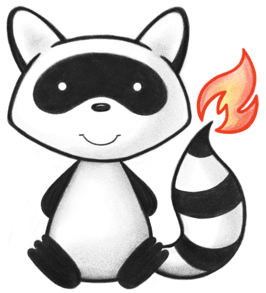
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 051 */ 052@ResourceDef(name="Provenance", profile="http://hl7.org/fhir/Profile/Provenance") 053public class Provenance extends DomainResource { 054 055 public enum ProvenanceEntityRole { 056 /** 057 * A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a preexisting entity. 058 */ 059 DERIVATION, 060 /** 061 * A derivation for which the resulting entity is a revised version of some original. 062 */ 063 REVISION, 064 /** 065 * The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author. 066 */ 067 QUOTATION, 068 /** 069 * A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight. 070 */ 071 SOURCE, 072 /** 073 * A derivation for which the entity is removed from accessibility usually through the use of the Delete operation. 074 */ 075 REMOVAL, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 public static ProvenanceEntityRole fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("derivation".equals(codeString)) 084 return DERIVATION; 085 if ("revision".equals(codeString)) 086 return REVISION; 087 if ("quotation".equals(codeString)) 088 return QUOTATION; 089 if ("source".equals(codeString)) 090 return SOURCE; 091 if ("removal".equals(codeString)) 092 return REMOVAL; 093 if (Configuration.isAcceptInvalidEnums()) 094 return null; 095 else 096 throw new FHIRException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 097 } 098 public String toCode() { 099 switch (this) { 100 case DERIVATION: return "derivation"; 101 case REVISION: return "revision"; 102 case QUOTATION: return "quotation"; 103 case SOURCE: return "source"; 104 case REMOVAL: return "removal"; 105 case NULL: return null; 106 default: return "?"; 107 } 108 } 109 public String getSystem() { 110 switch (this) { 111 case DERIVATION: return "http://hl7.org/fhir/provenance-entity-role"; 112 case REVISION: return "http://hl7.org/fhir/provenance-entity-role"; 113 case QUOTATION: return "http://hl7.org/fhir/provenance-entity-role"; 114 case SOURCE: return "http://hl7.org/fhir/provenance-entity-role"; 115 case REMOVAL: return "http://hl7.org/fhir/provenance-entity-role"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDefinition() { 121 switch (this) { 122 case DERIVATION: return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a preexisting entity."; 123 case REVISION: return "A derivation for which the resulting entity is a revised version of some original."; 124 case QUOTATION: return "The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author."; 125 case SOURCE: return "A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight."; 126 case REMOVAL: return "A derivation for which the entity is removed from accessibility usually through the use of the Delete operation."; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getDisplay() { 132 switch (this) { 133 case DERIVATION: return "Derivation"; 134 case REVISION: return "Revision"; 135 case QUOTATION: return "Quotation"; 136 case SOURCE: return "Source"; 137 case REMOVAL: return "Removal"; 138 case NULL: return null; 139 default: return "?"; 140 } 141 } 142 } 143 144 public static class ProvenanceEntityRoleEnumFactory implements EnumFactory<ProvenanceEntityRole> { 145 public ProvenanceEntityRole fromCode(String codeString) throws IllegalArgumentException { 146 if (codeString == null || "".equals(codeString)) 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("derivation".equals(codeString)) 150 return ProvenanceEntityRole.DERIVATION; 151 if ("revision".equals(codeString)) 152 return ProvenanceEntityRole.REVISION; 153 if ("quotation".equals(codeString)) 154 return ProvenanceEntityRole.QUOTATION; 155 if ("source".equals(codeString)) 156 return ProvenanceEntityRole.SOURCE; 157 if ("removal".equals(codeString)) 158 return ProvenanceEntityRole.REMOVAL; 159 throw new IllegalArgumentException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 160 } 161 public Enumeration<ProvenanceEntityRole> fromType(PrimitiveType<?> code) throws FHIRException { 162 if (code == null) 163 return null; 164 if (code.isEmpty()) 165 return new Enumeration<ProvenanceEntityRole>(this); 166 String codeString = code.asStringValue(); 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("derivation".equals(codeString)) 170 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.DERIVATION); 171 if ("revision".equals(codeString)) 172 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REVISION); 173 if ("quotation".equals(codeString)) 174 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.QUOTATION); 175 if ("source".equals(codeString)) 176 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.SOURCE); 177 if ("removal".equals(codeString)) 178 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REMOVAL); 179 throw new FHIRException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 180 } 181 public String toCode(ProvenanceEntityRole code) { 182 if (code == ProvenanceEntityRole.NULL) 183 return null; 184 if (code == ProvenanceEntityRole.DERIVATION) 185 return "derivation"; 186 if (code == ProvenanceEntityRole.REVISION) 187 return "revision"; 188 if (code == ProvenanceEntityRole.QUOTATION) 189 return "quotation"; 190 if (code == ProvenanceEntityRole.SOURCE) 191 return "source"; 192 if (code == ProvenanceEntityRole.REMOVAL) 193 return "removal"; 194 return "?"; 195 } 196 public String toSystem(ProvenanceEntityRole code) { 197 return code.getSystem(); 198 } 199 } 200 201 @Block() 202 public static class ProvenanceAgentComponent extends BackboneElement implements IBaseBackboneElement { 203 /** 204 * The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity. 205 */ 206 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 207 @Description(shortDefinition="What the agents role was", formalDefinition="The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity." ) 208 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 209 protected List<CodeableConcept> role; 210 211 /** 212 * The individual, device or organization that participated in the event. 213 */ 214 @Child(name = "who", type = {UriType.class, Practitioner.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=true) 215 @Description(shortDefinition="Who participated", formalDefinition="The individual, device or organization that participated in the event." ) 216 protected Type who; 217 218 /** 219 * The individual, device, or organization for whom the change was made. 220 */ 221 @Child(name = "onBehalfOf", type = {UriType.class, Practitioner.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Who the agent is representing", formalDefinition="The individual, device, or organization for whom the change was made." ) 223 protected Type onBehalfOf; 224 225 /** 226 * The type of relationship between agents. 227 */ 228 @Child(name = "relatedAgentType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="Type of relationship between agents", formalDefinition="The type of relationship between agents." ) 230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-RoleLinkType") 231 protected CodeableConcept relatedAgentType; 232 233 private static final long serialVersionUID = -1431948744L; 234 235 /** 236 * Constructor 237 */ 238 public ProvenanceAgentComponent() { 239 super(); 240 } 241 242 /** 243 * Constructor 244 */ 245 public ProvenanceAgentComponent(Type who) { 246 super(); 247 this.who = who; 248 } 249 250 /** 251 * @return {@link #role} (The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.) 252 */ 253 public List<CodeableConcept> getRole() { 254 if (this.role == null) 255 this.role = new ArrayList<CodeableConcept>(); 256 return this.role; 257 } 258 259 /** 260 * @return Returns a reference to <code>this</code> for easy method chaining 261 */ 262 public ProvenanceAgentComponent setRole(List<CodeableConcept> theRole) { 263 this.role = theRole; 264 return this; 265 } 266 267 public boolean hasRole() { 268 if (this.role == null) 269 return false; 270 for (CodeableConcept item : this.role) 271 if (!item.isEmpty()) 272 return true; 273 return false; 274 } 275 276 public CodeableConcept addRole() { //3 277 CodeableConcept t = new CodeableConcept(); 278 if (this.role == null) 279 this.role = new ArrayList<CodeableConcept>(); 280 this.role.add(t); 281 return t; 282 } 283 284 public ProvenanceAgentComponent addRole(CodeableConcept t) { //3 285 if (t == null) 286 return this; 287 if (this.role == null) 288 this.role = new ArrayList<CodeableConcept>(); 289 this.role.add(t); 290 return this; 291 } 292 293 /** 294 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist 295 */ 296 public CodeableConcept getRoleFirstRep() { 297 if (getRole().isEmpty()) { 298 addRole(); 299 } 300 return getRole().get(0); 301 } 302 303 /** 304 * @return {@link #who} (The individual, device or organization that participated in the event.) 305 */ 306 public Type getWho() { 307 return this.who; 308 } 309 310 /** 311 * @return {@link #who} (The individual, device or organization that participated in the event.) 312 */ 313 public UriType getWhoUriType() throws FHIRException { 314 if (this.who == null) 315 return null; 316 if (!(this.who instanceof UriType)) 317 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.who.getClass().getName()+" was encountered"); 318 return (UriType) this.who; 319 } 320 321 public boolean hasWhoUriType() { 322 return this != null && this.who instanceof UriType; 323 } 324 325 /** 326 * @return {@link #who} (The individual, device or organization that participated in the event.) 327 */ 328 public Reference getWhoReference() throws FHIRException { 329 if (this.who == null) 330 return null; 331 if (!(this.who instanceof Reference)) 332 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.who.getClass().getName()+" was encountered"); 333 return (Reference) this.who; 334 } 335 336 public boolean hasWhoReference() { 337 return this != null && this.who instanceof Reference; 338 } 339 340 public boolean hasWho() { 341 return this.who != null && !this.who.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #who} (The individual, device or organization that participated in the event.) 346 */ 347 public ProvenanceAgentComponent setWho(Type value) throws FHIRFormatError { 348 if (value != null && !(value instanceof UriType || value instanceof Reference)) 349 throw new FHIRFormatError("Not the right type for Provenance.agent.who[x]: "+value.fhirType()); 350 this.who = value; 351 return this; 352 } 353 354 /** 355 * @return {@link #onBehalfOf} (The individual, device, or organization for whom the change was made.) 356 */ 357 public Type getOnBehalfOf() { 358 return this.onBehalfOf; 359 } 360 361 /** 362 * @return {@link #onBehalfOf} (The individual, device, or organization for whom the change was made.) 363 */ 364 public UriType getOnBehalfOfUriType() throws FHIRException { 365 if (this.onBehalfOf == null) 366 return null; 367 if (!(this.onBehalfOf instanceof UriType)) 368 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.onBehalfOf.getClass().getName()+" was encountered"); 369 return (UriType) this.onBehalfOf; 370 } 371 372 public boolean hasOnBehalfOfUriType() { 373 return this != null && this.onBehalfOf instanceof UriType; 374 } 375 376 /** 377 * @return {@link #onBehalfOf} (The individual, device, or organization for whom the change was made.) 378 */ 379 public Reference getOnBehalfOfReference() throws FHIRException { 380 if (this.onBehalfOf == null) 381 return null; 382 if (!(this.onBehalfOf instanceof Reference)) 383 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.onBehalfOf.getClass().getName()+" was encountered"); 384 return (Reference) this.onBehalfOf; 385 } 386 387 public boolean hasOnBehalfOfReference() { 388 return this != null && this.onBehalfOf instanceof Reference; 389 } 390 391 public boolean hasOnBehalfOf() { 392 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 393 } 394 395 /** 396 * @param value {@link #onBehalfOf} (The individual, device, or organization for whom the change was made.) 397 */ 398 public ProvenanceAgentComponent setOnBehalfOf(Type value) throws FHIRFormatError { 399 if (value != null && !(value instanceof UriType || value instanceof Reference)) 400 throw new FHIRFormatError("Not the right type for Provenance.agent.onBehalfOf[x]: "+value.fhirType()); 401 this.onBehalfOf = value; 402 return this; 403 } 404 405 /** 406 * @return {@link #relatedAgentType} (The type of relationship between agents.) 407 */ 408 public CodeableConcept getRelatedAgentType() { 409 if (this.relatedAgentType == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create ProvenanceAgentComponent.relatedAgentType"); 412 else if (Configuration.doAutoCreate()) 413 this.relatedAgentType = new CodeableConcept(); // cc 414 return this.relatedAgentType; 415 } 416 417 public boolean hasRelatedAgentType() { 418 return this.relatedAgentType != null && !this.relatedAgentType.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #relatedAgentType} (The type of relationship between agents.) 423 */ 424 public ProvenanceAgentComponent setRelatedAgentType(CodeableConcept value) { 425 this.relatedAgentType = value; 426 return this; 427 } 428 429 protected void listChildren(List<Property> children) { 430 super.listChildren(children); 431 children.add(new Property("role", "CodeableConcept", "The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role)); 432 children.add(new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device or organization that participated in the event.", 0, 1, who)); 433 children.add(new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf)); 434 children.add(new Property("relatedAgentType", "CodeableConcept", "The type of relationship between agents.", 0, 1, relatedAgentType)); 435 } 436 437 @Override 438 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 439 switch (_hash) { 440 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The function of the agent with respect to the activity. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role); 441 case -788654078: /*who[x]*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device or organization that participated in the event.", 0, 1, who); 442 case 117694: /*who*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device or organization that participated in the event.", 0, 1, who); 443 case -788660018: /*whoUri*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device or organization that participated in the event.", 0, 1, who); 444 case 1017243949: /*whoReference*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device or organization that participated in the event.", 0, 1, who); 445 case 418120340: /*onBehalfOf[x]*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf); 446 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf); 447 case 418114400: /*onBehalfOfUri*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf); 448 case -1136255425: /*onBehalfOfReference*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "The individual, device, or organization for whom the change was made.", 0, 1, onBehalfOf); 449 case 1228161012: /*relatedAgentType*/ return new Property("relatedAgentType", "CodeableConcept", "The type of relationship between agents.", 0, 1, relatedAgentType); 450 default: return super.getNamedProperty(_hash, _name, _checkValid); 451 } 452 453 } 454 455 @Override 456 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 457 switch (hash) { 458 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 459 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Type 460 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Type 461 case 1228161012: /*relatedAgentType*/ return this.relatedAgentType == null ? new Base[0] : new Base[] {this.relatedAgentType}; // CodeableConcept 462 default: return super.getProperty(hash, name, checkValid); 463 } 464 465 } 466 467 @Override 468 public Base setProperty(int hash, String name, Base value) throws FHIRException { 469 switch (hash) { 470 case 3506294: // role 471 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 472 return value; 473 case 117694: // who 474 this.who = castToType(value); // Type 475 return value; 476 case -14402964: // onBehalfOf 477 this.onBehalfOf = castToType(value); // Type 478 return value; 479 case 1228161012: // relatedAgentType 480 this.relatedAgentType = castToCodeableConcept(value); // CodeableConcept 481 return value; 482 default: return super.setProperty(hash, name, value); 483 } 484 485 } 486 487 @Override 488 public Base setProperty(String name, Base value) throws FHIRException { 489 if (name.equals("role")) { 490 this.getRole().add(castToCodeableConcept(value)); 491 } else if (name.equals("who[x]")) { 492 this.who = castToType(value); // Type 493 } else if (name.equals("onBehalfOf[x]")) { 494 this.onBehalfOf = castToType(value); // Type 495 } else if (name.equals("relatedAgentType")) { 496 this.relatedAgentType = castToCodeableConcept(value); // CodeableConcept 497 } else 498 return super.setProperty(name, value); 499 return value; 500 } 501 502 @Override 503 public Base makeProperty(int hash, String name) throws FHIRException { 504 switch (hash) { 505 case 3506294: return addRole(); 506 case -788654078: return getWho(); 507 case 117694: return getWho(); 508 case 418120340: return getOnBehalfOf(); 509 case -14402964: return getOnBehalfOf(); 510 case 1228161012: return getRelatedAgentType(); 511 default: return super.makeProperty(hash, name); 512 } 513 514 } 515 516 @Override 517 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 518 switch (hash) { 519 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 520 case 117694: /*who*/ return new String[] {"uri", "Reference"}; 521 case -14402964: /*onBehalfOf*/ return new String[] {"uri", "Reference"}; 522 case 1228161012: /*relatedAgentType*/ return new String[] {"CodeableConcept"}; 523 default: return super.getTypesForProperty(hash, name); 524 } 525 526 } 527 528 @Override 529 public Base addChild(String name) throws FHIRException { 530 if (name.equals("role")) { 531 return addRole(); 532 } 533 else if (name.equals("whoUri")) { 534 this.who = new UriType(); 535 return this.who; 536 } 537 else if (name.equals("whoReference")) { 538 this.who = new Reference(); 539 return this.who; 540 } 541 else if (name.equals("onBehalfOfUri")) { 542 this.onBehalfOf = new UriType(); 543 return this.onBehalfOf; 544 } 545 else if (name.equals("onBehalfOfReference")) { 546 this.onBehalfOf = new Reference(); 547 return this.onBehalfOf; 548 } 549 else if (name.equals("relatedAgentType")) { 550 this.relatedAgentType = new CodeableConcept(); 551 return this.relatedAgentType; 552 } 553 else 554 return super.addChild(name); 555 } 556 557 public ProvenanceAgentComponent copy() { 558 ProvenanceAgentComponent dst = new ProvenanceAgentComponent(); 559 copyValues(dst); 560 if (role != null) { 561 dst.role = new ArrayList<CodeableConcept>(); 562 for (CodeableConcept i : role) 563 dst.role.add(i.copy()); 564 }; 565 dst.who = who == null ? null : who.copy(); 566 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 567 dst.relatedAgentType = relatedAgentType == null ? null : relatedAgentType.copy(); 568 return dst; 569 } 570 571 @Override 572 public boolean equalsDeep(Base other_) { 573 if (!super.equalsDeep(other_)) 574 return false; 575 if (!(other_ instanceof ProvenanceAgentComponent)) 576 return false; 577 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 578 return compareDeep(role, o.role, true) && compareDeep(who, o.who, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 579 && compareDeep(relatedAgentType, o.relatedAgentType, true); 580 } 581 582 @Override 583 public boolean equalsShallow(Base other_) { 584 if (!super.equalsShallow(other_)) 585 return false; 586 if (!(other_ instanceof ProvenanceAgentComponent)) 587 return false; 588 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other_; 589 return true; 590 } 591 592 public boolean isEmpty() { 593 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, who, onBehalfOf, relatedAgentType 594 ); 595 } 596 597 public String fhirType() { 598 return "Provenance.agent"; 599 600 } 601 602 } 603 604 @Block() 605 public static class ProvenanceEntityComponent extends BackboneElement implements IBaseBackboneElement { 606 /** 607 * How the entity was used during the activity. 608 */ 609 @Child(name = "role", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 610 @Description(shortDefinition="derivation | revision | quotation | source | removal", formalDefinition="How the entity was used during the activity." ) 611 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provenance-entity-role") 612 protected Enumeration<ProvenanceEntityRole> role; 613 614 /** 615 * Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative. 616 */ 617 @Child(name = "what", type = {UriType.class, Reference.class, Identifier.class}, order=2, min=1, max=1, modifier=false, summary=true) 618 @Description(shortDefinition="Identity of entity", formalDefinition="Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative." ) 619 protected Type what; 620 621 /** 622 * The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity. 623 */ 624 @Child(name = "agent", type = {ProvenanceAgentComponent.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 625 @Description(shortDefinition="Entity is attributed to this agent", formalDefinition="The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity." ) 626 protected List<ProvenanceAgentComponent> agent; 627 628 private static final long serialVersionUID = 1436676923L; 629 630 /** 631 * Constructor 632 */ 633 public ProvenanceEntityComponent() { 634 super(); 635 } 636 637 /** 638 * Constructor 639 */ 640 public ProvenanceEntityComponent(Enumeration<ProvenanceEntityRole> role, Type what) { 641 super(); 642 this.role = role; 643 this.what = what; 644 } 645 646 /** 647 * @return {@link #role} (How the entity was used during the activity.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 648 */ 649 public Enumeration<ProvenanceEntityRole> getRoleElement() { 650 if (this.role == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create ProvenanceEntityComponent.role"); 653 else if (Configuration.doAutoCreate()) 654 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); // bb 655 return this.role; 656 } 657 658 public boolean hasRoleElement() { 659 return this.role != null && !this.role.isEmpty(); 660 } 661 662 public boolean hasRole() { 663 return this.role != null && !this.role.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #role} (How the entity was used during the activity.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 668 */ 669 public ProvenanceEntityComponent setRoleElement(Enumeration<ProvenanceEntityRole> value) { 670 this.role = value; 671 return this; 672 } 673 674 /** 675 * @return How the entity was used during the activity. 676 */ 677 public ProvenanceEntityRole getRole() { 678 return this.role == null ? null : this.role.getValue(); 679 } 680 681 /** 682 * @param value How the entity was used during the activity. 683 */ 684 public ProvenanceEntityComponent setRole(ProvenanceEntityRole value) { 685 if (this.role == null) 686 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); 687 this.role.setValue(value); 688 return this; 689 } 690 691 /** 692 * @return {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 693 */ 694 public Type getWhat() { 695 return this.what; 696 } 697 698 /** 699 * @return {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 700 */ 701 public UriType getWhatUriType() throws FHIRException { 702 if (this.what == null) 703 return null; 704 if (!(this.what instanceof UriType)) 705 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.what.getClass().getName()+" was encountered"); 706 return (UriType) this.what; 707 } 708 709 public boolean hasWhatUriType() { 710 return this != null && this.what instanceof UriType; 711 } 712 713 /** 714 * @return {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 715 */ 716 public Reference getWhatReference() throws FHIRException { 717 if (this.what == null) 718 return null; 719 if (!(this.what instanceof Reference)) 720 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.what.getClass().getName()+" was encountered"); 721 return (Reference) this.what; 722 } 723 724 public boolean hasWhatReference() { 725 return this != null && this.what instanceof Reference; 726 } 727 728 /** 729 * @return {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 730 */ 731 public Identifier getWhatIdentifier() throws FHIRException { 732 if (this.what == null) 733 return null; 734 if (!(this.what instanceof Identifier)) 735 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.what.getClass().getName()+" was encountered"); 736 return (Identifier) this.what; 737 } 738 739 public boolean hasWhatIdentifier() { 740 return this != null && this.what instanceof Identifier; 741 } 742 743 public boolean hasWhat() { 744 return this.what != null && !this.what.isEmpty(); 745 } 746 747 /** 748 * @param value {@link #what} (Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.) 749 */ 750 public ProvenanceEntityComponent setWhat(Type value) throws FHIRFormatError { 751 if (value != null && !(value instanceof UriType || value instanceof Reference || value instanceof Identifier)) 752 throw new FHIRFormatError("Not the right type for Provenance.entity.what[x]: "+value.fhirType()); 753 this.what = value; 754 return this; 755 } 756 757 /** 758 * @return {@link #agent} (The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.) 759 */ 760 public List<ProvenanceAgentComponent> getAgent() { 761 if (this.agent == null) 762 this.agent = new ArrayList<ProvenanceAgentComponent>(); 763 return this.agent; 764 } 765 766 /** 767 * @return Returns a reference to <code>this</code> for easy method chaining 768 */ 769 public ProvenanceEntityComponent setAgent(List<ProvenanceAgentComponent> theAgent) { 770 this.agent = theAgent; 771 return this; 772 } 773 774 public boolean hasAgent() { 775 if (this.agent == null) 776 return false; 777 for (ProvenanceAgentComponent item : this.agent) 778 if (!item.isEmpty()) 779 return true; 780 return false; 781 } 782 783 public ProvenanceAgentComponent addAgent() { //3 784 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 785 if (this.agent == null) 786 this.agent = new ArrayList<ProvenanceAgentComponent>(); 787 this.agent.add(t); 788 return t; 789 } 790 791 public ProvenanceEntityComponent addAgent(ProvenanceAgentComponent t) { //3 792 if (t == null) 793 return this; 794 if (this.agent == null) 795 this.agent = new ArrayList<ProvenanceAgentComponent>(); 796 this.agent.add(t); 797 return this; 798 } 799 800 /** 801 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist 802 */ 803 public ProvenanceAgentComponent getAgentFirstRep() { 804 if (getAgent().isEmpty()) { 805 addAgent(); 806 } 807 return getAgent().get(0); 808 } 809 810 protected void listChildren(List<Property> children) { 811 super.listChildren(children); 812 children.add(new Property("role", "code", "How the entity was used during the activity.", 0, 1, role)); 813 children.add(new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what)); 814 children.add(new Property("agent", "@Provenance.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.", 0, java.lang.Integer.MAX_VALUE, agent)); 815 } 816 817 @Override 818 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 819 switch (_hash) { 820 case 3506294: /*role*/ return new Property("role", "code", "How the entity was used during the activity.", 0, 1, role); 821 case 1309315900: /*what[x]*/ return new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 822 case 3648196: /*what*/ return new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 823 case 1309309960: /*whatUri*/ return new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 824 case 1531941095: /*whatReference*/ return new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 825 case 1537117837: /*whatIdentifier*/ return new Property("what[x]", "uri|Reference(Any)|Identifier", "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 1, what); 826 case 92750597: /*agent*/ return new Property("agent", "@Provenance.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.", 0, java.lang.Integer.MAX_VALUE, agent); 827 default: return super.getNamedProperty(_hash, _name, _checkValid); 828 } 829 830 } 831 832 @Override 833 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 834 switch (hash) { 835 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // Enumeration<ProvenanceEntityRole> 836 case 3648196: /*what*/ return this.what == null ? new Base[0] : new Base[] {this.what}; // Type 837 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 838 default: return super.getProperty(hash, name, checkValid); 839 } 840 841 } 842 843 @Override 844 public Base setProperty(int hash, String name, Base value) throws FHIRException { 845 switch (hash) { 846 case 3506294: // role 847 value = new ProvenanceEntityRoleEnumFactory().fromType(castToCode(value)); 848 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 849 return value; 850 case 3648196: // what 851 this.what = castToType(value); // Type 852 return value; 853 case 92750597: // agent 854 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 855 return value; 856 default: return super.setProperty(hash, name, value); 857 } 858 859 } 860 861 @Override 862 public Base setProperty(String name, Base value) throws FHIRException { 863 if (name.equals("role")) { 864 value = new ProvenanceEntityRoleEnumFactory().fromType(castToCode(value)); 865 this.role = (Enumeration) value; // Enumeration<ProvenanceEntityRole> 866 } else if (name.equals("what[x]")) { 867 this.what = castToType(value); // Type 868 } else if (name.equals("agent")) { 869 this.getAgent().add((ProvenanceAgentComponent) value); 870 } else 871 return super.setProperty(name, value); 872 return value; 873 } 874 875 @Override 876 public Base makeProperty(int hash, String name) throws FHIRException { 877 switch (hash) { 878 case 3506294: return getRoleElement(); 879 case 1309315900: return getWhat(); 880 case 3648196: return getWhat(); 881 case 92750597: return addAgent(); 882 default: return super.makeProperty(hash, name); 883 } 884 885 } 886 887 @Override 888 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 889 switch (hash) { 890 case 3506294: /*role*/ return new String[] {"code"}; 891 case 3648196: /*what*/ return new String[] {"uri", "Reference", "Identifier"}; 892 case 92750597: /*agent*/ return new String[] {"@Provenance.agent"}; 893 default: return super.getTypesForProperty(hash, name); 894 } 895 896 } 897 898 @Override 899 public Base addChild(String name) throws FHIRException { 900 if (name.equals("role")) { 901 throw new FHIRException("Cannot call addChild on a singleton property Provenance.role"); 902 } 903 else if (name.equals("whatUri")) { 904 this.what = new UriType(); 905 return this.what; 906 } 907 else if (name.equals("whatReference")) { 908 this.what = new Reference(); 909 return this.what; 910 } 911 else if (name.equals("whatIdentifier")) { 912 this.what = new Identifier(); 913 return this.what; 914 } 915 else if (name.equals("agent")) { 916 return addAgent(); 917 } 918 else 919 return super.addChild(name); 920 } 921 922 public ProvenanceEntityComponent copy() { 923 ProvenanceEntityComponent dst = new ProvenanceEntityComponent(); 924 copyValues(dst); 925 dst.role = role == null ? null : role.copy(); 926 dst.what = what == null ? null : what.copy(); 927 if (agent != null) { 928 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 929 for (ProvenanceAgentComponent i : agent) 930 dst.agent.add(i.copy()); 931 }; 932 return dst; 933 } 934 935 @Override 936 public boolean equalsDeep(Base other_) { 937 if (!super.equalsDeep(other_)) 938 return false; 939 if (!(other_ instanceof ProvenanceEntityComponent)) 940 return false; 941 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 942 return compareDeep(role, o.role, true) && compareDeep(what, o.what, true) && compareDeep(agent, o.agent, true) 943 ; 944 } 945 946 @Override 947 public boolean equalsShallow(Base other_) { 948 if (!super.equalsShallow(other_)) 949 return false; 950 if (!(other_ instanceof ProvenanceEntityComponent)) 951 return false; 952 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other_; 953 return compareValues(role, o.role, true); 954 } 955 956 public boolean isEmpty() { 957 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, what, agent); 958 } 959 960 public String fhirType() { 961 return "Provenance.entity"; 962 963 } 964 965 } 966 967 /** 968 * The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity. 969 */ 970 @Child(name = "target", type = {Reference.class}, order=0, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 971 @Description(shortDefinition="Target Reference(s) (usually version specific)", formalDefinition="The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity." ) 972 protected List<Reference> target; 973 /** 974 * The actual objects that are the target of the reference (The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.) 975 */ 976 protected List<Resource> targetTarget; 977 978 979 /** 980 * The period during which the activity occurred. 981 */ 982 @Child(name = "period", type = {Period.class}, order=1, min=0, max=1, modifier=false, summary=false) 983 @Description(shortDefinition="When the activity occurred", formalDefinition="The period during which the activity occurred." ) 984 protected Period period; 985 986 /** 987 * The instant of time at which the activity was recorded. 988 */ 989 @Child(name = "recorded", type = {InstantType.class}, order=2, min=1, max=1, modifier=false, summary=true) 990 @Description(shortDefinition="When the activity was recorded / updated", formalDefinition="The instant of time at which the activity was recorded." ) 991 protected InstantType recorded; 992 993 /** 994 * Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc. 995 */ 996 @Child(name = "policy", type = {UriType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 997 @Description(shortDefinition="Policy or plan the activity was defined by", formalDefinition="Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc." ) 998 protected List<UriType> policy; 999 1000 /** 1001 * Where the activity occurred, if relevant. 1002 */ 1003 @Child(name = "location", type = {Location.class}, order=4, min=0, max=1, modifier=false, summary=false) 1004 @Description(shortDefinition="Where the activity occurred, if relevant", formalDefinition="Where the activity occurred, if relevant." ) 1005 protected Reference location; 1006 1007 /** 1008 * The actual object that is the target of the reference (Where the activity occurred, if relevant.) 1009 */ 1010 protected Location locationTarget; 1011 1012 /** 1013 * The reason that the activity was taking place. 1014 */ 1015 @Child(name = "reason", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1016 @Description(shortDefinition="Reason the activity is occurring", formalDefinition="The reason that the activity was taking place." ) 1017 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-PurposeOfUse") 1018 protected List<Coding> reason; 1019 1020 /** 1021 * An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities. 1022 */ 1023 @Child(name = "activity", type = {Coding.class}, order=6, min=0, max=1, modifier=false, summary=false) 1024 @Description(shortDefinition="Activity that occurred", formalDefinition="An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities." ) 1025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provenance-activity-type") 1026 protected Coding activity; 1027 1028 /** 1029 * An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place. 1030 */ 1031 @Child(name = "agent", type = {}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1032 @Description(shortDefinition="Actor involved", formalDefinition="An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place." ) 1033 protected List<ProvenanceAgentComponent> agent; 1034 1035 /** 1036 * An entity used in this activity. 1037 */ 1038 @Child(name = "entity", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1039 @Description(shortDefinition="An entity used in this activity", formalDefinition="An entity used in this activity." ) 1040 protected List<ProvenanceEntityComponent> entity; 1041 1042 /** 1043 * A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated. 1044 */ 1045 @Child(name = "signature", type = {Signature.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1046 @Description(shortDefinition="Signature on target", formalDefinition="A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated." ) 1047 protected List<Signature> signature; 1048 1049 private static final long serialVersionUID = -1668640371L; 1050 1051 /** 1052 * Constructor 1053 */ 1054 public Provenance() { 1055 super(); 1056 } 1057 1058 /** 1059 * Constructor 1060 */ 1061 public Provenance(InstantType recorded) { 1062 super(); 1063 this.recorded = recorded; 1064 } 1065 1066 /** 1067 * @return {@link #target} (The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.) 1068 */ 1069 public List<Reference> getTarget() { 1070 if (this.target == null) 1071 this.target = new ArrayList<Reference>(); 1072 return this.target; 1073 } 1074 1075 /** 1076 * @return Returns a reference to <code>this</code> for easy method chaining 1077 */ 1078 public Provenance setTarget(List<Reference> theTarget) { 1079 this.target = theTarget; 1080 return this; 1081 } 1082 1083 public boolean hasTarget() { 1084 if (this.target == null) 1085 return false; 1086 for (Reference item : this.target) 1087 if (!item.isEmpty()) 1088 return true; 1089 return false; 1090 } 1091 1092 public Reference addTarget() { //3 1093 Reference t = new Reference(); 1094 if (this.target == null) 1095 this.target = new ArrayList<Reference>(); 1096 this.target.add(t); 1097 return t; 1098 } 1099 1100 public Provenance addTarget(Reference t) { //3 1101 if (t == null) 1102 return this; 1103 if (this.target == null) 1104 this.target = new ArrayList<Reference>(); 1105 this.target.add(t); 1106 return this; 1107 } 1108 1109 /** 1110 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist 1111 */ 1112 public Reference getTargetFirstRep() { 1113 if (getTarget().isEmpty()) { 1114 addTarget(); 1115 } 1116 return getTarget().get(0); 1117 } 1118 1119 /** 1120 * @deprecated Use Reference#setResource(IBaseResource) instead 1121 */ 1122 @Deprecated 1123 public List<Resource> getTargetTarget() { 1124 if (this.targetTarget == null) 1125 this.targetTarget = new ArrayList<Resource>(); 1126 return this.targetTarget; 1127 } 1128 1129 /** 1130 * @return {@link #period} (The period during which the activity occurred.) 1131 */ 1132 public Period getPeriod() { 1133 if (this.period == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create Provenance.period"); 1136 else if (Configuration.doAutoCreate()) 1137 this.period = new Period(); // cc 1138 return this.period; 1139 } 1140 1141 public boolean hasPeriod() { 1142 return this.period != null && !this.period.isEmpty(); 1143 } 1144 1145 /** 1146 * @param value {@link #period} (The period during which the activity occurred.) 1147 */ 1148 public Provenance setPeriod(Period value) { 1149 this.period = value; 1150 return this; 1151 } 1152 1153 /** 1154 * @return {@link #recorded} (The instant of time at which the activity was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1155 */ 1156 public InstantType getRecordedElement() { 1157 if (this.recorded == null) 1158 if (Configuration.errorOnAutoCreate()) 1159 throw new Error("Attempt to auto-create Provenance.recorded"); 1160 else if (Configuration.doAutoCreate()) 1161 this.recorded = new InstantType(); // bb 1162 return this.recorded; 1163 } 1164 1165 public boolean hasRecordedElement() { 1166 return this.recorded != null && !this.recorded.isEmpty(); 1167 } 1168 1169 public boolean hasRecorded() { 1170 return this.recorded != null && !this.recorded.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #recorded} (The instant of time at which the activity was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1175 */ 1176 public Provenance setRecordedElement(InstantType value) { 1177 this.recorded = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return The instant of time at which the activity was recorded. 1183 */ 1184 public Date getRecorded() { 1185 return this.recorded == null ? null : this.recorded.getValue(); 1186 } 1187 1188 /** 1189 * @param value The instant of time at which the activity was recorded. 1190 */ 1191 public Provenance setRecorded(Date value) { 1192 if (this.recorded == null) 1193 this.recorded = new InstantType(); 1194 this.recorded.setValue(value); 1195 return this; 1196 } 1197 1198 /** 1199 * @return {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1200 */ 1201 public List<UriType> getPolicy() { 1202 if (this.policy == null) 1203 this.policy = new ArrayList<UriType>(); 1204 return this.policy; 1205 } 1206 1207 /** 1208 * @return Returns a reference to <code>this</code> for easy method chaining 1209 */ 1210 public Provenance setPolicy(List<UriType> thePolicy) { 1211 this.policy = thePolicy; 1212 return this; 1213 } 1214 1215 public boolean hasPolicy() { 1216 if (this.policy == null) 1217 return false; 1218 for (UriType item : this.policy) 1219 if (!item.isEmpty()) 1220 return true; 1221 return false; 1222 } 1223 1224 /** 1225 * @return {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1226 */ 1227 public UriType addPolicyElement() {//2 1228 UriType t = new UriType(); 1229 if (this.policy == null) 1230 this.policy = new ArrayList<UriType>(); 1231 this.policy.add(t); 1232 return t; 1233 } 1234 1235 /** 1236 * @param value {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1237 */ 1238 public Provenance addPolicy(String value) { //1 1239 UriType t = new UriType(); 1240 t.setValue(value); 1241 if (this.policy == null) 1242 this.policy = new ArrayList<UriType>(); 1243 this.policy.add(t); 1244 return this; 1245 } 1246 1247 /** 1248 * @param value {@link #policy} (Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.) 1249 */ 1250 public boolean hasPolicy(String value) { 1251 if (this.policy == null) 1252 return false; 1253 for (UriType v : this.policy) 1254 if (v.getValue().equals(value)) // uri 1255 return true; 1256 return false; 1257 } 1258 1259 /** 1260 * @return {@link #location} (Where the activity occurred, if relevant.) 1261 */ 1262 public Reference getLocation() { 1263 if (this.location == null) 1264 if (Configuration.errorOnAutoCreate()) 1265 throw new Error("Attempt to auto-create Provenance.location"); 1266 else if (Configuration.doAutoCreate()) 1267 this.location = new Reference(); // cc 1268 return this.location; 1269 } 1270 1271 public boolean hasLocation() { 1272 return this.location != null && !this.location.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #location} (Where the activity occurred, if relevant.) 1277 */ 1278 public Provenance setLocation(Reference value) { 1279 this.location = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the activity occurred, if relevant.) 1285 */ 1286 public Location getLocationTarget() { 1287 if (this.locationTarget == null) 1288 if (Configuration.errorOnAutoCreate()) 1289 throw new Error("Attempt to auto-create Provenance.location"); 1290 else if (Configuration.doAutoCreate()) 1291 this.locationTarget = new Location(); // aa 1292 return this.locationTarget; 1293 } 1294 1295 /** 1296 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the activity occurred, if relevant.) 1297 */ 1298 public Provenance setLocationTarget(Location value) { 1299 this.locationTarget = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #reason} (The reason that the activity was taking place.) 1305 */ 1306 public List<Coding> getReason() { 1307 if (this.reason == null) 1308 this.reason = new ArrayList<Coding>(); 1309 return this.reason; 1310 } 1311 1312 /** 1313 * @return Returns a reference to <code>this</code> for easy method chaining 1314 */ 1315 public Provenance setReason(List<Coding> theReason) { 1316 this.reason = theReason; 1317 return this; 1318 } 1319 1320 public boolean hasReason() { 1321 if (this.reason == null) 1322 return false; 1323 for (Coding item : this.reason) 1324 if (!item.isEmpty()) 1325 return true; 1326 return false; 1327 } 1328 1329 public Coding addReason() { //3 1330 Coding t = new Coding(); 1331 if (this.reason == null) 1332 this.reason = new ArrayList<Coding>(); 1333 this.reason.add(t); 1334 return t; 1335 } 1336 1337 public Provenance addReason(Coding t) { //3 1338 if (t == null) 1339 return this; 1340 if (this.reason == null) 1341 this.reason = new ArrayList<Coding>(); 1342 this.reason.add(t); 1343 return this; 1344 } 1345 1346 /** 1347 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 1348 */ 1349 public Coding getReasonFirstRep() { 1350 if (getReason().isEmpty()) { 1351 addReason(); 1352 } 1353 return getReason().get(0); 1354 } 1355 1356 /** 1357 * @return {@link #activity} (An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.) 1358 */ 1359 public Coding getActivity() { 1360 if (this.activity == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create Provenance.activity"); 1363 else if (Configuration.doAutoCreate()) 1364 this.activity = new Coding(); // cc 1365 return this.activity; 1366 } 1367 1368 public boolean hasActivity() { 1369 return this.activity != null && !this.activity.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #activity} (An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.) 1374 */ 1375 public Provenance setActivity(Coding value) { 1376 this.activity = value; 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #agent} (An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.) 1382 */ 1383 public List<ProvenanceAgentComponent> getAgent() { 1384 if (this.agent == null) 1385 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1386 return this.agent; 1387 } 1388 1389 /** 1390 * @return Returns a reference to <code>this</code> for easy method chaining 1391 */ 1392 public Provenance setAgent(List<ProvenanceAgentComponent> theAgent) { 1393 this.agent = theAgent; 1394 return this; 1395 } 1396 1397 public boolean hasAgent() { 1398 if (this.agent == null) 1399 return false; 1400 for (ProvenanceAgentComponent item : this.agent) 1401 if (!item.isEmpty()) 1402 return true; 1403 return false; 1404 } 1405 1406 public ProvenanceAgentComponent addAgent() { //3 1407 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 1408 if (this.agent == null) 1409 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1410 this.agent.add(t); 1411 return t; 1412 } 1413 1414 public Provenance addAgent(ProvenanceAgentComponent t) { //3 1415 if (t == null) 1416 return this; 1417 if (this.agent == null) 1418 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1419 this.agent.add(t); 1420 return this; 1421 } 1422 1423 /** 1424 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist 1425 */ 1426 public ProvenanceAgentComponent getAgentFirstRep() { 1427 if (getAgent().isEmpty()) { 1428 addAgent(); 1429 } 1430 return getAgent().get(0); 1431 } 1432 1433 /** 1434 * @return {@link #entity} (An entity used in this activity.) 1435 */ 1436 public List<ProvenanceEntityComponent> getEntity() { 1437 if (this.entity == null) 1438 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1439 return this.entity; 1440 } 1441 1442 /** 1443 * @return Returns a reference to <code>this</code> for easy method chaining 1444 */ 1445 public Provenance setEntity(List<ProvenanceEntityComponent> theEntity) { 1446 this.entity = theEntity; 1447 return this; 1448 } 1449 1450 public boolean hasEntity() { 1451 if (this.entity == null) 1452 return false; 1453 for (ProvenanceEntityComponent item : this.entity) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 public ProvenanceEntityComponent addEntity() { //3 1460 ProvenanceEntityComponent t = new ProvenanceEntityComponent(); 1461 if (this.entity == null) 1462 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1463 this.entity.add(t); 1464 return t; 1465 } 1466 1467 public Provenance addEntity(ProvenanceEntityComponent t) { //3 1468 if (t == null) 1469 return this; 1470 if (this.entity == null) 1471 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1472 this.entity.add(t); 1473 return this; 1474 } 1475 1476 /** 1477 * @return The first repetition of repeating field {@link #entity}, creating it if it does not already exist 1478 */ 1479 public ProvenanceEntityComponent getEntityFirstRep() { 1480 if (getEntity().isEmpty()) { 1481 addEntity(); 1482 } 1483 return getEntity().get(0); 1484 } 1485 1486 /** 1487 * @return {@link #signature} (A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.) 1488 */ 1489 public List<Signature> getSignature() { 1490 if (this.signature == null) 1491 this.signature = new ArrayList<Signature>(); 1492 return this.signature; 1493 } 1494 1495 /** 1496 * @return Returns a reference to <code>this</code> for easy method chaining 1497 */ 1498 public Provenance setSignature(List<Signature> theSignature) { 1499 this.signature = theSignature; 1500 return this; 1501 } 1502 1503 public boolean hasSignature() { 1504 if (this.signature == null) 1505 return false; 1506 for (Signature item : this.signature) 1507 if (!item.isEmpty()) 1508 return true; 1509 return false; 1510 } 1511 1512 public Signature addSignature() { //3 1513 Signature t = new Signature(); 1514 if (this.signature == null) 1515 this.signature = new ArrayList<Signature>(); 1516 this.signature.add(t); 1517 return t; 1518 } 1519 1520 public Provenance addSignature(Signature t) { //3 1521 if (t == null) 1522 return this; 1523 if (this.signature == null) 1524 this.signature = new ArrayList<Signature>(); 1525 this.signature.add(t); 1526 return this; 1527 } 1528 1529 /** 1530 * @return The first repetition of repeating field {@link #signature}, creating it if it does not already exist 1531 */ 1532 public Signature getSignatureFirstRep() { 1533 if (getSignature().isEmpty()) { 1534 addSignature(); 1535 } 1536 return getSignature().get(0); 1537 } 1538 1539 protected void listChildren(List<Property> children) { 1540 super.listChildren(children); 1541 children.add(new Property("target", "Reference(Any)", "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 0, java.lang.Integer.MAX_VALUE, target)); 1542 children.add(new Property("period", "Period", "The period during which the activity occurred.", 0, 1, period)); 1543 children.add(new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1, recorded)); 1544 children.add(new Property("policy", "uri", "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 0, java.lang.Integer.MAX_VALUE, policy)); 1545 children.add(new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1, location)); 1546 children.add(new Property("reason", "Coding", "The reason that the activity was taking place.", 0, java.lang.Integer.MAX_VALUE, reason)); 1547 children.add(new Property("activity", "Coding", "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 0, 1, activity)); 1548 children.add(new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent)); 1549 children.add(new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity)); 1550 children.add(new Property("signature", "Signature", "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 0, java.lang.Integer.MAX_VALUE, signature)); 1551 } 1552 1553 @Override 1554 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1555 switch (_hash) { 1556 case -880905839: /*target*/ return new Property("target", "Reference(Any)", "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 0, java.lang.Integer.MAX_VALUE, target); 1557 case -991726143: /*period*/ return new Property("period", "Period", "The period during which the activity occurred.", 0, 1, period); 1558 case -799233872: /*recorded*/ return new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1, recorded); 1559 case -982670030: /*policy*/ return new Property("policy", "uri", "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 0, java.lang.Integer.MAX_VALUE, policy); 1560 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1, location); 1561 case -934964668: /*reason*/ return new Property("reason", "Coding", "The reason that the activity was taking place.", 0, java.lang.Integer.MAX_VALUE, reason); 1562 case -1655966961: /*activity*/ return new Property("activity", "Coding", "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 0, 1, activity); 1563 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, agent); 1564 case -1298275357: /*entity*/ return new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity); 1565 case 1073584312: /*signature*/ return new Property("signature", "Signature", "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 0, java.lang.Integer.MAX_VALUE, signature); 1566 default: return super.getNamedProperty(_hash, _name, _checkValid); 1567 } 1568 1569 } 1570 1571 @Override 1572 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1573 switch (hash) { 1574 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 1575 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1576 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // InstantType 1577 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1578 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1579 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // Coding 1580 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : new Base[] {this.activity}; // Coding 1581 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // ProvenanceAgentComponent 1582 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // ProvenanceEntityComponent 1583 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : this.signature.toArray(new Base[this.signature.size()]); // Signature 1584 default: return super.getProperty(hash, name, checkValid); 1585 } 1586 1587 } 1588 1589 @Override 1590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1591 switch (hash) { 1592 case -880905839: // target 1593 this.getTarget().add(castToReference(value)); // Reference 1594 return value; 1595 case -991726143: // period 1596 this.period = castToPeriod(value); // Period 1597 return value; 1598 case -799233872: // recorded 1599 this.recorded = castToInstant(value); // InstantType 1600 return value; 1601 case -982670030: // policy 1602 this.getPolicy().add(castToUri(value)); // UriType 1603 return value; 1604 case 1901043637: // location 1605 this.location = castToReference(value); // Reference 1606 return value; 1607 case -934964668: // reason 1608 this.getReason().add(castToCoding(value)); // Coding 1609 return value; 1610 case -1655966961: // activity 1611 this.activity = castToCoding(value); // Coding 1612 return value; 1613 case 92750597: // agent 1614 this.getAgent().add((ProvenanceAgentComponent) value); // ProvenanceAgentComponent 1615 return value; 1616 case -1298275357: // entity 1617 this.getEntity().add((ProvenanceEntityComponent) value); // ProvenanceEntityComponent 1618 return value; 1619 case 1073584312: // signature 1620 this.getSignature().add(castToSignature(value)); // Signature 1621 return value; 1622 default: return super.setProperty(hash, name, value); 1623 } 1624 1625 } 1626 1627 @Override 1628 public Base setProperty(String name, Base value) throws FHIRException { 1629 if (name.equals("target")) { 1630 this.getTarget().add(castToReference(value)); 1631 } else if (name.equals("period")) { 1632 this.period = castToPeriod(value); // Period 1633 } else if (name.equals("recorded")) { 1634 this.recorded = castToInstant(value); // InstantType 1635 } else if (name.equals("policy")) { 1636 this.getPolicy().add(castToUri(value)); 1637 } else if (name.equals("location")) { 1638 this.location = castToReference(value); // Reference 1639 } else if (name.equals("reason")) { 1640 this.getReason().add(castToCoding(value)); 1641 } else if (name.equals("activity")) { 1642 this.activity = castToCoding(value); // Coding 1643 } else if (name.equals("agent")) { 1644 this.getAgent().add((ProvenanceAgentComponent) value); 1645 } else if (name.equals("entity")) { 1646 this.getEntity().add((ProvenanceEntityComponent) value); 1647 } else if (name.equals("signature")) { 1648 this.getSignature().add(castToSignature(value)); 1649 } else 1650 return super.setProperty(name, value); 1651 return value; 1652 } 1653 1654 @Override 1655 public Base makeProperty(int hash, String name) throws FHIRException { 1656 switch (hash) { 1657 case -880905839: return addTarget(); 1658 case -991726143: return getPeriod(); 1659 case -799233872: return getRecordedElement(); 1660 case -982670030: return addPolicyElement(); 1661 case 1901043637: return getLocation(); 1662 case -934964668: return addReason(); 1663 case -1655966961: return getActivity(); 1664 case 92750597: return addAgent(); 1665 case -1298275357: return addEntity(); 1666 case 1073584312: return addSignature(); 1667 default: return super.makeProperty(hash, name); 1668 } 1669 1670 } 1671 1672 @Override 1673 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1674 switch (hash) { 1675 case -880905839: /*target*/ return new String[] {"Reference"}; 1676 case -991726143: /*period*/ return new String[] {"Period"}; 1677 case -799233872: /*recorded*/ return new String[] {"instant"}; 1678 case -982670030: /*policy*/ return new String[] {"uri"}; 1679 case 1901043637: /*location*/ return new String[] {"Reference"}; 1680 case -934964668: /*reason*/ return new String[] {"Coding"}; 1681 case -1655966961: /*activity*/ return new String[] {"Coding"}; 1682 case 92750597: /*agent*/ return new String[] {}; 1683 case -1298275357: /*entity*/ return new String[] {}; 1684 case 1073584312: /*signature*/ return new String[] {"Signature"}; 1685 default: return super.getTypesForProperty(hash, name); 1686 } 1687 1688 } 1689 1690 @Override 1691 public Base addChild(String name) throws FHIRException { 1692 if (name.equals("target")) { 1693 return addTarget(); 1694 } 1695 else if (name.equals("period")) { 1696 this.period = new Period(); 1697 return this.period; 1698 } 1699 else if (name.equals("recorded")) { 1700 throw new FHIRException("Cannot call addChild on a singleton property Provenance.recorded"); 1701 } 1702 else if (name.equals("policy")) { 1703 throw new FHIRException("Cannot call addChild on a singleton property Provenance.policy"); 1704 } 1705 else if (name.equals("location")) { 1706 this.location = new Reference(); 1707 return this.location; 1708 } 1709 else if (name.equals("reason")) { 1710 return addReason(); 1711 } 1712 else if (name.equals("activity")) { 1713 this.activity = new Coding(); 1714 return this.activity; 1715 } 1716 else if (name.equals("agent")) { 1717 return addAgent(); 1718 } 1719 else if (name.equals("entity")) { 1720 return addEntity(); 1721 } 1722 else if (name.equals("signature")) { 1723 return addSignature(); 1724 } 1725 else 1726 return super.addChild(name); 1727 } 1728 1729 public String fhirType() { 1730 return "Provenance"; 1731 1732 } 1733 1734 public Provenance copy() { 1735 Provenance dst = new Provenance(); 1736 copyValues(dst); 1737 if (target != null) { 1738 dst.target = new ArrayList<Reference>(); 1739 for (Reference i : target) 1740 dst.target.add(i.copy()); 1741 }; 1742 dst.period = period == null ? null : period.copy(); 1743 dst.recorded = recorded == null ? null : recorded.copy(); 1744 if (policy != null) { 1745 dst.policy = new ArrayList<UriType>(); 1746 for (UriType i : policy) 1747 dst.policy.add(i.copy()); 1748 }; 1749 dst.location = location == null ? null : location.copy(); 1750 if (reason != null) { 1751 dst.reason = new ArrayList<Coding>(); 1752 for (Coding i : reason) 1753 dst.reason.add(i.copy()); 1754 }; 1755 dst.activity = activity == null ? null : activity.copy(); 1756 if (agent != null) { 1757 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 1758 for (ProvenanceAgentComponent i : agent) 1759 dst.agent.add(i.copy()); 1760 }; 1761 if (entity != null) { 1762 dst.entity = new ArrayList<ProvenanceEntityComponent>(); 1763 for (ProvenanceEntityComponent i : entity) 1764 dst.entity.add(i.copy()); 1765 }; 1766 if (signature != null) { 1767 dst.signature = new ArrayList<Signature>(); 1768 for (Signature i : signature) 1769 dst.signature.add(i.copy()); 1770 }; 1771 return dst; 1772 } 1773 1774 protected Provenance typedCopy() { 1775 return copy(); 1776 } 1777 1778 @Override 1779 public boolean equalsDeep(Base other_) { 1780 if (!super.equalsDeep(other_)) 1781 return false; 1782 if (!(other_ instanceof Provenance)) 1783 return false; 1784 Provenance o = (Provenance) other_; 1785 return compareDeep(target, o.target, true) && compareDeep(period, o.period, true) && compareDeep(recorded, o.recorded, true) 1786 && compareDeep(policy, o.policy, true) && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) 1787 && compareDeep(activity, o.activity, true) && compareDeep(agent, o.agent, true) && compareDeep(entity, o.entity, true) 1788 && compareDeep(signature, o.signature, true); 1789 } 1790 1791 @Override 1792 public boolean equalsShallow(Base other_) { 1793 if (!super.equalsShallow(other_)) 1794 return false; 1795 if (!(other_ instanceof Provenance)) 1796 return false; 1797 Provenance o = (Provenance) other_; 1798 return compareValues(recorded, o.recorded, true) && compareValues(policy, o.policy, true); 1799 } 1800 1801 public boolean isEmpty() { 1802 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, period, recorded 1803 , policy, location, reason, activity, agent, entity, signature); 1804 } 1805 1806 @Override 1807 public ResourceType getResourceType() { 1808 return ResourceType.Provenance; 1809 } 1810 1811 /** 1812 * Search parameter: <b>entity-ref</b> 1813 * <p> 1814 * Description: <b>Identity of entity</b><br> 1815 * Type: <b>reference</b><br> 1816 * Path: <b>Provenance.entity.whatReference</b><br> 1817 * </p> 1818 */ 1819 @SearchParamDefinition(name="entity-ref", path="Provenance.entity.what.as(Reference)", description="Identity of entity", type="reference" ) 1820 public static final String SP_ENTITY_REF = "entity-ref"; 1821 /** 1822 * <b>Fluent Client</b> search parameter constant for <b>entity-ref</b> 1823 * <p> 1824 * Description: <b>Identity of entity</b><br> 1825 * Type: <b>reference</b><br> 1826 * Path: <b>Provenance.entity.whatReference</b><br> 1827 * </p> 1828 */ 1829 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTITY_REF); 1830 1831/** 1832 * Constant for fluent queries to be used to add include statements. Specifies 1833 * the path value of "<b>Provenance:entity-ref</b>". 1834 */ 1835 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY_REF = new ca.uhn.fhir.model.api.Include("Provenance:entity-ref").toLocked(); 1836 1837 /** 1838 * Search parameter: <b>agent</b> 1839 * <p> 1840 * Description: <b>Who participated</b><br> 1841 * Type: <b>reference</b><br> 1842 * Path: <b>Provenance.agent.who[x]</b><br> 1843 * </p> 1844 */ 1845 @SearchParamDefinition(name="agent", path="Provenance.agent.who", description="Who participated", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1846 public static final String SP_AGENT = "agent"; 1847 /** 1848 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 1849 * <p> 1850 * Description: <b>Who participated</b><br> 1851 * Type: <b>reference</b><br> 1852 * Path: <b>Provenance.agent.who[x]</b><br> 1853 * </p> 1854 */ 1855 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AGENT); 1856 1857/** 1858 * Constant for fluent queries to be used to add include statements. Specifies 1859 * the path value of "<b>Provenance:agent</b>". 1860 */ 1861 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include("Provenance:agent").toLocked(); 1862 1863 /** 1864 * Search parameter: <b>signature-type</b> 1865 * <p> 1866 * Description: <b>Indication of the reason the entity signed the object(s)</b><br> 1867 * Type: <b>token</b><br> 1868 * Path: <b>Provenance.signature.type</b><br> 1869 * </p> 1870 */ 1871 @SearchParamDefinition(name="signature-type", path="Provenance.signature.type", description="Indication of the reason the entity signed the object(s)", type="token" ) 1872 public static final String SP_SIGNATURE_TYPE = "signature-type"; 1873 /** 1874 * <b>Fluent Client</b> search parameter constant for <b>signature-type</b> 1875 * <p> 1876 * Description: <b>Indication of the reason the entity signed the object(s)</b><br> 1877 * Type: <b>token</b><br> 1878 * Path: <b>Provenance.signature.type</b><br> 1879 * </p> 1880 */ 1881 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SIGNATURE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SIGNATURE_TYPE); 1882 1883 /** 1884 * Search parameter: <b>patient</b> 1885 * <p> 1886 * Description: <b>Target Reference(s) (usually version specific)</b><br> 1887 * Type: <b>reference</b><br> 1888 * Path: <b>Provenance.target</b><br> 1889 * </p> 1890 */ 1891 @SearchParamDefinition(name="patient", path="Provenance.target", description="Target Reference(s) (usually version specific)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1892 public static final String SP_PATIENT = "patient"; 1893 /** 1894 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1895 * <p> 1896 * Description: <b>Target Reference(s) (usually version specific)</b><br> 1897 * Type: <b>reference</b><br> 1898 * Path: <b>Provenance.target</b><br> 1899 * </p> 1900 */ 1901 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1902 1903/** 1904 * Constant for fluent queries to be used to add include statements. Specifies 1905 * the path value of "<b>Provenance:patient</b>". 1906 */ 1907 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Provenance:patient").toLocked(); 1908 1909 /** 1910 * Search parameter: <b>start</b> 1911 * <p> 1912 * Description: <b>Starting time with inclusive boundary</b><br> 1913 * Type: <b>date</b><br> 1914 * Path: <b>Provenance.period.start</b><br> 1915 * </p> 1916 */ 1917 @SearchParamDefinition(name="start", path="Provenance.period.start", description="Starting time with inclusive boundary", type="date" ) 1918 public static final String SP_START = "start"; 1919 /** 1920 * <b>Fluent Client</b> search parameter constant for <b>start</b> 1921 * <p> 1922 * Description: <b>Starting time with inclusive boundary</b><br> 1923 * Type: <b>date</b><br> 1924 * Path: <b>Provenance.period.start</b><br> 1925 * </p> 1926 */ 1927 public static final ca.uhn.fhir.rest.gclient.DateClientParam START = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_START); 1928 1929 /** 1930 * Search parameter: <b>end</b> 1931 * <p> 1932 * Description: <b>End time with inclusive boundary, if not ongoing</b><br> 1933 * Type: <b>date</b><br> 1934 * Path: <b>Provenance.period.end</b><br> 1935 * </p> 1936 */ 1937 @SearchParamDefinition(name="end", path="Provenance.period.end", description="End time with inclusive boundary, if not ongoing", type="date" ) 1938 public static final String SP_END = "end"; 1939 /** 1940 * <b>Fluent Client</b> search parameter constant for <b>end</b> 1941 * <p> 1942 * Description: <b>End time with inclusive boundary, if not ongoing</b><br> 1943 * Type: <b>date</b><br> 1944 * Path: <b>Provenance.period.end</b><br> 1945 * </p> 1946 */ 1947 public static final ca.uhn.fhir.rest.gclient.DateClientParam END = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_END); 1948 1949 /** 1950 * Search parameter: <b>location</b> 1951 * <p> 1952 * Description: <b>Where the activity occurred, if relevant</b><br> 1953 * Type: <b>reference</b><br> 1954 * Path: <b>Provenance.location</b><br> 1955 * </p> 1956 */ 1957 @SearchParamDefinition(name="location", path="Provenance.location", description="Where the activity occurred, if relevant", type="reference", target={Location.class } ) 1958 public static final String SP_LOCATION = "location"; 1959 /** 1960 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1961 * <p> 1962 * Description: <b>Where the activity occurred, if relevant</b><br> 1963 * Type: <b>reference</b><br> 1964 * Path: <b>Provenance.location</b><br> 1965 * </p> 1966 */ 1967 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 1968 1969/** 1970 * Constant for fluent queries to be used to add include statements. Specifies 1971 * the path value of "<b>Provenance:location</b>". 1972 */ 1973 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Provenance:location").toLocked(); 1974 1975 /** 1976 * Search parameter: <b>recorded</b> 1977 * <p> 1978 * Description: <b>When the activity was recorded / updated</b><br> 1979 * Type: <b>date</b><br> 1980 * Path: <b>Provenance.recorded</b><br> 1981 * </p> 1982 */ 1983 @SearchParamDefinition(name="recorded", path="Provenance.recorded", description="When the activity was recorded / updated", type="date" ) 1984 public static final String SP_RECORDED = "recorded"; 1985 /** 1986 * <b>Fluent Client</b> search parameter constant for <b>recorded</b> 1987 * <p> 1988 * Description: <b>When the activity was recorded / updated</b><br> 1989 * Type: <b>date</b><br> 1990 * Path: <b>Provenance.recorded</b><br> 1991 * </p> 1992 */ 1993 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECORDED); 1994 1995 /** 1996 * Search parameter: <b>agent-role</b> 1997 * <p> 1998 * Description: <b>What the agents role was</b><br> 1999 * Type: <b>token</b><br> 2000 * Path: <b>Provenance.agent.role</b><br> 2001 * </p> 2002 */ 2003 @SearchParamDefinition(name="agent-role", path="Provenance.agent.role", description="What the agents role was", type="token" ) 2004 public static final String SP_AGENT_ROLE = "agent-role"; 2005 /** 2006 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 2007 * <p> 2008 * Description: <b>What the agents role was</b><br> 2009 * Type: <b>token</b><br> 2010 * Path: <b>Provenance.agent.role</b><br> 2011 * </p> 2012 */ 2013 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_AGENT_ROLE); 2014 2015 /** 2016 * Search parameter: <b>entity-id</b> 2017 * <p> 2018 * Description: <b>Identity of entity</b><br> 2019 * Type: <b>token</b><br> 2020 * Path: <b>Provenance.entity.whatIdentifier</b><br> 2021 * </p> 2022 */ 2023 @SearchParamDefinition(name="entity-id", path="Provenance.entity.what.as(Identifier)", description="Identity of entity", type="token" ) 2024 public static final String SP_ENTITY_ID = "entity-id"; 2025 /** 2026 * <b>Fluent Client</b> search parameter constant for <b>entity-id</b> 2027 * <p> 2028 * Description: <b>Identity of entity</b><br> 2029 * Type: <b>token</b><br> 2030 * Path: <b>Provenance.entity.whatIdentifier</b><br> 2031 * </p> 2032 */ 2033 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ENTITY_ID); 2034 2035 /** 2036 * Search parameter: <b>target</b> 2037 * <p> 2038 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2039 * Type: <b>reference</b><br> 2040 * Path: <b>Provenance.target</b><br> 2041 * </p> 2042 */ 2043 @SearchParamDefinition(name="target", path="Provenance.target", description="Target Reference(s) (usually version specific)", type="reference" ) 2044 public static final String SP_TARGET = "target"; 2045 /** 2046 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2047 * <p> 2048 * Description: <b>Target Reference(s) (usually version specific)</b><br> 2049 * Type: <b>reference</b><br> 2050 * Path: <b>Provenance.target</b><br> 2051 * </p> 2052 */ 2053 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET); 2054 2055/** 2056 * Constant for fluent queries to be used to add include statements. Specifies 2057 * the path value of "<b>Provenance:target</b>". 2058 */ 2059 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include("Provenance:target").toLocked(); 2060 2061 2062}