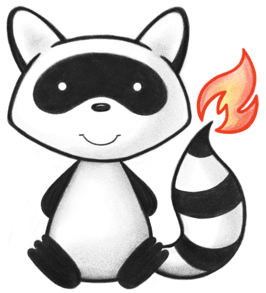
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 050 */ 051@ResourceDef(name="ProcedureRequest", profile="http://hl7.org/fhir/Profile/ProcedureRequest") 052public class ProcedureRequest extends DomainResource { 053 054 public enum ProcedureRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action 057 */ 058 DRAFT, 059 /** 060 * The request is ready to be acted upon 061 */ 062 ACTIVE, 063 /** 064 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 069 */ 070 CANCELLED, 071 /** 072 * Activity against the request has been sufficiently completed to the satisfaction of the requester 073 */ 074 COMPLETED, 075 /** 076 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 077 */ 078 ENTEREDINERROR, 079 /** 080 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static ProcedureRequestStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("suspended".equals(codeString)) 095 return SUSPENDED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("unknown".equals(codeString)) 103 return UNKNOWN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case DRAFT: return "draft"; 112 case ACTIVE: return "active"; 113 case SUSPENDED: return "suspended"; 114 case CANCELLED: return "cancelled"; 115 case COMPLETED: return "completed"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case UNKNOWN: return "unknown"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case DRAFT: return "http://hl7.org/fhir/request-status"; 125 case ACTIVE: return "http://hl7.org/fhir/request-status"; 126 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 127 case CANCELLED: return "http://hl7.org/fhir/request-status"; 128 case COMPLETED: return "http://hl7.org/fhir/request-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 130 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 138 case ACTIVE: return "The request is ready to be acted upon"; 139 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 140 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 141 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 142 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 143 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case DRAFT: return "Draft"; 151 case ACTIVE: return "Active"; 152 case SUSPENDED: return "Suspended"; 153 case CANCELLED: return "Cancelled"; 154 case COMPLETED: return "Completed"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case UNKNOWN: return "Unknown"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class ProcedureRequestStatusEnumFactory implements EnumFactory<ProcedureRequestStatus> { 164 public ProcedureRequestStatus fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("draft".equals(codeString)) 169 return ProcedureRequestStatus.DRAFT; 170 if ("active".equals(codeString)) 171 return ProcedureRequestStatus.ACTIVE; 172 if ("suspended".equals(codeString)) 173 return ProcedureRequestStatus.SUSPENDED; 174 if ("cancelled".equals(codeString)) 175 return ProcedureRequestStatus.CANCELLED; 176 if ("completed".equals(codeString)) 177 return ProcedureRequestStatus.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return ProcedureRequestStatus.ENTEREDINERROR; 180 if ("unknown".equals(codeString)) 181 return ProcedureRequestStatus.UNKNOWN; 182 throw new IllegalArgumentException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 183 } 184 public Enumeration<ProcedureRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<ProcedureRequestStatus>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("draft".equals(codeString)) 193 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.DRAFT); 194 if ("active".equals(codeString)) 195 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ACTIVE); 196 if ("suspended".equals(codeString)) 197 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.SUSPENDED); 198 if ("cancelled".equals(codeString)) 199 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.CANCELLED); 200 if ("completed".equals(codeString)) 201 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.COMPLETED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ENTEREDINERROR); 204 if ("unknown".equals(codeString)) 205 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.UNKNOWN); 206 throw new FHIRException("Unknown ProcedureRequestStatus code '"+codeString+"'"); 207 } 208 public String toCode(ProcedureRequestStatus code) { 209 if (code == ProcedureRequestStatus.NULL) 210 return null; 211 if (code == ProcedureRequestStatus.DRAFT) 212 return "draft"; 213 if (code == ProcedureRequestStatus.ACTIVE) 214 return "active"; 215 if (code == ProcedureRequestStatus.SUSPENDED) 216 return "suspended"; 217 if (code == ProcedureRequestStatus.CANCELLED) 218 return "cancelled"; 219 if (code == ProcedureRequestStatus.COMPLETED) 220 return "completed"; 221 if (code == ProcedureRequestStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == ProcedureRequestStatus.UNKNOWN) 224 return "unknown"; 225 return "?"; 226 } 227 public String toSystem(ProcedureRequestStatus code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum ProcedureRequestIntent { 233 /** 234 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 235 */ 236 PROPOSAL, 237 /** 238 * The request represents an intension to ensure something occurs without providing an authorization for others to act 239 */ 240 PLAN, 241 /** 242 * The request represents a request/demand and authorization for action 243 */ 244 ORDER, 245 /** 246 * The request represents an original authorization for action 247 */ 248 ORIGINALORDER, 249 /** 250 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 251 */ 252 REFLEXORDER, 253 /** 254 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 255 */ 256 FILLERORDER, 257 /** 258 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 259 */ 260 INSTANCEORDER, 261 /** 262 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 263 264Refer to [[[RequestGroup]]] for additional information on how this status is used 265 */ 266 OPTION, 267 /** 268 * added to help the parsers with the generic types 269 */ 270 NULL; 271 public static ProcedureRequestIntent fromCode(String codeString) throws FHIRException { 272 if (codeString == null || "".equals(codeString)) 273 return null; 274 if ("proposal".equals(codeString)) 275 return PROPOSAL; 276 if ("plan".equals(codeString)) 277 return PLAN; 278 if ("order".equals(codeString)) 279 return ORDER; 280 if ("original-order".equals(codeString)) 281 return ORIGINALORDER; 282 if ("reflex-order".equals(codeString)) 283 return REFLEXORDER; 284 if ("filler-order".equals(codeString)) 285 return FILLERORDER; 286 if ("instance-order".equals(codeString)) 287 return INSTANCEORDER; 288 if ("option".equals(codeString)) 289 return OPTION; 290 if (Configuration.isAcceptInvalidEnums()) 291 return null; 292 else 293 throw new FHIRException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 294 } 295 public String toCode() { 296 switch (this) { 297 case PROPOSAL: return "proposal"; 298 case PLAN: return "plan"; 299 case ORDER: return "order"; 300 case ORIGINALORDER: return "original-order"; 301 case REFLEXORDER: return "reflex-order"; 302 case FILLERORDER: return "filler-order"; 303 case INSTANCEORDER: return "instance-order"; 304 case OPTION: return "option"; 305 case NULL: return null; 306 default: return "?"; 307 } 308 } 309 public String getSystem() { 310 switch (this) { 311 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 312 case PLAN: return "http://hl7.org/fhir/request-intent"; 313 case ORDER: return "http://hl7.org/fhir/request-intent"; 314 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 315 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 316 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 317 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 318 case OPTION: return "http://hl7.org/fhir/request-intent"; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getDefinition() { 324 switch (this) { 325 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 326 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 327 case ORDER: return "The request represents a request/demand and authorization for action"; 328 case ORIGINALORDER: return "The request represents an original authorization for action"; 329 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 330 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 331 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 332 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 333 case NULL: return null; 334 default: return "?"; 335 } 336 } 337 public String getDisplay() { 338 switch (this) { 339 case PROPOSAL: return "Proposal"; 340 case PLAN: return "Plan"; 341 case ORDER: return "Order"; 342 case ORIGINALORDER: return "Original Order"; 343 case REFLEXORDER: return "Reflex Order"; 344 case FILLERORDER: return "Filler Order"; 345 case INSTANCEORDER: return "Instance Order"; 346 case OPTION: return "Option"; 347 case NULL: return null; 348 default: return "?"; 349 } 350 } 351 } 352 353 public static class ProcedureRequestIntentEnumFactory implements EnumFactory<ProcedureRequestIntent> { 354 public ProcedureRequestIntent fromCode(String codeString) throws IllegalArgumentException { 355 if (codeString == null || "".equals(codeString)) 356 if (codeString == null || "".equals(codeString)) 357 return null; 358 if ("proposal".equals(codeString)) 359 return ProcedureRequestIntent.PROPOSAL; 360 if ("plan".equals(codeString)) 361 return ProcedureRequestIntent.PLAN; 362 if ("order".equals(codeString)) 363 return ProcedureRequestIntent.ORDER; 364 if ("original-order".equals(codeString)) 365 return ProcedureRequestIntent.ORIGINALORDER; 366 if ("reflex-order".equals(codeString)) 367 return ProcedureRequestIntent.REFLEXORDER; 368 if ("filler-order".equals(codeString)) 369 return ProcedureRequestIntent.FILLERORDER; 370 if ("instance-order".equals(codeString)) 371 return ProcedureRequestIntent.INSTANCEORDER; 372 if ("option".equals(codeString)) 373 return ProcedureRequestIntent.OPTION; 374 throw new IllegalArgumentException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 375 } 376 public Enumeration<ProcedureRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 377 if (code == null) 378 return null; 379 if (code.isEmpty()) 380 return new Enumeration<ProcedureRequestIntent>(this); 381 String codeString = code.asStringValue(); 382 if (codeString == null || "".equals(codeString)) 383 return null; 384 if ("proposal".equals(codeString)) 385 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.PROPOSAL); 386 if ("plan".equals(codeString)) 387 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.PLAN); 388 if ("order".equals(codeString)) 389 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.ORDER); 390 if ("original-order".equals(codeString)) 391 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.ORIGINALORDER); 392 if ("reflex-order".equals(codeString)) 393 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.REFLEXORDER); 394 if ("filler-order".equals(codeString)) 395 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.FILLERORDER); 396 if ("instance-order".equals(codeString)) 397 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.INSTANCEORDER); 398 if ("option".equals(codeString)) 399 return new Enumeration<ProcedureRequestIntent>(this, ProcedureRequestIntent.OPTION); 400 throw new FHIRException("Unknown ProcedureRequestIntent code '"+codeString+"'"); 401 } 402 public String toCode(ProcedureRequestIntent code) { 403 if (code == ProcedureRequestIntent.NULL) 404 return null; 405 if (code == ProcedureRequestIntent.PROPOSAL) 406 return "proposal"; 407 if (code == ProcedureRequestIntent.PLAN) 408 return "plan"; 409 if (code == ProcedureRequestIntent.ORDER) 410 return "order"; 411 if (code == ProcedureRequestIntent.ORIGINALORDER) 412 return "original-order"; 413 if (code == ProcedureRequestIntent.REFLEXORDER) 414 return "reflex-order"; 415 if (code == ProcedureRequestIntent.FILLERORDER) 416 return "filler-order"; 417 if (code == ProcedureRequestIntent.INSTANCEORDER) 418 return "instance-order"; 419 if (code == ProcedureRequestIntent.OPTION) 420 return "option"; 421 return "?"; 422 } 423 public String toSystem(ProcedureRequestIntent code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum ProcedureRequestPriority { 429 /** 430 * The request has normal priority 431 */ 432 ROUTINE, 433 /** 434 * The request should be actioned promptly - higher priority than routine 435 */ 436 URGENT, 437 /** 438 * The request should be actioned as soon as possible - higher priority than urgent 439 */ 440 ASAP, 441 /** 442 * The request should be actioned immediately - highest possible priority. E.g. an emergency 443 */ 444 STAT, 445 /** 446 * added to help the parsers with the generic types 447 */ 448 NULL; 449 public static ProcedureRequestPriority fromCode(String codeString) throws FHIRException { 450 if (codeString == null || "".equals(codeString)) 451 return null; 452 if ("routine".equals(codeString)) 453 return ROUTINE; 454 if ("urgent".equals(codeString)) 455 return URGENT; 456 if ("asap".equals(codeString)) 457 return ASAP; 458 if ("stat".equals(codeString)) 459 return STAT; 460 if (Configuration.isAcceptInvalidEnums()) 461 return null; 462 else 463 throw new FHIRException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 464 } 465 public String toCode() { 466 switch (this) { 467 case ROUTINE: return "routine"; 468 case URGENT: return "urgent"; 469 case ASAP: return "asap"; 470 case STAT: return "stat"; 471 case NULL: return null; 472 default: return "?"; 473 } 474 } 475 public String getSystem() { 476 switch (this) { 477 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 478 case URGENT: return "http://hl7.org/fhir/request-priority"; 479 case ASAP: return "http://hl7.org/fhir/request-priority"; 480 case STAT: return "http://hl7.org/fhir/request-priority"; 481 case NULL: return null; 482 default: return "?"; 483 } 484 } 485 public String getDefinition() { 486 switch (this) { 487 case ROUTINE: return "The request has normal priority"; 488 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 489 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 490 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 491 case NULL: return null; 492 default: return "?"; 493 } 494 } 495 public String getDisplay() { 496 switch (this) { 497 case ROUTINE: return "Routine"; 498 case URGENT: return "Urgent"; 499 case ASAP: return "ASAP"; 500 case STAT: return "STAT"; 501 case NULL: return null; 502 default: return "?"; 503 } 504 } 505 } 506 507 public static class ProcedureRequestPriorityEnumFactory implements EnumFactory<ProcedureRequestPriority> { 508 public ProcedureRequestPriority fromCode(String codeString) throws IllegalArgumentException { 509 if (codeString == null || "".equals(codeString)) 510 if (codeString == null || "".equals(codeString)) 511 return null; 512 if ("routine".equals(codeString)) 513 return ProcedureRequestPriority.ROUTINE; 514 if ("urgent".equals(codeString)) 515 return ProcedureRequestPriority.URGENT; 516 if ("asap".equals(codeString)) 517 return ProcedureRequestPriority.ASAP; 518 if ("stat".equals(codeString)) 519 return ProcedureRequestPriority.STAT; 520 throw new IllegalArgumentException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 521 } 522 public Enumeration<ProcedureRequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 523 if (code == null) 524 return null; 525 if (code.isEmpty()) 526 return new Enumeration<ProcedureRequestPriority>(this); 527 String codeString = code.asStringValue(); 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("routine".equals(codeString)) 531 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ROUTINE); 532 if ("urgent".equals(codeString)) 533 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.URGENT); 534 if ("asap".equals(codeString)) 535 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ASAP); 536 if ("stat".equals(codeString)) 537 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.STAT); 538 throw new FHIRException("Unknown ProcedureRequestPriority code '"+codeString+"'"); 539 } 540 public String toCode(ProcedureRequestPriority code) { 541 if (code == ProcedureRequestPriority.NULL) 542 return null; 543 if (code == ProcedureRequestPriority.ROUTINE) 544 return "routine"; 545 if (code == ProcedureRequestPriority.URGENT) 546 return "urgent"; 547 if (code == ProcedureRequestPriority.ASAP) 548 return "asap"; 549 if (code == ProcedureRequestPriority.STAT) 550 return "stat"; 551 return "?"; 552 } 553 public String toSystem(ProcedureRequestPriority code) { 554 return code.getSystem(); 555 } 556 } 557 558 @Block() 559 public static class ProcedureRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 560 /** 561 * The device, practitioner or organization who initiated the request. 562 */ 563 @Child(name = "agent", type = {Device.class, Practitioner.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=true) 564 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner or organization who initiated the request." ) 565 protected Reference agent; 566 567 /** 568 * The actual object that is the target of the reference (The device, practitioner or organization who initiated the request.) 569 */ 570 protected Resource agentTarget; 571 572 /** 573 * The organization the device or practitioner was acting on behalf of. 574 */ 575 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 576 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 577 protected Reference onBehalfOf; 578 579 /** 580 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 581 */ 582 protected Organization onBehalfOfTarget; 583 584 private static final long serialVersionUID = -71453027L; 585 586 /** 587 * Constructor 588 */ 589 public ProcedureRequestRequesterComponent() { 590 super(); 591 } 592 593 /** 594 * Constructor 595 */ 596 public ProcedureRequestRequesterComponent(Reference agent) { 597 super(); 598 this.agent = agent; 599 } 600 601 /** 602 * @return {@link #agent} (The device, practitioner or organization who initiated the request.) 603 */ 604 public Reference getAgent() { 605 if (this.agent == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.agent"); 608 else if (Configuration.doAutoCreate()) 609 this.agent = new Reference(); // cc 610 return this.agent; 611 } 612 613 public boolean hasAgent() { 614 return this.agent != null && !this.agent.isEmpty(); 615 } 616 617 /** 618 * @param value {@link #agent} (The device, practitioner or organization who initiated the request.) 619 */ 620 public ProcedureRequestRequesterComponent setAgent(Reference value) { 621 this.agent = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner or organization who initiated the request.) 627 */ 628 public Resource getAgentTarget() { 629 return this.agentTarget; 630 } 631 632 /** 633 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner or organization who initiated the request.) 634 */ 635 public ProcedureRequestRequesterComponent setAgentTarget(Resource value) { 636 this.agentTarget = value; 637 return this; 638 } 639 640 /** 641 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 642 */ 643 public Reference getOnBehalfOf() { 644 if (this.onBehalfOf == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.onBehalfOf"); 647 else if (Configuration.doAutoCreate()) 648 this.onBehalfOf = new Reference(); // cc 649 return this.onBehalfOf; 650 } 651 652 public boolean hasOnBehalfOf() { 653 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 658 */ 659 public ProcedureRequestRequesterComponent setOnBehalfOf(Reference value) { 660 this.onBehalfOf = value; 661 return this; 662 } 663 664 /** 665 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 666 */ 667 public Organization getOnBehalfOfTarget() { 668 if (this.onBehalfOfTarget == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create ProcedureRequestRequesterComponent.onBehalfOf"); 671 else if (Configuration.doAutoCreate()) 672 this.onBehalfOfTarget = new Organization(); // aa 673 return this.onBehalfOfTarget; 674 } 675 676 /** 677 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 678 */ 679 public ProcedureRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 680 this.onBehalfOfTarget = value; 681 return this; 682 } 683 684 protected void listChildren(List<Property> children) { 685 super.listChildren(children); 686 children.add(new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner or organization who initiated the request.", 0, 1, agent)); 687 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 688 } 689 690 @Override 691 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 692 switch (_hash) { 693 case 92750597: /*agent*/ return new Property("agent", "Reference(Device|Practitioner|Organization)", "The device, practitioner or organization who initiated the request.", 0, 1, agent); 694 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 695 default: return super.getNamedProperty(_hash, _name, _checkValid); 696 } 697 698 } 699 700 @Override 701 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 702 switch (hash) { 703 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 704 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 705 default: return super.getProperty(hash, name, checkValid); 706 } 707 708 } 709 710 @Override 711 public Base setProperty(int hash, String name, Base value) throws FHIRException { 712 switch (hash) { 713 case 92750597: // agent 714 this.agent = castToReference(value); // Reference 715 return value; 716 case -14402964: // onBehalfOf 717 this.onBehalfOf = castToReference(value); // Reference 718 return value; 719 default: return super.setProperty(hash, name, value); 720 } 721 722 } 723 724 @Override 725 public Base setProperty(String name, Base value) throws FHIRException { 726 if (name.equals("agent")) { 727 this.agent = castToReference(value); // Reference 728 } else if (name.equals("onBehalfOf")) { 729 this.onBehalfOf = castToReference(value); // Reference 730 } else 731 return super.setProperty(name, value); 732 return value; 733 } 734 735 @Override 736 public Base makeProperty(int hash, String name) throws FHIRException { 737 switch (hash) { 738 case 92750597: return getAgent(); 739 case -14402964: return getOnBehalfOf(); 740 default: return super.makeProperty(hash, name); 741 } 742 743 } 744 745 @Override 746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 747 switch (hash) { 748 case 92750597: /*agent*/ return new String[] {"Reference"}; 749 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 750 default: return super.getTypesForProperty(hash, name); 751 } 752 753 } 754 755 @Override 756 public Base addChild(String name) throws FHIRException { 757 if (name.equals("agent")) { 758 this.agent = new Reference(); 759 return this.agent; 760 } 761 else if (name.equals("onBehalfOf")) { 762 this.onBehalfOf = new Reference(); 763 return this.onBehalfOf; 764 } 765 else 766 return super.addChild(name); 767 } 768 769 public ProcedureRequestRequesterComponent copy() { 770 ProcedureRequestRequesterComponent dst = new ProcedureRequestRequesterComponent(); 771 copyValues(dst); 772 dst.agent = agent == null ? null : agent.copy(); 773 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 774 return dst; 775 } 776 777 @Override 778 public boolean equalsDeep(Base other_) { 779 if (!super.equalsDeep(other_)) 780 return false; 781 if (!(other_ instanceof ProcedureRequestRequesterComponent)) 782 return false; 783 ProcedureRequestRequesterComponent o = (ProcedureRequestRequesterComponent) other_; 784 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 785 } 786 787 @Override 788 public boolean equalsShallow(Base other_) { 789 if (!super.equalsShallow(other_)) 790 return false; 791 if (!(other_ instanceof ProcedureRequestRequesterComponent)) 792 return false; 793 ProcedureRequestRequesterComponent o = (ProcedureRequestRequesterComponent) other_; 794 return true; 795 } 796 797 public boolean isEmpty() { 798 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 799 } 800 801 public String fhirType() { 802 return "ProcedureRequest.requester"; 803 804 } 805 806 } 807 808 /** 809 * Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller. 810 */ 811 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 812 @Description(shortDefinition="Identifiers assigned to this order", formalDefinition="Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller." ) 813 protected List<Identifier> identifier; 814 815 /** 816 * Protocol or definition followed by this request. 817 */ 818 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 819 @Description(shortDefinition="Protocol or definition", formalDefinition="Protocol or definition followed by this request." ) 820 protected List<Reference> definition; 821 /** 822 * The actual objects that are the target of the reference (Protocol or definition followed by this request.) 823 */ 824 protected List<Resource> definitionTarget; 825 826 827 /** 828 * Plan/proposal/order fulfilled by this request. 829 */ 830 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 831 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 832 protected List<Reference> basedOn; 833 /** 834 * The actual objects that are the target of the reference (Plan/proposal/order fulfilled by this request.) 835 */ 836 protected List<Resource> basedOnTarget; 837 838 839 /** 840 * The request takes the place of the referenced completed or terminated request(s). 841 */ 842 @Child(name = "replaces", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 843 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 844 protected List<Reference> replaces; 845 /** 846 * The actual objects that are the target of the reference (The request takes the place of the referenced completed or terminated request(s).) 847 */ 848 protected List<Resource> replacesTarget; 849 850 851 /** 852 * A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier. 853 */ 854 @Child(name = "requisition", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 855 @Description(shortDefinition="Composite Request ID", formalDefinition="A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier." ) 856 protected Identifier requisition; 857 858 /** 859 * The status of the order. 860 */ 861 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 862 @Description(shortDefinition="draft | active | suspended | completed | entered-in-error | cancelled", formalDefinition="The status of the order." ) 863 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 864 protected Enumeration<ProcedureRequestStatus> status; 865 866 /** 867 * Whether the request is a proposal, plan, an original order or a reflex order. 868 */ 869 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 870 @Description(shortDefinition="proposal | plan | order +", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 871 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 872 protected Enumeration<ProcedureRequestIntent> intent; 873 874 /** 875 * Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 876 */ 877 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 878 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the ProcedureRequest should be addressed with respect to other requests." ) 879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 880 protected Enumeration<ProcedureRequestPriority> priority; 881 882 /** 883 * Set this to true if the record is saying that the procedure should NOT be performed. 884 */ 885 @Child(name = "doNotPerform", type = {BooleanType.class}, order=8, min=0, max=1, modifier=true, summary=true) 886 @Description(shortDefinition="True if procedure should not be performed", formalDefinition="Set this to true if the record is saying that the procedure should NOT be performed." ) 887 protected BooleanType doNotPerform; 888 889 /** 890 * A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure"). 891 */ 892 @Child(name = "category", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 893 @Description(shortDefinition="Classification of procedure", formalDefinition="A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\")." ) 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-category") 895 protected List<CodeableConcept> category; 896 897 /** 898 * A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested. 899 */ 900 @Child(name = "code", type = {CodeableConcept.class}, order=10, min=1, max=1, modifier=false, summary=true) 901 @Description(shortDefinition="What is being requested/ordered", formalDefinition="A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 903 protected CodeableConcept code; 904 905 /** 906 * On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans). 907 */ 908 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=11, min=1, max=1, modifier=false, summary=true) 909 @Description(shortDefinition="Individual the service is ordered for", formalDefinition="On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans)." ) 910 protected Reference subject; 911 912 /** 913 * The actual object that is the target of the reference (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 914 */ 915 protected Resource subjectTarget; 916 917 /** 918 * An encounter or episode of care that provides additional information about the healthcare context in which this request is made. 919 */ 920 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=12, min=0, max=1, modifier=false, summary=true) 921 @Description(shortDefinition="Encounter or Episode during which request was created", formalDefinition="An encounter or episode of care that provides additional information about the healthcare context in which this request is made." ) 922 protected Reference context; 923 924 /** 925 * The actual object that is the target of the reference (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 926 */ 927 protected Resource contextTarget; 928 929 /** 930 * The date/time at which the diagnostic testing should occur. 931 */ 932 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=13, min=0, max=1, modifier=false, summary=true) 933 @Description(shortDefinition="When procedure should occur", formalDefinition="The date/time at which the diagnostic testing should occur." ) 934 protected Type occurrence; 935 936 /** 937 * If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc. 938 */ 939 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=true) 940 @Description(shortDefinition="Preconditions for procedure or diagnostic", formalDefinition="If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 942 protected Type asNeeded; 943 944 /** 945 * When the request transitioned to being actionable. 946 */ 947 @Child(name = "authoredOn", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="Date request signed", formalDefinition="When the request transitioned to being actionable." ) 949 protected DateTimeType authoredOn; 950 951 /** 952 * The individual who initiated the request and has responsibility for its activation. 953 */ 954 @Child(name = "requester", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 955 @Description(shortDefinition="Who/what is requesting procedure or diagnostic", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 956 protected ProcedureRequestRequesterComponent requester; 957 958 /** 959 * Desired type of performer for doing the diagnostic testing. 960 */ 961 @Child(name = "performerType", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 962 @Description(shortDefinition="Performer role", formalDefinition="Desired type of performer for doing the diagnostic testing." ) 963 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 964 protected CodeableConcept performerType; 965 966 /** 967 * The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc. 968 */ 969 @Child(name = "performer", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, HealthcareService.class}, order=18, min=0, max=1, modifier=false, summary=true) 970 @Description(shortDefinition="Requested perfomer", formalDefinition="The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc." ) 971 protected Reference performer; 972 973 /** 974 * The actual object that is the target of the reference (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 975 */ 976 protected Resource performerTarget; 977 978 /** 979 * An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation. 980 */ 981 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 982 @Description(shortDefinition="Explanation/Justification for test", formalDefinition="An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation." ) 983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 984 protected List<CodeableConcept> reasonCode; 985 986 /** 987 * Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation. 988 */ 989 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 990 @Description(shortDefinition="Explanation/Justification for test", formalDefinition="Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation." ) 991 protected List<Reference> reasonReference; 992 /** 993 * The actual objects that are the target of the reference (Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.) 994 */ 995 protected List<Resource> reasonReferenceTarget; 996 997 998 /** 999 * Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements. 1000 */ 1001 @Child(name = "supportingInfo", type = {Reference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1002 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements." ) 1003 protected List<Reference> supportingInfo; 1004 /** 1005 * The actual objects that are the target of the reference (Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.) 1006 */ 1007 protected List<Resource> supportingInfoTarget; 1008 1009 1010 /** 1011 * One or more specimens that the laboratory procedure will use. 1012 */ 1013 @Child(name = "specimen", type = {Specimen.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1014 @Description(shortDefinition="Procedure Samples", formalDefinition="One or more specimens that the laboratory procedure will use." ) 1015 protected List<Reference> specimen; 1016 /** 1017 * The actual objects that are the target of the reference (One or more specimens that the laboratory procedure will use.) 1018 */ 1019 protected List<Specimen> specimenTarget; 1020 1021 1022 /** 1023 * Anatomic location where the procedure should be performed. This is the target site. 1024 */ 1025 @Child(name = "bodySite", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1026 @Description(shortDefinition="Location on Body", formalDefinition="Anatomic location where the procedure should be performed. This is the target site." ) 1027 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1028 protected List<CodeableConcept> bodySite; 1029 1030 /** 1031 * Any other notes and comments made about the service request. For example, letting provider know that "patient hates needles" or other provider instructions. 1032 */ 1033 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1034 @Description(shortDefinition="Comments", formalDefinition="Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions." ) 1035 protected List<Annotation> note; 1036 1037 /** 1038 * Key events in the history of the request. 1039 */ 1040 @Child(name = "relevantHistory", type = {Provenance.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1041 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 1042 protected List<Reference> relevantHistory; 1043 /** 1044 * The actual objects that are the target of the reference (Key events in the history of the request.) 1045 */ 1046 protected List<Provenance> relevantHistoryTarget; 1047 1048 1049 private static final long serialVersionUID = 184396216L; 1050 1051 /** 1052 * Constructor 1053 */ 1054 public ProcedureRequest() { 1055 super(); 1056 } 1057 1058 /** 1059 * Constructor 1060 */ 1061 public ProcedureRequest(Enumeration<ProcedureRequestStatus> status, Enumeration<ProcedureRequestIntent> intent, CodeableConcept code, Reference subject) { 1062 super(); 1063 this.status = status; 1064 this.intent = intent; 1065 this.code = code; 1066 this.subject = subject; 1067 } 1068 1069 /** 1070 * @return {@link #identifier} (Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.) 1071 */ 1072 public List<Identifier> getIdentifier() { 1073 if (this.identifier == null) 1074 this.identifier = new ArrayList<Identifier>(); 1075 return this.identifier; 1076 } 1077 1078 /** 1079 * @return Returns a reference to <code>this</code> for easy method chaining 1080 */ 1081 public ProcedureRequest setIdentifier(List<Identifier> theIdentifier) { 1082 this.identifier = theIdentifier; 1083 return this; 1084 } 1085 1086 public boolean hasIdentifier() { 1087 if (this.identifier == null) 1088 return false; 1089 for (Identifier item : this.identifier) 1090 if (!item.isEmpty()) 1091 return true; 1092 return false; 1093 } 1094 1095 public Identifier addIdentifier() { //3 1096 Identifier t = new Identifier(); 1097 if (this.identifier == null) 1098 this.identifier = new ArrayList<Identifier>(); 1099 this.identifier.add(t); 1100 return t; 1101 } 1102 1103 public ProcedureRequest addIdentifier(Identifier t) { //3 1104 if (t == null) 1105 return this; 1106 if (this.identifier == null) 1107 this.identifier = new ArrayList<Identifier>(); 1108 this.identifier.add(t); 1109 return this; 1110 } 1111 1112 /** 1113 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1114 */ 1115 public Identifier getIdentifierFirstRep() { 1116 if (getIdentifier().isEmpty()) { 1117 addIdentifier(); 1118 } 1119 return getIdentifier().get(0); 1120 } 1121 1122 /** 1123 * @return {@link #definition} (Protocol or definition followed by this request.) 1124 */ 1125 public List<Reference> getDefinition() { 1126 if (this.definition == null) 1127 this.definition = new ArrayList<Reference>(); 1128 return this.definition; 1129 } 1130 1131 /** 1132 * @return Returns a reference to <code>this</code> for easy method chaining 1133 */ 1134 public ProcedureRequest setDefinition(List<Reference> theDefinition) { 1135 this.definition = theDefinition; 1136 return this; 1137 } 1138 1139 public boolean hasDefinition() { 1140 if (this.definition == null) 1141 return false; 1142 for (Reference item : this.definition) 1143 if (!item.isEmpty()) 1144 return true; 1145 return false; 1146 } 1147 1148 public Reference addDefinition() { //3 1149 Reference t = new Reference(); 1150 if (this.definition == null) 1151 this.definition = new ArrayList<Reference>(); 1152 this.definition.add(t); 1153 return t; 1154 } 1155 1156 public ProcedureRequest addDefinition(Reference t) { //3 1157 if (t == null) 1158 return this; 1159 if (this.definition == null) 1160 this.definition = new ArrayList<Reference>(); 1161 this.definition.add(t); 1162 return this; 1163 } 1164 1165 /** 1166 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1167 */ 1168 public Reference getDefinitionFirstRep() { 1169 if (getDefinition().isEmpty()) { 1170 addDefinition(); 1171 } 1172 return getDefinition().get(0); 1173 } 1174 1175 /** 1176 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 1177 */ 1178 public List<Reference> getBasedOn() { 1179 if (this.basedOn == null) 1180 this.basedOn = new ArrayList<Reference>(); 1181 return this.basedOn; 1182 } 1183 1184 /** 1185 * @return Returns a reference to <code>this</code> for easy method chaining 1186 */ 1187 public ProcedureRequest setBasedOn(List<Reference> theBasedOn) { 1188 this.basedOn = theBasedOn; 1189 return this; 1190 } 1191 1192 public boolean hasBasedOn() { 1193 if (this.basedOn == null) 1194 return false; 1195 for (Reference item : this.basedOn) 1196 if (!item.isEmpty()) 1197 return true; 1198 return false; 1199 } 1200 1201 public Reference addBasedOn() { //3 1202 Reference t = new Reference(); 1203 if (this.basedOn == null) 1204 this.basedOn = new ArrayList<Reference>(); 1205 this.basedOn.add(t); 1206 return t; 1207 } 1208 1209 public ProcedureRequest addBasedOn(Reference t) { //3 1210 if (t == null) 1211 return this; 1212 if (this.basedOn == null) 1213 this.basedOn = new ArrayList<Reference>(); 1214 this.basedOn.add(t); 1215 return this; 1216 } 1217 1218 /** 1219 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1220 */ 1221 public Reference getBasedOnFirstRep() { 1222 if (getBasedOn().isEmpty()) { 1223 addBasedOn(); 1224 } 1225 return getBasedOn().get(0); 1226 } 1227 1228 /** 1229 * @return {@link #replaces} (The request takes the place of the referenced completed or terminated request(s).) 1230 */ 1231 public List<Reference> getReplaces() { 1232 if (this.replaces == null) 1233 this.replaces = new ArrayList<Reference>(); 1234 return this.replaces; 1235 } 1236 1237 /** 1238 * @return Returns a reference to <code>this</code> for easy method chaining 1239 */ 1240 public ProcedureRequest setReplaces(List<Reference> theReplaces) { 1241 this.replaces = theReplaces; 1242 return this; 1243 } 1244 1245 public boolean hasReplaces() { 1246 if (this.replaces == null) 1247 return false; 1248 for (Reference item : this.replaces) 1249 if (!item.isEmpty()) 1250 return true; 1251 return false; 1252 } 1253 1254 public Reference addReplaces() { //3 1255 Reference t = new Reference(); 1256 if (this.replaces == null) 1257 this.replaces = new ArrayList<Reference>(); 1258 this.replaces.add(t); 1259 return t; 1260 } 1261 1262 public ProcedureRequest addReplaces(Reference t) { //3 1263 if (t == null) 1264 return this; 1265 if (this.replaces == null) 1266 this.replaces = new ArrayList<Reference>(); 1267 this.replaces.add(t); 1268 return this; 1269 } 1270 1271 /** 1272 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 1273 */ 1274 public Reference getReplacesFirstRep() { 1275 if (getReplaces().isEmpty()) { 1276 addReplaces(); 1277 } 1278 return getReplaces().get(0); 1279 } 1280 1281 /** 1282 * @return {@link #requisition} (A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1283 */ 1284 public Identifier getRequisition() { 1285 if (this.requisition == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create ProcedureRequest.requisition"); 1288 else if (Configuration.doAutoCreate()) 1289 this.requisition = new Identifier(); // cc 1290 return this.requisition; 1291 } 1292 1293 public boolean hasRequisition() { 1294 return this.requisition != null && !this.requisition.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #requisition} (A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1299 */ 1300 public ProcedureRequest setRequisition(Identifier value) { 1301 this.requisition = value; 1302 return this; 1303 } 1304 1305 /** 1306 * @return {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1307 */ 1308 public Enumeration<ProcedureRequestStatus> getStatusElement() { 1309 if (this.status == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create ProcedureRequest.status"); 1312 else if (Configuration.doAutoCreate()) 1313 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); // bb 1314 return this.status; 1315 } 1316 1317 public boolean hasStatusElement() { 1318 return this.status != null && !this.status.isEmpty(); 1319 } 1320 1321 public boolean hasStatus() { 1322 return this.status != null && !this.status.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1327 */ 1328 public ProcedureRequest setStatusElement(Enumeration<ProcedureRequestStatus> value) { 1329 this.status = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return The status of the order. 1335 */ 1336 public ProcedureRequestStatus getStatus() { 1337 return this.status == null ? null : this.status.getValue(); 1338 } 1339 1340 /** 1341 * @param value The status of the order. 1342 */ 1343 public ProcedureRequest setStatus(ProcedureRequestStatus value) { 1344 if (this.status == null) 1345 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); 1346 this.status.setValue(value); 1347 return this; 1348 } 1349 1350 /** 1351 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1352 */ 1353 public Enumeration<ProcedureRequestIntent> getIntentElement() { 1354 if (this.intent == null) 1355 if (Configuration.errorOnAutoCreate()) 1356 throw new Error("Attempt to auto-create ProcedureRequest.intent"); 1357 else if (Configuration.doAutoCreate()) 1358 this.intent = new Enumeration<ProcedureRequestIntent>(new ProcedureRequestIntentEnumFactory()); // bb 1359 return this.intent; 1360 } 1361 1362 public boolean hasIntentElement() { 1363 return this.intent != null && !this.intent.isEmpty(); 1364 } 1365 1366 public boolean hasIntent() { 1367 return this.intent != null && !this.intent.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1372 */ 1373 public ProcedureRequest setIntentElement(Enumeration<ProcedureRequestIntent> value) { 1374 this.intent = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return Whether the request is a proposal, plan, an original order or a reflex order. 1380 */ 1381 public ProcedureRequestIntent getIntent() { 1382 return this.intent == null ? null : this.intent.getValue(); 1383 } 1384 1385 /** 1386 * @param value Whether the request is a proposal, plan, an original order or a reflex order. 1387 */ 1388 public ProcedureRequest setIntent(ProcedureRequestIntent value) { 1389 if (this.intent == null) 1390 this.intent = new Enumeration<ProcedureRequestIntent>(new ProcedureRequestIntentEnumFactory()); 1391 this.intent.setValue(value); 1392 return this; 1393 } 1394 1395 /** 1396 * @return {@link #priority} (Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1397 */ 1398 public Enumeration<ProcedureRequestPriority> getPriorityElement() { 1399 if (this.priority == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create ProcedureRequest.priority"); 1402 else if (Configuration.doAutoCreate()) 1403 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); // bb 1404 return this.priority; 1405 } 1406 1407 public boolean hasPriorityElement() { 1408 return this.priority != null && !this.priority.isEmpty(); 1409 } 1410 1411 public boolean hasPriority() { 1412 return this.priority != null && !this.priority.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #priority} (Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1417 */ 1418 public ProcedureRequest setPriorityElement(Enumeration<ProcedureRequestPriority> value) { 1419 this.priority = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 1425 */ 1426 public ProcedureRequestPriority getPriority() { 1427 return this.priority == null ? null : this.priority.getValue(); 1428 } 1429 1430 /** 1431 * @param value Indicates how quickly the ProcedureRequest should be addressed with respect to other requests. 1432 */ 1433 public ProcedureRequest setPriority(ProcedureRequestPriority value) { 1434 if (value == null) 1435 this.priority = null; 1436 else { 1437 if (this.priority == null) 1438 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); 1439 this.priority.setValue(value); 1440 } 1441 return this; 1442 } 1443 1444 /** 1445 * @return {@link #doNotPerform} (Set this to true if the record is saying that the procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1446 */ 1447 public BooleanType getDoNotPerformElement() { 1448 if (this.doNotPerform == null) 1449 if (Configuration.errorOnAutoCreate()) 1450 throw new Error("Attempt to auto-create ProcedureRequest.doNotPerform"); 1451 else if (Configuration.doAutoCreate()) 1452 this.doNotPerform = new BooleanType(); // bb 1453 return this.doNotPerform; 1454 } 1455 1456 public boolean hasDoNotPerformElement() { 1457 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1458 } 1459 1460 public boolean hasDoNotPerform() { 1461 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1462 } 1463 1464 /** 1465 * @param value {@link #doNotPerform} (Set this to true if the record is saying that the procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1466 */ 1467 public ProcedureRequest setDoNotPerformElement(BooleanType value) { 1468 this.doNotPerform = value; 1469 return this; 1470 } 1471 1472 /** 1473 * @return Set this to true if the record is saying that the procedure should NOT be performed. 1474 */ 1475 public boolean getDoNotPerform() { 1476 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1477 } 1478 1479 /** 1480 * @param value Set this to true if the record is saying that the procedure should NOT be performed. 1481 */ 1482 public ProcedureRequest setDoNotPerform(boolean value) { 1483 if (this.doNotPerform == null) 1484 this.doNotPerform = new BooleanType(); 1485 this.doNotPerform.setValue(value); 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #category} (A code that classifies the procedure for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1491 */ 1492 public List<CodeableConcept> getCategory() { 1493 if (this.category == null) 1494 this.category = new ArrayList<CodeableConcept>(); 1495 return this.category; 1496 } 1497 1498 /** 1499 * @return Returns a reference to <code>this</code> for easy method chaining 1500 */ 1501 public ProcedureRequest setCategory(List<CodeableConcept> theCategory) { 1502 this.category = theCategory; 1503 return this; 1504 } 1505 1506 public boolean hasCategory() { 1507 if (this.category == null) 1508 return false; 1509 for (CodeableConcept item : this.category) 1510 if (!item.isEmpty()) 1511 return true; 1512 return false; 1513 } 1514 1515 public CodeableConcept addCategory() { //3 1516 CodeableConcept t = new CodeableConcept(); 1517 if (this.category == null) 1518 this.category = new ArrayList<CodeableConcept>(); 1519 this.category.add(t); 1520 return t; 1521 } 1522 1523 public ProcedureRequest addCategory(CodeableConcept t) { //3 1524 if (t == null) 1525 return this; 1526 if (this.category == null) 1527 this.category = new ArrayList<CodeableConcept>(); 1528 this.category.add(t); 1529 return this; 1530 } 1531 1532 /** 1533 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1534 */ 1535 public CodeableConcept getCategoryFirstRep() { 1536 if (getCategory().isEmpty()) { 1537 addCategory(); 1538 } 1539 return getCategory().get(0); 1540 } 1541 1542 /** 1543 * @return {@link #code} (A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.) 1544 */ 1545 public CodeableConcept getCode() { 1546 if (this.code == null) 1547 if (Configuration.errorOnAutoCreate()) 1548 throw new Error("Attempt to auto-create ProcedureRequest.code"); 1549 else if (Configuration.doAutoCreate()) 1550 this.code = new CodeableConcept(); // cc 1551 return this.code; 1552 } 1553 1554 public boolean hasCode() { 1555 return this.code != null && !this.code.isEmpty(); 1556 } 1557 1558 /** 1559 * @param value {@link #code} (A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.) 1560 */ 1561 public ProcedureRequest setCode(CodeableConcept value) { 1562 this.code = value; 1563 return this; 1564 } 1565 1566 /** 1567 * @return {@link #subject} (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1568 */ 1569 public Reference getSubject() { 1570 if (this.subject == null) 1571 if (Configuration.errorOnAutoCreate()) 1572 throw new Error("Attempt to auto-create ProcedureRequest.subject"); 1573 else if (Configuration.doAutoCreate()) 1574 this.subject = new Reference(); // cc 1575 return this.subject; 1576 } 1577 1578 public boolean hasSubject() { 1579 return this.subject != null && !this.subject.isEmpty(); 1580 } 1581 1582 /** 1583 * @param value {@link #subject} (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1584 */ 1585 public ProcedureRequest setSubject(Reference value) { 1586 this.subject = value; 1587 return this; 1588 } 1589 1590 /** 1591 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1592 */ 1593 public Resource getSubjectTarget() { 1594 return this.subjectTarget; 1595 } 1596 1597 /** 1598 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1599 */ 1600 public ProcedureRequest setSubjectTarget(Resource value) { 1601 this.subjectTarget = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #context} (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1607 */ 1608 public Reference getContext() { 1609 if (this.context == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create ProcedureRequest.context"); 1612 else if (Configuration.doAutoCreate()) 1613 this.context = new Reference(); // cc 1614 return this.context; 1615 } 1616 1617 public boolean hasContext() { 1618 return this.context != null && !this.context.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #context} (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1623 */ 1624 public ProcedureRequest setContext(Reference value) { 1625 this.context = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1631 */ 1632 public Resource getContextTarget() { 1633 return this.contextTarget; 1634 } 1635 1636 /** 1637 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An encounter or episode of care that provides additional information about the healthcare context in which this request is made.) 1638 */ 1639 public ProcedureRequest setContextTarget(Resource value) { 1640 this.contextTarget = value; 1641 return this; 1642 } 1643 1644 /** 1645 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1646 */ 1647 public Type getOccurrence() { 1648 return this.occurrence; 1649 } 1650 1651 /** 1652 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1653 */ 1654 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1655 if (this.occurrence == null) 1656 return null; 1657 if (!(this.occurrence instanceof DateTimeType)) 1658 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1659 return (DateTimeType) this.occurrence; 1660 } 1661 1662 public boolean hasOccurrenceDateTimeType() { 1663 return this.occurrence instanceof DateTimeType; 1664 } 1665 1666 /** 1667 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1668 */ 1669 public Period getOccurrencePeriod() throws FHIRException { 1670 if (this.occurrence == null) 1671 return null; 1672 if (!(this.occurrence instanceof Period)) 1673 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1674 return (Period) this.occurrence; 1675 } 1676 1677 public boolean hasOccurrencePeriod() { 1678 return this.occurrence instanceof Period; 1679 } 1680 1681 /** 1682 * @return {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1683 */ 1684 public Timing getOccurrenceTiming() throws FHIRException { 1685 if (this.occurrence == null) 1686 return null; 1687 if (!(this.occurrence instanceof Timing)) 1688 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1689 return (Timing) this.occurrence; 1690 } 1691 1692 public boolean hasOccurrenceTiming() { 1693 return this.occurrence instanceof Timing; 1694 } 1695 1696 public boolean hasOccurrence() { 1697 return this.occurrence != null && !this.occurrence.isEmpty(); 1698 } 1699 1700 /** 1701 * @param value {@link #occurrence} (The date/time at which the diagnostic testing should occur.) 1702 */ 1703 public ProcedureRequest setOccurrence(Type value) throws FHIRFormatError { 1704 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1705 throw new FHIRFormatError("Not the right type for ProcedureRequest.occurrence[x]: "+value.fhirType()); 1706 this.occurrence = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1712 */ 1713 public Type getAsNeeded() { 1714 return this.asNeeded; 1715 } 1716 1717 /** 1718 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1719 */ 1720 public BooleanType getAsNeededBooleanType() throws FHIRException { 1721 if (this.asNeeded == null) 1722 return null; 1723 if (!(this.asNeeded instanceof BooleanType)) 1724 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 1725 return (BooleanType) this.asNeeded; 1726 } 1727 1728 public boolean hasAsNeededBooleanType() { 1729 return this.asNeeded instanceof BooleanType; 1730 } 1731 1732 /** 1733 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1734 */ 1735 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 1736 if (this.asNeeded == null) 1737 return null; 1738 if (!(this.asNeeded instanceof CodeableConcept)) 1739 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 1740 return (CodeableConcept) this.asNeeded; 1741 } 1742 1743 public boolean hasAsNeededCodeableConcept() { 1744 return this.asNeeded instanceof CodeableConcept; 1745 } 1746 1747 public boolean hasAsNeeded() { 1748 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1749 } 1750 1751 /** 1752 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example "pain", "on flare-up", etc.) 1753 */ 1754 public ProcedureRequest setAsNeeded(Type value) throws FHIRFormatError { 1755 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 1756 throw new FHIRFormatError("Not the right type for ProcedureRequest.asNeeded[x]: "+value.fhirType()); 1757 this.asNeeded = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1763 */ 1764 public DateTimeType getAuthoredOnElement() { 1765 if (this.authoredOn == null) 1766 if (Configuration.errorOnAutoCreate()) 1767 throw new Error("Attempt to auto-create ProcedureRequest.authoredOn"); 1768 else if (Configuration.doAutoCreate()) 1769 this.authoredOn = new DateTimeType(); // bb 1770 return this.authoredOn; 1771 } 1772 1773 public boolean hasAuthoredOnElement() { 1774 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1775 } 1776 1777 public boolean hasAuthoredOn() { 1778 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1779 } 1780 1781 /** 1782 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1783 */ 1784 public ProcedureRequest setAuthoredOnElement(DateTimeType value) { 1785 this.authoredOn = value; 1786 return this; 1787 } 1788 1789 /** 1790 * @return When the request transitioned to being actionable. 1791 */ 1792 public Date getAuthoredOn() { 1793 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1794 } 1795 1796 /** 1797 * @param value When the request transitioned to being actionable. 1798 */ 1799 public ProcedureRequest setAuthoredOn(Date value) { 1800 if (value == null) 1801 this.authoredOn = null; 1802 else { 1803 if (this.authoredOn == null) 1804 this.authoredOn = new DateTimeType(); 1805 this.authoredOn.setValue(value); 1806 } 1807 return this; 1808 } 1809 1810 /** 1811 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1812 */ 1813 public ProcedureRequestRequesterComponent getRequester() { 1814 if (this.requester == null) 1815 if (Configuration.errorOnAutoCreate()) 1816 throw new Error("Attempt to auto-create ProcedureRequest.requester"); 1817 else if (Configuration.doAutoCreate()) 1818 this.requester = new ProcedureRequestRequesterComponent(); // cc 1819 return this.requester; 1820 } 1821 1822 public boolean hasRequester() { 1823 return this.requester != null && !this.requester.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1828 */ 1829 public ProcedureRequest setRequester(ProcedureRequestRequesterComponent value) { 1830 this.requester = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1836 */ 1837 public CodeableConcept getPerformerType() { 1838 if (this.performerType == null) 1839 if (Configuration.errorOnAutoCreate()) 1840 throw new Error("Attempt to auto-create ProcedureRequest.performerType"); 1841 else if (Configuration.doAutoCreate()) 1842 this.performerType = new CodeableConcept(); // cc 1843 return this.performerType; 1844 } 1845 1846 public boolean hasPerformerType() { 1847 return this.performerType != null && !this.performerType.isEmpty(); 1848 } 1849 1850 /** 1851 * @param value {@link #performerType} (Desired type of performer for doing the diagnostic testing.) 1852 */ 1853 public ProcedureRequest setPerformerType(CodeableConcept value) { 1854 this.performerType = value; 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #performer} (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1860 */ 1861 public Reference getPerformer() { 1862 if (this.performer == null) 1863 if (Configuration.errorOnAutoCreate()) 1864 throw new Error("Attempt to auto-create ProcedureRequest.performer"); 1865 else if (Configuration.doAutoCreate()) 1866 this.performer = new Reference(); // cc 1867 return this.performer; 1868 } 1869 1870 public boolean hasPerformer() { 1871 return this.performer != null && !this.performer.isEmpty(); 1872 } 1873 1874 /** 1875 * @param value {@link #performer} (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1876 */ 1877 public ProcedureRequest setPerformer(Reference value) { 1878 this.performer = value; 1879 return this; 1880 } 1881 1882 /** 1883 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1884 */ 1885 public Resource getPerformerTarget() { 1886 return this.performerTarget; 1887 } 1888 1889 /** 1890 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.) 1891 */ 1892 public ProcedureRequest setPerformerTarget(Resource value) { 1893 this.performerTarget = value; 1894 return this; 1895 } 1896 1897 /** 1898 * @return {@link #reasonCode} (An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.) 1899 */ 1900 public List<CodeableConcept> getReasonCode() { 1901 if (this.reasonCode == null) 1902 this.reasonCode = new ArrayList<CodeableConcept>(); 1903 return this.reasonCode; 1904 } 1905 1906 /** 1907 * @return Returns a reference to <code>this</code> for easy method chaining 1908 */ 1909 public ProcedureRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1910 this.reasonCode = theReasonCode; 1911 return this; 1912 } 1913 1914 public boolean hasReasonCode() { 1915 if (this.reasonCode == null) 1916 return false; 1917 for (CodeableConcept item : this.reasonCode) 1918 if (!item.isEmpty()) 1919 return true; 1920 return false; 1921 } 1922 1923 public CodeableConcept addReasonCode() { //3 1924 CodeableConcept t = new CodeableConcept(); 1925 if (this.reasonCode == null) 1926 this.reasonCode = new ArrayList<CodeableConcept>(); 1927 this.reasonCode.add(t); 1928 return t; 1929 } 1930 1931 public ProcedureRequest addReasonCode(CodeableConcept t) { //3 1932 if (t == null) 1933 return this; 1934 if (this.reasonCode == null) 1935 this.reasonCode = new ArrayList<CodeableConcept>(); 1936 this.reasonCode.add(t); 1937 return this; 1938 } 1939 1940 /** 1941 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1942 */ 1943 public CodeableConcept getReasonCodeFirstRep() { 1944 if (getReasonCode().isEmpty()) { 1945 addReasonCode(); 1946 } 1947 return getReasonCode().get(0); 1948 } 1949 1950 /** 1951 * @return {@link #reasonReference} (Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.) 1952 */ 1953 public List<Reference> getReasonReference() { 1954 if (this.reasonReference == null) 1955 this.reasonReference = new ArrayList<Reference>(); 1956 return this.reasonReference; 1957 } 1958 1959 /** 1960 * @return Returns a reference to <code>this</code> for easy method chaining 1961 */ 1962 public ProcedureRequest setReasonReference(List<Reference> theReasonReference) { 1963 this.reasonReference = theReasonReference; 1964 return this; 1965 } 1966 1967 public boolean hasReasonReference() { 1968 if (this.reasonReference == null) 1969 return false; 1970 for (Reference item : this.reasonReference) 1971 if (!item.isEmpty()) 1972 return true; 1973 return false; 1974 } 1975 1976 public Reference addReasonReference() { //3 1977 Reference t = new Reference(); 1978 if (this.reasonReference == null) 1979 this.reasonReference = new ArrayList<Reference>(); 1980 this.reasonReference.add(t); 1981 return t; 1982 } 1983 1984 public ProcedureRequest addReasonReference(Reference t) { //3 1985 if (t == null) 1986 return this; 1987 if (this.reasonReference == null) 1988 this.reasonReference = new ArrayList<Reference>(); 1989 this.reasonReference.add(t); 1990 return this; 1991 } 1992 1993 /** 1994 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1995 */ 1996 public Reference getReasonReferenceFirstRep() { 1997 if (getReasonReference().isEmpty()) { 1998 addReasonReference(); 1999 } 2000 return getReasonReference().get(0); 2001 } 2002 2003 /** 2004 * @return {@link #supportingInfo} (Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.) 2005 */ 2006 public List<Reference> getSupportingInfo() { 2007 if (this.supportingInfo == null) 2008 this.supportingInfo = new ArrayList<Reference>(); 2009 return this.supportingInfo; 2010 } 2011 2012 /** 2013 * @return Returns a reference to <code>this</code> for easy method chaining 2014 */ 2015 public ProcedureRequest setSupportingInfo(List<Reference> theSupportingInfo) { 2016 this.supportingInfo = theSupportingInfo; 2017 return this; 2018 } 2019 2020 public boolean hasSupportingInfo() { 2021 if (this.supportingInfo == null) 2022 return false; 2023 for (Reference item : this.supportingInfo) 2024 if (!item.isEmpty()) 2025 return true; 2026 return false; 2027 } 2028 2029 public Reference addSupportingInfo() { //3 2030 Reference t = new Reference(); 2031 if (this.supportingInfo == null) 2032 this.supportingInfo = new ArrayList<Reference>(); 2033 this.supportingInfo.add(t); 2034 return t; 2035 } 2036 2037 public ProcedureRequest addSupportingInfo(Reference t) { //3 2038 if (t == null) 2039 return this; 2040 if (this.supportingInfo == null) 2041 this.supportingInfo = new ArrayList<Reference>(); 2042 this.supportingInfo.add(t); 2043 return this; 2044 } 2045 2046 /** 2047 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 2048 */ 2049 public Reference getSupportingInfoFirstRep() { 2050 if (getSupportingInfo().isEmpty()) { 2051 addSupportingInfo(); 2052 } 2053 return getSupportingInfo().get(0); 2054 } 2055 2056 /** 2057 * @return {@link #specimen} (One or more specimens that the laboratory procedure will use.) 2058 */ 2059 public List<Reference> getSpecimen() { 2060 if (this.specimen == null) 2061 this.specimen = new ArrayList<Reference>(); 2062 return this.specimen; 2063 } 2064 2065 /** 2066 * @return Returns a reference to <code>this</code> for easy method chaining 2067 */ 2068 public ProcedureRequest setSpecimen(List<Reference> theSpecimen) { 2069 this.specimen = theSpecimen; 2070 return this; 2071 } 2072 2073 public boolean hasSpecimen() { 2074 if (this.specimen == null) 2075 return false; 2076 for (Reference item : this.specimen) 2077 if (!item.isEmpty()) 2078 return true; 2079 return false; 2080 } 2081 2082 public Reference addSpecimen() { //3 2083 Reference t = new Reference(); 2084 if (this.specimen == null) 2085 this.specimen = new ArrayList<Reference>(); 2086 this.specimen.add(t); 2087 return t; 2088 } 2089 2090 public ProcedureRequest addSpecimen(Reference t) { //3 2091 if (t == null) 2092 return this; 2093 if (this.specimen == null) 2094 this.specimen = new ArrayList<Reference>(); 2095 this.specimen.add(t); 2096 return this; 2097 } 2098 2099 /** 2100 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist 2101 */ 2102 public Reference getSpecimenFirstRep() { 2103 if (getSpecimen().isEmpty()) { 2104 addSpecimen(); 2105 } 2106 return getSpecimen().get(0); 2107 } 2108 2109 /** 2110 * @return {@link #bodySite} (Anatomic location where the procedure should be performed. This is the target site.) 2111 */ 2112 public List<CodeableConcept> getBodySite() { 2113 if (this.bodySite == null) 2114 this.bodySite = new ArrayList<CodeableConcept>(); 2115 return this.bodySite; 2116 } 2117 2118 /** 2119 * @return Returns a reference to <code>this</code> for easy method chaining 2120 */ 2121 public ProcedureRequest setBodySite(List<CodeableConcept> theBodySite) { 2122 this.bodySite = theBodySite; 2123 return this; 2124 } 2125 2126 public boolean hasBodySite() { 2127 if (this.bodySite == null) 2128 return false; 2129 for (CodeableConcept item : this.bodySite) 2130 if (!item.isEmpty()) 2131 return true; 2132 return false; 2133 } 2134 2135 public CodeableConcept addBodySite() { //3 2136 CodeableConcept t = new CodeableConcept(); 2137 if (this.bodySite == null) 2138 this.bodySite = new ArrayList<CodeableConcept>(); 2139 this.bodySite.add(t); 2140 return t; 2141 } 2142 2143 public ProcedureRequest addBodySite(CodeableConcept t) { //3 2144 if (t == null) 2145 return this; 2146 if (this.bodySite == null) 2147 this.bodySite = new ArrayList<CodeableConcept>(); 2148 this.bodySite.add(t); 2149 return this; 2150 } 2151 2152 /** 2153 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist 2154 */ 2155 public CodeableConcept getBodySiteFirstRep() { 2156 if (getBodySite().isEmpty()) { 2157 addBodySite(); 2158 } 2159 return getBodySite().get(0); 2160 } 2161 2162 /** 2163 * @return {@link #note} (Any other notes and comments made about the service request. For example, letting provider know that "patient hates needles" or other provider instructions.) 2164 */ 2165 public List<Annotation> getNote() { 2166 if (this.note == null) 2167 this.note = new ArrayList<Annotation>(); 2168 return this.note; 2169 } 2170 2171 /** 2172 * @return Returns a reference to <code>this</code> for easy method chaining 2173 */ 2174 public ProcedureRequest setNote(List<Annotation> theNote) { 2175 this.note = theNote; 2176 return this; 2177 } 2178 2179 public boolean hasNote() { 2180 if (this.note == null) 2181 return false; 2182 for (Annotation item : this.note) 2183 if (!item.isEmpty()) 2184 return true; 2185 return false; 2186 } 2187 2188 public Annotation addNote() { //3 2189 Annotation t = new Annotation(); 2190 if (this.note == null) 2191 this.note = new ArrayList<Annotation>(); 2192 this.note.add(t); 2193 return t; 2194 } 2195 2196 public ProcedureRequest addNote(Annotation t) { //3 2197 if (t == null) 2198 return this; 2199 if (this.note == null) 2200 this.note = new ArrayList<Annotation>(); 2201 this.note.add(t); 2202 return this; 2203 } 2204 2205 /** 2206 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2207 */ 2208 public Annotation getNoteFirstRep() { 2209 if (getNote().isEmpty()) { 2210 addNote(); 2211 } 2212 return getNote().get(0); 2213 } 2214 2215 /** 2216 * @return {@link #relevantHistory} (Key events in the history of the request.) 2217 */ 2218 public List<Reference> getRelevantHistory() { 2219 if (this.relevantHistory == null) 2220 this.relevantHistory = new ArrayList<Reference>(); 2221 return this.relevantHistory; 2222 } 2223 2224 /** 2225 * @return Returns a reference to <code>this</code> for easy method chaining 2226 */ 2227 public ProcedureRequest setRelevantHistory(List<Reference> theRelevantHistory) { 2228 this.relevantHistory = theRelevantHistory; 2229 return this; 2230 } 2231 2232 public boolean hasRelevantHistory() { 2233 if (this.relevantHistory == null) 2234 return false; 2235 for (Reference item : this.relevantHistory) 2236 if (!item.isEmpty()) 2237 return true; 2238 return false; 2239 } 2240 2241 public Reference addRelevantHistory() { //3 2242 Reference t = new Reference(); 2243 if (this.relevantHistory == null) 2244 this.relevantHistory = new ArrayList<Reference>(); 2245 this.relevantHistory.add(t); 2246 return t; 2247 } 2248 2249 public ProcedureRequest addRelevantHistory(Reference t) { //3 2250 if (t == null) 2251 return this; 2252 if (this.relevantHistory == null) 2253 this.relevantHistory = new ArrayList<Reference>(); 2254 this.relevantHistory.add(t); 2255 return this; 2256 } 2257 2258 /** 2259 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 2260 */ 2261 public Reference getRelevantHistoryFirstRep() { 2262 if (getRelevantHistory().isEmpty()) { 2263 addRelevantHistory(); 2264 } 2265 return getRelevantHistory().get(0); 2266 } 2267 2268 protected void listChildren(List<Property> children) { 2269 super.listChildren(children); 2270 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2271 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 2272 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2273 children.add(new Property("replaces", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces)); 2274 children.add(new Property("requisition", "Identifier", "A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition)); 2275 children.add(new Property("status", "code", "The status of the order.", 0, 1, status)); 2276 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 2277 children.add(new Property("priority", "code", "Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.", 0, 1, priority)); 2278 children.add(new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the procedure should NOT be performed.", 0, 1, doNotPerform)); 2279 children.add(new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category)); 2280 children.add(new Property("code", "CodeableConcept", "A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.", 0, 1, code)); 2281 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject)); 2282 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter or episode of care that provides additional information about the healthcare context in which this request is made.", 0, 1, context)); 2283 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence)); 2284 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded)); 2285 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 2286 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2287 children.add(new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType)); 2288 children.add(new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, 1, performer)); 2289 children.add(new Property("reasonCode", "CodeableConcept", "An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2290 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2291 children.add(new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2292 children.add(new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen)); 2293 children.add(new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2294 children.add(new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions.", 0, java.lang.Integer.MAX_VALUE, note)); 2295 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 2296 } 2297 2298 @Override 2299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2300 switch (_hash) { 2301 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier); 2302 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 2303 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2304 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Any)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces); 2305 case 395923612: /*requisition*/ return new Property("requisition", "Identifier", "A shared identifier common to all procedure or diagnostic requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition); 2306 case -892481550: /*status*/ return new Property("status", "code", "The status of the order.", 0, 1, status); 2307 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 2308 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the ProcedureRequest should be addressed with respect to other requests.", 0, 1, priority); 2309 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the procedure should NOT be performed.", 0, 1, doNotPerform); 2310 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category); 2311 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies a particular procedure, diagnostic investigation, or panel of investigations, that have been requested.", 0, 1, code); 2312 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the procedure or diagnostic is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject); 2313 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "An encounter or episode of care that provides additional information about the healthcare context in which this request is made.", 0, 1, context); 2314 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2315 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2316 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2317 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2318 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the diagnostic testing should occur.", 0, 1, occurrence); 2319 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2320 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2321 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2322 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2323 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 2324 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2325 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType); 2326 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson|HealthcareService)", "The desired perfomer for doing the diagnostic testing. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, 1, performer); 2327 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "An explanation or justification for why this diagnostic investigation is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2328 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource that provides a justification for why this diagnostic investigation is being requested. May relate to the resources referred to in supportingInformation.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2329 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient or specimen that may influence the procedure or diagnostics or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2330 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen); 2331 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2332 case 3387378: /*note*/ return new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, letting provider know that \"patient hates needles\" or other provider instructions.", 0, java.lang.Integer.MAX_VALUE, note); 2333 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 2334 default: return super.getNamedProperty(_hash, _name, _checkValid); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2341 switch (hash) { 2342 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2343 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2344 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2345 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2346 case 395923612: /*requisition*/ return this.requisition == null ? new Base[0] : new Base[] {this.requisition}; // Identifier 2347 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ProcedureRequestStatus> 2348 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<ProcedureRequestIntent> 2349 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<ProcedureRequestPriority> 2350 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 2351 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2352 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2353 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2354 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2355 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 2356 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // Type 2357 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2358 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // ProcedureRequestRequesterComponent 2359 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 2360 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 2361 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2362 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2363 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2364 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 2365 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2366 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2367 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 2368 default: return super.getProperty(hash, name, checkValid); 2369 } 2370 2371 } 2372 2373 @Override 2374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2375 switch (hash) { 2376 case -1618432855: // identifier 2377 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2378 return value; 2379 case -1014418093: // definition 2380 this.getDefinition().add(castToReference(value)); // Reference 2381 return value; 2382 case -332612366: // basedOn 2383 this.getBasedOn().add(castToReference(value)); // Reference 2384 return value; 2385 case -430332865: // replaces 2386 this.getReplaces().add(castToReference(value)); // Reference 2387 return value; 2388 case 395923612: // requisition 2389 this.requisition = castToIdentifier(value); // Identifier 2390 return value; 2391 case -892481550: // status 2392 value = new ProcedureRequestStatusEnumFactory().fromType(castToCode(value)); 2393 this.status = (Enumeration) value; // Enumeration<ProcedureRequestStatus> 2394 return value; 2395 case -1183762788: // intent 2396 value = new ProcedureRequestIntentEnumFactory().fromType(castToCode(value)); 2397 this.intent = (Enumeration) value; // Enumeration<ProcedureRequestIntent> 2398 return value; 2399 case -1165461084: // priority 2400 value = new ProcedureRequestPriorityEnumFactory().fromType(castToCode(value)); 2401 this.priority = (Enumeration) value; // Enumeration<ProcedureRequestPriority> 2402 return value; 2403 case -1788508167: // doNotPerform 2404 this.doNotPerform = castToBoolean(value); // BooleanType 2405 return value; 2406 case 50511102: // category 2407 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2408 return value; 2409 case 3059181: // code 2410 this.code = castToCodeableConcept(value); // CodeableConcept 2411 return value; 2412 case -1867885268: // subject 2413 this.subject = castToReference(value); // Reference 2414 return value; 2415 case 951530927: // context 2416 this.context = castToReference(value); // Reference 2417 return value; 2418 case 1687874001: // occurrence 2419 this.occurrence = castToType(value); // Type 2420 return value; 2421 case -1432923513: // asNeeded 2422 this.asNeeded = castToType(value); // Type 2423 return value; 2424 case -1500852503: // authoredOn 2425 this.authoredOn = castToDateTime(value); // DateTimeType 2426 return value; 2427 case 693933948: // requester 2428 this.requester = (ProcedureRequestRequesterComponent) value; // ProcedureRequestRequesterComponent 2429 return value; 2430 case -901444568: // performerType 2431 this.performerType = castToCodeableConcept(value); // CodeableConcept 2432 return value; 2433 case 481140686: // performer 2434 this.performer = castToReference(value); // Reference 2435 return value; 2436 case 722137681: // reasonCode 2437 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2438 return value; 2439 case -1146218137: // reasonReference 2440 this.getReasonReference().add(castToReference(value)); // Reference 2441 return value; 2442 case 1922406657: // supportingInfo 2443 this.getSupportingInfo().add(castToReference(value)); // Reference 2444 return value; 2445 case -2132868344: // specimen 2446 this.getSpecimen().add(castToReference(value)); // Reference 2447 return value; 2448 case 1702620169: // bodySite 2449 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 2450 return value; 2451 case 3387378: // note 2452 this.getNote().add(castToAnnotation(value)); // Annotation 2453 return value; 2454 case 1538891575: // relevantHistory 2455 this.getRelevantHistory().add(castToReference(value)); // Reference 2456 return value; 2457 default: return super.setProperty(hash, name, value); 2458 } 2459 2460 } 2461 2462 @Override 2463 public Base setProperty(String name, Base value) throws FHIRException { 2464 if (name.equals("identifier")) { 2465 this.getIdentifier().add(castToIdentifier(value)); 2466 } else if (name.equals("definition")) { 2467 this.getDefinition().add(castToReference(value)); 2468 } else if (name.equals("basedOn")) { 2469 this.getBasedOn().add(castToReference(value)); 2470 } else if (name.equals("replaces")) { 2471 this.getReplaces().add(castToReference(value)); 2472 } else if (name.equals("requisition")) { 2473 this.requisition = castToIdentifier(value); // Identifier 2474 } else if (name.equals("status")) { 2475 value = new ProcedureRequestStatusEnumFactory().fromType(castToCode(value)); 2476 this.status = (Enumeration) value; // Enumeration<ProcedureRequestStatus> 2477 } else if (name.equals("intent")) { 2478 value = new ProcedureRequestIntentEnumFactory().fromType(castToCode(value)); 2479 this.intent = (Enumeration) value; // Enumeration<ProcedureRequestIntent> 2480 } else if (name.equals("priority")) { 2481 value = new ProcedureRequestPriorityEnumFactory().fromType(castToCode(value)); 2482 this.priority = (Enumeration) value; // Enumeration<ProcedureRequestPriority> 2483 } else if (name.equals("doNotPerform")) { 2484 this.doNotPerform = castToBoolean(value); // BooleanType 2485 } else if (name.equals("category")) { 2486 this.getCategory().add(castToCodeableConcept(value)); 2487 } else if (name.equals("code")) { 2488 this.code = castToCodeableConcept(value); // CodeableConcept 2489 } else if (name.equals("subject")) { 2490 this.subject = castToReference(value); // Reference 2491 } else if (name.equals("context")) { 2492 this.context = castToReference(value); // Reference 2493 } else if (name.equals("occurrence[x]")) { 2494 this.occurrence = castToType(value); // Type 2495 } else if (name.equals("asNeeded[x]")) { 2496 this.asNeeded = castToType(value); // Type 2497 } else if (name.equals("authoredOn")) { 2498 this.authoredOn = castToDateTime(value); // DateTimeType 2499 } else if (name.equals("requester")) { 2500 this.requester = (ProcedureRequestRequesterComponent) value; // ProcedureRequestRequesterComponent 2501 } else if (name.equals("performerType")) { 2502 this.performerType = castToCodeableConcept(value); // CodeableConcept 2503 } else if (name.equals("performer")) { 2504 this.performer = castToReference(value); // Reference 2505 } else if (name.equals("reasonCode")) { 2506 this.getReasonCode().add(castToCodeableConcept(value)); 2507 } else if (name.equals("reasonReference")) { 2508 this.getReasonReference().add(castToReference(value)); 2509 } else if (name.equals("supportingInfo")) { 2510 this.getSupportingInfo().add(castToReference(value)); 2511 } else if (name.equals("specimen")) { 2512 this.getSpecimen().add(castToReference(value)); 2513 } else if (name.equals("bodySite")) { 2514 this.getBodySite().add(castToCodeableConcept(value)); 2515 } else if (name.equals("note")) { 2516 this.getNote().add(castToAnnotation(value)); 2517 } else if (name.equals("relevantHistory")) { 2518 this.getRelevantHistory().add(castToReference(value)); 2519 } else 2520 return super.setProperty(name, value); 2521 return value; 2522 } 2523 2524 @Override 2525 public Base makeProperty(int hash, String name) throws FHIRException { 2526 switch (hash) { 2527 case -1618432855: return addIdentifier(); 2528 case -1014418093: return addDefinition(); 2529 case -332612366: return addBasedOn(); 2530 case -430332865: return addReplaces(); 2531 case 395923612: return getRequisition(); 2532 case -892481550: return getStatusElement(); 2533 case -1183762788: return getIntentElement(); 2534 case -1165461084: return getPriorityElement(); 2535 case -1788508167: return getDoNotPerformElement(); 2536 case 50511102: return addCategory(); 2537 case 3059181: return getCode(); 2538 case -1867885268: return getSubject(); 2539 case 951530927: return getContext(); 2540 case -2022646513: return getOccurrence(); 2541 case 1687874001: return getOccurrence(); 2542 case -544329575: return getAsNeeded(); 2543 case -1432923513: return getAsNeeded(); 2544 case -1500852503: return getAuthoredOnElement(); 2545 case 693933948: return getRequester(); 2546 case -901444568: return getPerformerType(); 2547 case 481140686: return getPerformer(); 2548 case 722137681: return addReasonCode(); 2549 case -1146218137: return addReasonReference(); 2550 case 1922406657: return addSupportingInfo(); 2551 case -2132868344: return addSpecimen(); 2552 case 1702620169: return addBodySite(); 2553 case 3387378: return addNote(); 2554 case 1538891575: return addRelevantHistory(); 2555 default: return super.makeProperty(hash, name); 2556 } 2557 2558 } 2559 2560 @Override 2561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2562 switch (hash) { 2563 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2564 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2565 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2566 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2567 case 395923612: /*requisition*/ return new String[] {"Identifier"}; 2568 case -892481550: /*status*/ return new String[] {"code"}; 2569 case -1183762788: /*intent*/ return new String[] {"code"}; 2570 case -1165461084: /*priority*/ return new String[] {"code"}; 2571 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 2572 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2573 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2574 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2575 case 951530927: /*context*/ return new String[] {"Reference"}; 2576 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2577 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 2578 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2579 case 693933948: /*requester*/ return new String[] {}; 2580 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 2581 case 481140686: /*performer*/ return new String[] {"Reference"}; 2582 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2583 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2584 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2585 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2586 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 2587 case 3387378: /*note*/ return new String[] {"Annotation"}; 2588 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2589 default: return super.getTypesForProperty(hash, name); 2590 } 2591 2592 } 2593 2594 @Override 2595 public Base addChild(String name) throws FHIRException { 2596 if (name.equals("identifier")) { 2597 return addIdentifier(); 2598 } 2599 else if (name.equals("definition")) { 2600 return addDefinition(); 2601 } 2602 else if (name.equals("basedOn")) { 2603 return addBasedOn(); 2604 } 2605 else if (name.equals("replaces")) { 2606 return addReplaces(); 2607 } 2608 else if (name.equals("requisition")) { 2609 this.requisition = new Identifier(); 2610 return this.requisition; 2611 } 2612 else if (name.equals("status")) { 2613 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.status"); 2614 } 2615 else if (name.equals("intent")) { 2616 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.intent"); 2617 } 2618 else if (name.equals("priority")) { 2619 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.priority"); 2620 } 2621 else if (name.equals("doNotPerform")) { 2622 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.doNotPerform"); 2623 } 2624 else if (name.equals("category")) { 2625 return addCategory(); 2626 } 2627 else if (name.equals("code")) { 2628 this.code = new CodeableConcept(); 2629 return this.code; 2630 } 2631 else if (name.equals("subject")) { 2632 this.subject = new Reference(); 2633 return this.subject; 2634 } 2635 else if (name.equals("context")) { 2636 this.context = new Reference(); 2637 return this.context; 2638 } 2639 else if (name.equals("occurrenceDateTime")) { 2640 this.occurrence = new DateTimeType(); 2641 return this.occurrence; 2642 } 2643 else if (name.equals("occurrencePeriod")) { 2644 this.occurrence = new Period(); 2645 return this.occurrence; 2646 } 2647 else if (name.equals("occurrenceTiming")) { 2648 this.occurrence = new Timing(); 2649 return this.occurrence; 2650 } 2651 else if (name.equals("asNeededBoolean")) { 2652 this.asNeeded = new BooleanType(); 2653 return this.asNeeded; 2654 } 2655 else if (name.equals("asNeededCodeableConcept")) { 2656 this.asNeeded = new CodeableConcept(); 2657 return this.asNeeded; 2658 } 2659 else if (name.equals("authoredOn")) { 2660 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.authoredOn"); 2661 } 2662 else if (name.equals("requester")) { 2663 this.requester = new ProcedureRequestRequesterComponent(); 2664 return this.requester; 2665 } 2666 else if (name.equals("performerType")) { 2667 this.performerType = new CodeableConcept(); 2668 return this.performerType; 2669 } 2670 else if (name.equals("performer")) { 2671 this.performer = new Reference(); 2672 return this.performer; 2673 } 2674 else if (name.equals("reasonCode")) { 2675 return addReasonCode(); 2676 } 2677 else if (name.equals("reasonReference")) { 2678 return addReasonReference(); 2679 } 2680 else if (name.equals("supportingInfo")) { 2681 return addSupportingInfo(); 2682 } 2683 else if (name.equals("specimen")) { 2684 return addSpecimen(); 2685 } 2686 else if (name.equals("bodySite")) { 2687 return addBodySite(); 2688 } 2689 else if (name.equals("note")) { 2690 return addNote(); 2691 } 2692 else if (name.equals("relevantHistory")) { 2693 return addRelevantHistory(); 2694 } 2695 else 2696 return super.addChild(name); 2697 } 2698 2699 public String fhirType() { 2700 return "ProcedureRequest"; 2701 2702 } 2703 2704 public ProcedureRequest copy() { 2705 ProcedureRequest dst = new ProcedureRequest(); 2706 copyValues(dst); 2707 if (identifier != null) { 2708 dst.identifier = new ArrayList<Identifier>(); 2709 for (Identifier i : identifier) 2710 dst.identifier.add(i.copy()); 2711 }; 2712 if (definition != null) { 2713 dst.definition = new ArrayList<Reference>(); 2714 for (Reference i : definition) 2715 dst.definition.add(i.copy()); 2716 }; 2717 if (basedOn != null) { 2718 dst.basedOn = new ArrayList<Reference>(); 2719 for (Reference i : basedOn) 2720 dst.basedOn.add(i.copy()); 2721 }; 2722 if (replaces != null) { 2723 dst.replaces = new ArrayList<Reference>(); 2724 for (Reference i : replaces) 2725 dst.replaces.add(i.copy()); 2726 }; 2727 dst.requisition = requisition == null ? null : requisition.copy(); 2728 dst.status = status == null ? null : status.copy(); 2729 dst.intent = intent == null ? null : intent.copy(); 2730 dst.priority = priority == null ? null : priority.copy(); 2731 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2732 if (category != null) { 2733 dst.category = new ArrayList<CodeableConcept>(); 2734 for (CodeableConcept i : category) 2735 dst.category.add(i.copy()); 2736 }; 2737 dst.code = code == null ? null : code.copy(); 2738 dst.subject = subject == null ? null : subject.copy(); 2739 dst.context = context == null ? null : context.copy(); 2740 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2741 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 2742 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2743 dst.requester = requester == null ? null : requester.copy(); 2744 dst.performerType = performerType == null ? null : performerType.copy(); 2745 dst.performer = performer == null ? null : performer.copy(); 2746 if (reasonCode != null) { 2747 dst.reasonCode = new ArrayList<CodeableConcept>(); 2748 for (CodeableConcept i : reasonCode) 2749 dst.reasonCode.add(i.copy()); 2750 }; 2751 if (reasonReference != null) { 2752 dst.reasonReference = new ArrayList<Reference>(); 2753 for (Reference i : reasonReference) 2754 dst.reasonReference.add(i.copy()); 2755 }; 2756 if (supportingInfo != null) { 2757 dst.supportingInfo = new ArrayList<Reference>(); 2758 for (Reference i : supportingInfo) 2759 dst.supportingInfo.add(i.copy()); 2760 }; 2761 if (specimen != null) { 2762 dst.specimen = new ArrayList<Reference>(); 2763 for (Reference i : specimen) 2764 dst.specimen.add(i.copy()); 2765 }; 2766 if (bodySite != null) { 2767 dst.bodySite = new ArrayList<CodeableConcept>(); 2768 for (CodeableConcept i : bodySite) 2769 dst.bodySite.add(i.copy()); 2770 }; 2771 if (note != null) { 2772 dst.note = new ArrayList<Annotation>(); 2773 for (Annotation i : note) 2774 dst.note.add(i.copy()); 2775 }; 2776 if (relevantHistory != null) { 2777 dst.relevantHistory = new ArrayList<Reference>(); 2778 for (Reference i : relevantHistory) 2779 dst.relevantHistory.add(i.copy()); 2780 }; 2781 return dst; 2782 } 2783 2784 protected ProcedureRequest typedCopy() { 2785 return copy(); 2786 } 2787 2788 @Override 2789 public boolean equalsDeep(Base other_) { 2790 if (!super.equalsDeep(other_)) 2791 return false; 2792 if (!(other_ instanceof ProcedureRequest)) 2793 return false; 2794 ProcedureRequest o = (ProcedureRequest) other_; 2795 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2796 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(requisition, o.requisition, true) 2797 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 2798 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 2799 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) 2800 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2801 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 2802 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2803 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(specimen, o.specimen, true) 2804 && compareDeep(bodySite, o.bodySite, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 2805 ; 2806 } 2807 2808 @Override 2809 public boolean equalsShallow(Base other_) { 2810 if (!super.equalsShallow(other_)) 2811 return false; 2812 if (!(other_ instanceof ProcedureRequest)) 2813 return false; 2814 ProcedureRequest o = (ProcedureRequest) other_; 2815 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 2816 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true) 2817 ; 2818 } 2819 2820 public boolean isEmpty() { 2821 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2822 , replaces, requisition, status, intent, priority, doNotPerform, category, code 2823 , subject, context, occurrence, asNeeded, authoredOn, requester, performerType 2824 , performer, reasonCode, reasonReference, supportingInfo, specimen, bodySite, note 2825 , relevantHistory); 2826 } 2827 2828 @Override 2829 public ResourceType getResourceType() { 2830 return ResourceType.ProcedureRequest; 2831 } 2832 2833 /** 2834 * Search parameter: <b>authored</b> 2835 * <p> 2836 * Description: <b>Date request signed</b><br> 2837 * Type: <b>date</b><br> 2838 * Path: <b>ProcedureRequest.authoredOn</b><br> 2839 * </p> 2840 */ 2841 @SearchParamDefinition(name="authored", path="ProcedureRequest.authoredOn", description="Date request signed", type="date" ) 2842 public static final String SP_AUTHORED = "authored"; 2843 /** 2844 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2845 * <p> 2846 * Description: <b>Date request signed</b><br> 2847 * Type: <b>date</b><br> 2848 * Path: <b>ProcedureRequest.authoredOn</b><br> 2849 * </p> 2850 */ 2851 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2852 2853 /** 2854 * Search parameter: <b>requester</b> 2855 * <p> 2856 * Description: <b>Individual making the request</b><br> 2857 * Type: <b>reference</b><br> 2858 * Path: <b>ProcedureRequest.requester.agent</b><br> 2859 * </p> 2860 */ 2861 @SearchParamDefinition(name="requester", path="ProcedureRequest.requester.agent", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Practitioner.class } ) 2862 public static final String SP_REQUESTER = "requester"; 2863 /** 2864 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2865 * <p> 2866 * Description: <b>Individual making the request</b><br> 2867 * Type: <b>reference</b><br> 2868 * Path: <b>ProcedureRequest.requester.agent</b><br> 2869 * </p> 2870 */ 2871 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2872 2873/** 2874 * Constant for fluent queries to be used to add include statements. Specifies 2875 * the path value of "<b>ProcedureRequest:requester</b>". 2876 */ 2877 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:requester").toLocked(); 2878 2879 /** 2880 * Search parameter: <b>identifier</b> 2881 * <p> 2882 * Description: <b>Identifiers assigned to this order</b><br> 2883 * Type: <b>token</b><br> 2884 * Path: <b>ProcedureRequest.identifier</b><br> 2885 * </p> 2886 */ 2887 @SearchParamDefinition(name="identifier", path="ProcedureRequest.identifier", description="Identifiers assigned to this order", type="token" ) 2888 public static final String SP_IDENTIFIER = "identifier"; 2889 /** 2890 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2891 * <p> 2892 * Description: <b>Identifiers assigned to this order</b><br> 2893 * Type: <b>token</b><br> 2894 * Path: <b>ProcedureRequest.identifier</b><br> 2895 * </p> 2896 */ 2897 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2898 2899 /** 2900 * Search parameter: <b>code</b> 2901 * <p> 2902 * Description: <b>What is being requested/ordered</b><br> 2903 * Type: <b>token</b><br> 2904 * Path: <b>ProcedureRequest.code</b><br> 2905 * </p> 2906 */ 2907 @SearchParamDefinition(name="code", path="ProcedureRequest.code", description="What is being requested/ordered", type="token" ) 2908 public static final String SP_CODE = "code"; 2909 /** 2910 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2911 * <p> 2912 * Description: <b>What is being requested/ordered</b><br> 2913 * Type: <b>token</b><br> 2914 * Path: <b>ProcedureRequest.code</b><br> 2915 * </p> 2916 */ 2917 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2918 2919 /** 2920 * Search parameter: <b>performer</b> 2921 * <p> 2922 * Description: <b>Requested perfomer</b><br> 2923 * Type: <b>reference</b><br> 2924 * Path: <b>ProcedureRequest.performer</b><br> 2925 * </p> 2926 */ 2927 @SearchParamDefinition(name="performer", path="ProcedureRequest.performer", description="Requested perfomer", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2928 public static final String SP_PERFORMER = "performer"; 2929 /** 2930 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2931 * <p> 2932 * Description: <b>Requested perfomer</b><br> 2933 * Type: <b>reference</b><br> 2934 * Path: <b>ProcedureRequest.performer</b><br> 2935 * </p> 2936 */ 2937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2938 2939/** 2940 * Constant for fluent queries to be used to add include statements. Specifies 2941 * the path value of "<b>ProcedureRequest:performer</b>". 2942 */ 2943 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:performer").toLocked(); 2944 2945 /** 2946 * Search parameter: <b>requisition</b> 2947 * <p> 2948 * Description: <b>Composite Request ID</b><br> 2949 * Type: <b>token</b><br> 2950 * Path: <b>ProcedureRequest.requisition</b><br> 2951 * </p> 2952 */ 2953 @SearchParamDefinition(name="requisition", path="ProcedureRequest.requisition", description="Composite Request ID", type="token" ) 2954 public static final String SP_REQUISITION = "requisition"; 2955 /** 2956 * <b>Fluent Client</b> search parameter constant for <b>requisition</b> 2957 * <p> 2958 * Description: <b>Composite Request ID</b><br> 2959 * Type: <b>token</b><br> 2960 * Path: <b>ProcedureRequest.requisition</b><br> 2961 * </p> 2962 */ 2963 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUISITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REQUISITION); 2964 2965 /** 2966 * Search parameter: <b>replaces</b> 2967 * <p> 2968 * Description: <b>What request replaces</b><br> 2969 * Type: <b>reference</b><br> 2970 * Path: <b>ProcedureRequest.replaces</b><br> 2971 * </p> 2972 */ 2973 @SearchParamDefinition(name="replaces", path="ProcedureRequest.replaces", description="What request replaces", type="reference" ) 2974 public static final String SP_REPLACES = "replaces"; 2975 /** 2976 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2977 * <p> 2978 * Description: <b>What request replaces</b><br> 2979 * Type: <b>reference</b><br> 2980 * Path: <b>ProcedureRequest.replaces</b><br> 2981 * </p> 2982 */ 2983 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 2984 2985/** 2986 * Constant for fluent queries to be used to add include statements. Specifies 2987 * the path value of "<b>ProcedureRequest:replaces</b>". 2988 */ 2989 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("ProcedureRequest:replaces").toLocked(); 2990 2991 /** 2992 * Search parameter: <b>subject</b> 2993 * <p> 2994 * Description: <b>Search by subject</b><br> 2995 * Type: <b>reference</b><br> 2996 * Path: <b>ProcedureRequest.subject</b><br> 2997 * </p> 2998 */ 2999 @SearchParamDefinition(name="subject", path="ProcedureRequest.subject", description="Search by subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 3000 public static final String SP_SUBJECT = "subject"; 3001 /** 3002 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3003 * <p> 3004 * Description: <b>Search by subject</b><br> 3005 * Type: <b>reference</b><br> 3006 * Path: <b>ProcedureRequest.subject</b><br> 3007 * </p> 3008 */ 3009 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3010 3011/** 3012 * Constant for fluent queries to be used to add include statements. Specifies 3013 * the path value of "<b>ProcedureRequest:subject</b>". 3014 */ 3015 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:subject").toLocked(); 3016 3017 /** 3018 * Search parameter: <b>encounter</b> 3019 * <p> 3020 * Description: <b>An encounter in which this request is made</b><br> 3021 * Type: <b>reference</b><br> 3022 * Path: <b>ProcedureRequest.context</b><br> 3023 * </p> 3024 */ 3025 @SearchParamDefinition(name="encounter", path="ProcedureRequest.context", description="An encounter in which this request is made", type="reference", target={Encounter.class } ) 3026 public static final String SP_ENCOUNTER = "encounter"; 3027 /** 3028 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3029 * <p> 3030 * Description: <b>An encounter in which this request is made</b><br> 3031 * Type: <b>reference</b><br> 3032 * Path: <b>ProcedureRequest.context</b><br> 3033 * </p> 3034 */ 3035 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3036 3037/** 3038 * Constant for fluent queries to be used to add include statements. Specifies 3039 * the path value of "<b>ProcedureRequest:encounter</b>". 3040 */ 3041 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ProcedureRequest:encounter").toLocked(); 3042 3043 /** 3044 * Search parameter: <b>occurrence</b> 3045 * <p> 3046 * Description: <b>When procedure should occur</b><br> 3047 * Type: <b>date</b><br> 3048 * Path: <b>ProcedureRequest.occurrence[x]</b><br> 3049 * </p> 3050 */ 3051 @SearchParamDefinition(name="occurrence", path="ProcedureRequest.occurrence", description="When procedure should occur", type="date" ) 3052 public static final String SP_OCCURRENCE = "occurrence"; 3053 /** 3054 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3055 * <p> 3056 * Description: <b>When procedure should occur</b><br> 3057 * Type: <b>date</b><br> 3058 * Path: <b>ProcedureRequest.occurrence[x]</b><br> 3059 * </p> 3060 */ 3061 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 3062 3063 /** 3064 * Search parameter: <b>priority</b> 3065 * <p> 3066 * Description: <b>routine | urgent | asap | stat</b><br> 3067 * Type: <b>token</b><br> 3068 * Path: <b>ProcedureRequest.priority</b><br> 3069 * </p> 3070 */ 3071 @SearchParamDefinition(name="priority", path="ProcedureRequest.priority", description="routine | urgent | asap | stat", type="token" ) 3072 public static final String SP_PRIORITY = "priority"; 3073 /** 3074 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3075 * <p> 3076 * Description: <b>routine | urgent | asap | stat</b><br> 3077 * Type: <b>token</b><br> 3078 * Path: <b>ProcedureRequest.priority</b><br> 3079 * </p> 3080 */ 3081 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 3082 3083 /** 3084 * Search parameter: <b>intent</b> 3085 * <p> 3086 * Description: <b>proposal | plan | order +</b><br> 3087 * Type: <b>token</b><br> 3088 * Path: <b>ProcedureRequest.intent</b><br> 3089 * </p> 3090 */ 3091 @SearchParamDefinition(name="intent", path="ProcedureRequest.intent", description="proposal | plan | order +", type="token" ) 3092 public static final String SP_INTENT = "intent"; 3093 /** 3094 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 3095 * <p> 3096 * Description: <b>proposal | plan | order +</b><br> 3097 * Type: <b>token</b><br> 3098 * Path: <b>ProcedureRequest.intent</b><br> 3099 * </p> 3100 */ 3101 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 3102 3103 /** 3104 * Search parameter: <b>performer-type</b> 3105 * <p> 3106 * Description: <b>Performer role</b><br> 3107 * Type: <b>token</b><br> 3108 * Path: <b>ProcedureRequest.performerType</b><br> 3109 * </p> 3110 */ 3111 @SearchParamDefinition(name="performer-type", path="ProcedureRequest.performerType", description="Performer role", type="token" ) 3112 public static final String SP_PERFORMER_TYPE = "performer-type"; 3113 /** 3114 * <b>Fluent Client</b> search parameter constant for <b>performer-type</b> 3115 * <p> 3116 * Description: <b>Performer role</b><br> 3117 * Type: <b>token</b><br> 3118 * Path: <b>ProcedureRequest.performerType</b><br> 3119 * </p> 3120 */ 3121 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_TYPE); 3122 3123 /** 3124 * Search parameter: <b>based-on</b> 3125 * <p> 3126 * Description: <b>What request fulfills</b><br> 3127 * Type: <b>reference</b><br> 3128 * Path: <b>ProcedureRequest.basedOn</b><br> 3129 * </p> 3130 */ 3131 @SearchParamDefinition(name="based-on", path="ProcedureRequest.basedOn", description="What request fulfills", type="reference" ) 3132 public static final String SP_BASED_ON = "based-on"; 3133 /** 3134 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3135 * <p> 3136 * Description: <b>What request fulfills</b><br> 3137 * Type: <b>reference</b><br> 3138 * Path: <b>ProcedureRequest.basedOn</b><br> 3139 * </p> 3140 */ 3141 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3142 3143/** 3144 * Constant for fluent queries to be used to add include statements. Specifies 3145 * the path value of "<b>ProcedureRequest:based-on</b>". 3146 */ 3147 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ProcedureRequest:based-on").toLocked(); 3148 3149 /** 3150 * Search parameter: <b>patient</b> 3151 * <p> 3152 * Description: <b>Search by subject - a patient</b><br> 3153 * Type: <b>reference</b><br> 3154 * Path: <b>ProcedureRequest.subject</b><br> 3155 * </p> 3156 */ 3157 @SearchParamDefinition(name="patient", path="ProcedureRequest.subject", description="Search by subject - a patient", type="reference", target={Patient.class } ) 3158 public static final String SP_PATIENT = "patient"; 3159 /** 3160 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3161 * <p> 3162 * Description: <b>Search by subject - a patient</b><br> 3163 * Type: <b>reference</b><br> 3164 * Path: <b>ProcedureRequest.subject</b><br> 3165 * </p> 3166 */ 3167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3168 3169/** 3170 * Constant for fluent queries to be used to add include statements. Specifies 3171 * the path value of "<b>ProcedureRequest:patient</b>". 3172 */ 3173 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:patient").toLocked(); 3174 3175 /** 3176 * Search parameter: <b>specimen</b> 3177 * <p> 3178 * Description: <b>Specimen to be tested</b><br> 3179 * Type: <b>reference</b><br> 3180 * Path: <b>ProcedureRequest.specimen</b><br> 3181 * </p> 3182 */ 3183 @SearchParamDefinition(name="specimen", path="ProcedureRequest.specimen", description="Specimen to be tested", type="reference", target={Specimen.class } ) 3184 public static final String SP_SPECIMEN = "specimen"; 3185 /** 3186 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 3187 * <p> 3188 * Description: <b>Specimen to be tested</b><br> 3189 * Type: <b>reference</b><br> 3190 * Path: <b>ProcedureRequest.specimen</b><br> 3191 * </p> 3192 */ 3193 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 3194 3195/** 3196 * Constant for fluent queries to be used to add include statements. Specifies 3197 * the path value of "<b>ProcedureRequest:specimen</b>". 3198 */ 3199 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("ProcedureRequest:specimen").toLocked(); 3200 3201 /** 3202 * Search parameter: <b>context</b> 3203 * <p> 3204 * Description: <b>Encounter or Episode during which request was created</b><br> 3205 * Type: <b>reference</b><br> 3206 * Path: <b>ProcedureRequest.context</b><br> 3207 * </p> 3208 */ 3209 @SearchParamDefinition(name="context", path="ProcedureRequest.context", description="Encounter or Episode during which request was created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 3210 public static final String SP_CONTEXT = "context"; 3211 /** 3212 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3213 * <p> 3214 * Description: <b>Encounter or Episode during which request was created</b><br> 3215 * Type: <b>reference</b><br> 3216 * Path: <b>ProcedureRequest.context</b><br> 3217 * </p> 3218 */ 3219 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3220 3221/** 3222 * Constant for fluent queries to be used to add include statements. Specifies 3223 * the path value of "<b>ProcedureRequest:context</b>". 3224 */ 3225 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ProcedureRequest:context").toLocked(); 3226 3227 /** 3228 * Search parameter: <b>definition</b> 3229 * <p> 3230 * Description: <b>Protocol or definition</b><br> 3231 * Type: <b>reference</b><br> 3232 * Path: <b>ProcedureRequest.definition</b><br> 3233 * </p> 3234 */ 3235 @SearchParamDefinition(name="definition", path="ProcedureRequest.definition", description="Protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 3236 public static final String SP_DEFINITION = "definition"; 3237 /** 3238 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 3239 * <p> 3240 * Description: <b>Protocol or definition</b><br> 3241 * Type: <b>reference</b><br> 3242 * Path: <b>ProcedureRequest.definition</b><br> 3243 * </p> 3244 */ 3245 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 3246 3247/** 3248 * Constant for fluent queries to be used to add include statements. Specifies 3249 * the path value of "<b>ProcedureRequest:definition</b>". 3250 */ 3251 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("ProcedureRequest:definition").toLocked(); 3252 3253 /** 3254 * Search parameter: <b>body-site</b> 3255 * <p> 3256 * Description: <b>Where procedure is going to be done</b><br> 3257 * Type: <b>token</b><br> 3258 * Path: <b>ProcedureRequest.bodySite</b><br> 3259 * </p> 3260 */ 3261 @SearchParamDefinition(name="body-site", path="ProcedureRequest.bodySite", description="Where procedure is going to be done", type="token" ) 3262 public static final String SP_BODY_SITE = "body-site"; 3263 /** 3264 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3265 * <p> 3266 * Description: <b>Where procedure is going to be done</b><br> 3267 * Type: <b>token</b><br> 3268 * Path: <b>ProcedureRequest.bodySite</b><br> 3269 * </p> 3270 */ 3271 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3272 3273 /** 3274 * Search parameter: <b>status</b> 3275 * <p> 3276 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled</b><br> 3277 * Type: <b>token</b><br> 3278 * Path: <b>ProcedureRequest.status</b><br> 3279 * </p> 3280 */ 3281 @SearchParamDefinition(name="status", path="ProcedureRequest.status", description="draft | active | suspended | completed | entered-in-error | cancelled", type="token" ) 3282 public static final String SP_STATUS = "status"; 3283 /** 3284 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3285 * <p> 3286 * Description: <b>draft | active | suspended | completed | entered-in-error | cancelled</b><br> 3287 * Type: <b>token</b><br> 3288 * Path: <b>ProcedureRequest.status</b><br> 3289 * </p> 3290 */ 3291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3292 3293 3294}