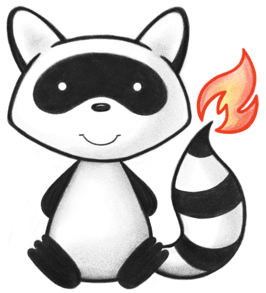
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * A set of ordered Quantities defined by a low and high limit. 046 */ 047@DatatypeDef(name="Range") 048public class Range extends Type implements ICompositeType { 049 050 /** 051 * The low limit. The boundary is inclusive. 052 */ 053 @Child(name = "low", type = {SimpleQuantity.class}, order=0, min=0, max=1, modifier=false, summary=true) 054 @Description(shortDefinition="Low limit", formalDefinition="The low limit. The boundary is inclusive." ) 055 protected SimpleQuantity low; 056 057 /** 058 * The high limit. The boundary is inclusive. 059 */ 060 @Child(name = "high", type = {SimpleQuantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="High limit", formalDefinition="The high limit. The boundary is inclusive." ) 062 protected SimpleQuantity high; 063 064 private static final long serialVersionUID = 1699187994L; 065 066 /** 067 * Constructor 068 */ 069 public Range() { 070 super(); 071 } 072 073 /** 074 * @return {@link #low} (The low limit. The boundary is inclusive.) 075 */ 076 public SimpleQuantity getLow() { 077 if (this.low == null) 078 if (Configuration.errorOnAutoCreate()) 079 throw new Error("Attempt to auto-create Range.low"); 080 else if (Configuration.doAutoCreate()) 081 this.low = new SimpleQuantity(); // cc 082 return this.low; 083 } 084 085 public boolean hasLow() { 086 return this.low != null && !this.low.isEmpty(); 087 } 088 089 /** 090 * @param value {@link #low} (The low limit. The boundary is inclusive.) 091 */ 092 public Range setLow(SimpleQuantity value) { 093 this.low = value; 094 return this; 095 } 096 097 /** 098 * @return {@link #high} (The high limit. The boundary is inclusive.) 099 */ 100 public SimpleQuantity getHigh() { 101 if (this.high == null) 102 if (Configuration.errorOnAutoCreate()) 103 throw new Error("Attempt to auto-create Range.high"); 104 else if (Configuration.doAutoCreate()) 105 this.high = new SimpleQuantity(); // cc 106 return this.high; 107 } 108 109 public boolean hasHigh() { 110 return this.high != null && !this.high.isEmpty(); 111 } 112 113 /** 114 * @param value {@link #high} (The high limit. The boundary is inclusive.) 115 */ 116 public Range setHigh(SimpleQuantity value) { 117 this.high = value; 118 return this; 119 } 120 121 protected void listChildren(List<Property> children) { 122 super.listChildren(children); 123 children.add(new Property("low", "SimpleQuantity", "The low limit. The boundary is inclusive.", 0, 1, low)); 124 children.add(new Property("high", "SimpleQuantity", "The high limit. The boundary is inclusive.", 0, 1, high)); 125 } 126 127 @Override 128 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 129 switch (_hash) { 130 case 107348: /*low*/ return new Property("low", "SimpleQuantity", "The low limit. The boundary is inclusive.", 0, 1, low); 131 case 3202466: /*high*/ return new Property("high", "SimpleQuantity", "The high limit. The boundary is inclusive.", 0, 1, high); 132 default: return super.getNamedProperty(_hash, _name, _checkValid); 133 } 134 135 } 136 137 @Override 138 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 139 switch (hash) { 140 case 107348: /*low*/ return this.low == null ? new Base[0] : new Base[] {this.low}; // SimpleQuantity 141 case 3202466: /*high*/ return this.high == null ? new Base[0] : new Base[] {this.high}; // SimpleQuantity 142 default: return super.getProperty(hash, name, checkValid); 143 } 144 145 } 146 147 @Override 148 public Base setProperty(int hash, String name, Base value) throws FHIRException { 149 switch (hash) { 150 case 107348: // low 151 this.low = castToSimpleQuantity(value); // SimpleQuantity 152 return value; 153 case 3202466: // high 154 this.high = castToSimpleQuantity(value); // SimpleQuantity 155 return value; 156 default: return super.setProperty(hash, name, value); 157 } 158 159 } 160 161 @Override 162 public Base setProperty(String name, Base value) throws FHIRException { 163 if (name.equals("low")) { 164 this.low = castToSimpleQuantity(value); // SimpleQuantity 165 } else if (name.equals("high")) { 166 this.high = castToSimpleQuantity(value); // SimpleQuantity 167 } else 168 return super.setProperty(name, value); 169 return value; 170 } 171 172 @Override 173 public Base makeProperty(int hash, String name) throws FHIRException { 174 switch (hash) { 175 case 107348: return getLow(); 176 case 3202466: return getHigh(); 177 default: return super.makeProperty(hash, name); 178 } 179 180 } 181 182 @Override 183 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 184 switch (hash) { 185 case 107348: /*low*/ return new String[] {"SimpleQuantity"}; 186 case 3202466: /*high*/ return new String[] {"SimpleQuantity"}; 187 default: return super.getTypesForProperty(hash, name); 188 } 189 190 } 191 192 @Override 193 public Base addChild(String name) throws FHIRException { 194 if (name.equals("low")) { 195 this.low = new SimpleQuantity(); 196 return this.low; 197 } 198 else if (name.equals("high")) { 199 this.high = new SimpleQuantity(); 200 return this.high; 201 } 202 else 203 return super.addChild(name); 204 } 205 206 public String fhirType() { 207 return "Range"; 208 209 } 210 211 public Range copy() { 212 Range dst = new Range(); 213 copyValues(dst); 214 dst.low = low == null ? null : low.copy(); 215 dst.high = high == null ? null : high.copy(); 216 return dst; 217 } 218 219 protected Range typedCopy() { 220 return copy(); 221 } 222 223 @Override 224 public boolean equalsDeep(Base other_) { 225 if (!super.equalsDeep(other_)) 226 return false; 227 if (!(other_ instanceof Range)) 228 return false; 229 Range o = (Range) other_; 230 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true); 231 } 232 233 @Override 234 public boolean equalsShallow(Base other_) { 235 if (!super.equalsShallow(other_)) 236 return false; 237 if (!(other_ instanceof Range)) 238 return false; 239 Range o = (Range) other_; 240 return true; 241 } 242 243 public boolean isEmpty() { 244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high); 245 } 246 247 248}