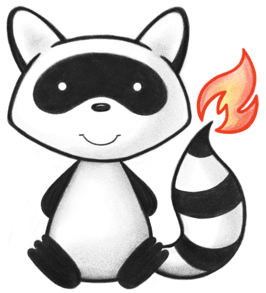
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * Related artifacts such as additional documentation, justification, or bibliographic references. 046 */ 047@DatatypeDef(name="RelatedArtifact") 048public class RelatedArtifact extends Type implements ICompositeType { 049 050 public enum RelatedArtifactType { 051 /** 052 * Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness 053 */ 054 DOCUMENTATION, 055 /** 056 * A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource 057 */ 058 JUSTIFICATION, 059 /** 060 * Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource 061 */ 062 CITATION, 063 /** 064 * The previous version of the knowledge resource 065 */ 066 PREDECESSOR, 067 /** 068 * The next version of the knowledge resource 069 */ 070 SUCCESSOR, 071 /** 072 * The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting 073 */ 074 DERIVEDFROM, 075 /** 076 * The knowledge resource depends on the given related artifact 077 */ 078 DEPENDSON, 079 /** 080 * The knowledge resource is composed of the given related artifact 081 */ 082 COMPOSEDOF, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("documentation".equals(codeString)) 091 return DOCUMENTATION; 092 if ("justification".equals(codeString)) 093 return JUSTIFICATION; 094 if ("citation".equals(codeString)) 095 return CITATION; 096 if ("predecessor".equals(codeString)) 097 return PREDECESSOR; 098 if ("successor".equals(codeString)) 099 return SUCCESSOR; 100 if ("derived-from".equals(codeString)) 101 return DERIVEDFROM; 102 if ("depends-on".equals(codeString)) 103 return DEPENDSON; 104 if ("composed-of".equals(codeString)) 105 return COMPOSEDOF; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown RelatedArtifactType code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case DOCUMENTATION: return "documentation"; 114 case JUSTIFICATION: return "justification"; 115 case CITATION: return "citation"; 116 case PREDECESSOR: return "predecessor"; 117 case SUCCESSOR: return "successor"; 118 case DERIVEDFROM: return "derived-from"; 119 case DEPENDSON: return "depends-on"; 120 case COMPOSEDOF: return "composed-of"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getSystem() { 126 switch (this) { 127 case DOCUMENTATION: return "http://hl7.org/fhir/related-artifact-type"; 128 case JUSTIFICATION: return "http://hl7.org/fhir/related-artifact-type"; 129 case CITATION: return "http://hl7.org/fhir/related-artifact-type"; 130 case PREDECESSOR: return "http://hl7.org/fhir/related-artifact-type"; 131 case SUCCESSOR: return "http://hl7.org/fhir/related-artifact-type"; 132 case DERIVEDFROM: return "http://hl7.org/fhir/related-artifact-type"; 133 case DEPENDSON: return "http://hl7.org/fhir/related-artifact-type"; 134 case COMPOSEDOF: return "http://hl7.org/fhir/related-artifact-type"; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 public String getDefinition() { 140 switch (this) { 141 case DOCUMENTATION: return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness"; 142 case JUSTIFICATION: return "A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource"; 143 case CITATION: return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource"; 144 case PREDECESSOR: return "The previous version of the knowledge resource"; 145 case SUCCESSOR: return "The next version of the knowledge resource"; 146 case DERIVEDFROM: return "The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting"; 147 case DEPENDSON: return "The knowledge resource depends on the given related artifact"; 148 case COMPOSEDOF: return "The knowledge resource is composed of the given related artifact"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDisplay() { 154 switch (this) { 155 case DOCUMENTATION: return "Documentation"; 156 case JUSTIFICATION: return "Justification"; 157 case CITATION: return "Citation"; 158 case PREDECESSOR: return "Predecessor"; 159 case SUCCESSOR: return "Successor"; 160 case DERIVEDFROM: return "Derived From"; 161 case DEPENDSON: return "Depends On"; 162 case COMPOSEDOF: return "Composed Of"; 163 case NULL: return null; 164 default: return "?"; 165 } 166 } 167 } 168 169 public static class RelatedArtifactTypeEnumFactory implements EnumFactory<RelatedArtifactType> { 170 public RelatedArtifactType fromCode(String codeString) throws IllegalArgumentException { 171 if (codeString == null || "".equals(codeString)) 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("documentation".equals(codeString)) 175 return RelatedArtifactType.DOCUMENTATION; 176 if ("justification".equals(codeString)) 177 return RelatedArtifactType.JUSTIFICATION; 178 if ("citation".equals(codeString)) 179 return RelatedArtifactType.CITATION; 180 if ("predecessor".equals(codeString)) 181 return RelatedArtifactType.PREDECESSOR; 182 if ("successor".equals(codeString)) 183 return RelatedArtifactType.SUCCESSOR; 184 if ("derived-from".equals(codeString)) 185 return RelatedArtifactType.DERIVEDFROM; 186 if ("depends-on".equals(codeString)) 187 return RelatedArtifactType.DEPENDSON; 188 if ("composed-of".equals(codeString)) 189 return RelatedArtifactType.COMPOSEDOF; 190 throw new IllegalArgumentException("Unknown RelatedArtifactType code '"+codeString+"'"); 191 } 192 public Enumeration<RelatedArtifactType> fromType(PrimitiveType<?> code) throws FHIRException { 193 if (code == null) 194 return null; 195 if (code.isEmpty()) 196 return new Enumeration<RelatedArtifactType>(this); 197 String codeString = code.asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("documentation".equals(codeString)) 201 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DOCUMENTATION); 202 if ("justification".equals(codeString)) 203 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.JUSTIFICATION); 204 if ("citation".equals(codeString)) 205 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITATION); 206 if ("predecessor".equals(codeString)) 207 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.PREDECESSOR); 208 if ("successor".equals(codeString)) 209 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUCCESSOR); 210 if ("derived-from".equals(codeString)) 211 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DERIVEDFROM); 212 if ("depends-on".equals(codeString)) 213 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DEPENDSON); 214 if ("composed-of".equals(codeString)) 215 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMPOSEDOF); 216 throw new FHIRException("Unknown RelatedArtifactType code '"+codeString+"'"); 217 } 218 public String toCode(RelatedArtifactType code) { 219 if (code == RelatedArtifactType.NULL) 220 return null; 221 if (code == RelatedArtifactType.DOCUMENTATION) 222 return "documentation"; 223 if (code == RelatedArtifactType.JUSTIFICATION) 224 return "justification"; 225 if (code == RelatedArtifactType.CITATION) 226 return "citation"; 227 if (code == RelatedArtifactType.PREDECESSOR) 228 return "predecessor"; 229 if (code == RelatedArtifactType.SUCCESSOR) 230 return "successor"; 231 if (code == RelatedArtifactType.DERIVEDFROM) 232 return "derived-from"; 233 if (code == RelatedArtifactType.DEPENDSON) 234 return "depends-on"; 235 if (code == RelatedArtifactType.COMPOSEDOF) 236 return "composed-of"; 237 return "?"; 238 } 239 public String toSystem(RelatedArtifactType code) { 240 return code.getSystem(); 241 } 242 } 243 244 /** 245 * The type of relationship to the related artifact. 246 */ 247 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 248 @Description(shortDefinition="documentation | justification | citation | predecessor | successor | derived-from | depends-on | composed-of", formalDefinition="The type of relationship to the related artifact." ) 249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-artifact-type") 250 protected Enumeration<RelatedArtifactType> type; 251 252 /** 253 * A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 254 */ 255 @Child(name = "display", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 256 @Description(shortDefinition="Brief description of the related artifact", formalDefinition="A brief description of the document or knowledge resource being referenced, suitable for display to a consumer." ) 257 protected StringType display; 258 259 /** 260 * A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 261 */ 262 @Child(name = "citation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 263 @Description(shortDefinition="Bibliographic citation for the artifact", formalDefinition="A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format." ) 264 protected StringType citation; 265 266 /** 267 * A url for the artifact that can be followed to access the actual content. 268 */ 269 @Child(name = "url", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=true) 270 @Description(shortDefinition="Where the artifact can be accessed", formalDefinition="A url for the artifact that can be followed to access the actual content." ) 271 protected UriType url; 272 273 /** 274 * The document being referenced, represented as an attachment. This is exclusive with the resource element. 275 */ 276 @Child(name = "document", type = {Attachment.class}, order=4, min=0, max=1, modifier=false, summary=true) 277 @Description(shortDefinition="What document is being referenced", formalDefinition="The document being referenced, represented as an attachment. This is exclusive with the resource element." ) 278 protected Attachment document; 279 280 /** 281 * The related resource, such as a library, value set, profile, or other knowledge resource. 282 */ 283 @Child(name = "resource", type = {Reference.class}, order=5, min=0, max=1, modifier=false, summary=true) 284 @Description(shortDefinition="What resource is being referenced", formalDefinition="The related resource, such as a library, value set, profile, or other knowledge resource." ) 285 protected Reference resource; 286 287 /** 288 * The actual object that is the target of the reference (The related resource, such as a library, value set, profile, or other knowledge resource.) 289 */ 290 protected Resource resourceTarget; 291 292 private static final long serialVersionUID = -660871462L; 293 294 /** 295 * Constructor 296 */ 297 public RelatedArtifact() { 298 super(); 299 } 300 301 /** 302 * Constructor 303 */ 304 public RelatedArtifact(Enumeration<RelatedArtifactType> type) { 305 super(); 306 this.type = type; 307 } 308 309 /** 310 * @return {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 311 */ 312 public Enumeration<RelatedArtifactType> getTypeElement() { 313 if (this.type == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create RelatedArtifact.type"); 316 else if (Configuration.doAutoCreate()) 317 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); // bb 318 return this.type; 319 } 320 321 public boolean hasTypeElement() { 322 return this.type != null && !this.type.isEmpty(); 323 } 324 325 public boolean hasType() { 326 return this.type != null && !this.type.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 331 */ 332 public RelatedArtifact setTypeElement(Enumeration<RelatedArtifactType> value) { 333 this.type = value; 334 return this; 335 } 336 337 /** 338 * @return The type of relationship to the related artifact. 339 */ 340 public RelatedArtifactType getType() { 341 return this.type == null ? null : this.type.getValue(); 342 } 343 344 /** 345 * @param value The type of relationship to the related artifact. 346 */ 347 public RelatedArtifact setType(RelatedArtifactType value) { 348 if (this.type == null) 349 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); 350 this.type.setValue(value); 351 return this; 352 } 353 354 /** 355 * @return {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 356 */ 357 public StringType getDisplayElement() { 358 if (this.display == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create RelatedArtifact.display"); 361 else if (Configuration.doAutoCreate()) 362 this.display = new StringType(); // bb 363 return this.display; 364 } 365 366 public boolean hasDisplayElement() { 367 return this.display != null && !this.display.isEmpty(); 368 } 369 370 public boolean hasDisplay() { 371 return this.display != null && !this.display.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 376 */ 377 public RelatedArtifact setDisplayElement(StringType value) { 378 this.display = value; 379 return this; 380 } 381 382 /** 383 * @return A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 384 */ 385 public String getDisplay() { 386 return this.display == null ? null : this.display.getValue(); 387 } 388 389 /** 390 * @param value A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 391 */ 392 public RelatedArtifact setDisplay(String value) { 393 if (Utilities.noString(value)) 394 this.display = null; 395 else { 396 if (this.display == null) 397 this.display = new StringType(); 398 this.display.setValue(value); 399 } 400 return this; 401 } 402 403 /** 404 * @return {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 405 */ 406 public StringType getCitationElement() { 407 if (this.citation == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create RelatedArtifact.citation"); 410 else if (Configuration.doAutoCreate()) 411 this.citation = new StringType(); // bb 412 return this.citation; 413 } 414 415 public boolean hasCitationElement() { 416 return this.citation != null && !this.citation.isEmpty(); 417 } 418 419 public boolean hasCitation() { 420 return this.citation != null && !this.citation.isEmpty(); 421 } 422 423 /** 424 * @param value {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 425 */ 426 public RelatedArtifact setCitationElement(StringType value) { 427 this.citation = value; 428 return this; 429 } 430 431 /** 432 * @return A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 433 */ 434 public String getCitation() { 435 return this.citation == null ? null : this.citation.getValue(); 436 } 437 438 /** 439 * @param value A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 440 */ 441 public RelatedArtifact setCitation(String value) { 442 if (Utilities.noString(value)) 443 this.citation = null; 444 else { 445 if (this.citation == null) 446 this.citation = new StringType(); 447 this.citation.setValue(value); 448 } 449 return this; 450 } 451 452 /** 453 * @return {@link #url} (A url for the artifact that can be followed to access the actual content.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 454 */ 455 public UriType getUrlElement() { 456 if (this.url == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create RelatedArtifact.url"); 459 else if (Configuration.doAutoCreate()) 460 this.url = new UriType(); // bb 461 return this.url; 462 } 463 464 public boolean hasUrlElement() { 465 return this.url != null && !this.url.isEmpty(); 466 } 467 468 public boolean hasUrl() { 469 return this.url != null && !this.url.isEmpty(); 470 } 471 472 /** 473 * @param value {@link #url} (A url for the artifact that can be followed to access the actual content.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 474 */ 475 public RelatedArtifact setUrlElement(UriType value) { 476 this.url = value; 477 return this; 478 } 479 480 /** 481 * @return A url for the artifact that can be followed to access the actual content. 482 */ 483 public String getUrl() { 484 return this.url == null ? null : this.url.getValue(); 485 } 486 487 /** 488 * @param value A url for the artifact that can be followed to access the actual content. 489 */ 490 public RelatedArtifact setUrl(String value) { 491 if (Utilities.noString(value)) 492 this.url = null; 493 else { 494 if (this.url == null) 495 this.url = new UriType(); 496 this.url.setValue(value); 497 } 498 return this; 499 } 500 501 /** 502 * @return {@link #document} (The document being referenced, represented as an attachment. This is exclusive with the resource element.) 503 */ 504 public Attachment getDocument() { 505 if (this.document == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create RelatedArtifact.document"); 508 else if (Configuration.doAutoCreate()) 509 this.document = new Attachment(); // cc 510 return this.document; 511 } 512 513 public boolean hasDocument() { 514 return this.document != null && !this.document.isEmpty(); 515 } 516 517 /** 518 * @param value {@link #document} (The document being referenced, represented as an attachment. This is exclusive with the resource element.) 519 */ 520 public RelatedArtifact setDocument(Attachment value) { 521 this.document = value; 522 return this; 523 } 524 525 /** 526 * @return {@link #resource} (The related resource, such as a library, value set, profile, or other knowledge resource.) 527 */ 528 public Reference getResource() { 529 if (this.resource == null) 530 if (Configuration.errorOnAutoCreate()) 531 throw new Error("Attempt to auto-create RelatedArtifact.resource"); 532 else if (Configuration.doAutoCreate()) 533 this.resource = new Reference(); // cc 534 return this.resource; 535 } 536 537 public boolean hasResource() { 538 return this.resource != null && !this.resource.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #resource} (The related resource, such as a library, value set, profile, or other knowledge resource.) 543 */ 544 public RelatedArtifact setResource(Reference value) { 545 this.resource = value; 546 return this; 547 } 548 549 /** 550 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The related resource, such as a library, value set, profile, or other knowledge resource.) 551 */ 552 public Resource getResourceTarget() { 553 return this.resourceTarget; 554 } 555 556 /** 557 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The related resource, such as a library, value set, profile, or other knowledge resource.) 558 */ 559 public RelatedArtifact setResourceTarget(Resource value) { 560 this.resourceTarget = value; 561 return this; 562 } 563 564 protected void listChildren(List<Property> children) { 565 super.listChildren(children); 566 children.add(new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type)); 567 children.add(new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display)); 568 children.add(new Property("citation", "string", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation)); 569 children.add(new Property("url", "uri", "A url for the artifact that can be followed to access the actual content.", 0, 1, url)); 570 children.add(new Property("document", "Attachment", "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 1, document)); 571 children.add(new Property("resource", "Reference(Any)", "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource)); 572 } 573 574 @Override 575 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 576 switch (_hash) { 577 case 3575610: /*type*/ return new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type); 578 case 1671764162: /*display*/ return new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display); 579 case -1442706713: /*citation*/ return new Property("citation", "string", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation); 580 case 116079: /*url*/ return new Property("url", "uri", "A url for the artifact that can be followed to access the actual content.", 0, 1, url); 581 case 861720859: /*document*/ return new Property("document", "Attachment", "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 1, document); 582 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource); 583 default: return super.getNamedProperty(_hash, _name, _checkValid); 584 } 585 586 } 587 588 @Override 589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 590 switch (hash) { 591 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<RelatedArtifactType> 592 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 593 case -1442706713: /*citation*/ return this.citation == null ? new Base[0] : new Base[] {this.citation}; // StringType 594 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 595 case 861720859: /*document*/ return this.document == null ? new Base[0] : new Base[] {this.document}; // Attachment 596 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 597 default: return super.getProperty(hash, name, checkValid); 598 } 599 600 } 601 602 @Override 603 public Base setProperty(int hash, String name, Base value) throws FHIRException { 604 switch (hash) { 605 case 3575610: // type 606 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 607 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 608 return value; 609 case 1671764162: // display 610 this.display = castToString(value); // StringType 611 return value; 612 case -1442706713: // citation 613 this.citation = castToString(value); // StringType 614 return value; 615 case 116079: // url 616 this.url = castToUri(value); // UriType 617 return value; 618 case 861720859: // document 619 this.document = castToAttachment(value); // Attachment 620 return value; 621 case -341064690: // resource 622 this.resource = castToReference(value); // Reference 623 return value; 624 default: return super.setProperty(hash, name, value); 625 } 626 627 } 628 629 @Override 630 public Base setProperty(String name, Base value) throws FHIRException { 631 if (name.equals("type")) { 632 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 633 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 634 } else if (name.equals("display")) { 635 this.display = castToString(value); // StringType 636 } else if (name.equals("citation")) { 637 this.citation = castToString(value); // StringType 638 } else if (name.equals("url")) { 639 this.url = castToUri(value); // UriType 640 } else if (name.equals("document")) { 641 this.document = castToAttachment(value); // Attachment 642 } else if (name.equals("resource")) { 643 this.resource = castToReference(value); // Reference 644 } else 645 return super.setProperty(name, value); 646 return value; 647 } 648 649 @Override 650 public Base makeProperty(int hash, String name) throws FHIRException { 651 switch (hash) { 652 case 3575610: return getTypeElement(); 653 case 1671764162: return getDisplayElement(); 654 case -1442706713: return getCitationElement(); 655 case 116079: return getUrlElement(); 656 case 861720859: return getDocument(); 657 case -341064690: return getResource(); 658 default: return super.makeProperty(hash, name); 659 } 660 661 } 662 663 @Override 664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 665 switch (hash) { 666 case 3575610: /*type*/ return new String[] {"code"}; 667 case 1671764162: /*display*/ return new String[] {"string"}; 668 case -1442706713: /*citation*/ return new String[] {"string"}; 669 case 116079: /*url*/ return new String[] {"uri"}; 670 case 861720859: /*document*/ return new String[] {"Attachment"}; 671 case -341064690: /*resource*/ return new String[] {"Reference"}; 672 default: return super.getTypesForProperty(hash, name); 673 } 674 675 } 676 677 @Override 678 public Base addChild(String name) throws FHIRException { 679 if (name.equals("type")) { 680 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.type"); 681 } 682 else if (name.equals("display")) { 683 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.display"); 684 } 685 else if (name.equals("citation")) { 686 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.citation"); 687 } 688 else if (name.equals("url")) { 689 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.url"); 690 } 691 else if (name.equals("document")) { 692 this.document = new Attachment(); 693 return this.document; 694 } 695 else if (name.equals("resource")) { 696 this.resource = new Reference(); 697 return this.resource; 698 } 699 else 700 return super.addChild(name); 701 } 702 703 public String fhirType() { 704 return "RelatedArtifact"; 705 706 } 707 708 public RelatedArtifact copy() { 709 RelatedArtifact dst = new RelatedArtifact(); 710 copyValues(dst); 711 dst.type = type == null ? null : type.copy(); 712 dst.display = display == null ? null : display.copy(); 713 dst.citation = citation == null ? null : citation.copy(); 714 dst.url = url == null ? null : url.copy(); 715 dst.document = document == null ? null : document.copy(); 716 dst.resource = resource == null ? null : resource.copy(); 717 return dst; 718 } 719 720 protected RelatedArtifact typedCopy() { 721 return copy(); 722 } 723 724 @Override 725 public boolean equalsDeep(Base other_) { 726 if (!super.equalsDeep(other_)) 727 return false; 728 if (!(other_ instanceof RelatedArtifact)) 729 return false; 730 RelatedArtifact o = (RelatedArtifact) other_; 731 return compareDeep(type, o.type, true) && compareDeep(display, o.display, true) && compareDeep(citation, o.citation, true) 732 && compareDeep(url, o.url, true) && compareDeep(document, o.document, true) && compareDeep(resource, o.resource, true) 733 ; 734 } 735 736 @Override 737 public boolean equalsShallow(Base other_) { 738 if (!super.equalsShallow(other_)) 739 return false; 740 if (!(other_ instanceof RelatedArtifact)) 741 return false; 742 RelatedArtifact o = (RelatedArtifact) other_; 743 return compareValues(type, o.type, true) && compareValues(display, o.display, true) && compareValues(citation, o.citation, true) 744 && compareValues(url, o.url, true); 745 } 746 747 public boolean isEmpty() { 748 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, display, citation 749 , url, document, resource); 750 } 751 752 753}