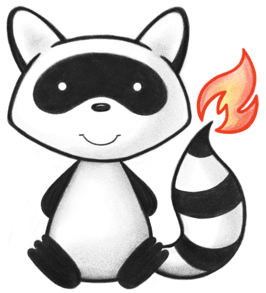
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 052 */ 053@ResourceDef(name="QuestionnaireResponse", profile="http://hl7.org/fhir/Profile/QuestionnaireResponse") 054public class QuestionnaireResponse extends DomainResource { 055 056 public enum QuestionnaireResponseStatus { 057 /** 058 * This QuestionnaireResponse has been partially filled out with answers, but changes or additions are still expected to be made to it. 059 */ 060 INPROGRESS, 061 /** 062 * This QuestionnaireResponse has been filled out with answers, and the current content is regarded as definitive. 063 */ 064 COMPLETED, 065 /** 066 * This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards. 067 */ 068 AMENDED, 069 /** 070 * This QuestionnaireResponse was entered in error and voided. 071 */ 072 ENTEREDINERROR, 073 /** 074 * This QuestionnaireResponse has been partially filled out with answers, but has been abandoned. It is unknown whether changes or additions are expected to be made to it. 075 */ 076 STOPPED, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("in-progress".equals(codeString)) 085 return INPROGRESS; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if ("amended".equals(codeString)) 089 return AMENDED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("stopped".equals(codeString)) 093 return STOPPED; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case INPROGRESS: return "in-progress"; 102 case COMPLETED: return "completed"; 103 case AMENDED: return "amended"; 104 case ENTEREDINERROR: return "entered-in-error"; 105 case STOPPED: return "stopped"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case INPROGRESS: return "http://hl7.org/fhir/questionnaire-answers-status"; 113 case COMPLETED: return "http://hl7.org/fhir/questionnaire-answers-status"; 114 case AMENDED: return "http://hl7.org/fhir/questionnaire-answers-status"; 115 case ENTEREDINERROR: return "http://hl7.org/fhir/questionnaire-answers-status"; 116 case STOPPED: return "http://hl7.org/fhir/questionnaire-answers-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case INPROGRESS: return "This QuestionnaireResponse has been partially filled out with answers, but changes or additions are still expected to be made to it."; 124 case COMPLETED: return "This QuestionnaireResponse has been filled out with answers, and the current content is regarded as definitive."; 125 case AMENDED: return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 126 case ENTEREDINERROR: return "This QuestionnaireResponse was entered in error and voided."; 127 case STOPPED: return "This QuestionnaireResponse has been partially filled out with answers, but has been abandoned. It is unknown whether changes or additions are expected to be made to it."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case INPROGRESS: return "In Progress"; 135 case COMPLETED: return "Completed"; 136 case AMENDED: return "Amended"; 137 case ENTEREDINERROR: return "Entered in Error"; 138 case STOPPED: return "Stopped"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 146 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("in-progress".equals(codeString)) 151 return QuestionnaireResponseStatus.INPROGRESS; 152 if ("completed".equals(codeString)) 153 return QuestionnaireResponseStatus.COMPLETED; 154 if ("amended".equals(codeString)) 155 return QuestionnaireResponseStatus.AMENDED; 156 if ("entered-in-error".equals(codeString)) 157 return QuestionnaireResponseStatus.ENTEREDINERROR; 158 if ("stopped".equals(codeString)) 159 return QuestionnaireResponseStatus.STOPPED; 160 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 161 } 162 public Enumeration<QuestionnaireResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<QuestionnaireResponseStatus>(this); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("in-progress".equals(codeString)) 171 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS); 172 if ("completed".equals(codeString)) 173 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED); 174 if ("amended".equals(codeString)) 175 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.ENTEREDINERROR); 178 if ("stopped".equals(codeString)) 179 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.STOPPED); 180 throw new FHIRException("Unknown QuestionnaireResponseStatus code '"+codeString+"'"); 181 } 182 public String toCode(QuestionnaireResponseStatus code) { 183 if (code == QuestionnaireResponseStatus.NULL) 184 return null; 185 if (code == QuestionnaireResponseStatus.INPROGRESS) 186 return "in-progress"; 187 if (code == QuestionnaireResponseStatus.COMPLETED) 188 return "completed"; 189 if (code == QuestionnaireResponseStatus.AMENDED) 190 return "amended"; 191 if (code == QuestionnaireResponseStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 if (code == QuestionnaireResponseStatus.STOPPED) 194 return "stopped"; 195 return "?"; 196 } 197 public String toSystem(QuestionnaireResponseStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class QuestionnaireResponseItemComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 206 */ 207 @Child(name = "linkId", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="Pointer to specific item from Questionnaire", formalDefinition="The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource." ) 209 protected StringType linkId; 210 211 /** 212 * A reference to an [[[ElementDefinition]]] that provides the details for the item. 213 */ 214 @Child(name = "definition", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 215 @Description(shortDefinition="ElementDefinition - details for the item", formalDefinition="A reference to an [[[ElementDefinition]]] that provides the details for the item." ) 216 protected UriType definition; 217 218 /** 219 * Text that is displayed above the contents of the group or as the text of the question being answered. 220 */ 221 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Name for group or question text", formalDefinition="Text that is displayed above the contents of the group or as the text of the question being answered." ) 223 protected StringType text; 224 225 /** 226 * More specific subject this section's answers are about, details the subject given in QuestionnaireResponse. 227 */ 228 @Child(name = "subject", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="The subject this group's answers are about", formalDefinition="More specific subject this section's answers are about, details the subject given in QuestionnaireResponse." ) 230 protected Reference subject; 231 232 /** 233 * The actual object that is the target of the reference (More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.) 234 */ 235 protected Resource subjectTarget; 236 237 /** 238 * The respondent's answer(s) to the question. 239 */ 240 @Child(name = "answer", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 241 @Description(shortDefinition="The response(s) to the question", formalDefinition="The respondent's answer(s) to the question." ) 242 protected List<QuestionnaireResponseItemAnswerComponent> answer; 243 244 /** 245 * Questions or sub-groups nested beneath a question or group. 246 */ 247 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 248 @Description(shortDefinition="Nested questionnaire response items", formalDefinition="Questions or sub-groups nested beneath a question or group." ) 249 protected List<QuestionnaireResponseItemComponent> item; 250 251 private static final long serialVersionUID = -154786340L; 252 253 /** 254 * Constructor 255 */ 256 public QuestionnaireResponseItemComponent() { 257 super(); 258 } 259 260 /** 261 * Constructor 262 */ 263 public QuestionnaireResponseItemComponent(StringType linkId) { 264 super(); 265 this.linkId = linkId; 266 } 267 268 /** 269 * @return {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 270 */ 271 public StringType getLinkIdElement() { 272 if (this.linkId == null) 273 if (Configuration.errorOnAutoCreate()) 274 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.linkId"); 275 else if (Configuration.doAutoCreate()) 276 this.linkId = new StringType(); // bb 277 return this.linkId; 278 } 279 280 public boolean hasLinkIdElement() { 281 return this.linkId != null && !this.linkId.isEmpty(); 282 } 283 284 public boolean hasLinkId() { 285 return this.linkId != null && !this.linkId.isEmpty(); 286 } 287 288 /** 289 * @param value {@link #linkId} (The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 290 */ 291 public QuestionnaireResponseItemComponent setLinkIdElement(StringType value) { 292 this.linkId = value; 293 return this; 294 } 295 296 /** 297 * @return The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 298 */ 299 public String getLinkId() { 300 return this.linkId == null ? null : this.linkId.getValue(); 301 } 302 303 /** 304 * @param value The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource. 305 */ 306 public QuestionnaireResponseItemComponent setLinkId(String value) { 307 if (this.linkId == null) 308 this.linkId = new StringType(); 309 this.linkId.setValue(value); 310 return this; 311 } 312 313 /** 314 * @return {@link #definition} (A reference to an [[[ElementDefinition]]] that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 315 */ 316 public UriType getDefinitionElement() { 317 if (this.definition == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.definition"); 320 else if (Configuration.doAutoCreate()) 321 this.definition = new UriType(); // bb 322 return this.definition; 323 } 324 325 public boolean hasDefinitionElement() { 326 return this.definition != null && !this.definition.isEmpty(); 327 } 328 329 public boolean hasDefinition() { 330 return this.definition != null && !this.definition.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #definition} (A reference to an [[[ElementDefinition]]] that provides the details for the item.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 335 */ 336 public QuestionnaireResponseItemComponent setDefinitionElement(UriType value) { 337 this.definition = value; 338 return this; 339 } 340 341 /** 342 * @return A reference to an [[[ElementDefinition]]] that provides the details for the item. 343 */ 344 public String getDefinition() { 345 return this.definition == null ? null : this.definition.getValue(); 346 } 347 348 /** 349 * @param value A reference to an [[[ElementDefinition]]] that provides the details for the item. 350 */ 351 public QuestionnaireResponseItemComponent setDefinition(String value) { 352 if (Utilities.noString(value)) 353 this.definition = null; 354 else { 355 if (this.definition == null) 356 this.definition = new UriType(); 357 this.definition.setValue(value); 358 } 359 return this; 360 } 361 362 /** 363 * @return {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 364 */ 365 public StringType getTextElement() { 366 if (this.text == null) 367 if (Configuration.errorOnAutoCreate()) 368 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.text"); 369 else if (Configuration.doAutoCreate()) 370 this.text = new StringType(); // bb 371 return this.text; 372 } 373 374 public boolean hasTextElement() { 375 return this.text != null && !this.text.isEmpty(); 376 } 377 378 public boolean hasText() { 379 return this.text != null && !this.text.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #text} (Text that is displayed above the contents of the group or as the text of the question being answered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 384 */ 385 public QuestionnaireResponseItemComponent setTextElement(StringType value) { 386 this.text = value; 387 return this; 388 } 389 390 /** 391 * @return Text that is displayed above the contents of the group or as the text of the question being answered. 392 */ 393 public String getText() { 394 return this.text == null ? null : this.text.getValue(); 395 } 396 397 /** 398 * @param value Text that is displayed above the contents of the group or as the text of the question being answered. 399 */ 400 public QuestionnaireResponseItemComponent setText(String value) { 401 if (Utilities.noString(value)) 402 this.text = null; 403 else { 404 if (this.text == null) 405 this.text = new StringType(); 406 this.text.setValue(value); 407 } 408 return this; 409 } 410 411 /** 412 * @return {@link #subject} (More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.) 413 */ 414 public Reference getSubject() { 415 if (this.subject == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.subject"); 418 else if (Configuration.doAutoCreate()) 419 this.subject = new Reference(); // cc 420 return this.subject; 421 } 422 423 public boolean hasSubject() { 424 return this.subject != null && !this.subject.isEmpty(); 425 } 426 427 /** 428 * @param value {@link #subject} (More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.) 429 */ 430 public QuestionnaireResponseItemComponent setSubject(Reference value) { 431 this.subject = value; 432 return this; 433 } 434 435 /** 436 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.) 437 */ 438 public Resource getSubjectTarget() { 439 return this.subjectTarget; 440 } 441 442 /** 443 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.) 444 */ 445 public QuestionnaireResponseItemComponent setSubjectTarget(Resource value) { 446 this.subjectTarget = value; 447 return this; 448 } 449 450 /** 451 * @return {@link #answer} (The respondent's answer(s) to the question.) 452 */ 453 public List<QuestionnaireResponseItemAnswerComponent> getAnswer() { 454 if (this.answer == null) 455 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 456 return this.answer; 457 } 458 459 /** 460 * @return Returns a reference to <code>this</code> for easy method chaining 461 */ 462 public QuestionnaireResponseItemComponent setAnswer(List<QuestionnaireResponseItemAnswerComponent> theAnswer) { 463 this.answer = theAnswer; 464 return this; 465 } 466 467 public boolean hasAnswer() { 468 if (this.answer == null) 469 return false; 470 for (QuestionnaireResponseItemAnswerComponent item : this.answer) 471 if (!item.isEmpty()) 472 return true; 473 return false; 474 } 475 476 public QuestionnaireResponseItemAnswerComponent addAnswer() { //3 477 QuestionnaireResponseItemAnswerComponent t = new QuestionnaireResponseItemAnswerComponent(); 478 if (this.answer == null) 479 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 480 this.answer.add(t); 481 return t; 482 } 483 484 public QuestionnaireResponseItemComponent addAnswer(QuestionnaireResponseItemAnswerComponent t) { //3 485 if (t == null) 486 return this; 487 if (this.answer == null) 488 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 489 this.answer.add(t); 490 return this; 491 } 492 493 /** 494 * @return The first repetition of repeating field {@link #answer}, creating it if it does not already exist 495 */ 496 public QuestionnaireResponseItemAnswerComponent getAnswerFirstRep() { 497 if (getAnswer().isEmpty()) { 498 addAnswer(); 499 } 500 return getAnswer().get(0); 501 } 502 503 /** 504 * @return {@link #item} (Questions or sub-groups nested beneath a question or group.) 505 */ 506 public List<QuestionnaireResponseItemComponent> getItem() { 507 if (this.item == null) 508 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 509 return this.item; 510 } 511 512 /** 513 * @return Returns a reference to <code>this</code> for easy method chaining 514 */ 515 public QuestionnaireResponseItemComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 516 this.item = theItem; 517 return this; 518 } 519 520 public boolean hasItem() { 521 if (this.item == null) 522 return false; 523 for (QuestionnaireResponseItemComponent item : this.item) 524 if (!item.isEmpty()) 525 return true; 526 return false; 527 } 528 529 public QuestionnaireResponseItemComponent addItem() { //3 530 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 531 if (this.item == null) 532 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 533 this.item.add(t); 534 return t; 535 } 536 537 public QuestionnaireResponseItemComponent addItem(QuestionnaireResponseItemComponent t) { //3 538 if (t == null) 539 return this; 540 if (this.item == null) 541 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 542 this.item.add(t); 543 return this; 544 } 545 546 /** 547 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 548 */ 549 public QuestionnaireResponseItemComponent getItemFirstRep() { 550 if (getItem().isEmpty()) { 551 addItem(); 552 } 553 return getItem().get(0); 554 } 555 556 protected void listChildren(List<Property> children) { 557 super.listChildren(children); 558 children.add(new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId)); 559 children.add(new Property("definition", "uri", "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition)); 560 children.add(new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text)); 561 children.add(new Property("subject", "Reference(Any)", "More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.", 0, 1, subject)); 562 children.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer)); 563 children.add(new Property("item", "@QuestionnaireResponse.item", "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item)); 564 } 565 566 @Override 567 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 568 switch (_hash) { 569 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, linkId); 570 case -1014418093: /*definition*/ return new Property("definition", "uri", "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition); 571 case 3556653: /*text*/ return new Property("text", "string", "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, text); 572 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.", 0, 1, subject); 573 case -1412808770: /*answer*/ return new Property("answer", "", "The respondent's answer(s) to the question.", 0, java.lang.Integer.MAX_VALUE, answer); 574 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item); 575 default: return super.getNamedProperty(_hash, _name, _checkValid); 576 } 577 578 } 579 580 @Override 581 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 582 switch (hash) { 583 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 584 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // UriType 585 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 586 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 587 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // QuestionnaireResponseItemAnswerComponent 588 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 589 default: return super.getProperty(hash, name, checkValid); 590 } 591 592 } 593 594 @Override 595 public Base setProperty(int hash, String name, Base value) throws FHIRException { 596 switch (hash) { 597 case -1102667083: // linkId 598 this.linkId = castToString(value); // StringType 599 return value; 600 case -1014418093: // definition 601 this.definition = castToUri(value); // UriType 602 return value; 603 case 3556653: // text 604 this.text = castToString(value); // StringType 605 return value; 606 case -1867885268: // subject 607 this.subject = castToReference(value); // Reference 608 return value; 609 case -1412808770: // answer 610 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); // QuestionnaireResponseItemAnswerComponent 611 return value; 612 case 3242771: // item 613 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 614 return value; 615 default: return super.setProperty(hash, name, value); 616 } 617 618 } 619 620 @Override 621 public Base setProperty(String name, Base value) throws FHIRException { 622 if (name.equals("linkId")) { 623 this.linkId = castToString(value); // StringType 624 } else if (name.equals("definition")) { 625 this.definition = castToUri(value); // UriType 626 } else if (name.equals("text")) { 627 this.text = castToString(value); // StringType 628 } else if (name.equals("subject")) { 629 this.subject = castToReference(value); // Reference 630 } else if (name.equals("answer")) { 631 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); 632 } else if (name.equals("item")) { 633 this.getItem().add((QuestionnaireResponseItemComponent) value); 634 } else 635 return super.setProperty(name, value); 636 return value; 637 } 638 639 @Override 640 public Base makeProperty(int hash, String name) throws FHIRException { 641 switch (hash) { 642 case -1102667083: return getLinkIdElement(); 643 case -1014418093: return getDefinitionElement(); 644 case 3556653: return getTextElement(); 645 case -1867885268: return getSubject(); 646 case -1412808770: return addAnswer(); 647 case 3242771: return addItem(); 648 default: return super.makeProperty(hash, name); 649 } 650 651 } 652 653 @Override 654 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 655 switch (hash) { 656 case -1102667083: /*linkId*/ return new String[] {"string"}; 657 case -1014418093: /*definition*/ return new String[] {"uri"}; 658 case 3556653: /*text*/ return new String[] {"string"}; 659 case -1867885268: /*subject*/ return new String[] {"Reference"}; 660 case -1412808770: /*answer*/ return new String[] {}; 661 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 662 default: return super.getTypesForProperty(hash, name); 663 } 664 665 } 666 667 @Override 668 public Base addChild(String name) throws FHIRException { 669 if (name.equals("linkId")) { 670 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.linkId"); 671 } 672 else if (name.equals("definition")) { 673 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.definition"); 674 } 675 else if (name.equals("text")) { 676 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.text"); 677 } 678 else if (name.equals("subject")) { 679 this.subject = new Reference(); 680 return this.subject; 681 } 682 else if (name.equals("answer")) { 683 return addAnswer(); 684 } 685 else if (name.equals("item")) { 686 return addItem(); 687 } 688 else 689 return super.addChild(name); 690 } 691 692 public QuestionnaireResponseItemComponent copy() { 693 QuestionnaireResponseItemComponent dst = new QuestionnaireResponseItemComponent(); 694 copyValues(dst); 695 dst.linkId = linkId == null ? null : linkId.copy(); 696 dst.definition = definition == null ? null : definition.copy(); 697 dst.text = text == null ? null : text.copy(); 698 dst.subject = subject == null ? null : subject.copy(); 699 if (answer != null) { 700 dst.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 701 for (QuestionnaireResponseItemAnswerComponent i : answer) 702 dst.answer.add(i.copy()); 703 }; 704 if (item != null) { 705 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 706 for (QuestionnaireResponseItemComponent i : item) 707 dst.item.add(i.copy()); 708 }; 709 return dst; 710 } 711 712 @Override 713 public boolean equalsDeep(Base other_) { 714 if (!super.equalsDeep(other_)) 715 return false; 716 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 717 return false; 718 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 719 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) && compareDeep(text, o.text, true) 720 && compareDeep(subject, o.subject, true) && compareDeep(answer, o.answer, true) && compareDeep(item, o.item, true) 721 ; 722 } 723 724 @Override 725 public boolean equalsShallow(Base other_) { 726 if (!super.equalsShallow(other_)) 727 return false; 728 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 729 return false; 730 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 731 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) && compareValues(text, o.text, true) 732 ; 733 } 734 735 public boolean isEmpty() { 736 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, text 737 , subject, answer, item); 738 } 739 740 public String fhirType() { 741 return "QuestionnaireResponse.item"; 742 743 } 744 745 } 746 747 @Block() 748 public static class QuestionnaireResponseItemAnswerComponent extends BackboneElement implements IBaseBackboneElement { 749 /** 750 * The answer (or one of the answers) provided by the respondent to the question. 751 */ 752 @Child(name = "value", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 753 @Description(shortDefinition="Single-valued answer to the question", formalDefinition="The answer (or one of the answers) provided by the respondent to the question." ) 754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers") 755 protected Type value; 756 757 /** 758 * Nested groups and/or questions found within this particular answer. 759 */ 760 @Child(name = "item", type = {QuestionnaireResponseItemComponent.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 761 @Description(shortDefinition="Nested groups and questions", formalDefinition="Nested groups and/or questions found within this particular answer." ) 762 protected List<QuestionnaireResponseItemComponent> item; 763 764 private static final long serialVersionUID = 2052422636L; 765 766 /** 767 * Constructor 768 */ 769 public QuestionnaireResponseItemAnswerComponent() { 770 super(); 771 } 772 773 /** 774 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 775 */ 776 public Type getValue() { 777 return this.value; 778 } 779 780 /** 781 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 782 */ 783 public BooleanType getValueBooleanType() throws FHIRException { 784 if (this.value == null) 785 return null; 786 if (!(this.value instanceof BooleanType)) 787 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 788 return (BooleanType) this.value; 789 } 790 791 public boolean hasValueBooleanType() { 792 return this != null && this.value instanceof BooleanType; 793 } 794 795 /** 796 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 797 */ 798 public DecimalType getValueDecimalType() throws FHIRException { 799 if (this.value == null) 800 return null; 801 if (!(this.value instanceof DecimalType)) 802 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 803 return (DecimalType) this.value; 804 } 805 806 public boolean hasValueDecimalType() { 807 return this != null && this.value instanceof DecimalType; 808 } 809 810 /** 811 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 812 */ 813 public IntegerType getValueIntegerType() throws FHIRException { 814 if (this.value == null) 815 return null; 816 if (!(this.value instanceof IntegerType)) 817 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 818 return (IntegerType) this.value; 819 } 820 821 public boolean hasValueIntegerType() { 822 return this != null && this.value instanceof IntegerType; 823 } 824 825 /** 826 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 827 */ 828 public DateType getValueDateType() throws FHIRException { 829 if (this.value == null) 830 return null; 831 if (!(this.value instanceof DateType)) 832 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 833 return (DateType) this.value; 834 } 835 836 public boolean hasValueDateType() { 837 return this != null && this.value instanceof DateType; 838 } 839 840 /** 841 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 842 */ 843 public DateTimeType getValueDateTimeType() throws FHIRException { 844 if (this.value == null) 845 return null; 846 if (!(this.value instanceof DateTimeType)) 847 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 848 return (DateTimeType) this.value; 849 } 850 851 public boolean hasValueDateTimeType() { 852 return this != null && this.value instanceof DateTimeType; 853 } 854 855 /** 856 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 857 */ 858 public TimeType getValueTimeType() throws FHIRException { 859 if (this.value == null) 860 return null; 861 if (!(this.value instanceof TimeType)) 862 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 863 return (TimeType) this.value; 864 } 865 866 public boolean hasValueTimeType() { 867 return this != null && this.value instanceof TimeType; 868 } 869 870 /** 871 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 872 */ 873 public StringType getValueStringType() throws FHIRException { 874 if (this.value == null) 875 return null; 876 if (!(this.value instanceof StringType)) 877 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 878 return (StringType) this.value; 879 } 880 881 public boolean hasValueStringType() { 882 return this != null && this.value instanceof StringType; 883 } 884 885 /** 886 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 887 */ 888 public UriType getValueUriType() throws FHIRException { 889 if (this.value == null) 890 return null; 891 if (!(this.value instanceof UriType)) 892 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 893 return (UriType) this.value; 894 } 895 896 public boolean hasValueUriType() { 897 return this != null && this.value instanceof UriType; 898 } 899 900 /** 901 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 902 */ 903 public Attachment getValueAttachment() throws FHIRException { 904 if (this.value == null) 905 return null; 906 if (!(this.value instanceof Attachment)) 907 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 908 return (Attachment) this.value; 909 } 910 911 public boolean hasValueAttachment() { 912 return this != null && this.value instanceof Attachment; 913 } 914 915 /** 916 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 917 */ 918 public Coding getValueCoding() throws FHIRException { 919 if (this.value == null) 920 return null; 921 if (!(this.value instanceof Coding)) 922 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 923 return (Coding) this.value; 924 } 925 926 public boolean hasValueCoding() { 927 return this != null && this.value instanceof Coding; 928 } 929 930 /** 931 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 932 */ 933 public Quantity getValueQuantity() throws FHIRException { 934 if (this.value == null) 935 return null; 936 if (!(this.value instanceof Quantity)) 937 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 938 return (Quantity) this.value; 939 } 940 941 public boolean hasValueQuantity() { 942 return this != null && this.value instanceof Quantity; 943 } 944 945 /** 946 * @return {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 947 */ 948 public Reference getValueReference() throws FHIRException { 949 if (this.value == null) 950 return null; 951 if (!(this.value instanceof Reference)) 952 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 953 return (Reference) this.value; 954 } 955 956 public boolean hasValueReference() { 957 return this != null && this.value instanceof Reference; 958 } 959 960 public boolean hasValue() { 961 return this.value != null && !this.value.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #value} (The answer (or one of the answers) provided by the respondent to the question.) 966 */ 967 public QuestionnaireResponseItemAnswerComponent setValue(Type value) throws FHIRFormatError { 968 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 969 throw new FHIRFormatError("Not the right type for QuestionnaireResponse.item.answer.value[x]: "+value.fhirType()); 970 this.value = value; 971 return this; 972 } 973 974 /** 975 * @return {@link #item} (Nested groups and/or questions found within this particular answer.) 976 */ 977 public List<QuestionnaireResponseItemComponent> getItem() { 978 if (this.item == null) 979 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 980 return this.item; 981 } 982 983 /** 984 * @return Returns a reference to <code>this</code> for easy method chaining 985 */ 986 public QuestionnaireResponseItemAnswerComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 987 this.item = theItem; 988 return this; 989 } 990 991 public boolean hasItem() { 992 if (this.item == null) 993 return false; 994 for (QuestionnaireResponseItemComponent item : this.item) 995 if (!item.isEmpty()) 996 return true; 997 return false; 998 } 999 1000 public QuestionnaireResponseItemComponent addItem() { //3 1001 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1002 if (this.item == null) 1003 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1004 this.item.add(t); 1005 return t; 1006 } 1007 1008 public QuestionnaireResponseItemAnswerComponent addItem(QuestionnaireResponseItemComponent t) { //3 1009 if (t == null) 1010 return this; 1011 if (this.item == null) 1012 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1013 this.item.add(t); 1014 return this; 1015 } 1016 1017 /** 1018 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 1019 */ 1020 public QuestionnaireResponseItemComponent getItemFirstRep() { 1021 if (getItem().isEmpty()) { 1022 addItem(); 1023 } 1024 return getItem().get(0); 1025 } 1026 1027 protected void listChildren(List<Property> children) { 1028 super.listChildren(children); 1029 children.add(new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value)); 1030 children.add(new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item)); 1031 } 1032 1033 @Override 1034 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1035 switch (_hash) { 1036 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1037 case 111972721: /*value*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1038 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1039 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1040 case -1668204915: /*valueInteger*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1041 case -766192449: /*valueDate*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1042 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1043 case -765708322: /*valueTime*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1044 case -1424603934: /*valueString*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1045 case -1410172357: /*valueUri*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1046 case -475566732: /*valueAttachment*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1047 case -1887705029: /*valueCoding*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1048 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1049 case 1755241690: /*valueReference*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1050 case 3242771: /*item*/ return new Property("item", "@QuestionnaireResponse.item", "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item); 1051 default: return super.getNamedProperty(_hash, _name, _checkValid); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1058 switch (hash) { 1059 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1060 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1061 default: return super.getProperty(hash, name, checkValid); 1062 } 1063 1064 } 1065 1066 @Override 1067 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1068 switch (hash) { 1069 case 111972721: // value 1070 this.value = castToType(value); // Type 1071 return value; 1072 case 3242771: // item 1073 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1074 return value; 1075 default: return super.setProperty(hash, name, value); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base setProperty(String name, Base value) throws FHIRException { 1082 if (name.equals("value[x]")) { 1083 this.value = castToType(value); // Type 1084 } else if (name.equals("item")) { 1085 this.getItem().add((QuestionnaireResponseItemComponent) value); 1086 } else 1087 return super.setProperty(name, value); 1088 return value; 1089 } 1090 1091 @Override 1092 public Base makeProperty(int hash, String name) throws FHIRException { 1093 switch (hash) { 1094 case -1410166417: return getValue(); 1095 case 111972721: return getValue(); 1096 case 3242771: return addItem(); 1097 default: return super.makeProperty(hash, name); 1098 } 1099 1100 } 1101 1102 @Override 1103 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1104 switch (hash) { 1105 case 111972721: /*value*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 1106 case 3242771: /*item*/ return new String[] {"@QuestionnaireResponse.item"}; 1107 default: return super.getTypesForProperty(hash, name); 1108 } 1109 1110 } 1111 1112 @Override 1113 public Base addChild(String name) throws FHIRException { 1114 if (name.equals("valueBoolean")) { 1115 this.value = new BooleanType(); 1116 return this.value; 1117 } 1118 else if (name.equals("valueDecimal")) { 1119 this.value = new DecimalType(); 1120 return this.value; 1121 } 1122 else if (name.equals("valueInteger")) { 1123 this.value = new IntegerType(); 1124 return this.value; 1125 } 1126 else if (name.equals("valueDate")) { 1127 this.value = new DateType(); 1128 return this.value; 1129 } 1130 else if (name.equals("valueDateTime")) { 1131 this.value = new DateTimeType(); 1132 return this.value; 1133 } 1134 else if (name.equals("valueTime")) { 1135 this.value = new TimeType(); 1136 return this.value; 1137 } 1138 else if (name.equals("valueString")) { 1139 this.value = new StringType(); 1140 return this.value; 1141 } 1142 else if (name.equals("valueUri")) { 1143 this.value = new UriType(); 1144 return this.value; 1145 } 1146 else if (name.equals("valueAttachment")) { 1147 this.value = new Attachment(); 1148 return this.value; 1149 } 1150 else if (name.equals("valueCoding")) { 1151 this.value = new Coding(); 1152 return this.value; 1153 } 1154 else if (name.equals("valueQuantity")) { 1155 this.value = new Quantity(); 1156 return this.value; 1157 } 1158 else if (name.equals("valueReference")) { 1159 this.value = new Reference(); 1160 return this.value; 1161 } 1162 else if (name.equals("item")) { 1163 return addItem(); 1164 } 1165 else 1166 return super.addChild(name); 1167 } 1168 1169 public QuestionnaireResponseItemAnswerComponent copy() { 1170 QuestionnaireResponseItemAnswerComponent dst = new QuestionnaireResponseItemAnswerComponent(); 1171 copyValues(dst); 1172 dst.value = value == null ? null : value.copy(); 1173 if (item != null) { 1174 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1175 for (QuestionnaireResponseItemComponent i : item) 1176 dst.item.add(i.copy()); 1177 }; 1178 return dst; 1179 } 1180 1181 @Override 1182 public boolean equalsDeep(Base other_) { 1183 if (!super.equalsDeep(other_)) 1184 return false; 1185 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1186 return false; 1187 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1188 return compareDeep(value, o.value, true) && compareDeep(item, o.item, true); 1189 } 1190 1191 @Override 1192 public boolean equalsShallow(Base other_) { 1193 if (!super.equalsShallow(other_)) 1194 return false; 1195 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1196 return false; 1197 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1198 return true; 1199 } 1200 1201 public boolean isEmpty() { 1202 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, item); 1203 } 1204 1205 public String fhirType() { 1206 return "QuestionnaireResponse.item.answer"; 1207 1208 } 1209 1210 } 1211 1212 /** 1213 * A business identifier assigned to a particular completed (or partially completed) questionnaire. 1214 */ 1215 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1216 @Description(shortDefinition="Unique id for this set of answers", formalDefinition="A business identifier assigned to a particular completed (or partially completed) questionnaire." ) 1217 protected Identifier identifier; 1218 1219 /** 1220 * The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression. 1221 */ 1222 @Child(name = "basedOn", type = {ReferralRequest.class, CarePlan.class, ProcedureRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1223 @Description(shortDefinition="Request fulfilled by this QuestionnaireResponse", formalDefinition="The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression." ) 1224 protected List<Reference> basedOn; 1225 /** 1226 * The actual objects that are the target of the reference (The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.) 1227 */ 1228 protected List<Resource> basedOnTarget; 1229 1230 1231 /** 1232 * A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of. 1233 */ 1234 @Child(name = "parent", type = {Observation.class, Procedure.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1235 @Description(shortDefinition="Part of this action", formalDefinition="A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of." ) 1236 protected List<Reference> parent; 1237 /** 1238 * The actual objects that are the target of the reference (A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.) 1239 */ 1240 protected List<Resource> parentTarget; 1241 1242 1243 /** 1244 * The Questionnaire that defines and organizes the questions for which answers are being provided. 1245 */ 1246 @Child(name = "questionnaire", type = {Questionnaire.class}, order=3, min=0, max=1, modifier=false, summary=true) 1247 @Description(shortDefinition="Form being answered", formalDefinition="The Questionnaire that defines and organizes the questions for which answers are being provided." ) 1248 protected Reference questionnaire; 1249 1250 /** 1251 * The actual object that is the target of the reference (The Questionnaire that defines and organizes the questions for which answers are being provided.) 1252 */ 1253 protected Questionnaire questionnaireTarget; 1254 1255 /** 1256 * The position of the questionnaire response within its overall lifecycle. 1257 */ 1258 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 1259 @Description(shortDefinition="in-progress | completed | amended | entered-in-error | stopped", formalDefinition="The position of the questionnaire response within its overall lifecycle." ) 1260 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/questionnaire-answers-status") 1261 protected Enumeration<QuestionnaireResponseStatus> status; 1262 1263 /** 1264 * The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information. 1265 */ 1266 @Child(name = "subject", type = {Reference.class}, order=5, min=0, max=1, modifier=false, summary=true) 1267 @Description(shortDefinition="The subject of the questions", formalDefinition="The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information." ) 1268 protected Reference subject; 1269 1270 /** 1271 * The actual object that is the target of the reference (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1272 */ 1273 protected Resource subjectTarget; 1274 1275 /** 1276 * The encounter or episode of care with primary association to the questionnaire response. 1277 */ 1278 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 1279 @Description(shortDefinition="Encounter or Episode during which questionnaire was completed", formalDefinition="The encounter or episode of care with primary association to the questionnaire response." ) 1280 protected Reference context; 1281 1282 /** 1283 * The actual object that is the target of the reference (The encounter or episode of care with primary association to the questionnaire response.) 1284 */ 1285 protected Resource contextTarget; 1286 1287 /** 1288 * The date and/or time that this set of answers were last changed. 1289 */ 1290 @Child(name = "authored", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1291 @Description(shortDefinition="Date the answers were gathered", formalDefinition="The date and/or time that this set of answers were last changed." ) 1292 protected DateTimeType authored; 1293 1294 /** 1295 * Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system. 1296 */ 1297 @Child(name = "author", type = {Device.class, Practitioner.class, Patient.class, RelatedPerson.class}, order=8, min=0, max=1, modifier=false, summary=true) 1298 @Description(shortDefinition="Person who received and recorded the answers", formalDefinition="Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system." ) 1299 protected Reference author; 1300 1301 /** 1302 * The actual object that is the target of the reference (Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1303 */ 1304 protected Resource authorTarget; 1305 1306 /** 1307 * The person who answered the questions about the subject. 1308 */ 1309 @Child(name = "source", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=true) 1310 @Description(shortDefinition="The person who answered the questions", formalDefinition="The person who answered the questions about the subject." ) 1311 protected Reference source; 1312 1313 /** 1314 * The actual object that is the target of the reference (The person who answered the questions about the subject.) 1315 */ 1316 protected Resource sourceTarget; 1317 1318 /** 1319 * A group or question item from the original questionnaire for which answers are provided. 1320 */ 1321 @Child(name = "item", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1322 @Description(shortDefinition="Groups and questions", formalDefinition="A group or question item from the original questionnaire for which answers are provided." ) 1323 protected List<QuestionnaireResponseItemComponent> item; 1324 1325 private static final long serialVersionUID = -1559552776L; 1326 1327 /** 1328 * Constructor 1329 */ 1330 public QuestionnaireResponse() { 1331 super(); 1332 } 1333 1334 /** 1335 * Constructor 1336 */ 1337 public QuestionnaireResponse(Enumeration<QuestionnaireResponseStatus> status) { 1338 super(); 1339 this.status = status; 1340 } 1341 1342 /** 1343 * @return {@link #identifier} (A business identifier assigned to a particular completed (or partially completed) questionnaire.) 1344 */ 1345 public Identifier getIdentifier() { 1346 if (this.identifier == null) 1347 if (Configuration.errorOnAutoCreate()) 1348 throw new Error("Attempt to auto-create QuestionnaireResponse.identifier"); 1349 else if (Configuration.doAutoCreate()) 1350 this.identifier = new Identifier(); // cc 1351 return this.identifier; 1352 } 1353 1354 public boolean hasIdentifier() { 1355 return this.identifier != null && !this.identifier.isEmpty(); 1356 } 1357 1358 /** 1359 * @param value {@link #identifier} (A business identifier assigned to a particular completed (or partially completed) questionnaire.) 1360 */ 1361 public QuestionnaireResponse setIdentifier(Identifier value) { 1362 this.identifier = value; 1363 return this; 1364 } 1365 1366 /** 1367 * @return {@link #basedOn} (The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.) 1368 */ 1369 public List<Reference> getBasedOn() { 1370 if (this.basedOn == null) 1371 this.basedOn = new ArrayList<Reference>(); 1372 return this.basedOn; 1373 } 1374 1375 /** 1376 * @return Returns a reference to <code>this</code> for easy method chaining 1377 */ 1378 public QuestionnaireResponse setBasedOn(List<Reference> theBasedOn) { 1379 this.basedOn = theBasedOn; 1380 return this; 1381 } 1382 1383 public boolean hasBasedOn() { 1384 if (this.basedOn == null) 1385 return false; 1386 for (Reference item : this.basedOn) 1387 if (!item.isEmpty()) 1388 return true; 1389 return false; 1390 } 1391 1392 public Reference addBasedOn() { //3 1393 Reference t = new Reference(); 1394 if (this.basedOn == null) 1395 this.basedOn = new ArrayList<Reference>(); 1396 this.basedOn.add(t); 1397 return t; 1398 } 1399 1400 public QuestionnaireResponse addBasedOn(Reference t) { //3 1401 if (t == null) 1402 return this; 1403 if (this.basedOn == null) 1404 this.basedOn = new ArrayList<Reference>(); 1405 this.basedOn.add(t); 1406 return this; 1407 } 1408 1409 /** 1410 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1411 */ 1412 public Reference getBasedOnFirstRep() { 1413 if (getBasedOn().isEmpty()) { 1414 addBasedOn(); 1415 } 1416 return getBasedOn().get(0); 1417 } 1418 1419 /** 1420 * @deprecated Use Reference#setResource(IBaseResource) instead 1421 */ 1422 @Deprecated 1423 public List<Resource> getBasedOnTarget() { 1424 if (this.basedOnTarget == null) 1425 this.basedOnTarget = new ArrayList<Resource>(); 1426 return this.basedOnTarget; 1427 } 1428 1429 /** 1430 * @return {@link #parent} (A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.) 1431 */ 1432 public List<Reference> getParent() { 1433 if (this.parent == null) 1434 this.parent = new ArrayList<Reference>(); 1435 return this.parent; 1436 } 1437 1438 /** 1439 * @return Returns a reference to <code>this</code> for easy method chaining 1440 */ 1441 public QuestionnaireResponse setParent(List<Reference> theParent) { 1442 this.parent = theParent; 1443 return this; 1444 } 1445 1446 public boolean hasParent() { 1447 if (this.parent == null) 1448 return false; 1449 for (Reference item : this.parent) 1450 if (!item.isEmpty()) 1451 return true; 1452 return false; 1453 } 1454 1455 public Reference addParent() { //3 1456 Reference t = new Reference(); 1457 if (this.parent == null) 1458 this.parent = new ArrayList<Reference>(); 1459 this.parent.add(t); 1460 return t; 1461 } 1462 1463 public QuestionnaireResponse addParent(Reference t) { //3 1464 if (t == null) 1465 return this; 1466 if (this.parent == null) 1467 this.parent = new ArrayList<Reference>(); 1468 this.parent.add(t); 1469 return this; 1470 } 1471 1472 /** 1473 * @return The first repetition of repeating field {@link #parent}, creating it if it does not already exist 1474 */ 1475 public Reference getParentFirstRep() { 1476 if (getParent().isEmpty()) { 1477 addParent(); 1478 } 1479 return getParent().get(0); 1480 } 1481 1482 /** 1483 * @deprecated Use Reference#setResource(IBaseResource) instead 1484 */ 1485 @Deprecated 1486 public List<Resource> getParentTarget() { 1487 if (this.parentTarget == null) 1488 this.parentTarget = new ArrayList<Resource>(); 1489 return this.parentTarget; 1490 } 1491 1492 /** 1493 * @return {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.) 1494 */ 1495 public Reference getQuestionnaire() { 1496 if (this.questionnaire == null) 1497 if (Configuration.errorOnAutoCreate()) 1498 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1499 else if (Configuration.doAutoCreate()) 1500 this.questionnaire = new Reference(); // cc 1501 return this.questionnaire; 1502 } 1503 1504 public boolean hasQuestionnaire() { 1505 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1506 } 1507 1508 /** 1509 * @param value {@link #questionnaire} (The Questionnaire that defines and organizes the questions for which answers are being provided.) 1510 */ 1511 public QuestionnaireResponse setQuestionnaire(Reference value) { 1512 this.questionnaire = value; 1513 return this; 1514 } 1515 1516 /** 1517 * @return {@link #questionnaire} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Questionnaire that defines and organizes the questions for which answers are being provided.) 1518 */ 1519 public Questionnaire getQuestionnaireTarget() { 1520 if (this.questionnaireTarget == null) 1521 if (Configuration.errorOnAutoCreate()) 1522 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1523 else if (Configuration.doAutoCreate()) 1524 this.questionnaireTarget = new Questionnaire(); // aa 1525 return this.questionnaireTarget; 1526 } 1527 1528 /** 1529 * @param value {@link #questionnaire} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Questionnaire that defines and organizes the questions for which answers are being provided.) 1530 */ 1531 public QuestionnaireResponse setQuestionnaireTarget(Questionnaire value) { 1532 this.questionnaireTarget = value; 1533 return this; 1534 } 1535 1536 /** 1537 * @return {@link #status} (The position of the questionnaire response within its overall lifecycle.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1538 */ 1539 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1540 if (this.status == null) 1541 if (Configuration.errorOnAutoCreate()) 1542 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1543 else if (Configuration.doAutoCreate()) 1544 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1545 return this.status; 1546 } 1547 1548 public boolean hasStatusElement() { 1549 return this.status != null && !this.status.isEmpty(); 1550 } 1551 1552 public boolean hasStatus() { 1553 return this.status != null && !this.status.isEmpty(); 1554 } 1555 1556 /** 1557 * @param value {@link #status} (The position of the questionnaire response within its overall lifecycle.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1558 */ 1559 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1560 this.status = value; 1561 return this; 1562 } 1563 1564 /** 1565 * @return The position of the questionnaire response within its overall lifecycle. 1566 */ 1567 public QuestionnaireResponseStatus getStatus() { 1568 return this.status == null ? null : this.status.getValue(); 1569 } 1570 1571 /** 1572 * @param value The position of the questionnaire response within its overall lifecycle. 1573 */ 1574 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1575 if (this.status == null) 1576 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1577 this.status.setValue(value); 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1583 */ 1584 public Reference getSubject() { 1585 if (this.subject == null) 1586 if (Configuration.errorOnAutoCreate()) 1587 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1588 else if (Configuration.doAutoCreate()) 1589 this.subject = new Reference(); // cc 1590 return this.subject; 1591 } 1592 1593 public boolean hasSubject() { 1594 return this.subject != null && !this.subject.isEmpty(); 1595 } 1596 1597 /** 1598 * @param value {@link #subject} (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1599 */ 1600 public QuestionnaireResponse setSubject(Reference value) { 1601 this.subject = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1607 */ 1608 public Resource getSubjectTarget() { 1609 return this.subjectTarget; 1610 } 1611 1612 /** 1613 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.) 1614 */ 1615 public QuestionnaireResponse setSubjectTarget(Resource value) { 1616 this.subjectTarget = value; 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #context} (The encounter or episode of care with primary association to the questionnaire response.) 1622 */ 1623 public Reference getContext() { 1624 if (this.context == null) 1625 if (Configuration.errorOnAutoCreate()) 1626 throw new Error("Attempt to auto-create QuestionnaireResponse.context"); 1627 else if (Configuration.doAutoCreate()) 1628 this.context = new Reference(); // cc 1629 return this.context; 1630 } 1631 1632 public boolean hasContext() { 1633 return this.context != null && !this.context.isEmpty(); 1634 } 1635 1636 /** 1637 * @param value {@link #context} (The encounter or episode of care with primary association to the questionnaire response.) 1638 */ 1639 public QuestionnaireResponse setContext(Reference value) { 1640 this.context = value; 1641 return this; 1642 } 1643 1644 /** 1645 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care with primary association to the questionnaire response.) 1646 */ 1647 public Resource getContextTarget() { 1648 return this.contextTarget; 1649 } 1650 1651 /** 1652 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care with primary association to the questionnaire response.) 1653 */ 1654 public QuestionnaireResponse setContextTarget(Resource value) { 1655 this.contextTarget = value; 1656 return this; 1657 } 1658 1659 /** 1660 * @return {@link #authored} (The date and/or time that this set of answers were last changed.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1661 */ 1662 public DateTimeType getAuthoredElement() { 1663 if (this.authored == null) 1664 if (Configuration.errorOnAutoCreate()) 1665 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1666 else if (Configuration.doAutoCreate()) 1667 this.authored = new DateTimeType(); // bb 1668 return this.authored; 1669 } 1670 1671 public boolean hasAuthoredElement() { 1672 return this.authored != null && !this.authored.isEmpty(); 1673 } 1674 1675 public boolean hasAuthored() { 1676 return this.authored != null && !this.authored.isEmpty(); 1677 } 1678 1679 /** 1680 * @param value {@link #authored} (The date and/or time that this set of answers were last changed.). This is the underlying object with id, value and extensions. The accessor "getAuthored" gives direct access to the value 1681 */ 1682 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1683 this.authored = value; 1684 return this; 1685 } 1686 1687 /** 1688 * @return The date and/or time that this set of answers were last changed. 1689 */ 1690 public Date getAuthored() { 1691 return this.authored == null ? null : this.authored.getValue(); 1692 } 1693 1694 /** 1695 * @param value The date and/or time that this set of answers were last changed. 1696 */ 1697 public QuestionnaireResponse setAuthored(Date value) { 1698 if (value == null) 1699 this.authored = null; 1700 else { 1701 if (this.authored == null) 1702 this.authored = new DateTimeType(); 1703 this.authored.setValue(value); 1704 } 1705 return this; 1706 } 1707 1708 /** 1709 * @return {@link #author} (Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1710 */ 1711 public Reference getAuthor() { 1712 if (this.author == null) 1713 if (Configuration.errorOnAutoCreate()) 1714 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1715 else if (Configuration.doAutoCreate()) 1716 this.author = new Reference(); // cc 1717 return this.author; 1718 } 1719 1720 public boolean hasAuthor() { 1721 return this.author != null && !this.author.isEmpty(); 1722 } 1723 1724 /** 1725 * @param value {@link #author} (Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1726 */ 1727 public QuestionnaireResponse setAuthor(Reference value) { 1728 this.author = value; 1729 return this; 1730 } 1731 1732 /** 1733 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1734 */ 1735 public Resource getAuthorTarget() { 1736 return this.authorTarget; 1737 } 1738 1739 /** 1740 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.) 1741 */ 1742 public QuestionnaireResponse setAuthorTarget(Resource value) { 1743 this.authorTarget = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return {@link #source} (The person who answered the questions about the subject.) 1749 */ 1750 public Reference getSource() { 1751 if (this.source == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 1754 else if (Configuration.doAutoCreate()) 1755 this.source = new Reference(); // cc 1756 return this.source; 1757 } 1758 1759 public boolean hasSource() { 1760 return this.source != null && !this.source.isEmpty(); 1761 } 1762 1763 /** 1764 * @param value {@link #source} (The person who answered the questions about the subject.) 1765 */ 1766 public QuestionnaireResponse setSource(Reference value) { 1767 this.source = value; 1768 return this; 1769 } 1770 1771 /** 1772 * @return {@link #source} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who answered the questions about the subject.) 1773 */ 1774 public Resource getSourceTarget() { 1775 return this.sourceTarget; 1776 } 1777 1778 /** 1779 * @param value {@link #source} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who answered the questions about the subject.) 1780 */ 1781 public QuestionnaireResponse setSourceTarget(Resource value) { 1782 this.sourceTarget = value; 1783 return this; 1784 } 1785 1786 /** 1787 * @return {@link #item} (A group or question item from the original questionnaire for which answers are provided.) 1788 */ 1789 public List<QuestionnaireResponseItemComponent> getItem() { 1790 if (this.item == null) 1791 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1792 return this.item; 1793 } 1794 1795 /** 1796 * @return Returns a reference to <code>this</code> for easy method chaining 1797 */ 1798 public QuestionnaireResponse setItem(List<QuestionnaireResponseItemComponent> theItem) { 1799 this.item = theItem; 1800 return this; 1801 } 1802 1803 public boolean hasItem() { 1804 if (this.item == null) 1805 return false; 1806 for (QuestionnaireResponseItemComponent item : this.item) 1807 if (!item.isEmpty()) 1808 return true; 1809 return false; 1810 } 1811 1812 public QuestionnaireResponseItemComponent addItem() { //3 1813 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1814 if (this.item == null) 1815 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1816 this.item.add(t); 1817 return t; 1818 } 1819 1820 public QuestionnaireResponse addItem(QuestionnaireResponseItemComponent t) { //3 1821 if (t == null) 1822 return this; 1823 if (this.item == null) 1824 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1825 this.item.add(t); 1826 return this; 1827 } 1828 1829 /** 1830 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 1831 */ 1832 public QuestionnaireResponseItemComponent getItemFirstRep() { 1833 if (getItem().isEmpty()) { 1834 addItem(); 1835 } 1836 return getItem().get(0); 1837 } 1838 1839 protected void listChildren(List<Property> children) { 1840 super.listChildren(children); 1841 children.add(new Property("identifier", "Identifier", "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, identifier)); 1842 children.add(new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1843 children.add(new Property("parent", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, parent)); 1844 children.add(new Property("questionnaire", "Reference(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire)); 1845 children.add(new Property("status", "code", "The position of the questionnaire response within its overall lifecycle.", 0, 1, status)); 1846 children.add(new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject)); 1847 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care with primary association to the questionnaire response.", 0, 1, context)); 1848 children.add(new Property("authored", "dateTime", "The date and/or time that this set of answers were last changed.", 0, 1, authored)); 1849 children.add(new Property("author", "Reference(Device|Practitioner|Patient|RelatedPerson)", "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author)); 1850 children.add(new Property("source", "Reference(Patient|Practitioner|RelatedPerson)", "The person who answered the questions about the subject.", 0, 1, source)); 1851 children.add(new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item)); 1852 } 1853 1854 @Override 1855 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1856 switch (_hash) { 1857 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, identifier); 1858 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ProcedureRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1859 case -995424086: /*parent*/ return new Property("parent", "Reference(Observation|Procedure)", "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 0, java.lang.Integer.MAX_VALUE, parent); 1860 case -1017049693: /*questionnaire*/ return new Property("questionnaire", "Reference(Questionnaire)", "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, questionnaire); 1861 case -892481550: /*status*/ return new Property("status", "code", "The position of the questionnaire response within its overall lifecycle.", 0, 1, status); 1862 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 0, 1, subject); 1863 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care with primary association to the questionnaire response.", 0, 1, context); 1864 case 1433073514: /*authored*/ return new Property("authored", "dateTime", "The date and/or time that this set of answers were last changed.", 0, 1, authored); 1865 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner|Patient|RelatedPerson)", "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 0, 1, author); 1866 case -896505829: /*source*/ return new Property("source", "Reference(Patient|Practitioner|RelatedPerson)", "The person who answered the questions about the subject.", 0, 1, source); 1867 case 3242771: /*item*/ return new Property("item", "", "A group or question item from the original questionnaire for which answers are provided.", 0, java.lang.Integer.MAX_VALUE, item); 1868 default: return super.getNamedProperty(_hash, _name, _checkValid); 1869 } 1870 1871 } 1872 1873 @Override 1874 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1875 switch (hash) { 1876 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1877 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1878 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 1879 case -1017049693: /*questionnaire*/ return this.questionnaire == null ? new Base[0] : new Base[] {this.questionnaire}; // Reference 1880 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<QuestionnaireResponseStatus> 1881 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1882 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1883 case 1433073514: /*authored*/ return this.authored == null ? new Base[0] : new Base[] {this.authored}; // DateTimeType 1884 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1885 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1886 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1887 default: return super.getProperty(hash, name, checkValid); 1888 } 1889 1890 } 1891 1892 @Override 1893 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1894 switch (hash) { 1895 case -1618432855: // identifier 1896 this.identifier = castToIdentifier(value); // Identifier 1897 return value; 1898 case -332612366: // basedOn 1899 this.getBasedOn().add(castToReference(value)); // Reference 1900 return value; 1901 case -995424086: // parent 1902 this.getParent().add(castToReference(value)); // Reference 1903 return value; 1904 case -1017049693: // questionnaire 1905 this.questionnaire = castToReference(value); // Reference 1906 return value; 1907 case -892481550: // status 1908 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 1909 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1910 return value; 1911 case -1867885268: // subject 1912 this.subject = castToReference(value); // Reference 1913 return value; 1914 case 951530927: // context 1915 this.context = castToReference(value); // Reference 1916 return value; 1917 case 1433073514: // authored 1918 this.authored = castToDateTime(value); // DateTimeType 1919 return value; 1920 case -1406328437: // author 1921 this.author = castToReference(value); // Reference 1922 return value; 1923 case -896505829: // source 1924 this.source = castToReference(value); // Reference 1925 return value; 1926 case 3242771: // item 1927 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1928 return value; 1929 default: return super.setProperty(hash, name, value); 1930 } 1931 1932 } 1933 1934 @Override 1935 public Base setProperty(String name, Base value) throws FHIRException { 1936 if (name.equals("identifier")) { 1937 this.identifier = castToIdentifier(value); // Identifier 1938 } else if (name.equals("basedOn")) { 1939 this.getBasedOn().add(castToReference(value)); 1940 } else if (name.equals("parent")) { 1941 this.getParent().add(castToReference(value)); 1942 } else if (name.equals("questionnaire")) { 1943 this.questionnaire = castToReference(value); // Reference 1944 } else if (name.equals("status")) { 1945 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 1946 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 1947 } else if (name.equals("subject")) { 1948 this.subject = castToReference(value); // Reference 1949 } else if (name.equals("context")) { 1950 this.context = castToReference(value); // Reference 1951 } else if (name.equals("authored")) { 1952 this.authored = castToDateTime(value); // DateTimeType 1953 } else if (name.equals("author")) { 1954 this.author = castToReference(value); // Reference 1955 } else if (name.equals("source")) { 1956 this.source = castToReference(value); // Reference 1957 } else if (name.equals("item")) { 1958 this.getItem().add((QuestionnaireResponseItemComponent) value); 1959 } else 1960 return super.setProperty(name, value); 1961 return value; 1962 } 1963 1964 @Override 1965 public Base makeProperty(int hash, String name) throws FHIRException { 1966 switch (hash) { 1967 case -1618432855: return getIdentifier(); 1968 case -332612366: return addBasedOn(); 1969 case -995424086: return addParent(); 1970 case -1017049693: return getQuestionnaire(); 1971 case -892481550: return getStatusElement(); 1972 case -1867885268: return getSubject(); 1973 case 951530927: return getContext(); 1974 case 1433073514: return getAuthoredElement(); 1975 case -1406328437: return getAuthor(); 1976 case -896505829: return getSource(); 1977 case 3242771: return addItem(); 1978 default: return super.makeProperty(hash, name); 1979 } 1980 1981 } 1982 1983 @Override 1984 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1985 switch (hash) { 1986 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1987 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1988 case -995424086: /*parent*/ return new String[] {"Reference"}; 1989 case -1017049693: /*questionnaire*/ return new String[] {"Reference"}; 1990 case -892481550: /*status*/ return new String[] {"code"}; 1991 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1992 case 951530927: /*context*/ return new String[] {"Reference"}; 1993 case 1433073514: /*authored*/ return new String[] {"dateTime"}; 1994 case -1406328437: /*author*/ return new String[] {"Reference"}; 1995 case -896505829: /*source*/ return new String[] {"Reference"}; 1996 case 3242771: /*item*/ return new String[] {}; 1997 default: return super.getTypesForProperty(hash, name); 1998 } 1999 2000 } 2001 2002 @Override 2003 public Base addChild(String name) throws FHIRException { 2004 if (name.equals("identifier")) { 2005 this.identifier = new Identifier(); 2006 return this.identifier; 2007 } 2008 else if (name.equals("basedOn")) { 2009 return addBasedOn(); 2010 } 2011 else if (name.equals("parent")) { 2012 return addParent(); 2013 } 2014 else if (name.equals("questionnaire")) { 2015 this.questionnaire = new Reference(); 2016 return this.questionnaire; 2017 } 2018 else if (name.equals("status")) { 2019 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 2020 } 2021 else if (name.equals("subject")) { 2022 this.subject = new Reference(); 2023 return this.subject; 2024 } 2025 else if (name.equals("context")) { 2026 this.context = new Reference(); 2027 return this.context; 2028 } 2029 else if (name.equals("authored")) { 2030 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 2031 } 2032 else if (name.equals("author")) { 2033 this.author = new Reference(); 2034 return this.author; 2035 } 2036 else if (name.equals("source")) { 2037 this.source = new Reference(); 2038 return this.source; 2039 } 2040 else if (name.equals("item")) { 2041 return addItem(); 2042 } 2043 else 2044 return super.addChild(name); 2045 } 2046 2047 public String fhirType() { 2048 return "QuestionnaireResponse"; 2049 2050 } 2051 2052 public QuestionnaireResponse copy() { 2053 QuestionnaireResponse dst = new QuestionnaireResponse(); 2054 copyValues(dst); 2055 dst.identifier = identifier == null ? null : identifier.copy(); 2056 if (basedOn != null) { 2057 dst.basedOn = new ArrayList<Reference>(); 2058 for (Reference i : basedOn) 2059 dst.basedOn.add(i.copy()); 2060 }; 2061 if (parent != null) { 2062 dst.parent = new ArrayList<Reference>(); 2063 for (Reference i : parent) 2064 dst.parent.add(i.copy()); 2065 }; 2066 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 2067 dst.status = status == null ? null : status.copy(); 2068 dst.subject = subject == null ? null : subject.copy(); 2069 dst.context = context == null ? null : context.copy(); 2070 dst.authored = authored == null ? null : authored.copy(); 2071 dst.author = author == null ? null : author.copy(); 2072 dst.source = source == null ? null : source.copy(); 2073 if (item != null) { 2074 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2075 for (QuestionnaireResponseItemComponent i : item) 2076 dst.item.add(i.copy()); 2077 }; 2078 return dst; 2079 } 2080 2081 protected QuestionnaireResponse typedCopy() { 2082 return copy(); 2083 } 2084 2085 @Override 2086 public boolean equalsDeep(Base other_) { 2087 if (!super.equalsDeep(other_)) 2088 return false; 2089 if (!(other_ instanceof QuestionnaireResponse)) 2090 return false; 2091 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2092 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(parent, o.parent, true) 2093 && compareDeep(questionnaire, o.questionnaire, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 2094 && compareDeep(context, o.context, true) && compareDeep(authored, o.authored, true) && compareDeep(author, o.author, true) 2095 && compareDeep(source, o.source, true) && compareDeep(item, o.item, true); 2096 } 2097 2098 @Override 2099 public boolean equalsShallow(Base other_) { 2100 if (!super.equalsShallow(other_)) 2101 return false; 2102 if (!(other_ instanceof QuestionnaireResponse)) 2103 return false; 2104 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2105 return compareValues(status, o.status, true) && compareValues(authored, o.authored, true); 2106 } 2107 2108 public boolean isEmpty() { 2109 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, parent 2110 , questionnaire, status, subject, context, authored, author, source, item); 2111 } 2112 2113 @Override 2114 public ResourceType getResourceType() { 2115 return ResourceType.QuestionnaireResponse; 2116 } 2117 2118 /** 2119 * Search parameter: <b>authored</b> 2120 * <p> 2121 * Description: <b>When the questionnaire response was last changed</b><br> 2122 * Type: <b>date</b><br> 2123 * Path: <b>QuestionnaireResponse.authored</b><br> 2124 * </p> 2125 */ 2126 @SearchParamDefinition(name="authored", path="QuestionnaireResponse.authored", description="When the questionnaire response was last changed", type="date" ) 2127 public static final String SP_AUTHORED = "authored"; 2128 /** 2129 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2130 * <p> 2131 * Description: <b>When the questionnaire response was last changed</b><br> 2132 * Type: <b>date</b><br> 2133 * Path: <b>QuestionnaireResponse.authored</b><br> 2134 * </p> 2135 */ 2136 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2137 2138 /** 2139 * Search parameter: <b>identifier</b> 2140 * <p> 2141 * Description: <b>The unique identifier for the questionnaire response</b><br> 2142 * Type: <b>token</b><br> 2143 * Path: <b>QuestionnaireResponse.identifier</b><br> 2144 * </p> 2145 */ 2146 @SearchParamDefinition(name="identifier", path="QuestionnaireResponse.identifier", description="The unique identifier for the questionnaire response", type="token" ) 2147 public static final String SP_IDENTIFIER = "identifier"; 2148 /** 2149 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2150 * <p> 2151 * Description: <b>The unique identifier for the questionnaire response</b><br> 2152 * Type: <b>token</b><br> 2153 * Path: <b>QuestionnaireResponse.identifier</b><br> 2154 * </p> 2155 */ 2156 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2157 2158 /** 2159 * Search parameter: <b>parent</b> 2160 * <p> 2161 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2162 * Type: <b>reference</b><br> 2163 * Path: <b>QuestionnaireResponse.parent</b><br> 2164 * </p> 2165 */ 2166 @SearchParamDefinition(name="parent", path="QuestionnaireResponse.parent", description="Procedure or observation this questionnaire response was performed as a part of", type="reference", target={Observation.class, Procedure.class } ) 2167 public static final String SP_PARENT = "parent"; 2168 /** 2169 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 2170 * <p> 2171 * Description: <b>Procedure or observation this questionnaire response was performed as a part of</b><br> 2172 * Type: <b>reference</b><br> 2173 * Path: <b>QuestionnaireResponse.parent</b><br> 2174 * </p> 2175 */ 2176 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 2177 2178/** 2179 * Constant for fluent queries to be used to add include statements. Specifies 2180 * the path value of "<b>QuestionnaireResponse:parent</b>". 2181 */ 2182 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:parent").toLocked(); 2183 2184 /** 2185 * Search parameter: <b>questionnaire</b> 2186 * <p> 2187 * Description: <b>The questionnaire the answers are provided for</b><br> 2188 * Type: <b>reference</b><br> 2189 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2190 * </p> 2191 */ 2192 @SearchParamDefinition(name="questionnaire", path="QuestionnaireResponse.questionnaire", description="The questionnaire the answers are provided for", type="reference", target={Questionnaire.class } ) 2193 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2194 /** 2195 * <b>Fluent Client</b> search parameter constant for <b>questionnaire</b> 2196 * <p> 2197 * Description: <b>The questionnaire the answers are provided for</b><br> 2198 * Type: <b>reference</b><br> 2199 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2200 * </p> 2201 */ 2202 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam QUESTIONNAIRE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_QUESTIONNAIRE); 2203 2204/** 2205 * Constant for fluent queries to be used to add include statements. Specifies 2206 * the path value of "<b>QuestionnaireResponse:questionnaire</b>". 2207 */ 2208 public static final ca.uhn.fhir.model.api.Include INCLUDE_QUESTIONNAIRE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:questionnaire").toLocked(); 2209 2210 /** 2211 * Search parameter: <b>based-on</b> 2212 * <p> 2213 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2214 * Type: <b>reference</b><br> 2215 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2216 * </p> 2217 */ 2218 @SearchParamDefinition(name="based-on", path="QuestionnaireResponse.basedOn", description="Plan/proposal/order fulfilled by this questionnaire response", type="reference", target={CarePlan.class, ProcedureRequest.class, ReferralRequest.class } ) 2219 public static final String SP_BASED_ON = "based-on"; 2220 /** 2221 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2222 * <p> 2223 * Description: <b>Plan/proposal/order fulfilled by this questionnaire response</b><br> 2224 * Type: <b>reference</b><br> 2225 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2226 * </p> 2227 */ 2228 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2229 2230/** 2231 * Constant for fluent queries to be used to add include statements. Specifies 2232 * the path value of "<b>QuestionnaireResponse:based-on</b>". 2233 */ 2234 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:based-on").toLocked(); 2235 2236 /** 2237 * Search parameter: <b>subject</b> 2238 * <p> 2239 * Description: <b>The subject of the questionnaire response</b><br> 2240 * Type: <b>reference</b><br> 2241 * Path: <b>QuestionnaireResponse.subject</b><br> 2242 * </p> 2243 */ 2244 @SearchParamDefinition(name="subject", path="QuestionnaireResponse.subject", description="The subject of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") } ) 2245 public static final String SP_SUBJECT = "subject"; 2246 /** 2247 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2248 * <p> 2249 * Description: <b>The subject of the questionnaire response</b><br> 2250 * Type: <b>reference</b><br> 2251 * Path: <b>QuestionnaireResponse.subject</b><br> 2252 * </p> 2253 */ 2254 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2255 2256/** 2257 * Constant for fluent queries to be used to add include statements. Specifies 2258 * the path value of "<b>QuestionnaireResponse:subject</b>". 2259 */ 2260 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:subject").toLocked(); 2261 2262 /** 2263 * Search parameter: <b>author</b> 2264 * <p> 2265 * Description: <b>The author of the questionnaire response</b><br> 2266 * Type: <b>reference</b><br> 2267 * Path: <b>QuestionnaireResponse.author</b><br> 2268 * </p> 2269 */ 2270 @SearchParamDefinition(name="author", path="QuestionnaireResponse.author", description="The author of the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2271 public static final String SP_AUTHOR = "author"; 2272 /** 2273 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2274 * <p> 2275 * Description: <b>The author of the questionnaire response</b><br> 2276 * Type: <b>reference</b><br> 2277 * Path: <b>QuestionnaireResponse.author</b><br> 2278 * </p> 2279 */ 2280 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2281 2282/** 2283 * Constant for fluent queries to be used to add include statements. Specifies 2284 * the path value of "<b>QuestionnaireResponse:author</b>". 2285 */ 2286 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:author").toLocked(); 2287 2288 /** 2289 * Search parameter: <b>patient</b> 2290 * <p> 2291 * Description: <b>The patient that is the subject of the questionnaire response</b><br> 2292 * Type: <b>reference</b><br> 2293 * Path: <b>QuestionnaireResponse.subject</b><br> 2294 * </p> 2295 */ 2296 @SearchParamDefinition(name="patient", path="QuestionnaireResponse.subject", description="The patient that is the subject of the questionnaire response", type="reference", target={Patient.class } ) 2297 public static final String SP_PATIENT = "patient"; 2298 /** 2299 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2300 * <p> 2301 * Description: <b>The patient that is the subject of the questionnaire response</b><br> 2302 * Type: <b>reference</b><br> 2303 * Path: <b>QuestionnaireResponse.subject</b><br> 2304 * </p> 2305 */ 2306 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2307 2308/** 2309 * Constant for fluent queries to be used to add include statements. Specifies 2310 * the path value of "<b>QuestionnaireResponse:patient</b>". 2311 */ 2312 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:patient").toLocked(); 2313 2314 /** 2315 * Search parameter: <b>context</b> 2316 * <p> 2317 * Description: <b>Encounter or episode associated with the questionnaire response</b><br> 2318 * Type: <b>reference</b><br> 2319 * Path: <b>QuestionnaireResponse.context</b><br> 2320 * </p> 2321 */ 2322 @SearchParamDefinition(name="context", path="QuestionnaireResponse.context", description="Encounter or episode associated with the questionnaire response", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2323 public static final String SP_CONTEXT = "context"; 2324 /** 2325 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2326 * <p> 2327 * Description: <b>Encounter or episode associated with the questionnaire response</b><br> 2328 * Type: <b>reference</b><br> 2329 * Path: <b>QuestionnaireResponse.context</b><br> 2330 * </p> 2331 */ 2332 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2333 2334/** 2335 * Constant for fluent queries to be used to add include statements. Specifies 2336 * the path value of "<b>QuestionnaireResponse:context</b>". 2337 */ 2338 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:context").toLocked(); 2339 2340 /** 2341 * Search parameter: <b>source</b> 2342 * <p> 2343 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2344 * Type: <b>reference</b><br> 2345 * Path: <b>QuestionnaireResponse.source</b><br> 2346 * </p> 2347 */ 2348 @SearchParamDefinition(name="source", path="QuestionnaireResponse.source", description="The individual providing the information reflected in the questionnaire respose", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 2349 public static final String SP_SOURCE = "source"; 2350 /** 2351 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2352 * <p> 2353 * Description: <b>The individual providing the information reflected in the questionnaire respose</b><br> 2354 * Type: <b>reference</b><br> 2355 * Path: <b>QuestionnaireResponse.source</b><br> 2356 * </p> 2357 */ 2358 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2359 2360/** 2361 * Constant for fluent queries to be used to add include statements. Specifies 2362 * the path value of "<b>QuestionnaireResponse:source</b>". 2363 */ 2364 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("QuestionnaireResponse:source").toLocked(); 2365 2366 /** 2367 * Search parameter: <b>status</b> 2368 * <p> 2369 * Description: <b>The status of the questionnaire response</b><br> 2370 * Type: <b>token</b><br> 2371 * Path: <b>QuestionnaireResponse.status</b><br> 2372 * </p> 2373 */ 2374 @SearchParamDefinition(name="status", path="QuestionnaireResponse.status", description="The status of the questionnaire response", type="token" ) 2375 public static final String SP_STATUS = "status"; 2376 /** 2377 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2378 * <p> 2379 * Description: <b>The status of the questionnaire response</b><br> 2380 * Type: <b>token</b><br> 2381 * Path: <b>QuestionnaireResponse.status</b><br> 2382 * </p> 2383 */ 2384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2385 2386 2387}