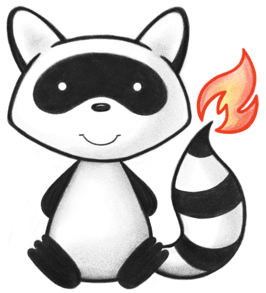
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 051 */ 052@ResourceDef(name="ProcessRequest", profile="http://hl7.org/fhir/Profile/ProcessRequest") 053public class ProcessRequest extends DomainResource { 054 055 public enum ProcessRequestStatus { 056 /** 057 * The instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ProcessRequestStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ProcessRequestStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 105 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 106 case DRAFT: return "http://hl7.org/fhir/fm-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The instance is currently in-force."; 115 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class ProcessRequestStatusEnumFactory implements EnumFactory<ProcessRequestStatus> { 135 public ProcessRequestStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return ProcessRequestStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return ProcessRequestStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return ProcessRequestStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return ProcessRequestStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown ProcessRequestStatus code '"+codeString+"'"); 148 } 149 public Enumeration<ProcessRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<ProcessRequestStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<ProcessRequestStatus>(this, ProcessRequestStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<ProcessRequestStatus>(this, ProcessRequestStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<ProcessRequestStatus>(this, ProcessRequestStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<ProcessRequestStatus>(this, ProcessRequestStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown ProcessRequestStatus code '"+codeString+"'"); 166 } 167 public String toCode(ProcessRequestStatus code) { 168 if (code == ProcessRequestStatus.NULL) 169 return null; 170 if (code == ProcessRequestStatus.ACTIVE) 171 return "active"; 172 if (code == ProcessRequestStatus.CANCELLED) 173 return "cancelled"; 174 if (code == ProcessRequestStatus.DRAFT) 175 return "draft"; 176 if (code == ProcessRequestStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(ProcessRequestStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 public enum ActionList { 186 /** 187 * Cancel, reverse or nullify the target resource. 188 */ 189 CANCEL, 190 /** 191 * Check for previously un-read/ not-retrieved resources. 192 */ 193 POLL, 194 /** 195 * Re-process the target resource. 196 */ 197 REPROCESS, 198 /** 199 * Retrieve the processing status of the target resource. 200 */ 201 STATUS, 202 /** 203 * added to help the parsers with the generic types 204 */ 205 NULL; 206 public static ActionList fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("cancel".equals(codeString)) 210 return CANCEL; 211 if ("poll".equals(codeString)) 212 return POLL; 213 if ("reprocess".equals(codeString)) 214 return REPROCESS; 215 if ("status".equals(codeString)) 216 return STATUS; 217 if (Configuration.isAcceptInvalidEnums()) 218 return null; 219 else 220 throw new FHIRException("Unknown ActionList code '"+codeString+"'"); 221 } 222 public String toCode() { 223 switch (this) { 224 case CANCEL: return "cancel"; 225 case POLL: return "poll"; 226 case REPROCESS: return "reprocess"; 227 case STATUS: return "status"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 public String getSystem() { 233 switch (this) { 234 case CANCEL: return "http://hl7.org/fhir/actionlist"; 235 case POLL: return "http://hl7.org/fhir/actionlist"; 236 case REPROCESS: return "http://hl7.org/fhir/actionlist"; 237 case STATUS: return "http://hl7.org/fhir/actionlist"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getDefinition() { 243 switch (this) { 244 case CANCEL: return "Cancel, reverse or nullify the target resource."; 245 case POLL: return "Check for previously un-read/ not-retrieved resources."; 246 case REPROCESS: return "Re-process the target resource."; 247 case STATUS: return "Retrieve the processing status of the target resource."; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 public String getDisplay() { 253 switch (this) { 254 case CANCEL: return "Cancel, Reverse or Nullify"; 255 case POLL: return "Poll"; 256 case REPROCESS: return "Re-Process"; 257 case STATUS: return "Status Check"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 } 263 264 public static class ActionListEnumFactory implements EnumFactory<ActionList> { 265 public ActionList fromCode(String codeString) throws IllegalArgumentException { 266 if (codeString == null || "".equals(codeString)) 267 if (codeString == null || "".equals(codeString)) 268 return null; 269 if ("cancel".equals(codeString)) 270 return ActionList.CANCEL; 271 if ("poll".equals(codeString)) 272 return ActionList.POLL; 273 if ("reprocess".equals(codeString)) 274 return ActionList.REPROCESS; 275 if ("status".equals(codeString)) 276 return ActionList.STATUS; 277 throw new IllegalArgumentException("Unknown ActionList code '"+codeString+"'"); 278 } 279 public Enumeration<ActionList> fromType(PrimitiveType<?> code) throws FHIRException { 280 if (code == null) 281 return null; 282 if (code.isEmpty()) 283 return new Enumeration<ActionList>(this); 284 String codeString = code.asStringValue(); 285 if (codeString == null || "".equals(codeString)) 286 return null; 287 if ("cancel".equals(codeString)) 288 return new Enumeration<ActionList>(this, ActionList.CANCEL); 289 if ("poll".equals(codeString)) 290 return new Enumeration<ActionList>(this, ActionList.POLL); 291 if ("reprocess".equals(codeString)) 292 return new Enumeration<ActionList>(this, ActionList.REPROCESS); 293 if ("status".equals(codeString)) 294 return new Enumeration<ActionList>(this, ActionList.STATUS); 295 throw new FHIRException("Unknown ActionList code '"+codeString+"'"); 296 } 297 public String toCode(ActionList code) { 298 if (code == ActionList.NULL) 299 return null; 300 if (code == ActionList.CANCEL) 301 return "cancel"; 302 if (code == ActionList.POLL) 303 return "poll"; 304 if (code == ActionList.REPROCESS) 305 return "reprocess"; 306 if (code == ActionList.STATUS) 307 return "status"; 308 return "?"; 309 } 310 public String toSystem(ActionList code) { 311 return code.getSystem(); 312 } 313 } 314 315 @Block() 316 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 317 /** 318 * A service line number. 319 */ 320 @Child(name = "sequenceLinkId", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=false) 321 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 322 protected IntegerType sequenceLinkId; 323 324 private static final long serialVersionUID = -1598360600L; 325 326 /** 327 * Constructor 328 */ 329 public ItemsComponent() { 330 super(); 331 } 332 333 /** 334 * Constructor 335 */ 336 public ItemsComponent(IntegerType sequenceLinkId) { 337 super(); 338 this.sequenceLinkId = sequenceLinkId; 339 } 340 341 /** 342 * @return {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 343 */ 344 public IntegerType getSequenceLinkIdElement() { 345 if (this.sequenceLinkId == null) 346 if (Configuration.errorOnAutoCreate()) 347 throw new Error("Attempt to auto-create ItemsComponent.sequenceLinkId"); 348 else if (Configuration.doAutoCreate()) 349 this.sequenceLinkId = new IntegerType(); // bb 350 return this.sequenceLinkId; 351 } 352 353 public boolean hasSequenceLinkIdElement() { 354 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 355 } 356 357 public boolean hasSequenceLinkId() { 358 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #sequenceLinkId} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequenceLinkId" gives direct access to the value 363 */ 364 public ItemsComponent setSequenceLinkIdElement(IntegerType value) { 365 this.sequenceLinkId = value; 366 return this; 367 } 368 369 /** 370 * @return A service line number. 371 */ 372 public int getSequenceLinkId() { 373 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 374 } 375 376 /** 377 * @param value A service line number. 378 */ 379 public ItemsComponent setSequenceLinkId(int value) { 380 if (this.sequenceLinkId == null) 381 this.sequenceLinkId = new IntegerType(); 382 this.sequenceLinkId.setValue(value); 383 return this; 384 } 385 386 protected void listChildren(List<Property> children) { 387 super.listChildren(children); 388 children.add(new Property("sequenceLinkId", "integer", "A service line number.", 0, 1, sequenceLinkId)); 389 } 390 391 @Override 392 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 393 switch (_hash) { 394 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "integer", "A service line number.", 0, 1, sequenceLinkId); 395 default: return super.getNamedProperty(_hash, _name, _checkValid); 396 } 397 398 } 399 400 @Override 401 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 402 switch (hash) { 403 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : new Base[] {this.sequenceLinkId}; // IntegerType 404 default: return super.getProperty(hash, name, checkValid); 405 } 406 407 } 408 409 @Override 410 public Base setProperty(int hash, String name, Base value) throws FHIRException { 411 switch (hash) { 412 case -1422298666: // sequenceLinkId 413 this.sequenceLinkId = castToInteger(value); // IntegerType 414 return value; 415 default: return super.setProperty(hash, name, value); 416 } 417 418 } 419 420 @Override 421 public Base setProperty(String name, Base value) throws FHIRException { 422 if (name.equals("sequenceLinkId")) { 423 this.sequenceLinkId = castToInteger(value); // IntegerType 424 } else 425 return super.setProperty(name, value); 426 return value; 427 } 428 429 @Override 430 public Base makeProperty(int hash, String name) throws FHIRException { 431 switch (hash) { 432 case -1422298666: return getSequenceLinkIdElement(); 433 default: return super.makeProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 440 switch (hash) { 441 case -1422298666: /*sequenceLinkId*/ return new String[] {"integer"}; 442 default: return super.getTypesForProperty(hash, name); 443 } 444 445 } 446 447 @Override 448 public Base addChild(String name) throws FHIRException { 449 if (name.equals("sequenceLinkId")) { 450 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.sequenceLinkId"); 451 } 452 else 453 return super.addChild(name); 454 } 455 456 public ItemsComponent copy() { 457 ItemsComponent dst = new ItemsComponent(); 458 copyValues(dst); 459 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 460 return dst; 461 } 462 463 @Override 464 public boolean equalsDeep(Base other_) { 465 if (!super.equalsDeep(other_)) 466 return false; 467 if (!(other_ instanceof ItemsComponent)) 468 return false; 469 ItemsComponent o = (ItemsComponent) other_; 470 return compareDeep(sequenceLinkId, o.sequenceLinkId, true); 471 } 472 473 @Override 474 public boolean equalsShallow(Base other_) { 475 if (!super.equalsShallow(other_)) 476 return false; 477 if (!(other_ instanceof ItemsComponent)) 478 return false; 479 ItemsComponent o = (ItemsComponent) other_; 480 return compareValues(sequenceLinkId, o.sequenceLinkId, true); 481 } 482 483 public boolean isEmpty() { 484 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId); 485 } 486 487 public String fhirType() { 488 return "ProcessRequest.item"; 489 490 } 491 492 } 493 494 /** 495 * The ProcessRequest business identifier. 496 */ 497 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 498 @Description(shortDefinition="Business Identifier", formalDefinition="The ProcessRequest business identifier." ) 499 protected List<Identifier> identifier; 500 501 /** 502 * The status of the resource instance. 503 */ 504 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 505 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 506 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 507 protected Enumeration<ProcessRequestStatus> status; 508 509 /** 510 * The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest. 511 */ 512 @Child(name = "action", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 513 @Description(shortDefinition="cancel | poll | reprocess | status", formalDefinition="The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest." ) 514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/actionlist") 515 protected Enumeration<ActionList> action; 516 517 /** 518 * The organization which is the target of the request. 519 */ 520 @Child(name = "target", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 521 @Description(shortDefinition="Party which is the target of the request", formalDefinition="The organization which is the target of the request." ) 522 protected Reference target; 523 524 /** 525 * The actual object that is the target of the reference (The organization which is the target of the request.) 526 */ 527 protected Organization targetTarget; 528 529 /** 530 * The date when this resource was created. 531 */ 532 @Child(name = "created", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 533 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 534 protected DateTimeType created; 535 536 /** 537 * The practitioner who is responsible for the action specified in this request. 538 */ 539 @Child(name = "provider", type = {Practitioner.class}, order=5, min=0, max=1, modifier=false, summary=false) 540 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the action specified in this request." ) 541 protected Reference provider; 542 543 /** 544 * The actual object that is the target of the reference (The practitioner who is responsible for the action specified in this request.) 545 */ 546 protected Practitioner providerTarget; 547 548 /** 549 * The organization which is responsible for the action speccified in this request. 550 */ 551 @Child(name = "organization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 552 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the action speccified in this request." ) 553 protected Reference organization; 554 555 /** 556 * The actual object that is the target of the reference (The organization which is responsible for the action speccified in this request.) 557 */ 558 protected Organization organizationTarget; 559 560 /** 561 * Reference of resource which is the target or subject of this action. 562 */ 563 @Child(name = "request", type = {Reference.class}, order=7, min=0, max=1, modifier=false, summary=false) 564 @Description(shortDefinition="Reference to the Request resource", formalDefinition="Reference of resource which is the target or subject of this action." ) 565 protected Reference request; 566 567 /** 568 * The actual object that is the target of the reference (Reference of resource which is the target or subject of this action.) 569 */ 570 protected Resource requestTarget; 571 572 /** 573 * Reference of a prior response to resource which is the target or subject of this action. 574 */ 575 @Child(name = "response", type = {Reference.class}, order=8, min=0, max=1, modifier=false, summary=false) 576 @Description(shortDefinition="Reference to the Response resource", formalDefinition="Reference of a prior response to resource which is the target or subject of this action." ) 577 protected Reference response; 578 579 /** 580 * The actual object that is the target of the reference (Reference of a prior response to resource which is the target or subject of this action.) 581 */ 582 protected Resource responseTarget; 583 584 /** 585 * If true remove all history excluding audit. 586 */ 587 @Child(name = "nullify", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 588 @Description(shortDefinition="Remove history", formalDefinition="If true remove all history excluding audit." ) 589 protected BooleanType nullify; 590 591 /** 592 * A reference to supply which authenticates the process. 593 */ 594 @Child(name = "reference", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 595 @Description(shortDefinition="Reference number/string", formalDefinition="A reference to supply which authenticates the process." ) 596 protected StringType reference; 597 598 /** 599 * List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated. 600 */ 601 @Child(name = "item", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 602 @Description(shortDefinition="Items to re-adjudicate", formalDefinition="List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated." ) 603 protected List<ItemsComponent> item; 604 605 /** 606 * Names of resource types to include. 607 */ 608 @Child(name = "include", type = {StringType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 609 @Description(shortDefinition="Resource type(s) to include", formalDefinition="Names of resource types to include." ) 610 protected List<StringType> include; 611 612 /** 613 * Names of resource types to exclude. 614 */ 615 @Child(name = "exclude", type = {StringType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 616 @Description(shortDefinition="Resource type(s) to exclude", formalDefinition="Names of resource types to exclude." ) 617 protected List<StringType> exclude; 618 619 /** 620 * A period of time during which the fulfilling resources would have been created. 621 */ 622 @Child(name = "period", type = {Period.class}, order=14, min=0, max=1, modifier=false, summary=false) 623 @Description(shortDefinition="Selection period", formalDefinition="A period of time during which the fulfilling resources would have been created." ) 624 protected Period period; 625 626 private static final long serialVersionUID = -346692020L; 627 628 /** 629 * Constructor 630 */ 631 public ProcessRequest() { 632 super(); 633 } 634 635 /** 636 * @return {@link #identifier} (The ProcessRequest business identifier.) 637 */ 638 public List<Identifier> getIdentifier() { 639 if (this.identifier == null) 640 this.identifier = new ArrayList<Identifier>(); 641 return this.identifier; 642 } 643 644 /** 645 * @return Returns a reference to <code>this</code> for easy method chaining 646 */ 647 public ProcessRequest setIdentifier(List<Identifier> theIdentifier) { 648 this.identifier = theIdentifier; 649 return this; 650 } 651 652 public boolean hasIdentifier() { 653 if (this.identifier == null) 654 return false; 655 for (Identifier item : this.identifier) 656 if (!item.isEmpty()) 657 return true; 658 return false; 659 } 660 661 public Identifier addIdentifier() { //3 662 Identifier t = new Identifier(); 663 if (this.identifier == null) 664 this.identifier = new ArrayList<Identifier>(); 665 this.identifier.add(t); 666 return t; 667 } 668 669 public ProcessRequest addIdentifier(Identifier t) { //3 670 if (t == null) 671 return this; 672 if (this.identifier == null) 673 this.identifier = new ArrayList<Identifier>(); 674 this.identifier.add(t); 675 return this; 676 } 677 678 /** 679 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 680 */ 681 public Identifier getIdentifierFirstRep() { 682 if (getIdentifier().isEmpty()) { 683 addIdentifier(); 684 } 685 return getIdentifier().get(0); 686 } 687 688 /** 689 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 690 */ 691 public Enumeration<ProcessRequestStatus> getStatusElement() { 692 if (this.status == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create ProcessRequest.status"); 695 else if (Configuration.doAutoCreate()) 696 this.status = new Enumeration<ProcessRequestStatus>(new ProcessRequestStatusEnumFactory()); // bb 697 return this.status; 698 } 699 700 public boolean hasStatusElement() { 701 return this.status != null && !this.status.isEmpty(); 702 } 703 704 public boolean hasStatus() { 705 return this.status != null && !this.status.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 710 */ 711 public ProcessRequest setStatusElement(Enumeration<ProcessRequestStatus> value) { 712 this.status = value; 713 return this; 714 } 715 716 /** 717 * @return The status of the resource instance. 718 */ 719 public ProcessRequestStatus getStatus() { 720 return this.status == null ? null : this.status.getValue(); 721 } 722 723 /** 724 * @param value The status of the resource instance. 725 */ 726 public ProcessRequest setStatus(ProcessRequestStatus value) { 727 if (value == null) 728 this.status = null; 729 else { 730 if (this.status == null) 731 this.status = new Enumeration<ProcessRequestStatus>(new ProcessRequestStatusEnumFactory()); 732 this.status.setValue(value); 733 } 734 return this; 735 } 736 737 /** 738 * @return {@link #action} (The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 739 */ 740 public Enumeration<ActionList> getActionElement() { 741 if (this.action == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create ProcessRequest.action"); 744 else if (Configuration.doAutoCreate()) 745 this.action = new Enumeration<ActionList>(new ActionListEnumFactory()); // bb 746 return this.action; 747 } 748 749 public boolean hasActionElement() { 750 return this.action != null && !this.action.isEmpty(); 751 } 752 753 public boolean hasAction() { 754 return this.action != null && !this.action.isEmpty(); 755 } 756 757 /** 758 * @param value {@link #action} (The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 759 */ 760 public ProcessRequest setActionElement(Enumeration<ActionList> value) { 761 this.action = value; 762 return this; 763 } 764 765 /** 766 * @return The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest. 767 */ 768 public ActionList getAction() { 769 return this.action == null ? null : this.action.getValue(); 770 } 771 772 /** 773 * @param value The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest. 774 */ 775 public ProcessRequest setAction(ActionList value) { 776 if (value == null) 777 this.action = null; 778 else { 779 if (this.action == null) 780 this.action = new Enumeration<ActionList>(new ActionListEnumFactory()); 781 this.action.setValue(value); 782 } 783 return this; 784 } 785 786 /** 787 * @return {@link #target} (The organization which is the target of the request.) 788 */ 789 public Reference getTarget() { 790 if (this.target == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create ProcessRequest.target"); 793 else if (Configuration.doAutoCreate()) 794 this.target = new Reference(); // cc 795 return this.target; 796 } 797 798 public boolean hasTarget() { 799 return this.target != null && !this.target.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #target} (The organization which is the target of the request.) 804 */ 805 public ProcessRequest setTarget(Reference value) { 806 this.target = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #target} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is the target of the request.) 812 */ 813 public Organization getTargetTarget() { 814 if (this.targetTarget == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create ProcessRequest.target"); 817 else if (Configuration.doAutoCreate()) 818 this.targetTarget = new Organization(); // aa 819 return this.targetTarget; 820 } 821 822 /** 823 * @param value {@link #target} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is the target of the request.) 824 */ 825 public ProcessRequest setTargetTarget(Organization value) { 826 this.targetTarget = value; 827 return this; 828 } 829 830 /** 831 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 832 */ 833 public DateTimeType getCreatedElement() { 834 if (this.created == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create ProcessRequest.created"); 837 else if (Configuration.doAutoCreate()) 838 this.created = new DateTimeType(); // bb 839 return this.created; 840 } 841 842 public boolean hasCreatedElement() { 843 return this.created != null && !this.created.isEmpty(); 844 } 845 846 public boolean hasCreated() { 847 return this.created != null && !this.created.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 852 */ 853 public ProcessRequest setCreatedElement(DateTimeType value) { 854 this.created = value; 855 return this; 856 } 857 858 /** 859 * @return The date when this resource was created. 860 */ 861 public Date getCreated() { 862 return this.created == null ? null : this.created.getValue(); 863 } 864 865 /** 866 * @param value The date when this resource was created. 867 */ 868 public ProcessRequest setCreated(Date value) { 869 if (value == null) 870 this.created = null; 871 else { 872 if (this.created == null) 873 this.created = new DateTimeType(); 874 this.created.setValue(value); 875 } 876 return this; 877 } 878 879 /** 880 * @return {@link #provider} (The practitioner who is responsible for the action specified in this request.) 881 */ 882 public Reference getProvider() { 883 if (this.provider == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create ProcessRequest.provider"); 886 else if (Configuration.doAutoCreate()) 887 this.provider = new Reference(); // cc 888 return this.provider; 889 } 890 891 public boolean hasProvider() { 892 return this.provider != null && !this.provider.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #provider} (The practitioner who is responsible for the action specified in this request.) 897 */ 898 public ProcessRequest setProvider(Reference value) { 899 this.provider = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the action specified in this request.) 905 */ 906 public Practitioner getProviderTarget() { 907 if (this.providerTarget == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create ProcessRequest.provider"); 910 else if (Configuration.doAutoCreate()) 911 this.providerTarget = new Practitioner(); // aa 912 return this.providerTarget; 913 } 914 915 /** 916 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the action specified in this request.) 917 */ 918 public ProcessRequest setProviderTarget(Practitioner value) { 919 this.providerTarget = value; 920 return this; 921 } 922 923 /** 924 * @return {@link #organization} (The organization which is responsible for the action speccified in this request.) 925 */ 926 public Reference getOrganization() { 927 if (this.organization == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create ProcessRequest.organization"); 930 else if (Configuration.doAutoCreate()) 931 this.organization = new Reference(); // cc 932 return this.organization; 933 } 934 935 public boolean hasOrganization() { 936 return this.organization != null && !this.organization.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #organization} (The organization which is responsible for the action speccified in this request.) 941 */ 942 public ProcessRequest setOrganization(Reference value) { 943 this.organization = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the action speccified in this request.) 949 */ 950 public Organization getOrganizationTarget() { 951 if (this.organizationTarget == null) 952 if (Configuration.errorOnAutoCreate()) 953 throw new Error("Attempt to auto-create ProcessRequest.organization"); 954 else if (Configuration.doAutoCreate()) 955 this.organizationTarget = new Organization(); // aa 956 return this.organizationTarget; 957 } 958 959 /** 960 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the action speccified in this request.) 961 */ 962 public ProcessRequest setOrganizationTarget(Organization value) { 963 this.organizationTarget = value; 964 return this; 965 } 966 967 /** 968 * @return {@link #request} (Reference of resource which is the target or subject of this action.) 969 */ 970 public Reference getRequest() { 971 if (this.request == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create ProcessRequest.request"); 974 else if (Configuration.doAutoCreate()) 975 this.request = new Reference(); // cc 976 return this.request; 977 } 978 979 public boolean hasRequest() { 980 return this.request != null && !this.request.isEmpty(); 981 } 982 983 /** 984 * @param value {@link #request} (Reference of resource which is the target or subject of this action.) 985 */ 986 public ProcessRequest setRequest(Reference value) { 987 this.request = value; 988 return this; 989 } 990 991 /** 992 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference of resource which is the target or subject of this action.) 993 */ 994 public Resource getRequestTarget() { 995 return this.requestTarget; 996 } 997 998 /** 999 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference of resource which is the target or subject of this action.) 1000 */ 1001 public ProcessRequest setRequestTarget(Resource value) { 1002 this.requestTarget = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #response} (Reference of a prior response to resource which is the target or subject of this action.) 1008 */ 1009 public Reference getResponse() { 1010 if (this.response == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create ProcessRequest.response"); 1013 else if (Configuration.doAutoCreate()) 1014 this.response = new Reference(); // cc 1015 return this.response; 1016 } 1017 1018 public boolean hasResponse() { 1019 return this.response != null && !this.response.isEmpty(); 1020 } 1021 1022 /** 1023 * @param value {@link #response} (Reference of a prior response to resource which is the target or subject of this action.) 1024 */ 1025 public ProcessRequest setResponse(Reference value) { 1026 this.response = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return {@link #response} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference of a prior response to resource which is the target or subject of this action.) 1032 */ 1033 public Resource getResponseTarget() { 1034 return this.responseTarget; 1035 } 1036 1037 /** 1038 * @param value {@link #response} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference of a prior response to resource which is the target or subject of this action.) 1039 */ 1040 public ProcessRequest setResponseTarget(Resource value) { 1041 this.responseTarget = value; 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #nullify} (If true remove all history excluding audit.). This is the underlying object with id, value and extensions. The accessor "getNullify" gives direct access to the value 1047 */ 1048 public BooleanType getNullifyElement() { 1049 if (this.nullify == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create ProcessRequest.nullify"); 1052 else if (Configuration.doAutoCreate()) 1053 this.nullify = new BooleanType(); // bb 1054 return this.nullify; 1055 } 1056 1057 public boolean hasNullifyElement() { 1058 return this.nullify != null && !this.nullify.isEmpty(); 1059 } 1060 1061 public boolean hasNullify() { 1062 return this.nullify != null && !this.nullify.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #nullify} (If true remove all history excluding audit.). This is the underlying object with id, value and extensions. The accessor "getNullify" gives direct access to the value 1067 */ 1068 public ProcessRequest setNullifyElement(BooleanType value) { 1069 this.nullify = value; 1070 return this; 1071 } 1072 1073 /** 1074 * @return If true remove all history excluding audit. 1075 */ 1076 public boolean getNullify() { 1077 return this.nullify == null || this.nullify.isEmpty() ? false : this.nullify.getValue(); 1078 } 1079 1080 /** 1081 * @param value If true remove all history excluding audit. 1082 */ 1083 public ProcessRequest setNullify(boolean value) { 1084 if (this.nullify == null) 1085 this.nullify = new BooleanType(); 1086 this.nullify.setValue(value); 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #reference} (A reference to supply which authenticates the process.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1092 */ 1093 public StringType getReferenceElement() { 1094 if (this.reference == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error("Attempt to auto-create ProcessRequest.reference"); 1097 else if (Configuration.doAutoCreate()) 1098 this.reference = new StringType(); // bb 1099 return this.reference; 1100 } 1101 1102 public boolean hasReferenceElement() { 1103 return this.reference != null && !this.reference.isEmpty(); 1104 } 1105 1106 public boolean hasReference() { 1107 return this.reference != null && !this.reference.isEmpty(); 1108 } 1109 1110 /** 1111 * @param value {@link #reference} (A reference to supply which authenticates the process.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1112 */ 1113 public ProcessRequest setReferenceElement(StringType value) { 1114 this.reference = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return A reference to supply which authenticates the process. 1120 */ 1121 public String getReference() { 1122 return this.reference == null ? null : this.reference.getValue(); 1123 } 1124 1125 /** 1126 * @param value A reference to supply which authenticates the process. 1127 */ 1128 public ProcessRequest setReference(String value) { 1129 if (Utilities.noString(value)) 1130 this.reference = null; 1131 else { 1132 if (this.reference == null) 1133 this.reference = new StringType(); 1134 this.reference.setValue(value); 1135 } 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #item} (List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated.) 1141 */ 1142 public List<ItemsComponent> getItem() { 1143 if (this.item == null) 1144 this.item = new ArrayList<ItemsComponent>(); 1145 return this.item; 1146 } 1147 1148 /** 1149 * @return Returns a reference to <code>this</code> for easy method chaining 1150 */ 1151 public ProcessRequest setItem(List<ItemsComponent> theItem) { 1152 this.item = theItem; 1153 return this; 1154 } 1155 1156 public boolean hasItem() { 1157 if (this.item == null) 1158 return false; 1159 for (ItemsComponent item : this.item) 1160 if (!item.isEmpty()) 1161 return true; 1162 return false; 1163 } 1164 1165 public ItemsComponent addItem() { //3 1166 ItemsComponent t = new ItemsComponent(); 1167 if (this.item == null) 1168 this.item = new ArrayList<ItemsComponent>(); 1169 this.item.add(t); 1170 return t; 1171 } 1172 1173 public ProcessRequest addItem(ItemsComponent t) { //3 1174 if (t == null) 1175 return this; 1176 if (this.item == null) 1177 this.item = new ArrayList<ItemsComponent>(); 1178 this.item.add(t); 1179 return this; 1180 } 1181 1182 /** 1183 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 1184 */ 1185 public ItemsComponent getItemFirstRep() { 1186 if (getItem().isEmpty()) { 1187 addItem(); 1188 } 1189 return getItem().get(0); 1190 } 1191 1192 /** 1193 * @return {@link #include} (Names of resource types to include.) 1194 */ 1195 public List<StringType> getInclude() { 1196 if (this.include == null) 1197 this.include = new ArrayList<StringType>(); 1198 return this.include; 1199 } 1200 1201 /** 1202 * @return Returns a reference to <code>this</code> for easy method chaining 1203 */ 1204 public ProcessRequest setInclude(List<StringType> theInclude) { 1205 this.include = theInclude; 1206 return this; 1207 } 1208 1209 public boolean hasInclude() { 1210 if (this.include == null) 1211 return false; 1212 for (StringType item : this.include) 1213 if (!item.isEmpty()) 1214 return true; 1215 return false; 1216 } 1217 1218 /** 1219 * @return {@link #include} (Names of resource types to include.) 1220 */ 1221 public StringType addIncludeElement() {//2 1222 StringType t = new StringType(); 1223 if (this.include == null) 1224 this.include = new ArrayList<StringType>(); 1225 this.include.add(t); 1226 return t; 1227 } 1228 1229 /** 1230 * @param value {@link #include} (Names of resource types to include.) 1231 */ 1232 public ProcessRequest addInclude(String value) { //1 1233 StringType t = new StringType(); 1234 t.setValue(value); 1235 if (this.include == null) 1236 this.include = new ArrayList<StringType>(); 1237 this.include.add(t); 1238 return this; 1239 } 1240 1241 /** 1242 * @param value {@link #include} (Names of resource types to include.) 1243 */ 1244 public boolean hasInclude(String value) { 1245 if (this.include == null) 1246 return false; 1247 for (StringType v : this.include) 1248 if (v.getValue().equals(value)) // string 1249 return true; 1250 return false; 1251 } 1252 1253 /** 1254 * @return {@link #exclude} (Names of resource types to exclude.) 1255 */ 1256 public List<StringType> getExclude() { 1257 if (this.exclude == null) 1258 this.exclude = new ArrayList<StringType>(); 1259 return this.exclude; 1260 } 1261 1262 /** 1263 * @return Returns a reference to <code>this</code> for easy method chaining 1264 */ 1265 public ProcessRequest setExclude(List<StringType> theExclude) { 1266 this.exclude = theExclude; 1267 return this; 1268 } 1269 1270 public boolean hasExclude() { 1271 if (this.exclude == null) 1272 return false; 1273 for (StringType item : this.exclude) 1274 if (!item.isEmpty()) 1275 return true; 1276 return false; 1277 } 1278 1279 /** 1280 * @return {@link #exclude} (Names of resource types to exclude.) 1281 */ 1282 public StringType addExcludeElement() {//2 1283 StringType t = new StringType(); 1284 if (this.exclude == null) 1285 this.exclude = new ArrayList<StringType>(); 1286 this.exclude.add(t); 1287 return t; 1288 } 1289 1290 /** 1291 * @param value {@link #exclude} (Names of resource types to exclude.) 1292 */ 1293 public ProcessRequest addExclude(String value) { //1 1294 StringType t = new StringType(); 1295 t.setValue(value); 1296 if (this.exclude == null) 1297 this.exclude = new ArrayList<StringType>(); 1298 this.exclude.add(t); 1299 return this; 1300 } 1301 1302 /** 1303 * @param value {@link #exclude} (Names of resource types to exclude.) 1304 */ 1305 public boolean hasExclude(String value) { 1306 if (this.exclude == null) 1307 return false; 1308 for (StringType v : this.exclude) 1309 if (v.getValue().equals(value)) // string 1310 return true; 1311 return false; 1312 } 1313 1314 /** 1315 * @return {@link #period} (A period of time during which the fulfilling resources would have been created.) 1316 */ 1317 public Period getPeriod() { 1318 if (this.period == null) 1319 if (Configuration.errorOnAutoCreate()) 1320 throw new Error("Attempt to auto-create ProcessRequest.period"); 1321 else if (Configuration.doAutoCreate()) 1322 this.period = new Period(); // cc 1323 return this.period; 1324 } 1325 1326 public boolean hasPeriod() { 1327 return this.period != null && !this.period.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #period} (A period of time during which the fulfilling resources would have been created.) 1332 */ 1333 public ProcessRequest setPeriod(Period value) { 1334 this.period = value; 1335 return this; 1336 } 1337 1338 protected void listChildren(List<Property> children) { 1339 super.listChildren(children); 1340 children.add(new Property("identifier", "Identifier", "The ProcessRequest business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1341 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 1342 children.add(new Property("action", "code", "The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.", 0, 1, action)); 1343 children.add(new Property("target", "Reference(Organization)", "The organization which is the target of the request.", 0, 1, target)); 1344 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 1345 children.add(new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the action specified in this request.", 0, 1, provider)); 1346 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the action speccified in this request.", 0, 1, organization)); 1347 children.add(new Property("request", "Reference(Any)", "Reference of resource which is the target or subject of this action.", 0, 1, request)); 1348 children.add(new Property("response", "Reference(Any)", "Reference of a prior response to resource which is the target or subject of this action.", 0, 1, response)); 1349 children.add(new Property("nullify", "boolean", "If true remove all history excluding audit.", 0, 1, nullify)); 1350 children.add(new Property("reference", "string", "A reference to supply which authenticates the process.", 0, 1, reference)); 1351 children.add(new Property("item", "", "List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated.", 0, java.lang.Integer.MAX_VALUE, item)); 1352 children.add(new Property("include", "string", "Names of resource types to include.", 0, java.lang.Integer.MAX_VALUE, include)); 1353 children.add(new Property("exclude", "string", "Names of resource types to exclude.", 0, java.lang.Integer.MAX_VALUE, exclude)); 1354 children.add(new Property("period", "Period", "A period of time during which the fulfilling resources would have been created.", 0, 1, period)); 1355 } 1356 1357 @Override 1358 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1359 switch (_hash) { 1360 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The ProcessRequest business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1361 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 1362 case -1422950858: /*action*/ return new Property("action", "code", "The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.", 0, 1, action); 1363 case -880905839: /*target*/ return new Property("target", "Reference(Organization)", "The organization which is the target of the request.", 0, 1, target); 1364 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 1365 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the action specified in this request.", 0, 1, provider); 1366 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the action speccified in this request.", 0, 1, organization); 1367 case 1095692943: /*request*/ return new Property("request", "Reference(Any)", "Reference of resource which is the target or subject of this action.", 0, 1, request); 1368 case -340323263: /*response*/ return new Property("response", "Reference(Any)", "Reference of a prior response to resource which is the target or subject of this action.", 0, 1, response); 1369 case -2001137643: /*nullify*/ return new Property("nullify", "boolean", "If true remove all history excluding audit.", 0, 1, nullify); 1370 case -925155509: /*reference*/ return new Property("reference", "string", "A reference to supply which authenticates the process.", 0, 1, reference); 1371 case 3242771: /*item*/ return new Property("item", "", "List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated.", 0, java.lang.Integer.MAX_VALUE, item); 1372 case 1942574248: /*include*/ return new Property("include", "string", "Names of resource types to include.", 0, java.lang.Integer.MAX_VALUE, include); 1373 case -1321148966: /*exclude*/ return new Property("exclude", "string", "Names of resource types to exclude.", 0, java.lang.Integer.MAX_VALUE, exclude); 1374 case -991726143: /*period*/ return new Property("period", "Period", "A period of time during which the fulfilling resources would have been created.", 0, 1, period); 1375 default: return super.getNamedProperty(_hash, _name, _checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1382 switch (hash) { 1383 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1384 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ProcessRequestStatus> 1385 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // Enumeration<ActionList> 1386 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 1387 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1388 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1389 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1390 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 1391 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Reference 1392 case -2001137643: /*nullify*/ return this.nullify == null ? new Base[0] : new Base[] {this.nullify}; // BooleanType 1393 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // StringType 1394 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemsComponent 1395 case 1942574248: /*include*/ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // StringType 1396 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : this.exclude.toArray(new Base[this.exclude.size()]); // StringType 1397 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1398 default: return super.getProperty(hash, name, checkValid); 1399 } 1400 1401 } 1402 1403 @Override 1404 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1405 switch (hash) { 1406 case -1618432855: // identifier 1407 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1408 return value; 1409 case -892481550: // status 1410 value = new ProcessRequestStatusEnumFactory().fromType(castToCode(value)); 1411 this.status = (Enumeration) value; // Enumeration<ProcessRequestStatus> 1412 return value; 1413 case -1422950858: // action 1414 value = new ActionListEnumFactory().fromType(castToCode(value)); 1415 this.action = (Enumeration) value; // Enumeration<ActionList> 1416 return value; 1417 case -880905839: // target 1418 this.target = castToReference(value); // Reference 1419 return value; 1420 case 1028554472: // created 1421 this.created = castToDateTime(value); // DateTimeType 1422 return value; 1423 case -987494927: // provider 1424 this.provider = castToReference(value); // Reference 1425 return value; 1426 case 1178922291: // organization 1427 this.organization = castToReference(value); // Reference 1428 return value; 1429 case 1095692943: // request 1430 this.request = castToReference(value); // Reference 1431 return value; 1432 case -340323263: // response 1433 this.response = castToReference(value); // Reference 1434 return value; 1435 case -2001137643: // nullify 1436 this.nullify = castToBoolean(value); // BooleanType 1437 return value; 1438 case -925155509: // reference 1439 this.reference = castToString(value); // StringType 1440 return value; 1441 case 3242771: // item 1442 this.getItem().add((ItemsComponent) value); // ItemsComponent 1443 return value; 1444 case 1942574248: // include 1445 this.getInclude().add(castToString(value)); // StringType 1446 return value; 1447 case -1321148966: // exclude 1448 this.getExclude().add(castToString(value)); // StringType 1449 return value; 1450 case -991726143: // period 1451 this.period = castToPeriod(value); // Period 1452 return value; 1453 default: return super.setProperty(hash, name, value); 1454 } 1455 1456 } 1457 1458 @Override 1459 public Base setProperty(String name, Base value) throws FHIRException { 1460 if (name.equals("identifier")) { 1461 this.getIdentifier().add(castToIdentifier(value)); 1462 } else if (name.equals("status")) { 1463 value = new ProcessRequestStatusEnumFactory().fromType(castToCode(value)); 1464 this.status = (Enumeration) value; // Enumeration<ProcessRequestStatus> 1465 } else if (name.equals("action")) { 1466 value = new ActionListEnumFactory().fromType(castToCode(value)); 1467 this.action = (Enumeration) value; // Enumeration<ActionList> 1468 } else if (name.equals("target")) { 1469 this.target = castToReference(value); // Reference 1470 } else if (name.equals("created")) { 1471 this.created = castToDateTime(value); // DateTimeType 1472 } else if (name.equals("provider")) { 1473 this.provider = castToReference(value); // Reference 1474 } else if (name.equals("organization")) { 1475 this.organization = castToReference(value); // Reference 1476 } else if (name.equals("request")) { 1477 this.request = castToReference(value); // Reference 1478 } else if (name.equals("response")) { 1479 this.response = castToReference(value); // Reference 1480 } else if (name.equals("nullify")) { 1481 this.nullify = castToBoolean(value); // BooleanType 1482 } else if (name.equals("reference")) { 1483 this.reference = castToString(value); // StringType 1484 } else if (name.equals("item")) { 1485 this.getItem().add((ItemsComponent) value); 1486 } else if (name.equals("include")) { 1487 this.getInclude().add(castToString(value)); 1488 } else if (name.equals("exclude")) { 1489 this.getExclude().add(castToString(value)); 1490 } else if (name.equals("period")) { 1491 this.period = castToPeriod(value); // Period 1492 } else 1493 return super.setProperty(name, value); 1494 return value; 1495 } 1496 1497 @Override 1498 public Base makeProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case -1618432855: return addIdentifier(); 1501 case -892481550: return getStatusElement(); 1502 case -1422950858: return getActionElement(); 1503 case -880905839: return getTarget(); 1504 case 1028554472: return getCreatedElement(); 1505 case -987494927: return getProvider(); 1506 case 1178922291: return getOrganization(); 1507 case 1095692943: return getRequest(); 1508 case -340323263: return getResponse(); 1509 case -2001137643: return getNullifyElement(); 1510 case -925155509: return getReferenceElement(); 1511 case 3242771: return addItem(); 1512 case 1942574248: return addIncludeElement(); 1513 case -1321148966: return addExcludeElement(); 1514 case -991726143: return getPeriod(); 1515 default: return super.makeProperty(hash, name); 1516 } 1517 1518 } 1519 1520 @Override 1521 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1522 switch (hash) { 1523 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1524 case -892481550: /*status*/ return new String[] {"code"}; 1525 case -1422950858: /*action*/ return new String[] {"code"}; 1526 case -880905839: /*target*/ return new String[] {"Reference"}; 1527 case 1028554472: /*created*/ return new String[] {"dateTime"}; 1528 case -987494927: /*provider*/ return new String[] {"Reference"}; 1529 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1530 case 1095692943: /*request*/ return new String[] {"Reference"}; 1531 case -340323263: /*response*/ return new String[] {"Reference"}; 1532 case -2001137643: /*nullify*/ return new String[] {"boolean"}; 1533 case -925155509: /*reference*/ return new String[] {"string"}; 1534 case 3242771: /*item*/ return new String[] {}; 1535 case 1942574248: /*include*/ return new String[] {"string"}; 1536 case -1321148966: /*exclude*/ return new String[] {"string"}; 1537 case -991726143: /*period*/ return new String[] {"Period"}; 1538 default: return super.getTypesForProperty(hash, name); 1539 } 1540 1541 } 1542 1543 @Override 1544 public Base addChild(String name) throws FHIRException { 1545 if (name.equals("identifier")) { 1546 return addIdentifier(); 1547 } 1548 else if (name.equals("status")) { 1549 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.status"); 1550 } 1551 else if (name.equals("action")) { 1552 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.action"); 1553 } 1554 else if (name.equals("target")) { 1555 this.target = new Reference(); 1556 return this.target; 1557 } 1558 else if (name.equals("created")) { 1559 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.created"); 1560 } 1561 else if (name.equals("provider")) { 1562 this.provider = new Reference(); 1563 return this.provider; 1564 } 1565 else if (name.equals("organization")) { 1566 this.organization = new Reference(); 1567 return this.organization; 1568 } 1569 else if (name.equals("request")) { 1570 this.request = new Reference(); 1571 return this.request; 1572 } 1573 else if (name.equals("response")) { 1574 this.response = new Reference(); 1575 return this.response; 1576 } 1577 else if (name.equals("nullify")) { 1578 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.nullify"); 1579 } 1580 else if (name.equals("reference")) { 1581 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.reference"); 1582 } 1583 else if (name.equals("item")) { 1584 return addItem(); 1585 } 1586 else if (name.equals("include")) { 1587 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.include"); 1588 } 1589 else if (name.equals("exclude")) { 1590 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.exclude"); 1591 } 1592 else if (name.equals("period")) { 1593 this.period = new Period(); 1594 return this.period; 1595 } 1596 else 1597 return super.addChild(name); 1598 } 1599 1600 public String fhirType() { 1601 return "ProcessRequest"; 1602 1603 } 1604 1605 public ProcessRequest copy() { 1606 ProcessRequest dst = new ProcessRequest(); 1607 copyValues(dst); 1608 if (identifier != null) { 1609 dst.identifier = new ArrayList<Identifier>(); 1610 for (Identifier i : identifier) 1611 dst.identifier.add(i.copy()); 1612 }; 1613 dst.status = status == null ? null : status.copy(); 1614 dst.action = action == null ? null : action.copy(); 1615 dst.target = target == null ? null : target.copy(); 1616 dst.created = created == null ? null : created.copy(); 1617 dst.provider = provider == null ? null : provider.copy(); 1618 dst.organization = organization == null ? null : organization.copy(); 1619 dst.request = request == null ? null : request.copy(); 1620 dst.response = response == null ? null : response.copy(); 1621 dst.nullify = nullify == null ? null : nullify.copy(); 1622 dst.reference = reference == null ? null : reference.copy(); 1623 if (item != null) { 1624 dst.item = new ArrayList<ItemsComponent>(); 1625 for (ItemsComponent i : item) 1626 dst.item.add(i.copy()); 1627 }; 1628 if (include != null) { 1629 dst.include = new ArrayList<StringType>(); 1630 for (StringType i : include) 1631 dst.include.add(i.copy()); 1632 }; 1633 if (exclude != null) { 1634 dst.exclude = new ArrayList<StringType>(); 1635 for (StringType i : exclude) 1636 dst.exclude.add(i.copy()); 1637 }; 1638 dst.period = period == null ? null : period.copy(); 1639 return dst; 1640 } 1641 1642 protected ProcessRequest typedCopy() { 1643 return copy(); 1644 } 1645 1646 @Override 1647 public boolean equalsDeep(Base other_) { 1648 if (!super.equalsDeep(other_)) 1649 return false; 1650 if (!(other_ instanceof ProcessRequest)) 1651 return false; 1652 ProcessRequest o = (ProcessRequest) other_; 1653 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(action, o.action, true) 1654 && compareDeep(target, o.target, true) && compareDeep(created, o.created, true) && compareDeep(provider, o.provider, true) 1655 && compareDeep(organization, o.organization, true) && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 1656 && compareDeep(nullify, o.nullify, true) && compareDeep(reference, o.reference, true) && compareDeep(item, o.item, true) 1657 && compareDeep(include, o.include, true) && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true) 1658 ; 1659 } 1660 1661 @Override 1662 public boolean equalsShallow(Base other_) { 1663 if (!super.equalsShallow(other_)) 1664 return false; 1665 if (!(other_ instanceof ProcessRequest)) 1666 return false; 1667 ProcessRequest o = (ProcessRequest) other_; 1668 return compareValues(status, o.status, true) && compareValues(action, o.action, true) && compareValues(created, o.created, true) 1669 && compareValues(nullify, o.nullify, true) && compareValues(reference, o.reference, true) && compareValues(include, o.include, true) 1670 && compareValues(exclude, o.exclude, true); 1671 } 1672 1673 public boolean isEmpty() { 1674 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, action 1675 , target, created, provider, organization, request, response, nullify, reference 1676 , item, include, exclude, period); 1677 } 1678 1679 @Override 1680 public ResourceType getResourceType() { 1681 return ResourceType.ProcessRequest; 1682 } 1683 1684 /** 1685 * Search parameter: <b>identifier</b> 1686 * <p> 1687 * Description: <b>The business identifier of the ProcessRequest</b><br> 1688 * Type: <b>token</b><br> 1689 * Path: <b>ProcessRequest.identifier</b><br> 1690 * </p> 1691 */ 1692 @SearchParamDefinition(name="identifier", path="ProcessRequest.identifier", description="The business identifier of the ProcessRequest", type="token" ) 1693 public static final String SP_IDENTIFIER = "identifier"; 1694 /** 1695 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1696 * <p> 1697 * Description: <b>The business identifier of the ProcessRequest</b><br> 1698 * Type: <b>token</b><br> 1699 * Path: <b>ProcessRequest.identifier</b><br> 1700 * </p> 1701 */ 1702 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1703 1704 /** 1705 * Search parameter: <b>provider</b> 1706 * <p> 1707 * Description: <b>The provider who regenerated this request</b><br> 1708 * Type: <b>reference</b><br> 1709 * Path: <b>ProcessRequest.provider</b><br> 1710 * </p> 1711 */ 1712 @SearchParamDefinition(name="provider", path="ProcessRequest.provider", description="The provider who regenerated this request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1713 public static final String SP_PROVIDER = "provider"; 1714 /** 1715 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 1716 * <p> 1717 * Description: <b>The provider who regenerated this request</b><br> 1718 * Type: <b>reference</b><br> 1719 * Path: <b>ProcessRequest.provider</b><br> 1720 * </p> 1721 */ 1722 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 1723 1724/** 1725 * Constant for fluent queries to be used to add include statements. Specifies 1726 * the path value of "<b>ProcessRequest:provider</b>". 1727 */ 1728 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("ProcessRequest:provider").toLocked(); 1729 1730 /** 1731 * Search parameter: <b>organization</b> 1732 * <p> 1733 * Description: <b>The organization who generated this request</b><br> 1734 * Type: <b>reference</b><br> 1735 * Path: <b>ProcessRequest.organization</b><br> 1736 * </p> 1737 */ 1738 @SearchParamDefinition(name="organization", path="ProcessRequest.organization", description="The organization who generated this request", type="reference", target={Organization.class } ) 1739 public static final String SP_ORGANIZATION = "organization"; 1740 /** 1741 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1742 * <p> 1743 * Description: <b>The organization who generated this request</b><br> 1744 * Type: <b>reference</b><br> 1745 * Path: <b>ProcessRequest.organization</b><br> 1746 * </p> 1747 */ 1748 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1749 1750/** 1751 * Constant for fluent queries to be used to add include statements. Specifies 1752 * the path value of "<b>ProcessRequest:organization</b>". 1753 */ 1754 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ProcessRequest:organization").toLocked(); 1755 1756 /** 1757 * Search parameter: <b>action</b> 1758 * <p> 1759 * Description: <b>The action requested by this resource</b><br> 1760 * Type: <b>token</b><br> 1761 * Path: <b>ProcessRequest.action</b><br> 1762 * </p> 1763 */ 1764 @SearchParamDefinition(name="action", path="ProcessRequest.action", description="The action requested by this resource", type="token" ) 1765 public static final String SP_ACTION = "action"; 1766 /** 1767 * <b>Fluent Client</b> search parameter constant for <b>action</b> 1768 * <p> 1769 * Description: <b>The action requested by this resource</b><br> 1770 * Type: <b>token</b><br> 1771 * Path: <b>ProcessRequest.action</b><br> 1772 * </p> 1773 */ 1774 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 1775 1776 1777}