDate", description="The patient's date of birth", type="date" ) 2845 public static final String SP_BIRTHDATE = "birthdate"; 2846 /** 2847 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 2848 * <p> 2849 * Description: <b>The patient's date of birth</b><br> 2850 * Type: <b>date</b><br> 2851 * Path: <b>Patient.birthDate</b><br> 2852 * </p> 2853 */ 2854 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 2855 2856 /** 2857 * Search parameter: <b>deceased</b> 2858 * <p> 2859 * Description: <b>This patient has been marked as deceased, or as a death date entered</b><br> 2860 * Type: <b>token</b><br> 2861 * Path: <b>Patient.deceased[x]</b><br> 2862 * </p> 2863 */ 2864 @SearchParamDefinition(name="deceased", path="Patient.deceased.exists()", description="This patient has been marked as deceased, or as a death date entered", type="token" ) 2865 public static final String SP_DECEASED = "deceased"; 2866 /** 2867 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 2868 * <p> 2869 * Description: <b>This patient has been marked as deceased, or as a death date entered</b><br> 2870 * Type: <b>token</b><br> 2871 * Path: <b>Patient.deceased[x]</b><br> 2872 * </p> 2873 */ 2874 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DECEASED); 2875 2876 /** 2877 * Search parameter: <b>address-state</b> 2878 * <p> 2879 * Description: <b>A state specified in an address</b><br> 2880 * Type: <b>string</b><br> 2881 * Path: <b>Patient.address.state</b><br> 2882 * </p> 2883 */ 2884 @SearchParamDefinition(name="address-state", path="Patient.address.state", description="A state specified in an address", type="string" ) 2885 public static final String SP_ADDRESS_STATE = "address-state"; 2886 /** 2887 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 2888 * <p> 2889 * Description: <b>A state specified in an address</b><br> 2890 * Type: <b>string</b><br> 2891 * Path: <b>Patient.address.state</b><br> 2892 * </p> 2893 */ 2894 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 2895 2896 /** 2897 * Search parameter: <b>gender</b> 2898 * <p> 2899 * Description: <b>Gender of the patient</b><br> 2900 * Type: <b>token</b><br> 2901 * Path: <b>Patient.gender</b><br> 2902 * </p> 2903 */ 2904 @SearchParamDefinition(name="gender", path="Patient.gender", description="Gender of the patient", type="token" ) 2905 public static final String SP_GENDER = "gender"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 2908 * <p> 2909 * Description: <b>Gender of the patient</b><br> 2910 * Type: <b>token</b><br> 2911 * Path: <b>Patient.gender</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 2915 2916 /** 2917 * Search parameter: <b>animal-species</b> 2918 * <p> 2919 * Description: <b>The species for animal patients</b><br> 2920 * Type: <b>token</b><br> 2921 * Path: <b>Patient.animal.species</b><br> 2922 * </p> 2923 */ 2924 @SearchParamDefinition(name="animal-species", path="Patient.animal.species", description="The species for animal patients", type="token" ) 2925 public static final String SP_ANIMAL_SPECIES = "animal-species"; 2926 /** 2927 * <b>Fluent Client</b> search parameter constant for <b>animal-species</b> 2928 * <p> 2929 * Description: <b>The species for animal patients</b><br> 2930 * Type: <b>token</b><br> 2931 * Path: <b>Patient.animal.species</b><br> 2932 * </p> 2933 */ 2934 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ANIMAL_SPECIES = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ANIMAL_SPECIES); 2935 2936 /** 2937 * Search parameter: <b>link</b> 2938 * <p> 2939 * Description: <b>All patients linked to the given patient</b><br> 2940 * Type: <b>reference</b><br> 2941 * Path: <b>Patient.link.other</b><br> 2942 * </p> 2943 */ 2944 @SearchParamDefinition(name="link", path="Patient.link.other", description="All patients linked to the given patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, RelatedPerson.class } ) 2945 public static final String SP_LINK = "link"; 2946 /** 2947 * <b>Fluent Client</b> search parameter constant for <b>link</b> 2948 * <p> 2949 * Description: <b>All patients linked to the given patient</b><br> 2950 * Type: <b>reference</b><br> 2951 * Path: <b>Patient.link.other</b><br> 2952 * </p> 2953 */ 2954 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LINK); 2955 2956/** 2957 * Constant for fluent queries to be used to add include statements. Specifies 2958 * the path value of "<b>Patient:link</b>". 2959 */ 2960 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Patient:link").toLocked(); 2961 2962 /** 2963 * Search parameter: <b>language</b> 2964 * <p> 2965 * Description: <b>Language code (irrespective of use value)</b><br> 2966 * Type: <b>token</b><br> 2967 * Path: <b>Patient.communication.language</b><br> 2968 * </p> 2969 */ 2970 @SearchParamDefinition(name="language", path="Patient.communication.language", description="Language code (irrespective of use value)", type="token" ) 2971 public static final String SP_LANGUAGE = "language"; 2972 /** 2973 * <b>Fluent Client</b> search parameter constant for <b>language</b> 2974 * <p> 2975 * Description: <b>Language code (irrespective of use value)</b><br> 2976 * Type: <b>token</b><br> 2977 * Path: <b>Patient.communication.language</b><br> 2978 * </p> 2979 */ 2980 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 2981 2982 /** 2983 * Search parameter: <b>animal-breed</b> 2984 * <p> 2985 * Description: <b>The breed for animal patients</b><br> 2986 * Type: <b>token</b><br> 2987 * Path: <b>Patient.animal.breed</b><br> 2988 * </p> 2989 */ 2990 @SearchParamDefinition(name="animal-breed", path="Patient.animal.breed", description="The breed for animal patients", type="token" ) 2991 public static final String SP_ANIMAL_BREED = "animal-breed"; 2992 /** 2993 * <b>Fluent Client</b> search parameter constant for <b>animal-breed</b> 2994 * <p> 2995 * Description: <b>The breed for animal patients</b><br> 2996 * Type: <b>token</b><br> 2997 * Path: <b>Patient.animal.breed</b><br> 2998 * </p> 2999 */ 3000 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ANIMAL_BREED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ANIMAL_BREED); 3001 3002 /** 3003 * Search parameter: <b>address-country</b> 3004 * <p> 3005 * Description: <b>A country specified in an address</b><br> 3006 * Type: <b>string</b><br> 3007 * Path: <b>Patient.address.country</b><br> 3008 * </p> 3009 */ 3010 @SearchParamDefinition(name="address-country", path="Patient.address.country", description="A country specified in an address", type="string" ) 3011 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3012 /** 3013 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3014 * <p> 3015 * Description: <b>A country specified in an address</b><br> 3016 * Type: <b>string</b><br> 3017 * Path: <b>Patient.address.country</b><br> 3018 * </p> 3019 */ 3020 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 3021 3022 /** 3023 * Search parameter: <b>death-date</b> 3024 * <p> 3025 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 3026 * Type: <b>date</b><br> 3027 * Path: <b>Patient.deceasedDateTime</b><br> 3028 * </p> 3029 */ 3030 @SearchParamDefinition(name="death-date", path="Patient.deceased.as(DateTime)", description="The date of death has been provided and satisfies this search value", type="date" ) 3031 public static final String SP_DEATH_DATE = "death-date"; 3032 /** 3033 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 3034 * <p> 3035 * Description: <b>The date of death has been provided and satisfies this search value</b><br> 3036 * Type: <b>date</b><br> 3037 * Path: <b>Patient.deceasedDateTime</b><br> 3038 * </p> 3039 */ 3040 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DEATH_DATE); 3041 3042 /** 3043 * Search parameter: <b>phonetic</b> 3044 * <p> 3045 * Description: <b>A portion of either family or given name using some kind of phonetic matching algorithm</b><br> 3046 * Type: <b>string</b><br> 3047 * Path: <b>Patient.name</b><br> 3048 * </p> 3049 */ 3050 @SearchParamDefinition(name="phonetic", path="Patient.name", description="A portion of either family or given name using some kind of phonetic matching algorithm", type="string" ) 3051 public static final String SP_PHONETIC = "phonetic"; 3052 /** 3053 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 3054 * <p> 3055 * Description: <b>A portion of either family or given name using some kind of phonetic matching algorithm</b><br> 3056 * Type: <b>string</b><br> 3057 * Path: <b>Patient.name</b><br> 3058 * </p> 3059 */ 3060 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 3061 3062 /** 3063 * Search parameter: <b>telecom</b> 3064 * <p> 3065 * Description: <b>The value in any kind of telecom details of the patient</b><br> 3066 * Type: <b>token</b><br> 3067 * Path: <b>Patient.telecom</b><br> 3068 * </p> 3069 */ 3070 @SearchParamDefinition(name="telecom", path="Patient.telecom", description="The value in any kind of telecom details of the patient", type="token" ) 3071 public static final String SP_TELECOM = "telecom"; 3072 /** 3073 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 3074 * <p> 3075 * Description: <b>The value in any kind of telecom details of the patient</b><br> 3076 * Type: <b>token</b><br> 3077 * Path: <b>Patient.telecom</b><br> 3078 * </p> 3079 */ 3080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 3081 3082 /** 3083 * Search parameter: <b>address-city</b> 3084 * <p> 3085 * Description: <b>A city specified in an address</b><br> 3086 * Type: <b>string</b><br> 3087 * Path: <b>Patient.address.city</b><br> 3088 * </p> 3089 */ 3090 @SearchParamDefinition(name="address-city", path="Patient.address.city", description="A city specified in an address", type="string" ) 3091 public static final String SP_ADDRESS_CITY = "address-city"; 3092 /** 3093 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3094 * <p> 3095 * Description: <b>A city specified in an address</b><br> 3096 * Type: <b>string</b><br> 3097 * Path: <b>Patient.address.city</b><br> 3098 * </p> 3099 */ 3100 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 3101 3102 /** 3103 * Search parameter: <b>email</b> 3104 * <p> 3105 * Description: <b>A value in an email contact</b><br> 3106 * Type: <b>token</b><br> 3107 * Path: <b>Patient.telecom(system=email)</b><br> 3108 * </p> 3109 */ 3110 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email')", description="A value in an email contact", type="token" ) 3111 public static final String SP_EMAIL = "email"; 3112 /** 3113 * <b>Fluent Client</b> search parameter constant for <b>email</b> 3114 * <p> 3115 * Description: <b>A value in an email contact</b><br> 3116 * Type: <b>token</b><br> 3117 * Path: <b>Patient.telecom(system=email)</b><br> 3118 * </p> 3119 */ 3120 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 3121 3122 /** 3123 * Search parameter: <b>identifier</b> 3124 * <p> 3125 * Description: <b>A patient identifier</b><br> 3126 * Type: <b>token</b><br> 3127 * Path: <b>Patient.identifier</b><br> 3128 * </p> 3129 */ 3130 @SearchParamDefinition(name="identifier", path="Patient.identifier", description="A patient identifier", type="token" ) 3131 public static final String SP_IDENTIFIER = "identifier"; 3132 /** 3133 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3134 * <p> 3135 * Description: <b>A patient identifier</b><br> 3136 * Type: <b>token</b><br> 3137 * Path: <b>Patient.identifier</b><br> 3138 * </p> 3139 */ 3140 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3141 3142 /** 3143 * Search parameter: <b>given</b> 3144 * <p> 3145 * Description: <b>A portion of the given name of the patient</b><br> 3146 * Type: <b>string</b><br> 3147 * Path: <b>Patient.name.given</b><br> 3148 * </p> 3149 */ 3150 @SearchParamDefinition(name="given", path="Patient.name.given", description="A portion of the given name of the patient", type="string" ) 3151 public static final String SP_GIVEN = "given"; 3152 /** 3153 * <b>Fluent Client</b> search parameter constant for <b>given</b> 3154 * <p> 3155 * Description: <b>A portion of the given name of the patient</b><br> 3156 * Type: <b>string</b><br> 3157 * Path: <b>Patient.name.given</b><br> 3158 * </p> 3159 */ 3160 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 3161 3162 /** 3163 * Search parameter: <b>address</b> 3164 * <p> 3165 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 3166 * Type: <b>string</b><br> 3167 * Path: <b>Patient.address</b><br> 3168 * </p> 3169 */ 3170 @SearchParamDefinition(name="address", path="Patient.address", description="A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text", type="string" ) 3171 public static final String SP_ADDRESS = "address"; 3172 /** 3173 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3174 * <p> 3175 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 3176 * Type: <b>string</b><br> 3177 * Path: <b>Patient.address</b><br> 3178 * </p> 3179 */ 3180 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 3181 3182 /** 3183 * Search parameter: <b>general-practitioner</b> 3184 * <p> 3185 * Description: <b>Patient's nominated general practitioner, not the organization that manages the record</b><br> 3186 * Type: <b>reference</b><br> 3187 * Path: <b>Patient.generalPractitioner</b><br> 3188 * </p> 3189 */ 3190 @SearchParamDefinition(name="general-practitioner", path="Patient.generalPractitioner", description="Patient's nominated general practitioner, not the organization that manages the record", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 3191 public static final String SP_GENERAL_PRACTITIONER = "general-practitioner"; 3192 /** 3193 * <b>Fluent Client</b> search parameter constant for <b>general-practitioner</b> 3194 * <p> 3195 * Description: <b>Patient's nominated general practitioner, not the organization that manages the record</b><br> 3196 * Type: <b>reference</b><br> 3197 * Path: <b>Patient.generalPractitioner</b><br> 3198 * </p> 3199 */ 3200 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GENERAL_PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GENERAL_PRACTITIONER); 3201 3202/** 3203 * Constant for fluent queries to be used to add include statements. Specifies 3204 * the path value of "<b>Patient:general-practitioner</b>". 3205 */ 3206 public static final ca.uhn.fhir.model.api.Include INCLUDE_GENERAL_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Patient:general-practitioner").toLocked(); 3207 3208 /** 3209 * Search parameter: <b>active</b> 3210 * <p> 3211 * Description: <b>Whether the patient record is active</b><br> 3212 * Type: <b>token</b><br> 3213 * Path: <b>Patient.active</b><br> 3214 * </p> 3215 */ 3216 @SearchParamDefinition(name="active", path="Patient.active", description="Whether the patient record is active", type="token" ) 3217 public static final String SP_ACTIVE = "active"; 3218 /** 3219 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3220 * <p> 3221 * Description: <b>Whether the patient record is active</b><br> 3222 * Type: <b>token</b><br> 3223 * Path: <b>Patient.active</b><br> 3224 * </p> 3225 */ 3226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 3227 3228 /** 3229 * Search parameter: <b>address-postalcode</b> 3230 * <p> 3231 * Description: <b>A postalCode specified in an address</b><br> 3232 * Type: <b>string</b><br> 3233 * Path: <b>Patient.address.postalCode</b><br> 3234 * </p> 3235 */ 3236 @SearchParamDefinition(name="address-postalcode", path="Patient.address.postalCode", description="A postalCode specified in an address", type="string" ) 3237 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3238 /** 3239 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3240 * <p> 3241 * Description: <b>A postalCode specified in an address</b><br> 3242 * Type: <b>string</b><br> 3243 * Path: <b>Patient.address.postalCode</b><br> 3244 * </p> 3245 */ 3246 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 3247 3248 /** 3249 * Search parameter: <b>phone</b> 3250 * <p> 3251 * Description: <b>A value in a phone contact</b><br> 3252 * Type: <b>token</b><br> 3253 * Path: <b>Patient.telecom(system=phone)</b><br> 3254 * </p> 3255 */ 3256 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 3257 public static final String SP_PHONE = "phone"; 3258 /** 3259 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 3260 * <p> 3261 * Description: <b>A value in a phone contact</b><br> 3262 * Type: <b>token</b><br> 3263 * Path: <b>Patient.telecom(system=phone)</b><br> 3264 * </p> 3265 */ 3266 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 3267 3268 /** 3269 * Search parameter: <b>organization</b> 3270 * <p> 3271 * Description: <b>The organization at which this person is a patient</b><br> 3272 * Type: <b>reference</b><br> 3273 * Path: <b>Patient.managingOrganization</b><br> 3274 * </p> 3275 */ 3276 @SearchParamDefinition(name="organization", path="Patient.managingOrganization", description="The organization at which this person is a patient", type="reference", target={Organization.class } ) 3277 public static final String SP_ORGANIZATION = "organization"; 3278 /** 3279 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3280 * <p> 3281 * Description: <b>The organization at which this person is a patient</b><br> 3282 * Type: <b>reference</b><br> 3283 * Path: <b>Patient.managingOrganization</b><br> 3284 * </p> 3285 */ 3286 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 3287 3288/** 3289 * Constant for fluent queries to be used to add include statements. Specifies 3290 * the path value of "<b>Patient:organization</b>". 3291 */ 3292 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Patient:organization").toLocked(); 3293 3294 /** 3295 * Search parameter: <b>name</b> 3296 * <p> 3297 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 3298 * Type: <b>string</b><br> 3299 * Path: <b>Patient.name</b><br> 3300 * </p> 3301 */ 3302 @SearchParamDefinition(name="name", path="Patient.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 3303 public static final String SP_NAME = "name"; 3304 /** 3305 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3306 * <p> 3307 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 3308 * Type: <b>string</b><br> 3309 * Path: <b>Patient.name</b><br> 3310 * </p> 3311 */ 3312 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3313 3314 /** 3315 * Search parameter: <b>address-use</b> 3316 * <p> 3317 * Description: <b>A use code specified in an address</b><br> 3318 * Type: <b>token</b><br> 3319 * Path: <b>Patient.address.use</b><br> 3320 * </p> 3321 */ 3322 @SearchParamDefinition(name="address-use", path="Patient.address.use", description="A use code specified in an address", type="token" ) 3323 public static final String SP_ADDRESS_USE = "address-use"; 3324 /** 3325 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3326 * <p> 3327 * Description: <b>A use code specified in an address</b><br> 3328 * Type: <b>token</b><br> 3329 * Path: <b>Patient.address.use</b><br> 3330 * </p> 3331 */ 3332 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 3333 3334 /** 3335 * Search parameter: <b>family</b> 3336 * <p> 3337 * Description: <b>A portion of the family name of the patient</b><br> 3338 * Type: <b>string</b><br> 3339 * Path: <b>Patient.name.family</b><br> 3340 * </p> 3341 */ 3342 @SearchParamDefinition(name="family", path="Patient.name.family", description="A portion of the family name of the patient", type="string" ) 3343 public static final String SP_FAMILY = "family"; 3344 /** 3345 * <b>Fluent Client</b> search parameter constant for <b>family</b> 3346 * <p> 3347 * Description: <b>A portion of the family name of the patient</b><br> 3348 * Type: <b>string</b><br> 3349 * Path: <b>Patient.name.family</b><br> 3350 * </p> 3351 */ 3352 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 3353 3354 3355}