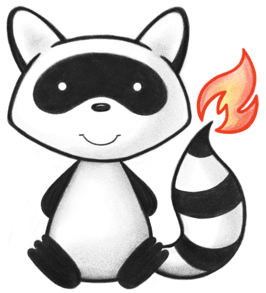
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 050 */ 051@ResourceDef(name="ProcedureRequest", profile="http://hl7.org/fhir/Profile/ProcedureRequest") 052public class ProcedureRequest extends DomainResource { 053 054 public enum ProcedureRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action 057 */ 058 DRAFT, 059 /** 060 * The request is ready to be acted upon 061 */ 062 ACTIVE, 063 /** 064 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 069 */ 070 CANCELLED, 071 /** 072 * Activity against the request has been sufficiently completed to the satisfaction of the requester 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static ProcedureRequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case CANCELLED: return "cancelled"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/request-status"; 125 case ACTIVE: return "http://hl7.org/fhir/request-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 127 case CANCELLED: return "http://hl7.org/fhir/request-status"; 128 case COMPLETED: return "http://hl7.org/fhir/request-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 138 case ACTIVE: return "The request is ready to be acted upon"; 139 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 140 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 141 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Draft"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case CANCELLED: return "Cancelled"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class ProcedureRequestStatusEnumFactory implements EnumFactory<ProcedureRequestStatus> { 164 public ProcedureRequestStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return ProcedureRequestStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return ProcedureRequestStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return ProcedureRequestStatus.SUSPENDED; 174 if ("cancelled".equals(codeString)) 175 return ProcedureRequestStatus.CANCELLED; 176 if ("completed".equals(codeString)) 177 return ProcedureRequestStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return ProcedureRequestStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return ProcedureRequestStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 183 } 184 public Enumeration<ProcedureRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<ProcedureRequestStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.SUSPENDED); 198 if ("cancelled".equals(codeString)) 199 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.CANCELLED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.UNKNOWN); 206 throw new FHIRException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 207 } 208 public String toCode(ProcedureRequestStatus code) { 209 if (code == ProcedureRequestStatus.NULL) 210 return null; 211 if (code == ProcedureRequestStatus.DRAFT) 212 return "draft"; 213 if (code == ProcedureRequestStatus.ACTIVE) 214 return "active"; 215 if (code == ProcedureRequestStatus.SUSPENDED) 216 return "suspended"; 217 if (code == ProcedureRequestStatus.CANCELLED) 218 return "cancelled"; 219 if (code == ProcedureRequestStatus.COMPLETED) 220 return "completed"; 221 if (code == ProcedureRequestStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == ProcedureRequestStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(ProcedureRequestStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum ProcedureRequestIntent { 233 /** 234 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 235 */ 236 PROPOSAL, 237 /** 238 * The request represents an intension to ensure something occurs without providing an authorization for others to act 239 */ 240 PLAN, 241 /** 242 * The request represents a request/demand and authorization for action 243 */ 244 ORDER, 245 /** 246 * The request represents an original authorization for action 247 */ 248 ORIGINALORDER, 249 /** 250 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 251 */ 252 REFLEXORDER, 253 /** 254 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 255 */ 256 FILLERORDER, 257 /** 258 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 259 */ 260 INSTANCEORDER, 261 /** 262 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 263 264Refer to [[[RequestGroup]]] for additional information on how this status is used 265 */ 266 OPTION, 267 /** 268 * added to help the parsers with the generic types 269 */ 270 NULL; 271 public static ProcedureRequestIntent fromCode(String codeString) throws FHIRException { 272 if (codeString == null || "".equals(codeString)) 273 return null; 274 if ("proposal".equals(codeString)) 275 return PROPOSAL; 276 if ("plan".equals(codeString)) 277 return PLAN; 278 if ("order".equals(codeString)) 279 return ORDER; 280 if ("original-order".equals(codeString)) 281 return ORIGINALORDER; 282 if ("reflex-order".equals(codeString)) 283 return REFLEXORDER; 284 if ("filler-order".equals(codeString)) 285 return FILLERORDER; 286 if ("instance-order".equals(codeString)) 287 return INSTANCEORDER; 288 if ("option".equals(codeString)) 289 return OPTION; 290 if (Configuration.isAcceptInvalidEnums()) 291 return null; 292 else 293 throw new FHIRException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 294 } 295 public String toCode() { 296 switch (this) { 297 case PROPOSAL: return "proposal"; 298 case PLAN: return "plan"; 299 case ORDER: return "order"; 300 case ORIGINALORDER: return "original-order"; 301 case REFLEXORDER: return "reflex-order"; 302 case FILLERORDER: return "filler-order"; 303 case INSTANCEORDER: return "instance-order"; 304 case OPTION: return "option"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 public String getSystem() { 310 switch (this) { 311 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 312 case PLAN: return "http://hl7.org/fhir/request-intent"; 313 case ORDER: return "http://hl7.org/fhir/request-intent"; 314 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 315 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 316 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 317 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 318 case OPTION: return "http://hl7.org/fhir/request-intent"; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getDefinition() { 324 switch (this) { 325 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 326 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 327 case ORDER: return "The request represents a request/demand and authorization for action"; 328 case ORIGINALORDER: return "The request represents an original authorization for action"; 329 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 330 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 331 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 332 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 333 case NULL: return null; 334 default: return "?"; 335 } 336 } 337 public String getDisplay() { 338 switch (this) { 339 case PROPOSAL: return "Proposal"; 340 case PLAN: return "Plan"; 341 case ORDER: return "Order"; 342 case ORIGINALORDER: return "Original Order"; 343 case REFLEXORDER: return "Reflex Order"; 344 case FILLERORDER: return "Filler Order"; 345 case INSTANCEORDER: return "Instance Order"; 346 case OPTION: return "Option"; 347 case NULL: return null; 348 default: return "?"; 349 } 350 } 351 } 352 353 public static class ProcedureRequestIntentEnumFactory implements EnumFactory<ProcedureRequestIntent> { 354 public ProcedureRequestIntent fromCode(String codeString) throws IllegalArgumentException { 355 if (codeString == null || "".equals(codeString)) 356 if (codeString == null || "".equals(codeString)) 357 return null; 358 if ("proposal".equals(codeString)) 359 return ProcedureRequestIntent.PROPOSAL; 360 if ("plan".equals(codeString)) 361 return ProcedureRequestIntent.PLAN; 362 if ("order".equals(codeString)) 363 return ProcedureRequestIntent.ORDER; 364 if ("original-order".equals(codeString)) 365 return ProcedureRequestIntent.ORIGINALORDER; 366 if ("reflex-order".equals(codeString)) 367 return ProcedureRequestIntent.REFLEXORDER; 368 if ("filler-order".equals(codeString)) 369 return ProcedureRequestIntent.FILLERORDER; 370 if ("instance-order".equals(codeString)) 371 return ProcedureRequestIntent.INSTANCEORDER; 372 if ("option".equals(codeString)) 373 return ProcedureRequestIntent.OPTION; 374 throw new IllegalArgumentException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 375 } 376 public Enumeration<ProcedureRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 377 if (code == null) 378 return null; 379 if (code.isEmpty()) 380 return new Enumeration<ProcedureRequestIntent>(this); 381 String codeString = code.asStringValue(); 382 if (codeString == null || "".equals(codeString)) 383 return null; 384 if ("proposal".equals(codeString)) 385 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.PROPOSAL); 386 if ("plan".equals(codeString)) 387 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.PLAN); 388 if ("order".equals(codeString)) 389 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.ORDER); 390 if ("original-order".equals(codeString)) 391 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.ORIGINALORDER); 392 if ("reflex-order".equals(codeString)) 393 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.REFLEXORDER); 394 if ("filler-order".equals(codeString)) 395 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.FILLERORDER); 396 if ("instance-order".equals(codeString)) 397 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.INSTANCEORDER); 398 if ("option".equals(codeString)) 399 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.OPTION); 400 throw new FHIRException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 401 } 402 public String toCode(ProcedureRequestIntent code) { 403 if (code == ProcedureRequestIntent.NULL) 404 return null; 405 if (code == ProcedureRequestIntent.PROPOSAL) 406 return "proposal"; 407 if (code == ProcedureRequestIntent.PLAN) 408 return "plan"; 409 if (code == ProcedureRequestIntent.ORDER) 410 return "order"; 411 if (code == ProcedureRequestIntent.ORIGINALORDER) 412 return "original-order"; 413 if (code == ProcedureRequestIntent.REFLEXORDER) 414 return "reflex-order"; 415 if (code == ProcedureRequestIntent.FILLERORDER) 416 return "filler-order"; 417 if (code == ProcedureRequestIntent.INSTANCEORDER) 418 return "instance-order"; 419 if (code == ProcedureRequestIntent.OPTION) 420 return "option"; 421 return "?"; 422 } 423 public String toSystem(ProcedureRequestIntent code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum ProcedureRequestPriority { 429 /** 430 * The request has normal priority 431 */ 432 ROUTINE, 433 /** 434 * The request should be actioned promptly - higher priority than routine 435 */ 436 URGENT, 437 /** 438 * The request should be actioned as soon as possible - higher priority than urgent 439 */ 440 ASAP, 441 /** 442 * The request should be actioned immediately - highest possible priority. E.g. an emergency 443 */ 444 STAT, 445 /** 446 * added to help the parsers with the generic types 447 */ 448 NULL; 449 public static ProcedureRequestPriority fromCode(String codeString) throws FHIRException { 450 if (codeString == null || "".equals(codeString)) 451 return null; 452 if ("routine".equals(codeString)) 453 return ROUTINE; 454 if ("urgent".equals(codeString)) 455 return URGENT; 456 if ("asap".equals(codeString)) 457 return ASAP; 458 if ("stat".equals(codeString)) 459 return STAT; 460 if (Configuration.isAcceptInvalidEnums()) 461 return null; 462 else 463 throw new FHIRException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 464 } 465 public String toCode() { 466 switch (this) { 467 case ROUTINE: return "routine"; 468 case URGENT: return "urgent"; 469 case ASAP: return "asap"; 470 case STAT: return "stat"; 471 case NULL: return null; 472 default: return "?"; 473 } 474 } 475 public String getSystem() { 476 switch (this) { 477 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 478 case URGENT: return "http://hl7.org/fhir/request-priority"; 479 case ASAP: return "http://hl7.org/fhir/request-priority"; 480 case STAT: return "http://hl7.org/fhir/request-priority"; 481 case NULL: return null; 482 default: return "?"; 483 } 484 } 485 public String getDefinition() { 486 switch (this) { 487 case ROUTINE: return "The request has normal priority"; 488 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 489 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 490 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 491 case NULL: return null; 492 default: return "?"; 493 } 494 } 495 public String getDisplay() { 496 switch (this) { 497 case ROUTINE: return "Routine"; 498 case URGENT: return "Urgent"; 499 case ASAP: return "ASAP"; 500 case STAT: return "STAT"; 501 case NULL: return null; 502 default: return "?"; 503 } 504 } 505 } 506 507 public static class ProcedureRequestPriorityEnumFactory implements EnumFactory<ProcedureRequestPriority> { 508 public ProcedureRequestPriority fromCode(String codeString) throws IllegalArgumentException { 509 if (codeString == null || "".equals(codeString)) 510 if (codeString == null || "".equals(codeString)) 511 return null; 512 if ("routine".equals(codeString)) 513 return ProcedureRequestPriority.ROUTINE; 514 if ("urgent".equals(codeString)) 515 return ProcedureRequestPriority.URGENT; 516 if ("asap".equals(codeString)) 517 return ProcedureRequestPriority.ASAP; 518 if ("stat".equals(codeString)) 519 return ProcedureRequestPriority.STAT; 520 throw new IllegalArgumentException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 521 } 522 public Enumeration<ProcedureRequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 523 if (code == null) 524 return null; 525 if (code.isEmpty()) 526 return new Enumeration<ProcedureRequestPriority>(this); 527 String codeString = code.asStringValue(); 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("routine".equals(codeString)) 531 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ROUTINE); 532 if ("urgent".equals(codeString)) 533 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.URGENT); 534 if ("asap".equals(codeString)) 535 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ASAP); 536 if ("stat".equals(codeString)) 537 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.STAT); 538 throw new FHIRException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 539 } 540 public String toCode(ProcedureRequestPriority code) { 541 if (code == ProcedureRequestPriority.NULL) 542 return null; 543 if (code == ProcedureRequestPriority.ROUTINE) 544 return "routine"; 545 if (code == ProcedureRequestPriority.URGENT) 546 return "urgent"; 547 if (code == ProcedureRequestPriority.ASAP) 548 return "asap"; 549 if (code == ProcedureRequestPriority.STAT) 550 return "stat"; 551 return "?"; 552 } 553 public String toSystem(ProcedureRequestPriority code) { 554 return code.getSystem(); 555 } 556 } 557 558 @Block() 559 public static class ProcedureRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 560 /** 561 * The device, practitioner or organization who initiated the request. 562 */ 563 @Child(name = "agent", type = {Device.class, Practitioner.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=true) 564 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner or organization who initiated the request." ) 565 protected Reference agent; 566 567 /** 568 * The actual object that is the target of the reference (The device, practitioner or organization who initiated the request.) 569 */ 570 protected Resource agentTarget; 571 572 /** 573 * The organization the device or practitioner was acting on behalf of. 574 */ 575 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 576 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 577 protected Reference onBehalfOf; 578 579 /** 580 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 581 */ 582 protected Organization onBehalfOfTarget; 583 584 private static final long serialVersionUID = -71453027L; 585 586 /** 587 * Constructor 588 */ 589 public ProcedureRequestRequesterComponent() { 590 super(); 591 } 592 593 /** 594 * Constructor 595 */ 596 public ProcedureRequestRequesterComponent(Reference agent) { 597 super(); 598 this.agent = agent; 599 } 600 601 /** 602 * @return {@link #agent} (The device, practitioner or organization who initiated the request.) 603 */ 604 public Reference getAgent() { 605 if (this.agent == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.agent"); 608 else if (Configuration.doAutoCreate()) 609 this.agent = new Reference(); // cc 610 return this.agent; 611 } 612 613 public boolean hasAgent() { 614 return this.agent != null && !this.agent.isEmpty(); 615 } 616 617 /** 618 * @param value {@link #agent} (The device, practitioner or organization who initiated the request.) 619 */ 620 public ProcedureRequestRequesterComponent setAgent(Reference value) { 621 this.agent = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner or organization who initiated the request.) 627 */ 628 public Resource getAgentTarget() { 629 return this.agentTarget; 630 } 631 632 /** 633 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner or organization who initiated the request.) 634 */ 635 public ProcedureRequestRequesterComponent setAgentTarget(Resource value) { 636 this.agentTarget = value; 637 return this; 638 } 639 640 /** 641 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 642 */ 643 public Reference getOnBehalfOf() { 644 if (this.onBehalfOf == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.onBehalfOf"); 647 else if (Configuration.doAutoCreate()) 648 this.onBehalfOf = new Reference(); // cc 649 return this.onBehalfOf; 650 } 651 652 public boolean hasOnBehalfOf() { 653 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 658 */ 659 public ProcedureRequestRequesterComponent setOnBehalfOf(Reference value) { 660 this.onBehalfOf = value; 661 return this; 662 } 663 664 /** 665 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 666 */ 667 public Organization getOnBehalfOfTarget() { 668 if (this.onBehalfOfTarget == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.onBehalfOf"); 671 else if (Configuration.doAutoCreate()) 672 this.onBehalfOfTarget = new Organization(); // aa 673 return this.onBehalfOfTarget; 674 } 675 676 /** 677 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 678 */ 679 public ProcedureRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 680 this.onBehalfOfTarget = value; 681 return this; 682 } 683 684 protected void listChildren(List<Property> children) { 685 super.listChildren(children); 686 children.add(new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner or organization who initiated the request.", 0, 1, agent)); 687 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 688 } 689 690 @Override 691 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 692 switch (_hash) { 693 case 92750597: /*agent*/ return new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner or organization who initiated the request.", 0, 1, agent); 694 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 695 default: return super.getNamedProperty(_hash, _name, _checkValid); 696 } 697 698 } 699 700 @Override 701 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 702 switch (hash) { 703 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 704 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 705 default: return super.getProperty(hash, name, checkValid); 706 } 707 708 } 709 710 @Override 711 public Base setProperty(int hash, String name, Base value) throws FHIRException { 712 switch (hash) { 713 case 92750597: // agent 714 this.agent = castToReference(value); // Reference 715 return value; 716 case -14402964: // onBehalfOf 717 this.onBehalfOf = castToReference(value); // Reference 718 return value; 719 default: return super.setProperty(hash, name, value); 720 } 721 722 } 723 724 @Override 725 public Base setProperty(String name, Base value) throws FHIRException { 726 if (name.equals("agent")) { 727 this.agent = castToReference(value); // Reference 728 } else if (name.equals("onBehalfOf")) { 729 this.onBehalfOf = castToReference(value); // Reference 730 } else 731 return super.setProperty(name, value); 732 return value; 733 } 734 735 @Override 736 public Base makeProperty(int hash, String name) throws FHIRException { 737 switch (hash) { 738 case 92750597: return getAgent(); 739 case -14402964: return getOnBehalfOf(); 740 default: return super.makeProperty(hash, name); 741 } 742 743 } 744 745 @Override 746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 747 switch (hash) { 748 case 92750597: /*agent*/ return new String[] {"Reference"}; 749 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 750 default: return super.getTypesForProperty(hash, name); 751 } 752 753 } 754 755 @Override 756 public Base addChild(String name) throws FHIRException { 757 if (name.equals("agent")) { 758 this.agent = new Reference(); 759 return this.agent; 760 } 761 else if (name.equals("onBehalfOf")) { 762 this.onBehalfOf = new Reference(); 763 return this.onBehalfOf; 764 } 765 else 766 return super.addChild(name); 767 } 768 769 public ProcedureRequestRequesterComponent copy() { 770 ProcedureRequestRequesterComponent dst = new ProcedureRequestRequesterComponent(); 771 copyValues(dst); 772 dst.agent = agent == null ? null : agent.copy(); 773 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 774 return dst; 775 } 776 777 @Override 778 public boolean equalsDeep(Base other_) { 779 if (!super.equalsDeep(other_)) 780 return false; 781 if (!(other_ instanceof ProcedureRequestRequesterComponent)) 782 return false; 783 ProcedureRequestRequesterComponent o = (ProcedureRequestRequesterComponent) other_; 784 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 785 } 786 787 @Override 788 public boolean equalsShallow(Base other_) { 789 if (!super.equalsShallow(other_)) 790 return false; 791 if (!(other_ instanceof ProcedureRequestRequesterComponent)) 792 return false; 793 ProcedureRequestRequesterComponent o = (ProcedureRequestRequesterComponent) other_; 794 return true; 795 } 796 797 public boolean isEmpty() { 798 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 799 } 800 801 public String fhirType() { 802 return "ProcedureRequest.requester"; 803 804 } 805 806 } 807 808 /** 809 * Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller. 810 */ 811 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 812 @Description(shortDefinition="Identifiers assigned to this order", formalDefinition="Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller." ) 813 protected List<Identifier> identifier; 814 815 /** 816 * Protocol or definition followed by this request. 817 */ 818 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 819 @Description(shortDefinition="Protocol or definition", formalDefinition="Protocol or definition followed by this request." ) 820 protected List<Reference> definition; 821 /** 822 * The actual objects that are the target of the reference (Protocol or definition followed by this request.) 823 */ 824 protected List<Resource> definitionTarget; 825 826 827 /** 828 * Plan/proposal/order fulfilled by this request. 829 */ 830 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 831 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 832 protected List<Reference> basedOn; 833 /** 834 * The actual objects that are the target of the reference (Plan/proposal/order fulfilled by this request.) 835 */ 836 protected List<Resource> basedOnTarget; 837 838 839 /** 840 * The request takes the place of the referenced completed or terminated request(s). 841 */ 842 @Child(name = "replaces", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 843 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 844 protected List<Reference> replaces; 845 /** 846 * The actual objects that are the target of the reference (The request takes the place of the referenced completed or terminated request(s).) 847 */ 848 protected List<Resource> replacesTarget; 849 850 851 /** 852 * A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier. 853 */ 854 @Child(name = "requisition", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 855 @Description(shortDefinition="Composite Request ID", formalDefinition="A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier." ) 856 protected Identifier requisition; 857 858 /** 859 * The status of the order. 860 */ 861 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 862 @Description(shortDefinition="draft | active | suspended | completed | entered-in-error | cancelled", formalDefinition="The status of the order." ) 863 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 864 protected Enumeration<ProcedureRequestStatus> status; 865 866 /** 867 * Whether the request is a proposal, plan, an original order or a reflex order. 868 */ 869 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 870 @Description(shortDefinition="proposal | plan | order +", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 871 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 872 protected Enumeration<ProcedureRequestIntent> intent; 873 874 /** 875 * Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 876 */ 877 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 878 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the ProcedureRequest should be addressed with respect to other requests." ) 879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 880 protected Enumeration<ProcedureRequestPriority> priority; 881 882 /** 883 * Set this to true if the record is saying that the procedure should NOT be performed. 884 */ 885 @Child(name = "doNotPerform", type = {BooleanType.class}, order=8, min=0, max=1, modifier=true, summary=true) 886 @Description(shortDefinition="True if procedure should not be performed", formalDefinition="Set this to true if the record is saying that the procedure should NOT be performed." ) 887 protected BooleanType doNotPerform; 888 889 /** 890 * A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure"). 891 */ 892 @Child(name = "category", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 893 @Description(shortDefinition="Classification of procedure", formalDefinition="A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\")." ) 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-category") 895 protected List<CodeableConcept> category; 896 897 /** 898 * A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested. 899 */ 900 @Child(name = "code", type = {CodeableConcept.class}, order=10, min=1, max=1, modifier=false, summary=true) 901 @Description(shortDefinition="What is being requested/ordered", formalDefinition="A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 903 protected CodeableConcept code; 904 905 /** 906 * On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans). 907 */ 908 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=11, min=1, max=1, modifier=false, summary=true) 909 @Description(shortDefinition="Individual the service is ordered for", formalDefinition="On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans)." ) 910 protected Reference subject; 911 912 /** 913 * The actual object that is the target of the reference (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 914 */ 915 protected Resource subjectTarget; 916 917 /** 918 * An encounter or episode of care that provides additional information about the healthcare context in which this request is made. 919 */ 920 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=12, min=0, max=1, modifier=false, summary=true) 921 @Description(shortDefinition="Encounter or Episode during which request was created", formalDefinition="An encounter or episode of care that provides additional information about the healthcare context in which this request is made." ) 922 protected Reference context; 923 924 /** 925 * The actual object that is the target of the reference (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 926 */ 927 protected Resource contextTarget; 928 929 /** 930 * The date/time at which the diagnostic testing should occur. 931 */ 932 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=13, min=0, max=1, modifier=false, summary=true) 933 @Description(shortDefinition="When procedure should occur", formalDefinition="The date/time at which the diagnostic testing should occur." ) 934 protected Type occurrence; 935 936 /** 937 * If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc. 938 */ 939 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=true) 940 @Description(shortDefinition="Preconditions for procedure or diagnostic", formalDefinition="If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 942 protected Type asNeeded; 943 944 /** 945 * When the request transitioned to being actionable. 946 */ 947 @Child(name = "authoredOn", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="Date request signed", formalDefinition="When the request transitioned to being actionable." ) 949 protected DateTimeType authoredOn; 950 951 /** 952 * The individual who initiated the request and has responsibility for its activation. 953 */ 954 @Child(name = "requester", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 955 @Description(shortDefinition="Who/what is requesting procedure or diagnostic", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 956 protected ProcedureRequestRequesterComponent requester; 957 958 /** 959 * Desired type of performer for doing the diagnostic testing. 960 */ 961 @Child(name = "performerType", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 962 @Description(shortDefinition="Performer role", formalDefinition="Desired type of performer for doing the diagnostic testing." ) 963 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 964 protected CodeableConcept performerType; 965 966 /** 967 * The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc. 968 */ 969 @Child(name = "performer", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, HealthcareService.class}, order=18, min=0, max=1, modifier=false, summary=true) 970 @Description(shortDefinition="Requested perfomer", formalDefinition="The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc." ) 971 protected Reference performer; 972 973 /** 974 * The actual object that is the target of the reference (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 975 */ 976 protected Resource performerTarget; 977 978 /** 979 * An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation. 980 */ 981 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 982 @Description(shortDefinition="Explanation/Justification for test", formalDefinition="An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation." ) 983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 984 protected List<CodeableConcept> reasonCode; 985 986 /** 987 * Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation. 988 */ 989 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 990 @Description(shortDefinition="Explanation/Justification for test", formalDefinition="Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation." ) 991 protected List<Reference> reasonReference; 992 /** 993 * The actual objects that are the target of the reference (Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.) 994 */ 995 protected List<Resource> reasonReferenceTarget; 996 997 998 /** 999 * Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements. 1000 */ 1001 @Child(name = "supportingInfo", type = {Reference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1002 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements." ) 1003 protected List<Reference> supportingInfo; 1004 /** 1005 * The actual objects that are the target of the reference (Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.) 1006 */ 1007 protected List<Resource> supportingInfoTarget; 1008 1009 1010 /** 1011 * One or more specimens that the laboratory procedure will use. 1012 */ 1013 @Child(name = "specimen", type = {Specimen.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1014 @Description(shortDefinition="Procedure Samples", formalDefinition="One or more specimens that the laboratory procedure will use." ) 1015 protected List<Reference> specimen; 1016 /** 1017 * The actual objects that are the target of the reference (One or more specimens that the laboratory procedure will use.) 1018 */ 1019 protected List<Specimen> specimenTarget; 1020 1021 1022 /** 1023 * Anatomic location where the procedure should be performed. This is the target site. 1024 */ 1025 @Child(name = "bodySite", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1026 @Description(shortDefinition="Location on Body", formalDefinition="Anatomic location where the procedure should be performed. This is the target site." ) 1027 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1028 protected List<CodeableConcept> bodySite; 1029 1030 /** 1031 * Any other notes and comments made about the service request. For example, letting provider know that "patient hates needles" or other provider instructions. 1032 */ 1033 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1034 @Description(shortDefinition="Comments", formalDefinition="Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions." ) 1035 protected List<Annotation> note; 1036 1037 /** 1038 * Key events in the history of the request. 1039 */ 1040 @Child(name = "relevantHistory", type = {Provenance.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1041 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 1042 protected List<Reference> relevantHistory; 1043 /** 1044 * The actual objects that are the target of the reference (Key events in the history of the request.) 1045 */ 1046 protected List<Provenance> relevantHistoryTarget; 1047 1048 1049 private static final long serialVersionUID = 184396216L; 1050 1051 /** 1052 * Constructor 1053 */ 1054 public ProcedureRequest() { 1055 super(); 1056 } 1057 1058 /** 1059 * Constructor 1060 */ 1061 public ProcedureRequest(Enumeration<ProcedureRequestStatus> status, Enumeration<ProcedureRequestIntent> intent, CodeableConcept code, Reference subject) { 1062 super(); 1063 this.status = status; 1064 this.intent = intent; 1065 this.code = code; 1066 this.subject = subject; 1067 } 1068 1069 /** 1070 * @return {@link #identifier} (Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.) 1071 */ 1072 public List<Identifier> getIdentifier() { 1073 if (this.identifier == null) 1074 this.identifier = new ArrayList<Identifier>(); 1075 return this.identifier; 1076 } 1077 1078 /** 1079 * @return Returns a reference to <code>this</code> for easy method chaining 1080 */ 1081 public ProcedureRequest setIdentifier(List<Identifier> theIdentifier) { 1082 this.identifier = theIdentifier; 1083 return this; 1084 } 1085 1086 public boolean hasIdentifier() { 1087 if (this.identifier == null) 1088 return false; 1089 for (Identifier item : this.identifier) 1090 if (!item.isEmpty()) 1091 return true; 1092 return false; 1093 } 1094 1095 public Identifier addIdentifier() { //3 1096 Identifier t = new Identifier(); 1097 if (this.identifier == null) 1098 this.identifier = new ArrayList<Identifier>(); 1099 this.identifier.add(t); 1100 return t; 1101 } 1102 1103 public ProcedureRequest addIdentifier(Identifier t) { //3 1104 if (t == null) 1105 return this; 1106 if (this.identifier == null) 1107 this.identifier = new ArrayList<Identifier>(); 1108 this.identifier.add(t); 1109 return this; 1110 } 1111 1112 /** 1113 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1114 */ 1115 public Identifier getIdentifierFirstRep() { 1116 if (getIdentifier().isEmpty()) { 1117 addIdentifier(); 1118 } 1119 return getIdentifier().get(0); 1120 } 1121 1122 /** 1123 * @return {@link #definition} (Protocol or definition followed by this request.) 1124 */ 1125 public List<Reference> getDefinition() { 1126 if (this.definition == null) 1127 this.definition = new ArrayList<Reference>(); 1128 return this.definition; 1129 } 1130 1131 /** 1132 * @return Returns a reference to <code>this</code> for easy method chaining 1133 */ 1134 public ProcedureRequest setDefinition(List<Reference> theDefinition) { 1135 this.definition = theDefinition; 1136 return this; 1137 } 1138 1139 public boolean hasDefinition() { 1140 if (this.definition == null) 1141 return false; 1142 for (Reference item : this.definition) 1143 if (!item.isEmpty()) 1144 return true; 1145 return false; 1146 } 1147 1148 public Reference addDefinition() { //3 1149 Reference t = new Reference(); 1150 if (this.definition == null) 1151 this.definition = new ArrayList<Reference>(); 1152 this.definition.add(t); 1153 return t; 1154 } 1155 1156 public ProcedureRequest addDefinition(Reference t) { //3 1157 if (t == null) 1158 return this; 1159 if (this.definition == null) 1160 this.definition = new ArrayList<Reference>(); 1161 this.definition.add(t); 1162 return this; 1163 } 1164 1165 /** 1166 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1167 */ 1168 public Reference getDefinitionFirstRep() { 1169 if (getDefinition().isEmpty()) { 1170 addDefinition(); 1171 } 1172 return getDefinition().get(0); 1173 } 1174 1175 /** 1176 * @deprecated Use Reference#setResource(IBaseResource) instead 1177 */ 1178 @Deprecated 1179 public List<Resource> getDefinitionTarget() { 1180 if (this.definitionTarget == null) 1181 this.definitionTarget = new ArrayList<Resource>(); 1182 return this.definitionTarget; 1183 } 1184 1185 /** 1186 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 1187 */ 1188 public List<Reference> getBasedOn() { 1189 if (this.basedOn == null) 1190 this.basedOn = new ArrayList<Reference>(); 1191 return this.basedOn; 1192 } 1193 1194 /** 1195 * @return Returns a reference to <code>this</code> for easy method chaining 1196 */ 1197 public ProcedureRequest setBasedOn(List<Reference> theBasedOn) { 1198 this.basedOn = theBasedOn; 1199 return this; 1200 } 1201 1202 public boolean hasBasedOn() { 1203 if (this.basedOn == null) 1204 return false; 1205 for (Reference item : this.basedOn) 1206 if (!item.isEmpty()) 1207 return true; 1208 return false; 1209 } 1210 1211 public Reference addBasedOn() { //3 1212 Reference t = new Reference(); 1213 if (this.basedOn == null) 1214 this.basedOn = new ArrayList<Reference>(); 1215 this.basedOn.add(t); 1216 return t; 1217 } 1218 1219 public ProcedureRequest addBasedOn(Reference t) { //3 1220 if (t == null) 1221 return this; 1222 if (this.basedOn == null) 1223 this.basedOn = new ArrayList<Reference>(); 1224 this.basedOn.add(t); 1225 return this; 1226 } 1227 1228 /** 1229 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1230 */ 1231 public Reference getBasedOnFirstRep() { 1232 if (getBasedOn().isEmpty()) { 1233 addBasedOn(); 1234 } 1235 return getBasedOn().get(0); 1236 } 1237 1238 /** 1239 * @deprecated Use Reference#setResource(IBaseResource) instead 1240 */ 1241 @Deprecated 1242 public List<Resource> getBasedOnTarget() { 1243 if (this.basedOnTarget == null) 1244 this.basedOnTarget = new ArrayList<Resource>(); 1245 return this.basedOnTarget; 1246 } 1247 1248 /** 1249 * @return {@link #replaces} (The request takes the place of the referenced completed or terminated request(s).) 1250 */ 1251 public List<Reference> getReplaces() { 1252 if (this.replaces == null) 1253 this.replaces = new ArrayList<Reference>(); 1254 return this.replaces; 1255 } 1256 1257 /** 1258 * @return Returns a reference to <code>this</code> for easy method chaining 1259 */ 1260 public ProcedureRequest setReplaces(List<Reference> theReplaces) { 1261 this.replaces = theReplaces; 1262 return this; 1263 } 1264 1265 public boolean hasReplaces() { 1266 if (this.replaces == null) 1267 return false; 1268 for (Reference item : this.replaces) 1269 if (!item.isEmpty()) 1270 return true; 1271 return false; 1272 } 1273 1274 public Reference addReplaces() { //3 1275 Reference t = new Reference(); 1276 if (this.replaces == null) 1277 this.replaces = new ArrayList<Reference>(); 1278 this.replaces.add(t); 1279 return t; 1280 } 1281 1282 public ProcedureRequest addReplaces(Reference t) { //3 1283 if (t == null) 1284 return this; 1285 if (this.replaces == null) 1286 this.replaces = new ArrayList<Reference>(); 1287 this.replaces.add(t); 1288 return this; 1289 } 1290 1291 /** 1292 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 1293 */ 1294 public Reference getReplacesFirstRep() { 1295 if (getReplaces().isEmpty()) { 1296 addReplaces(); 1297 } 1298 return getReplaces().get(0); 1299 } 1300 1301 /** 1302 * @deprecated Use Reference#setResource(IBaseResource) instead 1303 */ 1304 @Deprecated 1305 public List<Resource> getReplacesTarget() { 1306 if (this.replacesTarget == null) 1307 this.replacesTarget = new ArrayList<Resource>(); 1308 return this.replacesTarget; 1309 } 1310 1311 /** 1312 * @return {@link #requisition} (A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1313 */ 1314 public Identifier getRequisition() { 1315 if (this.requisition == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create ProcedureRequest.requisition"); 1318 else if (Configuration.doAutoCreate()) 1319 this.requisition = new Identifier(); // cc 1320 return this.requisition; 1321 } 1322 1323 public boolean hasRequisition() { 1324 return this.requisition != null && !this.requisition.isEmpty(); 1325 } 1326 1327 /** 1328 * @param value {@link #requisition} (A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1329 */ 1330 public ProcedureRequest setRequisition(Identifier value) { 1331 this.requisition = value; 1332 return this; 1333 } 1334 1335 /** 1336 * @return {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1337 */ 1338 public Enumeration<ProcedureRequestStatus> getStatusElement() { 1339 if (this.status == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create ProcedureRequest.status"); 1342 else if (Configuration.doAutoCreate()) 1343 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); // bb 1344 return this.status; 1345 } 1346 1347 public boolean hasStatusElement() { 1348 return this.status != null && !this.status.isEmpty(); 1349 } 1350 1351 public boolean hasStatus() { 1352 return this.status != null && !this.status.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1357 */ 1358 public ProcedureRequest setStatusElement(Enumeration<ProcedureRequestStatus> value) { 1359 this.status = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return The status of the order. 1365 */ 1366 public ProcedureRequestStatus getStatus() { 1367 return this.status == null ? null : this.status.getValue(); 1368 } 1369 1370 /** 1371 * @param value The status of the order. 1372 */ 1373 public ProcedureRequest setStatus(ProcedureRequestStatus value) { 1374 if (this.status == null) 1375 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); 1376 this.status.setValue(value); 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1382 */ 1383 public Enumeration<ProcedureRequestIntent> getIntentElement() { 1384 if (this.intent == null) 1385 if (Configuration.errorOnAutoCreate()) 1386 throw new Error("Attempt to auto-create ProcedureRequest.intent"); 1387 else if (Configuration.doAutoCreate()) 1388 this.intent = new Enumeration<ProcedureRequestIntent>(new ProcedureRequestIntentEnumFactory()); // bb 1389 return this.intent; 1390 } 1391 1392 public boolean hasIntentElement() { 1393 return this.intent != null && !this.intent.isEmpty(); 1394 } 1395 1396 public boolean hasIntent() { 1397 return this.intent != null && !this.intent.isEmpty(); 1398 } 1399 1400 /** 1401 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1402 */ 1403 public ProcedureRequest setIntentElement(Enumeration<ProcedureRequestIntent> value) { 1404 this.intent = value; 1405 return this; 1406 } 1407 1408 /** 1409 * @return Whether the request is a proposal, plan, an original order or a reflex order. 1410 */ 1411 public ProcedureRequestIntent getIntent() { 1412 return this.intent == null ? null : this.intent.getValue(); 1413 } 1414 1415 /** 1416 * @param value Whether the request is a proposal, plan, an original order or a reflex order. 1417 */ 1418 public ProcedureRequest setIntent(ProcedureRequestIntent value) { 1419 if (this.intent == null) 1420 this.intent = new Enumeration<ProcedureRequestIntent>(new ProcedureRequestIntentEnumFactory()); 1421 this.intent.setValue(value); 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #priority} (Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1427 */ 1428 public Enumeration<ProcedureRequestPriority> getPriorityElement() { 1429 if (this.priority == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create ProcedureRequest.priority"); 1432 else if (Configuration.doAutoCreate()) 1433 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); // bb 1434 return this.priority; 1435 } 1436 1437 public boolean hasPriorityElement() { 1438 return this.priority != null && !this.priority.isEmpty(); 1439 } 1440 1441 public boolean hasPriority() { 1442 return this.priority != null && !this.priority.isEmpty(); 1443 } 1444 1445 /** 1446 * @param value {@link #priority} (Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1447 */ 1448 public ProcedureRequest setPriorityElement(Enumeration<ProcedureRequestPriority> value) { 1449 this.priority = value; 1450 return this; 1451 } 1452 1453 /** 1454 * @return Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 1455 */ 1456 public ProcedureRequestPriority getPriority() { 1457 return this.priority == null ? null : this.priority.getValue(); 1458 } 1459 1460 /** 1461 * @param value Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 1462 */ 1463 public ProcedureRequest setPriority(ProcedureRequestPriority value) { 1464 if (value == null) 1465 this.priority = null; 1466 else { 1467 if (this.priority == null) 1468 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); 1469 this.priority.setValue(value); 1470 } 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #doNotPerform} (Set this to true if the record is saying that the procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1476 */ 1477 public BooleanType getDoNotPerformElement() { 1478 if (this.doNotPerform == null) 1479 if (Configuration.errorOnAutoCreate()) 1480 throw new Error("Attempt to auto-create ProcedureRequest.doNotPerform"); 1481 else if (Configuration.doAutoCreate()) 1482 this.doNotPerform = new BooleanType(); // bb 1483 return this.doNotPerform; 1484 } 1485 1486 public boolean hasDoNotPerformElement() { 1487 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1488 } 1489 1490 public boolean hasDoNotPerform() { 1491 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1492 } 1493 1494 /** 1495 * @param value {@link #doNotPerform} (Set this to true if the record is saying that the procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1496 */ 1497 public ProcedureRequest setDoNotPerformElement(BooleanType value) { 1498 this.doNotPerform = value; 1499 return this; 1500 } 1501 1502 /** 1503 * @return Set this to true if the record is saying that the procedure should NOT be performed. 1504 */ 1505 public boolean getDoNotPerform() { 1506 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1507 } 1508 1509 /** 1510 * @param value Set this to true if the record is saying that the procedure should NOT be performed. 1511 */ 1512 public ProcedureRequest setDoNotPerform(boolean value) { 1513 if (this.doNotPerform == null) 1514 this.doNotPerform = new BooleanType(); 1515 this.doNotPerform.setValue(value); 1516 return this; 1517 } 1518 1519 /** 1520 * @return {@link #category} (A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1521 */ 1522 public List<CodeableConcept> getCategory() { 1523 if (this.category == null) 1524 this.category = new ArrayList<CodeableConcept>(); 1525 return this.category; 1526 } 1527 1528 /** 1529 * @return Returns a reference to <code>this</code> for easy method chaining 1530 */ 1531 public ProcedureRequest setCategory(List<CodeableConcept> theCategory) { 1532 this.category = theCategory; 1533 return this; 1534 } 1535 1536 public boolean hasCategory() { 1537 if (this.category == null) 1538 return false; 1539 for (CodeableConcept item : this.category) 1540 if (!item.isEmpty()) 1541 return true; 1542 return false; 1543 } 1544 1545 public CodeableConcept addCategory() { //3 1546 CodeableConcept t = new CodeableConcept(); 1547 if (this.category == null) 1548 this.category = new ArrayList<CodeableConcept>(); 1549 this.category.add(t); 1550 return t; 1551 } 1552 1553 public ProcedureRequest addCategory(CodeableConcept t) { //3 1554 if (t == null) 1555 return this; 1556 if (this.category == null) 1557 this.category = new ArrayList<CodeableConcept>(); 1558 this.category.add(t); 1559 return this; 1560 } 1561 1562 /** 1563 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1564 */ 1565 public CodeableConcept getCategoryFirstRep() { 1566 if (getCategory().isEmpty()) { 1567 addCategory(); 1568 } 1569 return getCategory().get(0); 1570 } 1571 1572 /** 1573 * @return {@link #code} (A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.) 1574 */ 1575 public CodeableConcept getCode() { 1576 if (this.code == null) 1577 if (Configuration.errorOnAutoCreate()) 1578 throw new Error("Attempt to auto-create ProcedureRequest.code"); 1579 else if (Configuration.doAutoCreate()) 1580 this.code = new CodeableConcept(); // cc 1581 return this.code; 1582 } 1583 1584 public boolean hasCode() { 1585 return this.code != null && !this.code.isEmpty(); 1586 } 1587 1588 /** 1589 * @param value {@link #code} (A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.) 1590 */ 1591 public ProcedureRequest setCode(CodeableConcept value) { 1592 this.code = value; 1593 return this; 1594 } 1595 1596 /** 1597 * @return {@link #subject} (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1598 */ 1599 public Reference getSubject() { 1600 if (this.subject == null) 1601 if (Configuration.errorOnAutoCreate()) 1602 throw new Error("Attempt to auto-create ProcedureRequest.subject"); 1603 else if (Configuration.doAutoCreate()) 1604 this.subject = new Reference(); // cc 1605 return this.subject; 1606 } 1607 1608 public boolean hasSubject() { 1609 return this.subject != null && !this.subject.isEmpty(); 1610 } 1611 1612 /** 1613 * @param value {@link #subject} (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1614 */ 1615 public ProcedureRequest setSubject(Reference value) { 1616 this.subject = value; 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1622 */ 1623 public Resource getSubjectTarget() { 1624 return this.subjectTarget; 1625 } 1626 1627 /** 1628 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1629 */ 1630 public ProcedureRequest setSubjectTarget(Resource value) { 1631 this.subjectTarget = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #context} (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1637 */ 1638 public Reference getContext() { 1639 if (this.context == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create ProcedureRequest.context"); 1642 else if (Configuration.doAutoCreate()) 1643 this.context = new Reference(); // cc 1644 return this.context; 1645 } 1646 1647 public boolean hasContext() { 1648 return this.context != null && !this.context.isEmpty(); 1649 } 1650 1651 /** 1652 * @param value {@link #context} (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1653 */ 1654 public ProcedureRequest setContext(Reference value) { 1655 this.context = value; 1656 return this; 1657 } 1658 1659 /** 1660 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1661 */ 1662 public Resource getContextTarget() { 1663 return this.contextTarget; 1664 } 1665 1666 /** 1667 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1668 */ 1669 public ProcedureRequest setContextTarget(Resource value) { 1670 this.contextTarget = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1676 */ 1677 public Type getOccurrence() { 1678 return this.occurrence; 1679 } 1680 1681 /** 1682 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1683 */ 1684 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1685 if (this.occurrence == null) 1686 return null; 1687 if (!(this.occurrence instanceof DateTimeType)) 1688 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1689 return (DateTimeType) this.occurrence; 1690 } 1691 1692 public boolean hasOccurrenceDateTimeType() { 1693 return this.occurrence instanceof DateTimeType; 1694 } 1695 1696 /** 1697 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1698 */ 1699 public Period getOccurrencePeriod() throws FHIRException { 1700 if (this.occurrence == null) 1701 return null; 1702 if (!(this.occurrence instanceof Period)) 1703 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1704 return (Period) this.occurrence; 1705 } 1706 1707 public boolean hasOccurrencePeriod() { 1708 return this.occurrence instanceof Period; 1709 } 1710 1711 /** 1712 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1713 */ 1714 public Timing getOccurrenceTiming() throws FHIRException { 1715 if (this.occurrence == null) 1716 return null; 1717 if (!(this.occurrence instanceof Timing)) 1718 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1719 return (Timing) this.occurrence; 1720 } 1721 1722 public boolean hasOccurrenceTiming() { 1723 return this.occurrence instanceof Timing; 1724 } 1725 1726 public boolean hasOccurrence() { 1727 return this.occurrence != null && !this.occurrence.isEmpty(); 1728 } 1729 1730 /** 1731 * @param value {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1732 */ 1733 public ProcedureRequest setOccurrence(Type value) throws FHIRFormatError { 1734 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1735 throw new FHIRFormatError("Not the right type for ProcedureRequest.occurrence[x]: "+value.fhirType()); 1736 this.occurrence = value; 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1742 */ 1743 public Type getAsNeeded() { 1744 return this.asNeeded; 1745 } 1746 1747 /** 1748 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1749 */ 1750 public BooleanType getAsNeededBooleanType() throws FHIRException { 1751 if (this.asNeeded == null) 1752 return null; 1753 if (!(this.asNeeded instanceof BooleanType)) 1754 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 1755 return (BooleanType) this.asNeeded; 1756 } 1757 1758 public boolean hasAsNeededBooleanType() { 1759 return this.asNeeded instanceof BooleanType; 1760 } 1761 1762 /** 1763 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1764 */ 1765 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 1766 if (this.asNeeded == null) 1767 return null; 1768 if (!(this.asNeeded instanceof CodeableConcept)) 1769 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 1770 return (CodeableConcept) this.asNeeded; 1771 } 1772 1773 public boolean hasAsNeededCodeableConcept() { 1774 return this.asNeeded instanceof CodeableConcept; 1775 } 1776 1777 public boolean hasAsNeeded() { 1778 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1779 } 1780 1781 /** 1782 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1783 */ 1784 public ProcedureRequest setAsNeeded(Type value) throws FHIRFormatError { 1785 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 1786 throw new FHIRFormatError("Not the right type for ProcedureRequest.asNeeded[x]: "+value.fhirType()); 1787 this.asNeeded = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1793 */ 1794 public DateTimeType getAuthoredOnElement() { 1795 if (this.authoredOn == null) 1796 if (Configuration.errorOnAutoCreate()) 1797 throw new Error("Attempt to auto-create ProcedureRequest.authoredOn"); 1798 else if (Configuration.doAutoCreate()) 1799 this.authoredOn = new DateTimeType(); // bb 1800 return this.authoredOn; 1801 } 1802 1803 public boolean hasAuthoredOnElement() { 1804 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1805 } 1806 1807 public boolean hasAuthoredOn() { 1808 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1809 } 1810 1811 /** 1812 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1813 */ 1814 public ProcedureRequest setAuthoredOnElement(DateTimeType value) { 1815 this.authoredOn = value; 1816 return this; 1817 } 1818 1819 /** 1820 * @return When the request transitioned to being actionable. 1821 */ 1822 public Date getAuthoredOn() { 1823 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1824 } 1825 1826 /** 1827 * @param value When the request transitioned to being actionable. 1828 */ 1829 public ProcedureRequest setAuthoredOn(Date value) { 1830 if (value == null) 1831 this.authoredOn = null; 1832 else { 1833 if (this.authoredOn == null) 1834 this.authoredOn = new DateTimeType(); 1835 this.authoredOn.setValue(value); 1836 } 1837 return this; 1838 } 1839 1840 /** 1841 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1842 */ 1843 public ProcedureRequestRequesterComponent getRequester() { 1844 if (this.requester == null) 1845 if (Configuration.errorOnAutoCreate()) 1846 throw new Error("Attempt to auto-create ProcedureRequest.requester"); 1847 else if (Configuration.doAutoCreate()) 1848 this.requester = new ProcedureRequestRequesterComponent(); // cc 1849 return this.requester; 1850 } 1851 1852 public boolean hasRequester() { 1853 return this.requester != null && !this.requester.isEmpty(); 1854 } 1855 1856 /** 1857 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1858 */ 1859 public ProcedureRequest setRequester(ProcedureRequestRequesterComponent value) { 1860 this.requester = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1866 */ 1867 public CodeableConcept getPerformerType() { 1868 if (this.performerType == null) 1869 if (Configuration.errorOnAutoCreate()) 1870 throw new Error("Attempt to auto-create ProcedureRequest.performerType"); 1871 else if (Configuration.doAutoCreate()) 1872 this.performerType = new CodeableConcept(); // cc 1873 return this.performerType; 1874 } 1875 1876 public boolean hasPerformerType() { 1877 return this.performerType != null && !this.performerType.isEmpty(); 1878 } 1879 1880 /** 1881 * @param value {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1882 */ 1883 public ProcedureRequest setPerformerType(CodeableConcept value) { 1884 this.performerType = value; 1885 return this; 1886 } 1887 1888 /** 1889 * @return {@link #performer} (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1890 */ 1891 public Reference getPerformer() { 1892 if (this.performer == null) 1893 if (Configuration.errorOnAutoCreate()) 1894 throw new Error("Attempt to auto-create ProcedureRequest.performer"); 1895 else if (Configuration.doAutoCreate()) 1896 this.performer = new Reference(); // cc 1897 return this.performer; 1898 } 1899 1900 public boolean hasPerformer() { 1901 return this.performer != null && !this.performer.isEmpty(); 1902 } 1903 1904 /** 1905 * @param value {@link #performer} (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1906 */ 1907 public ProcedureRequest setPerformer(Reference value) { 1908 this.performer = value; 1909 return this; 1910 } 1911 1912 /** 1913 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1914 */ 1915 public Resource getPerformerTarget() { 1916 return this.performerTarget; 1917 } 1918 1919 /** 1920 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1921 */ 1922 public ProcedureRequest setPerformerTarget(Resource value) { 1923 this.performerTarget = value; 1924 return this; 1925 } 1926 1927 /** 1928 * @return {@link #reasonCode} (An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.) 1929 */ 1930 public List<CodeableConcept> getReasonCode() { 1931 if (this.reasonCode == null) 1932 this.reasonCode = new ArrayList<CodeableConcept>(); 1933 return this.reasonCode; 1934 } 1935 1936 /** 1937 * @return Returns a reference to <code>this</code> for easy method chaining 1938 */ 1939 public ProcedureRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1940 this.reasonCode = theReasonCode; 1941 return this; 1942 } 1943 1944 public boolean hasReasonCode() { 1945 if (this.reasonCode == null) 1946 return false; 1947 for (CodeableConcept item : this.reasonCode) 1948 if (!item.isEmpty()) 1949 return true; 1950 return false; 1951 } 1952 1953 public CodeableConcept addReasonCode() { //3 1954 CodeableConcept t = new CodeableConcept(); 1955 if (this.reasonCode == null) 1956 this.reasonCode = new ArrayList<CodeableConcept>(); 1957 this.reasonCode.add(t); 1958 return t; 1959 } 1960 1961 public ProcedureRequest addReasonCode(CodeableConcept t) { //3 1962 if (t == null) 1963 return this; 1964 if (this.reasonCode == null) 1965 this.reasonCode = new ArrayList<CodeableConcept>(); 1966 this.reasonCode.add(t); 1967 return this; 1968 } 1969 1970 /** 1971 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1972 */ 1973 public CodeableConcept getReasonCodeFirstRep() { 1974 if (getReasonCode().isEmpty()) { 1975 addReasonCode(); 1976 } 1977 return getReasonCode().get(0); 1978 } 1979 1980 /** 1981 * @return {@link #reasonReference} (Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.) 1982 */ 1983 public List<Reference> getReasonReference() { 1984 if (this.reasonReference == null) 1985 this.reasonReference = new ArrayList<Reference>(); 1986 return this.reasonReference; 1987 } 1988 1989 /** 1990 * @return Returns a reference to <code>this</code> for easy method chaining 1991 */ 1992 public ProcedureRequest setReasonReference(List<Reference> theReasonReference) { 1993 this.reasonReference = theReasonReference; 1994 return this; 1995 } 1996 1997 public boolean hasReasonReference() { 1998 if (this.reasonReference == null) 1999 return false; 2000 for (Reference item : this.reasonReference) 2001 if (!item.isEmpty()) 2002 return true; 2003 return false; 2004 } 2005 2006 public Reference addReasonReference() { //3 2007 Reference t = new Reference(); 2008 if (this.reasonReference == null) 2009 this.reasonReference = new ArrayList<Reference>(); 2010 this.reasonReference.add(t); 2011 return t; 2012 } 2013 2014 public ProcedureRequest addReasonReference(Reference t) { //3 2015 if (t == null) 2016 return this; 2017 if (this.reasonReference == null) 2018 this.reasonReference = new ArrayList<Reference>(); 2019 this.reasonReference.add(t); 2020 return this; 2021 } 2022 2023 /** 2024 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 2025 */ 2026 public Reference getReasonReferenceFirstRep() { 2027 if (getReasonReference().isEmpty()) { 2028 addReasonReference(); 2029 } 2030 return getReasonReference().get(0); 2031 } 2032 2033 /** 2034 * @deprecated Use Reference#setResource(IBaseResource) instead 2035 */ 2036 @Deprecated 2037 public List<Resource> getReasonReferenceTarget() { 2038 if (this.reasonReferenceTarget == null) 2039 this.reasonReferenceTarget = new ArrayList<Resource>(); 2040 return this.reasonReferenceTarget; 2041 } 2042 2043 /** 2044 * @return {@link #supportingInfo} (Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.) 2045 */ 2046 public List<Reference> getSupportingInfo() { 2047 if (this.supportingInfo == null) 2048 this.supportingInfo = new ArrayList<Reference>(); 2049 return this.supportingInfo; 2050 } 2051 2052 /** 2053 * @return Returns a reference to <code>this</code> for easy method chaining 2054 */ 2055 public ProcedureRequest setSupportingInfo(List<Reference> theSupportingInfo) { 2056 this.supportingInfo = theSupportingInfo; 2057 return this; 2058 } 2059 2060 public boolean hasSupportingInfo() { 2061 if (this.supportingInfo == null) 2062 return false; 2063 for (Reference item : this.supportingInfo) 2064 if (!item.isEmpty()) 2065 return true; 2066 return false; 2067 } 2068 2069 public Reference addSupportingInfo() { //3 2070 Reference t = new Reference(); 2071 if (this.supportingInfo == null) 2072 this.supportingInfo = new ArrayList<Reference>(); 2073 this.supportingInfo.add(t); 2074 return t; 2075 } 2076 2077 public ProcedureRequest addSupportingInfo(Reference t) { //3 2078 if (t == null) 2079 return this; 2080 if (this.supportingInfo == null) 2081 this.supportingInfo = new ArrayList<Reference>(); 2082 this.supportingInfo.add(t); 2083 return this; 2084 } 2085 2086 /** 2087 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 2088 */ 2089 public Reference getSupportingInfoFirstRep() { 2090 if (getSupportingInfo().isEmpty()) { 2091 addSupportingInfo(); 2092 } 2093 return getSupportingInfo().get(0); 2094 } 2095 2096 /** 2097 * @deprecated Use Reference#setResource(IBaseResource) instead 2098 */ 2099 @Deprecated 2100 public List<Resource> getSupportingInfoTarget() { 2101 if (this.supportingInfoTarget == null) 2102 this.supportingInfoTarget = new ArrayList<Resource>(); 2103 return this.supportingInfoTarget; 2104 } 2105 2106 /** 2107 * @return {@link #specimen} (One or more specimens that the laboratory procedure will use.) 2108 */ 2109 public List<Reference> getSpecimen() { 2110 if (this.specimen == null) 2111 this.specimen = new ArrayList<Reference>(); 2112 return this.specimen; 2113 } 2114 2115 /** 2116 * @return Returns a reference to <code>this</code> for easy method chaining 2117 */ 2118 public ProcedureRequest setSpecimen(List<Reference> theSpecimen) { 2119 this.specimen = theSpecimen; 2120 return this; 2121 } 2122 2123 public boolean hasSpecimen() { 2124 if (this.specimen == null) 2125 return false; 2126 for (Reference item : this.specimen) 2127 if (!item.isEmpty()) 2128 return true; 2129 return false; 2130 } 2131 2132 public Reference addSpecimen() { //3 2133 Reference t = new Reference(); 2134 if (this.specimen == null) 2135 this.specimen = new ArrayList<Reference>(); 2136 this.specimen.add(t); 2137 return t; 2138 } 2139 2140 public ProcedureRequest addSpecimen(Reference t) { //3 2141 if (t == null) 2142 return this; 2143 if (this.specimen == null) 2144 this.specimen = new ArrayList<Reference>(); 2145 this.specimen.add(t); 2146 return this; 2147 } 2148 2149 /** 2150 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist 2151 */ 2152 public Reference getSpecimenFirstRep() { 2153 if (getSpecimen().isEmpty()) { 2154 addSpecimen(); 2155 } 2156 return getSpecimen().get(0); 2157 } 2158 2159 /** 2160 * @deprecated Use Reference#setResource(IBaseResource) instead 2161 */ 2162 @Deprecated 2163 public List<Specimen> getSpecimenTarget() { 2164 if (this.specimenTarget == null) 2165 this.specimenTarget = new ArrayList<Specimen>(); 2166 return this.specimenTarget; 2167 } 2168 2169 /** 2170 * @deprecated Use Reference#setResource(IBaseResource) instead 2171 */ 2172 @Deprecated 2173 public Specimen addSpecimenTarget() { 2174 Specimen r = new Specimen(); 2175 if (this.specimenTarget == null) 2176 this.specimenTarget = new ArrayList<Specimen>(); 2177 this.specimenTarget.add(r); 2178 return r; 2179 } 2180 2181 /** 2182 * @return {@link #bodySite} (Anatomic location where the procedure should be performed. This is the target site.) 2183 */ 2184 public List<CodeableConcept> getBodySite() { 2185 if (this.bodySite == null) 2186 this.bodySite = new ArrayList<CodeableConcept>(); 2187 return this.bodySite; 2188 } 2189 2190 /** 2191 * @return Returns a reference to <code>this</code> for easy method chaining 2192 */ 2193 public ProcedureRequest setBodySite(List<CodeableConcept> theBodySite) { 2194 this.bodySite = theBodySite; 2195 return this; 2196 } 2197 2198 public boolean hasBodySite() { 2199 if (this.bodySite == null) 2200 return false; 2201 for (CodeableConcept item : this.bodySite) 2202 if (!item.isEmpty()) 2203 return true; 2204 return false; 2205 } 2206 2207 public CodeableConcept addBodySite() { //3 2208 CodeableConcept t = new CodeableConcept(); 2209 if (this.bodySite == null) 2210 this.bodySite = new ArrayList<CodeableConcept>(); 2211 this.bodySite.add(t); 2212 return t; 2213 } 2214 2215 public ProcedureRequest addBodySite(CodeableConcept t) { //3 2216 if (t == null) 2217 return this; 2218 if (this.bodySite == null) 2219 this.bodySite = new ArrayList<CodeableConcept>(); 2220 this.bodySite.add(t); 2221 return this; 2222 } 2223 2224 /** 2225 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist 2226 */ 2227 public CodeableConcept getBodySiteFirstRep() { 2228 if (getBodySite().isEmpty()) { 2229 addBodySite(); 2230 } 2231 return getBodySite().get(0); 2232 } 2233 2234 /** 2235 * @return {@link #note} (Any other notes and comments made about the service request. For example, letting provider know that "patient hates needles" or other provider instructions.) 2236 */ 2237 public List<Annotation> getNote() { 2238 if (this.note == null) 2239 this.note = new ArrayList<Annotation>(); 2240 return this.note; 2241 } 2242 2243 /** 2244 * @return Returns a reference to <code>this</code> for easy method chaining 2245 */ 2246 public ProcedureRequest setNote(List<Annotation> theNote) { 2247 this.note = theNote; 2248 return this; 2249 } 2250 2251 public boolean hasNote() { 2252 if (this.note == null) 2253 return false; 2254 for (Annotation item : this.note) 2255 if (!item.isEmpty()) 2256 return true; 2257 return false; 2258 } 2259 2260 public Annotation addNote() { //3 2261 Annotation t = new Annotation(); 2262 if (this.note == null) 2263 this.note = new ArrayList<Annotation>(); 2264 this.note.add(t); 2265 return t; 2266 } 2267 2268 public ProcedureRequest addNote(Annotation t) { //3 2269 if (t == null) 2270 return this; 2271 if (this.note == null) 2272 this.note = new ArrayList<Annotation>(); 2273 this.note.add(t); 2274 return this; 2275 } 2276 2277 /** 2278 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2279 */ 2280 public Annotation getNoteFirstRep() { 2281 if (getNote().isEmpty()) { 2282 addNote(); 2283 } 2284 return getNote().get(0); 2285 } 2286 2287 /** 2288 * @return {@link #relevantHistory} (Key events in the history of the request.) 2289 */ 2290 public List<Reference> getRelevantHistory() { 2291 if (this.relevantHistory == null) 2292 this.relevantHistory = new ArrayList<Reference>(); 2293 return this.relevantHistory; 2294 } 2295 2296 /** 2297 * @return Returns a reference to <code>this</code> for easy method chaining 2298 */ 2299 public ProcedureRequest setRelevantHistory(List<Reference> theRelevantHistory) { 2300 this.relevantHistory = theRelevantHistory; 2301 return this; 2302 } 2303 2304 public boolean hasRelevantHistory() { 2305 if (this.relevantHistory == null) 2306 return false; 2307 for (Reference item : this.relevantHistory) 2308 if (!item.isEmpty()) 2309 return true; 2310 return false; 2311 } 2312 2313 public Reference addRelevantHistory() { //3 2314 Reference t = new Reference(); 2315 if (this.relevantHistory == null) 2316 this.relevantHistory = new ArrayList<Reference>(); 2317 this.relevantHistory.add(t); 2318 return t; 2319 } 2320 2321 public ProcedureRequest addRelevantHistory(Reference t) { //3 2322 if (t == null) 2323 return this; 2324 if (this.relevantHistory == null) 2325 this.relevantHistory = new ArrayList<Reference>(); 2326 this.relevantHistory.add(t); 2327 return this; 2328 } 2329 2330 /** 2331 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 2332 */ 2333 public Reference getRelevantHistoryFirstRep() { 2334 if (getRelevantHistory().isEmpty()) { 2335 addRelevantHistory(); 2336 } 2337 return getRelevantHistory().get(0); 2338 } 2339 2340 /** 2341 * @deprecated Use Reference#setResource(IBaseResource) instead 2342 */ 2343 @Deprecated 2344 public List<Provenance> getRelevantHistoryTarget() { 2345 if (this.relevantHistoryTarget == null) 2346 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2347 return this.relevantHistoryTarget; 2348 } 2349 2350 /** 2351 * @deprecated Use Reference#setResource(IBaseResource) instead 2352 */ 2353 @Deprecated 2354 public Provenance addRelevantHistoryTarget() { 2355 Provenance r = new Provenance(); 2356 if (this.relevantHistoryTarget == null) 2357 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2358 this.relevantHistoryTarget.add(r); 2359 return r; 2360 } 2361 2362 protected void listChildren(List<Property> children) { 2363 super.listChildren(children); 2364 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2365 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 2366 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2367 children.add(new Property("replaces", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces)); 2368 children.add(new Property("requisition", "Identifier", "A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition)); 2369 children.add(new Property("status", "code", "The status of the order.", 0, 1, status)); 2370 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 2371 children.add(new Property("priority", "code", "Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.", 0, 1, priority)); 2372 children.add(new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the procedure should NOT be performed.", 0, 1, doNotPerform)); 2373 children.add(new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category)); 2374 children.add(new Property("code", "CodeableConcept", "A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.", 0, 1, code)); 2375 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject)); 2376 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter or episode of care that provides additional information about the healthcare context in which this request is made.", 0, 1, context)); 2377 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence)); 2378 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded)); 2379 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 2380 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2381 children.add(new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType)); 2382 children.add(new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, 1, performer)); 2383 children.add(new Property("reasonCode", "CodeableConcept", "An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2384 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2385 children.add(new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2386 children.add(new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen)); 2387 children.add(new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2388 children.add(new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions.", 0, java.lang.Integer.MAX_VALUE, note)); 2389 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 2390 } 2391 2392 @Override 2393 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2394 switch (_hash) { 2395 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier); 2396 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 2397 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2398 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces); 2399 case 395923612: /*requisition*/ return new Property("requisition", "Identifier", "A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition); 2400 case -892481550: /*status*/ return new Property("status", "code", "The status of the order.", 0, 1, status); 2401 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 2402 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.", 0, 1, priority); 2403 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the procedure should NOT be performed.", 0, 1, doNotPerform); 2404 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category); 2405 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.", 0, 1, code); 2406 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject); 2407 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter or episode of care that provides additional information about the healthcare context in which this request is made.", 0, 1, context); 2408 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2409 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2410 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2411 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2412 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2413 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2414 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2415 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2416 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2417 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 2418 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2419 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType); 2420 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, 1, performer); 2421 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2422 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2423 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2424 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen); 2425 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2426 case 3387378: /*note*/ return new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions.", 0, java.lang.Integer.MAX_VALUE, note); 2427 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 2428 default: return super.getNamedProperty(_hash, _name, _checkValid); 2429 } 2430 2431 } 2432 2433 @Override 2434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2435 switch (hash) { 2436 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2437 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2438 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2439 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2440 case 395923612: /*requisition*/ return this.requisition == null ? new Base[0] : new Base[] {this.requisition}; // Identifier 2441 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ProcedureRequestStatus> 2442 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<ProcedureRequestIntent> 2443 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<ProcedureRequestPriority> 2444 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 2445 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2446 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2447 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2448 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2449 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 2450 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // Type 2451 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2452 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // ProcedureRequestRequesterComponent 2453 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 2454 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 2455 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2456 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2457 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2458 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 2459 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2460 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2461 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 2462 default: return super.getProperty(hash, name, checkValid); 2463 } 2464 2465 } 2466 2467 @Override 2468 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2469 switch (hash) { 2470 case -1618432855: // identifier 2471 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2472 return value; 2473 case -1014418093: // definition 2474 this.getDefinition().add(castToReference(value)); // Reference 2475 return value; 2476 case -332612366: // basedOn 2477 this.getBasedOn().add(castToReference(value)); // Reference 2478 return value; 2479 case -430332865: // replaces 2480 this.getReplaces().add(castToReference(value)); // Reference 2481 return value; 2482 case 395923612: // requisition 2483 this.requisition = castToIdentifier(value); // Identifier 2484 return value; 2485 case -892481550: // status 2486 value = new ProcedureRequestStatusEnumFactory().fromType(castToCode(value)); 2487 this.status = (Enumeration) value; // Enumeration<ProcedureRequestStatus> 2488 return value; 2489 case -1183762788: // intent 2490 value = new ProcedureRequestIntentEnumFactory().fromType(castToCode(value)); 2491 this.intent = (Enumeration) value; // Enumeration<ProcedureRequestIntent> 2492 return value; 2493 case -1165461084: // priority 2494 value = new ProcedureRequestPriorityEnumFactory().fromType(castToCode(value)); 2495 this.priority = (Enumeration) value; // Enumeration<ProcedureRequestPriority> 2496 return value; 2497 case -1788508167: // doNotPerform 2498 this.doNotPerform = castToBoolean(value); // BooleanType 2499 return value; 2500 case 50511102: // category 2501 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2502 return value; 2503 case 3059181: // code 2504 this.code = castToCodeableConcept(value); // CodeableConcept 2505 return value; 2506 case -1867885268: // subject 2507 this.subject = castToReference(value); // Reference 2508 return value; 2509 case 951530927: // context 2510 this.context = castToReference(value); // Reference 2511 return value; 2512 case 1687874001: // occurrence 2513 this.occurrence = castToType(value); // Type 2514 return value; 2515 case -1432923513: // asNeeded 2516 this.asNeeded = castToType(value); // Type 2517 return value; 2518 case -1500852503: // authoredOn 2519 this.authoredOn = castToDateTime(value); // DateTimeType 2520 return value; 2521 case 693933948: // requester 2522 this.requester = (ProcedureRequestRequesterComponent) value; // ProcedureRequestRequesterComponent 2523 return value; 2524 case -901444568: // performerType 2525 this.performerType = castToCodeableConcept(value); // CodeableConcept 2526 return value; 2527 case 481140686: // performer 2528 this.performer = castToReference(value); // Reference 2529 return value; 2530 case 722137681: // reasonCode 2531 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2532 return value; 2533 case -1146218137: // reasonReference 2534 this.getReasonReference().add(castToReference(value)); // Reference 2535 return value; 2536 case 1922406657: // supportingInfo 2537 this.getSupportingInfo().add(castToReference(value)); // Reference 2538 return value; 2539 case -2132868344: // specimen 2540 this.getSpecimen().add(castToReference(value)); // Reference 2541 return value; 2542 case 1702620169: // bodySite 2543 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 2544 return value; 2545 case 3387378: // note 2546 this.getNote().add(castToAnnotation(value)); // Annotation 2547 return value; 2548 case 1538891575: // relevantHistory 2549 this.getRelevantHistory().add(castToReference(value)); // Reference 2550 return value; 2551 default: return super.setProperty(hash, name, value); 2552 } 2553 2554 } 2555 2556 @Override 2557 public Base setProperty(String name, Base value) throws FHIRException { 2558 if (name.equals("identifier")) { 2559 this.getIdentifier().add(castToIdentifier(value)); 2560 } else if (name.equals("definition")) { 2561 this.getDefinition().add(castToReference(value)); 2562 } else if (name.equals("basedOn")) { 2563 this.getBasedOn().add(castToReference(value)); 2564 } else if (name.equals("replaces")) { 2565 this.getReplaces().add(castToReference(value)); 2566 } else if (name.equals("requisition")) { 2567 this.requisition = castToIdentifier(value); // Identifier 2568 } else if (name.equals("status")) { 2569 value = new ProcedureRequestStatusEnumFactory().fromType(castToCode(value)); 2570 this.status = (Enumeration) value; // Enumeration<ProcedureRequestStatus> 2571 } else if (name.equals("intent")) { 2572 value = new ProcedureRequestIntentEnumFactory().fromType(castToCode(value)); 2573 this.intent = (Enumeration) value; // Enumeration<ProcedureRequestIntent> 2574 } else if (name.equals("priority")) { 2575 value = new ProcedureRequestPriorityEnumFactory().fromType(castToCode(value)); 2576 this.priority = (Enumeration) value; // Enumeration<ProcedureRequestPriority> 2577 } else if (name.equals("doNotPerform")) { 2578 this.doNotPerform = castToBoolean(value); // BooleanType 2579 } else if (name.equals("category")) { 2580 this.getCategory().add(castToCodeableConcept(value)); 2581 } else if (name.equals("code")) { 2582 this.code = castToCodeableConcept(value); // CodeableConcept 2583 } else if (name.equals("subject")) { 2584 this.subject = castToReference(value); // Reference 2585 } else if (name.equals("context")) { 2586 this.context = castToReference(value); // Reference 2587 } else if (name.equals("occurrence[x]")) { 2588 this.occurrence = castToType(value); // Type 2589 } else if (name.equals("asNeeded[x]")) { 2590 this.asNeeded = castToType(value); // Type 2591 } else if (name.equals("authoredOn")) { 2592 this.authoredOn = castToDateTime(value); // DateTimeType 2593 } else if (name.equals("requester")) { 2594 this.requester = (ProcedureRequestRequesterComponent) value; // ProcedureRequestRequesterComponent 2595 } else if (name.equals("performerType")) { 2596 this.performerType = castToCodeableConcept(value); // CodeableConcept 2597 } else if (name.equals("performer")) { 2598 this.performer = castToReference(value); // Reference 2599 } else if (name.equals("reasonCode")) { 2600 this.getReasonCode().add(castToCodeableConcept(value)); 2601 } else if (name.equals("reasonReference")) { 2602 this.getReasonReference().add(castToReference(value)); 2603 } else if (name.equals("supportingInfo")) { 2604 this.getSupportingInfo().add(castToReference(value)); 2605 } else if (name.equals("specimen")) { 2606 this.getSpecimen().add(castToReference(value)); 2607 } else if (name.equals("bodySite")) { 2608 this.getBodySite().add(castToCodeableConcept(value)); 2609 } else if (name.equals("note")) { 2610 this.getNote().add(castToAnnotation(value)); 2611 } else if (name.equals("relevantHistory")) { 2612 this.getRelevantHistory().add(castToReference(value)); 2613 } else 2614 return super.setProperty(name, value); 2615 return value; 2616 } 2617 2618 @Override 2619 public Base makeProperty(int hash, String name) throws FHIRException { 2620 switch (hash) { 2621 case -1618432855: return addIdentifier(); 2622 case -1014418093: return addDefinition(); 2623 case -332612366: return addBasedOn(); 2624 case -430332865: return addReplaces(); 2625 case 395923612: return getRequisition(); 2626 case -892481550: return getStatusElement(); 2627 case -1183762788: return getIntentElement(); 2628 case -1165461084: return getPriorityElement(); 2629 case -1788508167: return getDoNotPerformElement(); 2630 case 50511102: return addCategory(); 2631 case 3059181: return getCode(); 2632 case -1867885268: return getSubject(); 2633 case 951530927: return getContext(); 2634 case -2022646513: return getOccurrence(); 2635 case 1687874001: return getOccurrence(); 2636 case -544329575: return getAsNeeded(); 2637 case -1432923513: return getAsNeeded(); 2638 case -1500852503: return getAuthoredOnElement(); 2639 case 693933948: return getRequester(); 2640 case -901444568: return getPerformerType(); 2641 case 481140686: return getPerformer(); 2642 case 722137681: return addReasonCode(); 2643 case -1146218137: return addReasonReference(); 2644 case 1922406657: return addSupportingInfo(); 2645 case -2132868344: return addSpecimen(); 2646 case 1702620169: return addBodySite(); 2647 case 3387378: return addNote(); 2648 case 1538891575: return addRelevantHistory(); 2649 default: return super.makeProperty(hash, name); 2650 } 2651 2652 } 2653 2654 @Override 2655 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2656 switch (hash) { 2657 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2658 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2659 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2660 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2661 case 395923612: /*requisition*/ return new String[] {"Identifier"}; 2662 case -892481550: /*status*/ return new String[] {"code"}; 2663 case -1183762788: /*intent*/ return new String[] {"code"}; 2664 case -1165461084: /*priority*/ return new String[] {"code"}; 2665 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 2666 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2667 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2668 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2669 case 951530927: /*context*/ return new String[] {"Reference"}; 2670 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2671 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 2672 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2673 case 693933948: /*requester*/ return new String[] {}; 2674 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 2675 case 481140686: /*performer*/ return new String[] {"Reference"}; 2676 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2677 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2678 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2679 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2680 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 2681 case 3387378: /*note*/ return new String[] {"Annotation"}; 2682 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2683 default: return super.getTypesForProperty(hash, name); 2684 } 2685 2686 } 2687 2688 @Override 2689 public Base addChild(String name) throws FHIRException { 2690 if (name.equals("identifier")) { 2691 return addIdentifier(); 2692 } 2693 else if (name.equals("definition")) { 2694 return addDefinition(); 2695 } 2696 else if (name.equals("basedOn")) { 2697 return addBasedOn(); 2698 } 2699 else if (name.equals("replaces")) { 2700 return addReplaces(); 2701 } 2702 else if (name.equals("requisition")) { 2703 this.requisition = new Identifier(); 2704 return this.requisition; 2705 } 2706 else if (name.equals("status")) { 2707 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.status"); 2708 } 2709 else if (name.equals("intent")) { 2710 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.intent"); 2711 } 2712 else if (name.equals("priority")) { 2713 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.priority"); 2714 } 2715 else if (name.equals("doNotPerform")) { 2716 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.doNotPerform"); 2717 } 2718 else if (name.equals("category")) { 2719 return addCategory(); 2720 } 2721 else if (name.equals("code")) { 2722 this.code = new CodeableConcept(); 2723 return this.code; 2724 } 2725 else if (name.equals("subject")) { 2726 this.subject = new Reference(); 2727 return this.subject; 2728 } 2729 else if (name.equals("context")) { 2730 this.context = new Reference(); 2731 return this.context; 2732 } 2733 else if (name.equals("occurrenceDateTime")) { 2734 this.occurrence = new DateTimeType(); 2735 return this.occurrence; 2736 } 2737 else if (name.equals("occurrencePeriod")) { 2738 this.occurrence = new Period(); 2739 return this.occurrence; 2740 } 2741 else if (name.equals("occurrenceTiming")) { 2742 this.occurrence = new Timing(); 2743 return this.occurrence; 2744 } 2745 else if (name.equals("asNeededBoolean")) { 2746 this.asNeeded = new BooleanType(); 2747 return this.asNeeded; 2748 } 2749 else if (name.equals("asNeededCodeableConcept")) { 2750 this.asNeeded = new CodeableConcept(); 2751 return this.asNeeded; 2752 } 2753 else if (name.equals("authoredOn")) { 2754 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.authoredOn"); 2755 } 2756 else if (name.equals("requester")) { 2757 this.requester = new ProcedureRequestRequesterComponent(); 2758 return this.requester; 2759 } 2760 else if (name.equals("performerType")) { 2761 this.performerType = new CodeableConcept(); 2762 return this.performerType; 2763 } 2764 else if (name.equals("performer")) { 2765 this.performer = new Reference(); 2766 return this.performer; 2767 } 2768 else if (name.equals("reasonCode")) { 2769 return addReasonCode(); 2770 } 2771 else if (name.equals("reasonReference")) { 2772 return addReasonReference(); 2773 } 2774 else if (name.equals("supportingInfo")) { 2775 return addSupportingInfo(); 2776 } 2777 else if (name.equals("specimen")) { 2778 return addSpecimen(); 2779 } 2780 else if (name.equals("bodySite")) { 2781 return addBodySite(); 2782 } 2783 else if (name.equals("note")) { 2784 return addNote(); 2785 } 2786 else if (name.equals("relevantHistory")) { 2787 return addRelevantHistory(); 2788 } 2789 else 2790 return super.addChild(name); 2791 } 2792 2793 public String fhirType() { 2794 return "ProcedureRequest"; 2795 2796 } 2797 2798 public ProcedureRequest copy() { 2799 ProcedureRequest dst = new ProcedureRequest(); 2800 copyValues(dst); 2801 if (identifier != null) { 2802 dst.identifier = new ArrayList<Identifier>(); 2803 for (Identifier i : identifier) 2804 dst.identifier.add(i.copy()); 2805 }; 2806 if (definition != null) { 2807 dst.definition = new ArrayList<Reference>(); 2808 for (Reference i : definition) 2809 dst.definition.add(i.copy()); 2810 }; 2811 if (basedOn != null) { 2812 dst.basedOn = new ArrayList<Reference>(); 2813 for (Reference i : basedOn) 2814 dst.basedOn.add(i.copy()); 2815 }; 2816 if (replaces != null) { 2817 dst.replaces = new ArrayList<Reference>(); 2818 for (Reference i : replaces) 2819 dst.replaces.add(i.copy()); 2820 }; 2821 dst.requisition = requisition == null ? null : requisition.copy(); 2822 dst.status = status == null ? null : status.copy(); 2823 dst.intent = intent == null ? null : intent.copy(); 2824 dst.priority = priority == null ? null : priority.copy(); 2825 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2826 if (category != null) { 2827 dst.category = new ArrayList<CodeableConcept>(); 2828 for (CodeableConcept i : category) 2829 dst.category.add(i.copy()); 2830 }; 2831 dst.code = code == null ? null : code.copy(); 2832 dst.subject = subject == null ? null : subject.copy(); 2833 dst.context = context == null ? null : context.copy(); 2834 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2835 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 2836 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2837 dst.requester = requester == null ? null : requester.copy(); 2838 dst.performerType = performerType == null ? null : performerType.copy(); 2839 dst.performer = performer == null ? null : performer.copy(); 2840 if (reasonCode != null) { 2841 dst.reasonCode = new ArrayList<CodeableConcept>(); 2842 for (CodeableConcept i : reasonCode) 2843 dst.reasonCode.add(i.copy()); 2844 }; 2845 if (reasonReference != null) { 2846 dst.reasonReference = new ArrayList<Reference>(); 2847 for (Reference i : reasonReference) 2848 dst.reasonReference.add(i.copy()); 2849 }; 2850 if (supportingInfo != null) { 2851 dst.supportingInfo = new ArrayList<Reference>(); 2852 for (Reference i : supportingInfo) 2853 dst.supportingInfo.add(i.copy()); 2854 }; 2855 if (specimen != null) { 2856 dst.specimen = new ArrayList<Reference>(); 2857 for (Reference i : specimen) 2858 dst.specimen.add(i.copy()); 2859 }; 2860 if (bodySite != null) { 2861 dst.bodySite = new ArrayList<CodeableConcept>(); 2862 for (CodeableConcept i : bodySite) 2863 dst.bodySite.add(i.copy()); 2864 }; 2865 if (note != null) { 2866 dst.note = new ArrayList<Annotation>(); 2867 for (Annotation i : note) 2868 dst.note.add(i.copy()); 2869 }; 2870 if (relevantHistory != null) { 2871 dst.relevantHistory = new ArrayList<Reference>(); 2872 for (Reference i : relevantHistory) 2873 dst.relevantHistory.add(i.copy()); 2874 }; 2875 return dst; 2876 } 2877 2878 protected ProcedureRequest typedCopy() { 2879 return copy(); 2880 } 2881 2882 @Override 2883 public boolean equalsDeep(Base other_) { 2884 if (!super.equalsDeep(other_)) 2885 return false; 2886 if (!(other_ instanceof ProcedureRequest)) 2887 return false; 2888 ProcedureRequest o = (ProcedureRequest) other_; 2889 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2890 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(requisition, o.requisition, true) 2891 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 2892 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 2893 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) 2894 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2895 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 2896 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2897 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(specimen, o.specimen, true) 2898 && compareDeep(bodySite, o.bodySite, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 2899 ; 2900 } 2901 2902 @Override 2903 public boolean equalsShallow(Base other_) { 2904 if (!super.equalsShallow(other_)) 2905 return false; 2906 if (!(other_ instanceof ProcedureRequest)) 2907 return false; 2908 ProcedureRequest o = (ProcedureRequest) other_; 2909 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 2910 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true) 2911 ; 2912 } 2913 2914 public boolean isEmpty() { 2915 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2916 , replaces, requisition, status, intent, priority, doNotPerform, category, code 2917 , subject, context, occurrence, asNeeded, authoredOn, requester, performerType 2918 , performer, reasonCode, reasonReference, supportingInfo, specimen, bodySite, note 2919 , relevantHistory); 2920 } 2921 2922 @Override 2923 public ResourceType getResourceType() { 2924 return ResourceType.ProcedureRequest; 2925 } 2926 2927 /** 2928 * Search parameter: <b>authored</b> 2929 * <p> 2930 * Description: <b>Date request signed</b><br> 2931 * Type: <b>date</b><br> 2932 * Path: <b>ProcedureRequest.authoredOn</b><br> 2933 * </p> 2934 */ 2935 @SearchParamDefinition(name="authored", path="ProcedureRequest.authoredOn", description="Date request signed", type="date" ) 2936 public static final String SP_AUTHORED = "authored"; 2937 /** 2938 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2939 * <p> 2940 * Description: <b>Date request signed</b><br> 2941 * Type: <b>date</b><br> 2942 * Path: <b>ProcedureRequest.authoredOn</b><br> 2943 * </p> 2944 */ 2945 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2946 2947 /** 2948 * Search parameter: <b>requester</b> 2949 * <p> 2950 * Description: <b>Individual making the request</b><br> 2951 * Type: <b>reference</b><br> 2952 * Path: <b>ProcedureRequest.requester.agent</b><br> 2953 * </p> 2954 */ 2955 @SearchParamDefinition(name="requester", path="ProcedureRequest.requester.agent", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Practitioner.class } ) 2956 public static final String SP_REQUESTER = "requester"; 2957 /** 2958 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2959 * <p> 2960 * Description: <b>Individual making the request</b><br> 2961 * Type: <b>reference</b><br> 2962 * Path: <b>ProcedureRequest.requester.agent</b><br> 2963 * </p> 2964 */ 2965 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2966 2967/** 2968 * Constant for fluent queries to be used to add include statements. Specifies 2969 * the path value of "<b>ProcedureRequest:requester</b>". 2970 */ 2971 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:requester").toLocked(); 2972 2973 /** 2974 * Search parameter: <b>identifier</b> 2975 * <p> 2976 * Description: <b>Identifiers assigned to this order</b><br> 2977 * Type: <b>token</b><br> 2978 * Path: <b>ProcedureRequest.identifier</b><br> 2979 * </p> 2980 */ 2981 @SearchParamDefinition(name="identifier", path="ProcedureRequest.identifier", description="Identifiers assigned to this order", type="token" ) 2982 public static final String SP_IDENTIFIER = "identifier"; 2983 /** 2984 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2985 * <p> 2986 * Description: <b>Identifiers assigned to this order</b><br> 2987 * Type: <b>token</b><br> 2988 * Path: <b>ProcedureRequest.identifier</b><br> 2989 * </p> 2990 */ 2991 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2992 2993 /** 2994 * Search parameter: <b>code</b> 2995 * <p> 2996 * Description: <b>What is being requested/ordered</b><br> 2997 * Type: <b>token</b><br> 2998 * Path: <b>ProcedureRequest.code</b><br> 2999 * </p> 3000 */ 3001 @SearchParamDefinition(name="code", path="ProcedureRequest.code", description="What is being requested/ordered", type="token" ) 3002 public static final String SP_CODE = "code"; 3003 /** 3004 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3005 * <p> 3006 * Description: <b>What is being requested/ordered</b><br> 3007 * Type: <b>token</b><br> 3008 * Path: <b>ProcedureRequest.code</b><br> 3009 * </p> 3010 */ 3011 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3012 3013 /** 3014 * Search parameter: <b>performer</b> 3015 * <p> 3016 * Description: <b>Requested perfomer</b><br> 3017 * Type: <b>reference</b><br> 3018 * Path: <b>ProcedureRequest.performer</b><br> 3019 * </p> 3020 */ 3021 @SearchParamDefinition(name="performer", path="ProcedureRequest.performer", description="Requested perfomer", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3022 public static final String SP_PERFORMER = "performer"; 3023 /** 3024 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3025 * <p> 3026 * Description: <b>Requested perfomer</b><br> 3027 * Type: <b>reference</b><br> 3028 * Path: <b>ProcedureRequest.performer</b><br> 3029 * </p> 3030 */ 3031 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3032 3033/** 3034 * Constant for fluent queries to be used to add include statements. Specifies 3035 * the path value of "<b>ProcedureRequest:performer</b>". 3036 */ 3037 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:performer").toLocked(); 3038 3039 /** 3040 * Search parameter: <b>requisition</b> 3041 * <p> 3042 * Description: <b>Composite Request ID</b><br> 3043 * Type: <b>token</b><br> 3044 * Path: <b>ProcedureRequest.requisition</b><br> 3045 * </p> 3046 */ 3047 @SearchParamDefinition(name="requisition", path="ProcedureRequest.requisition", description="Composite Request ID", type="token" ) 3048 public static final String SP_REQUISITION = "requisition"; 3049 /** 3050 * <b>Fluent Client</b> search parameter constant for <b>requisition</b> 3051 * <p> 3052 * Description: <b>Composite Request ID</b><br> 3053 * Type: <b>token</b><br> 3054 * Path: <b>ProcedureRequest.requisition</b><br> 3055 * </p> 3056 */ 3057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUISITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REQUISITION); 3058 3059 /** 3060 * Search parameter: <b>replaces</b> 3061 * <p> 3062 * Description: <b>What request replaces</b><br> 3063 * Type: <b>reference</b><br> 3064 * Path: <b>ProcedureRequest.replaces</b><br> 3065 * </p> 3066 */ 3067 @SearchParamDefinition(name="replaces", path="ProcedureRequest.replaces", description="What request replaces", type="reference" ) 3068 public static final String SP_REPLACES = "replaces"; 3069 /** 3070 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 3071 * <p> 3072 * Description: <b>What request replaces</b><br> 3073 * Type: <b>reference</b><br> 3074 * Path: <b>ProcedureRequest.replaces</b><br> 3075 * </p> 3076 */ 3077 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 3078 3079/** 3080 * Constant for fluent queries to be used to add include statements. Specifies 3081 * the path value of "<b>ProcedureRequest:replaces</b>". 3082 */ 3083 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("ProcedureRequest:replaces").toLocked(); 3084 3085 /** 3086 * Search parameter: <b>subject</b> 3087 * <p> 3088 * Description: <b>Search by subject</b><br> 3089 * Type: <b>reference</b><br> 3090 * Path: <b>ProcedureRequest.subject</b><br> 3091 * </p> 3092 */ 3093 @SearchParamDefinition(name="subject", path="ProcedureRequest.subject", description="Search by subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 3094 public static final String SP_SUBJECT = "subject"; 3095 /** 3096 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3097 * <p> 3098 * Description: <b>Search by subject</b><br> 3099 * Type: <b>reference</b><br> 3100 * Path: <b>ProcedureRequest.subject</b><br> 3101 * </p> 3102 */ 3103 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3104 3105/** 3106 * Constant for fluent queries to be used to add include statements. Specifies 3107 * the path value of "<b>ProcedureRequest:subject</b>". 3108 */ 3109 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:subject").toLocked(); 3110 3111 /** 3112 * Search parameter: <b>encounter</b> 3113 * <p> 3114 * Description: <b>An encounter in which this request is made</b><br> 3115 * Type: <b>reference</b><br> 3116 * Path: <b>ProcedureRequest.context</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name="encounter", path="ProcedureRequest.context", description="An encounter in which this request is made", type="reference", target={Encounter.class } ) 3120 public static final String SP_ENCOUNTER = "encounter"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3123 * <p> 3124 * Description: <b>An encounter in which this request is made</b><br> 3125 * Type: <b>reference</b><br> 3126 * Path: <b>ProcedureRequest.context</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3130 3131/** 3132 * Constant for fluent queries to be used to add include statements. Specifies 3133 * the path value of "<b>ProcedureRequest:encounter</b>". 3134 */ 3135 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:encounter").toLocked(); 3136 3137 /** 3138 * Search parameter: <b>occurrence</b> 3139 * <p> 3140 * Description: <b>When procedure should occur</b><br> 3141 * Type: <b>date</b><br> 3142 * Path: <b>ProcedureRequest.occurrence[x]</b><br> 3143 * </p> 3144 */ 3145 @SearchParamDefinition(name="occurrence", path="ProcedureRequest.occurrence", description="When procedure should occur", type="date" ) 3146 public static final String SP_OCCURRENCE = "occurrence"; 3147 /** 3148 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3149 * <p> 3150 * Description: <b>When procedure should occur</b><br> 3151 * Type: <b>date</b><br> 3152 * Path: <b>ProcedureRequest.occurrence[x]</b><br> 3153 * </p> 3154 */ 3155 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 3156 3157 /** 3158 * Search parameter: <b>priority</b> 3159 * <p> 3160 * Description: <b>routine | urgent | asap | stat</b><br> 3161 * Type: <b>token</b><br> 3162 * Path: <b>ProcedureRequest.priority</b><br> 3163 * </p> 3164 */ 3165 @SearchParamDefinition(name="priority", path="ProcedureRequest.priority", description="routine | urgent | asap | stat", type="token" ) 3166 public static final String SP_PRIORITY = "priority"; 3167 /** 3168 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3169 * <p> 3170 * Description: <b>routine | urgent | asap | stat</b><br> 3171 * Type: <b>token</b><br> 3172 * Path: <b>ProcedureRequest.priority</b><br> 3173 * </p> 3174 */ 3175 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 3176 3177 /** 3178 * Search parameter: <b>intent</b> 3179 * <p> 3180 * Description: <b>proposal | plan | order +</b><br> 3181 * Type: <b>token</b><br> 3182 * Path: <b>ProcedureRequest.intent</b><br> 3183 * </p> 3184 */ 3185 @SearchParamDefinition(name="intent", path="ProcedureRequest.intent", description="proposal | plan | order +", type="token" ) 3186 public static final String SP_INTENT = "intent"; 3187 /** 3188 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 3189 * <p> 3190 * Description: <b>proposal | plan | order +</b><br> 3191 * Type: <b>token</b><br> 3192 * Path: <b>ProcedureRequest.intent</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 3196 3197 /** 3198 * Search parameter: <b>performer-type</b> 3199 * <p> 3200 * Description: <b>Performer role</b><br> 3201 * Type: <b>token</b><br> 3202 * Path: <b>ProcedureRequest.performerType</b><br> 3203 * </p> 3204 */ 3205 @SearchParamDefinition(name="performer-type", path="ProcedureRequest.performerType", description="Performer role", type="token" ) 3206 public static final String SP_PERFORMER_TYPE = "performer-type"; 3207 /** 3208 * <b>Fluent Client</b> search parameter constant for <b>performer-type</b> 3209 * <p> 3210 * Description: <b>Performer role</b><br> 3211 * Type: <b>token</b><br> 3212 * Path: <b>ProcedureRequest.performerType</b><br> 3213 * </p> 3214 */ 3215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_TYPE); 3216 3217 /** 3218 * Search parameter: <b>based-on</b> 3219 * <p> 3220 * Description: <b>What request fulfills</b><br> 3221 * Type: <b>reference</b><br> 3222 * Path: <b>ProcedureRequest.basedOn</b><br> 3223 * </p> 3224 */ 3225 @SearchParamDefinition(name="based-on", path="ProcedureRequest.basedOn", description="What request fulfills", type="reference" ) 3226 public static final String SP_BASED_ON = "based-on"; 3227 /** 3228 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3229 * <p> 3230 * Description: <b>What request fulfills</b><br> 3231 * Type: <b>reference</b><br> 3232 * Path: <b>ProcedureRequest.basedOn</b><br> 3233 * </p> 3234 */ 3235 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3236 3237/** 3238 * Constant for fluent queries to be used to add include statements. Specifies 3239 * the path value of "<b>ProcedureRequest:based-on</b>". 3240 */ 3241 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ProcedureRequest:based-on").toLocked(); 3242 3243 /** 3244 * Search parameter: <b>patient</b> 3245 * <p> 3246 * Description: <b>Search by subject - a patient</b><br> 3247 * Type: <b>reference</b><br> 3248 * Path: <b>ProcedureRequest.subject</b><br> 3249 * </p> 3250 */ 3251 @SearchParamDefinition(name="patient", path="ProcedureRequest.subject", description="Search by subject - a patient", type="reference", target={Patient.class } ) 3252 public static final String SP_PATIENT = "patient"; 3253 /** 3254 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3255 * <p> 3256 * Description: <b>Search by subject - a patient</b><br> 3257 * Type: <b>reference</b><br> 3258 * Path: <b>ProcedureRequest.subject</b><br> 3259 * </p> 3260 */ 3261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3262 3263/** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>ProcedureRequest:patient</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:patient").toLocked(); 3268 3269 /** 3270 * Search parameter: <b>specimen</b> 3271 * <p> 3272 * Description: <b>Specimen to be tested</b><br> 3273 * Type: <b>reference</b><br> 3274 * Path: <b>ProcedureRequest.specimen</b><br> 3275 * </p> 3276 */ 3277 @SearchParamDefinition(name="specimen", path="ProcedureRequest.specimen", description="Specimen to be tested", type="reference", target={Specimen.class } ) 3278 public static final String SP_SPECIMEN = "specimen"; 3279 /** 3280 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 3281 * <p> 3282 * Description: <b>Specimen to be tested</b><br> 3283 * Type: <b>reference</b><br> 3284 * Path: <b>ProcedureRequest.specimen</b><br> 3285 * </p> 3286 */ 3287 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 3288 3289/** 3290 * Constant for fluent queries to be used to add include statements. Specifies 3291 * the path value of "<b>ProcedureRequest:specimen</b>". 3292 */ 3293 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("ProcedureRequest:specimen").toLocked(); 3294 3295 /** 3296 * Search parameter: <b>context</b> 3297 * <p> 3298 * Description: <b>Encounter or Episode during which request was created</b><br> 3299 * Type: <b>reference</b><br> 3300 * Path: <b>ProcedureRequest.context</b><br> 3301 * </p> 3302 */ 3303 @SearchParamDefinition(name="context", path="ProcedureRequest.context", description="Encounter or Episode during which request was created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 3304 public static final String SP_CONTEXT = "context"; 3305 /** 3306 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3307 * <p> 3308 * Description: <b>Encounter or Episode during which request was created</b><br> 3309 * Type: <b>reference</b><br> 3310 * Path: <b>ProcedureRequest.context</b><br> 3311 * </p> 3312 */ 3313 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3314 3315/** 3316 * Constant for fluent queries to be used to add include statements. Specifies 3317 * the path value of "<b>ProcedureRequest:context</b>". 3318 */ 3319 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:context").toLocked(); 3320 3321 /** 3322 * Search parameter: <b>definition</b> 3323 * <p> 3324 * Description: <b>Protocol or definition</b><br> 3325 * Type: <b>reference</b><br> 3326 * Path: <b>ProcedureRequest.definition</b><br> 3327 * </p> 3328 */ 3329 @SearchParamDefinition(name="definition", path="ProcedureRequest.definition", description="Protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 3330 public static final String SP_DEFINITION = "definition"; 3331 /** 3332 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 3333 * <p> 3334 * Description: <b>Protocol or definition</b><br> 3335 * Type: <b>reference</b><br> 3336 * Path: <b>ProcedureRequest.definition</b><br> 3337 * </p> 3338 */ 3339 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 3340 3341/** 3342 * Constant for fluent queries to be used to add include statements. Specifies 3343 * the path value of "<b>ProcedureRequest:definition</b>". 3344 */ 3345 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("ProcedureRequest:definition").toLocked(); 3346 3347 /** 3348 * Search parameter: <b>body-site</b> 3349 * <p> 3350 * Description: <b>Where procedure is going to be done</b><br> 3351 * Type: <b>token</b><br> 3352 * Path: <b>ProcedureRequest.bodySite</b><br> 3353 * </p> 3354 */ 3355 @SearchParamDefinition(name="body-site", path="ProcedureRequest.bodySite", description="Where procedure is going to be done", type="token" ) 3356 public static final String SP_BODY_SITE = "body-site"; 3357 /** 3358 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3359 * <p> 3360 * Description: <b>Where procedure is going to be done</b><br> 3361 * Type: <b>token</b><br> 3362 * Path: <b>ProcedureRequest.bodySite</b><br> 3363 * </p> 3364 */ 3365 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3366 3367 /** 3368 * Search parameter: <b>status</b> 3369 * <p> 3370 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled</b><br> 3371 * Type: <b>token</b><br> 3372 * Path: <b>ProcedureRequest.status</b><br> 3373 * </p> 3374 */ 3375 @SearchParamDefinition(name="status", path="ProcedureRequest.status", description="draft | active | suspended | completed | entered-in-error | cancelled", type="token" ) 3376 public static final String SP_STATUS = "status"; 3377 /** 3378 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3379 * <p> 3380 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled</b><br> 3381 * Type: <b>token</b><br> 3382 * Path: <b>ProcedureRequest.status</b><br> 3383 * </p> 3384 */ 3385 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3386 3387 3388}