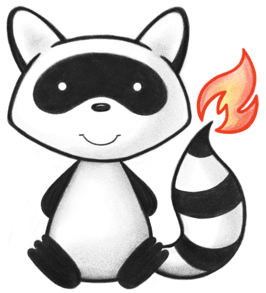
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IAnyResource; 039import org.hl7.fhir.instance.model.api.IBaseReference; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.instance.model.api.IIdType; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047/** 048 * A reference from one resource to another. 049 */ 050@DatatypeDef(name="Reference") 051public class Reference extends BaseReference implements IBaseReference, ICompositeType { 052 053 /** 054 * A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 055 */ 056 @Child(name = "reference", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="Literal reference, Relative, internal or absolute URL", formalDefinition="A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources." ) 058 protected StringType reference; 059 060 /** 061 * An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference. 062 */ 063 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="Logical reference, when literal reference is not known", formalDefinition="An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference." ) 065 protected Identifier identifier; 066 067 /** 068 * Plain text narrative that identifies the resource in addition to the resource reference. 069 */ 070 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="Text alternative for the resource", formalDefinition="Plain text narrative that identifies the resource in addition to the resource reference." ) 072 protected StringType display; 073 074 private static final long serialVersionUID = -909353281L; 075 076 /** 077 * Constructor 078 */ 079 public Reference() { 080 super(); 081 } 082 083 /** 084 * Constructor 085 * 086 * @param theReference The given reference string (e.g. "Patient/123" or "http://example.com/Patient/123") 087 */ 088 public Reference(String theReference) { 089 super(theReference); 090 } 091 092 /** 093 * Constructor 094 * 095 * @param theReference The given reference as an IdType (e.g. "Patient/123" or "http://example.com/Patient/123") 096 */ 097 public Reference(IIdType theReference) { 098 super(theReference); 099 } 100 101 /** 102 * Constructor 103 * 104 * @param theResource The resource represented by this reference 105 */ 106 public Reference(IAnyResource theResource) { 107 super(theResource); 108 } 109 110 /** 111 * @return {@link #reference} (A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 112 */ 113 public StringType getReferenceElement_() { 114 if (this.reference == null) 115 if (Configuration.errorOnAutoCreate()) 116 throw new Error("Attempt to auto-create Reference.reference"); 117 else if (Configuration.doAutoCreate()) 118 this.reference = new StringType(); // bb 119 return this.reference; 120 } 121 122 public boolean hasReferenceElement() { 123 return this.reference != null && !this.reference.isEmpty(); 124 } 125 126 public boolean hasReference() { 127 return this.reference != null && !this.reference.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #reference} (A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 132 */ 133 public Reference setReferenceElement(StringType value) { 134 this.reference = value; 135 return this; 136 } 137 138 /** 139 * @return A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 140 */ 141 public String getReference() { 142 return this.reference == null ? null : this.reference.getValue(); 143 } 144 145 /** 146 * @param value A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 147 */ 148 public Reference setReference(String value) { 149 if (Utilities.noString(value)) 150 this.reference = null; 151 else { 152 if (this.reference == null) 153 this.reference = new StringType(); 154 this.reference.setValue(value); 155 } 156 return this; 157 } 158 159 /** 160 * @return {@link #identifier} (An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.) 161 */ 162 public Identifier getIdentifier() { 163 if (this.identifier == null) 164 if (Configuration.errorOnAutoCreate()) 165 throw new Error("Attempt to auto-create Reference.identifier"); 166 else if (Configuration.doAutoCreate()) 167 this.identifier = new Identifier(); // cc 168 return this.identifier; 169 } 170 171 public boolean hasIdentifier() { 172 return this.identifier != null && !this.identifier.isEmpty(); 173 } 174 175 /** 176 * @param value {@link #identifier} (An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.) 177 */ 178 public Reference setIdentifier(Identifier value) { 179 this.identifier = value; 180 return this; 181 } 182 183 /** 184 * @return {@link #display} (Plain text narrative that identifies the resource in addition to the resource reference.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 185 */ 186 public StringType getDisplayElement() { 187 if (this.display == null) 188 if (Configuration.errorOnAutoCreate()) 189 throw new Error("Attempt to auto-create Reference.display"); 190 else if (Configuration.doAutoCreate()) 191 this.display = new StringType(); // bb 192 return this.display; 193 } 194 195 public boolean hasDisplayElement() { 196 return this.display != null && !this.display.isEmpty(); 197 } 198 199 public boolean hasDisplay() { 200 return this.display != null && !this.display.isEmpty(); 201 } 202 203 /** 204 * @param value {@link #display} (Plain text narrative that identifies the resource in addition to the resource reference.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 205 */ 206 public Reference setDisplayElement(StringType value) { 207 this.display = value; 208 return this; 209 } 210 211 /** 212 * @return Plain text narrative that identifies the resource in addition to the resource reference. 213 */ 214 public String getDisplay() { 215 return this.display == null ? null : this.display.getValue(); 216 } 217 218 /** 219 * @param value Plain text narrative that identifies the resource in addition to the resource reference. 220 */ 221 public Reference setDisplay(String value) { 222 if (Utilities.noString(value)) 223 this.display = null; 224 else { 225 if (this.display == null) 226 this.display = new StringType(); 227 this.display.setValue(value); 228 } 229 return this; 230 } 231 232 /** 233 * Convenience setter which sets the reference to the complete {@link IIdType#getValue() value} of the given 234 * reference. 235 * 236 * @param theReference The reference, or <code>null</code> 237 * @return 238 * @return Returns a reference to this 239 */ 240 public Reference setReferenceElement(IIdType theReference) { 241 if (theReference != null) { 242 setReference(theReference.getValue()); 243 } else { 244 setReference(null); 245 } 246 return this; 247 } 248 protected void listChildren(List<Property> children) { 249 super.listChildren(children); 250 children.add(new Property("reference", "string", "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 0, 1, reference)); 251 children.add(new Property("identifier", "Identifier", "An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 0, 1, identifier)); 252 children.add(new Property("display", "string", "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display)); 253 } 254 255 @Override 256 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 257 switch (_hash) { 258 case -925155509: /*reference*/ return new Property("reference", "string", "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 0, 1, reference); 259 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for the other resource. This is used when there is no way to reference the other resource directly, either because the entity is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 0, 1, identifier); 260 case 1671764162: /*display*/ return new Property("display", "string", "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display); 261 default: return super.getNamedProperty(_hash, _name, _checkValid); 262 } 263 264 } 265 266 @Override 267 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 268 switch (hash) { 269 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // StringType 270 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 271 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 272 default: return super.getProperty(hash, name, checkValid); 273 } 274 275 } 276 277 @Override 278 public Base setProperty(int hash, String name, Base value) throws FHIRException { 279 switch (hash) { 280 case -925155509: // reference 281 this.reference = castToString(value); // StringType 282 return value; 283 case -1618432855: // identifier 284 this.identifier = castToIdentifier(value); // Identifier 285 return value; 286 case 1671764162: // display 287 this.display = castToString(value); // StringType 288 return value; 289 default: return super.setProperty(hash, name, value); 290 } 291 292 } 293 294 @Override 295 public Base setProperty(String name, Base value) throws FHIRException { 296 if (name.equals("reference")) { 297 this.reference = castToString(value); // StringType 298 } else if (name.equals("identifier")) { 299 this.identifier = castToIdentifier(value); // Identifier 300 } else if (name.equals("display")) { 301 this.display = castToString(value); // StringType 302 } else 303 return super.setProperty(name, value); 304 return value; 305 } 306 307 @Override 308 public Base makeProperty(int hash, String name) throws FHIRException { 309 switch (hash) { 310 case -925155509: return getReferenceElement_(); 311 case -1618432855: return getIdentifier(); 312 case 1671764162: return getDisplayElement(); 313 default: return super.makeProperty(hash, name); 314 } 315 316 } 317 318 @Override 319 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 320 switch (hash) { 321 case -925155509: /*reference*/ return new String[] {"string"}; 322 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 323 case 1671764162: /*display*/ return new String[] {"string"}; 324 default: return super.getTypesForProperty(hash, name); 325 } 326 327 } 328 329 @Override 330 public Base addChild(String name) throws FHIRException { 331 if (name.equals("reference")) { 332 throw new FHIRException("Cannot call addChild on a singleton property Reference.reference"); 333 } 334 else if (name.equals("identifier")) { 335 this.identifier = new Identifier(); 336 return this.identifier; 337 } 338 else if (name.equals("display")) { 339 throw new FHIRException("Cannot call addChild on a singleton property Reference.display"); 340 } 341 else 342 return super.addChild(name); 343 } 344 345 public String fhirType() { 346 return "Reference"; 347 348 } 349 350 public Reference copy() { 351 Reference dst = new Reference(); 352 copyValues(dst); 353 dst.reference = reference == null ? null : reference.copy(); 354 dst.identifier = identifier == null ? null : identifier.copy(); 355 dst.display = display == null ? null : display.copy(); 356 return dst; 357 } 358 359 protected Reference typedCopy() { 360 return copy(); 361 } 362 363 @Override 364 public boolean equalsDeep(Base other_) { 365 if (!super.equalsDeep(other_)) 366 return false; 367 if (!(other_ instanceof Reference)) 368 return false; 369 Reference o = (Reference) other_; 370 return compareDeep(reference, o.reference, true) && compareDeep(identifier, o.identifier, true) 371 && compareDeep(display, o.display, true); 372 } 373 374 @Override 375 public boolean equalsShallow(Base other_) { 376 if (!super.equalsShallow(other_)) 377 return false; 378 if (!(other_ instanceof Reference)) 379 return false; 380 Reference o = (Reference) other_; 381 return compareValues(reference, o.reference, true) && compareValues(display, o.display, true); 382 } 383 384 public boolean isEmpty() { 385 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, identifier, display 386 ); 387 } 388 389 390}