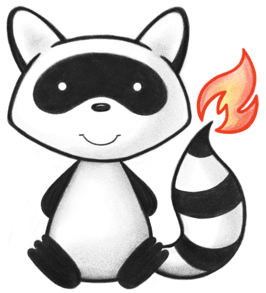
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * This resource provides payment details and claim references supporting a bulk payment. 050 */ 051@ResourceDef(name="PaymentReconciliation", profile="http://hl7.org/fhir/Profile/PaymentReconciliation") 052public class PaymentReconciliation extends DomainResource { 053 054 public enum PaymentReconciliationStatus { 055 /** 056 * The instance is currently in-force. 057 */ 058 ACTIVE, 059 /** 060 * The instance is withdrawn, rescinded or reversed. 061 */ 062 CANCELLED, 063 /** 064 * A new instance the contents of which is not complete. 065 */ 066 DRAFT, 067 /** 068 * The instance was entered in error. 069 */ 070 ENTEREDINERROR, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static PaymentReconciliationStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("active".equals(codeString)) 079 return ACTIVE; 080 if ("cancelled".equals(codeString)) 081 return CANCELLED; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown PaymentReconciliationStatus code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case ACTIVE: return "active"; 094 case CANCELLED: return "cancelled"; 095 case DRAFT: return "draft"; 096 case ENTEREDINERROR: return "entered-in-error"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 104 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 105 case DRAFT: return "http://hl7.org/fhir/fm-status"; 106 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case ACTIVE: return "The instance is currently in-force."; 114 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 115 case DRAFT: return "A new instance the contents of which is not complete."; 116 case ENTEREDINERROR: return "The instance was entered in error."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case ACTIVE: return "Active"; 124 case CANCELLED: return "Cancelled"; 125 case DRAFT: return "Draft"; 126 case ENTEREDINERROR: return "Entered in Error"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class PaymentReconciliationStatusEnumFactory implements EnumFactory<PaymentReconciliationStatus> { 134 public PaymentReconciliationStatus fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("active".equals(codeString)) 139 return PaymentReconciliationStatus.ACTIVE; 140 if ("cancelled".equals(codeString)) 141 return PaymentReconciliationStatus.CANCELLED; 142 if ("draft".equals(codeString)) 143 return PaymentReconciliationStatus.DRAFT; 144 if ("entered-in-error".equals(codeString)) 145 return PaymentReconciliationStatus.ENTEREDINERROR; 146 throw new IllegalArgumentException("Unknown PaymentReconciliationStatus code '"+codeString+"'"); 147 } 148 public Enumeration<PaymentReconciliationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<PaymentReconciliationStatus>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("active".equals(codeString)) 157 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.ACTIVE); 158 if ("cancelled".equals(codeString)) 159 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.CANCELLED); 160 if ("draft".equals(codeString)) 161 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.DRAFT); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<PaymentReconciliationStatus>(this, PaymentReconciliationStatus.ENTEREDINERROR); 164 throw new FHIRException("Unknown PaymentReconciliationStatus code '"+codeString+"'"); 165 } 166 public String toCode(PaymentReconciliationStatus code) { 167 if (code == PaymentReconciliationStatus.NULL) 168 return null; 169 if (code == PaymentReconciliationStatus.ACTIVE) 170 return "active"; 171 if (code == PaymentReconciliationStatus.CANCELLED) 172 return "cancelled"; 173 if (code == PaymentReconciliationStatus.DRAFT) 174 return "draft"; 175 if (code == PaymentReconciliationStatus.ENTEREDINERROR) 176 return "entered-in-error"; 177 return "?"; 178 } 179 public String toSystem(PaymentReconciliationStatus code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class DetailsComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * Code to indicate the nature of the payment, adjustment, funds advance, etc. 188 */ 189 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Type code", formalDefinition="Code to indicate the nature of the payment, adjustment, funds advance, etc." ) 191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-type") 192 protected CodeableConcept type; 193 194 /** 195 * The claim or financial resource. 196 */ 197 @Child(name = "request", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="Claim", formalDefinition="The claim or financial resource." ) 199 protected Reference request; 200 201 /** 202 * The actual object that is the target of the reference (The claim or financial resource.) 203 */ 204 protected Resource requestTarget; 205 206 /** 207 * The claim response resource. 208 */ 209 @Child(name = "response", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 210 @Description(shortDefinition="Claim Response", formalDefinition="The claim response resource." ) 211 protected Reference response; 212 213 /** 214 * The actual object that is the target of the reference (The claim response resource.) 215 */ 216 protected Resource responseTarget; 217 218 /** 219 * The Organization which submitted the claim or financial transaction. 220 */ 221 @Child(name = "submitter", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Organization which submitted the claim", formalDefinition="The Organization which submitted the claim or financial transaction." ) 223 protected Reference submitter; 224 225 /** 226 * The actual object that is the target of the reference (The Organization which submitted the claim or financial transaction.) 227 */ 228 protected Organization submitterTarget; 229 230 /** 231 * The organization which is receiving the payment. 232 */ 233 @Child(name = "payee", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 234 @Description(shortDefinition="Organization which is receiving the payment", formalDefinition="The organization which is receiving the payment." ) 235 protected Reference payee; 236 237 /** 238 * The actual object that is the target of the reference (The organization which is receiving the payment.) 239 */ 240 protected Organization payeeTarget; 241 242 /** 243 * The date of the invoice or financial resource. 244 */ 245 @Child(name = "date", type = {DateType.class}, order=6, min=0, max=1, modifier=false, summary=false) 246 @Description(shortDefinition="Invoice date", formalDefinition="The date of the invoice or financial resource." ) 247 protected DateType date; 248 249 /** 250 * Amount paid for this detail. 251 */ 252 @Child(name = "amount", type = {Money.class}, order=7, min=0, max=1, modifier=false, summary=false) 253 @Description(shortDefinition="Amount being paid", formalDefinition="Amount paid for this detail." ) 254 protected Money amount; 255 256 private static final long serialVersionUID = 661095855L; 257 258 /** 259 * Constructor 260 */ 261 public DetailsComponent() { 262 super(); 263 } 264 265 /** 266 * Constructor 267 */ 268 public DetailsComponent(CodeableConcept type) { 269 super(); 270 this.type = type; 271 } 272 273 /** 274 * @return {@link #type} (Code to indicate the nature of the payment, adjustment, funds advance, etc.) 275 */ 276 public CodeableConcept getType() { 277 if (this.type == null) 278 if (Configuration.errorOnAutoCreate()) 279 throw new Error("Attempt to auto-create DetailsComponent.type"); 280 else if (Configuration.doAutoCreate()) 281 this.type = new CodeableConcept(); // cc 282 return this.type; 283 } 284 285 public boolean hasType() { 286 return this.type != null && !this.type.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #type} (Code to indicate the nature of the payment, adjustment, funds advance, etc.) 291 */ 292 public DetailsComponent setType(CodeableConcept value) { 293 this.type = value; 294 return this; 295 } 296 297 /** 298 * @return {@link #request} (The claim or financial resource.) 299 */ 300 public Reference getRequest() { 301 if (this.request == null) 302 if (Configuration.errorOnAutoCreate()) 303 throw new Error("Attempt to auto-create DetailsComponent.request"); 304 else if (Configuration.doAutoCreate()) 305 this.request = new Reference(); // cc 306 return this.request; 307 } 308 309 public boolean hasRequest() { 310 return this.request != null && !this.request.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #request} (The claim or financial resource.) 315 */ 316 public DetailsComponent setRequest(Reference value) { 317 this.request = value; 318 return this; 319 } 320 321 /** 322 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The claim or financial resource.) 323 */ 324 public Resource getRequestTarget() { 325 return this.requestTarget; 326 } 327 328 /** 329 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The claim or financial resource.) 330 */ 331 public DetailsComponent setRequestTarget(Resource value) { 332 this.requestTarget = value; 333 return this; 334 } 335 336 /** 337 * @return {@link #response} (The claim response resource.) 338 */ 339 public Reference getResponse() { 340 if (this.response == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create DetailsComponent.response"); 343 else if (Configuration.doAutoCreate()) 344 this.response = new Reference(); // cc 345 return this.response; 346 } 347 348 public boolean hasResponse() { 349 return this.response != null && !this.response.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #response} (The claim response resource.) 354 */ 355 public DetailsComponent setResponse(Reference value) { 356 this.response = value; 357 return this; 358 } 359 360 /** 361 * @return {@link #response} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The claim response resource.) 362 */ 363 public Resource getResponseTarget() { 364 return this.responseTarget; 365 } 366 367 /** 368 * @param value {@link #response} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The claim response resource.) 369 */ 370 public DetailsComponent setResponseTarget(Resource value) { 371 this.responseTarget = value; 372 return this; 373 } 374 375 /** 376 * @return {@link #submitter} (The Organization which submitted the claim or financial transaction.) 377 */ 378 public Reference getSubmitter() { 379 if (this.submitter == null) 380 if (Configuration.errorOnAutoCreate()) 381 throw new Error("Attempt to auto-create DetailsComponent.submitter"); 382 else if (Configuration.doAutoCreate()) 383 this.submitter = new Reference(); // cc 384 return this.submitter; 385 } 386 387 public boolean hasSubmitter() { 388 return this.submitter != null && !this.submitter.isEmpty(); 389 } 390 391 /** 392 * @param value {@link #submitter} (The Organization which submitted the claim or financial transaction.) 393 */ 394 public DetailsComponent setSubmitter(Reference value) { 395 this.submitter = value; 396 return this; 397 } 398 399 /** 400 * @return {@link #submitter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Organization which submitted the claim or financial transaction.) 401 */ 402 public Organization getSubmitterTarget() { 403 if (this.submitterTarget == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create DetailsComponent.submitter"); 406 else if (Configuration.doAutoCreate()) 407 this.submitterTarget = new Organization(); // aa 408 return this.submitterTarget; 409 } 410 411 /** 412 * @param value {@link #submitter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Organization which submitted the claim or financial transaction.) 413 */ 414 public DetailsComponent setSubmitterTarget(Organization value) { 415 this.submitterTarget = value; 416 return this; 417 } 418 419 /** 420 * @return {@link #payee} (The organization which is receiving the payment.) 421 */ 422 public Reference getPayee() { 423 if (this.payee == null) 424 if (Configuration.errorOnAutoCreate()) 425 throw new Error("Attempt to auto-create DetailsComponent.payee"); 426 else if (Configuration.doAutoCreate()) 427 this.payee = new Reference(); // cc 428 return this.payee; 429 } 430 431 public boolean hasPayee() { 432 return this.payee != null && !this.payee.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #payee} (The organization which is receiving the payment.) 437 */ 438 public DetailsComponent setPayee(Reference value) { 439 this.payee = value; 440 return this; 441 } 442 443 /** 444 * @return {@link #payee} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is receiving the payment.) 445 */ 446 public Organization getPayeeTarget() { 447 if (this.payeeTarget == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create DetailsComponent.payee"); 450 else if (Configuration.doAutoCreate()) 451 this.payeeTarget = new Organization(); // aa 452 return this.payeeTarget; 453 } 454 455 /** 456 * @param value {@link #payee} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is receiving the payment.) 457 */ 458 public DetailsComponent setPayeeTarget(Organization value) { 459 this.payeeTarget = value; 460 return this; 461 } 462 463 /** 464 * @return {@link #date} (The date of the invoice or financial resource.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 465 */ 466 public DateType getDateElement() { 467 if (this.date == null) 468 if (Configuration.errorOnAutoCreate()) 469 throw new Error("Attempt to auto-create DetailsComponent.date"); 470 else if (Configuration.doAutoCreate()) 471 this.date = new DateType(); // bb 472 return this.date; 473 } 474 475 public boolean hasDateElement() { 476 return this.date != null && !this.date.isEmpty(); 477 } 478 479 public boolean hasDate() { 480 return this.date != null && !this.date.isEmpty(); 481 } 482 483 /** 484 * @param value {@link #date} (The date of the invoice or financial resource.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 485 */ 486 public DetailsComponent setDateElement(DateType value) { 487 this.date = value; 488 return this; 489 } 490 491 /** 492 * @return The date of the invoice or financial resource. 493 */ 494 public Date getDate() { 495 return this.date == null ? null : this.date.getValue(); 496 } 497 498 /** 499 * @param value The date of the invoice or financial resource. 500 */ 501 public DetailsComponent setDate(Date value) { 502 if (value == null) 503 this.date = null; 504 else { 505 if (this.date == null) 506 this.date = new DateType(); 507 this.date.setValue(value); 508 } 509 return this; 510 } 511 512 /** 513 * @return {@link #amount} (Amount paid for this detail.) 514 */ 515 public Money getAmount() { 516 if (this.amount == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create DetailsComponent.amount"); 519 else if (Configuration.doAutoCreate()) 520 this.amount = new Money(); // cc 521 return this.amount; 522 } 523 524 public boolean hasAmount() { 525 return this.amount != null && !this.amount.isEmpty(); 526 } 527 528 /** 529 * @param value {@link #amount} (Amount paid for this detail.) 530 */ 531 public DetailsComponent setAmount(Money value) { 532 this.amount = value; 533 return this; 534 } 535 536 protected void listChildren(List<Property> children) { 537 super.listChildren(children); 538 children.add(new Property("type", "CodeableConcept", "Code to indicate the nature of the payment, adjustment, funds advance, etc.", 0, 1, type)); 539 children.add(new Property("request", "Reference(Any)", "The claim or financial resource.", 0, 1, request)); 540 children.add(new Property("response", "Reference(Any)", "The claim response resource.", 0, 1, response)); 541 children.add(new Property("submitter", "Reference(Organization)", "The Organization which submitted the claim or financial transaction.", 0, 1, submitter)); 542 children.add(new Property("payee", "Reference(Organization)", "The organization which is receiving the payment.", 0, 1, payee)); 543 children.add(new Property("date", "date", "The date of the invoice or financial resource.", 0, 1, date)); 544 children.add(new Property("amount", "Money", "Amount paid for this detail.", 0, 1, amount)); 545 } 546 547 @Override 548 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 549 switch (_hash) { 550 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code to indicate the nature of the payment, adjustment, funds advance, etc.", 0, 1, type); 551 case 1095692943: /*request*/ return new Property("request", "Reference(Any)", "The claim or financial resource.", 0, 1, request); 552 case -340323263: /*response*/ return new Property("response", "Reference(Any)", "The claim response resource.", 0, 1, response); 553 case 348678409: /*submitter*/ return new Property("submitter", "Reference(Organization)", "The Organization which submitted the claim or financial transaction.", 0, 1, submitter); 554 case 106443592: /*payee*/ return new Property("payee", "Reference(Organization)", "The organization which is receiving the payment.", 0, 1, payee); 555 case 3076014: /*date*/ return new Property("date", "date", "The date of the invoice or financial resource.", 0, 1, date); 556 case -1413853096: /*amount*/ return new Property("amount", "Money", "Amount paid for this detail.", 0, 1, amount); 557 default: return super.getNamedProperty(_hash, _name, _checkValid); 558 } 559 560 } 561 562 @Override 563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 564 switch (hash) { 565 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 566 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 567 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 568 case 348678409: /*submitter*/ return this.submitter == null ? new Base[0] : new Base[] {this.submitter}; // Reference 569 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // Reference 570 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 571 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 572 default: return super.getProperty(hash, name, checkValid); 573 } 574 575 } 576 577 @Override 578 public Base setProperty(int hash, String name, Base value) throws FHIRException { 579 switch (hash) { 580 case 3575610: // type 581 this.type = castToCodeableConcept(value); // CodeableConcept 582 return value; 583 case 1095692943: // request 584 this.request = castToReference(value); // Reference 585 return value; 586 case -340323263: // response 587 this.response = castToReference(value); // Reference 588 return value; 589 case 348678409: // submitter 590 this.submitter = castToReference(value); // Reference 591 return value; 592 case 106443592: // payee 593 this.payee = castToReference(value); // Reference 594 return value; 595 case 3076014: // date 596 this.date = castToDate(value); // DateType 597 return value; 598 case -1413853096: // amount 599 this.amount = castToMoney(value); // Money 600 return value; 601 default: return super.setProperty(hash, name, value); 602 } 603 604 } 605 606 @Override 607 public Base setProperty(String name, Base value) throws FHIRException { 608 if (name.equals("type")) { 609 this.type = castToCodeableConcept(value); // CodeableConcept 610 } else if (name.equals("request")) { 611 this.request = castToReference(value); // Reference 612 } else if (name.equals("response")) { 613 this.response = castToReference(value); // Reference 614 } else if (name.equals("submitter")) { 615 this.submitter = castToReference(value); // Reference 616 } else if (name.equals("payee")) { 617 this.payee = castToReference(value); // Reference 618 } else if (name.equals("date")) { 619 this.date = castToDate(value); // DateType 620 } else if (name.equals("amount")) { 621 this.amount = castToMoney(value); // Money 622 } else 623 return super.setProperty(name, value); 624 return value; 625 } 626 627 @Override 628 public Base makeProperty(int hash, String name) throws FHIRException { 629 switch (hash) { 630 case 3575610: return getType(); 631 case 1095692943: return getRequest(); 632 case -340323263: return getResponse(); 633 case 348678409: return getSubmitter(); 634 case 106443592: return getPayee(); 635 case 3076014: return getDateElement(); 636 case -1413853096: return getAmount(); 637 default: return super.makeProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 644 switch (hash) { 645 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 646 case 1095692943: /*request*/ return new String[] {"Reference"}; 647 case -340323263: /*response*/ return new String[] {"Reference"}; 648 case 348678409: /*submitter*/ return new String[] {"Reference"}; 649 case 106443592: /*payee*/ return new String[] {"Reference"}; 650 case 3076014: /*date*/ return new String[] {"date"}; 651 case -1413853096: /*amount*/ return new String[] {"Money"}; 652 default: return super.getTypesForProperty(hash, name); 653 } 654 655 } 656 657 @Override 658 public Base addChild(String name) throws FHIRException { 659 if (name.equals("type")) { 660 this.type = new CodeableConcept(); 661 return this.type; 662 } 663 else if (name.equals("request")) { 664 this.request = new Reference(); 665 return this.request; 666 } 667 else if (name.equals("response")) { 668 this.response = new Reference(); 669 return this.response; 670 } 671 else if (name.equals("submitter")) { 672 this.submitter = new Reference(); 673 return this.submitter; 674 } 675 else if (name.equals("payee")) { 676 this.payee = new Reference(); 677 return this.payee; 678 } 679 else if (name.equals("date")) { 680 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.date"); 681 } 682 else if (name.equals("amount")) { 683 this.amount = new Money(); 684 return this.amount; 685 } 686 else 687 return super.addChild(name); 688 } 689 690 public DetailsComponent copy() { 691 DetailsComponent dst = new DetailsComponent(); 692 copyValues(dst); 693 dst.type = type == null ? null : type.copy(); 694 dst.request = request == null ? null : request.copy(); 695 dst.response = response == null ? null : response.copy(); 696 dst.submitter = submitter == null ? null : submitter.copy(); 697 dst.payee = payee == null ? null : payee.copy(); 698 dst.date = date == null ? null : date.copy(); 699 dst.amount = amount == null ? null : amount.copy(); 700 return dst; 701 } 702 703 @Override 704 public boolean equalsDeep(Base other_) { 705 if (!super.equalsDeep(other_)) 706 return false; 707 if (!(other_ instanceof DetailsComponent)) 708 return false; 709 DetailsComponent o = (DetailsComponent) other_; 710 return compareDeep(type, o.type, true) && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 711 && compareDeep(submitter, o.submitter, true) && compareDeep(payee, o.payee, true) && compareDeep(date, o.date, true) 712 && compareDeep(amount, o.amount, true); 713 } 714 715 @Override 716 public boolean equalsShallow(Base other_) { 717 if (!super.equalsShallow(other_)) 718 return false; 719 if (!(other_ instanceof DetailsComponent)) 720 return false; 721 DetailsComponent o = (DetailsComponent) other_; 722 return compareValues(date, o.date, true); 723 } 724 725 public boolean isEmpty() { 726 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, request, response 727 , submitter, payee, date, amount); 728 } 729 730 public String fhirType() { 731 return "PaymentReconciliation.detail"; 732 733 } 734 735 } 736 737 @Block() 738 public static class NotesComponent extends BackboneElement implements IBaseBackboneElement { 739 /** 740 * The note purpose: Print/Display. 741 */ 742 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 743 @Description(shortDefinition="display | print | printoper", formalDefinition="The note purpose: Print/Display." ) 744 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 745 protected CodeableConcept type; 746 747 /** 748 * The note text. 749 */ 750 @Child(name = "text", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 751 @Description(shortDefinition="Comment on the processing", formalDefinition="The note text." ) 752 protected StringType text; 753 754 private static final long serialVersionUID = 874830709L; 755 756 /** 757 * Constructor 758 */ 759 public NotesComponent() { 760 super(); 761 } 762 763 /** 764 * @return {@link #type} (The note purpose: Print/Display.) 765 */ 766 public CodeableConcept getType() { 767 if (this.type == null) 768 if (Configuration.errorOnAutoCreate()) 769 throw new Error("Attempt to auto-create NotesComponent.type"); 770 else if (Configuration.doAutoCreate()) 771 this.type = new CodeableConcept(); // cc 772 return this.type; 773 } 774 775 public boolean hasType() { 776 return this.type != null && !this.type.isEmpty(); 777 } 778 779 /** 780 * @param value {@link #type} (The note purpose: Print/Display.) 781 */ 782 public NotesComponent setType(CodeableConcept value) { 783 this.type = value; 784 return this; 785 } 786 787 /** 788 * @return {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 789 */ 790 public StringType getTextElement() { 791 if (this.text == null) 792 if (Configuration.errorOnAutoCreate()) 793 throw new Error("Attempt to auto-create NotesComponent.text"); 794 else if (Configuration.doAutoCreate()) 795 this.text = new StringType(); // bb 796 return this.text; 797 } 798 799 public boolean hasTextElement() { 800 return this.text != null && !this.text.isEmpty(); 801 } 802 803 public boolean hasText() { 804 return this.text != null && !this.text.isEmpty(); 805 } 806 807 /** 808 * @param value {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 809 */ 810 public NotesComponent setTextElement(StringType value) { 811 this.text = value; 812 return this; 813 } 814 815 /** 816 * @return The note text. 817 */ 818 public String getText() { 819 return this.text == null ? null : this.text.getValue(); 820 } 821 822 /** 823 * @param value The note text. 824 */ 825 public NotesComponent setText(String value) { 826 if (Utilities.noString(value)) 827 this.text = null; 828 else { 829 if (this.text == null) 830 this.text = new StringType(); 831 this.text.setValue(value); 832 } 833 return this; 834 } 835 836 protected void listChildren(List<Property> children) { 837 super.listChildren(children); 838 children.add(new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type)); 839 children.add(new Property("text", "string", "The note text.", 0, 1, text)); 840 } 841 842 @Override 843 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 844 switch (_hash) { 845 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type); 846 case 3556653: /*text*/ return new Property("text", "string", "The note text.", 0, 1, text); 847 default: return super.getNamedProperty(_hash, _name, _checkValid); 848 } 849 850 } 851 852 @Override 853 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 854 switch (hash) { 855 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 856 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 857 default: return super.getProperty(hash, name, checkValid); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(int hash, String name, Base value) throws FHIRException { 864 switch (hash) { 865 case 3575610: // type 866 this.type = castToCodeableConcept(value); // CodeableConcept 867 return value; 868 case 3556653: // text 869 this.text = castToString(value); // StringType 870 return value; 871 default: return super.setProperty(hash, name, value); 872 } 873 874 } 875 876 @Override 877 public Base setProperty(String name, Base value) throws FHIRException { 878 if (name.equals("type")) { 879 this.type = castToCodeableConcept(value); // CodeableConcept 880 } else if (name.equals("text")) { 881 this.text = castToString(value); // StringType 882 } else 883 return super.setProperty(name, value); 884 return value; 885 } 886 887 @Override 888 public Base makeProperty(int hash, String name) throws FHIRException { 889 switch (hash) { 890 case 3575610: return getType(); 891 case 3556653: return getTextElement(); 892 default: return super.makeProperty(hash, name); 893 } 894 895 } 896 897 @Override 898 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 899 switch (hash) { 900 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 901 case 3556653: /*text*/ return new String[] {"string"}; 902 default: return super.getTypesForProperty(hash, name); 903 } 904 905 } 906 907 @Override 908 public Base addChild(String name) throws FHIRException { 909 if (name.equals("type")) { 910 this.type = new CodeableConcept(); 911 return this.type; 912 } 913 else if (name.equals("text")) { 914 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.text"); 915 } 916 else 917 return super.addChild(name); 918 } 919 920 public NotesComponent copy() { 921 NotesComponent dst = new NotesComponent(); 922 copyValues(dst); 923 dst.type = type == null ? null : type.copy(); 924 dst.text = text == null ? null : text.copy(); 925 return dst; 926 } 927 928 @Override 929 public boolean equalsDeep(Base other_) { 930 if (!super.equalsDeep(other_)) 931 return false; 932 if (!(other_ instanceof NotesComponent)) 933 return false; 934 NotesComponent o = (NotesComponent) other_; 935 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 936 } 937 938 @Override 939 public boolean equalsShallow(Base other_) { 940 if (!super.equalsShallow(other_)) 941 return false; 942 if (!(other_ instanceof NotesComponent)) 943 return false; 944 NotesComponent o = (NotesComponent) other_; 945 return compareValues(text, o.text, true); 946 } 947 948 public boolean isEmpty() { 949 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, text); 950 } 951 952 public String fhirType() { 953 return "PaymentReconciliation.processNote"; 954 955 } 956 957 } 958 959 /** 960 * The Response business identifier. 961 */ 962 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 963 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 964 protected List<Identifier> identifier; 965 966 /** 967 * The status of the resource instance. 968 */ 969 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 970 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 972 protected Enumeration<PaymentReconciliationStatus> status; 973 974 /** 975 * The period of time for which payments have been gathered into this bulk payment for settlement. 976 */ 977 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 978 @Description(shortDefinition="Period covered", formalDefinition="The period of time for which payments have been gathered into this bulk payment for settlement." ) 979 protected Period period; 980 981 /** 982 * The date when the enclosed suite of services were performed or completed. 983 */ 984 @Child(name = "created", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 985 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 986 protected DateTimeType created; 987 988 /** 989 * The Insurer who produced this adjudicated response. 990 */ 991 @Child(name = "organization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 992 @Description(shortDefinition="Insurer", formalDefinition="The Insurer who produced this adjudicated response." ) 993 protected Reference organization; 994 995 /** 996 * The actual object that is the target of the reference (The Insurer who produced this adjudicated response.) 997 */ 998 protected Organization organizationTarget; 999 1000 /** 1001 * Original request resource reference. 1002 */ 1003 @Child(name = "request", type = {ProcessRequest.class}, order=5, min=0, max=1, modifier=false, summary=false) 1004 @Description(shortDefinition="Claim reference", formalDefinition="Original request resource reference." ) 1005 protected Reference request; 1006 1007 /** 1008 * The actual object that is the target of the reference (Original request resource reference.) 1009 */ 1010 protected ProcessRequest requestTarget; 1011 1012 /** 1013 * Transaction status: error, complete. 1014 */ 1015 @Child(name = "outcome", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1016 @Description(shortDefinition="complete | error | partial", formalDefinition="Transaction status: error, complete." ) 1017 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 1018 protected CodeableConcept outcome; 1019 1020 /** 1021 * A description of the status of the adjudication. 1022 */ 1023 @Child(name = "disposition", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1024 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 1025 protected StringType disposition; 1026 1027 /** 1028 * The practitioner who is responsible for the services rendered to the patient. 1029 */ 1030 @Child(name = "requestProvider", type = {Practitioner.class}, order=8, min=0, max=1, modifier=false, summary=false) 1031 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 1032 protected Reference requestProvider; 1033 1034 /** 1035 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 1036 */ 1037 protected Practitioner requestProviderTarget; 1038 1039 /** 1040 * The organization which is responsible for the services rendered to the patient. 1041 */ 1042 @Child(name = "requestOrganization", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 1043 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 1044 protected Reference requestOrganization; 1045 1046 /** 1047 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 1048 */ 1049 protected Organization requestOrganizationTarget; 1050 1051 /** 1052 * List of individual settlement amounts and the corresponding transaction. 1053 */ 1054 @Child(name = "detail", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1055 @Description(shortDefinition="List of settlements", formalDefinition="List of individual settlement amounts and the corresponding transaction." ) 1056 protected List<DetailsComponent> detail; 1057 1058 /** 1059 * The form to be used for printing the content. 1060 */ 1061 @Child(name = "form", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 1062 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 1063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 1064 protected CodeableConcept form; 1065 1066 /** 1067 * Total payment amount. 1068 */ 1069 @Child(name = "total", type = {Money.class}, order=12, min=0, max=1, modifier=false, summary=false) 1070 @Description(shortDefinition="Total amount of Payment", formalDefinition="Total payment amount." ) 1071 protected Money total; 1072 1073 /** 1074 * Suite of notes. 1075 */ 1076 @Child(name = "processNote", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1077 @Description(shortDefinition="Processing comments", formalDefinition="Suite of notes." ) 1078 protected List<NotesComponent> processNote; 1079 1080 private static final long serialVersionUID = -665475468L; 1081 1082 /** 1083 * Constructor 1084 */ 1085 public PaymentReconciliation() { 1086 super(); 1087 } 1088 1089 /** 1090 * @return {@link #identifier} (The Response business identifier.) 1091 */ 1092 public List<Identifier> getIdentifier() { 1093 if (this.identifier == null) 1094 this.identifier = new ArrayList<Identifier>(); 1095 return this.identifier; 1096 } 1097 1098 /** 1099 * @return Returns a reference to <code>this</code> for easy method chaining 1100 */ 1101 public PaymentReconciliation setIdentifier(List<Identifier> theIdentifier) { 1102 this.identifier = theIdentifier; 1103 return this; 1104 } 1105 1106 public boolean hasIdentifier() { 1107 if (this.identifier == null) 1108 return false; 1109 for (Identifier item : this.identifier) 1110 if (!item.isEmpty()) 1111 return true; 1112 return false; 1113 } 1114 1115 public Identifier addIdentifier() { //3 1116 Identifier t = new Identifier(); 1117 if (this.identifier == null) 1118 this.identifier = new ArrayList<Identifier>(); 1119 this.identifier.add(t); 1120 return t; 1121 } 1122 1123 public PaymentReconciliation addIdentifier(Identifier t) { //3 1124 if (t == null) 1125 return this; 1126 if (this.identifier == null) 1127 this.identifier = new ArrayList<Identifier>(); 1128 this.identifier.add(t); 1129 return this; 1130 } 1131 1132 /** 1133 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1134 */ 1135 public Identifier getIdentifierFirstRep() { 1136 if (getIdentifier().isEmpty()) { 1137 addIdentifier(); 1138 } 1139 return getIdentifier().get(0); 1140 } 1141 1142 /** 1143 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1144 */ 1145 public Enumeration<PaymentReconciliationStatus> getStatusElement() { 1146 if (this.status == null) 1147 if (Configuration.errorOnAutoCreate()) 1148 throw new Error("Attempt to auto-create PaymentReconciliation.status"); 1149 else if (Configuration.doAutoCreate()) 1150 this.status = new Enumeration<PaymentReconciliationStatus>(new PaymentReconciliationStatusEnumFactory()); // bb 1151 return this.status; 1152 } 1153 1154 public boolean hasStatusElement() { 1155 return this.status != null && !this.status.isEmpty(); 1156 } 1157 1158 public boolean hasStatus() { 1159 return this.status != null && !this.status.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1164 */ 1165 public PaymentReconciliation setStatusElement(Enumeration<PaymentReconciliationStatus> value) { 1166 this.status = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return The status of the resource instance. 1172 */ 1173 public PaymentReconciliationStatus getStatus() { 1174 return this.status == null ? null : this.status.getValue(); 1175 } 1176 1177 /** 1178 * @param value The status of the resource instance. 1179 */ 1180 public PaymentReconciliation setStatus(PaymentReconciliationStatus value) { 1181 if (value == null) 1182 this.status = null; 1183 else { 1184 if (this.status == null) 1185 this.status = new Enumeration<PaymentReconciliationStatus>(new PaymentReconciliationStatusEnumFactory()); 1186 this.status.setValue(value); 1187 } 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #period} (The period of time for which payments have been gathered into this bulk payment for settlement.) 1193 */ 1194 public Period getPeriod() { 1195 if (this.period == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create PaymentReconciliation.period"); 1198 else if (Configuration.doAutoCreate()) 1199 this.period = new Period(); // cc 1200 return this.period; 1201 } 1202 1203 public boolean hasPeriod() { 1204 return this.period != null && !this.period.isEmpty(); 1205 } 1206 1207 /** 1208 * @param value {@link #period} (The period of time for which payments have been gathered into this bulk payment for settlement.) 1209 */ 1210 public PaymentReconciliation setPeriod(Period value) { 1211 this.period = value; 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1217 */ 1218 public DateTimeType getCreatedElement() { 1219 if (this.created == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create PaymentReconciliation.created"); 1222 else if (Configuration.doAutoCreate()) 1223 this.created = new DateTimeType(); // bb 1224 return this.created; 1225 } 1226 1227 public boolean hasCreatedElement() { 1228 return this.created != null && !this.created.isEmpty(); 1229 } 1230 1231 public boolean hasCreated() { 1232 return this.created != null && !this.created.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1237 */ 1238 public PaymentReconciliation setCreatedElement(DateTimeType value) { 1239 this.created = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return The date when the enclosed suite of services were performed or completed. 1245 */ 1246 public Date getCreated() { 1247 return this.created == null ? null : this.created.getValue(); 1248 } 1249 1250 /** 1251 * @param value The date when the enclosed suite of services were performed or completed. 1252 */ 1253 public PaymentReconciliation setCreated(Date value) { 1254 if (value == null) 1255 this.created = null; 1256 else { 1257 if (this.created == null) 1258 this.created = new DateTimeType(); 1259 this.created.setValue(value); 1260 } 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #organization} (The Insurer who produced this adjudicated response.) 1266 */ 1267 public Reference getOrganization() { 1268 if (this.organization == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create PaymentReconciliation.organization"); 1271 else if (Configuration.doAutoCreate()) 1272 this.organization = new Reference(); // cc 1273 return this.organization; 1274 } 1275 1276 public boolean hasOrganization() { 1277 return this.organization != null && !this.organization.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #organization} (The Insurer who produced this adjudicated response.) 1282 */ 1283 public PaymentReconciliation setOrganization(Reference value) { 1284 this.organization = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 1290 */ 1291 public Organization getOrganizationTarget() { 1292 if (this.organizationTarget == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create PaymentReconciliation.organization"); 1295 else if (Configuration.doAutoCreate()) 1296 this.organizationTarget = new Organization(); // aa 1297 return this.organizationTarget; 1298 } 1299 1300 /** 1301 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 1302 */ 1303 public PaymentReconciliation setOrganizationTarget(Organization value) { 1304 this.organizationTarget = value; 1305 return this; 1306 } 1307 1308 /** 1309 * @return {@link #request} (Original request resource reference.) 1310 */ 1311 public Reference getRequest() { 1312 if (this.request == null) 1313 if (Configuration.errorOnAutoCreate()) 1314 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 1315 else if (Configuration.doAutoCreate()) 1316 this.request = new Reference(); // cc 1317 return this.request; 1318 } 1319 1320 public boolean hasRequest() { 1321 return this.request != null && !this.request.isEmpty(); 1322 } 1323 1324 /** 1325 * @param value {@link #request} (Original request resource reference.) 1326 */ 1327 public PaymentReconciliation setRequest(Reference value) { 1328 this.request = value; 1329 return this; 1330 } 1331 1332 /** 1333 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 1334 */ 1335 public ProcessRequest getRequestTarget() { 1336 if (this.requestTarget == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 1339 else if (Configuration.doAutoCreate()) 1340 this.requestTarget = new ProcessRequest(); // aa 1341 return this.requestTarget; 1342 } 1343 1344 /** 1345 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 1346 */ 1347 public PaymentReconciliation setRequestTarget(ProcessRequest value) { 1348 this.requestTarget = value; 1349 return this; 1350 } 1351 1352 /** 1353 * @return {@link #outcome} (Transaction status: error, complete.) 1354 */ 1355 public CodeableConcept getOutcome() { 1356 if (this.outcome == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create PaymentReconciliation.outcome"); 1359 else if (Configuration.doAutoCreate()) 1360 this.outcome = new CodeableConcept(); // cc 1361 return this.outcome; 1362 } 1363 1364 public boolean hasOutcome() { 1365 return this.outcome != null && !this.outcome.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #outcome} (Transaction status: error, complete.) 1370 */ 1371 public PaymentReconciliation setOutcome(CodeableConcept value) { 1372 this.outcome = value; 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 1378 */ 1379 public StringType getDispositionElement() { 1380 if (this.disposition == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create PaymentReconciliation.disposition"); 1383 else if (Configuration.doAutoCreate()) 1384 this.disposition = new StringType(); // bb 1385 return this.disposition; 1386 } 1387 1388 public boolean hasDispositionElement() { 1389 return this.disposition != null && !this.disposition.isEmpty(); 1390 } 1391 1392 public boolean hasDisposition() { 1393 return this.disposition != null && !this.disposition.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 1398 */ 1399 public PaymentReconciliation setDispositionElement(StringType value) { 1400 this.disposition = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return A description of the status of the adjudication. 1406 */ 1407 public String getDisposition() { 1408 return this.disposition == null ? null : this.disposition.getValue(); 1409 } 1410 1411 /** 1412 * @param value A description of the status of the adjudication. 1413 */ 1414 public PaymentReconciliation setDisposition(String value) { 1415 if (Utilities.noString(value)) 1416 this.disposition = null; 1417 else { 1418 if (this.disposition == null) 1419 this.disposition = new StringType(); 1420 this.disposition.setValue(value); 1421 } 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 1427 */ 1428 public Reference getRequestProvider() { 1429 if (this.requestProvider == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create PaymentReconciliation.requestProvider"); 1432 else if (Configuration.doAutoCreate()) 1433 this.requestProvider = new Reference(); // cc 1434 return this.requestProvider; 1435 } 1436 1437 public boolean hasRequestProvider() { 1438 return this.requestProvider != null && !this.requestProvider.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 1443 */ 1444 public PaymentReconciliation setRequestProvider(Reference value) { 1445 this.requestProvider = value; 1446 return this; 1447 } 1448 1449 /** 1450 * @return {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 1451 */ 1452 public Practitioner getRequestProviderTarget() { 1453 if (this.requestProviderTarget == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create PaymentReconciliation.requestProvider"); 1456 else if (Configuration.doAutoCreate()) 1457 this.requestProviderTarget = new Practitioner(); // aa 1458 return this.requestProviderTarget; 1459 } 1460 1461 /** 1462 * @param value {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 1463 */ 1464 public PaymentReconciliation setRequestProviderTarget(Practitioner value) { 1465 this.requestProviderTarget = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 1471 */ 1472 public Reference getRequestOrganization() { 1473 if (this.requestOrganization == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create PaymentReconciliation.requestOrganization"); 1476 else if (Configuration.doAutoCreate()) 1477 this.requestOrganization = new Reference(); // cc 1478 return this.requestOrganization; 1479 } 1480 1481 public boolean hasRequestOrganization() { 1482 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 1483 } 1484 1485 /** 1486 * @param value {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 1487 */ 1488 public PaymentReconciliation setRequestOrganization(Reference value) { 1489 this.requestOrganization = value; 1490 return this; 1491 } 1492 1493 /** 1494 * @return {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 1495 */ 1496 public Organization getRequestOrganizationTarget() { 1497 if (this.requestOrganizationTarget == null) 1498 if (Configuration.errorOnAutoCreate()) 1499 throw new Error("Attempt to auto-create PaymentReconciliation.requestOrganization"); 1500 else if (Configuration.doAutoCreate()) 1501 this.requestOrganizationTarget = new Organization(); // aa 1502 return this.requestOrganizationTarget; 1503 } 1504 1505 /** 1506 * @param value {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 1507 */ 1508 public PaymentReconciliation setRequestOrganizationTarget(Organization value) { 1509 this.requestOrganizationTarget = value; 1510 return this; 1511 } 1512 1513 /** 1514 * @return {@link #detail} (List of individual settlement amounts and the corresponding transaction.) 1515 */ 1516 public List<DetailsComponent> getDetail() { 1517 if (this.detail == null) 1518 this.detail = new ArrayList<DetailsComponent>(); 1519 return this.detail; 1520 } 1521 1522 /** 1523 * @return Returns a reference to <code>this</code> for easy method chaining 1524 */ 1525 public PaymentReconciliation setDetail(List<DetailsComponent> theDetail) { 1526 this.detail = theDetail; 1527 return this; 1528 } 1529 1530 public boolean hasDetail() { 1531 if (this.detail == null) 1532 return false; 1533 for (DetailsComponent item : this.detail) 1534 if (!item.isEmpty()) 1535 return true; 1536 return false; 1537 } 1538 1539 public DetailsComponent addDetail() { //3 1540 DetailsComponent t = new DetailsComponent(); 1541 if (this.detail == null) 1542 this.detail = new ArrayList<DetailsComponent>(); 1543 this.detail.add(t); 1544 return t; 1545 } 1546 1547 public PaymentReconciliation addDetail(DetailsComponent t) { //3 1548 if (t == null) 1549 return this; 1550 if (this.detail == null) 1551 this.detail = new ArrayList<DetailsComponent>(); 1552 this.detail.add(t); 1553 return this; 1554 } 1555 1556 /** 1557 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 1558 */ 1559 public DetailsComponent getDetailFirstRep() { 1560 if (getDetail().isEmpty()) { 1561 addDetail(); 1562 } 1563 return getDetail().get(0); 1564 } 1565 1566 /** 1567 * @return {@link #form} (The form to be used for printing the content.) 1568 */ 1569 public CodeableConcept getForm() { 1570 if (this.form == null) 1571 if (Configuration.errorOnAutoCreate()) 1572 throw new Error("Attempt to auto-create PaymentReconciliation.form"); 1573 else if (Configuration.doAutoCreate()) 1574 this.form = new CodeableConcept(); // cc 1575 return this.form; 1576 } 1577 1578 public boolean hasForm() { 1579 return this.form != null && !this.form.isEmpty(); 1580 } 1581 1582 /** 1583 * @param value {@link #form} (The form to be used for printing the content.) 1584 */ 1585 public PaymentReconciliation setForm(CodeableConcept value) { 1586 this.form = value; 1587 return this; 1588 } 1589 1590 /** 1591 * @return {@link #total} (Total payment amount.) 1592 */ 1593 public Money getTotal() { 1594 if (this.total == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create PaymentReconciliation.total"); 1597 else if (Configuration.doAutoCreate()) 1598 this.total = new Money(); // cc 1599 return this.total; 1600 } 1601 1602 public boolean hasTotal() { 1603 return this.total != null && !this.total.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #total} (Total payment amount.) 1608 */ 1609 public PaymentReconciliation setTotal(Money value) { 1610 this.total = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return {@link #processNote} (Suite of notes.) 1616 */ 1617 public List<NotesComponent> getProcessNote() { 1618 if (this.processNote == null) 1619 this.processNote = new ArrayList<NotesComponent>(); 1620 return this.processNote; 1621 } 1622 1623 /** 1624 * @return Returns a reference to <code>this</code> for easy method chaining 1625 */ 1626 public PaymentReconciliation setProcessNote(List<NotesComponent> theProcessNote) { 1627 this.processNote = theProcessNote; 1628 return this; 1629 } 1630 1631 public boolean hasProcessNote() { 1632 if (this.processNote == null) 1633 return false; 1634 for (NotesComponent item : this.processNote) 1635 if (!item.isEmpty()) 1636 return true; 1637 return false; 1638 } 1639 1640 public NotesComponent addProcessNote() { //3 1641 NotesComponent t = new NotesComponent(); 1642 if (this.processNote == null) 1643 this.processNote = new ArrayList<NotesComponent>(); 1644 this.processNote.add(t); 1645 return t; 1646 } 1647 1648 public PaymentReconciliation addProcessNote(NotesComponent t) { //3 1649 if (t == null) 1650 return this; 1651 if (this.processNote == null) 1652 this.processNote = new ArrayList<NotesComponent>(); 1653 this.processNote.add(t); 1654 return this; 1655 } 1656 1657 /** 1658 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist 1659 */ 1660 public NotesComponent getProcessNoteFirstRep() { 1661 if (getProcessNote().isEmpty()) { 1662 addProcessNote(); 1663 } 1664 return getProcessNote().get(0); 1665 } 1666 1667 protected void listChildren(List<Property> children) { 1668 super.listChildren(children); 1669 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1670 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 1671 children.add(new Property("period", "Period", "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, period)); 1672 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 1673 children.add(new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization)); 1674 children.add(new Property("request", "Reference(ProcessRequest)", "Original request resource reference.", 0, 1, request)); 1675 children.add(new Property("outcome", "CodeableConcept", "Transaction status: error, complete.", 0, 1, outcome)); 1676 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 1677 children.add(new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 1678 children.add(new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization)); 1679 children.add(new Property("detail", "", "List of individual settlement amounts and the corresponding transaction.", 0, java.lang.Integer.MAX_VALUE, detail)); 1680 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 1681 children.add(new Property("total", "Money", "Total payment amount.", 0, 1, total)); 1682 children.add(new Property("processNote", "", "Suite of notes.", 0, java.lang.Integer.MAX_VALUE, processNote)); 1683 } 1684 1685 @Override 1686 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1687 switch (_hash) { 1688 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1689 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 1690 case -991726143: /*period*/ return new Property("period", "Period", "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1, period); 1691 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 1692 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization); 1693 case 1095692943: /*request*/ return new Property("request", "Reference(ProcessRequest)", "Original request resource reference.", 0, 1, request); 1694 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Transaction status: error, complete.", 0, 1, outcome); 1695 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 1696 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 1697 case 599053666: /*requestOrganization*/ return new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization); 1698 case -1335224239: /*detail*/ return new Property("detail", "", "List of individual settlement amounts and the corresponding transaction.", 0, java.lang.Integer.MAX_VALUE, detail); 1699 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 1700 case 110549828: /*total*/ return new Property("total", "Money", "Total payment amount.", 0, 1, total); 1701 case 202339073: /*processNote*/ return new Property("processNote", "", "Suite of notes.", 0, java.lang.Integer.MAX_VALUE, processNote); 1702 default: return super.getNamedProperty(_hash, _name, _checkValid); 1703 } 1704 1705 } 1706 1707 @Override 1708 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1709 switch (hash) { 1710 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1711 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PaymentReconciliationStatus> 1712 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1713 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1714 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1715 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 1716 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 1717 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 1718 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 1719 case 599053666: /*requestOrganization*/ return this.requestOrganization == null ? new Base[0] : new Base[] {this.requestOrganization}; // Reference 1720 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailsComponent 1721 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 1722 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // Money 1723 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NotesComponent 1724 default: return super.getProperty(hash, name, checkValid); 1725 } 1726 1727 } 1728 1729 @Override 1730 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1731 switch (hash) { 1732 case -1618432855: // identifier 1733 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1734 return value; 1735 case -892481550: // status 1736 value = new PaymentReconciliationStatusEnumFactory().fromType(castToCode(value)); 1737 this.status = (Enumeration) value; // Enumeration<PaymentReconciliationStatus> 1738 return value; 1739 case -991726143: // period 1740 this.period = castToPeriod(value); // Period 1741 return value; 1742 case 1028554472: // created 1743 this.created = castToDateTime(value); // DateTimeType 1744 return value; 1745 case 1178922291: // organization 1746 this.organization = castToReference(value); // Reference 1747 return value; 1748 case 1095692943: // request 1749 this.request = castToReference(value); // Reference 1750 return value; 1751 case -1106507950: // outcome 1752 this.outcome = castToCodeableConcept(value); // CodeableConcept 1753 return value; 1754 case 583380919: // disposition 1755 this.disposition = castToString(value); // StringType 1756 return value; 1757 case 1601527200: // requestProvider 1758 this.requestProvider = castToReference(value); // Reference 1759 return value; 1760 case 599053666: // requestOrganization 1761 this.requestOrganization = castToReference(value); // Reference 1762 return value; 1763 case -1335224239: // detail 1764 this.getDetail().add((DetailsComponent) value); // DetailsComponent 1765 return value; 1766 case 3148996: // form 1767 this.form = castToCodeableConcept(value); // CodeableConcept 1768 return value; 1769 case 110549828: // total 1770 this.total = castToMoney(value); // Money 1771 return value; 1772 case 202339073: // processNote 1773 this.getProcessNote().add((NotesComponent) value); // NotesComponent 1774 return value; 1775 default: return super.setProperty(hash, name, value); 1776 } 1777 1778 } 1779 1780 @Override 1781 public Base setProperty(String name, Base value) throws FHIRException { 1782 if (name.equals("identifier")) { 1783 this.getIdentifier().add(castToIdentifier(value)); 1784 } else if (name.equals("status")) { 1785 value = new PaymentReconciliationStatusEnumFactory().fromType(castToCode(value)); 1786 this.status = (Enumeration) value; // Enumeration<PaymentReconciliationStatus> 1787 } else if (name.equals("period")) { 1788 this.period = castToPeriod(value); // Period 1789 } else if (name.equals("created")) { 1790 this.created = castToDateTime(value); // DateTimeType 1791 } else if (name.equals("organization")) { 1792 this.organization = castToReference(value); // Reference 1793 } else if (name.equals("request")) { 1794 this.request = castToReference(value); // Reference 1795 } else if (name.equals("outcome")) { 1796 this.outcome = castToCodeableConcept(value); // CodeableConcept 1797 } else if (name.equals("disposition")) { 1798 this.disposition = castToString(value); // StringType 1799 } else if (name.equals("requestProvider")) { 1800 this.requestProvider = castToReference(value); // Reference 1801 } else if (name.equals("requestOrganization")) { 1802 this.requestOrganization = castToReference(value); // Reference 1803 } else if (name.equals("detail")) { 1804 this.getDetail().add((DetailsComponent) value); 1805 } else if (name.equals("form")) { 1806 this.form = castToCodeableConcept(value); // CodeableConcept 1807 } else if (name.equals("total")) { 1808 this.total = castToMoney(value); // Money 1809 } else if (name.equals("processNote")) { 1810 this.getProcessNote().add((NotesComponent) value); 1811 } else 1812 return super.setProperty(name, value); 1813 return value; 1814 } 1815 1816 @Override 1817 public Base makeProperty(int hash, String name) throws FHIRException { 1818 switch (hash) { 1819 case -1618432855: return addIdentifier(); 1820 case -892481550: return getStatusElement(); 1821 case -991726143: return getPeriod(); 1822 case 1028554472: return getCreatedElement(); 1823 case 1178922291: return getOrganization(); 1824 case 1095692943: return getRequest(); 1825 case -1106507950: return getOutcome(); 1826 case 583380919: return getDispositionElement(); 1827 case 1601527200: return getRequestProvider(); 1828 case 599053666: return getRequestOrganization(); 1829 case -1335224239: return addDetail(); 1830 case 3148996: return getForm(); 1831 case 110549828: return getTotal(); 1832 case 202339073: return addProcessNote(); 1833 default: return super.makeProperty(hash, name); 1834 } 1835 1836 } 1837 1838 @Override 1839 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1840 switch (hash) { 1841 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1842 case -892481550: /*status*/ return new String[] {"code"}; 1843 case -991726143: /*period*/ return new String[] {"Period"}; 1844 case 1028554472: /*created*/ return new String[] {"dateTime"}; 1845 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1846 case 1095692943: /*request*/ return new String[] {"Reference"}; 1847 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 1848 case 583380919: /*disposition*/ return new String[] {"string"}; 1849 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 1850 case 599053666: /*requestOrganization*/ return new String[] {"Reference"}; 1851 case -1335224239: /*detail*/ return new String[] {}; 1852 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 1853 case 110549828: /*total*/ return new String[] {"Money"}; 1854 case 202339073: /*processNote*/ return new String[] {}; 1855 default: return super.getTypesForProperty(hash, name); 1856 } 1857 1858 } 1859 1860 @Override 1861 public Base addChild(String name) throws FHIRException { 1862 if (name.equals("identifier")) { 1863 return addIdentifier(); 1864 } 1865 else if (name.equals("status")) { 1866 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.status"); 1867 } 1868 else if (name.equals("period")) { 1869 this.period = new Period(); 1870 return this.period; 1871 } 1872 else if (name.equals("created")) { 1873 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.created"); 1874 } 1875 else if (name.equals("organization")) { 1876 this.organization = new Reference(); 1877 return this.organization; 1878 } 1879 else if (name.equals("request")) { 1880 this.request = new Reference(); 1881 return this.request; 1882 } 1883 else if (name.equals("outcome")) { 1884 this.outcome = new CodeableConcept(); 1885 return this.outcome; 1886 } 1887 else if (name.equals("disposition")) { 1888 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.disposition"); 1889 } 1890 else if (name.equals("requestProvider")) { 1891 this.requestProvider = new Reference(); 1892 return this.requestProvider; 1893 } 1894 else if (name.equals("requestOrganization")) { 1895 this.requestOrganization = new Reference(); 1896 return this.requestOrganization; 1897 } 1898 else if (name.equals("detail")) { 1899 return addDetail(); 1900 } 1901 else if (name.equals("form")) { 1902 this.form = new CodeableConcept(); 1903 return this.form; 1904 } 1905 else if (name.equals("total")) { 1906 this.total = new Money(); 1907 return this.total; 1908 } 1909 else if (name.equals("processNote")) { 1910 return addProcessNote(); 1911 } 1912 else 1913 return super.addChild(name); 1914 } 1915 1916 public String fhirType() { 1917 return "PaymentReconciliation"; 1918 1919 } 1920 1921 public PaymentReconciliation copy() { 1922 PaymentReconciliation dst = new PaymentReconciliation(); 1923 copyValues(dst); 1924 if (identifier != null) { 1925 dst.identifier = new ArrayList<Identifier>(); 1926 for (Identifier i : identifier) 1927 dst.identifier.add(i.copy()); 1928 }; 1929 dst.status = status == null ? null : status.copy(); 1930 dst.period = period == null ? null : period.copy(); 1931 dst.created = created == null ? null : created.copy(); 1932 dst.organization = organization == null ? null : organization.copy(); 1933 dst.request = request == null ? null : request.copy(); 1934 dst.outcome = outcome == null ? null : outcome.copy(); 1935 dst.disposition = disposition == null ? null : disposition.copy(); 1936 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 1937 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 1938 if (detail != null) { 1939 dst.detail = new ArrayList<DetailsComponent>(); 1940 for (DetailsComponent i : detail) 1941 dst.detail.add(i.copy()); 1942 }; 1943 dst.form = form == null ? null : form.copy(); 1944 dst.total = total == null ? null : total.copy(); 1945 if (processNote != null) { 1946 dst.processNote = new ArrayList<NotesComponent>(); 1947 for (NotesComponent i : processNote) 1948 dst.processNote.add(i.copy()); 1949 }; 1950 return dst; 1951 } 1952 1953 protected PaymentReconciliation typedCopy() { 1954 return copy(); 1955 } 1956 1957 @Override 1958 public boolean equalsDeep(Base other_) { 1959 if (!super.equalsDeep(other_)) 1960 return false; 1961 if (!(other_ instanceof PaymentReconciliation)) 1962 return false; 1963 PaymentReconciliation o = (PaymentReconciliation) other_; 1964 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(period, o.period, true) 1965 && compareDeep(created, o.created, true) && compareDeep(organization, o.organization, true) && compareDeep(request, o.request, true) 1966 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(requestProvider, o.requestProvider, true) 1967 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(detail, o.detail, true) 1968 && compareDeep(form, o.form, true) && compareDeep(total, o.total, true) && compareDeep(processNote, o.processNote, true) 1969 ; 1970 } 1971 1972 @Override 1973 public boolean equalsShallow(Base other_) { 1974 if (!super.equalsShallow(other_)) 1975 return false; 1976 if (!(other_ instanceof PaymentReconciliation)) 1977 return false; 1978 PaymentReconciliation o = (PaymentReconciliation) other_; 1979 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 1980 ; 1981 } 1982 1983 public boolean isEmpty() { 1984 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, period 1985 , created, organization, request, outcome, disposition, requestProvider, requestOrganization 1986 , detail, form, total, processNote); 1987 } 1988 1989 @Override 1990 public ResourceType getResourceType() { 1991 return ResourceType.PaymentReconciliation; 1992 } 1993 1994 /** 1995 * Search parameter: <b>identifier</b> 1996 * <p> 1997 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 1998 * Type: <b>token</b><br> 1999 * Path: <b>PaymentReconciliation.identifier</b><br> 2000 * </p> 2001 */ 2002 @SearchParamDefinition(name="identifier", path="PaymentReconciliation.identifier", description="The business identifier of the Explanation of Benefit", type="token" ) 2003 public static final String SP_IDENTIFIER = "identifier"; 2004 /** 2005 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2006 * <p> 2007 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 2008 * Type: <b>token</b><br> 2009 * Path: <b>PaymentReconciliation.identifier</b><br> 2010 * </p> 2011 */ 2012 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2013 2014 /** 2015 * Search parameter: <b>request</b> 2016 * <p> 2017 * Description: <b>The reference to the claim</b><br> 2018 * Type: <b>reference</b><br> 2019 * Path: <b>PaymentReconciliation.request</b><br> 2020 * </p> 2021 */ 2022 @SearchParamDefinition(name="request", path="PaymentReconciliation.request", description="The reference to the claim", type="reference", target={ProcessRequest.class } ) 2023 public static final String SP_REQUEST = "request"; 2024 /** 2025 * <b>Fluent Client</b> search parameter constant for <b>request</b> 2026 * <p> 2027 * Description: <b>The reference to the claim</b><br> 2028 * Type: <b>reference</b><br> 2029 * Path: <b>PaymentReconciliation.request</b><br> 2030 * </p> 2031 */ 2032 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 2033 2034/** 2035 * Constant for fluent queries to be used to add include statements. Specifies 2036 * the path value of "<b>PaymentReconciliation:request</b>". 2037 */ 2038 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:request").toLocked(); 2039 2040 /** 2041 * Search parameter: <b>disposition</b> 2042 * <p> 2043 * Description: <b>The contents of the disposition message</b><br> 2044 * Type: <b>string</b><br> 2045 * Path: <b>PaymentReconciliation.disposition</b><br> 2046 * </p> 2047 */ 2048 @SearchParamDefinition(name="disposition", path="PaymentReconciliation.disposition", description="The contents of the disposition message", type="string" ) 2049 public static final String SP_DISPOSITION = "disposition"; 2050 /** 2051 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 2052 * <p> 2053 * Description: <b>The contents of the disposition message</b><br> 2054 * Type: <b>string</b><br> 2055 * Path: <b>PaymentReconciliation.disposition</b><br> 2056 * </p> 2057 */ 2058 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 2059 2060 /** 2061 * Search parameter: <b>created</b> 2062 * <p> 2063 * Description: <b>The creation date</b><br> 2064 * Type: <b>date</b><br> 2065 * Path: <b>PaymentReconciliation.created</b><br> 2066 * </p> 2067 */ 2068 @SearchParamDefinition(name="created", path="PaymentReconciliation.created", description="The creation date", type="date" ) 2069 public static final String SP_CREATED = "created"; 2070 /** 2071 * <b>Fluent Client</b> search parameter constant for <b>created</b> 2072 * <p> 2073 * Description: <b>The creation date</b><br> 2074 * Type: <b>date</b><br> 2075 * Path: <b>PaymentReconciliation.created</b><br> 2076 * </p> 2077 */ 2078 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 2079 2080 /** 2081 * Search parameter: <b>organization</b> 2082 * <p> 2083 * Description: <b>The organization who generated this resource</b><br> 2084 * Type: <b>reference</b><br> 2085 * Path: <b>PaymentReconciliation.organization</b><br> 2086 * </p> 2087 */ 2088 @SearchParamDefinition(name="organization", path="PaymentReconciliation.organization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 2089 public static final String SP_ORGANIZATION = "organization"; 2090 /** 2091 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2092 * <p> 2093 * Description: <b>The organization who generated this resource</b><br> 2094 * Type: <b>reference</b><br> 2095 * Path: <b>PaymentReconciliation.organization</b><br> 2096 * </p> 2097 */ 2098 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2099 2100/** 2101 * Constant for fluent queries to be used to add include statements. Specifies 2102 * the path value of "<b>PaymentReconciliation:organization</b>". 2103 */ 2104 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:organization").toLocked(); 2105 2106 /** 2107 * Search parameter: <b>request-organization</b> 2108 * <p> 2109 * Description: <b>The organization who generated this resource</b><br> 2110 * Type: <b>reference</b><br> 2111 * Path: <b>PaymentReconciliation.requestOrganization</b><br> 2112 * </p> 2113 */ 2114 @SearchParamDefinition(name="request-organization", path="PaymentReconciliation.requestOrganization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 2115 public static final String SP_REQUEST_ORGANIZATION = "request-organization"; 2116 /** 2117 * <b>Fluent Client</b> search parameter constant for <b>request-organization</b> 2118 * <p> 2119 * Description: <b>The organization who generated this resource</b><br> 2120 * Type: <b>reference</b><br> 2121 * Path: <b>PaymentReconciliation.requestOrganization</b><br> 2122 * </p> 2123 */ 2124 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_ORGANIZATION); 2125 2126/** 2127 * Constant for fluent queries to be used to add include statements. Specifies 2128 * the path value of "<b>PaymentReconciliation:request-organization</b>". 2129 */ 2130 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:request-organization").toLocked(); 2131 2132 /** 2133 * Search parameter: <b>request-provider</b> 2134 * <p> 2135 * Description: <b>The reference to the provider who sumbitted the claim</b><br> 2136 * Type: <b>reference</b><br> 2137 * Path: <b>PaymentReconciliation.requestProvider</b><br> 2138 * </p> 2139 */ 2140 @SearchParamDefinition(name="request-provider", path="PaymentReconciliation.requestProvider", description="The reference to the provider who sumbitted the claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2141 public static final String SP_REQUEST_PROVIDER = "request-provider"; 2142 /** 2143 * <b>Fluent Client</b> search parameter constant for <b>request-provider</b> 2144 * <p> 2145 * Description: <b>The reference to the provider who sumbitted the claim</b><br> 2146 * Type: <b>reference</b><br> 2147 * Path: <b>PaymentReconciliation.requestProvider</b><br> 2148 * </p> 2149 */ 2150 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_PROVIDER); 2151 2152/** 2153 * Constant for fluent queries to be used to add include statements. Specifies 2154 * the path value of "<b>PaymentReconciliation:request-provider</b>". 2155 */ 2156 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_PROVIDER = new ca.uhn.fhir.model.api.Include("PaymentReconciliation:request-provider").toLocked(); 2157 2158 /** 2159 * Search parameter: <b>outcome</b> 2160 * <p> 2161 * Description: <b>The processing outcome</b><br> 2162 * Type: <b>token</b><br> 2163 * Path: <b>PaymentReconciliation.outcome</b><br> 2164 * </p> 2165 */ 2166 @SearchParamDefinition(name="outcome", path="PaymentReconciliation.outcome", description="The processing outcome", type="token" ) 2167 public static final String SP_OUTCOME = "outcome"; 2168 /** 2169 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 2170 * <p> 2171 * Description: <b>The processing outcome</b><br> 2172 * Type: <b>token</b><br> 2173 * Path: <b>PaymentReconciliation.outcome</b><br> 2174 * </p> 2175 */ 2176 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 2177 2178 2179}