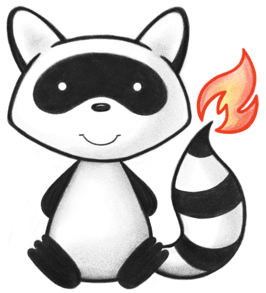
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 050 */ 051@ResourceDef(name="PractitionerRole", profile="http://hl7.org/fhir/Profile/PractitionerRole") 052public class PractitionerRole extends DomainResource { 053 054 public enum DaysOfWeek { 055 /** 056 * Monday 057 */ 058 MON, 059 /** 060 * Tuesday 061 */ 062 TUE, 063 /** 064 * Wednesday 065 */ 066 WED, 067 /** 068 * Thursday 069 */ 070 THU, 071 /** 072 * Friday 073 */ 074 FRI, 075 /** 076 * Saturday 077 */ 078 SAT, 079 /** 080 * Sunday 081 */ 082 SUN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("mon".equals(codeString)) 091 return MON; 092 if ("tue".equals(codeString)) 093 return TUE; 094 if ("wed".equals(codeString)) 095 return WED; 096 if ("thu".equals(codeString)) 097 return THU; 098 if ("fri".equals(codeString)) 099 return FRI; 100 if ("sat".equals(codeString)) 101 return SAT; 102 if ("sun".equals(codeString)) 103 return SUN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case MON: return "mon"; 112 case TUE: return "tue"; 113 case WED: return "wed"; 114 case THU: return "thu"; 115 case FRI: return "fri"; 116 case SAT: return "sat"; 117 case SUN: return "sun"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case MON: return "http://hl7.org/fhir/days-of-week"; 125 case TUE: return "http://hl7.org/fhir/days-of-week"; 126 case WED: return "http://hl7.org/fhir/days-of-week"; 127 case THU: return "http://hl7.org/fhir/days-of-week"; 128 case FRI: return "http://hl7.org/fhir/days-of-week"; 129 case SAT: return "http://hl7.org/fhir/days-of-week"; 130 case SUN: return "http://hl7.org/fhir/days-of-week"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case MON: return "Monday"; 138 case TUE: return "Tuesday"; 139 case WED: return "Wednesday"; 140 case THU: return "Thursday"; 141 case FRI: return "Friday"; 142 case SAT: return "Saturday"; 143 case SUN: return "Sunday"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case MON: return "Monday"; 151 case TUE: return "Tuesday"; 152 case WED: return "Wednesday"; 153 case THU: return "Thursday"; 154 case FRI: return "Friday"; 155 case SAT: return "Saturday"; 156 case SUN: return "Sunday"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 164 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("mon".equals(codeString)) 169 return DaysOfWeek.MON; 170 if ("tue".equals(codeString)) 171 return DaysOfWeek.TUE; 172 if ("wed".equals(codeString)) 173 return DaysOfWeek.WED; 174 if ("thu".equals(codeString)) 175 return DaysOfWeek.THU; 176 if ("fri".equals(codeString)) 177 return DaysOfWeek.FRI; 178 if ("sat".equals(codeString)) 179 return DaysOfWeek.SAT; 180 if ("sun".equals(codeString)) 181 return DaysOfWeek.SUN; 182 throw new IllegalArgumentException("Unknown DaysOfWeek code '"+codeString+"'"); 183 } 184 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<DaysOfWeek>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("mon".equals(codeString)) 193 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON); 194 if ("tue".equals(codeString)) 195 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE); 196 if ("wed".equals(codeString)) 197 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED); 198 if ("thu".equals(codeString)) 199 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU); 200 if ("fri".equals(codeString)) 201 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI); 202 if ("sat".equals(codeString)) 203 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT); 204 if ("sun".equals(codeString)) 205 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN); 206 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 207 } 208 public String toCode(DaysOfWeek code) { 209 if (code == DaysOfWeek.MON) 210 return "mon"; 211 if (code == DaysOfWeek.TUE) 212 return "tue"; 213 if (code == DaysOfWeek.WED) 214 return "wed"; 215 if (code == DaysOfWeek.THU) 216 return "thu"; 217 if (code == DaysOfWeek.FRI) 218 return "fri"; 219 if (code == DaysOfWeek.SAT) 220 return "sat"; 221 if (code == DaysOfWeek.SUN) 222 return "sun"; 223 return "?"; 224 } 225 public String toSystem(DaysOfWeek code) { 226 return code.getSystem(); 227 } 228 } 229 230 @Block() 231 public static class PractitionerRoleAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 232 /** 233 * Indicates which days of the week are available between the start and end Times. 234 */ 235 @Child(name = "daysOfWeek", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 236 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="Indicates which days of the week are available between the start and end Times." ) 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 238 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 239 240 /** 241 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 242 */ 243 @Child(name = "allDay", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 244 @Description(shortDefinition="Always available? e.g. 24 hour service", formalDefinition="Is this always available? (hence times are irrelevant) e.g. 24 hour service." ) 245 protected BooleanType allDay; 246 247 /** 248 * The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 249 */ 250 @Child(name = "availableStartTime", type = {TimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 251 @Description(shortDefinition="Opening time of day (ignored if allDay = true)", formalDefinition="The opening time of day. Note: If the AllDay flag is set, then this time is ignored." ) 252 protected TimeType availableStartTime; 253 254 /** 255 * The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 256 */ 257 @Child(name = "availableEndTime", type = {TimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 258 @Description(shortDefinition="Closing time of day (ignored if allDay = true)", formalDefinition="The closing time of day. Note: If the AllDay flag is set, then this time is ignored." ) 259 protected TimeType availableEndTime; 260 261 private static final long serialVersionUID = -2139510127L; 262 263 /** 264 * Constructor 265 */ 266 public PractitionerRoleAvailableTimeComponent() { 267 super(); 268 } 269 270 /** 271 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 272 */ 273 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 274 if (this.daysOfWeek == null) 275 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 276 return this.daysOfWeek; 277 } 278 279 /** 280 * @return Returns a reference to <code>this</code> for easy method chaining 281 */ 282 public PractitionerRoleAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 283 this.daysOfWeek = theDaysOfWeek; 284 return this; 285 } 286 287 public boolean hasDaysOfWeek() { 288 if (this.daysOfWeek == null) 289 return false; 290 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 291 if (!item.isEmpty()) 292 return true; 293 return false; 294 } 295 296 /** 297 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 298 */ 299 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {//2 300 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 301 if (this.daysOfWeek == null) 302 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 303 this.daysOfWeek.add(t); 304 return t; 305 } 306 307 /** 308 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 309 */ 310 public PractitionerRoleAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { //1 311 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 312 t.setValue(value); 313 if (this.daysOfWeek == null) 314 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 315 this.daysOfWeek.add(t); 316 return this; 317 } 318 319 /** 320 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 321 */ 322 public boolean hasDaysOfWeek(DaysOfWeek value) { 323 if (this.daysOfWeek == null) 324 return false; 325 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 326 if (v.getValue().equals(value)) // code 327 return true; 328 return false; 329 } 330 331 /** 332 * @return {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 333 */ 334 public BooleanType getAllDayElement() { 335 if (this.allDay == null) 336 if (Configuration.errorOnAutoCreate()) 337 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.allDay"); 338 else if (Configuration.doAutoCreate()) 339 this.allDay = new BooleanType(); // bb 340 return this.allDay; 341 } 342 343 public boolean hasAllDayElement() { 344 return this.allDay != null && !this.allDay.isEmpty(); 345 } 346 347 public boolean hasAllDay() { 348 return this.allDay != null && !this.allDay.isEmpty(); 349 } 350 351 /** 352 * @param value {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 353 */ 354 public PractitionerRoleAvailableTimeComponent setAllDayElement(BooleanType value) { 355 this.allDay = value; 356 return this; 357 } 358 359 /** 360 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour service. 361 */ 362 public boolean getAllDay() { 363 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 364 } 365 366 /** 367 * @param value Is this always available? (hence times are irrelevant) e.g. 24 hour service. 368 */ 369 public PractitionerRoleAvailableTimeComponent setAllDay(boolean value) { 370 if (this.allDay == null) 371 this.allDay = new BooleanType(); 372 this.allDay.setValue(value); 373 return this; 374 } 375 376 /** 377 * @return {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 378 */ 379 public TimeType getAvailableStartTimeElement() { 380 if (this.availableStartTime == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableStartTime"); 383 else if (Configuration.doAutoCreate()) 384 this.availableStartTime = new TimeType(); // bb 385 return this.availableStartTime; 386 } 387 388 public boolean hasAvailableStartTimeElement() { 389 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 390 } 391 392 public boolean hasAvailableStartTime() { 393 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 398 */ 399 public PractitionerRoleAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 400 this.availableStartTime = value; 401 return this; 402 } 403 404 /** 405 * @return The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 406 */ 407 public String getAvailableStartTime() { 408 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 409 } 410 411 /** 412 * @param value The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 413 */ 414 public PractitionerRoleAvailableTimeComponent setAvailableStartTime(String value) { 415 if (value == null) 416 this.availableStartTime = null; 417 else { 418 if (this.availableStartTime == null) 419 this.availableStartTime = new TimeType(); 420 this.availableStartTime.setValue(value); 421 } 422 return this; 423 } 424 425 /** 426 * @return {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 427 */ 428 public TimeType getAvailableEndTimeElement() { 429 if (this.availableEndTime == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableEndTime"); 432 else if (Configuration.doAutoCreate()) 433 this.availableEndTime = new TimeType(); // bb 434 return this.availableEndTime; 435 } 436 437 public boolean hasAvailableEndTimeElement() { 438 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 439 } 440 441 public boolean hasAvailableEndTime() { 442 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 447 */ 448 public PractitionerRoleAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 449 this.availableEndTime = value; 450 return this; 451 } 452 453 /** 454 * @return The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 455 */ 456 public String getAvailableEndTime() { 457 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 458 } 459 460 /** 461 * @param value The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 462 */ 463 public PractitionerRoleAvailableTimeComponent setAvailableEndTime(String value) { 464 if (value == null) 465 this.availableEndTime = null; 466 else { 467 if (this.availableEndTime == null) 468 this.availableEndTime = new TimeType(); 469 this.availableEndTime.setValue(value); 470 } 471 return this; 472 } 473 474 protected void listChildren(List<Property> children) { 475 super.listChildren(children); 476 children.add(new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek)); 477 children.add(new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 478 children.add(new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime)); 479 children.add(new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime)); 480 } 481 482 @Override 483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 484 switch (_hash) { 485 case 68050338: /*daysOfWeek*/ return new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek); 486 case -1414913477: /*allDay*/ return new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 487 case -1039453818: /*availableStartTime*/ return new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime); 488 case 101151551: /*availableEndTime*/ return new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime); 489 default: return super.getNamedProperty(_hash, _name, _checkValid); 490 } 491 492 } 493 494 @Override 495 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 496 switch (hash) { 497 case 68050338: /*daysOfWeek*/ return this.daysOfWeek == null ? new Base[0] : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 498 case -1414913477: /*allDay*/ return this.allDay == null ? new Base[0] : new Base[] {this.allDay}; // BooleanType 499 case -1039453818: /*availableStartTime*/ return this.availableStartTime == null ? new Base[0] : new Base[] {this.availableStartTime}; // TimeType 500 case 101151551: /*availableEndTime*/ return this.availableEndTime == null ? new Base[0] : new Base[] {this.availableEndTime}; // TimeType 501 default: return super.getProperty(hash, name, checkValid); 502 } 503 504 } 505 506 @Override 507 public Base setProperty(int hash, String name, Base value) throws FHIRException { 508 switch (hash) { 509 case 68050338: // daysOfWeek 510 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 511 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 512 return value; 513 case -1414913477: // allDay 514 this.allDay = castToBoolean(value); // BooleanType 515 return value; 516 case -1039453818: // availableStartTime 517 this.availableStartTime = castToTime(value); // TimeType 518 return value; 519 case 101151551: // availableEndTime 520 this.availableEndTime = castToTime(value); // TimeType 521 return value; 522 default: return super.setProperty(hash, name, value); 523 } 524 525 } 526 527 @Override 528 public Base setProperty(String name, Base value) throws FHIRException { 529 if (name.equals("daysOfWeek")) { 530 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 531 this.getDaysOfWeek().add((Enumeration) value); 532 } else if (name.equals("allDay")) { 533 this.allDay = castToBoolean(value); // BooleanType 534 } else if (name.equals("availableStartTime")) { 535 this.availableStartTime = castToTime(value); // TimeType 536 } else if (name.equals("availableEndTime")) { 537 this.availableEndTime = castToTime(value); // TimeType 538 } else 539 return super.setProperty(name, value); 540 return value; 541 } 542 543 @Override 544 public Base makeProperty(int hash, String name) throws FHIRException { 545 switch (hash) { 546 case 68050338: return addDaysOfWeekElement(); 547 case -1414913477: return getAllDayElement(); 548 case -1039453818: return getAvailableStartTimeElement(); 549 case 101151551: return getAvailableEndTimeElement(); 550 default: return super.makeProperty(hash, name); 551 } 552 553 } 554 555 @Override 556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 557 switch (hash) { 558 case 68050338: /*daysOfWeek*/ return new String[] {"code"}; 559 case -1414913477: /*allDay*/ return new String[] {"boolean"}; 560 case -1039453818: /*availableStartTime*/ return new String[] {"time"}; 561 case 101151551: /*availableEndTime*/ return new String[] {"time"}; 562 default: return super.getTypesForProperty(hash, name); 563 } 564 565 } 566 567 @Override 568 public Base addChild(String name) throws FHIRException { 569 if (name.equals("daysOfWeek")) { 570 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.daysOfWeek"); 571 } 572 else if (name.equals("allDay")) { 573 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.allDay"); 574 } 575 else if (name.equals("availableStartTime")) { 576 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableStartTime"); 577 } 578 else if (name.equals("availableEndTime")) { 579 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableEndTime"); 580 } 581 else 582 return super.addChild(name); 583 } 584 585 public PractitionerRoleAvailableTimeComponent copy() { 586 PractitionerRoleAvailableTimeComponent dst = new PractitionerRoleAvailableTimeComponent(); 587 copyValues(dst); 588 if (daysOfWeek != null) { 589 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 590 for (Enumeration<DaysOfWeek> i : daysOfWeek) 591 dst.daysOfWeek.add(i.copy()); 592 }; 593 dst.allDay = allDay == null ? null : allDay.copy(); 594 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 595 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 596 return dst; 597 } 598 599 @Override 600 public boolean equalsDeep(Base other_) { 601 if (!super.equalsDeep(other_)) 602 return false; 603 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 604 return false; 605 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 606 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) && compareDeep(availableStartTime, o.availableStartTime, true) 607 && compareDeep(availableEndTime, o.availableEndTime, true); 608 } 609 610 @Override 611 public boolean equalsShallow(Base other_) { 612 if (!super.equalsShallow(other_)) 613 return false; 614 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 615 return false; 616 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 617 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) && compareValues(availableStartTime, o.availableStartTime, true) 618 && compareValues(availableEndTime, o.availableEndTime, true); 619 } 620 621 public boolean isEmpty() { 622 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime 623 , availableEndTime); 624 } 625 626 public String fhirType() { 627 return "PractitionerRole.availableTime"; 628 629 } 630 631 } 632 633 @Block() 634 public static class PractitionerRoleNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 635 /** 636 * The reason that can be presented to the user as to why this time is not available. 637 */ 638 @Child(name = "description", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 639 @Description(shortDefinition="Reason presented to the user explaining why time not available", formalDefinition="The reason that can be presented to the user as to why this time is not available." ) 640 protected StringType description; 641 642 /** 643 * Service is not available (seasonally or for a public holiday) from this date. 644 */ 645 @Child(name = "during", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 646 @Description(shortDefinition="Service not availablefrom this date", formalDefinition="Service is not available (seasonally or for a public holiday) from this date." ) 647 protected Period during; 648 649 private static final long serialVersionUID = 310849929L; 650 651 /** 652 * Constructor 653 */ 654 public PractitionerRoleNotAvailableComponent() { 655 super(); 656 } 657 658 /** 659 * Constructor 660 */ 661 public PractitionerRoleNotAvailableComponent(StringType description) { 662 super(); 663 this.description = description; 664 } 665 666 /** 667 * @return {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 668 */ 669 public StringType getDescriptionElement() { 670 if (this.description == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.description"); 673 else if (Configuration.doAutoCreate()) 674 this.description = new StringType(); // bb 675 return this.description; 676 } 677 678 public boolean hasDescriptionElement() { 679 return this.description != null && !this.description.isEmpty(); 680 } 681 682 public boolean hasDescription() { 683 return this.description != null && !this.description.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 688 */ 689 public PractitionerRoleNotAvailableComponent setDescriptionElement(StringType value) { 690 this.description = value; 691 return this; 692 } 693 694 /** 695 * @return The reason that can be presented to the user as to why this time is not available. 696 */ 697 public String getDescription() { 698 return this.description == null ? null : this.description.getValue(); 699 } 700 701 /** 702 * @param value The reason that can be presented to the user as to why this time is not available. 703 */ 704 public PractitionerRoleNotAvailableComponent setDescription(String value) { 705 if (this.description == null) 706 this.description = new StringType(); 707 this.description.setValue(value); 708 return this; 709 } 710 711 /** 712 * @return {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 713 */ 714 public Period getDuring() { 715 if (this.during == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.during"); 718 else if (Configuration.doAutoCreate()) 719 this.during = new Period(); // cc 720 return this.during; 721 } 722 723 public boolean hasDuring() { 724 return this.during != null && !this.during.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 729 */ 730 public PractitionerRoleNotAvailableComponent setDuring(Period value) { 731 this.during = value; 732 return this; 733 } 734 735 protected void listChildren(List<Property> children) { 736 super.listChildren(children); 737 children.add(new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 738 children.add(new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 739 } 740 741 @Override 742 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 743 switch (_hash) { 744 case -1724546052: /*description*/ return new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 745 case -1320499647: /*during*/ return new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 746 default: return super.getNamedProperty(_hash, _name, _checkValid); 747 } 748 749 } 750 751 @Override 752 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 753 switch (hash) { 754 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 755 case -1320499647: /*during*/ return this.during == null ? new Base[0] : new Base[] {this.during}; // Period 756 default: return super.getProperty(hash, name, checkValid); 757 } 758 759 } 760 761 @Override 762 public Base setProperty(int hash, String name, Base value) throws FHIRException { 763 switch (hash) { 764 case -1724546052: // description 765 this.description = castToString(value); // StringType 766 return value; 767 case -1320499647: // during 768 this.during = castToPeriod(value); // Period 769 return value; 770 default: return super.setProperty(hash, name, value); 771 } 772 773 } 774 775 @Override 776 public Base setProperty(String name, Base value) throws FHIRException { 777 if (name.equals("description")) { 778 this.description = castToString(value); // StringType 779 } else if (name.equals("during")) { 780 this.during = castToPeriod(value); // Period 781 } else 782 return super.setProperty(name, value); 783 return value; 784 } 785 786 @Override 787 public Base makeProperty(int hash, String name) throws FHIRException { 788 switch (hash) { 789 case -1724546052: return getDescriptionElement(); 790 case -1320499647: return getDuring(); 791 default: return super.makeProperty(hash, name); 792 } 793 794 } 795 796 @Override 797 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 798 switch (hash) { 799 case -1724546052: /*description*/ return new String[] {"string"}; 800 case -1320499647: /*during*/ return new String[] {"Period"}; 801 default: return super.getTypesForProperty(hash, name); 802 } 803 804 } 805 806 @Override 807 public Base addChild(String name) throws FHIRException { 808 if (name.equals("description")) { 809 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.description"); 810 } 811 else if (name.equals("during")) { 812 this.during = new Period(); 813 return this.during; 814 } 815 else 816 return super.addChild(name); 817 } 818 819 public PractitionerRoleNotAvailableComponent copy() { 820 PractitionerRoleNotAvailableComponent dst = new PractitionerRoleNotAvailableComponent(); 821 copyValues(dst); 822 dst.description = description == null ? null : description.copy(); 823 dst.during = during == null ? null : during.copy(); 824 return dst; 825 } 826 827 @Override 828 public boolean equalsDeep(Base other_) { 829 if (!super.equalsDeep(other_)) 830 return false; 831 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 832 return false; 833 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 834 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 835 } 836 837 @Override 838 public boolean equalsShallow(Base other_) { 839 if (!super.equalsShallow(other_)) 840 return false; 841 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 842 return false; 843 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 844 return compareValues(description, o.description, true); 845 } 846 847 public boolean isEmpty() { 848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 849 } 850 851 public String fhirType() { 852 return "PractitionerRole.notAvailable"; 853 854 } 855 856 } 857 858 /** 859 * Business Identifiers that are specific to a role/location. 860 */ 861 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 862 @Description(shortDefinition="Business Identifiers that are specific to a role/location", formalDefinition="Business Identifiers that are specific to a role/location." ) 863 protected List<Identifier> identifier; 864 865 /** 866 * Whether this practitioner's record is in active use. 867 */ 868 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 869 @Description(shortDefinition="Whether this practitioner's record is in active use", formalDefinition="Whether this practitioner's record is in active use." ) 870 protected BooleanType active; 871 872 /** 873 * The period during which the person is authorized to act as a practitioner in these role(s) for the organization. 874 */ 875 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 876 @Description(shortDefinition="The period during which the practitioner is authorized to perform in these role(s)", formalDefinition="The period during which the person is authorized to act as a practitioner in these role(s) for the organization." ) 877 protected Period period; 878 879 /** 880 * Practitioner that is able to provide the defined services for the organation. 881 */ 882 @Child(name = "practitioner", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=true) 883 @Description(shortDefinition="Practitioner that is able to provide the defined services for the organation", formalDefinition="Practitioner that is able to provide the defined services for the organation." ) 884 protected Reference practitioner; 885 886 /** 887 * The actual object that is the target of the reference (Practitioner that is able to provide the defined services for the organation.) 888 */ 889 protected Practitioner practitionerTarget; 890 891 /** 892 * The organization where the Practitioner performs the roles associated. 893 */ 894 @Child(name = "organization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 895 @Description(shortDefinition="Organization where the roles are available", formalDefinition="The organization where the Practitioner performs the roles associated." ) 896 protected Reference organization; 897 898 /** 899 * The actual object that is the target of the reference (The organization where the Practitioner performs the roles associated.) 900 */ 901 protected Organization organizationTarget; 902 903 /** 904 * Roles which this practitioner is authorized to perform for the organization. 905 */ 906 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 907 @Description(shortDefinition="Roles which this practitioner may perform", formalDefinition="Roles which this practitioner is authorized to perform for the organization." ) 908 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/practitioner-role") 909 protected List<CodeableConcept> code; 910 911 /** 912 * Specific specialty of the practitioner. 913 */ 914 @Child(name = "specialty", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 915 @Description(shortDefinition="Specific specialty of the practitioner", formalDefinition="Specific specialty of the practitioner." ) 916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 917 protected List<CodeableConcept> specialty; 918 919 /** 920 * The location(s) at which this practitioner provides care. 921 */ 922 @Child(name = "location", type = {Location.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 923 @Description(shortDefinition="The location(s) at which this practitioner provides care", formalDefinition="The location(s) at which this practitioner provides care." ) 924 protected List<Reference> location; 925 /** 926 * The actual objects that are the target of the reference (The location(s) at which this practitioner provides care.) 927 */ 928 protected List<Location> locationTarget; 929 930 931 /** 932 * The list of healthcare services that this worker provides for this role's Organization/Location(s). 933 */ 934 @Child(name = "healthcareService", type = {HealthcareService.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 935 @Description(shortDefinition="The list of healthcare services that this worker provides for this role's Organization/Location(s)", formalDefinition="The list of healthcare services that this worker provides for this role's Organization/Location(s)." ) 936 protected List<Reference> healthcareService; 937 /** 938 * The actual objects that are the target of the reference (The list of healthcare services that this worker provides for this role's Organization/Location(s).) 939 */ 940 protected List<HealthcareService> healthcareServiceTarget; 941 942 943 /** 944 * Contact details that are specific to the role/location/service. 945 */ 946 @Child(name = "telecom", type = {ContactPoint.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 947 @Description(shortDefinition="Contact details that are specific to the role/location/service", formalDefinition="Contact details that are specific to the role/location/service." ) 948 protected List<ContactPoint> telecom; 949 950 /** 951 * A collection of times that the Service Site is available. 952 */ 953 @Child(name = "availableTime", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 954 @Description(shortDefinition="Times the Service Site is available", formalDefinition="A collection of times that the Service Site is available." ) 955 protected List<PractitionerRoleAvailableTimeComponent> availableTime; 956 957 /** 958 * The HealthcareService is not available during this period of time due to the provided reason. 959 */ 960 @Child(name = "notAvailable", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 961 @Description(shortDefinition="Not available during this time due to provided reason", formalDefinition="The HealthcareService is not available during this period of time due to the provided reason." ) 962 protected List<PractitionerRoleNotAvailableComponent> notAvailable; 963 964 /** 965 * A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 966 */ 967 @Child(name = "availabilityExceptions", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 968 @Description(shortDefinition="Description of availability exceptions", formalDefinition="A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times." ) 969 protected StringType availabilityExceptions; 970 971 /** 972 * Technical endpoints providing access to services operated for the practitioner with this role. 973 */ 974 @Child(name = "endpoint", type = {Endpoint.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 975 @Description(shortDefinition="Technical endpoints providing access to services operated for the practitioner with this role", formalDefinition="Technical endpoints providing access to services operated for the practitioner with this role." ) 976 protected List<Reference> endpoint; 977 /** 978 * The actual objects that are the target of the reference (Technical endpoints providing access to services operated for the practitioner with this role.) 979 */ 980 protected List<Endpoint> endpointTarget; 981 982 983 private static final long serialVersionUID = 423338051L; 984 985 /** 986 * Constructor 987 */ 988 public PractitionerRole() { 989 super(); 990 } 991 992 /** 993 * @return {@link #identifier} (Business Identifiers that are specific to a role/location.) 994 */ 995 public List<Identifier> getIdentifier() { 996 if (this.identifier == null) 997 this.identifier = new ArrayList<Identifier>(); 998 return this.identifier; 999 } 1000 1001 /** 1002 * @return Returns a reference to <code>this</code> for easy method chaining 1003 */ 1004 public PractitionerRole setIdentifier(List<Identifier> theIdentifier) { 1005 this.identifier = theIdentifier; 1006 return this; 1007 } 1008 1009 public boolean hasIdentifier() { 1010 if (this.identifier == null) 1011 return false; 1012 for (Identifier item : this.identifier) 1013 if (!item.isEmpty()) 1014 return true; 1015 return false; 1016 } 1017 1018 public Identifier addIdentifier() { //3 1019 Identifier t = new Identifier(); 1020 if (this.identifier == null) 1021 this.identifier = new ArrayList<Identifier>(); 1022 this.identifier.add(t); 1023 return t; 1024 } 1025 1026 public PractitionerRole addIdentifier(Identifier t) { //3 1027 if (t == null) 1028 return this; 1029 if (this.identifier == null) 1030 this.identifier = new ArrayList<Identifier>(); 1031 this.identifier.add(t); 1032 return this; 1033 } 1034 1035 /** 1036 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1037 */ 1038 public Identifier getIdentifierFirstRep() { 1039 if (getIdentifier().isEmpty()) { 1040 addIdentifier(); 1041 } 1042 return getIdentifier().get(0); 1043 } 1044 1045 /** 1046 * @return {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1047 */ 1048 public BooleanType getActiveElement() { 1049 if (this.active == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create PractitionerRole.active"); 1052 else if (Configuration.doAutoCreate()) 1053 this.active = new BooleanType(); // bb 1054 return this.active; 1055 } 1056 1057 public boolean hasActiveElement() { 1058 return this.active != null && !this.active.isEmpty(); 1059 } 1060 1061 public boolean hasActive() { 1062 return this.active != null && !this.active.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1067 */ 1068 public PractitionerRole setActiveElement(BooleanType value) { 1069 this.active = value; 1070 return this; 1071 } 1072 1073 /** 1074 * @return Whether this practitioner's record is in active use. 1075 */ 1076 public boolean getActive() { 1077 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1078 } 1079 1080 /** 1081 * @param value Whether this practitioner's record is in active use. 1082 */ 1083 public PractitionerRole setActive(boolean value) { 1084 if (this.active == null) 1085 this.active = new BooleanType(); 1086 this.active.setValue(value); 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 1092 */ 1093 public Period getPeriod() { 1094 if (this.period == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error("Attempt to auto-create PractitionerRole.period"); 1097 else if (Configuration.doAutoCreate()) 1098 this.period = new Period(); // cc 1099 return this.period; 1100 } 1101 1102 public boolean hasPeriod() { 1103 return this.period != null && !this.period.isEmpty(); 1104 } 1105 1106 /** 1107 * @param value {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 1108 */ 1109 public PractitionerRole setPeriod(Period value) { 1110 this.period = value; 1111 return this; 1112 } 1113 1114 /** 1115 * @return {@link #practitioner} (Practitioner that is able to provide the defined services for the organation.) 1116 */ 1117 public Reference getPractitioner() { 1118 if (this.practitioner == null) 1119 if (Configuration.errorOnAutoCreate()) 1120 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1121 else if (Configuration.doAutoCreate()) 1122 this.practitioner = new Reference(); // cc 1123 return this.practitioner; 1124 } 1125 1126 public boolean hasPractitioner() { 1127 return this.practitioner != null && !this.practitioner.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #practitioner} (Practitioner that is able to provide the defined services for the organation.) 1132 */ 1133 public PractitionerRole setPractitioner(Reference value) { 1134 this.practitioner = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return {@link #practitioner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Practitioner that is able to provide the defined services for the organation.) 1140 */ 1141 public Practitioner getPractitionerTarget() { 1142 if (this.practitionerTarget == null) 1143 if (Configuration.errorOnAutoCreate()) 1144 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1145 else if (Configuration.doAutoCreate()) 1146 this.practitionerTarget = new Practitioner(); // aa 1147 return this.practitionerTarget; 1148 } 1149 1150 /** 1151 * @param value {@link #practitioner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Practitioner that is able to provide the defined services for the organation.) 1152 */ 1153 public PractitionerRole setPractitionerTarget(Practitioner value) { 1154 this.practitionerTarget = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #organization} (The organization where the Practitioner performs the roles associated.) 1160 */ 1161 public Reference getOrganization() { 1162 if (this.organization == null) 1163 if (Configuration.errorOnAutoCreate()) 1164 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1165 else if (Configuration.doAutoCreate()) 1166 this.organization = new Reference(); // cc 1167 return this.organization; 1168 } 1169 1170 public boolean hasOrganization() { 1171 return this.organization != null && !this.organization.isEmpty(); 1172 } 1173 1174 /** 1175 * @param value {@link #organization} (The organization where the Practitioner performs the roles associated.) 1176 */ 1177 public PractitionerRole setOrganization(Reference value) { 1178 this.organization = value; 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization where the Practitioner performs the roles associated.) 1184 */ 1185 public Organization getOrganizationTarget() { 1186 if (this.organizationTarget == null) 1187 if (Configuration.errorOnAutoCreate()) 1188 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1189 else if (Configuration.doAutoCreate()) 1190 this.organizationTarget = new Organization(); // aa 1191 return this.organizationTarget; 1192 } 1193 1194 /** 1195 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization where the Practitioner performs the roles associated.) 1196 */ 1197 public PractitionerRole setOrganizationTarget(Organization value) { 1198 this.organizationTarget = value; 1199 return this; 1200 } 1201 1202 /** 1203 * @return {@link #code} (Roles which this practitioner is authorized to perform for the organization.) 1204 */ 1205 public List<CodeableConcept> getCode() { 1206 if (this.code == null) 1207 this.code = new ArrayList<CodeableConcept>(); 1208 return this.code; 1209 } 1210 1211 /** 1212 * @return Returns a reference to <code>this</code> for easy method chaining 1213 */ 1214 public PractitionerRole setCode(List<CodeableConcept> theCode) { 1215 this.code = theCode; 1216 return this; 1217 } 1218 1219 public boolean hasCode() { 1220 if (this.code == null) 1221 return false; 1222 for (CodeableConcept item : this.code) 1223 if (!item.isEmpty()) 1224 return true; 1225 return false; 1226 } 1227 1228 public CodeableConcept addCode() { //3 1229 CodeableConcept t = new CodeableConcept(); 1230 if (this.code == null) 1231 this.code = new ArrayList<CodeableConcept>(); 1232 this.code.add(t); 1233 return t; 1234 } 1235 1236 public PractitionerRole addCode(CodeableConcept t) { //3 1237 if (t == null) 1238 return this; 1239 if (this.code == null) 1240 this.code = new ArrayList<CodeableConcept>(); 1241 this.code.add(t); 1242 return this; 1243 } 1244 1245 /** 1246 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1247 */ 1248 public CodeableConcept getCodeFirstRep() { 1249 if (getCode().isEmpty()) { 1250 addCode(); 1251 } 1252 return getCode().get(0); 1253 } 1254 1255 /** 1256 * @return {@link #specialty} (Specific specialty of the practitioner.) 1257 */ 1258 public List<CodeableConcept> getSpecialty() { 1259 if (this.specialty == null) 1260 this.specialty = new ArrayList<CodeableConcept>(); 1261 return this.specialty; 1262 } 1263 1264 /** 1265 * @return Returns a reference to <code>this</code> for easy method chaining 1266 */ 1267 public PractitionerRole setSpecialty(List<CodeableConcept> theSpecialty) { 1268 this.specialty = theSpecialty; 1269 return this; 1270 } 1271 1272 public boolean hasSpecialty() { 1273 if (this.specialty == null) 1274 return false; 1275 for (CodeableConcept item : this.specialty) 1276 if (!item.isEmpty()) 1277 return true; 1278 return false; 1279 } 1280 1281 public CodeableConcept addSpecialty() { //3 1282 CodeableConcept t = new CodeableConcept(); 1283 if (this.specialty == null) 1284 this.specialty = new ArrayList<CodeableConcept>(); 1285 this.specialty.add(t); 1286 return t; 1287 } 1288 1289 public PractitionerRole addSpecialty(CodeableConcept t) { //3 1290 if (t == null) 1291 return this; 1292 if (this.specialty == null) 1293 this.specialty = new ArrayList<CodeableConcept>(); 1294 this.specialty.add(t); 1295 return this; 1296 } 1297 1298 /** 1299 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 1300 */ 1301 public CodeableConcept getSpecialtyFirstRep() { 1302 if (getSpecialty().isEmpty()) { 1303 addSpecialty(); 1304 } 1305 return getSpecialty().get(0); 1306 } 1307 1308 /** 1309 * @return {@link #location} (The location(s) at which this practitioner provides care.) 1310 */ 1311 public List<Reference> getLocation() { 1312 if (this.location == null) 1313 this.location = new ArrayList<Reference>(); 1314 return this.location; 1315 } 1316 1317 /** 1318 * @return Returns a reference to <code>this</code> for easy method chaining 1319 */ 1320 public PractitionerRole setLocation(List<Reference> theLocation) { 1321 this.location = theLocation; 1322 return this; 1323 } 1324 1325 public boolean hasLocation() { 1326 if (this.location == null) 1327 return false; 1328 for (Reference item : this.location) 1329 if (!item.isEmpty()) 1330 return true; 1331 return false; 1332 } 1333 1334 public Reference addLocation() { //3 1335 Reference t = new Reference(); 1336 if (this.location == null) 1337 this.location = new ArrayList<Reference>(); 1338 this.location.add(t); 1339 return t; 1340 } 1341 1342 public PractitionerRole addLocation(Reference t) { //3 1343 if (t == null) 1344 return this; 1345 if (this.location == null) 1346 this.location = new ArrayList<Reference>(); 1347 this.location.add(t); 1348 return this; 1349 } 1350 1351 /** 1352 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist 1353 */ 1354 public Reference getLocationFirstRep() { 1355 if (getLocation().isEmpty()) { 1356 addLocation(); 1357 } 1358 return getLocation().get(0); 1359 } 1360 1361 /** 1362 * @deprecated Use Reference#setResource(IBaseResource) instead 1363 */ 1364 @Deprecated 1365 public List<Location> getLocationTarget() { 1366 if (this.locationTarget == null) 1367 this.locationTarget = new ArrayList<Location>(); 1368 return this.locationTarget; 1369 } 1370 1371 /** 1372 * @deprecated Use Reference#setResource(IBaseResource) instead 1373 */ 1374 @Deprecated 1375 public Location addLocationTarget() { 1376 Location r = new Location(); 1377 if (this.locationTarget == null) 1378 this.locationTarget = new ArrayList<Location>(); 1379 this.locationTarget.add(r); 1380 return r; 1381 } 1382 1383 /** 1384 * @return {@link #healthcareService} (The list of healthcare services that this worker provides for this role's Organization/Location(s).) 1385 */ 1386 public List<Reference> getHealthcareService() { 1387 if (this.healthcareService == null) 1388 this.healthcareService = new ArrayList<Reference>(); 1389 return this.healthcareService; 1390 } 1391 1392 /** 1393 * @return Returns a reference to <code>this</code> for easy method chaining 1394 */ 1395 public PractitionerRole setHealthcareService(List<Reference> theHealthcareService) { 1396 this.healthcareService = theHealthcareService; 1397 return this; 1398 } 1399 1400 public boolean hasHealthcareService() { 1401 if (this.healthcareService == null) 1402 return false; 1403 for (Reference item : this.healthcareService) 1404 if (!item.isEmpty()) 1405 return true; 1406 return false; 1407 } 1408 1409 public Reference addHealthcareService() { //3 1410 Reference t = new Reference(); 1411 if (this.healthcareService == null) 1412 this.healthcareService = new ArrayList<Reference>(); 1413 this.healthcareService.add(t); 1414 return t; 1415 } 1416 1417 public PractitionerRole addHealthcareService(Reference t) { //3 1418 if (t == null) 1419 return this; 1420 if (this.healthcareService == null) 1421 this.healthcareService = new ArrayList<Reference>(); 1422 this.healthcareService.add(t); 1423 return this; 1424 } 1425 1426 /** 1427 * @return The first repetition of repeating field {@link #healthcareService}, creating it if it does not already exist 1428 */ 1429 public Reference getHealthcareServiceFirstRep() { 1430 if (getHealthcareService().isEmpty()) { 1431 addHealthcareService(); 1432 } 1433 return getHealthcareService().get(0); 1434 } 1435 1436 /** 1437 * @deprecated Use Reference#setResource(IBaseResource) instead 1438 */ 1439 @Deprecated 1440 public List<HealthcareService> getHealthcareServiceTarget() { 1441 if (this.healthcareServiceTarget == null) 1442 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1443 return this.healthcareServiceTarget; 1444 } 1445 1446 /** 1447 * @deprecated Use Reference#setResource(IBaseResource) instead 1448 */ 1449 @Deprecated 1450 public HealthcareService addHealthcareServiceTarget() { 1451 HealthcareService r = new HealthcareService(); 1452 if (this.healthcareServiceTarget == null) 1453 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1454 this.healthcareServiceTarget.add(r); 1455 return r; 1456 } 1457 1458 /** 1459 * @return {@link #telecom} (Contact details that are specific to the role/location/service.) 1460 */ 1461 public List<ContactPoint> getTelecom() { 1462 if (this.telecom == null) 1463 this.telecom = new ArrayList<ContactPoint>(); 1464 return this.telecom; 1465 } 1466 1467 /** 1468 * @return Returns a reference to <code>this</code> for easy method chaining 1469 */ 1470 public PractitionerRole setTelecom(List<ContactPoint> theTelecom) { 1471 this.telecom = theTelecom; 1472 return this; 1473 } 1474 1475 public boolean hasTelecom() { 1476 if (this.telecom == null) 1477 return false; 1478 for (ContactPoint item : this.telecom) 1479 if (!item.isEmpty()) 1480 return true; 1481 return false; 1482 } 1483 1484 public ContactPoint addTelecom() { //3 1485 ContactPoint t = new ContactPoint(); 1486 if (this.telecom == null) 1487 this.telecom = new ArrayList<ContactPoint>(); 1488 this.telecom.add(t); 1489 return t; 1490 } 1491 1492 public PractitionerRole addTelecom(ContactPoint t) { //3 1493 if (t == null) 1494 return this; 1495 if (this.telecom == null) 1496 this.telecom = new ArrayList<ContactPoint>(); 1497 this.telecom.add(t); 1498 return this; 1499 } 1500 1501 /** 1502 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 1503 */ 1504 public ContactPoint getTelecomFirstRep() { 1505 if (getTelecom().isEmpty()) { 1506 addTelecom(); 1507 } 1508 return getTelecom().get(0); 1509 } 1510 1511 /** 1512 * @return {@link #availableTime} (A collection of times that the Service Site is available.) 1513 */ 1514 public List<PractitionerRoleAvailableTimeComponent> getAvailableTime() { 1515 if (this.availableTime == null) 1516 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1517 return this.availableTime; 1518 } 1519 1520 /** 1521 * @return Returns a reference to <code>this</code> for easy method chaining 1522 */ 1523 public PractitionerRole setAvailableTime(List<PractitionerRoleAvailableTimeComponent> theAvailableTime) { 1524 this.availableTime = theAvailableTime; 1525 return this; 1526 } 1527 1528 public boolean hasAvailableTime() { 1529 if (this.availableTime == null) 1530 return false; 1531 for (PractitionerRoleAvailableTimeComponent item : this.availableTime) 1532 if (!item.isEmpty()) 1533 return true; 1534 return false; 1535 } 1536 1537 public PractitionerRoleAvailableTimeComponent addAvailableTime() { //3 1538 PractitionerRoleAvailableTimeComponent t = new PractitionerRoleAvailableTimeComponent(); 1539 if (this.availableTime == null) 1540 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1541 this.availableTime.add(t); 1542 return t; 1543 } 1544 1545 public PractitionerRole addAvailableTime(PractitionerRoleAvailableTimeComponent t) { //3 1546 if (t == null) 1547 return this; 1548 if (this.availableTime == null) 1549 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1550 this.availableTime.add(t); 1551 return this; 1552 } 1553 1554 /** 1555 * @return The first repetition of repeating field {@link #availableTime}, creating it if it does not already exist 1556 */ 1557 public PractitionerRoleAvailableTimeComponent getAvailableTimeFirstRep() { 1558 if (getAvailableTime().isEmpty()) { 1559 addAvailableTime(); 1560 } 1561 return getAvailableTime().get(0); 1562 } 1563 1564 /** 1565 * @return {@link #notAvailable} (The HealthcareService is not available during this period of time due to the provided reason.) 1566 */ 1567 public List<PractitionerRoleNotAvailableComponent> getNotAvailable() { 1568 if (this.notAvailable == null) 1569 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1570 return this.notAvailable; 1571 } 1572 1573 /** 1574 * @return Returns a reference to <code>this</code> for easy method chaining 1575 */ 1576 public PractitionerRole setNotAvailable(List<PractitionerRoleNotAvailableComponent> theNotAvailable) { 1577 this.notAvailable = theNotAvailable; 1578 return this; 1579 } 1580 1581 public boolean hasNotAvailable() { 1582 if (this.notAvailable == null) 1583 return false; 1584 for (PractitionerRoleNotAvailableComponent item : this.notAvailable) 1585 if (!item.isEmpty()) 1586 return true; 1587 return false; 1588 } 1589 1590 public PractitionerRoleNotAvailableComponent addNotAvailable() { //3 1591 PractitionerRoleNotAvailableComponent t = new PractitionerRoleNotAvailableComponent(); 1592 if (this.notAvailable == null) 1593 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1594 this.notAvailable.add(t); 1595 return t; 1596 } 1597 1598 public PractitionerRole addNotAvailable(PractitionerRoleNotAvailableComponent t) { //3 1599 if (t == null) 1600 return this; 1601 if (this.notAvailable == null) 1602 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1603 this.notAvailable.add(t); 1604 return this; 1605 } 1606 1607 /** 1608 * @return The first repetition of repeating field {@link #notAvailable}, creating it if it does not already exist 1609 */ 1610 public PractitionerRoleNotAvailableComponent getNotAvailableFirstRep() { 1611 if (getNotAvailable().isEmpty()) { 1612 addNotAvailable(); 1613 } 1614 return getNotAvailable().get(0); 1615 } 1616 1617 /** 1618 * @return {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 1619 */ 1620 public StringType getAvailabilityExceptionsElement() { 1621 if (this.availabilityExceptions == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create PractitionerRole.availabilityExceptions"); 1624 else if (Configuration.doAutoCreate()) 1625 this.availabilityExceptions = new StringType(); // bb 1626 return this.availabilityExceptions; 1627 } 1628 1629 public boolean hasAvailabilityExceptionsElement() { 1630 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1631 } 1632 1633 public boolean hasAvailabilityExceptions() { 1634 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 1639 */ 1640 public PractitionerRole setAvailabilityExceptionsElement(StringType value) { 1641 this.availabilityExceptions = value; 1642 return this; 1643 } 1644 1645 /** 1646 * @return A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 1647 */ 1648 public String getAvailabilityExceptions() { 1649 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 1650 } 1651 1652 /** 1653 * @param value A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 1654 */ 1655 public PractitionerRole setAvailabilityExceptions(String value) { 1656 if (Utilities.noString(value)) 1657 this.availabilityExceptions = null; 1658 else { 1659 if (this.availabilityExceptions == null) 1660 this.availabilityExceptions = new StringType(); 1661 this.availabilityExceptions.setValue(value); 1662 } 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the practitioner with this role.) 1668 */ 1669 public List<Reference> getEndpoint() { 1670 if (this.endpoint == null) 1671 this.endpoint = new ArrayList<Reference>(); 1672 return this.endpoint; 1673 } 1674 1675 /** 1676 * @return Returns a reference to <code>this</code> for easy method chaining 1677 */ 1678 public PractitionerRole setEndpoint(List<Reference> theEndpoint) { 1679 this.endpoint = theEndpoint; 1680 return this; 1681 } 1682 1683 public boolean hasEndpoint() { 1684 if (this.endpoint == null) 1685 return false; 1686 for (Reference item : this.endpoint) 1687 if (!item.isEmpty()) 1688 return true; 1689 return false; 1690 } 1691 1692 public Reference addEndpoint() { //3 1693 Reference t = new Reference(); 1694 if (this.endpoint == null) 1695 this.endpoint = new ArrayList<Reference>(); 1696 this.endpoint.add(t); 1697 return t; 1698 } 1699 1700 public PractitionerRole addEndpoint(Reference t) { //3 1701 if (t == null) 1702 return this; 1703 if (this.endpoint == null) 1704 this.endpoint = new ArrayList<Reference>(); 1705 this.endpoint.add(t); 1706 return this; 1707 } 1708 1709 /** 1710 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 1711 */ 1712 public Reference getEndpointFirstRep() { 1713 if (getEndpoint().isEmpty()) { 1714 addEndpoint(); 1715 } 1716 return getEndpoint().get(0); 1717 } 1718 1719 /** 1720 * @deprecated Use Reference#setResource(IBaseResource) instead 1721 */ 1722 @Deprecated 1723 public List<Endpoint> getEndpointTarget() { 1724 if (this.endpointTarget == null) 1725 this.endpointTarget = new ArrayList<Endpoint>(); 1726 return this.endpointTarget; 1727 } 1728 1729 /** 1730 * @deprecated Use Reference#setResource(IBaseResource) instead 1731 */ 1732 @Deprecated 1733 public Endpoint addEndpointTarget() { 1734 Endpoint r = new Endpoint(); 1735 if (this.endpointTarget == null) 1736 this.endpointTarget = new ArrayList<Endpoint>(); 1737 this.endpointTarget.add(r); 1738 return r; 1739 } 1740 1741 protected void listChildren(List<Property> children) { 1742 super.listChildren(children); 1743 children.add(new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1744 children.add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active)); 1745 children.add(new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period)); 1746 children.add(new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organation.", 0, 1, practitioner)); 1747 children.add(new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization)); 1748 children.add(new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code)); 1749 children.add(new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, java.lang.Integer.MAX_VALUE, specialty)); 1750 children.add(new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 1751 children.add(new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService)); 1752 children.add(new Property("telecom", "ContactPoint", "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1753 children.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime)); 1754 children.add(new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable)); 1755 children.add(new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions)); 1756 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the practitioner with this role.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1757 } 1758 1759 @Override 1760 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1761 switch (_hash) { 1762 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier); 1763 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active); 1764 case -991726143: /*period*/ return new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period); 1765 case 574573338: /*practitioner*/ return new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organation.", 0, 1, practitioner); 1766 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization); 1767 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code); 1768 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, java.lang.Integer.MAX_VALUE, specialty); 1769 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location); 1770 case 1289661064: /*healthcareService*/ return new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService); 1771 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom); 1772 case 1873069366: /*availableTime*/ return new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 1773 case -629572298: /*notAvailable*/ return new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable); 1774 case -1149143617: /*availabilityExceptions*/ return new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions); 1775 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the practitioner with this role.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1776 default: return super.getNamedProperty(_hash, _name, _checkValid); 1777 } 1778 1779 } 1780 1781 @Override 1782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1783 switch (hash) { 1784 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1785 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1786 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1787 case 574573338: /*practitioner*/ return this.practitioner == null ? new Base[0] : new Base[] {this.practitioner}; // Reference 1788 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1789 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1790 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 1791 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 1792 case 1289661064: /*healthcareService*/ return this.healthcareService == null ? new Base[0] : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 1793 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1794 case 1873069366: /*availableTime*/ return this.availableTime == null ? new Base[0] : this.availableTime.toArray(new Base[this.availableTime.size()]); // PractitionerRoleAvailableTimeComponent 1795 case -629572298: /*notAvailable*/ return this.notAvailable == null ? new Base[0] : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // PractitionerRoleNotAvailableComponent 1796 case -1149143617: /*availabilityExceptions*/ return this.availabilityExceptions == null ? new Base[0] : new Base[] {this.availabilityExceptions}; // StringType 1797 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1798 default: return super.getProperty(hash, name, checkValid); 1799 } 1800 1801 } 1802 1803 @Override 1804 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1805 switch (hash) { 1806 case -1618432855: // identifier 1807 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1808 return value; 1809 case -1422950650: // active 1810 this.active = castToBoolean(value); // BooleanType 1811 return value; 1812 case -991726143: // period 1813 this.period = castToPeriod(value); // Period 1814 return value; 1815 case 574573338: // practitioner 1816 this.practitioner = castToReference(value); // Reference 1817 return value; 1818 case 1178922291: // organization 1819 this.organization = castToReference(value); // Reference 1820 return value; 1821 case 3059181: // code 1822 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1823 return value; 1824 case -1694759682: // specialty 1825 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 1826 return value; 1827 case 1901043637: // location 1828 this.getLocation().add(castToReference(value)); // Reference 1829 return value; 1830 case 1289661064: // healthcareService 1831 this.getHealthcareService().add(castToReference(value)); // Reference 1832 return value; 1833 case -1429363305: // telecom 1834 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1835 return value; 1836 case 1873069366: // availableTime 1837 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); // PractitionerRoleAvailableTimeComponent 1838 return value; 1839 case -629572298: // notAvailable 1840 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); // PractitionerRoleNotAvailableComponent 1841 return value; 1842 case -1149143617: // availabilityExceptions 1843 this.availabilityExceptions = castToString(value); // StringType 1844 return value; 1845 case 1741102485: // endpoint 1846 this.getEndpoint().add(castToReference(value)); // Reference 1847 return value; 1848 default: return super.setProperty(hash, name, value); 1849 } 1850 1851 } 1852 1853 @Override 1854 public Base setProperty(String name, Base value) throws FHIRException { 1855 if (name.equals("identifier")) { 1856 this.getIdentifier().add(castToIdentifier(value)); 1857 } else if (name.equals("active")) { 1858 this.active = castToBoolean(value); // BooleanType 1859 } else if (name.equals("period")) { 1860 this.period = castToPeriod(value); // Period 1861 } else if (name.equals("practitioner")) { 1862 this.practitioner = castToReference(value); // Reference 1863 } else if (name.equals("organization")) { 1864 this.organization = castToReference(value); // Reference 1865 } else if (name.equals("code")) { 1866 this.getCode().add(castToCodeableConcept(value)); 1867 } else if (name.equals("specialty")) { 1868 this.getSpecialty().add(castToCodeableConcept(value)); 1869 } else if (name.equals("location")) { 1870 this.getLocation().add(castToReference(value)); 1871 } else if (name.equals("healthcareService")) { 1872 this.getHealthcareService().add(castToReference(value)); 1873 } else if (name.equals("telecom")) { 1874 this.getTelecom().add(castToContactPoint(value)); 1875 } else if (name.equals("availableTime")) { 1876 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); 1877 } else if (name.equals("notAvailable")) { 1878 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); 1879 } else if (name.equals("availabilityExceptions")) { 1880 this.availabilityExceptions = castToString(value); // StringType 1881 } else if (name.equals("endpoint")) { 1882 this.getEndpoint().add(castToReference(value)); 1883 } else 1884 return super.setProperty(name, value); 1885 return value; 1886 } 1887 1888 @Override 1889 public Base makeProperty(int hash, String name) throws FHIRException { 1890 switch (hash) { 1891 case -1618432855: return addIdentifier(); 1892 case -1422950650: return getActiveElement(); 1893 case -991726143: return getPeriod(); 1894 case 574573338: return getPractitioner(); 1895 case 1178922291: return getOrganization(); 1896 case 3059181: return addCode(); 1897 case -1694759682: return addSpecialty(); 1898 case 1901043637: return addLocation(); 1899 case 1289661064: return addHealthcareService(); 1900 case -1429363305: return addTelecom(); 1901 case 1873069366: return addAvailableTime(); 1902 case -629572298: return addNotAvailable(); 1903 case -1149143617: return getAvailabilityExceptionsElement(); 1904 case 1741102485: return addEndpoint(); 1905 default: return super.makeProperty(hash, name); 1906 } 1907 1908 } 1909 1910 @Override 1911 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1912 switch (hash) { 1913 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1914 case -1422950650: /*active*/ return new String[] {"boolean"}; 1915 case -991726143: /*period*/ return new String[] {"Period"}; 1916 case 574573338: /*practitioner*/ return new String[] {"Reference"}; 1917 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1918 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1919 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1920 case 1901043637: /*location*/ return new String[] {"Reference"}; 1921 case 1289661064: /*healthcareService*/ return new String[] {"Reference"}; 1922 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1923 case 1873069366: /*availableTime*/ return new String[] {}; 1924 case -629572298: /*notAvailable*/ return new String[] {}; 1925 case -1149143617: /*availabilityExceptions*/ return new String[] {"string"}; 1926 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1927 default: return super.getTypesForProperty(hash, name); 1928 } 1929 1930 } 1931 1932 @Override 1933 public Base addChild(String name) throws FHIRException { 1934 if (name.equals("identifier")) { 1935 return addIdentifier(); 1936 } 1937 else if (name.equals("active")) { 1938 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.active"); 1939 } 1940 else if (name.equals("period")) { 1941 this.period = new Period(); 1942 return this.period; 1943 } 1944 else if (name.equals("practitioner")) { 1945 this.practitioner = new Reference(); 1946 return this.practitioner; 1947 } 1948 else if (name.equals("organization")) { 1949 this.organization = new Reference(); 1950 return this.organization; 1951 } 1952 else if (name.equals("code")) { 1953 return addCode(); 1954 } 1955 else if (name.equals("specialty")) { 1956 return addSpecialty(); 1957 } 1958 else if (name.equals("location")) { 1959 return addLocation(); 1960 } 1961 else if (name.equals("healthcareService")) { 1962 return addHealthcareService(); 1963 } 1964 else if (name.equals("telecom")) { 1965 return addTelecom(); 1966 } 1967 else if (name.equals("availableTime")) { 1968 return addAvailableTime(); 1969 } 1970 else if (name.equals("notAvailable")) { 1971 return addNotAvailable(); 1972 } 1973 else if (name.equals("availabilityExceptions")) { 1974 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availabilityExceptions"); 1975 } 1976 else if (name.equals("endpoint")) { 1977 return addEndpoint(); 1978 } 1979 else 1980 return super.addChild(name); 1981 } 1982 1983 public String fhirType() { 1984 return "PractitionerRole"; 1985 1986 } 1987 1988 public PractitionerRole copy() { 1989 PractitionerRole dst = new PractitionerRole(); 1990 copyValues(dst); 1991 if (identifier != null) { 1992 dst.identifier = new ArrayList<Identifier>(); 1993 for (Identifier i : identifier) 1994 dst.identifier.add(i.copy()); 1995 }; 1996 dst.active = active == null ? null : active.copy(); 1997 dst.period = period == null ? null : period.copy(); 1998 dst.practitioner = practitioner == null ? null : practitioner.copy(); 1999 dst.organization = organization == null ? null : organization.copy(); 2000 if (code != null) { 2001 dst.code = new ArrayList<CodeableConcept>(); 2002 for (CodeableConcept i : code) 2003 dst.code.add(i.copy()); 2004 }; 2005 if (specialty != null) { 2006 dst.specialty = new ArrayList<CodeableConcept>(); 2007 for (CodeableConcept i : specialty) 2008 dst.specialty.add(i.copy()); 2009 }; 2010 if (location != null) { 2011 dst.location = new ArrayList<Reference>(); 2012 for (Reference i : location) 2013 dst.location.add(i.copy()); 2014 }; 2015 if (healthcareService != null) { 2016 dst.healthcareService = new ArrayList<Reference>(); 2017 for (Reference i : healthcareService) 2018 dst.healthcareService.add(i.copy()); 2019 }; 2020 if (telecom != null) { 2021 dst.telecom = new ArrayList<ContactPoint>(); 2022 for (ContactPoint i : telecom) 2023 dst.telecom.add(i.copy()); 2024 }; 2025 if (availableTime != null) { 2026 dst.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 2027 for (PractitionerRoleAvailableTimeComponent i : availableTime) 2028 dst.availableTime.add(i.copy()); 2029 }; 2030 if (notAvailable != null) { 2031 dst.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 2032 for (PractitionerRoleNotAvailableComponent i : notAvailable) 2033 dst.notAvailable.add(i.copy()); 2034 }; 2035 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2036 if (endpoint != null) { 2037 dst.endpoint = new ArrayList<Reference>(); 2038 for (Reference i : endpoint) 2039 dst.endpoint.add(i.copy()); 2040 }; 2041 return dst; 2042 } 2043 2044 protected PractitionerRole typedCopy() { 2045 return copy(); 2046 } 2047 2048 @Override 2049 public boolean equalsDeep(Base other_) { 2050 if (!super.equalsDeep(other_)) 2051 return false; 2052 if (!(other_ instanceof PractitionerRole)) 2053 return false; 2054 PractitionerRole o = (PractitionerRole) other_; 2055 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(period, o.period, true) 2056 && compareDeep(practitioner, o.practitioner, true) && compareDeep(organization, o.organization, true) 2057 && compareDeep(code, o.code, true) && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 2058 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(telecom, o.telecom, true) 2059 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 2060 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) && compareDeep(endpoint, o.endpoint, true) 2061 ; 2062 } 2063 2064 @Override 2065 public boolean equalsShallow(Base other_) { 2066 if (!super.equalsShallow(other_)) 2067 return false; 2068 if (!(other_ instanceof PractitionerRole)) 2069 return false; 2070 PractitionerRole o = (PractitionerRole) other_; 2071 return compareValues(active, o.active, true) && compareValues(availabilityExceptions, o.availabilityExceptions, true) 2072 ; 2073 } 2074 2075 public boolean isEmpty() { 2076 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period 2077 , practitioner, organization, code, specialty, location, healthcareService, telecom 2078 , availableTime, notAvailable, availabilityExceptions, endpoint); 2079 } 2080 2081 @Override 2082 public ResourceType getResourceType() { 2083 return ResourceType.PractitionerRole; 2084 } 2085 2086 /** 2087 * Search parameter: <b>date</b> 2088 * <p> 2089 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 2090 * Type: <b>date</b><br> 2091 * Path: <b>PractitionerRole.period</b><br> 2092 * </p> 2093 */ 2094 @SearchParamDefinition(name="date", path="PractitionerRole.period", description="The period during which the practitioner is authorized to perform in these role(s)", type="date" ) 2095 public static final String SP_DATE = "date"; 2096 /** 2097 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2098 * <p> 2099 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 2100 * Type: <b>date</b><br> 2101 * Path: <b>PractitionerRole.period</b><br> 2102 * </p> 2103 */ 2104 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2105 2106 /** 2107 * Search parameter: <b>identifier</b> 2108 * <p> 2109 * Description: <b>A practitioner's Identifier</b><br> 2110 * Type: <b>token</b><br> 2111 * Path: <b>PractitionerRole.identifier</b><br> 2112 * </p> 2113 */ 2114 @SearchParamDefinition(name="identifier", path="PractitionerRole.identifier", description="A practitioner's Identifier", type="token" ) 2115 public static final String SP_IDENTIFIER = "identifier"; 2116 /** 2117 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2118 * <p> 2119 * Description: <b>A practitioner's Identifier</b><br> 2120 * Type: <b>token</b><br> 2121 * Path: <b>PractitionerRole.identifier</b><br> 2122 * </p> 2123 */ 2124 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2125 2126 /** 2127 * Search parameter: <b>specialty</b> 2128 * <p> 2129 * Description: <b>The practitioner has this specialty at an organization</b><br> 2130 * Type: <b>token</b><br> 2131 * Path: <b>PractitionerRole.specialty</b><br> 2132 * </p> 2133 */ 2134 @SearchParamDefinition(name="specialty", path="PractitionerRole.specialty", description="The practitioner has this specialty at an organization", type="token" ) 2135 public static final String SP_SPECIALTY = "specialty"; 2136 /** 2137 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2138 * <p> 2139 * Description: <b>The practitioner has this specialty at an organization</b><br> 2140 * Type: <b>token</b><br> 2141 * Path: <b>PractitionerRole.specialty</b><br> 2142 * </p> 2143 */ 2144 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 2145 2146 /** 2147 * Search parameter: <b>role</b> 2148 * <p> 2149 * Description: <b>The practitioner can perform this role at for the organization</b><br> 2150 * Type: <b>token</b><br> 2151 * Path: <b>PractitionerRole.code</b><br> 2152 * </p> 2153 */ 2154 @SearchParamDefinition(name="role", path="PractitionerRole.code", description="The practitioner can perform this role at for the organization", type="token" ) 2155 public static final String SP_ROLE = "role"; 2156 /** 2157 * <b>Fluent Client</b> search parameter constant for <b>role</b> 2158 * <p> 2159 * Description: <b>The practitioner can perform this role at for the organization</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>PractitionerRole.code</b><br> 2162 * </p> 2163 */ 2164 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROLE); 2165 2166 /** 2167 * Search parameter: <b>practitioner</b> 2168 * <p> 2169 * Description: <b>Practitioner that is able to provide the defined services for the organation</b><br> 2170 * Type: <b>reference</b><br> 2171 * Path: <b>PractitionerRole.practitioner</b><br> 2172 * </p> 2173 */ 2174 @SearchParamDefinition(name="practitioner", path="PractitionerRole.practitioner", description="Practitioner that is able to provide the defined services for the organation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2175 public static final String SP_PRACTITIONER = "practitioner"; 2176 /** 2177 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 2178 * <p> 2179 * Description: <b>Practitioner that is able to provide the defined services for the organation</b><br> 2180 * Type: <b>reference</b><br> 2181 * Path: <b>PractitionerRole.practitioner</b><br> 2182 * </p> 2183 */ 2184 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 2185 2186/** 2187 * Constant for fluent queries to be used to add include statements. Specifies 2188 * the path value of "<b>PractitionerRole:practitioner</b>". 2189 */ 2190 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("PractitionerRole:practitioner").toLocked(); 2191 2192 /** 2193 * Search parameter: <b>active</b> 2194 * <p> 2195 * Description: <b>Whether this practitioner's record is in active use</b><br> 2196 * Type: <b>token</b><br> 2197 * Path: <b>PractitionerRole.active</b><br> 2198 * </p> 2199 */ 2200 @SearchParamDefinition(name="active", path="PractitionerRole.active", description="Whether this practitioner's record is in active use", type="token" ) 2201 public static final String SP_ACTIVE = "active"; 2202 /** 2203 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2204 * <p> 2205 * Description: <b>Whether this practitioner's record is in active use</b><br> 2206 * Type: <b>token</b><br> 2207 * Path: <b>PractitionerRole.active</b><br> 2208 * </p> 2209 */ 2210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 2211 2212 /** 2213 * Search parameter: <b>endpoint</b> 2214 * <p> 2215 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 2216 * Type: <b>reference</b><br> 2217 * Path: <b>PractitionerRole.endpoint</b><br> 2218 * </p> 2219 */ 2220 @SearchParamDefinition(name="endpoint", path="PractitionerRole.endpoint", description="Technical endpoints providing access to services operated for the practitioner with this role", type="reference", target={Endpoint.class } ) 2221 public static final String SP_ENDPOINT = "endpoint"; 2222 /** 2223 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2224 * <p> 2225 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 2226 * Type: <b>reference</b><br> 2227 * Path: <b>PractitionerRole.endpoint</b><br> 2228 * </p> 2229 */ 2230 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 2231 2232/** 2233 * Constant for fluent queries to be used to add include statements. Specifies 2234 * the path value of "<b>PractitionerRole:endpoint</b>". 2235 */ 2236 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("PractitionerRole:endpoint").toLocked(); 2237 2238 /** 2239 * Search parameter: <b>phone</b> 2240 * <p> 2241 * Description: <b>A value in a phone contact</b><br> 2242 * Type: <b>token</b><br> 2243 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2244 * </p> 2245 */ 2246 @SearchParamDefinition(name="phone", path="PractitionerRole.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 2247 public static final String SP_PHONE = "phone"; 2248 /** 2249 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2250 * <p> 2251 * Description: <b>A value in a phone contact</b><br> 2252 * Type: <b>token</b><br> 2253 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2254 * </p> 2255 */ 2256 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 2257 2258 /** 2259 * Search parameter: <b>service</b> 2260 * <p> 2261 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 2262 * Type: <b>reference</b><br> 2263 * Path: <b>PractitionerRole.healthcareService</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name="service", path="PractitionerRole.healthcareService", description="The list of healthcare services that this worker provides for this role's Organization/Location(s)", type="reference", target={HealthcareService.class } ) 2267 public static final String SP_SERVICE = "service"; 2268 /** 2269 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2270 * <p> 2271 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 2272 * Type: <b>reference</b><br> 2273 * Path: <b>PractitionerRole.healthcareService</b><br> 2274 * </p> 2275 */ 2276 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 2277 2278/** 2279 * Constant for fluent queries to be used to add include statements. Specifies 2280 * the path value of "<b>PractitionerRole:service</b>". 2281 */ 2282 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("PractitionerRole:service").toLocked(); 2283 2284 /** 2285 * Search parameter: <b>organization</b> 2286 * <p> 2287 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 2288 * Type: <b>reference</b><br> 2289 * Path: <b>PractitionerRole.organization</b><br> 2290 * </p> 2291 */ 2292 @SearchParamDefinition(name="organization", path="PractitionerRole.organization", description="The identity of the organization the practitioner represents / acts on behalf of", type="reference", target={Organization.class } ) 2293 public static final String SP_ORGANIZATION = "organization"; 2294 /** 2295 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2296 * <p> 2297 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 2298 * Type: <b>reference</b><br> 2299 * Path: <b>PractitionerRole.organization</b><br> 2300 * </p> 2301 */ 2302 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2303 2304/** 2305 * Constant for fluent queries to be used to add include statements. Specifies 2306 * the path value of "<b>PractitionerRole:organization</b>". 2307 */ 2308 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:organization").toLocked(); 2309 2310 /** 2311 * Search parameter: <b>telecom</b> 2312 * <p> 2313 * Description: <b>The value in any kind of contact</b><br> 2314 * Type: <b>token</b><br> 2315 * Path: <b>PractitionerRole.telecom</b><br> 2316 * </p> 2317 */ 2318 @SearchParamDefinition(name="telecom", path="PractitionerRole.telecom", description="The value in any kind of contact", type="token" ) 2319 public static final String SP_TELECOM = "telecom"; 2320 /** 2321 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2322 * <p> 2323 * Description: <b>The value in any kind of contact</b><br> 2324 * Type: <b>token</b><br> 2325 * Path: <b>PractitionerRole.telecom</b><br> 2326 * </p> 2327 */ 2328 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2329 2330 /** 2331 * Search parameter: <b>location</b> 2332 * <p> 2333 * Description: <b>One of the locations at which this practitioner provides care</b><br> 2334 * Type: <b>reference</b><br> 2335 * Path: <b>PractitionerRole.location</b><br> 2336 * </p> 2337 */ 2338 @SearchParamDefinition(name="location", path="PractitionerRole.location", description="One of the locations at which this practitioner provides care", type="reference", target={Location.class } ) 2339 public static final String SP_LOCATION = "location"; 2340 /** 2341 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2342 * <p> 2343 * Description: <b>One of the locations at which this practitioner provides care</b><br> 2344 * Type: <b>reference</b><br> 2345 * Path: <b>PractitionerRole.location</b><br> 2346 * </p> 2347 */ 2348 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2349 2350/** 2351 * Constant for fluent queries to be used to add include statements. Specifies 2352 * the path value of "<b>PractitionerRole:location</b>". 2353 */ 2354 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:location").toLocked(); 2355 2356 /** 2357 * Search parameter: <b>email</b> 2358 * <p> 2359 * Description: <b>A value in an email contact</b><br> 2360 * Type: <b>token</b><br> 2361 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2362 * </p> 2363 */ 2364 @SearchParamDefinition(name="email", path="PractitionerRole.telecom.where(system='email')", description="A value in an email contact", type="token" ) 2365 public static final String SP_EMAIL = "email"; 2366 /** 2367 * <b>Fluent Client</b> search parameter constant for <b>email</b> 2368 * <p> 2369 * Description: <b>A value in an email contact</b><br> 2370 * Type: <b>token</b><br> 2371 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2372 * </p> 2373 */ 2374 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 2375 2376 2377}