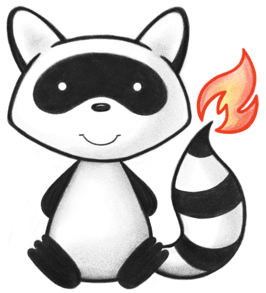
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.ArrayList; 035import java.util.List; 036 037/** 038 * A child element or property defined by the FHIR specification 039 * This class is defined as a helper class when iterating the 040 * children of an element in a generic fashion 041 * 042 * At present, iteration is only based on the specification, but 043 * this may be changed to allow profile based expression at a 044 * later date 045 * 046 * note: there's no point in creating one of these classes outside this package 047 */ 048public class Property { 049 050 /** 051 * The name of the property as found in the FHIR specification 052 */ 053 private String name; 054 055 /** 056 * The type of the property as specified in the FHIR specification (e.g. type|type|Reference(Name|Name) 057 */ 058 private String typeCode; 059 060 /** 061 * The formal definition of the element given in the FHIR specification 062 */ 063 private String definition; 064 065 /** 066 * The minimum allowed cardinality - 0 or 1 when based on the specification 067 */ 068 private int minCardinality; 069 070 /** 071 * The maximum allowed cardinality - 1 or MAX_INT when based on the specification 072 */ 073 private int maxCardinality; 074 075 /** 076 * The actual elements that exist on this instance 077 */ 078 private List<Base> values = new ArrayList<Base>(); 079 080 /** 081 * For run time, if/once a property is hooked up to it's definition 082 */ 083 private StructureDefinition structure; 084 085 /** 086 * Internal constructor 087 */ 088 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, Base value) { 089 super(); 090 this.name = name; 091 this.typeCode = typeCode; 092 this.definition = definition; 093 this.minCardinality = minCardinality; 094 this.maxCardinality = maxCardinality; 095 if (value != null) 096 this.values.add(value); 097 } 098 099 /** 100 * Internal constructor 101 */ 102 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, List<? extends Base> values) { 103 super(); 104 this.name = name; 105 this.typeCode = typeCode; 106 this.definition = definition; 107 this.minCardinality = minCardinality; 108 this.maxCardinality = maxCardinality; 109 if (values != null) 110 this.values.addAll(values); 111 } 112 113 /** 114 * @return The name of this property in the FHIR Specification 115 */ 116 public String getName() { 117 return name; 118 } 119 120 /** 121 * @return The stated type in the FHIR specification 122 */ 123 public String getTypeCode() { 124 return typeCode; 125 } 126 127 /** 128 * @return The definition of this element in the FHIR spec 129 */ 130 public String getDefinition() { 131 return definition; 132 } 133 134 /** 135 * @return the minimum cardinality for this element 136 */ 137 public int getMinCardinality() { 138 return minCardinality; 139 } 140 141 /** 142 * @return the maximum cardinality for this element 143 */ 144 public int getMaxCardinality() { 145 return maxCardinality; 146 } 147 148 /** 149 * @return the actual values - will only be 1 unless maximum cardinality == MAX_INT 150 */ 151 public List<Base> getValues() { 152 return values; 153 } 154 155 public boolean hasValues() { 156 for (Base e : getValues()) 157 if (e != null) 158 return true; 159 return false; 160 } 161 162 public StructureDefinition getStructure() { 163 return structure; 164 } 165 166 public void setStructure(StructureDefinition structure) { 167 this.structure = structure; 168 } 169 170 public boolean isList() { 171 return maxCardinality > 1; 172 } 173 174}