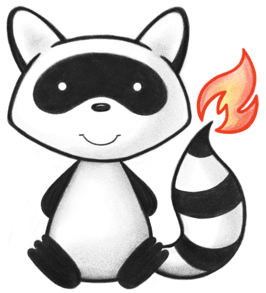
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 049 */ 050@ResourceDef(name="Procedure", profile="http://hl7.org/fhir/Profile/Procedure") 051public class Procedure extends DomainResource { 052 053 public enum ProcedureStatus { 054 /** 055 * The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes. 056 */ 057 PREPARATION, 058 /** 059 * The event is currently occurring 060 */ 061 INPROGRESS, 062 /** 063 * The event has been temporarily stopped but is expected to resume in the future 064 */ 065 SUSPENDED, 066 /** 067 * The event was prior to the full completion of the intended actions 068 */ 069 ABORTED, 070 /** 071 * The event has now concluded 072 */ 073 COMPLETED, 074 /** 075 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 076 */ 077 ENTEREDINERROR, 078 /** 079 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 080 */ 081 UNKNOWN, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 public static ProcedureStatus fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("preparation".equals(codeString)) 090 return PREPARATION; 091 if ("in-progress".equals(codeString)) 092 return INPROGRESS; 093 if ("suspended".equals(codeString)) 094 return SUSPENDED; 095 if ("aborted".equals(codeString)) 096 return ABORTED; 097 if ("completed".equals(codeString)) 098 return COMPLETED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if ("unknown".equals(codeString)) 102 return UNKNOWN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown ProcedureStatus code '"+codeString+"'"); 107 } 108 public String toCode() { 109 switch (this) { 110 case PREPARATION: return "preparation"; 111 case INPROGRESS: return "in-progress"; 112 case SUSPENDED: return "suspended"; 113 case ABORTED: return "aborted"; 114 case COMPLETED: return "completed"; 115 case ENTEREDINERROR: return "entered-in-error"; 116 case UNKNOWN: return "unknown"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getSystem() { 122 switch (this) { 123 case PREPARATION: return "http://hl7.org/fhir/event-status"; 124 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 125 case SUSPENDED: return "http://hl7.org/fhir/event-status"; 126 case ABORTED: return "http://hl7.org/fhir/event-status"; 127 case COMPLETED: return "http://hl7.org/fhir/event-status"; 128 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 129 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getDefinition() { 135 switch (this) { 136 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 137 case INPROGRESS: return "The event is currently occurring"; 138 case SUSPENDED: return "The event has been temporarily stopped but is expected to resume in the future"; 139 case ABORTED: return "The event was prior to the full completion of the intended actions"; 140 case COMPLETED: return "The event has now concluded"; 141 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 142 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 public String getDisplay() { 148 switch (this) { 149 case PREPARATION: return "Preparation"; 150 case INPROGRESS: return "In Progress"; 151 case SUSPENDED: return "Suspended"; 152 case ABORTED: return "Aborted"; 153 case COMPLETED: return "Completed"; 154 case ENTEREDINERROR: return "Entered in Error"; 155 case UNKNOWN: return "Unknown"; 156 case NULL: return null; 157 default: return "?"; 158 } 159 } 160 } 161 162 public static class ProcedureStatusEnumFactory implements EnumFactory<ProcedureStatus> { 163 public ProcedureStatus fromCode(String codeString) throws IllegalArgumentException { 164 if (codeString == null || "".equals(codeString)) 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("preparation".equals(codeString)) 168 return ProcedureStatus.PREPARATION; 169 if ("in-progress".equals(codeString)) 170 return ProcedureStatus.INPROGRESS; 171 if ("suspended".equals(codeString)) 172 return ProcedureStatus.SUSPENDED; 173 if ("aborted".equals(codeString)) 174 return ProcedureStatus.ABORTED; 175 if ("completed".equals(codeString)) 176 return ProcedureStatus.COMPLETED; 177 if ("entered-in-error".equals(codeString)) 178 return ProcedureStatus.ENTEREDINERROR; 179 if ("unknown".equals(codeString)) 180 return ProcedureStatus.UNKNOWN; 181 throw new IllegalArgumentException("Unknown ProcedureStatus code '"+codeString+"'"); 182 } 183 public Enumeration<ProcedureStatus> fromType(PrimitiveType<?> code) throws FHIRException { 184 if (code == null) 185 return null; 186 if (code.isEmpty()) 187 return new Enumeration<ProcedureStatus>(this); 188 String codeString = code.asStringValue(); 189 if (codeString == null || "".equals(codeString)) 190 return null; 191 if ("preparation".equals(codeString)) 192 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.PREPARATION); 193 if ("in-progress".equals(codeString)) 194 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.INPROGRESS); 195 if ("suspended".equals(codeString)) 196 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.SUSPENDED); 197 if ("aborted".equals(codeString)) 198 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ABORTED); 199 if ("completed".equals(codeString)) 200 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.COMPLETED); 201 if ("entered-in-error".equals(codeString)) 202 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ENTEREDINERROR); 203 if ("unknown".equals(codeString)) 204 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.UNKNOWN); 205 throw new FHIRException("Unknown ProcedureStatus code '"+codeString+"'"); 206 } 207 public String toCode(ProcedureStatus code) { 208 if (code == ProcedureStatus.NULL) 209 return null; 210 if (code == ProcedureStatus.PREPARATION) 211 return "preparation"; 212 if (code == ProcedureStatus.INPROGRESS) 213 return "in-progress"; 214 if (code == ProcedureStatus.SUSPENDED) 215 return "suspended"; 216 if (code == ProcedureStatus.ABORTED) 217 return "aborted"; 218 if (code == ProcedureStatus.COMPLETED) 219 return "completed"; 220 if (code == ProcedureStatus.ENTEREDINERROR) 221 return "entered-in-error"; 222 if (code == ProcedureStatus.UNKNOWN) 223 return "unknown"; 224 return "?"; 225 } 226 public String toSystem(ProcedureStatus code) { 227 return code.getSystem(); 228 } 229 } 230 231 @Block() 232 public static class ProcedurePerformerComponent extends BackboneElement implements IBaseBackboneElement { 233 /** 234 * For example: surgeon, anaethetist, endoscopist. 235 */ 236 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 237 @Description(shortDefinition="The role the actor was in", formalDefinition="For example: surgeon, anaethetist, endoscopist." ) 238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 239 protected CodeableConcept role; 240 241 /** 242 * The practitioner who was involved in the procedure. 243 */ 244 @Child(name = "actor", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=2, min=1, max=1, modifier=false, summary=true) 245 @Description(shortDefinition="The reference to the practitioner", formalDefinition="The practitioner who was involved in the procedure." ) 246 protected Reference actor; 247 248 /** 249 * The actual object that is the target of the reference (The practitioner who was involved in the procedure.) 250 */ 251 protected Resource actorTarget; 252 253 /** 254 * The organization the device or practitioner was acting on behalf of. 255 */ 256 @Child(name = "onBehalfOf", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 257 @Description(shortDefinition="Organization the device or practitioner was acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 258 protected Reference onBehalfOf; 259 260 /** 261 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 262 */ 263 protected Organization onBehalfOfTarget; 264 265 private static final long serialVersionUID = 213950062L; 266 267 /** 268 * Constructor 269 */ 270 public ProcedurePerformerComponent() { 271 super(); 272 } 273 274 /** 275 * Constructor 276 */ 277 public ProcedurePerformerComponent(Reference actor) { 278 super(); 279 this.actor = actor; 280 } 281 282 /** 283 * @return {@link #role} (For example: surgeon, anaethetist, endoscopist.) 284 */ 285 public CodeableConcept getRole() { 286 if (this.role == null) 287 if (Configuration.errorOnAutoCreate()) 288 throw new Error("Attempt to auto-create ProcedurePerformerComponent.role"); 289 else if (Configuration.doAutoCreate()) 290 this.role = new CodeableConcept(); // cc 291 return this.role; 292 } 293 294 public boolean hasRole() { 295 return this.role != null && !this.role.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #role} (For example: surgeon, anaethetist, endoscopist.) 300 */ 301 public ProcedurePerformerComponent setRole(CodeableConcept value) { 302 this.role = value; 303 return this; 304 } 305 306 /** 307 * @return {@link #actor} (The practitioner who was involved in the procedure.) 308 */ 309 public Reference getActor() { 310 if (this.actor == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create ProcedurePerformerComponent.actor"); 313 else if (Configuration.doAutoCreate()) 314 this.actor = new Reference(); // cc 315 return this.actor; 316 } 317 318 public boolean hasActor() { 319 return this.actor != null && !this.actor.isEmpty(); 320 } 321 322 /** 323 * @param value {@link #actor} (The practitioner who was involved in the procedure.) 324 */ 325 public ProcedurePerformerComponent setActor(Reference value) { 326 this.actor = value; 327 return this; 328 } 329 330 /** 331 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who was involved in the procedure.) 332 */ 333 public Resource getActorTarget() { 334 return this.actorTarget; 335 } 336 337 /** 338 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who was involved in the procedure.) 339 */ 340 public ProcedurePerformerComponent setActorTarget(Resource value) { 341 this.actorTarget = value; 342 return this; 343 } 344 345 /** 346 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 347 */ 348 public Reference getOnBehalfOf() { 349 if (this.onBehalfOf == null) 350 if (Configuration.errorOnAutoCreate()) 351 throw new Error("Attempt to auto-create ProcedurePerformerComponent.onBehalfOf"); 352 else if (Configuration.doAutoCreate()) 353 this.onBehalfOf = new Reference(); // cc 354 return this.onBehalfOf; 355 } 356 357 public boolean hasOnBehalfOf() { 358 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 363 */ 364 public ProcedurePerformerComponent setOnBehalfOf(Reference value) { 365 this.onBehalfOf = value; 366 return this; 367 } 368 369 /** 370 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 371 */ 372 public Organization getOnBehalfOfTarget() { 373 if (this.onBehalfOfTarget == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create ProcedurePerformerComponent.onBehalfOf"); 376 else if (Configuration.doAutoCreate()) 377 this.onBehalfOfTarget = new Organization(); // aa 378 return this.onBehalfOfTarget; 379 } 380 381 /** 382 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 383 */ 384 public ProcedurePerformerComponent setOnBehalfOfTarget(Organization value) { 385 this.onBehalfOfTarget = value; 386 return this; 387 } 388 389 protected void listChildren(List<Property> children) { 390 super.listChildren(children); 391 children.add(new Property("role", "CodeableConcept", "For example: surgeon, anaethetist, endoscopist.", 0, 1, role)); 392 children.add(new Property("actor", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The practitioner who was involved in the procedure.", 0, 1, actor)); 393 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 394 } 395 396 @Override 397 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 398 switch (_hash) { 399 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "For example: surgeon, anaethetist, endoscopist.", 0, 1, role); 400 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The practitioner who was involved in the procedure.", 0, 1, actor); 401 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 402 default: return super.getNamedProperty(_hash, _name, _checkValid); 403 } 404 405 } 406 407 @Override 408 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 409 switch (hash) { 410 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 411 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 412 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 413 default: return super.getProperty(hash, name, checkValid); 414 } 415 416 } 417 418 @Override 419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 420 switch (hash) { 421 case 3506294: // role 422 this.role = castToCodeableConcept(value); // CodeableConcept 423 return value; 424 case 92645877: // actor 425 this.actor = castToReference(value); // Reference 426 return value; 427 case -14402964: // onBehalfOf 428 this.onBehalfOf = castToReference(value); // Reference 429 return value; 430 default: return super.setProperty(hash, name, value); 431 } 432 433 } 434 435 @Override 436 public Base setProperty(String name, Base value) throws FHIRException { 437 if (name.equals("role")) { 438 this.role = castToCodeableConcept(value); // CodeableConcept 439 } else if (name.equals("actor")) { 440 this.actor = castToReference(value); // Reference 441 } else if (name.equals("onBehalfOf")) { 442 this.onBehalfOf = castToReference(value); // Reference 443 } else 444 return super.setProperty(name, value); 445 return value; 446 } 447 448 @Override 449 public Base makeProperty(int hash, String name) throws FHIRException { 450 switch (hash) { 451 case 3506294: return getRole(); 452 case 92645877: return getActor(); 453 case -14402964: return getOnBehalfOf(); 454 default: return super.makeProperty(hash, name); 455 } 456 457 } 458 459 @Override 460 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 461 switch (hash) { 462 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 463 case 92645877: /*actor*/ return new String[] {"Reference"}; 464 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 465 default: return super.getTypesForProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public Base addChild(String name) throws FHIRException { 472 if (name.equals("role")) { 473 this.role = new CodeableConcept(); 474 return this.role; 475 } 476 else if (name.equals("actor")) { 477 this.actor = new Reference(); 478 return this.actor; 479 } 480 else if (name.equals("onBehalfOf")) { 481 this.onBehalfOf = new Reference(); 482 return this.onBehalfOf; 483 } 484 else 485 return super.addChild(name); 486 } 487 488 public ProcedurePerformerComponent copy() { 489 ProcedurePerformerComponent dst = new ProcedurePerformerComponent(); 490 copyValues(dst); 491 dst.role = role == null ? null : role.copy(); 492 dst.actor = actor == null ? null : actor.copy(); 493 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 494 return dst; 495 } 496 497 @Override 498 public boolean equalsDeep(Base other_) { 499 if (!super.equalsDeep(other_)) 500 return false; 501 if (!(other_ instanceof ProcedurePerformerComponent)) 502 return false; 503 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 504 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 505 ; 506 } 507 508 @Override 509 public boolean equalsShallow(Base other_) { 510 if (!super.equalsShallow(other_)) 511 return false; 512 if (!(other_ instanceof ProcedurePerformerComponent)) 513 return false; 514 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 515 return true; 516 } 517 518 public boolean isEmpty() { 519 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor, onBehalfOf 520 ); 521 } 522 523 public String fhirType() { 524 return "Procedure.performer"; 525 526 } 527 528 } 529 530 @Block() 531 public static class ProcedureFocalDeviceComponent extends BackboneElement implements IBaseBackboneElement { 532 /** 533 * The kind of change that happened to the device during the procedure. 534 */ 535 @Child(name = "action", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 536 @Description(shortDefinition="Kind of change to device", formalDefinition="The kind of change that happened to the device during the procedure." ) 537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-action") 538 protected CodeableConcept action; 539 540 /** 541 * The device that was manipulated (changed) during the procedure. 542 */ 543 @Child(name = "manipulated", type = {Device.class}, order=2, min=1, max=1, modifier=false, summary=false) 544 @Description(shortDefinition="Device that was changed", formalDefinition="The device that was manipulated (changed) during the procedure." ) 545 protected Reference manipulated; 546 547 /** 548 * The actual object that is the target of the reference (The device that was manipulated (changed) during the procedure.) 549 */ 550 protected Device manipulatedTarget; 551 552 private static final long serialVersionUID = 1779937807L; 553 554 /** 555 * Constructor 556 */ 557 public ProcedureFocalDeviceComponent() { 558 super(); 559 } 560 561 /** 562 * Constructor 563 */ 564 public ProcedureFocalDeviceComponent(Reference manipulated) { 565 super(); 566 this.manipulated = manipulated; 567 } 568 569 /** 570 * @return {@link #action} (The kind of change that happened to the device during the procedure.) 571 */ 572 public CodeableConcept getAction() { 573 if (this.action == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.action"); 576 else if (Configuration.doAutoCreate()) 577 this.action = new CodeableConcept(); // cc 578 return this.action; 579 } 580 581 public boolean hasAction() { 582 return this.action != null && !this.action.isEmpty(); 583 } 584 585 /** 586 * @param value {@link #action} (The kind of change that happened to the device during the procedure.) 587 */ 588 public ProcedureFocalDeviceComponent setAction(CodeableConcept value) { 589 this.action = value; 590 return this; 591 } 592 593 /** 594 * @return {@link #manipulated} (The device that was manipulated (changed) during the procedure.) 595 */ 596 public Reference getManipulated() { 597 if (this.manipulated == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 600 else if (Configuration.doAutoCreate()) 601 this.manipulated = new Reference(); // cc 602 return this.manipulated; 603 } 604 605 public boolean hasManipulated() { 606 return this.manipulated != null && !this.manipulated.isEmpty(); 607 } 608 609 /** 610 * @param value {@link #manipulated} (The device that was manipulated (changed) during the procedure.) 611 */ 612 public ProcedureFocalDeviceComponent setManipulated(Reference value) { 613 this.manipulated = value; 614 return this; 615 } 616 617 /** 618 * @return {@link #manipulated} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device that was manipulated (changed) during the procedure.) 619 */ 620 public Device getManipulatedTarget() { 621 if (this.manipulatedTarget == null) 622 if (Configuration.errorOnAutoCreate()) 623 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 624 else if (Configuration.doAutoCreate()) 625 this.manipulatedTarget = new Device(); // aa 626 return this.manipulatedTarget; 627 } 628 629 /** 630 * @param value {@link #manipulated} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device that was manipulated (changed) during the procedure.) 631 */ 632 public ProcedureFocalDeviceComponent setManipulatedTarget(Device value) { 633 this.manipulatedTarget = value; 634 return this; 635 } 636 637 protected void listChildren(List<Property> children) { 638 super.listChildren(children); 639 children.add(new Property("action", "CodeableConcept", "The kind of change that happened to the device during the procedure.", 0, 1, action)); 640 children.add(new Property("manipulated", "Reference(Device)", "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated)); 641 } 642 643 @Override 644 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 645 switch (_hash) { 646 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "The kind of change that happened to the device during the procedure.", 0, 1, action); 647 case 947372650: /*manipulated*/ return new Property("manipulated", "Reference(Device)", "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated); 648 default: return super.getNamedProperty(_hash, _name, _checkValid); 649 } 650 651 } 652 653 @Override 654 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 655 switch (hash) { 656 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // CodeableConcept 657 case 947372650: /*manipulated*/ return this.manipulated == null ? new Base[0] : new Base[] {this.manipulated}; // Reference 658 default: return super.getProperty(hash, name, checkValid); 659 } 660 661 } 662 663 @Override 664 public Base setProperty(int hash, String name, Base value) throws FHIRException { 665 switch (hash) { 666 case -1422950858: // action 667 this.action = castToCodeableConcept(value); // CodeableConcept 668 return value; 669 case 947372650: // manipulated 670 this.manipulated = castToReference(value); // Reference 671 return value; 672 default: return super.setProperty(hash, name, value); 673 } 674 675 } 676 677 @Override 678 public Base setProperty(String name, Base value) throws FHIRException { 679 if (name.equals("action")) { 680 this.action = castToCodeableConcept(value); // CodeableConcept 681 } else if (name.equals("manipulated")) { 682 this.manipulated = castToReference(value); // Reference 683 } else 684 return super.setProperty(name, value); 685 return value; 686 } 687 688 @Override 689 public Base makeProperty(int hash, String name) throws FHIRException { 690 switch (hash) { 691 case -1422950858: return getAction(); 692 case 947372650: return getManipulated(); 693 default: return super.makeProperty(hash, name); 694 } 695 696 } 697 698 @Override 699 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 700 switch (hash) { 701 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 702 case 947372650: /*manipulated*/ return new String[] {"Reference"}; 703 default: return super.getTypesForProperty(hash, name); 704 } 705 706 } 707 708 @Override 709 public Base addChild(String name) throws FHIRException { 710 if (name.equals("action")) { 711 this.action = new CodeableConcept(); 712 return this.action; 713 } 714 else if (name.equals("manipulated")) { 715 this.manipulated = new Reference(); 716 return this.manipulated; 717 } 718 else 719 return super.addChild(name); 720 } 721 722 public ProcedureFocalDeviceComponent copy() { 723 ProcedureFocalDeviceComponent dst = new ProcedureFocalDeviceComponent(); 724 copyValues(dst); 725 dst.action = action == null ? null : action.copy(); 726 dst.manipulated = manipulated == null ? null : manipulated.copy(); 727 return dst; 728 } 729 730 @Override 731 public boolean equalsDeep(Base other_) { 732 if (!super.equalsDeep(other_)) 733 return false; 734 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 735 return false; 736 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 737 return compareDeep(action, o.action, true) && compareDeep(manipulated, o.manipulated, true); 738 } 739 740 @Override 741 public boolean equalsShallow(Base other_) { 742 if (!super.equalsShallow(other_)) 743 return false; 744 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 745 return false; 746 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 747 return true; 748 } 749 750 public boolean isEmpty() { 751 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, manipulated); 752 } 753 754 public String fhirType() { 755 return "Procedure.focalDevice"; 756 757 } 758 759 } 760 761 /** 762 * This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 763 */ 764 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 765 @Description(shortDefinition="External Identifiers for this procedure", formalDefinition="This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 766 protected List<Identifier> identifier; 767 768 /** 769 * A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure. 770 */ 771 @Child(name = "definition", type = {PlanDefinition.class, ActivityDefinition.class, HealthcareService.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 772 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure." ) 773 protected List<Reference> definition; 774 /** 775 * The actual objects that are the target of the reference (A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure.) 776 */ 777 protected List<Resource> definitionTarget; 778 779 780 /** 781 * A reference to a resource that contains details of the request for this procedure. 782 */ 783 @Child(name = "basedOn", type = {CarePlan.class, ProcedureRequest.class, ReferralRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 784 @Description(shortDefinition="A request for this procedure", formalDefinition="A reference to a resource that contains details of the request for this procedure." ) 785 protected List<Reference> basedOn; 786 /** 787 * The actual objects that are the target of the reference (A reference to a resource that contains details of the request for this procedure.) 788 */ 789 protected List<Resource> basedOnTarget; 790 791 792 /** 793 * A larger event of which this particular procedure is a component or step. 794 */ 795 @Child(name = "partOf", type = {Procedure.class, Observation.class, MedicationAdministration.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 796 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular procedure is a component or step." ) 797 protected List<Reference> partOf; 798 /** 799 * The actual objects that are the target of the reference (A larger event of which this particular procedure is a component or step.) 800 */ 801 protected List<Resource> partOfTarget; 802 803 804 /** 805 * A code specifying the state of the procedure. Generally this will be in-progress or completed state. 806 */ 807 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 808 @Description(shortDefinition="preparation | in-progress | suspended | aborted | completed | entered-in-error | unknown", formalDefinition="A code specifying the state of the procedure. Generally this will be in-progress or completed state." ) 809 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 810 protected Enumeration<ProcedureStatus> status; 811 812 /** 813 * Set this to true if the record is saying that the procedure was NOT performed. 814 */ 815 @Child(name = "notDone", type = {BooleanType.class}, order=5, min=0, max=1, modifier=true, summary=true) 816 @Description(shortDefinition="True if procedure was not performed as scheduled", formalDefinition="Set this to true if the record is saying that the procedure was NOT performed." ) 817 protected BooleanType notDone; 818 819 /** 820 * A code indicating why the procedure was not performed. 821 */ 822 @Child(name = "notDoneReason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 823 @Description(shortDefinition="Reason procedure was not performed", formalDefinition="A code indicating why the procedure was not performed." ) 824 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-not-performed-reason") 825 protected CodeableConcept notDoneReason; 826 827 /** 828 * A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure"). 829 */ 830 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 831 @Description(shortDefinition="Classification of the procedure", formalDefinition="A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\")." ) 832 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-category") 833 protected CodeableConcept category; 834 835 /** 836 * The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy"). 837 */ 838 @Child(name = "code", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 839 @Description(shortDefinition="Identification of the procedure", formalDefinition="The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\")." ) 840 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 841 protected CodeableConcept code; 842 843 /** 844 * The person, animal or group on which the procedure was performed. 845 */ 846 @Child(name = "subject", type = {Patient.class, Group.class}, order=9, min=1, max=1, modifier=false, summary=true) 847 @Description(shortDefinition="Who the procedure was performed on", formalDefinition="The person, animal or group on which the procedure was performed." ) 848 protected Reference subject; 849 850 /** 851 * The actual object that is the target of the reference (The person, animal or group on which the procedure was performed.) 852 */ 853 protected Resource subjectTarget; 854 855 /** 856 * The encounter during which the procedure was performed. 857 */ 858 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=10, min=0, max=1, modifier=false, summary=true) 859 @Description(shortDefinition="Encounter or episode associated with the procedure", formalDefinition="The encounter during which the procedure was performed." ) 860 protected Reference context; 861 862 /** 863 * The actual object that is the target of the reference (The encounter during which the procedure was performed.) 864 */ 865 protected Resource contextTarget; 866 867 /** 868 * The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured. 869 */ 870 @Child(name = "performed", type = {DateTimeType.class, Period.class}, order=11, min=0, max=1, modifier=false, summary=true) 871 @Description(shortDefinition="Date/Period the procedure was performed", formalDefinition="The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured." ) 872 protected Type performed; 873 874 /** 875 * Limited to 'real' people rather than equipment. 876 */ 877 @Child(name = "performer", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 878 @Description(shortDefinition="The people who performed the procedure", formalDefinition="Limited to 'real' people rather than equipment." ) 879 protected List<ProcedurePerformerComponent> performer; 880 881 /** 882 * The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant. 883 */ 884 @Child(name = "location", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=true) 885 @Description(shortDefinition="Where the procedure happened", formalDefinition="The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant." ) 886 protected Reference location; 887 888 /** 889 * The actual object that is the target of the reference (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 890 */ 891 protected Location locationTarget; 892 893 /** 894 * The coded reason why the procedure was performed. This may be coded entity of some type, or may simply be present as text. 895 */ 896 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 897 @Description(shortDefinition="Coded reason procedure performed", formalDefinition="The coded reason why the procedure was performed. This may be coded entity of some type, or may simply be present as text." ) 898 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 899 protected List<CodeableConcept> reasonCode; 900 901 /** 902 * The condition that is the reason why the procedure was performed. 903 */ 904 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 905 @Description(shortDefinition="Condition that is the reason the procedure performed", formalDefinition="The condition that is the reason why the procedure was performed." ) 906 protected List<Reference> reasonReference; 907 /** 908 * The actual objects that are the target of the reference (The condition that is the reason why the procedure was performed.) 909 */ 910 protected List<Resource> reasonReferenceTarget; 911 912 913 /** 914 * Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion. 915 */ 916 @Child(name = "bodySite", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 917 @Description(shortDefinition="Target body sites", formalDefinition="Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion." ) 918 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 919 protected List<CodeableConcept> bodySite; 920 921 /** 922 * The outcome of the procedure - did it resolve reasons for the procedure being performed? 923 */ 924 @Child(name = "outcome", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 925 @Description(shortDefinition="The result of procedure", formalDefinition="The outcome of the procedure - did it resolve reasons for the procedure being performed?" ) 926 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-outcome") 927 protected CodeableConcept outcome; 928 929 /** 930 * This could be a histology result, pathology report, surgical report, etc.. 931 */ 932 @Child(name = "report", type = {DiagnosticReport.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 933 @Description(shortDefinition="Any report resulting from the procedure", formalDefinition="This could be a histology result, pathology report, surgical report, etc.." ) 934 protected List<Reference> report; 935 /** 936 * The actual objects that are the target of the reference (This could be a histology result, pathology report, surgical report, etc..) 937 */ 938 protected List<DiagnosticReport> reportTarget; 939 940 941 /** 942 * Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues. 943 */ 944 @Child(name = "complication", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 945 @Description(shortDefinition="Complication following the procedure", formalDefinition="Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues." ) 946 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 947 protected List<CodeableConcept> complication; 948 949 /** 950 * Any complications that occurred during the procedure, or in the immediate post-performance period. 951 */ 952 @Child(name = "complicationDetail", type = {Condition.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 953 @Description(shortDefinition="A condition that is a result of the procedure", formalDefinition="Any complications that occurred during the procedure, or in the immediate post-performance period." ) 954 protected List<Reference> complicationDetail; 955 /** 956 * The actual objects that are the target of the reference (Any complications that occurred during the procedure, or in the immediate post-performance period.) 957 */ 958 protected List<Condition> complicationDetailTarget; 959 960 961 /** 962 * If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used. 963 */ 964 @Child(name = "followUp", type = {CodeableConcept.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 965 @Description(shortDefinition="Instructions for follow up", formalDefinition="If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-followup") 967 protected List<CodeableConcept> followUp; 968 969 /** 970 * Any other notes about the procedure. E.g. the operative notes. 971 */ 972 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 973 @Description(shortDefinition="Additional information about the procedure", formalDefinition="Any other notes about the procedure. E.g. the operative notes." ) 974 protected List<Annotation> note; 975 976 /** 977 * A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure. 978 */ 979 @Child(name = "focalDevice", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 980 @Description(shortDefinition="Device changed in procedure", formalDefinition="A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure." ) 981 protected List<ProcedureFocalDeviceComponent> focalDevice; 982 983 /** 984 * Identifies medications, devices and any other substance used as part of the procedure. 985 */ 986 @Child(name = "usedReference", type = {Device.class, Medication.class, Substance.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 987 @Description(shortDefinition="Items used during procedure", formalDefinition="Identifies medications, devices and any other substance used as part of the procedure." ) 988 protected List<Reference> usedReference; 989 /** 990 * The actual objects that are the target of the reference (Identifies medications, devices and any other substance used as part of the procedure.) 991 */ 992 protected List<Resource> usedReferenceTarget; 993 994 995 /** 996 * Identifies coded items that were used as part of the procedure. 997 */ 998 @Child(name = "usedCode", type = {CodeableConcept.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 999 @Description(shortDefinition="Coded items used during the procedure", formalDefinition="Identifies coded items that were used as part of the procedure." ) 1000 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-kind") 1001 protected List<CodeableConcept> usedCode; 1002 1003 private static final long serialVersionUID = 7729906L; 1004 1005 /** 1006 * Constructor 1007 */ 1008 public Procedure() { 1009 super(); 1010 } 1011 1012 /** 1013 * Constructor 1014 */ 1015 public Procedure(Enumeration<ProcedureStatus> status, Reference subject) { 1016 super(); 1017 this.status = status; 1018 this.subject = subject; 1019 } 1020 1021 /** 1022 * @return {@link #identifier} (This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 1023 */ 1024 public List<Identifier> getIdentifier() { 1025 if (this.identifier == null) 1026 this.identifier = new ArrayList<Identifier>(); 1027 return this.identifier; 1028 } 1029 1030 /** 1031 * @return Returns a reference to <code>this</code> for easy method chaining 1032 */ 1033 public Procedure setIdentifier(List<Identifier> theIdentifier) { 1034 this.identifier = theIdentifier; 1035 return this; 1036 } 1037 1038 public boolean hasIdentifier() { 1039 if (this.identifier == null) 1040 return false; 1041 for (Identifier item : this.identifier) 1042 if (!item.isEmpty()) 1043 return true; 1044 return false; 1045 } 1046 1047 public Identifier addIdentifier() { //3 1048 Identifier t = new Identifier(); 1049 if (this.identifier == null) 1050 this.identifier = new ArrayList<Identifier>(); 1051 this.identifier.add(t); 1052 return t; 1053 } 1054 1055 public Procedure addIdentifier(Identifier t) { //3 1056 if (t == null) 1057 return this; 1058 if (this.identifier == null) 1059 this.identifier = new ArrayList<Identifier>(); 1060 this.identifier.add(t); 1061 return this; 1062 } 1063 1064 /** 1065 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1066 */ 1067 public Identifier getIdentifierFirstRep() { 1068 if (getIdentifier().isEmpty()) { 1069 addIdentifier(); 1070 } 1071 return getIdentifier().get(0); 1072 } 1073 1074 /** 1075 * @return {@link #definition} (A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure.) 1076 */ 1077 public List<Reference> getDefinition() { 1078 if (this.definition == null) 1079 this.definition = new ArrayList<Reference>(); 1080 return this.definition; 1081 } 1082 1083 /** 1084 * @return Returns a reference to <code>this</code> for easy method chaining 1085 */ 1086 public Procedure setDefinition(List<Reference> theDefinition) { 1087 this.definition = theDefinition; 1088 return this; 1089 } 1090 1091 public boolean hasDefinition() { 1092 if (this.definition == null) 1093 return false; 1094 for (Reference item : this.definition) 1095 if (!item.isEmpty()) 1096 return true; 1097 return false; 1098 } 1099 1100 public Reference addDefinition() { //3 1101 Reference t = new Reference(); 1102 if (this.definition == null) 1103 this.definition = new ArrayList<Reference>(); 1104 this.definition.add(t); 1105 return t; 1106 } 1107 1108 public Procedure addDefinition(Reference t) { //3 1109 if (t == null) 1110 return this; 1111 if (this.definition == null) 1112 this.definition = new ArrayList<Reference>(); 1113 this.definition.add(t); 1114 return this; 1115 } 1116 1117 /** 1118 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1119 */ 1120 public Reference getDefinitionFirstRep() { 1121 if (getDefinition().isEmpty()) { 1122 addDefinition(); 1123 } 1124 return getDefinition().get(0); 1125 } 1126 1127 /** 1128 * @deprecated Use Reference#setResource(IBaseResource) instead 1129 */ 1130 @Deprecated 1131 public List<Resource> getDefinitionTarget() { 1132 if (this.definitionTarget == null) 1133 this.definitionTarget = new ArrayList<Resource>(); 1134 return this.definitionTarget; 1135 } 1136 1137 /** 1138 * @return {@link #basedOn} (A reference to a resource that contains details of the request for this procedure.) 1139 */ 1140 public List<Reference> getBasedOn() { 1141 if (this.basedOn == null) 1142 this.basedOn = new ArrayList<Reference>(); 1143 return this.basedOn; 1144 } 1145 1146 /** 1147 * @return Returns a reference to <code>this</code> for easy method chaining 1148 */ 1149 public Procedure setBasedOn(List<Reference> theBasedOn) { 1150 this.basedOn = theBasedOn; 1151 return this; 1152 } 1153 1154 public boolean hasBasedOn() { 1155 if (this.basedOn == null) 1156 return false; 1157 for (Reference item : this.basedOn) 1158 if (!item.isEmpty()) 1159 return true; 1160 return false; 1161 } 1162 1163 public Reference addBasedOn() { //3 1164 Reference t = new Reference(); 1165 if (this.basedOn == null) 1166 this.basedOn = new ArrayList<Reference>(); 1167 this.basedOn.add(t); 1168 return t; 1169 } 1170 1171 public Procedure addBasedOn(Reference t) { //3 1172 if (t == null) 1173 return this; 1174 if (this.basedOn == null) 1175 this.basedOn = new ArrayList<Reference>(); 1176 this.basedOn.add(t); 1177 return this; 1178 } 1179 1180 /** 1181 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1182 */ 1183 public Reference getBasedOnFirstRep() { 1184 if (getBasedOn().isEmpty()) { 1185 addBasedOn(); 1186 } 1187 return getBasedOn().get(0); 1188 } 1189 1190 /** 1191 * @deprecated Use Reference#setResource(IBaseResource) instead 1192 */ 1193 @Deprecated 1194 public List<Resource> getBasedOnTarget() { 1195 if (this.basedOnTarget == null) 1196 this.basedOnTarget = new ArrayList<Resource>(); 1197 return this.basedOnTarget; 1198 } 1199 1200 /** 1201 * @return {@link #partOf} (A larger event of which this particular procedure is a component or step.) 1202 */ 1203 public List<Reference> getPartOf() { 1204 if (this.partOf == null) 1205 this.partOf = new ArrayList<Reference>(); 1206 return this.partOf; 1207 } 1208 1209 /** 1210 * @return Returns a reference to <code>this</code> for easy method chaining 1211 */ 1212 public Procedure setPartOf(List<Reference> thePartOf) { 1213 this.partOf = thePartOf; 1214 return this; 1215 } 1216 1217 public boolean hasPartOf() { 1218 if (this.partOf == null) 1219 return false; 1220 for (Reference item : this.partOf) 1221 if (!item.isEmpty()) 1222 return true; 1223 return false; 1224 } 1225 1226 public Reference addPartOf() { //3 1227 Reference t = new Reference(); 1228 if (this.partOf == null) 1229 this.partOf = new ArrayList<Reference>(); 1230 this.partOf.add(t); 1231 return t; 1232 } 1233 1234 public Procedure addPartOf(Reference t) { //3 1235 if (t == null) 1236 return this; 1237 if (this.partOf == null) 1238 this.partOf = new ArrayList<Reference>(); 1239 this.partOf.add(t); 1240 return this; 1241 } 1242 1243 /** 1244 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 1245 */ 1246 public Reference getPartOfFirstRep() { 1247 if (getPartOf().isEmpty()) { 1248 addPartOf(); 1249 } 1250 return getPartOf().get(0); 1251 } 1252 1253 /** 1254 * @deprecated Use Reference#setResource(IBaseResource) instead 1255 */ 1256 @Deprecated 1257 public List<Resource> getPartOfTarget() { 1258 if (this.partOfTarget == null) 1259 this.partOfTarget = new ArrayList<Resource>(); 1260 return this.partOfTarget; 1261 } 1262 1263 /** 1264 * @return {@link #status} (A code specifying the state of the procedure. Generally this will be in-progress or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1265 */ 1266 public Enumeration<ProcedureStatus> getStatusElement() { 1267 if (this.status == null) 1268 if (Configuration.errorOnAutoCreate()) 1269 throw new Error("Attempt to auto-create Procedure.status"); 1270 else if (Configuration.doAutoCreate()) 1271 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); // bb 1272 return this.status; 1273 } 1274 1275 public boolean hasStatusElement() { 1276 return this.status != null && !this.status.isEmpty(); 1277 } 1278 1279 public boolean hasStatus() { 1280 return this.status != null && !this.status.isEmpty(); 1281 } 1282 1283 /** 1284 * @param value {@link #status} (A code specifying the state of the procedure. Generally this will be in-progress or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1285 */ 1286 public Procedure setStatusElement(Enumeration<ProcedureStatus> value) { 1287 this.status = value; 1288 return this; 1289 } 1290 1291 /** 1292 * @return A code specifying the state of the procedure. Generally this will be in-progress or completed state. 1293 */ 1294 public ProcedureStatus getStatus() { 1295 return this.status == null ? null : this.status.getValue(); 1296 } 1297 1298 /** 1299 * @param value A code specifying the state of the procedure. Generally this will be in-progress or completed state. 1300 */ 1301 public Procedure setStatus(ProcedureStatus value) { 1302 if (this.status == null) 1303 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); 1304 this.status.setValue(value); 1305 return this; 1306 } 1307 1308 /** 1309 * @return {@link #notDone} (Set this to true if the record is saying that the procedure was NOT performed.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 1310 */ 1311 public BooleanType getNotDoneElement() { 1312 if (this.notDone == null) 1313 if (Configuration.errorOnAutoCreate()) 1314 throw new Error("Attempt to auto-create Procedure.notDone"); 1315 else if (Configuration.doAutoCreate()) 1316 this.notDone = new BooleanType(); // bb 1317 return this.notDone; 1318 } 1319 1320 public boolean hasNotDoneElement() { 1321 return this.notDone != null && !this.notDone.isEmpty(); 1322 } 1323 1324 public boolean hasNotDone() { 1325 return this.notDone != null && !this.notDone.isEmpty(); 1326 } 1327 1328 /** 1329 * @param value {@link #notDone} (Set this to true if the record is saying that the procedure was NOT performed.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 1330 */ 1331 public Procedure setNotDoneElement(BooleanType value) { 1332 this.notDone = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return Set this to true if the record is saying that the procedure was NOT performed. 1338 */ 1339 public boolean getNotDone() { 1340 return this.notDone == null || this.notDone.isEmpty() ? false : this.notDone.getValue(); 1341 } 1342 1343 /** 1344 * @param value Set this to true if the record is saying that the procedure was NOT performed. 1345 */ 1346 public Procedure setNotDone(boolean value) { 1347 if (this.notDone == null) 1348 this.notDone = new BooleanType(); 1349 this.notDone.setValue(value); 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #notDoneReason} (A code indicating why the procedure was not performed.) 1355 */ 1356 public CodeableConcept getNotDoneReason() { 1357 if (this.notDoneReason == null) 1358 if (Configuration.errorOnAutoCreate()) 1359 throw new Error("Attempt to auto-create Procedure.notDoneReason"); 1360 else if (Configuration.doAutoCreate()) 1361 this.notDoneReason = new CodeableConcept(); // cc 1362 return this.notDoneReason; 1363 } 1364 1365 public boolean hasNotDoneReason() { 1366 return this.notDoneReason != null && !this.notDoneReason.isEmpty(); 1367 } 1368 1369 /** 1370 * @param value {@link #notDoneReason} (A code indicating why the procedure was not performed.) 1371 */ 1372 public Procedure setNotDoneReason(CodeableConcept value) { 1373 this.notDoneReason = value; 1374 return this; 1375 } 1376 1377 /** 1378 * @return {@link #category} (A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1379 */ 1380 public CodeableConcept getCategory() { 1381 if (this.category == null) 1382 if (Configuration.errorOnAutoCreate()) 1383 throw new Error("Attempt to auto-create Procedure.category"); 1384 else if (Configuration.doAutoCreate()) 1385 this.category = new CodeableConcept(); // cc 1386 return this.category; 1387 } 1388 1389 public boolean hasCategory() { 1390 return this.category != null && !this.category.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #category} (A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1395 */ 1396 public Procedure setCategory(CodeableConcept value) { 1397 this.category = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #code} (The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy").) 1403 */ 1404 public CodeableConcept getCode() { 1405 if (this.code == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create Procedure.code"); 1408 else if (Configuration.doAutoCreate()) 1409 this.code = new CodeableConcept(); // cc 1410 return this.code; 1411 } 1412 1413 public boolean hasCode() { 1414 return this.code != null && !this.code.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #code} (The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. "Laparoscopic Appendectomy").) 1419 */ 1420 public Procedure setCode(CodeableConcept value) { 1421 this.code = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #subject} (The person, animal or group on which the procedure was performed.) 1427 */ 1428 public Reference getSubject() { 1429 if (this.subject == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create Procedure.subject"); 1432 else if (Configuration.doAutoCreate()) 1433 this.subject = new Reference(); // cc 1434 return this.subject; 1435 } 1436 1437 public boolean hasSubject() { 1438 return this.subject != null && !this.subject.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #subject} (The person, animal or group on which the procedure was performed.) 1443 */ 1444 public Procedure setSubject(Reference value) { 1445 this.subject = value; 1446 return this; 1447 } 1448 1449 /** 1450 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person, animal or group on which the procedure was performed.) 1451 */ 1452 public Resource getSubjectTarget() { 1453 return this.subjectTarget; 1454 } 1455 1456 /** 1457 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person, animal or group on which the procedure was performed.) 1458 */ 1459 public Procedure setSubjectTarget(Resource value) { 1460 this.subjectTarget = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #context} (The encounter during which the procedure was performed.) 1466 */ 1467 public Reference getContext() { 1468 if (this.context == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create Procedure.context"); 1471 else if (Configuration.doAutoCreate()) 1472 this.context = new Reference(); // cc 1473 return this.context; 1474 } 1475 1476 public boolean hasContext() { 1477 return this.context != null && !this.context.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #context} (The encounter during which the procedure was performed.) 1482 */ 1483 public Procedure setContext(Reference value) { 1484 this.context = value; 1485 return this; 1486 } 1487 1488 /** 1489 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter during which the procedure was performed.) 1490 */ 1491 public Resource getContextTarget() { 1492 return this.contextTarget; 1493 } 1494 1495 /** 1496 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter during which the procedure was performed.) 1497 */ 1498 public Procedure setContextTarget(Resource value) { 1499 this.contextTarget = value; 1500 return this; 1501 } 1502 1503 /** 1504 * @return {@link #performed} (The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1505 */ 1506 public Type getPerformed() { 1507 return this.performed; 1508 } 1509 1510 /** 1511 * @return {@link #performed} (The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1512 */ 1513 public DateTimeType getPerformedDateTimeType() throws FHIRException { 1514 if (this.performed == null) 1515 return null; 1516 if (!(this.performed instanceof DateTimeType)) 1517 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.performed.getClass().getName()+" was encountered"); 1518 return (DateTimeType) this.performed; 1519 } 1520 1521 public boolean hasPerformedDateTimeType() { 1522 return this != null && this.performed instanceof DateTimeType; 1523 } 1524 1525 /** 1526 * @return {@link #performed} (The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1527 */ 1528 public Period getPerformedPeriod() throws FHIRException { 1529 if (this.performed == null) 1530 return null; 1531 if (!(this.performed instanceof Period)) 1532 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.performed.getClass().getName()+" was encountered"); 1533 return (Period) this.performed; 1534 } 1535 1536 public boolean hasPerformedPeriod() { 1537 return this != null && this.performed instanceof Period; 1538 } 1539 1540 public boolean hasPerformed() { 1541 return this.performed != null && !this.performed.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #performed} (The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1546 */ 1547 public Procedure setPerformed(Type value) throws FHIRFormatError { 1548 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1549 throw new FHIRFormatError("Not the right type for Procedure.performed[x]: "+value.fhirType()); 1550 this.performed = value; 1551 return this; 1552 } 1553 1554 /** 1555 * @return {@link #performer} (Limited to 'real' people rather than equipment.) 1556 */ 1557 public List<ProcedurePerformerComponent> getPerformer() { 1558 if (this.performer == null) 1559 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1560 return this.performer; 1561 } 1562 1563 /** 1564 * @return Returns a reference to <code>this</code> for easy method chaining 1565 */ 1566 public Procedure setPerformer(List<ProcedurePerformerComponent> thePerformer) { 1567 this.performer = thePerformer; 1568 return this; 1569 } 1570 1571 public boolean hasPerformer() { 1572 if (this.performer == null) 1573 return false; 1574 for (ProcedurePerformerComponent item : this.performer) 1575 if (!item.isEmpty()) 1576 return true; 1577 return false; 1578 } 1579 1580 public ProcedurePerformerComponent addPerformer() { //3 1581 ProcedurePerformerComponent t = new ProcedurePerformerComponent(); 1582 if (this.performer == null) 1583 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1584 this.performer.add(t); 1585 return t; 1586 } 1587 1588 public Procedure addPerformer(ProcedurePerformerComponent t) { //3 1589 if (t == null) 1590 return this; 1591 if (this.performer == null) 1592 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1593 this.performer.add(t); 1594 return this; 1595 } 1596 1597 /** 1598 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1599 */ 1600 public ProcedurePerformerComponent getPerformerFirstRep() { 1601 if (getPerformer().isEmpty()) { 1602 addPerformer(); 1603 } 1604 return getPerformer().get(0); 1605 } 1606 1607 /** 1608 * @return {@link #location} (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1609 */ 1610 public Reference getLocation() { 1611 if (this.location == null) 1612 if (Configuration.errorOnAutoCreate()) 1613 throw new Error("Attempt to auto-create Procedure.location"); 1614 else if (Configuration.doAutoCreate()) 1615 this.location = new Reference(); // cc 1616 return this.location; 1617 } 1618 1619 public boolean hasLocation() { 1620 return this.location != null && !this.location.isEmpty(); 1621 } 1622 1623 /** 1624 * @param value {@link #location} (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1625 */ 1626 public Procedure setLocation(Reference value) { 1627 this.location = value; 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1633 */ 1634 public Location getLocationTarget() { 1635 if (this.locationTarget == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create Procedure.location"); 1638 else if (Configuration.doAutoCreate()) 1639 this.locationTarget = new Location(); // aa 1640 return this.locationTarget; 1641 } 1642 1643 /** 1644 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1645 */ 1646 public Procedure setLocationTarget(Location value) { 1647 this.locationTarget = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #reasonCode} (The coded reason why the procedure was performed. This may be coded entity of some type, or may simply be present as text.) 1653 */ 1654 public List<CodeableConcept> getReasonCode() { 1655 if (this.reasonCode == null) 1656 this.reasonCode = new ArrayList<CodeableConcept>(); 1657 return this.reasonCode; 1658 } 1659 1660 /** 1661 * @return Returns a reference to <code>this</code> for easy method chaining 1662 */ 1663 public Procedure setReasonCode(List<CodeableConcept> theReasonCode) { 1664 this.reasonCode = theReasonCode; 1665 return this; 1666 } 1667 1668 public boolean hasReasonCode() { 1669 if (this.reasonCode == null) 1670 return false; 1671 for (CodeableConcept item : this.reasonCode) 1672 if (!item.isEmpty()) 1673 return true; 1674 return false; 1675 } 1676 1677 public CodeableConcept addReasonCode() { //3 1678 CodeableConcept t = new CodeableConcept(); 1679 if (this.reasonCode == null) 1680 this.reasonCode = new ArrayList<CodeableConcept>(); 1681 this.reasonCode.add(t); 1682 return t; 1683 } 1684 1685 public Procedure addReasonCode(CodeableConcept t) { //3 1686 if (t == null) 1687 return this; 1688 if (this.reasonCode == null) 1689 this.reasonCode = new ArrayList<CodeableConcept>(); 1690 this.reasonCode.add(t); 1691 return this; 1692 } 1693 1694 /** 1695 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1696 */ 1697 public CodeableConcept getReasonCodeFirstRep() { 1698 if (getReasonCode().isEmpty()) { 1699 addReasonCode(); 1700 } 1701 return getReasonCode().get(0); 1702 } 1703 1704 /** 1705 * @return {@link #reasonReference} (The condition that is the reason why the procedure was performed.) 1706 */ 1707 public List<Reference> getReasonReference() { 1708 if (this.reasonReference == null) 1709 this.reasonReference = new ArrayList<Reference>(); 1710 return this.reasonReference; 1711 } 1712 1713 /** 1714 * @return Returns a reference to <code>this</code> for easy method chaining 1715 */ 1716 public Procedure setReasonReference(List<Reference> theReasonReference) { 1717 this.reasonReference = theReasonReference; 1718 return this; 1719 } 1720 1721 public boolean hasReasonReference() { 1722 if (this.reasonReference == null) 1723 return false; 1724 for (Reference item : this.reasonReference) 1725 if (!item.isEmpty()) 1726 return true; 1727 return false; 1728 } 1729 1730 public Reference addReasonReference() { //3 1731 Reference t = new Reference(); 1732 if (this.reasonReference == null) 1733 this.reasonReference = new ArrayList<Reference>(); 1734 this.reasonReference.add(t); 1735 return t; 1736 } 1737 1738 public Procedure addReasonReference(Reference t) { //3 1739 if (t == null) 1740 return this; 1741 if (this.reasonReference == null) 1742 this.reasonReference = new ArrayList<Reference>(); 1743 this.reasonReference.add(t); 1744 return this; 1745 } 1746 1747 /** 1748 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1749 */ 1750 public Reference getReasonReferenceFirstRep() { 1751 if (getReasonReference().isEmpty()) { 1752 addReasonReference(); 1753 } 1754 return getReasonReference().get(0); 1755 } 1756 1757 /** 1758 * @deprecated Use Reference#setResource(IBaseResource) instead 1759 */ 1760 @Deprecated 1761 public List<Resource> getReasonReferenceTarget() { 1762 if (this.reasonReferenceTarget == null) 1763 this.reasonReferenceTarget = new ArrayList<Resource>(); 1764 return this.reasonReferenceTarget; 1765 } 1766 1767 /** 1768 * @return {@link #bodySite} (Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.) 1769 */ 1770 public List<CodeableConcept> getBodySite() { 1771 if (this.bodySite == null) 1772 this.bodySite = new ArrayList<CodeableConcept>(); 1773 return this.bodySite; 1774 } 1775 1776 /** 1777 * @return Returns a reference to <code>this</code> for easy method chaining 1778 */ 1779 public Procedure setBodySite(List<CodeableConcept> theBodySite) { 1780 this.bodySite = theBodySite; 1781 return this; 1782 } 1783 1784 public boolean hasBodySite() { 1785 if (this.bodySite == null) 1786 return false; 1787 for (CodeableConcept item : this.bodySite) 1788 if (!item.isEmpty()) 1789 return true; 1790 return false; 1791 } 1792 1793 public CodeableConcept addBodySite() { //3 1794 CodeableConcept t = new CodeableConcept(); 1795 if (this.bodySite == null) 1796 this.bodySite = new ArrayList<CodeableConcept>(); 1797 this.bodySite.add(t); 1798 return t; 1799 } 1800 1801 public Procedure addBodySite(CodeableConcept t) { //3 1802 if (t == null) 1803 return this; 1804 if (this.bodySite == null) 1805 this.bodySite = new ArrayList<CodeableConcept>(); 1806 this.bodySite.add(t); 1807 return this; 1808 } 1809 1810 /** 1811 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist 1812 */ 1813 public CodeableConcept getBodySiteFirstRep() { 1814 if (getBodySite().isEmpty()) { 1815 addBodySite(); 1816 } 1817 return getBodySite().get(0); 1818 } 1819 1820 /** 1821 * @return {@link #outcome} (The outcome of the procedure - did it resolve reasons for the procedure being performed?) 1822 */ 1823 public CodeableConcept getOutcome() { 1824 if (this.outcome == null) 1825 if (Configuration.errorOnAutoCreate()) 1826 throw new Error("Attempt to auto-create Procedure.outcome"); 1827 else if (Configuration.doAutoCreate()) 1828 this.outcome = new CodeableConcept(); // cc 1829 return this.outcome; 1830 } 1831 1832 public boolean hasOutcome() { 1833 return this.outcome != null && !this.outcome.isEmpty(); 1834 } 1835 1836 /** 1837 * @param value {@link #outcome} (The outcome of the procedure - did it resolve reasons for the procedure being performed?) 1838 */ 1839 public Procedure setOutcome(CodeableConcept value) { 1840 this.outcome = value; 1841 return this; 1842 } 1843 1844 /** 1845 * @return {@link #report} (This could be a histology result, pathology report, surgical report, etc..) 1846 */ 1847 public List<Reference> getReport() { 1848 if (this.report == null) 1849 this.report = new ArrayList<Reference>(); 1850 return this.report; 1851 } 1852 1853 /** 1854 * @return Returns a reference to <code>this</code> for easy method chaining 1855 */ 1856 public Procedure setReport(List<Reference> theReport) { 1857 this.report = theReport; 1858 return this; 1859 } 1860 1861 public boolean hasReport() { 1862 if (this.report == null) 1863 return false; 1864 for (Reference item : this.report) 1865 if (!item.isEmpty()) 1866 return true; 1867 return false; 1868 } 1869 1870 public Reference addReport() { //3 1871 Reference t = new Reference(); 1872 if (this.report == null) 1873 this.report = new ArrayList<Reference>(); 1874 this.report.add(t); 1875 return t; 1876 } 1877 1878 public Procedure addReport(Reference t) { //3 1879 if (t == null) 1880 return this; 1881 if (this.report == null) 1882 this.report = new ArrayList<Reference>(); 1883 this.report.add(t); 1884 return this; 1885 } 1886 1887 /** 1888 * @return The first repetition of repeating field {@link #report}, creating it if it does not already exist 1889 */ 1890 public Reference getReportFirstRep() { 1891 if (getReport().isEmpty()) { 1892 addReport(); 1893 } 1894 return getReport().get(0); 1895 } 1896 1897 /** 1898 * @deprecated Use Reference#setResource(IBaseResource) instead 1899 */ 1900 @Deprecated 1901 public List<DiagnosticReport> getReportTarget() { 1902 if (this.reportTarget == null) 1903 this.reportTarget = new ArrayList<DiagnosticReport>(); 1904 return this.reportTarget; 1905 } 1906 1907 /** 1908 * @deprecated Use Reference#setResource(IBaseResource) instead 1909 */ 1910 @Deprecated 1911 public DiagnosticReport addReportTarget() { 1912 DiagnosticReport r = new DiagnosticReport(); 1913 if (this.reportTarget == null) 1914 this.reportTarget = new ArrayList<DiagnosticReport>(); 1915 this.reportTarget.add(r); 1916 return r; 1917 } 1918 1919 /** 1920 * @return {@link #complication} (Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.) 1921 */ 1922 public List<CodeableConcept> getComplication() { 1923 if (this.complication == null) 1924 this.complication = new ArrayList<CodeableConcept>(); 1925 return this.complication; 1926 } 1927 1928 /** 1929 * @return Returns a reference to <code>this</code> for easy method chaining 1930 */ 1931 public Procedure setComplication(List<CodeableConcept> theComplication) { 1932 this.complication = theComplication; 1933 return this; 1934 } 1935 1936 public boolean hasComplication() { 1937 if (this.complication == null) 1938 return false; 1939 for (CodeableConcept item : this.complication) 1940 if (!item.isEmpty()) 1941 return true; 1942 return false; 1943 } 1944 1945 public CodeableConcept addComplication() { //3 1946 CodeableConcept t = new CodeableConcept(); 1947 if (this.complication == null) 1948 this.complication = new ArrayList<CodeableConcept>(); 1949 this.complication.add(t); 1950 return t; 1951 } 1952 1953 public Procedure addComplication(CodeableConcept t) { //3 1954 if (t == null) 1955 return this; 1956 if (this.complication == null) 1957 this.complication = new ArrayList<CodeableConcept>(); 1958 this.complication.add(t); 1959 return this; 1960 } 1961 1962 /** 1963 * @return The first repetition of repeating field {@link #complication}, creating it if it does not already exist 1964 */ 1965 public CodeableConcept getComplicationFirstRep() { 1966 if (getComplication().isEmpty()) { 1967 addComplication(); 1968 } 1969 return getComplication().get(0); 1970 } 1971 1972 /** 1973 * @return {@link #complicationDetail} (Any complications that occurred during the procedure, or in the immediate post-performance period.) 1974 */ 1975 public List<Reference> getComplicationDetail() { 1976 if (this.complicationDetail == null) 1977 this.complicationDetail = new ArrayList<Reference>(); 1978 return this.complicationDetail; 1979 } 1980 1981 /** 1982 * @return Returns a reference to <code>this</code> for easy method chaining 1983 */ 1984 public Procedure setComplicationDetail(List<Reference> theComplicationDetail) { 1985 this.complicationDetail = theComplicationDetail; 1986 return this; 1987 } 1988 1989 public boolean hasComplicationDetail() { 1990 if (this.complicationDetail == null) 1991 return false; 1992 for (Reference item : this.complicationDetail) 1993 if (!item.isEmpty()) 1994 return true; 1995 return false; 1996 } 1997 1998 public Reference addComplicationDetail() { //3 1999 Reference t = new Reference(); 2000 if (this.complicationDetail == null) 2001 this.complicationDetail = new ArrayList<Reference>(); 2002 this.complicationDetail.add(t); 2003 return t; 2004 } 2005 2006 public Procedure addComplicationDetail(Reference t) { //3 2007 if (t == null) 2008 return this; 2009 if (this.complicationDetail == null) 2010 this.complicationDetail = new ArrayList<Reference>(); 2011 this.complicationDetail.add(t); 2012 return this; 2013 } 2014 2015 /** 2016 * @return The first repetition of repeating field {@link #complicationDetail}, creating it if it does not already exist 2017 */ 2018 public Reference getComplicationDetailFirstRep() { 2019 if (getComplicationDetail().isEmpty()) { 2020 addComplicationDetail(); 2021 } 2022 return getComplicationDetail().get(0); 2023 } 2024 2025 /** 2026 * @deprecated Use Reference#setResource(IBaseResource) instead 2027 */ 2028 @Deprecated 2029 public List<Condition> getComplicationDetailTarget() { 2030 if (this.complicationDetailTarget == null) 2031 this.complicationDetailTarget = new ArrayList<Condition>(); 2032 return this.complicationDetailTarget; 2033 } 2034 2035 /** 2036 * @deprecated Use Reference#setResource(IBaseResource) instead 2037 */ 2038 @Deprecated 2039 public Condition addComplicationDetailTarget() { 2040 Condition r = new Condition(); 2041 if (this.complicationDetailTarget == null) 2042 this.complicationDetailTarget = new ArrayList<Condition>(); 2043 this.complicationDetailTarget.add(r); 2044 return r; 2045 } 2046 2047 /** 2048 * @return {@link #followUp} (If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used.) 2049 */ 2050 public List<CodeableConcept> getFollowUp() { 2051 if (this.followUp == null) 2052 this.followUp = new ArrayList<CodeableConcept>(); 2053 return this.followUp; 2054 } 2055 2056 /** 2057 * @return Returns a reference to <code>this</code> for easy method chaining 2058 */ 2059 public Procedure setFollowUp(List<CodeableConcept> theFollowUp) { 2060 this.followUp = theFollowUp; 2061 return this; 2062 } 2063 2064 public boolean hasFollowUp() { 2065 if (this.followUp == null) 2066 return false; 2067 for (CodeableConcept item : this.followUp) 2068 if (!item.isEmpty()) 2069 return true; 2070 return false; 2071 } 2072 2073 public CodeableConcept addFollowUp() { //3 2074 CodeableConcept t = new CodeableConcept(); 2075 if (this.followUp == null) 2076 this.followUp = new ArrayList<CodeableConcept>(); 2077 this.followUp.add(t); 2078 return t; 2079 } 2080 2081 public Procedure addFollowUp(CodeableConcept t) { //3 2082 if (t == null) 2083 return this; 2084 if (this.followUp == null) 2085 this.followUp = new ArrayList<CodeableConcept>(); 2086 this.followUp.add(t); 2087 return this; 2088 } 2089 2090 /** 2091 * @return The first repetition of repeating field {@link #followUp}, creating it if it does not already exist 2092 */ 2093 public CodeableConcept getFollowUpFirstRep() { 2094 if (getFollowUp().isEmpty()) { 2095 addFollowUp(); 2096 } 2097 return getFollowUp().get(0); 2098 } 2099 2100 /** 2101 * @return {@link #note} (Any other notes about the procedure. E.g. the operative notes.) 2102 */ 2103 public List<Annotation> getNote() { 2104 if (this.note == null) 2105 this.note = new ArrayList<Annotation>(); 2106 return this.note; 2107 } 2108 2109 /** 2110 * @return Returns a reference to <code>this</code> for easy method chaining 2111 */ 2112 public Procedure setNote(List<Annotation> theNote) { 2113 this.note = theNote; 2114 return this; 2115 } 2116 2117 public boolean hasNote() { 2118 if (this.note == null) 2119 return false; 2120 for (Annotation item : this.note) 2121 if (!item.isEmpty()) 2122 return true; 2123 return false; 2124 } 2125 2126 public Annotation addNote() { //3 2127 Annotation t = new Annotation(); 2128 if (this.note == null) 2129 this.note = new ArrayList<Annotation>(); 2130 this.note.add(t); 2131 return t; 2132 } 2133 2134 public Procedure addNote(Annotation t) { //3 2135 if (t == null) 2136 return this; 2137 if (this.note == null) 2138 this.note = new ArrayList<Annotation>(); 2139 this.note.add(t); 2140 return this; 2141 } 2142 2143 /** 2144 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2145 */ 2146 public Annotation getNoteFirstRep() { 2147 if (getNote().isEmpty()) { 2148 addNote(); 2149 } 2150 return getNote().get(0); 2151 } 2152 2153 /** 2154 * @return {@link #focalDevice} (A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.) 2155 */ 2156 public List<ProcedureFocalDeviceComponent> getFocalDevice() { 2157 if (this.focalDevice == null) 2158 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2159 return this.focalDevice; 2160 } 2161 2162 /** 2163 * @return Returns a reference to <code>this</code> for easy method chaining 2164 */ 2165 public Procedure setFocalDevice(List<ProcedureFocalDeviceComponent> theFocalDevice) { 2166 this.focalDevice = theFocalDevice; 2167 return this; 2168 } 2169 2170 public boolean hasFocalDevice() { 2171 if (this.focalDevice == null) 2172 return false; 2173 for (ProcedureFocalDeviceComponent item : this.focalDevice) 2174 if (!item.isEmpty()) 2175 return true; 2176 return false; 2177 } 2178 2179 public ProcedureFocalDeviceComponent addFocalDevice() { //3 2180 ProcedureFocalDeviceComponent t = new ProcedureFocalDeviceComponent(); 2181 if (this.focalDevice == null) 2182 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2183 this.focalDevice.add(t); 2184 return t; 2185 } 2186 2187 public Procedure addFocalDevice(ProcedureFocalDeviceComponent t) { //3 2188 if (t == null) 2189 return this; 2190 if (this.focalDevice == null) 2191 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2192 this.focalDevice.add(t); 2193 return this; 2194 } 2195 2196 /** 2197 * @return The first repetition of repeating field {@link #focalDevice}, creating it if it does not already exist 2198 */ 2199 public ProcedureFocalDeviceComponent getFocalDeviceFirstRep() { 2200 if (getFocalDevice().isEmpty()) { 2201 addFocalDevice(); 2202 } 2203 return getFocalDevice().get(0); 2204 } 2205 2206 /** 2207 * @return {@link #usedReference} (Identifies medications, devices and any other substance used as part of the procedure.) 2208 */ 2209 public List<Reference> getUsedReference() { 2210 if (this.usedReference == null) 2211 this.usedReference = new ArrayList<Reference>(); 2212 return this.usedReference; 2213 } 2214 2215 /** 2216 * @return Returns a reference to <code>this</code> for easy method chaining 2217 */ 2218 public Procedure setUsedReference(List<Reference> theUsedReference) { 2219 this.usedReference = theUsedReference; 2220 return this; 2221 } 2222 2223 public boolean hasUsedReference() { 2224 if (this.usedReference == null) 2225 return false; 2226 for (Reference item : this.usedReference) 2227 if (!item.isEmpty()) 2228 return true; 2229 return false; 2230 } 2231 2232 public Reference addUsedReference() { //3 2233 Reference t = new Reference(); 2234 if (this.usedReference == null) 2235 this.usedReference = new ArrayList<Reference>(); 2236 this.usedReference.add(t); 2237 return t; 2238 } 2239 2240 public Procedure addUsedReference(Reference t) { //3 2241 if (t == null) 2242 return this; 2243 if (this.usedReference == null) 2244 this.usedReference = new ArrayList<Reference>(); 2245 this.usedReference.add(t); 2246 return this; 2247 } 2248 2249 /** 2250 * @return The first repetition of repeating field {@link #usedReference}, creating it if it does not already exist 2251 */ 2252 public Reference getUsedReferenceFirstRep() { 2253 if (getUsedReference().isEmpty()) { 2254 addUsedReference(); 2255 } 2256 return getUsedReference().get(0); 2257 } 2258 2259 /** 2260 * @deprecated Use Reference#setResource(IBaseResource) instead 2261 */ 2262 @Deprecated 2263 public List<Resource> getUsedReferenceTarget() { 2264 if (this.usedReferenceTarget == null) 2265 this.usedReferenceTarget = new ArrayList<Resource>(); 2266 return this.usedReferenceTarget; 2267 } 2268 2269 /** 2270 * @return {@link #usedCode} (Identifies coded items that were used as part of the procedure.) 2271 */ 2272 public List<CodeableConcept> getUsedCode() { 2273 if (this.usedCode == null) 2274 this.usedCode = new ArrayList<CodeableConcept>(); 2275 return this.usedCode; 2276 } 2277 2278 /** 2279 * @return Returns a reference to <code>this</code> for easy method chaining 2280 */ 2281 public Procedure setUsedCode(List<CodeableConcept> theUsedCode) { 2282 this.usedCode = theUsedCode; 2283 return this; 2284 } 2285 2286 public boolean hasUsedCode() { 2287 if (this.usedCode == null) 2288 return false; 2289 for (CodeableConcept item : this.usedCode) 2290 if (!item.isEmpty()) 2291 return true; 2292 return false; 2293 } 2294 2295 public CodeableConcept addUsedCode() { //3 2296 CodeableConcept t = new CodeableConcept(); 2297 if (this.usedCode == null) 2298 this.usedCode = new ArrayList<CodeableConcept>(); 2299 this.usedCode.add(t); 2300 return t; 2301 } 2302 2303 public Procedure addUsedCode(CodeableConcept t) { //3 2304 if (t == null) 2305 return this; 2306 if (this.usedCode == null) 2307 this.usedCode = new ArrayList<CodeableConcept>(); 2308 this.usedCode.add(t); 2309 return this; 2310 } 2311 2312 /** 2313 * @return The first repetition of repeating field {@link #usedCode}, creating it if it does not already exist 2314 */ 2315 public CodeableConcept getUsedCodeFirstRep() { 2316 if (getUsedCode().isEmpty()) { 2317 addUsedCode(); 2318 } 2319 return getUsedCode().get(0); 2320 } 2321 2322 protected void listChildren(List<Property> children) { 2323 super.listChildren(children); 2324 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 2325 children.add(new Property("definition", "Reference(PlanDefinition|ActivityDefinition|HealthcareService)", "A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure.", 0, java.lang.Integer.MAX_VALUE, definition)); 2326 children.add(new Property("basedOn", "Reference(CarePlan|ProcedureRequest|ReferralRequest)", "A reference to a resource that contains details of the request for this procedure.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2327 children.add(new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2328 children.add(new Property("status", "code", "A code specifying the state of the procedure. Generally this will be in-progress or completed state.", 0, 1, status)); 2329 children.add(new Property("notDone", "boolean", "Set this to true if the record is saying that the procedure was NOT performed.", 0, 1, notDone)); 2330 children.add(new Property("notDoneReason", "CodeableConcept", "A code indicating why the procedure was not performed.", 0, 1, notDoneReason)); 2331 children.add(new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, 1, category)); 2332 children.add(new Property("code", "CodeableConcept", "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 0, 1, code)); 2333 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group on which the procedure was performed.", 0, 1, subject)); 2334 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter during which the procedure was performed.", 0, 1, context)); 2335 children.add(new Property("performed[x]", "dateTime|Period", "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed)); 2336 children.add(new Property("performer", "", "Limited to 'real' people rather than equipment.", 0, java.lang.Integer.MAX_VALUE, performer)); 2337 children.add(new Property("location", "Reference(Location)", "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 0, 1, location)); 2338 children.add(new Property("reasonCode", "CodeableConcept", "The coded reason why the procedure was performed. This may be coded entity of some type, or may simply be present as text.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2339 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "The condition that is the reason why the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2340 children.add(new Property("bodySite", "CodeableConcept", "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2341 children.add(new Property("outcome", "CodeableConcept", "The outcome of the procedure - did it resolve reasons for the procedure being performed?", 0, 1, outcome)); 2342 children.add(new Property("report", "Reference(DiagnosticReport)", "This could be a histology result, pathology report, surgical report, etc..", 0, java.lang.Integer.MAX_VALUE, report)); 2343 children.add(new Property("complication", "CodeableConcept", "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 0, java.lang.Integer.MAX_VALUE, complication)); 2344 children.add(new Property("complicationDetail", "Reference(Condition)", "Any complications that occurred during the procedure, or in the immediate post-performance period.", 0, java.lang.Integer.MAX_VALUE, complicationDetail)); 2345 children.add(new Property("followUp", "CodeableConcept", "If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used.", 0, java.lang.Integer.MAX_VALUE, followUp)); 2346 children.add(new Property("note", "Annotation", "Any other notes about the procedure. E.g. the operative notes.", 0, java.lang.Integer.MAX_VALUE, note)); 2347 children.add(new Property("focalDevice", "", "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 0, java.lang.Integer.MAX_VALUE, focalDevice)); 2348 children.add(new Property("usedReference", "Reference(Device|Medication|Substance)", "Identifies medications, devices and any other substance used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedReference)); 2349 children.add(new Property("usedCode", "CodeableConcept", "Identifies coded items that were used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedCode)); 2350 } 2351 2352 @Override 2353 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2354 switch (_hash) { 2355 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 2356 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|ActivityDefinition|HealthcareService)", "A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this procedure.", 0, java.lang.Integer.MAX_VALUE, definition); 2357 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ProcedureRequest|ReferralRequest)", "A reference to a resource that contains details of the request for this procedure.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2358 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2359 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the procedure. Generally this will be in-progress or completed state.", 0, 1, status); 2360 case 2128257269: /*notDone*/ return new Property("notDone", "boolean", "Set this to true if the record is saying that the procedure was NOT performed.", 0, 1, notDone); 2361 case -1973169255: /*notDoneReason*/ return new Property("notDoneReason", "CodeableConcept", "A code indicating why the procedure was not performed.", 0, 1, notDoneReason); 2362 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, 1, category); 2363 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 0, 1, code); 2364 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group on which the procedure was performed.", 0, 1, subject); 2365 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter during which the procedure was performed.", 0, 1, context); 2366 case 1355984064: /*performed[x]*/ return new Property("performed[x]", "dateTime|Period", "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 2367 case 481140672: /*performed*/ return new Property("performed[x]", "dateTime|Period", "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 2368 case 1118270331: /*performedDateTime*/ return new Property("performed[x]", "dateTime|Period", "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 2369 case 1622094241: /*performedPeriod*/ return new Property("performed[x]", "dateTime|Period", "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 2370 case 481140686: /*performer*/ return new Property("performer", "", "Limited to 'real' people rather than equipment.", 0, java.lang.Integer.MAX_VALUE, performer); 2371 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 0, 1, location); 2372 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "The coded reason why the procedure was performed. This may be coded entity of some type, or may simply be present as text.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2373 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "The condition that is the reason why the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2374 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2375 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "The outcome of the procedure - did it resolve reasons for the procedure being performed?", 0, 1, outcome); 2376 case -934521548: /*report*/ return new Property("report", "Reference(DiagnosticReport)", "This could be a histology result, pathology report, surgical report, etc..", 0, java.lang.Integer.MAX_VALUE, report); 2377 case -1644401602: /*complication*/ return new Property("complication", "CodeableConcept", "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 0, java.lang.Integer.MAX_VALUE, complication); 2378 case -1685272017: /*complicationDetail*/ return new Property("complicationDetail", "Reference(Condition)", "Any complications that occurred during the procedure, or in the immediate post-performance period.", 0, java.lang.Integer.MAX_VALUE, complicationDetail); 2379 case 301801004: /*followUp*/ return new Property("followUp", "CodeableConcept", "If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used.", 0, java.lang.Integer.MAX_VALUE, followUp); 2380 case 3387378: /*note*/ return new Property("note", "Annotation", "Any other notes about the procedure. E.g. the operative notes.", 0, java.lang.Integer.MAX_VALUE, note); 2381 case -1129235173: /*focalDevice*/ return new Property("focalDevice", "", "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 0, java.lang.Integer.MAX_VALUE, focalDevice); 2382 case -504932338: /*usedReference*/ return new Property("usedReference", "Reference(Device|Medication|Substance)", "Identifies medications, devices and any other substance used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedReference); 2383 case -279910582: /*usedCode*/ return new Property("usedCode", "CodeableConcept", "Identifies coded items that were used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedCode); 2384 default: return super.getNamedProperty(_hash, _name, _checkValid); 2385 } 2386 2387 } 2388 2389 @Override 2390 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2391 switch (hash) { 2392 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2393 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2394 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2395 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2396 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ProcedureStatus> 2397 case 2128257269: /*notDone*/ return this.notDone == null ? new Base[0] : new Base[] {this.notDone}; // BooleanType 2398 case -1973169255: /*notDoneReason*/ return this.notDoneReason == null ? new Base[0] : new Base[] {this.notDoneReason}; // CodeableConcept 2399 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2400 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2401 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2402 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2403 case 481140672: /*performed*/ return this.performed == null ? new Base[0] : new Base[] {this.performed}; // Type 2404 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ProcedurePerformerComponent 2405 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2406 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2407 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2408 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2409 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 2410 case -934521548: /*report*/ return this.report == null ? new Base[0] : this.report.toArray(new Base[this.report.size()]); // Reference 2411 case -1644401602: /*complication*/ return this.complication == null ? new Base[0] : this.complication.toArray(new Base[this.complication.size()]); // CodeableConcept 2412 case -1685272017: /*complicationDetail*/ return this.complicationDetail == null ? new Base[0] : this.complicationDetail.toArray(new Base[this.complicationDetail.size()]); // Reference 2413 case 301801004: /*followUp*/ return this.followUp == null ? new Base[0] : this.followUp.toArray(new Base[this.followUp.size()]); // CodeableConcept 2414 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2415 case -1129235173: /*focalDevice*/ return this.focalDevice == null ? new Base[0] : this.focalDevice.toArray(new Base[this.focalDevice.size()]); // ProcedureFocalDeviceComponent 2416 case -504932338: /*usedReference*/ return this.usedReference == null ? new Base[0] : this.usedReference.toArray(new Base[this.usedReference.size()]); // Reference 2417 case -279910582: /*usedCode*/ return this.usedCode == null ? new Base[0] : this.usedCode.toArray(new Base[this.usedCode.size()]); // CodeableConcept 2418 default: return super.getProperty(hash, name, checkValid); 2419 } 2420 2421 } 2422 2423 @Override 2424 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2425 switch (hash) { 2426 case -1618432855: // identifier 2427 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2428 return value; 2429 case -1014418093: // definition 2430 this.getDefinition().add(castToReference(value)); // Reference 2431 return value; 2432 case -332612366: // basedOn 2433 this.getBasedOn().add(castToReference(value)); // Reference 2434 return value; 2435 case -995410646: // partOf 2436 this.getPartOf().add(castToReference(value)); // Reference 2437 return value; 2438 case -892481550: // status 2439 value = new ProcedureStatusEnumFactory().fromType(castToCode(value)); 2440 this.status = (Enumeration) value; // Enumeration<ProcedureStatus> 2441 return value; 2442 case 2128257269: // notDone 2443 this.notDone = castToBoolean(value); // BooleanType 2444 return value; 2445 case -1973169255: // notDoneReason 2446 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 2447 return value; 2448 case 50511102: // category 2449 this.category = castToCodeableConcept(value); // CodeableConcept 2450 return value; 2451 case 3059181: // code 2452 this.code = castToCodeableConcept(value); // CodeableConcept 2453 return value; 2454 case -1867885268: // subject 2455 this.subject = castToReference(value); // Reference 2456 return value; 2457 case 951530927: // context 2458 this.context = castToReference(value); // Reference 2459 return value; 2460 case 481140672: // performed 2461 this.performed = castToType(value); // Type 2462 return value; 2463 case 481140686: // performer 2464 this.getPerformer().add((ProcedurePerformerComponent) value); // ProcedurePerformerComponent 2465 return value; 2466 case 1901043637: // location 2467 this.location = castToReference(value); // Reference 2468 return value; 2469 case 722137681: // reasonCode 2470 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2471 return value; 2472 case -1146218137: // reasonReference 2473 this.getReasonReference().add(castToReference(value)); // Reference 2474 return value; 2475 case 1702620169: // bodySite 2476 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 2477 return value; 2478 case -1106507950: // outcome 2479 this.outcome = castToCodeableConcept(value); // CodeableConcept 2480 return value; 2481 case -934521548: // report 2482 this.getReport().add(castToReference(value)); // Reference 2483 return value; 2484 case -1644401602: // complication 2485 this.getComplication().add(castToCodeableConcept(value)); // CodeableConcept 2486 return value; 2487 case -1685272017: // complicationDetail 2488 this.getComplicationDetail().add(castToReference(value)); // Reference 2489 return value; 2490 case 301801004: // followUp 2491 this.getFollowUp().add(castToCodeableConcept(value)); // CodeableConcept 2492 return value; 2493 case 3387378: // note 2494 this.getNote().add(castToAnnotation(value)); // Annotation 2495 return value; 2496 case -1129235173: // focalDevice 2497 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); // ProcedureFocalDeviceComponent 2498 return value; 2499 case -504932338: // usedReference 2500 this.getUsedReference().add(castToReference(value)); // Reference 2501 return value; 2502 case -279910582: // usedCode 2503 this.getUsedCode().add(castToCodeableConcept(value)); // CodeableConcept 2504 return value; 2505 default: return super.setProperty(hash, name, value); 2506 } 2507 2508 } 2509 2510 @Override 2511 public Base setProperty(String name, Base value) throws FHIRException { 2512 if (name.equals("identifier")) { 2513 this.getIdentifier().add(castToIdentifier(value)); 2514 } else if (name.equals("definition")) { 2515 this.getDefinition().add(castToReference(value)); 2516 } else if (name.equals("basedOn")) { 2517 this.getBasedOn().add(castToReference(value)); 2518 } else if (name.equals("partOf")) { 2519 this.getPartOf().add(castToReference(value)); 2520 } else if (name.equals("status")) { 2521 value = new ProcedureStatusEnumFactory().fromType(castToCode(value)); 2522 this.status = (Enumeration) value; // Enumeration<ProcedureStatus> 2523 } else if (name.equals("notDone")) { 2524 this.notDone = castToBoolean(value); // BooleanType 2525 } else if (name.equals("notDoneReason")) { 2526 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 2527 } else if (name.equals("category")) { 2528 this.category = castToCodeableConcept(value); // CodeableConcept 2529 } else if (name.equals("code")) { 2530 this.code = castToCodeableConcept(value); // CodeableConcept 2531 } else if (name.equals("subject")) { 2532 this.subject = castToReference(value); // Reference 2533 } else if (name.equals("context")) { 2534 this.context = castToReference(value); // Reference 2535 } else if (name.equals("performed[x]")) { 2536 this.performed = castToType(value); // Type 2537 } else if (name.equals("performer")) { 2538 this.getPerformer().add((ProcedurePerformerComponent) value); 2539 } else if (name.equals("location")) { 2540 this.location = castToReference(value); // Reference 2541 } else if (name.equals("reasonCode")) { 2542 this.getReasonCode().add(castToCodeableConcept(value)); 2543 } else if (name.equals("reasonReference")) { 2544 this.getReasonReference().add(castToReference(value)); 2545 } else if (name.equals("bodySite")) { 2546 this.getBodySite().add(castToCodeableConcept(value)); 2547 } else if (name.equals("outcome")) { 2548 this.outcome = castToCodeableConcept(value); // CodeableConcept 2549 } else if (name.equals("report")) { 2550 this.getReport().add(castToReference(value)); 2551 } else if (name.equals("complication")) { 2552 this.getComplication().add(castToCodeableConcept(value)); 2553 } else if (name.equals("complicationDetail")) { 2554 this.getComplicationDetail().add(castToReference(value)); 2555 } else if (name.equals("followUp")) { 2556 this.getFollowUp().add(castToCodeableConcept(value)); 2557 } else if (name.equals("note")) { 2558 this.getNote().add(castToAnnotation(value)); 2559 } else if (name.equals("focalDevice")) { 2560 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); 2561 } else if (name.equals("usedReference")) { 2562 this.getUsedReference().add(castToReference(value)); 2563 } else if (name.equals("usedCode")) { 2564 this.getUsedCode().add(castToCodeableConcept(value)); 2565 } else 2566 return super.setProperty(name, value); 2567 return value; 2568 } 2569 2570 @Override 2571 public Base makeProperty(int hash, String name) throws FHIRException { 2572 switch (hash) { 2573 case -1618432855: return addIdentifier(); 2574 case -1014418093: return addDefinition(); 2575 case -332612366: return addBasedOn(); 2576 case -995410646: return addPartOf(); 2577 case -892481550: return getStatusElement(); 2578 case 2128257269: return getNotDoneElement(); 2579 case -1973169255: return getNotDoneReason(); 2580 case 50511102: return getCategory(); 2581 case 3059181: return getCode(); 2582 case -1867885268: return getSubject(); 2583 case 951530927: return getContext(); 2584 case 1355984064: return getPerformed(); 2585 case 481140672: return getPerformed(); 2586 case 481140686: return addPerformer(); 2587 case 1901043637: return getLocation(); 2588 case 722137681: return addReasonCode(); 2589 case -1146218137: return addReasonReference(); 2590 case 1702620169: return addBodySite(); 2591 case -1106507950: return getOutcome(); 2592 case -934521548: return addReport(); 2593 case -1644401602: return addComplication(); 2594 case -1685272017: return addComplicationDetail(); 2595 case 301801004: return addFollowUp(); 2596 case 3387378: return addNote(); 2597 case -1129235173: return addFocalDevice(); 2598 case -504932338: return addUsedReference(); 2599 case -279910582: return addUsedCode(); 2600 default: return super.makeProperty(hash, name); 2601 } 2602 2603 } 2604 2605 @Override 2606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2607 switch (hash) { 2608 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2609 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2610 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2611 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2612 case -892481550: /*status*/ return new String[] {"code"}; 2613 case 2128257269: /*notDone*/ return new String[] {"boolean"}; 2614 case -1973169255: /*notDoneReason*/ return new String[] {"CodeableConcept"}; 2615 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2616 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2617 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2618 case 951530927: /*context*/ return new String[] {"Reference"}; 2619 case 481140672: /*performed*/ return new String[] {"dateTime", "Period"}; 2620 case 481140686: /*performer*/ return new String[] {}; 2621 case 1901043637: /*location*/ return new String[] {"Reference"}; 2622 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2623 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2624 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 2625 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 2626 case -934521548: /*report*/ return new String[] {"Reference"}; 2627 case -1644401602: /*complication*/ return new String[] {"CodeableConcept"}; 2628 case -1685272017: /*complicationDetail*/ return new String[] {"Reference"}; 2629 case 301801004: /*followUp*/ return new String[] {"CodeableConcept"}; 2630 case 3387378: /*note*/ return new String[] {"Annotation"}; 2631 case -1129235173: /*focalDevice*/ return new String[] {}; 2632 case -504932338: /*usedReference*/ return new String[] {"Reference"}; 2633 case -279910582: /*usedCode*/ return new String[] {"CodeableConcept"}; 2634 default: return super.getTypesForProperty(hash, name); 2635 } 2636 2637 } 2638 2639 @Override 2640 public Base addChild(String name) throws FHIRException { 2641 if (name.equals("identifier")) { 2642 return addIdentifier(); 2643 } 2644 else if (name.equals("definition")) { 2645 return addDefinition(); 2646 } 2647 else if (name.equals("basedOn")) { 2648 return addBasedOn(); 2649 } 2650 else if (name.equals("partOf")) { 2651 return addPartOf(); 2652 } 2653 else if (name.equals("status")) { 2654 throw new FHIRException("Cannot call addChild on a singleton property Procedure.status"); 2655 } 2656 else if (name.equals("notDone")) { 2657 throw new FHIRException("Cannot call addChild on a singleton property Procedure.notDone"); 2658 } 2659 else if (name.equals("notDoneReason")) { 2660 this.notDoneReason = new CodeableConcept(); 2661 return this.notDoneReason; 2662 } 2663 else if (name.equals("category")) { 2664 this.category = new CodeableConcept(); 2665 return this.category; 2666 } 2667 else if (name.equals("code")) { 2668 this.code = new CodeableConcept(); 2669 return this.code; 2670 } 2671 else if (name.equals("subject")) { 2672 this.subject = new Reference(); 2673 return this.subject; 2674 } 2675 else if (name.equals("context")) { 2676 this.context = new Reference(); 2677 return this.context; 2678 } 2679 else if (name.equals("performedDateTime")) { 2680 this.performed = new DateTimeType(); 2681 return this.performed; 2682 } 2683 else if (name.equals("performedPeriod")) { 2684 this.performed = new Period(); 2685 return this.performed; 2686 } 2687 else if (name.equals("performer")) { 2688 return addPerformer(); 2689 } 2690 else if (name.equals("location")) { 2691 this.location = new Reference(); 2692 return this.location; 2693 } 2694 else if (name.equals("reasonCode")) { 2695 return addReasonCode(); 2696 } 2697 else if (name.equals("reasonReference")) { 2698 return addReasonReference(); 2699 } 2700 else if (name.equals("bodySite")) { 2701 return addBodySite(); 2702 } 2703 else if (name.equals("outcome")) { 2704 this.outcome = new CodeableConcept(); 2705 return this.outcome; 2706 } 2707 else if (name.equals("report")) { 2708 return addReport(); 2709 } 2710 else if (name.equals("complication")) { 2711 return addComplication(); 2712 } 2713 else if (name.equals("complicationDetail")) { 2714 return addComplicationDetail(); 2715 } 2716 else if (name.equals("followUp")) { 2717 return addFollowUp(); 2718 } 2719 else if (name.equals("note")) { 2720 return addNote(); 2721 } 2722 else if (name.equals("focalDevice")) { 2723 return addFocalDevice(); 2724 } 2725 else if (name.equals("usedReference")) { 2726 return addUsedReference(); 2727 } 2728 else if (name.equals("usedCode")) { 2729 return addUsedCode(); 2730 } 2731 else 2732 return super.addChild(name); 2733 } 2734 2735 public String fhirType() { 2736 return "Procedure"; 2737 2738 } 2739 2740 public Procedure copy() { 2741 Procedure dst = new Procedure(); 2742 copyValues(dst); 2743 if (identifier != null) { 2744 dst.identifier = new ArrayList<Identifier>(); 2745 for (Identifier i : identifier) 2746 dst.identifier.add(i.copy()); 2747 }; 2748 if (definition != null) { 2749 dst.definition = new ArrayList<Reference>(); 2750 for (Reference i : definition) 2751 dst.definition.add(i.copy()); 2752 }; 2753 if (basedOn != null) { 2754 dst.basedOn = new ArrayList<Reference>(); 2755 for (Reference i : basedOn) 2756 dst.basedOn.add(i.copy()); 2757 }; 2758 if (partOf != null) { 2759 dst.partOf = new ArrayList<Reference>(); 2760 for (Reference i : partOf) 2761 dst.partOf.add(i.copy()); 2762 }; 2763 dst.status = status == null ? null : status.copy(); 2764 dst.notDone = notDone == null ? null : notDone.copy(); 2765 dst.notDoneReason = notDoneReason == null ? null : notDoneReason.copy(); 2766 dst.category = category == null ? null : category.copy(); 2767 dst.code = code == null ? null : code.copy(); 2768 dst.subject = subject == null ? null : subject.copy(); 2769 dst.context = context == null ? null : context.copy(); 2770 dst.performed = performed == null ? null : performed.copy(); 2771 if (performer != null) { 2772 dst.performer = new ArrayList<ProcedurePerformerComponent>(); 2773 for (ProcedurePerformerComponent i : performer) 2774 dst.performer.add(i.copy()); 2775 }; 2776 dst.location = location == null ? null : location.copy(); 2777 if (reasonCode != null) { 2778 dst.reasonCode = new ArrayList<CodeableConcept>(); 2779 for (CodeableConcept i : reasonCode) 2780 dst.reasonCode.add(i.copy()); 2781 }; 2782 if (reasonReference != null) { 2783 dst.reasonReference = new ArrayList<Reference>(); 2784 for (Reference i : reasonReference) 2785 dst.reasonReference.add(i.copy()); 2786 }; 2787 if (bodySite != null) { 2788 dst.bodySite = new ArrayList<CodeableConcept>(); 2789 for (CodeableConcept i : bodySite) 2790 dst.bodySite.add(i.copy()); 2791 }; 2792 dst.outcome = outcome == null ? null : outcome.copy(); 2793 if (report != null) { 2794 dst.report = new ArrayList<Reference>(); 2795 for (Reference i : report) 2796 dst.report.add(i.copy()); 2797 }; 2798 if (complication != null) { 2799 dst.complication = new ArrayList<CodeableConcept>(); 2800 for (CodeableConcept i : complication) 2801 dst.complication.add(i.copy()); 2802 }; 2803 if (complicationDetail != null) { 2804 dst.complicationDetail = new ArrayList<Reference>(); 2805 for (Reference i : complicationDetail) 2806 dst.complicationDetail.add(i.copy()); 2807 }; 2808 if (followUp != null) { 2809 dst.followUp = new ArrayList<CodeableConcept>(); 2810 for (CodeableConcept i : followUp) 2811 dst.followUp.add(i.copy()); 2812 }; 2813 if (note != null) { 2814 dst.note = new ArrayList<Annotation>(); 2815 for (Annotation i : note) 2816 dst.note.add(i.copy()); 2817 }; 2818 if (focalDevice != null) { 2819 dst.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2820 for (ProcedureFocalDeviceComponent i : focalDevice) 2821 dst.focalDevice.add(i.copy()); 2822 }; 2823 if (usedReference != null) { 2824 dst.usedReference = new ArrayList<Reference>(); 2825 for (Reference i : usedReference) 2826 dst.usedReference.add(i.copy()); 2827 }; 2828 if (usedCode != null) { 2829 dst.usedCode = new ArrayList<CodeableConcept>(); 2830 for (CodeableConcept i : usedCode) 2831 dst.usedCode.add(i.copy()); 2832 }; 2833 return dst; 2834 } 2835 2836 protected Procedure typedCopy() { 2837 return copy(); 2838 } 2839 2840 @Override 2841 public boolean equalsDeep(Base other_) { 2842 if (!super.equalsDeep(other_)) 2843 return false; 2844 if (!(other_ instanceof Procedure)) 2845 return false; 2846 Procedure o = (Procedure) other_; 2847 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2848 && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2849 && compareDeep(notDone, o.notDone, true) && compareDeep(notDoneReason, o.notDoneReason, true) && compareDeep(category, o.category, true) 2850 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 2851 && compareDeep(performed, o.performed, true) && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) 2852 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2853 && compareDeep(bodySite, o.bodySite, true) && compareDeep(outcome, o.outcome, true) && compareDeep(report, o.report, true) 2854 && compareDeep(complication, o.complication, true) && compareDeep(complicationDetail, o.complicationDetail, true) 2855 && compareDeep(followUp, o.followUp, true) && compareDeep(note, o.note, true) && compareDeep(focalDevice, o.focalDevice, true) 2856 && compareDeep(usedReference, o.usedReference, true) && compareDeep(usedCode, o.usedCode, true) 2857 ; 2858 } 2859 2860 @Override 2861 public boolean equalsShallow(Base other_) { 2862 if (!super.equalsShallow(other_)) 2863 return false; 2864 if (!(other_ instanceof Procedure)) 2865 return false; 2866 Procedure o = (Procedure) other_; 2867 return compareValues(status, o.status, true) && compareValues(notDone, o.notDone, true); 2868 } 2869 2870 public boolean isEmpty() { 2871 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2872 , partOf, status, notDone, notDoneReason, category, code, subject, context, performed 2873 , performer, location, reasonCode, reasonReference, bodySite, outcome, report 2874 , complication, complicationDetail, followUp, note, focalDevice, usedReference, usedCode 2875 ); 2876 } 2877 2878 @Override 2879 public ResourceType getResourceType() { 2880 return ResourceType.Procedure; 2881 } 2882 2883 /** 2884 * Search parameter: <b>date</b> 2885 * <p> 2886 * Description: <b>Date/Period the procedure was performed</b><br> 2887 * Type: <b>date</b><br> 2888 * Path: <b>Procedure.performed[x]</b><br> 2889 * </p> 2890 */ 2891 @SearchParamDefinition(name="date", path="Procedure.performed", description="Date/Period the procedure was performed", type="date" ) 2892 public static final String SP_DATE = "date"; 2893 /** 2894 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2895 * <p> 2896 * Description: <b>Date/Period the procedure was performed</b><br> 2897 * Type: <b>date</b><br> 2898 * Path: <b>Procedure.performed[x]</b><br> 2899 * </p> 2900 */ 2901 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2902 2903 /** 2904 * Search parameter: <b>identifier</b> 2905 * <p> 2906 * Description: <b>A unique identifier for a procedure</b><br> 2907 * Type: <b>token</b><br> 2908 * Path: <b>Procedure.identifier</b><br> 2909 * </p> 2910 */ 2911 @SearchParamDefinition(name="identifier", path="Procedure.identifier", description="A unique identifier for a procedure", type="token" ) 2912 public static final String SP_IDENTIFIER = "identifier"; 2913 /** 2914 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2915 * <p> 2916 * Description: <b>A unique identifier for a procedure</b><br> 2917 * Type: <b>token</b><br> 2918 * Path: <b>Procedure.identifier</b><br> 2919 * </p> 2920 */ 2921 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2922 2923 /** 2924 * Search parameter: <b>code</b> 2925 * <p> 2926 * Description: <b>A code to identify a procedure</b><br> 2927 * Type: <b>token</b><br> 2928 * Path: <b>Procedure.code</b><br> 2929 * </p> 2930 */ 2931 @SearchParamDefinition(name="code", path="Procedure.code", description="A code to identify a procedure", type="token" ) 2932 public static final String SP_CODE = "code"; 2933 /** 2934 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2935 * <p> 2936 * Description: <b>A code to identify a procedure</b><br> 2937 * Type: <b>token</b><br> 2938 * Path: <b>Procedure.code</b><br> 2939 * </p> 2940 */ 2941 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2942 2943 /** 2944 * Search parameter: <b>performer</b> 2945 * <p> 2946 * Description: <b>The reference to the practitioner</b><br> 2947 * Type: <b>reference</b><br> 2948 * Path: <b>Procedure.performer.actor</b><br> 2949 * </p> 2950 */ 2951 @SearchParamDefinition(name="performer", path="Procedure.performer.actor", description="The reference to the practitioner", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2952 public static final String SP_PERFORMER = "performer"; 2953 /** 2954 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2955 * <p> 2956 * Description: <b>The reference to the practitioner</b><br> 2957 * Type: <b>reference</b><br> 2958 * Path: <b>Procedure.performer.actor</b><br> 2959 * </p> 2960 */ 2961 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2962 2963/** 2964 * Constant for fluent queries to be used to add include statements. Specifies 2965 * the path value of "<b>Procedure:performer</b>". 2966 */ 2967 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Procedure:performer").toLocked(); 2968 2969 /** 2970 * Search parameter: <b>subject</b> 2971 * <p> 2972 * Description: <b>Search by subject</b><br> 2973 * Type: <b>reference</b><br> 2974 * Path: <b>Procedure.subject</b><br> 2975 * </p> 2976 */ 2977 @SearchParamDefinition(name="subject", path="Procedure.subject", description="Search by subject", type="reference", target={Group.class, Patient.class } ) 2978 public static final String SP_SUBJECT = "subject"; 2979 /** 2980 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2981 * <p> 2982 * Description: <b>Search by subject</b><br> 2983 * Type: <b>reference</b><br> 2984 * Path: <b>Procedure.subject</b><br> 2985 * </p> 2986 */ 2987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2988 2989/** 2990 * Constant for fluent queries to be used to add include statements. Specifies 2991 * the path value of "<b>Procedure:subject</b>". 2992 */ 2993 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Procedure:subject").toLocked(); 2994 2995 /** 2996 * Search parameter: <b>part-of</b> 2997 * <p> 2998 * Description: <b>Part of referenced event</b><br> 2999 * Type: <b>reference</b><br> 3000 * Path: <b>Procedure.partOf</b><br> 3001 * </p> 3002 */ 3003 @SearchParamDefinition(name="part-of", path="Procedure.partOf", description="Part of referenced event", type="reference", target={MedicationAdministration.class, Observation.class, Procedure.class } ) 3004 public static final String SP_PART_OF = "part-of"; 3005 /** 3006 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 3007 * <p> 3008 * Description: <b>Part of referenced event</b><br> 3009 * Type: <b>reference</b><br> 3010 * Path: <b>Procedure.partOf</b><br> 3011 * </p> 3012 */ 3013 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 3014 3015/** 3016 * Constant for fluent queries to be used to add include statements. Specifies 3017 * the path value of "<b>Procedure:part-of</b>". 3018 */ 3019 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Procedure:part-of").toLocked(); 3020 3021 /** 3022 * Search parameter: <b>encounter</b> 3023 * <p> 3024 * Description: <b>Search by encounter</b><br> 3025 * Type: <b>reference</b><br> 3026 * Path: <b>Procedure.context</b><br> 3027 * </p> 3028 */ 3029 @SearchParamDefinition(name="encounter", path="Procedure.context", description="Search by encounter", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 3030 public static final String SP_ENCOUNTER = "encounter"; 3031 /** 3032 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3033 * <p> 3034 * Description: <b>Search by encounter</b><br> 3035 * Type: <b>reference</b><br> 3036 * Path: <b>Procedure.context</b><br> 3037 * </p> 3038 */ 3039 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3040 3041/** 3042 * Constant for fluent queries to be used to add include statements. Specifies 3043 * the path value of "<b>Procedure:encounter</b>". 3044 */ 3045 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Procedure:encounter").toLocked(); 3046 3047 /** 3048 * Search parameter: <b>based-on</b> 3049 * <p> 3050 * Description: <b>A request for this procedure</b><br> 3051 * Type: <b>reference</b><br> 3052 * Path: <b>Procedure.basedOn</b><br> 3053 * </p> 3054 */ 3055 @SearchParamDefinition(name="based-on", path="Procedure.basedOn", description="A request for this procedure", type="reference", target={CarePlan.class, ProcedureRequest.class, ReferralRequest.class } ) 3056 public static final String SP_BASED_ON = "based-on"; 3057 /** 3058 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3059 * <p> 3060 * Description: <b>A request for this procedure</b><br> 3061 * Type: <b>reference</b><br> 3062 * Path: <b>Procedure.basedOn</b><br> 3063 * </p> 3064 */ 3065 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3066 3067/** 3068 * Constant for fluent queries to be used to add include statements. Specifies 3069 * the path value of "<b>Procedure:based-on</b>". 3070 */ 3071 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Procedure:based-on").toLocked(); 3072 3073 /** 3074 * Search parameter: <b>patient</b> 3075 * <p> 3076 * Description: <b>Search by subject - a patient</b><br> 3077 * Type: <b>reference</b><br> 3078 * Path: <b>Procedure.subject</b><br> 3079 * </p> 3080 */ 3081 @SearchParamDefinition(name="patient", path="Procedure.subject", description="Search by subject - a patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3082 public static final String SP_PATIENT = "patient"; 3083 /** 3084 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3085 * <p> 3086 * Description: <b>Search by subject - a patient</b><br> 3087 * Type: <b>reference</b><br> 3088 * Path: <b>Procedure.subject</b><br> 3089 * </p> 3090 */ 3091 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3092 3093/** 3094 * Constant for fluent queries to be used to add include statements. Specifies 3095 * the path value of "<b>Procedure:patient</b>". 3096 */ 3097 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Procedure:patient").toLocked(); 3098 3099 /** 3100 * Search parameter: <b>context</b> 3101 * <p> 3102 * Description: <b>Encounter or episode associated with the procedure</b><br> 3103 * Type: <b>reference</b><br> 3104 * Path: <b>Procedure.context</b><br> 3105 * </p> 3106 */ 3107 @SearchParamDefinition(name="context", path="Procedure.context", description="Encounter or episode associated with the procedure", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 3108 public static final String SP_CONTEXT = "context"; 3109 /** 3110 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3111 * <p> 3112 * Description: <b>Encounter or episode associated with the procedure</b><br> 3113 * Type: <b>reference</b><br> 3114 * Path: <b>Procedure.context</b><br> 3115 * </p> 3116 */ 3117 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3118 3119/** 3120 * Constant for fluent queries to be used to add include statements. Specifies 3121 * the path value of "<b>Procedure:context</b>". 3122 */ 3123 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Procedure:context").toLocked(); 3124 3125 /** 3126 * Search parameter: <b>location</b> 3127 * <p> 3128 * Description: <b>Where the procedure happened</b><br> 3129 * Type: <b>reference</b><br> 3130 * Path: <b>Procedure.location</b><br> 3131 * </p> 3132 */ 3133 @SearchParamDefinition(name="location", path="Procedure.location", description="Where the procedure happened", type="reference", target={Location.class } ) 3134 public static final String SP_LOCATION = "location"; 3135 /** 3136 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3137 * <p> 3138 * Description: <b>Where the procedure happened</b><br> 3139 * Type: <b>reference</b><br> 3140 * Path: <b>Procedure.location</b><br> 3141 * </p> 3142 */ 3143 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3144 3145/** 3146 * Constant for fluent queries to be used to add include statements. Specifies 3147 * the path value of "<b>Procedure:location</b>". 3148 */ 3149 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Procedure:location").toLocked(); 3150 3151 /** 3152 * Search parameter: <b>definition</b> 3153 * <p> 3154 * Description: <b>Instantiates protocol or definition</b><br> 3155 * Type: <b>reference</b><br> 3156 * Path: <b>Procedure.definition</b><br> 3157 * </p> 3158 */ 3159 @SearchParamDefinition(name="definition", path="Procedure.definition", description="Instantiates protocol or definition", type="reference", target={ActivityDefinition.class, HealthcareService.class, PlanDefinition.class } ) 3160 public static final String SP_DEFINITION = "definition"; 3161 /** 3162 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 3163 * <p> 3164 * Description: <b>Instantiates protocol or definition</b><br> 3165 * Type: <b>reference</b><br> 3166 * Path: <b>Procedure.definition</b><br> 3167 * </p> 3168 */ 3169 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 3170 3171/** 3172 * Constant for fluent queries to be used to add include statements. Specifies 3173 * the path value of "<b>Procedure:definition</b>". 3174 */ 3175 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("Procedure:definition").toLocked(); 3176 3177 /** 3178 * Search parameter: <b>category</b> 3179 * <p> 3180 * Description: <b>Classification of the procedure</b><br> 3181 * Type: <b>token</b><br> 3182 * Path: <b>Procedure.category</b><br> 3183 * </p> 3184 */ 3185 @SearchParamDefinition(name="category", path="Procedure.category", description="Classification of the procedure", type="token" ) 3186 public static final String SP_CATEGORY = "category"; 3187 /** 3188 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3189 * <p> 3190 * Description: <b>Classification of the procedure</b><br> 3191 * Type: <b>token</b><br> 3192 * Path: <b>Procedure.category</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3196 3197 /** 3198 * Search parameter: <b>status</b> 3199 * <p> 3200 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error | unknown</b><br> 3201 * Type: <b>token</b><br> 3202 * Path: <b>Procedure.status</b><br> 3203 * </p> 3204 */ 3205 @SearchParamDefinition(name="status", path="Procedure.status", description="preparation | in-progress | suspended | aborted | completed | entered-in-error | unknown", type="token" ) 3206 public static final String SP_STATUS = "status"; 3207 /** 3208 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3209 * <p> 3210 * Description: <b>preparation | in-progress | suspended | aborted | completed | entered-in-error | unknown</b><br> 3211 * Type: <b>token</b><br> 3212 * Path: <b>Procedure.status</b><br> 3213 * </p> 3214 */ 3215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3216 3217 3218}