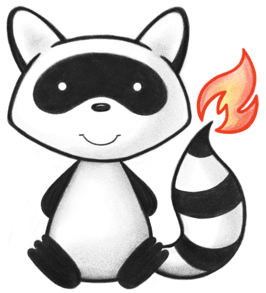
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Demographics and administrative information about a person independent of a specific health-related context. 051 */ 052@ResourceDef(name="Person", profile="http://hl7.org/fhir/Profile/Person") 053public class Person extends DomainResource { 054 055 public enum IdentityAssuranceLevel { 056 /** 057 * Little or no confidence in the asserted identity's accuracy. 058 */ 059 LEVEL1, 060 /** 061 * Some confidence in the asserted identity's accuracy. 062 */ 063 LEVEL2, 064 /** 065 * High confidence in the asserted identity's accuracy. 066 */ 067 LEVEL3, 068 /** 069 * Very high confidence in the asserted identity's accuracy. 070 */ 071 LEVEL4, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static IdentityAssuranceLevel fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("level1".equals(codeString)) 080 return LEVEL1; 081 if ("level2".equals(codeString)) 082 return LEVEL2; 083 if ("level3".equals(codeString)) 084 return LEVEL3; 085 if ("level4".equals(codeString)) 086 return LEVEL4; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case LEVEL1: return "level1"; 095 case LEVEL2: return "level2"; 096 case LEVEL3: return "level3"; 097 case LEVEL4: return "level4"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case LEVEL1: return "http://hl7.org/fhir/identity-assuranceLevel"; 105 case LEVEL2: return "http://hl7.org/fhir/identity-assuranceLevel"; 106 case LEVEL3: return "http://hl7.org/fhir/identity-assuranceLevel"; 107 case LEVEL4: return "http://hl7.org/fhir/identity-assuranceLevel"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case LEVEL1: return "Little or no confidence in the asserted identity's accuracy."; 115 case LEVEL2: return "Some confidence in the asserted identity's accuracy."; 116 case LEVEL3: return "High confidence in the asserted identity's accuracy."; 117 case LEVEL4: return "Very high confidence in the asserted identity's accuracy."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case LEVEL1: return "Level 1"; 125 case LEVEL2: return "Level 2"; 126 case LEVEL3: return "Level 3"; 127 case LEVEL4: return "Level 4"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class IdentityAssuranceLevelEnumFactory implements EnumFactory<IdentityAssuranceLevel> { 135 public IdentityAssuranceLevel fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("level1".equals(codeString)) 140 return IdentityAssuranceLevel.LEVEL1; 141 if ("level2".equals(codeString)) 142 return IdentityAssuranceLevel.LEVEL2; 143 if ("level3".equals(codeString)) 144 return IdentityAssuranceLevel.LEVEL3; 145 if ("level4".equals(codeString)) 146 return IdentityAssuranceLevel.LEVEL4; 147 throw new IllegalArgumentException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 148 } 149 public Enumeration<IdentityAssuranceLevel> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<IdentityAssuranceLevel>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("level1".equals(codeString)) 158 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL1); 159 if ("level2".equals(codeString)) 160 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL2); 161 if ("level3".equals(codeString)) 162 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL3); 163 if ("level4".equals(codeString)) 164 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL4); 165 throw new FHIRException("Unknown IdentityAssuranceLevel code '"+codeString+"'"); 166 } 167 public String toCode(IdentityAssuranceLevel code) { 168 if (code == IdentityAssuranceLevel.NULL) 169 return null; 170 if (code == IdentityAssuranceLevel.LEVEL1) 171 return "level1"; 172 if (code == IdentityAssuranceLevel.LEVEL2) 173 return "level2"; 174 if (code == IdentityAssuranceLevel.LEVEL3) 175 return "level3"; 176 if (code == IdentityAssuranceLevel.LEVEL4) 177 return "level4"; 178 return "?"; 179 } 180 public String toSystem(IdentityAssuranceLevel code) { 181 return code.getSystem(); 182 } 183 } 184 185 @Block() 186 public static class PersonLinkComponent extends BackboneElement implements IBaseBackboneElement { 187 /** 188 * The resource to which this actual person is associated. 189 */ 190 @Child(name = "target", type = {Patient.class, Practitioner.class, RelatedPerson.class, Person.class}, order=1, min=1, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="The resource to which this actual person is associated", formalDefinition="The resource to which this actual person is associated." ) 192 protected Reference target; 193 194 /** 195 * The actual object that is the target of the reference (The resource to which this actual person is associated.) 196 */ 197 protected Resource targetTarget; 198 199 /** 200 * Level of assurance that this link is actually associated with the target resource. 201 */ 202 @Child(name = "assurance", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 203 @Description(shortDefinition="level1 | level2 | level3 | level4", formalDefinition="Level of assurance that this link is actually associated with the target resource." ) 204 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identity-assuranceLevel") 205 protected Enumeration<IdentityAssuranceLevel> assurance; 206 207 private static final long serialVersionUID = 508763647L; 208 209 /** 210 * Constructor 211 */ 212 public PersonLinkComponent() { 213 super(); 214 } 215 216 /** 217 * Constructor 218 */ 219 public PersonLinkComponent(Reference target) { 220 super(); 221 this.target = target; 222 } 223 224 /** 225 * @return {@link #target} (The resource to which this actual person is associated.) 226 */ 227 public Reference getTarget() { 228 if (this.target == null) 229 if (Configuration.errorOnAutoCreate()) 230 throw new Error("Attempt to auto-create PersonLinkComponent.target"); 231 else if (Configuration.doAutoCreate()) 232 this.target = new Reference(); // cc 233 return this.target; 234 } 235 236 public boolean hasTarget() { 237 return this.target != null && !this.target.isEmpty(); 238 } 239 240 /** 241 * @param value {@link #target} (The resource to which this actual person is associated.) 242 */ 243 public PersonLinkComponent setTarget(Reference value) { 244 this.target = value; 245 return this; 246 } 247 248 /** 249 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource to which this actual person is associated.) 250 */ 251 public Resource getTargetTarget() { 252 return this.targetTarget; 253 } 254 255 /** 256 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource to which this actual person is associated.) 257 */ 258 public PersonLinkComponent setTargetTarget(Resource value) { 259 this.targetTarget = value; 260 return this; 261 } 262 263 /** 264 * @return {@link #assurance} (Level of assurance that this link is actually associated with the target resource.). This is the underlying object with id, value and extensions. The accessor "getAssurance" gives direct access to the value 265 */ 266 public Enumeration<IdentityAssuranceLevel> getAssuranceElement() { 267 if (this.assurance == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create PersonLinkComponent.assurance"); 270 else if (Configuration.doAutoCreate()) 271 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); // bb 272 return this.assurance; 273 } 274 275 public boolean hasAssuranceElement() { 276 return this.assurance != null && !this.assurance.isEmpty(); 277 } 278 279 public boolean hasAssurance() { 280 return this.assurance != null && !this.assurance.isEmpty(); 281 } 282 283 /** 284 * @param value {@link #assurance} (Level of assurance that this link is actually associated with the target resource.). This is the underlying object with id, value and extensions. The accessor "getAssurance" gives direct access to the value 285 */ 286 public PersonLinkComponent setAssuranceElement(Enumeration<IdentityAssuranceLevel> value) { 287 this.assurance = value; 288 return this; 289 } 290 291 /** 292 * @return Level of assurance that this link is actually associated with the target resource. 293 */ 294 public IdentityAssuranceLevel getAssurance() { 295 return this.assurance == null ? null : this.assurance.getValue(); 296 } 297 298 /** 299 * @param value Level of assurance that this link is actually associated with the target resource. 300 */ 301 public PersonLinkComponent setAssurance(IdentityAssuranceLevel value) { 302 if (value == null) 303 this.assurance = null; 304 else { 305 if (this.assurance == null) 306 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); 307 this.assurance.setValue(value); 308 } 309 return this; 310 } 311 312 protected void listChildren(List<Property> children) { 313 super.listChildren(children); 314 children.add(new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", "The resource to which this actual person is associated.", 0, 1, target)); 315 children.add(new Property("assurance", "code", "Level of assurance that this link is actually associated with the target resource.", 0, 1, assurance)); 316 } 317 318 @Override 319 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 320 switch (_hash) { 321 case -880905839: /*target*/ return new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", "The resource to which this actual person is associated.", 0, 1, target); 322 case 1771900717: /*assurance*/ return new Property("assurance", "code", "Level of assurance that this link is actually associated with the target resource.", 0, 1, assurance); 323 default: return super.getNamedProperty(_hash, _name, _checkValid); 324 } 325 326 } 327 328 @Override 329 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 330 switch (hash) { 331 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 332 case 1771900717: /*assurance*/ return this.assurance == null ? new Base[0] : new Base[] {this.assurance}; // Enumeration<IdentityAssuranceLevel> 333 default: return super.getProperty(hash, name, checkValid); 334 } 335 336 } 337 338 @Override 339 public Base setProperty(int hash, String name, Base value) throws FHIRException { 340 switch (hash) { 341 case -880905839: // target 342 this.target = castToReference(value); // Reference 343 return value; 344 case 1771900717: // assurance 345 value = new IdentityAssuranceLevelEnumFactory().fromType(castToCode(value)); 346 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 347 return value; 348 default: return super.setProperty(hash, name, value); 349 } 350 351 } 352 353 @Override 354 public Base setProperty(String name, Base value) throws FHIRException { 355 if (name.equals("target")) { 356 this.target = castToReference(value); // Reference 357 } else if (name.equals("assurance")) { 358 value = new IdentityAssuranceLevelEnumFactory().fromType(castToCode(value)); 359 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 360 } else 361 return super.setProperty(name, value); 362 return value; 363 } 364 365 @Override 366 public Base makeProperty(int hash, String name) throws FHIRException { 367 switch (hash) { 368 case -880905839: return getTarget(); 369 case 1771900717: return getAssuranceElement(); 370 default: return super.makeProperty(hash, name); 371 } 372 373 } 374 375 @Override 376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 377 switch (hash) { 378 case -880905839: /*target*/ return new String[] {"Reference"}; 379 case 1771900717: /*assurance*/ return new String[] {"code"}; 380 default: return super.getTypesForProperty(hash, name); 381 } 382 383 } 384 385 @Override 386 public Base addChild(String name) throws FHIRException { 387 if (name.equals("target")) { 388 this.target = new Reference(); 389 return this.target; 390 } 391 else if (name.equals("assurance")) { 392 throw new FHIRException("Cannot call addChild on a singleton property Person.assurance"); 393 } 394 else 395 return super.addChild(name); 396 } 397 398 public PersonLinkComponent copy() { 399 PersonLinkComponent dst = new PersonLinkComponent(); 400 copyValues(dst); 401 dst.target = target == null ? null : target.copy(); 402 dst.assurance = assurance == null ? null : assurance.copy(); 403 return dst; 404 } 405 406 @Override 407 public boolean equalsDeep(Base other_) { 408 if (!super.equalsDeep(other_)) 409 return false; 410 if (!(other_ instanceof PersonLinkComponent)) 411 return false; 412 PersonLinkComponent o = (PersonLinkComponent) other_; 413 return compareDeep(target, o.target, true) && compareDeep(assurance, o.assurance, true); 414 } 415 416 @Override 417 public boolean equalsShallow(Base other_) { 418 if (!super.equalsShallow(other_)) 419 return false; 420 if (!(other_ instanceof PersonLinkComponent)) 421 return false; 422 PersonLinkComponent o = (PersonLinkComponent) other_; 423 return compareValues(assurance, o.assurance, true); 424 } 425 426 public boolean isEmpty() { 427 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, assurance); 428 } 429 430 public String fhirType() { 431 return "Person.link"; 432 433 } 434 435 } 436 437 /** 438 * Identifier for a person within a particular scope. 439 */ 440 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 441 @Description(shortDefinition="A human identifier for this person", formalDefinition="Identifier for a person within a particular scope." ) 442 protected List<Identifier> identifier; 443 444 /** 445 * A name associated with the person. 446 */ 447 @Child(name = "name", type = {HumanName.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 448 @Description(shortDefinition="A name associated with the person", formalDefinition="A name associated with the person." ) 449 protected List<HumanName> name; 450 451 /** 452 * A contact detail for the person, e.g. a telephone number or an email address. 453 */ 454 @Child(name = "telecom", type = {ContactPoint.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 455 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 456 protected List<ContactPoint> telecom; 457 458 /** 459 * Administrative Gender. 460 */ 461 @Child(name = "gender", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 462 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender." ) 463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 464 protected Enumeration<AdministrativeGender> gender; 465 466 /** 467 * The birth date for the person. 468 */ 469 @Child(name = "birthDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=true) 470 @Description(shortDefinition="The date on which the person was born", formalDefinition="The birth date for the person." ) 471 protected DateType birthDate; 472 473 /** 474 * One or more addresses for the person. 475 */ 476 @Child(name = "address", type = {Address.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 477 @Description(shortDefinition="One or more addresses for the person", formalDefinition="One or more addresses for the person." ) 478 protected List<Address> address; 479 480 /** 481 * An image that can be displayed as a thumbnail of the person to enhance the identification of the individual. 482 */ 483 @Child(name = "photo", type = {Attachment.class}, order=6, min=0, max=1, modifier=false, summary=false) 484 @Description(shortDefinition="Image of the person", formalDefinition="An image that can be displayed as a thumbnail of the person to enhance the identification of the individual." ) 485 protected Attachment photo; 486 487 /** 488 * The organization that is the custodian of the person record. 489 */ 490 @Child(name = "managingOrganization", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=true) 491 @Description(shortDefinition="The organization that is the custodian of the person record", formalDefinition="The organization that is the custodian of the person record." ) 492 protected Reference managingOrganization; 493 494 /** 495 * The actual object that is the target of the reference (The organization that is the custodian of the person record.) 496 */ 497 protected Organization managingOrganizationTarget; 498 499 /** 500 * Whether this person's record is in active use. 501 */ 502 @Child(name = "active", type = {BooleanType.class}, order=8, min=0, max=1, modifier=true, summary=true) 503 @Description(shortDefinition="This person's record is in active use", formalDefinition="Whether this person's record is in active use." ) 504 protected BooleanType active; 505 506 /** 507 * Link to a resource that concerns the same actual person. 508 */ 509 @Child(name = "link", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 510 @Description(shortDefinition="Link to a resource that concerns the same actual person", formalDefinition="Link to a resource that concerns the same actual person." ) 511 protected List<PersonLinkComponent> link; 512 513 private static final long serialVersionUID = -117464654L; 514 515 /** 516 * Constructor 517 */ 518 public Person() { 519 super(); 520 } 521 522 /** 523 * @return {@link #identifier} (Identifier for a person within a particular scope.) 524 */ 525 public List<Identifier> getIdentifier() { 526 if (this.identifier == null) 527 this.identifier = new ArrayList<Identifier>(); 528 return this.identifier; 529 } 530 531 /** 532 * @return Returns a reference to <code>this</code> for easy method chaining 533 */ 534 public Person setIdentifier(List<Identifier> theIdentifier) { 535 this.identifier = theIdentifier; 536 return this; 537 } 538 539 public boolean hasIdentifier() { 540 if (this.identifier == null) 541 return false; 542 for (Identifier item : this.identifier) 543 if (!item.isEmpty()) 544 return true; 545 return false; 546 } 547 548 public Identifier addIdentifier() { //3 549 Identifier t = new Identifier(); 550 if (this.identifier == null) 551 this.identifier = new ArrayList<Identifier>(); 552 this.identifier.add(t); 553 return t; 554 } 555 556 public Person addIdentifier(Identifier t) { //3 557 if (t == null) 558 return this; 559 if (this.identifier == null) 560 this.identifier = new ArrayList<Identifier>(); 561 this.identifier.add(t); 562 return this; 563 } 564 565 /** 566 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 567 */ 568 public Identifier getIdentifierFirstRep() { 569 if (getIdentifier().isEmpty()) { 570 addIdentifier(); 571 } 572 return getIdentifier().get(0); 573 } 574 575 /** 576 * @return {@link #name} (A name associated with the person.) 577 */ 578 public List<HumanName> getName() { 579 if (this.name == null) 580 this.name = new ArrayList<HumanName>(); 581 return this.name; 582 } 583 584 /** 585 * @return Returns a reference to <code>this</code> for easy method chaining 586 */ 587 public Person setName(List<HumanName> theName) { 588 this.name = theName; 589 return this; 590 } 591 592 public boolean hasName() { 593 if (this.name == null) 594 return false; 595 for (HumanName item : this.name) 596 if (!item.isEmpty()) 597 return true; 598 return false; 599 } 600 601 public HumanName addName() { //3 602 HumanName t = new HumanName(); 603 if (this.name == null) 604 this.name = new ArrayList<HumanName>(); 605 this.name.add(t); 606 return t; 607 } 608 609 public Person addName(HumanName t) { //3 610 if (t == null) 611 return this; 612 if (this.name == null) 613 this.name = new ArrayList<HumanName>(); 614 this.name.add(t); 615 return this; 616 } 617 618 /** 619 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist 620 */ 621 public HumanName getNameFirstRep() { 622 if (getName().isEmpty()) { 623 addName(); 624 } 625 return getName().get(0); 626 } 627 628 /** 629 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 630 */ 631 public List<ContactPoint> getTelecom() { 632 if (this.telecom == null) 633 this.telecom = new ArrayList<ContactPoint>(); 634 return this.telecom; 635 } 636 637 /** 638 * @return Returns a reference to <code>this</code> for easy method chaining 639 */ 640 public Person setTelecom(List<ContactPoint> theTelecom) { 641 this.telecom = theTelecom; 642 return this; 643 } 644 645 public boolean hasTelecom() { 646 if (this.telecom == null) 647 return false; 648 for (ContactPoint item : this.telecom) 649 if (!item.isEmpty()) 650 return true; 651 return false; 652 } 653 654 public ContactPoint addTelecom() { //3 655 ContactPoint t = new ContactPoint(); 656 if (this.telecom == null) 657 this.telecom = new ArrayList<ContactPoint>(); 658 this.telecom.add(t); 659 return t; 660 } 661 662 public Person addTelecom(ContactPoint t) { //3 663 if (t == null) 664 return this; 665 if (this.telecom == null) 666 this.telecom = new ArrayList<ContactPoint>(); 667 this.telecom.add(t); 668 return this; 669 } 670 671 /** 672 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 673 */ 674 public ContactPoint getTelecomFirstRep() { 675 if (getTelecom().isEmpty()) { 676 addTelecom(); 677 } 678 return getTelecom().get(0); 679 } 680 681 /** 682 * @return {@link #gender} (Administrative Gender.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 683 */ 684 public Enumeration<AdministrativeGender> getGenderElement() { 685 if (this.gender == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create Person.gender"); 688 else if (Configuration.doAutoCreate()) 689 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 690 return this.gender; 691 } 692 693 public boolean hasGenderElement() { 694 return this.gender != null && !this.gender.isEmpty(); 695 } 696 697 public boolean hasGender() { 698 return this.gender != null && !this.gender.isEmpty(); 699 } 700 701 /** 702 * @param value {@link #gender} (Administrative Gender.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 703 */ 704 public Person setGenderElement(Enumeration<AdministrativeGender> value) { 705 this.gender = value; 706 return this; 707 } 708 709 /** 710 * @return Administrative Gender. 711 */ 712 public AdministrativeGender getGender() { 713 return this.gender == null ? null : this.gender.getValue(); 714 } 715 716 /** 717 * @param value Administrative Gender. 718 */ 719 public Person setGender(AdministrativeGender value) { 720 if (value == null) 721 this.gender = null; 722 else { 723 if (this.gender == null) 724 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 725 this.gender.setValue(value); 726 } 727 return this; 728 } 729 730 /** 731 * @return {@link #birthDate} (The birth date for the person.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 732 */ 733 public DateType getBirthDateElement() { 734 if (this.birthDate == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create Person.birthDate"); 737 else if (Configuration.doAutoCreate()) 738 this.birthDate = new DateType(); // bb 739 return this.birthDate; 740 } 741 742 public boolean hasBirthDateElement() { 743 return this.birthDate != null && !this.birthDate.isEmpty(); 744 } 745 746 public boolean hasBirthDate() { 747 return this.birthDate != null && !this.birthDate.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #birthDate} (The birth date for the person.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 752 */ 753 public Person setBirthDateElement(DateType value) { 754 this.birthDate = value; 755 return this; 756 } 757 758 /** 759 * @return The birth date for the person. 760 */ 761 public Date getBirthDate() { 762 return this.birthDate == null ? null : this.birthDate.getValue(); 763 } 764 765 /** 766 * @param value The birth date for the person. 767 */ 768 public Person setBirthDate(Date value) { 769 if (value == null) 770 this.birthDate = null; 771 else { 772 if (this.birthDate == null) 773 this.birthDate = new DateType(); 774 this.birthDate.setValue(value); 775 } 776 return this; 777 } 778 779 /** 780 * @return {@link #address} (One or more addresses for the person.) 781 */ 782 public List<Address> getAddress() { 783 if (this.address == null) 784 this.address = new ArrayList<Address>(); 785 return this.address; 786 } 787 788 /** 789 * @return Returns a reference to <code>this</code> for easy method chaining 790 */ 791 public Person setAddress(List<Address> theAddress) { 792 this.address = theAddress; 793 return this; 794 } 795 796 public boolean hasAddress() { 797 if (this.address == null) 798 return false; 799 for (Address item : this.address) 800 if (!item.isEmpty()) 801 return true; 802 return false; 803 } 804 805 public Address addAddress() { //3 806 Address t = new Address(); 807 if (this.address == null) 808 this.address = new ArrayList<Address>(); 809 this.address.add(t); 810 return t; 811 } 812 813 public Person addAddress(Address t) { //3 814 if (t == null) 815 return this; 816 if (this.address == null) 817 this.address = new ArrayList<Address>(); 818 this.address.add(t); 819 return this; 820 } 821 822 /** 823 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist 824 */ 825 public Address getAddressFirstRep() { 826 if (getAddress().isEmpty()) { 827 addAddress(); 828 } 829 return getAddress().get(0); 830 } 831 832 /** 833 * @return {@link #photo} (An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.) 834 */ 835 public Attachment getPhoto() { 836 if (this.photo == null) 837 if (Configuration.errorOnAutoCreate()) 838 throw new Error("Attempt to auto-create Person.photo"); 839 else if (Configuration.doAutoCreate()) 840 this.photo = new Attachment(); // cc 841 return this.photo; 842 } 843 844 public boolean hasPhoto() { 845 return this.photo != null && !this.photo.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #photo} (An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.) 850 */ 851 public Person setPhoto(Attachment value) { 852 this.photo = value; 853 return this; 854 } 855 856 /** 857 * @return {@link #managingOrganization} (The organization that is the custodian of the person record.) 858 */ 859 public Reference getManagingOrganization() { 860 if (this.managingOrganization == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create Person.managingOrganization"); 863 else if (Configuration.doAutoCreate()) 864 this.managingOrganization = new Reference(); // cc 865 return this.managingOrganization; 866 } 867 868 public boolean hasManagingOrganization() { 869 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 870 } 871 872 /** 873 * @param value {@link #managingOrganization} (The organization that is the custodian of the person record.) 874 */ 875 public Person setManagingOrganization(Reference value) { 876 this.managingOrganization = value; 877 return this; 878 } 879 880 /** 881 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization that is the custodian of the person record.) 882 */ 883 public Organization getManagingOrganizationTarget() { 884 if (this.managingOrganizationTarget == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create Person.managingOrganization"); 887 else if (Configuration.doAutoCreate()) 888 this.managingOrganizationTarget = new Organization(); // aa 889 return this.managingOrganizationTarget; 890 } 891 892 /** 893 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization that is the custodian of the person record.) 894 */ 895 public Person setManagingOrganizationTarget(Organization value) { 896 this.managingOrganizationTarget = value; 897 return this; 898 } 899 900 /** 901 * @return {@link #active} (Whether this person's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 902 */ 903 public BooleanType getActiveElement() { 904 if (this.active == null) 905 if (Configuration.errorOnAutoCreate()) 906 throw new Error("Attempt to auto-create Person.active"); 907 else if (Configuration.doAutoCreate()) 908 this.active = new BooleanType(); // bb 909 return this.active; 910 } 911 912 public boolean hasActiveElement() { 913 return this.active != null && !this.active.isEmpty(); 914 } 915 916 public boolean hasActive() { 917 return this.active != null && !this.active.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #active} (Whether this person's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 922 */ 923 public Person setActiveElement(BooleanType value) { 924 this.active = value; 925 return this; 926 } 927 928 /** 929 * @return Whether this person's record is in active use. 930 */ 931 public boolean getActive() { 932 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 933 } 934 935 /** 936 * @param value Whether this person's record is in active use. 937 */ 938 public Person setActive(boolean value) { 939 if (this.active == null) 940 this.active = new BooleanType(); 941 this.active.setValue(value); 942 return this; 943 } 944 945 /** 946 * @return {@link #link} (Link to a resource that concerns the same actual person.) 947 */ 948 public List<PersonLinkComponent> getLink() { 949 if (this.link == null) 950 this.link = new ArrayList<PersonLinkComponent>(); 951 return this.link; 952 } 953 954 /** 955 * @return Returns a reference to <code>this</code> for easy method chaining 956 */ 957 public Person setLink(List<PersonLinkComponent> theLink) { 958 this.link = theLink; 959 return this; 960 } 961 962 public boolean hasLink() { 963 if (this.link == null) 964 return false; 965 for (PersonLinkComponent item : this.link) 966 if (!item.isEmpty()) 967 return true; 968 return false; 969 } 970 971 public PersonLinkComponent addLink() { //3 972 PersonLinkComponent t = new PersonLinkComponent(); 973 if (this.link == null) 974 this.link = new ArrayList<PersonLinkComponent>(); 975 this.link.add(t); 976 return t; 977 } 978 979 public Person addLink(PersonLinkComponent t) { //3 980 if (t == null) 981 return this; 982 if (this.link == null) 983 this.link = new ArrayList<PersonLinkComponent>(); 984 this.link.add(t); 985 return this; 986 } 987 988 /** 989 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 990 */ 991 public PersonLinkComponent getLinkFirstRep() { 992 if (getLink().isEmpty()) { 993 addLink(); 994 } 995 return getLink().get(0); 996 } 997 998 protected void listChildren(List<Property> children) { 999 super.listChildren(children); 1000 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1001 children.add(new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 1002 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1003 children.add(new Property("gender", "code", "Administrative Gender.", 0, 1, gender)); 1004 children.add(new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate)); 1005 children.add(new Property("address", "Address", "One or more addresses for the person.", 0, java.lang.Integer.MAX_VALUE, address)); 1006 children.add(new Property("photo", "Attachment", "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 0, 1, photo)); 1007 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that is the custodian of the person record.", 0, 1, managingOrganization)); 1008 children.add(new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, active)); 1009 children.add(new Property("link", "", "Link to a resource that concerns the same actual person.", 0, java.lang.Integer.MAX_VALUE, link)); 1010 } 1011 1012 @Override 1013 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1014 switch (_hash) { 1015 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 1016 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name); 1017 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 1018 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender.", 0, 1, gender); 1019 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate); 1020 case -1147692044: /*address*/ return new Property("address", "Address", "One or more addresses for the person.", 0, java.lang.Integer.MAX_VALUE, address); 1021 case 106642994: /*photo*/ return new Property("photo", "Attachment", "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 0, 1, photo); 1022 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that is the custodian of the person record.", 0, 1, managingOrganization); 1023 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, active); 1024 case 3321850: /*link*/ return new Property("link", "", "Link to a resource that concerns the same actual person.", 0, java.lang.Integer.MAX_VALUE, link); 1025 default: return super.getNamedProperty(_hash, _name, _checkValid); 1026 } 1027 1028 } 1029 1030 @Override 1031 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1032 switch (hash) { 1033 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1034 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1035 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1036 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1037 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 1038 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1039 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : new Base[] {this.photo}; // Attachment 1040 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1041 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1042 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PersonLinkComponent 1043 default: return super.getProperty(hash, name, checkValid); 1044 } 1045 1046 } 1047 1048 @Override 1049 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1050 switch (hash) { 1051 case -1618432855: // identifier 1052 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1053 return value; 1054 case 3373707: // name 1055 this.getName().add(castToHumanName(value)); // HumanName 1056 return value; 1057 case -1429363305: // telecom 1058 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1059 return value; 1060 case -1249512767: // gender 1061 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1062 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1063 return value; 1064 case -1210031859: // birthDate 1065 this.birthDate = castToDate(value); // DateType 1066 return value; 1067 case -1147692044: // address 1068 this.getAddress().add(castToAddress(value)); // Address 1069 return value; 1070 case 106642994: // photo 1071 this.photo = castToAttachment(value); // Attachment 1072 return value; 1073 case -2058947787: // managingOrganization 1074 this.managingOrganization = castToReference(value); // Reference 1075 return value; 1076 case -1422950650: // active 1077 this.active = castToBoolean(value); // BooleanType 1078 return value; 1079 case 3321850: // link 1080 this.getLink().add((PersonLinkComponent) value); // PersonLinkComponent 1081 return value; 1082 default: return super.setProperty(hash, name, value); 1083 } 1084 1085 } 1086 1087 @Override 1088 public Base setProperty(String name, Base value) throws FHIRException { 1089 if (name.equals("identifier")) { 1090 this.getIdentifier().add(castToIdentifier(value)); 1091 } else if (name.equals("name")) { 1092 this.getName().add(castToHumanName(value)); 1093 } else if (name.equals("telecom")) { 1094 this.getTelecom().add(castToContactPoint(value)); 1095 } else if (name.equals("gender")) { 1096 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1097 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1098 } else if (name.equals("birthDate")) { 1099 this.birthDate = castToDate(value); // DateType 1100 } else if (name.equals("address")) { 1101 this.getAddress().add(castToAddress(value)); 1102 } else if (name.equals("photo")) { 1103 this.photo = castToAttachment(value); // Attachment 1104 } else if (name.equals("managingOrganization")) { 1105 this.managingOrganization = castToReference(value); // Reference 1106 } else if (name.equals("active")) { 1107 this.active = castToBoolean(value); // BooleanType 1108 } else if (name.equals("link")) { 1109 this.getLink().add((PersonLinkComponent) value); 1110 } else 1111 return super.setProperty(name, value); 1112 return value; 1113 } 1114 1115 @Override 1116 public Base makeProperty(int hash, String name) throws FHIRException { 1117 switch (hash) { 1118 case -1618432855: return addIdentifier(); 1119 case 3373707: return addName(); 1120 case -1429363305: return addTelecom(); 1121 case -1249512767: return getGenderElement(); 1122 case -1210031859: return getBirthDateElement(); 1123 case -1147692044: return addAddress(); 1124 case 106642994: return getPhoto(); 1125 case -2058947787: return getManagingOrganization(); 1126 case -1422950650: return getActiveElement(); 1127 case 3321850: return addLink(); 1128 default: return super.makeProperty(hash, name); 1129 } 1130 1131 } 1132 1133 @Override 1134 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1135 switch (hash) { 1136 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1137 case 3373707: /*name*/ return new String[] {"HumanName"}; 1138 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1139 case -1249512767: /*gender*/ return new String[] {"code"}; 1140 case -1210031859: /*birthDate*/ return new String[] {"date"}; 1141 case -1147692044: /*address*/ return new String[] {"Address"}; 1142 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1143 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1144 case -1422950650: /*active*/ return new String[] {"boolean"}; 1145 case 3321850: /*link*/ return new String[] {}; 1146 default: return super.getTypesForProperty(hash, name); 1147 } 1148 1149 } 1150 1151 @Override 1152 public Base addChild(String name) throws FHIRException { 1153 if (name.equals("identifier")) { 1154 return addIdentifier(); 1155 } 1156 else if (name.equals("name")) { 1157 return addName(); 1158 } 1159 else if (name.equals("telecom")) { 1160 return addTelecom(); 1161 } 1162 else if (name.equals("gender")) { 1163 throw new FHIRException("Cannot call addChild on a singleton property Person.gender"); 1164 } 1165 else if (name.equals("birthDate")) { 1166 throw new FHIRException("Cannot call addChild on a singleton property Person.birthDate"); 1167 } 1168 else if (name.equals("address")) { 1169 return addAddress(); 1170 } 1171 else if (name.equals("photo")) { 1172 this.photo = new Attachment(); 1173 return this.photo; 1174 } 1175 else if (name.equals("managingOrganization")) { 1176 this.managingOrganization = new Reference(); 1177 return this.managingOrganization; 1178 } 1179 else if (name.equals("active")) { 1180 throw new FHIRException("Cannot call addChild on a singleton property Person.active"); 1181 } 1182 else if (name.equals("link")) { 1183 return addLink(); 1184 } 1185 else 1186 return super.addChild(name); 1187 } 1188 1189 public String fhirType() { 1190 return "Person"; 1191 1192 } 1193 1194 public Person copy() { 1195 Person dst = new Person(); 1196 copyValues(dst); 1197 if (identifier != null) { 1198 dst.identifier = new ArrayList<Identifier>(); 1199 for (Identifier i : identifier) 1200 dst.identifier.add(i.copy()); 1201 }; 1202 if (name != null) { 1203 dst.name = new ArrayList<HumanName>(); 1204 for (HumanName i : name) 1205 dst.name.add(i.copy()); 1206 }; 1207 if (telecom != null) { 1208 dst.telecom = new ArrayList<ContactPoint>(); 1209 for (ContactPoint i : telecom) 1210 dst.telecom.add(i.copy()); 1211 }; 1212 dst.gender = gender == null ? null : gender.copy(); 1213 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1214 if (address != null) { 1215 dst.address = new ArrayList<Address>(); 1216 for (Address i : address) 1217 dst.address.add(i.copy()); 1218 }; 1219 dst.photo = photo == null ? null : photo.copy(); 1220 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1221 dst.active = active == null ? null : active.copy(); 1222 if (link != null) { 1223 dst.link = new ArrayList<PersonLinkComponent>(); 1224 for (PersonLinkComponent i : link) 1225 dst.link.add(i.copy()); 1226 }; 1227 return dst; 1228 } 1229 1230 protected Person typedCopy() { 1231 return copy(); 1232 } 1233 1234 @Override 1235 public boolean equalsDeep(Base other_) { 1236 if (!super.equalsDeep(other_)) 1237 return false; 1238 if (!(other_ instanceof Person)) 1239 return false; 1240 Person o = (Person) other_; 1241 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 1242 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 1243 && compareDeep(photo, o.photo, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1244 && compareDeep(active, o.active, true) && compareDeep(link, o.link, true); 1245 } 1246 1247 @Override 1248 public boolean equalsShallow(Base other_) { 1249 if (!super.equalsShallow(other_)) 1250 return false; 1251 if (!(other_ instanceof Person)) 1252 return false; 1253 Person o = (Person) other_; 1254 return compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) && compareValues(active, o.active, true) 1255 ; 1256 } 1257 1258 public boolean isEmpty() { 1259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, telecom 1260 , gender, birthDate, address, photo, managingOrganization, active, link); 1261 } 1262 1263 @Override 1264 public ResourceType getResourceType() { 1265 return ResourceType.Person; 1266 } 1267 1268 /** 1269 * Search parameter: <b>identifier</b> 1270 * <p> 1271 * Description: <b>A person Identifier</b><br> 1272 * Type: <b>token</b><br> 1273 * Path: <b>Person.identifier</b><br> 1274 * </p> 1275 */ 1276 @SearchParamDefinition(name="identifier", path="Person.identifier", description="A person Identifier", type="token" ) 1277 public static final String SP_IDENTIFIER = "identifier"; 1278 /** 1279 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1280 * <p> 1281 * Description: <b>A person Identifier</b><br> 1282 * Type: <b>token</b><br> 1283 * Path: <b>Person.identifier</b><br> 1284 * </p> 1285 */ 1286 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1287 1288 /** 1289 * Search parameter: <b>address</b> 1290 * <p> 1291 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1292 * Type: <b>string</b><br> 1293 * Path: <b>Person.address</b><br> 1294 * </p> 1295 */ 1296 @SearchParamDefinition(name="address", path="Person.address", description="A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text", type="string" ) 1297 public static final String SP_ADDRESS = "address"; 1298 /** 1299 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1300 * <p> 1301 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1302 * Type: <b>string</b><br> 1303 * Path: <b>Person.address</b><br> 1304 * </p> 1305 */ 1306 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1307 1308 /** 1309 * Search parameter: <b>birthdate</b> 1310 * <p> 1311 * Description: <b>The person's date of birth</b><br> 1312 * Type: <b>date</b><br> 1313 * Path: <b>Person.birthDate</b><br> 1314 * </p> 1315 */ 1316 @SearchParamDefinition(name="birthdate", path="Person.birthDate", description="The person's date of birth", type="date" ) 1317 public static final String SP_BIRTHDATE = "birthdate"; 1318 /** 1319 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 1320 * <p> 1321 * Description: <b>The person's date of birth</b><br> 1322 * Type: <b>date</b><br> 1323 * Path: <b>Person.birthDate</b><br> 1324 * </p> 1325 */ 1326 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 1327 1328 /** 1329 * Search parameter: <b>address-state</b> 1330 * <p> 1331 * Description: <b>A state specified in an address</b><br> 1332 * Type: <b>string</b><br> 1333 * Path: <b>Person.address.state</b><br> 1334 * </p> 1335 */ 1336 @SearchParamDefinition(name="address-state", path="Person.address.state", description="A state specified in an address", type="string" ) 1337 public static final String SP_ADDRESS_STATE = "address-state"; 1338 /** 1339 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1340 * <p> 1341 * Description: <b>A state specified in an address</b><br> 1342 * Type: <b>string</b><br> 1343 * Path: <b>Person.address.state</b><br> 1344 * </p> 1345 */ 1346 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1347 1348 /** 1349 * Search parameter: <b>gender</b> 1350 * <p> 1351 * Description: <b>The gender of the person</b><br> 1352 * Type: <b>token</b><br> 1353 * Path: <b>Person.gender</b><br> 1354 * </p> 1355 */ 1356 @SearchParamDefinition(name="gender", path="Person.gender", description="The gender of the person", type="token" ) 1357 public static final String SP_GENDER = "gender"; 1358 /** 1359 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1360 * <p> 1361 * Description: <b>The gender of the person</b><br> 1362 * Type: <b>token</b><br> 1363 * Path: <b>Person.gender</b><br> 1364 * </p> 1365 */ 1366 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 1367 1368 /** 1369 * Search parameter: <b>practitioner</b> 1370 * <p> 1371 * Description: <b>The Person links to this Practitioner</b><br> 1372 * Type: <b>reference</b><br> 1373 * Path: <b>Person.link.target</b><br> 1374 * </p> 1375 */ 1376 @SearchParamDefinition(name="practitioner", path="Person.link.target", description="The Person links to this Practitioner", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1377 public static final String SP_PRACTITIONER = "practitioner"; 1378 /** 1379 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1380 * <p> 1381 * Description: <b>The Person links to this Practitioner</b><br> 1382 * Type: <b>reference</b><br> 1383 * Path: <b>Person.link.target</b><br> 1384 * </p> 1385 */ 1386 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 1387 1388/** 1389 * Constant for fluent queries to be used to add include statements. Specifies 1390 * the path value of "<b>Person:practitioner</b>". 1391 */ 1392 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Person:practitioner").toLocked(); 1393 1394 /** 1395 * Search parameter: <b>link</b> 1396 * <p> 1397 * Description: <b>Any link has this Patient, Person, RelatedPerson or Practitioner reference</b><br> 1398 * Type: <b>reference</b><br> 1399 * Path: <b>Person.link.target</b><br> 1400 * </p> 1401 */ 1402 @SearchParamDefinition(name="link", path="Person.link.target", description="Any link has this Patient, Person, RelatedPerson or Practitioner reference", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Person.class, Practitioner.class, RelatedPerson.class } ) 1403 public static final String SP_LINK = "link"; 1404 /** 1405 * <b>Fluent Client</b> search parameter constant for <b>link</b> 1406 * <p> 1407 * Description: <b>Any link has this Patient, Person, RelatedPerson or Practitioner reference</b><br> 1408 * Type: <b>reference</b><br> 1409 * Path: <b>Person.link.target</b><br> 1410 * </p> 1411 */ 1412 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LINK); 1413 1414/** 1415 * Constant for fluent queries to be used to add include statements. Specifies 1416 * the path value of "<b>Person:link</b>". 1417 */ 1418 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Person:link").toLocked(); 1419 1420 /** 1421 * Search parameter: <b>relatedperson</b> 1422 * <p> 1423 * Description: <b>The Person links to this RelatedPerson</b><br> 1424 * Type: <b>reference</b><br> 1425 * Path: <b>Person.link.target</b><br> 1426 * </p> 1427 */ 1428 @SearchParamDefinition(name="relatedperson", path="Person.link.target", description="The Person links to this RelatedPerson", type="reference", target={RelatedPerson.class } ) 1429 public static final String SP_RELATEDPERSON = "relatedperson"; 1430 /** 1431 * <b>Fluent Client</b> search parameter constant for <b>relatedperson</b> 1432 * <p> 1433 * Description: <b>The Person links to this RelatedPerson</b><br> 1434 * Type: <b>reference</b><br> 1435 * Path: <b>Person.link.target</b><br> 1436 * </p> 1437 */ 1438 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATEDPERSON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATEDPERSON); 1439 1440/** 1441 * Constant for fluent queries to be used to add include statements. Specifies 1442 * the path value of "<b>Person:relatedperson</b>". 1443 */ 1444 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATEDPERSON = new ca.uhn.fhir.model.api.Include("Person:relatedperson").toLocked(); 1445 1446 /** 1447 * Search parameter: <b>address-postalcode</b> 1448 * <p> 1449 * Description: <b>A postal code specified in an address</b><br> 1450 * Type: <b>string</b><br> 1451 * Path: <b>Person.address.postalCode</b><br> 1452 * </p> 1453 */ 1454 @SearchParamDefinition(name="address-postalcode", path="Person.address.postalCode", description="A postal code specified in an address", type="string" ) 1455 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1456 /** 1457 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1458 * <p> 1459 * Description: <b>A postal code specified in an address</b><br> 1460 * Type: <b>string</b><br> 1461 * Path: <b>Person.address.postalCode</b><br> 1462 * </p> 1463 */ 1464 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1465 1466 /** 1467 * Search parameter: <b>address-country</b> 1468 * <p> 1469 * Description: <b>A country specified in an address</b><br> 1470 * Type: <b>string</b><br> 1471 * Path: <b>Person.address.country</b><br> 1472 * </p> 1473 */ 1474 @SearchParamDefinition(name="address-country", path="Person.address.country", description="A country specified in an address", type="string" ) 1475 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1476 /** 1477 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1478 * <p> 1479 * Description: <b>A country specified in an address</b><br> 1480 * Type: <b>string</b><br> 1481 * Path: <b>Person.address.country</b><br> 1482 * </p> 1483 */ 1484 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1485 1486 /** 1487 * Search parameter: <b>phonetic</b> 1488 * <p> 1489 * Description: <b>A portion of name using some kind of phonetic matching algorithm</b><br> 1490 * Type: <b>string</b><br> 1491 * Path: <b>Person.name</b><br> 1492 * </p> 1493 */ 1494 @SearchParamDefinition(name="phonetic", path="Person.name", description="A portion of name using some kind of phonetic matching algorithm", type="string" ) 1495 public static final String SP_PHONETIC = "phonetic"; 1496 /** 1497 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1498 * <p> 1499 * Description: <b>A portion of name using some kind of phonetic matching algorithm</b><br> 1500 * Type: <b>string</b><br> 1501 * Path: <b>Person.name</b><br> 1502 * </p> 1503 */ 1504 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 1505 1506 /** 1507 * Search parameter: <b>phone</b> 1508 * <p> 1509 * Description: <b>A value in a phone contact</b><br> 1510 * Type: <b>token</b><br> 1511 * Path: <b>Person.telecom(system=phone)</b><br> 1512 * </p> 1513 */ 1514 @SearchParamDefinition(name="phone", path="Person.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 1515 public static final String SP_PHONE = "phone"; 1516 /** 1517 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1518 * <p> 1519 * Description: <b>A value in a phone contact</b><br> 1520 * Type: <b>token</b><br> 1521 * Path: <b>Person.telecom(system=phone)</b><br> 1522 * </p> 1523 */ 1524 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 1525 1526 /** 1527 * Search parameter: <b>patient</b> 1528 * <p> 1529 * Description: <b>The Person links to this Patient</b><br> 1530 * Type: <b>reference</b><br> 1531 * Path: <b>Person.link.target</b><br> 1532 * </p> 1533 */ 1534 @SearchParamDefinition(name="patient", path="Person.link.target", description="The Person links to this Patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1535 public static final String SP_PATIENT = "patient"; 1536 /** 1537 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1538 * <p> 1539 * Description: <b>The Person links to this Patient</b><br> 1540 * Type: <b>reference</b><br> 1541 * Path: <b>Person.link.target</b><br> 1542 * </p> 1543 */ 1544 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1545 1546/** 1547 * Constant for fluent queries to be used to add include statements. Specifies 1548 * the path value of "<b>Person:patient</b>". 1549 */ 1550 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Person:patient").toLocked(); 1551 1552 /** 1553 * Search parameter: <b>organization</b> 1554 * <p> 1555 * Description: <b>The organization at which this person record is being managed</b><br> 1556 * Type: <b>reference</b><br> 1557 * Path: <b>Person.managingOrganization</b><br> 1558 * </p> 1559 */ 1560 @SearchParamDefinition(name="organization", path="Person.managingOrganization", description="The organization at which this person record is being managed", type="reference", target={Organization.class } ) 1561 public static final String SP_ORGANIZATION = "organization"; 1562 /** 1563 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1564 * <p> 1565 * Description: <b>The organization at which this person record is being managed</b><br> 1566 * Type: <b>reference</b><br> 1567 * Path: <b>Person.managingOrganization</b><br> 1568 * </p> 1569 */ 1570 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1571 1572/** 1573 * Constant for fluent queries to be used to add include statements. Specifies 1574 * the path value of "<b>Person:organization</b>". 1575 */ 1576 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Person:organization").toLocked(); 1577 1578 /** 1579 * Search parameter: <b>name</b> 1580 * <p> 1581 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1582 * Type: <b>string</b><br> 1583 * Path: <b>Person.name</b><br> 1584 * </p> 1585 */ 1586 @SearchParamDefinition(name="name", path="Person.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1587 public static final String SP_NAME = "name"; 1588 /** 1589 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1590 * <p> 1591 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1592 * Type: <b>string</b><br> 1593 * Path: <b>Person.name</b><br> 1594 * </p> 1595 */ 1596 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1597 1598 /** 1599 * Search parameter: <b>address-use</b> 1600 * <p> 1601 * Description: <b>A use code specified in an address</b><br> 1602 * Type: <b>token</b><br> 1603 * Path: <b>Person.address.use</b><br> 1604 * </p> 1605 */ 1606 @SearchParamDefinition(name="address-use", path="Person.address.use", description="A use code specified in an address", type="token" ) 1607 public static final String SP_ADDRESS_USE = "address-use"; 1608 /** 1609 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1610 * <p> 1611 * Description: <b>A use code specified in an address</b><br> 1612 * Type: <b>token</b><br> 1613 * Path: <b>Person.address.use</b><br> 1614 * </p> 1615 */ 1616 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1617 1618 /** 1619 * Search parameter: <b>telecom</b> 1620 * <p> 1621 * Description: <b>The value in any kind of contact</b><br> 1622 * Type: <b>token</b><br> 1623 * Path: <b>Person.telecom</b><br> 1624 * </p> 1625 */ 1626 @SearchParamDefinition(name="telecom", path="Person.telecom", description="The value in any kind of contact", type="token" ) 1627 public static final String SP_TELECOM = "telecom"; 1628 /** 1629 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1630 * <p> 1631 * Description: <b>The value in any kind of contact</b><br> 1632 * Type: <b>token</b><br> 1633 * Path: <b>Person.telecom</b><br> 1634 * </p> 1635 */ 1636 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 1637 1638 /** 1639 * Search parameter: <b>address-city</b> 1640 * <p> 1641 * Description: <b>A city specified in an address</b><br> 1642 * Type: <b>string</b><br> 1643 * Path: <b>Person.address.city</b><br> 1644 * </p> 1645 */ 1646 @SearchParamDefinition(name="address-city", path="Person.address.city", description="A city specified in an address", type="string" ) 1647 public static final String SP_ADDRESS_CITY = "address-city"; 1648 /** 1649 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1650 * <p> 1651 * Description: <b>A city specified in an address</b><br> 1652 * Type: <b>string</b><br> 1653 * Path: <b>Person.address.city</b><br> 1654 * </p> 1655 */ 1656 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1657 1658 /** 1659 * Search parameter: <b>email</b> 1660 * <p> 1661 * Description: <b>A value in an email contact</b><br> 1662 * Type: <b>token</b><br> 1663 * Path: <b>Person.telecom(system=email)</b><br> 1664 * </p> 1665 */ 1666 @SearchParamDefinition(name="email", path="Person.telecom.where(system='email')", description="A value in an email contact", type="token" ) 1667 public static final String SP_EMAIL = "email"; 1668 /** 1669 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1670 * <p> 1671 * Description: <b>A value in an email contact</b><br> 1672 * Type: <b>token</b><br> 1673 * Path: <b>Person.telecom(system=email)</b><br> 1674 * </p> 1675 */ 1676 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1677 1678 1679}