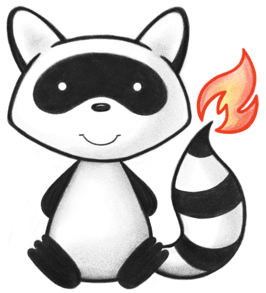
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043/** 044 * A relationship of two Quantity values - expressed as a numerator and a denominator. 045 */ 046@DatatypeDef(name="Ratio") 047public class Ratio extends Type implements ICompositeType { 048 049 /** 050 * The value of the numerator. 051 */ 052 @Child(name = "numerator", type = {Quantity.class}, order=0, min=0, max=1, modifier=false, summary=true) 053 @Description(shortDefinition="Numerator value", formalDefinition="The value of the numerator." ) 054 protected Quantity numerator; 055 056 /** 057 * The value of the denominator. 058 */ 059 @Child(name = "denominator", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Denominator value", formalDefinition="The value of the denominator." ) 061 protected Quantity denominator; 062 063 private static final long serialVersionUID = 479922563L; 064 065 /** 066 * Constructor 067 */ 068 public Ratio() { 069 super(); 070 } 071 072 /** 073 * @return {@link #numerator} (The value of the numerator.) 074 */ 075 public Quantity getNumerator() { 076 if (this.numerator == null) 077 if (Configuration.errorOnAutoCreate()) 078 throw new Error("Attempt to auto-create Ratio.numerator"); 079 else if (Configuration.doAutoCreate()) 080 this.numerator = new Quantity(); // cc 081 return this.numerator; 082 } 083 084 public boolean hasNumerator() { 085 return this.numerator != null && !this.numerator.isEmpty(); 086 } 087 088 /** 089 * @param value {@link #numerator} (The value of the numerator.) 090 */ 091 public Ratio setNumerator(Quantity value) { 092 this.numerator = value; 093 return this; 094 } 095 096 /** 097 * @return {@link #denominator} (The value of the denominator.) 098 */ 099 public Quantity getDenominator() { 100 if (this.denominator == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create Ratio.denominator"); 103 else if (Configuration.doAutoCreate()) 104 this.denominator = new Quantity(); // cc 105 return this.denominator; 106 } 107 108 public boolean hasDenominator() { 109 return this.denominator != null && !this.denominator.isEmpty(); 110 } 111 112 /** 113 * @param value {@link #denominator} (The value of the denominator.) 114 */ 115 public Ratio setDenominator(Quantity value) { 116 this.denominator = value; 117 return this; 118 } 119 120 protected void listChildren(List<Property> children) { 121 super.listChildren(children); 122 children.add(new Property("numerator", "Quantity", "The value of the numerator.", 0, 1, numerator)); 123 children.add(new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, denominator)); 124 } 125 126 @Override 127 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 128 switch (_hash) { 129 case 1747334793: /*numerator*/ return new Property("numerator", "Quantity", "The value of the numerator.", 0, 1, numerator); 130 case -1983274394: /*denominator*/ return new Property("denominator", "Quantity", "The value of the denominator.", 0, 1, denominator); 131 default: return super.getNamedProperty(_hash, _name, _checkValid); 132 } 133 134 } 135 136 @Override 137 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 138 switch (hash) { 139 case 1747334793: /*numerator*/ return this.numerator == null ? new Base[0] : new Base[] {this.numerator}; // Quantity 140 case -1983274394: /*denominator*/ return this.denominator == null ? new Base[0] : new Base[] {this.denominator}; // Quantity 141 default: return super.getProperty(hash, name, checkValid); 142 } 143 144 } 145 146 @Override 147 public Base setProperty(int hash, String name, Base value) throws FHIRException { 148 switch (hash) { 149 case 1747334793: // numerator 150 this.numerator = castToQuantity(value); // Quantity 151 return value; 152 case -1983274394: // denominator 153 this.denominator = castToQuantity(value); // Quantity 154 return value; 155 default: return super.setProperty(hash, name, value); 156 } 157 158 } 159 160 @Override 161 public Base setProperty(String name, Base value) throws FHIRException { 162 if (name.equals("numerator")) { 163 this.numerator = castToQuantity(value); // Quantity 164 } else if (name.equals("denominator")) { 165 this.denominator = castToQuantity(value); // Quantity 166 } else 167 return super.setProperty(name, value); 168 return value; 169 } 170 171 @Override 172 public Base makeProperty(int hash, String name) throws FHIRException { 173 switch (hash) { 174 case 1747334793: return getNumerator(); 175 case -1983274394: return getDenominator(); 176 default: return super.makeProperty(hash, name); 177 } 178 179 } 180 181 @Override 182 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 183 switch (hash) { 184 case 1747334793: /*numerator*/ return new String[] {"Quantity"}; 185 case -1983274394: /*denominator*/ return new String[] {"Quantity"}; 186 default: return super.getTypesForProperty(hash, name); 187 } 188 189 } 190 191 @Override 192 public Base addChild(String name) throws FHIRException { 193 if (name.equals("numerator")) { 194 this.numerator = new Quantity(); 195 return this.numerator; 196 } 197 else if (name.equals("denominator")) { 198 this.denominator = new Quantity(); 199 return this.denominator; 200 } 201 else 202 return super.addChild(name); 203 } 204 205 public String fhirType() { 206 return "Ratio"; 207 208 } 209 210 public Ratio copy() { 211 Ratio dst = new Ratio(); 212 copyValues(dst); 213 dst.numerator = numerator == null ? null : numerator.copy(); 214 dst.denominator = denominator == null ? null : denominator.copy(); 215 return dst; 216 } 217 218 protected Ratio typedCopy() { 219 return copy(); 220 } 221 222 @Override 223 public boolean equalsDeep(Base other_) { 224 if (!super.equalsDeep(other_)) 225 return false; 226 if (!(other_ instanceof Ratio)) 227 return false; 228 Ratio o = (Ratio) other_; 229 return compareDeep(numerator, o.numerator, true) && compareDeep(denominator, o.denominator, true) 230 ; 231 } 232 233 @Override 234 public boolean equalsShallow(Base other_) { 235 if (!super.equalsShallow(other_)) 236 return false; 237 if (!(other_ instanceof Ratio)) 238 return false; 239 Ratio o = (Ratio) other_; 240 return true; 241 } 242 243 public boolean isEmpty() { 244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(numerator, denominator); 245 } 246 247 248}