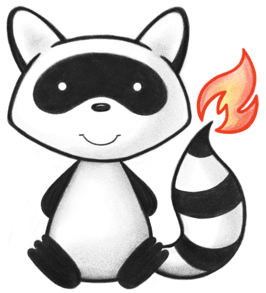
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.Date; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040 041import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * A time period defined by a start and end date and optionally time. 047 */ 048@DatatypeDef(name="Period") 049public class Period extends Type implements ICompositeType { 050 051 /** 052 * The start of the period. The boundary is inclusive. 053 */ 054 @Child(name = "start", type = {DateTimeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 055 @Description(shortDefinition="Starting time with inclusive boundary", formalDefinition="The start of the period. The boundary is inclusive." ) 056 protected DateTimeType start; 057 058 /** 059 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 060 */ 061 @Child(name = "end", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="End time with inclusive boundary, if not ongoing", formalDefinition="The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time." ) 063 protected DateTimeType end; 064 065 private static final long serialVersionUID = 649791751L; 066 067 /** 068 * Constructor 069 */ 070 public Period() { 071 super(); 072 } 073 074 /** 075 * @return {@link #start} (The start of the period. The boundary is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 076 */ 077 public DateTimeType getStartElement() { 078 if (this.start == null) 079 if (Configuration.errorOnAutoCreate()) 080 throw new Error("Attempt to auto-create Period.start"); 081 else if (Configuration.doAutoCreate()) 082 this.start = new DateTimeType(); // bb 083 return this.start; 084 } 085 086 public boolean hasStartElement() { 087 return this.start != null && !this.start.isEmpty(); 088 } 089 090 public boolean hasStart() { 091 return this.start != null && !this.start.isEmpty(); 092 } 093 094 /** 095 * @param value {@link #start} (The start of the period. The boundary is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 096 */ 097 public Period setStartElement(DateTimeType value) { 098 this.start = value; 099 return this; 100 } 101 102 /** 103 * @return The start of the period. The boundary is inclusive. 104 */ 105 public Date getStart() { 106 return this.start == null ? null : this.start.getValue(); 107 } 108 109 /** 110 * @param value The start of the period. The boundary is inclusive. 111 */ 112 public Period setStart(Date value) { 113 if (value == null) 114 this.start = null; 115 else { 116 if (this.start == null) 117 this.start = new DateTimeType(); 118 this.start.setValue(value); 119 } 120 return this; 121 } 122 123 /** 124 * @return {@link #end} (The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 125 */ 126 public DateTimeType getEndElement() { 127 if (this.end == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create Period.end"); 130 else if (Configuration.doAutoCreate()) 131 this.end = new DateTimeType(); // bb 132 return this.end; 133 } 134 135 public boolean hasEndElement() { 136 return this.end != null && !this.end.isEmpty(); 137 } 138 139 public boolean hasEnd() { 140 return this.end != null && !this.end.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #end} (The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 145 */ 146 public Period setEndElement(DateTimeType value) { 147 this.end = value; 148 return this; 149 } 150 151 /** 152 * @return The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 153 */ 154 public Date getEnd() { 155 return this.end == null ? null : this.end.getValue(); 156 } 157 158 /** 159 * @param value The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time. 160 */ 161 public Period setEnd(Date value) { 162 if (value == null) 163 this.end = null; 164 else { 165 if (this.end == null) 166 this.end = new DateTimeType(); 167 this.end.setValue(value); 168 } 169 return this; 170 } 171 172 /** 173 * Sets the value for <b>start</b> () 174 * 175 * <p> 176 * <b>Definition:</b> 177 * The start of the period. The boundary is inclusive. 178 * </p> 179 */ 180 public Period setStart( Date theDate, TemporalPrecisionEnum thePrecision) { 181 start = new DateTimeType(theDate, thePrecision); 182 return this; 183 } 184 185 /** 186 * Sets the value for <b>end</b> () 187 * 188 * <p> 189 * <b>Definition:</b> 190 * The end of the period. The boundary is inclusive. 191 * </p> 192 */ 193 public Period setEnd( Date theDate, TemporalPrecisionEnum thePrecision) { 194 end = new DateTimeType(theDate, thePrecision); 195 return this; 196 } 197 198 protected void listChildren(List<Property> children) { 199 super.listChildren(children); 200 children.add(new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, start)); 201 children.add(new Property("end", "dateTime", "The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 0, 1, end)); 202 } 203 204 @Override 205 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 206 switch (_hash) { 207 case 109757538: /*start*/ return new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, start); 208 case 100571: /*end*/ return new Property("end", "dateTime", "The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 0, 1, end); 209 default: return super.getNamedProperty(_hash, _name, _checkValid); 210 } 211 212 } 213 214 @Override 215 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 216 switch (hash) { 217 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // DateTimeType 218 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // DateTimeType 219 default: return super.getProperty(hash, name, checkValid); 220 } 221 222 } 223 224 @Override 225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 226 switch (hash) { 227 case 109757538: // start 228 this.start = castToDateTime(value); // DateTimeType 229 return value; 230 case 100571: // end 231 this.end = castToDateTime(value); // DateTimeType 232 return value; 233 default: return super.setProperty(hash, name, value); 234 } 235 236 } 237 238 @Override 239 public Base setProperty(String name, Base value) throws FHIRException { 240 if (name.equals("start")) { 241 this.start = castToDateTime(value); // DateTimeType 242 } else if (name.equals("end")) { 243 this.end = castToDateTime(value); // DateTimeType 244 } else 245 return super.setProperty(name, value); 246 return value; 247 } 248 249 @Override 250 public Base makeProperty(int hash, String name) throws FHIRException { 251 switch (hash) { 252 case 109757538: return getStartElement(); 253 case 100571: return getEndElement(); 254 default: return super.makeProperty(hash, name); 255 } 256 257 } 258 259 @Override 260 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 261 switch (hash) { 262 case 109757538: /*start*/ return new String[] {"dateTime"}; 263 case 100571: /*end*/ return new String[] {"dateTime"}; 264 default: return super.getTypesForProperty(hash, name); 265 } 266 267 } 268 269 @Override 270 public Base addChild(String name) throws FHIRException { 271 if (name.equals("start")) { 272 throw new FHIRException("Cannot call addChild on a singleton property Period.start"); 273 } 274 else if (name.equals("end")) { 275 throw new FHIRException("Cannot call addChild on a singleton property Period.end"); 276 } 277 else 278 return super.addChild(name); 279 } 280 281 public String fhirType() { 282 return "Period"; 283 284 } 285 286 public Period copy() { 287 Period dst = new Period(); 288 copyValues(dst); 289 dst.start = start == null ? null : start.copy(); 290 dst.end = end == null ? null : end.copy(); 291 return dst; 292 } 293 294 protected Period typedCopy() { 295 return copy(); 296 } 297 298 @Override 299 public boolean equalsDeep(Base other_) { 300 if (!super.equalsDeep(other_)) 301 return false; 302 if (!(other_ instanceof Period)) 303 return false; 304 Period o = (Period) other_; 305 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 306 } 307 308 @Override 309 public boolean equalsShallow(Base other_) { 310 if (!super.equalsShallow(other_)) 311 return false; 312 if (!(other_ instanceof Period)) 313 return false; 314 Period o = (Period) other_; 315 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 316 } 317 318 public boolean isEmpty() { 319 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 320 } 321 322 323}