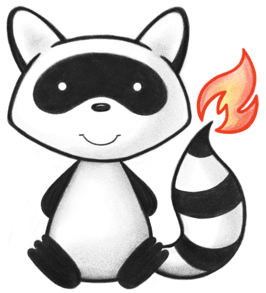
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 054 */ 055@ResourceDef(name="PlanDefinition", profile="http://hl7.org/fhir/Profile/PlanDefinition") 056@ChildOrder(names={"url", "identifier", "version", "name", "title", "type", "status", "experimental", "date", "publisher", "description", "purpose", "usage", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "topic", "contributor", "contact", "copyright", "relatedArtifact", "library", "goal", "action"}) 057public class PlanDefinition extends MetadataResource { 058 059 public enum ActionConditionKind { 060 /** 061 * The condition describes whether or not a given action is applicable 062 */ 063 APPLICABILITY, 064 /** 065 * The condition is a starting condition for the action 066 */ 067 START, 068 /** 069 * The condition is a stop, or exit condition for the action 070 */ 071 STOP, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("applicability".equals(codeString)) 080 return APPLICABILITY; 081 if ("start".equals(codeString)) 082 return START; 083 if ("stop".equals(codeString)) 084 return STOP; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case APPLICABILITY: return "applicability"; 093 case START: return "start"; 094 case STOP: return "stop"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 switch (this) { 101 case APPLICABILITY: return "http://hl7.org/fhir/action-condition-kind"; 102 case START: return "http://hl7.org/fhir/action-condition-kind"; 103 case STOP: return "http://hl7.org/fhir/action-condition-kind"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDefinition() { 109 switch (this) { 110 case APPLICABILITY: return "The condition describes whether or not a given action is applicable"; 111 case START: return "The condition is a starting condition for the action"; 112 case STOP: return "The condition is a stop, or exit condition for the action"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getDisplay() { 118 switch (this) { 119 case APPLICABILITY: return "Applicability"; 120 case START: return "Start"; 121 case STOP: return "Stop"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 } 127 128 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 129 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 130 if (codeString == null || "".equals(codeString)) 131 if (codeString == null || "".equals(codeString)) 132 return null; 133 if ("applicability".equals(codeString)) 134 return ActionConditionKind.APPLICABILITY; 135 if ("start".equals(codeString)) 136 return ActionConditionKind.START; 137 if ("stop".equals(codeString)) 138 return ActionConditionKind.STOP; 139 throw new IllegalArgumentException("Unknown ActionConditionKind code '"+codeString+"'"); 140 } 141 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 142 if (code == null) 143 return null; 144 if (code.isEmpty()) 145 return new Enumeration<ActionConditionKind>(this); 146 String codeString = code.asStringValue(); 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("applicability".equals(codeString)) 150 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY); 151 if ("start".equals(codeString)) 152 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START); 153 if ("stop".equals(codeString)) 154 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP); 155 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 156 } 157 public String toCode(ActionConditionKind code) { 158 if (code == ActionConditionKind.NULL) 159 return null; 160 if (code == ActionConditionKind.APPLICABILITY) 161 return "applicability"; 162 if (code == ActionConditionKind.START) 163 return "start"; 164 if (code == ActionConditionKind.STOP) 165 return "stop"; 166 return "?"; 167 } 168 public String toSystem(ActionConditionKind code) { 169 return code.getSystem(); 170 } 171 } 172 173 public enum ActionRelationshipType { 174 /** 175 * The action must be performed before the start of the related action 176 */ 177 BEFORESTART, 178 /** 179 * The action must be performed before the related action 180 */ 181 BEFORE, 182 /** 183 * The action must be performed before the end of the related action 184 */ 185 BEFOREEND, 186 /** 187 * The action must be performed concurrent with the start of the related action 188 */ 189 CONCURRENTWITHSTART, 190 /** 191 * The action must be performed concurrent with the related action 192 */ 193 CONCURRENT, 194 /** 195 * The action must be performed concurrent with the end of the related action 196 */ 197 CONCURRENTWITHEND, 198 /** 199 * The action must be performed after the start of the related action 200 */ 201 AFTERSTART, 202 /** 203 * The action must be performed after the related action 204 */ 205 AFTER, 206 /** 207 * The action must be performed after the end of the related action 208 */ 209 AFTEREND, 210 /** 211 * added to help the parsers with the generic types 212 */ 213 NULL; 214 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("before-start".equals(codeString)) 218 return BEFORESTART; 219 if ("before".equals(codeString)) 220 return BEFORE; 221 if ("before-end".equals(codeString)) 222 return BEFOREEND; 223 if ("concurrent-with-start".equals(codeString)) 224 return CONCURRENTWITHSTART; 225 if ("concurrent".equals(codeString)) 226 return CONCURRENT; 227 if ("concurrent-with-end".equals(codeString)) 228 return CONCURRENTWITHEND; 229 if ("after-start".equals(codeString)) 230 return AFTERSTART; 231 if ("after".equals(codeString)) 232 return AFTER; 233 if ("after-end".equals(codeString)) 234 return AFTEREND; 235 if (Configuration.isAcceptInvalidEnums()) 236 return null; 237 else 238 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 239 } 240 public String toCode() { 241 switch (this) { 242 case BEFORESTART: return "before-start"; 243 case BEFORE: return "before"; 244 case BEFOREEND: return "before-end"; 245 case CONCURRENTWITHSTART: return "concurrent-with-start"; 246 case CONCURRENT: return "concurrent"; 247 case CONCURRENTWITHEND: return "concurrent-with-end"; 248 case AFTERSTART: return "after-start"; 249 case AFTER: return "after"; 250 case AFTEREND: return "after-end"; 251 case NULL: return null; 252 default: return "?"; 253 } 254 } 255 public String getSystem() { 256 switch (this) { 257 case BEFORESTART: return "http://hl7.org/fhir/action-relationship-type"; 258 case BEFORE: return "http://hl7.org/fhir/action-relationship-type"; 259 case BEFOREEND: return "http://hl7.org/fhir/action-relationship-type"; 260 case CONCURRENTWITHSTART: return "http://hl7.org/fhir/action-relationship-type"; 261 case CONCURRENT: return "http://hl7.org/fhir/action-relationship-type"; 262 case CONCURRENTWITHEND: return "http://hl7.org/fhir/action-relationship-type"; 263 case AFTERSTART: return "http://hl7.org/fhir/action-relationship-type"; 264 case AFTER: return "http://hl7.org/fhir/action-relationship-type"; 265 case AFTEREND: return "http://hl7.org/fhir/action-relationship-type"; 266 case NULL: return null; 267 default: return "?"; 268 } 269 } 270 public String getDefinition() { 271 switch (this) { 272 case BEFORESTART: return "The action must be performed before the start of the related action"; 273 case BEFORE: return "The action must be performed before the related action"; 274 case BEFOREEND: return "The action must be performed before the end of the related action"; 275 case CONCURRENTWITHSTART: return "The action must be performed concurrent with the start of the related action"; 276 case CONCURRENT: return "The action must be performed concurrent with the related action"; 277 case CONCURRENTWITHEND: return "The action must be performed concurrent with the end of the related action"; 278 case AFTERSTART: return "The action must be performed after the start of the related action"; 279 case AFTER: return "The action must be performed after the related action"; 280 case AFTEREND: return "The action must be performed after the end of the related action"; 281 case NULL: return null; 282 default: return "?"; 283 } 284 } 285 public String getDisplay() { 286 switch (this) { 287 case BEFORESTART: return "Before Start"; 288 case BEFORE: return "Before"; 289 case BEFOREEND: return "Before End"; 290 case CONCURRENTWITHSTART: return "Concurrent With Start"; 291 case CONCURRENT: return "Concurrent"; 292 case CONCURRENTWITHEND: return "Concurrent With End"; 293 case AFTERSTART: return "After Start"; 294 case AFTER: return "After"; 295 case AFTEREND: return "After End"; 296 case NULL: return null; 297 default: return "?"; 298 } 299 } 300 } 301 302 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 303 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 304 if (codeString == null || "".equals(codeString)) 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("before-start".equals(codeString)) 308 return ActionRelationshipType.BEFORESTART; 309 if ("before".equals(codeString)) 310 return ActionRelationshipType.BEFORE; 311 if ("before-end".equals(codeString)) 312 return ActionRelationshipType.BEFOREEND; 313 if ("concurrent-with-start".equals(codeString)) 314 return ActionRelationshipType.CONCURRENTWITHSTART; 315 if ("concurrent".equals(codeString)) 316 return ActionRelationshipType.CONCURRENT; 317 if ("concurrent-with-end".equals(codeString)) 318 return ActionRelationshipType.CONCURRENTWITHEND; 319 if ("after-start".equals(codeString)) 320 return ActionRelationshipType.AFTERSTART; 321 if ("after".equals(codeString)) 322 return ActionRelationshipType.AFTER; 323 if ("after-end".equals(codeString)) 324 return ActionRelationshipType.AFTEREND; 325 throw new IllegalArgumentException("Unknown ActionRelationshipType code '"+codeString+"'"); 326 } 327 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 328 if (code == null) 329 return null; 330 if (code.isEmpty()) 331 return new Enumeration<ActionRelationshipType>(this); 332 String codeString = code.asStringValue(); 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("before-start".equals(codeString)) 336 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART); 337 if ("before".equals(codeString)) 338 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE); 339 if ("before-end".equals(codeString)) 340 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND); 341 if ("concurrent-with-start".equals(codeString)) 342 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART); 343 if ("concurrent".equals(codeString)) 344 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT); 345 if ("concurrent-with-end".equals(codeString)) 346 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND); 347 if ("after-start".equals(codeString)) 348 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART); 349 if ("after".equals(codeString)) 350 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER); 351 if ("after-end".equals(codeString)) 352 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND); 353 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 354 } 355 public String toCode(ActionRelationshipType code) { 356 if (code == ActionRelationshipType.NULL) 357 return null; 358 if (code == ActionRelationshipType.BEFORESTART) 359 return "before-start"; 360 if (code == ActionRelationshipType.BEFORE) 361 return "before"; 362 if (code == ActionRelationshipType.BEFOREEND) 363 return "before-end"; 364 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 365 return "concurrent-with-start"; 366 if (code == ActionRelationshipType.CONCURRENT) 367 return "concurrent"; 368 if (code == ActionRelationshipType.CONCURRENTWITHEND) 369 return "concurrent-with-end"; 370 if (code == ActionRelationshipType.AFTERSTART) 371 return "after-start"; 372 if (code == ActionRelationshipType.AFTER) 373 return "after"; 374 if (code == ActionRelationshipType.AFTEREND) 375 return "after-end"; 376 return "?"; 377 } 378 public String toSystem(ActionRelationshipType code) { 379 return code.getSystem(); 380 } 381 } 382 383 public enum ActionParticipantType { 384 /** 385 * The participant is the patient under evaluation 386 */ 387 PATIENT, 388 /** 389 * The participant is a practitioner involved in the patient's care 390 */ 391 PRACTITIONER, 392 /** 393 * The participant is a person related to the patient 394 */ 395 RELATEDPERSON, 396 /** 397 * added to help the parsers with the generic types 398 */ 399 NULL; 400 public static ActionParticipantType fromCode(String codeString) throws FHIRException { 401 if (codeString == null || "".equals(codeString)) 402 return null; 403 if ("patient".equals(codeString)) 404 return PATIENT; 405 if ("practitioner".equals(codeString)) 406 return PRACTITIONER; 407 if ("related-person".equals(codeString)) 408 return RELATEDPERSON; 409 if (Configuration.isAcceptInvalidEnums()) 410 return null; 411 else 412 throw new FHIRException("Unknown ActionParticipantType code '"+codeString+"'"); 413 } 414 public String toCode() { 415 switch (this) { 416 case PATIENT: return "patient"; 417 case PRACTITIONER: return "practitioner"; 418 case RELATEDPERSON: return "related-person"; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 public String getSystem() { 424 switch (this) { 425 case PATIENT: return "http://hl7.org/fhir/action-participant-type"; 426 case PRACTITIONER: return "http://hl7.org/fhir/action-participant-type"; 427 case RELATEDPERSON: return "http://hl7.org/fhir/action-participant-type"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 public String getDefinition() { 433 switch (this) { 434 case PATIENT: return "The participant is the patient under evaluation"; 435 case PRACTITIONER: return "The participant is a practitioner involved in the patient's care"; 436 case RELATEDPERSON: return "The participant is a person related to the patient"; 437 case NULL: return null; 438 default: return "?"; 439 } 440 } 441 public String getDisplay() { 442 switch (this) { 443 case PATIENT: return "Patient"; 444 case PRACTITIONER: return "Practitioner"; 445 case RELATEDPERSON: return "Related Person"; 446 case NULL: return null; 447 default: return "?"; 448 } 449 } 450 } 451 452 public static class ActionParticipantTypeEnumFactory implements EnumFactory<ActionParticipantType> { 453 public ActionParticipantType fromCode(String codeString) throws IllegalArgumentException { 454 if (codeString == null || "".equals(codeString)) 455 if (codeString == null || "".equals(codeString)) 456 return null; 457 if ("patient".equals(codeString)) 458 return ActionParticipantType.PATIENT; 459 if ("practitioner".equals(codeString)) 460 return ActionParticipantType.PRACTITIONER; 461 if ("related-person".equals(codeString)) 462 return ActionParticipantType.RELATEDPERSON; 463 throw new IllegalArgumentException("Unknown ActionParticipantType code '"+codeString+"'"); 464 } 465 public Enumeration<ActionParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 466 if (code == null) 467 return null; 468 if (code.isEmpty()) 469 return new Enumeration<ActionParticipantType>(this); 470 String codeString = code.asStringValue(); 471 if (codeString == null || "".equals(codeString)) 472 return null; 473 if ("patient".equals(codeString)) 474 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PATIENT); 475 if ("practitioner".equals(codeString)) 476 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PRACTITIONER); 477 if ("related-person".equals(codeString)) 478 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.RELATEDPERSON); 479 throw new FHIRException("Unknown ActionParticipantType code '"+codeString+"'"); 480 } 481 public String toCode(ActionParticipantType code) { 482 if (code == ActionParticipantType.NULL) 483 return null; 484 if (code == ActionParticipantType.PATIENT) 485 return "patient"; 486 if (code == ActionParticipantType.PRACTITIONER) 487 return "practitioner"; 488 if (code == ActionParticipantType.RELATEDPERSON) 489 return "related-person"; 490 return "?"; 491 } 492 public String toSystem(ActionParticipantType code) { 493 return code.getSystem(); 494 } 495 } 496 497 public enum ActionGroupingBehavior { 498 /** 499 * Any group marked with this behavior should be displayed as a visual group to the end user 500 */ 501 VISUALGROUP, 502 /** 503 * A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so 504 */ 505 LOGICALGROUP, 506 /** 507 * A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of "AtMostOne", unless it's a required action, in which case, it would be "ExactlyOne" 508 */ 509 SENTENCEGROUP, 510 /** 511 * added to help the parsers with the generic types 512 */ 513 NULL; 514 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 515 if (codeString == null || "".equals(codeString)) 516 return null; 517 if ("visual-group".equals(codeString)) 518 return VISUALGROUP; 519 if ("logical-group".equals(codeString)) 520 return LOGICALGROUP; 521 if ("sentence-group".equals(codeString)) 522 return SENTENCEGROUP; 523 if (Configuration.isAcceptInvalidEnums()) 524 return null; 525 else 526 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 527 } 528 public String toCode() { 529 switch (this) { 530 case VISUALGROUP: return "visual-group"; 531 case LOGICALGROUP: return "logical-group"; 532 case SENTENCEGROUP: return "sentence-group"; 533 case NULL: return null; 534 default: return "?"; 535 } 536 } 537 public String getSystem() { 538 switch (this) { 539 case VISUALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 540 case LOGICALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 541 case SENTENCEGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 542 case NULL: return null; 543 default: return "?"; 544 } 545 } 546 public String getDefinition() { 547 switch (this) { 548 case VISUALGROUP: return "Any group marked with this behavior should be displayed as a visual group to the end user"; 549 case LOGICALGROUP: return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so"; 550 case SENTENCEGROUP: return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\""; 551 case NULL: return null; 552 default: return "?"; 553 } 554 } 555 public String getDisplay() { 556 switch (this) { 557 case VISUALGROUP: return "Visual Group"; 558 case LOGICALGROUP: return "Logical Group"; 559 case SENTENCEGROUP: return "Sentence Group"; 560 case NULL: return null; 561 default: return "?"; 562 } 563 } 564 } 565 566 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 567 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 568 if (codeString == null || "".equals(codeString)) 569 if (codeString == null || "".equals(codeString)) 570 return null; 571 if ("visual-group".equals(codeString)) 572 return ActionGroupingBehavior.VISUALGROUP; 573 if ("logical-group".equals(codeString)) 574 return ActionGroupingBehavior.LOGICALGROUP; 575 if ("sentence-group".equals(codeString)) 576 return ActionGroupingBehavior.SENTENCEGROUP; 577 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 578 } 579 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 580 if (code == null) 581 return null; 582 if (code.isEmpty()) 583 return new Enumeration<ActionGroupingBehavior>(this); 584 String codeString = code.asStringValue(); 585 if (codeString == null || "".equals(codeString)) 586 return null; 587 if ("visual-group".equals(codeString)) 588 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP); 589 if ("logical-group".equals(codeString)) 590 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP); 591 if ("sentence-group".equals(codeString)) 592 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP); 593 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 594 } 595 public String toCode(ActionGroupingBehavior code) { 596 if (code == ActionGroupingBehavior.NULL) 597 return null; 598 if (code == ActionGroupingBehavior.VISUALGROUP) 599 return "visual-group"; 600 if (code == ActionGroupingBehavior.LOGICALGROUP) 601 return "logical-group"; 602 if (code == ActionGroupingBehavior.SENTENCEGROUP) 603 return "sentence-group"; 604 return "?"; 605 } 606 public String toSystem(ActionGroupingBehavior code) { 607 return code.getSystem(); 608 } 609 } 610 611 public enum ActionSelectionBehavior { 612 /** 613 * Any number of the actions in the group may be chosen, from zero to all 614 */ 615 ANY, 616 /** 617 * All the actions in the group must be selected as a single unit 618 */ 619 ALL, 620 /** 621 * All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected 622 */ 623 ALLORNONE, 624 /** 625 * The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group 626 */ 627 EXACTLYONE, 628 /** 629 * The end user may choose zero or at most one of the actions in the group 630 */ 631 ATMOSTONE, 632 /** 633 * The end user must choose a minimum of one, and as many additional as desired 634 */ 635 ONEORMORE, 636 /** 637 * added to help the parsers with the generic types 638 */ 639 NULL; 640 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 641 if (codeString == null || "".equals(codeString)) 642 return null; 643 if ("any".equals(codeString)) 644 return ANY; 645 if ("all".equals(codeString)) 646 return ALL; 647 if ("all-or-none".equals(codeString)) 648 return ALLORNONE; 649 if ("exactly-one".equals(codeString)) 650 return EXACTLYONE; 651 if ("at-most-one".equals(codeString)) 652 return ATMOSTONE; 653 if ("one-or-more".equals(codeString)) 654 return ONEORMORE; 655 if (Configuration.isAcceptInvalidEnums()) 656 return null; 657 else 658 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 659 } 660 public String toCode() { 661 switch (this) { 662 case ANY: return "any"; 663 case ALL: return "all"; 664 case ALLORNONE: return "all-or-none"; 665 case EXACTLYONE: return "exactly-one"; 666 case ATMOSTONE: return "at-most-one"; 667 case ONEORMORE: return "one-or-more"; 668 case NULL: return null; 669 default: return "?"; 670 } 671 } 672 public String getSystem() { 673 switch (this) { 674 case ANY: return "http://hl7.org/fhir/action-selection-behavior"; 675 case ALL: return "http://hl7.org/fhir/action-selection-behavior"; 676 case ALLORNONE: return "http://hl7.org/fhir/action-selection-behavior"; 677 case EXACTLYONE: return "http://hl7.org/fhir/action-selection-behavior"; 678 case ATMOSTONE: return "http://hl7.org/fhir/action-selection-behavior"; 679 case ONEORMORE: return "http://hl7.org/fhir/action-selection-behavior"; 680 case NULL: return null; 681 default: return "?"; 682 } 683 } 684 public String getDefinition() { 685 switch (this) { 686 case ANY: return "Any number of the actions in the group may be chosen, from zero to all"; 687 case ALL: return "All the actions in the group must be selected as a single unit"; 688 case ALLORNONE: return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected"; 689 case EXACTLYONE: return "The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group"; 690 case ATMOSTONE: return "The end user may choose zero or at most one of the actions in the group"; 691 case ONEORMORE: return "The end user must choose a minimum of one, and as many additional as desired"; 692 case NULL: return null; 693 default: return "?"; 694 } 695 } 696 public String getDisplay() { 697 switch (this) { 698 case ANY: return "Any"; 699 case ALL: return "All"; 700 case ALLORNONE: return "All Or None"; 701 case EXACTLYONE: return "Exactly One"; 702 case ATMOSTONE: return "At Most One"; 703 case ONEORMORE: return "One Or More"; 704 case NULL: return null; 705 default: return "?"; 706 } 707 } 708 } 709 710 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 711 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 712 if (codeString == null || "".equals(codeString)) 713 if (codeString == null || "".equals(codeString)) 714 return null; 715 if ("any".equals(codeString)) 716 return ActionSelectionBehavior.ANY; 717 if ("all".equals(codeString)) 718 return ActionSelectionBehavior.ALL; 719 if ("all-or-none".equals(codeString)) 720 return ActionSelectionBehavior.ALLORNONE; 721 if ("exactly-one".equals(codeString)) 722 return ActionSelectionBehavior.EXACTLYONE; 723 if ("at-most-one".equals(codeString)) 724 return ActionSelectionBehavior.ATMOSTONE; 725 if ("one-or-more".equals(codeString)) 726 return ActionSelectionBehavior.ONEORMORE; 727 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 728 } 729 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 730 if (code == null) 731 return null; 732 if (code.isEmpty()) 733 return new Enumeration<ActionSelectionBehavior>(this); 734 String codeString = code.asStringValue(); 735 if (codeString == null || "".equals(codeString)) 736 return null; 737 if ("any".equals(codeString)) 738 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY); 739 if ("all".equals(codeString)) 740 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL); 741 if ("all-or-none".equals(codeString)) 742 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE); 743 if ("exactly-one".equals(codeString)) 744 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE); 745 if ("at-most-one".equals(codeString)) 746 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE); 747 if ("one-or-more".equals(codeString)) 748 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE); 749 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 750 } 751 public String toCode(ActionSelectionBehavior code) { 752 if (code == ActionSelectionBehavior.NULL) 753 return null; 754 if (code == ActionSelectionBehavior.ANY) 755 return "any"; 756 if (code == ActionSelectionBehavior.ALL) 757 return "all"; 758 if (code == ActionSelectionBehavior.ALLORNONE) 759 return "all-or-none"; 760 if (code == ActionSelectionBehavior.EXACTLYONE) 761 return "exactly-one"; 762 if (code == ActionSelectionBehavior.ATMOSTONE) 763 return "at-most-one"; 764 if (code == ActionSelectionBehavior.ONEORMORE) 765 return "one-or-more"; 766 return "?"; 767 } 768 public String toSystem(ActionSelectionBehavior code) { 769 return code.getSystem(); 770 } 771 } 772 773 public enum ActionRequiredBehavior { 774 /** 775 * An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action 776 */ 777 MUST, 778 /** 779 * An action with this behavior may be included in the set of actions processed by the end user 780 */ 781 COULD, 782 /** 783 * An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included 784 */ 785 MUSTUNLESSDOCUMENTED, 786 /** 787 * added to help the parsers with the generic types 788 */ 789 NULL; 790 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 791 if (codeString == null || "".equals(codeString)) 792 return null; 793 if ("must".equals(codeString)) 794 return MUST; 795 if ("could".equals(codeString)) 796 return COULD; 797 if ("must-unless-documented".equals(codeString)) 798 return MUSTUNLESSDOCUMENTED; 799 if (Configuration.isAcceptInvalidEnums()) 800 return null; 801 else 802 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 803 } 804 public String toCode() { 805 switch (this) { 806 case MUST: return "must"; 807 case COULD: return "could"; 808 case MUSTUNLESSDOCUMENTED: return "must-unless-documented"; 809 case NULL: return null; 810 default: return "?"; 811 } 812 } 813 public String getSystem() { 814 switch (this) { 815 case MUST: return "http://hl7.org/fhir/action-required-behavior"; 816 case COULD: return "http://hl7.org/fhir/action-required-behavior"; 817 case MUSTUNLESSDOCUMENTED: return "http://hl7.org/fhir/action-required-behavior"; 818 case NULL: return null; 819 default: return "?"; 820 } 821 } 822 public String getDefinition() { 823 switch (this) { 824 case MUST: return "An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action"; 825 case COULD: return "An action with this behavior may be included in the set of actions processed by the end user"; 826 case MUSTUNLESSDOCUMENTED: return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included"; 827 case NULL: return null; 828 default: return "?"; 829 } 830 } 831 public String getDisplay() { 832 switch (this) { 833 case MUST: return "Must"; 834 case COULD: return "Could"; 835 case MUSTUNLESSDOCUMENTED: return "Must Unless Documented"; 836 case NULL: return null; 837 default: return "?"; 838 } 839 } 840 } 841 842 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 843 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 844 if (codeString == null || "".equals(codeString)) 845 if (codeString == null || "".equals(codeString)) 846 return null; 847 if ("must".equals(codeString)) 848 return ActionRequiredBehavior.MUST; 849 if ("could".equals(codeString)) 850 return ActionRequiredBehavior.COULD; 851 if ("must-unless-documented".equals(codeString)) 852 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 853 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 854 } 855 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 856 if (code == null) 857 return null; 858 if (code.isEmpty()) 859 return new Enumeration<ActionRequiredBehavior>(this); 860 String codeString = code.asStringValue(); 861 if (codeString == null || "".equals(codeString)) 862 return null; 863 if ("must".equals(codeString)) 864 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST); 865 if ("could".equals(codeString)) 866 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD); 867 if ("must-unless-documented".equals(codeString)) 868 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED); 869 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 870 } 871 public String toCode(ActionRequiredBehavior code) { 872 if (code == ActionRequiredBehavior.NULL) 873 return null; 874 if (code == ActionRequiredBehavior.MUST) 875 return "must"; 876 if (code == ActionRequiredBehavior.COULD) 877 return "could"; 878 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 879 return "must-unless-documented"; 880 return "?"; 881 } 882 public String toSystem(ActionRequiredBehavior code) { 883 return code.getSystem(); 884 } 885 } 886 887 public enum ActionPrecheckBehavior { 888 /** 889 * An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider "pre-checking" such an action as a convenience for the user 890 */ 891 YES, 892 /** 893 * An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not "pre-check" such an action 894 */ 895 NO, 896 /** 897 * added to help the parsers with the generic types 898 */ 899 NULL; 900 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 901 if (codeString == null || "".equals(codeString)) 902 return null; 903 if ("yes".equals(codeString)) 904 return YES; 905 if ("no".equals(codeString)) 906 return NO; 907 if (Configuration.isAcceptInvalidEnums()) 908 return null; 909 else 910 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 911 } 912 public String toCode() { 913 switch (this) { 914 case YES: return "yes"; 915 case NO: return "no"; 916 case NULL: return null; 917 default: return "?"; 918 } 919 } 920 public String getSystem() { 921 switch (this) { 922 case YES: return "http://hl7.org/fhir/action-precheck-behavior"; 923 case NO: return "http://hl7.org/fhir/action-precheck-behavior"; 924 case NULL: return null; 925 default: return "?"; 926 } 927 } 928 public String getDefinition() { 929 switch (this) { 930 case YES: return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user"; 931 case NO: return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action"; 932 case NULL: return null; 933 default: return "?"; 934 } 935 } 936 public String getDisplay() { 937 switch (this) { 938 case YES: return "Yes"; 939 case NO: return "No"; 940 case NULL: return null; 941 default: return "?"; 942 } 943 } 944 } 945 946 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 947 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 948 if (codeString == null || "".equals(codeString)) 949 if (codeString == null || "".equals(codeString)) 950 return null; 951 if ("yes".equals(codeString)) 952 return ActionPrecheckBehavior.YES; 953 if ("no".equals(codeString)) 954 return ActionPrecheckBehavior.NO; 955 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 956 } 957 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 958 if (code == null) 959 return null; 960 if (code.isEmpty()) 961 return new Enumeration<ActionPrecheckBehavior>(this); 962 String codeString = code.asStringValue(); 963 if (codeString == null || "".equals(codeString)) 964 return null; 965 if ("yes".equals(codeString)) 966 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES); 967 if ("no".equals(codeString)) 968 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO); 969 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 970 } 971 public String toCode(ActionPrecheckBehavior code) { 972 if (code == ActionPrecheckBehavior.NULL) 973 return null; 974 if (code == ActionPrecheckBehavior.YES) 975 return "yes"; 976 if (code == ActionPrecheckBehavior.NO) 977 return "no"; 978 return "?"; 979 } 980 public String toSystem(ActionPrecheckBehavior code) { 981 return code.getSystem(); 982 } 983 } 984 985 public enum ActionCardinalityBehavior { 986 /** 987 * The action may only be selected one time 988 */ 989 SINGLE, 990 /** 991 * The action may be selected multiple times 992 */ 993 MULTIPLE, 994 /** 995 * added to help the parsers with the generic types 996 */ 997 NULL; 998 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 999 if (codeString == null || "".equals(codeString)) 1000 return null; 1001 if ("single".equals(codeString)) 1002 return SINGLE; 1003 if ("multiple".equals(codeString)) 1004 return MULTIPLE; 1005 if (Configuration.isAcceptInvalidEnums()) 1006 return null; 1007 else 1008 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1009 } 1010 public String toCode() { 1011 switch (this) { 1012 case SINGLE: return "single"; 1013 case MULTIPLE: return "multiple"; 1014 case NULL: return null; 1015 default: return "?"; 1016 } 1017 } 1018 public String getSystem() { 1019 switch (this) { 1020 case SINGLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1021 case MULTIPLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1022 case NULL: return null; 1023 default: return "?"; 1024 } 1025 } 1026 public String getDefinition() { 1027 switch (this) { 1028 case SINGLE: return "The action may only be selected one time"; 1029 case MULTIPLE: return "The action may be selected multiple times"; 1030 case NULL: return null; 1031 default: return "?"; 1032 } 1033 } 1034 public String getDisplay() { 1035 switch (this) { 1036 case SINGLE: return "Single"; 1037 case MULTIPLE: return "Multiple"; 1038 case NULL: return null; 1039 default: return "?"; 1040 } 1041 } 1042 } 1043 1044 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1045 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1046 if (codeString == null || "".equals(codeString)) 1047 if (codeString == null || "".equals(codeString)) 1048 return null; 1049 if ("single".equals(codeString)) 1050 return ActionCardinalityBehavior.SINGLE; 1051 if ("multiple".equals(codeString)) 1052 return ActionCardinalityBehavior.MULTIPLE; 1053 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1054 } 1055 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1056 if (code == null) 1057 return null; 1058 if (code.isEmpty()) 1059 return new Enumeration<ActionCardinalityBehavior>(this); 1060 String codeString = code.asStringValue(); 1061 if (codeString == null || "".equals(codeString)) 1062 return null; 1063 if ("single".equals(codeString)) 1064 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE); 1065 if ("multiple".equals(codeString)) 1066 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE); 1067 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1068 } 1069 public String toCode(ActionCardinalityBehavior code) { 1070 if (code == ActionCardinalityBehavior.NULL) 1071 return null; 1072 if (code == ActionCardinalityBehavior.SINGLE) 1073 return "single"; 1074 if (code == ActionCardinalityBehavior.MULTIPLE) 1075 return "multiple"; 1076 return "?"; 1077 } 1078 public String toSystem(ActionCardinalityBehavior code) { 1079 return code.getSystem(); 1080 } 1081 } 1082 1083 @Block() 1084 public static class PlanDefinitionGoalComponent extends BackboneElement implements IBaseBackboneElement { 1085 /** 1086 * Indicates a category the goal falls within. 1087 */ 1088 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1089 @Description(shortDefinition="E.g. Treatment, dietary, behavioral, etc", formalDefinition="Indicates a category the goal falls within." ) 1090 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-category") 1091 protected CodeableConcept category; 1092 1093 /** 1094 * Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding". 1095 */ 1096 @Child(name = "description", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1097 @Description(shortDefinition="Code or text describing the goal", formalDefinition="Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\"." ) 1098 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 1099 protected CodeableConcept description; 1100 1101 /** 1102 * Identifies the expected level of importance associated with reaching/sustaining the defined goal. 1103 */ 1104 @Child(name = "priority", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1105 @Description(shortDefinition="high-priority | medium-priority | low-priority", formalDefinition="Identifies the expected level of importance associated with reaching/sustaining the defined goal." ) 1106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-priority") 1107 protected CodeableConcept priority; 1108 1109 /** 1110 * The event after which the goal should begin being pursued. 1111 */ 1112 @Child(name = "start", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1113 @Description(shortDefinition="When goal pursuit begins", formalDefinition="The event after which the goal should begin being pursued." ) 1114 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-start-event") 1115 protected CodeableConcept start; 1116 1117 /** 1118 * Identifies problems, conditions, issues, or concerns the goal is intended to address. 1119 */ 1120 @Child(name = "addresses", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1121 @Description(shortDefinition="What does the goal address", formalDefinition="Identifies problems, conditions, issues, or concerns the goal is intended to address." ) 1122 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1123 protected List<CodeableConcept> addresses; 1124 1125 /** 1126 * Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources. 1127 */ 1128 @Child(name = "documentation", type = {RelatedArtifact.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1129 @Description(shortDefinition="Supporting documentation for the goal", formalDefinition="Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources." ) 1130 protected List<RelatedArtifact> documentation; 1131 1132 /** 1133 * Indicates what should be done and within what timeframe. 1134 */ 1135 @Child(name = "target", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1136 @Description(shortDefinition="Target outcome for the goal", formalDefinition="Indicates what should be done and within what timeframe." ) 1137 protected List<PlanDefinitionGoalTargetComponent> target; 1138 1139 private static final long serialVersionUID = -795308926L; 1140 1141 /** 1142 * Constructor 1143 */ 1144 public PlanDefinitionGoalComponent() { 1145 super(); 1146 } 1147 1148 /** 1149 * Constructor 1150 */ 1151 public PlanDefinitionGoalComponent(CodeableConcept description) { 1152 super(); 1153 this.description = description; 1154 } 1155 1156 /** 1157 * @return {@link #category} (Indicates a category the goal falls within.) 1158 */ 1159 public CodeableConcept getCategory() { 1160 if (this.category == null) 1161 if (Configuration.errorOnAutoCreate()) 1162 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.category"); 1163 else if (Configuration.doAutoCreate()) 1164 this.category = new CodeableConcept(); // cc 1165 return this.category; 1166 } 1167 1168 public boolean hasCategory() { 1169 return this.category != null && !this.category.isEmpty(); 1170 } 1171 1172 /** 1173 * @param value {@link #category} (Indicates a category the goal falls within.) 1174 */ 1175 public PlanDefinitionGoalComponent setCategory(CodeableConcept value) { 1176 this.category = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 1182 */ 1183 public CodeableConcept getDescription() { 1184 if (this.description == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.description"); 1187 else if (Configuration.doAutoCreate()) 1188 this.description = new CodeableConcept(); // cc 1189 return this.description; 1190 } 1191 1192 public boolean hasDescription() { 1193 return this.description != null && !this.description.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 1198 */ 1199 public PlanDefinitionGoalComponent setDescription(CodeableConcept value) { 1200 this.description = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #priority} (Identifies the expected level of importance associated with reaching/sustaining the defined goal.) 1206 */ 1207 public CodeableConcept getPriority() { 1208 if (this.priority == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.priority"); 1211 else if (Configuration.doAutoCreate()) 1212 this.priority = new CodeableConcept(); // cc 1213 return this.priority; 1214 } 1215 1216 public boolean hasPriority() { 1217 return this.priority != null && !this.priority.isEmpty(); 1218 } 1219 1220 /** 1221 * @param value {@link #priority} (Identifies the expected level of importance associated with reaching/sustaining the defined goal.) 1222 */ 1223 public PlanDefinitionGoalComponent setPriority(CodeableConcept value) { 1224 this.priority = value; 1225 return this; 1226 } 1227 1228 /** 1229 * @return {@link #start} (The event after which the goal should begin being pursued.) 1230 */ 1231 public CodeableConcept getStart() { 1232 if (this.start == null) 1233 if (Configuration.errorOnAutoCreate()) 1234 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.start"); 1235 else if (Configuration.doAutoCreate()) 1236 this.start = new CodeableConcept(); // cc 1237 return this.start; 1238 } 1239 1240 public boolean hasStart() { 1241 return this.start != null && !this.start.isEmpty(); 1242 } 1243 1244 /** 1245 * @param value {@link #start} (The event after which the goal should begin being pursued.) 1246 */ 1247 public PlanDefinitionGoalComponent setStart(CodeableConcept value) { 1248 this.start = value; 1249 return this; 1250 } 1251 1252 /** 1253 * @return {@link #addresses} (Identifies problems, conditions, issues, or concerns the goal is intended to address.) 1254 */ 1255 public List<CodeableConcept> getAddresses() { 1256 if (this.addresses == null) 1257 this.addresses = new ArrayList<CodeableConcept>(); 1258 return this.addresses; 1259 } 1260 1261 /** 1262 * @return Returns a reference to <code>this</code> for easy method chaining 1263 */ 1264 public PlanDefinitionGoalComponent setAddresses(List<CodeableConcept> theAddresses) { 1265 this.addresses = theAddresses; 1266 return this; 1267 } 1268 1269 public boolean hasAddresses() { 1270 if (this.addresses == null) 1271 return false; 1272 for (CodeableConcept item : this.addresses) 1273 if (!item.isEmpty()) 1274 return true; 1275 return false; 1276 } 1277 1278 public CodeableConcept addAddresses() { //3 1279 CodeableConcept t = new CodeableConcept(); 1280 if (this.addresses == null) 1281 this.addresses = new ArrayList<CodeableConcept>(); 1282 this.addresses.add(t); 1283 return t; 1284 } 1285 1286 public PlanDefinitionGoalComponent addAddresses(CodeableConcept t) { //3 1287 if (t == null) 1288 return this; 1289 if (this.addresses == null) 1290 this.addresses = new ArrayList<CodeableConcept>(); 1291 this.addresses.add(t); 1292 return this; 1293 } 1294 1295 /** 1296 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist 1297 */ 1298 public CodeableConcept getAddressesFirstRep() { 1299 if (getAddresses().isEmpty()) { 1300 addAddresses(); 1301 } 1302 return getAddresses().get(0); 1303 } 1304 1305 /** 1306 * @return {@link #documentation} (Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.) 1307 */ 1308 public List<RelatedArtifact> getDocumentation() { 1309 if (this.documentation == null) 1310 this.documentation = new ArrayList<RelatedArtifact>(); 1311 return this.documentation; 1312 } 1313 1314 /** 1315 * @return Returns a reference to <code>this</code> for easy method chaining 1316 */ 1317 public PlanDefinitionGoalComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 1318 this.documentation = theDocumentation; 1319 return this; 1320 } 1321 1322 public boolean hasDocumentation() { 1323 if (this.documentation == null) 1324 return false; 1325 for (RelatedArtifact item : this.documentation) 1326 if (!item.isEmpty()) 1327 return true; 1328 return false; 1329 } 1330 1331 public RelatedArtifact addDocumentation() { //3 1332 RelatedArtifact t = new RelatedArtifact(); 1333 if (this.documentation == null) 1334 this.documentation = new ArrayList<RelatedArtifact>(); 1335 this.documentation.add(t); 1336 return t; 1337 } 1338 1339 public PlanDefinitionGoalComponent addDocumentation(RelatedArtifact t) { //3 1340 if (t == null) 1341 return this; 1342 if (this.documentation == null) 1343 this.documentation = new ArrayList<RelatedArtifact>(); 1344 this.documentation.add(t); 1345 return this; 1346 } 1347 1348 /** 1349 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist 1350 */ 1351 public RelatedArtifact getDocumentationFirstRep() { 1352 if (getDocumentation().isEmpty()) { 1353 addDocumentation(); 1354 } 1355 return getDocumentation().get(0); 1356 } 1357 1358 /** 1359 * @return {@link #target} (Indicates what should be done and within what timeframe.) 1360 */ 1361 public List<PlanDefinitionGoalTargetComponent> getTarget() { 1362 if (this.target == null) 1363 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1364 return this.target; 1365 } 1366 1367 /** 1368 * @return Returns a reference to <code>this</code> for easy method chaining 1369 */ 1370 public PlanDefinitionGoalComponent setTarget(List<PlanDefinitionGoalTargetComponent> theTarget) { 1371 this.target = theTarget; 1372 return this; 1373 } 1374 1375 public boolean hasTarget() { 1376 if (this.target == null) 1377 return false; 1378 for (PlanDefinitionGoalTargetComponent item : this.target) 1379 if (!item.isEmpty()) 1380 return true; 1381 return false; 1382 } 1383 1384 public PlanDefinitionGoalTargetComponent addTarget() { //3 1385 PlanDefinitionGoalTargetComponent t = new PlanDefinitionGoalTargetComponent(); 1386 if (this.target == null) 1387 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1388 this.target.add(t); 1389 return t; 1390 } 1391 1392 public PlanDefinitionGoalComponent addTarget(PlanDefinitionGoalTargetComponent t) { //3 1393 if (t == null) 1394 return this; 1395 if (this.target == null) 1396 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1397 this.target.add(t); 1398 return this; 1399 } 1400 1401 /** 1402 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist 1403 */ 1404 public PlanDefinitionGoalTargetComponent getTargetFirstRep() { 1405 if (getTarget().isEmpty()) { 1406 addTarget(); 1407 } 1408 return getTarget().get(0); 1409 } 1410 1411 protected void listChildren(List<Property> children) { 1412 super.listChildren(children); 1413 children.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category)); 1414 children.add(new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description)); 1415 children.add(new Property("priority", "CodeableConcept", "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, priority)); 1416 children.add(new Property("start", "CodeableConcept", "The event after which the goal should begin being pursued.", 0, 1, start)); 1417 children.add(new Property("addresses", "CodeableConcept", "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, java.lang.Integer.MAX_VALUE, addresses)); 1418 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 1419 children.add(new Property("target", "", "Indicates what should be done and within what timeframe.", 0, java.lang.Integer.MAX_VALUE, target)); 1420 } 1421 1422 @Override 1423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1424 switch (_hash) { 1425 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category); 1426 case -1724546052: /*description*/ return new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description); 1427 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, priority); 1428 case 109757538: /*start*/ return new Property("start", "CodeableConcept", "The event after which the goal should begin being pursued.", 0, 1, start); 1429 case 874544034: /*addresses*/ return new Property("addresses", "CodeableConcept", "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, java.lang.Integer.MAX_VALUE, addresses); 1430 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 1431 case -880905839: /*target*/ return new Property("target", "", "Indicates what should be done and within what timeframe.", 0, java.lang.Integer.MAX_VALUE, target); 1432 default: return super.getNamedProperty(_hash, _name, _checkValid); 1433 } 1434 1435 } 1436 1437 @Override 1438 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1439 switch (hash) { 1440 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1441 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // CodeableConcept 1442 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 1443 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // CodeableConcept 1444 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // CodeableConcept 1445 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 1446 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // PlanDefinitionGoalTargetComponent 1447 default: return super.getProperty(hash, name, checkValid); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1454 switch (hash) { 1455 case 50511102: // category 1456 this.category = castToCodeableConcept(value); // CodeableConcept 1457 return value; 1458 case -1724546052: // description 1459 this.description = castToCodeableConcept(value); // CodeableConcept 1460 return value; 1461 case -1165461084: // priority 1462 this.priority = castToCodeableConcept(value); // CodeableConcept 1463 return value; 1464 case 109757538: // start 1465 this.start = castToCodeableConcept(value); // CodeableConcept 1466 return value; 1467 case 874544034: // addresses 1468 this.getAddresses().add(castToCodeableConcept(value)); // CodeableConcept 1469 return value; 1470 case 1587405498: // documentation 1471 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 1472 return value; 1473 case -880905839: // target 1474 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); // PlanDefinitionGoalTargetComponent 1475 return value; 1476 default: return super.setProperty(hash, name, value); 1477 } 1478 1479 } 1480 1481 @Override 1482 public Base setProperty(String name, Base value) throws FHIRException { 1483 if (name.equals("category")) { 1484 this.category = castToCodeableConcept(value); // CodeableConcept 1485 } else if (name.equals("description")) { 1486 this.description = castToCodeableConcept(value); // CodeableConcept 1487 } else if (name.equals("priority")) { 1488 this.priority = castToCodeableConcept(value); // CodeableConcept 1489 } else if (name.equals("start")) { 1490 this.start = castToCodeableConcept(value); // CodeableConcept 1491 } else if (name.equals("addresses")) { 1492 this.getAddresses().add(castToCodeableConcept(value)); 1493 } else if (name.equals("documentation")) { 1494 this.getDocumentation().add(castToRelatedArtifact(value)); 1495 } else if (name.equals("target")) { 1496 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); 1497 } else 1498 return super.setProperty(name, value); 1499 return value; 1500 } 1501 1502 @Override 1503 public Base makeProperty(int hash, String name) throws FHIRException { 1504 switch (hash) { 1505 case 50511102: return getCategory(); 1506 case -1724546052: return getDescription(); 1507 case -1165461084: return getPriority(); 1508 case 109757538: return getStart(); 1509 case 874544034: return addAddresses(); 1510 case 1587405498: return addDocumentation(); 1511 case -880905839: return addTarget(); 1512 default: return super.makeProperty(hash, name); 1513 } 1514 1515 } 1516 1517 @Override 1518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1519 switch (hash) { 1520 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1521 case -1724546052: /*description*/ return new String[] {"CodeableConcept"}; 1522 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 1523 case 109757538: /*start*/ return new String[] {"CodeableConcept"}; 1524 case 874544034: /*addresses*/ return new String[] {"CodeableConcept"}; 1525 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 1526 case -880905839: /*target*/ return new String[] {}; 1527 default: return super.getTypesForProperty(hash, name); 1528 } 1529 1530 } 1531 1532 @Override 1533 public Base addChild(String name) throws FHIRException { 1534 if (name.equals("category")) { 1535 this.category = new CodeableConcept(); 1536 return this.category; 1537 } 1538 else if (name.equals("description")) { 1539 this.description = new CodeableConcept(); 1540 return this.description; 1541 } 1542 else if (name.equals("priority")) { 1543 this.priority = new CodeableConcept(); 1544 return this.priority; 1545 } 1546 else if (name.equals("start")) { 1547 this.start = new CodeableConcept(); 1548 return this.start; 1549 } 1550 else if (name.equals("addresses")) { 1551 return addAddresses(); 1552 } 1553 else if (name.equals("documentation")) { 1554 return addDocumentation(); 1555 } 1556 else if (name.equals("target")) { 1557 return addTarget(); 1558 } 1559 else 1560 return super.addChild(name); 1561 } 1562 1563 public PlanDefinitionGoalComponent copy() { 1564 PlanDefinitionGoalComponent dst = new PlanDefinitionGoalComponent(); 1565 copyValues(dst); 1566 dst.category = category == null ? null : category.copy(); 1567 dst.description = description == null ? null : description.copy(); 1568 dst.priority = priority == null ? null : priority.copy(); 1569 dst.start = start == null ? null : start.copy(); 1570 if (addresses != null) { 1571 dst.addresses = new ArrayList<CodeableConcept>(); 1572 for (CodeableConcept i : addresses) 1573 dst.addresses.add(i.copy()); 1574 }; 1575 if (documentation != null) { 1576 dst.documentation = new ArrayList<RelatedArtifact>(); 1577 for (RelatedArtifact i : documentation) 1578 dst.documentation.add(i.copy()); 1579 }; 1580 if (target != null) { 1581 dst.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1582 for (PlanDefinitionGoalTargetComponent i : target) 1583 dst.target.add(i.copy()); 1584 }; 1585 return dst; 1586 } 1587 1588 @Override 1589 public boolean equalsDeep(Base other_) { 1590 if (!super.equalsDeep(other_)) 1591 return false; 1592 if (!(other_ instanceof PlanDefinitionGoalComponent)) 1593 return false; 1594 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 1595 return compareDeep(category, o.category, true) && compareDeep(description, o.description, true) 1596 && compareDeep(priority, o.priority, true) && compareDeep(start, o.start, true) && compareDeep(addresses, o.addresses, true) 1597 && compareDeep(documentation, o.documentation, true) && compareDeep(target, o.target, true); 1598 } 1599 1600 @Override 1601 public boolean equalsShallow(Base other_) { 1602 if (!super.equalsShallow(other_)) 1603 return false; 1604 if (!(other_ instanceof PlanDefinitionGoalComponent)) 1605 return false; 1606 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 1607 return true; 1608 } 1609 1610 public boolean isEmpty() { 1611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, description, priority 1612 , start, addresses, documentation, target); 1613 } 1614 1615 public String fhirType() { 1616 return "PlanDefinition.goal"; 1617 1618 } 1619 1620 } 1621 1622 @Block() 1623 public static class PlanDefinitionGoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 1624 /** 1625 * The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level. 1626 */ 1627 @Child(name = "measure", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1628 @Description(shortDefinition="The parameter whose value is to be tracked", formalDefinition="The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level." ) 1629 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 1630 protected CodeableConcept measure; 1631 1632 /** 1633 * The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value. 1634 */ 1635 @Child(name = "detail", type = {Quantity.class, Range.class, CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1636 @Description(shortDefinition="The target value to be achieved", formalDefinition="The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value." ) 1637 protected Type detail; 1638 1639 /** 1640 * Indicates the timeframe after the start of the goal in which the goal should be met. 1641 */ 1642 @Child(name = "due", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=false) 1643 @Description(shortDefinition="Reach goal within", formalDefinition="Indicates the timeframe after the start of the goal in which the goal should be met." ) 1644 protected Duration due; 1645 1646 private static final long serialVersionUID = -131874144L; 1647 1648 /** 1649 * Constructor 1650 */ 1651 public PlanDefinitionGoalTargetComponent() { 1652 super(); 1653 } 1654 1655 /** 1656 * @return {@link #measure} (The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level.) 1657 */ 1658 public CodeableConcept getMeasure() { 1659 if (this.measure == null) 1660 if (Configuration.errorOnAutoCreate()) 1661 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.measure"); 1662 else if (Configuration.doAutoCreate()) 1663 this.measure = new CodeableConcept(); // cc 1664 return this.measure; 1665 } 1666 1667 public boolean hasMeasure() { 1668 return this.measure != null && !this.measure.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #measure} (The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level.) 1673 */ 1674 public PlanDefinitionGoalTargetComponent setMeasure(CodeableConcept value) { 1675 this.measure = value; 1676 return this; 1677 } 1678 1679 /** 1680 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 1681 */ 1682 public Type getDetail() { 1683 return this.detail; 1684 } 1685 1686 /** 1687 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 1688 */ 1689 public Quantity getDetailQuantity() throws FHIRException { 1690 if (this.detail == null) 1691 return null; 1692 if (!(this.detail instanceof Quantity)) 1693 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.detail.getClass().getName()+" was encountered"); 1694 return (Quantity) this.detail; 1695 } 1696 1697 public boolean hasDetailQuantity() { 1698 return this != null && this.detail instanceof Quantity; 1699 } 1700 1701 /** 1702 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 1703 */ 1704 public Range getDetailRange() throws FHIRException { 1705 if (this.detail == null) 1706 return null; 1707 if (!(this.detail instanceof Range)) 1708 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.detail.getClass().getName()+" was encountered"); 1709 return (Range) this.detail; 1710 } 1711 1712 public boolean hasDetailRange() { 1713 return this != null && this.detail instanceof Range; 1714 } 1715 1716 /** 1717 * @return {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 1718 */ 1719 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 1720 if (this.detail == null) 1721 return null; 1722 if (!(this.detail instanceof CodeableConcept)) 1723 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.detail.getClass().getName()+" was encountered"); 1724 return (CodeableConcept) this.detail; 1725 } 1726 1727 public boolean hasDetailCodeableConcept() { 1728 return this != null && this.detail instanceof CodeableConcept; 1729 } 1730 1731 public boolean hasDetail() { 1732 return this.detail != null && !this.detail.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #detail} (The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.) 1737 */ 1738 public PlanDefinitionGoalTargetComponent setDetail(Type value) throws FHIRFormatError { 1739 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 1740 throw new FHIRFormatError("Not the right type for PlanDefinition.goal.target.detail[x]: "+value.fhirType()); 1741 this.detail = value; 1742 return this; 1743 } 1744 1745 /** 1746 * @return {@link #due} (Indicates the timeframe after the start of the goal in which the goal should be met.) 1747 */ 1748 public Duration getDue() { 1749 if (this.due == null) 1750 if (Configuration.errorOnAutoCreate()) 1751 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.due"); 1752 else if (Configuration.doAutoCreate()) 1753 this.due = new Duration(); // cc 1754 return this.due; 1755 } 1756 1757 public boolean hasDue() { 1758 return this.due != null && !this.due.isEmpty(); 1759 } 1760 1761 /** 1762 * @param value {@link #due} (Indicates the timeframe after the start of the goal in which the goal should be met.) 1763 */ 1764 public PlanDefinitionGoalTargetComponent setDue(Duration value) { 1765 this.due = value; 1766 return this; 1767 } 1768 1769 protected void listChildren(List<Property> children) { 1770 super.listChildren(children); 1771 children.add(new Property("measure", "CodeableConcept", "The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level.", 0, 1, measure)); 1772 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail)); 1773 children.add(new Property("due", "Duration", "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due)); 1774 } 1775 1776 @Override 1777 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1778 switch (_hash) { 1779 case 938321246: /*measure*/ return new Property("measure", "CodeableConcept", "The parameter whose value is to be tracked, e.g. body weigth, blood pressure, or hemoglobin A1c level.", 0, 1, measure); 1780 case -1973084529: /*detail[x]*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 1781 case -1335224239: /*detail*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 1782 case -1313079300: /*detailQuantity*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 1783 case -2062632084: /*detailRange*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 1784 case -175586544: /*detailCodeableConcept*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. Whan a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 0, 1, detail); 1785 case 99828: /*due*/ return new Property("due", "Duration", "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due); 1786 default: return super.getNamedProperty(_hash, _name, _checkValid); 1787 } 1788 1789 } 1790 1791 @Override 1792 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1793 switch (hash) { 1794 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // CodeableConcept 1795 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // Type 1796 case 99828: /*due*/ return this.due == null ? new Base[0] : new Base[] {this.due}; // Duration 1797 default: return super.getProperty(hash, name, checkValid); 1798 } 1799 1800 } 1801 1802 @Override 1803 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1804 switch (hash) { 1805 case 938321246: // measure 1806 this.measure = castToCodeableConcept(value); // CodeableConcept 1807 return value; 1808 case -1335224239: // detail 1809 this.detail = castToType(value); // Type 1810 return value; 1811 case 99828: // due 1812 this.due = castToDuration(value); // Duration 1813 return value; 1814 default: return super.setProperty(hash, name, value); 1815 } 1816 1817 } 1818 1819 @Override 1820 public Base setProperty(String name, Base value) throws FHIRException { 1821 if (name.equals("measure")) { 1822 this.measure = castToCodeableConcept(value); // CodeableConcept 1823 } else if (name.equals("detail[x]")) { 1824 this.detail = castToType(value); // Type 1825 } else if (name.equals("due")) { 1826 this.due = castToDuration(value); // Duration 1827 } else 1828 return super.setProperty(name, value); 1829 return value; 1830 } 1831 1832 @Override 1833 public Base makeProperty(int hash, String name) throws FHIRException { 1834 switch (hash) { 1835 case 938321246: return getMeasure(); 1836 case -1973084529: return getDetail(); 1837 case -1335224239: return getDetail(); 1838 case 99828: return getDue(); 1839 default: return super.makeProperty(hash, name); 1840 } 1841 1842 } 1843 1844 @Override 1845 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1846 switch (hash) { 1847 case 938321246: /*measure*/ return new String[] {"CodeableConcept"}; 1848 case -1335224239: /*detail*/ return new String[] {"Quantity", "Range", "CodeableConcept"}; 1849 case 99828: /*due*/ return new String[] {"Duration"}; 1850 default: return super.getTypesForProperty(hash, name); 1851 } 1852 1853 } 1854 1855 @Override 1856 public Base addChild(String name) throws FHIRException { 1857 if (name.equals("measure")) { 1858 this.measure = new CodeableConcept(); 1859 return this.measure; 1860 } 1861 else if (name.equals("detailQuantity")) { 1862 this.detail = new Quantity(); 1863 return this.detail; 1864 } 1865 else if (name.equals("detailRange")) { 1866 this.detail = new Range(); 1867 return this.detail; 1868 } 1869 else if (name.equals("detailCodeableConcept")) { 1870 this.detail = new CodeableConcept(); 1871 return this.detail; 1872 } 1873 else if (name.equals("due")) { 1874 this.due = new Duration(); 1875 return this.due; 1876 } 1877 else 1878 return super.addChild(name); 1879 } 1880 1881 public PlanDefinitionGoalTargetComponent copy() { 1882 PlanDefinitionGoalTargetComponent dst = new PlanDefinitionGoalTargetComponent(); 1883 copyValues(dst); 1884 dst.measure = measure == null ? null : measure.copy(); 1885 dst.detail = detail == null ? null : detail.copy(); 1886 dst.due = due == null ? null : due.copy(); 1887 return dst; 1888 } 1889 1890 @Override 1891 public boolean equalsDeep(Base other_) { 1892 if (!super.equalsDeep(other_)) 1893 return false; 1894 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 1895 return false; 1896 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 1897 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) && compareDeep(due, o.due, true) 1898 ; 1899 } 1900 1901 @Override 1902 public boolean equalsShallow(Base other_) { 1903 if (!super.equalsShallow(other_)) 1904 return false; 1905 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 1906 return false; 1907 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 1908 return true; 1909 } 1910 1911 public boolean isEmpty() { 1912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 1913 } 1914 1915 public String fhirType() { 1916 return "PlanDefinition.goal.target"; 1917 1918 } 1919 1920 } 1921 1922 @Block() 1923 public static class PlanDefinitionActionComponent extends BackboneElement implements IBaseBackboneElement { 1924 /** 1925 * A user-visible label for the action. 1926 */ 1927 @Child(name = "label", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1928 @Description(shortDefinition="User-visible label for the action (e.g. 1. or A.)", formalDefinition="A user-visible label for the action." ) 1929 protected StringType label; 1930 1931 /** 1932 * The title of the action displayed to a user. 1933 */ 1934 @Child(name = "title", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1935 @Description(shortDefinition="User-visible title", formalDefinition="The title of the action displayed to a user." ) 1936 protected StringType title; 1937 1938 /** 1939 * A short description of the action used to provide a summary to display to the user. 1940 */ 1941 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1942 @Description(shortDefinition="Short description of the action", formalDefinition="A short description of the action used to provide a summary to display to the user." ) 1943 protected StringType description; 1944 1945 /** 1946 * A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1947 */ 1948 @Child(name = "textEquivalent", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1949 @Description(shortDefinition="Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition="A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically." ) 1950 protected StringType textEquivalent; 1951 1952 /** 1953 * A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template. 1954 */ 1955 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1956 @Description(shortDefinition="Code representing the meaning of the action or sub-actions", formalDefinition="A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template." ) 1957 protected List<CodeableConcept> code; 1958 1959 /** 1960 * A description of why this action is necessary or appropriate. 1961 */ 1962 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1963 @Description(shortDefinition="Why the action should be performed", formalDefinition="A description of why this action is necessary or appropriate." ) 1964 protected List<CodeableConcept> reason; 1965 1966 /** 1967 * Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources. 1968 */ 1969 @Child(name = "documentation", type = {RelatedArtifact.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1970 @Description(shortDefinition="Supporting documentation for the intended performer of the action", formalDefinition="Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources." ) 1971 protected List<RelatedArtifact> documentation; 1972 1973 /** 1974 * Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition. 1975 */ 1976 @Child(name = "goalId", type = {IdType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1977 @Description(shortDefinition="What goals this action supports", formalDefinition="Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition." ) 1978 protected List<IdType> goalId; 1979 1980 /** 1981 * A description of when the action should be triggered. 1982 */ 1983 @Child(name = "triggerDefinition", type = {TriggerDefinition.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1984 @Description(shortDefinition="When the action should be triggered", formalDefinition="A description of when the action should be triggered." ) 1985 protected List<TriggerDefinition> triggerDefinition; 1986 1987 /** 1988 * An expression that describes applicability criteria, or start/stop conditions for the action. 1989 */ 1990 @Child(name = "condition", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1991 @Description(shortDefinition="Whether or not the action is applicable", formalDefinition="An expression that describes applicability criteria, or start/stop conditions for the action." ) 1992 protected List<PlanDefinitionActionConditionComponent> condition; 1993 1994 /** 1995 * Defines input data requirements for the action. 1996 */ 1997 @Child(name = "input", type = {DataRequirement.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1998 @Description(shortDefinition="Input data requirements", formalDefinition="Defines input data requirements for the action." ) 1999 protected List<DataRequirement> input; 2000 2001 /** 2002 * Defines the outputs of the action, if any. 2003 */ 2004 @Child(name = "output", type = {DataRequirement.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2005 @Description(shortDefinition="Output data definition", formalDefinition="Defines the outputs of the action, if any." ) 2006 protected List<DataRequirement> output; 2007 2008 /** 2009 * A relationship to another action such as "before" or "30-60 minutes after start of". 2010 */ 2011 @Child(name = "relatedAction", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2012 @Description(shortDefinition="Relationship to another action", formalDefinition="A relationship to another action such as \"before\" or \"30-60 minutes after start of\"." ) 2013 protected List<PlanDefinitionActionRelatedActionComponent> relatedAction; 2014 2015 /** 2016 * An optional value describing when the action should be performed. 2017 */ 2018 @Child(name = "timing", type = {DateTimeType.class, Period.class, Duration.class, Range.class, Timing.class}, order=14, min=0, max=1, modifier=false, summary=false) 2019 @Description(shortDefinition="When the action should take place", formalDefinition="An optional value describing when the action should be performed." ) 2020 protected Type timing; 2021 2022 /** 2023 * Indicates who should participate in performing the action described. 2024 */ 2025 @Child(name = "participant", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2026 @Description(shortDefinition="Who should participate in the action", formalDefinition="Indicates who should participate in performing the action described." ) 2027 protected List<PlanDefinitionActionParticipantComponent> participant; 2028 2029 /** 2030 * The type of action to perform (create, update, remove). 2031 */ 2032 @Child(name = "type", type = {Coding.class}, order=16, min=0, max=1, modifier=false, summary=false) 2033 @Description(shortDefinition="create | update | remove | fire-event", formalDefinition="The type of action to perform (create, update, remove)." ) 2034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-type") 2035 protected Coding type; 2036 2037 /** 2038 * Defines the grouping behavior for the action and its children. 2039 */ 2040 @Child(name = "groupingBehavior", type = {CodeType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2041 @Description(shortDefinition="visual-group | logical-group | sentence-group", formalDefinition="Defines the grouping behavior for the action and its children." ) 2042 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-grouping-behavior") 2043 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 2044 2045 /** 2046 * Defines the selection behavior for the action and its children. 2047 */ 2048 @Child(name = "selectionBehavior", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2049 @Description(shortDefinition="any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition="Defines the selection behavior for the action and its children." ) 2050 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-selection-behavior") 2051 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 2052 2053 /** 2054 * Defines the requiredness behavior for the action. 2055 */ 2056 @Child(name = "requiredBehavior", type = {CodeType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2057 @Description(shortDefinition="must | could | must-unless-documented", formalDefinition="Defines the requiredness behavior for the action." ) 2058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-required-behavior") 2059 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 2060 2061 /** 2062 * Defines whether the action should usually be preselected. 2063 */ 2064 @Child(name = "precheckBehavior", type = {CodeType.class}, order=20, min=0, max=1, modifier=false, summary=false) 2065 @Description(shortDefinition="yes | no", formalDefinition="Defines whether the action should usually be preselected." ) 2066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-precheck-behavior") 2067 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 2068 2069 /** 2070 * Defines whether the action can be selected multiple times. 2071 */ 2072 @Child(name = "cardinalityBehavior", type = {CodeType.class}, order=21, min=0, max=1, modifier=false, summary=false) 2073 @Description(shortDefinition="single | multiple", formalDefinition="Defines whether the action can be selected multiple times." ) 2074 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 2075 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 2076 2077 /** 2078 * A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken. 2079 */ 2080 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=22, min=0, max=1, modifier=false, summary=false) 2081 @Description(shortDefinition="Description of the activity to be performed", formalDefinition="A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken." ) 2082 protected Reference definition; 2083 2084 /** 2085 * The actual object that is the target of the reference (A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.) 2086 */ 2087 protected Resource definitionTarget; 2088 2089 /** 2090 * A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 2091 */ 2092 @Child(name = "transform", type = {StructureMap.class}, order=23, min=0, max=1, modifier=false, summary=false) 2093 @Description(shortDefinition="Transform to apply the template", formalDefinition="A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input." ) 2094 protected Reference transform; 2095 2096 /** 2097 * The actual object that is the target of the reference (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 2098 */ 2099 protected StructureMap transformTarget; 2100 2101 /** 2102 * Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result. 2103 */ 2104 @Child(name = "dynamicValue", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2105 @Description(shortDefinition="Dynamic aspects of the definition", formalDefinition="Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result." ) 2106 protected List<PlanDefinitionActionDynamicValueComponent> dynamicValue; 2107 2108 /** 2109 * Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition. 2110 */ 2111 @Child(name = "action", type = {PlanDefinitionActionComponent.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2112 @Description(shortDefinition="A sub-action", formalDefinition="Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition." ) 2113 protected List<PlanDefinitionActionComponent> action; 2114 2115 private static final long serialVersionUID = -1404963512L; 2116 2117 /** 2118 * Constructor 2119 */ 2120 public PlanDefinitionActionComponent() { 2121 super(); 2122 } 2123 2124 /** 2125 * @return {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2126 */ 2127 public StringType getLabelElement() { 2128 if (this.label == null) 2129 if (Configuration.errorOnAutoCreate()) 2130 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.label"); 2131 else if (Configuration.doAutoCreate()) 2132 this.label = new StringType(); // bb 2133 return this.label; 2134 } 2135 2136 public boolean hasLabelElement() { 2137 return this.label != null && !this.label.isEmpty(); 2138 } 2139 2140 public boolean hasLabel() { 2141 return this.label != null && !this.label.isEmpty(); 2142 } 2143 2144 /** 2145 * @param value {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2146 */ 2147 public PlanDefinitionActionComponent setLabelElement(StringType value) { 2148 this.label = value; 2149 return this; 2150 } 2151 2152 /** 2153 * @return A user-visible label for the action. 2154 */ 2155 public String getLabel() { 2156 return this.label == null ? null : this.label.getValue(); 2157 } 2158 2159 /** 2160 * @param value A user-visible label for the action. 2161 */ 2162 public PlanDefinitionActionComponent setLabel(String value) { 2163 if (Utilities.noString(value)) 2164 this.label = null; 2165 else { 2166 if (this.label == null) 2167 this.label = new StringType(); 2168 this.label.setValue(value); 2169 } 2170 return this; 2171 } 2172 2173 /** 2174 * @return {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2175 */ 2176 public StringType getTitleElement() { 2177 if (this.title == null) 2178 if (Configuration.errorOnAutoCreate()) 2179 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.title"); 2180 else if (Configuration.doAutoCreate()) 2181 this.title = new StringType(); // bb 2182 return this.title; 2183 } 2184 2185 public boolean hasTitleElement() { 2186 return this.title != null && !this.title.isEmpty(); 2187 } 2188 2189 public boolean hasTitle() { 2190 return this.title != null && !this.title.isEmpty(); 2191 } 2192 2193 /** 2194 * @param value {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2195 */ 2196 public PlanDefinitionActionComponent setTitleElement(StringType value) { 2197 this.title = value; 2198 return this; 2199 } 2200 2201 /** 2202 * @return The title of the action displayed to a user. 2203 */ 2204 public String getTitle() { 2205 return this.title == null ? null : this.title.getValue(); 2206 } 2207 2208 /** 2209 * @param value The title of the action displayed to a user. 2210 */ 2211 public PlanDefinitionActionComponent setTitle(String value) { 2212 if (Utilities.noString(value)) 2213 this.title = null; 2214 else { 2215 if (this.title == null) 2216 this.title = new StringType(); 2217 this.title.setValue(value); 2218 } 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2224 */ 2225 public StringType getDescriptionElement() { 2226 if (this.description == null) 2227 if (Configuration.errorOnAutoCreate()) 2228 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.description"); 2229 else if (Configuration.doAutoCreate()) 2230 this.description = new StringType(); // bb 2231 return this.description; 2232 } 2233 2234 public boolean hasDescriptionElement() { 2235 return this.description != null && !this.description.isEmpty(); 2236 } 2237 2238 public boolean hasDescription() { 2239 return this.description != null && !this.description.isEmpty(); 2240 } 2241 2242 /** 2243 * @param value {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2244 */ 2245 public PlanDefinitionActionComponent setDescriptionElement(StringType value) { 2246 this.description = value; 2247 return this; 2248 } 2249 2250 /** 2251 * @return A short description of the action used to provide a summary to display to the user. 2252 */ 2253 public String getDescription() { 2254 return this.description == null ? null : this.description.getValue(); 2255 } 2256 2257 /** 2258 * @param value A short description of the action used to provide a summary to display to the user. 2259 */ 2260 public PlanDefinitionActionComponent setDescription(String value) { 2261 if (Utilities.noString(value)) 2262 this.description = null; 2263 else { 2264 if (this.description == null) 2265 this.description = new StringType(); 2266 this.description.setValue(value); 2267 } 2268 return this; 2269 } 2270 2271 /** 2272 * @return {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 2273 */ 2274 public StringType getTextEquivalentElement() { 2275 if (this.textEquivalent == null) 2276 if (Configuration.errorOnAutoCreate()) 2277 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.textEquivalent"); 2278 else if (Configuration.doAutoCreate()) 2279 this.textEquivalent = new StringType(); // bb 2280 return this.textEquivalent; 2281 } 2282 2283 public boolean hasTextEquivalentElement() { 2284 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2285 } 2286 2287 public boolean hasTextEquivalent() { 2288 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2289 } 2290 2291 /** 2292 * @param value {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 2293 */ 2294 public PlanDefinitionActionComponent setTextEquivalentElement(StringType value) { 2295 this.textEquivalent = value; 2296 return this; 2297 } 2298 2299 /** 2300 * @return A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 2301 */ 2302 public String getTextEquivalent() { 2303 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 2304 } 2305 2306 /** 2307 * @param value A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 2308 */ 2309 public PlanDefinitionActionComponent setTextEquivalent(String value) { 2310 if (Utilities.noString(value)) 2311 this.textEquivalent = null; 2312 else { 2313 if (this.textEquivalent == null) 2314 this.textEquivalent = new StringType(); 2315 this.textEquivalent.setValue(value); 2316 } 2317 return this; 2318 } 2319 2320 /** 2321 * @return {@link #code} (A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.) 2322 */ 2323 public List<CodeableConcept> getCode() { 2324 if (this.code == null) 2325 this.code = new ArrayList<CodeableConcept>(); 2326 return this.code; 2327 } 2328 2329 /** 2330 * @return Returns a reference to <code>this</code> for easy method chaining 2331 */ 2332 public PlanDefinitionActionComponent setCode(List<CodeableConcept> theCode) { 2333 this.code = theCode; 2334 return this; 2335 } 2336 2337 public boolean hasCode() { 2338 if (this.code == null) 2339 return false; 2340 for (CodeableConcept item : this.code) 2341 if (!item.isEmpty()) 2342 return true; 2343 return false; 2344 } 2345 2346 public CodeableConcept addCode() { //3 2347 CodeableConcept t = new CodeableConcept(); 2348 if (this.code == null) 2349 this.code = new ArrayList<CodeableConcept>(); 2350 this.code.add(t); 2351 return t; 2352 } 2353 2354 public PlanDefinitionActionComponent addCode(CodeableConcept t) { //3 2355 if (t == null) 2356 return this; 2357 if (this.code == null) 2358 this.code = new ArrayList<CodeableConcept>(); 2359 this.code.add(t); 2360 return this; 2361 } 2362 2363 /** 2364 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 2365 */ 2366 public CodeableConcept getCodeFirstRep() { 2367 if (getCode().isEmpty()) { 2368 addCode(); 2369 } 2370 return getCode().get(0); 2371 } 2372 2373 /** 2374 * @return {@link #reason} (A description of why this action is necessary or appropriate.) 2375 */ 2376 public List<CodeableConcept> getReason() { 2377 if (this.reason == null) 2378 this.reason = new ArrayList<CodeableConcept>(); 2379 return this.reason; 2380 } 2381 2382 /** 2383 * @return Returns a reference to <code>this</code> for easy method chaining 2384 */ 2385 public PlanDefinitionActionComponent setReason(List<CodeableConcept> theReason) { 2386 this.reason = theReason; 2387 return this; 2388 } 2389 2390 public boolean hasReason() { 2391 if (this.reason == null) 2392 return false; 2393 for (CodeableConcept item : this.reason) 2394 if (!item.isEmpty()) 2395 return true; 2396 return false; 2397 } 2398 2399 public CodeableConcept addReason() { //3 2400 CodeableConcept t = new CodeableConcept(); 2401 if (this.reason == null) 2402 this.reason = new ArrayList<CodeableConcept>(); 2403 this.reason.add(t); 2404 return t; 2405 } 2406 2407 public PlanDefinitionActionComponent addReason(CodeableConcept t) { //3 2408 if (t == null) 2409 return this; 2410 if (this.reason == null) 2411 this.reason = new ArrayList<CodeableConcept>(); 2412 this.reason.add(t); 2413 return this; 2414 } 2415 2416 /** 2417 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 2418 */ 2419 public CodeableConcept getReasonFirstRep() { 2420 if (getReason().isEmpty()) { 2421 addReason(); 2422 } 2423 return getReason().get(0); 2424 } 2425 2426 /** 2427 * @return {@link #documentation} (Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.) 2428 */ 2429 public List<RelatedArtifact> getDocumentation() { 2430 if (this.documentation == null) 2431 this.documentation = new ArrayList<RelatedArtifact>(); 2432 return this.documentation; 2433 } 2434 2435 /** 2436 * @return Returns a reference to <code>this</code> for easy method chaining 2437 */ 2438 public PlanDefinitionActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 2439 this.documentation = theDocumentation; 2440 return this; 2441 } 2442 2443 public boolean hasDocumentation() { 2444 if (this.documentation == null) 2445 return false; 2446 for (RelatedArtifact item : this.documentation) 2447 if (!item.isEmpty()) 2448 return true; 2449 return false; 2450 } 2451 2452 public RelatedArtifact addDocumentation() { //3 2453 RelatedArtifact t = new RelatedArtifact(); 2454 if (this.documentation == null) 2455 this.documentation = new ArrayList<RelatedArtifact>(); 2456 this.documentation.add(t); 2457 return t; 2458 } 2459 2460 public PlanDefinitionActionComponent addDocumentation(RelatedArtifact t) { //3 2461 if (t == null) 2462 return this; 2463 if (this.documentation == null) 2464 this.documentation = new ArrayList<RelatedArtifact>(); 2465 this.documentation.add(t); 2466 return this; 2467 } 2468 2469 /** 2470 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist 2471 */ 2472 public RelatedArtifact getDocumentationFirstRep() { 2473 if (getDocumentation().isEmpty()) { 2474 addDocumentation(); 2475 } 2476 return getDocumentation().get(0); 2477 } 2478 2479 /** 2480 * @return {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.) 2481 */ 2482 public List<IdType> getGoalId() { 2483 if (this.goalId == null) 2484 this.goalId = new ArrayList<IdType>(); 2485 return this.goalId; 2486 } 2487 2488 /** 2489 * @return Returns a reference to <code>this</code> for easy method chaining 2490 */ 2491 public PlanDefinitionActionComponent setGoalId(List<IdType> theGoalId) { 2492 this.goalId = theGoalId; 2493 return this; 2494 } 2495 2496 public boolean hasGoalId() { 2497 if (this.goalId == null) 2498 return false; 2499 for (IdType item : this.goalId) 2500 if (!item.isEmpty()) 2501 return true; 2502 return false; 2503 } 2504 2505 /** 2506 * @return {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.) 2507 */ 2508 public IdType addGoalIdElement() {//2 2509 IdType t = new IdType(); 2510 if (this.goalId == null) 2511 this.goalId = new ArrayList<IdType>(); 2512 this.goalId.add(t); 2513 return t; 2514 } 2515 2516 /** 2517 * @param value {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.) 2518 */ 2519 public PlanDefinitionActionComponent addGoalId(String value) { //1 2520 IdType t = new IdType(); 2521 t.setValue(value); 2522 if (this.goalId == null) 2523 this.goalId = new ArrayList<IdType>(); 2524 this.goalId.add(t); 2525 return this; 2526 } 2527 2528 /** 2529 * @param value {@link #goalId} (Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.) 2530 */ 2531 public boolean hasGoalId(String value) { 2532 if (this.goalId == null) 2533 return false; 2534 for (IdType v : this.goalId) 2535 if (v.getValue().equals(value)) // id 2536 return true; 2537 return false; 2538 } 2539 2540 /** 2541 * @return {@link #triggerDefinition} (A description of when the action should be triggered.) 2542 */ 2543 public List<TriggerDefinition> getTriggerDefinition() { 2544 if (this.triggerDefinition == null) 2545 this.triggerDefinition = new ArrayList<TriggerDefinition>(); 2546 return this.triggerDefinition; 2547 } 2548 2549 /** 2550 * @return Returns a reference to <code>this</code> for easy method chaining 2551 */ 2552 public PlanDefinitionActionComponent setTriggerDefinition(List<TriggerDefinition> theTriggerDefinition) { 2553 this.triggerDefinition = theTriggerDefinition; 2554 return this; 2555 } 2556 2557 public boolean hasTriggerDefinition() { 2558 if (this.triggerDefinition == null) 2559 return false; 2560 for (TriggerDefinition item : this.triggerDefinition) 2561 if (!item.isEmpty()) 2562 return true; 2563 return false; 2564 } 2565 2566 public TriggerDefinition addTriggerDefinition() { //3 2567 TriggerDefinition t = new TriggerDefinition(); 2568 if (this.triggerDefinition == null) 2569 this.triggerDefinition = new ArrayList<TriggerDefinition>(); 2570 this.triggerDefinition.add(t); 2571 return t; 2572 } 2573 2574 public PlanDefinitionActionComponent addTriggerDefinition(TriggerDefinition t) { //3 2575 if (t == null) 2576 return this; 2577 if (this.triggerDefinition == null) 2578 this.triggerDefinition = new ArrayList<TriggerDefinition>(); 2579 this.triggerDefinition.add(t); 2580 return this; 2581 } 2582 2583 /** 2584 * @return The first repetition of repeating field {@link #triggerDefinition}, creating it if it does not already exist 2585 */ 2586 public TriggerDefinition getTriggerDefinitionFirstRep() { 2587 if (getTriggerDefinition().isEmpty()) { 2588 addTriggerDefinition(); 2589 } 2590 return getTriggerDefinition().get(0); 2591 } 2592 2593 /** 2594 * @return {@link #condition} (An expression that describes applicability criteria, or start/stop conditions for the action.) 2595 */ 2596 public List<PlanDefinitionActionConditionComponent> getCondition() { 2597 if (this.condition == null) 2598 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2599 return this.condition; 2600 } 2601 2602 /** 2603 * @return Returns a reference to <code>this</code> for easy method chaining 2604 */ 2605 public PlanDefinitionActionComponent setCondition(List<PlanDefinitionActionConditionComponent> theCondition) { 2606 this.condition = theCondition; 2607 return this; 2608 } 2609 2610 public boolean hasCondition() { 2611 if (this.condition == null) 2612 return false; 2613 for (PlanDefinitionActionConditionComponent item : this.condition) 2614 if (!item.isEmpty()) 2615 return true; 2616 return false; 2617 } 2618 2619 public PlanDefinitionActionConditionComponent addCondition() { //3 2620 PlanDefinitionActionConditionComponent t = new PlanDefinitionActionConditionComponent(); 2621 if (this.condition == null) 2622 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2623 this.condition.add(t); 2624 return t; 2625 } 2626 2627 public PlanDefinitionActionComponent addCondition(PlanDefinitionActionConditionComponent t) { //3 2628 if (t == null) 2629 return this; 2630 if (this.condition == null) 2631 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 2632 this.condition.add(t); 2633 return this; 2634 } 2635 2636 /** 2637 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist 2638 */ 2639 public PlanDefinitionActionConditionComponent getConditionFirstRep() { 2640 if (getCondition().isEmpty()) { 2641 addCondition(); 2642 } 2643 return getCondition().get(0); 2644 } 2645 2646 /** 2647 * @return {@link #input} (Defines input data requirements for the action.) 2648 */ 2649 public List<DataRequirement> getInput() { 2650 if (this.input == null) 2651 this.input = new ArrayList<DataRequirement>(); 2652 return this.input; 2653 } 2654 2655 /** 2656 * @return Returns a reference to <code>this</code> for easy method chaining 2657 */ 2658 public PlanDefinitionActionComponent setInput(List<DataRequirement> theInput) { 2659 this.input = theInput; 2660 return this; 2661 } 2662 2663 public boolean hasInput() { 2664 if (this.input == null) 2665 return false; 2666 for (DataRequirement item : this.input) 2667 if (!item.isEmpty()) 2668 return true; 2669 return false; 2670 } 2671 2672 public DataRequirement addInput() { //3 2673 DataRequirement t = new DataRequirement(); 2674 if (this.input == null) 2675 this.input = new ArrayList<DataRequirement>(); 2676 this.input.add(t); 2677 return t; 2678 } 2679 2680 public PlanDefinitionActionComponent addInput(DataRequirement t) { //3 2681 if (t == null) 2682 return this; 2683 if (this.input == null) 2684 this.input = new ArrayList<DataRequirement>(); 2685 this.input.add(t); 2686 return this; 2687 } 2688 2689 /** 2690 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist 2691 */ 2692 public DataRequirement getInputFirstRep() { 2693 if (getInput().isEmpty()) { 2694 addInput(); 2695 } 2696 return getInput().get(0); 2697 } 2698 2699 /** 2700 * @return {@link #output} (Defines the outputs of the action, if any.) 2701 */ 2702 public List<DataRequirement> getOutput() { 2703 if (this.output == null) 2704 this.output = new ArrayList<DataRequirement>(); 2705 return this.output; 2706 } 2707 2708 /** 2709 * @return Returns a reference to <code>this</code> for easy method chaining 2710 */ 2711 public PlanDefinitionActionComponent setOutput(List<DataRequirement> theOutput) { 2712 this.output = theOutput; 2713 return this; 2714 } 2715 2716 public boolean hasOutput() { 2717 if (this.output == null) 2718 return false; 2719 for (DataRequirement item : this.output) 2720 if (!item.isEmpty()) 2721 return true; 2722 return false; 2723 } 2724 2725 public DataRequirement addOutput() { //3 2726 DataRequirement t = new DataRequirement(); 2727 if (this.output == null) 2728 this.output = new ArrayList<DataRequirement>(); 2729 this.output.add(t); 2730 return t; 2731 } 2732 2733 public PlanDefinitionActionComponent addOutput(DataRequirement t) { //3 2734 if (t == null) 2735 return this; 2736 if (this.output == null) 2737 this.output = new ArrayList<DataRequirement>(); 2738 this.output.add(t); 2739 return this; 2740 } 2741 2742 /** 2743 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist 2744 */ 2745 public DataRequirement getOutputFirstRep() { 2746 if (getOutput().isEmpty()) { 2747 addOutput(); 2748 } 2749 return getOutput().get(0); 2750 } 2751 2752 /** 2753 * @return {@link #relatedAction} (A relationship to another action such as "before" or "30-60 minutes after start of".) 2754 */ 2755 public List<PlanDefinitionActionRelatedActionComponent> getRelatedAction() { 2756 if (this.relatedAction == null) 2757 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2758 return this.relatedAction; 2759 } 2760 2761 /** 2762 * @return Returns a reference to <code>this</code> for easy method chaining 2763 */ 2764 public PlanDefinitionActionComponent setRelatedAction(List<PlanDefinitionActionRelatedActionComponent> theRelatedAction) { 2765 this.relatedAction = theRelatedAction; 2766 return this; 2767 } 2768 2769 public boolean hasRelatedAction() { 2770 if (this.relatedAction == null) 2771 return false; 2772 for (PlanDefinitionActionRelatedActionComponent item : this.relatedAction) 2773 if (!item.isEmpty()) 2774 return true; 2775 return false; 2776 } 2777 2778 public PlanDefinitionActionRelatedActionComponent addRelatedAction() { //3 2779 PlanDefinitionActionRelatedActionComponent t = new PlanDefinitionActionRelatedActionComponent(); 2780 if (this.relatedAction == null) 2781 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2782 this.relatedAction.add(t); 2783 return t; 2784 } 2785 2786 public PlanDefinitionActionComponent addRelatedAction(PlanDefinitionActionRelatedActionComponent t) { //3 2787 if (t == null) 2788 return this; 2789 if (this.relatedAction == null) 2790 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 2791 this.relatedAction.add(t); 2792 return this; 2793 } 2794 2795 /** 2796 * @return The first repetition of repeating field {@link #relatedAction}, creating it if it does not already exist 2797 */ 2798 public PlanDefinitionActionRelatedActionComponent getRelatedActionFirstRep() { 2799 if (getRelatedAction().isEmpty()) { 2800 addRelatedAction(); 2801 } 2802 return getRelatedAction().get(0); 2803 } 2804 2805 /** 2806 * @return {@link #timing} (An optional value describing when the action should be performed.) 2807 */ 2808 public Type getTiming() { 2809 return this.timing; 2810 } 2811 2812 /** 2813 * @return {@link #timing} (An optional value describing when the action should be performed.) 2814 */ 2815 public DateTimeType getTimingDateTimeType() throws FHIRException { 2816 if (this.timing == null) 2817 return null; 2818 if (!(this.timing instanceof DateTimeType)) 2819 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 2820 return (DateTimeType) this.timing; 2821 } 2822 2823 public boolean hasTimingDateTimeType() { 2824 return this != null && this.timing instanceof DateTimeType; 2825 } 2826 2827 /** 2828 * @return {@link #timing} (An optional value describing when the action should be performed.) 2829 */ 2830 public Period getTimingPeriod() throws FHIRException { 2831 if (this.timing == null) 2832 return null; 2833 if (!(this.timing instanceof Period)) 2834 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 2835 return (Period) this.timing; 2836 } 2837 2838 public boolean hasTimingPeriod() { 2839 return this != null && this.timing instanceof Period; 2840 } 2841 2842 /** 2843 * @return {@link #timing} (An optional value describing when the action should be performed.) 2844 */ 2845 public Duration getTimingDuration() throws FHIRException { 2846 if (this.timing == null) 2847 return null; 2848 if (!(this.timing instanceof Duration)) 2849 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 2850 return (Duration) this.timing; 2851 } 2852 2853 public boolean hasTimingDuration() { 2854 return this != null && this.timing instanceof Duration; 2855 } 2856 2857 /** 2858 * @return {@link #timing} (An optional value describing when the action should be performed.) 2859 */ 2860 public Range getTimingRange() throws FHIRException { 2861 if (this.timing == null) 2862 return null; 2863 if (!(this.timing instanceof Range)) 2864 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 2865 return (Range) this.timing; 2866 } 2867 2868 public boolean hasTimingRange() { 2869 return this != null && this.timing instanceof Range; 2870 } 2871 2872 /** 2873 * @return {@link #timing} (An optional value describing when the action should be performed.) 2874 */ 2875 public Timing getTimingTiming() throws FHIRException { 2876 if (this.timing == null) 2877 return null; 2878 if (!(this.timing instanceof Timing)) 2879 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 2880 return (Timing) this.timing; 2881 } 2882 2883 public boolean hasTimingTiming() { 2884 return this != null && this.timing instanceof Timing; 2885 } 2886 2887 public boolean hasTiming() { 2888 return this.timing != null && !this.timing.isEmpty(); 2889 } 2890 2891 /** 2892 * @param value {@link #timing} (An optional value describing when the action should be performed.) 2893 */ 2894 public PlanDefinitionActionComponent setTiming(Type value) throws FHIRFormatError { 2895 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2896 throw new FHIRFormatError("Not the right type for PlanDefinition.action.timing[x]: "+value.fhirType()); 2897 this.timing = value; 2898 return this; 2899 } 2900 2901 /** 2902 * @return {@link #participant} (Indicates who should participate in performing the action described.) 2903 */ 2904 public List<PlanDefinitionActionParticipantComponent> getParticipant() { 2905 if (this.participant == null) 2906 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2907 return this.participant; 2908 } 2909 2910 /** 2911 * @return Returns a reference to <code>this</code> for easy method chaining 2912 */ 2913 public PlanDefinitionActionComponent setParticipant(List<PlanDefinitionActionParticipantComponent> theParticipant) { 2914 this.participant = theParticipant; 2915 return this; 2916 } 2917 2918 public boolean hasParticipant() { 2919 if (this.participant == null) 2920 return false; 2921 for (PlanDefinitionActionParticipantComponent item : this.participant) 2922 if (!item.isEmpty()) 2923 return true; 2924 return false; 2925 } 2926 2927 public PlanDefinitionActionParticipantComponent addParticipant() { //3 2928 PlanDefinitionActionParticipantComponent t = new PlanDefinitionActionParticipantComponent(); 2929 if (this.participant == null) 2930 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2931 this.participant.add(t); 2932 return t; 2933 } 2934 2935 public PlanDefinitionActionComponent addParticipant(PlanDefinitionActionParticipantComponent t) { //3 2936 if (t == null) 2937 return this; 2938 if (this.participant == null) 2939 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 2940 this.participant.add(t); 2941 return this; 2942 } 2943 2944 /** 2945 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 2946 */ 2947 public PlanDefinitionActionParticipantComponent getParticipantFirstRep() { 2948 if (getParticipant().isEmpty()) { 2949 addParticipant(); 2950 } 2951 return getParticipant().get(0); 2952 } 2953 2954 /** 2955 * @return {@link #type} (The type of action to perform (create, update, remove).) 2956 */ 2957 public Coding getType() { 2958 if (this.type == null) 2959 if (Configuration.errorOnAutoCreate()) 2960 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.type"); 2961 else if (Configuration.doAutoCreate()) 2962 this.type = new Coding(); // cc 2963 return this.type; 2964 } 2965 2966 public boolean hasType() { 2967 return this.type != null && !this.type.isEmpty(); 2968 } 2969 2970 /** 2971 * @param value {@link #type} (The type of action to perform (create, update, remove).) 2972 */ 2973 public PlanDefinitionActionComponent setType(Coding value) { 2974 this.type = value; 2975 return this; 2976 } 2977 2978 /** 2979 * @return {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2980 */ 2981 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2982 if (this.groupingBehavior == null) 2983 if (Configuration.errorOnAutoCreate()) 2984 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.groupingBehavior"); 2985 else if (Configuration.doAutoCreate()) 2986 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2987 return this.groupingBehavior; 2988 } 2989 2990 public boolean hasGroupingBehaviorElement() { 2991 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2992 } 2993 2994 public boolean hasGroupingBehavior() { 2995 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2996 } 2997 2998 /** 2999 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 3000 */ 3001 public PlanDefinitionActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 3002 this.groupingBehavior = value; 3003 return this; 3004 } 3005 3006 /** 3007 * @return Defines the grouping behavior for the action and its children. 3008 */ 3009 public ActionGroupingBehavior getGroupingBehavior() { 3010 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 3011 } 3012 3013 /** 3014 * @param value Defines the grouping behavior for the action and its children. 3015 */ 3016 public PlanDefinitionActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 3017 if (value == null) 3018 this.groupingBehavior = null; 3019 else { 3020 if (this.groupingBehavior == null) 3021 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 3022 this.groupingBehavior.setValue(value); 3023 } 3024 return this; 3025 } 3026 3027 /** 3028 * @return {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 3029 */ 3030 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 3031 if (this.selectionBehavior == null) 3032 if (Configuration.errorOnAutoCreate()) 3033 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.selectionBehavior"); 3034 else if (Configuration.doAutoCreate()) 3035 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 3036 return this.selectionBehavior; 3037 } 3038 3039 public boolean hasSelectionBehaviorElement() { 3040 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3041 } 3042 3043 public boolean hasSelectionBehavior() { 3044 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3045 } 3046 3047 /** 3048 * @param value {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 3049 */ 3050 public PlanDefinitionActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 3051 this.selectionBehavior = value; 3052 return this; 3053 } 3054 3055 /** 3056 * @return Defines the selection behavior for the action and its children. 3057 */ 3058 public ActionSelectionBehavior getSelectionBehavior() { 3059 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 3060 } 3061 3062 /** 3063 * @param value Defines the selection behavior for the action and its children. 3064 */ 3065 public PlanDefinitionActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 3066 if (value == null) 3067 this.selectionBehavior = null; 3068 else { 3069 if (this.selectionBehavior == null) 3070 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 3071 this.selectionBehavior.setValue(value); 3072 } 3073 return this; 3074 } 3075 3076 /** 3077 * @return {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 3078 */ 3079 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 3080 if (this.requiredBehavior == null) 3081 if (Configuration.errorOnAutoCreate()) 3082 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.requiredBehavior"); 3083 else if (Configuration.doAutoCreate()) 3084 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 3085 return this.requiredBehavior; 3086 } 3087 3088 public boolean hasRequiredBehaviorElement() { 3089 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3090 } 3091 3092 public boolean hasRequiredBehavior() { 3093 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3094 } 3095 3096 /** 3097 * @param value {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 3098 */ 3099 public PlanDefinitionActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 3100 this.requiredBehavior = value; 3101 return this; 3102 } 3103 3104 /** 3105 * @return Defines the requiredness behavior for the action. 3106 */ 3107 public ActionRequiredBehavior getRequiredBehavior() { 3108 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 3109 } 3110 3111 /** 3112 * @param value Defines the requiredness behavior for the action. 3113 */ 3114 public PlanDefinitionActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 3115 if (value == null) 3116 this.requiredBehavior = null; 3117 else { 3118 if (this.requiredBehavior == null) 3119 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 3120 this.requiredBehavior.setValue(value); 3121 } 3122 return this; 3123 } 3124 3125 /** 3126 * @return {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 3127 */ 3128 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 3129 if (this.precheckBehavior == null) 3130 if (Configuration.errorOnAutoCreate()) 3131 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.precheckBehavior"); 3132 else if (Configuration.doAutoCreate()) 3133 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 3134 return this.precheckBehavior; 3135 } 3136 3137 public boolean hasPrecheckBehaviorElement() { 3138 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 3139 } 3140 3141 public boolean hasPrecheckBehavior() { 3142 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 3143 } 3144 3145 /** 3146 * @param value {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 3147 */ 3148 public PlanDefinitionActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 3149 this.precheckBehavior = value; 3150 return this; 3151 } 3152 3153 /** 3154 * @return Defines whether the action should usually be preselected. 3155 */ 3156 public ActionPrecheckBehavior getPrecheckBehavior() { 3157 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 3158 } 3159 3160 /** 3161 * @param value Defines whether the action should usually be preselected. 3162 */ 3163 public PlanDefinitionActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 3164 if (value == null) 3165 this.precheckBehavior = null; 3166 else { 3167 if (this.precheckBehavior == null) 3168 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 3169 this.precheckBehavior.setValue(value); 3170 } 3171 return this; 3172 } 3173 3174 /** 3175 * @return {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 3176 */ 3177 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 3178 if (this.cardinalityBehavior == null) 3179 if (Configuration.errorOnAutoCreate()) 3180 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.cardinalityBehavior"); 3181 else if (Configuration.doAutoCreate()) 3182 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); // bb 3183 return this.cardinalityBehavior; 3184 } 3185 3186 public boolean hasCardinalityBehaviorElement() { 3187 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3188 } 3189 3190 public boolean hasCardinalityBehavior() { 3191 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3192 } 3193 3194 /** 3195 * @param value {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 3196 */ 3197 public PlanDefinitionActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 3198 this.cardinalityBehavior = value; 3199 return this; 3200 } 3201 3202 /** 3203 * @return Defines whether the action can be selected multiple times. 3204 */ 3205 public ActionCardinalityBehavior getCardinalityBehavior() { 3206 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 3207 } 3208 3209 /** 3210 * @param value Defines whether the action can be selected multiple times. 3211 */ 3212 public PlanDefinitionActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 3213 if (value == null) 3214 this.cardinalityBehavior = null; 3215 else { 3216 if (this.cardinalityBehavior == null) 3217 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); 3218 this.cardinalityBehavior.setValue(value); 3219 } 3220 return this; 3221 } 3222 3223 /** 3224 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.) 3225 */ 3226 public Reference getDefinition() { 3227 if (this.definition == null) 3228 if (Configuration.errorOnAutoCreate()) 3229 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.definition"); 3230 else if (Configuration.doAutoCreate()) 3231 this.definition = new Reference(); // cc 3232 return this.definition; 3233 } 3234 3235 public boolean hasDefinition() { 3236 return this.definition != null && !this.definition.isEmpty(); 3237 } 3238 3239 /** 3240 * @param value {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.) 3241 */ 3242 public PlanDefinitionActionComponent setDefinition(Reference value) { 3243 this.definition = value; 3244 return this; 3245 } 3246 3247 /** 3248 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.) 3249 */ 3250 public Resource getDefinitionTarget() { 3251 return this.definitionTarget; 3252 } 3253 3254 /** 3255 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.) 3256 */ 3257 public PlanDefinitionActionComponent setDefinitionTarget(Resource value) { 3258 this.definitionTarget = value; 3259 return this; 3260 } 3261 3262 /** 3263 * @return {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 3264 */ 3265 public Reference getTransform() { 3266 if (this.transform == null) 3267 if (Configuration.errorOnAutoCreate()) 3268 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.transform"); 3269 else if (Configuration.doAutoCreate()) 3270 this.transform = new Reference(); // cc 3271 return this.transform; 3272 } 3273 3274 public boolean hasTransform() { 3275 return this.transform != null && !this.transform.isEmpty(); 3276 } 3277 3278 /** 3279 * @param value {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 3280 */ 3281 public PlanDefinitionActionComponent setTransform(Reference value) { 3282 this.transform = value; 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #transform} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 3288 */ 3289 public StructureMap getTransformTarget() { 3290 if (this.transformTarget == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.transform"); 3293 else if (Configuration.doAutoCreate()) 3294 this.transformTarget = new StructureMap(); // aa 3295 return this.transformTarget; 3296 } 3297 3298 /** 3299 * @param value {@link #transform} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.) 3300 */ 3301 public PlanDefinitionActionComponent setTransformTarget(StructureMap value) { 3302 this.transformTarget = value; 3303 return this; 3304 } 3305 3306 /** 3307 * @return {@link #dynamicValue} (Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.) 3308 */ 3309 public List<PlanDefinitionActionDynamicValueComponent> getDynamicValue() { 3310 if (this.dynamicValue == null) 3311 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3312 return this.dynamicValue; 3313 } 3314 3315 /** 3316 * @return Returns a reference to <code>this</code> for easy method chaining 3317 */ 3318 public PlanDefinitionActionComponent setDynamicValue(List<PlanDefinitionActionDynamicValueComponent> theDynamicValue) { 3319 this.dynamicValue = theDynamicValue; 3320 return this; 3321 } 3322 3323 public boolean hasDynamicValue() { 3324 if (this.dynamicValue == null) 3325 return false; 3326 for (PlanDefinitionActionDynamicValueComponent item : this.dynamicValue) 3327 if (!item.isEmpty()) 3328 return true; 3329 return false; 3330 } 3331 3332 public PlanDefinitionActionDynamicValueComponent addDynamicValue() { //3 3333 PlanDefinitionActionDynamicValueComponent t = new PlanDefinitionActionDynamicValueComponent(); 3334 if (this.dynamicValue == null) 3335 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3336 this.dynamicValue.add(t); 3337 return t; 3338 } 3339 3340 public PlanDefinitionActionComponent addDynamicValue(PlanDefinitionActionDynamicValueComponent t) { //3 3341 if (t == null) 3342 return this; 3343 if (this.dynamicValue == null) 3344 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3345 this.dynamicValue.add(t); 3346 return this; 3347 } 3348 3349 /** 3350 * @return The first repetition of repeating field {@link #dynamicValue}, creating it if it does not already exist 3351 */ 3352 public PlanDefinitionActionDynamicValueComponent getDynamicValueFirstRep() { 3353 if (getDynamicValue().isEmpty()) { 3354 addDynamicValue(); 3355 } 3356 return getDynamicValue().get(0); 3357 } 3358 3359 /** 3360 * @return {@link #action} (Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.) 3361 */ 3362 public List<PlanDefinitionActionComponent> getAction() { 3363 if (this.action == null) 3364 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3365 return this.action; 3366 } 3367 3368 /** 3369 * @return Returns a reference to <code>this</code> for easy method chaining 3370 */ 3371 public PlanDefinitionActionComponent setAction(List<PlanDefinitionActionComponent> theAction) { 3372 this.action = theAction; 3373 return this; 3374 } 3375 3376 public boolean hasAction() { 3377 if (this.action == null) 3378 return false; 3379 for (PlanDefinitionActionComponent item : this.action) 3380 if (!item.isEmpty()) 3381 return true; 3382 return false; 3383 } 3384 3385 public PlanDefinitionActionComponent addAction() { //3 3386 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 3387 if (this.action == null) 3388 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3389 this.action.add(t); 3390 return t; 3391 } 3392 3393 public PlanDefinitionActionComponent addAction(PlanDefinitionActionComponent t) { //3 3394 if (t == null) 3395 return this; 3396 if (this.action == null) 3397 this.action = new ArrayList<PlanDefinitionActionComponent>(); 3398 this.action.add(t); 3399 return this; 3400 } 3401 3402 /** 3403 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 3404 */ 3405 public PlanDefinitionActionComponent getActionFirstRep() { 3406 if (getAction().isEmpty()) { 3407 addAction(); 3408 } 3409 return getAction().get(0); 3410 } 3411 3412 protected void listChildren(List<Property> children) { 3413 super.listChildren(children); 3414 children.add(new Property("label", "string", "A user-visible label for the action.", 0, 1, label)); 3415 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 3416 children.add(new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 3417 children.add(new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent)); 3418 children.add(new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code)); 3419 children.add(new Property("reason", "CodeableConcept", "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason)); 3420 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 3421 children.add(new Property("goalId", "id", "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 0, java.lang.Integer.MAX_VALUE, goalId)); 3422 children.add(new Property("triggerDefinition", "TriggerDefinition", "A description of when the action should be triggered.", 0, java.lang.Integer.MAX_VALUE, triggerDefinition)); 3423 children.add(new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition)); 3424 children.add(new Property("input", "DataRequirement", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input)); 3425 children.add(new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output)); 3426 children.add(new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction)); 3427 children.add(new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing)); 3428 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant)); 3429 children.add(new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type)); 3430 children.add(new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 3431 children.add(new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 3432 children.add(new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior)); 3433 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior)); 3434 children.add(new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 3435 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 0, 1, definition)); 3436 children.add(new Property("transform", "Reference(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform)); 3437 children.add(new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 3438 children.add(new Property("action", "@PlanDefinition.action", "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 0, java.lang.Integer.MAX_VALUE, action)); 3439 } 3440 3441 @Override 3442 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3443 switch (_hash) { 3444 case 102727412: /*label*/ return new Property("label", "string", "A user-visible label for the action.", 0, 1, label); 3445 case 110371416: /*title*/ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 3446 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 3447 case -900391049: /*textEquivalent*/ return new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent); 3448 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code); 3449 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason); 3450 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 3451 case -1240658034: /*goalId*/ return new Property("goalId", "id", "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 0, java.lang.Integer.MAX_VALUE, goalId); 3452 case 1126736171: /*triggerDefinition*/ return new Property("triggerDefinition", "TriggerDefinition", "A description of when the action should be triggered.", 0, java.lang.Integer.MAX_VALUE, triggerDefinition); 3453 case -861311717: /*condition*/ return new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition); 3454 case 100358090: /*input*/ return new Property("input", "DataRequirement", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input); 3455 case -1005512447: /*output*/ return new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output); 3456 case -384107967: /*relatedAction*/ return new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction); 3457 case 164632566: /*timing[x]*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3458 case -873664438: /*timing*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3459 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3460 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3461 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3462 case -710871277: /*timingRange*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3463 case -497554124: /*timingTiming*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 3464 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant); 3465 case 3575610: /*type*/ return new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type); 3466 case 586678389: /*groupingBehavior*/ return new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 3467 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 3468 case -1163906287: /*requiredBehavior*/ return new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior); 3469 case -1174249033: /*precheckBehavior*/ return new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 3470 case -922577408: /*cardinalityBehavior*/ return new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 3471 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 0, 1, definition); 3472 case 1052666732: /*transform*/ return new Property("transform", "Reference(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform); 3473 case 572625010: /*dynamicValue*/ return new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue); 3474 case -1422950858: /*action*/ return new Property("action", "@PlanDefinition.action", "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 0, java.lang.Integer.MAX_VALUE, action); 3475 default: return super.getNamedProperty(_hash, _name, _checkValid); 3476 } 3477 3478 } 3479 3480 @Override 3481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3482 switch (hash) { 3483 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 3484 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3485 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3486 case -900391049: /*textEquivalent*/ return this.textEquivalent == null ? new Base[0] : new Base[] {this.textEquivalent}; // StringType 3487 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 3488 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 3489 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 3490 case -1240658034: /*goalId*/ return this.goalId == null ? new Base[0] : this.goalId.toArray(new Base[this.goalId.size()]); // IdType 3491 case 1126736171: /*triggerDefinition*/ return this.triggerDefinition == null ? new Base[0] : this.triggerDefinition.toArray(new Base[this.triggerDefinition.size()]); // TriggerDefinition 3492 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // PlanDefinitionActionConditionComponent 3493 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // DataRequirement 3494 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // DataRequirement 3495 case -384107967: /*relatedAction*/ return this.relatedAction == null ? new Base[0] : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // PlanDefinitionActionRelatedActionComponent 3496 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 3497 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // PlanDefinitionActionParticipantComponent 3498 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 3499 case 586678389: /*groupingBehavior*/ return this.groupingBehavior == null ? new Base[0] : new Base[] {this.groupingBehavior}; // Enumeration<ActionGroupingBehavior> 3500 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<ActionSelectionBehavior> 3501 case -1163906287: /*requiredBehavior*/ return this.requiredBehavior == null ? new Base[0] : new Base[] {this.requiredBehavior}; // Enumeration<ActionRequiredBehavior> 3502 case -1174249033: /*precheckBehavior*/ return this.precheckBehavior == null ? new Base[0] : new Base[] {this.precheckBehavior}; // Enumeration<ActionPrecheckBehavior> 3503 case -922577408: /*cardinalityBehavior*/ return this.cardinalityBehavior == null ? new Base[0] : new Base[] {this.cardinalityBehavior}; // Enumeration<ActionCardinalityBehavior> 3504 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 3505 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // Reference 3506 case 572625010: /*dynamicValue*/ return this.dynamicValue == null ? new Base[0] : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // PlanDefinitionActionDynamicValueComponent 3507 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 3508 default: return super.getProperty(hash, name, checkValid); 3509 } 3510 3511 } 3512 3513 @Override 3514 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3515 switch (hash) { 3516 case 102727412: // label 3517 this.label = castToString(value); // StringType 3518 return value; 3519 case 110371416: // title 3520 this.title = castToString(value); // StringType 3521 return value; 3522 case -1724546052: // description 3523 this.description = castToString(value); // StringType 3524 return value; 3525 case -900391049: // textEquivalent 3526 this.textEquivalent = castToString(value); // StringType 3527 return value; 3528 case 3059181: // code 3529 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 3530 return value; 3531 case -934964668: // reason 3532 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 3533 return value; 3534 case 1587405498: // documentation 3535 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 3536 return value; 3537 case -1240658034: // goalId 3538 this.getGoalId().add(castToId(value)); // IdType 3539 return value; 3540 case 1126736171: // triggerDefinition 3541 this.getTriggerDefinition().add(castToTriggerDefinition(value)); // TriggerDefinition 3542 return value; 3543 case -861311717: // condition 3544 this.getCondition().add((PlanDefinitionActionConditionComponent) value); // PlanDefinitionActionConditionComponent 3545 return value; 3546 case 100358090: // input 3547 this.getInput().add(castToDataRequirement(value)); // DataRequirement 3548 return value; 3549 case -1005512447: // output 3550 this.getOutput().add(castToDataRequirement(value)); // DataRequirement 3551 return value; 3552 case -384107967: // relatedAction 3553 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); // PlanDefinitionActionRelatedActionComponent 3554 return value; 3555 case -873664438: // timing 3556 this.timing = castToType(value); // Type 3557 return value; 3558 case 767422259: // participant 3559 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); // PlanDefinitionActionParticipantComponent 3560 return value; 3561 case 3575610: // type 3562 this.type = castToCoding(value); // Coding 3563 return value; 3564 case 586678389: // groupingBehavior 3565 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3566 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3567 return value; 3568 case 168639486: // selectionBehavior 3569 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3570 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3571 return value; 3572 case -1163906287: // requiredBehavior 3573 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3574 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3575 return value; 3576 case -1174249033: // precheckBehavior 3577 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3578 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3579 return value; 3580 case -922577408: // cardinalityBehavior 3581 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3582 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3583 return value; 3584 case -1014418093: // definition 3585 this.definition = castToReference(value); // Reference 3586 return value; 3587 case 1052666732: // transform 3588 this.transform = castToReference(value); // Reference 3589 return value; 3590 case 572625010: // dynamicValue 3591 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); // PlanDefinitionActionDynamicValueComponent 3592 return value; 3593 case -1422950858: // action 3594 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 3595 return value; 3596 default: return super.setProperty(hash, name, value); 3597 } 3598 3599 } 3600 3601 @Override 3602 public Base setProperty(String name, Base value) throws FHIRException { 3603 if (name.equals("label")) { 3604 this.label = castToString(value); // StringType 3605 } else if (name.equals("title")) { 3606 this.title = castToString(value); // StringType 3607 } else if (name.equals("description")) { 3608 this.description = castToString(value); // StringType 3609 } else if (name.equals("textEquivalent")) { 3610 this.textEquivalent = castToString(value); // StringType 3611 } else if (name.equals("code")) { 3612 this.getCode().add(castToCodeableConcept(value)); 3613 } else if (name.equals("reason")) { 3614 this.getReason().add(castToCodeableConcept(value)); 3615 } else if (name.equals("documentation")) { 3616 this.getDocumentation().add(castToRelatedArtifact(value)); 3617 } else if (name.equals("goalId")) { 3618 this.getGoalId().add(castToId(value)); 3619 } else if (name.equals("triggerDefinition")) { 3620 this.getTriggerDefinition().add(castToTriggerDefinition(value)); 3621 } else if (name.equals("condition")) { 3622 this.getCondition().add((PlanDefinitionActionConditionComponent) value); 3623 } else if (name.equals("input")) { 3624 this.getInput().add(castToDataRequirement(value)); 3625 } else if (name.equals("output")) { 3626 this.getOutput().add(castToDataRequirement(value)); 3627 } else if (name.equals("relatedAction")) { 3628 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); 3629 } else if (name.equals("timing[x]")) { 3630 this.timing = castToType(value); // Type 3631 } else if (name.equals("participant")) { 3632 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); 3633 } else if (name.equals("type")) { 3634 this.type = castToCoding(value); // Coding 3635 } else if (name.equals("groupingBehavior")) { 3636 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3637 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3638 } else if (name.equals("selectionBehavior")) { 3639 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3640 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3641 } else if (name.equals("requiredBehavior")) { 3642 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3643 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3644 } else if (name.equals("precheckBehavior")) { 3645 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3646 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3647 } else if (name.equals("cardinalityBehavior")) { 3648 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3649 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3650 } else if (name.equals("definition")) { 3651 this.definition = castToReference(value); // Reference 3652 } else if (name.equals("transform")) { 3653 this.transform = castToReference(value); // Reference 3654 } else if (name.equals("dynamicValue")) { 3655 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); 3656 } else if (name.equals("action")) { 3657 this.getAction().add((PlanDefinitionActionComponent) value); 3658 } else 3659 return super.setProperty(name, value); 3660 return value; 3661 } 3662 3663 @Override 3664 public Base makeProperty(int hash, String name) throws FHIRException { 3665 switch (hash) { 3666 case 102727412: return getLabelElement(); 3667 case 110371416: return getTitleElement(); 3668 case -1724546052: return getDescriptionElement(); 3669 case -900391049: return getTextEquivalentElement(); 3670 case 3059181: return addCode(); 3671 case -934964668: return addReason(); 3672 case 1587405498: return addDocumentation(); 3673 case -1240658034: return addGoalIdElement(); 3674 case 1126736171: return addTriggerDefinition(); 3675 case -861311717: return addCondition(); 3676 case 100358090: return addInput(); 3677 case -1005512447: return addOutput(); 3678 case -384107967: return addRelatedAction(); 3679 case 164632566: return getTiming(); 3680 case -873664438: return getTiming(); 3681 case 767422259: return addParticipant(); 3682 case 3575610: return getType(); 3683 case 586678389: return getGroupingBehaviorElement(); 3684 case 168639486: return getSelectionBehaviorElement(); 3685 case -1163906287: return getRequiredBehaviorElement(); 3686 case -1174249033: return getPrecheckBehaviorElement(); 3687 case -922577408: return getCardinalityBehaviorElement(); 3688 case -1014418093: return getDefinition(); 3689 case 1052666732: return getTransform(); 3690 case 572625010: return addDynamicValue(); 3691 case -1422950858: return addAction(); 3692 default: return super.makeProperty(hash, name); 3693 } 3694 3695 } 3696 3697 @Override 3698 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3699 switch (hash) { 3700 case 102727412: /*label*/ return new String[] {"string"}; 3701 case 110371416: /*title*/ return new String[] {"string"}; 3702 case -1724546052: /*description*/ return new String[] {"string"}; 3703 case -900391049: /*textEquivalent*/ return new String[] {"string"}; 3704 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3705 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 3706 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 3707 case -1240658034: /*goalId*/ return new String[] {"id"}; 3708 case 1126736171: /*triggerDefinition*/ return new String[] {"TriggerDefinition"}; 3709 case -861311717: /*condition*/ return new String[] {}; 3710 case 100358090: /*input*/ return new String[] {"DataRequirement"}; 3711 case -1005512447: /*output*/ return new String[] {"DataRequirement"}; 3712 case -384107967: /*relatedAction*/ return new String[] {}; 3713 case -873664438: /*timing*/ return new String[] {"dateTime", "Period", "Duration", "Range", "Timing"}; 3714 case 767422259: /*participant*/ return new String[] {}; 3715 case 3575610: /*type*/ return new String[] {"Coding"}; 3716 case 586678389: /*groupingBehavior*/ return new String[] {"code"}; 3717 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 3718 case -1163906287: /*requiredBehavior*/ return new String[] {"code"}; 3719 case -1174249033: /*precheckBehavior*/ return new String[] {"code"}; 3720 case -922577408: /*cardinalityBehavior*/ return new String[] {"code"}; 3721 case -1014418093: /*definition*/ return new String[] {"Reference"}; 3722 case 1052666732: /*transform*/ return new String[] {"Reference"}; 3723 case 572625010: /*dynamicValue*/ return new String[] {}; 3724 case -1422950858: /*action*/ return new String[] {"@PlanDefinition.action"}; 3725 default: return super.getTypesForProperty(hash, name); 3726 } 3727 3728 } 3729 3730 @Override 3731 public Base addChild(String name) throws FHIRException { 3732 if (name.equals("label")) { 3733 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.label"); 3734 } 3735 else if (name.equals("title")) { 3736 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 3737 } 3738 else if (name.equals("description")) { 3739 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 3740 } 3741 else if (name.equals("textEquivalent")) { 3742 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.textEquivalent"); 3743 } 3744 else if (name.equals("code")) { 3745 return addCode(); 3746 } 3747 else if (name.equals("reason")) { 3748 return addReason(); 3749 } 3750 else if (name.equals("documentation")) { 3751 return addDocumentation(); 3752 } 3753 else if (name.equals("goalId")) { 3754 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.goalId"); 3755 } 3756 else if (name.equals("triggerDefinition")) { 3757 return addTriggerDefinition(); 3758 } 3759 else if (name.equals("condition")) { 3760 return addCondition(); 3761 } 3762 else if (name.equals("input")) { 3763 return addInput(); 3764 } 3765 else if (name.equals("output")) { 3766 return addOutput(); 3767 } 3768 else if (name.equals("relatedAction")) { 3769 return addRelatedAction(); 3770 } 3771 else if (name.equals("timingDateTime")) { 3772 this.timing = new DateTimeType(); 3773 return this.timing; 3774 } 3775 else if (name.equals("timingPeriod")) { 3776 this.timing = new Period(); 3777 return this.timing; 3778 } 3779 else if (name.equals("timingDuration")) { 3780 this.timing = new Duration(); 3781 return this.timing; 3782 } 3783 else if (name.equals("timingRange")) { 3784 this.timing = new Range(); 3785 return this.timing; 3786 } 3787 else if (name.equals("timingTiming")) { 3788 this.timing = new Timing(); 3789 return this.timing; 3790 } 3791 else if (name.equals("participant")) { 3792 return addParticipant(); 3793 } 3794 else if (name.equals("type")) { 3795 this.type = new Coding(); 3796 return this.type; 3797 } 3798 else if (name.equals("groupingBehavior")) { 3799 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.groupingBehavior"); 3800 } 3801 else if (name.equals("selectionBehavior")) { 3802 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.selectionBehavior"); 3803 } 3804 else if (name.equals("requiredBehavior")) { 3805 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.requiredBehavior"); 3806 } 3807 else if (name.equals("precheckBehavior")) { 3808 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.precheckBehavior"); 3809 } 3810 else if (name.equals("cardinalityBehavior")) { 3811 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.cardinalityBehavior"); 3812 } 3813 else if (name.equals("definition")) { 3814 this.definition = new Reference(); 3815 return this.definition; 3816 } 3817 else if (name.equals("transform")) { 3818 this.transform = new Reference(); 3819 return this.transform; 3820 } 3821 else if (name.equals("dynamicValue")) { 3822 return addDynamicValue(); 3823 } 3824 else if (name.equals("action")) { 3825 return addAction(); 3826 } 3827 else 3828 return super.addChild(name); 3829 } 3830 3831 public PlanDefinitionActionComponent copy() { 3832 PlanDefinitionActionComponent dst = new PlanDefinitionActionComponent(); 3833 copyValues(dst); 3834 dst.label = label == null ? null : label.copy(); 3835 dst.title = title == null ? null : title.copy(); 3836 dst.description = description == null ? null : description.copy(); 3837 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 3838 if (code != null) { 3839 dst.code = new ArrayList<CodeableConcept>(); 3840 for (CodeableConcept i : code) 3841 dst.code.add(i.copy()); 3842 }; 3843 if (reason != null) { 3844 dst.reason = new ArrayList<CodeableConcept>(); 3845 for (CodeableConcept i : reason) 3846 dst.reason.add(i.copy()); 3847 }; 3848 if (documentation != null) { 3849 dst.documentation = new ArrayList<RelatedArtifact>(); 3850 for (RelatedArtifact i : documentation) 3851 dst.documentation.add(i.copy()); 3852 }; 3853 if (goalId != null) { 3854 dst.goalId = new ArrayList<IdType>(); 3855 for (IdType i : goalId) 3856 dst.goalId.add(i.copy()); 3857 }; 3858 if (triggerDefinition != null) { 3859 dst.triggerDefinition = new ArrayList<TriggerDefinition>(); 3860 for (TriggerDefinition i : triggerDefinition) 3861 dst.triggerDefinition.add(i.copy()); 3862 }; 3863 if (condition != null) { 3864 dst.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3865 for (PlanDefinitionActionConditionComponent i : condition) 3866 dst.condition.add(i.copy()); 3867 }; 3868 if (input != null) { 3869 dst.input = new ArrayList<DataRequirement>(); 3870 for (DataRequirement i : input) 3871 dst.input.add(i.copy()); 3872 }; 3873 if (output != null) { 3874 dst.output = new ArrayList<DataRequirement>(); 3875 for (DataRequirement i : output) 3876 dst.output.add(i.copy()); 3877 }; 3878 if (relatedAction != null) { 3879 dst.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3880 for (PlanDefinitionActionRelatedActionComponent i : relatedAction) 3881 dst.relatedAction.add(i.copy()); 3882 }; 3883 dst.timing = timing == null ? null : timing.copy(); 3884 if (participant != null) { 3885 dst.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3886 for (PlanDefinitionActionParticipantComponent i : participant) 3887 dst.participant.add(i.copy()); 3888 }; 3889 dst.type = type == null ? null : type.copy(); 3890 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 3891 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 3892 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 3893 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 3894 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 3895 dst.definition = definition == null ? null : definition.copy(); 3896 dst.transform = transform == null ? null : transform.copy(); 3897 if (dynamicValue != null) { 3898 dst.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 3899 for (PlanDefinitionActionDynamicValueComponent i : dynamicValue) 3900 dst.dynamicValue.add(i.copy()); 3901 }; 3902 if (action != null) { 3903 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 3904 for (PlanDefinitionActionComponent i : action) 3905 dst.action.add(i.copy()); 3906 }; 3907 return dst; 3908 } 3909 3910 @Override 3911 public boolean equalsDeep(Base other_) { 3912 if (!super.equalsDeep(other_)) 3913 return false; 3914 if (!(other_ instanceof PlanDefinitionActionComponent)) 3915 return false; 3916 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 3917 return compareDeep(label, o.label, true) && compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 3918 && compareDeep(textEquivalent, o.textEquivalent, true) && compareDeep(code, o.code, true) && compareDeep(reason, o.reason, true) 3919 && compareDeep(documentation, o.documentation, true) && compareDeep(goalId, o.goalId, true) && compareDeep(triggerDefinition, o.triggerDefinition, true) 3920 && compareDeep(condition, o.condition, true) && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) 3921 && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) && compareDeep(participant, o.participant, true) 3922 && compareDeep(type, o.type, true) && compareDeep(groupingBehavior, o.groupingBehavior, true) && compareDeep(selectionBehavior, o.selectionBehavior, true) 3923 && compareDeep(requiredBehavior, o.requiredBehavior, true) && compareDeep(precheckBehavior, o.precheckBehavior, true) 3924 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(definition, o.definition, true) 3925 && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true) 3926 && compareDeep(action, o.action, true); 3927 } 3928 3929 @Override 3930 public boolean equalsShallow(Base other_) { 3931 if (!super.equalsShallow(other_)) 3932 return false; 3933 if (!(other_ instanceof PlanDefinitionActionComponent)) 3934 return false; 3935 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 3936 return compareValues(label, o.label, true) && compareValues(title, o.title, true) && compareValues(description, o.description, true) 3937 && compareValues(textEquivalent, o.textEquivalent, true) && compareValues(goalId, o.goalId, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 3938 && compareValues(selectionBehavior, o.selectionBehavior, true) && compareValues(requiredBehavior, o.requiredBehavior, true) 3939 && compareValues(precheckBehavior, o.precheckBehavior, true) && compareValues(cardinalityBehavior, o.cardinalityBehavior, true) 3940 ; 3941 } 3942 3943 public boolean isEmpty() { 3944 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, title, description 3945 , textEquivalent, code, reason, documentation, goalId, triggerDefinition, condition 3946 , input, output, relatedAction, timing, participant, type, groupingBehavior, selectionBehavior 3947 , requiredBehavior, precheckBehavior, cardinalityBehavior, definition, transform, dynamicValue 3948 , action); 3949 } 3950 3951 public String fhirType() { 3952 return "PlanDefinition.action"; 3953 3954 } 3955 3956 } 3957 3958 @Block() 3959 public static class PlanDefinitionActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 3960 /** 3961 * The kind of condition. 3962 */ 3963 @Child(name = "kind", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3964 @Description(shortDefinition="applicability | start | stop", formalDefinition="The kind of condition." ) 3965 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-condition-kind") 3966 protected Enumeration<ActionConditionKind> kind; 3967 3968 /** 3969 * A brief, natural language description of the condition that effectively communicates the intended semantics. 3970 */ 3971 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3972 @Description(shortDefinition="Natural language description of the condition", formalDefinition="A brief, natural language description of the condition that effectively communicates the intended semantics." ) 3973 protected StringType description; 3974 3975 /** 3976 * The media type of the language for the expression. 3977 */ 3978 @Child(name = "language", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3979 @Description(shortDefinition="Language of the expression", formalDefinition="The media type of the language for the expression." ) 3980 protected StringType language; 3981 3982 /** 3983 * An expression that returns true or false, indicating whether or not the condition is satisfied. 3984 */ 3985 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3986 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether or not the condition is satisfied." ) 3987 protected StringType expression; 3988 3989 private static final long serialVersionUID = 944300105L; 3990 3991 /** 3992 * Constructor 3993 */ 3994 public PlanDefinitionActionConditionComponent() { 3995 super(); 3996 } 3997 3998 /** 3999 * Constructor 4000 */ 4001 public PlanDefinitionActionConditionComponent(Enumeration<ActionConditionKind> kind) { 4002 super(); 4003 this.kind = kind; 4004 } 4005 4006 /** 4007 * @return {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4008 */ 4009 public Enumeration<ActionConditionKind> getKindElement() { 4010 if (this.kind == null) 4011 if (Configuration.errorOnAutoCreate()) 4012 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.kind"); 4013 else if (Configuration.doAutoCreate()) 4014 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 4015 return this.kind; 4016 } 4017 4018 public boolean hasKindElement() { 4019 return this.kind != null && !this.kind.isEmpty(); 4020 } 4021 4022 public boolean hasKind() { 4023 return this.kind != null && !this.kind.isEmpty(); 4024 } 4025 4026 /** 4027 * @param value {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4028 */ 4029 public PlanDefinitionActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 4030 this.kind = value; 4031 return this; 4032 } 4033 4034 /** 4035 * @return The kind of condition. 4036 */ 4037 public ActionConditionKind getKind() { 4038 return this.kind == null ? null : this.kind.getValue(); 4039 } 4040 4041 /** 4042 * @param value The kind of condition. 4043 */ 4044 public PlanDefinitionActionConditionComponent setKind(ActionConditionKind value) { 4045 if (this.kind == null) 4046 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 4047 this.kind.setValue(value); 4048 return this; 4049 } 4050 4051 /** 4052 * @return {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4053 */ 4054 public StringType getDescriptionElement() { 4055 if (this.description == null) 4056 if (Configuration.errorOnAutoCreate()) 4057 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.description"); 4058 else if (Configuration.doAutoCreate()) 4059 this.description = new StringType(); // bb 4060 return this.description; 4061 } 4062 4063 public boolean hasDescriptionElement() { 4064 return this.description != null && !this.description.isEmpty(); 4065 } 4066 4067 public boolean hasDescription() { 4068 return this.description != null && !this.description.isEmpty(); 4069 } 4070 4071 /** 4072 * @param value {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4073 */ 4074 public PlanDefinitionActionConditionComponent setDescriptionElement(StringType value) { 4075 this.description = value; 4076 return this; 4077 } 4078 4079 /** 4080 * @return A brief, natural language description of the condition that effectively communicates the intended semantics. 4081 */ 4082 public String getDescription() { 4083 return this.description == null ? null : this.description.getValue(); 4084 } 4085 4086 /** 4087 * @param value A brief, natural language description of the condition that effectively communicates the intended semantics. 4088 */ 4089 public PlanDefinitionActionConditionComponent setDescription(String value) { 4090 if (Utilities.noString(value)) 4091 this.description = null; 4092 else { 4093 if (this.description == null) 4094 this.description = new StringType(); 4095 this.description.setValue(value); 4096 } 4097 return this; 4098 } 4099 4100 /** 4101 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 4102 */ 4103 public StringType getLanguageElement() { 4104 if (this.language == null) 4105 if (Configuration.errorOnAutoCreate()) 4106 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.language"); 4107 else if (Configuration.doAutoCreate()) 4108 this.language = new StringType(); // bb 4109 return this.language; 4110 } 4111 4112 public boolean hasLanguageElement() { 4113 return this.language != null && !this.language.isEmpty(); 4114 } 4115 4116 public boolean hasLanguage() { 4117 return this.language != null && !this.language.isEmpty(); 4118 } 4119 4120 /** 4121 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 4122 */ 4123 public PlanDefinitionActionConditionComponent setLanguageElement(StringType value) { 4124 this.language = value; 4125 return this; 4126 } 4127 4128 /** 4129 * @return The media type of the language for the expression. 4130 */ 4131 public String getLanguage() { 4132 return this.language == null ? null : this.language.getValue(); 4133 } 4134 4135 /** 4136 * @param value The media type of the language for the expression. 4137 */ 4138 public PlanDefinitionActionConditionComponent setLanguage(String value) { 4139 if (Utilities.noString(value)) 4140 this.language = null; 4141 else { 4142 if (this.language == null) 4143 this.language = new StringType(); 4144 this.language.setValue(value); 4145 } 4146 return this; 4147 } 4148 4149 /** 4150 * @return {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4151 */ 4152 public StringType getExpressionElement() { 4153 if (this.expression == null) 4154 if (Configuration.errorOnAutoCreate()) 4155 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.expression"); 4156 else if (Configuration.doAutoCreate()) 4157 this.expression = new StringType(); // bb 4158 return this.expression; 4159 } 4160 4161 public boolean hasExpressionElement() { 4162 return this.expression != null && !this.expression.isEmpty(); 4163 } 4164 4165 public boolean hasExpression() { 4166 return this.expression != null && !this.expression.isEmpty(); 4167 } 4168 4169 /** 4170 * @param value {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4171 */ 4172 public PlanDefinitionActionConditionComponent setExpressionElement(StringType value) { 4173 this.expression = value; 4174 return this; 4175 } 4176 4177 /** 4178 * @return An expression that returns true or false, indicating whether or not the condition is satisfied. 4179 */ 4180 public String getExpression() { 4181 return this.expression == null ? null : this.expression.getValue(); 4182 } 4183 4184 /** 4185 * @param value An expression that returns true or false, indicating whether or not the condition is satisfied. 4186 */ 4187 public PlanDefinitionActionConditionComponent setExpression(String value) { 4188 if (Utilities.noString(value)) 4189 this.expression = null; 4190 else { 4191 if (this.expression == null) 4192 this.expression = new StringType(); 4193 this.expression.setValue(value); 4194 } 4195 return this; 4196 } 4197 4198 protected void listChildren(List<Property> children) { 4199 super.listChildren(children); 4200 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 4201 children.add(new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description)); 4202 children.add(new Property("language", "string", "The media type of the language for the expression.", 0, 1, language)); 4203 children.add(new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression)); 4204 } 4205 4206 @Override 4207 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4208 switch (_hash) { 4209 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 4210 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description); 4211 case -1613589672: /*language*/ return new Property("language", "string", "The media type of the language for the expression.", 0, 1, language); 4212 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression); 4213 default: return super.getNamedProperty(_hash, _name, _checkValid); 4214 } 4215 4216 } 4217 4218 @Override 4219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4220 switch (hash) { 4221 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActionConditionKind> 4222 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 4223 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // StringType 4224 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 4225 default: return super.getProperty(hash, name, checkValid); 4226 } 4227 4228 } 4229 4230 @Override 4231 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4232 switch (hash) { 4233 case 3292052: // kind 4234 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 4235 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 4236 return value; 4237 case -1724546052: // description 4238 this.description = castToString(value); // StringType 4239 return value; 4240 case -1613589672: // language 4241 this.language = castToString(value); // StringType 4242 return value; 4243 case -1795452264: // expression 4244 this.expression = castToString(value); // StringType 4245 return value; 4246 default: return super.setProperty(hash, name, value); 4247 } 4248 4249 } 4250 4251 @Override 4252 public Base setProperty(String name, Base value) throws FHIRException { 4253 if (name.equals("kind")) { 4254 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 4255 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 4256 } else if (name.equals("description")) { 4257 this.description = castToString(value); // StringType 4258 } else if (name.equals("language")) { 4259 this.language = castToString(value); // StringType 4260 } else if (name.equals("expression")) { 4261 this.expression = castToString(value); // StringType 4262 } else 4263 return super.setProperty(name, value); 4264 return value; 4265 } 4266 4267 @Override 4268 public Base makeProperty(int hash, String name) throws FHIRException { 4269 switch (hash) { 4270 case 3292052: return getKindElement(); 4271 case -1724546052: return getDescriptionElement(); 4272 case -1613589672: return getLanguageElement(); 4273 case -1795452264: return getExpressionElement(); 4274 default: return super.makeProperty(hash, name); 4275 } 4276 4277 } 4278 4279 @Override 4280 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4281 switch (hash) { 4282 case 3292052: /*kind*/ return new String[] {"code"}; 4283 case -1724546052: /*description*/ return new String[] {"string"}; 4284 case -1613589672: /*language*/ return new String[] {"string"}; 4285 case -1795452264: /*expression*/ return new String[] {"string"}; 4286 default: return super.getTypesForProperty(hash, name); 4287 } 4288 4289 } 4290 4291 @Override 4292 public Base addChild(String name) throws FHIRException { 4293 if (name.equals("kind")) { 4294 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.kind"); 4295 } 4296 else if (name.equals("description")) { 4297 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 4298 } 4299 else if (name.equals("language")) { 4300 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.language"); 4301 } 4302 else if (name.equals("expression")) { 4303 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.expression"); 4304 } 4305 else 4306 return super.addChild(name); 4307 } 4308 4309 public PlanDefinitionActionConditionComponent copy() { 4310 PlanDefinitionActionConditionComponent dst = new PlanDefinitionActionConditionComponent(); 4311 copyValues(dst); 4312 dst.kind = kind == null ? null : kind.copy(); 4313 dst.description = description == null ? null : description.copy(); 4314 dst.language = language == null ? null : language.copy(); 4315 dst.expression = expression == null ? null : expression.copy(); 4316 return dst; 4317 } 4318 4319 @Override 4320 public boolean equalsDeep(Base other_) { 4321 if (!super.equalsDeep(other_)) 4322 return false; 4323 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 4324 return false; 4325 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 4326 return compareDeep(kind, o.kind, true) && compareDeep(description, o.description, true) && compareDeep(language, o.language, true) 4327 && compareDeep(expression, o.expression, true); 4328 } 4329 4330 @Override 4331 public boolean equalsShallow(Base other_) { 4332 if (!super.equalsShallow(other_)) 4333 return false; 4334 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 4335 return false; 4336 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 4337 return compareValues(kind, o.kind, true) && compareValues(description, o.description, true) && compareValues(language, o.language, true) 4338 && compareValues(expression, o.expression, true); 4339 } 4340 4341 public boolean isEmpty() { 4342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, description, language 4343 , expression); 4344 } 4345 4346 public String fhirType() { 4347 return "PlanDefinition.action.condition"; 4348 4349 } 4350 4351 } 4352 4353 @Block() 4354 public static class PlanDefinitionActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 4355 /** 4356 * The element id of the related action. 4357 */ 4358 @Child(name = "actionId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4359 @Description(shortDefinition="What action is this related to", formalDefinition="The element id of the related action." ) 4360 protected IdType actionId; 4361 4362 /** 4363 * The relationship of this action to the related action. 4364 */ 4365 @Child(name = "relationship", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4366 @Description(shortDefinition="before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition="The relationship of this action to the related action." ) 4367 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 4368 protected Enumeration<ActionRelationshipType> relationship; 4369 4370 /** 4371 * A duration or range of durations to apply to the relationship. For example, 30-60 minutes before. 4372 */ 4373 @Child(name = "offset", type = {Duration.class, Range.class}, order=3, min=0, max=1, modifier=false, summary=false) 4374 @Description(shortDefinition="Time offset for the relationship", formalDefinition="A duration or range of durations to apply to the relationship. For example, 30-60 minutes before." ) 4375 protected Type offset; 4376 4377 private static final long serialVersionUID = 1063306770L; 4378 4379 /** 4380 * Constructor 4381 */ 4382 public PlanDefinitionActionRelatedActionComponent() { 4383 super(); 4384 } 4385 4386 /** 4387 * Constructor 4388 */ 4389 public PlanDefinitionActionRelatedActionComponent(IdType actionId, Enumeration<ActionRelationshipType> relationship) { 4390 super(); 4391 this.actionId = actionId; 4392 this.relationship = relationship; 4393 } 4394 4395 /** 4396 * @return {@link #actionId} (The element id of the related action.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 4397 */ 4398 public IdType getActionIdElement() { 4399 if (this.actionId == null) 4400 if (Configuration.errorOnAutoCreate()) 4401 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.actionId"); 4402 else if (Configuration.doAutoCreate()) 4403 this.actionId = new IdType(); // bb 4404 return this.actionId; 4405 } 4406 4407 public boolean hasActionIdElement() { 4408 return this.actionId != null && !this.actionId.isEmpty(); 4409 } 4410 4411 public boolean hasActionId() { 4412 return this.actionId != null && !this.actionId.isEmpty(); 4413 } 4414 4415 /** 4416 * @param value {@link #actionId} (The element id of the related action.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 4417 */ 4418 public PlanDefinitionActionRelatedActionComponent setActionIdElement(IdType value) { 4419 this.actionId = value; 4420 return this; 4421 } 4422 4423 /** 4424 * @return The element id of the related action. 4425 */ 4426 public String getActionId() { 4427 return this.actionId == null ? null : this.actionId.getValue(); 4428 } 4429 4430 /** 4431 * @param value The element id of the related action. 4432 */ 4433 public PlanDefinitionActionRelatedActionComponent setActionId(String value) { 4434 if (this.actionId == null) 4435 this.actionId = new IdType(); 4436 this.actionId.setValue(value); 4437 return this; 4438 } 4439 4440 /** 4441 * @return {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 4442 */ 4443 public Enumeration<ActionRelationshipType> getRelationshipElement() { 4444 if (this.relationship == null) 4445 if (Configuration.errorOnAutoCreate()) 4446 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.relationship"); 4447 else if (Configuration.doAutoCreate()) 4448 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 4449 return this.relationship; 4450 } 4451 4452 public boolean hasRelationshipElement() { 4453 return this.relationship != null && !this.relationship.isEmpty(); 4454 } 4455 4456 public boolean hasRelationship() { 4457 return this.relationship != null && !this.relationship.isEmpty(); 4458 } 4459 4460 /** 4461 * @param value {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 4462 */ 4463 public PlanDefinitionActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 4464 this.relationship = value; 4465 return this; 4466 } 4467 4468 /** 4469 * @return The relationship of this action to the related action. 4470 */ 4471 public ActionRelationshipType getRelationship() { 4472 return this.relationship == null ? null : this.relationship.getValue(); 4473 } 4474 4475 /** 4476 * @param value The relationship of this action to the related action. 4477 */ 4478 public PlanDefinitionActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 4479 if (this.relationship == null) 4480 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 4481 this.relationship.setValue(value); 4482 return this; 4483 } 4484 4485 /** 4486 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 4487 */ 4488 public Type getOffset() { 4489 return this.offset; 4490 } 4491 4492 /** 4493 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 4494 */ 4495 public Duration getOffsetDuration() throws FHIRException { 4496 if (this.offset == null) 4497 return null; 4498 if (!(this.offset instanceof Duration)) 4499 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.offset.getClass().getName()+" was encountered"); 4500 return (Duration) this.offset; 4501 } 4502 4503 public boolean hasOffsetDuration() { 4504 return this != null && this.offset instanceof Duration; 4505 } 4506 4507 /** 4508 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 4509 */ 4510 public Range getOffsetRange() throws FHIRException { 4511 if (this.offset == null) 4512 return null; 4513 if (!(this.offset instanceof Range)) 4514 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.offset.getClass().getName()+" was encountered"); 4515 return (Range) this.offset; 4516 } 4517 4518 public boolean hasOffsetRange() { 4519 return this != null && this.offset instanceof Range; 4520 } 4521 4522 public boolean hasOffset() { 4523 return this.offset != null && !this.offset.isEmpty(); 4524 } 4525 4526 /** 4527 * @param value {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 4528 */ 4529 public PlanDefinitionActionRelatedActionComponent setOffset(Type value) throws FHIRFormatError { 4530 if (value != null && !(value instanceof Duration || value instanceof Range)) 4531 throw new FHIRFormatError("Not the right type for PlanDefinition.action.relatedAction.offset[x]: "+value.fhirType()); 4532 this.offset = value; 4533 return this; 4534 } 4535 4536 protected void listChildren(List<Property> children) { 4537 super.listChildren(children); 4538 children.add(new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId)); 4539 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship)); 4540 children.add(new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset)); 4541 } 4542 4543 @Override 4544 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4545 switch (_hash) { 4546 case -1656172047: /*actionId*/ return new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId); 4547 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship); 4548 case -1960684787: /*offset[x]*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 4549 case -1019779949: /*offset*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 4550 case 134075207: /*offsetDuration*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 4551 case 1263585386: /*offsetRange*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 4552 default: return super.getNamedProperty(_hash, _name, _checkValid); 4553 } 4554 4555 } 4556 4557 @Override 4558 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4559 switch (hash) { 4560 case -1656172047: /*actionId*/ return this.actionId == null ? new Base[0] : new Base[] {this.actionId}; // IdType 4561 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ActionRelationshipType> 4562 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // Type 4563 default: return super.getProperty(hash, name, checkValid); 4564 } 4565 4566 } 4567 4568 @Override 4569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4570 switch (hash) { 4571 case -1656172047: // actionId 4572 this.actionId = castToId(value); // IdType 4573 return value; 4574 case -261851592: // relationship 4575 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4576 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4577 return value; 4578 case -1019779949: // offset 4579 this.offset = castToType(value); // Type 4580 return value; 4581 default: return super.setProperty(hash, name, value); 4582 } 4583 4584 } 4585 4586 @Override 4587 public Base setProperty(String name, Base value) throws FHIRException { 4588 if (name.equals("actionId")) { 4589 this.actionId = castToId(value); // IdType 4590 } else if (name.equals("relationship")) { 4591 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4592 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4593 } else if (name.equals("offset[x]")) { 4594 this.offset = castToType(value); // Type 4595 } else 4596 return super.setProperty(name, value); 4597 return value; 4598 } 4599 4600 @Override 4601 public Base makeProperty(int hash, String name) throws FHIRException { 4602 switch (hash) { 4603 case -1656172047: return getActionIdElement(); 4604 case -261851592: return getRelationshipElement(); 4605 case -1960684787: return getOffset(); 4606 case -1019779949: return getOffset(); 4607 default: return super.makeProperty(hash, name); 4608 } 4609 4610 } 4611 4612 @Override 4613 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4614 switch (hash) { 4615 case -1656172047: /*actionId*/ return new String[] {"id"}; 4616 case -261851592: /*relationship*/ return new String[] {"code"}; 4617 case -1019779949: /*offset*/ return new String[] {"Duration", "Range"}; 4618 default: return super.getTypesForProperty(hash, name); 4619 } 4620 4621 } 4622 4623 @Override 4624 public Base addChild(String name) throws FHIRException { 4625 if (name.equals("actionId")) { 4626 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actionId"); 4627 } 4628 else if (name.equals("relationship")) { 4629 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.relationship"); 4630 } 4631 else if (name.equals("offsetDuration")) { 4632 this.offset = new Duration(); 4633 return this.offset; 4634 } 4635 else if (name.equals("offsetRange")) { 4636 this.offset = new Range(); 4637 return this.offset; 4638 } 4639 else 4640 return super.addChild(name); 4641 } 4642 4643 public PlanDefinitionActionRelatedActionComponent copy() { 4644 PlanDefinitionActionRelatedActionComponent dst = new PlanDefinitionActionRelatedActionComponent(); 4645 copyValues(dst); 4646 dst.actionId = actionId == null ? null : actionId.copy(); 4647 dst.relationship = relationship == null ? null : relationship.copy(); 4648 dst.offset = offset == null ? null : offset.copy(); 4649 return dst; 4650 } 4651 4652 @Override 4653 public boolean equalsDeep(Base other_) { 4654 if (!super.equalsDeep(other_)) 4655 return false; 4656 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 4657 return false; 4658 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 4659 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 4660 && compareDeep(offset, o.offset, true); 4661 } 4662 4663 @Override 4664 public boolean equalsShallow(Base other_) { 4665 if (!super.equalsShallow(other_)) 4666 return false; 4667 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 4668 return false; 4669 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 4670 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true) 4671 ; 4672 } 4673 4674 public boolean isEmpty() { 4675 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset 4676 ); 4677 } 4678 4679 public String fhirType() { 4680 return "PlanDefinition.action.relatedAction"; 4681 4682 } 4683 4684 } 4685 4686 @Block() 4687 public static class PlanDefinitionActionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 4688 /** 4689 * The type of participant in the action. 4690 */ 4691 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4692 @Description(shortDefinition="patient | practitioner | related-person", formalDefinition="The type of participant in the action." ) 4693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 4694 protected Enumeration<ActionParticipantType> type; 4695 4696 /** 4697 * The role the participant should play in performing the described action. 4698 */ 4699 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4700 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent, etc", formalDefinition="The role the participant should play in performing the described action." ) 4701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-role") 4702 protected CodeableConcept role; 4703 4704 private static final long serialVersionUID = -1152013659L; 4705 4706 /** 4707 * Constructor 4708 */ 4709 public PlanDefinitionActionParticipantComponent() { 4710 super(); 4711 } 4712 4713 /** 4714 * Constructor 4715 */ 4716 public PlanDefinitionActionParticipantComponent(Enumeration<ActionParticipantType> type) { 4717 super(); 4718 this.type = type; 4719 } 4720 4721 /** 4722 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4723 */ 4724 public Enumeration<ActionParticipantType> getTypeElement() { 4725 if (this.type == null) 4726 if (Configuration.errorOnAutoCreate()) 4727 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.type"); 4728 else if (Configuration.doAutoCreate()) 4729 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 4730 return this.type; 4731 } 4732 4733 public boolean hasTypeElement() { 4734 return this.type != null && !this.type.isEmpty(); 4735 } 4736 4737 public boolean hasType() { 4738 return this.type != null && !this.type.isEmpty(); 4739 } 4740 4741 /** 4742 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4743 */ 4744 public PlanDefinitionActionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 4745 this.type = value; 4746 return this; 4747 } 4748 4749 /** 4750 * @return The type of participant in the action. 4751 */ 4752 public ActionParticipantType getType() { 4753 return this.type == null ? null : this.type.getValue(); 4754 } 4755 4756 /** 4757 * @param value The type of participant in the action. 4758 */ 4759 public PlanDefinitionActionParticipantComponent setType(ActionParticipantType value) { 4760 if (this.type == null) 4761 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 4762 this.type.setValue(value); 4763 return this; 4764 } 4765 4766 /** 4767 * @return {@link #role} (The role the participant should play in performing the described action.) 4768 */ 4769 public CodeableConcept getRole() { 4770 if (this.role == null) 4771 if (Configuration.errorOnAutoCreate()) 4772 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.role"); 4773 else if (Configuration.doAutoCreate()) 4774 this.role = new CodeableConcept(); // cc 4775 return this.role; 4776 } 4777 4778 public boolean hasRole() { 4779 return this.role != null && !this.role.isEmpty(); 4780 } 4781 4782 /** 4783 * @param value {@link #role} (The role the participant should play in performing the described action.) 4784 */ 4785 public PlanDefinitionActionParticipantComponent setRole(CodeableConcept value) { 4786 this.role = value; 4787 return this; 4788 } 4789 4790 protected void listChildren(List<Property> children) { 4791 super.listChildren(children); 4792 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 4793 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 4794 } 4795 4796 @Override 4797 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4798 switch (_hash) { 4799 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 4800 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 4801 default: return super.getNamedProperty(_hash, _name, _checkValid); 4802 } 4803 4804 } 4805 4806 @Override 4807 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4808 switch (hash) { 4809 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActionParticipantType> 4810 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 4811 default: return super.getProperty(hash, name, checkValid); 4812 } 4813 4814 } 4815 4816 @Override 4817 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4818 switch (hash) { 4819 case 3575610: // type 4820 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 4821 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 4822 return value; 4823 case 3506294: // role 4824 this.role = castToCodeableConcept(value); // CodeableConcept 4825 return value; 4826 default: return super.setProperty(hash, name, value); 4827 } 4828 4829 } 4830 4831 @Override 4832 public Base setProperty(String name, Base value) throws FHIRException { 4833 if (name.equals("type")) { 4834 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 4835 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 4836 } else if (name.equals("role")) { 4837 this.role = castToCodeableConcept(value); // CodeableConcept 4838 } else 4839 return super.setProperty(name, value); 4840 return value; 4841 } 4842 4843 @Override 4844 public Base makeProperty(int hash, String name) throws FHIRException { 4845 switch (hash) { 4846 case 3575610: return getTypeElement(); 4847 case 3506294: return getRole(); 4848 default: return super.makeProperty(hash, name); 4849 } 4850 4851 } 4852 4853 @Override 4854 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4855 switch (hash) { 4856 case 3575610: /*type*/ return new String[] {"code"}; 4857 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 4858 default: return super.getTypesForProperty(hash, name); 4859 } 4860 4861 } 4862 4863 @Override 4864 public Base addChild(String name) throws FHIRException { 4865 if (name.equals("type")) { 4866 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.type"); 4867 } 4868 else if (name.equals("role")) { 4869 this.role = new CodeableConcept(); 4870 return this.role; 4871 } 4872 else 4873 return super.addChild(name); 4874 } 4875 4876 public PlanDefinitionActionParticipantComponent copy() { 4877 PlanDefinitionActionParticipantComponent dst = new PlanDefinitionActionParticipantComponent(); 4878 copyValues(dst); 4879 dst.type = type == null ? null : type.copy(); 4880 dst.role = role == null ? null : role.copy(); 4881 return dst; 4882 } 4883 4884 @Override 4885 public boolean equalsDeep(Base other_) { 4886 if (!super.equalsDeep(other_)) 4887 return false; 4888 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 4889 return false; 4890 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 4891 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 4892 } 4893 4894 @Override 4895 public boolean equalsShallow(Base other_) { 4896 if (!super.equalsShallow(other_)) 4897 return false; 4898 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 4899 return false; 4900 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 4901 return compareValues(type, o.type, true); 4902 } 4903 4904 public boolean isEmpty() { 4905 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 4906 } 4907 4908 public String fhirType() { 4909 return "PlanDefinition.action.participant"; 4910 4911 } 4912 4913 } 4914 4915 @Block() 4916 public static class PlanDefinitionActionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 4917 /** 4918 * A brief, natural language description of the intended semantics of the dynamic value. 4919 */ 4920 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 4921 @Description(shortDefinition="Natural language description of the dynamic value", formalDefinition="A brief, natural language description of the intended semantics of the dynamic value." ) 4922 protected StringType description; 4923 4924 /** 4925 * The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 4926 */ 4927 @Child(name = "path", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4928 @Description(shortDefinition="The path to the element to be set dynamically", formalDefinition="The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression." ) 4929 protected StringType path; 4930 4931 /** 4932 * The media type of the language for the expression. 4933 */ 4934 @Child(name = "language", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4935 @Description(shortDefinition="Language of the expression", formalDefinition="The media type of the language for the expression." ) 4936 protected StringType language; 4937 4938 /** 4939 * An expression specifying the value of the customized element. 4940 */ 4941 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4942 @Description(shortDefinition="An expression that provides the dynamic value for the customization", formalDefinition="An expression specifying the value of the customized element." ) 4943 protected StringType expression; 4944 4945 private static final long serialVersionUID = 448404361L; 4946 4947 /** 4948 * Constructor 4949 */ 4950 public PlanDefinitionActionDynamicValueComponent() { 4951 super(); 4952 } 4953 4954 /** 4955 * @return {@link #description} (A brief, natural language description of the intended semantics of the dynamic value.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4956 */ 4957 public StringType getDescriptionElement() { 4958 if (this.description == null) 4959 if (Configuration.errorOnAutoCreate()) 4960 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.description"); 4961 else if (Configuration.doAutoCreate()) 4962 this.description = new StringType(); // bb 4963 return this.description; 4964 } 4965 4966 public boolean hasDescriptionElement() { 4967 return this.description != null && !this.description.isEmpty(); 4968 } 4969 4970 public boolean hasDescription() { 4971 return this.description != null && !this.description.isEmpty(); 4972 } 4973 4974 /** 4975 * @param value {@link #description} (A brief, natural language description of the intended semantics of the dynamic value.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4976 */ 4977 public PlanDefinitionActionDynamicValueComponent setDescriptionElement(StringType value) { 4978 this.description = value; 4979 return this; 4980 } 4981 4982 /** 4983 * @return A brief, natural language description of the intended semantics of the dynamic value. 4984 */ 4985 public String getDescription() { 4986 return this.description == null ? null : this.description.getValue(); 4987 } 4988 4989 /** 4990 * @param value A brief, natural language description of the intended semantics of the dynamic value. 4991 */ 4992 public PlanDefinitionActionDynamicValueComponent setDescription(String value) { 4993 if (Utilities.noString(value)) 4994 this.description = null; 4995 else { 4996 if (this.description == null) 4997 this.description = new StringType(); 4998 this.description.setValue(value); 4999 } 5000 return this; 5001 } 5002 5003 /** 5004 * @return {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 5005 */ 5006 public StringType getPathElement() { 5007 if (this.path == null) 5008 if (Configuration.errorOnAutoCreate()) 5009 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.path"); 5010 else if (Configuration.doAutoCreate()) 5011 this.path = new StringType(); // bb 5012 return this.path; 5013 } 5014 5015 public boolean hasPathElement() { 5016 return this.path != null && !this.path.isEmpty(); 5017 } 5018 5019 public boolean hasPath() { 5020 return this.path != null && !this.path.isEmpty(); 5021 } 5022 5023 /** 5024 * @param value {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 5025 */ 5026 public PlanDefinitionActionDynamicValueComponent setPathElement(StringType value) { 5027 this.path = value; 5028 return this; 5029 } 5030 5031 /** 5032 * @return The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 5033 */ 5034 public String getPath() { 5035 return this.path == null ? null : this.path.getValue(); 5036 } 5037 5038 /** 5039 * @param value The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. 5040 */ 5041 public PlanDefinitionActionDynamicValueComponent setPath(String value) { 5042 if (Utilities.noString(value)) 5043 this.path = null; 5044 else { 5045 if (this.path == null) 5046 this.path = new StringType(); 5047 this.path.setValue(value); 5048 } 5049 return this; 5050 } 5051 5052 /** 5053 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5054 */ 5055 public StringType getLanguageElement() { 5056 if (this.language == null) 5057 if (Configuration.errorOnAutoCreate()) 5058 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.language"); 5059 else if (Configuration.doAutoCreate()) 5060 this.language = new StringType(); // bb 5061 return this.language; 5062 } 5063 5064 public boolean hasLanguageElement() { 5065 return this.language != null && !this.language.isEmpty(); 5066 } 5067 5068 public boolean hasLanguage() { 5069 return this.language != null && !this.language.isEmpty(); 5070 } 5071 5072 /** 5073 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5074 */ 5075 public PlanDefinitionActionDynamicValueComponent setLanguageElement(StringType value) { 5076 this.language = value; 5077 return this; 5078 } 5079 5080 /** 5081 * @return The media type of the language for the expression. 5082 */ 5083 public String getLanguage() { 5084 return this.language == null ? null : this.language.getValue(); 5085 } 5086 5087 /** 5088 * @param value The media type of the language for the expression. 5089 */ 5090 public PlanDefinitionActionDynamicValueComponent setLanguage(String value) { 5091 if (Utilities.noString(value)) 5092 this.language = null; 5093 else { 5094 if (this.language == null) 5095 this.language = new StringType(); 5096 this.language.setValue(value); 5097 } 5098 return this; 5099 } 5100 5101 /** 5102 * @return {@link #expression} (An expression specifying the value of the customized element.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 5103 */ 5104 public StringType getExpressionElement() { 5105 if (this.expression == null) 5106 if (Configuration.errorOnAutoCreate()) 5107 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.expression"); 5108 else if (Configuration.doAutoCreate()) 5109 this.expression = new StringType(); // bb 5110 return this.expression; 5111 } 5112 5113 public boolean hasExpressionElement() { 5114 return this.expression != null && !this.expression.isEmpty(); 5115 } 5116 5117 public boolean hasExpression() { 5118 return this.expression != null && !this.expression.isEmpty(); 5119 } 5120 5121 /** 5122 * @param value {@link #expression} (An expression specifying the value of the customized element.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 5123 */ 5124 public PlanDefinitionActionDynamicValueComponent setExpressionElement(StringType value) { 5125 this.expression = value; 5126 return this; 5127 } 5128 5129 /** 5130 * @return An expression specifying the value of the customized element. 5131 */ 5132 public String getExpression() { 5133 return this.expression == null ? null : this.expression.getValue(); 5134 } 5135 5136 /** 5137 * @param value An expression specifying the value of the customized element. 5138 */ 5139 public PlanDefinitionActionDynamicValueComponent setExpression(String value) { 5140 if (Utilities.noString(value)) 5141 this.expression = null; 5142 else { 5143 if (this.expression == null) 5144 this.expression = new StringType(); 5145 this.expression.setValue(value); 5146 } 5147 return this; 5148 } 5149 5150 protected void listChildren(List<Property> children) { 5151 super.listChildren(children); 5152 children.add(new Property("description", "string", "A brief, natural language description of the intended semantics of the dynamic value.", 0, 1, description)); 5153 children.add(new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.", 0, 1, path)); 5154 children.add(new Property("language", "string", "The media type of the language for the expression.", 0, 1, language)); 5155 children.add(new Property("expression", "string", "An expression specifying the value of the customized element.", 0, 1, expression)); 5156 } 5157 5158 @Override 5159 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5160 switch (_hash) { 5161 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the intended semantics of the dynamic value.", 0, 1, description); 5162 case 3433509: /*path*/ return new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression.", 0, 1, path); 5163 case -1613589672: /*language*/ return new Property("language", "string", "The media type of the language for the expression.", 0, 1, language); 5164 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression specifying the value of the customized element.", 0, 1, expression); 5165 default: return super.getNamedProperty(_hash, _name, _checkValid); 5166 } 5167 5168 } 5169 5170 @Override 5171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5172 switch (hash) { 5173 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 5174 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 5175 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // StringType 5176 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 5177 default: return super.getProperty(hash, name, checkValid); 5178 } 5179 5180 } 5181 5182 @Override 5183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5184 switch (hash) { 5185 case -1724546052: // description 5186 this.description = castToString(value); // StringType 5187 return value; 5188 case 3433509: // path 5189 this.path = castToString(value); // StringType 5190 return value; 5191 case -1613589672: // language 5192 this.language = castToString(value); // StringType 5193 return value; 5194 case -1795452264: // expression 5195 this.expression = castToString(value); // StringType 5196 return value; 5197 default: return super.setProperty(hash, name, value); 5198 } 5199 5200 } 5201 5202 @Override 5203 public Base setProperty(String name, Base value) throws FHIRException { 5204 if (name.equals("description")) { 5205 this.description = castToString(value); // StringType 5206 } else if (name.equals("path")) { 5207 this.path = castToString(value); // StringType 5208 } else if (name.equals("language")) { 5209 this.language = castToString(value); // StringType 5210 } else if (name.equals("expression")) { 5211 this.expression = castToString(value); // StringType 5212 } else 5213 return super.setProperty(name, value); 5214 return value; 5215 } 5216 5217 @Override 5218 public Base makeProperty(int hash, String name) throws FHIRException { 5219 switch (hash) { 5220 case -1724546052: return getDescriptionElement(); 5221 case 3433509: return getPathElement(); 5222 case -1613589672: return getLanguageElement(); 5223 case -1795452264: return getExpressionElement(); 5224 default: return super.makeProperty(hash, name); 5225 } 5226 5227 } 5228 5229 @Override 5230 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5231 switch (hash) { 5232 case -1724546052: /*description*/ return new String[] {"string"}; 5233 case 3433509: /*path*/ return new String[] {"string"}; 5234 case -1613589672: /*language*/ return new String[] {"string"}; 5235 case -1795452264: /*expression*/ return new String[] {"string"}; 5236 default: return super.getTypesForProperty(hash, name); 5237 } 5238 5239 } 5240 5241 @Override 5242 public Base addChild(String name) throws FHIRException { 5243 if (name.equals("description")) { 5244 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 5245 } 5246 else if (name.equals("path")) { 5247 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.path"); 5248 } 5249 else if (name.equals("language")) { 5250 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.language"); 5251 } 5252 else if (name.equals("expression")) { 5253 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.expression"); 5254 } 5255 else 5256 return super.addChild(name); 5257 } 5258 5259 public PlanDefinitionActionDynamicValueComponent copy() { 5260 PlanDefinitionActionDynamicValueComponent dst = new PlanDefinitionActionDynamicValueComponent(); 5261 copyValues(dst); 5262 dst.description = description == null ? null : description.copy(); 5263 dst.path = path == null ? null : path.copy(); 5264 dst.language = language == null ? null : language.copy(); 5265 dst.expression = expression == null ? null : expression.copy(); 5266 return dst; 5267 } 5268 5269 @Override 5270 public boolean equalsDeep(Base other_) { 5271 if (!super.equalsDeep(other_)) 5272 return false; 5273 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 5274 return false; 5275 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 5276 return compareDeep(description, o.description, true) && compareDeep(path, o.path, true) && compareDeep(language, o.language, true) 5277 && compareDeep(expression, o.expression, true); 5278 } 5279 5280 @Override 5281 public boolean equalsShallow(Base other_) { 5282 if (!super.equalsShallow(other_)) 5283 return false; 5284 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 5285 return false; 5286 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 5287 return compareValues(description, o.description, true) && compareValues(path, o.path, true) && compareValues(language, o.language, true) 5288 && compareValues(expression, o.expression, true); 5289 } 5290 5291 public boolean isEmpty() { 5292 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, path, language 5293 , expression); 5294 } 5295 5296 public String fhirType() { 5297 return "PlanDefinition.action.dynamicValue"; 5298 5299 } 5300 5301 } 5302 5303 /** 5304 * A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 5305 */ 5306 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5307 @Description(shortDefinition="Additional identifier for the plan definition", formalDefinition="A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 5308 protected List<Identifier> identifier; 5309 5310 /** 5311 * The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule. 5312 */ 5313 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 5314 @Description(shortDefinition="order-set | protocol | eca-rule", formalDefinition="The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule." ) 5315 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/plan-definition-type") 5316 protected CodeableConcept type; 5317 5318 /** 5319 * Explaination of why this plan definition is needed and why it has been designed as it has. 5320 */ 5321 @Child(name = "purpose", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5322 @Description(shortDefinition="Why this plan definition is defined", formalDefinition="Explaination of why this plan definition is needed and why it has been designed as it has." ) 5323 protected MarkdownType purpose; 5324 5325 /** 5326 * A detailed description of how the asset is used from a clinical perspective. 5327 */ 5328 @Child(name = "usage", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5329 @Description(shortDefinition="Describes the clinical usage of the asset", formalDefinition="A detailed description of how the asset is used from a clinical perspective." ) 5330 protected StringType usage; 5331 5332 /** 5333 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 5334 */ 5335 @Child(name = "approvalDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 5336 @Description(shortDefinition="When the plan definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 5337 protected DateType approvalDate; 5338 5339 /** 5340 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 5341 */ 5342 @Child(name = "lastReviewDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=false) 5343 @Description(shortDefinition="When the plan definition was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 5344 protected DateType lastReviewDate; 5345 5346 /** 5347 * The period during which the plan definition content was or is planned to be in active use. 5348 */ 5349 @Child(name = "effectivePeriod", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 5350 @Description(shortDefinition="When the plan definition is expected to be used", formalDefinition="The period during which the plan definition content was or is planned to be in active use." ) 5351 protected Period effectivePeriod; 5352 5353 /** 5354 * Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching. 5355 */ 5356 @Child(name = "topic", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5357 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching." ) 5358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 5359 protected List<CodeableConcept> topic; 5360 5361 /** 5362 * A contributor to the content of the asset, including authors, editors, reviewers, and endorsers. 5363 */ 5364 @Child(name = "contributor", type = {Contributor.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5365 @Description(shortDefinition="A content contributor", formalDefinition="A contributor to the content of the asset, including authors, editors, reviewers, and endorsers." ) 5366 protected List<Contributor> contributor; 5367 5368 /** 5369 * A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 5370 */ 5371 @Child(name = "copyright", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5372 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition." ) 5373 protected MarkdownType copyright; 5374 5375 /** 5376 * Related artifacts such as additional documentation, justification, or bibliographic references. 5377 */ 5378 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5379 @Description(shortDefinition="Related artifacts for the asset", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 5380 protected List<RelatedArtifact> relatedArtifact; 5381 5382 /** 5383 * A reference to a Library resource containing any formal logic used by the plan definition. 5384 */ 5385 @Child(name = "library", type = {Library.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5386 @Description(shortDefinition="Logic used by the plan definition", formalDefinition="A reference to a Library resource containing any formal logic used by the plan definition." ) 5387 protected List<Reference> library; 5388 /** 5389 * The actual objects that are the target of the reference (A reference to a Library resource containing any formal logic used by the plan definition.) 5390 */ 5391 protected List<Library> libraryTarget; 5392 5393 5394 /** 5395 * Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 5396 */ 5397 @Child(name = "goal", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5398 @Description(shortDefinition="What the plan is trying to accomplish", formalDefinition="Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc." ) 5399 protected List<PlanDefinitionGoalComponent> goal; 5400 5401 /** 5402 * An action to be taken as part of the plan. 5403 */ 5404 @Child(name = "action", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5405 @Description(shortDefinition="Action defined by the plan", formalDefinition="An action to be taken as part of the plan." ) 5406 protected List<PlanDefinitionActionComponent> action; 5407 5408 private static final long serialVersionUID = -1191108677L; 5409 5410 /** 5411 * Constructor 5412 */ 5413 public PlanDefinition() { 5414 super(); 5415 } 5416 5417 /** 5418 * Constructor 5419 */ 5420 public PlanDefinition(Enumeration<PublicationStatus> status) { 5421 super(); 5422 this.status = status; 5423 } 5424 5425 /** 5426 * @return {@link #url} (An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5427 */ 5428 public UriType getUrlElement() { 5429 if (this.url == null) 5430 if (Configuration.errorOnAutoCreate()) 5431 throw new Error("Attempt to auto-create PlanDefinition.url"); 5432 else if (Configuration.doAutoCreate()) 5433 this.url = new UriType(); // bb 5434 return this.url; 5435 } 5436 5437 public boolean hasUrlElement() { 5438 return this.url != null && !this.url.isEmpty(); 5439 } 5440 5441 public boolean hasUrl() { 5442 return this.url != null && !this.url.isEmpty(); 5443 } 5444 5445 /** 5446 * @param value {@link #url} (An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5447 */ 5448 public PlanDefinition setUrlElement(UriType value) { 5449 this.url = value; 5450 return this; 5451 } 5452 5453 /** 5454 * @return An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions). 5455 */ 5456 public String getUrl() { 5457 return this.url == null ? null : this.url.getValue(); 5458 } 5459 5460 /** 5461 * @param value An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions). 5462 */ 5463 public PlanDefinition setUrl(String value) { 5464 if (Utilities.noString(value)) 5465 this.url = null; 5466 else { 5467 if (this.url == null) 5468 this.url = new UriType(); 5469 this.url.setValue(value); 5470 } 5471 return this; 5472 } 5473 5474 /** 5475 * @return {@link #identifier} (A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 5476 */ 5477 public List<Identifier> getIdentifier() { 5478 if (this.identifier == null) 5479 this.identifier = new ArrayList<Identifier>(); 5480 return this.identifier; 5481 } 5482 5483 /** 5484 * @return Returns a reference to <code>this</code> for easy method chaining 5485 */ 5486 public PlanDefinition setIdentifier(List<Identifier> theIdentifier) { 5487 this.identifier = theIdentifier; 5488 return this; 5489 } 5490 5491 public boolean hasIdentifier() { 5492 if (this.identifier == null) 5493 return false; 5494 for (Identifier item : this.identifier) 5495 if (!item.isEmpty()) 5496 return true; 5497 return false; 5498 } 5499 5500 public Identifier addIdentifier() { //3 5501 Identifier t = new Identifier(); 5502 if (this.identifier == null) 5503 this.identifier = new ArrayList<Identifier>(); 5504 this.identifier.add(t); 5505 return t; 5506 } 5507 5508 public PlanDefinition addIdentifier(Identifier t) { //3 5509 if (t == null) 5510 return this; 5511 if (this.identifier == null) 5512 this.identifier = new ArrayList<Identifier>(); 5513 this.identifier.add(t); 5514 return this; 5515 } 5516 5517 /** 5518 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 5519 */ 5520 public Identifier getIdentifierFirstRep() { 5521 if (getIdentifier().isEmpty()) { 5522 addIdentifier(); 5523 } 5524 return getIdentifier().get(0); 5525 } 5526 5527 /** 5528 * @return {@link #version} (The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5529 */ 5530 public StringType getVersionElement() { 5531 if (this.version == null) 5532 if (Configuration.errorOnAutoCreate()) 5533 throw new Error("Attempt to auto-create PlanDefinition.version"); 5534 else if (Configuration.doAutoCreate()) 5535 this.version = new StringType(); // bb 5536 return this.version; 5537 } 5538 5539 public boolean hasVersionElement() { 5540 return this.version != null && !this.version.isEmpty(); 5541 } 5542 5543 public boolean hasVersion() { 5544 return this.version != null && !this.version.isEmpty(); 5545 } 5546 5547 /** 5548 * @param value {@link #version} (The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5549 */ 5550 public PlanDefinition setVersionElement(StringType value) { 5551 this.version = value; 5552 return this; 5553 } 5554 5555 /** 5556 * @return The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 5557 */ 5558 public String getVersion() { 5559 return this.version == null ? null : this.version.getValue(); 5560 } 5561 5562 /** 5563 * @param value The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 5564 */ 5565 public PlanDefinition setVersion(String value) { 5566 if (Utilities.noString(value)) 5567 this.version = null; 5568 else { 5569 if (this.version == null) 5570 this.version = new StringType(); 5571 this.version.setValue(value); 5572 } 5573 return this; 5574 } 5575 5576 /** 5577 * @return {@link #name} (A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5578 */ 5579 public StringType getNameElement() { 5580 if (this.name == null) 5581 if (Configuration.errorOnAutoCreate()) 5582 throw new Error("Attempt to auto-create PlanDefinition.name"); 5583 else if (Configuration.doAutoCreate()) 5584 this.name = new StringType(); // bb 5585 return this.name; 5586 } 5587 5588 public boolean hasNameElement() { 5589 return this.name != null && !this.name.isEmpty(); 5590 } 5591 5592 public boolean hasName() { 5593 return this.name != null && !this.name.isEmpty(); 5594 } 5595 5596 /** 5597 * @param value {@link #name} (A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5598 */ 5599 public PlanDefinition setNameElement(StringType value) { 5600 this.name = value; 5601 return this; 5602 } 5603 5604 /** 5605 * @return A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5606 */ 5607 public String getName() { 5608 return this.name == null ? null : this.name.getValue(); 5609 } 5610 5611 /** 5612 * @param value A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5613 */ 5614 public PlanDefinition setName(String value) { 5615 if (Utilities.noString(value)) 5616 this.name = null; 5617 else { 5618 if (this.name == null) 5619 this.name = new StringType(); 5620 this.name.setValue(value); 5621 } 5622 return this; 5623 } 5624 5625 /** 5626 * @return {@link #title} (A short, descriptive, user-friendly title for the plan definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5627 */ 5628 public StringType getTitleElement() { 5629 if (this.title == null) 5630 if (Configuration.errorOnAutoCreate()) 5631 throw new Error("Attempt to auto-create PlanDefinition.title"); 5632 else if (Configuration.doAutoCreate()) 5633 this.title = new StringType(); // bb 5634 return this.title; 5635 } 5636 5637 public boolean hasTitleElement() { 5638 return this.title != null && !this.title.isEmpty(); 5639 } 5640 5641 public boolean hasTitle() { 5642 return this.title != null && !this.title.isEmpty(); 5643 } 5644 5645 /** 5646 * @param value {@link #title} (A short, descriptive, user-friendly title for the plan definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5647 */ 5648 public PlanDefinition setTitleElement(StringType value) { 5649 this.title = value; 5650 return this; 5651 } 5652 5653 /** 5654 * @return A short, descriptive, user-friendly title for the plan definition. 5655 */ 5656 public String getTitle() { 5657 return this.title == null ? null : this.title.getValue(); 5658 } 5659 5660 /** 5661 * @param value A short, descriptive, user-friendly title for the plan definition. 5662 */ 5663 public PlanDefinition setTitle(String value) { 5664 if (Utilities.noString(value)) 5665 this.title = null; 5666 else { 5667 if (this.title == null) 5668 this.title = new StringType(); 5669 this.title.setValue(value); 5670 } 5671 return this; 5672 } 5673 5674 /** 5675 * @return {@link #type} (The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule.) 5676 */ 5677 public CodeableConcept getType() { 5678 if (this.type == null) 5679 if (Configuration.errorOnAutoCreate()) 5680 throw new Error("Attempt to auto-create PlanDefinition.type"); 5681 else if (Configuration.doAutoCreate()) 5682 this.type = new CodeableConcept(); // cc 5683 return this.type; 5684 } 5685 5686 public boolean hasType() { 5687 return this.type != null && !this.type.isEmpty(); 5688 } 5689 5690 /** 5691 * @param value {@link #type} (The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule.) 5692 */ 5693 public PlanDefinition setType(CodeableConcept value) { 5694 this.type = value; 5695 return this; 5696 } 5697 5698 /** 5699 * @return {@link #status} (The status of this plan definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5700 */ 5701 public Enumeration<PublicationStatus> getStatusElement() { 5702 if (this.status == null) 5703 if (Configuration.errorOnAutoCreate()) 5704 throw new Error("Attempt to auto-create PlanDefinition.status"); 5705 else if (Configuration.doAutoCreate()) 5706 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 5707 return this.status; 5708 } 5709 5710 public boolean hasStatusElement() { 5711 return this.status != null && !this.status.isEmpty(); 5712 } 5713 5714 public boolean hasStatus() { 5715 return this.status != null && !this.status.isEmpty(); 5716 } 5717 5718 /** 5719 * @param value {@link #status} (The status of this plan definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5720 */ 5721 public PlanDefinition setStatusElement(Enumeration<PublicationStatus> value) { 5722 this.status = value; 5723 return this; 5724 } 5725 5726 /** 5727 * @return The status of this plan definition. Enables tracking the life-cycle of the content. 5728 */ 5729 public PublicationStatus getStatus() { 5730 return this.status == null ? null : this.status.getValue(); 5731 } 5732 5733 /** 5734 * @param value The status of this plan definition. Enables tracking the life-cycle of the content. 5735 */ 5736 public PlanDefinition setStatus(PublicationStatus value) { 5737 if (this.status == null) 5738 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 5739 this.status.setValue(value); 5740 return this; 5741 } 5742 5743 /** 5744 * @return {@link #experimental} (A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5745 */ 5746 public BooleanType getExperimentalElement() { 5747 if (this.experimental == null) 5748 if (Configuration.errorOnAutoCreate()) 5749 throw new Error("Attempt to auto-create PlanDefinition.experimental"); 5750 else if (Configuration.doAutoCreate()) 5751 this.experimental = new BooleanType(); // bb 5752 return this.experimental; 5753 } 5754 5755 public boolean hasExperimentalElement() { 5756 return this.experimental != null && !this.experimental.isEmpty(); 5757 } 5758 5759 public boolean hasExperimental() { 5760 return this.experimental != null && !this.experimental.isEmpty(); 5761 } 5762 5763 /** 5764 * @param value {@link #experimental} (A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5765 */ 5766 public PlanDefinition setExperimentalElement(BooleanType value) { 5767 this.experimental = value; 5768 return this; 5769 } 5770 5771 /** 5772 * @return A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 5773 */ 5774 public boolean getExperimental() { 5775 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 5776 } 5777 5778 /** 5779 * @param value A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 5780 */ 5781 public PlanDefinition setExperimental(boolean value) { 5782 if (this.experimental == null) 5783 this.experimental = new BooleanType(); 5784 this.experimental.setValue(value); 5785 return this; 5786 } 5787 5788 /** 5789 * @return {@link #date} (The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5790 */ 5791 public DateTimeType getDateElement() { 5792 if (this.date == null) 5793 if (Configuration.errorOnAutoCreate()) 5794 throw new Error("Attempt to auto-create PlanDefinition.date"); 5795 else if (Configuration.doAutoCreate()) 5796 this.date = new DateTimeType(); // bb 5797 return this.date; 5798 } 5799 5800 public boolean hasDateElement() { 5801 return this.date != null && !this.date.isEmpty(); 5802 } 5803 5804 public boolean hasDate() { 5805 return this.date != null && !this.date.isEmpty(); 5806 } 5807 5808 /** 5809 * @param value {@link #date} (The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5810 */ 5811 public PlanDefinition setDateElement(DateTimeType value) { 5812 this.date = value; 5813 return this; 5814 } 5815 5816 /** 5817 * @return The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes. 5818 */ 5819 public Date getDate() { 5820 return this.date == null ? null : this.date.getValue(); 5821 } 5822 5823 /** 5824 * @param value The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes. 5825 */ 5826 public PlanDefinition setDate(Date value) { 5827 if (value == null) 5828 this.date = null; 5829 else { 5830 if (this.date == null) 5831 this.date = new DateTimeType(); 5832 this.date.setValue(value); 5833 } 5834 return this; 5835 } 5836 5837 /** 5838 * @return {@link #publisher} (The name of the individual or organization that published the plan definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5839 */ 5840 public StringType getPublisherElement() { 5841 if (this.publisher == null) 5842 if (Configuration.errorOnAutoCreate()) 5843 throw new Error("Attempt to auto-create PlanDefinition.publisher"); 5844 else if (Configuration.doAutoCreate()) 5845 this.publisher = new StringType(); // bb 5846 return this.publisher; 5847 } 5848 5849 public boolean hasPublisherElement() { 5850 return this.publisher != null && !this.publisher.isEmpty(); 5851 } 5852 5853 public boolean hasPublisher() { 5854 return this.publisher != null && !this.publisher.isEmpty(); 5855 } 5856 5857 /** 5858 * @param value {@link #publisher} (The name of the individual or organization that published the plan definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5859 */ 5860 public PlanDefinition setPublisherElement(StringType value) { 5861 this.publisher = value; 5862 return this; 5863 } 5864 5865 /** 5866 * @return The name of the individual or organization that published the plan definition. 5867 */ 5868 public String getPublisher() { 5869 return this.publisher == null ? null : this.publisher.getValue(); 5870 } 5871 5872 /** 5873 * @param value The name of the individual or organization that published the plan definition. 5874 */ 5875 public PlanDefinition setPublisher(String value) { 5876 if (Utilities.noString(value)) 5877 this.publisher = null; 5878 else { 5879 if (this.publisher == null) 5880 this.publisher = new StringType(); 5881 this.publisher.setValue(value); 5882 } 5883 return this; 5884 } 5885 5886 /** 5887 * @return {@link #description} (A free text natural language description of the plan definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5888 */ 5889 public MarkdownType getDescriptionElement() { 5890 if (this.description == null) 5891 if (Configuration.errorOnAutoCreate()) 5892 throw new Error("Attempt to auto-create PlanDefinition.description"); 5893 else if (Configuration.doAutoCreate()) 5894 this.description = new MarkdownType(); // bb 5895 return this.description; 5896 } 5897 5898 public boolean hasDescriptionElement() { 5899 return this.description != null && !this.description.isEmpty(); 5900 } 5901 5902 public boolean hasDescription() { 5903 return this.description != null && !this.description.isEmpty(); 5904 } 5905 5906 /** 5907 * @param value {@link #description} (A free text natural language description of the plan definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5908 */ 5909 public PlanDefinition setDescriptionElement(MarkdownType value) { 5910 this.description = value; 5911 return this; 5912 } 5913 5914 /** 5915 * @return A free text natural language description of the plan definition from a consumer's perspective. 5916 */ 5917 public String getDescription() { 5918 return this.description == null ? null : this.description.getValue(); 5919 } 5920 5921 /** 5922 * @param value A free text natural language description of the plan definition from a consumer's perspective. 5923 */ 5924 public PlanDefinition setDescription(String value) { 5925 if (value == null) 5926 this.description = null; 5927 else { 5928 if (this.description == null) 5929 this.description = new MarkdownType(); 5930 this.description.setValue(value); 5931 } 5932 return this; 5933 } 5934 5935 /** 5936 * @return {@link #purpose} (Explaination of why this plan definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5937 */ 5938 public MarkdownType getPurposeElement() { 5939 if (this.purpose == null) 5940 if (Configuration.errorOnAutoCreate()) 5941 throw new Error("Attempt to auto-create PlanDefinition.purpose"); 5942 else if (Configuration.doAutoCreate()) 5943 this.purpose = new MarkdownType(); // bb 5944 return this.purpose; 5945 } 5946 5947 public boolean hasPurposeElement() { 5948 return this.purpose != null && !this.purpose.isEmpty(); 5949 } 5950 5951 public boolean hasPurpose() { 5952 return this.purpose != null && !this.purpose.isEmpty(); 5953 } 5954 5955 /** 5956 * @param value {@link #purpose} (Explaination of why this plan definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5957 */ 5958 public PlanDefinition setPurposeElement(MarkdownType value) { 5959 this.purpose = value; 5960 return this; 5961 } 5962 5963 /** 5964 * @return Explaination of why this plan definition is needed and why it has been designed as it has. 5965 */ 5966 public String getPurpose() { 5967 return this.purpose == null ? null : this.purpose.getValue(); 5968 } 5969 5970 /** 5971 * @param value Explaination of why this plan definition is needed and why it has been designed as it has. 5972 */ 5973 public PlanDefinition setPurpose(String value) { 5974 if (value == null) 5975 this.purpose = null; 5976 else { 5977 if (this.purpose == null) 5978 this.purpose = new MarkdownType(); 5979 this.purpose.setValue(value); 5980 } 5981 return this; 5982 } 5983 5984 /** 5985 * @return {@link #usage} (A detailed description of how the asset is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 5986 */ 5987 public StringType getUsageElement() { 5988 if (this.usage == null) 5989 if (Configuration.errorOnAutoCreate()) 5990 throw new Error("Attempt to auto-create PlanDefinition.usage"); 5991 else if (Configuration.doAutoCreate()) 5992 this.usage = new StringType(); // bb 5993 return this.usage; 5994 } 5995 5996 public boolean hasUsageElement() { 5997 return this.usage != null && !this.usage.isEmpty(); 5998 } 5999 6000 public boolean hasUsage() { 6001 return this.usage != null && !this.usage.isEmpty(); 6002 } 6003 6004 /** 6005 * @param value {@link #usage} (A detailed description of how the asset is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 6006 */ 6007 public PlanDefinition setUsageElement(StringType value) { 6008 this.usage = value; 6009 return this; 6010 } 6011 6012 /** 6013 * @return A detailed description of how the asset is used from a clinical perspective. 6014 */ 6015 public String getUsage() { 6016 return this.usage == null ? null : this.usage.getValue(); 6017 } 6018 6019 /** 6020 * @param value A detailed description of how the asset is used from a clinical perspective. 6021 */ 6022 public PlanDefinition setUsage(String value) { 6023 if (Utilities.noString(value)) 6024 this.usage = null; 6025 else { 6026 if (this.usage == null) 6027 this.usage = new StringType(); 6028 this.usage.setValue(value); 6029 } 6030 return this; 6031 } 6032 6033 /** 6034 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 6035 */ 6036 public DateType getApprovalDateElement() { 6037 if (this.approvalDate == null) 6038 if (Configuration.errorOnAutoCreate()) 6039 throw new Error("Attempt to auto-create PlanDefinition.approvalDate"); 6040 else if (Configuration.doAutoCreate()) 6041 this.approvalDate = new DateType(); // bb 6042 return this.approvalDate; 6043 } 6044 6045 public boolean hasApprovalDateElement() { 6046 return this.approvalDate != null && !this.approvalDate.isEmpty(); 6047 } 6048 6049 public boolean hasApprovalDate() { 6050 return this.approvalDate != null && !this.approvalDate.isEmpty(); 6051 } 6052 6053 /** 6054 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 6055 */ 6056 public PlanDefinition setApprovalDateElement(DateType value) { 6057 this.approvalDate = value; 6058 return this; 6059 } 6060 6061 /** 6062 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 6063 */ 6064 public Date getApprovalDate() { 6065 return this.approvalDate == null ? null : this.approvalDate.getValue(); 6066 } 6067 6068 /** 6069 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 6070 */ 6071 public PlanDefinition setApprovalDate(Date value) { 6072 if (value == null) 6073 this.approvalDate = null; 6074 else { 6075 if (this.approvalDate == null) 6076 this.approvalDate = new DateType(); 6077 this.approvalDate.setValue(value); 6078 } 6079 return this; 6080 } 6081 6082 /** 6083 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 6084 */ 6085 public DateType getLastReviewDateElement() { 6086 if (this.lastReviewDate == null) 6087 if (Configuration.errorOnAutoCreate()) 6088 throw new Error("Attempt to auto-create PlanDefinition.lastReviewDate"); 6089 else if (Configuration.doAutoCreate()) 6090 this.lastReviewDate = new DateType(); // bb 6091 return this.lastReviewDate; 6092 } 6093 6094 public boolean hasLastReviewDateElement() { 6095 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 6096 } 6097 6098 public boolean hasLastReviewDate() { 6099 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 6100 } 6101 6102 /** 6103 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 6104 */ 6105 public PlanDefinition setLastReviewDateElement(DateType value) { 6106 this.lastReviewDate = value; 6107 return this; 6108 } 6109 6110 /** 6111 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 6112 */ 6113 public Date getLastReviewDate() { 6114 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 6115 } 6116 6117 /** 6118 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 6119 */ 6120 public PlanDefinition setLastReviewDate(Date value) { 6121 if (value == null) 6122 this.lastReviewDate = null; 6123 else { 6124 if (this.lastReviewDate == null) 6125 this.lastReviewDate = new DateType(); 6126 this.lastReviewDate.setValue(value); 6127 } 6128 return this; 6129 } 6130 6131 /** 6132 * @return {@link #effectivePeriod} (The period during which the plan definition content was or is planned to be in active use.) 6133 */ 6134 public Period getEffectivePeriod() { 6135 if (this.effectivePeriod == null) 6136 if (Configuration.errorOnAutoCreate()) 6137 throw new Error("Attempt to auto-create PlanDefinition.effectivePeriod"); 6138 else if (Configuration.doAutoCreate()) 6139 this.effectivePeriod = new Period(); // cc 6140 return this.effectivePeriod; 6141 } 6142 6143 public boolean hasEffectivePeriod() { 6144 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 6145 } 6146 6147 /** 6148 * @param value {@link #effectivePeriod} (The period during which the plan definition content was or is planned to be in active use.) 6149 */ 6150 public PlanDefinition setEffectivePeriod(Period value) { 6151 this.effectivePeriod = value; 6152 return this; 6153 } 6154 6155 /** 6156 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate plan definition instances.) 6157 */ 6158 public List<UsageContext> getUseContext() { 6159 if (this.useContext == null) 6160 this.useContext = new ArrayList<UsageContext>(); 6161 return this.useContext; 6162 } 6163 6164 /** 6165 * @return Returns a reference to <code>this</code> for easy method chaining 6166 */ 6167 public PlanDefinition setUseContext(List<UsageContext> theUseContext) { 6168 this.useContext = theUseContext; 6169 return this; 6170 } 6171 6172 public boolean hasUseContext() { 6173 if (this.useContext == null) 6174 return false; 6175 for (UsageContext item : this.useContext) 6176 if (!item.isEmpty()) 6177 return true; 6178 return false; 6179 } 6180 6181 public UsageContext addUseContext() { //3 6182 UsageContext t = new UsageContext(); 6183 if (this.useContext == null) 6184 this.useContext = new ArrayList<UsageContext>(); 6185 this.useContext.add(t); 6186 return t; 6187 } 6188 6189 public PlanDefinition addUseContext(UsageContext t) { //3 6190 if (t == null) 6191 return this; 6192 if (this.useContext == null) 6193 this.useContext = new ArrayList<UsageContext>(); 6194 this.useContext.add(t); 6195 return this; 6196 } 6197 6198 /** 6199 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 6200 */ 6201 public UsageContext getUseContextFirstRep() { 6202 if (getUseContext().isEmpty()) { 6203 addUseContext(); 6204 } 6205 return getUseContext().get(0); 6206 } 6207 6208 /** 6209 * @return {@link #jurisdiction} (A legal or geographic region in which the plan definition is intended to be used.) 6210 */ 6211 public List<CodeableConcept> getJurisdiction() { 6212 if (this.jurisdiction == null) 6213 this.jurisdiction = new ArrayList<CodeableConcept>(); 6214 return this.jurisdiction; 6215 } 6216 6217 /** 6218 * @return Returns a reference to <code>this</code> for easy method chaining 6219 */ 6220 public PlanDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 6221 this.jurisdiction = theJurisdiction; 6222 return this; 6223 } 6224 6225 public boolean hasJurisdiction() { 6226 if (this.jurisdiction == null) 6227 return false; 6228 for (CodeableConcept item : this.jurisdiction) 6229 if (!item.isEmpty()) 6230 return true; 6231 return false; 6232 } 6233 6234 public CodeableConcept addJurisdiction() { //3 6235 CodeableConcept t = new CodeableConcept(); 6236 if (this.jurisdiction == null) 6237 this.jurisdiction = new ArrayList<CodeableConcept>(); 6238 this.jurisdiction.add(t); 6239 return t; 6240 } 6241 6242 public PlanDefinition addJurisdiction(CodeableConcept t) { //3 6243 if (t == null) 6244 return this; 6245 if (this.jurisdiction == null) 6246 this.jurisdiction = new ArrayList<CodeableConcept>(); 6247 this.jurisdiction.add(t); 6248 return this; 6249 } 6250 6251 /** 6252 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 6253 */ 6254 public CodeableConcept getJurisdictionFirstRep() { 6255 if (getJurisdiction().isEmpty()) { 6256 addJurisdiction(); 6257 } 6258 return getJurisdiction().get(0); 6259 } 6260 6261 /** 6262 * @return {@link #topic} (Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.) 6263 */ 6264 public List<CodeableConcept> getTopic() { 6265 if (this.topic == null) 6266 this.topic = new ArrayList<CodeableConcept>(); 6267 return this.topic; 6268 } 6269 6270 /** 6271 * @return Returns a reference to <code>this</code> for easy method chaining 6272 */ 6273 public PlanDefinition setTopic(List<CodeableConcept> theTopic) { 6274 this.topic = theTopic; 6275 return this; 6276 } 6277 6278 public boolean hasTopic() { 6279 if (this.topic == null) 6280 return false; 6281 for (CodeableConcept item : this.topic) 6282 if (!item.isEmpty()) 6283 return true; 6284 return false; 6285 } 6286 6287 public CodeableConcept addTopic() { //3 6288 CodeableConcept t = new CodeableConcept(); 6289 if (this.topic == null) 6290 this.topic = new ArrayList<CodeableConcept>(); 6291 this.topic.add(t); 6292 return t; 6293 } 6294 6295 public PlanDefinition addTopic(CodeableConcept t) { //3 6296 if (t == null) 6297 return this; 6298 if (this.topic == null) 6299 this.topic = new ArrayList<CodeableConcept>(); 6300 this.topic.add(t); 6301 return this; 6302 } 6303 6304 /** 6305 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 6306 */ 6307 public CodeableConcept getTopicFirstRep() { 6308 if (getTopic().isEmpty()) { 6309 addTopic(); 6310 } 6311 return getTopic().get(0); 6312 } 6313 6314 /** 6315 * @return {@link #contributor} (A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.) 6316 */ 6317 public List<Contributor> getContributor() { 6318 if (this.contributor == null) 6319 this.contributor = new ArrayList<Contributor>(); 6320 return this.contributor; 6321 } 6322 6323 /** 6324 * @return Returns a reference to <code>this</code> for easy method chaining 6325 */ 6326 public PlanDefinition setContributor(List<Contributor> theContributor) { 6327 this.contributor = theContributor; 6328 return this; 6329 } 6330 6331 public boolean hasContributor() { 6332 if (this.contributor == null) 6333 return false; 6334 for (Contributor item : this.contributor) 6335 if (!item.isEmpty()) 6336 return true; 6337 return false; 6338 } 6339 6340 public Contributor addContributor() { //3 6341 Contributor t = new Contributor(); 6342 if (this.contributor == null) 6343 this.contributor = new ArrayList<Contributor>(); 6344 this.contributor.add(t); 6345 return t; 6346 } 6347 6348 public PlanDefinition addContributor(Contributor t) { //3 6349 if (t == null) 6350 return this; 6351 if (this.contributor == null) 6352 this.contributor = new ArrayList<Contributor>(); 6353 this.contributor.add(t); 6354 return this; 6355 } 6356 6357 /** 6358 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist 6359 */ 6360 public Contributor getContributorFirstRep() { 6361 if (getContributor().isEmpty()) { 6362 addContributor(); 6363 } 6364 return getContributor().get(0); 6365 } 6366 6367 /** 6368 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 6369 */ 6370 public List<ContactDetail> getContact() { 6371 if (this.contact == null) 6372 this.contact = new ArrayList<ContactDetail>(); 6373 return this.contact; 6374 } 6375 6376 /** 6377 * @return Returns a reference to <code>this</code> for easy method chaining 6378 */ 6379 public PlanDefinition setContact(List<ContactDetail> theContact) { 6380 this.contact = theContact; 6381 return this; 6382 } 6383 6384 public boolean hasContact() { 6385 if (this.contact == null) 6386 return false; 6387 for (ContactDetail item : this.contact) 6388 if (!item.isEmpty()) 6389 return true; 6390 return false; 6391 } 6392 6393 public ContactDetail addContact() { //3 6394 ContactDetail t = new ContactDetail(); 6395 if (this.contact == null) 6396 this.contact = new ArrayList<ContactDetail>(); 6397 this.contact.add(t); 6398 return t; 6399 } 6400 6401 public PlanDefinition addContact(ContactDetail t) { //3 6402 if (t == null) 6403 return this; 6404 if (this.contact == null) 6405 this.contact = new ArrayList<ContactDetail>(); 6406 this.contact.add(t); 6407 return this; 6408 } 6409 6410 /** 6411 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 6412 */ 6413 public ContactDetail getContactFirstRep() { 6414 if (getContact().isEmpty()) { 6415 addContact(); 6416 } 6417 return getContact().get(0); 6418 } 6419 6420 /** 6421 * @return {@link #copyright} (A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6422 */ 6423 public MarkdownType getCopyrightElement() { 6424 if (this.copyright == null) 6425 if (Configuration.errorOnAutoCreate()) 6426 throw new Error("Attempt to auto-create PlanDefinition.copyright"); 6427 else if (Configuration.doAutoCreate()) 6428 this.copyright = new MarkdownType(); // bb 6429 return this.copyright; 6430 } 6431 6432 public boolean hasCopyrightElement() { 6433 return this.copyright != null && !this.copyright.isEmpty(); 6434 } 6435 6436 public boolean hasCopyright() { 6437 return this.copyright != null && !this.copyright.isEmpty(); 6438 } 6439 6440 /** 6441 * @param value {@link #copyright} (A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6442 */ 6443 public PlanDefinition setCopyrightElement(MarkdownType value) { 6444 this.copyright = value; 6445 return this; 6446 } 6447 6448 /** 6449 * @return A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 6450 */ 6451 public String getCopyright() { 6452 return this.copyright == null ? null : this.copyright.getValue(); 6453 } 6454 6455 /** 6456 * @param value A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition. 6457 */ 6458 public PlanDefinition setCopyright(String value) { 6459 if (value == null) 6460 this.copyright = null; 6461 else { 6462 if (this.copyright == null) 6463 this.copyright = new MarkdownType(); 6464 this.copyright.setValue(value); 6465 } 6466 return this; 6467 } 6468 6469 /** 6470 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 6471 */ 6472 public List<RelatedArtifact> getRelatedArtifact() { 6473 if (this.relatedArtifact == null) 6474 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 6475 return this.relatedArtifact; 6476 } 6477 6478 /** 6479 * @return Returns a reference to <code>this</code> for easy method chaining 6480 */ 6481 public PlanDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 6482 this.relatedArtifact = theRelatedArtifact; 6483 return this; 6484 } 6485 6486 public boolean hasRelatedArtifact() { 6487 if (this.relatedArtifact == null) 6488 return false; 6489 for (RelatedArtifact item : this.relatedArtifact) 6490 if (!item.isEmpty()) 6491 return true; 6492 return false; 6493 } 6494 6495 public RelatedArtifact addRelatedArtifact() { //3 6496 RelatedArtifact t = new RelatedArtifact(); 6497 if (this.relatedArtifact == null) 6498 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 6499 this.relatedArtifact.add(t); 6500 return t; 6501 } 6502 6503 public PlanDefinition addRelatedArtifact(RelatedArtifact t) { //3 6504 if (t == null) 6505 return this; 6506 if (this.relatedArtifact == null) 6507 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 6508 this.relatedArtifact.add(t); 6509 return this; 6510 } 6511 6512 /** 6513 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 6514 */ 6515 public RelatedArtifact getRelatedArtifactFirstRep() { 6516 if (getRelatedArtifact().isEmpty()) { 6517 addRelatedArtifact(); 6518 } 6519 return getRelatedArtifact().get(0); 6520 } 6521 6522 /** 6523 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the plan definition.) 6524 */ 6525 public List<Reference> getLibrary() { 6526 if (this.library == null) 6527 this.library = new ArrayList<Reference>(); 6528 return this.library; 6529 } 6530 6531 /** 6532 * @return Returns a reference to <code>this</code> for easy method chaining 6533 */ 6534 public PlanDefinition setLibrary(List<Reference> theLibrary) { 6535 this.library = theLibrary; 6536 return this; 6537 } 6538 6539 public boolean hasLibrary() { 6540 if (this.library == null) 6541 return false; 6542 for (Reference item : this.library) 6543 if (!item.isEmpty()) 6544 return true; 6545 return false; 6546 } 6547 6548 public Reference addLibrary() { //3 6549 Reference t = new Reference(); 6550 if (this.library == null) 6551 this.library = new ArrayList<Reference>(); 6552 this.library.add(t); 6553 return t; 6554 } 6555 6556 public PlanDefinition addLibrary(Reference t) { //3 6557 if (t == null) 6558 return this; 6559 if (this.library == null) 6560 this.library = new ArrayList<Reference>(); 6561 this.library.add(t); 6562 return this; 6563 } 6564 6565 /** 6566 * @return The first repetition of repeating field {@link #library}, creating it if it does not already exist 6567 */ 6568 public Reference getLibraryFirstRep() { 6569 if (getLibrary().isEmpty()) { 6570 addLibrary(); 6571 } 6572 return getLibrary().get(0); 6573 } 6574 6575 /** 6576 * @deprecated Use Reference#setResource(IBaseResource) instead 6577 */ 6578 @Deprecated 6579 public List<Library> getLibraryTarget() { 6580 if (this.libraryTarget == null) 6581 this.libraryTarget = new ArrayList<Library>(); 6582 return this.libraryTarget; 6583 } 6584 6585 /** 6586 * @deprecated Use Reference#setResource(IBaseResource) instead 6587 */ 6588 @Deprecated 6589 public Library addLibraryTarget() { 6590 Library r = new Library(); 6591 if (this.libraryTarget == null) 6592 this.libraryTarget = new ArrayList<Library>(); 6593 this.libraryTarget.add(r); 6594 return r; 6595 } 6596 6597 /** 6598 * @return {@link #goal} (Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.) 6599 */ 6600 public List<PlanDefinitionGoalComponent> getGoal() { 6601 if (this.goal == null) 6602 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 6603 return this.goal; 6604 } 6605 6606 /** 6607 * @return Returns a reference to <code>this</code> for easy method chaining 6608 */ 6609 public PlanDefinition setGoal(List<PlanDefinitionGoalComponent> theGoal) { 6610 this.goal = theGoal; 6611 return this; 6612 } 6613 6614 public boolean hasGoal() { 6615 if (this.goal == null) 6616 return false; 6617 for (PlanDefinitionGoalComponent item : this.goal) 6618 if (!item.isEmpty()) 6619 return true; 6620 return false; 6621 } 6622 6623 public PlanDefinitionGoalComponent addGoal() { //3 6624 PlanDefinitionGoalComponent t = new PlanDefinitionGoalComponent(); 6625 if (this.goal == null) 6626 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 6627 this.goal.add(t); 6628 return t; 6629 } 6630 6631 public PlanDefinition addGoal(PlanDefinitionGoalComponent t) { //3 6632 if (t == null) 6633 return this; 6634 if (this.goal == null) 6635 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 6636 this.goal.add(t); 6637 return this; 6638 } 6639 6640 /** 6641 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist 6642 */ 6643 public PlanDefinitionGoalComponent getGoalFirstRep() { 6644 if (getGoal().isEmpty()) { 6645 addGoal(); 6646 } 6647 return getGoal().get(0); 6648 } 6649 6650 /** 6651 * @return {@link #action} (An action to be taken as part of the plan.) 6652 */ 6653 public List<PlanDefinitionActionComponent> getAction() { 6654 if (this.action == null) 6655 this.action = new ArrayList<PlanDefinitionActionComponent>(); 6656 return this.action; 6657 } 6658 6659 /** 6660 * @return Returns a reference to <code>this</code> for easy method chaining 6661 */ 6662 public PlanDefinition setAction(List<PlanDefinitionActionComponent> theAction) { 6663 this.action = theAction; 6664 return this; 6665 } 6666 6667 public boolean hasAction() { 6668 if (this.action == null) 6669 return false; 6670 for (PlanDefinitionActionComponent item : this.action) 6671 if (!item.isEmpty()) 6672 return true; 6673 return false; 6674 } 6675 6676 public PlanDefinitionActionComponent addAction() { //3 6677 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 6678 if (this.action == null) 6679 this.action = new ArrayList<PlanDefinitionActionComponent>(); 6680 this.action.add(t); 6681 return t; 6682 } 6683 6684 public PlanDefinition addAction(PlanDefinitionActionComponent t) { //3 6685 if (t == null) 6686 return this; 6687 if (this.action == null) 6688 this.action = new ArrayList<PlanDefinitionActionComponent>(); 6689 this.action.add(t); 6690 return this; 6691 } 6692 6693 /** 6694 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 6695 */ 6696 public PlanDefinitionActionComponent getActionFirstRep() { 6697 if (getAction().isEmpty()) { 6698 addAction(); 6699 } 6700 return getAction().get(0); 6701 } 6702 6703 protected void listChildren(List<Property> children) { 6704 super.listChildren(children); 6705 children.add(new Property("url", "uri", "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 6706 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6707 children.add(new Property("version", "string", "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 6708 children.add(new Property("name", "string", "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 6709 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title)); 6710 children.add(new Property("type", "CodeableConcept", "The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule.", 0, 1, type)); 6711 children.add(new Property("status", "code", "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 6712 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 6713 children.add(new Property("date", "dateTime", "The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 0, 1, date)); 6714 children.add(new Property("publisher", "string", "The name of the individual or organization that published the plan definition.", 0, 1, publisher)); 6715 children.add(new Property("description", "markdown", "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, description)); 6716 children.add(new Property("purpose", "markdown", "Explaination of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose)); 6717 children.add(new Property("usage", "string", "A detailed description of how the asset is used from a clinical perspective.", 0, 1, usage)); 6718 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 6719 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 6720 children.add(new Property("effectivePeriod", "Period", "The period during which the plan definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 6721 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate plan definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 6722 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the plan definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 6723 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 6724 children.add(new Property("contributor", "Contributor", "A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor)); 6725 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 6726 children.add(new Property("copyright", "markdown", "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 0, 1, copyright)); 6727 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 6728 children.add(new Property("library", "Reference(Library)", "A reference to a Library resource containing any formal logic used by the plan definition.", 0, java.lang.Integer.MAX_VALUE, library)); 6729 children.add(new Property("goal", "", "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 0, java.lang.Integer.MAX_VALUE, goal)); 6730 children.add(new Property("action", "", "An action to be taken as part of the plan.", 0, java.lang.Integer.MAX_VALUE, action)); 6731 } 6732 6733 @Override 6734 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6735 switch (_hash) { 6736 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this plan definition is (or will be) published. The URL SHOULD include the major version of the plan definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 6737 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 6738 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 6739 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 6740 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title); 6741 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of asset the plan definition represents, e.g. an order set, protocol, or event-condition-action rule.", 0, 1, type); 6742 case -892481550: /*status*/ return new Property("status", "code", "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status); 6743 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 6744 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the plan definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 0, 1, date); 6745 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the plan definition.", 0, 1, publisher); 6746 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, description); 6747 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose); 6748 case 111574433: /*usage*/ return new Property("usage", "string", "A detailed description of how the asset is used from a clinical perspective.", 0, 1, usage); 6749 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 6750 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 6751 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the plan definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 6752 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate plan definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 6753 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the plan definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 6754 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 6755 case -1895276325: /*contributor*/ return new Property("contributor", "Contributor", "A contributor to the content of the asset, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor); 6756 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 6757 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 0, 1, copyright); 6758 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 6759 case 166208699: /*library*/ return new Property("library", "Reference(Library)", "A reference to a Library resource containing any formal logic used by the plan definition.", 0, java.lang.Integer.MAX_VALUE, library); 6760 case 3178259: /*goal*/ return new Property("goal", "", "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 0, java.lang.Integer.MAX_VALUE, goal); 6761 case -1422950858: /*action*/ return new Property("action", "", "An action to be taken as part of the plan.", 0, java.lang.Integer.MAX_VALUE, action); 6762 default: return super.getNamedProperty(_hash, _name, _checkValid); 6763 } 6764 6765 } 6766 6767 @Override 6768 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6769 switch (hash) { 6770 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6771 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6772 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6773 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 6774 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6775 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 6776 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 6777 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 6778 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 6779 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 6780 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6781 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 6782 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 6783 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 6784 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 6785 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 6786 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 6787 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 6788 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 6789 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Contributor 6790 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 6791 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 6792 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 6793 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // Reference 6794 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // PlanDefinitionGoalComponent 6795 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 6796 default: return super.getProperty(hash, name, checkValid); 6797 } 6798 6799 } 6800 6801 @Override 6802 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6803 switch (hash) { 6804 case 116079: // url 6805 this.url = castToUri(value); // UriType 6806 return value; 6807 case -1618432855: // identifier 6808 this.getIdentifier().add(castToIdentifier(value)); // Identifier 6809 return value; 6810 case 351608024: // version 6811 this.version = castToString(value); // StringType 6812 return value; 6813 case 3373707: // name 6814 this.name = castToString(value); // StringType 6815 return value; 6816 case 110371416: // title 6817 this.title = castToString(value); // StringType 6818 return value; 6819 case 3575610: // type 6820 this.type = castToCodeableConcept(value); // CodeableConcept 6821 return value; 6822 case -892481550: // status 6823 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 6824 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6825 return value; 6826 case -404562712: // experimental 6827 this.experimental = castToBoolean(value); // BooleanType 6828 return value; 6829 case 3076014: // date 6830 this.date = castToDateTime(value); // DateTimeType 6831 return value; 6832 case 1447404028: // publisher 6833 this.publisher = castToString(value); // StringType 6834 return value; 6835 case -1724546052: // description 6836 this.description = castToMarkdown(value); // MarkdownType 6837 return value; 6838 case -220463842: // purpose 6839 this.purpose = castToMarkdown(value); // MarkdownType 6840 return value; 6841 case 111574433: // usage 6842 this.usage = castToString(value); // StringType 6843 return value; 6844 case 223539345: // approvalDate 6845 this.approvalDate = castToDate(value); // DateType 6846 return value; 6847 case -1687512484: // lastReviewDate 6848 this.lastReviewDate = castToDate(value); // DateType 6849 return value; 6850 case -403934648: // effectivePeriod 6851 this.effectivePeriod = castToPeriod(value); // Period 6852 return value; 6853 case -669707736: // useContext 6854 this.getUseContext().add(castToUsageContext(value)); // UsageContext 6855 return value; 6856 case -507075711: // jurisdiction 6857 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 6858 return value; 6859 case 110546223: // topic 6860 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 6861 return value; 6862 case -1895276325: // contributor 6863 this.getContributor().add(castToContributor(value)); // Contributor 6864 return value; 6865 case 951526432: // contact 6866 this.getContact().add(castToContactDetail(value)); // ContactDetail 6867 return value; 6868 case 1522889671: // copyright 6869 this.copyright = castToMarkdown(value); // MarkdownType 6870 return value; 6871 case 666807069: // relatedArtifact 6872 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 6873 return value; 6874 case 166208699: // library 6875 this.getLibrary().add(castToReference(value)); // Reference 6876 return value; 6877 case 3178259: // goal 6878 this.getGoal().add((PlanDefinitionGoalComponent) value); // PlanDefinitionGoalComponent 6879 return value; 6880 case -1422950858: // action 6881 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 6882 return value; 6883 default: return super.setProperty(hash, name, value); 6884 } 6885 6886 } 6887 6888 @Override 6889 public Base setProperty(String name, Base value) throws FHIRException { 6890 if (name.equals("url")) { 6891 this.url = castToUri(value); // UriType 6892 } else if (name.equals("identifier")) { 6893 this.getIdentifier().add(castToIdentifier(value)); 6894 } else if (name.equals("version")) { 6895 this.version = castToString(value); // StringType 6896 } else if (name.equals("name")) { 6897 this.name = castToString(value); // StringType 6898 } else if (name.equals("title")) { 6899 this.title = castToString(value); // StringType 6900 } else if (name.equals("type")) { 6901 this.type = castToCodeableConcept(value); // CodeableConcept 6902 } else if (name.equals("status")) { 6903 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 6904 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6905 } else if (name.equals("experimental")) { 6906 this.experimental = castToBoolean(value); // BooleanType 6907 } else if (name.equals("date")) { 6908 this.date = castToDateTime(value); // DateTimeType 6909 } else if (name.equals("publisher")) { 6910 this.publisher = castToString(value); // StringType 6911 } else if (name.equals("description")) { 6912 this.description = castToMarkdown(value); // MarkdownType 6913 } else if (name.equals("purpose")) { 6914 this.purpose = castToMarkdown(value); // MarkdownType 6915 } else if (name.equals("usage")) { 6916 this.usage = castToString(value); // StringType 6917 } else if (name.equals("approvalDate")) { 6918 this.approvalDate = castToDate(value); // DateType 6919 } else if (name.equals("lastReviewDate")) { 6920 this.lastReviewDate = castToDate(value); // DateType 6921 } else if (name.equals("effectivePeriod")) { 6922 this.effectivePeriod = castToPeriod(value); // Period 6923 } else if (name.equals("useContext")) { 6924 this.getUseContext().add(castToUsageContext(value)); 6925 } else if (name.equals("jurisdiction")) { 6926 this.getJurisdiction().add(castToCodeableConcept(value)); 6927 } else if (name.equals("topic")) { 6928 this.getTopic().add(castToCodeableConcept(value)); 6929 } else if (name.equals("contributor")) { 6930 this.getContributor().add(castToContributor(value)); 6931 } else if (name.equals("contact")) { 6932 this.getContact().add(castToContactDetail(value)); 6933 } else if (name.equals("copyright")) { 6934 this.copyright = castToMarkdown(value); // MarkdownType 6935 } else if (name.equals("relatedArtifact")) { 6936 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 6937 } else if (name.equals("library")) { 6938 this.getLibrary().add(castToReference(value)); 6939 } else if (name.equals("goal")) { 6940 this.getGoal().add((PlanDefinitionGoalComponent) value); 6941 } else if (name.equals("action")) { 6942 this.getAction().add((PlanDefinitionActionComponent) value); 6943 } else 6944 return super.setProperty(name, value); 6945 return value; 6946 } 6947 6948 @Override 6949 public Base makeProperty(int hash, String name) throws FHIRException { 6950 switch (hash) { 6951 case 116079: return getUrlElement(); 6952 case -1618432855: return addIdentifier(); 6953 case 351608024: return getVersionElement(); 6954 case 3373707: return getNameElement(); 6955 case 110371416: return getTitleElement(); 6956 case 3575610: return getType(); 6957 case -892481550: return getStatusElement(); 6958 case -404562712: return getExperimentalElement(); 6959 case 3076014: return getDateElement(); 6960 case 1447404028: return getPublisherElement(); 6961 case -1724546052: return getDescriptionElement(); 6962 case -220463842: return getPurposeElement(); 6963 case 111574433: return getUsageElement(); 6964 case 223539345: return getApprovalDateElement(); 6965 case -1687512484: return getLastReviewDateElement(); 6966 case -403934648: return getEffectivePeriod(); 6967 case -669707736: return addUseContext(); 6968 case -507075711: return addJurisdiction(); 6969 case 110546223: return addTopic(); 6970 case -1895276325: return addContributor(); 6971 case 951526432: return addContact(); 6972 case 1522889671: return getCopyrightElement(); 6973 case 666807069: return addRelatedArtifact(); 6974 case 166208699: return addLibrary(); 6975 case 3178259: return addGoal(); 6976 case -1422950858: return addAction(); 6977 default: return super.makeProperty(hash, name); 6978 } 6979 6980 } 6981 6982 @Override 6983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6984 switch (hash) { 6985 case 116079: /*url*/ return new String[] {"uri"}; 6986 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6987 case 351608024: /*version*/ return new String[] {"string"}; 6988 case 3373707: /*name*/ return new String[] {"string"}; 6989 case 110371416: /*title*/ return new String[] {"string"}; 6990 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 6991 case -892481550: /*status*/ return new String[] {"code"}; 6992 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6993 case 3076014: /*date*/ return new String[] {"dateTime"}; 6994 case 1447404028: /*publisher*/ return new String[] {"string"}; 6995 case -1724546052: /*description*/ return new String[] {"markdown"}; 6996 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6997 case 111574433: /*usage*/ return new String[] {"string"}; 6998 case 223539345: /*approvalDate*/ return new String[] {"date"}; 6999 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 7000 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 7001 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 7002 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 7003 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 7004 case -1895276325: /*contributor*/ return new String[] {"Contributor"}; 7005 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 7006 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 7007 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 7008 case 166208699: /*library*/ return new String[] {"Reference"}; 7009 case 3178259: /*goal*/ return new String[] {}; 7010 case -1422950858: /*action*/ return new String[] {}; 7011 default: return super.getTypesForProperty(hash, name); 7012 } 7013 7014 } 7015 7016 @Override 7017 public Base addChild(String name) throws FHIRException { 7018 if (name.equals("url")) { 7019 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.url"); 7020 } 7021 else if (name.equals("identifier")) { 7022 return addIdentifier(); 7023 } 7024 else if (name.equals("version")) { 7025 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.version"); 7026 } 7027 else if (name.equals("name")) { 7028 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.name"); 7029 } 7030 else if (name.equals("title")) { 7031 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 7032 } 7033 else if (name.equals("type")) { 7034 this.type = new CodeableConcept(); 7035 return this.type; 7036 } 7037 else if (name.equals("status")) { 7038 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.status"); 7039 } 7040 else if (name.equals("experimental")) { 7041 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.experimental"); 7042 } 7043 else if (name.equals("date")) { 7044 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.date"); 7045 } 7046 else if (name.equals("publisher")) { 7047 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.publisher"); 7048 } 7049 else if (name.equals("description")) { 7050 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 7051 } 7052 else if (name.equals("purpose")) { 7053 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.purpose"); 7054 } 7055 else if (name.equals("usage")) { 7056 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.usage"); 7057 } 7058 else if (name.equals("approvalDate")) { 7059 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.approvalDate"); 7060 } 7061 else if (name.equals("lastReviewDate")) { 7062 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.lastReviewDate"); 7063 } 7064 else if (name.equals("effectivePeriod")) { 7065 this.effectivePeriod = new Period(); 7066 return this.effectivePeriod; 7067 } 7068 else if (name.equals("useContext")) { 7069 return addUseContext(); 7070 } 7071 else if (name.equals("jurisdiction")) { 7072 return addJurisdiction(); 7073 } 7074 else if (name.equals("topic")) { 7075 return addTopic(); 7076 } 7077 else if (name.equals("contributor")) { 7078 return addContributor(); 7079 } 7080 else if (name.equals("contact")) { 7081 return addContact(); 7082 } 7083 else if (name.equals("copyright")) { 7084 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.copyright"); 7085 } 7086 else if (name.equals("relatedArtifact")) { 7087 return addRelatedArtifact(); 7088 } 7089 else if (name.equals("library")) { 7090 return addLibrary(); 7091 } 7092 else if (name.equals("goal")) { 7093 return addGoal(); 7094 } 7095 else if (name.equals("action")) { 7096 return addAction(); 7097 } 7098 else 7099 return super.addChild(name); 7100 } 7101 7102 public String fhirType() { 7103 return "PlanDefinition"; 7104 7105 } 7106 7107 public PlanDefinition copy() { 7108 PlanDefinition dst = new PlanDefinition(); 7109 copyValues(dst); 7110 dst.url = url == null ? null : url.copy(); 7111 if (identifier != null) { 7112 dst.identifier = new ArrayList<Identifier>(); 7113 for (Identifier i : identifier) 7114 dst.identifier.add(i.copy()); 7115 }; 7116 dst.version = version == null ? null : version.copy(); 7117 dst.name = name == null ? null : name.copy(); 7118 dst.title = title == null ? null : title.copy(); 7119 dst.type = type == null ? null : type.copy(); 7120 dst.status = status == null ? null : status.copy(); 7121 dst.experimental = experimental == null ? null : experimental.copy(); 7122 dst.date = date == null ? null : date.copy(); 7123 dst.publisher = publisher == null ? null : publisher.copy(); 7124 dst.description = description == null ? null : description.copy(); 7125 dst.purpose = purpose == null ? null : purpose.copy(); 7126 dst.usage = usage == null ? null : usage.copy(); 7127 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 7128 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 7129 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 7130 if (useContext != null) { 7131 dst.useContext = new ArrayList<UsageContext>(); 7132 for (UsageContext i : useContext) 7133 dst.useContext.add(i.copy()); 7134 }; 7135 if (jurisdiction != null) { 7136 dst.jurisdiction = new ArrayList<CodeableConcept>(); 7137 for (CodeableConcept i : jurisdiction) 7138 dst.jurisdiction.add(i.copy()); 7139 }; 7140 if (topic != null) { 7141 dst.topic = new ArrayList<CodeableConcept>(); 7142 for (CodeableConcept i : topic) 7143 dst.topic.add(i.copy()); 7144 }; 7145 if (contributor != null) { 7146 dst.contributor = new ArrayList<Contributor>(); 7147 for (Contributor i : contributor) 7148 dst.contributor.add(i.copy()); 7149 }; 7150 if (contact != null) { 7151 dst.contact = new ArrayList<ContactDetail>(); 7152 for (ContactDetail i : contact) 7153 dst.contact.add(i.copy()); 7154 }; 7155 dst.copyright = copyright == null ? null : copyright.copy(); 7156 if (relatedArtifact != null) { 7157 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 7158 for (RelatedArtifact i : relatedArtifact) 7159 dst.relatedArtifact.add(i.copy()); 7160 }; 7161 if (library != null) { 7162 dst.library = new ArrayList<Reference>(); 7163 for (Reference i : library) 7164 dst.library.add(i.copy()); 7165 }; 7166 if (goal != null) { 7167 dst.goal = new ArrayList<PlanDefinitionGoalComponent>(); 7168 for (PlanDefinitionGoalComponent i : goal) 7169 dst.goal.add(i.copy()); 7170 }; 7171 if (action != null) { 7172 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 7173 for (PlanDefinitionActionComponent i : action) 7174 dst.action.add(i.copy()); 7175 }; 7176 return dst; 7177 } 7178 7179 protected PlanDefinition typedCopy() { 7180 return copy(); 7181 } 7182 7183 @Override 7184 public boolean equalsDeep(Base other_) { 7185 if (!super.equalsDeep(other_)) 7186 return false; 7187 if (!(other_ instanceof PlanDefinition)) 7188 return false; 7189 PlanDefinition o = (PlanDefinition) other_; 7190 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(purpose, o.purpose, true) 7191 && compareDeep(usage, o.usage, true) && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 7192 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(contributor, o.contributor, true) 7193 && compareDeep(copyright, o.copyright, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 7194 && compareDeep(library, o.library, true) && compareDeep(goal, o.goal, true) && compareDeep(action, o.action, true) 7195 ; 7196 } 7197 7198 @Override 7199 public boolean equalsShallow(Base other_) { 7200 if (!super.equalsShallow(other_)) 7201 return false; 7202 if (!(other_ instanceof PlanDefinition)) 7203 return false; 7204 PlanDefinition o = (PlanDefinition) other_; 7205 return compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) && compareValues(approvalDate, o.approvalDate, true) 7206 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 7207 ; 7208 } 7209 7210 public boolean isEmpty() { 7211 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, purpose 7212 , usage, approvalDate, lastReviewDate, effectivePeriod, topic, contributor, copyright 7213 , relatedArtifact, library, goal, action); 7214 } 7215 7216 @Override 7217 public ResourceType getResourceType() { 7218 return ResourceType.PlanDefinition; 7219 } 7220 7221 /** 7222 * Search parameter: <b>date</b> 7223 * <p> 7224 * Description: <b>The plan definition publication date</b><br> 7225 * Type: <b>date</b><br> 7226 * Path: <b>PlanDefinition.date</b><br> 7227 * </p> 7228 */ 7229 @SearchParamDefinition(name="date", path="PlanDefinition.date", description="The plan definition publication date", type="date" ) 7230 public static final String SP_DATE = "date"; 7231 /** 7232 * <b>Fluent Client</b> search parameter constant for <b>date</b> 7233 * <p> 7234 * Description: <b>The plan definition publication date</b><br> 7235 * Type: <b>date</b><br> 7236 * Path: <b>PlanDefinition.date</b><br> 7237 * </p> 7238 */ 7239 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 7240 7241 /** 7242 * Search parameter: <b>identifier</b> 7243 * <p> 7244 * Description: <b>External identifier for the plan definition</b><br> 7245 * Type: <b>token</b><br> 7246 * Path: <b>PlanDefinition.identifier</b><br> 7247 * </p> 7248 */ 7249 @SearchParamDefinition(name="identifier", path="PlanDefinition.identifier", description="External identifier for the plan definition", type="token" ) 7250 public static final String SP_IDENTIFIER = "identifier"; 7251 /** 7252 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7253 * <p> 7254 * Description: <b>External identifier for the plan definition</b><br> 7255 * Type: <b>token</b><br> 7256 * Path: <b>PlanDefinition.identifier</b><br> 7257 * </p> 7258 */ 7259 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7260 7261 /** 7262 * Search parameter: <b>successor</b> 7263 * <p> 7264 * Description: <b>What resource is being referenced</b><br> 7265 * Type: <b>reference</b><br> 7266 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7267 * </p> 7268 */ 7269 @SearchParamDefinition(name="successor", path="PlanDefinition.relatedArtifact.where(type='successor').resource", description="What resource is being referenced", type="reference" ) 7270 public static final String SP_SUCCESSOR = "successor"; 7271 /** 7272 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 7273 * <p> 7274 * Description: <b>What resource is being referenced</b><br> 7275 * Type: <b>reference</b><br> 7276 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7277 * </p> 7278 */ 7279 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 7280 7281/** 7282 * Constant for fluent queries to be used to add include statements. Specifies 7283 * the path value of "<b>PlanDefinition:successor</b>". 7284 */ 7285 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("PlanDefinition:successor").toLocked(); 7286 7287 /** 7288 * Search parameter: <b>jurisdiction</b> 7289 * <p> 7290 * Description: <b>Intended jurisdiction for the plan definition</b><br> 7291 * Type: <b>token</b><br> 7292 * Path: <b>PlanDefinition.jurisdiction</b><br> 7293 * </p> 7294 */ 7295 @SearchParamDefinition(name="jurisdiction", path="PlanDefinition.jurisdiction", description="Intended jurisdiction for the plan definition", type="token" ) 7296 public static final String SP_JURISDICTION = "jurisdiction"; 7297 /** 7298 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 7299 * <p> 7300 * Description: <b>Intended jurisdiction for the plan definition</b><br> 7301 * Type: <b>token</b><br> 7302 * Path: <b>PlanDefinition.jurisdiction</b><br> 7303 * </p> 7304 */ 7305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 7306 7307 /** 7308 * Search parameter: <b>description</b> 7309 * <p> 7310 * Description: <b>The description of the plan definition</b><br> 7311 * Type: <b>string</b><br> 7312 * Path: <b>PlanDefinition.description</b><br> 7313 * </p> 7314 */ 7315 @SearchParamDefinition(name="description", path="PlanDefinition.description", description="The description of the plan definition", type="string" ) 7316 public static final String SP_DESCRIPTION = "description"; 7317 /** 7318 * <b>Fluent Client</b> search parameter constant for <b>description</b> 7319 * <p> 7320 * Description: <b>The description of the plan definition</b><br> 7321 * Type: <b>string</b><br> 7322 * Path: <b>PlanDefinition.description</b><br> 7323 * </p> 7324 */ 7325 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 7326 7327 /** 7328 * Search parameter: <b>derived-from</b> 7329 * <p> 7330 * Description: <b>What resource is being referenced</b><br> 7331 * Type: <b>reference</b><br> 7332 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7333 * </p> 7334 */ 7335 @SearchParamDefinition(name="derived-from", path="PlanDefinition.relatedArtifact.where(type='derived-from').resource", description="What resource is being referenced", type="reference" ) 7336 public static final String SP_DERIVED_FROM = "derived-from"; 7337 /** 7338 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 7339 * <p> 7340 * Description: <b>What resource is being referenced</b><br> 7341 * Type: <b>reference</b><br> 7342 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7343 * </p> 7344 */ 7345 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 7346 7347/** 7348 * Constant for fluent queries to be used to add include statements. Specifies 7349 * the path value of "<b>PlanDefinition:derived-from</b>". 7350 */ 7351 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("PlanDefinition:derived-from").toLocked(); 7352 7353 /** 7354 * Search parameter: <b>predecessor</b> 7355 * <p> 7356 * Description: <b>What resource is being referenced</b><br> 7357 * Type: <b>reference</b><br> 7358 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7359 * </p> 7360 */ 7361 @SearchParamDefinition(name="predecessor", path="PlanDefinition.relatedArtifact.where(type='predecessor').resource", description="What resource is being referenced", type="reference" ) 7362 public static final String SP_PREDECESSOR = "predecessor"; 7363 /** 7364 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 7365 * <p> 7366 * Description: <b>What resource is being referenced</b><br> 7367 * Type: <b>reference</b><br> 7368 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7369 * </p> 7370 */ 7371 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 7372 7373/** 7374 * Constant for fluent queries to be used to add include statements. Specifies 7375 * the path value of "<b>PlanDefinition:predecessor</b>". 7376 */ 7377 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("PlanDefinition:predecessor").toLocked(); 7378 7379 /** 7380 * Search parameter: <b>title</b> 7381 * <p> 7382 * Description: <b>The human-friendly name of the plan definition</b><br> 7383 * Type: <b>string</b><br> 7384 * Path: <b>PlanDefinition.title</b><br> 7385 * </p> 7386 */ 7387 @SearchParamDefinition(name="title", path="PlanDefinition.title", description="The human-friendly name of the plan definition", type="string" ) 7388 public static final String SP_TITLE = "title"; 7389 /** 7390 * <b>Fluent Client</b> search parameter constant for <b>title</b> 7391 * <p> 7392 * Description: <b>The human-friendly name of the plan definition</b><br> 7393 * Type: <b>string</b><br> 7394 * Path: <b>PlanDefinition.title</b><br> 7395 * </p> 7396 */ 7397 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 7398 7399 /** 7400 * Search parameter: <b>composed-of</b> 7401 * <p> 7402 * Description: <b>What resource is being referenced</b><br> 7403 * Type: <b>reference</b><br> 7404 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7405 * </p> 7406 */ 7407 @SearchParamDefinition(name="composed-of", path="PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="What resource is being referenced", type="reference" ) 7408 public static final String SP_COMPOSED_OF = "composed-of"; 7409 /** 7410 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 7411 * <p> 7412 * Description: <b>What resource is being referenced</b><br> 7413 * Type: <b>reference</b><br> 7414 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 7415 * </p> 7416 */ 7417 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 7418 7419/** 7420 * Constant for fluent queries to be used to add include statements. Specifies 7421 * the path value of "<b>PlanDefinition:composed-of</b>". 7422 */ 7423 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("PlanDefinition:composed-of").toLocked(); 7424 7425 /** 7426 * Search parameter: <b>version</b> 7427 * <p> 7428 * Description: <b>The business version of the plan definition</b><br> 7429 * Type: <b>token</b><br> 7430 * Path: <b>PlanDefinition.version</b><br> 7431 * </p> 7432 */ 7433 @SearchParamDefinition(name="version", path="PlanDefinition.version", description="The business version of the plan definition", type="token" ) 7434 public static final String SP_VERSION = "version"; 7435 /** 7436 * <b>Fluent Client</b> search parameter constant for <b>version</b> 7437 * <p> 7438 * Description: <b>The business version of the plan definition</b><br> 7439 * Type: <b>token</b><br> 7440 * Path: <b>PlanDefinition.version</b><br> 7441 * </p> 7442 */ 7443 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 7444 7445 /** 7446 * Search parameter: <b>url</b> 7447 * <p> 7448 * Description: <b>The uri that identifies the plan definition</b><br> 7449 * Type: <b>uri</b><br> 7450 * Path: <b>PlanDefinition.url</b><br> 7451 * </p> 7452 */ 7453 @SearchParamDefinition(name="url", path="PlanDefinition.url", description="The uri that identifies the plan definition", type="uri" ) 7454 public static final String SP_URL = "url"; 7455 /** 7456 * <b>Fluent Client</b> search parameter constant for <b>url</b> 7457 * <p> 7458 * Description: <b>The uri that identifies the plan definition</b><br> 7459 * Type: <b>uri</b><br> 7460 * Path: <b>PlanDefinition.url</b><br> 7461 * </p> 7462 */ 7463 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 7464 7465 /** 7466 * Search parameter: <b>effective</b> 7467 * <p> 7468 * Description: <b>The time during which the plan definition is intended to be in use</b><br> 7469 * Type: <b>date</b><br> 7470 * Path: <b>PlanDefinition.effectivePeriod</b><br> 7471 * </p> 7472 */ 7473 @SearchParamDefinition(name="effective", path="PlanDefinition.effectivePeriod", description="The time during which the plan definition is intended to be in use", type="date" ) 7474 public static final String SP_EFFECTIVE = "effective"; 7475 /** 7476 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 7477 * <p> 7478 * Description: <b>The time during which the plan definition is intended to be in use</b><br> 7479 * Type: <b>date</b><br> 7480 * Path: <b>PlanDefinition.effectivePeriod</b><br> 7481 * </p> 7482 */ 7483 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 7484 7485 /** 7486 * Search parameter: <b>depends-on</b> 7487 * <p> 7488 * Description: <b>What resource is being referenced</b><br> 7489 * Type: <b>reference</b><br> 7490 * Path: <b>PlanDefinition.relatedArtifact.resource, PlanDefinition.library</b><br> 7491 * </p> 7492 */ 7493 @SearchParamDefinition(name="depends-on", path="PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="What resource is being referenced", type="reference" ) 7494 public static final String SP_DEPENDS_ON = "depends-on"; 7495 /** 7496 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 7497 * <p> 7498 * Description: <b>What resource is being referenced</b><br> 7499 * Type: <b>reference</b><br> 7500 * Path: <b>PlanDefinition.relatedArtifact.resource, PlanDefinition.library</b><br> 7501 * </p> 7502 */ 7503 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 7504 7505/** 7506 * Constant for fluent queries to be used to add include statements. Specifies 7507 * the path value of "<b>PlanDefinition:depends-on</b>". 7508 */ 7509 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("PlanDefinition:depends-on").toLocked(); 7510 7511 /** 7512 * Search parameter: <b>name</b> 7513 * <p> 7514 * Description: <b>Computationally friendly name of the plan definition</b><br> 7515 * Type: <b>string</b><br> 7516 * Path: <b>PlanDefinition.name</b><br> 7517 * </p> 7518 */ 7519 @SearchParamDefinition(name="name", path="PlanDefinition.name", description="Computationally friendly name of the plan definition", type="string" ) 7520 public static final String SP_NAME = "name"; 7521 /** 7522 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7523 * <p> 7524 * Description: <b>Computationally friendly name of the plan definition</b><br> 7525 * Type: <b>string</b><br> 7526 * Path: <b>PlanDefinition.name</b><br> 7527 * </p> 7528 */ 7529 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 7530 7531 /** 7532 * Search parameter: <b>publisher</b> 7533 * <p> 7534 * Description: <b>Name of the publisher of the plan definition</b><br> 7535 * Type: <b>string</b><br> 7536 * Path: <b>PlanDefinition.publisher</b><br> 7537 * </p> 7538 */ 7539 @SearchParamDefinition(name="publisher", path="PlanDefinition.publisher", description="Name of the publisher of the plan definition", type="string" ) 7540 public static final String SP_PUBLISHER = "publisher"; 7541 /** 7542 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 7543 * <p> 7544 * Description: <b>Name of the publisher of the plan definition</b><br> 7545 * Type: <b>string</b><br> 7546 * Path: <b>PlanDefinition.publisher</b><br> 7547 * </p> 7548 */ 7549 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 7550 7551 /** 7552 * Search parameter: <b>topic</b> 7553 * <p> 7554 * Description: <b>Topics associated with the module</b><br> 7555 * Type: <b>token</b><br> 7556 * Path: <b>PlanDefinition.topic</b><br> 7557 * </p> 7558 */ 7559 @SearchParamDefinition(name="topic", path="PlanDefinition.topic", description="Topics associated with the module", type="token" ) 7560 public static final String SP_TOPIC = "topic"; 7561 /** 7562 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 7563 * <p> 7564 * Description: <b>Topics associated with the module</b><br> 7565 * Type: <b>token</b><br> 7566 * Path: <b>PlanDefinition.topic</b><br> 7567 * </p> 7568 */ 7569 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 7570 7571 /** 7572 * Search parameter: <b>status</b> 7573 * <p> 7574 * Description: <b>The current status of the plan definition</b><br> 7575 * Type: <b>token</b><br> 7576 * Path: <b>PlanDefinition.status</b><br> 7577 * </p> 7578 */ 7579 @SearchParamDefinition(name="status", path="PlanDefinition.status", description="The current status of the plan definition", type="token" ) 7580 public static final String SP_STATUS = "status"; 7581 /** 7582 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7583 * <p> 7584 * Description: <b>The current status of the plan definition</b><br> 7585 * Type: <b>token</b><br> 7586 * Path: <b>PlanDefinition.status</b><br> 7587 * </p> 7588 */ 7589 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7590 7591 7592}