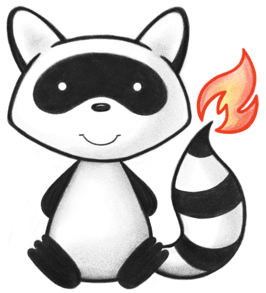
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 052 */ 053@ResourceDef(name="ReferralRequest", profile="http://hl7.org/fhir/Profile/ReferralRequest") 054public class ReferralRequest extends DomainResource { 055 056 public enum ReferralRequestStatus { 057 /** 058 * The request has been created but is not yet complete or ready for action 059 */ 060 DRAFT, 061 /** 062 * The request is ready to be acted upon 063 */ 064 ACTIVE, 065 /** 066 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 067 */ 068 SUSPENDED, 069 /** 070 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 071 */ 072 CANCELLED, 073 /** 074 * Activity against the request has been sufficiently completed to the satisfaction of the requester 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 079 */ 080 ENTEREDINERROR, 081 /** 082 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static ReferralRequestStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("draft".equals(codeString)) 093 return DRAFT; 094 if ("active".equals(codeString)) 095 return ACTIVE; 096 if ("suspended".equals(codeString)) 097 return SUSPENDED; 098 if ("cancelled".equals(codeString)) 099 return CANCELLED; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown ReferralRequestStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case DRAFT: return "draft"; 114 case ACTIVE: return "active"; 115 case SUSPENDED: return "suspended"; 116 case CANCELLED: return "cancelled"; 117 case COMPLETED: return "completed"; 118 case ENTEREDINERROR: return "entered-in-error"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case DRAFT: return "http://hl7.org/fhir/request-status"; 127 case ACTIVE: return "http://hl7.org/fhir/request-status"; 128 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 129 case CANCELLED: return "http://hl7.org/fhir/request-status"; 130 case COMPLETED: return "http://hl7.org/fhir/request-status"; 131 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 140 case ACTIVE: return "The request is ready to be acted upon"; 141 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 142 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 143 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 144 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 145 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case DRAFT: return "Draft"; 153 case ACTIVE: return "Active"; 154 case SUSPENDED: return "Suspended"; 155 case CANCELLED: return "Cancelled"; 156 case COMPLETED: return "Completed"; 157 case ENTEREDINERROR: return "Entered in Error"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class ReferralRequestStatusEnumFactory implements EnumFactory<ReferralRequestStatus> { 166 public ReferralRequestStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("draft".equals(codeString)) 171 return ReferralRequestStatus.DRAFT; 172 if ("active".equals(codeString)) 173 return ReferralRequestStatus.ACTIVE; 174 if ("suspended".equals(codeString)) 175 return ReferralRequestStatus.SUSPENDED; 176 if ("cancelled".equals(codeString)) 177 return ReferralRequestStatus.CANCELLED; 178 if ("completed".equals(codeString)) 179 return ReferralRequestStatus.COMPLETED; 180 if ("entered-in-error".equals(codeString)) 181 return ReferralRequestStatus.ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return ReferralRequestStatus.UNKNOWN; 184 throw new IllegalArgumentException("Unknown ReferralRequestStatus code '"+codeString+"'"); 185 } 186 public Enumeration<ReferralRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<ReferralRequestStatus>(this); 191 String codeString = code.asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("draft".equals(codeString)) 195 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.DRAFT); 196 if ("active".equals(codeString)) 197 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.ACTIVE); 198 if ("suspended".equals(codeString)) 199 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.SUSPENDED); 200 if ("cancelled".equals(codeString)) 201 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.CANCELLED); 202 if ("completed".equals(codeString)) 203 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.COMPLETED); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.ENTEREDINERROR); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<ReferralRequestStatus>(this, ReferralRequestStatus.UNKNOWN); 208 throw new FHIRException("Unknown ReferralRequestStatus code '"+codeString+"'"); 209 } 210 public String toCode(ReferralRequestStatus code) { 211 if (code == ReferralRequestStatus.NULL) 212 return null; 213 if (code == ReferralRequestStatus.DRAFT) 214 return "draft"; 215 if (code == ReferralRequestStatus.ACTIVE) 216 return "active"; 217 if (code == ReferralRequestStatus.SUSPENDED) 218 return "suspended"; 219 if (code == ReferralRequestStatus.CANCELLED) 220 return "cancelled"; 221 if (code == ReferralRequestStatus.COMPLETED) 222 return "completed"; 223 if (code == ReferralRequestStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == ReferralRequestStatus.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(ReferralRequestStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 public enum ReferralCategory { 235 /** 236 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 237 */ 238 PROPOSAL, 239 /** 240 * The request represents an intension to ensure something occurs without providing an authorization for others to act 241 */ 242 PLAN, 243 /** 244 * The request represents a request/demand and authorization for action 245 */ 246 ORDER, 247 /** 248 * The request represents an original authorization for action 249 */ 250 ORIGINALORDER, 251 /** 252 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 253 */ 254 REFLEXORDER, 255 /** 256 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 257 */ 258 FILLERORDER, 259 /** 260 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 261 */ 262 INSTANCEORDER, 263 /** 264 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 265 266Refer to [[[RequestGroup]]] for additional information on how this status is used 267 */ 268 OPTION, 269 /** 270 * added to help the parsers with the generic types 271 */ 272 NULL; 273 public static ReferralCategory fromCode(String codeString) throws FHIRException { 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("proposal".equals(codeString)) 277 return PROPOSAL; 278 if ("plan".equals(codeString)) 279 return PLAN; 280 if ("order".equals(codeString)) 281 return ORDER; 282 if ("original-order".equals(codeString)) 283 return ORIGINALORDER; 284 if ("reflex-order".equals(codeString)) 285 return REFLEXORDER; 286 if ("filler-order".equals(codeString)) 287 return FILLERORDER; 288 if ("instance-order".equals(codeString)) 289 return INSTANCEORDER; 290 if ("option".equals(codeString)) 291 return OPTION; 292 if (Configuration.isAcceptInvalidEnums()) 293 return null; 294 else 295 throw new FHIRException("Unknown ReferralCategory code '"+codeString+"'"); 296 } 297 public String toCode() { 298 switch (this) { 299 case PROPOSAL: return "proposal"; 300 case PLAN: return "plan"; 301 case ORDER: return "order"; 302 case ORIGINALORDER: return "original-order"; 303 case REFLEXORDER: return "reflex-order"; 304 case FILLERORDER: return "filler-order"; 305 case INSTANCEORDER: return "instance-order"; 306 case OPTION: return "option"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 public String getSystem() { 312 switch (this) { 313 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 314 case PLAN: return "http://hl7.org/fhir/request-intent"; 315 case ORDER: return "http://hl7.org/fhir/request-intent"; 316 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 317 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 318 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 319 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 320 case OPTION: return "http://hl7.org/fhir/request-intent"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 public String getDefinition() { 326 switch (this) { 327 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 328 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 329 case ORDER: return "The request represents a request/demand and authorization for action"; 330 case ORIGINALORDER: return "The request represents an original authorization for action"; 331 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 332 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 333 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 334 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getDisplay() { 340 switch (this) { 341 case PROPOSAL: return "Proposal"; 342 case PLAN: return "Plan"; 343 case ORDER: return "Order"; 344 case ORIGINALORDER: return "Original Order"; 345 case REFLEXORDER: return "Reflex Order"; 346 case FILLERORDER: return "Filler Order"; 347 case INSTANCEORDER: return "Instance Order"; 348 case OPTION: return "Option"; 349 case NULL: return null; 350 default: return "?"; 351 } 352 } 353 } 354 355 public static class ReferralCategoryEnumFactory implements EnumFactory<ReferralCategory> { 356 public ReferralCategory fromCode(String codeString) throws IllegalArgumentException { 357 if (codeString == null || "".equals(codeString)) 358 if (codeString == null || "".equals(codeString)) 359 return null; 360 if ("proposal".equals(codeString)) 361 return ReferralCategory.PROPOSAL; 362 if ("plan".equals(codeString)) 363 return ReferralCategory.PLAN; 364 if ("order".equals(codeString)) 365 return ReferralCategory.ORDER; 366 if ("original-order".equals(codeString)) 367 return ReferralCategory.ORIGINALORDER; 368 if ("reflex-order".equals(codeString)) 369 return ReferralCategory.REFLEXORDER; 370 if ("filler-order".equals(codeString)) 371 return ReferralCategory.FILLERORDER; 372 if ("instance-order".equals(codeString)) 373 return ReferralCategory.INSTANCEORDER; 374 if ("option".equals(codeString)) 375 return ReferralCategory.OPTION; 376 throw new IllegalArgumentException("Unknown ReferralCategory code '"+codeString+"'"); 377 } 378 public Enumeration<ReferralCategory> fromType(PrimitiveType<?> code) throws FHIRException { 379 if (code == null) 380 return null; 381 if (code.isEmpty()) 382 return new Enumeration<ReferralCategory>(this); 383 String codeString = code.asStringValue(); 384 if (codeString == null || "".equals(codeString)) 385 return null; 386 if ("proposal".equals(codeString)) 387 return new Enumeration<ReferralCategory>(this, ReferralCategory.PROPOSAL); 388 if ("plan".equals(codeString)) 389 return new Enumeration<ReferralCategory>(this, ReferralCategory.PLAN); 390 if ("order".equals(codeString)) 391 return new Enumeration<ReferralCategory>(this, ReferralCategory.ORDER); 392 if ("original-order".equals(codeString)) 393 return new Enumeration<ReferralCategory>(this, ReferralCategory.ORIGINALORDER); 394 if ("reflex-order".equals(codeString)) 395 return new Enumeration<ReferralCategory>(this, ReferralCategory.REFLEXORDER); 396 if ("filler-order".equals(codeString)) 397 return new Enumeration<ReferralCategory>(this, ReferralCategory.FILLERORDER); 398 if ("instance-order".equals(codeString)) 399 return new Enumeration<ReferralCategory>(this, ReferralCategory.INSTANCEORDER); 400 if ("option".equals(codeString)) 401 return new Enumeration<ReferralCategory>(this, ReferralCategory.OPTION); 402 throw new FHIRException("Unknown ReferralCategory code '"+codeString+"'"); 403 } 404 public String toCode(ReferralCategory code) { 405 if (code == ReferralCategory.NULL) 406 return null; 407 if (code == ReferralCategory.PROPOSAL) 408 return "proposal"; 409 if (code == ReferralCategory.PLAN) 410 return "plan"; 411 if (code == ReferralCategory.ORDER) 412 return "order"; 413 if (code == ReferralCategory.ORIGINALORDER) 414 return "original-order"; 415 if (code == ReferralCategory.REFLEXORDER) 416 return "reflex-order"; 417 if (code == ReferralCategory.FILLERORDER) 418 return "filler-order"; 419 if (code == ReferralCategory.INSTANCEORDER) 420 return "instance-order"; 421 if (code == ReferralCategory.OPTION) 422 return "option"; 423 return "?"; 424 } 425 public String toSystem(ReferralCategory code) { 426 return code.getSystem(); 427 } 428 } 429 430 public enum ReferralPriority { 431 /** 432 * The request has normal priority 433 */ 434 ROUTINE, 435 /** 436 * The request should be actioned promptly - higher priority than routine 437 */ 438 URGENT, 439 /** 440 * The request should be actioned as soon as possible - higher priority than urgent 441 */ 442 ASAP, 443 /** 444 * The request should be actioned immediately - highest possible priority. E.g. an emergency 445 */ 446 STAT, 447 /** 448 * added to help the parsers with the generic types 449 */ 450 NULL; 451 public static ReferralPriority fromCode(String codeString) throws FHIRException { 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("routine".equals(codeString)) 455 return ROUTINE; 456 if ("urgent".equals(codeString)) 457 return URGENT; 458 if ("asap".equals(codeString)) 459 return ASAP; 460 if ("stat".equals(codeString)) 461 return STAT; 462 if (Configuration.isAcceptInvalidEnums()) 463 return null; 464 else 465 throw new FHIRException("Unknown ReferralPriority code '"+codeString+"'"); 466 } 467 public String toCode() { 468 switch (this) { 469 case ROUTINE: return "routine"; 470 case URGENT: return "urgent"; 471 case ASAP: return "asap"; 472 case STAT: return "stat"; 473 case NULL: return null; 474 default: return "?"; 475 } 476 } 477 public String getSystem() { 478 switch (this) { 479 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 480 case URGENT: return "http://hl7.org/fhir/request-priority"; 481 case ASAP: return "http://hl7.org/fhir/request-priority"; 482 case STAT: return "http://hl7.org/fhir/request-priority"; 483 case NULL: return null; 484 default: return "?"; 485 } 486 } 487 public String getDefinition() { 488 switch (this) { 489 case ROUTINE: return "The request has normal priority"; 490 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 491 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 492 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 493 case NULL: return null; 494 default: return "?"; 495 } 496 } 497 public String getDisplay() { 498 switch (this) { 499 case ROUTINE: return "Routine"; 500 case URGENT: return "Urgent"; 501 case ASAP: return "ASAP"; 502 case STAT: return "STAT"; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 } 508 509 public static class ReferralPriorityEnumFactory implements EnumFactory<ReferralPriority> { 510 public ReferralPriority fromCode(String codeString) throws IllegalArgumentException { 511 if (codeString == null || "".equals(codeString)) 512 if (codeString == null || "".equals(codeString)) 513 return null; 514 if ("routine".equals(codeString)) 515 return ReferralPriority.ROUTINE; 516 if ("urgent".equals(codeString)) 517 return ReferralPriority.URGENT; 518 if ("asap".equals(codeString)) 519 return ReferralPriority.ASAP; 520 if ("stat".equals(codeString)) 521 return ReferralPriority.STAT; 522 throw new IllegalArgumentException("Unknown ReferralPriority code '"+codeString+"'"); 523 } 524 public Enumeration<ReferralPriority> fromType(PrimitiveType<?> code) throws FHIRException { 525 if (code == null) 526 return null; 527 if (code.isEmpty()) 528 return new Enumeration<ReferralPriority>(this); 529 String codeString = code.asStringValue(); 530 if (codeString == null || "".equals(codeString)) 531 return null; 532 if ("routine".equals(codeString)) 533 return new Enumeration<ReferralPriority>(this, ReferralPriority.ROUTINE); 534 if ("urgent".equals(codeString)) 535 return new Enumeration<ReferralPriority>(this, ReferralPriority.URGENT); 536 if ("asap".equals(codeString)) 537 return new Enumeration<ReferralPriority>(this, ReferralPriority.ASAP); 538 if ("stat".equals(codeString)) 539 return new Enumeration<ReferralPriority>(this, ReferralPriority.STAT); 540 throw new FHIRException("Unknown ReferralPriority code '"+codeString+"'"); 541 } 542 public String toCode(ReferralPriority code) { 543 if (code == ReferralPriority.NULL) 544 return null; 545 if (code == ReferralPriority.ROUTINE) 546 return "routine"; 547 if (code == ReferralPriority.URGENT) 548 return "urgent"; 549 if (code == ReferralPriority.ASAP) 550 return "asap"; 551 if (code == ReferralPriority.STAT) 552 return "stat"; 553 return "?"; 554 } 555 public String toSystem(ReferralPriority code) { 556 return code.getSystem(); 557 } 558 } 559 560 @Block() 561 public static class ReferralRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 562 /** 563 * The device, practitioner, etc. who initiated the request. 564 */ 565 @Child(name = "agent", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 566 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 567 protected Reference agent; 568 569 /** 570 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the request.) 571 */ 572 protected Resource agentTarget; 573 574 /** 575 * The organization the device or practitioner was acting on behalf of. 576 */ 577 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 578 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 579 protected Reference onBehalfOf; 580 581 /** 582 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 583 */ 584 protected Organization onBehalfOfTarget; 585 586 private static final long serialVersionUID = -71453027L; 587 588 /** 589 * Constructor 590 */ 591 public ReferralRequestRequesterComponent() { 592 super(); 593 } 594 595 /** 596 * Constructor 597 */ 598 public ReferralRequestRequesterComponent(Reference agent) { 599 super(); 600 this.agent = agent; 601 } 602 603 /** 604 * @return {@link #agent} (The device, practitioner, etc. who initiated the request.) 605 */ 606 public Reference getAgent() { 607 if (this.agent == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create ReferralRequestRequesterComponent.agent"); 610 else if (Configuration.doAutoCreate()) 611 this.agent = new Reference(); // cc 612 return this.agent; 613 } 614 615 public boolean hasAgent() { 616 return this.agent != null && !this.agent.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #agent} (The device, practitioner, etc. who initiated the request.) 621 */ 622 public ReferralRequestRequesterComponent setAgent(Reference value) { 623 this.agent = value; 624 return this; 625 } 626 627 /** 628 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 629 */ 630 public Resource getAgentTarget() { 631 return this.agentTarget; 632 } 633 634 /** 635 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 636 */ 637 public ReferralRequestRequesterComponent setAgentTarget(Resource value) { 638 this.agentTarget = value; 639 return this; 640 } 641 642 /** 643 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 644 */ 645 public Reference getOnBehalfOf() { 646 if (this.onBehalfOf == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create ReferralRequestRequesterComponent.onBehalfOf"); 649 else if (Configuration.doAutoCreate()) 650 this.onBehalfOf = new Reference(); // cc 651 return this.onBehalfOf; 652 } 653 654 public boolean hasOnBehalfOf() { 655 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 660 */ 661 public ReferralRequestRequesterComponent setOnBehalfOf(Reference value) { 662 this.onBehalfOf = value; 663 return this; 664 } 665 666 /** 667 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 668 */ 669 public Organization getOnBehalfOfTarget() { 670 if (this.onBehalfOfTarget == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create ReferralRequestRequesterComponent.onBehalfOf"); 673 else if (Configuration.doAutoCreate()) 674 this.onBehalfOfTarget = new Organization(); // aa 675 return this.onBehalfOfTarget; 676 } 677 678 /** 679 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 680 */ 681 public ReferralRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 682 this.onBehalfOfTarget = value; 683 return this; 684 } 685 686 protected void listChildren(List<Property> children) { 687 super.listChildren(children); 688 children.add(new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent)); 689 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 690 } 691 692 @Override 693 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 694 switch (_hash) { 695 case 92750597: /*agent*/ return new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent); 696 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 697 default: return super.getNamedProperty(_hash, _name, _checkValid); 698 } 699 700 } 701 702 @Override 703 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 704 switch (hash) { 705 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 706 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 707 default: return super.getProperty(hash, name, checkValid); 708 } 709 710 } 711 712 @Override 713 public Base setProperty(int hash, String name, Base value) throws FHIRException { 714 switch (hash) { 715 case 92750597: // agent 716 this.agent = castToReference(value); // Reference 717 return value; 718 case -14402964: // onBehalfOf 719 this.onBehalfOf = castToReference(value); // Reference 720 return value; 721 default: return super.setProperty(hash, name, value); 722 } 723 724 } 725 726 @Override 727 public Base setProperty(String name, Base value) throws FHIRException { 728 if (name.equals("agent")) { 729 this.agent = castToReference(value); // Reference 730 } else if (name.equals("onBehalfOf")) { 731 this.onBehalfOf = castToReference(value); // Reference 732 } else 733 return super.setProperty(name, value); 734 return value; 735 } 736 737 @Override 738 public Base makeProperty(int hash, String name) throws FHIRException { 739 switch (hash) { 740 case 92750597: return getAgent(); 741 case -14402964: return getOnBehalfOf(); 742 default: return super.makeProperty(hash, name); 743 } 744 745 } 746 747 @Override 748 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 749 switch (hash) { 750 case 92750597: /*agent*/ return new String[] {"Reference"}; 751 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 752 default: return super.getTypesForProperty(hash, name); 753 } 754 755 } 756 757 @Override 758 public Base addChild(String name) throws FHIRException { 759 if (name.equals("agent")) { 760 this.agent = new Reference(); 761 return this.agent; 762 } 763 else if (name.equals("onBehalfOf")) { 764 this.onBehalfOf = new Reference(); 765 return this.onBehalfOf; 766 } 767 else 768 return super.addChild(name); 769 } 770 771 public ReferralRequestRequesterComponent copy() { 772 ReferralRequestRequesterComponent dst = new ReferralRequestRequesterComponent(); 773 copyValues(dst); 774 dst.agent = agent == null ? null : agent.copy(); 775 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 776 return dst; 777 } 778 779 @Override 780 public boolean equalsDeep(Base other_) { 781 if (!super.equalsDeep(other_)) 782 return false; 783 if (!(other_ instanceof ReferralRequestRequesterComponent)) 784 return false; 785 ReferralRequestRequesterComponent o = (ReferralRequestRequesterComponent) other_; 786 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 787 } 788 789 @Override 790 public boolean equalsShallow(Base other_) { 791 if (!super.equalsShallow(other_)) 792 return false; 793 if (!(other_ instanceof ReferralRequestRequesterComponent)) 794 return false; 795 ReferralRequestRequesterComponent o = (ReferralRequestRequesterComponent) other_; 796 return true; 797 } 798 799 public boolean isEmpty() { 800 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 801 } 802 803 public String fhirType() { 804 return "ReferralRequest.requester"; 805 806 } 807 808 } 809 810 /** 811 * Business identifier that uniquely identifies the referral/care transfer request instance. 812 */ 813 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 814 @Description(shortDefinition="Business identifier", formalDefinition="Business identifier that uniquely identifies the referral/care transfer request instance." ) 815 protected List<Identifier> identifier; 816 817 /** 818 * A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request. 819 */ 820 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 821 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request." ) 822 protected List<Reference> definition; 823 /** 824 * The actual objects that are the target of the reference (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 825 */ 826 protected List<Resource> definitionTarget; 827 828 829 /** 830 * Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part. 831 */ 832 @Child(name = "basedOn", type = {ReferralRequest.class, CarePlan.class, ProcedureRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 833 @Description(shortDefinition="Request fulfilled by this request", formalDefinition="Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part." ) 834 protected List<Reference> basedOn; 835 /** 836 * The actual objects that are the target of the reference (Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part.) 837 */ 838 protected List<Resource> basedOnTarget; 839 840 841 /** 842 * Completed or terminated request(s) whose function is taken by this new request. 843 */ 844 @Child(name = "replaces", type = {ReferralRequest.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 845 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 846 protected List<Reference> replaces; 847 /** 848 * The actual objects that are the target of the reference (Completed or terminated request(s) whose function is taken by this new request.) 849 */ 850 protected List<ReferralRequest> replacesTarget; 851 852 853 /** 854 * The business identifier of the logical "grouping" request/order that this referral is a part of. 855 */ 856 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 857 @Description(shortDefinition="Composite request this is part of", formalDefinition="The business identifier of the logical \"grouping\" request/order that this referral is a part of." ) 858 protected Identifier groupIdentifier; 859 860 /** 861 * The status of the authorization/intention reflected by the referral request record. 862 */ 863 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 864 @Description(shortDefinition="draft | active | suspended | cancelled | completed | entered-in-error | unknown", formalDefinition="The status of the authorization/intention reflected by the referral request record." ) 865 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 866 protected Enumeration<ReferralRequestStatus> status; 867 868 /** 869 * Distinguishes the "level" of authorization/demand implicit in this request. 870 */ 871 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 872 @Description(shortDefinition="proposal | plan | order", formalDefinition="Distinguishes the \"level\" of authorization/demand implicit in this request." ) 873 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 874 protected Enumeration<ReferralCategory> intent; 875 876 /** 877 * An indication of the type of referral (or where applicable the type of transfer of care) request. 878 */ 879 @Child(name = "type", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 880 @Description(shortDefinition="Referral/Transition of care request type", formalDefinition="An indication of the type of referral (or where applicable the type of transfer of care) request." ) 881 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referral-type") 882 protected CodeableConcept type; 883 884 /** 885 * An indication of the urgency of referral (or where applicable the type of transfer of care) request. 886 */ 887 @Child(name = "priority", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 888 @Description(shortDefinition="Urgency of referral / transfer of care request", formalDefinition="An indication of the urgency of referral (or where applicable the type of transfer of care) request." ) 889 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 890 protected Enumeration<ReferralPriority> priority; 891 892 /** 893 * The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion. 894 */ 895 @Child(name = "serviceRequested", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 896 @Description(shortDefinition="Actions requested as part of the referral", formalDefinition="The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion." ) 897 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 898 protected List<CodeableConcept> serviceRequested; 899 900 /** 901 * The patient who is the subject of a referral or transfer of care request. 902 */ 903 @Child(name = "subject", type = {Patient.class, Group.class}, order=10, min=1, max=1, modifier=false, summary=true) 904 @Description(shortDefinition="Patient referred to care or transfer", formalDefinition="The patient who is the subject of a referral or transfer of care request." ) 905 protected Reference subject; 906 907 /** 908 * The actual object that is the target of the reference (The patient who is the subject of a referral or transfer of care request.) 909 */ 910 protected Resource subjectTarget; 911 912 /** 913 * The encounter at which the request for referral or transfer of care is initiated. 914 */ 915 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=11, min=0, max=1, modifier=false, summary=true) 916 @Description(shortDefinition="Originating encounter", formalDefinition="The encounter at which the request for referral or transfer of care is initiated." ) 917 protected Reference context; 918 919 /** 920 * The actual object that is the target of the reference (The encounter at which the request for referral or transfer of care is initiated.) 921 */ 922 protected Resource contextTarget; 923 924 /** 925 * The period of time within which the services identified in the referral/transfer of care is specified or required to occur. 926 */ 927 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=12, min=0, max=1, modifier=false, summary=true) 928 @Description(shortDefinition="When the service(s) requested in the referral should occur", formalDefinition="The period of time within which the services identified in the referral/transfer of care is specified or required to occur." ) 929 protected Type occurrence; 930 931 /** 932 * Date/DateTime of creation for draft requests and date of activation for active requests. 933 */ 934 @Child(name = "authoredOn", type = {DateTimeType.class}, order=13, min=0, max=1, modifier=false, summary=true) 935 @Description(shortDefinition="Date of creation/activation", formalDefinition="Date/DateTime of creation for draft requests and date of activation for active requests." ) 936 protected DateTimeType authoredOn; 937 938 /** 939 * The individual who initiated the request and has responsibility for its activation. 940 */ 941 @Child(name = "requester", type = {}, order=14, min=0, max=1, modifier=false, summary=true) 942 @Description(shortDefinition="Who/what is requesting service", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 943 protected ReferralRequestRequesterComponent requester; 944 945 /** 946 * Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology. 947 */ 948 @Child(name = "specialty", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="The clinical specialty (discipline) that the referral is requested for", formalDefinition="Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology." ) 950 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/practitioner-specialty") 951 protected CodeableConcept specialty; 952 953 /** 954 * The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request. 955 */ 956 @Child(name = "recipient", type = {Practitioner.class, Organization.class, HealthcareService.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 957 @Description(shortDefinition="Receiver of referral / transfer of care request", formalDefinition="The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request." ) 958 protected List<Reference> recipient; 959 /** 960 * The actual objects that are the target of the reference (The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.) 961 */ 962 protected List<Resource> recipientTarget; 963 964 965 /** 966 * Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management. 967 */ 968 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 969 @Description(shortDefinition="Reason for referral / transfer of care request", formalDefinition="Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management." ) 970 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 971 protected List<CodeableConcept> reasonCode; 972 973 /** 974 * Indicates another resource whose existence justifies this request. 975 */ 976 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 977 @Description(shortDefinition="Why is service needed?", formalDefinition="Indicates another resource whose existence justifies this request." ) 978 protected List<Reference> reasonReference; 979 /** 980 * The actual objects that are the target of the reference (Indicates another resource whose existence justifies this request.) 981 */ 982 protected List<Resource> reasonReferenceTarget; 983 984 985 /** 986 * The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary. 987 */ 988 @Child(name = "description", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 989 @Description(shortDefinition="A textual description of the referral", formalDefinition="The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary." ) 990 protected StringType description; 991 992 /** 993 * Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan. 994 */ 995 @Child(name = "supportingInfo", type = {Reference.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 996 @Description(shortDefinition="Additonal information to support referral or transfer of care request", formalDefinition="Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan." ) 997 protected List<Reference> supportingInfo; 998 /** 999 * The actual objects that are the target of the reference (Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.) 1000 */ 1001 protected List<Resource> supportingInfoTarget; 1002 1003 1004 /** 1005 * Comments made about the referral request by any of the participants. 1006 */ 1007 @Child(name = "note", type = {Annotation.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1008 @Description(shortDefinition="Comments made about referral request", formalDefinition="Comments made about the referral request by any of the participants." ) 1009 protected List<Annotation> note; 1010 1011 /** 1012 * Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource. 1013 */ 1014 @Child(name = "relevantHistory", type = {Provenance.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1015 @Description(shortDefinition="Key events in history of request", formalDefinition="Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource." ) 1016 protected List<Reference> relevantHistory; 1017 /** 1018 * The actual objects that are the target of the reference (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 1019 */ 1020 protected List<Provenance> relevantHistoryTarget; 1021 1022 1023 private static final long serialVersionUID = -404424161L; 1024 1025 /** 1026 * Constructor 1027 */ 1028 public ReferralRequest() { 1029 super(); 1030 } 1031 1032 /** 1033 * Constructor 1034 */ 1035 public ReferralRequest(Enumeration<ReferralRequestStatus> status, Enumeration<ReferralCategory> intent, Reference subject) { 1036 super(); 1037 this.status = status; 1038 this.intent = intent; 1039 this.subject = subject; 1040 } 1041 1042 /** 1043 * @return {@link #identifier} (Business identifier that uniquely identifies the referral/care transfer request instance.) 1044 */ 1045 public List<Identifier> getIdentifier() { 1046 if (this.identifier == null) 1047 this.identifier = new ArrayList<Identifier>(); 1048 return this.identifier; 1049 } 1050 1051 /** 1052 * @return Returns a reference to <code>this</code> for easy method chaining 1053 */ 1054 public ReferralRequest setIdentifier(List<Identifier> theIdentifier) { 1055 this.identifier = theIdentifier; 1056 return this; 1057 } 1058 1059 public boolean hasIdentifier() { 1060 if (this.identifier == null) 1061 return false; 1062 for (Identifier item : this.identifier) 1063 if (!item.isEmpty()) 1064 return true; 1065 return false; 1066 } 1067 1068 public Identifier addIdentifier() { //3 1069 Identifier t = new Identifier(); 1070 if (this.identifier == null) 1071 this.identifier = new ArrayList<Identifier>(); 1072 this.identifier.add(t); 1073 return t; 1074 } 1075 1076 public ReferralRequest addIdentifier(Identifier t) { //3 1077 if (t == null) 1078 return this; 1079 if (this.identifier == null) 1080 this.identifier = new ArrayList<Identifier>(); 1081 this.identifier.add(t); 1082 return this; 1083 } 1084 1085 /** 1086 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1087 */ 1088 public Identifier getIdentifierFirstRep() { 1089 if (getIdentifier().isEmpty()) { 1090 addIdentifier(); 1091 } 1092 return getIdentifier().get(0); 1093 } 1094 1095 /** 1096 * @return {@link #definition} (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 1097 */ 1098 public List<Reference> getDefinition() { 1099 if (this.definition == null) 1100 this.definition = new ArrayList<Reference>(); 1101 return this.definition; 1102 } 1103 1104 /** 1105 * @return Returns a reference to <code>this</code> for easy method chaining 1106 */ 1107 public ReferralRequest setDefinition(List<Reference> theDefinition) { 1108 this.definition = theDefinition; 1109 return this; 1110 } 1111 1112 public boolean hasDefinition() { 1113 if (this.definition == null) 1114 return false; 1115 for (Reference item : this.definition) 1116 if (!item.isEmpty()) 1117 return true; 1118 return false; 1119 } 1120 1121 public Reference addDefinition() { //3 1122 Reference t = new Reference(); 1123 if (this.definition == null) 1124 this.definition = new ArrayList<Reference>(); 1125 this.definition.add(t); 1126 return t; 1127 } 1128 1129 public ReferralRequest addDefinition(Reference t) { //3 1130 if (t == null) 1131 return this; 1132 if (this.definition == null) 1133 this.definition = new ArrayList<Reference>(); 1134 this.definition.add(t); 1135 return this; 1136 } 1137 1138 /** 1139 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1140 */ 1141 public Reference getDefinitionFirstRep() { 1142 if (getDefinition().isEmpty()) { 1143 addDefinition(); 1144 } 1145 return getDefinition().get(0); 1146 } 1147 1148 /** 1149 * @deprecated Use Reference#setResource(IBaseResource) instead 1150 */ 1151 @Deprecated 1152 public List<Resource> getDefinitionTarget() { 1153 if (this.definitionTarget == null) 1154 this.definitionTarget = new ArrayList<Resource>(); 1155 return this.definitionTarget; 1156 } 1157 1158 /** 1159 * @return {@link #basedOn} (Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part.) 1160 */ 1161 public List<Reference> getBasedOn() { 1162 if (this.basedOn == null) 1163 this.basedOn = new ArrayList<Reference>(); 1164 return this.basedOn; 1165 } 1166 1167 /** 1168 * @return Returns a reference to <code>this</code> for easy method chaining 1169 */ 1170 public ReferralRequest setBasedOn(List<Reference> theBasedOn) { 1171 this.basedOn = theBasedOn; 1172 return this; 1173 } 1174 1175 public boolean hasBasedOn() { 1176 if (this.basedOn == null) 1177 return false; 1178 for (Reference item : this.basedOn) 1179 if (!item.isEmpty()) 1180 return true; 1181 return false; 1182 } 1183 1184 public Reference addBasedOn() { //3 1185 Reference t = new Reference(); 1186 if (this.basedOn == null) 1187 this.basedOn = new ArrayList<Reference>(); 1188 this.basedOn.add(t); 1189 return t; 1190 } 1191 1192 public ReferralRequest addBasedOn(Reference t) { //3 1193 if (t == null) 1194 return this; 1195 if (this.basedOn == null) 1196 this.basedOn = new ArrayList<Reference>(); 1197 this.basedOn.add(t); 1198 return this; 1199 } 1200 1201 /** 1202 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1203 */ 1204 public Reference getBasedOnFirstRep() { 1205 if (getBasedOn().isEmpty()) { 1206 addBasedOn(); 1207 } 1208 return getBasedOn().get(0); 1209 } 1210 1211 /** 1212 * @deprecated Use Reference#setResource(IBaseResource) instead 1213 */ 1214 @Deprecated 1215 public List<Resource> getBasedOnTarget() { 1216 if (this.basedOnTarget == null) 1217 this.basedOnTarget = new ArrayList<Resource>(); 1218 return this.basedOnTarget; 1219 } 1220 1221 /** 1222 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 1223 */ 1224 public List<Reference> getReplaces() { 1225 if (this.replaces == null) 1226 this.replaces = new ArrayList<Reference>(); 1227 return this.replaces; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public ReferralRequest setReplaces(List<Reference> theReplaces) { 1234 this.replaces = theReplaces; 1235 return this; 1236 } 1237 1238 public boolean hasReplaces() { 1239 if (this.replaces == null) 1240 return false; 1241 for (Reference item : this.replaces) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 public Reference addReplaces() { //3 1248 Reference t = new Reference(); 1249 if (this.replaces == null) 1250 this.replaces = new ArrayList<Reference>(); 1251 this.replaces.add(t); 1252 return t; 1253 } 1254 1255 public ReferralRequest addReplaces(Reference t) { //3 1256 if (t == null) 1257 return this; 1258 if (this.replaces == null) 1259 this.replaces = new ArrayList<Reference>(); 1260 this.replaces.add(t); 1261 return this; 1262 } 1263 1264 /** 1265 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 1266 */ 1267 public Reference getReplacesFirstRep() { 1268 if (getReplaces().isEmpty()) { 1269 addReplaces(); 1270 } 1271 return getReplaces().get(0); 1272 } 1273 1274 /** 1275 * @deprecated Use Reference#setResource(IBaseResource) instead 1276 */ 1277 @Deprecated 1278 public List<ReferralRequest> getReplacesTarget() { 1279 if (this.replacesTarget == null) 1280 this.replacesTarget = new ArrayList<ReferralRequest>(); 1281 return this.replacesTarget; 1282 } 1283 1284 /** 1285 * @deprecated Use Reference#setResource(IBaseResource) instead 1286 */ 1287 @Deprecated 1288 public ReferralRequest addReplacesTarget() { 1289 ReferralRequest r = new ReferralRequest(); 1290 if (this.replacesTarget == null) 1291 this.replacesTarget = new ArrayList<ReferralRequest>(); 1292 this.replacesTarget.add(r); 1293 return r; 1294 } 1295 1296 /** 1297 * @return {@link #groupIdentifier} (The business identifier of the logical "grouping" request/order that this referral is a part of.) 1298 */ 1299 public Identifier getGroupIdentifier() { 1300 if (this.groupIdentifier == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create ReferralRequest.groupIdentifier"); 1303 else if (Configuration.doAutoCreate()) 1304 this.groupIdentifier = new Identifier(); // cc 1305 return this.groupIdentifier; 1306 } 1307 1308 public boolean hasGroupIdentifier() { 1309 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1310 } 1311 1312 /** 1313 * @param value {@link #groupIdentifier} (The business identifier of the logical "grouping" request/order that this referral is a part of.) 1314 */ 1315 public ReferralRequest setGroupIdentifier(Identifier value) { 1316 this.groupIdentifier = value; 1317 return this; 1318 } 1319 1320 /** 1321 * @return {@link #status} (The status of the authorization/intention reflected by the referral request record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1322 */ 1323 public Enumeration<ReferralRequestStatus> getStatusElement() { 1324 if (this.status == null) 1325 if (Configuration.errorOnAutoCreate()) 1326 throw new Error("Attempt to auto-create ReferralRequest.status"); 1327 else if (Configuration.doAutoCreate()) 1328 this.status = new Enumeration<ReferralRequestStatus>(new ReferralRequestStatusEnumFactory()); // bb 1329 return this.status; 1330 } 1331 1332 public boolean hasStatusElement() { 1333 return this.status != null && !this.status.isEmpty(); 1334 } 1335 1336 public boolean hasStatus() { 1337 return this.status != null && !this.status.isEmpty(); 1338 } 1339 1340 /** 1341 * @param value {@link #status} (The status of the authorization/intention reflected by the referral request record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1342 */ 1343 public ReferralRequest setStatusElement(Enumeration<ReferralRequestStatus> value) { 1344 this.status = value; 1345 return this; 1346 } 1347 1348 /** 1349 * @return The status of the authorization/intention reflected by the referral request record. 1350 */ 1351 public ReferralRequestStatus getStatus() { 1352 return this.status == null ? null : this.status.getValue(); 1353 } 1354 1355 /** 1356 * @param value The status of the authorization/intention reflected by the referral request record. 1357 */ 1358 public ReferralRequest setStatus(ReferralRequestStatus value) { 1359 if (this.status == null) 1360 this.status = new Enumeration<ReferralRequestStatus>(new ReferralRequestStatusEnumFactory()); 1361 this.status.setValue(value); 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #intent} (Distinguishes the "level" of authorization/demand implicit in this request.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1367 */ 1368 public Enumeration<ReferralCategory> getIntentElement() { 1369 if (this.intent == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create ReferralRequest.intent"); 1372 else if (Configuration.doAutoCreate()) 1373 this.intent = new Enumeration<ReferralCategory>(new ReferralCategoryEnumFactory()); // bb 1374 return this.intent; 1375 } 1376 1377 public boolean hasIntentElement() { 1378 return this.intent != null && !this.intent.isEmpty(); 1379 } 1380 1381 public boolean hasIntent() { 1382 return this.intent != null && !this.intent.isEmpty(); 1383 } 1384 1385 /** 1386 * @param value {@link #intent} (Distinguishes the "level" of authorization/demand implicit in this request.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1387 */ 1388 public ReferralRequest setIntentElement(Enumeration<ReferralCategory> value) { 1389 this.intent = value; 1390 return this; 1391 } 1392 1393 /** 1394 * @return Distinguishes the "level" of authorization/demand implicit in this request. 1395 */ 1396 public ReferralCategory getIntent() { 1397 return this.intent == null ? null : this.intent.getValue(); 1398 } 1399 1400 /** 1401 * @param value Distinguishes the "level" of authorization/demand implicit in this request. 1402 */ 1403 public ReferralRequest setIntent(ReferralCategory value) { 1404 if (this.intent == null) 1405 this.intent = new Enumeration<ReferralCategory>(new ReferralCategoryEnumFactory()); 1406 this.intent.setValue(value); 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #type} (An indication of the type of referral (or where applicable the type of transfer of care) request.) 1412 */ 1413 public CodeableConcept getType() { 1414 if (this.type == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create ReferralRequest.type"); 1417 else if (Configuration.doAutoCreate()) 1418 this.type = new CodeableConcept(); // cc 1419 return this.type; 1420 } 1421 1422 public boolean hasType() { 1423 return this.type != null && !this.type.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #type} (An indication of the type of referral (or where applicable the type of transfer of care) request.) 1428 */ 1429 public ReferralRequest setType(CodeableConcept value) { 1430 this.type = value; 1431 return this; 1432 } 1433 1434 /** 1435 * @return {@link #priority} (An indication of the urgency of referral (or where applicable the type of transfer of care) request.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1436 */ 1437 public Enumeration<ReferralPriority> getPriorityElement() { 1438 if (this.priority == null) 1439 if (Configuration.errorOnAutoCreate()) 1440 throw new Error("Attempt to auto-create ReferralRequest.priority"); 1441 else if (Configuration.doAutoCreate()) 1442 this.priority = new Enumeration<ReferralPriority>(new ReferralPriorityEnumFactory()); // bb 1443 return this.priority; 1444 } 1445 1446 public boolean hasPriorityElement() { 1447 return this.priority != null && !this.priority.isEmpty(); 1448 } 1449 1450 public boolean hasPriority() { 1451 return this.priority != null && !this.priority.isEmpty(); 1452 } 1453 1454 /** 1455 * @param value {@link #priority} (An indication of the urgency of referral (or where applicable the type of transfer of care) request.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1456 */ 1457 public ReferralRequest setPriorityElement(Enumeration<ReferralPriority> value) { 1458 this.priority = value; 1459 return this; 1460 } 1461 1462 /** 1463 * @return An indication of the urgency of referral (or where applicable the type of transfer of care) request. 1464 */ 1465 public ReferralPriority getPriority() { 1466 return this.priority == null ? null : this.priority.getValue(); 1467 } 1468 1469 /** 1470 * @param value An indication of the urgency of referral (or where applicable the type of transfer of care) request. 1471 */ 1472 public ReferralRequest setPriority(ReferralPriority value) { 1473 if (value == null) 1474 this.priority = null; 1475 else { 1476 if (this.priority == null) 1477 this.priority = new Enumeration<ReferralPriority>(new ReferralPriorityEnumFactory()); 1478 this.priority.setValue(value); 1479 } 1480 return this; 1481 } 1482 1483 /** 1484 * @return {@link #serviceRequested} (The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion.) 1485 */ 1486 public List<CodeableConcept> getServiceRequested() { 1487 if (this.serviceRequested == null) 1488 this.serviceRequested = new ArrayList<CodeableConcept>(); 1489 return this.serviceRequested; 1490 } 1491 1492 /** 1493 * @return Returns a reference to <code>this</code> for easy method chaining 1494 */ 1495 public ReferralRequest setServiceRequested(List<CodeableConcept> theServiceRequested) { 1496 this.serviceRequested = theServiceRequested; 1497 return this; 1498 } 1499 1500 public boolean hasServiceRequested() { 1501 if (this.serviceRequested == null) 1502 return false; 1503 for (CodeableConcept item : this.serviceRequested) 1504 if (!item.isEmpty()) 1505 return true; 1506 return false; 1507 } 1508 1509 public CodeableConcept addServiceRequested() { //3 1510 CodeableConcept t = new CodeableConcept(); 1511 if (this.serviceRequested == null) 1512 this.serviceRequested = new ArrayList<CodeableConcept>(); 1513 this.serviceRequested.add(t); 1514 return t; 1515 } 1516 1517 public ReferralRequest addServiceRequested(CodeableConcept t) { //3 1518 if (t == null) 1519 return this; 1520 if (this.serviceRequested == null) 1521 this.serviceRequested = new ArrayList<CodeableConcept>(); 1522 this.serviceRequested.add(t); 1523 return this; 1524 } 1525 1526 /** 1527 * @return The first repetition of repeating field {@link #serviceRequested}, creating it if it does not already exist 1528 */ 1529 public CodeableConcept getServiceRequestedFirstRep() { 1530 if (getServiceRequested().isEmpty()) { 1531 addServiceRequested(); 1532 } 1533 return getServiceRequested().get(0); 1534 } 1535 1536 /** 1537 * @return {@link #subject} (The patient who is the subject of a referral or transfer of care request.) 1538 */ 1539 public Reference getSubject() { 1540 if (this.subject == null) 1541 if (Configuration.errorOnAutoCreate()) 1542 throw new Error("Attempt to auto-create ReferralRequest.subject"); 1543 else if (Configuration.doAutoCreate()) 1544 this.subject = new Reference(); // cc 1545 return this.subject; 1546 } 1547 1548 public boolean hasSubject() { 1549 return this.subject != null && !this.subject.isEmpty(); 1550 } 1551 1552 /** 1553 * @param value {@link #subject} (The patient who is the subject of a referral or transfer of care request.) 1554 */ 1555 public ReferralRequest setSubject(Reference value) { 1556 this.subject = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who is the subject of a referral or transfer of care request.) 1562 */ 1563 public Resource getSubjectTarget() { 1564 return this.subjectTarget; 1565 } 1566 1567 /** 1568 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who is the subject of a referral or transfer of care request.) 1569 */ 1570 public ReferralRequest setSubjectTarget(Resource value) { 1571 this.subjectTarget = value; 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #context} (The encounter at which the request for referral or transfer of care is initiated.) 1577 */ 1578 public Reference getContext() { 1579 if (this.context == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create ReferralRequest.context"); 1582 else if (Configuration.doAutoCreate()) 1583 this.context = new Reference(); // cc 1584 return this.context; 1585 } 1586 1587 public boolean hasContext() { 1588 return this.context != null && !this.context.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #context} (The encounter at which the request for referral or transfer of care is initiated.) 1593 */ 1594 public ReferralRequest setContext(Reference value) { 1595 this.context = value; 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter at which the request for referral or transfer of care is initiated.) 1601 */ 1602 public Resource getContextTarget() { 1603 return this.contextTarget; 1604 } 1605 1606 /** 1607 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter at which the request for referral or transfer of care is initiated.) 1608 */ 1609 public ReferralRequest setContextTarget(Resource value) { 1610 this.contextTarget = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return {@link #occurrence} (The period of time within which the services identified in the referral/transfer of care is specified or required to occur.) 1616 */ 1617 public Type getOccurrence() { 1618 return this.occurrence; 1619 } 1620 1621 /** 1622 * @return {@link #occurrence} (The period of time within which the services identified in the referral/transfer of care is specified or required to occur.) 1623 */ 1624 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1625 if (this.occurrence == null) 1626 return null; 1627 if (!(this.occurrence instanceof DateTimeType)) 1628 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1629 return (DateTimeType) this.occurrence; 1630 } 1631 1632 public boolean hasOccurrenceDateTimeType() { 1633 return this.occurrence instanceof DateTimeType; 1634 } 1635 1636 /** 1637 * @return {@link #occurrence} (The period of time within which the services identified in the referral/transfer of care is specified or required to occur.) 1638 */ 1639 public Period getOccurrencePeriod() throws FHIRException { 1640 if (this.occurrence == null) 1641 return null; 1642 if (!(this.occurrence instanceof Period)) 1643 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1644 return (Period) this.occurrence; 1645 } 1646 1647 public boolean hasOccurrencePeriod() { 1648 return this.occurrence instanceof Period; 1649 } 1650 1651 public boolean hasOccurrence() { 1652 return this.occurrence != null && !this.occurrence.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #occurrence} (The period of time within which the services identified in the referral/transfer of care is specified or required to occur.) 1657 */ 1658 public ReferralRequest setOccurrence(Type value) throws FHIRFormatError { 1659 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1660 throw new FHIRFormatError("Not the right type for ReferralRequest.occurrence[x]: "+value.fhirType()); 1661 this.occurrence = value; 1662 return this; 1663 } 1664 1665 /** 1666 * @return {@link #authoredOn} (Date/DateTime of creation for draft requests and date of activation for active requests.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1667 */ 1668 public DateTimeType getAuthoredOnElement() { 1669 if (this.authoredOn == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create ReferralRequest.authoredOn"); 1672 else if (Configuration.doAutoCreate()) 1673 this.authoredOn = new DateTimeType(); // bb 1674 return this.authoredOn; 1675 } 1676 1677 public boolean hasAuthoredOnElement() { 1678 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1679 } 1680 1681 public boolean hasAuthoredOn() { 1682 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1683 } 1684 1685 /** 1686 * @param value {@link #authoredOn} (Date/DateTime of creation for draft requests and date of activation for active requests.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1687 */ 1688 public ReferralRequest setAuthoredOnElement(DateTimeType value) { 1689 this.authoredOn = value; 1690 return this; 1691 } 1692 1693 /** 1694 * @return Date/DateTime of creation for draft requests and date of activation for active requests. 1695 */ 1696 public Date getAuthoredOn() { 1697 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1698 } 1699 1700 /** 1701 * @param value Date/DateTime of creation for draft requests and date of activation for active requests. 1702 */ 1703 public ReferralRequest setAuthoredOn(Date value) { 1704 if (value == null) 1705 this.authoredOn = null; 1706 else { 1707 if (this.authoredOn == null) 1708 this.authoredOn = new DateTimeType(); 1709 this.authoredOn.setValue(value); 1710 } 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1716 */ 1717 public ReferralRequestRequesterComponent getRequester() { 1718 if (this.requester == null) 1719 if (Configuration.errorOnAutoCreate()) 1720 throw new Error("Attempt to auto-create ReferralRequest.requester"); 1721 else if (Configuration.doAutoCreate()) 1722 this.requester = new ReferralRequestRequesterComponent(); // cc 1723 return this.requester; 1724 } 1725 1726 public boolean hasRequester() { 1727 return this.requester != null && !this.requester.isEmpty(); 1728 } 1729 1730 /** 1731 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1732 */ 1733 public ReferralRequest setRequester(ReferralRequestRequesterComponent value) { 1734 this.requester = value; 1735 return this; 1736 } 1737 1738 /** 1739 * @return {@link #specialty} (Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.) 1740 */ 1741 public CodeableConcept getSpecialty() { 1742 if (this.specialty == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create ReferralRequest.specialty"); 1745 else if (Configuration.doAutoCreate()) 1746 this.specialty = new CodeableConcept(); // cc 1747 return this.specialty; 1748 } 1749 1750 public boolean hasSpecialty() { 1751 return this.specialty != null && !this.specialty.isEmpty(); 1752 } 1753 1754 /** 1755 * @param value {@link #specialty} (Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.) 1756 */ 1757 public ReferralRequest setSpecialty(CodeableConcept value) { 1758 this.specialty = value; 1759 return this; 1760 } 1761 1762 /** 1763 * @return {@link #recipient} (The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.) 1764 */ 1765 public List<Reference> getRecipient() { 1766 if (this.recipient == null) 1767 this.recipient = new ArrayList<Reference>(); 1768 return this.recipient; 1769 } 1770 1771 /** 1772 * @return Returns a reference to <code>this</code> for easy method chaining 1773 */ 1774 public ReferralRequest setRecipient(List<Reference> theRecipient) { 1775 this.recipient = theRecipient; 1776 return this; 1777 } 1778 1779 public boolean hasRecipient() { 1780 if (this.recipient == null) 1781 return false; 1782 for (Reference item : this.recipient) 1783 if (!item.isEmpty()) 1784 return true; 1785 return false; 1786 } 1787 1788 public Reference addRecipient() { //3 1789 Reference t = new Reference(); 1790 if (this.recipient == null) 1791 this.recipient = new ArrayList<Reference>(); 1792 this.recipient.add(t); 1793 return t; 1794 } 1795 1796 public ReferralRequest addRecipient(Reference t) { //3 1797 if (t == null) 1798 return this; 1799 if (this.recipient == null) 1800 this.recipient = new ArrayList<Reference>(); 1801 this.recipient.add(t); 1802 return this; 1803 } 1804 1805 /** 1806 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 1807 */ 1808 public Reference getRecipientFirstRep() { 1809 if (getRecipient().isEmpty()) { 1810 addRecipient(); 1811 } 1812 return getRecipient().get(0); 1813 } 1814 1815 /** 1816 * @deprecated Use Reference#setResource(IBaseResource) instead 1817 */ 1818 @Deprecated 1819 public List<Resource> getRecipientTarget() { 1820 if (this.recipientTarget == null) 1821 this.recipientTarget = new ArrayList<Resource>(); 1822 return this.recipientTarget; 1823 } 1824 1825 /** 1826 * @return {@link #reasonCode} (Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management.) 1827 */ 1828 public List<CodeableConcept> getReasonCode() { 1829 if (this.reasonCode == null) 1830 this.reasonCode = new ArrayList<CodeableConcept>(); 1831 return this.reasonCode; 1832 } 1833 1834 /** 1835 * @return Returns a reference to <code>this</code> for easy method chaining 1836 */ 1837 public ReferralRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1838 this.reasonCode = theReasonCode; 1839 return this; 1840 } 1841 1842 public boolean hasReasonCode() { 1843 if (this.reasonCode == null) 1844 return false; 1845 for (CodeableConcept item : this.reasonCode) 1846 if (!item.isEmpty()) 1847 return true; 1848 return false; 1849 } 1850 1851 public CodeableConcept addReasonCode() { //3 1852 CodeableConcept t = new CodeableConcept(); 1853 if (this.reasonCode == null) 1854 this.reasonCode = new ArrayList<CodeableConcept>(); 1855 this.reasonCode.add(t); 1856 return t; 1857 } 1858 1859 public ReferralRequest addReasonCode(CodeableConcept t) { //3 1860 if (t == null) 1861 return this; 1862 if (this.reasonCode == null) 1863 this.reasonCode = new ArrayList<CodeableConcept>(); 1864 this.reasonCode.add(t); 1865 return this; 1866 } 1867 1868 /** 1869 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1870 */ 1871 public CodeableConcept getReasonCodeFirstRep() { 1872 if (getReasonCode().isEmpty()) { 1873 addReasonCode(); 1874 } 1875 return getReasonCode().get(0); 1876 } 1877 1878 /** 1879 * @return {@link #reasonReference} (Indicates another resource whose existence justifies this request.) 1880 */ 1881 public List<Reference> getReasonReference() { 1882 if (this.reasonReference == null) 1883 this.reasonReference = new ArrayList<Reference>(); 1884 return this.reasonReference; 1885 } 1886 1887 /** 1888 * @return Returns a reference to <code>this</code> for easy method chaining 1889 */ 1890 public ReferralRequest setReasonReference(List<Reference> theReasonReference) { 1891 this.reasonReference = theReasonReference; 1892 return this; 1893 } 1894 1895 public boolean hasReasonReference() { 1896 if (this.reasonReference == null) 1897 return false; 1898 for (Reference item : this.reasonReference) 1899 if (!item.isEmpty()) 1900 return true; 1901 return false; 1902 } 1903 1904 public Reference addReasonReference() { //3 1905 Reference t = new Reference(); 1906 if (this.reasonReference == null) 1907 this.reasonReference = new ArrayList<Reference>(); 1908 this.reasonReference.add(t); 1909 return t; 1910 } 1911 1912 public ReferralRequest addReasonReference(Reference t) { //3 1913 if (t == null) 1914 return this; 1915 if (this.reasonReference == null) 1916 this.reasonReference = new ArrayList<Reference>(); 1917 this.reasonReference.add(t); 1918 return this; 1919 } 1920 1921 /** 1922 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1923 */ 1924 public Reference getReasonReferenceFirstRep() { 1925 if (getReasonReference().isEmpty()) { 1926 addReasonReference(); 1927 } 1928 return getReasonReference().get(0); 1929 } 1930 1931 /** 1932 * @deprecated Use Reference#setResource(IBaseResource) instead 1933 */ 1934 @Deprecated 1935 public List<Resource> getReasonReferenceTarget() { 1936 if (this.reasonReferenceTarget == null) 1937 this.reasonReferenceTarget = new ArrayList<Resource>(); 1938 return this.reasonReferenceTarget; 1939 } 1940 1941 /** 1942 * @return {@link #description} (The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1943 */ 1944 public StringType getDescriptionElement() { 1945 if (this.description == null) 1946 if (Configuration.errorOnAutoCreate()) 1947 throw new Error("Attempt to auto-create ReferralRequest.description"); 1948 else if (Configuration.doAutoCreate()) 1949 this.description = new StringType(); // bb 1950 return this.description; 1951 } 1952 1953 public boolean hasDescriptionElement() { 1954 return this.description != null && !this.description.isEmpty(); 1955 } 1956 1957 public boolean hasDescription() { 1958 return this.description != null && !this.description.isEmpty(); 1959 } 1960 1961 /** 1962 * @param value {@link #description} (The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1963 */ 1964 public ReferralRequest setDescriptionElement(StringType value) { 1965 this.description = value; 1966 return this; 1967 } 1968 1969 /** 1970 * @return The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary. 1971 */ 1972 public String getDescription() { 1973 return this.description == null ? null : this.description.getValue(); 1974 } 1975 1976 /** 1977 * @param value The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary. 1978 */ 1979 public ReferralRequest setDescription(String value) { 1980 if (Utilities.noString(value)) 1981 this.description = null; 1982 else { 1983 if (this.description == null) 1984 this.description = new StringType(); 1985 this.description.setValue(value); 1986 } 1987 return this; 1988 } 1989 1990 /** 1991 * @return {@link #supportingInfo} (Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.) 1992 */ 1993 public List<Reference> getSupportingInfo() { 1994 if (this.supportingInfo == null) 1995 this.supportingInfo = new ArrayList<Reference>(); 1996 return this.supportingInfo; 1997 } 1998 1999 /** 2000 * @return Returns a reference to <code>this</code> for easy method chaining 2001 */ 2002 public ReferralRequest setSupportingInfo(List<Reference> theSupportingInfo) { 2003 this.supportingInfo = theSupportingInfo; 2004 return this; 2005 } 2006 2007 public boolean hasSupportingInfo() { 2008 if (this.supportingInfo == null) 2009 return false; 2010 for (Reference item : this.supportingInfo) 2011 if (!item.isEmpty()) 2012 return true; 2013 return false; 2014 } 2015 2016 public Reference addSupportingInfo() { //3 2017 Reference t = new Reference(); 2018 if (this.supportingInfo == null) 2019 this.supportingInfo = new ArrayList<Reference>(); 2020 this.supportingInfo.add(t); 2021 return t; 2022 } 2023 2024 public ReferralRequest addSupportingInfo(Reference t) { //3 2025 if (t == null) 2026 return this; 2027 if (this.supportingInfo == null) 2028 this.supportingInfo = new ArrayList<Reference>(); 2029 this.supportingInfo.add(t); 2030 return this; 2031 } 2032 2033 /** 2034 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist 2035 */ 2036 public Reference getSupportingInfoFirstRep() { 2037 if (getSupportingInfo().isEmpty()) { 2038 addSupportingInfo(); 2039 } 2040 return getSupportingInfo().get(0); 2041 } 2042 2043 /** 2044 * @deprecated Use Reference#setResource(IBaseResource) instead 2045 */ 2046 @Deprecated 2047 public List<Resource> getSupportingInfoTarget() { 2048 if (this.supportingInfoTarget == null) 2049 this.supportingInfoTarget = new ArrayList<Resource>(); 2050 return this.supportingInfoTarget; 2051 } 2052 2053 /** 2054 * @return {@link #note} (Comments made about the referral request by any of the participants.) 2055 */ 2056 public List<Annotation> getNote() { 2057 if (this.note == null) 2058 this.note = new ArrayList<Annotation>(); 2059 return this.note; 2060 } 2061 2062 /** 2063 * @return Returns a reference to <code>this</code> for easy method chaining 2064 */ 2065 public ReferralRequest setNote(List<Annotation> theNote) { 2066 this.note = theNote; 2067 return this; 2068 } 2069 2070 public boolean hasNote() { 2071 if (this.note == null) 2072 return false; 2073 for (Annotation item : this.note) 2074 if (!item.isEmpty()) 2075 return true; 2076 return false; 2077 } 2078 2079 public Annotation addNote() { //3 2080 Annotation t = new Annotation(); 2081 if (this.note == null) 2082 this.note = new ArrayList<Annotation>(); 2083 this.note.add(t); 2084 return t; 2085 } 2086 2087 public ReferralRequest addNote(Annotation t) { //3 2088 if (t == null) 2089 return this; 2090 if (this.note == null) 2091 this.note = new ArrayList<Annotation>(); 2092 this.note.add(t); 2093 return this; 2094 } 2095 2096 /** 2097 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2098 */ 2099 public Annotation getNoteFirstRep() { 2100 if (getNote().isEmpty()) { 2101 addNote(); 2102 } 2103 return getNote().get(0); 2104 } 2105 2106 /** 2107 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 2108 */ 2109 public List<Reference> getRelevantHistory() { 2110 if (this.relevantHistory == null) 2111 this.relevantHistory = new ArrayList<Reference>(); 2112 return this.relevantHistory; 2113 } 2114 2115 /** 2116 * @return Returns a reference to <code>this</code> for easy method chaining 2117 */ 2118 public ReferralRequest setRelevantHistory(List<Reference> theRelevantHistory) { 2119 this.relevantHistory = theRelevantHistory; 2120 return this; 2121 } 2122 2123 public boolean hasRelevantHistory() { 2124 if (this.relevantHistory == null) 2125 return false; 2126 for (Reference item : this.relevantHistory) 2127 if (!item.isEmpty()) 2128 return true; 2129 return false; 2130 } 2131 2132 public Reference addRelevantHistory() { //3 2133 Reference t = new Reference(); 2134 if (this.relevantHistory == null) 2135 this.relevantHistory = new ArrayList<Reference>(); 2136 this.relevantHistory.add(t); 2137 return t; 2138 } 2139 2140 public ReferralRequest addRelevantHistory(Reference t) { //3 2141 if (t == null) 2142 return this; 2143 if (this.relevantHistory == null) 2144 this.relevantHistory = new ArrayList<Reference>(); 2145 this.relevantHistory.add(t); 2146 return this; 2147 } 2148 2149 /** 2150 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 2151 */ 2152 public Reference getRelevantHistoryFirstRep() { 2153 if (getRelevantHistory().isEmpty()) { 2154 addRelevantHistory(); 2155 } 2156 return getRelevantHistory().get(0); 2157 } 2158 2159 /** 2160 * @deprecated Use Reference#setResource(IBaseResource) instead 2161 */ 2162 @Deprecated 2163 public List<Provenance> getRelevantHistoryTarget() { 2164 if (this.relevantHistoryTarget == null) 2165 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2166 return this.relevantHistoryTarget; 2167 } 2168 2169 /** 2170 * @deprecated Use Reference#setResource(IBaseResource) instead 2171 */ 2172 @Deprecated 2173 public Provenance addRelevantHistoryTarget() { 2174 Provenance r = new Provenance(); 2175 if (this.relevantHistoryTarget == null) 2176 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2177 this.relevantHistoryTarget.add(r); 2178 return r; 2179 } 2180 2181 protected void listChildren(List<Property> children) { 2182 super.listChildren(children); 2183 children.add(new Property("identifier", "Identifier", "Business identifier that uniquely identifies the referral/care transfer request instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2184 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 2185 children.add(new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2186 children.add(new Property("replaces", "Reference(ReferralRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2187 children.add(new Property("groupIdentifier", "Identifier", "The business identifier of the logical \"grouping\" request/order that this referral is a part of.", 0, 1, groupIdentifier)); 2188 children.add(new Property("status", "code", "The status of the authorization/intention reflected by the referral request record.", 0, 1, status)); 2189 children.add(new Property("intent", "code", "Distinguishes the \"level\" of authorization/demand implicit in this request.", 0, 1, intent)); 2190 children.add(new Property("type", "CodeableConcept", "An indication of the type of referral (or where applicable the type of transfer of care) request.", 0, 1, type)); 2191 children.add(new Property("priority", "code", "An indication of the urgency of referral (or where applicable the type of transfer of care) request.", 0, 1, priority)); 2192 children.add(new Property("serviceRequested", "CodeableConcept", "The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion.", 0, java.lang.Integer.MAX_VALUE, serviceRequested)); 2193 children.add(new Property("subject", "Reference(Patient|Group)", "The patient who is the subject of a referral or transfer of care request.", 0, 1, subject)); 2194 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter at which the request for referral or transfer of care is initiated.", 0, 1, context)); 2195 children.add(new Property("occurrence[x]", "dateTime|Period", "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 0, 1, occurrence)); 2196 children.add(new Property("authoredOn", "dateTime", "Date/DateTime of creation for draft requests and date of activation for active requests.", 0, 1, authoredOn)); 2197 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2198 children.add(new Property("specialty", "CodeableConcept", "Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.", 0, 1, specialty)); 2199 children.add(new Property("recipient", "Reference(Practitioner|Organization|HealthcareService)", "The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.", 0, java.lang.Integer.MAX_VALUE, recipient)); 2200 children.add(new Property("reasonCode", "CodeableConcept", "Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2201 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2202 children.add(new Property("description", "string", "The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.", 0, 1, description)); 2203 children.add(new Property("supportingInfo", "Reference(Any)", "Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2204 children.add(new Property("note", "Annotation", "Comments made about the referral request by any of the participants.", 0, java.lang.Integer.MAX_VALUE, note)); 2205 children.add(new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 2206 } 2207 2208 @Override 2209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2210 switch (_hash) { 2211 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier that uniquely identifies the referral/care transfer request instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2212 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 2213 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "Indicates any plans, proposals or orders that this request is intended to satisfy - in whole or in part.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2214 case -430332865: /*replaces*/ return new Property("replaces", "Reference(ReferralRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 2215 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "The business identifier of the logical \"grouping\" request/order that this referral is a part of.", 0, 1, groupIdentifier); 2216 case -892481550: /*status*/ return new Property("status", "code", "The status of the authorization/intention reflected by the referral request record.", 0, 1, status); 2217 case -1183762788: /*intent*/ return new Property("intent", "code", "Distinguishes the \"level\" of authorization/demand implicit in this request.", 0, 1, intent); 2218 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "An indication of the type of referral (or where applicable the type of transfer of care) request.", 0, 1, type); 2219 case -1165461084: /*priority*/ return new Property("priority", "code", "An indication of the urgency of referral (or where applicable the type of transfer of care) request.", 0, 1, priority); 2220 case 190229561: /*serviceRequested*/ return new Property("serviceRequested", "CodeableConcept", "The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion.", 0, java.lang.Integer.MAX_VALUE, serviceRequested); 2221 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient who is the subject of a referral or transfer of care request.", 0, 1, subject); 2222 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter at which the request for referral or transfer of care is initiated.", 0, 1, context); 2223 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 0, 1, occurrence); 2224 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 0, 1, occurrence); 2225 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period", "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 0, 1, occurrence); 2226 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period", "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 0, 1, occurrence); 2227 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "Date/DateTime of creation for draft requests and date of activation for active requests.", 0, 1, authoredOn); 2228 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2229 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.", 0, 1, specialty); 2230 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Practitioner|Organization|HealthcareService)", "The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.", 0, java.lang.Integer.MAX_VALUE, recipient); 2231 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2232 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2233 case -1724546052: /*description*/ return new Property("description", "string", "The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.", 0, 1, description); 2234 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2235 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the referral request by any of the participants.", 0, java.lang.Integer.MAX_VALUE, note); 2236 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 2237 default: return super.getNamedProperty(_hash, _name, _checkValid); 2238 } 2239 2240 } 2241 2242 @Override 2243 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2244 switch (hash) { 2245 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2246 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2247 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2248 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2249 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 2250 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ReferralRequestStatus> 2251 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<ReferralCategory> 2252 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2253 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<ReferralPriority> 2254 case 190229561: /*serviceRequested*/ return this.serviceRequested == null ? new Base[0] : this.serviceRequested.toArray(new Base[this.serviceRequested.size()]); // CodeableConcept 2255 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2256 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2257 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 2258 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2259 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // ReferralRequestRequesterComponent 2260 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : new Base[] {this.specialty}; // CodeableConcept 2261 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2262 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2263 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2264 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2265 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2266 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2267 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 2268 default: return super.getProperty(hash, name, checkValid); 2269 } 2270 2271 } 2272 2273 @Override 2274 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2275 switch (hash) { 2276 case -1618432855: // identifier 2277 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2278 return value; 2279 case -1014418093: // definition 2280 this.getDefinition().add(castToReference(value)); // Reference 2281 return value; 2282 case -332612366: // basedOn 2283 this.getBasedOn().add(castToReference(value)); // Reference 2284 return value; 2285 case -430332865: // replaces 2286 this.getReplaces().add(castToReference(value)); // Reference 2287 return value; 2288 case -445338488: // groupIdentifier 2289 this.groupIdentifier = castToIdentifier(value); // Identifier 2290 return value; 2291 case -892481550: // status 2292 value = new ReferralRequestStatusEnumFactory().fromType(castToCode(value)); 2293 this.status = (Enumeration) value; // Enumeration<ReferralRequestStatus> 2294 return value; 2295 case -1183762788: // intent 2296 value = new ReferralCategoryEnumFactory().fromType(castToCode(value)); 2297 this.intent = (Enumeration) value; // Enumeration<ReferralCategory> 2298 return value; 2299 case 3575610: // type 2300 this.type = castToCodeableConcept(value); // CodeableConcept 2301 return value; 2302 case -1165461084: // priority 2303 value = new ReferralPriorityEnumFactory().fromType(castToCode(value)); 2304 this.priority = (Enumeration) value; // Enumeration<ReferralPriority> 2305 return value; 2306 case 190229561: // serviceRequested 2307 this.getServiceRequested().add(castToCodeableConcept(value)); // CodeableConcept 2308 return value; 2309 case -1867885268: // subject 2310 this.subject = castToReference(value); // Reference 2311 return value; 2312 case 951530927: // context 2313 this.context = castToReference(value); // Reference 2314 return value; 2315 case 1687874001: // occurrence 2316 this.occurrence = castToType(value); // Type 2317 return value; 2318 case -1500852503: // authoredOn 2319 this.authoredOn = castToDateTime(value); // DateTimeType 2320 return value; 2321 case 693933948: // requester 2322 this.requester = (ReferralRequestRequesterComponent) value; // ReferralRequestRequesterComponent 2323 return value; 2324 case -1694759682: // specialty 2325 this.specialty = castToCodeableConcept(value); // CodeableConcept 2326 return value; 2327 case 820081177: // recipient 2328 this.getRecipient().add(castToReference(value)); // Reference 2329 return value; 2330 case 722137681: // reasonCode 2331 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2332 return value; 2333 case -1146218137: // reasonReference 2334 this.getReasonReference().add(castToReference(value)); // Reference 2335 return value; 2336 case -1724546052: // description 2337 this.description = castToString(value); // StringType 2338 return value; 2339 case 1922406657: // supportingInfo 2340 this.getSupportingInfo().add(castToReference(value)); // Reference 2341 return value; 2342 case 3387378: // note 2343 this.getNote().add(castToAnnotation(value)); // Annotation 2344 return value; 2345 case 1538891575: // relevantHistory 2346 this.getRelevantHistory().add(castToReference(value)); // Reference 2347 return value; 2348 default: return super.setProperty(hash, name, value); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base setProperty(String name, Base value) throws FHIRException { 2355 if (name.equals("identifier")) { 2356 this.getIdentifier().add(castToIdentifier(value)); 2357 } else if (name.equals("definition")) { 2358 this.getDefinition().add(castToReference(value)); 2359 } else if (name.equals("basedOn")) { 2360 this.getBasedOn().add(castToReference(value)); 2361 } else if (name.equals("replaces")) { 2362 this.getReplaces().add(castToReference(value)); 2363 } else if (name.equals("groupIdentifier")) { 2364 this.groupIdentifier = castToIdentifier(value); // Identifier 2365 } else if (name.equals("status")) { 2366 value = new ReferralRequestStatusEnumFactory().fromType(castToCode(value)); 2367 this.status = (Enumeration) value; // Enumeration<ReferralRequestStatus> 2368 } else if (name.equals("intent")) { 2369 value = new ReferralCategoryEnumFactory().fromType(castToCode(value)); 2370 this.intent = (Enumeration) value; // Enumeration<ReferralCategory> 2371 } else if (name.equals("type")) { 2372 this.type = castToCodeableConcept(value); // CodeableConcept 2373 } else if (name.equals("priority")) { 2374 value = new ReferralPriorityEnumFactory().fromType(castToCode(value)); 2375 this.priority = (Enumeration) value; // Enumeration<ReferralPriority> 2376 } else if (name.equals("serviceRequested")) { 2377 this.getServiceRequested().add(castToCodeableConcept(value)); 2378 } else if (name.equals("subject")) { 2379 this.subject = castToReference(value); // Reference 2380 } else if (name.equals("context")) { 2381 this.context = castToReference(value); // Reference 2382 } else if (name.equals("occurrence[x]")) { 2383 this.occurrence = castToType(value); // Type 2384 } else if (name.equals("authoredOn")) { 2385 this.authoredOn = castToDateTime(value); // DateTimeType 2386 } else if (name.equals("requester")) { 2387 this.requester = (ReferralRequestRequesterComponent) value; // ReferralRequestRequesterComponent 2388 } else if (name.equals("specialty")) { 2389 this.specialty = castToCodeableConcept(value); // CodeableConcept 2390 } else if (name.equals("recipient")) { 2391 this.getRecipient().add(castToReference(value)); 2392 } else if (name.equals("reasonCode")) { 2393 this.getReasonCode().add(castToCodeableConcept(value)); 2394 } else if (name.equals("reasonReference")) { 2395 this.getReasonReference().add(castToReference(value)); 2396 } else if (name.equals("description")) { 2397 this.description = castToString(value); // StringType 2398 } else if (name.equals("supportingInfo")) { 2399 this.getSupportingInfo().add(castToReference(value)); 2400 } else if (name.equals("note")) { 2401 this.getNote().add(castToAnnotation(value)); 2402 } else if (name.equals("relevantHistory")) { 2403 this.getRelevantHistory().add(castToReference(value)); 2404 } else 2405 return super.setProperty(name, value); 2406 return value; 2407 } 2408 2409 @Override 2410 public Base makeProperty(int hash, String name) throws FHIRException { 2411 switch (hash) { 2412 case -1618432855: return addIdentifier(); 2413 case -1014418093: return addDefinition(); 2414 case -332612366: return addBasedOn(); 2415 case -430332865: return addReplaces(); 2416 case -445338488: return getGroupIdentifier(); 2417 case -892481550: return getStatusElement(); 2418 case -1183762788: return getIntentElement(); 2419 case 3575610: return getType(); 2420 case -1165461084: return getPriorityElement(); 2421 case 190229561: return addServiceRequested(); 2422 case -1867885268: return getSubject(); 2423 case 951530927: return getContext(); 2424 case -2022646513: return getOccurrence(); 2425 case 1687874001: return getOccurrence(); 2426 case -1500852503: return getAuthoredOnElement(); 2427 case 693933948: return getRequester(); 2428 case -1694759682: return getSpecialty(); 2429 case 820081177: return addRecipient(); 2430 case 722137681: return addReasonCode(); 2431 case -1146218137: return addReasonReference(); 2432 case -1724546052: return getDescriptionElement(); 2433 case 1922406657: return addSupportingInfo(); 2434 case 3387378: return addNote(); 2435 case 1538891575: return addRelevantHistory(); 2436 default: return super.makeProperty(hash, name); 2437 } 2438 2439 } 2440 2441 @Override 2442 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2443 switch (hash) { 2444 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2445 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2446 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2447 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2448 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 2449 case -892481550: /*status*/ return new String[] {"code"}; 2450 case -1183762788: /*intent*/ return new String[] {"code"}; 2451 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2452 case -1165461084: /*priority*/ return new String[] {"code"}; 2453 case 190229561: /*serviceRequested*/ return new String[] {"CodeableConcept"}; 2454 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2455 case 951530927: /*context*/ return new String[] {"Reference"}; 2456 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 2457 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2458 case 693933948: /*requester*/ return new String[] {}; 2459 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 2460 case 820081177: /*recipient*/ return new String[] {"Reference"}; 2461 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2462 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2463 case -1724546052: /*description*/ return new String[] {"string"}; 2464 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2465 case 3387378: /*note*/ return new String[] {"Annotation"}; 2466 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2467 default: return super.getTypesForProperty(hash, name); 2468 } 2469 2470 } 2471 2472 @Override 2473 public Base addChild(String name) throws FHIRException { 2474 if (name.equals("identifier")) { 2475 return addIdentifier(); 2476 } 2477 else if (name.equals("definition")) { 2478 return addDefinition(); 2479 } 2480 else if (name.equals("basedOn")) { 2481 return addBasedOn(); 2482 } 2483 else if (name.equals("replaces")) { 2484 return addReplaces(); 2485 } 2486 else if (name.equals("groupIdentifier")) { 2487 this.groupIdentifier = new Identifier(); 2488 return this.groupIdentifier; 2489 } 2490 else if (name.equals("status")) { 2491 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.status"); 2492 } 2493 else if (name.equals("intent")) { 2494 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.intent"); 2495 } 2496 else if (name.equals("type")) { 2497 this.type = new CodeableConcept(); 2498 return this.type; 2499 } 2500 else if (name.equals("priority")) { 2501 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.priority"); 2502 } 2503 else if (name.equals("serviceRequested")) { 2504 return addServiceRequested(); 2505 } 2506 else if (name.equals("subject")) { 2507 this.subject = new Reference(); 2508 return this.subject; 2509 } 2510 else if (name.equals("context")) { 2511 this.context = new Reference(); 2512 return this.context; 2513 } 2514 else if (name.equals("occurrenceDateTime")) { 2515 this.occurrence = new DateTimeType(); 2516 return this.occurrence; 2517 } 2518 else if (name.equals("occurrencePeriod")) { 2519 this.occurrence = new Period(); 2520 return this.occurrence; 2521 } 2522 else if (name.equals("authoredOn")) { 2523 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.authoredOn"); 2524 } 2525 else if (name.equals("requester")) { 2526 this.requester = new ReferralRequestRequesterComponent(); 2527 return this.requester; 2528 } 2529 else if (name.equals("specialty")) { 2530 this.specialty = new CodeableConcept(); 2531 return this.specialty; 2532 } 2533 else if (name.equals("recipient")) { 2534 return addRecipient(); 2535 } 2536 else if (name.equals("reasonCode")) { 2537 return addReasonCode(); 2538 } 2539 else if (name.equals("reasonReference")) { 2540 return addReasonReference(); 2541 } 2542 else if (name.equals("description")) { 2543 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.description"); 2544 } 2545 else if (name.equals("supportingInfo")) { 2546 return addSupportingInfo(); 2547 } 2548 else if (name.equals("note")) { 2549 return addNote(); 2550 } 2551 else if (name.equals("relevantHistory")) { 2552 return addRelevantHistory(); 2553 } 2554 else 2555 return super.addChild(name); 2556 } 2557 2558 public String fhirType() { 2559 return "ReferralRequest"; 2560 2561 } 2562 2563 public ReferralRequest copy() { 2564 ReferralRequest dst = new ReferralRequest(); 2565 copyValues(dst); 2566 if (identifier != null) { 2567 dst.identifier = new ArrayList<Identifier>(); 2568 for (Identifier i : identifier) 2569 dst.identifier.add(i.copy()); 2570 }; 2571 if (definition != null) { 2572 dst.definition = new ArrayList<Reference>(); 2573 for (Reference i : definition) 2574 dst.definition.add(i.copy()); 2575 }; 2576 if (basedOn != null) { 2577 dst.basedOn = new ArrayList<Reference>(); 2578 for (Reference i : basedOn) 2579 dst.basedOn.add(i.copy()); 2580 }; 2581 if (replaces != null) { 2582 dst.replaces = new ArrayList<Reference>(); 2583 for (Reference i : replaces) 2584 dst.replaces.add(i.copy()); 2585 }; 2586 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2587 dst.status = status == null ? null : status.copy(); 2588 dst.intent = intent == null ? null : intent.copy(); 2589 dst.type = type == null ? null : type.copy(); 2590 dst.priority = priority == null ? null : priority.copy(); 2591 if (serviceRequested != null) { 2592 dst.serviceRequested = new ArrayList<CodeableConcept>(); 2593 for (CodeableConcept i : serviceRequested) 2594 dst.serviceRequested.add(i.copy()); 2595 }; 2596 dst.subject = subject == null ? null : subject.copy(); 2597 dst.context = context == null ? null : context.copy(); 2598 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2599 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2600 dst.requester = requester == null ? null : requester.copy(); 2601 dst.specialty = specialty == null ? null : specialty.copy(); 2602 if (recipient != null) { 2603 dst.recipient = new ArrayList<Reference>(); 2604 for (Reference i : recipient) 2605 dst.recipient.add(i.copy()); 2606 }; 2607 if (reasonCode != null) { 2608 dst.reasonCode = new ArrayList<CodeableConcept>(); 2609 for (CodeableConcept i : reasonCode) 2610 dst.reasonCode.add(i.copy()); 2611 }; 2612 if (reasonReference != null) { 2613 dst.reasonReference = new ArrayList<Reference>(); 2614 for (Reference i : reasonReference) 2615 dst.reasonReference.add(i.copy()); 2616 }; 2617 dst.description = description == null ? null : description.copy(); 2618 if (supportingInfo != null) { 2619 dst.supportingInfo = new ArrayList<Reference>(); 2620 for (Reference i : supportingInfo) 2621 dst.supportingInfo.add(i.copy()); 2622 }; 2623 if (note != null) { 2624 dst.note = new ArrayList<Annotation>(); 2625 for (Annotation i : note) 2626 dst.note.add(i.copy()); 2627 }; 2628 if (relevantHistory != null) { 2629 dst.relevantHistory = new ArrayList<Reference>(); 2630 for (Reference i : relevantHistory) 2631 dst.relevantHistory.add(i.copy()); 2632 }; 2633 return dst; 2634 } 2635 2636 protected ReferralRequest typedCopy() { 2637 return copy(); 2638 } 2639 2640 @Override 2641 public boolean equalsDeep(Base other_) { 2642 if (!super.equalsDeep(other_)) 2643 return false; 2644 if (!(other_ instanceof ReferralRequest)) 2645 return false; 2646 ReferralRequest o = (ReferralRequest) other_; 2647 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2648 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2649 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(type, o.type, true) 2650 && compareDeep(priority, o.priority, true) && compareDeep(serviceRequested, o.serviceRequested, true) 2651 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) 2652 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(specialty, o.specialty, true) 2653 && compareDeep(recipient, o.recipient, true) && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2654 && compareDeep(description, o.description, true) && compareDeep(supportingInfo, o.supportingInfo, true) 2655 && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true); 2656 } 2657 2658 @Override 2659 public boolean equalsShallow(Base other_) { 2660 if (!super.equalsShallow(other_)) 2661 return false; 2662 if (!(other_ instanceof ReferralRequest)) 2663 return false; 2664 ReferralRequest o = (ReferralRequest) other_; 2665 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 2666 && compareValues(authoredOn, o.authoredOn, true) && compareValues(description, o.description, true) 2667 ; 2668 } 2669 2670 public boolean isEmpty() { 2671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 2672 , replaces, groupIdentifier, status, intent, type, priority, serviceRequested 2673 , subject, context, occurrence, authoredOn, requester, specialty, recipient, reasonCode 2674 , reasonReference, description, supportingInfo, note, relevantHistory); 2675 } 2676 2677 @Override 2678 public ResourceType getResourceType() { 2679 return ResourceType.ReferralRequest; 2680 } 2681 2682 /** 2683 * Search parameter: <b>requester</b> 2684 * <p> 2685 * Description: <b>Individual making the request</b><br> 2686 * Type: <b>reference</b><br> 2687 * Path: <b>ReferralRequest.requester.agent</b><br> 2688 * </p> 2689 */ 2690 @SearchParamDefinition(name="requester", path="ReferralRequest.requester.agent", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2691 public static final String SP_REQUESTER = "requester"; 2692 /** 2693 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2694 * <p> 2695 * Description: <b>Individual making the request</b><br> 2696 * Type: <b>reference</b><br> 2697 * Path: <b>ReferralRequest.requester.agent</b><br> 2698 * </p> 2699 */ 2700 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2701 2702/** 2703 * Constant for fluent queries to be used to add include statements. Specifies 2704 * the path value of "<b>ReferralRequest:requester</b>". 2705 */ 2706 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("ReferralRequest:requester").toLocked(); 2707 2708 /** 2709 * Search parameter: <b>identifier</b> 2710 * <p> 2711 * Description: <b>Business identifier</b><br> 2712 * Type: <b>token</b><br> 2713 * Path: <b>ReferralRequest.identifier</b><br> 2714 * </p> 2715 */ 2716 @SearchParamDefinition(name="identifier", path="ReferralRequest.identifier", description="Business identifier", type="token" ) 2717 public static final String SP_IDENTIFIER = "identifier"; 2718 /** 2719 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2720 * <p> 2721 * Description: <b>Business identifier</b><br> 2722 * Type: <b>token</b><br> 2723 * Path: <b>ReferralRequest.identifier</b><br> 2724 * </p> 2725 */ 2726 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2727 2728 /** 2729 * Search parameter: <b>specialty</b> 2730 * <p> 2731 * Description: <b>The specialty that the referral is for</b><br> 2732 * Type: <b>token</b><br> 2733 * Path: <b>ReferralRequest.specialty</b><br> 2734 * </p> 2735 */ 2736 @SearchParamDefinition(name="specialty", path="ReferralRequest.specialty", description="The specialty that the referral is for", type="token" ) 2737 public static final String SP_SPECIALTY = "specialty"; 2738 /** 2739 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2740 * <p> 2741 * Description: <b>The specialty that the referral is for</b><br> 2742 * Type: <b>token</b><br> 2743 * Path: <b>ReferralRequest.specialty</b><br> 2744 * </p> 2745 */ 2746 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 2747 2748 /** 2749 * Search parameter: <b>replaces</b> 2750 * <p> 2751 * Description: <b>Request(s) replaced by this request</b><br> 2752 * Type: <b>reference</b><br> 2753 * Path: <b>ReferralRequest.replaces</b><br> 2754 * </p> 2755 */ 2756 @SearchParamDefinition(name="replaces", path="ReferralRequest.replaces", description="Request(s) replaced by this request", type="reference", target={ReferralRequest.class } ) 2757 public static final String SP_REPLACES = "replaces"; 2758 /** 2759 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2760 * <p> 2761 * Description: <b>Request(s) replaced by this request</b><br> 2762 * Type: <b>reference</b><br> 2763 * Path: <b>ReferralRequest.replaces</b><br> 2764 * </p> 2765 */ 2766 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 2767 2768/** 2769 * Constant for fluent queries to be used to add include statements. Specifies 2770 * the path value of "<b>ReferralRequest:replaces</b>". 2771 */ 2772 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("ReferralRequest:replaces").toLocked(); 2773 2774 /** 2775 * Search parameter: <b>subject</b> 2776 * <p> 2777 * Description: <b>Patient referred to care or transfer</b><br> 2778 * Type: <b>reference</b><br> 2779 * Path: <b>ReferralRequest.subject</b><br> 2780 * </p> 2781 */ 2782 @SearchParamDefinition(name="subject", path="ReferralRequest.subject", description="Patient referred to care or transfer", type="reference", target={Group.class, Patient.class } ) 2783 public static final String SP_SUBJECT = "subject"; 2784 /** 2785 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2786 * <p> 2787 * Description: <b>Patient referred to care or transfer</b><br> 2788 * Type: <b>reference</b><br> 2789 * Path: <b>ReferralRequest.subject</b><br> 2790 * </p> 2791 */ 2792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2793 2794/** 2795 * Constant for fluent queries to be used to add include statements. Specifies 2796 * the path value of "<b>ReferralRequest:subject</b>". 2797 */ 2798 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ReferralRequest:subject").toLocked(); 2799 2800 /** 2801 * Search parameter: <b>encounter</b> 2802 * <p> 2803 * Description: <b>Originating encounter</b><br> 2804 * Type: <b>reference</b><br> 2805 * Path: <b>ReferralRequest.context</b><br> 2806 * </p> 2807 */ 2808 @SearchParamDefinition(name="encounter", path="ReferralRequest.context", description="Originating encounter", type="reference", target={Encounter.class } ) 2809 public static final String SP_ENCOUNTER = "encounter"; 2810 /** 2811 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2812 * <p> 2813 * Description: <b>Originating encounter</b><br> 2814 * Type: <b>reference</b><br> 2815 * Path: <b>ReferralRequest.context</b><br> 2816 * </p> 2817 */ 2818 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2819 2820/** 2821 * Constant for fluent queries to be used to add include statements. Specifies 2822 * the path value of "<b>ReferralRequest:encounter</b>". 2823 */ 2824 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ReferralRequest:encounter").toLocked(); 2825 2826 /** 2827 * Search parameter: <b>authored-on</b> 2828 * <p> 2829 * Description: <b>Creation or activation date</b><br> 2830 * Type: <b>date</b><br> 2831 * Path: <b>ReferralRequest.authoredOn</b><br> 2832 * </p> 2833 */ 2834 @SearchParamDefinition(name="authored-on", path="ReferralRequest.authoredOn", description="Creation or activation date", type="date" ) 2835 public static final String SP_AUTHORED_ON = "authored-on"; 2836 /** 2837 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 2838 * <p> 2839 * Description: <b>Creation or activation date</b><br> 2840 * Type: <b>date</b><br> 2841 * Path: <b>ReferralRequest.authoredOn</b><br> 2842 * </p> 2843 */ 2844 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 2845 2846 /** 2847 * Search parameter: <b>type</b> 2848 * <p> 2849 * Description: <b>The type of the referral</b><br> 2850 * Type: <b>token</b><br> 2851 * Path: <b>ReferralRequest.type</b><br> 2852 * </p> 2853 */ 2854 @SearchParamDefinition(name="type", path="ReferralRequest.type", description="The type of the referral", type="token" ) 2855 public static final String SP_TYPE = "type"; 2856 /** 2857 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2858 * <p> 2859 * Description: <b>The type of the referral</b><br> 2860 * Type: <b>token</b><br> 2861 * Path: <b>ReferralRequest.type</b><br> 2862 * </p> 2863 */ 2864 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2865 2866 /** 2867 * Search parameter: <b>priority</b> 2868 * <p> 2869 * Description: <b>The priority assigned to the referral</b><br> 2870 * Type: <b>token</b><br> 2871 * Path: <b>ReferralRequest.priority</b><br> 2872 * </p> 2873 */ 2874 @SearchParamDefinition(name="priority", path="ReferralRequest.priority", description="The priority assigned to the referral", type="token" ) 2875 public static final String SP_PRIORITY = "priority"; 2876 /** 2877 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 2878 * <p> 2879 * Description: <b>The priority assigned to the referral</b><br> 2880 * Type: <b>token</b><br> 2881 * Path: <b>ReferralRequest.priority</b><br> 2882 * </p> 2883 */ 2884 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 2885 2886 /** 2887 * Search parameter: <b>intent</b> 2888 * <p> 2889 * Description: <b>Proposal, plan or order</b><br> 2890 * Type: <b>token</b><br> 2891 * Path: <b>ReferralRequest.intent</b><br> 2892 * </p> 2893 */ 2894 @SearchParamDefinition(name="intent", path="ReferralRequest.intent", description="Proposal, plan or order", type="token" ) 2895 public static final String SP_INTENT = "intent"; 2896 /** 2897 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 2898 * <p> 2899 * Description: <b>Proposal, plan or order</b><br> 2900 * Type: <b>token</b><br> 2901 * Path: <b>ReferralRequest.intent</b><br> 2902 * </p> 2903 */ 2904 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 2905 2906 /** 2907 * Search parameter: <b>group-identifier</b> 2908 * <p> 2909 * Description: <b>Part of common request</b><br> 2910 * Type: <b>token</b><br> 2911 * Path: <b>ReferralRequest.groupIdentifier</b><br> 2912 * </p> 2913 */ 2914 @SearchParamDefinition(name="group-identifier", path="ReferralRequest.groupIdentifier", description="Part of common request", type="token" ) 2915 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2916 /** 2917 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2918 * <p> 2919 * Description: <b>Part of common request</b><br> 2920 * Type: <b>token</b><br> 2921 * Path: <b>ReferralRequest.groupIdentifier</b><br> 2922 * </p> 2923 */ 2924 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2925 2926 /** 2927 * Search parameter: <b>based-on</b> 2928 * <p> 2929 * Description: <b>Request being fulfilled</b><br> 2930 * Type: <b>reference</b><br> 2931 * Path: <b>ReferralRequest.basedOn</b><br> 2932 * </p> 2933 */ 2934 @SearchParamDefinition(name="based-on", path="ReferralRequest.basedOn", description="Request being fulfilled", type="reference", target={CarePlan.class, ProcedureRequest.class, ReferralRequest.class } ) 2935 public static final String SP_BASED_ON = "based-on"; 2936 /** 2937 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2938 * <p> 2939 * Description: <b>Request being fulfilled</b><br> 2940 * Type: <b>reference</b><br> 2941 * Path: <b>ReferralRequest.basedOn</b><br> 2942 * </p> 2943 */ 2944 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2945 2946/** 2947 * Constant for fluent queries to be used to add include statements. Specifies 2948 * the path value of "<b>ReferralRequest:based-on</b>". 2949 */ 2950 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ReferralRequest:based-on").toLocked(); 2951 2952 /** 2953 * Search parameter: <b>patient</b> 2954 * <p> 2955 * Description: <b>Who the referral is about</b><br> 2956 * Type: <b>reference</b><br> 2957 * Path: <b>ReferralRequest.subject</b><br> 2958 * </p> 2959 */ 2960 @SearchParamDefinition(name="patient", path="ReferralRequest.subject", description="Who the referral is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2961 public static final String SP_PATIENT = "patient"; 2962 /** 2963 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2964 * <p> 2965 * Description: <b>Who the referral is about</b><br> 2966 * Type: <b>reference</b><br> 2967 * Path: <b>ReferralRequest.subject</b><br> 2968 * </p> 2969 */ 2970 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2971 2972/** 2973 * Constant for fluent queries to be used to add include statements. Specifies 2974 * the path value of "<b>ReferralRequest:patient</b>". 2975 */ 2976 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ReferralRequest:patient").toLocked(); 2977 2978 /** 2979 * Search parameter: <b>service</b> 2980 * <p> 2981 * Description: <b>Actions requested as part of the referral</b><br> 2982 * Type: <b>token</b><br> 2983 * Path: <b>ReferralRequest.serviceRequested</b><br> 2984 * </p> 2985 */ 2986 @SearchParamDefinition(name="service", path="ReferralRequest.serviceRequested", description="Actions requested as part of the referral", type="token" ) 2987 public static final String SP_SERVICE = "service"; 2988 /** 2989 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2990 * <p> 2991 * Description: <b>Actions requested as part of the referral</b><br> 2992 * Type: <b>token</b><br> 2993 * Path: <b>ReferralRequest.serviceRequested</b><br> 2994 * </p> 2995 */ 2996 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE); 2997 2998 /** 2999 * Search parameter: <b>occurrence-date</b> 3000 * <p> 3001 * Description: <b>When the service(s) requested in the referral should occur</b><br> 3002 * Type: <b>date</b><br> 3003 * Path: <b>ReferralRequest.occurrence[x]</b><br> 3004 * </p> 3005 */ 3006 @SearchParamDefinition(name="occurrence-date", path="ReferralRequest.occurrence", description="When the service(s) requested in the referral should occur", type="date" ) 3007 public static final String SP_OCCURRENCE_DATE = "occurrence-date"; 3008 /** 3009 * <b>Fluent Client</b> search parameter constant for <b>occurrence-date</b> 3010 * <p> 3011 * Description: <b>When the service(s) requested in the referral should occur</b><br> 3012 * Type: <b>date</b><br> 3013 * Path: <b>ReferralRequest.occurrence[x]</b><br> 3014 * </p> 3015 */ 3016 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE_DATE); 3017 3018 /** 3019 * Search parameter: <b>recipient</b> 3020 * <p> 3021 * Description: <b>The person that the referral was sent to</b><br> 3022 * Type: <b>reference</b><br> 3023 * Path: <b>ReferralRequest.recipient</b><br> 3024 * </p> 3025 */ 3026 @SearchParamDefinition(name="recipient", path="ReferralRequest.recipient", description="The person that the referral was sent to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={HealthcareService.class, Organization.class, Practitioner.class } ) 3027 public static final String SP_RECIPIENT = "recipient"; 3028 /** 3029 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3030 * <p> 3031 * Description: <b>The person that the referral was sent to</b><br> 3032 * Type: <b>reference</b><br> 3033 * Path: <b>ReferralRequest.recipient</b><br> 3034 * </p> 3035 */ 3036 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 3037 3038/** 3039 * Constant for fluent queries to be used to add include statements. Specifies 3040 * the path value of "<b>ReferralRequest:recipient</b>". 3041 */ 3042 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("ReferralRequest:recipient").toLocked(); 3043 3044 /** 3045 * Search parameter: <b>context</b> 3046 * <p> 3047 * Description: <b>Part of encounter or episode of care</b><br> 3048 * Type: <b>reference</b><br> 3049 * Path: <b>ReferralRequest.context</b><br> 3050 * </p> 3051 */ 3052 @SearchParamDefinition(name="context", path="ReferralRequest.context", description="Part of encounter or episode of care", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 3053 public static final String SP_CONTEXT = "context"; 3054 /** 3055 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3056 * <p> 3057 * Description: <b>Part of encounter or episode of care</b><br> 3058 * Type: <b>reference</b><br> 3059 * Path: <b>ReferralRequest.context</b><br> 3060 * </p> 3061 */ 3062 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3063 3064/** 3065 * Constant for fluent queries to be used to add include statements. Specifies 3066 * the path value of "<b>ReferralRequest:context</b>". 3067 */ 3068 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ReferralRequest:context").toLocked(); 3069 3070 /** 3071 * Search parameter: <b>definition</b> 3072 * <p> 3073 * Description: <b>Instantiates protocol or definition</b><br> 3074 * Type: <b>reference</b><br> 3075 * Path: <b>ReferralRequest.definition</b><br> 3076 * </p> 3077 */ 3078 @SearchParamDefinition(name="definition", path="ReferralRequest.definition", description="Instantiates protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 3079 public static final String SP_DEFINITION = "definition"; 3080 /** 3081 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 3082 * <p> 3083 * Description: <b>Instantiates protocol or definition</b><br> 3084 * Type: <b>reference</b><br> 3085 * Path: <b>ReferralRequest.definition</b><br> 3086 * </p> 3087 */ 3088 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 3089 3090/** 3091 * Constant for fluent queries to be used to add include statements. Specifies 3092 * the path value of "<b>ReferralRequest:definition</b>". 3093 */ 3094 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("ReferralRequest:definition").toLocked(); 3095 3096 /** 3097 * Search parameter: <b>status</b> 3098 * <p> 3099 * Description: <b>The status of the referral</b><br> 3100 * Type: <b>token</b><br> 3101 * Path: <b>ReferralRequest.status</b><br> 3102 * </p> 3103 */ 3104 @SearchParamDefinition(name="status", path="ReferralRequest.status", description="The status of the referral", type="token" ) 3105 public static final String SP_STATUS = "status"; 3106 /** 3107 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3108 * <p> 3109 * Description: <b>The status of the referral</b><br> 3110 * Type: <b>token</b><br> 3111 * Path: <b>ReferralRequest.status</b><br> 3112 * </p> 3113 */ 3114 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3115 3116 3117}