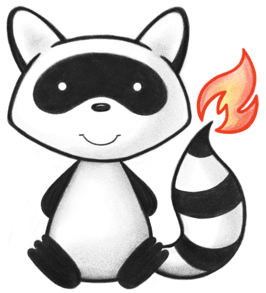
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004import java.util.ArrayList; 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 047 */ 048@DatatypeDef(name="Quantity") 049public class Quantity extends Type implements ICompositeType { 050 051 public enum QuantityComparator { 052 /** 053 * The actual value is less than the given value. 054 */ 055 LESS_THAN, 056 /** 057 * The actual value is less than or equal to the given value. 058 */ 059 LESS_OR_EQUAL, 060 /** 061 * The actual value is greater than or equal to the given value. 062 */ 063 GREATER_OR_EQUAL, 064 /** 065 * The actual value is greater than the given value. 066 */ 067 GREATER_THAN, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static QuantityComparator fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("<".equals(codeString)) 076 return LESS_THAN; 077 if ("<=".equals(codeString)) 078 return LESS_OR_EQUAL; 079 if (">=".equals(codeString)) 080 return GREATER_OR_EQUAL; 081 if (">".equals(codeString)) 082 return GREATER_THAN; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown QuantityComparator code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case LESS_THAN: return "<"; 091 case LESS_OR_EQUAL: return "<="; 092 case GREATER_OR_EQUAL: return ">="; 093 case GREATER_THAN: return ">"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case LESS_THAN: return "http://hl7.org/fhir/quantity-comparator"; 101 case LESS_OR_EQUAL: return "http://hl7.org/fhir/quantity-comparator"; 102 case GREATER_OR_EQUAL: return "http://hl7.org/fhir/quantity-comparator"; 103 case GREATER_THAN: return "http://hl7.org/fhir/quantity-comparator"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDefinition() { 109 switch (this) { 110 case LESS_THAN: return "The actual value is less than the given value."; 111 case LESS_OR_EQUAL: return "The actual value is less than or equal to the given value."; 112 case GREATER_OR_EQUAL: return "The actual value is greater than or equal to the given value."; 113 case GREATER_THAN: return "The actual value is greater than the given value."; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDisplay() { 119 switch (this) { 120 case LESS_THAN: return "Less than"; 121 case LESS_OR_EQUAL: return "Less or Equal to"; 122 case GREATER_OR_EQUAL: return "Greater or Equal to"; 123 case GREATER_THAN: return "Greater than"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 } 129 130 public static class QuantityComparatorEnumFactory implements EnumFactory<QuantityComparator> { 131 public QuantityComparator fromCode(String codeString) throws IllegalArgumentException { 132 if (codeString == null || "".equals(codeString)) 133 if (codeString == null || "".equals(codeString)) 134 return null; 135 if ("<".equals(codeString)) 136 return QuantityComparator.LESS_THAN; 137 if ("<=".equals(codeString)) 138 return QuantityComparator.LESS_OR_EQUAL; 139 if (">=".equals(codeString)) 140 return QuantityComparator.GREATER_OR_EQUAL; 141 if (">".equals(codeString)) 142 return QuantityComparator.GREATER_THAN; 143 throw new IllegalArgumentException("Unknown QuantityComparator code '"+codeString+"'"); 144 } 145 public Enumeration<QuantityComparator> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<QuantityComparator>(this); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("<".equals(codeString)) 154 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_THAN); 155 if ("<=".equals(codeString)) 156 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_OR_EQUAL); 157 if (">=".equals(codeString)) 158 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_OR_EQUAL); 159 if (">".equals(codeString)) 160 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_THAN); 161 throw new FHIRException("Unknown QuantityComparator code '"+codeString+"'"); 162 } 163 public String toCode(QuantityComparator code) { 164 if (code == QuantityComparator.NULL) 165 return null; 166 if (code == QuantityComparator.LESS_THAN) 167 return "<"; 168 if (code == QuantityComparator.LESS_OR_EQUAL) 169 return "<="; 170 if (code == QuantityComparator.GREATER_OR_EQUAL) 171 return ">="; 172 if (code == QuantityComparator.GREATER_THAN) 173 return ">"; 174 return "?"; 175 } 176 public String toSystem(QuantityComparator code) { 177 return code.getSystem(); 178 } 179 } 180 181 /** 182 * The value of the measured amount. The value includes an implicit precision in the presentation of the value. 183 */ 184 @Child(name = "value", type = {DecimalType.class}, order=0, min=0, max=1, modifier=false, summary=true) 185 @Description(shortDefinition="Numerical value (with implicit precision)", formalDefinition="The value of the measured amount. The value includes an implicit precision in the presentation of the value." ) 186 protected DecimalType value; 187 188 /** 189 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 190 */ 191 @Child(name = "comparator", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 192 @Description(shortDefinition="< | <= | >= | > - how to understand the value", formalDefinition="How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/quantity-comparator") 194 protected Enumeration<QuantityComparator> comparator; 195 196 /** 197 * A human-readable form of the unit. 198 */ 199 @Child(name = "unit", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 200 @Description(shortDefinition="Unit representation", formalDefinition="A human-readable form of the unit." ) 201 protected StringType unit; 202 203 /** 204 * The identification of the system that provides the coded form of the unit. 205 */ 206 @Child(name = "system", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=true) 207 @Description(shortDefinition="System that defines coded unit form", formalDefinition="The identification of the system that provides the coded form of the unit." ) 208 protected UriType system; 209 210 /** 211 * A computer processable form of the unit in some unit representation system. 212 */ 213 @Child(name = "code", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 214 @Description(shortDefinition="Coded form of the unit", formalDefinition="A computer processable form of the unit in some unit representation system." ) 215 protected CodeType code; 216 217 private static final long serialVersionUID = 1069574054L; 218 219 /** 220 * Constructor 221 */ 222 public Quantity() { 223 super(); 224 } 225 226 /** 227 * Convenience constructor 228 * 229 * @param theValue The {@link #setValue(double) value} 230 */ 231 public Quantity(double theValue) { 232 setValue(theValue); 233 } 234 235 /** 236 * Convenience constructor 237 * 238 * @param theValue The {@link #setValue(long) value} 239 */ 240 public Quantity(long theValue) { 241 setValue(theValue); 242 } 243 244 /** 245 * Convenience constructor 246 * 247 * @param theComparator The {@link #setComparator(QuantityComparator) comparator} 248 * @param theValue The {@link #setValue(BigDecimal) value} 249 * @param theSystem The {@link #setSystem(String)} (the code system for the units} 250 * @param theCode The {@link #setCode(String)} (the code for the units} 251 * @param theUnit The {@link #setUnit(String)} (the human readable display name for the units} 252 */ 253 public Quantity(QuantityComparator theComparator, double theValue, String theSystem, String theCode, String theUnit) { 254 setValue(theValue); 255 setComparator(theComparator); 256 setSystem(theSystem); 257 setCode(theCode); 258 setUnit(theUnit); 259 } 260 261 /** 262 * Convenience constructor 263 * 264 * @param theComparator The {@link #setComparator(QuantityComparator) comparator} 265 * @param theValue The {@link #setValue(BigDecimal) value} 266 * @param theSystem The {@link #setSystem(String)} (the code system for the units} 267 * @param theCode The {@link #setCode(String)} (the code for the units} 268 * @param theUnit The {@link #setUnit(String)} (the human readable display name for the units} 269 */ 270 public Quantity(QuantityComparator theComparator, long theValue, String theSystem, String theCode, String theUnit) { 271 setValue(theValue); 272 setComparator(theComparator); 273 setSystem(theSystem); 274 setCode(theCode); 275 setUnit(theUnit); 276 } 277 /** 278 * @return {@link #value} (The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 279 */ 280 public DecimalType getValueElement() { 281 if (this.value == null) 282 if (Configuration.errorOnAutoCreate()) 283 throw new Error("Attempt to auto-create Quantity.value"); 284 else if (Configuration.doAutoCreate()) 285 this.value = new DecimalType(); // bb 286 return this.value; 287 } 288 289 public boolean hasValueElement() { 290 return this.value != null && !this.value.isEmpty(); 291 } 292 293 public boolean hasValue() { 294 return this.value != null && !this.value.isEmpty(); 295 } 296 297 /** 298 * @param value {@link #value} (The value of the measured amount. The value includes an implicit precision in the presentation of the value.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 299 */ 300 public Quantity setValueElement(DecimalType value) { 301 this.value = value; 302 return this; 303 } 304 305 /** 306 * @return The value of the measured amount. The value includes an implicit precision in the presentation of the value. 307 */ 308 public BigDecimal getValue() { 309 return this.value == null ? null : this.value.getValue(); 310 } 311 312 /** 313 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 314 */ 315 public Quantity setValue(BigDecimal value) { 316 if (value == null) 317 this.value = null; 318 else { 319 if (this.value == null) 320 this.value = new DecimalType(); 321 this.value.setValue(value); 322 } 323 return this; 324 } 325 326 /** 327 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 328 */ 329 public Quantity setValue(long value) { 330 this.value = new DecimalType(); 331 this.value.setValue(value); 332 return this; 333 } 334 335 /** 336 * @param value The value of the measured amount. The value includes an implicit precision in the presentation of the value. 337 */ 338 public Quantity setValue(double value) { 339 this.value = new DecimalType(); 340 this.value.setValue(value); 341 return this; 342 } 343 344 /** 345 * @return {@link #comparator} (How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 346 */ 347 public Enumeration<QuantityComparator> getComparatorElement() { 348 if (this.comparator == null) 349 if (Configuration.errorOnAutoCreate()) 350 throw new Error("Attempt to auto-create Quantity.comparator"); 351 else if (Configuration.doAutoCreate()) 352 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); // bb 353 return this.comparator; 354 } 355 356 public boolean hasComparatorElement() { 357 return this.comparator != null && !this.comparator.isEmpty(); 358 } 359 360 public boolean hasComparator() { 361 return this.comparator != null && !this.comparator.isEmpty(); 362 } 363 364 /** 365 * @param value {@link #comparator} (How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 366 */ 367 public Quantity setComparatorElement(Enumeration<QuantityComparator> value) { 368 this.comparator = value; 369 return this; 370 } 371 372 /** 373 * @return How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 374 */ 375 public QuantityComparator getComparator() { 376 return this.comparator == null ? null : this.comparator.getValue(); 377 } 378 379 /** 380 * @param value How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is "<" , then the real value is < stated value. 381 */ 382 public Quantity setComparator(QuantityComparator value) { 383 if (value == null) 384 this.comparator = null; 385 else { 386 if (this.comparator == null) 387 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); 388 this.comparator.setValue(value); 389 } 390 return this; 391 } 392 393 /** 394 * @return {@link #unit} (A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 395 */ 396 public StringType getUnitElement() { 397 if (this.unit == null) 398 if (Configuration.errorOnAutoCreate()) 399 throw new Error("Attempt to auto-create Quantity.unit"); 400 else if (Configuration.doAutoCreate()) 401 this.unit = new StringType(); // bb 402 return this.unit; 403 } 404 405 public boolean hasUnitElement() { 406 return this.unit != null && !this.unit.isEmpty(); 407 } 408 409 public boolean hasUnit() { 410 return this.unit != null && !this.unit.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #unit} (A human-readable form of the unit.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 415 */ 416 public Quantity setUnitElement(StringType value) { 417 this.unit = value; 418 return this; 419 } 420 421 /** 422 * @return A human-readable form of the unit. 423 */ 424 public String getUnit() { 425 return this.unit == null ? null : this.unit.getValue(); 426 } 427 428 /** 429 * @param value A human-readable form of the unit. 430 */ 431 public Quantity setUnit(String value) { 432 if (Utilities.noString(value)) 433 this.unit = null; 434 else { 435 if (this.unit == null) 436 this.unit = new StringType(); 437 this.unit.setValue(value); 438 } 439 return this; 440 } 441 442 /** 443 * @return {@link #system} (The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 444 */ 445 public UriType getSystemElement() { 446 if (this.system == null) 447 if (Configuration.errorOnAutoCreate()) 448 throw new Error("Attempt to auto-create Quantity.system"); 449 else if (Configuration.doAutoCreate()) 450 this.system = new UriType(); // bb 451 return this.system; 452 } 453 454 public boolean hasSystemElement() { 455 return this.system != null && !this.system.isEmpty(); 456 } 457 458 public boolean hasSystem() { 459 return this.system != null && !this.system.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #system} (The identification of the system that provides the coded form of the unit.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 464 */ 465 public Quantity setSystemElement(UriType value) { 466 this.system = value; 467 return this; 468 } 469 470 /** 471 * @return The identification of the system that provides the coded form of the unit. 472 */ 473 public String getSystem() { 474 return this.system == null ? null : this.system.getValue(); 475 } 476 477 /** 478 * @param value The identification of the system that provides the coded form of the unit. 479 */ 480 public Quantity setSystem(String value) { 481 if (Utilities.noString(value)) 482 this.system = null; 483 else { 484 if (this.system == null) 485 this.system = new UriType(); 486 this.system.setValue(value); 487 } 488 return this; 489 } 490 491 /** 492 * @return {@link #code} (A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 493 */ 494 public CodeType getCodeElement() { 495 if (this.code == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create Quantity.code"); 498 else if (Configuration.doAutoCreate()) 499 this.code = new CodeType(); // bb 500 return this.code; 501 } 502 503 public boolean hasCodeElement() { 504 return this.code != null && !this.code.isEmpty(); 505 } 506 507 public boolean hasCode() { 508 return this.code != null && !this.code.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #code} (A computer processable form of the unit in some unit representation system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 513 */ 514 public Quantity setCodeElement(CodeType value) { 515 this.code = value; 516 return this; 517 } 518 519 /** 520 * @return A computer processable form of the unit in some unit representation system. 521 */ 522 public String getCode() { 523 return this.code == null ? null : this.code.getValue(); 524 } 525 526 /** 527 * @param value A computer processable form of the unit in some unit representation system. 528 */ 529 public Quantity setCode(String value) { 530 if (Utilities.noString(value)) 531 this.code = null; 532 else { 533 if (this.code == null) 534 this.code = new CodeType(); 535 this.code.setValue(value); 536 } 537 return this; 538 } 539 540 protected void listChildren(List<Property> children) { 541 super.listChildren(children); 542 children.add(new Property("value", "decimal", "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 0, 1, value)); 543 children.add(new Property("comparator", "code", "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 0, 1, comparator)); 544 children.add(new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit)); 545 children.add(new Property("system", "uri", "The identification of the system that provides the coded form of the unit.", 0, 1, system)); 546 children.add(new Property("code", "code", "A computer processable form of the unit in some unit representation system.", 0, 1, code)); 547 } 548 549 @Override 550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 551 switch (_hash) { 552 case 111972721: /*value*/ return new Property("value", "decimal", "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 0, 1, value); 553 case -844673834: /*comparator*/ return new Property("comparator", "code", "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 0, 1, comparator); 554 case 3594628: /*unit*/ return new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit); 555 case -887328209: /*system*/ return new Property("system", "uri", "The identification of the system that provides the coded form of the unit.", 0, 1, system); 556 case 3059181: /*code*/ return new Property("code", "code", "A computer processable form of the unit in some unit representation system.", 0, 1, code); 557 default: return super.getNamedProperty(_hash, _name, _checkValid); 558 } 559 560 } 561 562 @Override 563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 564 switch (hash) { 565 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 566 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : new Base[] {this.comparator}; // Enumeration<QuantityComparator> 567 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // StringType 568 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 569 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 570 default: return super.getProperty(hash, name, checkValid); 571 } 572 573 } 574 575 @Override 576 public Base setProperty(int hash, String name, Base value) throws FHIRException { 577 switch (hash) { 578 case 111972721: // value 579 this.value = castToDecimal(value); // DecimalType 580 return value; 581 case -844673834: // comparator 582 value = new QuantityComparatorEnumFactory().fromType(castToCode(value)); 583 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 584 return value; 585 case 3594628: // unit 586 this.unit = castToString(value); // StringType 587 return value; 588 case -887328209: // system 589 this.system = castToUri(value); // UriType 590 return value; 591 case 3059181: // code 592 this.code = castToCode(value); // CodeType 593 return value; 594 default: return super.setProperty(hash, name, value); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(String name, Base value) throws FHIRException { 601 if (name.equals("value")) { 602 this.value = castToDecimal(value); // DecimalType 603 } else if (name.equals("comparator")) { 604 value = new QuantityComparatorEnumFactory().fromType(castToCode(value)); 605 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 606 } else if (name.equals("unit")) { 607 this.unit = castToString(value); // StringType 608 } else if (name.equals("system")) { 609 this.system = castToUri(value); // UriType 610 } else if (name.equals("code")) { 611 this.code = castToCode(value); // CodeType 612 } else 613 return super.setProperty(name, value); 614 return value; 615 } 616 617 @Override 618 public Base makeProperty(int hash, String name) throws FHIRException { 619 switch (hash) { 620 case 111972721: return getValueElement(); 621 case -844673834: return getComparatorElement(); 622 case 3594628: return getUnitElement(); 623 case -887328209: return getSystemElement(); 624 case 3059181: return getCodeElement(); 625 default: return super.makeProperty(hash, name); 626 } 627 628 } 629 630 @Override 631 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 632 switch (hash) { 633 case 111972721: /*value*/ return new String[] {"decimal"}; 634 case -844673834: /*comparator*/ return new String[] {"code"}; 635 case 3594628: /*unit*/ return new String[] {"string"}; 636 case -887328209: /*system*/ return new String[] {"uri"}; 637 case 3059181: /*code*/ return new String[] {"code"}; 638 default: return super.getTypesForProperty(hash, name); 639 } 640 641 } 642 643 @Override 644 public Base addChild(String name) throws FHIRException { 645 if (name.equals("value")) { 646 throw new FHIRException("Cannot call addChild on a singleton property Quantity.value"); 647 } 648 else if (name.equals("comparator")) { 649 throw new FHIRException("Cannot call addChild on a singleton property Quantity.comparator"); 650 } 651 else if (name.equals("unit")) { 652 throw new FHIRException("Cannot call addChild on a singleton property Quantity.unit"); 653 } 654 else if (name.equals("system")) { 655 throw new FHIRException("Cannot call addChild on a singleton property Quantity.system"); 656 } 657 else if (name.equals("code")) { 658 throw new FHIRException("Cannot call addChild on a singleton property Quantity.code"); 659 } 660 else 661 return super.addChild(name); 662 } 663 664 public String fhirType() { 665 return "Quantity"; 666 667 } 668 669 public Quantity copy() { 670 Quantity dst = new Quantity(); 671 copyValues(dst); 672 return dst; 673 } 674 675 public void copyValues(Quantity dst) { 676 super.copyValues(dst); 677 dst.value = value == null ? null : value.copy(); 678 dst.comparator = comparator == null ? null : comparator.copy(); 679 dst.unit = unit == null ? null : unit.copy(); 680 dst.system = system == null ? null : system.copy(); 681 dst.code = code == null ? null : code.copy(); 682 } 683 684 protected Quantity typedCopy() { 685 return copy(); 686 } 687 688 @Override 689 public boolean equalsDeep(Base other_) { 690 if (!super.equalsDeep(other_)) 691 return false; 692 if (!(other_ instanceof Quantity)) 693 return false; 694 Quantity o = (Quantity) other_; 695 return compareDeep(value, o.value, true) && compareDeep(comparator, o.comparator, true) && compareDeep(unit, o.unit, true) 696 && compareDeep(system, o.system, true) && compareDeep(code, o.code, true); 697 } 698 699 @Override 700 public boolean equalsShallow(Base other_) { 701 if (!super.equalsShallow(other_)) 702 return false; 703 if (!(other_ instanceof Quantity)) 704 return false; 705 Quantity o = (Quantity) other_; 706 return compareValues(value, o.value, true) && compareValues(comparator, o.comparator, true) && compareValues(unit, o.unit, true) 707 && compareValues(system, o.system, true) && compareValues(code, o.code, true); 708 } 709 710 public boolean isEmpty() { 711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, comparator, unit 712 , system, code); 713 } 714 715 716}