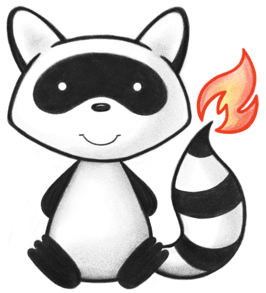
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * This resource provides processing status, errors and notes from the processing of a resource. 050 */ 051@ResourceDef(name="ProcessResponse", profile="http://hl7.org/fhir/Profile/ProcessResponse") 052public class ProcessResponse extends DomainResource { 053 054 public enum ProcessResponseStatus { 055 /** 056 * The instance is currently in-force. 057 */ 058 ACTIVE, 059 /** 060 * The instance is withdrawn, rescinded or reversed. 061 */ 062 CANCELLED, 063 /** 064 * A new instance the contents of which is not complete. 065 */ 066 DRAFT, 067 /** 068 * The instance was entered in error. 069 */ 070 ENTEREDINERROR, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static ProcessResponseStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("active".equals(codeString)) 079 return ACTIVE; 080 if ("cancelled".equals(codeString)) 081 return CANCELLED; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown ProcessResponseStatus code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case ACTIVE: return "active"; 094 case CANCELLED: return "cancelled"; 095 case DRAFT: return "draft"; 096 case ENTEREDINERROR: return "entered-in-error"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 104 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 105 case DRAFT: return "http://hl7.org/fhir/fm-status"; 106 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case ACTIVE: return "The instance is currently in-force."; 114 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 115 case DRAFT: return "A new instance the contents of which is not complete."; 116 case ENTEREDINERROR: return "The instance was entered in error."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case ACTIVE: return "Active"; 124 case CANCELLED: return "Cancelled"; 125 case DRAFT: return "Draft"; 126 case ENTEREDINERROR: return "Entered in Error"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class ProcessResponseStatusEnumFactory implements EnumFactory<ProcessResponseStatus> { 134 public ProcessResponseStatus fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("active".equals(codeString)) 139 return ProcessResponseStatus.ACTIVE; 140 if ("cancelled".equals(codeString)) 141 return ProcessResponseStatus.CANCELLED; 142 if ("draft".equals(codeString)) 143 return ProcessResponseStatus.DRAFT; 144 if ("entered-in-error".equals(codeString)) 145 return ProcessResponseStatus.ENTEREDINERROR; 146 throw new IllegalArgumentException("Unknown ProcessResponseStatus code '"+codeString+"'"); 147 } 148 public Enumeration<ProcessResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<ProcessResponseStatus>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("active".equals(codeString)) 157 return new Enumeration<ProcessResponseStatus>(this, ProcessResponseStatus.ACTIVE); 158 if ("cancelled".equals(codeString)) 159 return new Enumeration<ProcessResponseStatus>(this, ProcessResponseStatus.CANCELLED); 160 if ("draft".equals(codeString)) 161 return new Enumeration<ProcessResponseStatus>(this, ProcessResponseStatus.DRAFT); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<ProcessResponseStatus>(this, ProcessResponseStatus.ENTEREDINERROR); 164 throw new FHIRException("Unknown ProcessResponseStatus code '"+codeString+"'"); 165 } 166 public String toCode(ProcessResponseStatus code) { 167 if (code == ProcessResponseStatus.NULL) 168 return null; 169 if (code == ProcessResponseStatus.ACTIVE) 170 return "active"; 171 if (code == ProcessResponseStatus.CANCELLED) 172 return "cancelled"; 173 if (code == ProcessResponseStatus.DRAFT) 174 return "draft"; 175 if (code == ProcessResponseStatus.ENTEREDINERROR) 176 return "entered-in-error"; 177 return "?"; 178 } 179 public String toSystem(ProcessResponseStatus code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class ProcessResponseProcessNoteComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * The note purpose: Print/Display. 188 */ 189 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="display | print | printoper", formalDefinition="The note purpose: Print/Display." ) 191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 192 protected CodeableConcept type; 193 194 /** 195 * The note text. 196 */ 197 @Child(name = "text", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="Comment on the processing", formalDefinition="The note text." ) 199 protected StringType text; 200 201 private static final long serialVersionUID = 874830709L; 202 203 /** 204 * Constructor 205 */ 206 public ProcessResponseProcessNoteComponent() { 207 super(); 208 } 209 210 /** 211 * @return {@link #type} (The note purpose: Print/Display.) 212 */ 213 public CodeableConcept getType() { 214 if (this.type == null) 215 if (Configuration.errorOnAutoCreate()) 216 throw new Error("Attempt to auto-create ProcessResponseProcessNoteComponent.type"); 217 else if (Configuration.doAutoCreate()) 218 this.type = new CodeableConcept(); // cc 219 return this.type; 220 } 221 222 public boolean hasType() { 223 return this.type != null && !this.type.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #type} (The note purpose: Print/Display.) 228 */ 229 public ProcessResponseProcessNoteComponent setType(CodeableConcept value) { 230 this.type = value; 231 return this; 232 } 233 234 /** 235 * @return {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 236 */ 237 public StringType getTextElement() { 238 if (this.text == null) 239 if (Configuration.errorOnAutoCreate()) 240 throw new Error("Attempt to auto-create ProcessResponseProcessNoteComponent.text"); 241 else if (Configuration.doAutoCreate()) 242 this.text = new StringType(); // bb 243 return this.text; 244 } 245 246 public boolean hasTextElement() { 247 return this.text != null && !this.text.isEmpty(); 248 } 249 250 public boolean hasText() { 251 return this.text != null && !this.text.isEmpty(); 252 } 253 254 /** 255 * @param value {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 256 */ 257 public ProcessResponseProcessNoteComponent setTextElement(StringType value) { 258 this.text = value; 259 return this; 260 } 261 262 /** 263 * @return The note text. 264 */ 265 public String getText() { 266 return this.text == null ? null : this.text.getValue(); 267 } 268 269 /** 270 * @param value The note text. 271 */ 272 public ProcessResponseProcessNoteComponent setText(String value) { 273 if (Utilities.noString(value)) 274 this.text = null; 275 else { 276 if (this.text == null) 277 this.text = new StringType(); 278 this.text.setValue(value); 279 } 280 return this; 281 } 282 283 protected void listChildren(List<Property> children) { 284 super.listChildren(children); 285 children.add(new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type)); 286 children.add(new Property("text", "string", "The note text.", 0, 1, text)); 287 } 288 289 @Override 290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 291 switch (_hash) { 292 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type); 293 case 3556653: /*text*/ return new Property("text", "string", "The note text.", 0, 1, text); 294 default: return super.getNamedProperty(_hash, _name, _checkValid); 295 } 296 297 } 298 299 @Override 300 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 301 switch (hash) { 302 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 303 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 304 default: return super.getProperty(hash, name, checkValid); 305 } 306 307 } 308 309 @Override 310 public Base setProperty(int hash, String name, Base value) throws FHIRException { 311 switch (hash) { 312 case 3575610: // type 313 this.type = castToCodeableConcept(value); // CodeableConcept 314 return value; 315 case 3556653: // text 316 this.text = castToString(value); // StringType 317 return value; 318 default: return super.setProperty(hash, name, value); 319 } 320 321 } 322 323 @Override 324 public Base setProperty(String name, Base value) throws FHIRException { 325 if (name.equals("type")) { 326 this.type = castToCodeableConcept(value); // CodeableConcept 327 } else if (name.equals("text")) { 328 this.text = castToString(value); // StringType 329 } else 330 return super.setProperty(name, value); 331 return value; 332 } 333 334 @Override 335 public Base makeProperty(int hash, String name) throws FHIRException { 336 switch (hash) { 337 case 3575610: return getType(); 338 case 3556653: return getTextElement(); 339 default: return super.makeProperty(hash, name); 340 } 341 342 } 343 344 @Override 345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 346 switch (hash) { 347 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 348 case 3556653: /*text*/ return new String[] {"string"}; 349 default: return super.getTypesForProperty(hash, name); 350 } 351 352 } 353 354 @Override 355 public Base addChild(String name) throws FHIRException { 356 if (name.equals("type")) { 357 this.type = new CodeableConcept(); 358 return this.type; 359 } 360 else if (name.equals("text")) { 361 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.text"); 362 } 363 else 364 return super.addChild(name); 365 } 366 367 public ProcessResponseProcessNoteComponent copy() { 368 ProcessResponseProcessNoteComponent dst = new ProcessResponseProcessNoteComponent(); 369 copyValues(dst); 370 dst.type = type == null ? null : type.copy(); 371 dst.text = text == null ? null : text.copy(); 372 return dst; 373 } 374 375 @Override 376 public boolean equalsDeep(Base other_) { 377 if (!super.equalsDeep(other_)) 378 return false; 379 if (!(other_ instanceof ProcessResponseProcessNoteComponent)) 380 return false; 381 ProcessResponseProcessNoteComponent o = (ProcessResponseProcessNoteComponent) other_; 382 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 383 } 384 385 @Override 386 public boolean equalsShallow(Base other_) { 387 if (!super.equalsShallow(other_)) 388 return false; 389 if (!(other_ instanceof ProcessResponseProcessNoteComponent)) 390 return false; 391 ProcessResponseProcessNoteComponent o = (ProcessResponseProcessNoteComponent) other_; 392 return compareValues(text, o.text, true); 393 } 394 395 public boolean isEmpty() { 396 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, text); 397 } 398 399 public String fhirType() { 400 return "ProcessResponse.processNote"; 401 402 } 403 404 } 405 406 /** 407 * The Response business identifier. 408 */ 409 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 410 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 411 protected List<Identifier> identifier; 412 413 /** 414 * The status of the resource instance. 415 */ 416 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 417 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 419 protected Enumeration<ProcessResponseStatus> status; 420 421 /** 422 * The date when the enclosed suite of services were performed or completed. 423 */ 424 @Child(name = "created", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 425 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 426 protected DateTimeType created; 427 428 /** 429 * The organization who produced this adjudicated response. 430 */ 431 @Child(name = "organization", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 432 @Description(shortDefinition="Authoring Organization", formalDefinition="The organization who produced this adjudicated response." ) 433 protected Reference organization; 434 435 /** 436 * The actual object that is the target of the reference (The organization who produced this adjudicated response.) 437 */ 438 protected Organization organizationTarget; 439 440 /** 441 * Original request resource reference. 442 */ 443 @Child(name = "request", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 444 @Description(shortDefinition="Request reference", formalDefinition="Original request resource reference." ) 445 protected Reference request; 446 447 /** 448 * The actual object that is the target of the reference (Original request resource reference.) 449 */ 450 protected Resource requestTarget; 451 452 /** 453 * Transaction status: error, complete, held. 454 */ 455 @Child(name = "outcome", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 456 @Description(shortDefinition="Processing outcome", formalDefinition="Transaction status: error, complete, held." ) 457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-outcome") 458 protected CodeableConcept outcome; 459 460 /** 461 * A description of the status of the adjudication or processing. 462 */ 463 @Child(name = "disposition", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 464 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication or processing." ) 465 protected StringType disposition; 466 467 /** 468 * The practitioner who is responsible for the services rendered to the patient. 469 */ 470 @Child(name = "requestProvider", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 471 @Description(shortDefinition="Responsible Practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 472 protected Reference requestProvider; 473 474 /** 475 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 476 */ 477 protected Practitioner requestProviderTarget; 478 479 /** 480 * The organization which is responsible for the services rendered to the patient. 481 */ 482 @Child(name = "requestOrganization", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 483 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 484 protected Reference requestOrganization; 485 486 /** 487 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 488 */ 489 protected Organization requestOrganizationTarget; 490 491 /** 492 * The form to be used for printing the content. 493 */ 494 @Child(name = "form", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 495 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 496 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 497 protected CodeableConcept form; 498 499 /** 500 * Suite of processing notes or additional requirements if the processing has been held. 501 */ 502 @Child(name = "processNote", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 503 @Description(shortDefinition="Processing comments or additional requirements", formalDefinition="Suite of processing notes or additional requirements if the processing has been held." ) 504 protected List<ProcessResponseProcessNoteComponent> processNote; 505 506 /** 507 * Processing errors. 508 */ 509 @Child(name = "error", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 510 @Description(shortDefinition="Error code", formalDefinition="Processing errors." ) 511 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-error") 512 protected List<CodeableConcept> error; 513 514 /** 515 * Request for additional supporting or authorizing information, such as: documents, images or resources. 516 */ 517 @Child(name = "communicationRequest", type = {CommunicationRequest.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 518 @Description(shortDefinition="Request for additional information", formalDefinition="Request for additional supporting or authorizing information, such as: documents, images or resources." ) 519 protected List<Reference> communicationRequest; 520 /** 521 * The actual objects that are the target of the reference (Request for additional supporting or authorizing information, such as: documents, images or resources.) 522 */ 523 protected List<CommunicationRequest> communicationRequestTarget; 524 525 526 private static final long serialVersionUID = -2058462467L; 527 528 /** 529 * Constructor 530 */ 531 public ProcessResponse() { 532 super(); 533 } 534 535 /** 536 * @return {@link #identifier} (The Response business identifier.) 537 */ 538 public List<Identifier> getIdentifier() { 539 if (this.identifier == null) 540 this.identifier = new ArrayList<Identifier>(); 541 return this.identifier; 542 } 543 544 /** 545 * @return Returns a reference to <code>this</code> for easy method chaining 546 */ 547 public ProcessResponse setIdentifier(List<Identifier> theIdentifier) { 548 this.identifier = theIdentifier; 549 return this; 550 } 551 552 public boolean hasIdentifier() { 553 if (this.identifier == null) 554 return false; 555 for (Identifier item : this.identifier) 556 if (!item.isEmpty()) 557 return true; 558 return false; 559 } 560 561 public Identifier addIdentifier() { //3 562 Identifier t = new Identifier(); 563 if (this.identifier == null) 564 this.identifier = new ArrayList<Identifier>(); 565 this.identifier.add(t); 566 return t; 567 } 568 569 public ProcessResponse addIdentifier(Identifier t) { //3 570 if (t == null) 571 return this; 572 if (this.identifier == null) 573 this.identifier = new ArrayList<Identifier>(); 574 this.identifier.add(t); 575 return this; 576 } 577 578 /** 579 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 580 */ 581 public Identifier getIdentifierFirstRep() { 582 if (getIdentifier().isEmpty()) { 583 addIdentifier(); 584 } 585 return getIdentifier().get(0); 586 } 587 588 /** 589 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 590 */ 591 public Enumeration<ProcessResponseStatus> getStatusElement() { 592 if (this.status == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create ProcessResponse.status"); 595 else if (Configuration.doAutoCreate()) 596 this.status = new Enumeration<ProcessResponseStatus>(new ProcessResponseStatusEnumFactory()); // bb 597 return this.status; 598 } 599 600 public boolean hasStatusElement() { 601 return this.status != null && !this.status.isEmpty(); 602 } 603 604 public boolean hasStatus() { 605 return this.status != null && !this.status.isEmpty(); 606 } 607 608 /** 609 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 610 */ 611 public ProcessResponse setStatusElement(Enumeration<ProcessResponseStatus> value) { 612 this.status = value; 613 return this; 614 } 615 616 /** 617 * @return The status of the resource instance. 618 */ 619 public ProcessResponseStatus getStatus() { 620 return this.status == null ? null : this.status.getValue(); 621 } 622 623 /** 624 * @param value The status of the resource instance. 625 */ 626 public ProcessResponse setStatus(ProcessResponseStatus value) { 627 if (value == null) 628 this.status = null; 629 else { 630 if (this.status == null) 631 this.status = new Enumeration<ProcessResponseStatus>(new ProcessResponseStatusEnumFactory()); 632 this.status.setValue(value); 633 } 634 return this; 635 } 636 637 /** 638 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 639 */ 640 public DateTimeType getCreatedElement() { 641 if (this.created == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error("Attempt to auto-create ProcessResponse.created"); 644 else if (Configuration.doAutoCreate()) 645 this.created = new DateTimeType(); // bb 646 return this.created; 647 } 648 649 public boolean hasCreatedElement() { 650 return this.created != null && !this.created.isEmpty(); 651 } 652 653 public boolean hasCreated() { 654 return this.created != null && !this.created.isEmpty(); 655 } 656 657 /** 658 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 659 */ 660 public ProcessResponse setCreatedElement(DateTimeType value) { 661 this.created = value; 662 return this; 663 } 664 665 /** 666 * @return The date when the enclosed suite of services were performed or completed. 667 */ 668 public Date getCreated() { 669 return this.created == null ? null : this.created.getValue(); 670 } 671 672 /** 673 * @param value The date when the enclosed suite of services were performed or completed. 674 */ 675 public ProcessResponse setCreated(Date value) { 676 if (value == null) 677 this.created = null; 678 else { 679 if (this.created == null) 680 this.created = new DateTimeType(); 681 this.created.setValue(value); 682 } 683 return this; 684 } 685 686 /** 687 * @return {@link #organization} (The organization who produced this adjudicated response.) 688 */ 689 public Reference getOrganization() { 690 if (this.organization == null) 691 if (Configuration.errorOnAutoCreate()) 692 throw new Error("Attempt to auto-create ProcessResponse.organization"); 693 else if (Configuration.doAutoCreate()) 694 this.organization = new Reference(); // cc 695 return this.organization; 696 } 697 698 public boolean hasOrganization() { 699 return this.organization != null && !this.organization.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #organization} (The organization who produced this adjudicated response.) 704 */ 705 public ProcessResponse setOrganization(Reference value) { 706 this.organization = value; 707 return this; 708 } 709 710 /** 711 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization who produced this adjudicated response.) 712 */ 713 public Organization getOrganizationTarget() { 714 if (this.organizationTarget == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create ProcessResponse.organization"); 717 else if (Configuration.doAutoCreate()) 718 this.organizationTarget = new Organization(); // aa 719 return this.organizationTarget; 720 } 721 722 /** 723 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization who produced this adjudicated response.) 724 */ 725 public ProcessResponse setOrganizationTarget(Organization value) { 726 this.organizationTarget = value; 727 return this; 728 } 729 730 /** 731 * @return {@link #request} (Original request resource reference.) 732 */ 733 public Reference getRequest() { 734 if (this.request == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create ProcessResponse.request"); 737 else if (Configuration.doAutoCreate()) 738 this.request = new Reference(); // cc 739 return this.request; 740 } 741 742 public boolean hasRequest() { 743 return this.request != null && !this.request.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #request} (Original request resource reference.) 748 */ 749 public ProcessResponse setRequest(Reference value) { 750 this.request = value; 751 return this; 752 } 753 754 /** 755 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 756 */ 757 public Resource getRequestTarget() { 758 return this.requestTarget; 759 } 760 761 /** 762 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 763 */ 764 public ProcessResponse setRequestTarget(Resource value) { 765 this.requestTarget = value; 766 return this; 767 } 768 769 /** 770 * @return {@link #outcome} (Transaction status: error, complete, held.) 771 */ 772 public CodeableConcept getOutcome() { 773 if (this.outcome == null) 774 if (Configuration.errorOnAutoCreate()) 775 throw new Error("Attempt to auto-create ProcessResponse.outcome"); 776 else if (Configuration.doAutoCreate()) 777 this.outcome = new CodeableConcept(); // cc 778 return this.outcome; 779 } 780 781 public boolean hasOutcome() { 782 return this.outcome != null && !this.outcome.isEmpty(); 783 } 784 785 /** 786 * @param value {@link #outcome} (Transaction status: error, complete, held.) 787 */ 788 public ProcessResponse setOutcome(CodeableConcept value) { 789 this.outcome = value; 790 return this; 791 } 792 793 /** 794 * @return {@link #disposition} (A description of the status of the adjudication or processing.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 795 */ 796 public StringType getDispositionElement() { 797 if (this.disposition == null) 798 if (Configuration.errorOnAutoCreate()) 799 throw new Error("Attempt to auto-create ProcessResponse.disposition"); 800 else if (Configuration.doAutoCreate()) 801 this.disposition = new StringType(); // bb 802 return this.disposition; 803 } 804 805 public boolean hasDispositionElement() { 806 return this.disposition != null && !this.disposition.isEmpty(); 807 } 808 809 public boolean hasDisposition() { 810 return this.disposition != null && !this.disposition.isEmpty(); 811 } 812 813 /** 814 * @param value {@link #disposition} (A description of the status of the adjudication or processing.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 815 */ 816 public ProcessResponse setDispositionElement(StringType value) { 817 this.disposition = value; 818 return this; 819 } 820 821 /** 822 * @return A description of the status of the adjudication or processing. 823 */ 824 public String getDisposition() { 825 return this.disposition == null ? null : this.disposition.getValue(); 826 } 827 828 /** 829 * @param value A description of the status of the adjudication or processing. 830 */ 831 public ProcessResponse setDisposition(String value) { 832 if (Utilities.noString(value)) 833 this.disposition = null; 834 else { 835 if (this.disposition == null) 836 this.disposition = new StringType(); 837 this.disposition.setValue(value); 838 } 839 return this; 840 } 841 842 /** 843 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 844 */ 845 public Reference getRequestProvider() { 846 if (this.requestProvider == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create ProcessResponse.requestProvider"); 849 else if (Configuration.doAutoCreate()) 850 this.requestProvider = new Reference(); // cc 851 return this.requestProvider; 852 } 853 854 public boolean hasRequestProvider() { 855 return this.requestProvider != null && !this.requestProvider.isEmpty(); 856 } 857 858 /** 859 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 860 */ 861 public ProcessResponse setRequestProvider(Reference value) { 862 this.requestProvider = value; 863 return this; 864 } 865 866 /** 867 * @return {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 868 */ 869 public Practitioner getRequestProviderTarget() { 870 if (this.requestProviderTarget == null) 871 if (Configuration.errorOnAutoCreate()) 872 throw new Error("Attempt to auto-create ProcessResponse.requestProvider"); 873 else if (Configuration.doAutoCreate()) 874 this.requestProviderTarget = new Practitioner(); // aa 875 return this.requestProviderTarget; 876 } 877 878 /** 879 * @param value {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 880 */ 881 public ProcessResponse setRequestProviderTarget(Practitioner value) { 882 this.requestProviderTarget = value; 883 return this; 884 } 885 886 /** 887 * @return {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 888 */ 889 public Reference getRequestOrganization() { 890 if (this.requestOrganization == null) 891 if (Configuration.errorOnAutoCreate()) 892 throw new Error("Attempt to auto-create ProcessResponse.requestOrganization"); 893 else if (Configuration.doAutoCreate()) 894 this.requestOrganization = new Reference(); // cc 895 return this.requestOrganization; 896 } 897 898 public boolean hasRequestOrganization() { 899 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 904 */ 905 public ProcessResponse setRequestOrganization(Reference value) { 906 this.requestOrganization = value; 907 return this; 908 } 909 910 /** 911 * @return {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 912 */ 913 public Organization getRequestOrganizationTarget() { 914 if (this.requestOrganizationTarget == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create ProcessResponse.requestOrganization"); 917 else if (Configuration.doAutoCreate()) 918 this.requestOrganizationTarget = new Organization(); // aa 919 return this.requestOrganizationTarget; 920 } 921 922 /** 923 * @param value {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 924 */ 925 public ProcessResponse setRequestOrganizationTarget(Organization value) { 926 this.requestOrganizationTarget = value; 927 return this; 928 } 929 930 /** 931 * @return {@link #form} (The form to be used for printing the content.) 932 */ 933 public CodeableConcept getForm() { 934 if (this.form == null) 935 if (Configuration.errorOnAutoCreate()) 936 throw new Error("Attempt to auto-create ProcessResponse.form"); 937 else if (Configuration.doAutoCreate()) 938 this.form = new CodeableConcept(); // cc 939 return this.form; 940 } 941 942 public boolean hasForm() { 943 return this.form != null && !this.form.isEmpty(); 944 } 945 946 /** 947 * @param value {@link #form} (The form to be used for printing the content.) 948 */ 949 public ProcessResponse setForm(CodeableConcept value) { 950 this.form = value; 951 return this; 952 } 953 954 /** 955 * @return {@link #processNote} (Suite of processing notes or additional requirements if the processing has been held.) 956 */ 957 public List<ProcessResponseProcessNoteComponent> getProcessNote() { 958 if (this.processNote == null) 959 this.processNote = new ArrayList<ProcessResponseProcessNoteComponent>(); 960 return this.processNote; 961 } 962 963 /** 964 * @return Returns a reference to <code>this</code> for easy method chaining 965 */ 966 public ProcessResponse setProcessNote(List<ProcessResponseProcessNoteComponent> theProcessNote) { 967 this.processNote = theProcessNote; 968 return this; 969 } 970 971 public boolean hasProcessNote() { 972 if (this.processNote == null) 973 return false; 974 for (ProcessResponseProcessNoteComponent item : this.processNote) 975 if (!item.isEmpty()) 976 return true; 977 return false; 978 } 979 980 public ProcessResponseProcessNoteComponent addProcessNote() { //3 981 ProcessResponseProcessNoteComponent t = new ProcessResponseProcessNoteComponent(); 982 if (this.processNote == null) 983 this.processNote = new ArrayList<ProcessResponseProcessNoteComponent>(); 984 this.processNote.add(t); 985 return t; 986 } 987 988 public ProcessResponse addProcessNote(ProcessResponseProcessNoteComponent t) { //3 989 if (t == null) 990 return this; 991 if (this.processNote == null) 992 this.processNote = new ArrayList<ProcessResponseProcessNoteComponent>(); 993 this.processNote.add(t); 994 return this; 995 } 996 997 /** 998 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist 999 */ 1000 public ProcessResponseProcessNoteComponent getProcessNoteFirstRep() { 1001 if (getProcessNote().isEmpty()) { 1002 addProcessNote(); 1003 } 1004 return getProcessNote().get(0); 1005 } 1006 1007 /** 1008 * @return {@link #error} (Processing errors.) 1009 */ 1010 public List<CodeableConcept> getError() { 1011 if (this.error == null) 1012 this.error = new ArrayList<CodeableConcept>(); 1013 return this.error; 1014 } 1015 1016 /** 1017 * @return Returns a reference to <code>this</code> for easy method chaining 1018 */ 1019 public ProcessResponse setError(List<CodeableConcept> theError) { 1020 this.error = theError; 1021 return this; 1022 } 1023 1024 public boolean hasError() { 1025 if (this.error == null) 1026 return false; 1027 for (CodeableConcept item : this.error) 1028 if (!item.isEmpty()) 1029 return true; 1030 return false; 1031 } 1032 1033 public CodeableConcept addError() { //3 1034 CodeableConcept t = new CodeableConcept(); 1035 if (this.error == null) 1036 this.error = new ArrayList<CodeableConcept>(); 1037 this.error.add(t); 1038 return t; 1039 } 1040 1041 public ProcessResponse addError(CodeableConcept t) { //3 1042 if (t == null) 1043 return this; 1044 if (this.error == null) 1045 this.error = new ArrayList<CodeableConcept>(); 1046 this.error.add(t); 1047 return this; 1048 } 1049 1050 /** 1051 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist 1052 */ 1053 public CodeableConcept getErrorFirstRep() { 1054 if (getError().isEmpty()) { 1055 addError(); 1056 } 1057 return getError().get(0); 1058 } 1059 1060 /** 1061 * @return {@link #communicationRequest} (Request for additional supporting or authorizing information, such as: documents, images or resources.) 1062 */ 1063 public List<Reference> getCommunicationRequest() { 1064 if (this.communicationRequest == null) 1065 this.communicationRequest = new ArrayList<Reference>(); 1066 return this.communicationRequest; 1067 } 1068 1069 /** 1070 * @return Returns a reference to <code>this</code> for easy method chaining 1071 */ 1072 public ProcessResponse setCommunicationRequest(List<Reference> theCommunicationRequest) { 1073 this.communicationRequest = theCommunicationRequest; 1074 return this; 1075 } 1076 1077 public boolean hasCommunicationRequest() { 1078 if (this.communicationRequest == null) 1079 return false; 1080 for (Reference item : this.communicationRequest) 1081 if (!item.isEmpty()) 1082 return true; 1083 return false; 1084 } 1085 1086 public Reference addCommunicationRequest() { //3 1087 Reference t = new Reference(); 1088 if (this.communicationRequest == null) 1089 this.communicationRequest = new ArrayList<Reference>(); 1090 this.communicationRequest.add(t); 1091 return t; 1092 } 1093 1094 public ProcessResponse addCommunicationRequest(Reference t) { //3 1095 if (t == null) 1096 return this; 1097 if (this.communicationRequest == null) 1098 this.communicationRequest = new ArrayList<Reference>(); 1099 this.communicationRequest.add(t); 1100 return this; 1101 } 1102 1103 /** 1104 * @return The first repetition of repeating field {@link #communicationRequest}, creating it if it does not already exist 1105 */ 1106 public Reference getCommunicationRequestFirstRep() { 1107 if (getCommunicationRequest().isEmpty()) { 1108 addCommunicationRequest(); 1109 } 1110 return getCommunicationRequest().get(0); 1111 } 1112 1113 protected void listChildren(List<Property> children) { 1114 super.listChildren(children); 1115 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1116 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 1117 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 1118 children.add(new Property("organization", "Reference(Organization)", "The organization who produced this adjudicated response.", 0, 1, organization)); 1119 children.add(new Property("request", "Reference(Any)", "Original request resource reference.", 0, 1, request)); 1120 children.add(new Property("outcome", "CodeableConcept", "Transaction status: error, complete, held.", 0, 1, outcome)); 1121 children.add(new Property("disposition", "string", "A description of the status of the adjudication or processing.", 0, 1, disposition)); 1122 children.add(new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 1123 children.add(new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization)); 1124 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 1125 children.add(new Property("processNote", "", "Suite of processing notes or additional requirements if the processing has been held.", 0, java.lang.Integer.MAX_VALUE, processNote)); 1126 children.add(new Property("error", "CodeableConcept", "Processing errors.", 0, java.lang.Integer.MAX_VALUE, error)); 1127 children.add(new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information, such as: documents, images or resources.", 0, java.lang.Integer.MAX_VALUE, communicationRequest)); 1128 } 1129 1130 @Override 1131 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1132 switch (_hash) { 1133 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1134 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 1135 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 1136 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization who produced this adjudicated response.", 0, 1, organization); 1137 case 1095692943: /*request*/ return new Property("request", "Reference(Any)", "Original request resource reference.", 0, 1, request); 1138 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Transaction status: error, complete, held.", 0, 1, outcome); 1139 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication or processing.", 0, 1, disposition); 1140 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 1141 case 599053666: /*requestOrganization*/ return new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization); 1142 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 1143 case 202339073: /*processNote*/ return new Property("processNote", "", "Suite of processing notes or additional requirements if the processing has been held.", 0, java.lang.Integer.MAX_VALUE, processNote); 1144 case 96784904: /*error*/ return new Property("error", "CodeableConcept", "Processing errors.", 0, java.lang.Integer.MAX_VALUE, error); 1145 case -2071896615: /*communicationRequest*/ return new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information, such as: documents, images or resources.", 0, java.lang.Integer.MAX_VALUE, communicationRequest); 1146 default: return super.getNamedProperty(_hash, _name, _checkValid); 1147 } 1148 1149 } 1150 1151 @Override 1152 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1153 switch (hash) { 1154 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1155 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ProcessResponseStatus> 1156 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1157 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1158 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 1159 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 1160 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 1161 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 1162 case 599053666: /*requestOrganization*/ return this.requestOrganization == null ? new Base[0] : new Base[] {this.requestOrganization}; // Reference 1163 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 1164 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // ProcessResponseProcessNoteComponent 1165 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // CodeableConcept 1166 case -2071896615: /*communicationRequest*/ return this.communicationRequest == null ? new Base[0] : this.communicationRequest.toArray(new Base[this.communicationRequest.size()]); // Reference 1167 default: return super.getProperty(hash, name, checkValid); 1168 } 1169 1170 } 1171 1172 @Override 1173 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1174 switch (hash) { 1175 case -1618432855: // identifier 1176 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1177 return value; 1178 case -892481550: // status 1179 value = new ProcessResponseStatusEnumFactory().fromType(castToCode(value)); 1180 this.status = (Enumeration) value; // Enumeration<ProcessResponseStatus> 1181 return value; 1182 case 1028554472: // created 1183 this.created = castToDateTime(value); // DateTimeType 1184 return value; 1185 case 1178922291: // organization 1186 this.organization = castToReference(value); // Reference 1187 return value; 1188 case 1095692943: // request 1189 this.request = castToReference(value); // Reference 1190 return value; 1191 case -1106507950: // outcome 1192 this.outcome = castToCodeableConcept(value); // CodeableConcept 1193 return value; 1194 case 583380919: // disposition 1195 this.disposition = castToString(value); // StringType 1196 return value; 1197 case 1601527200: // requestProvider 1198 this.requestProvider = castToReference(value); // Reference 1199 return value; 1200 case 599053666: // requestOrganization 1201 this.requestOrganization = castToReference(value); // Reference 1202 return value; 1203 case 3148996: // form 1204 this.form = castToCodeableConcept(value); // CodeableConcept 1205 return value; 1206 case 202339073: // processNote 1207 this.getProcessNote().add((ProcessResponseProcessNoteComponent) value); // ProcessResponseProcessNoteComponent 1208 return value; 1209 case 96784904: // error 1210 this.getError().add(castToCodeableConcept(value)); // CodeableConcept 1211 return value; 1212 case -2071896615: // communicationRequest 1213 this.getCommunicationRequest().add(castToReference(value)); // Reference 1214 return value; 1215 default: return super.setProperty(hash, name, value); 1216 } 1217 1218 } 1219 1220 @Override 1221 public Base setProperty(String name, Base value) throws FHIRException { 1222 if (name.equals("identifier")) { 1223 this.getIdentifier().add(castToIdentifier(value)); 1224 } else if (name.equals("status")) { 1225 value = new ProcessResponseStatusEnumFactory().fromType(castToCode(value)); 1226 this.status = (Enumeration) value; // Enumeration<ProcessResponseStatus> 1227 } else if (name.equals("created")) { 1228 this.created = castToDateTime(value); // DateTimeType 1229 } else if (name.equals("organization")) { 1230 this.organization = castToReference(value); // Reference 1231 } else if (name.equals("request")) { 1232 this.request = castToReference(value); // Reference 1233 } else if (name.equals("outcome")) { 1234 this.outcome = castToCodeableConcept(value); // CodeableConcept 1235 } else if (name.equals("disposition")) { 1236 this.disposition = castToString(value); // StringType 1237 } else if (name.equals("requestProvider")) { 1238 this.requestProvider = castToReference(value); // Reference 1239 } else if (name.equals("requestOrganization")) { 1240 this.requestOrganization = castToReference(value); // Reference 1241 } else if (name.equals("form")) { 1242 this.form = castToCodeableConcept(value); // CodeableConcept 1243 } else if (name.equals("processNote")) { 1244 this.getProcessNote().add((ProcessResponseProcessNoteComponent) value); 1245 } else if (name.equals("error")) { 1246 this.getError().add(castToCodeableConcept(value)); 1247 } else if (name.equals("communicationRequest")) { 1248 this.getCommunicationRequest().add(castToReference(value)); 1249 } else 1250 return super.setProperty(name, value); 1251 return value; 1252 } 1253 1254 @Override 1255 public Base makeProperty(int hash, String name) throws FHIRException { 1256 switch (hash) { 1257 case -1618432855: return addIdentifier(); 1258 case -892481550: return getStatusElement(); 1259 case 1028554472: return getCreatedElement(); 1260 case 1178922291: return getOrganization(); 1261 case 1095692943: return getRequest(); 1262 case -1106507950: return getOutcome(); 1263 case 583380919: return getDispositionElement(); 1264 case 1601527200: return getRequestProvider(); 1265 case 599053666: return getRequestOrganization(); 1266 case 3148996: return getForm(); 1267 case 202339073: return addProcessNote(); 1268 case 96784904: return addError(); 1269 case -2071896615: return addCommunicationRequest(); 1270 default: return super.makeProperty(hash, name); 1271 } 1272 1273 } 1274 1275 @Override 1276 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1277 switch (hash) { 1278 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1279 case -892481550: /*status*/ return new String[] {"code"}; 1280 case 1028554472: /*created*/ return new String[] {"dateTime"}; 1281 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1282 case 1095692943: /*request*/ return new String[] {"Reference"}; 1283 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 1284 case 583380919: /*disposition*/ return new String[] {"string"}; 1285 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 1286 case 599053666: /*requestOrganization*/ return new String[] {"Reference"}; 1287 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 1288 case 202339073: /*processNote*/ return new String[] {}; 1289 case 96784904: /*error*/ return new String[] {"CodeableConcept"}; 1290 case -2071896615: /*communicationRequest*/ return new String[] {"Reference"}; 1291 default: return super.getTypesForProperty(hash, name); 1292 } 1293 1294 } 1295 1296 @Override 1297 public Base addChild(String name) throws FHIRException { 1298 if (name.equals("identifier")) { 1299 return addIdentifier(); 1300 } 1301 else if (name.equals("status")) { 1302 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.status"); 1303 } 1304 else if (name.equals("created")) { 1305 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.created"); 1306 } 1307 else if (name.equals("organization")) { 1308 this.organization = new Reference(); 1309 return this.organization; 1310 } 1311 else if (name.equals("request")) { 1312 this.request = new Reference(); 1313 return this.request; 1314 } 1315 else if (name.equals("outcome")) { 1316 this.outcome = new CodeableConcept(); 1317 return this.outcome; 1318 } 1319 else if (name.equals("disposition")) { 1320 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.disposition"); 1321 } 1322 else if (name.equals("requestProvider")) { 1323 this.requestProvider = new Reference(); 1324 return this.requestProvider; 1325 } 1326 else if (name.equals("requestOrganization")) { 1327 this.requestOrganization = new Reference(); 1328 return this.requestOrganization; 1329 } 1330 else if (name.equals("form")) { 1331 this.form = new CodeableConcept(); 1332 return this.form; 1333 } 1334 else if (name.equals("processNote")) { 1335 return addProcessNote(); 1336 } 1337 else if (name.equals("error")) { 1338 return addError(); 1339 } 1340 else if (name.equals("communicationRequest")) { 1341 return addCommunicationRequest(); 1342 } 1343 else 1344 return super.addChild(name); 1345 } 1346 1347 public String fhirType() { 1348 return "ProcessResponse"; 1349 1350 } 1351 1352 public ProcessResponse copy() { 1353 ProcessResponse dst = new ProcessResponse(); 1354 copyValues(dst); 1355 if (identifier != null) { 1356 dst.identifier = new ArrayList<Identifier>(); 1357 for (Identifier i : identifier) 1358 dst.identifier.add(i.copy()); 1359 }; 1360 dst.status = status == null ? null : status.copy(); 1361 dst.created = created == null ? null : created.copy(); 1362 dst.organization = organization == null ? null : organization.copy(); 1363 dst.request = request == null ? null : request.copy(); 1364 dst.outcome = outcome == null ? null : outcome.copy(); 1365 dst.disposition = disposition == null ? null : disposition.copy(); 1366 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 1367 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 1368 dst.form = form == null ? null : form.copy(); 1369 if (processNote != null) { 1370 dst.processNote = new ArrayList<ProcessResponseProcessNoteComponent>(); 1371 for (ProcessResponseProcessNoteComponent i : processNote) 1372 dst.processNote.add(i.copy()); 1373 }; 1374 if (error != null) { 1375 dst.error = new ArrayList<CodeableConcept>(); 1376 for (CodeableConcept i : error) 1377 dst.error.add(i.copy()); 1378 }; 1379 if (communicationRequest != null) { 1380 dst.communicationRequest = new ArrayList<Reference>(); 1381 for (Reference i : communicationRequest) 1382 dst.communicationRequest.add(i.copy()); 1383 }; 1384 return dst; 1385 } 1386 1387 protected ProcessResponse typedCopy() { 1388 return copy(); 1389 } 1390 1391 @Override 1392 public boolean equalsDeep(Base other_) { 1393 if (!super.equalsDeep(other_)) 1394 return false; 1395 if (!(other_ instanceof ProcessResponse)) 1396 return false; 1397 ProcessResponse o = (ProcessResponse) other_; 1398 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(created, o.created, true) 1399 && compareDeep(organization, o.organization, true) && compareDeep(request, o.request, true) && compareDeep(outcome, o.outcome, true) 1400 && compareDeep(disposition, o.disposition, true) && compareDeep(requestProvider, o.requestProvider, true) 1401 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(form, o.form, true) 1402 && compareDeep(processNote, o.processNote, true) && compareDeep(error, o.error, true) && compareDeep(communicationRequest, o.communicationRequest, true) 1403 ; 1404 } 1405 1406 @Override 1407 public boolean equalsShallow(Base other_) { 1408 if (!super.equalsShallow(other_)) 1409 return false; 1410 if (!(other_ instanceof ProcessResponse)) 1411 return false; 1412 ProcessResponse o = (ProcessResponse) other_; 1413 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 1414 ; 1415 } 1416 1417 public boolean isEmpty() { 1418 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created 1419 , organization, request, outcome, disposition, requestProvider, requestOrganization 1420 , form, processNote, error, communicationRequest); 1421 } 1422 1423 @Override 1424 public ResourceType getResourceType() { 1425 return ResourceType.ProcessResponse; 1426 } 1427 1428 /** 1429 * Search parameter: <b>identifier</b> 1430 * <p> 1431 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 1432 * Type: <b>token</b><br> 1433 * Path: <b>ProcessResponse.identifier</b><br> 1434 * </p> 1435 */ 1436 @SearchParamDefinition(name="identifier", path="ProcessResponse.identifier", description="The business identifier of the Explanation of Benefit", type="token" ) 1437 public static final String SP_IDENTIFIER = "identifier"; 1438 /** 1439 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1440 * <p> 1441 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 1442 * Type: <b>token</b><br> 1443 * Path: <b>ProcessResponse.identifier</b><br> 1444 * </p> 1445 */ 1446 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1447 1448 /** 1449 * Search parameter: <b>request</b> 1450 * <p> 1451 * Description: <b>The reference to the claim</b><br> 1452 * Type: <b>reference</b><br> 1453 * Path: <b>ProcessResponse.request</b><br> 1454 * </p> 1455 */ 1456 @SearchParamDefinition(name="request", path="ProcessResponse.request", description="The reference to the claim", type="reference" ) 1457 public static final String SP_REQUEST = "request"; 1458 /** 1459 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1460 * <p> 1461 * Description: <b>The reference to the claim</b><br> 1462 * Type: <b>reference</b><br> 1463 * Path: <b>ProcessResponse.request</b><br> 1464 * </p> 1465 */ 1466 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 1467 1468/** 1469 * Constant for fluent queries to be used to add include statements. Specifies 1470 * the path value of "<b>ProcessResponse:request</b>". 1471 */ 1472 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("ProcessResponse:request").toLocked(); 1473 1474 /** 1475 * Search parameter: <b>organization</b> 1476 * <p> 1477 * Description: <b>The organization who generated this resource</b><br> 1478 * Type: <b>reference</b><br> 1479 * Path: <b>ProcessResponse.organization</b><br> 1480 * </p> 1481 */ 1482 @SearchParamDefinition(name="organization", path="ProcessResponse.organization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 1483 public static final String SP_ORGANIZATION = "organization"; 1484 /** 1485 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1486 * <p> 1487 * Description: <b>The organization who generated this resource</b><br> 1488 * Type: <b>reference</b><br> 1489 * Path: <b>ProcessResponse.organization</b><br> 1490 * </p> 1491 */ 1492 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1493 1494/** 1495 * Constant for fluent queries to be used to add include statements. Specifies 1496 * the path value of "<b>ProcessResponse:organization</b>". 1497 */ 1498 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ProcessResponse:organization").toLocked(); 1499 1500 /** 1501 * Search parameter: <b>request-organization</b> 1502 * <p> 1503 * Description: <b>The Organization who is responsible the request transaction</b><br> 1504 * Type: <b>reference</b><br> 1505 * Path: <b>ProcessResponse.requestOrganization</b><br> 1506 * </p> 1507 */ 1508 @SearchParamDefinition(name="request-organization", path="ProcessResponse.requestOrganization", description="The Organization who is responsible the request transaction", type="reference", target={Organization.class } ) 1509 public static final String SP_REQUEST_ORGANIZATION = "request-organization"; 1510 /** 1511 * <b>Fluent Client</b> search parameter constant for <b>request-organization</b> 1512 * <p> 1513 * Description: <b>The Organization who is responsible the request transaction</b><br> 1514 * Type: <b>reference</b><br> 1515 * Path: <b>ProcessResponse.requestOrganization</b><br> 1516 * </p> 1517 */ 1518 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_ORGANIZATION); 1519 1520/** 1521 * Constant for fluent queries to be used to add include statements. Specifies 1522 * the path value of "<b>ProcessResponse:request-organization</b>". 1523 */ 1524 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ProcessResponse:request-organization").toLocked(); 1525 1526 /** 1527 * Search parameter: <b>request-provider</b> 1528 * <p> 1529 * Description: <b>The Provider who is responsible the request transaction</b><br> 1530 * Type: <b>reference</b><br> 1531 * Path: <b>ProcessResponse.requestProvider</b><br> 1532 * </p> 1533 */ 1534 @SearchParamDefinition(name="request-provider", path="ProcessResponse.requestProvider", description="The Provider who is responsible the request transaction", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1535 public static final String SP_REQUEST_PROVIDER = "request-provider"; 1536 /** 1537 * <b>Fluent Client</b> search parameter constant for <b>request-provider</b> 1538 * <p> 1539 * Description: <b>The Provider who is responsible the request transaction</b><br> 1540 * Type: <b>reference</b><br> 1541 * Path: <b>ProcessResponse.requestProvider</b><br> 1542 * </p> 1543 */ 1544 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_PROVIDER); 1545 1546/** 1547 * Constant for fluent queries to be used to add include statements. Specifies 1548 * the path value of "<b>ProcessResponse:request-provider</b>". 1549 */ 1550 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_PROVIDER = new ca.uhn.fhir.model.api.Include("ProcessResponse:request-provider").toLocked(); 1551 1552 1553}