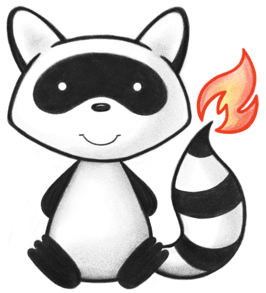
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 050 */ 051@ResourceDef(name="PractitionerRole", profile="http://hl7.org/fhir/Profile/PractitionerRole") 052public class PractitionerRole extends DomainResource { 053 054 public enum DaysOfWeek { 055 /** 056 * Monday 057 */ 058 MON, 059 /** 060 * Tuesday 061 */ 062 TUE, 063 /** 064 * Wednesday 065 */ 066 WED, 067 /** 068 * Thursday 069 */ 070 THU, 071 /** 072 * Friday 073 */ 074 FRI, 075 /** 076 * Saturday 077 */ 078 SAT, 079 /** 080 * Sunday 081 */ 082 SUN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("mon".equals(codeString)) 091 return MON; 092 if ("tue".equals(codeString)) 093 return TUE; 094 if ("wed".equals(codeString)) 095 return WED; 096 if ("thu".equals(codeString)) 097 return THU; 098 if ("fri".equals(codeString)) 099 return FRI; 100 if ("sat".equals(codeString)) 101 return SAT; 102 if ("sun".equals(codeString)) 103 return SUN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case MON: return "mon"; 112 case TUE: return "tue"; 113 case WED: return "wed"; 114 case THU: return "thu"; 115 case FRI: return "fri"; 116 case SAT: return "sat"; 117 case SUN: return "sun"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case MON: return "http://hl7.org/fhir/days-of-week"; 125 case TUE: return "http://hl7.org/fhir/days-of-week"; 126 case WED: return "http://hl7.org/fhir/days-of-week"; 127 case THU: return "http://hl7.org/fhir/days-of-week"; 128 case FRI: return "http://hl7.org/fhir/days-of-week"; 129 case SAT: return "http://hl7.org/fhir/days-of-week"; 130 case SUN: return "http://hl7.org/fhir/days-of-week"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case MON: return "Monday"; 138 case TUE: return "Tuesday"; 139 case WED: return "Wednesday"; 140 case THU: return "Thursday"; 141 case FRI: return "Friday"; 142 case SAT: return "Saturday"; 143 case SUN: return "Sunday"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case MON: return "Monday"; 151 case TUE: return "Tuesday"; 152 case WED: return "Wednesday"; 153 case THU: return "Thursday"; 154 case FRI: return "Friday"; 155 case SAT: return "Saturday"; 156 case SUN: return "Sunday"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 164 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("mon".equals(codeString)) 169 return DaysOfWeek.MON; 170 if ("tue".equals(codeString)) 171 return DaysOfWeek.TUE; 172 if ("wed".equals(codeString)) 173 return DaysOfWeek.WED; 174 if ("thu".equals(codeString)) 175 return DaysOfWeek.THU; 176 if ("fri".equals(codeString)) 177 return DaysOfWeek.FRI; 178 if ("sat".equals(codeString)) 179 return DaysOfWeek.SAT; 180 if ("sun".equals(codeString)) 181 return DaysOfWeek.SUN; 182 throw new IllegalArgumentException("Unknown DaysOfWeek code '"+codeString+"'"); 183 } 184 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<DaysOfWeek>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("mon".equals(codeString)) 193 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON); 194 if ("tue".equals(codeString)) 195 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE); 196 if ("wed".equals(codeString)) 197 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED); 198 if ("thu".equals(codeString)) 199 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU); 200 if ("fri".equals(codeString)) 201 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI); 202 if ("sat".equals(codeString)) 203 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT); 204 if ("sun".equals(codeString)) 205 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN); 206 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 207 } 208 public String toCode(DaysOfWeek code) { 209 if (code == DaysOfWeek.NULL) 210 return null; 211 if (code == DaysOfWeek.MON) 212 return "mon"; 213 if (code == DaysOfWeek.TUE) 214 return "tue"; 215 if (code == DaysOfWeek.WED) 216 return "wed"; 217 if (code == DaysOfWeek.THU) 218 return "thu"; 219 if (code == DaysOfWeek.FRI) 220 return "fri"; 221 if (code == DaysOfWeek.SAT) 222 return "sat"; 223 if (code == DaysOfWeek.SUN) 224 return "sun"; 225 return "?"; 226 } 227 public String toSystem(DaysOfWeek code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class PractitionerRoleAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * Indicates which days of the week are available between the start and end Times. 236 */ 237 @Child(name = "daysOfWeek", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 238 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="Indicates which days of the week are available between the start and end Times." ) 239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 240 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 241 242 /** 243 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 244 */ 245 @Child(name = "allDay", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 246 @Description(shortDefinition="Always available? e.g. 24 hour service", formalDefinition="Is this always available? (hence times are irrelevant) e.g. 24 hour service." ) 247 protected BooleanType allDay; 248 249 /** 250 * The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 251 */ 252 @Child(name = "availableStartTime", type = {TimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 253 @Description(shortDefinition="Opening time of day (ignored if allDay = true)", formalDefinition="The opening time of day. Note: If the AllDay flag is set, then this time is ignored." ) 254 protected TimeType availableStartTime; 255 256 /** 257 * The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 258 */ 259 @Child(name = "availableEndTime", type = {TimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 260 @Description(shortDefinition="Closing time of day (ignored if allDay = true)", formalDefinition="The closing time of day. Note: If the AllDay flag is set, then this time is ignored." ) 261 protected TimeType availableEndTime; 262 263 private static final long serialVersionUID = -2139510127L; 264 265 /** 266 * Constructor 267 */ 268 public PractitionerRoleAvailableTimeComponent() { 269 super(); 270 } 271 272 /** 273 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 274 */ 275 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 276 if (this.daysOfWeek == null) 277 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 278 return this.daysOfWeek; 279 } 280 281 /** 282 * @return Returns a reference to <code>this</code> for easy method chaining 283 */ 284 public PractitionerRoleAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 285 this.daysOfWeek = theDaysOfWeek; 286 return this; 287 } 288 289 public boolean hasDaysOfWeek() { 290 if (this.daysOfWeek == null) 291 return false; 292 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 293 if (!item.isEmpty()) 294 return true; 295 return false; 296 } 297 298 /** 299 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 300 */ 301 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {//2 302 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 303 if (this.daysOfWeek == null) 304 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 305 this.daysOfWeek.add(t); 306 return t; 307 } 308 309 /** 310 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 311 */ 312 public PractitionerRoleAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { //1 313 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 314 t.setValue(value); 315 if (this.daysOfWeek == null) 316 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 317 this.daysOfWeek.add(t); 318 return this; 319 } 320 321 /** 322 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 323 */ 324 public boolean hasDaysOfWeek(DaysOfWeek value) { 325 if (this.daysOfWeek == null) 326 return false; 327 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 328 if (v.getValue().equals(value)) // code 329 return true; 330 return false; 331 } 332 333 /** 334 * @return {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 335 */ 336 public BooleanType getAllDayElement() { 337 if (this.allDay == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.allDay"); 340 else if (Configuration.doAutoCreate()) 341 this.allDay = new BooleanType(); // bb 342 return this.allDay; 343 } 344 345 public boolean hasAllDayElement() { 346 return this.allDay != null && !this.allDay.isEmpty(); 347 } 348 349 public boolean hasAllDay() { 350 return this.allDay != null && !this.allDay.isEmpty(); 351 } 352 353 /** 354 * @param value {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 355 */ 356 public PractitionerRoleAvailableTimeComponent setAllDayElement(BooleanType value) { 357 this.allDay = value; 358 return this; 359 } 360 361 /** 362 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour service. 363 */ 364 public boolean getAllDay() { 365 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 366 } 367 368 /** 369 * @param value Is this always available? (hence times are irrelevant) e.g. 24 hour service. 370 */ 371 public PractitionerRoleAvailableTimeComponent setAllDay(boolean value) { 372 if (this.allDay == null) 373 this.allDay = new BooleanType(); 374 this.allDay.setValue(value); 375 return this; 376 } 377 378 /** 379 * @return {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 380 */ 381 public TimeType getAvailableStartTimeElement() { 382 if (this.availableStartTime == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableStartTime"); 385 else if (Configuration.doAutoCreate()) 386 this.availableStartTime = new TimeType(); // bb 387 return this.availableStartTime; 388 } 389 390 public boolean hasAvailableStartTimeElement() { 391 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 392 } 393 394 public boolean hasAvailableStartTime() { 395 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 400 */ 401 public PractitionerRoleAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 402 this.availableStartTime = value; 403 return this; 404 } 405 406 /** 407 * @return The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 408 */ 409 public String getAvailableStartTime() { 410 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 411 } 412 413 /** 414 * @param value The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 415 */ 416 public PractitionerRoleAvailableTimeComponent setAvailableStartTime(String value) { 417 if (value == null) 418 this.availableStartTime = null; 419 else { 420 if (this.availableStartTime == null) 421 this.availableStartTime = new TimeType(); 422 this.availableStartTime.setValue(value); 423 } 424 return this; 425 } 426 427 /** 428 * @return {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 429 */ 430 public TimeType getAvailableEndTimeElement() { 431 if (this.availableEndTime == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableEndTime"); 434 else if (Configuration.doAutoCreate()) 435 this.availableEndTime = new TimeType(); // bb 436 return this.availableEndTime; 437 } 438 439 public boolean hasAvailableEndTimeElement() { 440 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 441 } 442 443 public boolean hasAvailableEndTime() { 444 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 449 */ 450 public PractitionerRoleAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 451 this.availableEndTime = value; 452 return this; 453 } 454 455 /** 456 * @return The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 457 */ 458 public String getAvailableEndTime() { 459 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 460 } 461 462 /** 463 * @param value The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 464 */ 465 public PractitionerRoleAvailableTimeComponent setAvailableEndTime(String value) { 466 if (value == null) 467 this.availableEndTime = null; 468 else { 469 if (this.availableEndTime == null) 470 this.availableEndTime = new TimeType(); 471 this.availableEndTime.setValue(value); 472 } 473 return this; 474 } 475 476 protected void listChildren(List<Property> children) { 477 super.listChildren(children); 478 children.add(new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek)); 479 children.add(new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 480 children.add(new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime)); 481 children.add(new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime)); 482 } 483 484 @Override 485 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 486 switch (_hash) { 487 case 68050338: /*daysOfWeek*/ return new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek); 488 case -1414913477: /*allDay*/ return new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 489 case -1039453818: /*availableStartTime*/ return new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime); 490 case 101151551: /*availableEndTime*/ return new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime); 491 default: return super.getNamedProperty(_hash, _name, _checkValid); 492 } 493 494 } 495 496 @Override 497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 498 switch (hash) { 499 case 68050338: /*daysOfWeek*/ return this.daysOfWeek == null ? new Base[0] : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 500 case -1414913477: /*allDay*/ return this.allDay == null ? new Base[0] : new Base[] {this.allDay}; // BooleanType 501 case -1039453818: /*availableStartTime*/ return this.availableStartTime == null ? new Base[0] : new Base[] {this.availableStartTime}; // TimeType 502 case 101151551: /*availableEndTime*/ return this.availableEndTime == null ? new Base[0] : new Base[] {this.availableEndTime}; // TimeType 503 default: return super.getProperty(hash, name, checkValid); 504 } 505 506 } 507 508 @Override 509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 510 switch (hash) { 511 case 68050338: // daysOfWeek 512 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 513 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 514 return value; 515 case -1414913477: // allDay 516 this.allDay = castToBoolean(value); // BooleanType 517 return value; 518 case -1039453818: // availableStartTime 519 this.availableStartTime = castToTime(value); // TimeType 520 return value; 521 case 101151551: // availableEndTime 522 this.availableEndTime = castToTime(value); // TimeType 523 return value; 524 default: return super.setProperty(hash, name, value); 525 } 526 527 } 528 529 @Override 530 public Base setProperty(String name, Base value) throws FHIRException { 531 if (name.equals("daysOfWeek")) { 532 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 533 this.getDaysOfWeek().add((Enumeration) value); 534 } else if (name.equals("allDay")) { 535 this.allDay = castToBoolean(value); // BooleanType 536 } else if (name.equals("availableStartTime")) { 537 this.availableStartTime = castToTime(value); // TimeType 538 } else if (name.equals("availableEndTime")) { 539 this.availableEndTime = castToTime(value); // TimeType 540 } else 541 return super.setProperty(name, value); 542 return value; 543 } 544 545 @Override 546 public Base makeProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 case 68050338: return addDaysOfWeekElement(); 549 case -1414913477: return getAllDayElement(); 550 case -1039453818: return getAvailableStartTimeElement(); 551 case 101151551: return getAvailableEndTimeElement(); 552 default: return super.makeProperty(hash, name); 553 } 554 555 } 556 557 @Override 558 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 559 switch (hash) { 560 case 68050338: /*daysOfWeek*/ return new String[] {"code"}; 561 case -1414913477: /*allDay*/ return new String[] {"boolean"}; 562 case -1039453818: /*availableStartTime*/ return new String[] {"time"}; 563 case 101151551: /*availableEndTime*/ return new String[] {"time"}; 564 default: return super.getTypesForProperty(hash, name); 565 } 566 567 } 568 569 @Override 570 public Base addChild(String name) throws FHIRException { 571 if (name.equals("daysOfWeek")) { 572 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.daysOfWeek"); 573 } 574 else if (name.equals("allDay")) { 575 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.allDay"); 576 } 577 else if (name.equals("availableStartTime")) { 578 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableStartTime"); 579 } 580 else if (name.equals("availableEndTime")) { 581 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableEndTime"); 582 } 583 else 584 return super.addChild(name); 585 } 586 587 public PractitionerRoleAvailableTimeComponent copy() { 588 PractitionerRoleAvailableTimeComponent dst = new PractitionerRoleAvailableTimeComponent(); 589 copyValues(dst); 590 if (daysOfWeek != null) { 591 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 592 for (Enumeration<DaysOfWeek> i : daysOfWeek) 593 dst.daysOfWeek.add(i.copy()); 594 }; 595 dst.allDay = allDay == null ? null : allDay.copy(); 596 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 597 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 598 return dst; 599 } 600 601 @Override 602 public boolean equalsDeep(Base other_) { 603 if (!super.equalsDeep(other_)) 604 return false; 605 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 606 return false; 607 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 608 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) && compareDeep(availableStartTime, o.availableStartTime, true) 609 && compareDeep(availableEndTime, o.availableEndTime, true); 610 } 611 612 @Override 613 public boolean equalsShallow(Base other_) { 614 if (!super.equalsShallow(other_)) 615 return false; 616 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 617 return false; 618 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 619 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) && compareValues(availableStartTime, o.availableStartTime, true) 620 && compareValues(availableEndTime, o.availableEndTime, true); 621 } 622 623 public boolean isEmpty() { 624 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime 625 , availableEndTime); 626 } 627 628 public String fhirType() { 629 return "PractitionerRole.availableTime"; 630 631 } 632 633 } 634 635 @Block() 636 public static class PractitionerRoleNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 637 /** 638 * The reason that can be presented to the user as to why this time is not available. 639 */ 640 @Child(name = "description", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 641 @Description(shortDefinition="Reason presented to the user explaining why time not available", formalDefinition="The reason that can be presented to the user as to why this time is not available." ) 642 protected StringType description; 643 644 /** 645 * Service is not available (seasonally or for a public holiday) from this date. 646 */ 647 @Child(name = "during", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 648 @Description(shortDefinition="Service not availablefrom this date", formalDefinition="Service is not available (seasonally or for a public holiday) from this date." ) 649 protected Period during; 650 651 private static final long serialVersionUID = 310849929L; 652 653 /** 654 * Constructor 655 */ 656 public PractitionerRoleNotAvailableComponent() { 657 super(); 658 } 659 660 /** 661 * Constructor 662 */ 663 public PractitionerRoleNotAvailableComponent(StringType description) { 664 super(); 665 this.description = description; 666 } 667 668 /** 669 * @return {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 670 */ 671 public StringType getDescriptionElement() { 672 if (this.description == null) 673 if (Configuration.errorOnAutoCreate()) 674 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.description"); 675 else if (Configuration.doAutoCreate()) 676 this.description = new StringType(); // bb 677 return this.description; 678 } 679 680 public boolean hasDescriptionElement() { 681 return this.description != null && !this.description.isEmpty(); 682 } 683 684 public boolean hasDescription() { 685 return this.description != null && !this.description.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 690 */ 691 public PractitionerRoleNotAvailableComponent setDescriptionElement(StringType value) { 692 this.description = value; 693 return this; 694 } 695 696 /** 697 * @return The reason that can be presented to the user as to why this time is not available. 698 */ 699 public String getDescription() { 700 return this.description == null ? null : this.description.getValue(); 701 } 702 703 /** 704 * @param value The reason that can be presented to the user as to why this time is not available. 705 */ 706 public PractitionerRoleNotAvailableComponent setDescription(String value) { 707 if (this.description == null) 708 this.description = new StringType(); 709 this.description.setValue(value); 710 return this; 711 } 712 713 /** 714 * @return {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 715 */ 716 public Period getDuring() { 717 if (this.during == null) 718 if (Configuration.errorOnAutoCreate()) 719 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.during"); 720 else if (Configuration.doAutoCreate()) 721 this.during = new Period(); // cc 722 return this.during; 723 } 724 725 public boolean hasDuring() { 726 return this.during != null && !this.during.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 731 */ 732 public PractitionerRoleNotAvailableComponent setDuring(Period value) { 733 this.during = value; 734 return this; 735 } 736 737 protected void listChildren(List<Property> children) { 738 super.listChildren(children); 739 children.add(new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 740 children.add(new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 741 } 742 743 @Override 744 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 745 switch (_hash) { 746 case -1724546052: /*description*/ return new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 747 case -1320499647: /*during*/ return new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 748 default: return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 757 case -1320499647: /*during*/ return this.during == null ? new Base[0] : new Base[] {this.during}; // Period 758 default: return super.getProperty(hash, name, checkValid); 759 } 760 761 } 762 763 @Override 764 public Base setProperty(int hash, String name, Base value) throws FHIRException { 765 switch (hash) { 766 case -1724546052: // description 767 this.description = castToString(value); // StringType 768 return value; 769 case -1320499647: // during 770 this.during = castToPeriod(value); // Period 771 return value; 772 default: return super.setProperty(hash, name, value); 773 } 774 775 } 776 777 @Override 778 public Base setProperty(String name, Base value) throws FHIRException { 779 if (name.equals("description")) { 780 this.description = castToString(value); // StringType 781 } else if (name.equals("during")) { 782 this.during = castToPeriod(value); // Period 783 } else 784 return super.setProperty(name, value); 785 return value; 786 } 787 788 @Override 789 public Base makeProperty(int hash, String name) throws FHIRException { 790 switch (hash) { 791 case -1724546052: return getDescriptionElement(); 792 case -1320499647: return getDuring(); 793 default: return super.makeProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 800 switch (hash) { 801 case -1724546052: /*description*/ return new String[] {"string"}; 802 case -1320499647: /*during*/ return new String[] {"Period"}; 803 default: return super.getTypesForProperty(hash, name); 804 } 805 806 } 807 808 @Override 809 public Base addChild(String name) throws FHIRException { 810 if (name.equals("description")) { 811 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.description"); 812 } 813 else if (name.equals("during")) { 814 this.during = new Period(); 815 return this.during; 816 } 817 else 818 return super.addChild(name); 819 } 820 821 public PractitionerRoleNotAvailableComponent copy() { 822 PractitionerRoleNotAvailableComponent dst = new PractitionerRoleNotAvailableComponent(); 823 copyValues(dst); 824 dst.description = description == null ? null : description.copy(); 825 dst.during = during == null ? null : during.copy(); 826 return dst; 827 } 828 829 @Override 830 public boolean equalsDeep(Base other_) { 831 if (!super.equalsDeep(other_)) 832 return false; 833 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 834 return false; 835 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 836 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 837 } 838 839 @Override 840 public boolean equalsShallow(Base other_) { 841 if (!super.equalsShallow(other_)) 842 return false; 843 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 844 return false; 845 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 846 return compareValues(description, o.description, true); 847 } 848 849 public boolean isEmpty() { 850 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 851 } 852 853 public String fhirType() { 854 return "PractitionerRole.notAvailable"; 855 856 } 857 858 } 859 860 /** 861 * Business Identifiers that are specific to a role/location. 862 */ 863 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 864 @Description(shortDefinition="Business Identifiers that are specific to a role/location", formalDefinition="Business Identifiers that are specific to a role/location." ) 865 protected List<Identifier> identifier; 866 867 /** 868 * Whether this practitioner's record is in active use. 869 */ 870 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 871 @Description(shortDefinition="Whether this practitioner's record is in active use", formalDefinition="Whether this practitioner's record is in active use." ) 872 protected BooleanType active; 873 874 /** 875 * The period during which the person is authorized to act as a practitioner in these role(s) for the organization. 876 */ 877 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 878 @Description(shortDefinition="The period during which the practitioner is authorized to perform in these role(s)", formalDefinition="The period during which the person is authorized to act as a practitioner in these role(s) for the organization." ) 879 protected Period period; 880 881 /** 882 * Practitioner that is able to provide the defined services for the organation. 883 */ 884 @Child(name = "practitioner", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=true) 885 @Description(shortDefinition="Practitioner that is able to provide the defined services for the organation", formalDefinition="Practitioner that is able to provide the defined services for the organation." ) 886 protected Reference practitioner; 887 888 /** 889 * The actual object that is the target of the reference (Practitioner that is able to provide the defined services for the organation.) 890 */ 891 protected Practitioner practitionerTarget; 892 893 /** 894 * The organization where the Practitioner performs the roles associated. 895 */ 896 @Child(name = "organization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 897 @Description(shortDefinition="Organization where the roles are available", formalDefinition="The organization where the Practitioner performs the roles associated." ) 898 protected Reference organization; 899 900 /** 901 * The actual object that is the target of the reference (The organization where the Practitioner performs the roles associated.) 902 */ 903 protected Organization organizationTarget; 904 905 /** 906 * Roles which this practitioner is authorized to perform for the organization. 907 */ 908 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 909 @Description(shortDefinition="Roles which this practitioner may perform", formalDefinition="Roles which this practitioner is authorized to perform for the organization." ) 910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/practitioner-role") 911 protected List<CodeableConcept> code; 912 913 /** 914 * Specific specialty of the practitioner. 915 */ 916 @Child(name = "specialty", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 917 @Description(shortDefinition="Specific specialty of the practitioner", formalDefinition="Specific specialty of the practitioner." ) 918 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 919 protected List<CodeableConcept> specialty; 920 921 /** 922 * The location(s) at which this practitioner provides care. 923 */ 924 @Child(name = "location", type = {Location.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 925 @Description(shortDefinition="The location(s) at which this practitioner provides care", formalDefinition="The location(s) at which this practitioner provides care." ) 926 protected List<Reference> location; 927 /** 928 * The actual objects that are the target of the reference (The location(s) at which this practitioner provides care.) 929 */ 930 protected List<Location> locationTarget; 931 932 933 /** 934 * The list of healthcare services that this worker provides for this role's Organization/Location(s). 935 */ 936 @Child(name = "healthcareService", type = {HealthcareService.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 937 @Description(shortDefinition="The list of healthcare services that this worker provides for this role's Organization/Location(s)", formalDefinition="The list of healthcare services that this worker provides for this role's Organization/Location(s)." ) 938 protected List<Reference> healthcareService; 939 /** 940 * The actual objects that are the target of the reference (The list of healthcare services that this worker provides for this role's Organization/Location(s).) 941 */ 942 protected List<HealthcareService> healthcareServiceTarget; 943 944 945 /** 946 * Contact details that are specific to the role/location/service. 947 */ 948 @Child(name = "telecom", type = {ContactPoint.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 949 @Description(shortDefinition="Contact details that are specific to the role/location/service", formalDefinition="Contact details that are specific to the role/location/service." ) 950 protected List<ContactPoint> telecom; 951 952 /** 953 * A collection of times that the Service Site is available. 954 */ 955 @Child(name = "availableTime", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 956 @Description(shortDefinition="Times the Service Site is available", formalDefinition="A collection of times that the Service Site is available." ) 957 protected List<PractitionerRoleAvailableTimeComponent> availableTime; 958 959 /** 960 * The HealthcareService is not available during this period of time due to the provided reason. 961 */ 962 @Child(name = "notAvailable", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 963 @Description(shortDefinition="Not available during this time due to provided reason", formalDefinition="The HealthcareService is not available during this period of time due to the provided reason." ) 964 protected List<PractitionerRoleNotAvailableComponent> notAvailable; 965 966 /** 967 * A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 968 */ 969 @Child(name = "availabilityExceptions", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 970 @Description(shortDefinition="Description of availability exceptions", formalDefinition="A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times." ) 971 protected StringType availabilityExceptions; 972 973 /** 974 * Technical endpoints providing access to services operated for the practitioner with this role. 975 */ 976 @Child(name = "endpoint", type = {Endpoint.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 977 @Description(shortDefinition="Technical endpoints providing access to services operated for the practitioner with this role", formalDefinition="Technical endpoints providing access to services operated for the practitioner with this role." ) 978 protected List<Reference> endpoint; 979 /** 980 * The actual objects that are the target of the reference (Technical endpoints providing access to services operated for the practitioner with this role.) 981 */ 982 protected List<Endpoint> endpointTarget; 983 984 985 private static final long serialVersionUID = 423338051L; 986 987 /** 988 * Constructor 989 */ 990 public PractitionerRole() { 991 super(); 992 } 993 994 /** 995 * @return {@link #identifier} (Business Identifiers that are specific to a role/location.) 996 */ 997 public List<Identifier> getIdentifier() { 998 if (this.identifier == null) 999 this.identifier = new ArrayList<Identifier>(); 1000 return this.identifier; 1001 } 1002 1003 /** 1004 * @return Returns a reference to <code>this</code> for easy method chaining 1005 */ 1006 public PractitionerRole setIdentifier(List<Identifier> theIdentifier) { 1007 this.identifier = theIdentifier; 1008 return this; 1009 } 1010 1011 public boolean hasIdentifier() { 1012 if (this.identifier == null) 1013 return false; 1014 for (Identifier item : this.identifier) 1015 if (!item.isEmpty()) 1016 return true; 1017 return false; 1018 } 1019 1020 public Identifier addIdentifier() { //3 1021 Identifier t = new Identifier(); 1022 if (this.identifier == null) 1023 this.identifier = new ArrayList<Identifier>(); 1024 this.identifier.add(t); 1025 return t; 1026 } 1027 1028 public PractitionerRole addIdentifier(Identifier t) { //3 1029 if (t == null) 1030 return this; 1031 if (this.identifier == null) 1032 this.identifier = new ArrayList<Identifier>(); 1033 this.identifier.add(t); 1034 return this; 1035 } 1036 1037 /** 1038 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1039 */ 1040 public Identifier getIdentifierFirstRep() { 1041 if (getIdentifier().isEmpty()) { 1042 addIdentifier(); 1043 } 1044 return getIdentifier().get(0); 1045 } 1046 1047 /** 1048 * @return {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1049 */ 1050 public BooleanType getActiveElement() { 1051 if (this.active == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create PractitionerRole.active"); 1054 else if (Configuration.doAutoCreate()) 1055 this.active = new BooleanType(); // bb 1056 return this.active; 1057 } 1058 1059 public boolean hasActiveElement() { 1060 return this.active != null && !this.active.isEmpty(); 1061 } 1062 1063 public boolean hasActive() { 1064 return this.active != null && !this.active.isEmpty(); 1065 } 1066 1067 /** 1068 * @param value {@link #active} (Whether this practitioner's record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1069 */ 1070 public PractitionerRole setActiveElement(BooleanType value) { 1071 this.active = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return Whether this practitioner's record is in active use. 1077 */ 1078 public boolean getActive() { 1079 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1080 } 1081 1082 /** 1083 * @param value Whether this practitioner's record is in active use. 1084 */ 1085 public PractitionerRole setActive(boolean value) { 1086 if (this.active == null) 1087 this.active = new BooleanType(); 1088 this.active.setValue(value); 1089 return this; 1090 } 1091 1092 /** 1093 * @return {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 1094 */ 1095 public Period getPeriod() { 1096 if (this.period == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create PractitionerRole.period"); 1099 else if (Configuration.doAutoCreate()) 1100 this.period = new Period(); // cc 1101 return this.period; 1102 } 1103 1104 public boolean hasPeriod() { 1105 return this.period != null && !this.period.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #period} (The period during which the person is authorized to act as a practitioner in these role(s) for the organization.) 1110 */ 1111 public PractitionerRole setPeriod(Period value) { 1112 this.period = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #practitioner} (Practitioner that is able to provide the defined services for the organation.) 1118 */ 1119 public Reference getPractitioner() { 1120 if (this.practitioner == null) 1121 if (Configuration.errorOnAutoCreate()) 1122 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1123 else if (Configuration.doAutoCreate()) 1124 this.practitioner = new Reference(); // cc 1125 return this.practitioner; 1126 } 1127 1128 public boolean hasPractitioner() { 1129 return this.practitioner != null && !this.practitioner.isEmpty(); 1130 } 1131 1132 /** 1133 * @param value {@link #practitioner} (Practitioner that is able to provide the defined services for the organation.) 1134 */ 1135 public PractitionerRole setPractitioner(Reference value) { 1136 this.practitioner = value; 1137 return this; 1138 } 1139 1140 /** 1141 * @return {@link #practitioner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Practitioner that is able to provide the defined services for the organation.) 1142 */ 1143 public Practitioner getPractitionerTarget() { 1144 if (this.practitionerTarget == null) 1145 if (Configuration.errorOnAutoCreate()) 1146 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1147 else if (Configuration.doAutoCreate()) 1148 this.practitionerTarget = new Practitioner(); // aa 1149 return this.practitionerTarget; 1150 } 1151 1152 /** 1153 * @param value {@link #practitioner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Practitioner that is able to provide the defined services for the organation.) 1154 */ 1155 public PractitionerRole setPractitionerTarget(Practitioner value) { 1156 this.practitionerTarget = value; 1157 return this; 1158 } 1159 1160 /** 1161 * @return {@link #organization} (The organization where the Practitioner performs the roles associated.) 1162 */ 1163 public Reference getOrganization() { 1164 if (this.organization == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1167 else if (Configuration.doAutoCreate()) 1168 this.organization = new Reference(); // cc 1169 return this.organization; 1170 } 1171 1172 public boolean hasOrganization() { 1173 return this.organization != null && !this.organization.isEmpty(); 1174 } 1175 1176 /** 1177 * @param value {@link #organization} (The organization where the Practitioner performs the roles associated.) 1178 */ 1179 public PractitionerRole setOrganization(Reference value) { 1180 this.organization = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization where the Practitioner performs the roles associated.) 1186 */ 1187 public Organization getOrganizationTarget() { 1188 if (this.organizationTarget == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1191 else if (Configuration.doAutoCreate()) 1192 this.organizationTarget = new Organization(); // aa 1193 return this.organizationTarget; 1194 } 1195 1196 /** 1197 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization where the Practitioner performs the roles associated.) 1198 */ 1199 public PractitionerRole setOrganizationTarget(Organization value) { 1200 this.organizationTarget = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #code} (Roles which this practitioner is authorized to perform for the organization.) 1206 */ 1207 public List<CodeableConcept> getCode() { 1208 if (this.code == null) 1209 this.code = new ArrayList<CodeableConcept>(); 1210 return this.code; 1211 } 1212 1213 /** 1214 * @return Returns a reference to <code>this</code> for easy method chaining 1215 */ 1216 public PractitionerRole setCode(List<CodeableConcept> theCode) { 1217 this.code = theCode; 1218 return this; 1219 } 1220 1221 public boolean hasCode() { 1222 if (this.code == null) 1223 return false; 1224 for (CodeableConcept item : this.code) 1225 if (!item.isEmpty()) 1226 return true; 1227 return false; 1228 } 1229 1230 public CodeableConcept addCode() { //3 1231 CodeableConcept t = new CodeableConcept(); 1232 if (this.code == null) 1233 this.code = new ArrayList<CodeableConcept>(); 1234 this.code.add(t); 1235 return t; 1236 } 1237 1238 public PractitionerRole addCode(CodeableConcept t) { //3 1239 if (t == null) 1240 return this; 1241 if (this.code == null) 1242 this.code = new ArrayList<CodeableConcept>(); 1243 this.code.add(t); 1244 return this; 1245 } 1246 1247 /** 1248 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1249 */ 1250 public CodeableConcept getCodeFirstRep() { 1251 if (getCode().isEmpty()) { 1252 addCode(); 1253 } 1254 return getCode().get(0); 1255 } 1256 1257 /** 1258 * @return {@link #specialty} (Specific specialty of the practitioner.) 1259 */ 1260 public List<CodeableConcept> getSpecialty() { 1261 if (this.specialty == null) 1262 this.specialty = new ArrayList<CodeableConcept>(); 1263 return this.specialty; 1264 } 1265 1266 /** 1267 * @return Returns a reference to <code>this</code> for easy method chaining 1268 */ 1269 public PractitionerRole setSpecialty(List<CodeableConcept> theSpecialty) { 1270 this.specialty = theSpecialty; 1271 return this; 1272 } 1273 1274 public boolean hasSpecialty() { 1275 if (this.specialty == null) 1276 return false; 1277 for (CodeableConcept item : this.specialty) 1278 if (!item.isEmpty()) 1279 return true; 1280 return false; 1281 } 1282 1283 public CodeableConcept addSpecialty() { //3 1284 CodeableConcept t = new CodeableConcept(); 1285 if (this.specialty == null) 1286 this.specialty = new ArrayList<CodeableConcept>(); 1287 this.specialty.add(t); 1288 return t; 1289 } 1290 1291 public PractitionerRole addSpecialty(CodeableConcept t) { //3 1292 if (t == null) 1293 return this; 1294 if (this.specialty == null) 1295 this.specialty = new ArrayList<CodeableConcept>(); 1296 this.specialty.add(t); 1297 return this; 1298 } 1299 1300 /** 1301 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 1302 */ 1303 public CodeableConcept getSpecialtyFirstRep() { 1304 if (getSpecialty().isEmpty()) { 1305 addSpecialty(); 1306 } 1307 return getSpecialty().get(0); 1308 } 1309 1310 /** 1311 * @return {@link #location} (The location(s) at which this practitioner provides care.) 1312 */ 1313 public List<Reference> getLocation() { 1314 if (this.location == null) 1315 this.location = new ArrayList<Reference>(); 1316 return this.location; 1317 } 1318 1319 /** 1320 * @return Returns a reference to <code>this</code> for easy method chaining 1321 */ 1322 public PractitionerRole setLocation(List<Reference> theLocation) { 1323 this.location = theLocation; 1324 return this; 1325 } 1326 1327 public boolean hasLocation() { 1328 if (this.location == null) 1329 return false; 1330 for (Reference item : this.location) 1331 if (!item.isEmpty()) 1332 return true; 1333 return false; 1334 } 1335 1336 public Reference addLocation() { //3 1337 Reference t = new Reference(); 1338 if (this.location == null) 1339 this.location = new ArrayList<Reference>(); 1340 this.location.add(t); 1341 return t; 1342 } 1343 1344 public PractitionerRole addLocation(Reference t) { //3 1345 if (t == null) 1346 return this; 1347 if (this.location == null) 1348 this.location = new ArrayList<Reference>(); 1349 this.location.add(t); 1350 return this; 1351 } 1352 1353 /** 1354 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist 1355 */ 1356 public Reference getLocationFirstRep() { 1357 if (getLocation().isEmpty()) { 1358 addLocation(); 1359 } 1360 return getLocation().get(0); 1361 } 1362 1363 /** 1364 * @return {@link #healthcareService} (The list of healthcare services that this worker provides for this role's Organization/Location(s).) 1365 */ 1366 public List<Reference> getHealthcareService() { 1367 if (this.healthcareService == null) 1368 this.healthcareService = new ArrayList<Reference>(); 1369 return this.healthcareService; 1370 } 1371 1372 /** 1373 * @return Returns a reference to <code>this</code> for easy method chaining 1374 */ 1375 public PractitionerRole setHealthcareService(List<Reference> theHealthcareService) { 1376 this.healthcareService = theHealthcareService; 1377 return this; 1378 } 1379 1380 public boolean hasHealthcareService() { 1381 if (this.healthcareService == null) 1382 return false; 1383 for (Reference item : this.healthcareService) 1384 if (!item.isEmpty()) 1385 return true; 1386 return false; 1387 } 1388 1389 public Reference addHealthcareService() { //3 1390 Reference t = new Reference(); 1391 if (this.healthcareService == null) 1392 this.healthcareService = new ArrayList<Reference>(); 1393 this.healthcareService.add(t); 1394 return t; 1395 } 1396 1397 public PractitionerRole addHealthcareService(Reference t) { //3 1398 if (t == null) 1399 return this; 1400 if (this.healthcareService == null) 1401 this.healthcareService = new ArrayList<Reference>(); 1402 this.healthcareService.add(t); 1403 return this; 1404 } 1405 1406 /** 1407 * @return The first repetition of repeating field {@link #healthcareService}, creating it if it does not already exist 1408 */ 1409 public Reference getHealthcareServiceFirstRep() { 1410 if (getHealthcareService().isEmpty()) { 1411 addHealthcareService(); 1412 } 1413 return getHealthcareService().get(0); 1414 } 1415 1416 /** 1417 * @return {@link #telecom} (Contact details that are specific to the role/location/service.) 1418 */ 1419 public List<ContactPoint> getTelecom() { 1420 if (this.telecom == null) 1421 this.telecom = new ArrayList<ContactPoint>(); 1422 return this.telecom; 1423 } 1424 1425 /** 1426 * @return Returns a reference to <code>this</code> for easy method chaining 1427 */ 1428 public PractitionerRole setTelecom(List<ContactPoint> theTelecom) { 1429 this.telecom = theTelecom; 1430 return this; 1431 } 1432 1433 public boolean hasTelecom() { 1434 if (this.telecom == null) 1435 return false; 1436 for (ContactPoint item : this.telecom) 1437 if (!item.isEmpty()) 1438 return true; 1439 return false; 1440 } 1441 1442 public ContactPoint addTelecom() { //3 1443 ContactPoint t = new ContactPoint(); 1444 if (this.telecom == null) 1445 this.telecom = new ArrayList<ContactPoint>(); 1446 this.telecom.add(t); 1447 return t; 1448 } 1449 1450 public PractitionerRole addTelecom(ContactPoint t) { //3 1451 if (t == null) 1452 return this; 1453 if (this.telecom == null) 1454 this.telecom = new ArrayList<ContactPoint>(); 1455 this.telecom.add(t); 1456 return this; 1457 } 1458 1459 /** 1460 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 1461 */ 1462 public ContactPoint getTelecomFirstRep() { 1463 if (getTelecom().isEmpty()) { 1464 addTelecom(); 1465 } 1466 return getTelecom().get(0); 1467 } 1468 1469 /** 1470 * @return {@link #availableTime} (A collection of times that the Service Site is available.) 1471 */ 1472 public List<PractitionerRoleAvailableTimeComponent> getAvailableTime() { 1473 if (this.availableTime == null) 1474 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1475 return this.availableTime; 1476 } 1477 1478 /** 1479 * @return Returns a reference to <code>this</code> for easy method chaining 1480 */ 1481 public PractitionerRole setAvailableTime(List<PractitionerRoleAvailableTimeComponent> theAvailableTime) { 1482 this.availableTime = theAvailableTime; 1483 return this; 1484 } 1485 1486 public boolean hasAvailableTime() { 1487 if (this.availableTime == null) 1488 return false; 1489 for (PractitionerRoleAvailableTimeComponent item : this.availableTime) 1490 if (!item.isEmpty()) 1491 return true; 1492 return false; 1493 } 1494 1495 public PractitionerRoleAvailableTimeComponent addAvailableTime() { //3 1496 PractitionerRoleAvailableTimeComponent t = new PractitionerRoleAvailableTimeComponent(); 1497 if (this.availableTime == null) 1498 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1499 this.availableTime.add(t); 1500 return t; 1501 } 1502 1503 public PractitionerRole addAvailableTime(PractitionerRoleAvailableTimeComponent t) { //3 1504 if (t == null) 1505 return this; 1506 if (this.availableTime == null) 1507 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1508 this.availableTime.add(t); 1509 return this; 1510 } 1511 1512 /** 1513 * @return The first repetition of repeating field {@link #availableTime}, creating it if it does not already exist 1514 */ 1515 public PractitionerRoleAvailableTimeComponent getAvailableTimeFirstRep() { 1516 if (getAvailableTime().isEmpty()) { 1517 addAvailableTime(); 1518 } 1519 return getAvailableTime().get(0); 1520 } 1521 1522 /** 1523 * @return {@link #notAvailable} (The HealthcareService is not available during this period of time due to the provided reason.) 1524 */ 1525 public List<PractitionerRoleNotAvailableComponent> getNotAvailable() { 1526 if (this.notAvailable == null) 1527 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1528 return this.notAvailable; 1529 } 1530 1531 /** 1532 * @return Returns a reference to <code>this</code> for easy method chaining 1533 */ 1534 public PractitionerRole setNotAvailable(List<PractitionerRoleNotAvailableComponent> theNotAvailable) { 1535 this.notAvailable = theNotAvailable; 1536 return this; 1537 } 1538 1539 public boolean hasNotAvailable() { 1540 if (this.notAvailable == null) 1541 return false; 1542 for (PractitionerRoleNotAvailableComponent item : this.notAvailable) 1543 if (!item.isEmpty()) 1544 return true; 1545 return false; 1546 } 1547 1548 public PractitionerRoleNotAvailableComponent addNotAvailable() { //3 1549 PractitionerRoleNotAvailableComponent t = new PractitionerRoleNotAvailableComponent(); 1550 if (this.notAvailable == null) 1551 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1552 this.notAvailable.add(t); 1553 return t; 1554 } 1555 1556 public PractitionerRole addNotAvailable(PractitionerRoleNotAvailableComponent t) { //3 1557 if (t == null) 1558 return this; 1559 if (this.notAvailable == null) 1560 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1561 this.notAvailable.add(t); 1562 return this; 1563 } 1564 1565 /** 1566 * @return The first repetition of repeating field {@link #notAvailable}, creating it if it does not already exist 1567 */ 1568 public PractitionerRoleNotAvailableComponent getNotAvailableFirstRep() { 1569 if (getNotAvailable().isEmpty()) { 1570 addNotAvailable(); 1571 } 1572 return getNotAvailable().get(0); 1573 } 1574 1575 /** 1576 * @return {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 1577 */ 1578 public StringType getAvailabilityExceptionsElement() { 1579 if (this.availabilityExceptions == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create PractitionerRole.availabilityExceptions"); 1582 else if (Configuration.doAutoCreate()) 1583 this.availabilityExceptions = new StringType(); // bb 1584 return this.availabilityExceptions; 1585 } 1586 1587 public boolean hasAvailabilityExceptionsElement() { 1588 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1589 } 1590 1591 public boolean hasAvailabilityExceptions() { 1592 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 1597 */ 1598 public PractitionerRole setAvailabilityExceptionsElement(StringType value) { 1599 this.availabilityExceptions = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 1605 */ 1606 public String getAvailabilityExceptions() { 1607 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 1608 } 1609 1610 /** 1611 * @param value A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 1612 */ 1613 public PractitionerRole setAvailabilityExceptions(String value) { 1614 if (Utilities.noString(value)) 1615 this.availabilityExceptions = null; 1616 else { 1617 if (this.availabilityExceptions == null) 1618 this.availabilityExceptions = new StringType(); 1619 this.availabilityExceptions.setValue(value); 1620 } 1621 return this; 1622 } 1623 1624 /** 1625 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the practitioner with this role.) 1626 */ 1627 public List<Reference> getEndpoint() { 1628 if (this.endpoint == null) 1629 this.endpoint = new ArrayList<Reference>(); 1630 return this.endpoint; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public PractitionerRole setEndpoint(List<Reference> theEndpoint) { 1637 this.endpoint = theEndpoint; 1638 return this; 1639 } 1640 1641 public boolean hasEndpoint() { 1642 if (this.endpoint == null) 1643 return false; 1644 for (Reference item : this.endpoint) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public Reference addEndpoint() { //3 1651 Reference t = new Reference(); 1652 if (this.endpoint == null) 1653 this.endpoint = new ArrayList<Reference>(); 1654 this.endpoint.add(t); 1655 return t; 1656 } 1657 1658 public PractitionerRole addEndpoint(Reference t) { //3 1659 if (t == null) 1660 return this; 1661 if (this.endpoint == null) 1662 this.endpoint = new ArrayList<Reference>(); 1663 this.endpoint.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 1669 */ 1670 public Reference getEndpointFirstRep() { 1671 if (getEndpoint().isEmpty()) { 1672 addEndpoint(); 1673 } 1674 return getEndpoint().get(0); 1675 } 1676 1677 protected void listChildren(List<Property> children) { 1678 super.listChildren(children); 1679 children.add(new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1680 children.add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active)); 1681 children.add(new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period)); 1682 children.add(new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organation.", 0, 1, practitioner)); 1683 children.add(new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization)); 1684 children.add(new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code)); 1685 children.add(new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, java.lang.Integer.MAX_VALUE, specialty)); 1686 children.add(new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 1687 children.add(new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService)); 1688 children.add(new Property("telecom", "ContactPoint", "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1689 children.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime)); 1690 children.add(new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable)); 1691 children.add(new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions)); 1692 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the practitioner with this role.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1693 } 1694 1695 @Override 1696 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1697 switch (_hash) { 1698 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier); 1699 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active); 1700 case -991726143: /*period*/ return new Property("period", "Period", "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 0, 1, period); 1701 case 574573338: /*practitioner*/ return new Property("practitioner", "Reference(Practitioner)", "Practitioner that is able to provide the defined services for the organation.", 0, 1, practitioner); 1702 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization where the Practitioner performs the roles associated.", 0, 1, organization); 1703 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, code); 1704 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, java.lang.Integer.MAX_VALUE, specialty); 1705 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location); 1706 case 1289661064: /*healthcareService*/ return new Property("healthcareService", "Reference(HealthcareService)", "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, java.lang.Integer.MAX_VALUE, healthcareService); 1707 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom); 1708 case 1873069366: /*availableTime*/ return new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 1709 case -629572298: /*notAvailable*/ return new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable); 1710 case -1149143617: /*availabilityExceptions*/ return new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions); 1711 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the practitioner with this role.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1712 default: return super.getNamedProperty(_hash, _name, _checkValid); 1713 } 1714 1715 } 1716 1717 @Override 1718 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1719 switch (hash) { 1720 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1721 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1722 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1723 case 574573338: /*practitioner*/ return this.practitioner == null ? new Base[0] : new Base[] {this.practitioner}; // Reference 1724 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1725 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1726 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 1727 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 1728 case 1289661064: /*healthcareService*/ return this.healthcareService == null ? new Base[0] : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 1729 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1730 case 1873069366: /*availableTime*/ return this.availableTime == null ? new Base[0] : this.availableTime.toArray(new Base[this.availableTime.size()]); // PractitionerRoleAvailableTimeComponent 1731 case -629572298: /*notAvailable*/ return this.notAvailable == null ? new Base[0] : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // PractitionerRoleNotAvailableComponent 1732 case -1149143617: /*availabilityExceptions*/ return this.availabilityExceptions == null ? new Base[0] : new Base[] {this.availabilityExceptions}; // StringType 1733 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1734 default: return super.getProperty(hash, name, checkValid); 1735 } 1736 1737 } 1738 1739 @Override 1740 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1741 switch (hash) { 1742 case -1618432855: // identifier 1743 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1744 return value; 1745 case -1422950650: // active 1746 this.active = castToBoolean(value); // BooleanType 1747 return value; 1748 case -991726143: // period 1749 this.period = castToPeriod(value); // Period 1750 return value; 1751 case 574573338: // practitioner 1752 this.practitioner = castToReference(value); // Reference 1753 return value; 1754 case 1178922291: // organization 1755 this.organization = castToReference(value); // Reference 1756 return value; 1757 case 3059181: // code 1758 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1759 return value; 1760 case -1694759682: // specialty 1761 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 1762 return value; 1763 case 1901043637: // location 1764 this.getLocation().add(castToReference(value)); // Reference 1765 return value; 1766 case 1289661064: // healthcareService 1767 this.getHealthcareService().add(castToReference(value)); // Reference 1768 return value; 1769 case -1429363305: // telecom 1770 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1771 return value; 1772 case 1873069366: // availableTime 1773 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); // PractitionerRoleAvailableTimeComponent 1774 return value; 1775 case -629572298: // notAvailable 1776 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); // PractitionerRoleNotAvailableComponent 1777 return value; 1778 case -1149143617: // availabilityExceptions 1779 this.availabilityExceptions = castToString(value); // StringType 1780 return value; 1781 case 1741102485: // endpoint 1782 this.getEndpoint().add(castToReference(value)); // Reference 1783 return value; 1784 default: return super.setProperty(hash, name, value); 1785 } 1786 1787 } 1788 1789 @Override 1790 public Base setProperty(String name, Base value) throws FHIRException { 1791 if (name.equals("identifier")) { 1792 this.getIdentifier().add(castToIdentifier(value)); 1793 } else if (name.equals("active")) { 1794 this.active = castToBoolean(value); // BooleanType 1795 } else if (name.equals("period")) { 1796 this.period = castToPeriod(value); // Period 1797 } else if (name.equals("practitioner")) { 1798 this.practitioner = castToReference(value); // Reference 1799 } else if (name.equals("organization")) { 1800 this.organization = castToReference(value); // Reference 1801 } else if (name.equals("code")) { 1802 this.getCode().add(castToCodeableConcept(value)); 1803 } else if (name.equals("specialty")) { 1804 this.getSpecialty().add(castToCodeableConcept(value)); 1805 } else if (name.equals("location")) { 1806 this.getLocation().add(castToReference(value)); 1807 } else if (name.equals("healthcareService")) { 1808 this.getHealthcareService().add(castToReference(value)); 1809 } else if (name.equals("telecom")) { 1810 this.getTelecom().add(castToContactPoint(value)); 1811 } else if (name.equals("availableTime")) { 1812 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); 1813 } else if (name.equals("notAvailable")) { 1814 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); 1815 } else if (name.equals("availabilityExceptions")) { 1816 this.availabilityExceptions = castToString(value); // StringType 1817 } else if (name.equals("endpoint")) { 1818 this.getEndpoint().add(castToReference(value)); 1819 } else 1820 return super.setProperty(name, value); 1821 return value; 1822 } 1823 1824 @Override 1825 public Base makeProperty(int hash, String name) throws FHIRException { 1826 switch (hash) { 1827 case -1618432855: return addIdentifier(); 1828 case -1422950650: return getActiveElement(); 1829 case -991726143: return getPeriod(); 1830 case 574573338: return getPractitioner(); 1831 case 1178922291: return getOrganization(); 1832 case 3059181: return addCode(); 1833 case -1694759682: return addSpecialty(); 1834 case 1901043637: return addLocation(); 1835 case 1289661064: return addHealthcareService(); 1836 case -1429363305: return addTelecom(); 1837 case 1873069366: return addAvailableTime(); 1838 case -629572298: return addNotAvailable(); 1839 case -1149143617: return getAvailabilityExceptionsElement(); 1840 case 1741102485: return addEndpoint(); 1841 default: return super.makeProperty(hash, name); 1842 } 1843 1844 } 1845 1846 @Override 1847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1848 switch (hash) { 1849 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1850 case -1422950650: /*active*/ return new String[] {"boolean"}; 1851 case -991726143: /*period*/ return new String[] {"Period"}; 1852 case 574573338: /*practitioner*/ return new String[] {"Reference"}; 1853 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1854 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1855 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1856 case 1901043637: /*location*/ return new String[] {"Reference"}; 1857 case 1289661064: /*healthcareService*/ return new String[] {"Reference"}; 1858 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1859 case 1873069366: /*availableTime*/ return new String[] {}; 1860 case -629572298: /*notAvailable*/ return new String[] {}; 1861 case -1149143617: /*availabilityExceptions*/ return new String[] {"string"}; 1862 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1863 default: return super.getTypesForProperty(hash, name); 1864 } 1865 1866 } 1867 1868 @Override 1869 public Base addChild(String name) throws FHIRException { 1870 if (name.equals("identifier")) { 1871 return addIdentifier(); 1872 } 1873 else if (name.equals("active")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.active"); 1875 } 1876 else if (name.equals("period")) { 1877 this.period = new Period(); 1878 return this.period; 1879 } 1880 else if (name.equals("practitioner")) { 1881 this.practitioner = new Reference(); 1882 return this.practitioner; 1883 } 1884 else if (name.equals("organization")) { 1885 this.organization = new Reference(); 1886 return this.organization; 1887 } 1888 else if (name.equals("code")) { 1889 return addCode(); 1890 } 1891 else if (name.equals("specialty")) { 1892 return addSpecialty(); 1893 } 1894 else if (name.equals("location")) { 1895 return addLocation(); 1896 } 1897 else if (name.equals("healthcareService")) { 1898 return addHealthcareService(); 1899 } 1900 else if (name.equals("telecom")) { 1901 return addTelecom(); 1902 } 1903 else if (name.equals("availableTime")) { 1904 return addAvailableTime(); 1905 } 1906 else if (name.equals("notAvailable")) { 1907 return addNotAvailable(); 1908 } 1909 else if (name.equals("availabilityExceptions")) { 1910 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availabilityExceptions"); 1911 } 1912 else if (name.equals("endpoint")) { 1913 return addEndpoint(); 1914 } 1915 else 1916 return super.addChild(name); 1917 } 1918 1919 public String fhirType() { 1920 return "PractitionerRole"; 1921 1922 } 1923 1924 public PractitionerRole copy() { 1925 PractitionerRole dst = new PractitionerRole(); 1926 copyValues(dst); 1927 if (identifier != null) { 1928 dst.identifier = new ArrayList<Identifier>(); 1929 for (Identifier i : identifier) 1930 dst.identifier.add(i.copy()); 1931 }; 1932 dst.active = active == null ? null : active.copy(); 1933 dst.period = period == null ? null : period.copy(); 1934 dst.practitioner = practitioner == null ? null : practitioner.copy(); 1935 dst.organization = organization == null ? null : organization.copy(); 1936 if (code != null) { 1937 dst.code = new ArrayList<CodeableConcept>(); 1938 for (CodeableConcept i : code) 1939 dst.code.add(i.copy()); 1940 }; 1941 if (specialty != null) { 1942 dst.specialty = new ArrayList<CodeableConcept>(); 1943 for (CodeableConcept i : specialty) 1944 dst.specialty.add(i.copy()); 1945 }; 1946 if (location != null) { 1947 dst.location = new ArrayList<Reference>(); 1948 for (Reference i : location) 1949 dst.location.add(i.copy()); 1950 }; 1951 if (healthcareService != null) { 1952 dst.healthcareService = new ArrayList<Reference>(); 1953 for (Reference i : healthcareService) 1954 dst.healthcareService.add(i.copy()); 1955 }; 1956 if (telecom != null) { 1957 dst.telecom = new ArrayList<ContactPoint>(); 1958 for (ContactPoint i : telecom) 1959 dst.telecom.add(i.copy()); 1960 }; 1961 if (availableTime != null) { 1962 dst.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1963 for (PractitionerRoleAvailableTimeComponent i : availableTime) 1964 dst.availableTime.add(i.copy()); 1965 }; 1966 if (notAvailable != null) { 1967 dst.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1968 for (PractitionerRoleNotAvailableComponent i : notAvailable) 1969 dst.notAvailable.add(i.copy()); 1970 }; 1971 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 1972 if (endpoint != null) { 1973 dst.endpoint = new ArrayList<Reference>(); 1974 for (Reference i : endpoint) 1975 dst.endpoint.add(i.copy()); 1976 }; 1977 return dst; 1978 } 1979 1980 protected PractitionerRole typedCopy() { 1981 return copy(); 1982 } 1983 1984 @Override 1985 public boolean equalsDeep(Base other_) { 1986 if (!super.equalsDeep(other_)) 1987 return false; 1988 if (!(other_ instanceof PractitionerRole)) 1989 return false; 1990 PractitionerRole o = (PractitionerRole) other_; 1991 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(period, o.period, true) 1992 && compareDeep(practitioner, o.practitioner, true) && compareDeep(organization, o.organization, true) 1993 && compareDeep(code, o.code, true) && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 1994 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(telecom, o.telecom, true) 1995 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 1996 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) && compareDeep(endpoint, o.endpoint, true) 1997 ; 1998 } 1999 2000 @Override 2001 public boolean equalsShallow(Base other_) { 2002 if (!super.equalsShallow(other_)) 2003 return false; 2004 if (!(other_ instanceof PractitionerRole)) 2005 return false; 2006 PractitionerRole o = (PractitionerRole) other_; 2007 return compareValues(active, o.active, true) && compareValues(availabilityExceptions, o.availabilityExceptions, true) 2008 ; 2009 } 2010 2011 public boolean isEmpty() { 2012 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period 2013 , practitioner, organization, code, specialty, location, healthcareService, telecom 2014 , availableTime, notAvailable, availabilityExceptions, endpoint); 2015 } 2016 2017 @Override 2018 public ResourceType getResourceType() { 2019 return ResourceType.PractitionerRole; 2020 } 2021 2022 /** 2023 * Search parameter: <b>date</b> 2024 * <p> 2025 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 2026 * Type: <b>date</b><br> 2027 * Path: <b>PractitionerRole.period</b><br> 2028 * </p> 2029 */ 2030 @SearchParamDefinition(name="date", path="PractitionerRole.period", description="The period during which the practitioner is authorized to perform in these role(s)", type="date" ) 2031 public static final String SP_DATE = "date"; 2032 /** 2033 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2034 * <p> 2035 * Description: <b>The period during which the practitioner is authorized to perform in these role(s)</b><br> 2036 * Type: <b>date</b><br> 2037 * Path: <b>PractitionerRole.period</b><br> 2038 * </p> 2039 */ 2040 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2041 2042 /** 2043 * Search parameter: <b>identifier</b> 2044 * <p> 2045 * Description: <b>A practitioner's Identifier</b><br> 2046 * Type: <b>token</b><br> 2047 * Path: <b>PractitionerRole.identifier</b><br> 2048 * </p> 2049 */ 2050 @SearchParamDefinition(name="identifier", path="PractitionerRole.identifier", description="A practitioner's Identifier", type="token" ) 2051 public static final String SP_IDENTIFIER = "identifier"; 2052 /** 2053 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2054 * <p> 2055 * Description: <b>A practitioner's Identifier</b><br> 2056 * Type: <b>token</b><br> 2057 * Path: <b>PractitionerRole.identifier</b><br> 2058 * </p> 2059 */ 2060 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2061 2062 /** 2063 * Search parameter: <b>specialty</b> 2064 * <p> 2065 * Description: <b>The practitioner has this specialty at an organization</b><br> 2066 * Type: <b>token</b><br> 2067 * Path: <b>PractitionerRole.specialty</b><br> 2068 * </p> 2069 */ 2070 @SearchParamDefinition(name="specialty", path="PractitionerRole.specialty", description="The practitioner has this specialty at an organization", type="token" ) 2071 public static final String SP_SPECIALTY = "specialty"; 2072 /** 2073 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2074 * <p> 2075 * Description: <b>The practitioner has this specialty at an organization</b><br> 2076 * Type: <b>token</b><br> 2077 * Path: <b>PractitionerRole.specialty</b><br> 2078 * </p> 2079 */ 2080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 2081 2082 /** 2083 * Search parameter: <b>role</b> 2084 * <p> 2085 * Description: <b>The practitioner can perform this role at for the organization</b><br> 2086 * Type: <b>token</b><br> 2087 * Path: <b>PractitionerRole.code</b><br> 2088 * </p> 2089 */ 2090 @SearchParamDefinition(name="role", path="PractitionerRole.code", description="The practitioner can perform this role at for the organization", type="token" ) 2091 public static final String SP_ROLE = "role"; 2092 /** 2093 * <b>Fluent Client</b> search parameter constant for <b>role</b> 2094 * <p> 2095 * Description: <b>The practitioner can perform this role at for the organization</b><br> 2096 * Type: <b>token</b><br> 2097 * Path: <b>PractitionerRole.code</b><br> 2098 * </p> 2099 */ 2100 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROLE); 2101 2102 /** 2103 * Search parameter: <b>practitioner</b> 2104 * <p> 2105 * Description: <b>Practitioner that is able to provide the defined services for the organation</b><br> 2106 * Type: <b>reference</b><br> 2107 * Path: <b>PractitionerRole.practitioner</b><br> 2108 * </p> 2109 */ 2110 @SearchParamDefinition(name="practitioner", path="PractitionerRole.practitioner", description="Practitioner that is able to provide the defined services for the organation", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2111 public static final String SP_PRACTITIONER = "practitioner"; 2112 /** 2113 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 2114 * <p> 2115 * Description: <b>Practitioner that is able to provide the defined services for the organation</b><br> 2116 * Type: <b>reference</b><br> 2117 * Path: <b>PractitionerRole.practitioner</b><br> 2118 * </p> 2119 */ 2120 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 2121 2122/** 2123 * Constant for fluent queries to be used to add include statements. Specifies 2124 * the path value of "<b>PractitionerRole:practitioner</b>". 2125 */ 2126 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("PractitionerRole:practitioner").toLocked(); 2127 2128 /** 2129 * Search parameter: <b>active</b> 2130 * <p> 2131 * Description: <b>Whether this practitioner's record is in active use</b><br> 2132 * Type: <b>token</b><br> 2133 * Path: <b>PractitionerRole.active</b><br> 2134 * </p> 2135 */ 2136 @SearchParamDefinition(name="active", path="PractitionerRole.active", description="Whether this practitioner's record is in active use", type="token" ) 2137 public static final String SP_ACTIVE = "active"; 2138 /** 2139 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2140 * <p> 2141 * Description: <b>Whether this practitioner's record is in active use</b><br> 2142 * Type: <b>token</b><br> 2143 * Path: <b>PractitionerRole.active</b><br> 2144 * </p> 2145 */ 2146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 2147 2148 /** 2149 * Search parameter: <b>endpoint</b> 2150 * <p> 2151 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 2152 * Type: <b>reference</b><br> 2153 * Path: <b>PractitionerRole.endpoint</b><br> 2154 * </p> 2155 */ 2156 @SearchParamDefinition(name="endpoint", path="PractitionerRole.endpoint", description="Technical endpoints providing access to services operated for the practitioner with this role", type="reference", target={Endpoint.class } ) 2157 public static final String SP_ENDPOINT = "endpoint"; 2158 /** 2159 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2160 * <p> 2161 * Description: <b>Technical endpoints providing access to services operated for the practitioner with this role</b><br> 2162 * Type: <b>reference</b><br> 2163 * Path: <b>PractitionerRole.endpoint</b><br> 2164 * </p> 2165 */ 2166 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 2167 2168/** 2169 * Constant for fluent queries to be used to add include statements. Specifies 2170 * the path value of "<b>PractitionerRole:endpoint</b>". 2171 */ 2172 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("PractitionerRole:endpoint").toLocked(); 2173 2174 /** 2175 * Search parameter: <b>phone</b> 2176 * <p> 2177 * Description: <b>A value in a phone contact</b><br> 2178 * Type: <b>token</b><br> 2179 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2180 * </p> 2181 */ 2182 @SearchParamDefinition(name="phone", path="PractitionerRole.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 2183 public static final String SP_PHONE = "phone"; 2184 /** 2185 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2186 * <p> 2187 * Description: <b>A value in a phone contact</b><br> 2188 * Type: <b>token</b><br> 2189 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2190 * </p> 2191 */ 2192 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 2193 2194 /** 2195 * Search parameter: <b>service</b> 2196 * <p> 2197 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 2198 * Type: <b>reference</b><br> 2199 * Path: <b>PractitionerRole.healthcareService</b><br> 2200 * </p> 2201 */ 2202 @SearchParamDefinition(name="service", path="PractitionerRole.healthcareService", description="The list of healthcare services that this worker provides for this role's Organization/Location(s)", type="reference", target={HealthcareService.class } ) 2203 public static final String SP_SERVICE = "service"; 2204 /** 2205 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2206 * <p> 2207 * Description: <b>The list of healthcare services that this worker provides for this role's Organization/Location(s)</b><br> 2208 * Type: <b>reference</b><br> 2209 * Path: <b>PractitionerRole.healthcareService</b><br> 2210 * </p> 2211 */ 2212 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 2213 2214/** 2215 * Constant for fluent queries to be used to add include statements. Specifies 2216 * the path value of "<b>PractitionerRole:service</b>". 2217 */ 2218 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("PractitionerRole:service").toLocked(); 2219 2220 /** 2221 * Search parameter: <b>organization</b> 2222 * <p> 2223 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 2224 * Type: <b>reference</b><br> 2225 * Path: <b>PractitionerRole.organization</b><br> 2226 * </p> 2227 */ 2228 @SearchParamDefinition(name="organization", path="PractitionerRole.organization", description="The identity of the organization the practitioner represents / acts on behalf of", type="reference", target={Organization.class } ) 2229 public static final String SP_ORGANIZATION = "organization"; 2230 /** 2231 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2232 * <p> 2233 * Description: <b>The identity of the organization the practitioner represents / acts on behalf of</b><br> 2234 * Type: <b>reference</b><br> 2235 * Path: <b>PractitionerRole.organization</b><br> 2236 * </p> 2237 */ 2238 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2239 2240/** 2241 * Constant for fluent queries to be used to add include statements. Specifies 2242 * the path value of "<b>PractitionerRole:organization</b>". 2243 */ 2244 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:organization").toLocked(); 2245 2246 /** 2247 * Search parameter: <b>telecom</b> 2248 * <p> 2249 * Description: <b>The value in any kind of contact</b><br> 2250 * Type: <b>token</b><br> 2251 * Path: <b>PractitionerRole.telecom</b><br> 2252 * </p> 2253 */ 2254 @SearchParamDefinition(name="telecom", path="PractitionerRole.telecom", description="The value in any kind of contact", type="token" ) 2255 public static final String SP_TELECOM = "telecom"; 2256 /** 2257 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2258 * <p> 2259 * Description: <b>The value in any kind of contact</b><br> 2260 * Type: <b>token</b><br> 2261 * Path: <b>PractitionerRole.telecom</b><br> 2262 * </p> 2263 */ 2264 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2265 2266 /** 2267 * Search parameter: <b>location</b> 2268 * <p> 2269 * Description: <b>One of the locations at which this practitioner provides care</b><br> 2270 * Type: <b>reference</b><br> 2271 * Path: <b>PractitionerRole.location</b><br> 2272 * </p> 2273 */ 2274 @SearchParamDefinition(name="location", path="PractitionerRole.location", description="One of the locations at which this practitioner provides care", type="reference", target={Location.class } ) 2275 public static final String SP_LOCATION = "location"; 2276 /** 2277 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2278 * <p> 2279 * Description: <b>One of the locations at which this practitioner provides care</b><br> 2280 * Type: <b>reference</b><br> 2281 * Path: <b>PractitionerRole.location</b><br> 2282 * </p> 2283 */ 2284 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2285 2286/** 2287 * Constant for fluent queries to be used to add include statements. Specifies 2288 * the path value of "<b>PractitionerRole:location</b>". 2289 */ 2290 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("PractitionerRole:location").toLocked(); 2291 2292 /** 2293 * Search parameter: <b>email</b> 2294 * <p> 2295 * Description: <b>A value in an email contact</b><br> 2296 * Type: <b>token</b><br> 2297 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2298 * </p> 2299 */ 2300 @SearchParamDefinition(name="email", path="PractitionerRole.telecom.where(system='email')", description="A value in an email contact", type="token" ) 2301 public static final String SP_EMAIL = "email"; 2302 /** 2303 * <b>Fluent Client</b> search parameter constant for <b>email</b> 2304 * <p> 2305 * Description: <b>A value in an email contact</b><br> 2306 * Type: <b>token</b><br> 2307 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2308 * </p> 2309 */ 2310 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 2311 2312 2313}