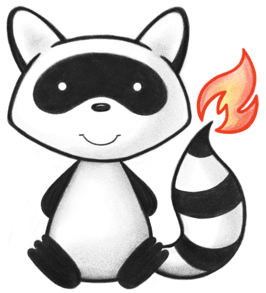
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A person who is directly or indirectly involved in the provisioning of healthcare. 051 */ 052@ResourceDef(name="Practitioner", profile="http://hl7.org/fhir/Profile/Practitioner") 053public class Practitioner extends DomainResource { 054 055 @Block() 056 public static class PractitionerQualificationComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * An identifier that applies to this person's qualification in this role. 059 */ 060 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 061 @Description(shortDefinition="An identifier for this qualification for the practitioner", formalDefinition="An identifier that applies to this person's qualification in this role." ) 062 protected List<Identifier> identifier; 063 064 /** 065 * Coded representation of the qualification. 066 */ 067 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 068 @Description(shortDefinition="Coded representation of the qualification", formalDefinition="Coded representation of the qualification." ) 069 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-2.7-0360") 070 protected CodeableConcept code; 071 072 /** 073 * Period during which the qualification is valid. 074 */ 075 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="Period during which the qualification is valid", formalDefinition="Period during which the qualification is valid." ) 077 protected Period period; 078 079 /** 080 * Organization that regulates and issues the qualification. 081 */ 082 @Child(name = "issuer", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 083 @Description(shortDefinition="Organization that regulates and issues the qualification", formalDefinition="Organization that regulates and issues the qualification." ) 084 protected Reference issuer; 085 086 /** 087 * The actual object that is the target of the reference (Organization that regulates and issues the qualification.) 088 */ 089 protected Organization issuerTarget; 090 091 private static final long serialVersionUID = 1095219071L; 092 093 /** 094 * Constructor 095 */ 096 public PractitionerQualificationComponent() { 097 super(); 098 } 099 100 /** 101 * Constructor 102 */ 103 public PractitionerQualificationComponent(CodeableConcept code) { 104 super(); 105 this.code = code; 106 } 107 108 /** 109 * @return {@link #identifier} (An identifier that applies to this person's qualification in this role.) 110 */ 111 public List<Identifier> getIdentifier() { 112 if (this.identifier == null) 113 this.identifier = new ArrayList<Identifier>(); 114 return this.identifier; 115 } 116 117 /** 118 * @return Returns a reference to <code>this</code> for easy method chaining 119 */ 120 public PractitionerQualificationComponent setIdentifier(List<Identifier> theIdentifier) { 121 this.identifier = theIdentifier; 122 return this; 123 } 124 125 public boolean hasIdentifier() { 126 if (this.identifier == null) 127 return false; 128 for (Identifier item : this.identifier) 129 if (!item.isEmpty()) 130 return true; 131 return false; 132 } 133 134 public Identifier addIdentifier() { //3 135 Identifier t = new Identifier(); 136 if (this.identifier == null) 137 this.identifier = new ArrayList<Identifier>(); 138 this.identifier.add(t); 139 return t; 140 } 141 142 public PractitionerQualificationComponent addIdentifier(Identifier t) { //3 143 if (t == null) 144 return this; 145 if (this.identifier == null) 146 this.identifier = new ArrayList<Identifier>(); 147 this.identifier.add(t); 148 return this; 149 } 150 151 /** 152 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 153 */ 154 public Identifier getIdentifierFirstRep() { 155 if (getIdentifier().isEmpty()) { 156 addIdentifier(); 157 } 158 return getIdentifier().get(0); 159 } 160 161 /** 162 * @return {@link #code} (Coded representation of the qualification.) 163 */ 164 public CodeableConcept getCode() { 165 if (this.code == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create PractitionerQualificationComponent.code"); 168 else if (Configuration.doAutoCreate()) 169 this.code = new CodeableConcept(); // cc 170 return this.code; 171 } 172 173 public boolean hasCode() { 174 return this.code != null && !this.code.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #code} (Coded representation of the qualification.) 179 */ 180 public PractitionerQualificationComponent setCode(CodeableConcept value) { 181 this.code = value; 182 return this; 183 } 184 185 /** 186 * @return {@link #period} (Period during which the qualification is valid.) 187 */ 188 public Period getPeriod() { 189 if (this.period == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create PractitionerQualificationComponent.period"); 192 else if (Configuration.doAutoCreate()) 193 this.period = new Period(); // cc 194 return this.period; 195 } 196 197 public boolean hasPeriod() { 198 return this.period != null && !this.period.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #period} (Period during which the qualification is valid.) 203 */ 204 public PractitionerQualificationComponent setPeriod(Period value) { 205 this.period = value; 206 return this; 207 } 208 209 /** 210 * @return {@link #issuer} (Organization that regulates and issues the qualification.) 211 */ 212 public Reference getIssuer() { 213 if (this.issuer == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 216 else if (Configuration.doAutoCreate()) 217 this.issuer = new Reference(); // cc 218 return this.issuer; 219 } 220 221 public boolean hasIssuer() { 222 return this.issuer != null && !this.issuer.isEmpty(); 223 } 224 225 /** 226 * @param value {@link #issuer} (Organization that regulates and issues the qualification.) 227 */ 228 public PractitionerQualificationComponent setIssuer(Reference value) { 229 this.issuer = value; 230 return this; 231 } 232 233 /** 234 * @return {@link #issuer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Organization that regulates and issues the qualification.) 235 */ 236 public Organization getIssuerTarget() { 237 if (this.issuerTarget == null) 238 if (Configuration.errorOnAutoCreate()) 239 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 240 else if (Configuration.doAutoCreate()) 241 this.issuerTarget = new Organization(); // aa 242 return this.issuerTarget; 243 } 244 245 /** 246 * @param value {@link #issuer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Organization that regulates and issues the qualification.) 247 */ 248 public PractitionerQualificationComponent setIssuerTarget(Organization value) { 249 this.issuerTarget = value; 250 return this; 251 } 252 253 protected void listChildren(List<Property> children) { 254 super.listChildren(children); 255 children.add(new Property("identifier", "Identifier", "An identifier that applies to this person's qualification in this role.", 0, java.lang.Integer.MAX_VALUE, identifier)); 256 children.add(new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code)); 257 children.add(new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period)); 258 children.add(new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer)); 259 } 260 261 @Override 262 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 263 switch (_hash) { 264 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier that applies to this person's qualification in this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 265 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code); 266 case -991726143: /*period*/ return new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period); 267 case -1179159879: /*issuer*/ return new Property("issuer", "Reference(Organization)", "Organization that regulates and issues the qualification.", 0, 1, issuer); 268 default: return super.getNamedProperty(_hash, _name, _checkValid); 269 } 270 271 } 272 273 @Override 274 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 275 switch (hash) { 276 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 277 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 278 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 279 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // Reference 280 default: return super.getProperty(hash, name, checkValid); 281 } 282 283 } 284 285 @Override 286 public Base setProperty(int hash, String name, Base value) throws FHIRException { 287 switch (hash) { 288 case -1618432855: // identifier 289 this.getIdentifier().add(castToIdentifier(value)); // Identifier 290 return value; 291 case 3059181: // code 292 this.code = castToCodeableConcept(value); // CodeableConcept 293 return value; 294 case -991726143: // period 295 this.period = castToPeriod(value); // Period 296 return value; 297 case -1179159879: // issuer 298 this.issuer = castToReference(value); // Reference 299 return value; 300 default: return super.setProperty(hash, name, value); 301 } 302 303 } 304 305 @Override 306 public Base setProperty(String name, Base value) throws FHIRException { 307 if (name.equals("identifier")) { 308 this.getIdentifier().add(castToIdentifier(value)); 309 } else if (name.equals("code")) { 310 this.code = castToCodeableConcept(value); // CodeableConcept 311 } else if (name.equals("period")) { 312 this.period = castToPeriod(value); // Period 313 } else if (name.equals("issuer")) { 314 this.issuer = castToReference(value); // Reference 315 } else 316 return super.setProperty(name, value); 317 return value; 318 } 319 320 @Override 321 public Base makeProperty(int hash, String name) throws FHIRException { 322 switch (hash) { 323 case -1618432855: return addIdentifier(); 324 case 3059181: return getCode(); 325 case -991726143: return getPeriod(); 326 case -1179159879: return getIssuer(); 327 default: return super.makeProperty(hash, name); 328 } 329 330 } 331 332 @Override 333 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 334 switch (hash) { 335 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 336 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 337 case -991726143: /*period*/ return new String[] {"Period"}; 338 case -1179159879: /*issuer*/ return new String[] {"Reference"}; 339 default: return super.getTypesForProperty(hash, name); 340 } 341 342 } 343 344 @Override 345 public Base addChild(String name) throws FHIRException { 346 if (name.equals("identifier")) { 347 return addIdentifier(); 348 } 349 else if (name.equals("code")) { 350 this.code = new CodeableConcept(); 351 return this.code; 352 } 353 else if (name.equals("period")) { 354 this.period = new Period(); 355 return this.period; 356 } 357 else if (name.equals("issuer")) { 358 this.issuer = new Reference(); 359 return this.issuer; 360 } 361 else 362 return super.addChild(name); 363 } 364 365 public PractitionerQualificationComponent copy() { 366 PractitionerQualificationComponent dst = new PractitionerQualificationComponent(); 367 copyValues(dst); 368 if (identifier != null) { 369 dst.identifier = new ArrayList<Identifier>(); 370 for (Identifier i : identifier) 371 dst.identifier.add(i.copy()); 372 }; 373 dst.code = code == null ? null : code.copy(); 374 dst.period = period == null ? null : period.copy(); 375 dst.issuer = issuer == null ? null : issuer.copy(); 376 return dst; 377 } 378 379 @Override 380 public boolean equalsDeep(Base other_) { 381 if (!super.equalsDeep(other_)) 382 return false; 383 if (!(other_ instanceof PractitionerQualificationComponent)) 384 return false; 385 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 386 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 387 && compareDeep(issuer, o.issuer, true); 388 } 389 390 @Override 391 public boolean equalsShallow(Base other_) { 392 if (!super.equalsShallow(other_)) 393 return false; 394 if (!(other_ instanceof PractitionerQualificationComponent)) 395 return false; 396 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 397 return true; 398 } 399 400 public boolean isEmpty() { 401 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, period 402 , issuer); 403 } 404 405 public String fhirType() { 406 return "Practitioner.qualification"; 407 408 } 409 410 } 411 412 /** 413 * An identifier that applies to this person in this role. 414 */ 415 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 416 @Description(shortDefinition="A identifier for the person as this agent", formalDefinition="An identifier that applies to this person in this role." ) 417 protected List<Identifier> identifier; 418 419 /** 420 * Whether this practitioner's record is in active use. 421 */ 422 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 423 @Description(shortDefinition="Whether this practitioner's record is in active use", formalDefinition="Whether this practitioner's record is in active use." ) 424 protected BooleanType active; 425 426 /** 427 * The name(s) associated with the practitioner. 428 */ 429 @Child(name = "name", type = {HumanName.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 430 @Description(shortDefinition="The name(s) associated with the practitioner", formalDefinition="The name(s) associated with the practitioner." ) 431 protected List<HumanName> name; 432 433 /** 434 * A contact detail for the practitioner, e.g. a telephone number or an email address. 435 */ 436 @Child(name = "telecom", type = {ContactPoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 437 @Description(shortDefinition="A contact detail for the practitioner (that apply to all roles)", formalDefinition="A contact detail for the practitioner, e.g. a telephone number or an email address." ) 438 protected List<ContactPoint> telecom; 439 440 /** 441 * Address(es) of the practitioner that are not role specific (typically home address). 442Work addresses are not typically entered in this property as they are usually role dependent. 443 */ 444 @Child(name = "address", type = {Address.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 445 @Description(shortDefinition="Address(es) of the practitioner that are not role specific (typically home address)", formalDefinition="Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent." ) 446 protected List<Address> address; 447 448 /** 449 * Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 450 */ 451 @Child(name = "gender", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 452 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes." ) 453 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 454 protected Enumeration<AdministrativeGender> gender; 455 456 /** 457 * The date of birth for the practitioner. 458 */ 459 @Child(name = "birthDate", type = {DateType.class}, order=6, min=0, max=1, modifier=false, summary=true) 460 @Description(shortDefinition="The date on which the practitioner was born", formalDefinition="The date of birth for the practitioner." ) 461 protected DateType birthDate; 462 463 /** 464 * Image of the person. 465 */ 466 @Child(name = "photo", type = {Attachment.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 467 @Description(shortDefinition="Image of the person", formalDefinition="Image of the person." ) 468 protected List<Attachment> photo; 469 470 /** 471 * Qualifications obtained by training and certification. 472 */ 473 @Child(name = "qualification", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 474 @Description(shortDefinition="Qualifications obtained by training and certification", formalDefinition="Qualifications obtained by training and certification." ) 475 protected List<PractitionerQualificationComponent> qualification; 476 477 /** 478 * A language the practitioner is able to use in patient communication. 479 */ 480 @Child(name = "communication", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 481 @Description(shortDefinition="A language the practitioner is able to use in patient communication", formalDefinition="A language the practitioner is able to use in patient communication." ) 482 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 483 protected List<CodeableConcept> communication; 484 485 private static final long serialVersionUID = 2128349259L; 486 487 /** 488 * Constructor 489 */ 490 public Practitioner() { 491 super(); 492 } 493 494 /** 495 * @return {@link #identifier} (An identifier that applies to this person in this role.) 496 */ 497 public List<Identifier> getIdentifier() { 498 if (this.identifier == null) 499 this.identifier = new ArrayList<Identifier>(); 500 return this.identifier; 501 } 502 503 /** 504 * @return Returns a reference to <code>this</code> for easy method chaining 505 */ 506 public Practitioner setIdentifier(List<Identifier> theIdentifier) { 507 this.identifier = theIdentifier; 508 return this; 509 } 510 511 public boolean hasIdentifier() { 512 if (this.identifier == null) 513 return false; 514 for (Identifier item : this.identifier) 515 if (!item.isEmpty()) 516 return true; 517 return false; 518 } 519 520 public Identifier addIdentifier() { //3 521 Identifier t = new Identifier(); 522 if (this.identifier == null) 523 this.identifier = new ArrayList<Identifier>(); 524 this.identifier.add(t); 525 return t; 526 } 527 528 public Practitioner addIdentifier(Identifier t) { //3 529 if (t == null) 530 return this; 531 if (this.identifier == null) 532 this.identifier = new ArrayList<Identifier>(); 533 this.identifier.add(t); 534 return this; 535 } 536 537 /** 538 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 539 */ 540 public Identifier getIdentifierFirstRep() { 541 if (getIdentifier().isEmpty()) { 542 addIdentifier(); 543 } 544 return getIdentifier().get(0); 545 } 546 547 /** 548 * @return {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 549 */ 550 public BooleanType getActiveElement() { 551 if (this.active == null) 552 if (Configuration.errorOnAutoCreate()) 553 throw new Error("Attempt to auto-create Practitioner.active"); 554 else if (Configuration.doAutoCreate()) 555 this.active = new BooleanType(); // bb 556 return this.active; 557 } 558 559 public boolean hasActiveElement() { 560 return this.active != null && !this.active.isEmpty(); 561 } 562 563 public boolean hasActive() { 564 return this.active != null && !this.active.isEmpty(); 565 } 566 567 /** 568 * @param value {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 569 */ 570 public Practitioner setActiveElement(BooleanType value) { 571 this.active = value; 572 return this; 573 } 574 575 /** 576 * @return Whether this practitioner's record is in active use. 577 */ 578 public boolean getActive() { 579 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 580 } 581 582 /** 583 * @param value Whether this practitioner's record is in active use. 584 */ 585 public Practitioner setActive(boolean value) { 586 if (this.active == null) 587 this.active = new BooleanType(); 588 this.active.setValue(value); 589 return this; 590 } 591 592 /** 593 * @return {@link #name} (The name(s) associated with the practitioner.) 594 */ 595 public List<HumanName> getName() { 596 if (this.name == null) 597 this.name = new ArrayList<HumanName>(); 598 return this.name; 599 } 600 601 /** 602 * @return Returns a reference to <code>this</code> for easy method chaining 603 */ 604 public Practitioner setName(List<HumanName> theName) { 605 this.name = theName; 606 return this; 607 } 608 609 public boolean hasName() { 610 if (this.name == null) 611 return false; 612 for (HumanName item : this.name) 613 if (!item.isEmpty()) 614 return true; 615 return false; 616 } 617 618 public HumanName addName() { //3 619 HumanName t = new HumanName(); 620 if (this.name == null) 621 this.name = new ArrayList<HumanName>(); 622 this.name.add(t); 623 return t; 624 } 625 626 public Practitioner addName(HumanName t) { //3 627 if (t == null) 628 return this; 629 if (this.name == null) 630 this.name = new ArrayList<HumanName>(); 631 this.name.add(t); 632 return this; 633 } 634 635 /** 636 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist 637 */ 638 public HumanName getNameFirstRep() { 639 if (getName().isEmpty()) { 640 addName(); 641 } 642 return getName().get(0); 643 } 644 645 /** 646 * @return {@link #telecom} (A contact detail for the practitioner, e.g. a telephone number or an email address.) 647 */ 648 public List<ContactPoint> getTelecom() { 649 if (this.telecom == null) 650 this.telecom = new ArrayList<ContactPoint>(); 651 return this.telecom; 652 } 653 654 /** 655 * @return Returns a reference to <code>this</code> for easy method chaining 656 */ 657 public Practitioner setTelecom(List<ContactPoint> theTelecom) { 658 this.telecom = theTelecom; 659 return this; 660 } 661 662 public boolean hasTelecom() { 663 if (this.telecom == null) 664 return false; 665 for (ContactPoint item : this.telecom) 666 if (!item.isEmpty()) 667 return true; 668 return false; 669 } 670 671 public ContactPoint addTelecom() { //3 672 ContactPoint t = new ContactPoint(); 673 if (this.telecom == null) 674 this.telecom = new ArrayList<ContactPoint>(); 675 this.telecom.add(t); 676 return t; 677 } 678 679 public Practitioner addTelecom(ContactPoint t) { //3 680 if (t == null) 681 return this; 682 if (this.telecom == null) 683 this.telecom = new ArrayList<ContactPoint>(); 684 this.telecom.add(t); 685 return this; 686 } 687 688 /** 689 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 690 */ 691 public ContactPoint getTelecomFirstRep() { 692 if (getTelecom().isEmpty()) { 693 addTelecom(); 694 } 695 return getTelecom().get(0); 696 } 697 698 /** 699 * @return {@link #address} (Address(es) of the practitioner that are not role specific (typically home address). 700Work addresses are not typically entered in this property as they are usually role dependent.) 701 */ 702 public List<Address> getAddress() { 703 if (this.address == null) 704 this.address = new ArrayList<Address>(); 705 return this.address; 706 } 707 708 /** 709 * @return Returns a reference to <code>this</code> for easy method chaining 710 */ 711 public Practitioner setAddress(List<Address> theAddress) { 712 this.address = theAddress; 713 return this; 714 } 715 716 public boolean hasAddress() { 717 if (this.address == null) 718 return false; 719 for (Address item : this.address) 720 if (!item.isEmpty()) 721 return true; 722 return false; 723 } 724 725 public Address addAddress() { //3 726 Address t = new Address(); 727 if (this.address == null) 728 this.address = new ArrayList<Address>(); 729 this.address.add(t); 730 return t; 731 } 732 733 public Practitioner addAddress(Address t) { //3 734 if (t == null) 735 return this; 736 if (this.address == null) 737 this.address = new ArrayList<Address>(); 738 this.address.add(t); 739 return this; 740 } 741 742 /** 743 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist 744 */ 745 public Address getAddressFirstRep() { 746 if (getAddress().isEmpty()) { 747 addAddress(); 748 } 749 return getAddress().get(0); 750 } 751 752 /** 753 * @return {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 754 */ 755 public Enumeration<AdministrativeGender> getGenderElement() { 756 if (this.gender == null) 757 if (Configuration.errorOnAutoCreate()) 758 throw new Error("Attempt to auto-create Practitioner.gender"); 759 else if (Configuration.doAutoCreate()) 760 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 761 return this.gender; 762 } 763 764 public boolean hasGenderElement() { 765 return this.gender != null && !this.gender.isEmpty(); 766 } 767 768 public boolean hasGender() { 769 return this.gender != null && !this.gender.isEmpty(); 770 } 771 772 /** 773 * @param value {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 774 */ 775 public Practitioner setGenderElement(Enumeration<AdministrativeGender> value) { 776 this.gender = value; 777 return this; 778 } 779 780 /** 781 * @return Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 782 */ 783 public AdministrativeGender getGender() { 784 return this.gender == null ? null : this.gender.getValue(); 785 } 786 787 /** 788 * @param value Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 789 */ 790 public Practitioner setGender(AdministrativeGender value) { 791 if (value == null) 792 this.gender = null; 793 else { 794 if (this.gender == null) 795 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 796 this.gender.setValue(value); 797 } 798 return this; 799 } 800 801 /** 802 * @return {@link #birthDate} (The date of birth for the practitioner.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 803 */ 804 public DateType getBirthDateElement() { 805 if (this.birthDate == null) 806 if (Configuration.errorOnAutoCreate()) 807 throw new Error("Attempt to auto-create Practitioner.birthDate"); 808 else if (Configuration.doAutoCreate()) 809 this.birthDate = new DateType(); // bb 810 return this.birthDate; 811 } 812 813 public boolean hasBirthDateElement() { 814 return this.birthDate != null && !this.birthDate.isEmpty(); 815 } 816 817 public boolean hasBirthDate() { 818 return this.birthDate != null && !this.birthDate.isEmpty(); 819 } 820 821 /** 822 * @param value {@link #birthDate} (The date of birth for the practitioner.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 823 */ 824 public Practitioner setBirthDateElement(DateType value) { 825 this.birthDate = value; 826 return this; 827 } 828 829 /** 830 * @return The date of birth for the practitioner. 831 */ 832 public Date getBirthDate() { 833 return this.birthDate == null ? null : this.birthDate.getValue(); 834 } 835 836 /** 837 * @param value The date of birth for the practitioner. 838 */ 839 public Practitioner setBirthDate(Date value) { 840 if (value == null) 841 this.birthDate = null; 842 else { 843 if (this.birthDate == null) 844 this.birthDate = new DateType(); 845 this.birthDate.setValue(value); 846 } 847 return this; 848 } 849 850 /** 851 * @return {@link #photo} (Image of the person.) 852 */ 853 public List<Attachment> getPhoto() { 854 if (this.photo == null) 855 this.photo = new ArrayList<Attachment>(); 856 return this.photo; 857 } 858 859 /** 860 * @return Returns a reference to <code>this</code> for easy method chaining 861 */ 862 public Practitioner setPhoto(List<Attachment> thePhoto) { 863 this.photo = thePhoto; 864 return this; 865 } 866 867 public boolean hasPhoto() { 868 if (this.photo == null) 869 return false; 870 for (Attachment item : this.photo) 871 if (!item.isEmpty()) 872 return true; 873 return false; 874 } 875 876 public Attachment addPhoto() { //3 877 Attachment t = new Attachment(); 878 if (this.photo == null) 879 this.photo = new ArrayList<Attachment>(); 880 this.photo.add(t); 881 return t; 882 } 883 884 public Practitioner addPhoto(Attachment t) { //3 885 if (t == null) 886 return this; 887 if (this.photo == null) 888 this.photo = new ArrayList<Attachment>(); 889 this.photo.add(t); 890 return this; 891 } 892 893 /** 894 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist 895 */ 896 public Attachment getPhotoFirstRep() { 897 if (getPhoto().isEmpty()) { 898 addPhoto(); 899 } 900 return getPhoto().get(0); 901 } 902 903 /** 904 * @return {@link #qualification} (Qualifications obtained by training and certification.) 905 */ 906 public List<PractitionerQualificationComponent> getQualification() { 907 if (this.qualification == null) 908 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 909 return this.qualification; 910 } 911 912 /** 913 * @return Returns a reference to <code>this</code> for easy method chaining 914 */ 915 public Practitioner setQualification(List<PractitionerQualificationComponent> theQualification) { 916 this.qualification = theQualification; 917 return this; 918 } 919 920 public boolean hasQualification() { 921 if (this.qualification == null) 922 return false; 923 for (PractitionerQualificationComponent item : this.qualification) 924 if (!item.isEmpty()) 925 return true; 926 return false; 927 } 928 929 public PractitionerQualificationComponent addQualification() { //3 930 PractitionerQualificationComponent t = new PractitionerQualificationComponent(); 931 if (this.qualification == null) 932 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 933 this.qualification.add(t); 934 return t; 935 } 936 937 public Practitioner addQualification(PractitionerQualificationComponent t) { //3 938 if (t == null) 939 return this; 940 if (this.qualification == null) 941 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 942 this.qualification.add(t); 943 return this; 944 } 945 946 /** 947 * @return The first repetition of repeating field {@link #qualification}, creating it if it does not already exist 948 */ 949 public PractitionerQualificationComponent getQualificationFirstRep() { 950 if (getQualification().isEmpty()) { 951 addQualification(); 952 } 953 return getQualification().get(0); 954 } 955 956 /** 957 * @return {@link #communication} (A language the practitioner is able to use in patient communication.) 958 */ 959 public List<CodeableConcept> getCommunication() { 960 if (this.communication == null) 961 this.communication = new ArrayList<CodeableConcept>(); 962 return this.communication; 963 } 964 965 /** 966 * @return Returns a reference to <code>this</code> for easy method chaining 967 */ 968 public Practitioner setCommunication(List<CodeableConcept> theCommunication) { 969 this.communication = theCommunication; 970 return this; 971 } 972 973 public boolean hasCommunication() { 974 if (this.communication == null) 975 return false; 976 for (CodeableConcept item : this.communication) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 public CodeableConcept addCommunication() { //3 983 CodeableConcept t = new CodeableConcept(); 984 if (this.communication == null) 985 this.communication = new ArrayList<CodeableConcept>(); 986 this.communication.add(t); 987 return t; 988 } 989 990 public Practitioner addCommunication(CodeableConcept t) { //3 991 if (t == null) 992 return this; 993 if (this.communication == null) 994 this.communication = new ArrayList<CodeableConcept>(); 995 this.communication.add(t); 996 return this; 997 } 998 999 /** 1000 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist 1001 */ 1002 public CodeableConcept getCommunicationFirstRep() { 1003 if (getCommunication().isEmpty()) { 1004 addCommunication(); 1005 } 1006 return getCommunication().get(0); 1007 } 1008 1009 protected void listChildren(List<Property> children) { 1010 super.listChildren(children); 1011 children.add(new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1012 children.add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active)); 1013 children.add(new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, java.lang.Integer.MAX_VALUE, name)); 1014 children.add(new Property("telecom", "ContactPoint", "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1015 children.add(new Property("address", "Address", "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 0, java.lang.Integer.MAX_VALUE, address)); 1016 children.add(new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 1017 children.add(new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, birthDate)); 1018 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 1019 children.add(new Property("qualification", "", "Qualifications obtained by training and certification.", 0, java.lang.Integer.MAX_VALUE, qualification)); 1020 children.add(new Property("communication", "CodeableConcept", "A language the practitioner is able to use in patient communication.", 0, java.lang.Integer.MAX_VALUE, communication)); 1021 } 1022 1023 @Override 1024 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1025 switch (_hash) { 1026 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 1027 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active); 1028 case 3373707: /*name*/ return new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, java.lang.Integer.MAX_VALUE, name); 1029 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 1030 case -1147692044: /*address*/ return new Property("address", "Address", "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 0, java.lang.Integer.MAX_VALUE, address); 1031 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender); 1032 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, birthDate); 1033 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo); 1034 case -631333393: /*qualification*/ return new Property("qualification", "", "Qualifications obtained by training and certification.", 0, java.lang.Integer.MAX_VALUE, qualification); 1035 case -1035284522: /*communication*/ return new Property("communication", "CodeableConcept", "A language the practitioner is able to use in patient communication.", 0, java.lang.Integer.MAX_VALUE, communication); 1036 default: return super.getNamedProperty(_hash, _name, _checkValid); 1037 } 1038 1039 } 1040 1041 @Override 1042 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1043 switch (hash) { 1044 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1045 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1046 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1047 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1048 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1049 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1050 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 1051 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1052 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : this.qualification.toArray(new Base[this.qualification.size()]); // PractitionerQualificationComponent 1053 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 1054 default: return super.getProperty(hash, name, checkValid); 1055 } 1056 1057 } 1058 1059 @Override 1060 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1061 switch (hash) { 1062 case -1618432855: // identifier 1063 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1064 return value; 1065 case -1422950650: // active 1066 this.active = castToBoolean(value); // BooleanType 1067 return value; 1068 case 3373707: // name 1069 this.getName().add(castToHumanName(value)); // HumanName 1070 return value; 1071 case -1429363305: // telecom 1072 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1073 return value; 1074 case -1147692044: // address 1075 this.getAddress().add(castToAddress(value)); // Address 1076 return value; 1077 case -1249512767: // gender 1078 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1079 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1080 return value; 1081 case -1210031859: // birthDate 1082 this.birthDate = castToDate(value); // DateType 1083 return value; 1084 case 106642994: // photo 1085 this.getPhoto().add(castToAttachment(value)); // Attachment 1086 return value; 1087 case -631333393: // qualification 1088 this.getQualification().add((PractitionerQualificationComponent) value); // PractitionerQualificationComponent 1089 return value; 1090 case -1035284522: // communication 1091 this.getCommunication().add(castToCodeableConcept(value)); // CodeableConcept 1092 return value; 1093 default: return super.setProperty(hash, name, value); 1094 } 1095 1096 } 1097 1098 @Override 1099 public Base setProperty(String name, Base value) throws FHIRException { 1100 if (name.equals("identifier")) { 1101 this.getIdentifier().add(castToIdentifier(value)); 1102 } else if (name.equals("active")) { 1103 this.active = castToBoolean(value); // BooleanType 1104 } else if (name.equals("name")) { 1105 this.getName().add(castToHumanName(value)); 1106 } else if (name.equals("telecom")) { 1107 this.getTelecom().add(castToContactPoint(value)); 1108 } else if (name.equals("address")) { 1109 this.getAddress().add(castToAddress(value)); 1110 } else if (name.equals("gender")) { 1111 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1112 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1113 } else if (name.equals("birthDate")) { 1114 this.birthDate = castToDate(value); // DateType 1115 } else if (name.equals("photo")) { 1116 this.getPhoto().add(castToAttachment(value)); 1117 } else if (name.equals("qualification")) { 1118 this.getQualification().add((PractitionerQualificationComponent) value); 1119 } else if (name.equals("communication")) { 1120 this.getCommunication().add(castToCodeableConcept(value)); 1121 } else 1122 return super.setProperty(name, value); 1123 return value; 1124 } 1125 1126 @Override 1127 public Base makeProperty(int hash, String name) throws FHIRException { 1128 switch (hash) { 1129 case -1618432855: return addIdentifier(); 1130 case -1422950650: return getActiveElement(); 1131 case 3373707: return addName(); 1132 case -1429363305: return addTelecom(); 1133 case -1147692044: return addAddress(); 1134 case -1249512767: return getGenderElement(); 1135 case -1210031859: return getBirthDateElement(); 1136 case 106642994: return addPhoto(); 1137 case -631333393: return addQualification(); 1138 case -1035284522: return addCommunication(); 1139 default: return super.makeProperty(hash, name); 1140 } 1141 1142 } 1143 1144 @Override 1145 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1146 switch (hash) { 1147 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1148 case -1422950650: /*active*/ return new String[] {"boolean"}; 1149 case 3373707: /*name*/ return new String[] {"HumanName"}; 1150 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1151 case -1147692044: /*address*/ return new String[] {"Address"}; 1152 case -1249512767: /*gender*/ return new String[] {"code"}; 1153 case -1210031859: /*birthDate*/ return new String[] {"date"}; 1154 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1155 case -631333393: /*qualification*/ return new String[] {}; 1156 case -1035284522: /*communication*/ return new String[] {"CodeableConcept"}; 1157 default: return super.getTypesForProperty(hash, name); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base addChild(String name) throws FHIRException { 1164 if (name.equals("identifier")) { 1165 return addIdentifier(); 1166 } 1167 else if (name.equals("active")) { 1168 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.active"); 1169 } 1170 else if (name.equals("name")) { 1171 return addName(); 1172 } 1173 else if (name.equals("telecom")) { 1174 return addTelecom(); 1175 } 1176 else if (name.equals("address")) { 1177 return addAddress(); 1178 } 1179 else if (name.equals("gender")) { 1180 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.gender"); 1181 } 1182 else if (name.equals("birthDate")) { 1183 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.birthDate"); 1184 } 1185 else if (name.equals("photo")) { 1186 return addPhoto(); 1187 } 1188 else if (name.equals("qualification")) { 1189 return addQualification(); 1190 } 1191 else if (name.equals("communication")) { 1192 return addCommunication(); 1193 } 1194 else 1195 return super.addChild(name); 1196 } 1197 1198 public String fhirType() { 1199 return "Practitioner"; 1200 1201 } 1202 1203 public Practitioner copy() { 1204 Practitioner dst = new Practitioner(); 1205 copyValues(dst); 1206 if (identifier != null) { 1207 dst.identifier = new ArrayList<Identifier>(); 1208 for (Identifier i : identifier) 1209 dst.identifier.add(i.copy()); 1210 }; 1211 dst.active = active == null ? null : active.copy(); 1212 if (name != null) { 1213 dst.name = new ArrayList<HumanName>(); 1214 for (HumanName i : name) 1215 dst.name.add(i.copy()); 1216 }; 1217 if (telecom != null) { 1218 dst.telecom = new ArrayList<ContactPoint>(); 1219 for (ContactPoint i : telecom) 1220 dst.telecom.add(i.copy()); 1221 }; 1222 if (address != null) { 1223 dst.address = new ArrayList<Address>(); 1224 for (Address i : address) 1225 dst.address.add(i.copy()); 1226 }; 1227 dst.gender = gender == null ? null : gender.copy(); 1228 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1229 if (photo != null) { 1230 dst.photo = new ArrayList<Attachment>(); 1231 for (Attachment i : photo) 1232 dst.photo.add(i.copy()); 1233 }; 1234 if (qualification != null) { 1235 dst.qualification = new ArrayList<PractitionerQualificationComponent>(); 1236 for (PractitionerQualificationComponent i : qualification) 1237 dst.qualification.add(i.copy()); 1238 }; 1239 if (communication != null) { 1240 dst.communication = new ArrayList<CodeableConcept>(); 1241 for (CodeableConcept i : communication) 1242 dst.communication.add(i.copy()); 1243 }; 1244 return dst; 1245 } 1246 1247 protected Practitioner typedCopy() { 1248 return copy(); 1249 } 1250 1251 @Override 1252 public boolean equalsDeep(Base other_) { 1253 if (!super.equalsDeep(other_)) 1254 return false; 1255 if (!(other_ instanceof Practitioner)) 1256 return false; 1257 Practitioner o = (Practitioner) other_; 1258 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(name, o.name, true) 1259 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) && compareDeep(gender, o.gender, true) 1260 && compareDeep(birthDate, o.birthDate, true) && compareDeep(photo, o.photo, true) && compareDeep(qualification, o.qualification, true) 1261 && compareDeep(communication, o.communication, true); 1262 } 1263 1264 @Override 1265 public boolean equalsShallow(Base other_) { 1266 if (!super.equalsShallow(other_)) 1267 return false; 1268 if (!(other_ instanceof Practitioner)) 1269 return false; 1270 Practitioner o = (Practitioner) other_; 1271 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1272 ; 1273 } 1274 1275 public boolean isEmpty() { 1276 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name 1277 , telecom, address, gender, birthDate, photo, qualification, communication); 1278 } 1279 1280 @Override 1281 public ResourceType getResourceType() { 1282 return ResourceType.Practitioner; 1283 } 1284 1285 /** 1286 * Search parameter: <b>identifier</b> 1287 * <p> 1288 * Description: <b>A practitioner's Identifier</b><br> 1289 * Type: <b>token</b><br> 1290 * Path: <b>Practitioner.identifier</b><br> 1291 * </p> 1292 */ 1293 @SearchParamDefinition(name="identifier", path="Practitioner.identifier", description="A practitioner's Identifier", type="token" ) 1294 public static final String SP_IDENTIFIER = "identifier"; 1295 /** 1296 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1297 * <p> 1298 * Description: <b>A practitioner's Identifier</b><br> 1299 * Type: <b>token</b><br> 1300 * Path: <b>Practitioner.identifier</b><br> 1301 * </p> 1302 */ 1303 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1304 1305 /** 1306 * Search parameter: <b>given</b> 1307 * <p> 1308 * Description: <b>A portion of the given name</b><br> 1309 * Type: <b>string</b><br> 1310 * Path: <b>Practitioner.name.given</b><br> 1311 * </p> 1312 */ 1313 @SearchParamDefinition(name="given", path="Practitioner.name.given", description="A portion of the given name", type="string" ) 1314 public static final String SP_GIVEN = "given"; 1315 /** 1316 * <b>Fluent Client</b> search parameter constant for <b>given</b> 1317 * <p> 1318 * Description: <b>A portion of the given name</b><br> 1319 * Type: <b>string</b><br> 1320 * Path: <b>Practitioner.name.given</b><br> 1321 * </p> 1322 */ 1323 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 1324 1325 /** 1326 * Search parameter: <b>address</b> 1327 * <p> 1328 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1329 * Type: <b>string</b><br> 1330 * Path: <b>Practitioner.address</b><br> 1331 * </p> 1332 */ 1333 @SearchParamDefinition(name="address", path="Practitioner.address", description="A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text", type="string" ) 1334 public static final String SP_ADDRESS = "address"; 1335 /** 1336 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1337 * <p> 1338 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 1339 * Type: <b>string</b><br> 1340 * Path: <b>Practitioner.address</b><br> 1341 * </p> 1342 */ 1343 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1344 1345 /** 1346 * Search parameter: <b>address-state</b> 1347 * <p> 1348 * Description: <b>A state specified in an address</b><br> 1349 * Type: <b>string</b><br> 1350 * Path: <b>Practitioner.address.state</b><br> 1351 * </p> 1352 */ 1353 @SearchParamDefinition(name="address-state", path="Practitioner.address.state", description="A state specified in an address", type="string" ) 1354 public static final String SP_ADDRESS_STATE = "address-state"; 1355 /** 1356 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1357 * <p> 1358 * Description: <b>A state specified in an address</b><br> 1359 * Type: <b>string</b><br> 1360 * Path: <b>Practitioner.address.state</b><br> 1361 * </p> 1362 */ 1363 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1364 1365 /** 1366 * Search parameter: <b>gender</b> 1367 * <p> 1368 * Description: <b>Gender of the practitioner</b><br> 1369 * Type: <b>token</b><br> 1370 * Path: <b>Practitioner.gender</b><br> 1371 * </p> 1372 */ 1373 @SearchParamDefinition(name="gender", path="Practitioner.gender", description="Gender of the practitioner", type="token" ) 1374 public static final String SP_GENDER = "gender"; 1375 /** 1376 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1377 * <p> 1378 * Description: <b>Gender of the practitioner</b><br> 1379 * Type: <b>token</b><br> 1380 * Path: <b>Practitioner.gender</b><br> 1381 * </p> 1382 */ 1383 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 1384 1385 /** 1386 * Search parameter: <b>active</b> 1387 * <p> 1388 * Description: <b>Whether the practitioner record is active</b><br> 1389 * Type: <b>token</b><br> 1390 * Path: <b>Practitioner.active</b><br> 1391 * </p> 1392 */ 1393 @SearchParamDefinition(name="active", path="Practitioner.active", description="Whether the practitioner record is active", type="token" ) 1394 public static final String SP_ACTIVE = "active"; 1395 /** 1396 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1397 * <p> 1398 * Description: <b>Whether the practitioner record is active</b><br> 1399 * Type: <b>token</b><br> 1400 * Path: <b>Practitioner.active</b><br> 1401 * </p> 1402 */ 1403 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1404 1405 /** 1406 * Search parameter: <b>address-postalcode</b> 1407 * <p> 1408 * Description: <b>A postalCode specified in an address</b><br> 1409 * Type: <b>string</b><br> 1410 * Path: <b>Practitioner.address.postalCode</b><br> 1411 * </p> 1412 */ 1413 @SearchParamDefinition(name="address-postalcode", path="Practitioner.address.postalCode", description="A postalCode specified in an address", type="string" ) 1414 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1415 /** 1416 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1417 * <p> 1418 * Description: <b>A postalCode specified in an address</b><br> 1419 * Type: <b>string</b><br> 1420 * Path: <b>Practitioner.address.postalCode</b><br> 1421 * </p> 1422 */ 1423 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1424 1425 /** 1426 * Search parameter: <b>address-country</b> 1427 * <p> 1428 * Description: <b>A country specified in an address</b><br> 1429 * Type: <b>string</b><br> 1430 * Path: <b>Practitioner.address.country</b><br> 1431 * </p> 1432 */ 1433 @SearchParamDefinition(name="address-country", path="Practitioner.address.country", description="A country specified in an address", type="string" ) 1434 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1435 /** 1436 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1437 * <p> 1438 * Description: <b>A country specified in an address</b><br> 1439 * Type: <b>string</b><br> 1440 * Path: <b>Practitioner.address.country</b><br> 1441 * </p> 1442 */ 1443 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1444 1445 /** 1446 * Search parameter: <b>phonetic</b> 1447 * <p> 1448 * Description: <b>A portion of either family or given name using some kind of phonetic matching algorithm</b><br> 1449 * Type: <b>string</b><br> 1450 * Path: <b>Practitioner.name</b><br> 1451 * </p> 1452 */ 1453 @SearchParamDefinition(name="phonetic", path="Practitioner.name", description="A portion of either family or given name using some kind of phonetic matching algorithm", type="string" ) 1454 public static final String SP_PHONETIC = "phonetic"; 1455 /** 1456 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1457 * <p> 1458 * Description: <b>A portion of either family or given name using some kind of phonetic matching algorithm</b><br> 1459 * Type: <b>string</b><br> 1460 * Path: <b>Practitioner.name</b><br> 1461 * </p> 1462 */ 1463 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 1464 1465 /** 1466 * Search parameter: <b>phone</b> 1467 * <p> 1468 * Description: <b>A value in a phone contact</b><br> 1469 * Type: <b>token</b><br> 1470 * Path: <b>Practitioner.telecom(system=phone)</b><br> 1471 * </p> 1472 */ 1473 @SearchParamDefinition(name="phone", path="Practitioner.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 1474 public static final String SP_PHONE = "phone"; 1475 /** 1476 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1477 * <p> 1478 * Description: <b>A value in a phone contact</b><br> 1479 * Type: <b>token</b><br> 1480 * Path: <b>Practitioner.telecom(system=phone)</b><br> 1481 * </p> 1482 */ 1483 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 1484 1485 /** 1486 * Search parameter: <b>name</b> 1487 * <p> 1488 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1489 * Type: <b>string</b><br> 1490 * Path: <b>Practitioner.name</b><br> 1491 * </p> 1492 */ 1493 @SearchParamDefinition(name="name", path="Practitioner.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1494 public static final String SP_NAME = "name"; 1495 /** 1496 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1497 * <p> 1498 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1499 * Type: <b>string</b><br> 1500 * Path: <b>Practitioner.name</b><br> 1501 * </p> 1502 */ 1503 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1504 1505 /** 1506 * Search parameter: <b>address-use</b> 1507 * <p> 1508 * Description: <b>A use code specified in an address</b><br> 1509 * Type: <b>token</b><br> 1510 * Path: <b>Practitioner.address.use</b><br> 1511 * </p> 1512 */ 1513 @SearchParamDefinition(name="address-use", path="Practitioner.address.use", description="A use code specified in an address", type="token" ) 1514 public static final String SP_ADDRESS_USE = "address-use"; 1515 /** 1516 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1517 * <p> 1518 * Description: <b>A use code specified in an address</b><br> 1519 * Type: <b>token</b><br> 1520 * Path: <b>Practitioner.address.use</b><br> 1521 * </p> 1522 */ 1523 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1524 1525 /** 1526 * Search parameter: <b>telecom</b> 1527 * <p> 1528 * Description: <b>The value in any kind of contact</b><br> 1529 * Type: <b>token</b><br> 1530 * Path: <b>Practitioner.telecom</b><br> 1531 * </p> 1532 */ 1533 @SearchParamDefinition(name="telecom", path="Practitioner.telecom", description="The value in any kind of contact", type="token" ) 1534 public static final String SP_TELECOM = "telecom"; 1535 /** 1536 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1537 * <p> 1538 * Description: <b>The value in any kind of contact</b><br> 1539 * Type: <b>token</b><br> 1540 * Path: <b>Practitioner.telecom</b><br> 1541 * </p> 1542 */ 1543 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 1544 1545 /** 1546 * Search parameter: <b>family</b> 1547 * <p> 1548 * Description: <b>A portion of the family name</b><br> 1549 * Type: <b>string</b><br> 1550 * Path: <b>Practitioner.name.family</b><br> 1551 * </p> 1552 */ 1553 @SearchParamDefinition(name="family", path="Practitioner.name.family", description="A portion of the family name", type="string" ) 1554 public static final String SP_FAMILY = "family"; 1555 /** 1556 * <b>Fluent Client</b> search parameter constant for <b>family</b> 1557 * <p> 1558 * Description: <b>A portion of the family name</b><br> 1559 * Type: <b>string</b><br> 1560 * Path: <b>Practitioner.name.family</b><br> 1561 * </p> 1562 */ 1563 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 1564 1565 /** 1566 * Search parameter: <b>address-city</b> 1567 * <p> 1568 * Description: <b>A city specified in an address</b><br> 1569 * Type: <b>string</b><br> 1570 * Path: <b>Practitioner.address.city</b><br> 1571 * </p> 1572 */ 1573 @SearchParamDefinition(name="address-city", path="Practitioner.address.city", description="A city specified in an address", type="string" ) 1574 public static final String SP_ADDRESS_CITY = "address-city"; 1575 /** 1576 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1577 * <p> 1578 * Description: <b>A city specified in an address</b><br> 1579 * Type: <b>string</b><br> 1580 * Path: <b>Practitioner.address.city</b><br> 1581 * </p> 1582 */ 1583 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1584 1585 /** 1586 * Search parameter: <b>communication</b> 1587 * <p> 1588 * Description: <b>One of the languages that the practitioner can communicate with</b><br> 1589 * Type: <b>token</b><br> 1590 * Path: <b>Practitioner.communication</b><br> 1591 * </p> 1592 */ 1593 @SearchParamDefinition(name="communication", path="Practitioner.communication", description="One of the languages that the practitioner can communicate with", type="token" ) 1594 public static final String SP_COMMUNICATION = "communication"; 1595 /** 1596 * <b>Fluent Client</b> search parameter constant for <b>communication</b> 1597 * <p> 1598 * Description: <b>One of the languages that the practitioner can communicate with</b><br> 1599 * Type: <b>token</b><br> 1600 * Path: <b>Practitioner.communication</b><br> 1601 * </p> 1602 */ 1603 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMMUNICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMMUNICATION); 1604 1605 /** 1606 * Search parameter: <b>email</b> 1607 * <p> 1608 * Description: <b>A value in an email contact</b><br> 1609 * Type: <b>token</b><br> 1610 * Path: <b>Practitioner.telecom(system=email)</b><br> 1611 * </p> 1612 */ 1613 @SearchParamDefinition(name="email", path="Practitioner.telecom.where(system='email')", description="A value in an email contact", type="token" ) 1614 public static final String SP_EMAIL = "email"; 1615 /** 1616 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1617 * <p> 1618 * Description: <b>A value in an email contact</b><br> 1619 * Type: <b>token</b><br> 1620 * Path: <b>Practitioner.telecom(system=email)</b><br> 1621 * </p> 1622 */ 1623 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1624 1625 1626}