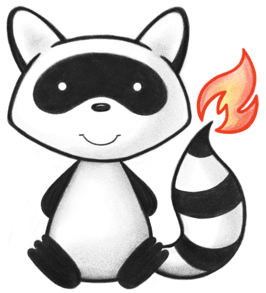
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.exceptions.FHIRFormatError; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053/** 054 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 055 */ 056@ResourceDef(name="Questionnaire", profile="http://hl7.org/fhir/Profile/Questionnaire") 057@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "description", "purpose", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "contact", "copyright", "code", "subjectType", "item"}) 058public class Questionnaire extends MetadataResource { 059 060 public enum QuestionnaireItemType { 061 /** 062 * An item with no direct answer but should have at least one child item. 063 */ 064 GROUP, 065 /** 066 * Text for display that will not capture an answer or have child items. 067 */ 068 DISPLAY, 069 /** 070 * An item that defines a specific answer to be captured, and may have child items. 071(the answer provided in the QuestionnaireResponse should be of the defined datatype) 072 */ 073 QUESTION, 074 /** 075 * Question with a yes/no answer (valueBoolean) 076 */ 077 BOOLEAN, 078 /** 079 * Question with is a real number answer (valueDecimal) 080 */ 081 DECIMAL, 082 /** 083 * Question with an integer answer (valueInteger) 084 */ 085 INTEGER, 086 /** 087 * Question with a date answer (valueDate) 088 */ 089 DATE, 090 /** 091 * Question with a date and time answer (valueDateTime) 092 */ 093 DATETIME, 094 /** 095 * Question with a time (hour:minute:second) answer independent of date. (valueTime) 096 */ 097 TIME, 098 /** 099 * Question with a short (few words to short sentence) free-text entry answer (valueString) 100 */ 101 STRING, 102 /** 103 * Question with a long (potentially multi-paragraph) free-text entry answer (valueString) 104 */ 105 TEXT, 106 /** 107 * Question with a URL (website, FTP site, etc.) answer (valueUri) 108 */ 109 URL, 110 /** 111 * Question with a Coding drawn from a list of options (specified in either the option property, or via the valueset referenced in the options property) as an answer (valueCoding) 112 */ 113 CHOICE, 114 /** 115 * Answer is a Coding drawn from a list of options (as with the choice type) or a free-text entry in a string (valueCoding or valueString) 116 */ 117 OPENCHOICE, 118 /** 119 * Question with binary content such as a image, PDF, etc. as an answer (valueAttachment) 120 */ 121 ATTACHMENT, 122 /** 123 * Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference) 124 */ 125 REFERENCE, 126 /** 127 * Question with a combination of a numeric value and unit, potentially with a comparator (<, >, etc.) as an answer. (valueQuantity) 128There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to define what unit whould be captured (or the a unit that has a ucum conversion from the provided unit) 129 */ 130 QUANTITY, 131 /** 132 * added to help the parsers with the generic types 133 */ 134 NULL; 135 public static QuestionnaireItemType fromCode(String codeString) throws FHIRException { 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("group".equals(codeString)) 139 return GROUP; 140 if ("display".equals(codeString)) 141 return DISPLAY; 142 if ("question".equals(codeString)) 143 return QUESTION; 144 if ("boolean".equals(codeString)) 145 return BOOLEAN; 146 if ("decimal".equals(codeString)) 147 return DECIMAL; 148 if ("integer".equals(codeString)) 149 return INTEGER; 150 if ("date".equals(codeString)) 151 return DATE; 152 if ("dateTime".equals(codeString)) 153 return DATETIME; 154 if ("time".equals(codeString)) 155 return TIME; 156 if ("string".equals(codeString)) 157 return STRING; 158 if ("text".equals(codeString)) 159 return TEXT; 160 if ("url".equals(codeString)) 161 return URL; 162 if ("choice".equals(codeString)) 163 return CHOICE; 164 if ("open-choice".equals(codeString)) 165 return OPENCHOICE; 166 if ("attachment".equals(codeString)) 167 return ATTACHMENT; 168 if ("reference".equals(codeString)) 169 return REFERENCE; 170 if ("quantity".equals(codeString)) 171 return QUANTITY; 172 if (Configuration.isAcceptInvalidEnums()) 173 return null; 174 else 175 throw new FHIRException("Unknown QuestionnaireItemType code '"+codeString+"'"); 176 } 177 public String toCode() { 178 switch (this) { 179 case GROUP: return "group"; 180 case DISPLAY: return "display"; 181 case QUESTION: return "question"; 182 case BOOLEAN: return "boolean"; 183 case DECIMAL: return "decimal"; 184 case INTEGER: return "integer"; 185 case DATE: return "date"; 186 case DATETIME: return "dateTime"; 187 case TIME: return "time"; 188 case STRING: return "string"; 189 case TEXT: return "text"; 190 case URL: return "url"; 191 case CHOICE: return "choice"; 192 case OPENCHOICE: return "open-choice"; 193 case ATTACHMENT: return "attachment"; 194 case REFERENCE: return "reference"; 195 case QUANTITY: return "quantity"; 196 case NULL: return null; 197 default: return "?"; 198 } 199 } 200 public String getSystem() { 201 switch (this) { 202 case GROUP: return "http://hl7.org/fhir/item-type"; 203 case DISPLAY: return "http://hl7.org/fhir/item-type"; 204 case QUESTION: return "http://hl7.org/fhir/item-type"; 205 case BOOLEAN: return "http://hl7.org/fhir/item-type"; 206 case DECIMAL: return "http://hl7.org/fhir/item-type"; 207 case INTEGER: return "http://hl7.org/fhir/item-type"; 208 case DATE: return "http://hl7.org/fhir/item-type"; 209 case DATETIME: return "http://hl7.org/fhir/item-type"; 210 case TIME: return "http://hl7.org/fhir/item-type"; 211 case STRING: return "http://hl7.org/fhir/item-type"; 212 case TEXT: return "http://hl7.org/fhir/item-type"; 213 case URL: return "http://hl7.org/fhir/item-type"; 214 case CHOICE: return "http://hl7.org/fhir/item-type"; 215 case OPENCHOICE: return "http://hl7.org/fhir/item-type"; 216 case ATTACHMENT: return "http://hl7.org/fhir/item-type"; 217 case REFERENCE: return "http://hl7.org/fhir/item-type"; 218 case QUANTITY: return "http://hl7.org/fhir/item-type"; 219 case NULL: return null; 220 default: return "?"; 221 } 222 } 223 public String getDefinition() { 224 switch (this) { 225 case GROUP: return "An item with no direct answer but should have at least one child item."; 226 case DISPLAY: return "Text for display that will not capture an answer or have child items."; 227 case QUESTION: return "An item that defines a specific answer to be captured, and may have child items.\n(the answer provided in the QuestionnaireResponse should be of the defined datatype)"; 228 case BOOLEAN: return "Question with a yes/no answer (valueBoolean)"; 229 case DECIMAL: return "Question with is a real number answer (valueDecimal)"; 230 case INTEGER: return "Question with an integer answer (valueInteger)"; 231 case DATE: return "Question with a date answer (valueDate)"; 232 case DATETIME: return "Question with a date and time answer (valueDateTime)"; 233 case TIME: return "Question with a time (hour:minute:second) answer independent of date. (valueTime)"; 234 case STRING: return "Question with a short (few words to short sentence) free-text entry answer (valueString)"; 235 case TEXT: return "Question with a long (potentially multi-paragraph) free-text entry answer (valueString)"; 236 case URL: return "Question with a URL (website, FTP site, etc.) answer (valueUri)"; 237 case CHOICE: return "Question with a Coding drawn from a list of options (specified in either the option property, or via the valueset referenced in the options property) as an answer (valueCoding)"; 238 case OPENCHOICE: return "Answer is a Coding drawn from a list of options (as with the choice type) or a free-text entry in a string (valueCoding or valueString)"; 239 case ATTACHMENT: return "Question with binary content such as a image, PDF, etc. as an answer (valueAttachment)"; 240 case REFERENCE: return "Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference)"; 241 case QUANTITY: return "Question with a combination of a numeric value and unit, potentially with a comparator (<, >, etc.) as an answer. (valueQuantity)\nThere is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to define what unit whould be captured (or the a unit that has a ucum conversion from the provided unit)"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 public String getDisplay() { 247 switch (this) { 248 case GROUP: return "Group"; 249 case DISPLAY: return "Display"; 250 case QUESTION: return "Question"; 251 case BOOLEAN: return "Boolean"; 252 case DECIMAL: return "Decimal"; 253 case INTEGER: return "Integer"; 254 case DATE: return "Date"; 255 case DATETIME: return "Date Time"; 256 case TIME: return "Time"; 257 case STRING: return "String"; 258 case TEXT: return "Text"; 259 case URL: return "Url"; 260 case CHOICE: return "Choice"; 261 case OPENCHOICE: return "Open Choice"; 262 case ATTACHMENT: return "Attachment"; 263 case REFERENCE: return "Reference"; 264 case QUANTITY: return "Quantity"; 265 case NULL: return null; 266 default: return "?"; 267 } 268 } 269 } 270 271 public static class QuestionnaireItemTypeEnumFactory implements EnumFactory<QuestionnaireItemType> { 272 public QuestionnaireItemType fromCode(String codeString) throws IllegalArgumentException { 273 if (codeString == null || "".equals(codeString)) 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("group".equals(codeString)) 277 return QuestionnaireItemType.GROUP; 278 if ("display".equals(codeString)) 279 return QuestionnaireItemType.DISPLAY; 280 if ("question".equals(codeString)) 281 return QuestionnaireItemType.QUESTION; 282 if ("boolean".equals(codeString)) 283 return QuestionnaireItemType.BOOLEAN; 284 if ("decimal".equals(codeString)) 285 return QuestionnaireItemType.DECIMAL; 286 if ("integer".equals(codeString)) 287 return QuestionnaireItemType.INTEGER; 288 if ("date".equals(codeString)) 289 return QuestionnaireItemType.DATE; 290 if ("dateTime".equals(codeString)) 291 return QuestionnaireItemType.DATETIME; 292 if ("time".equals(codeString)) 293 return QuestionnaireItemType.TIME; 294 if ("string".equals(codeString)) 295 return QuestionnaireItemType.STRING; 296 if ("text".equals(codeString)) 297 return QuestionnaireItemType.TEXT; 298 if ("url".equals(codeString)) 299 return QuestionnaireItemType.URL; 300 if ("choice".equals(codeString)) 301 return QuestionnaireItemType.CHOICE; 302 if ("open-choice".equals(codeString)) 303 return QuestionnaireItemType.OPENCHOICE; 304 if ("attachment".equals(codeString)) 305 return QuestionnaireItemType.ATTACHMENT; 306 if ("reference".equals(codeString)) 307 return QuestionnaireItemType.REFERENCE; 308 if ("quantity".equals(codeString)) 309 return QuestionnaireItemType.QUANTITY; 310 throw new IllegalArgumentException("Unknown QuestionnaireItemType code '"+codeString+"'"); 311 } 312 public Enumeration<QuestionnaireItemType> fromType(PrimitiveType<?> code) throws FHIRException { 313 if (code == null) 314 return null; 315 if (code.isEmpty()) 316 return new Enumeration<QuestionnaireItemType>(this); 317 String codeString = code.asStringValue(); 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("group".equals(codeString)) 321 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.GROUP); 322 if ("display".equals(codeString)) 323 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DISPLAY); 324 if ("question".equals(codeString)) 325 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUESTION); 326 if ("boolean".equals(codeString)) 327 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.BOOLEAN); 328 if ("decimal".equals(codeString)) 329 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DECIMAL); 330 if ("integer".equals(codeString)) 331 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.INTEGER); 332 if ("date".equals(codeString)) 333 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATE); 334 if ("dateTime".equals(codeString)) 335 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATETIME); 336 if ("time".equals(codeString)) 337 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TIME); 338 if ("string".equals(codeString)) 339 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.STRING); 340 if ("text".equals(codeString)) 341 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TEXT); 342 if ("url".equals(codeString)) 343 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.URL); 344 if ("choice".equals(codeString)) 345 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.CHOICE); 346 if ("open-choice".equals(codeString)) 347 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.OPENCHOICE); 348 if ("attachment".equals(codeString)) 349 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.ATTACHMENT); 350 if ("reference".equals(codeString)) 351 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.REFERENCE); 352 if ("quantity".equals(codeString)) 353 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUANTITY); 354 throw new FHIRException("Unknown QuestionnaireItemType code '"+codeString+"'"); 355 } 356 public String toCode(QuestionnaireItemType code) { 357 if (code == QuestionnaireItemType.NULL) 358 return null; 359 if (code == QuestionnaireItemType.GROUP) 360 return "group"; 361 if (code == QuestionnaireItemType.DISPLAY) 362 return "display"; 363 if (code == QuestionnaireItemType.QUESTION) 364 return "question"; 365 if (code == QuestionnaireItemType.BOOLEAN) 366 return "boolean"; 367 if (code == QuestionnaireItemType.DECIMAL) 368 return "decimal"; 369 if (code == QuestionnaireItemType.INTEGER) 370 return "integer"; 371 if (code == QuestionnaireItemType.DATE) 372 return "date"; 373 if (code == QuestionnaireItemType.DATETIME) 374 return "dateTime"; 375 if (code == QuestionnaireItemType.TIME) 376 return "time"; 377 if (code == QuestionnaireItemType.STRING) 378 return "string"; 379 if (code == QuestionnaireItemType.TEXT) 380 return "text"; 381 if (code == QuestionnaireItemType.URL) 382 return "url"; 383 if (code == QuestionnaireItemType.CHOICE) 384 return "choice"; 385 if (code == QuestionnaireItemType.OPENCHOICE) 386 return "open-choice"; 387 if (code == QuestionnaireItemType.ATTACHMENT) 388 return "attachment"; 389 if (code == QuestionnaireItemType.REFERENCE) 390 return "reference"; 391 if (code == QuestionnaireItemType.QUANTITY) 392 return "quantity"; 393 return "?"; 394 } 395 public String toSystem(QuestionnaireItemType code) { 396 return code.getSystem(); 397 } 398 } 399 400 @Block() 401 public static class QuestionnaireItemComponent extends BackboneElement implements IBaseBackboneElement { 402 /** 403 * An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 404 */ 405 @Child(name = "linkId", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 406 @Description(shortDefinition="Unique id for item in questionnaire", formalDefinition="An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource." ) 407 protected StringType linkId; 408 409 /** 410 * A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: 411 412* code (ElementDefinition.code) 413* type (ElementDefinition.type) 414* required (ElementDefinition.min) 415* repeats (ElementDefinition.max) 416* maxLength (ElementDefinition.maxLength) 417* options (ElementDefinition.binding) 418 419Any information provided in these elements on a Questionnaire Item overrides the information from the definition. 420 */ 421 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 422 @Description(shortDefinition="ElementDefinition - details for the item", formalDefinition="A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: \n\n* code (ElementDefinition.code)\n* type (ElementDefinition.type)\n* required (ElementDefinition.min)\n* repeats (ElementDefinition.max)\n* maxLength (ElementDefinition.maxLength)\n* options (ElementDefinition.binding)\n\nAny information provided in these elements on a Questionnaire Item overrides the information from the definition." ) 423 protected UriType definition; 424 425 /** 426 * A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers). 427 */ 428 @Child(name = "code", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 429 @Description(shortDefinition="Corresponding concept for this item in a terminology", formalDefinition="A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers)." ) 430 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-questions") 431 protected List<Coding> code; 432 433 /** 434 * A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 435 */ 436 @Child(name = "prefix", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 437 @Description(shortDefinition="E.g. \"1(a)\", \"2.5.3\"", formalDefinition="A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire." ) 438 protected StringType prefix; 439 440 /** 441 * The name of a section, the text of a question or text content for a display item. 442 */ 443 @Child(name = "text", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 444 @Description(shortDefinition="Primary text for the item", formalDefinition="The name of a section, the text of a question or text content for a display item." ) 445 protected StringType text; 446 447 /** 448 * The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.). 449 */ 450 @Child(name = "type", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=false) 451 @Description(shortDefinition="group | display | boolean | decimal | integer | date | dateTime +", formalDefinition="The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.)." ) 452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/item-type") 453 protected Enumeration<QuestionnaireItemType> type; 454 455 /** 456 * A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true. 457 */ 458 @Child(name = "enableWhen", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 459 @Description(shortDefinition="Only allow data when", formalDefinition="A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true." ) 460 protected List<QuestionnaireItemEnableWhenComponent> enableWhen; 461 462 /** 463 * An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 464 */ 465 @Child(name = "required", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 466 @Description(shortDefinition="Whether the item must be included in data results", formalDefinition="An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire." ) 467 protected BooleanType required; 468 469 /** 470 * An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups. 471 */ 472 @Child(name = "repeats", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 473 @Description(shortDefinition="Whether the item may repeat", formalDefinition="An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups." ) 474 protected BooleanType repeats; 475 476 /** 477 * An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 478 */ 479 @Child(name = "readOnly", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=false) 480 @Description(shortDefinition="Don't allow human editing", formalDefinition="An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire." ) 481 protected BooleanType readOnly; 482 483 /** 484 * The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 485 */ 486 @Child(name = "maxLength", type = {IntegerType.class}, order=11, min=0, max=1, modifier=false, summary=false) 487 @Description(shortDefinition="No more than this many characters", formalDefinition="The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse." ) 488 protected IntegerType maxLength; 489 490 /** 491 * A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question. 492 */ 493 @Child(name = "options", type = {ValueSet.class}, order=12, min=0, max=1, modifier=false, summary=false) 494 @Description(shortDefinition="Valueset containing permitted answers", formalDefinition="A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question." ) 495 protected Reference options; 496 497 /** 498 * The actual object that is the target of the reference (A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question.) 499 */ 500 protected ValueSet optionsTarget; 501 502 /** 503 * One of the permitted answers for a "choice" or "open-choice" question. 504 */ 505 @Child(name = "option", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 506 @Description(shortDefinition="Permitted answer", formalDefinition="One of the permitted answers for a \"choice\" or \"open-choice\" question." ) 507 protected List<QuestionnaireItemOptionComponent> option; 508 509 /** 510 * The value that should be defaulted when initially rendering the questionnaire for user input. 511 */ 512 @Child(name = "initial", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=14, min=0, max=1, modifier=false, summary=false) 513 @Description(shortDefinition="Default value when item is first rendered", formalDefinition="The value that should be defaulted when initially rendering the questionnaire for user input." ) 514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 515 protected Type initial; 516 517 /** 518 * Text, questions and other groups to be nested beneath a question or group. 519 */ 520 @Child(name = "item", type = {QuestionnaireItemComponent.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 521 @Description(shortDefinition="Nested questionnaire items", formalDefinition="Text, questions and other groups to be nested beneath a question or group." ) 522 protected List<QuestionnaireItemComponent> item; 523 524 private static final long serialVersionUID = -1997112302L; 525 526 /** 527 * Constructor 528 */ 529 public QuestionnaireItemComponent() { 530 super(); 531 } 532 533 /** 534 * Constructor 535 */ 536 public QuestionnaireItemComponent(StringType linkId, Enumeration<QuestionnaireItemType> type) { 537 super(); 538 this.linkId = linkId; 539 this.type = type; 540 } 541 542 /** 543 * @return {@link #linkId} (An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 544 */ 545 public StringType getLinkIdElement() { 546 if (this.linkId == null) 547 if (Configuration.errorOnAutoCreate()) 548 throw new Error("Attempt to auto-create QuestionnaireItemComponent.linkId"); 549 else if (Configuration.doAutoCreate()) 550 this.linkId = new StringType(); // bb 551 return this.linkId; 552 } 553 554 public boolean hasLinkIdElement() { 555 return this.linkId != null && !this.linkId.isEmpty(); 556 } 557 558 public boolean hasLinkId() { 559 return this.linkId != null && !this.linkId.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #linkId} (An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 564 */ 565 public QuestionnaireItemComponent setLinkIdElement(StringType value) { 566 this.linkId = value; 567 return this; 568 } 569 570 /** 571 * @return An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 572 */ 573 public String getLinkId() { 574 return this.linkId == null ? null : this.linkId.getValue(); 575 } 576 577 /** 578 * @param value An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource. 579 */ 580 public QuestionnaireItemComponent setLinkId(String value) { 581 if (this.linkId == null) 582 this.linkId = new StringType(); 583 this.linkId.setValue(value); 584 return this; 585 } 586 587 /** 588 * @return {@link #definition} (A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: 589 590* code (ElementDefinition.code) 591* type (ElementDefinition.type) 592* required (ElementDefinition.min) 593* repeats (ElementDefinition.max) 594* maxLength (ElementDefinition.maxLength) 595* options (ElementDefinition.binding) 596 597Any information provided in these elements on a Questionnaire Item overrides the information from the definition.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 598 */ 599 public UriType getDefinitionElement() { 600 if (this.definition == null) 601 if (Configuration.errorOnAutoCreate()) 602 throw new Error("Attempt to auto-create QuestionnaireItemComponent.definition"); 603 else if (Configuration.doAutoCreate()) 604 this.definition = new UriType(); // bb 605 return this.definition; 606 } 607 608 public boolean hasDefinitionElement() { 609 return this.definition != null && !this.definition.isEmpty(); 610 } 611 612 public boolean hasDefinition() { 613 return this.definition != null && !this.definition.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #definition} (A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: 618 619* code (ElementDefinition.code) 620* type (ElementDefinition.type) 621* required (ElementDefinition.min) 622* repeats (ElementDefinition.max) 623* maxLength (ElementDefinition.maxLength) 624* options (ElementDefinition.binding) 625 626Any information provided in these elements on a Questionnaire Item overrides the information from the definition.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 627 */ 628 public QuestionnaireItemComponent setDefinitionElement(UriType value) { 629 this.definition = value; 630 return this; 631 } 632 633 /** 634 * @return A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: 635 636* code (ElementDefinition.code) 637* type (ElementDefinition.type) 638* required (ElementDefinition.min) 639* repeats (ElementDefinition.max) 640* maxLength (ElementDefinition.maxLength) 641* options (ElementDefinition.binding) 642 643Any information provided in these elements on a Questionnaire Item overrides the information from the definition. 644 */ 645 public String getDefinition() { 646 return this.definition == null ? null : this.definition.getValue(); 647 } 648 649 /** 650 * @param value A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: 651 652* code (ElementDefinition.code) 653* type (ElementDefinition.type) 654* required (ElementDefinition.min) 655* repeats (ElementDefinition.max) 656* maxLength (ElementDefinition.maxLength) 657* options (ElementDefinition.binding) 658 659Any information provided in these elements on a Questionnaire Item overrides the information from the definition. 660 */ 661 public QuestionnaireItemComponent setDefinition(String value) { 662 if (Utilities.noString(value)) 663 this.definition = null; 664 else { 665 if (this.definition == null) 666 this.definition = new UriType(); 667 this.definition.setValue(value); 668 } 669 return this; 670 } 671 672 /** 673 * @return {@link #code} (A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).) 674 */ 675 public List<Coding> getCode() { 676 if (this.code == null) 677 this.code = new ArrayList<Coding>(); 678 return this.code; 679 } 680 681 /** 682 * @return Returns a reference to <code>this</code> for easy method chaining 683 */ 684 public QuestionnaireItemComponent setCode(List<Coding> theCode) { 685 this.code = theCode; 686 return this; 687 } 688 689 public boolean hasCode() { 690 if (this.code == null) 691 return false; 692 for (Coding item : this.code) 693 if (!item.isEmpty()) 694 return true; 695 return false; 696 } 697 698 public Coding addCode() { //3 699 Coding t = new Coding(); 700 if (this.code == null) 701 this.code = new ArrayList<Coding>(); 702 this.code.add(t); 703 return t; 704 } 705 706 public QuestionnaireItemComponent addCode(Coding t) { //3 707 if (t == null) 708 return this; 709 if (this.code == null) 710 this.code = new ArrayList<Coding>(); 711 this.code.add(t); 712 return this; 713 } 714 715 /** 716 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 717 */ 718 public Coding getCodeFirstRep() { 719 if (getCode().isEmpty()) { 720 addCode(); 721 } 722 return getCode().get(0); 723 } 724 725 /** 726 * @return {@link #prefix} (A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 727 */ 728 public StringType getPrefixElement() { 729 if (this.prefix == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create QuestionnaireItemComponent.prefix"); 732 else if (Configuration.doAutoCreate()) 733 this.prefix = new StringType(); // bb 734 return this.prefix; 735 } 736 737 public boolean hasPrefixElement() { 738 return this.prefix != null && !this.prefix.isEmpty(); 739 } 740 741 public boolean hasPrefix() { 742 return this.prefix != null && !this.prefix.isEmpty(); 743 } 744 745 /** 746 * @param value {@link #prefix} (A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 747 */ 748 public QuestionnaireItemComponent setPrefixElement(StringType value) { 749 this.prefix = value; 750 return this; 751 } 752 753 /** 754 * @return A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 755 */ 756 public String getPrefix() { 757 return this.prefix == null ? null : this.prefix.getValue(); 758 } 759 760 /** 761 * @param value A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire. 762 */ 763 public QuestionnaireItemComponent setPrefix(String value) { 764 if (Utilities.noString(value)) 765 this.prefix = null; 766 else { 767 if (this.prefix == null) 768 this.prefix = new StringType(); 769 this.prefix.setValue(value); 770 } 771 return this; 772 } 773 774 /** 775 * @return {@link #text} (The name of a section, the text of a question or text content for a display item.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 776 */ 777 public StringType getTextElement() { 778 if (this.text == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create QuestionnaireItemComponent.text"); 781 else if (Configuration.doAutoCreate()) 782 this.text = new StringType(); // bb 783 return this.text; 784 } 785 786 public boolean hasTextElement() { 787 return this.text != null && !this.text.isEmpty(); 788 } 789 790 public boolean hasText() { 791 return this.text != null && !this.text.isEmpty(); 792 } 793 794 /** 795 * @param value {@link #text} (The name of a section, the text of a question or text content for a display item.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 796 */ 797 public QuestionnaireItemComponent setTextElement(StringType value) { 798 this.text = value; 799 return this; 800 } 801 802 /** 803 * @return The name of a section, the text of a question or text content for a display item. 804 */ 805 public String getText() { 806 return this.text == null ? null : this.text.getValue(); 807 } 808 809 /** 810 * @param value The name of a section, the text of a question or text content for a display item. 811 */ 812 public QuestionnaireItemComponent setText(String value) { 813 if (Utilities.noString(value)) 814 this.text = null; 815 else { 816 if (this.text == null) 817 this.text = new StringType(); 818 this.text.setValue(value); 819 } 820 return this; 821 } 822 823 /** 824 * @return {@link #type} (The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 825 */ 826 public Enumeration<QuestionnaireItemType> getTypeElement() { 827 if (this.type == null) 828 if (Configuration.errorOnAutoCreate()) 829 throw new Error("Attempt to auto-create QuestionnaireItemComponent.type"); 830 else if (Configuration.doAutoCreate()) 831 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); // bb 832 return this.type; 833 } 834 835 public boolean hasTypeElement() { 836 return this.type != null && !this.type.isEmpty(); 837 } 838 839 public boolean hasType() { 840 return this.type != null && !this.type.isEmpty(); 841 } 842 843 /** 844 * @param value {@link #type} (The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 845 */ 846 public QuestionnaireItemComponent setTypeElement(Enumeration<QuestionnaireItemType> value) { 847 this.type = value; 848 return this; 849 } 850 851 /** 852 * @return The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.). 853 */ 854 public QuestionnaireItemType getType() { 855 return this.type == null ? null : this.type.getValue(); 856 } 857 858 /** 859 * @param value The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.). 860 */ 861 public QuestionnaireItemComponent setType(QuestionnaireItemType value) { 862 if (this.type == null) 863 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); 864 this.type.setValue(value); 865 return this; 866 } 867 868 /** 869 * @return {@link #enableWhen} (A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.) 870 */ 871 public List<QuestionnaireItemEnableWhenComponent> getEnableWhen() { 872 if (this.enableWhen == null) 873 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 874 return this.enableWhen; 875 } 876 877 /** 878 * @return Returns a reference to <code>this</code> for easy method chaining 879 */ 880 public QuestionnaireItemComponent setEnableWhen(List<QuestionnaireItemEnableWhenComponent> theEnableWhen) { 881 this.enableWhen = theEnableWhen; 882 return this; 883 } 884 885 public boolean hasEnableWhen() { 886 if (this.enableWhen == null) 887 return false; 888 for (QuestionnaireItemEnableWhenComponent item : this.enableWhen) 889 if (!item.isEmpty()) 890 return true; 891 return false; 892 } 893 894 public QuestionnaireItemEnableWhenComponent addEnableWhen() { //3 895 QuestionnaireItemEnableWhenComponent t = new QuestionnaireItemEnableWhenComponent(); 896 if (this.enableWhen == null) 897 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 898 this.enableWhen.add(t); 899 return t; 900 } 901 902 public QuestionnaireItemComponent addEnableWhen(QuestionnaireItemEnableWhenComponent t) { //3 903 if (t == null) 904 return this; 905 if (this.enableWhen == null) 906 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 907 this.enableWhen.add(t); 908 return this; 909 } 910 911 /** 912 * @return The first repetition of repeating field {@link #enableWhen}, creating it if it does not already exist 913 */ 914 public QuestionnaireItemEnableWhenComponent getEnableWhenFirstRep() { 915 if (getEnableWhen().isEmpty()) { 916 addEnableWhen(); 917 } 918 return getEnableWhen().get(0); 919 } 920 921 /** 922 * @return {@link #required} (An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 923 */ 924 public BooleanType getRequiredElement() { 925 if (this.required == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create QuestionnaireItemComponent.required"); 928 else if (Configuration.doAutoCreate()) 929 this.required = new BooleanType(); // bb 930 return this.required; 931 } 932 933 public boolean hasRequiredElement() { 934 return this.required != null && !this.required.isEmpty(); 935 } 936 937 public boolean hasRequired() { 938 return this.required != null && !this.required.isEmpty(); 939 } 940 941 /** 942 * @param value {@link #required} (An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 943 */ 944 public QuestionnaireItemComponent setRequiredElement(BooleanType value) { 945 this.required = value; 946 return this; 947 } 948 949 /** 950 * @return An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 951 */ 952 public boolean getRequired() { 953 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 954 } 955 956 /** 957 * @param value An indication, if true, that the item must be present in a "completed" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire. 958 */ 959 public QuestionnaireItemComponent setRequired(boolean value) { 960 if (this.required == null) 961 this.required = new BooleanType(); 962 this.required.setValue(value); 963 return this; 964 } 965 966 /** 967 * @return {@link #repeats} (An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups.). This is the underlying object with id, value and extensions. The accessor "getRepeats" gives direct access to the value 968 */ 969 public BooleanType getRepeatsElement() { 970 if (this.repeats == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create QuestionnaireItemComponent.repeats"); 973 else if (Configuration.doAutoCreate()) 974 this.repeats = new BooleanType(); // bb 975 return this.repeats; 976 } 977 978 public boolean hasRepeatsElement() { 979 return this.repeats != null && !this.repeats.isEmpty(); 980 } 981 982 public boolean hasRepeats() { 983 return this.repeats != null && !this.repeats.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #repeats} (An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups.). This is the underlying object with id, value and extensions. The accessor "getRepeats" gives direct access to the value 988 */ 989 public QuestionnaireItemComponent setRepeatsElement(BooleanType value) { 990 this.repeats = value; 991 return this; 992 } 993 994 /** 995 * @return An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups. 996 */ 997 public boolean getRepeats() { 998 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 999 } 1000 1001 /** 1002 * @param value An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups. 1003 */ 1004 public QuestionnaireItemComponent setRepeats(boolean value) { 1005 if (this.repeats == null) 1006 this.repeats = new BooleanType(); 1007 this.repeats.setValue(value); 1008 return this; 1009 } 1010 1011 /** 1012 * @return {@link #readOnly} (An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.). This is the underlying object with id, value and extensions. The accessor "getReadOnly" gives direct access to the value 1013 */ 1014 public BooleanType getReadOnlyElement() { 1015 if (this.readOnly == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create QuestionnaireItemComponent.readOnly"); 1018 else if (Configuration.doAutoCreate()) 1019 this.readOnly = new BooleanType(); // bb 1020 return this.readOnly; 1021 } 1022 1023 public boolean hasReadOnlyElement() { 1024 return this.readOnly != null && !this.readOnly.isEmpty(); 1025 } 1026 1027 public boolean hasReadOnly() { 1028 return this.readOnly != null && !this.readOnly.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #readOnly} (An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.). This is the underlying object with id, value and extensions. The accessor "getReadOnly" gives direct access to the value 1033 */ 1034 public QuestionnaireItemComponent setReadOnlyElement(BooleanType value) { 1035 this.readOnly = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 1041 */ 1042 public boolean getReadOnly() { 1043 return this.readOnly == null || this.readOnly.isEmpty() ? false : this.readOnly.getValue(); 1044 } 1045 1046 /** 1047 * @param value An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire. 1048 */ 1049 public QuestionnaireItemComponent setReadOnly(boolean value) { 1050 if (this.readOnly == null) 1051 this.readOnly = new BooleanType(); 1052 this.readOnly.setValue(value); 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #maxLength} (The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 1058 */ 1059 public IntegerType getMaxLengthElement() { 1060 if (this.maxLength == null) 1061 if (Configuration.errorOnAutoCreate()) 1062 throw new Error("Attempt to auto-create QuestionnaireItemComponent.maxLength"); 1063 else if (Configuration.doAutoCreate()) 1064 this.maxLength = new IntegerType(); // bb 1065 return this.maxLength; 1066 } 1067 1068 public boolean hasMaxLengthElement() { 1069 return this.maxLength != null && !this.maxLength.isEmpty(); 1070 } 1071 1072 public boolean hasMaxLength() { 1073 return this.maxLength != null && !this.maxLength.isEmpty(); 1074 } 1075 1076 /** 1077 * @param value {@link #maxLength} (The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 1078 */ 1079 public QuestionnaireItemComponent setMaxLengthElement(IntegerType value) { 1080 this.maxLength = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 1086 */ 1087 public int getMaxLength() { 1088 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 1089 } 1090 1091 /** 1092 * @param value The maximum number of characters that are permitted in the answer to be considered a "valid" QuestionnaireResponse. 1093 */ 1094 public QuestionnaireItemComponent setMaxLength(int value) { 1095 if (this.maxLength == null) 1096 this.maxLength = new IntegerType(); 1097 this.maxLength.setValue(value); 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #options} (A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question.) 1103 */ 1104 public Reference getOptions() { 1105 if (this.options == null) 1106 if (Configuration.errorOnAutoCreate()) 1107 throw new Error("Attempt to auto-create QuestionnaireItemComponent.options"); 1108 else if (Configuration.doAutoCreate()) 1109 this.options = new Reference(); // cc 1110 return this.options; 1111 } 1112 1113 public boolean hasOptions() { 1114 return this.options != null && !this.options.isEmpty(); 1115 } 1116 1117 /** 1118 * @param value {@link #options} (A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question.) 1119 */ 1120 public QuestionnaireItemComponent setOptions(Reference value) { 1121 this.options = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return {@link #options} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question.) 1127 */ 1128 public ValueSet getOptionsTarget() { 1129 if (this.optionsTarget == null) 1130 if (Configuration.errorOnAutoCreate()) 1131 throw new Error("Attempt to auto-create QuestionnaireItemComponent.options"); 1132 else if (Configuration.doAutoCreate()) 1133 this.optionsTarget = new ValueSet(); // aa 1134 return this.optionsTarget; 1135 } 1136 1137 /** 1138 * @param value {@link #options} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a value set containing a list of codes representing permitted answers for a "choice" or "open-choice" question.) 1139 */ 1140 public QuestionnaireItemComponent setOptionsTarget(ValueSet value) { 1141 this.optionsTarget = value; 1142 return this; 1143 } 1144 1145 /** 1146 * @return {@link #option} (One of the permitted answers for a "choice" or "open-choice" question.) 1147 */ 1148 public List<QuestionnaireItemOptionComponent> getOption() { 1149 if (this.option == null) 1150 this.option = new ArrayList<QuestionnaireItemOptionComponent>(); 1151 return this.option; 1152 } 1153 1154 /** 1155 * @return Returns a reference to <code>this</code> for easy method chaining 1156 */ 1157 public QuestionnaireItemComponent setOption(List<QuestionnaireItemOptionComponent> theOption) { 1158 this.option = theOption; 1159 return this; 1160 } 1161 1162 public boolean hasOption() { 1163 if (this.option == null) 1164 return false; 1165 for (QuestionnaireItemOptionComponent item : this.option) 1166 if (!item.isEmpty()) 1167 return true; 1168 return false; 1169 } 1170 1171 public QuestionnaireItemOptionComponent addOption() { //3 1172 QuestionnaireItemOptionComponent t = new QuestionnaireItemOptionComponent(); 1173 if (this.option == null) 1174 this.option = new ArrayList<QuestionnaireItemOptionComponent>(); 1175 this.option.add(t); 1176 return t; 1177 } 1178 1179 public QuestionnaireItemComponent addOption(QuestionnaireItemOptionComponent t) { //3 1180 if (t == null) 1181 return this; 1182 if (this.option == null) 1183 this.option = new ArrayList<QuestionnaireItemOptionComponent>(); 1184 this.option.add(t); 1185 return this; 1186 } 1187 1188 /** 1189 * @return The first repetition of repeating field {@link #option}, creating it if it does not already exist 1190 */ 1191 public QuestionnaireItemOptionComponent getOptionFirstRep() { 1192 if (getOption().isEmpty()) { 1193 addOption(); 1194 } 1195 return getOption().get(0); 1196 } 1197 1198 /** 1199 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1200 */ 1201 public Type getInitial() { 1202 return this.initial; 1203 } 1204 1205 /** 1206 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1207 */ 1208 public BooleanType getInitialBooleanType() throws FHIRException { 1209 if (this.initial == null) 1210 return null; 1211 if (!(this.initial instanceof BooleanType)) 1212 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1213 return (BooleanType) this.initial; 1214 } 1215 1216 public boolean hasInitialBooleanType() { 1217 return this.initial instanceof BooleanType; 1218 } 1219 1220 /** 1221 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1222 */ 1223 public DecimalType getInitialDecimalType() throws FHIRException { 1224 if (this.initial == null) 1225 return null; 1226 if (!(this.initial instanceof DecimalType)) 1227 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1228 return (DecimalType) this.initial; 1229 } 1230 1231 public boolean hasInitialDecimalType() { 1232 return this.initial instanceof DecimalType; 1233 } 1234 1235 /** 1236 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1237 */ 1238 public IntegerType getInitialIntegerType() throws FHIRException { 1239 if (this.initial == null) 1240 return null; 1241 if (!(this.initial instanceof IntegerType)) 1242 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1243 return (IntegerType) this.initial; 1244 } 1245 1246 public boolean hasInitialIntegerType() { 1247 return this.initial instanceof IntegerType; 1248 } 1249 1250 /** 1251 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1252 */ 1253 public DateType getInitialDateType() throws FHIRException { 1254 if (this.initial == null) 1255 return null; 1256 if (!(this.initial instanceof DateType)) 1257 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1258 return (DateType) this.initial; 1259 } 1260 1261 public boolean hasInitialDateType() { 1262 return this.initial instanceof DateType; 1263 } 1264 1265 /** 1266 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1267 */ 1268 public DateTimeType getInitialDateTimeType() throws FHIRException { 1269 if (this.initial == null) 1270 return null; 1271 if (!(this.initial instanceof DateTimeType)) 1272 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1273 return (DateTimeType) this.initial; 1274 } 1275 1276 public boolean hasInitialDateTimeType() { 1277 return this.initial instanceof DateTimeType; 1278 } 1279 1280 /** 1281 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1282 */ 1283 public TimeType getInitialTimeType() throws FHIRException { 1284 if (this.initial == null) 1285 return null; 1286 if (!(this.initial instanceof TimeType)) 1287 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1288 return (TimeType) this.initial; 1289 } 1290 1291 public boolean hasInitialTimeType() { 1292 return this.initial instanceof TimeType; 1293 } 1294 1295 /** 1296 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1297 */ 1298 public StringType getInitialStringType() throws FHIRException { 1299 if (this.initial == null) 1300 return null; 1301 if (!(this.initial instanceof StringType)) 1302 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1303 return (StringType) this.initial; 1304 } 1305 1306 public boolean hasInitialStringType() { 1307 return this.initial instanceof StringType; 1308 } 1309 1310 /** 1311 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1312 */ 1313 public UriType getInitialUriType() throws FHIRException { 1314 if (this.initial == null) 1315 return null; 1316 if (!(this.initial instanceof UriType)) 1317 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.initial.getClass().getName()+" was encountered"); 1318 return (UriType) this.initial; 1319 } 1320 1321 public boolean hasInitialUriType() { 1322 return this.initial instanceof UriType; 1323 } 1324 1325 /** 1326 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1327 */ 1328 public Attachment getInitialAttachment() throws FHIRException { 1329 if (this.initial == null) 1330 return null; 1331 if (!(this.initial instanceof Attachment)) 1332 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.initial.getClass().getName()+" was encountered"); 1333 return (Attachment) this.initial; 1334 } 1335 1336 public boolean hasInitialAttachment() { 1337 return this.initial instanceof Attachment; 1338 } 1339 1340 /** 1341 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1342 */ 1343 public Coding getInitialCoding() throws FHIRException { 1344 if (this.initial == null) 1345 return null; 1346 if (!(this.initial instanceof Coding)) 1347 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.initial.getClass().getName()+" was encountered"); 1348 return (Coding) this.initial; 1349 } 1350 1351 public boolean hasInitialCoding() { 1352 return this.initial instanceof Coding; 1353 } 1354 1355 /** 1356 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1357 */ 1358 public Quantity getInitialQuantity() throws FHIRException { 1359 if (this.initial == null) 1360 return null; 1361 if (!(this.initial instanceof Quantity)) 1362 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.initial.getClass().getName()+" was encountered"); 1363 return (Quantity) this.initial; 1364 } 1365 1366 public boolean hasInitialQuantity() { 1367 return this.initial instanceof Quantity; 1368 } 1369 1370 /** 1371 * @return {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1372 */ 1373 public Reference getInitialReference() throws FHIRException { 1374 if (this.initial == null) 1375 return null; 1376 if (!(this.initial instanceof Reference)) 1377 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.initial.getClass().getName()+" was encountered"); 1378 return (Reference) this.initial; 1379 } 1380 1381 public boolean hasInitialReference() { 1382 return this.initial instanceof Reference; 1383 } 1384 1385 public boolean hasInitial() { 1386 return this.initial != null && !this.initial.isEmpty(); 1387 } 1388 1389 /** 1390 * @param value {@link #initial} (The value that should be defaulted when initially rendering the questionnaire for user input.) 1391 */ 1392 public QuestionnaireItemComponent setInitial(Type value) throws FHIRFormatError { 1393 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 1394 throw new FHIRFormatError("Not the right type for Questionnaire.item.initial[x]: "+value.fhirType()); 1395 this.initial = value; 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #item} (Text, questions and other groups to be nested beneath a question or group.) 1401 */ 1402 public List<QuestionnaireItemComponent> getItem() { 1403 if (this.item == null) 1404 this.item = new ArrayList<QuestionnaireItemComponent>(); 1405 return this.item; 1406 } 1407 1408 /** 1409 * @return Returns a reference to <code>this</code> for easy method chaining 1410 */ 1411 public QuestionnaireItemComponent setItem(List<QuestionnaireItemComponent> theItem) { 1412 this.item = theItem; 1413 return this; 1414 } 1415 1416 public boolean hasItem() { 1417 if (this.item == null) 1418 return false; 1419 for (QuestionnaireItemComponent item : this.item) 1420 if (!item.isEmpty()) 1421 return true; 1422 return false; 1423 } 1424 1425 public QuestionnaireItemComponent addItem() { //3 1426 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 1427 if (this.item == null) 1428 this.item = new ArrayList<QuestionnaireItemComponent>(); 1429 this.item.add(t); 1430 return t; 1431 } 1432 1433 public QuestionnaireItemComponent addItem(QuestionnaireItemComponent t) { //3 1434 if (t == null) 1435 return this; 1436 if (this.item == null) 1437 this.item = new ArrayList<QuestionnaireItemComponent>(); 1438 this.item.add(t); 1439 return this; 1440 } 1441 1442 /** 1443 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 1444 */ 1445 public QuestionnaireItemComponent getItemFirstRep() { 1446 if (getItem().isEmpty()) { 1447 addItem(); 1448 } 1449 return getItem().get(0); 1450 } 1451 1452 protected void listChildren(List<Property> children) { 1453 super.listChildren(children); 1454 children.add(new Property("linkId", "string", "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 0, 1, linkId)); 1455 children.add(new Property("definition", "uri", "A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: \n\n* code (ElementDefinition.code)\n* type (ElementDefinition.type)\n* required (ElementDefinition.min)\n* repeats (ElementDefinition.max)\n* maxLength (ElementDefinition.maxLength)\n* options (ElementDefinition.binding)\n\nAny information provided in these elements on a Questionnaire Item overrides the information from the definition.", 0, 1, definition)); 1456 children.add(new Property("code", "Coding", "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 0, java.lang.Integer.MAX_VALUE, code)); 1457 children.add(new Property("prefix", "string", "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 0, 1, prefix)); 1458 children.add(new Property("text", "string", "The name of a section, the text of a question or text content for a display item.", 0, 1, text)); 1459 children.add(new Property("type", "code", "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).", 0, 1, type)); 1460 children.add(new Property("enableWhen", "", "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 0, java.lang.Integer.MAX_VALUE, enableWhen)); 1461 children.add(new Property("required", "boolean", "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 0, 1, required)); 1462 children.add(new Property("repeats", "boolean", "An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups.", 0, 1, repeats)); 1463 children.add(new Property("readOnly", "boolean", "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 1, readOnly)); 1464 children.add(new Property("maxLength", "integer", "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 0, 1, maxLength)); 1465 children.add(new Property("options", "Reference(ValueSet)", "A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question.", 0, 1, options)); 1466 children.add(new Property("option", "", "One of the permitted answers for a \"choice\" or \"open-choice\" question.", 0, java.lang.Integer.MAX_VALUE, option)); 1467 children.add(new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial)); 1468 children.add(new Property("item", "@Questionnaire.item", "Text, questions and other groups to be nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item)); 1469 } 1470 1471 @Override 1472 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1473 switch (_hash) { 1474 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 0, 1, linkId); 1475 case -1014418093: /*definition*/ return new Property("definition", "uri", "A reference to an [[[ElementDefinition]]] that provides the details for the item. If a definition is provided, then the following element values can be inferred from the definition: \n\n* code (ElementDefinition.code)\n* type (ElementDefinition.type)\n* required (ElementDefinition.min)\n* repeats (ElementDefinition.max)\n* maxLength (ElementDefinition.maxLength)\n* options (ElementDefinition.binding)\n\nAny information provided in these elements on a Questionnaire Item overrides the information from the definition.", 0, 1, definition); 1476 case 3059181: /*code*/ return new Property("code", "Coding", "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 0, java.lang.Integer.MAX_VALUE, code); 1477 case -980110702: /*prefix*/ return new Property("prefix", "string", "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 0, 1, prefix); 1478 case 3556653: /*text*/ return new Property("text", "string", "The name of a section, the text of a question or text content for a display item.", 0, 1, text); 1479 case 3575610: /*type*/ return new Property("type", "code", "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).", 0, 1, type); 1480 case 1893321565: /*enableWhen*/ return new Property("enableWhen", "", "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 0, java.lang.Integer.MAX_VALUE, enableWhen); 1481 case -393139297: /*required*/ return new Property("required", "boolean", "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 0, 1, required); 1482 case 1094288952: /*repeats*/ return new Property("repeats", "boolean", "An indication, if true, that the item may occur multiple times in the response, collecting multiple answers answers for questions or multiple sets of answers for groups.", 0, 1, repeats); 1483 case -867683742: /*readOnly*/ return new Property("readOnly", "boolean", "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 1, readOnly); 1484 case -791400086: /*maxLength*/ return new Property("maxLength", "integer", "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 0, 1, maxLength); 1485 case -1249474914: /*options*/ return new Property("options", "Reference(ValueSet)", "A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question.", 0, 1, options); 1486 case -1010136971: /*option*/ return new Property("option", "", "One of the permitted answers for a \"choice\" or \"open-choice\" question.", 0, java.lang.Integer.MAX_VALUE, option); 1487 case 871077564: /*initial[x]*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1488 case 1948342084: /*initial*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1489 case 1508030532: /*initialBoolean*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1490 case -1309384851: /*initialDecimal*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1491 case -893596326: /*initialInteger*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1492 case 1232894226: /*initialDate*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1493 case -709007617: /*initialDateTime*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1494 case 1233378353: /*initialTime*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1495 case -152690571: /*initialString*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1496 case 871071624: /*initialUri*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1497 case -970373241: /*initialAttachment*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1498 case -615791666: /*initialCoding*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1499 case 508206063: /*initialQuantity*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1500 case -1170213785: /*initialReference*/ return new Property("initial[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The value that should be defaulted when initially rendering the questionnaire for user input.", 0, 1, initial); 1501 case 3242771: /*item*/ return new Property("item", "@Questionnaire.item", "Text, questions and other groups to be nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item); 1502 default: return super.getNamedProperty(_hash, _name, _checkValid); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1509 switch (hash) { 1510 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1511 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 1512 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 1513 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : new Base[] {this.prefix}; // StringType 1514 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 1515 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<QuestionnaireItemType> 1516 case 1893321565: /*enableWhen*/ return this.enableWhen == null ? new Base[0] : this.enableWhen.toArray(new Base[this.enableWhen.size()]); // QuestionnaireItemEnableWhenComponent 1517 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // BooleanType 1518 case 1094288952: /*repeats*/ return this.repeats == null ? new Base[0] : new Base[] {this.repeats}; // BooleanType 1519 case -867683742: /*readOnly*/ return this.readOnly == null ? new Base[0] : new Base[] {this.readOnly}; // BooleanType 1520 case -791400086: /*maxLength*/ return this.maxLength == null ? new Base[0] : new Base[] {this.maxLength}; // IntegerType 1521 case -1249474914: /*options*/ return this.options == null ? new Base[0] : new Base[] {this.options}; // Reference 1522 case -1010136971: /*option*/ return this.option == null ? new Base[0] : this.option.toArray(new Base[this.option.size()]); // QuestionnaireItemOptionComponent 1523 case 1948342084: /*initial*/ return this.initial == null ? new Base[0] : new Base[] {this.initial}; // Type 1524 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 1525 default: return super.getProperty(hash, name, checkValid); 1526 } 1527 1528 } 1529 1530 @Override 1531 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1532 switch (hash) { 1533 case -1102667083: // linkId 1534 this.linkId = castToString(value); // StringType 1535 return value; 1536 case -1014418093: // definition 1537 this.definition = castToUri(value); // UriType 1538 return value; 1539 case 3059181: // code 1540 this.getCode().add(castToCoding(value)); // Coding 1541 return value; 1542 case -980110702: // prefix 1543 this.prefix = castToString(value); // StringType 1544 return value; 1545 case 3556653: // text 1546 this.text = castToString(value); // StringType 1547 return value; 1548 case 3575610: // type 1549 value = new QuestionnaireItemTypeEnumFactory().fromType(castToCode(value)); 1550 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 1551 return value; 1552 case 1893321565: // enableWhen 1553 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); // QuestionnaireItemEnableWhenComponent 1554 return value; 1555 case -393139297: // required 1556 this.required = castToBoolean(value); // BooleanType 1557 return value; 1558 case 1094288952: // repeats 1559 this.repeats = castToBoolean(value); // BooleanType 1560 return value; 1561 case -867683742: // readOnly 1562 this.readOnly = castToBoolean(value); // BooleanType 1563 return value; 1564 case -791400086: // maxLength 1565 this.maxLength = castToInteger(value); // IntegerType 1566 return value; 1567 case -1249474914: // options 1568 this.options = castToReference(value); // Reference 1569 return value; 1570 case -1010136971: // option 1571 this.getOption().add((QuestionnaireItemOptionComponent) value); // QuestionnaireItemOptionComponent 1572 return value; 1573 case 1948342084: // initial 1574 this.initial = castToType(value); // Type 1575 return value; 1576 case 3242771: // item 1577 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 1578 return value; 1579 default: return super.setProperty(hash, name, value); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base setProperty(String name, Base value) throws FHIRException { 1586 if (name.equals("linkId")) { 1587 this.linkId = castToString(value); // StringType 1588 } else if (name.equals("definition")) { 1589 this.definition = castToUri(value); // UriType 1590 } else if (name.equals("code")) { 1591 this.getCode().add(castToCoding(value)); 1592 } else if (name.equals("prefix")) { 1593 this.prefix = castToString(value); // StringType 1594 } else if (name.equals("text")) { 1595 this.text = castToString(value); // StringType 1596 } else if (name.equals("type")) { 1597 value = new QuestionnaireItemTypeEnumFactory().fromType(castToCode(value)); 1598 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 1599 } else if (name.equals("enableWhen")) { 1600 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); 1601 } else if (name.equals("required")) { 1602 this.required = castToBoolean(value); // BooleanType 1603 } else if (name.equals("repeats")) { 1604 this.repeats = castToBoolean(value); // BooleanType 1605 } else if (name.equals("readOnly")) { 1606 this.readOnly = castToBoolean(value); // BooleanType 1607 } else if (name.equals("maxLength")) { 1608 this.maxLength = castToInteger(value); // IntegerType 1609 } else if (name.equals("options")) { 1610 this.options = castToReference(value); // Reference 1611 } else if (name.equals("option")) { 1612 this.getOption().add((QuestionnaireItemOptionComponent) value); 1613 } else if (name.equals("initial[x]")) { 1614 this.initial = castToType(value); // Type 1615 } else if (name.equals("item")) { 1616 this.getItem().add((QuestionnaireItemComponent) value); 1617 } else 1618 return super.setProperty(name, value); 1619 return value; 1620 } 1621 1622 @Override 1623 public Base makeProperty(int hash, String name) throws FHIRException { 1624 switch (hash) { 1625 case -1102667083: return getLinkIdElement(); 1626 case -1014418093: return getDefinitionElement(); 1627 case 3059181: return addCode(); 1628 case -980110702: return getPrefixElement(); 1629 case 3556653: return getTextElement(); 1630 case 3575610: return getTypeElement(); 1631 case 1893321565: return addEnableWhen(); 1632 case -393139297: return getRequiredElement(); 1633 case 1094288952: return getRepeatsElement(); 1634 case -867683742: return getReadOnlyElement(); 1635 case -791400086: return getMaxLengthElement(); 1636 case -1249474914: return getOptions(); 1637 case -1010136971: return addOption(); 1638 case 871077564: return getInitial(); 1639 case 1948342084: return getInitial(); 1640 case 3242771: return addItem(); 1641 default: return super.makeProperty(hash, name); 1642 } 1643 1644 } 1645 1646 @Override 1647 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1648 switch (hash) { 1649 case -1102667083: /*linkId*/ return new String[] {"string"}; 1650 case -1014418093: /*definition*/ return new String[] {"uri"}; 1651 case 3059181: /*code*/ return new String[] {"Coding"}; 1652 case -980110702: /*prefix*/ return new String[] {"string"}; 1653 case 3556653: /*text*/ return new String[] {"string"}; 1654 case 3575610: /*type*/ return new String[] {"code"}; 1655 case 1893321565: /*enableWhen*/ return new String[] {}; 1656 case -393139297: /*required*/ return new String[] {"boolean"}; 1657 case 1094288952: /*repeats*/ return new String[] {"boolean"}; 1658 case -867683742: /*readOnly*/ return new String[] {"boolean"}; 1659 case -791400086: /*maxLength*/ return new String[] {"integer"}; 1660 case -1249474914: /*options*/ return new String[] {"Reference"}; 1661 case -1010136971: /*option*/ return new String[] {}; 1662 case 1948342084: /*initial*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 1663 case 3242771: /*item*/ return new String[] {"@Questionnaire.item"}; 1664 default: return super.getTypesForProperty(hash, name); 1665 } 1666 1667 } 1668 1669 @Override 1670 public Base addChild(String name) throws FHIRException { 1671 if (name.equals("linkId")) { 1672 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 1673 } 1674 else if (name.equals("definition")) { 1675 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.definition"); 1676 } 1677 else if (name.equals("code")) { 1678 return addCode(); 1679 } 1680 else if (name.equals("prefix")) { 1681 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.prefix"); 1682 } 1683 else if (name.equals("text")) { 1684 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 1685 } 1686 else if (name.equals("type")) { 1687 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.type"); 1688 } 1689 else if (name.equals("enableWhen")) { 1690 return addEnableWhen(); 1691 } 1692 else if (name.equals("required")) { 1693 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 1694 } 1695 else if (name.equals("repeats")) { 1696 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 1697 } 1698 else if (name.equals("readOnly")) { 1699 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.readOnly"); 1700 } 1701 else if (name.equals("maxLength")) { 1702 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.maxLength"); 1703 } 1704 else if (name.equals("options")) { 1705 this.options = new Reference(); 1706 return this.options; 1707 } 1708 else if (name.equals("option")) { 1709 return addOption(); 1710 } 1711 else if (name.equals("initialBoolean")) { 1712 this.initial = new BooleanType(); 1713 return this.initial; 1714 } 1715 else if (name.equals("initialDecimal")) { 1716 this.initial = new DecimalType(); 1717 return this.initial; 1718 } 1719 else if (name.equals("initialInteger")) { 1720 this.initial = new IntegerType(); 1721 return this.initial; 1722 } 1723 else if (name.equals("initialDate")) { 1724 this.initial = new DateType(); 1725 return this.initial; 1726 } 1727 else if (name.equals("initialDateTime")) { 1728 this.initial = new DateTimeType(); 1729 return this.initial; 1730 } 1731 else if (name.equals("initialTime")) { 1732 this.initial = new TimeType(); 1733 return this.initial; 1734 } 1735 else if (name.equals("initialString")) { 1736 this.initial = new StringType(); 1737 return this.initial; 1738 } 1739 else if (name.equals("initialUri")) { 1740 this.initial = new UriType(); 1741 return this.initial; 1742 } 1743 else if (name.equals("initialAttachment")) { 1744 this.initial = new Attachment(); 1745 return this.initial; 1746 } 1747 else if (name.equals("initialCoding")) { 1748 this.initial = new Coding(); 1749 return this.initial; 1750 } 1751 else if (name.equals("initialQuantity")) { 1752 this.initial = new Quantity(); 1753 return this.initial; 1754 } 1755 else if (name.equals("initialReference")) { 1756 this.initial = new Reference(); 1757 return this.initial; 1758 } 1759 else if (name.equals("item")) { 1760 return addItem(); 1761 } 1762 else 1763 return super.addChild(name); 1764 } 1765 1766 public QuestionnaireItemComponent copy() { 1767 QuestionnaireItemComponent dst = new QuestionnaireItemComponent(); 1768 copyValues(dst); 1769 dst.linkId = linkId == null ? null : linkId.copy(); 1770 dst.definition = definition == null ? null : definition.copy(); 1771 if (code != null) { 1772 dst.code = new ArrayList<Coding>(); 1773 for (Coding i : code) 1774 dst.code.add(i.copy()); 1775 }; 1776 dst.prefix = prefix == null ? null : prefix.copy(); 1777 dst.text = text == null ? null : text.copy(); 1778 dst.type = type == null ? null : type.copy(); 1779 if (enableWhen != null) { 1780 dst.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1781 for (QuestionnaireItemEnableWhenComponent i : enableWhen) 1782 dst.enableWhen.add(i.copy()); 1783 }; 1784 dst.required = required == null ? null : required.copy(); 1785 dst.repeats = repeats == null ? null : repeats.copy(); 1786 dst.readOnly = readOnly == null ? null : readOnly.copy(); 1787 dst.maxLength = maxLength == null ? null : maxLength.copy(); 1788 dst.options = options == null ? null : options.copy(); 1789 if (option != null) { 1790 dst.option = new ArrayList<QuestionnaireItemOptionComponent>(); 1791 for (QuestionnaireItemOptionComponent i : option) 1792 dst.option.add(i.copy()); 1793 }; 1794 dst.initial = initial == null ? null : initial.copy(); 1795 if (item != null) { 1796 dst.item = new ArrayList<QuestionnaireItemComponent>(); 1797 for (QuestionnaireItemComponent i : item) 1798 dst.item.add(i.copy()); 1799 }; 1800 return dst; 1801 } 1802 1803 @Override 1804 public boolean equalsDeep(Base other_) { 1805 if (!super.equalsDeep(other_)) 1806 return false; 1807 if (!(other_ instanceof QuestionnaireItemComponent)) 1808 return false; 1809 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 1810 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) && compareDeep(code, o.code, true) 1811 && compareDeep(prefix, o.prefix, true) && compareDeep(text, o.text, true) && compareDeep(type, o.type, true) 1812 && compareDeep(enableWhen, o.enableWhen, true) && compareDeep(required, o.required, true) && compareDeep(repeats, o.repeats, true) 1813 && compareDeep(readOnly, o.readOnly, true) && compareDeep(maxLength, o.maxLength, true) && compareDeep(options, o.options, true) 1814 && compareDeep(option, o.option, true) && compareDeep(initial, o.initial, true) && compareDeep(item, o.item, true) 1815 ; 1816 } 1817 1818 @Override 1819 public boolean equalsShallow(Base other_) { 1820 if (!super.equalsShallow(other_)) 1821 return false; 1822 if (!(other_ instanceof QuestionnaireItemComponent)) 1823 return false; 1824 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 1825 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) && compareValues(prefix, o.prefix, true) 1826 && compareValues(text, o.text, true) && compareValues(type, o.type, true) && compareValues(required, o.required, true) 1827 && compareValues(repeats, o.repeats, true) && compareValues(readOnly, o.readOnly, true) && compareValues(maxLength, o.maxLength, true) 1828 ; 1829 } 1830 1831 public boolean isEmpty() { 1832 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, code 1833 , prefix, text, type, enableWhen, required, repeats, readOnly, maxLength, options 1834 , option, initial, item); 1835 } 1836 1837 public String fhirType() { 1838 return "Questionnaire.item"; 1839 1840 } 1841 1842 } 1843 1844 @Block() 1845 public static class QuestionnaireItemEnableWhenComponent extends BackboneElement implements IBaseBackboneElement { 1846 /** 1847 * The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 1848 */ 1849 @Child(name = "question", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1850 @Description(shortDefinition="Question that determines whether item is enabled", formalDefinition="The linkId for the question whose answer (or lack of answer) governs whether this item is enabled." ) 1851 protected StringType question; 1852 1853 /** 1854 * An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false). 1855 */ 1856 @Child(name = "hasAnswer", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1857 @Description(shortDefinition="Enable when answered or not", formalDefinition="An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false)." ) 1858 protected BooleanType hasAnswer; 1859 1860 /** 1861 * An answer that the referenced question must match in order for the item to be enabled. 1862 */ 1863 @Child(name = "answer", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 1864 @Description(shortDefinition="Value question must have", formalDefinition="An answer that the referenced question must match in order for the item to be enabled." ) 1865 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 1866 protected Type answer; 1867 1868 private static final long serialVersionUID = -300241115L; 1869 1870 /** 1871 * Constructor 1872 */ 1873 public QuestionnaireItemEnableWhenComponent() { 1874 super(); 1875 } 1876 1877 /** 1878 * Constructor 1879 */ 1880 public QuestionnaireItemEnableWhenComponent(StringType question) { 1881 super(); 1882 this.question = question; 1883 } 1884 1885 /** 1886 * @return {@link #question} (The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.). This is the underlying object with id, value and extensions. The accessor "getQuestion" gives direct access to the value 1887 */ 1888 public StringType getQuestionElement() { 1889 if (this.question == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.question"); 1892 else if (Configuration.doAutoCreate()) 1893 this.question = new StringType(); // bb 1894 return this.question; 1895 } 1896 1897 public boolean hasQuestionElement() { 1898 return this.question != null && !this.question.isEmpty(); 1899 } 1900 1901 public boolean hasQuestion() { 1902 return this.question != null && !this.question.isEmpty(); 1903 } 1904 1905 /** 1906 * @param value {@link #question} (The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.). This is the underlying object with id, value and extensions. The accessor "getQuestion" gives direct access to the value 1907 */ 1908 public QuestionnaireItemEnableWhenComponent setQuestionElement(StringType value) { 1909 this.question = value; 1910 return this; 1911 } 1912 1913 /** 1914 * @return The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 1915 */ 1916 public String getQuestion() { 1917 return this.question == null ? null : this.question.getValue(); 1918 } 1919 1920 /** 1921 * @param value The linkId for the question whose answer (or lack of answer) governs whether this item is enabled. 1922 */ 1923 public QuestionnaireItemEnableWhenComponent setQuestion(String value) { 1924 if (this.question == null) 1925 this.question = new StringType(); 1926 this.question.setValue(value); 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #hasAnswer} (An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false).). This is the underlying object with id, value and extensions. The accessor "getHasAnswer" gives direct access to the value 1932 */ 1933 public BooleanType getHasAnswerElement() { 1934 if (this.hasAnswer == null) 1935 if (Configuration.errorOnAutoCreate()) 1936 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.hasAnswer"); 1937 else if (Configuration.doAutoCreate()) 1938 this.hasAnswer = new BooleanType(); // bb 1939 return this.hasAnswer; 1940 } 1941 1942 public boolean hasHasAnswerElement() { 1943 return this.hasAnswer != null && !this.hasAnswer.isEmpty(); 1944 } 1945 1946 public boolean hasHasAnswer() { 1947 return this.hasAnswer != null && !this.hasAnswer.isEmpty(); 1948 } 1949 1950 /** 1951 * @param value {@link #hasAnswer} (An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false).). This is the underlying object with id, value and extensions. The accessor "getHasAnswer" gives direct access to the value 1952 */ 1953 public QuestionnaireItemEnableWhenComponent setHasAnswerElement(BooleanType value) { 1954 this.hasAnswer = value; 1955 return this; 1956 } 1957 1958 /** 1959 * @return An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false). 1960 */ 1961 public boolean getHasAnswer() { 1962 return this.hasAnswer == null || this.hasAnswer.isEmpty() ? false : this.hasAnswer.getValue(); 1963 } 1964 1965 /** 1966 * @param value An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false). 1967 */ 1968 public QuestionnaireItemEnableWhenComponent setHasAnswer(boolean value) { 1969 if (this.hasAnswer == null) 1970 this.hasAnswer = new BooleanType(); 1971 this.hasAnswer.setValue(value); 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 1977 */ 1978 public Type getAnswer() { 1979 return this.answer; 1980 } 1981 1982 /** 1983 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 1984 */ 1985 public BooleanType getAnswerBooleanType() throws FHIRException { 1986 if (this.answer == null) 1987 return null; 1988 if (!(this.answer instanceof BooleanType)) 1989 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.answer.getClass().getName()+" was encountered"); 1990 return (BooleanType) this.answer; 1991 } 1992 1993 public boolean hasAnswerBooleanType() { 1994 return this.answer instanceof BooleanType; 1995 } 1996 1997 /** 1998 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 1999 */ 2000 public DecimalType getAnswerDecimalType() throws FHIRException { 2001 if (this.answer == null) 2002 return null; 2003 if (!(this.answer instanceof DecimalType)) 2004 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2005 return (DecimalType) this.answer; 2006 } 2007 2008 public boolean hasAnswerDecimalType() { 2009 return this.answer instanceof DecimalType; 2010 } 2011 2012 /** 2013 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2014 */ 2015 public IntegerType getAnswerIntegerType() throws FHIRException { 2016 if (this.answer == null) 2017 return null; 2018 if (!(this.answer instanceof IntegerType)) 2019 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2020 return (IntegerType) this.answer; 2021 } 2022 2023 public boolean hasAnswerIntegerType() { 2024 return this.answer instanceof IntegerType; 2025 } 2026 2027 /** 2028 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2029 */ 2030 public DateType getAnswerDateType() throws FHIRException { 2031 if (this.answer == null) 2032 return null; 2033 if (!(this.answer instanceof DateType)) 2034 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2035 return (DateType) this.answer; 2036 } 2037 2038 public boolean hasAnswerDateType() { 2039 return this.answer instanceof DateType; 2040 } 2041 2042 /** 2043 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2044 */ 2045 public DateTimeType getAnswerDateTimeType() throws FHIRException { 2046 if (this.answer == null) 2047 return null; 2048 if (!(this.answer instanceof DateTimeType)) 2049 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2050 return (DateTimeType) this.answer; 2051 } 2052 2053 public boolean hasAnswerDateTimeType() { 2054 return this.answer instanceof DateTimeType; 2055 } 2056 2057 /** 2058 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2059 */ 2060 public TimeType getAnswerTimeType() throws FHIRException { 2061 if (this.answer == null) 2062 return null; 2063 if (!(this.answer instanceof TimeType)) 2064 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2065 return (TimeType) this.answer; 2066 } 2067 2068 public boolean hasAnswerTimeType() { 2069 return this.answer instanceof TimeType; 2070 } 2071 2072 /** 2073 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2074 */ 2075 public StringType getAnswerStringType() throws FHIRException { 2076 if (this.answer == null) 2077 return null; 2078 if (!(this.answer instanceof StringType)) 2079 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2080 return (StringType) this.answer; 2081 } 2082 2083 public boolean hasAnswerStringType() { 2084 return this.answer instanceof StringType; 2085 } 2086 2087 /** 2088 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2089 */ 2090 public UriType getAnswerUriType() throws FHIRException { 2091 if (this.answer == null) 2092 return null; 2093 if (!(this.answer instanceof UriType)) 2094 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.answer.getClass().getName()+" was encountered"); 2095 return (UriType) this.answer; 2096 } 2097 2098 public boolean hasAnswerUriType() { 2099 return this.answer instanceof UriType; 2100 } 2101 2102 /** 2103 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2104 */ 2105 public Attachment getAnswerAttachment() throws FHIRException { 2106 if (this.answer == null) 2107 return null; 2108 if (!(this.answer instanceof Attachment)) 2109 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.answer.getClass().getName()+" was encountered"); 2110 return (Attachment) this.answer; 2111 } 2112 2113 public boolean hasAnswerAttachment() { 2114 return this.answer instanceof Attachment; 2115 } 2116 2117 /** 2118 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2119 */ 2120 public Coding getAnswerCoding() throws FHIRException { 2121 if (this.answer == null) 2122 return null; 2123 if (!(this.answer instanceof Coding)) 2124 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.answer.getClass().getName()+" was encountered"); 2125 return (Coding) this.answer; 2126 } 2127 2128 public boolean hasAnswerCoding() { 2129 return this.answer instanceof Coding; 2130 } 2131 2132 /** 2133 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2134 */ 2135 public Quantity getAnswerQuantity() throws FHIRException { 2136 if (this.answer == null) 2137 return null; 2138 if (!(this.answer instanceof Quantity)) 2139 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.answer.getClass().getName()+" was encountered"); 2140 return (Quantity) this.answer; 2141 } 2142 2143 public boolean hasAnswerQuantity() { 2144 return this.answer instanceof Quantity; 2145 } 2146 2147 /** 2148 * @return {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2149 */ 2150 public Reference getAnswerReference() throws FHIRException { 2151 if (this.answer == null) 2152 return null; 2153 if (!(this.answer instanceof Reference)) 2154 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.answer.getClass().getName()+" was encountered"); 2155 return (Reference) this.answer; 2156 } 2157 2158 public boolean hasAnswerReference() { 2159 return this.answer instanceof Reference; 2160 } 2161 2162 public boolean hasAnswer() { 2163 return this.answer != null && !this.answer.isEmpty(); 2164 } 2165 2166 /** 2167 * @param value {@link #answer} (An answer that the referenced question must match in order for the item to be enabled.) 2168 */ 2169 public QuestionnaireItemEnableWhenComponent setAnswer(Type value) throws FHIRFormatError { 2170 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 2171 throw new FHIRFormatError("Not the right type for Questionnaire.item.enableWhen.answer[x]: "+value.fhirType()); 2172 this.answer = value; 2173 return this; 2174 } 2175 2176 protected void listChildren(List<Property> children) { 2177 super.listChildren(children); 2178 children.add(new Property("question", "string", "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, question)); 2179 children.add(new Property("hasAnswer", "boolean", "An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false).", 0, 1, hasAnswer)); 2180 children.add(new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer)); 2181 } 2182 2183 @Override 2184 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2185 switch (_hash) { 2186 case -1165870106: /*question*/ return new Property("question", "string", "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, question); 2187 case -793058568: /*hasAnswer*/ return new Property("hasAnswer", "boolean", "An indication that this item should be enabled only if the specified question is answered (hasAnswer=true) or not answered (hasAnswer=false).", 0, 1, hasAnswer); 2188 case 1693524994: /*answer[x]*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2189 case -1412808770: /*answer*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2190 case 1194603146: /*answerBoolean*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2191 case -1622812237: /*answerDecimal*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2192 case -1207023712: /*answerInteger*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2193 case 958960780: /*answerDate*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2194 case -1835321991: /*answerDateTime*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2195 case 959444907: /*answerTime*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2196 case -1409727121: /*answerString*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2197 case 1693519054: /*answerUri*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2198 case -1026728063: /*answerAttachment*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2199 case -1872828216: /*answerCoding*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2200 case -618108311: /*answerQuantity*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2201 case -1726221011: /*answerReference*/ return new Property("answer[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "An answer that the referenced question must match in order for the item to be enabled.", 0, 1, answer); 2202 default: return super.getNamedProperty(_hash, _name, _checkValid); 2203 } 2204 2205 } 2206 2207 @Override 2208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2209 switch (hash) { 2210 case -1165870106: /*question*/ return this.question == null ? new Base[0] : new Base[] {this.question}; // StringType 2211 case -793058568: /*hasAnswer*/ return this.hasAnswer == null ? new Base[0] : new Base[] {this.hasAnswer}; // BooleanType 2212 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : new Base[] {this.answer}; // Type 2213 default: return super.getProperty(hash, name, checkValid); 2214 } 2215 2216 } 2217 2218 @Override 2219 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2220 switch (hash) { 2221 case -1165870106: // question 2222 this.question = castToString(value); // StringType 2223 return value; 2224 case -793058568: // hasAnswer 2225 this.hasAnswer = castToBoolean(value); // BooleanType 2226 return value; 2227 case -1412808770: // answer 2228 this.answer = castToType(value); // Type 2229 return value; 2230 default: return super.setProperty(hash, name, value); 2231 } 2232 2233 } 2234 2235 @Override 2236 public Base setProperty(String name, Base value) throws FHIRException { 2237 if (name.equals("question")) { 2238 this.question = castToString(value); // StringType 2239 } else if (name.equals("hasAnswer")) { 2240 this.hasAnswer = castToBoolean(value); // BooleanType 2241 } else if (name.equals("answer[x]")) { 2242 this.answer = castToType(value); // Type 2243 } else 2244 return super.setProperty(name, value); 2245 return value; 2246 } 2247 2248 @Override 2249 public Base makeProperty(int hash, String name) throws FHIRException { 2250 switch (hash) { 2251 case -1165870106: return getQuestionElement(); 2252 case -793058568: return getHasAnswerElement(); 2253 case 1693524994: return getAnswer(); 2254 case -1412808770: return getAnswer(); 2255 default: return super.makeProperty(hash, name); 2256 } 2257 2258 } 2259 2260 @Override 2261 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2262 switch (hash) { 2263 case -1165870106: /*question*/ return new String[] {"string"}; 2264 case -793058568: /*hasAnswer*/ return new String[] {"boolean"}; 2265 case -1412808770: /*answer*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 2266 default: return super.getTypesForProperty(hash, name); 2267 } 2268 2269 } 2270 2271 @Override 2272 public Base addChild(String name) throws FHIRException { 2273 if (name.equals("question")) { 2274 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.question"); 2275 } 2276 else if (name.equals("hasAnswer")) { 2277 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.hasAnswer"); 2278 } 2279 else if (name.equals("answerBoolean")) { 2280 this.answer = new BooleanType(); 2281 return this.answer; 2282 } 2283 else if (name.equals("answerDecimal")) { 2284 this.answer = new DecimalType(); 2285 return this.answer; 2286 } 2287 else if (name.equals("answerInteger")) { 2288 this.answer = new IntegerType(); 2289 return this.answer; 2290 } 2291 else if (name.equals("answerDate")) { 2292 this.answer = new DateType(); 2293 return this.answer; 2294 } 2295 else if (name.equals("answerDateTime")) { 2296 this.answer = new DateTimeType(); 2297 return this.answer; 2298 } 2299 else if (name.equals("answerTime")) { 2300 this.answer = new TimeType(); 2301 return this.answer; 2302 } 2303 else if (name.equals("answerString")) { 2304 this.answer = new StringType(); 2305 return this.answer; 2306 } 2307 else if (name.equals("answerUri")) { 2308 this.answer = new UriType(); 2309 return this.answer; 2310 } 2311 else if (name.equals("answerAttachment")) { 2312 this.answer = new Attachment(); 2313 return this.answer; 2314 } 2315 else if (name.equals("answerCoding")) { 2316 this.answer = new Coding(); 2317 return this.answer; 2318 } 2319 else if (name.equals("answerQuantity")) { 2320 this.answer = new Quantity(); 2321 return this.answer; 2322 } 2323 else if (name.equals("answerReference")) { 2324 this.answer = new Reference(); 2325 return this.answer; 2326 } 2327 else 2328 return super.addChild(name); 2329 } 2330 2331 public QuestionnaireItemEnableWhenComponent copy() { 2332 QuestionnaireItemEnableWhenComponent dst = new QuestionnaireItemEnableWhenComponent(); 2333 copyValues(dst); 2334 dst.question = question == null ? null : question.copy(); 2335 dst.hasAnswer = hasAnswer == null ? null : hasAnswer.copy(); 2336 dst.answer = answer == null ? null : answer.copy(); 2337 return dst; 2338 } 2339 2340 @Override 2341 public boolean equalsDeep(Base other_) { 2342 if (!super.equalsDeep(other_)) 2343 return false; 2344 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 2345 return false; 2346 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 2347 return compareDeep(question, o.question, true) && compareDeep(hasAnswer, o.hasAnswer, true) && compareDeep(answer, o.answer, true) 2348 ; 2349 } 2350 2351 @Override 2352 public boolean equalsShallow(Base other_) { 2353 if (!super.equalsShallow(other_)) 2354 return false; 2355 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 2356 return false; 2357 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 2358 return compareValues(question, o.question, true) && compareValues(hasAnswer, o.hasAnswer, true); 2359 } 2360 2361 public boolean isEmpty() { 2362 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(question, hasAnswer, answer 2363 ); 2364 } 2365 2366 public String fhirType() { 2367 return "Questionnaire.item.enableWhen"; 2368 2369 } 2370 2371 } 2372 2373 @Block() 2374 public static class QuestionnaireItemOptionComponent extends BackboneElement implements IBaseBackboneElement { 2375 /** 2376 * A potential answer that's allowed as the answer to this question. 2377 */ 2378 @Child(name = "value", type = {IntegerType.class, DateType.class, TimeType.class, StringType.class, Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 2379 @Description(shortDefinition="Answer value", formalDefinition="A potential answer that's allowed as the answer to this question." ) 2380 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 2381 protected Type value; 2382 2383 private static final long serialVersionUID = -732981989L; 2384 2385 /** 2386 * Constructor 2387 */ 2388 public QuestionnaireItemOptionComponent() { 2389 super(); 2390 } 2391 2392 /** 2393 * Constructor 2394 */ 2395 public QuestionnaireItemOptionComponent(Type value) { 2396 super(); 2397 this.value = value; 2398 } 2399 2400 /** 2401 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2402 */ 2403 public Type getValue() { 2404 return this.value; 2405 } 2406 2407 /** 2408 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2409 */ 2410 public IntegerType getValueIntegerType() throws FHIRException { 2411 if (this.value == null) 2412 return null; 2413 if (!(this.value instanceof IntegerType)) 2414 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2415 return (IntegerType) this.value; 2416 } 2417 2418 public boolean hasValueIntegerType() { 2419 return this.value instanceof IntegerType; 2420 } 2421 2422 /** 2423 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2424 */ 2425 public DateType getValueDateType() throws FHIRException { 2426 if (this.value == null) 2427 return null; 2428 if (!(this.value instanceof DateType)) 2429 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2430 return (DateType) this.value; 2431 } 2432 2433 public boolean hasValueDateType() { 2434 return this.value instanceof DateType; 2435 } 2436 2437 /** 2438 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2439 */ 2440 public TimeType getValueTimeType() throws FHIRException { 2441 if (this.value == null) 2442 return null; 2443 if (!(this.value instanceof TimeType)) 2444 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2445 return (TimeType) this.value; 2446 } 2447 2448 public boolean hasValueTimeType() { 2449 return this.value instanceof TimeType; 2450 } 2451 2452 /** 2453 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2454 */ 2455 public StringType getValueStringType() throws FHIRException { 2456 if (this.value == null) 2457 return null; 2458 if (!(this.value instanceof StringType)) 2459 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2460 return (StringType) this.value; 2461 } 2462 2463 public boolean hasValueStringType() { 2464 return this.value instanceof StringType; 2465 } 2466 2467 /** 2468 * @return {@link #value} (A potential answer that's allowed as the answer to this question.) 2469 */ 2470 public Coding getValueCoding() throws FHIRException { 2471 if (this.value == null) 2472 return null; 2473 if (!(this.value instanceof Coding)) 2474 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2475 return (Coding) this.value; 2476 } 2477 2478 public boolean hasValueCoding() { 2479 return this.value instanceof Coding; 2480 } 2481 2482 public boolean hasValue() { 2483 return this.value != null && !this.value.isEmpty(); 2484 } 2485 2486 /** 2487 * @param value {@link #value} (A potential answer that's allowed as the answer to this question.) 2488 */ 2489 public QuestionnaireItemOptionComponent setValue(Type value) throws FHIRFormatError { 2490 if (value != null && !(value instanceof IntegerType || value instanceof DateType || value instanceof TimeType || value instanceof StringType || value instanceof Coding)) 2491 throw new FHIRFormatError("Not the right type for Questionnaire.item.option.value[x]: "+value.fhirType()); 2492 this.value = value; 2493 return this; 2494 } 2495 2496 protected void listChildren(List<Property> children) { 2497 super.listChildren(children); 2498 children.add(new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value)); 2499 } 2500 2501 @Override 2502 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2503 switch (_hash) { 2504 case -1410166417: /*value[x]*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2505 case 111972721: /*value*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2506 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2507 case -766192449: /*valueDate*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2508 case -765708322: /*valueTime*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2509 case -1424603934: /*valueString*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2510 case -1887705029: /*valueCoding*/ return new Property("value[x]", "integer|date|time|string|Coding", "A potential answer that's allowed as the answer to this question.", 0, 1, value); 2511 default: return super.getNamedProperty(_hash, _name, _checkValid); 2512 } 2513 2514 } 2515 2516 @Override 2517 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2518 switch (hash) { 2519 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 2520 default: return super.getProperty(hash, name, checkValid); 2521 } 2522 2523 } 2524 2525 @Override 2526 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2527 switch (hash) { 2528 case 111972721: // value 2529 this.value = castToType(value); // Type 2530 return value; 2531 default: return super.setProperty(hash, name, value); 2532 } 2533 2534 } 2535 2536 @Override 2537 public Base setProperty(String name, Base value) throws FHIRException { 2538 if (name.equals("value[x]")) { 2539 this.value = castToType(value); // Type 2540 } else 2541 return super.setProperty(name, value); 2542 return value; 2543 } 2544 2545 @Override 2546 public Base makeProperty(int hash, String name) throws FHIRException { 2547 switch (hash) { 2548 case -1410166417: return getValue(); 2549 case 111972721: return getValue(); 2550 default: return super.makeProperty(hash, name); 2551 } 2552 2553 } 2554 2555 @Override 2556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2557 switch (hash) { 2558 case 111972721: /*value*/ return new String[] {"integer", "date", "time", "string", "Coding"}; 2559 default: return super.getTypesForProperty(hash, name); 2560 } 2561 2562 } 2563 2564 @Override 2565 public Base addChild(String name) throws FHIRException { 2566 if (name.equals("valueInteger")) { 2567 this.value = new IntegerType(); 2568 return this.value; 2569 } 2570 else if (name.equals("valueDate")) { 2571 this.value = new DateType(); 2572 return this.value; 2573 } 2574 else if (name.equals("valueTime")) { 2575 this.value = new TimeType(); 2576 return this.value; 2577 } 2578 else if (name.equals("valueString")) { 2579 this.value = new StringType(); 2580 return this.value; 2581 } 2582 else if (name.equals("valueCoding")) { 2583 this.value = new Coding(); 2584 return this.value; 2585 } 2586 else 2587 return super.addChild(name); 2588 } 2589 2590 public QuestionnaireItemOptionComponent copy() { 2591 QuestionnaireItemOptionComponent dst = new QuestionnaireItemOptionComponent(); 2592 copyValues(dst); 2593 dst.value = value == null ? null : value.copy(); 2594 return dst; 2595 } 2596 2597 @Override 2598 public boolean equalsDeep(Base other_) { 2599 if (!super.equalsDeep(other_)) 2600 return false; 2601 if (!(other_ instanceof QuestionnaireItemOptionComponent)) 2602 return false; 2603 QuestionnaireItemOptionComponent o = (QuestionnaireItemOptionComponent) other_; 2604 return compareDeep(value, o.value, true); 2605 } 2606 2607 @Override 2608 public boolean equalsShallow(Base other_) { 2609 if (!super.equalsShallow(other_)) 2610 return false; 2611 if (!(other_ instanceof QuestionnaireItemOptionComponent)) 2612 return false; 2613 QuestionnaireItemOptionComponent o = (QuestionnaireItemOptionComponent) other_; 2614 return true; 2615 } 2616 2617 public boolean isEmpty() { 2618 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 2619 } 2620 2621 public String fhirType() { 2622 return "Questionnaire.item.option"; 2623 2624 } 2625 2626 } 2627 2628 /** 2629 * A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance. 2630 */ 2631 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2632 @Description(shortDefinition="Additional identifier for the questionnaire", formalDefinition="A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2633 protected List<Identifier> identifier; 2634 2635 /** 2636 * Explaination of why this questionnaire is needed and why it has been designed as it has. 2637 */ 2638 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2639 @Description(shortDefinition="Why this questionnaire is defined", formalDefinition="Explaination of why this questionnaire is needed and why it has been designed as it has." ) 2640 protected MarkdownType purpose; 2641 2642 /** 2643 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2644 */ 2645 @Child(name = "approvalDate", type = {DateType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2646 @Description(shortDefinition="When the questionnaire was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2647 protected DateType approvalDate; 2648 2649 /** 2650 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 2651 */ 2652 @Child(name = "lastReviewDate", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2653 @Description(shortDefinition="When the questionnaire was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 2654 protected DateType lastReviewDate; 2655 2656 /** 2657 * The period during which the questionnaire content was or is planned to be in active use. 2658 */ 2659 @Child(name = "effectivePeriod", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 2660 @Description(shortDefinition="When the questionnaire is expected to be used", formalDefinition="The period during which the questionnaire content was or is planned to be in active use." ) 2661 protected Period effectivePeriod; 2662 2663 /** 2664 * A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 2665 */ 2666 @Child(name = "copyright", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2667 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire." ) 2668 protected MarkdownType copyright; 2669 2670 /** 2671 * An identifier for this question or group of questions in a particular terminology such as LOINC. 2672 */ 2673 @Child(name = "code", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2674 @Description(shortDefinition="Concept that represents the overall questionnaire", formalDefinition="An identifier for this question or group of questions in a particular terminology such as LOINC." ) 2675 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-questions") 2676 protected List<Coding> code; 2677 2678 /** 2679 * The types of subjects that can be the subject of responses created for the questionnaire. 2680 */ 2681 @Child(name = "subjectType", type = {CodeType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2682 @Description(shortDefinition="Resource that can be subject of QuestionnaireResponse", formalDefinition="The types of subjects that can be the subject of responses created for the questionnaire." ) 2683 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 2684 protected List<CodeType> subjectType; 2685 2686 /** 2687 * A particular question, question grouping or display text that is part of the questionnaire. 2688 */ 2689 @Child(name = "item", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2690 @Description(shortDefinition="Questions and sections within the Questionnaire", formalDefinition="A particular question, question grouping or display text that is part of the questionnaire." ) 2691 protected List<QuestionnaireItemComponent> item; 2692 2693 private static final long serialVersionUID = -1846925043L; 2694 2695 /** 2696 * Constructor 2697 */ 2698 public Questionnaire() { 2699 super(); 2700 } 2701 2702 /** 2703 * Constructor 2704 */ 2705 public Questionnaire(Enumeration<PublicationStatus> status) { 2706 super(); 2707 this.status = status; 2708 } 2709 2710 /** 2711 * @return {@link #url} (An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2712 */ 2713 public UriType getUrlElement() { 2714 if (this.url == null) 2715 if (Configuration.errorOnAutoCreate()) 2716 throw new Error("Attempt to auto-create Questionnaire.url"); 2717 else if (Configuration.doAutoCreate()) 2718 this.url = new UriType(); // bb 2719 return this.url; 2720 } 2721 2722 public boolean hasUrlElement() { 2723 return this.url != null && !this.url.isEmpty(); 2724 } 2725 2726 public boolean hasUrl() { 2727 return this.url != null && !this.url.isEmpty(); 2728 } 2729 2730 /** 2731 * @param value {@link #url} (An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2732 */ 2733 public Questionnaire setUrlElement(UriType value) { 2734 this.url = value; 2735 return this; 2736 } 2737 2738 /** 2739 * @return An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions). 2740 */ 2741 public String getUrl() { 2742 return this.url == null ? null : this.url.getValue(); 2743 } 2744 2745 /** 2746 * @param value An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions). 2747 */ 2748 public Questionnaire setUrl(String value) { 2749 if (Utilities.noString(value)) 2750 this.url = null; 2751 else { 2752 if (this.url == null) 2753 this.url = new UriType(); 2754 this.url.setValue(value); 2755 } 2756 return this; 2757 } 2758 2759 /** 2760 * @return {@link #identifier} (A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2761 */ 2762 public List<Identifier> getIdentifier() { 2763 if (this.identifier == null) 2764 this.identifier = new ArrayList<Identifier>(); 2765 return this.identifier; 2766 } 2767 2768 /** 2769 * @return Returns a reference to <code>this</code> for easy method chaining 2770 */ 2771 public Questionnaire setIdentifier(List<Identifier> theIdentifier) { 2772 this.identifier = theIdentifier; 2773 return this; 2774 } 2775 2776 public boolean hasIdentifier() { 2777 if (this.identifier == null) 2778 return false; 2779 for (Identifier item : this.identifier) 2780 if (!item.isEmpty()) 2781 return true; 2782 return false; 2783 } 2784 2785 public Identifier addIdentifier() { //3 2786 Identifier t = new Identifier(); 2787 if (this.identifier == null) 2788 this.identifier = new ArrayList<Identifier>(); 2789 this.identifier.add(t); 2790 return t; 2791 } 2792 2793 public Questionnaire addIdentifier(Identifier t) { //3 2794 if (t == null) 2795 return this; 2796 if (this.identifier == null) 2797 this.identifier = new ArrayList<Identifier>(); 2798 this.identifier.add(t); 2799 return this; 2800 } 2801 2802 /** 2803 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 2804 */ 2805 public Identifier getIdentifierFirstRep() { 2806 if (getIdentifier().isEmpty()) { 2807 addIdentifier(); 2808 } 2809 return getIdentifier().get(0); 2810 } 2811 2812 /** 2813 * @return {@link #version} (The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2814 */ 2815 public StringType getVersionElement() { 2816 if (this.version == null) 2817 if (Configuration.errorOnAutoCreate()) 2818 throw new Error("Attempt to auto-create Questionnaire.version"); 2819 else if (Configuration.doAutoCreate()) 2820 this.version = new StringType(); // bb 2821 return this.version; 2822 } 2823 2824 public boolean hasVersionElement() { 2825 return this.version != null && !this.version.isEmpty(); 2826 } 2827 2828 public boolean hasVersion() { 2829 return this.version != null && !this.version.isEmpty(); 2830 } 2831 2832 /** 2833 * @param value {@link #version} (The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2834 */ 2835 public Questionnaire setVersionElement(StringType value) { 2836 this.version = value; 2837 return this; 2838 } 2839 2840 /** 2841 * @return The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2842 */ 2843 public String getVersion() { 2844 return this.version == null ? null : this.version.getValue(); 2845 } 2846 2847 /** 2848 * @param value The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2849 */ 2850 public Questionnaire setVersion(String value) { 2851 if (Utilities.noString(value)) 2852 this.version = null; 2853 else { 2854 if (this.version == null) 2855 this.version = new StringType(); 2856 this.version.setValue(value); 2857 } 2858 return this; 2859 } 2860 2861 /** 2862 * @return {@link #name} (A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2863 */ 2864 public StringType getNameElement() { 2865 if (this.name == null) 2866 if (Configuration.errorOnAutoCreate()) 2867 throw new Error("Attempt to auto-create Questionnaire.name"); 2868 else if (Configuration.doAutoCreate()) 2869 this.name = new StringType(); // bb 2870 return this.name; 2871 } 2872 2873 public boolean hasNameElement() { 2874 return this.name != null && !this.name.isEmpty(); 2875 } 2876 2877 public boolean hasName() { 2878 return this.name != null && !this.name.isEmpty(); 2879 } 2880 2881 /** 2882 * @param value {@link #name} (A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2883 */ 2884 public Questionnaire setNameElement(StringType value) { 2885 this.name = value; 2886 return this; 2887 } 2888 2889 /** 2890 * @return A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2891 */ 2892 public String getName() { 2893 return this.name == null ? null : this.name.getValue(); 2894 } 2895 2896 /** 2897 * @param value A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2898 */ 2899 public Questionnaire setName(String value) { 2900 if (Utilities.noString(value)) 2901 this.name = null; 2902 else { 2903 if (this.name == null) 2904 this.name = new StringType(); 2905 this.name.setValue(value); 2906 } 2907 return this; 2908 } 2909 2910 /** 2911 * @return {@link #title} (A short, descriptive, user-friendly title for the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2912 */ 2913 public StringType getTitleElement() { 2914 if (this.title == null) 2915 if (Configuration.errorOnAutoCreate()) 2916 throw new Error("Attempt to auto-create Questionnaire.title"); 2917 else if (Configuration.doAutoCreate()) 2918 this.title = new StringType(); // bb 2919 return this.title; 2920 } 2921 2922 public boolean hasTitleElement() { 2923 return this.title != null && !this.title.isEmpty(); 2924 } 2925 2926 public boolean hasTitle() { 2927 return this.title != null && !this.title.isEmpty(); 2928 } 2929 2930 /** 2931 * @param value {@link #title} (A short, descriptive, user-friendly title for the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2932 */ 2933 public Questionnaire setTitleElement(StringType value) { 2934 this.title = value; 2935 return this; 2936 } 2937 2938 /** 2939 * @return A short, descriptive, user-friendly title for the questionnaire. 2940 */ 2941 public String getTitle() { 2942 return this.title == null ? null : this.title.getValue(); 2943 } 2944 2945 /** 2946 * @param value A short, descriptive, user-friendly title for the questionnaire. 2947 */ 2948 public Questionnaire setTitle(String value) { 2949 if (Utilities.noString(value)) 2950 this.title = null; 2951 else { 2952 if (this.title == null) 2953 this.title = new StringType(); 2954 this.title.setValue(value); 2955 } 2956 return this; 2957 } 2958 2959 /** 2960 * @return {@link #status} (The status of this questionnaire. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2961 */ 2962 public Enumeration<PublicationStatus> getStatusElement() { 2963 if (this.status == null) 2964 if (Configuration.errorOnAutoCreate()) 2965 throw new Error("Attempt to auto-create Questionnaire.status"); 2966 else if (Configuration.doAutoCreate()) 2967 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2968 return this.status; 2969 } 2970 2971 public boolean hasStatusElement() { 2972 return this.status != null && !this.status.isEmpty(); 2973 } 2974 2975 public boolean hasStatus() { 2976 return this.status != null && !this.status.isEmpty(); 2977 } 2978 2979 /** 2980 * @param value {@link #status} (The status of this questionnaire. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2981 */ 2982 public Questionnaire setStatusElement(Enumeration<PublicationStatus> value) { 2983 this.status = value; 2984 return this; 2985 } 2986 2987 /** 2988 * @return The status of this questionnaire. Enables tracking the life-cycle of the content. 2989 */ 2990 public PublicationStatus getStatus() { 2991 return this.status == null ? null : this.status.getValue(); 2992 } 2993 2994 /** 2995 * @param value The status of this questionnaire. Enables tracking the life-cycle of the content. 2996 */ 2997 public Questionnaire setStatus(PublicationStatus value) { 2998 if (this.status == null) 2999 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3000 this.status.setValue(value); 3001 return this; 3002 } 3003 3004 /** 3005 * @return {@link #experimental} (A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3006 */ 3007 public BooleanType getExperimentalElement() { 3008 if (this.experimental == null) 3009 if (Configuration.errorOnAutoCreate()) 3010 throw new Error("Attempt to auto-create Questionnaire.experimental"); 3011 else if (Configuration.doAutoCreate()) 3012 this.experimental = new BooleanType(); // bb 3013 return this.experimental; 3014 } 3015 3016 public boolean hasExperimentalElement() { 3017 return this.experimental != null && !this.experimental.isEmpty(); 3018 } 3019 3020 public boolean hasExperimental() { 3021 return this.experimental != null && !this.experimental.isEmpty(); 3022 } 3023 3024 /** 3025 * @param value {@link #experimental} (A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3026 */ 3027 public Questionnaire setExperimentalElement(BooleanType value) { 3028 this.experimental = value; 3029 return this; 3030 } 3031 3032 /** 3033 * @return A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3034 */ 3035 public boolean getExperimental() { 3036 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3037 } 3038 3039 /** 3040 * @param value A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3041 */ 3042 public Questionnaire setExperimental(boolean value) { 3043 if (this.experimental == null) 3044 this.experimental = new BooleanType(); 3045 this.experimental.setValue(value); 3046 return this; 3047 } 3048 3049 /** 3050 * @return {@link #date} (The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3051 */ 3052 public DateTimeType getDateElement() { 3053 if (this.date == null) 3054 if (Configuration.errorOnAutoCreate()) 3055 throw new Error("Attempt to auto-create Questionnaire.date"); 3056 else if (Configuration.doAutoCreate()) 3057 this.date = new DateTimeType(); // bb 3058 return this.date; 3059 } 3060 3061 public boolean hasDateElement() { 3062 return this.date != null && !this.date.isEmpty(); 3063 } 3064 3065 public boolean hasDate() { 3066 return this.date != null && !this.date.isEmpty(); 3067 } 3068 3069 /** 3070 * @param value {@link #date} (The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3071 */ 3072 public Questionnaire setDateElement(DateTimeType value) { 3073 this.date = value; 3074 return this; 3075 } 3076 3077 /** 3078 * @return The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes. 3079 */ 3080 public Date getDate() { 3081 return this.date == null ? null : this.date.getValue(); 3082 } 3083 3084 /** 3085 * @param value The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes. 3086 */ 3087 public Questionnaire setDate(Date value) { 3088 if (value == null) 3089 this.date = null; 3090 else { 3091 if (this.date == null) 3092 this.date = new DateTimeType(); 3093 this.date.setValue(value); 3094 } 3095 return this; 3096 } 3097 3098 /** 3099 * @return {@link #publisher} (The name of the individual or organization that published the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3100 */ 3101 public StringType getPublisherElement() { 3102 if (this.publisher == null) 3103 if (Configuration.errorOnAutoCreate()) 3104 throw new Error("Attempt to auto-create Questionnaire.publisher"); 3105 else if (Configuration.doAutoCreate()) 3106 this.publisher = new StringType(); // bb 3107 return this.publisher; 3108 } 3109 3110 public boolean hasPublisherElement() { 3111 return this.publisher != null && !this.publisher.isEmpty(); 3112 } 3113 3114 public boolean hasPublisher() { 3115 return this.publisher != null && !this.publisher.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #publisher} (The name of the individual or organization that published the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3120 */ 3121 public Questionnaire setPublisherElement(StringType value) { 3122 this.publisher = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return The name of the individual or organization that published the questionnaire. 3128 */ 3129 public String getPublisher() { 3130 return this.publisher == null ? null : this.publisher.getValue(); 3131 } 3132 3133 /** 3134 * @param value The name of the individual or organization that published the questionnaire. 3135 */ 3136 public Questionnaire setPublisher(String value) { 3137 if (Utilities.noString(value)) 3138 this.publisher = null; 3139 else { 3140 if (this.publisher == null) 3141 this.publisher = new StringType(); 3142 this.publisher.setValue(value); 3143 } 3144 return this; 3145 } 3146 3147 /** 3148 * @return {@link #description} (A free text natural language description of the questionnaire from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3149 */ 3150 public MarkdownType getDescriptionElement() { 3151 if (this.description == null) 3152 if (Configuration.errorOnAutoCreate()) 3153 throw new Error("Attempt to auto-create Questionnaire.description"); 3154 else if (Configuration.doAutoCreate()) 3155 this.description = new MarkdownType(); // bb 3156 return this.description; 3157 } 3158 3159 public boolean hasDescriptionElement() { 3160 return this.description != null && !this.description.isEmpty(); 3161 } 3162 3163 public boolean hasDescription() { 3164 return this.description != null && !this.description.isEmpty(); 3165 } 3166 3167 /** 3168 * @param value {@link #description} (A free text natural language description of the questionnaire from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3169 */ 3170 public Questionnaire setDescriptionElement(MarkdownType value) { 3171 this.description = value; 3172 return this; 3173 } 3174 3175 /** 3176 * @return A free text natural language description of the questionnaire from a consumer's perspective. 3177 */ 3178 public String getDescription() { 3179 return this.description == null ? null : this.description.getValue(); 3180 } 3181 3182 /** 3183 * @param value A free text natural language description of the questionnaire from a consumer's perspective. 3184 */ 3185 public Questionnaire setDescription(String value) { 3186 if (value == null) 3187 this.description = null; 3188 else { 3189 if (this.description == null) 3190 this.description = new MarkdownType(); 3191 this.description.setValue(value); 3192 } 3193 return this; 3194 } 3195 3196 /** 3197 * @return {@link #purpose} (Explaination of why this questionnaire is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3198 */ 3199 public MarkdownType getPurposeElement() { 3200 if (this.purpose == null) 3201 if (Configuration.errorOnAutoCreate()) 3202 throw new Error("Attempt to auto-create Questionnaire.purpose"); 3203 else if (Configuration.doAutoCreate()) 3204 this.purpose = new MarkdownType(); // bb 3205 return this.purpose; 3206 } 3207 3208 public boolean hasPurposeElement() { 3209 return this.purpose != null && !this.purpose.isEmpty(); 3210 } 3211 3212 public boolean hasPurpose() { 3213 return this.purpose != null && !this.purpose.isEmpty(); 3214 } 3215 3216 /** 3217 * @param value {@link #purpose} (Explaination of why this questionnaire is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3218 */ 3219 public Questionnaire setPurposeElement(MarkdownType value) { 3220 this.purpose = value; 3221 return this; 3222 } 3223 3224 /** 3225 * @return Explaination of why this questionnaire is needed and why it has been designed as it has. 3226 */ 3227 public String getPurpose() { 3228 return this.purpose == null ? null : this.purpose.getValue(); 3229 } 3230 3231 /** 3232 * @param value Explaination of why this questionnaire is needed and why it has been designed as it has. 3233 */ 3234 public Questionnaire setPurpose(String value) { 3235 if (value == null) 3236 this.purpose = null; 3237 else { 3238 if (this.purpose == null) 3239 this.purpose = new MarkdownType(); 3240 this.purpose.setValue(value); 3241 } 3242 return this; 3243 } 3244 3245 /** 3246 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3247 */ 3248 public DateType getApprovalDateElement() { 3249 if (this.approvalDate == null) 3250 if (Configuration.errorOnAutoCreate()) 3251 throw new Error("Attempt to auto-create Questionnaire.approvalDate"); 3252 else if (Configuration.doAutoCreate()) 3253 this.approvalDate = new DateType(); // bb 3254 return this.approvalDate; 3255 } 3256 3257 public boolean hasApprovalDateElement() { 3258 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3259 } 3260 3261 public boolean hasApprovalDate() { 3262 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3263 } 3264 3265 /** 3266 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3267 */ 3268 public Questionnaire setApprovalDateElement(DateType value) { 3269 this.approvalDate = value; 3270 return this; 3271 } 3272 3273 /** 3274 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3275 */ 3276 public Date getApprovalDate() { 3277 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3278 } 3279 3280 /** 3281 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3282 */ 3283 public Questionnaire setApprovalDate(Date value) { 3284 if (value == null) 3285 this.approvalDate = null; 3286 else { 3287 if (this.approvalDate == null) 3288 this.approvalDate = new DateType(); 3289 this.approvalDate.setValue(value); 3290 } 3291 return this; 3292 } 3293 3294 /** 3295 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3296 */ 3297 public DateType getLastReviewDateElement() { 3298 if (this.lastReviewDate == null) 3299 if (Configuration.errorOnAutoCreate()) 3300 throw new Error("Attempt to auto-create Questionnaire.lastReviewDate"); 3301 else if (Configuration.doAutoCreate()) 3302 this.lastReviewDate = new DateType(); // bb 3303 return this.lastReviewDate; 3304 } 3305 3306 public boolean hasLastReviewDateElement() { 3307 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3308 } 3309 3310 public boolean hasLastReviewDate() { 3311 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3312 } 3313 3314 /** 3315 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3316 */ 3317 public Questionnaire setLastReviewDateElement(DateType value) { 3318 this.lastReviewDate = value; 3319 return this; 3320 } 3321 3322 /** 3323 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 3324 */ 3325 public Date getLastReviewDate() { 3326 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3327 } 3328 3329 /** 3330 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 3331 */ 3332 public Questionnaire setLastReviewDate(Date value) { 3333 if (value == null) 3334 this.lastReviewDate = null; 3335 else { 3336 if (this.lastReviewDate == null) 3337 this.lastReviewDate = new DateType(); 3338 this.lastReviewDate.setValue(value); 3339 } 3340 return this; 3341 } 3342 3343 /** 3344 * @return {@link #effectivePeriod} (The period during which the questionnaire content was or is planned to be in active use.) 3345 */ 3346 public Period getEffectivePeriod() { 3347 if (this.effectivePeriod == null) 3348 if (Configuration.errorOnAutoCreate()) 3349 throw new Error("Attempt to auto-create Questionnaire.effectivePeriod"); 3350 else if (Configuration.doAutoCreate()) 3351 this.effectivePeriod = new Period(); // cc 3352 return this.effectivePeriod; 3353 } 3354 3355 public boolean hasEffectivePeriod() { 3356 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3357 } 3358 3359 /** 3360 * @param value {@link #effectivePeriod} (The period during which the questionnaire content was or is planned to be in active use.) 3361 */ 3362 public Questionnaire setEffectivePeriod(Period value) { 3363 this.effectivePeriod = value; 3364 return this; 3365 } 3366 3367 /** 3368 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate questionnaire instances.) 3369 */ 3370 public List<UsageContext> getUseContext() { 3371 if (this.useContext == null) 3372 this.useContext = new ArrayList<UsageContext>(); 3373 return this.useContext; 3374 } 3375 3376 /** 3377 * @return Returns a reference to <code>this</code> for easy method chaining 3378 */ 3379 public Questionnaire setUseContext(List<UsageContext> theUseContext) { 3380 this.useContext = theUseContext; 3381 return this; 3382 } 3383 3384 public boolean hasUseContext() { 3385 if (this.useContext == null) 3386 return false; 3387 for (UsageContext item : this.useContext) 3388 if (!item.isEmpty()) 3389 return true; 3390 return false; 3391 } 3392 3393 public UsageContext addUseContext() { //3 3394 UsageContext t = new UsageContext(); 3395 if (this.useContext == null) 3396 this.useContext = new ArrayList<UsageContext>(); 3397 this.useContext.add(t); 3398 return t; 3399 } 3400 3401 public Questionnaire addUseContext(UsageContext t) { //3 3402 if (t == null) 3403 return this; 3404 if (this.useContext == null) 3405 this.useContext = new ArrayList<UsageContext>(); 3406 this.useContext.add(t); 3407 return this; 3408 } 3409 3410 /** 3411 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 3412 */ 3413 public UsageContext getUseContextFirstRep() { 3414 if (getUseContext().isEmpty()) { 3415 addUseContext(); 3416 } 3417 return getUseContext().get(0); 3418 } 3419 3420 /** 3421 * @return {@link #jurisdiction} (A legal or geographic region in which the questionnaire is intended to be used.) 3422 */ 3423 public List<CodeableConcept> getJurisdiction() { 3424 if (this.jurisdiction == null) 3425 this.jurisdiction = new ArrayList<CodeableConcept>(); 3426 return this.jurisdiction; 3427 } 3428 3429 /** 3430 * @return Returns a reference to <code>this</code> for easy method chaining 3431 */ 3432 public Questionnaire setJurisdiction(List<CodeableConcept> theJurisdiction) { 3433 this.jurisdiction = theJurisdiction; 3434 return this; 3435 } 3436 3437 public boolean hasJurisdiction() { 3438 if (this.jurisdiction == null) 3439 return false; 3440 for (CodeableConcept item : this.jurisdiction) 3441 if (!item.isEmpty()) 3442 return true; 3443 return false; 3444 } 3445 3446 public CodeableConcept addJurisdiction() { //3 3447 CodeableConcept t = new CodeableConcept(); 3448 if (this.jurisdiction == null) 3449 this.jurisdiction = new ArrayList<CodeableConcept>(); 3450 this.jurisdiction.add(t); 3451 return t; 3452 } 3453 3454 public Questionnaire addJurisdiction(CodeableConcept t) { //3 3455 if (t == null) 3456 return this; 3457 if (this.jurisdiction == null) 3458 this.jurisdiction = new ArrayList<CodeableConcept>(); 3459 this.jurisdiction.add(t); 3460 return this; 3461 } 3462 3463 /** 3464 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 3465 */ 3466 public CodeableConcept getJurisdictionFirstRep() { 3467 if (getJurisdiction().isEmpty()) { 3468 addJurisdiction(); 3469 } 3470 return getJurisdiction().get(0); 3471 } 3472 3473 /** 3474 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3475 */ 3476 public List<ContactDetail> getContact() { 3477 if (this.contact == null) 3478 this.contact = new ArrayList<ContactDetail>(); 3479 return this.contact; 3480 } 3481 3482 /** 3483 * @return Returns a reference to <code>this</code> for easy method chaining 3484 */ 3485 public Questionnaire setContact(List<ContactDetail> theContact) { 3486 this.contact = theContact; 3487 return this; 3488 } 3489 3490 public boolean hasContact() { 3491 if (this.contact == null) 3492 return false; 3493 for (ContactDetail item : this.contact) 3494 if (!item.isEmpty()) 3495 return true; 3496 return false; 3497 } 3498 3499 public ContactDetail addContact() { //3 3500 ContactDetail t = new ContactDetail(); 3501 if (this.contact == null) 3502 this.contact = new ArrayList<ContactDetail>(); 3503 this.contact.add(t); 3504 return t; 3505 } 3506 3507 public Questionnaire addContact(ContactDetail t) { //3 3508 if (t == null) 3509 return this; 3510 if (this.contact == null) 3511 this.contact = new ArrayList<ContactDetail>(); 3512 this.contact.add(t); 3513 return this; 3514 } 3515 3516 /** 3517 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 3518 */ 3519 public ContactDetail getContactFirstRep() { 3520 if (getContact().isEmpty()) { 3521 addContact(); 3522 } 3523 return getContact().get(0); 3524 } 3525 3526 /** 3527 * @return {@link #copyright} (A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3528 */ 3529 public MarkdownType getCopyrightElement() { 3530 if (this.copyright == null) 3531 if (Configuration.errorOnAutoCreate()) 3532 throw new Error("Attempt to auto-create Questionnaire.copyright"); 3533 else if (Configuration.doAutoCreate()) 3534 this.copyright = new MarkdownType(); // bb 3535 return this.copyright; 3536 } 3537 3538 public boolean hasCopyrightElement() { 3539 return this.copyright != null && !this.copyright.isEmpty(); 3540 } 3541 3542 public boolean hasCopyright() { 3543 return this.copyright != null && !this.copyright.isEmpty(); 3544 } 3545 3546 /** 3547 * @param value {@link #copyright} (A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3548 */ 3549 public Questionnaire setCopyrightElement(MarkdownType value) { 3550 this.copyright = value; 3551 return this; 3552 } 3553 3554 /** 3555 * @return A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 3556 */ 3557 public String getCopyright() { 3558 return this.copyright == null ? null : this.copyright.getValue(); 3559 } 3560 3561 /** 3562 * @param value A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire. 3563 */ 3564 public Questionnaire setCopyright(String value) { 3565 if (value == null) 3566 this.copyright = null; 3567 else { 3568 if (this.copyright == null) 3569 this.copyright = new MarkdownType(); 3570 this.copyright.setValue(value); 3571 } 3572 return this; 3573 } 3574 3575 /** 3576 * @return {@link #code} (An identifier for this question or group of questions in a particular terminology such as LOINC.) 3577 */ 3578 public List<Coding> getCode() { 3579 if (this.code == null) 3580 this.code = new ArrayList<Coding>(); 3581 return this.code; 3582 } 3583 3584 /** 3585 * @return Returns a reference to <code>this</code> for easy method chaining 3586 */ 3587 public Questionnaire setCode(List<Coding> theCode) { 3588 this.code = theCode; 3589 return this; 3590 } 3591 3592 public boolean hasCode() { 3593 if (this.code == null) 3594 return false; 3595 for (Coding item : this.code) 3596 if (!item.isEmpty()) 3597 return true; 3598 return false; 3599 } 3600 3601 public Coding addCode() { //3 3602 Coding t = new Coding(); 3603 if (this.code == null) 3604 this.code = new ArrayList<Coding>(); 3605 this.code.add(t); 3606 return t; 3607 } 3608 3609 public Questionnaire addCode(Coding t) { //3 3610 if (t == null) 3611 return this; 3612 if (this.code == null) 3613 this.code = new ArrayList<Coding>(); 3614 this.code.add(t); 3615 return this; 3616 } 3617 3618 /** 3619 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 3620 */ 3621 public Coding getCodeFirstRep() { 3622 if (getCode().isEmpty()) { 3623 addCode(); 3624 } 3625 return getCode().get(0); 3626 } 3627 3628 /** 3629 * @return {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 3630 */ 3631 public List<CodeType> getSubjectType() { 3632 if (this.subjectType == null) 3633 this.subjectType = new ArrayList<CodeType>(); 3634 return this.subjectType; 3635 } 3636 3637 /** 3638 * @return Returns a reference to <code>this</code> for easy method chaining 3639 */ 3640 public Questionnaire setSubjectType(List<CodeType> theSubjectType) { 3641 this.subjectType = theSubjectType; 3642 return this; 3643 } 3644 3645 public boolean hasSubjectType() { 3646 if (this.subjectType == null) 3647 return false; 3648 for (CodeType item : this.subjectType) 3649 if (!item.isEmpty()) 3650 return true; 3651 return false; 3652 } 3653 3654 /** 3655 * @return {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 3656 */ 3657 public CodeType addSubjectTypeElement() {//2 3658 CodeType t = new CodeType(); 3659 if (this.subjectType == null) 3660 this.subjectType = new ArrayList<CodeType>(); 3661 this.subjectType.add(t); 3662 return t; 3663 } 3664 3665 /** 3666 * @param value {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 3667 */ 3668 public Questionnaire addSubjectType(String value) { //1 3669 CodeType t = new CodeType(); 3670 t.setValue(value); 3671 if (this.subjectType == null) 3672 this.subjectType = new ArrayList<CodeType>(); 3673 this.subjectType.add(t); 3674 return this; 3675 } 3676 3677 /** 3678 * @param value {@link #subjectType} (The types of subjects that can be the subject of responses created for the questionnaire.) 3679 */ 3680 public boolean hasSubjectType(String value) { 3681 if (this.subjectType == null) 3682 return false; 3683 for (CodeType v : this.subjectType) 3684 if (v.getValue().equals(value)) // code 3685 return true; 3686 return false; 3687 } 3688 3689 /** 3690 * @return {@link #item} (A particular question, question grouping or display text that is part of the questionnaire.) 3691 */ 3692 public List<QuestionnaireItemComponent> getItem() { 3693 if (this.item == null) 3694 this.item = new ArrayList<QuestionnaireItemComponent>(); 3695 return this.item; 3696 } 3697 3698 /** 3699 * @return Returns a reference to <code>this</code> for easy method chaining 3700 */ 3701 public Questionnaire setItem(List<QuestionnaireItemComponent> theItem) { 3702 this.item = theItem; 3703 return this; 3704 } 3705 3706 public boolean hasItem() { 3707 if (this.item == null) 3708 return false; 3709 for (QuestionnaireItemComponent item : this.item) 3710 if (!item.isEmpty()) 3711 return true; 3712 return false; 3713 } 3714 3715 public QuestionnaireItemComponent addItem() { //3 3716 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 3717 if (this.item == null) 3718 this.item = new ArrayList<QuestionnaireItemComponent>(); 3719 this.item.add(t); 3720 return t; 3721 } 3722 3723 public Questionnaire addItem(QuestionnaireItemComponent t) { //3 3724 if (t == null) 3725 return this; 3726 if (this.item == null) 3727 this.item = new ArrayList<QuestionnaireItemComponent>(); 3728 this.item.add(t); 3729 return this; 3730 } 3731 3732 /** 3733 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 3734 */ 3735 public QuestionnaireItemComponent getItemFirstRep() { 3736 if (getItem().isEmpty()) { 3737 addItem(); 3738 } 3739 return getItem().get(0); 3740 } 3741 3742 protected void listChildren(List<Property> children) { 3743 super.listChildren(children); 3744 children.add(new Property("url", "uri", "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 3745 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3746 children.add(new Property("version", "string", "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3747 children.add(new Property("name", "string", "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3748 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the questionnaire.", 0, 1, title)); 3749 children.add(new Property("status", "code", "The status of this questionnaire. Enables tracking the life-cycle of the content.", 0, 1, status)); 3750 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3751 children.add(new Property("date", "dateTime", "The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 0, 1, date)); 3752 children.add(new Property("publisher", "string", "The name of the individual or organization that published the questionnaire.", 0, 1, publisher)); 3753 children.add(new Property("description", "markdown", "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, description)); 3754 children.add(new Property("purpose", "markdown", "Explaination of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose)); 3755 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3756 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3757 children.add(new Property("effectivePeriod", "Period", "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, effectivePeriod)); 3758 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate questionnaire instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3759 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the questionnaire is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3760 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3761 children.add(new Property("copyright", "markdown", "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 0, 1, copyright)); 3762 children.add(new Property("code", "Coding", "An identifier for this question or group of questions in a particular terminology such as LOINC.", 0, java.lang.Integer.MAX_VALUE, code)); 3763 children.add(new Property("subjectType", "code", "The types of subjects that can be the subject of responses created for the questionnaire.", 0, java.lang.Integer.MAX_VALUE, subjectType)); 3764 children.add(new Property("item", "", "A particular question, question grouping or display text that is part of the questionnaire.", 0, java.lang.Integer.MAX_VALUE, item)); 3765 } 3766 3767 @Override 3768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3769 switch (_hash) { 3770 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this questionnaire is (or will be) published. The URL SHOULD include the major version of the questionnaire. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 3771 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3772 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3773 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3774 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the questionnaire.", 0, 1, title); 3775 case -892481550: /*status*/ return new Property("status", "code", "The status of this questionnaire. Enables tracking the life-cycle of the content.", 0, 1, status); 3776 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3777 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the questionnaire was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 0, 1, date); 3778 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the questionnaire.", 0, 1, publisher); 3779 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, description); 3780 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose); 3781 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3782 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 3783 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, effectivePeriod); 3784 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate questionnaire instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3785 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the questionnaire is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3786 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3787 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 0, 1, copyright); 3788 case 3059181: /*code*/ return new Property("code", "Coding", "An identifier for this question or group of questions in a particular terminology such as LOINC.", 0, java.lang.Integer.MAX_VALUE, code); 3789 case -603200890: /*subjectType*/ return new Property("subjectType", "code", "The types of subjects that can be the subject of responses created for the questionnaire.", 0, java.lang.Integer.MAX_VALUE, subjectType); 3790 case 3242771: /*item*/ return new Property("item", "", "A particular question, question grouping or display text that is part of the questionnaire.", 0, java.lang.Integer.MAX_VALUE, item); 3791 default: return super.getNamedProperty(_hash, _name, _checkValid); 3792 } 3793 3794 } 3795 3796 @Override 3797 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3798 switch (hash) { 3799 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3800 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3801 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3802 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3803 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3804 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3805 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3806 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3807 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3808 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3809 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3810 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 3811 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 3812 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 3813 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3814 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3815 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3816 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3817 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 3818 case -603200890: /*subjectType*/ return this.subjectType == null ? new Base[0] : this.subjectType.toArray(new Base[this.subjectType.size()]); // CodeType 3819 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 3820 default: return super.getProperty(hash, name, checkValid); 3821 } 3822 3823 } 3824 3825 @Override 3826 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3827 switch (hash) { 3828 case 116079: // url 3829 this.url = castToUri(value); // UriType 3830 return value; 3831 case -1618432855: // identifier 3832 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3833 return value; 3834 case 351608024: // version 3835 this.version = castToString(value); // StringType 3836 return value; 3837 case 3373707: // name 3838 this.name = castToString(value); // StringType 3839 return value; 3840 case 110371416: // title 3841 this.title = castToString(value); // StringType 3842 return value; 3843 case -892481550: // status 3844 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3845 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3846 return value; 3847 case -404562712: // experimental 3848 this.experimental = castToBoolean(value); // BooleanType 3849 return value; 3850 case 3076014: // date 3851 this.date = castToDateTime(value); // DateTimeType 3852 return value; 3853 case 1447404028: // publisher 3854 this.publisher = castToString(value); // StringType 3855 return value; 3856 case -1724546052: // description 3857 this.description = castToMarkdown(value); // MarkdownType 3858 return value; 3859 case -220463842: // purpose 3860 this.purpose = castToMarkdown(value); // MarkdownType 3861 return value; 3862 case 223539345: // approvalDate 3863 this.approvalDate = castToDate(value); // DateType 3864 return value; 3865 case -1687512484: // lastReviewDate 3866 this.lastReviewDate = castToDate(value); // DateType 3867 return value; 3868 case -403934648: // effectivePeriod 3869 this.effectivePeriod = castToPeriod(value); // Period 3870 return value; 3871 case -669707736: // useContext 3872 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3873 return value; 3874 case -507075711: // jurisdiction 3875 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3876 return value; 3877 case 951526432: // contact 3878 this.getContact().add(castToContactDetail(value)); // ContactDetail 3879 return value; 3880 case 1522889671: // copyright 3881 this.copyright = castToMarkdown(value); // MarkdownType 3882 return value; 3883 case 3059181: // code 3884 this.getCode().add(castToCoding(value)); // Coding 3885 return value; 3886 case -603200890: // subjectType 3887 this.getSubjectType().add(castToCode(value)); // CodeType 3888 return value; 3889 case 3242771: // item 3890 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 3891 return value; 3892 default: return super.setProperty(hash, name, value); 3893 } 3894 3895 } 3896 3897 @Override 3898 public Base setProperty(String name, Base value) throws FHIRException { 3899 if (name.equals("url")) { 3900 this.url = castToUri(value); // UriType 3901 } else if (name.equals("identifier")) { 3902 this.getIdentifier().add(castToIdentifier(value)); 3903 } else if (name.equals("version")) { 3904 this.version = castToString(value); // StringType 3905 } else if (name.equals("name")) { 3906 this.name = castToString(value); // StringType 3907 } else if (name.equals("title")) { 3908 this.title = castToString(value); // StringType 3909 } else if (name.equals("status")) { 3910 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3911 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3912 } else if (name.equals("experimental")) { 3913 this.experimental = castToBoolean(value); // BooleanType 3914 } else if (name.equals("date")) { 3915 this.date = castToDateTime(value); // DateTimeType 3916 } else if (name.equals("publisher")) { 3917 this.publisher = castToString(value); // StringType 3918 } else if (name.equals("description")) { 3919 this.description = castToMarkdown(value); // MarkdownType 3920 } else if (name.equals("purpose")) { 3921 this.purpose = castToMarkdown(value); // MarkdownType 3922 } else if (name.equals("approvalDate")) { 3923 this.approvalDate = castToDate(value); // DateType 3924 } else if (name.equals("lastReviewDate")) { 3925 this.lastReviewDate = castToDate(value); // DateType 3926 } else if (name.equals("effectivePeriod")) { 3927 this.effectivePeriod = castToPeriod(value); // Period 3928 } else if (name.equals("useContext")) { 3929 this.getUseContext().add(castToUsageContext(value)); 3930 } else if (name.equals("jurisdiction")) { 3931 this.getJurisdiction().add(castToCodeableConcept(value)); 3932 } else if (name.equals("contact")) { 3933 this.getContact().add(castToContactDetail(value)); 3934 } else if (name.equals("copyright")) { 3935 this.copyright = castToMarkdown(value); // MarkdownType 3936 } else if (name.equals("code")) { 3937 this.getCode().add(castToCoding(value)); 3938 } else if (name.equals("subjectType")) { 3939 this.getSubjectType().add(castToCode(value)); 3940 } else if (name.equals("item")) { 3941 this.getItem().add((QuestionnaireItemComponent) value); 3942 } else 3943 return super.setProperty(name, value); 3944 return value; 3945 } 3946 3947 @Override 3948 public Base makeProperty(int hash, String name) throws FHIRException { 3949 switch (hash) { 3950 case 116079: return getUrlElement(); 3951 case -1618432855: return addIdentifier(); 3952 case 351608024: return getVersionElement(); 3953 case 3373707: return getNameElement(); 3954 case 110371416: return getTitleElement(); 3955 case -892481550: return getStatusElement(); 3956 case -404562712: return getExperimentalElement(); 3957 case 3076014: return getDateElement(); 3958 case 1447404028: return getPublisherElement(); 3959 case -1724546052: return getDescriptionElement(); 3960 case -220463842: return getPurposeElement(); 3961 case 223539345: return getApprovalDateElement(); 3962 case -1687512484: return getLastReviewDateElement(); 3963 case -403934648: return getEffectivePeriod(); 3964 case -669707736: return addUseContext(); 3965 case -507075711: return addJurisdiction(); 3966 case 951526432: return addContact(); 3967 case 1522889671: return getCopyrightElement(); 3968 case 3059181: return addCode(); 3969 case -603200890: return addSubjectTypeElement(); 3970 case 3242771: return addItem(); 3971 default: return super.makeProperty(hash, name); 3972 } 3973 3974 } 3975 3976 @Override 3977 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3978 switch (hash) { 3979 case 116079: /*url*/ return new String[] {"uri"}; 3980 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3981 case 351608024: /*version*/ return new String[] {"string"}; 3982 case 3373707: /*name*/ return new String[] {"string"}; 3983 case 110371416: /*title*/ return new String[] {"string"}; 3984 case -892481550: /*status*/ return new String[] {"code"}; 3985 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3986 case 3076014: /*date*/ return new String[] {"dateTime"}; 3987 case 1447404028: /*publisher*/ return new String[] {"string"}; 3988 case -1724546052: /*description*/ return new String[] {"markdown"}; 3989 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3990 case 223539345: /*approvalDate*/ return new String[] {"date"}; 3991 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 3992 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 3993 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3994 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3995 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3996 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3997 case 3059181: /*code*/ return new String[] {"Coding"}; 3998 case -603200890: /*subjectType*/ return new String[] {"code"}; 3999 case 3242771: /*item*/ return new String[] {}; 4000 default: return super.getTypesForProperty(hash, name); 4001 } 4002 4003 } 4004 4005 @Override 4006 public Base addChild(String name) throws FHIRException { 4007 if (name.equals("url")) { 4008 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.url"); 4009 } 4010 else if (name.equals("identifier")) { 4011 return addIdentifier(); 4012 } 4013 else if (name.equals("version")) { 4014 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.version"); 4015 } 4016 else if (name.equals("name")) { 4017 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.name"); 4018 } 4019 else if (name.equals("title")) { 4020 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.title"); 4021 } 4022 else if (name.equals("status")) { 4023 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.status"); 4024 } 4025 else if (name.equals("experimental")) { 4026 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.experimental"); 4027 } 4028 else if (name.equals("date")) { 4029 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.date"); 4030 } 4031 else if (name.equals("publisher")) { 4032 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.publisher"); 4033 } 4034 else if (name.equals("description")) { 4035 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.description"); 4036 } 4037 else if (name.equals("purpose")) { 4038 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.purpose"); 4039 } 4040 else if (name.equals("approvalDate")) { 4041 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.approvalDate"); 4042 } 4043 else if (name.equals("lastReviewDate")) { 4044 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.lastReviewDate"); 4045 } 4046 else if (name.equals("effectivePeriod")) { 4047 this.effectivePeriod = new Period(); 4048 return this.effectivePeriod; 4049 } 4050 else if (name.equals("useContext")) { 4051 return addUseContext(); 4052 } 4053 else if (name.equals("jurisdiction")) { 4054 return addJurisdiction(); 4055 } 4056 else if (name.equals("contact")) { 4057 return addContact(); 4058 } 4059 else if (name.equals("copyright")) { 4060 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.copyright"); 4061 } 4062 else if (name.equals("code")) { 4063 return addCode(); 4064 } 4065 else if (name.equals("subjectType")) { 4066 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.subjectType"); 4067 } 4068 else if (name.equals("item")) { 4069 return addItem(); 4070 } 4071 else 4072 return super.addChild(name); 4073 } 4074 4075 public String fhirType() { 4076 return "Questionnaire"; 4077 4078 } 4079 4080 public Questionnaire copy() { 4081 Questionnaire dst = new Questionnaire(); 4082 copyValues(dst); 4083 dst.url = url == null ? null : url.copy(); 4084 if (identifier != null) { 4085 dst.identifier = new ArrayList<Identifier>(); 4086 for (Identifier i : identifier) 4087 dst.identifier.add(i.copy()); 4088 }; 4089 dst.version = version == null ? null : version.copy(); 4090 dst.name = name == null ? null : name.copy(); 4091 dst.title = title == null ? null : title.copy(); 4092 dst.status = status == null ? null : status.copy(); 4093 dst.experimental = experimental == null ? null : experimental.copy(); 4094 dst.date = date == null ? null : date.copy(); 4095 dst.publisher = publisher == null ? null : publisher.copy(); 4096 dst.description = description == null ? null : description.copy(); 4097 dst.purpose = purpose == null ? null : purpose.copy(); 4098 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4099 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4100 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4101 if (useContext != null) { 4102 dst.useContext = new ArrayList<UsageContext>(); 4103 for (UsageContext i : useContext) 4104 dst.useContext.add(i.copy()); 4105 }; 4106 if (jurisdiction != null) { 4107 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4108 for (CodeableConcept i : jurisdiction) 4109 dst.jurisdiction.add(i.copy()); 4110 }; 4111 if (contact != null) { 4112 dst.contact = new ArrayList<ContactDetail>(); 4113 for (ContactDetail i : contact) 4114 dst.contact.add(i.copy()); 4115 }; 4116 dst.copyright = copyright == null ? null : copyright.copy(); 4117 if (code != null) { 4118 dst.code = new ArrayList<Coding>(); 4119 for (Coding i : code) 4120 dst.code.add(i.copy()); 4121 }; 4122 if (subjectType != null) { 4123 dst.subjectType = new ArrayList<CodeType>(); 4124 for (CodeType i : subjectType) 4125 dst.subjectType.add(i.copy()); 4126 }; 4127 if (item != null) { 4128 dst.item = new ArrayList<QuestionnaireItemComponent>(); 4129 for (QuestionnaireItemComponent i : item) 4130 dst.item.add(i.copy()); 4131 }; 4132 return dst; 4133 } 4134 4135 protected Questionnaire typedCopy() { 4136 return copy(); 4137 } 4138 4139 @Override 4140 public boolean equalsDeep(Base other_) { 4141 if (!super.equalsDeep(other_)) 4142 return false; 4143 if (!(other_ instanceof Questionnaire)) 4144 return false; 4145 Questionnaire o = (Questionnaire) other_; 4146 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(approvalDate, o.approvalDate, true) 4147 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 4148 && compareDeep(copyright, o.copyright, true) && compareDeep(code, o.code, true) && compareDeep(subjectType, o.subjectType, true) 4149 && compareDeep(item, o.item, true); 4150 } 4151 4152 @Override 4153 public boolean equalsShallow(Base other_) { 4154 if (!super.equalsShallow(other_)) 4155 return false; 4156 if (!(other_ instanceof Questionnaire)) 4157 return false; 4158 Questionnaire o = (Questionnaire) other_; 4159 return compareValues(purpose, o.purpose, true) && compareValues(approvalDate, o.approvalDate, true) 4160 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 4161 && compareValues(subjectType, o.subjectType, true); 4162 } 4163 4164 public boolean isEmpty() { 4165 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, approvalDate 4166 , lastReviewDate, effectivePeriod, copyright, code, subjectType, item); 4167 } 4168 4169 @Override 4170 public ResourceType getResourceType() { 4171 return ResourceType.Questionnaire; 4172 } 4173 4174 /** 4175 * Search parameter: <b>date</b> 4176 * <p> 4177 * Description: <b>The questionnaire publication date</b><br> 4178 * Type: <b>date</b><br> 4179 * Path: <b>Questionnaire.date</b><br> 4180 * </p> 4181 */ 4182 @SearchParamDefinition(name="date", path="Questionnaire.date", description="The questionnaire publication date", type="date" ) 4183 public static final String SP_DATE = "date"; 4184 /** 4185 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4186 * <p> 4187 * Description: <b>The questionnaire publication date</b><br> 4188 * Type: <b>date</b><br> 4189 * Path: <b>Questionnaire.date</b><br> 4190 * </p> 4191 */ 4192 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4193 4194 /** 4195 * Search parameter: <b>identifier</b> 4196 * <p> 4197 * Description: <b>External identifier for the questionnaire</b><br> 4198 * Type: <b>token</b><br> 4199 * Path: <b>Questionnaire.identifier</b><br> 4200 * </p> 4201 */ 4202 @SearchParamDefinition(name="identifier", path="Questionnaire.identifier", description="External identifier for the questionnaire", type="token" ) 4203 public static final String SP_IDENTIFIER = "identifier"; 4204 /** 4205 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4206 * <p> 4207 * Description: <b>External identifier for the questionnaire</b><br> 4208 * Type: <b>token</b><br> 4209 * Path: <b>Questionnaire.identifier</b><br> 4210 * </p> 4211 */ 4212 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4213 4214 /** 4215 * Search parameter: <b>effective</b> 4216 * <p> 4217 * Description: <b>The time during which the questionnaire is intended to be in use</b><br> 4218 * Type: <b>date</b><br> 4219 * Path: <b>Questionnaire.effectivePeriod</b><br> 4220 * </p> 4221 */ 4222 @SearchParamDefinition(name="effective", path="Questionnaire.effectivePeriod", description="The time during which the questionnaire is intended to be in use", type="date" ) 4223 public static final String SP_EFFECTIVE = "effective"; 4224 /** 4225 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4226 * <p> 4227 * Description: <b>The time during which the questionnaire is intended to be in use</b><br> 4228 * Type: <b>date</b><br> 4229 * Path: <b>Questionnaire.effectivePeriod</b><br> 4230 * </p> 4231 */ 4232 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4233 4234 /** 4235 * Search parameter: <b>code</b> 4236 * <p> 4237 * Description: <b>A code that corresponds to one of its items in the questionnaire</b><br> 4238 * Type: <b>token</b><br> 4239 * Path: <b>Questionnaire.item.code</b><br> 4240 * </p> 4241 */ 4242 @SearchParamDefinition(name="code", path="Questionnaire.item.code", description="A code that corresponds to one of its items in the questionnaire", type="token" ) 4243 public static final String SP_CODE = "code"; 4244 /** 4245 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4246 * <p> 4247 * Description: <b>A code that corresponds to one of its items in the questionnaire</b><br> 4248 * Type: <b>token</b><br> 4249 * Path: <b>Questionnaire.item.code</b><br> 4250 * </p> 4251 */ 4252 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4253 4254 /** 4255 * Search parameter: <b>jurisdiction</b> 4256 * <p> 4257 * Description: <b>Intended jurisdiction for the questionnaire</b><br> 4258 * Type: <b>token</b><br> 4259 * Path: <b>Questionnaire.jurisdiction</b><br> 4260 * </p> 4261 */ 4262 @SearchParamDefinition(name="jurisdiction", path="Questionnaire.jurisdiction", description="Intended jurisdiction for the questionnaire", type="token" ) 4263 public static final String SP_JURISDICTION = "jurisdiction"; 4264 /** 4265 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4266 * <p> 4267 * Description: <b>Intended jurisdiction for the questionnaire</b><br> 4268 * Type: <b>token</b><br> 4269 * Path: <b>Questionnaire.jurisdiction</b><br> 4270 * </p> 4271 */ 4272 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4273 4274 /** 4275 * Search parameter: <b>name</b> 4276 * <p> 4277 * Description: <b>Computationally friendly name of the questionnaire</b><br> 4278 * Type: <b>string</b><br> 4279 * Path: <b>Questionnaire.name</b><br> 4280 * </p> 4281 */ 4282 @SearchParamDefinition(name="name", path="Questionnaire.name", description="Computationally friendly name of the questionnaire", type="string" ) 4283 public static final String SP_NAME = "name"; 4284 /** 4285 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4286 * <p> 4287 * Description: <b>Computationally friendly name of the questionnaire</b><br> 4288 * Type: <b>string</b><br> 4289 * Path: <b>Questionnaire.name</b><br> 4290 * </p> 4291 */ 4292 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4293 4294 /** 4295 * Search parameter: <b>description</b> 4296 * <p> 4297 * Description: <b>The description of the questionnaire</b><br> 4298 * Type: <b>string</b><br> 4299 * Path: <b>Questionnaire.description</b><br> 4300 * </p> 4301 */ 4302 @SearchParamDefinition(name="description", path="Questionnaire.description", description="The description of the questionnaire", type="string" ) 4303 public static final String SP_DESCRIPTION = "description"; 4304 /** 4305 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4306 * <p> 4307 * Description: <b>The description of the questionnaire</b><br> 4308 * Type: <b>string</b><br> 4309 * Path: <b>Questionnaire.description</b><br> 4310 * </p> 4311 */ 4312 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4313 4314 /** 4315 * Search parameter: <b>publisher</b> 4316 * <p> 4317 * Description: <b>Name of the publisher of the questionnaire</b><br> 4318 * Type: <b>string</b><br> 4319 * Path: <b>Questionnaire.publisher</b><br> 4320 * </p> 4321 */ 4322 @SearchParamDefinition(name="publisher", path="Questionnaire.publisher", description="Name of the publisher of the questionnaire", type="string" ) 4323 public static final String SP_PUBLISHER = "publisher"; 4324 /** 4325 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4326 * <p> 4327 * Description: <b>Name of the publisher of the questionnaire</b><br> 4328 * Type: <b>string</b><br> 4329 * Path: <b>Questionnaire.publisher</b><br> 4330 * </p> 4331 */ 4332 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4333 4334 /** 4335 * Search parameter: <b>title</b> 4336 * <p> 4337 * Description: <b>The human-friendly name of the questionnaire</b><br> 4338 * Type: <b>string</b><br> 4339 * Path: <b>Questionnaire.title</b><br> 4340 * </p> 4341 */ 4342 @SearchParamDefinition(name="title", path="Questionnaire.title", description="The human-friendly name of the questionnaire", type="string" ) 4343 public static final String SP_TITLE = "title"; 4344 /** 4345 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4346 * <p> 4347 * Description: <b>The human-friendly name of the questionnaire</b><br> 4348 * Type: <b>string</b><br> 4349 * Path: <b>Questionnaire.title</b><br> 4350 * </p> 4351 */ 4352 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4353 4354 /** 4355 * Search parameter: <b>version</b> 4356 * <p> 4357 * Description: <b>The business version of the questionnaire</b><br> 4358 * Type: <b>token</b><br> 4359 * Path: <b>Questionnaire.version</b><br> 4360 * </p> 4361 */ 4362 @SearchParamDefinition(name="version", path="Questionnaire.version", description="The business version of the questionnaire", type="token" ) 4363 public static final String SP_VERSION = "version"; 4364 /** 4365 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4366 * <p> 4367 * Description: <b>The business version of the questionnaire</b><br> 4368 * Type: <b>token</b><br> 4369 * Path: <b>Questionnaire.version</b><br> 4370 * </p> 4371 */ 4372 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4373 4374 /** 4375 * Search parameter: <b>url</b> 4376 * <p> 4377 * Description: <b>The uri that identifies the questionnaire</b><br> 4378 * Type: <b>uri</b><br> 4379 * Path: <b>Questionnaire.url</b><br> 4380 * </p> 4381 */ 4382 @SearchParamDefinition(name="url", path="Questionnaire.url", description="The uri that identifies the questionnaire", type="uri" ) 4383 public static final String SP_URL = "url"; 4384 /** 4385 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4386 * <p> 4387 * Description: <b>The uri that identifies the questionnaire</b><br> 4388 * Type: <b>uri</b><br> 4389 * Path: <b>Questionnaire.url</b><br> 4390 * </p> 4391 */ 4392 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4393 4394 /** 4395 * Search parameter: <b>status</b> 4396 * <p> 4397 * Description: <b>The current status of the questionnaire</b><br> 4398 * Type: <b>token</b><br> 4399 * Path: <b>Questionnaire.status</b><br> 4400 * </p> 4401 */ 4402 @SearchParamDefinition(name="status", path="Questionnaire.status", description="The current status of the questionnaire", type="token" ) 4403 public static final String SP_STATUS = "status"; 4404 /** 4405 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4406 * <p> 4407 * Description: <b>The current status of the questionnaire</b><br> 4408 * Type: <b>token</b><br> 4409 * Path: <b>Questionnaire.status</b><br> 4410 * </p> 4411 */ 4412 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4413 4414 4415}