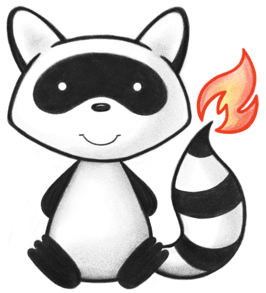
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 047 */ 048@ResourceDef(name="PaymentNotice", profile="http://hl7.org/fhir/Profile/PaymentNotice") 049public class PaymentNotice extends DomainResource { 050 051 public enum PaymentNoticeStatus { 052 /** 053 * The instance is currently in-force. 054 */ 055 ACTIVE, 056 /** 057 * The instance is withdrawn, rescinded or reversed. 058 */ 059 CANCELLED, 060 /** 061 * A new instance the contents of which is not complete. 062 */ 063 DRAFT, 064 /** 065 * The instance was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static PaymentNoticeStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("active".equals(codeString)) 076 return ACTIVE; 077 if ("cancelled".equals(codeString)) 078 return CANCELLED; 079 if ("draft".equals(codeString)) 080 return DRAFT; 081 if ("entered-in-error".equals(codeString)) 082 return ENTEREDINERROR; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown PaymentNoticeStatus code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case ACTIVE: return "active"; 091 case CANCELLED: return "cancelled"; 092 case DRAFT: return "draft"; 093 case ENTEREDINERROR: return "entered-in-error"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 101 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 102 case DRAFT: return "http://hl7.org/fhir/fm-status"; 103 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDefinition() { 109 switch (this) { 110 case ACTIVE: return "The instance is currently in-force."; 111 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 112 case DRAFT: return "A new instance the contents of which is not complete."; 113 case ENTEREDINERROR: return "The instance was entered in error."; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDisplay() { 119 switch (this) { 120 case ACTIVE: return "Active"; 121 case CANCELLED: return "Cancelled"; 122 case DRAFT: return "Draft"; 123 case ENTEREDINERROR: return "Entered in Error"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 } 129 130 public static class PaymentNoticeStatusEnumFactory implements EnumFactory<PaymentNoticeStatus> { 131 public PaymentNoticeStatus fromCode(String codeString) throws IllegalArgumentException { 132 if (codeString == null || "".equals(codeString)) 133 if (codeString == null || "".equals(codeString)) 134 return null; 135 if ("active".equals(codeString)) 136 return PaymentNoticeStatus.ACTIVE; 137 if ("cancelled".equals(codeString)) 138 return PaymentNoticeStatus.CANCELLED; 139 if ("draft".equals(codeString)) 140 return PaymentNoticeStatus.DRAFT; 141 if ("entered-in-error".equals(codeString)) 142 return PaymentNoticeStatus.ENTEREDINERROR; 143 throw new IllegalArgumentException("Unknown PaymentNoticeStatus code '"+codeString+"'"); 144 } 145 public Enumeration<PaymentNoticeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<PaymentNoticeStatus>(this); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("active".equals(codeString)) 154 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.ACTIVE); 155 if ("cancelled".equals(codeString)) 156 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.CANCELLED); 157 if ("draft".equals(codeString)) 158 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.DRAFT); 159 if ("entered-in-error".equals(codeString)) 160 return new Enumeration<PaymentNoticeStatus>(this, PaymentNoticeStatus.ENTEREDINERROR); 161 throw new FHIRException("Unknown PaymentNoticeStatus code '"+codeString+"'"); 162 } 163 public String toCode(PaymentNoticeStatus code) { 164 if (code == PaymentNoticeStatus.NULL) 165 return null; 166 if (code == PaymentNoticeStatus.ACTIVE) 167 return "active"; 168 if (code == PaymentNoticeStatus.CANCELLED) 169 return "cancelled"; 170 if (code == PaymentNoticeStatus.DRAFT) 171 return "draft"; 172 if (code == PaymentNoticeStatus.ENTEREDINERROR) 173 return "entered-in-error"; 174 return "?"; 175 } 176 public String toSystem(PaymentNoticeStatus code) { 177 return code.getSystem(); 178 } 179 } 180 181 /** 182 * The notice business identifier. 183 */ 184 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 185 @Description(shortDefinition="Business Identifier", formalDefinition="The notice business identifier." ) 186 protected List<Identifier> identifier; 187 188 /** 189 * The status of the resource instance. 190 */ 191 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 192 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 194 protected Enumeration<PaymentNoticeStatus> status; 195 196 /** 197 * Reference of resource for which payment is being made. 198 */ 199 @Child(name = "request", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="Request reference", formalDefinition="Reference of resource for which payment is being made." ) 201 protected Reference request; 202 203 /** 204 * The actual object that is the target of the reference (Reference of resource for which payment is being made.) 205 */ 206 protected Resource requestTarget; 207 208 /** 209 * Reference of response to resource for which payment is being made. 210 */ 211 @Child(name = "response", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 212 @Description(shortDefinition="Response reference", formalDefinition="Reference of response to resource for which payment is being made." ) 213 protected Reference response; 214 215 /** 216 * The actual object that is the target of the reference (Reference of response to resource for which payment is being made.) 217 */ 218 protected Resource responseTarget; 219 220 /** 221 * The date when the above payment action occurrred. 222 */ 223 @Child(name = "statusDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 224 @Description(shortDefinition="Payment or clearing date", formalDefinition="The date when the above payment action occurrred." ) 225 protected DateType statusDate; 226 227 /** 228 * The date when this resource was created. 229 */ 230 @Child(name = "created", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 232 protected DateTimeType created; 233 234 /** 235 * The Insurer who is target of the request. 236 */ 237 @Child(name = "target", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Insurer or Regulatory body", formalDefinition="The Insurer who is target of the request." ) 239 protected Reference target; 240 241 /** 242 * The actual object that is the target of the reference (The Insurer who is target of the request.) 243 */ 244 protected Organization targetTarget; 245 246 /** 247 * The practitioner who is responsible for the services rendered to the patient. 248 */ 249 @Child(name = "provider", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 250 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 251 protected Reference provider; 252 253 /** 254 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 255 */ 256 protected Practitioner providerTarget; 257 258 /** 259 * The organization which is responsible for the services rendered to the patient. 260 */ 261 @Child(name = "organization", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 262 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 263 protected Reference organization; 264 265 /** 266 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 267 */ 268 protected Organization organizationTarget; 269 270 /** 271 * The payment status, typically paid: payment sent, cleared: payment received. 272 */ 273 @Child(name = "paymentStatus", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="Whether payment has been sent or cleared", formalDefinition="The payment status, typically paid: payment sent, cleared: payment received." ) 275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-status") 276 protected CodeableConcept paymentStatus; 277 278 private static final long serialVersionUID = 37278603L; 279 280 /** 281 * Constructor 282 */ 283 public PaymentNotice() { 284 super(); 285 } 286 287 /** 288 * @return {@link #identifier} (The notice business identifier.) 289 */ 290 public List<Identifier> getIdentifier() { 291 if (this.identifier == null) 292 this.identifier = new ArrayList<Identifier>(); 293 return this.identifier; 294 } 295 296 /** 297 * @return Returns a reference to <code>this</code> for easy method chaining 298 */ 299 public PaymentNotice setIdentifier(List<Identifier> theIdentifier) { 300 this.identifier = theIdentifier; 301 return this; 302 } 303 304 public boolean hasIdentifier() { 305 if (this.identifier == null) 306 return false; 307 for (Identifier item : this.identifier) 308 if (!item.isEmpty()) 309 return true; 310 return false; 311 } 312 313 public Identifier addIdentifier() { //3 314 Identifier t = new Identifier(); 315 if (this.identifier == null) 316 this.identifier = new ArrayList<Identifier>(); 317 this.identifier.add(t); 318 return t; 319 } 320 321 public PaymentNotice addIdentifier(Identifier t) { //3 322 if (t == null) 323 return this; 324 if (this.identifier == null) 325 this.identifier = new ArrayList<Identifier>(); 326 this.identifier.add(t); 327 return this; 328 } 329 330 /** 331 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 332 */ 333 public Identifier getIdentifierFirstRep() { 334 if (getIdentifier().isEmpty()) { 335 addIdentifier(); 336 } 337 return getIdentifier().get(0); 338 } 339 340 /** 341 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 342 */ 343 public Enumeration<PaymentNoticeStatus> getStatusElement() { 344 if (this.status == null) 345 if (Configuration.errorOnAutoCreate()) 346 throw new Error("Attempt to auto-create PaymentNotice.status"); 347 else if (Configuration.doAutoCreate()) 348 this.status = new Enumeration<PaymentNoticeStatus>(new PaymentNoticeStatusEnumFactory()); // bb 349 return this.status; 350 } 351 352 public boolean hasStatusElement() { 353 return this.status != null && !this.status.isEmpty(); 354 } 355 356 public boolean hasStatus() { 357 return this.status != null && !this.status.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 362 */ 363 public PaymentNotice setStatusElement(Enumeration<PaymentNoticeStatus> value) { 364 this.status = value; 365 return this; 366 } 367 368 /** 369 * @return The status of the resource instance. 370 */ 371 public PaymentNoticeStatus getStatus() { 372 return this.status == null ? null : this.status.getValue(); 373 } 374 375 /** 376 * @param value The status of the resource instance. 377 */ 378 public PaymentNotice setStatus(PaymentNoticeStatus value) { 379 if (value == null) 380 this.status = null; 381 else { 382 if (this.status == null) 383 this.status = new Enumeration<PaymentNoticeStatus>(new PaymentNoticeStatusEnumFactory()); 384 this.status.setValue(value); 385 } 386 return this; 387 } 388 389 /** 390 * @return {@link #request} (Reference of resource for which payment is being made.) 391 */ 392 public Reference getRequest() { 393 if (this.request == null) 394 if (Configuration.errorOnAutoCreate()) 395 throw new Error("Attempt to auto-create PaymentNotice.request"); 396 else if (Configuration.doAutoCreate()) 397 this.request = new Reference(); // cc 398 return this.request; 399 } 400 401 public boolean hasRequest() { 402 return this.request != null && !this.request.isEmpty(); 403 } 404 405 /** 406 * @param value {@link #request} (Reference of resource for which payment is being made.) 407 */ 408 public PaymentNotice setRequest(Reference value) { 409 this.request = value; 410 return this; 411 } 412 413 /** 414 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference of resource for which payment is being made.) 415 */ 416 public Resource getRequestTarget() { 417 return this.requestTarget; 418 } 419 420 /** 421 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference of resource for which payment is being made.) 422 */ 423 public PaymentNotice setRequestTarget(Resource value) { 424 this.requestTarget = value; 425 return this; 426 } 427 428 /** 429 * @return {@link #response} (Reference of response to resource for which payment is being made.) 430 */ 431 public Reference getResponse() { 432 if (this.response == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error("Attempt to auto-create PaymentNotice.response"); 435 else if (Configuration.doAutoCreate()) 436 this.response = new Reference(); // cc 437 return this.response; 438 } 439 440 public boolean hasResponse() { 441 return this.response != null && !this.response.isEmpty(); 442 } 443 444 /** 445 * @param value {@link #response} (Reference of response to resource for which payment is being made.) 446 */ 447 public PaymentNotice setResponse(Reference value) { 448 this.response = value; 449 return this; 450 } 451 452 /** 453 * @return {@link #response} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference of response to resource for which payment is being made.) 454 */ 455 public Resource getResponseTarget() { 456 return this.responseTarget; 457 } 458 459 /** 460 * @param value {@link #response} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference of response to resource for which payment is being made.) 461 */ 462 public PaymentNotice setResponseTarget(Resource value) { 463 this.responseTarget = value; 464 return this; 465 } 466 467 /** 468 * @return {@link #statusDate} (The date when the above payment action occurrred.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 469 */ 470 public DateType getStatusDateElement() { 471 if (this.statusDate == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create PaymentNotice.statusDate"); 474 else if (Configuration.doAutoCreate()) 475 this.statusDate = new DateType(); // bb 476 return this.statusDate; 477 } 478 479 public boolean hasStatusDateElement() { 480 return this.statusDate != null && !this.statusDate.isEmpty(); 481 } 482 483 public boolean hasStatusDate() { 484 return this.statusDate != null && !this.statusDate.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #statusDate} (The date when the above payment action occurrred.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 489 */ 490 public PaymentNotice setStatusDateElement(DateType value) { 491 this.statusDate = value; 492 return this; 493 } 494 495 /** 496 * @return The date when the above payment action occurrred. 497 */ 498 public Date getStatusDate() { 499 return this.statusDate == null ? null : this.statusDate.getValue(); 500 } 501 502 /** 503 * @param value The date when the above payment action occurrred. 504 */ 505 public PaymentNotice setStatusDate(Date value) { 506 if (value == null) 507 this.statusDate = null; 508 else { 509 if (this.statusDate == null) 510 this.statusDate = new DateType(); 511 this.statusDate.setValue(value); 512 } 513 return this; 514 } 515 516 /** 517 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 518 */ 519 public DateTimeType getCreatedElement() { 520 if (this.created == null) 521 if (Configuration.errorOnAutoCreate()) 522 throw new Error("Attempt to auto-create PaymentNotice.created"); 523 else if (Configuration.doAutoCreate()) 524 this.created = new DateTimeType(); // bb 525 return this.created; 526 } 527 528 public boolean hasCreatedElement() { 529 return this.created != null && !this.created.isEmpty(); 530 } 531 532 public boolean hasCreated() { 533 return this.created != null && !this.created.isEmpty(); 534 } 535 536 /** 537 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 538 */ 539 public PaymentNotice setCreatedElement(DateTimeType value) { 540 this.created = value; 541 return this; 542 } 543 544 /** 545 * @return The date when this resource was created. 546 */ 547 public Date getCreated() { 548 return this.created == null ? null : this.created.getValue(); 549 } 550 551 /** 552 * @param value The date when this resource was created. 553 */ 554 public PaymentNotice setCreated(Date value) { 555 if (value == null) 556 this.created = null; 557 else { 558 if (this.created == null) 559 this.created = new DateTimeType(); 560 this.created.setValue(value); 561 } 562 return this; 563 } 564 565 /** 566 * @return {@link #target} (The Insurer who is target of the request.) 567 */ 568 public Reference getTarget() { 569 if (this.target == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create PaymentNotice.target"); 572 else if (Configuration.doAutoCreate()) 573 this.target = new Reference(); // cc 574 return this.target; 575 } 576 577 public boolean hasTarget() { 578 return this.target != null && !this.target.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #target} (The Insurer who is target of the request.) 583 */ 584 public PaymentNotice setTarget(Reference value) { 585 this.target = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 591 */ 592 public Organization getTargetTarget() { 593 if (this.targetTarget == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create PaymentNotice.target"); 596 else if (Configuration.doAutoCreate()) 597 this.targetTarget = new Organization(); // aa 598 return this.targetTarget; 599 } 600 601 /** 602 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 603 */ 604 public PaymentNotice setTargetTarget(Organization value) { 605 this.targetTarget = value; 606 return this; 607 } 608 609 /** 610 * @return {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 611 */ 612 public Reference getProvider() { 613 if (this.provider == null) 614 if (Configuration.errorOnAutoCreate()) 615 throw new Error("Attempt to auto-create PaymentNotice.provider"); 616 else if (Configuration.doAutoCreate()) 617 this.provider = new Reference(); // cc 618 return this.provider; 619 } 620 621 public boolean hasProvider() { 622 return this.provider != null && !this.provider.isEmpty(); 623 } 624 625 /** 626 * @param value {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 627 */ 628 public PaymentNotice setProvider(Reference value) { 629 this.provider = value; 630 return this; 631 } 632 633 /** 634 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 635 */ 636 public Practitioner getProviderTarget() { 637 if (this.providerTarget == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create PaymentNotice.provider"); 640 else if (Configuration.doAutoCreate()) 641 this.providerTarget = new Practitioner(); // aa 642 return this.providerTarget; 643 } 644 645 /** 646 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 647 */ 648 public PaymentNotice setProviderTarget(Practitioner value) { 649 this.providerTarget = value; 650 return this; 651 } 652 653 /** 654 * @return {@link #organization} (The organization which is responsible for the services rendered to the patient.) 655 */ 656 public Reference getOrganization() { 657 if (this.organization == null) 658 if (Configuration.errorOnAutoCreate()) 659 throw new Error("Attempt to auto-create PaymentNotice.organization"); 660 else if (Configuration.doAutoCreate()) 661 this.organization = new Reference(); // cc 662 return this.organization; 663 } 664 665 public boolean hasOrganization() { 666 return this.organization != null && !this.organization.isEmpty(); 667 } 668 669 /** 670 * @param value {@link #organization} (The organization which is responsible for the services rendered to the patient.) 671 */ 672 public PaymentNotice setOrganization(Reference value) { 673 this.organization = value; 674 return this; 675 } 676 677 /** 678 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 679 */ 680 public Organization getOrganizationTarget() { 681 if (this.organizationTarget == null) 682 if (Configuration.errorOnAutoCreate()) 683 throw new Error("Attempt to auto-create PaymentNotice.organization"); 684 else if (Configuration.doAutoCreate()) 685 this.organizationTarget = new Organization(); // aa 686 return this.organizationTarget; 687 } 688 689 /** 690 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 691 */ 692 public PaymentNotice setOrganizationTarget(Organization value) { 693 this.organizationTarget = value; 694 return this; 695 } 696 697 /** 698 * @return {@link #paymentStatus} (The payment status, typically paid: payment sent, cleared: payment received.) 699 */ 700 public CodeableConcept getPaymentStatus() { 701 if (this.paymentStatus == null) 702 if (Configuration.errorOnAutoCreate()) 703 throw new Error("Attempt to auto-create PaymentNotice.paymentStatus"); 704 else if (Configuration.doAutoCreate()) 705 this.paymentStatus = new CodeableConcept(); // cc 706 return this.paymentStatus; 707 } 708 709 public boolean hasPaymentStatus() { 710 return this.paymentStatus != null && !this.paymentStatus.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #paymentStatus} (The payment status, typically paid: payment sent, cleared: payment received.) 715 */ 716 public PaymentNotice setPaymentStatus(CodeableConcept value) { 717 this.paymentStatus = value; 718 return this; 719 } 720 721 protected void listChildren(List<Property> children) { 722 super.listChildren(children); 723 children.add(new Property("identifier", "Identifier", "The notice business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 724 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 725 children.add(new Property("request", "Reference(Any)", "Reference of resource for which payment is being made.", 0, 1, request)); 726 children.add(new Property("response", "Reference(Any)", "Reference of response to resource for which payment is being made.", 0, 1, response)); 727 children.add(new Property("statusDate", "date", "The date when the above payment action occurrred.", 0, 1, statusDate)); 728 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 729 children.add(new Property("target", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, target)); 730 children.add(new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 731 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization)); 732 children.add(new Property("paymentStatus", "CodeableConcept", "The payment status, typically paid: payment sent, cleared: payment received.", 0, 1, paymentStatus)); 733 } 734 735 @Override 736 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 737 switch (_hash) { 738 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The notice business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 739 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 740 case 1095692943: /*request*/ return new Property("request", "Reference(Any)", "Reference of resource for which payment is being made.", 0, 1, request); 741 case -340323263: /*response*/ return new Property("response", "Reference(Any)", "Reference of response to resource for which payment is being made.", 0, 1, response); 742 case 247524032: /*statusDate*/ return new Property("statusDate", "date", "The date when the above payment action occurrred.", 0, 1, statusDate); 743 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 744 case -880905839: /*target*/ return new Property("target", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, target); 745 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 746 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization); 747 case 1430704536: /*paymentStatus*/ return new Property("paymentStatus", "CodeableConcept", "The payment status, typically paid: payment sent, cleared: payment received.", 0, 1, paymentStatus); 748 default: return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 757 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PaymentNoticeStatus> 758 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 759 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 760 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateType 761 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 762 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 763 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 764 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 765 case 1430704536: /*paymentStatus*/ return this.paymentStatus == null ? new Base[0] : new Base[] {this.paymentStatus}; // CodeableConcept 766 default: return super.getProperty(hash, name, checkValid); 767 } 768 769 } 770 771 @Override 772 public Base setProperty(int hash, String name, Base value) throws FHIRException { 773 switch (hash) { 774 case -1618432855: // identifier 775 this.getIdentifier().add(castToIdentifier(value)); // Identifier 776 return value; 777 case -892481550: // status 778 value = new PaymentNoticeStatusEnumFactory().fromType(castToCode(value)); 779 this.status = (Enumeration) value; // Enumeration<PaymentNoticeStatus> 780 return value; 781 case 1095692943: // request 782 this.request = castToReference(value); // Reference 783 return value; 784 case -340323263: // response 785 this.response = castToReference(value); // Reference 786 return value; 787 case 247524032: // statusDate 788 this.statusDate = castToDate(value); // DateType 789 return value; 790 case 1028554472: // created 791 this.created = castToDateTime(value); // DateTimeType 792 return value; 793 case -880905839: // target 794 this.target = castToReference(value); // Reference 795 return value; 796 case -987494927: // provider 797 this.provider = castToReference(value); // Reference 798 return value; 799 case 1178922291: // organization 800 this.organization = castToReference(value); // Reference 801 return value; 802 case 1430704536: // paymentStatus 803 this.paymentStatus = castToCodeableConcept(value); // CodeableConcept 804 return value; 805 default: return super.setProperty(hash, name, value); 806 } 807 808 } 809 810 @Override 811 public Base setProperty(String name, Base value) throws FHIRException { 812 if (name.equals("identifier")) { 813 this.getIdentifier().add(castToIdentifier(value)); 814 } else if (name.equals("status")) { 815 value = new PaymentNoticeStatusEnumFactory().fromType(castToCode(value)); 816 this.status = (Enumeration) value; // Enumeration<PaymentNoticeStatus> 817 } else if (name.equals("request")) { 818 this.request = castToReference(value); // Reference 819 } else if (name.equals("response")) { 820 this.response = castToReference(value); // Reference 821 } else if (name.equals("statusDate")) { 822 this.statusDate = castToDate(value); // DateType 823 } else if (name.equals("created")) { 824 this.created = castToDateTime(value); // DateTimeType 825 } else if (name.equals("target")) { 826 this.target = castToReference(value); // Reference 827 } else if (name.equals("provider")) { 828 this.provider = castToReference(value); // Reference 829 } else if (name.equals("organization")) { 830 this.organization = castToReference(value); // Reference 831 } else if (name.equals("paymentStatus")) { 832 this.paymentStatus = castToCodeableConcept(value); // CodeableConcept 833 } else 834 return super.setProperty(name, value); 835 return value; 836 } 837 838 @Override 839 public Base makeProperty(int hash, String name) throws FHIRException { 840 switch (hash) { 841 case -1618432855: return addIdentifier(); 842 case -892481550: return getStatusElement(); 843 case 1095692943: return getRequest(); 844 case -340323263: return getResponse(); 845 case 247524032: return getStatusDateElement(); 846 case 1028554472: return getCreatedElement(); 847 case -880905839: return getTarget(); 848 case -987494927: return getProvider(); 849 case 1178922291: return getOrganization(); 850 case 1430704536: return getPaymentStatus(); 851 default: return super.makeProperty(hash, name); 852 } 853 854 } 855 856 @Override 857 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 858 switch (hash) { 859 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 860 case -892481550: /*status*/ return new String[] {"code"}; 861 case 1095692943: /*request*/ return new String[] {"Reference"}; 862 case -340323263: /*response*/ return new String[] {"Reference"}; 863 case 247524032: /*statusDate*/ return new String[] {"date"}; 864 case 1028554472: /*created*/ return new String[] {"dateTime"}; 865 case -880905839: /*target*/ return new String[] {"Reference"}; 866 case -987494927: /*provider*/ return new String[] {"Reference"}; 867 case 1178922291: /*organization*/ return new String[] {"Reference"}; 868 case 1430704536: /*paymentStatus*/ return new String[] {"CodeableConcept"}; 869 default: return super.getTypesForProperty(hash, name); 870 } 871 872 } 873 874 @Override 875 public Base addChild(String name) throws FHIRException { 876 if (name.equals("identifier")) { 877 return addIdentifier(); 878 } 879 else if (name.equals("status")) { 880 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.status"); 881 } 882 else if (name.equals("request")) { 883 this.request = new Reference(); 884 return this.request; 885 } 886 else if (name.equals("response")) { 887 this.response = new Reference(); 888 return this.response; 889 } 890 else if (name.equals("statusDate")) { 891 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.statusDate"); 892 } 893 else if (name.equals("created")) { 894 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.created"); 895 } 896 else if (name.equals("target")) { 897 this.target = new Reference(); 898 return this.target; 899 } 900 else if (name.equals("provider")) { 901 this.provider = new Reference(); 902 return this.provider; 903 } 904 else if (name.equals("organization")) { 905 this.organization = new Reference(); 906 return this.organization; 907 } 908 else if (name.equals("paymentStatus")) { 909 this.paymentStatus = new CodeableConcept(); 910 return this.paymentStatus; 911 } 912 else 913 return super.addChild(name); 914 } 915 916 public String fhirType() { 917 return "PaymentNotice"; 918 919 } 920 921 public PaymentNotice copy() { 922 PaymentNotice dst = new PaymentNotice(); 923 copyValues(dst); 924 if (identifier != null) { 925 dst.identifier = new ArrayList<Identifier>(); 926 for (Identifier i : identifier) 927 dst.identifier.add(i.copy()); 928 }; 929 dst.status = status == null ? null : status.copy(); 930 dst.request = request == null ? null : request.copy(); 931 dst.response = response == null ? null : response.copy(); 932 dst.statusDate = statusDate == null ? null : statusDate.copy(); 933 dst.created = created == null ? null : created.copy(); 934 dst.target = target == null ? null : target.copy(); 935 dst.provider = provider == null ? null : provider.copy(); 936 dst.organization = organization == null ? null : organization.copy(); 937 dst.paymentStatus = paymentStatus == null ? null : paymentStatus.copy(); 938 return dst; 939 } 940 941 protected PaymentNotice typedCopy() { 942 return copy(); 943 } 944 945 @Override 946 public boolean equalsDeep(Base other_) { 947 if (!super.equalsDeep(other_)) 948 return false; 949 if (!(other_ instanceof PaymentNotice)) 950 return false; 951 PaymentNotice o = (PaymentNotice) other_; 952 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(request, o.request, true) 953 && compareDeep(response, o.response, true) && compareDeep(statusDate, o.statusDate, true) && compareDeep(created, o.created, true) 954 && compareDeep(target, o.target, true) && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) 955 && compareDeep(paymentStatus, o.paymentStatus, true); 956 } 957 958 @Override 959 public boolean equalsShallow(Base other_) { 960 if (!super.equalsShallow(other_)) 961 return false; 962 if (!(other_ instanceof PaymentNotice)) 963 return false; 964 PaymentNotice o = (PaymentNotice) other_; 965 return compareValues(status, o.status, true) && compareValues(statusDate, o.statusDate, true) && compareValues(created, o.created, true) 966 ; 967 } 968 969 public boolean isEmpty() { 970 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request 971 , response, statusDate, created, target, provider, organization, paymentStatus 972 ); 973 } 974 975 @Override 976 public ResourceType getResourceType() { 977 return ResourceType.PaymentNotice; 978 } 979 980 /** 981 * Search parameter: <b>identifier</b> 982 * <p> 983 * Description: <b>The business identifier of the notice</b><br> 984 * Type: <b>token</b><br> 985 * Path: <b>PaymentNotice.identifier</b><br> 986 * </p> 987 */ 988 @SearchParamDefinition(name="identifier", path="PaymentNotice.identifier", description="The business identifier of the notice", type="token" ) 989 public static final String SP_IDENTIFIER = "identifier"; 990 /** 991 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 992 * <p> 993 * Description: <b>The business identifier of the notice</b><br> 994 * Type: <b>token</b><br> 995 * Path: <b>PaymentNotice.identifier</b><br> 996 * </p> 997 */ 998 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 999 1000 /** 1001 * Search parameter: <b>request</b> 1002 * <p> 1003 * Description: <b>The Claim</b><br> 1004 * Type: <b>reference</b><br> 1005 * Path: <b>PaymentNotice.request</b><br> 1006 * </p> 1007 */ 1008 @SearchParamDefinition(name="request", path="PaymentNotice.request", description="The Claim", type="reference" ) 1009 public static final String SP_REQUEST = "request"; 1010 /** 1011 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1012 * <p> 1013 * Description: <b>The Claim</b><br> 1014 * Type: <b>reference</b><br> 1015 * Path: <b>PaymentNotice.request</b><br> 1016 * </p> 1017 */ 1018 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 1019 1020/** 1021 * Constant for fluent queries to be used to add include statements. Specifies 1022 * the path value of "<b>PaymentNotice:request</b>". 1023 */ 1024 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("PaymentNotice:request").toLocked(); 1025 1026 /** 1027 * Search parameter: <b>provider</b> 1028 * <p> 1029 * Description: <b>The reference to the provider</b><br> 1030 * Type: <b>reference</b><br> 1031 * Path: <b>PaymentNotice.provider</b><br> 1032 * </p> 1033 */ 1034 @SearchParamDefinition(name="provider", path="PaymentNotice.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1035 public static final String SP_PROVIDER = "provider"; 1036 /** 1037 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 1038 * <p> 1039 * Description: <b>The reference to the provider</b><br> 1040 * Type: <b>reference</b><br> 1041 * Path: <b>PaymentNotice.provider</b><br> 1042 * </p> 1043 */ 1044 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 1045 1046/** 1047 * Constant for fluent queries to be used to add include statements. Specifies 1048 * the path value of "<b>PaymentNotice:provider</b>". 1049 */ 1050 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("PaymentNotice:provider").toLocked(); 1051 1052 /** 1053 * Search parameter: <b>created</b> 1054 * <p> 1055 * Description: <b>Creation date fro the notice</b><br> 1056 * Type: <b>date</b><br> 1057 * Path: <b>PaymentNotice.created</b><br> 1058 * </p> 1059 */ 1060 @SearchParamDefinition(name="created", path="PaymentNotice.created", description="Creation date fro the notice", type="date" ) 1061 public static final String SP_CREATED = "created"; 1062 /** 1063 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1064 * <p> 1065 * Description: <b>Creation date fro the notice</b><br> 1066 * Type: <b>date</b><br> 1067 * Path: <b>PaymentNotice.created</b><br> 1068 * </p> 1069 */ 1070 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 1071 1072 /** 1073 * Search parameter: <b>response</b> 1074 * <p> 1075 * Description: <b>The ClaimResponse</b><br> 1076 * Type: <b>reference</b><br> 1077 * Path: <b>PaymentNotice.response</b><br> 1078 * </p> 1079 */ 1080 @SearchParamDefinition(name="response", path="PaymentNotice.response", description="The ClaimResponse", type="reference" ) 1081 public static final String SP_RESPONSE = "response"; 1082 /** 1083 * <b>Fluent Client</b> search parameter constant for <b>response</b> 1084 * <p> 1085 * Description: <b>The ClaimResponse</b><br> 1086 * Type: <b>reference</b><br> 1087 * Path: <b>PaymentNotice.response</b><br> 1088 * </p> 1089 */ 1090 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSE); 1091 1092/** 1093 * Constant for fluent queries to be used to add include statements. Specifies 1094 * the path value of "<b>PaymentNotice:response</b>". 1095 */ 1096 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSE = new ca.uhn.fhir.model.api.Include("PaymentNotice:response").toLocked(); 1097 1098 /** 1099 * Search parameter: <b>organization</b> 1100 * <p> 1101 * Description: <b>The organization who generated this resource</b><br> 1102 * Type: <b>reference</b><br> 1103 * Path: <b>PaymentNotice.organization</b><br> 1104 * </p> 1105 */ 1106 @SearchParamDefinition(name="organization", path="PaymentNotice.organization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 1107 public static final String SP_ORGANIZATION = "organization"; 1108 /** 1109 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1110 * <p> 1111 * Description: <b>The organization who generated this resource</b><br> 1112 * Type: <b>reference</b><br> 1113 * Path: <b>PaymentNotice.organization</b><br> 1114 * </p> 1115 */ 1116 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1117 1118/** 1119 * Constant for fluent queries to be used to add include statements. Specifies 1120 * the path value of "<b>PaymentNotice:organization</b>". 1121 */ 1122 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PaymentNotice:organization").toLocked(); 1123 1124 /** 1125 * Search parameter: <b>payment-status</b> 1126 * <p> 1127 * Description: <b>The type of payment notice</b><br> 1128 * Type: <b>token</b><br> 1129 * Path: <b>PaymentNotice.paymentStatus</b><br> 1130 * </p> 1131 */ 1132 @SearchParamDefinition(name="payment-status", path="PaymentNotice.paymentStatus", description="The type of payment notice", type="token" ) 1133 public static final String SP_PAYMENT_STATUS = "payment-status"; 1134 /** 1135 * <b>Fluent Client</b> search parameter constant for <b>payment-status</b> 1136 * <p> 1137 * Description: <b>The type of payment notice</b><br> 1138 * Type: <b>token</b><br> 1139 * Path: <b>PaymentNotice.paymentStatus</b><br> 1140 * </p> 1141 */ 1142 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYMENT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYMENT_STATUS); 1143 1144 /** 1145 * Search parameter: <b>statusdate</b> 1146 * <p> 1147 * Description: <b>The date of the payment action</b><br> 1148 * Type: <b>date</b><br> 1149 * Path: <b>PaymentNotice.statusDate</b><br> 1150 * </p> 1151 */ 1152 @SearchParamDefinition(name="statusdate", path="PaymentNotice.statusDate", description="The date of the payment action", type="date" ) 1153 public static final String SP_STATUSDATE = "statusdate"; 1154 /** 1155 * <b>Fluent Client</b> search parameter constant for <b>statusdate</b> 1156 * <p> 1157 * Description: <b>The date of the payment action</b><br> 1158 * Type: <b>date</b><br> 1159 * Path: <b>PaymentNotice.statusDate</b><br> 1160 * </p> 1161 */ 1162 public static final ca.uhn.fhir.rest.gclient.DateClientParam STATUSDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_STATUSDATE); 1163 1164 1165}