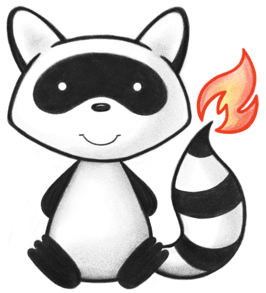
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 049 */ 050@ResourceDef(name="RelatedPerson", profile="http://hl7.org/fhir/Profile/RelatedPerson") 051public class RelatedPerson extends DomainResource { 052 053 /** 054 * Identifier for a person within a particular scope. 055 */ 056 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 057 @Description(shortDefinition="A human identifier for this person", formalDefinition="Identifier for a person within a particular scope." ) 058 protected List<Identifier> identifier; 059 060 /** 061 * Whether this related person record is in active use. 062 */ 063 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 064 @Description(shortDefinition="Whether this related person's record is in active use", formalDefinition="Whether this related person record is in active use." ) 065 protected BooleanType active; 066 067 /** 068 * The patient this person is related to. 069 */ 070 @Child(name = "patient", type = {Patient.class}, order=2, min=1, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="The patient this person is related to", formalDefinition="The patient this person is related to." ) 072 protected Reference patient; 073 074 /** 075 * The actual object that is the target of the reference (The patient this person is related to.) 076 */ 077 protected Patient patientTarget; 078 079 /** 080 * The nature of the relationship between a patient and the related person. 081 */ 082 @Child(name = "relationship", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="The nature of the relationship", formalDefinition="The nature of the relationship between a patient and the related person." ) 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/relatedperson-relationshiptype") 085 protected CodeableConcept relationship; 086 087 /** 088 * A name associated with the person. 089 */ 090 @Child(name = "name", type = {HumanName.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 091 @Description(shortDefinition="A name associated with the person", formalDefinition="A name associated with the person." ) 092 protected List<HumanName> name; 093 094 /** 095 * A contact detail for the person, e.g. a telephone number or an email address. 096 */ 097 @Child(name = "telecom", type = {ContactPoint.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 098 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 099 protected List<ContactPoint> telecom; 100 101 /** 102 * Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 103 */ 104 @Child(name = "gender", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 105 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes." ) 106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 107 protected Enumeration<AdministrativeGender> gender; 108 109 /** 110 * The date on which the related person was born. 111 */ 112 @Child(name = "birthDate", type = {DateType.class}, order=7, min=0, max=1, modifier=false, summary=true) 113 @Description(shortDefinition="The date on which the related person was born", formalDefinition="The date on which the related person was born." ) 114 protected DateType birthDate; 115 116 /** 117 * Address where the related person can be contacted or visited. 118 */ 119 @Child(name = "address", type = {Address.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 120 @Description(shortDefinition="Address where the related person can be contacted or visited", formalDefinition="Address where the related person can be contacted or visited." ) 121 protected List<Address> address; 122 123 /** 124 * Image of the person. 125 */ 126 @Child(name = "photo", type = {Attachment.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 127 @Description(shortDefinition="Image of the person", formalDefinition="Image of the person." ) 128 protected List<Attachment> photo; 129 130 /** 131 * The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown. 132 */ 133 @Child(name = "period", type = {Period.class}, order=10, min=0, max=1, modifier=false, summary=false) 134 @Description(shortDefinition="Period of time that this relationship is considered valid", formalDefinition="The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown." ) 135 protected Period period; 136 137 private static final long serialVersionUID = 1633785157L; 138 139 /** 140 * Constructor 141 */ 142 public RelatedPerson() { 143 super(); 144 } 145 146 /** 147 * Constructor 148 */ 149 public RelatedPerson(Reference patient) { 150 super(); 151 this.patient = patient; 152 } 153 154 /** 155 * @return {@link #identifier} (Identifier for a person within a particular scope.) 156 */ 157 public List<Identifier> getIdentifier() { 158 if (this.identifier == null) 159 this.identifier = new ArrayList<Identifier>(); 160 return this.identifier; 161 } 162 163 /** 164 * @return Returns a reference to <code>this</code> for easy method chaining 165 */ 166 public RelatedPerson setIdentifier(List<Identifier> theIdentifier) { 167 this.identifier = theIdentifier; 168 return this; 169 } 170 171 public boolean hasIdentifier() { 172 if (this.identifier == null) 173 return false; 174 for (Identifier item : this.identifier) 175 if (!item.isEmpty()) 176 return true; 177 return false; 178 } 179 180 public Identifier addIdentifier() { //3 181 Identifier t = new Identifier(); 182 if (this.identifier == null) 183 this.identifier = new ArrayList<Identifier>(); 184 this.identifier.add(t); 185 return t; 186 } 187 188 public RelatedPerson addIdentifier(Identifier t) { //3 189 if (t == null) 190 return this; 191 if (this.identifier == null) 192 this.identifier = new ArrayList<Identifier>(); 193 this.identifier.add(t); 194 return this; 195 } 196 197 /** 198 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 199 */ 200 public Identifier getIdentifierFirstRep() { 201 if (getIdentifier().isEmpty()) { 202 addIdentifier(); 203 } 204 return getIdentifier().get(0); 205 } 206 207 /** 208 * @return {@link #active} (Whether this related person record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 209 */ 210 public BooleanType getActiveElement() { 211 if (this.active == null) 212 if (Configuration.errorOnAutoCreate()) 213 throw new Error("Attempt to auto-create RelatedPerson.active"); 214 else if (Configuration.doAutoCreate()) 215 this.active = new BooleanType(); // bb 216 return this.active; 217 } 218 219 public boolean hasActiveElement() { 220 return this.active != null && !this.active.isEmpty(); 221 } 222 223 public boolean hasActive() { 224 return this.active != null && !this.active.isEmpty(); 225 } 226 227 /** 228 * @param value {@link #active} (Whether this related person record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 229 */ 230 public RelatedPerson setActiveElement(BooleanType value) { 231 this.active = value; 232 return this; 233 } 234 235 /** 236 * @return Whether this related person record is in active use. 237 */ 238 public boolean getActive() { 239 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 240 } 241 242 /** 243 * @param value Whether this related person record is in active use. 244 */ 245 public RelatedPerson setActive(boolean value) { 246 if (this.active == null) 247 this.active = new BooleanType(); 248 this.active.setValue(value); 249 return this; 250 } 251 252 /** 253 * @return {@link #patient} (The patient this person is related to.) 254 */ 255 public Reference getPatient() { 256 if (this.patient == null) 257 if (Configuration.errorOnAutoCreate()) 258 throw new Error("Attempt to auto-create RelatedPerson.patient"); 259 else if (Configuration.doAutoCreate()) 260 this.patient = new Reference(); // cc 261 return this.patient; 262 } 263 264 public boolean hasPatient() { 265 return this.patient != null && !this.patient.isEmpty(); 266 } 267 268 /** 269 * @param value {@link #patient} (The patient this person is related to.) 270 */ 271 public RelatedPerson setPatient(Reference value) { 272 this.patient = value; 273 return this; 274 } 275 276 /** 277 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient this person is related to.) 278 */ 279 public Patient getPatientTarget() { 280 if (this.patientTarget == null) 281 if (Configuration.errorOnAutoCreate()) 282 throw new Error("Attempt to auto-create RelatedPerson.patient"); 283 else if (Configuration.doAutoCreate()) 284 this.patientTarget = new Patient(); // aa 285 return this.patientTarget; 286 } 287 288 /** 289 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient this person is related to.) 290 */ 291 public RelatedPerson setPatientTarget(Patient value) { 292 this.patientTarget = value; 293 return this; 294 } 295 296 /** 297 * @return {@link #relationship} (The nature of the relationship between a patient and the related person.) 298 */ 299 public CodeableConcept getRelationship() { 300 if (this.relationship == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create RelatedPerson.relationship"); 303 else if (Configuration.doAutoCreate()) 304 this.relationship = new CodeableConcept(); // cc 305 return this.relationship; 306 } 307 308 public boolean hasRelationship() { 309 return this.relationship != null && !this.relationship.isEmpty(); 310 } 311 312 /** 313 * @param value {@link #relationship} (The nature of the relationship between a patient and the related person.) 314 */ 315 public RelatedPerson setRelationship(CodeableConcept value) { 316 this.relationship = value; 317 return this; 318 } 319 320 /** 321 * @return {@link #name} (A name associated with the person.) 322 */ 323 public List<HumanName> getName() { 324 if (this.name == null) 325 this.name = new ArrayList<HumanName>(); 326 return this.name; 327 } 328 329 /** 330 * @return Returns a reference to <code>this</code> for easy method chaining 331 */ 332 public RelatedPerson setName(List<HumanName> theName) { 333 this.name = theName; 334 return this; 335 } 336 337 public boolean hasName() { 338 if (this.name == null) 339 return false; 340 for (HumanName item : this.name) 341 if (!item.isEmpty()) 342 return true; 343 return false; 344 } 345 346 public HumanName addName() { //3 347 HumanName t = new HumanName(); 348 if (this.name == null) 349 this.name = new ArrayList<HumanName>(); 350 this.name.add(t); 351 return t; 352 } 353 354 public RelatedPerson addName(HumanName t) { //3 355 if (t == null) 356 return this; 357 if (this.name == null) 358 this.name = new ArrayList<HumanName>(); 359 this.name.add(t); 360 return this; 361 } 362 363 /** 364 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist 365 */ 366 public HumanName getNameFirstRep() { 367 if (getName().isEmpty()) { 368 addName(); 369 } 370 return getName().get(0); 371 } 372 373 /** 374 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 375 */ 376 public List<ContactPoint> getTelecom() { 377 if (this.telecom == null) 378 this.telecom = new ArrayList<ContactPoint>(); 379 return this.telecom; 380 } 381 382 /** 383 * @return Returns a reference to <code>this</code> for easy method chaining 384 */ 385 public RelatedPerson setTelecom(List<ContactPoint> theTelecom) { 386 this.telecom = theTelecom; 387 return this; 388 } 389 390 public boolean hasTelecom() { 391 if (this.telecom == null) 392 return false; 393 for (ContactPoint item : this.telecom) 394 if (!item.isEmpty()) 395 return true; 396 return false; 397 } 398 399 public ContactPoint addTelecom() { //3 400 ContactPoint t = new ContactPoint(); 401 if (this.telecom == null) 402 this.telecom = new ArrayList<ContactPoint>(); 403 this.telecom.add(t); 404 return t; 405 } 406 407 public RelatedPerson addTelecom(ContactPoint t) { //3 408 if (t == null) 409 return this; 410 if (this.telecom == null) 411 this.telecom = new ArrayList<ContactPoint>(); 412 this.telecom.add(t); 413 return this; 414 } 415 416 /** 417 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 418 */ 419 public ContactPoint getTelecomFirstRep() { 420 if (getTelecom().isEmpty()) { 421 addTelecom(); 422 } 423 return getTelecom().get(0); 424 } 425 426 /** 427 * @return {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 428 */ 429 public Enumeration<AdministrativeGender> getGenderElement() { 430 if (this.gender == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create RelatedPerson.gender"); 433 else if (Configuration.doAutoCreate()) 434 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 435 return this.gender; 436 } 437 438 public boolean hasGenderElement() { 439 return this.gender != null && !this.gender.isEmpty(); 440 } 441 442 public boolean hasGender() { 443 return this.gender != null && !this.gender.isEmpty(); 444 } 445 446 /** 447 * @param value {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 448 */ 449 public RelatedPerson setGenderElement(Enumeration<AdministrativeGender> value) { 450 this.gender = value; 451 return this; 452 } 453 454 /** 455 * @return Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 456 */ 457 public AdministrativeGender getGender() { 458 return this.gender == null ? null : this.gender.getValue(); 459 } 460 461 /** 462 * @param value Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 463 */ 464 public RelatedPerson setGender(AdministrativeGender value) { 465 if (value == null) 466 this.gender = null; 467 else { 468 if (this.gender == null) 469 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 470 this.gender.setValue(value); 471 } 472 return this; 473 } 474 475 /** 476 * @return {@link #birthDate} (The date on which the related person was born.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 477 */ 478 public DateType getBirthDateElement() { 479 if (this.birthDate == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create RelatedPerson.birthDate"); 482 else if (Configuration.doAutoCreate()) 483 this.birthDate = new DateType(); // bb 484 return this.birthDate; 485 } 486 487 public boolean hasBirthDateElement() { 488 return this.birthDate != null && !this.birthDate.isEmpty(); 489 } 490 491 public boolean hasBirthDate() { 492 return this.birthDate != null && !this.birthDate.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #birthDate} (The date on which the related person was born.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 497 */ 498 public RelatedPerson setBirthDateElement(DateType value) { 499 this.birthDate = value; 500 return this; 501 } 502 503 /** 504 * @return The date on which the related person was born. 505 */ 506 public Date getBirthDate() { 507 return this.birthDate == null ? null : this.birthDate.getValue(); 508 } 509 510 /** 511 * @param value The date on which the related person was born. 512 */ 513 public RelatedPerson setBirthDate(Date value) { 514 if (value == null) 515 this.birthDate = null; 516 else { 517 if (this.birthDate == null) 518 this.birthDate = new DateType(); 519 this.birthDate.setValue(value); 520 } 521 return this; 522 } 523 524 /** 525 * @return {@link #address} (Address where the related person can be contacted or visited.) 526 */ 527 public List<Address> getAddress() { 528 if (this.address == null) 529 this.address = new ArrayList<Address>(); 530 return this.address; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public RelatedPerson setAddress(List<Address> theAddress) { 537 this.address = theAddress; 538 return this; 539 } 540 541 public boolean hasAddress() { 542 if (this.address == null) 543 return false; 544 for (Address item : this.address) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 public Address addAddress() { //3 551 Address t = new Address(); 552 if (this.address == null) 553 this.address = new ArrayList<Address>(); 554 this.address.add(t); 555 return t; 556 } 557 558 public RelatedPerson addAddress(Address t) { //3 559 if (t == null) 560 return this; 561 if (this.address == null) 562 this.address = new ArrayList<Address>(); 563 this.address.add(t); 564 return this; 565 } 566 567 /** 568 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist 569 */ 570 public Address getAddressFirstRep() { 571 if (getAddress().isEmpty()) { 572 addAddress(); 573 } 574 return getAddress().get(0); 575 } 576 577 /** 578 * @return {@link #photo} (Image of the person.) 579 */ 580 public List<Attachment> getPhoto() { 581 if (this.photo == null) 582 this.photo = new ArrayList<Attachment>(); 583 return this.photo; 584 } 585 586 /** 587 * @return Returns a reference to <code>this</code> for easy method chaining 588 */ 589 public RelatedPerson setPhoto(List<Attachment> thePhoto) { 590 this.photo = thePhoto; 591 return this; 592 } 593 594 public boolean hasPhoto() { 595 if (this.photo == null) 596 return false; 597 for (Attachment item : this.photo) 598 if (!item.isEmpty()) 599 return true; 600 return false; 601 } 602 603 public Attachment addPhoto() { //3 604 Attachment t = new Attachment(); 605 if (this.photo == null) 606 this.photo = new ArrayList<Attachment>(); 607 this.photo.add(t); 608 return t; 609 } 610 611 public RelatedPerson addPhoto(Attachment t) { //3 612 if (t == null) 613 return this; 614 if (this.photo == null) 615 this.photo = new ArrayList<Attachment>(); 616 this.photo.add(t); 617 return this; 618 } 619 620 /** 621 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist 622 */ 623 public Attachment getPhotoFirstRep() { 624 if (getPhoto().isEmpty()) { 625 addPhoto(); 626 } 627 return getPhoto().get(0); 628 } 629 630 /** 631 * @return {@link #period} (The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.) 632 */ 633 public Period getPeriod() { 634 if (this.period == null) 635 if (Configuration.errorOnAutoCreate()) 636 throw new Error("Attempt to auto-create RelatedPerson.period"); 637 else if (Configuration.doAutoCreate()) 638 this.period = new Period(); // cc 639 return this.period; 640 } 641 642 public boolean hasPeriod() { 643 return this.period != null && !this.period.isEmpty(); 644 } 645 646 /** 647 * @param value {@link #period} (The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.) 648 */ 649 public RelatedPerson setPeriod(Period value) { 650 this.period = value; 651 return this; 652 } 653 654 protected void listChildren(List<Property> children) { 655 super.listChildren(children); 656 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier)); 657 children.add(new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1, active)); 658 children.add(new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, patient)); 659 children.add(new Property("relationship", "CodeableConcept", "The nature of the relationship between a patient and the related person.", 0, 1, relationship)); 660 children.add(new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 661 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 662 children.add(new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 663 children.add(new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, birthDate)); 664 children.add(new Property("address", "Address", "Address where the related person can be contacted or visited.", 0, java.lang.Integer.MAX_VALUE, address)); 665 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 666 children.add(new Property("period", "Period", "The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.", 0, 1, period)); 667 } 668 669 @Override 670 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 671 switch (_hash) { 672 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 673 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1, active); 674 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, patient); 675 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The nature of the relationship between a patient and the related person.", 0, 1, relationship); 676 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name); 677 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 678 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender); 679 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, birthDate); 680 case -1147692044: /*address*/ return new Property("address", "Address", "Address where the related person can be contacted or visited.", 0, java.lang.Integer.MAX_VALUE, address); 681 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo); 682 case -991726143: /*period*/ return new Property("period", "Period", "The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.", 0, 1, period); 683 default: return super.getNamedProperty(_hash, _name, _checkValid); 684 } 685 686 } 687 688 @Override 689 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 690 switch (hash) { 691 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 692 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 693 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 694 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 695 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 696 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 697 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 698 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 699 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 700 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 701 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 702 default: return super.getProperty(hash, name, checkValid); 703 } 704 705 } 706 707 @Override 708 public Base setProperty(int hash, String name, Base value) throws FHIRException { 709 switch (hash) { 710 case -1618432855: // identifier 711 this.getIdentifier().add(castToIdentifier(value)); // Identifier 712 return value; 713 case -1422950650: // active 714 this.active = castToBoolean(value); // BooleanType 715 return value; 716 case -791418107: // patient 717 this.patient = castToReference(value); // Reference 718 return value; 719 case -261851592: // relationship 720 this.relationship = castToCodeableConcept(value); // CodeableConcept 721 return value; 722 case 3373707: // name 723 this.getName().add(castToHumanName(value)); // HumanName 724 return value; 725 case -1429363305: // telecom 726 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 727 return value; 728 case -1249512767: // gender 729 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 730 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 731 return value; 732 case -1210031859: // birthDate 733 this.birthDate = castToDate(value); // DateType 734 return value; 735 case -1147692044: // address 736 this.getAddress().add(castToAddress(value)); // Address 737 return value; 738 case 106642994: // photo 739 this.getPhoto().add(castToAttachment(value)); // Attachment 740 return value; 741 case -991726143: // period 742 this.period = castToPeriod(value); // Period 743 return value; 744 default: return super.setProperty(hash, name, value); 745 } 746 747 } 748 749 @Override 750 public Base setProperty(String name, Base value) throws FHIRException { 751 if (name.equals("identifier")) { 752 this.getIdentifier().add(castToIdentifier(value)); 753 } else if (name.equals("active")) { 754 this.active = castToBoolean(value); // BooleanType 755 } else if (name.equals("patient")) { 756 this.patient = castToReference(value); // Reference 757 } else if (name.equals("relationship")) { 758 this.relationship = castToCodeableConcept(value); // CodeableConcept 759 } else if (name.equals("name")) { 760 this.getName().add(castToHumanName(value)); 761 } else if (name.equals("telecom")) { 762 this.getTelecom().add(castToContactPoint(value)); 763 } else if (name.equals("gender")) { 764 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 765 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 766 } else if (name.equals("birthDate")) { 767 this.birthDate = castToDate(value); // DateType 768 } else if (name.equals("address")) { 769 this.getAddress().add(castToAddress(value)); 770 } else if (name.equals("photo")) { 771 this.getPhoto().add(castToAttachment(value)); 772 } else if (name.equals("period")) { 773 this.period = castToPeriod(value); // Period 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public Base makeProperty(int hash, String name) throws FHIRException { 781 switch (hash) { 782 case -1618432855: return addIdentifier(); 783 case -1422950650: return getActiveElement(); 784 case -791418107: return getPatient(); 785 case -261851592: return getRelationship(); 786 case 3373707: return addName(); 787 case -1429363305: return addTelecom(); 788 case -1249512767: return getGenderElement(); 789 case -1210031859: return getBirthDateElement(); 790 case -1147692044: return addAddress(); 791 case 106642994: return addPhoto(); 792 case -991726143: return getPeriod(); 793 default: return super.makeProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 800 switch (hash) { 801 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 802 case -1422950650: /*active*/ return new String[] {"boolean"}; 803 case -791418107: /*patient*/ return new String[] {"Reference"}; 804 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 805 case 3373707: /*name*/ return new String[] {"HumanName"}; 806 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 807 case -1249512767: /*gender*/ return new String[] {"code"}; 808 case -1210031859: /*birthDate*/ return new String[] {"date"}; 809 case -1147692044: /*address*/ return new String[] {"Address"}; 810 case 106642994: /*photo*/ return new String[] {"Attachment"}; 811 case -991726143: /*period*/ return new String[] {"Period"}; 812 default: return super.getTypesForProperty(hash, name); 813 } 814 815 } 816 817 @Override 818 public Base addChild(String name) throws FHIRException { 819 if (name.equals("identifier")) { 820 return addIdentifier(); 821 } 822 else if (name.equals("active")) { 823 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.active"); 824 } 825 else if (name.equals("patient")) { 826 this.patient = new Reference(); 827 return this.patient; 828 } 829 else if (name.equals("relationship")) { 830 this.relationship = new CodeableConcept(); 831 return this.relationship; 832 } 833 else if (name.equals("name")) { 834 return addName(); 835 } 836 else if (name.equals("telecom")) { 837 return addTelecom(); 838 } 839 else if (name.equals("gender")) { 840 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.gender"); 841 } 842 else if (name.equals("birthDate")) { 843 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.birthDate"); 844 } 845 else if (name.equals("address")) { 846 return addAddress(); 847 } 848 else if (name.equals("photo")) { 849 return addPhoto(); 850 } 851 else if (name.equals("period")) { 852 this.period = new Period(); 853 return this.period; 854 } 855 else 856 return super.addChild(name); 857 } 858 859 public String fhirType() { 860 return "RelatedPerson"; 861 862 } 863 864 public RelatedPerson copy() { 865 RelatedPerson dst = new RelatedPerson(); 866 copyValues(dst); 867 if (identifier != null) { 868 dst.identifier = new ArrayList<Identifier>(); 869 for (Identifier i : identifier) 870 dst.identifier.add(i.copy()); 871 }; 872 dst.active = active == null ? null : active.copy(); 873 dst.patient = patient == null ? null : patient.copy(); 874 dst.relationship = relationship == null ? null : relationship.copy(); 875 if (name != null) { 876 dst.name = new ArrayList<HumanName>(); 877 for (HumanName i : name) 878 dst.name.add(i.copy()); 879 }; 880 if (telecom != null) { 881 dst.telecom = new ArrayList<ContactPoint>(); 882 for (ContactPoint i : telecom) 883 dst.telecom.add(i.copy()); 884 }; 885 dst.gender = gender == null ? null : gender.copy(); 886 dst.birthDate = birthDate == null ? null : birthDate.copy(); 887 if (address != null) { 888 dst.address = new ArrayList<Address>(); 889 for (Address i : address) 890 dst.address.add(i.copy()); 891 }; 892 if (photo != null) { 893 dst.photo = new ArrayList<Attachment>(); 894 for (Attachment i : photo) 895 dst.photo.add(i.copy()); 896 }; 897 dst.period = period == null ? null : period.copy(); 898 return dst; 899 } 900 901 protected RelatedPerson typedCopy() { 902 return copy(); 903 } 904 905 @Override 906 public boolean equalsDeep(Base other_) { 907 if (!super.equalsDeep(other_)) 908 return false; 909 if (!(other_ instanceof RelatedPerson)) 910 return false; 911 RelatedPerson o = (RelatedPerson) other_; 912 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(patient, o.patient, true) 913 && compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 914 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 915 && compareDeep(photo, o.photo, true) && compareDeep(period, o.period, true); 916 } 917 918 @Override 919 public boolean equalsShallow(Base other_) { 920 if (!super.equalsShallow(other_)) 921 return false; 922 if (!(other_ instanceof RelatedPerson)) 923 return false; 924 RelatedPerson o = (RelatedPerson) other_; 925 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 926 ; 927 } 928 929 public boolean isEmpty() { 930 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, patient 931 , relationship, name, telecom, gender, birthDate, address, photo, period); 932 } 933 934 @Override 935 public ResourceType getResourceType() { 936 return ResourceType.RelatedPerson; 937 } 938 939 /** 940 * Search parameter: <b>identifier</b> 941 * <p> 942 * Description: <b>An Identifier of the RelatedPerson</b><br> 943 * Type: <b>token</b><br> 944 * Path: <b>RelatedPerson.identifier</b><br> 945 * </p> 946 */ 947 @SearchParamDefinition(name="identifier", path="RelatedPerson.identifier", description="An Identifier of the RelatedPerson", type="token" ) 948 public static final String SP_IDENTIFIER = "identifier"; 949 /** 950 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 951 * <p> 952 * Description: <b>An Identifier of the RelatedPerson</b><br> 953 * Type: <b>token</b><br> 954 * Path: <b>RelatedPerson.identifier</b><br> 955 * </p> 956 */ 957 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 958 959 /** 960 * Search parameter: <b>address</b> 961 * <p> 962 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 963 * Type: <b>string</b><br> 964 * Path: <b>RelatedPerson.address</b><br> 965 * </p> 966 */ 967 @SearchParamDefinition(name="address", path="RelatedPerson.address", description="A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text", type="string" ) 968 public static final String SP_ADDRESS = "address"; 969 /** 970 * <b>Fluent Client</b> search parameter constant for <b>address</b> 971 * <p> 972 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, state, country, postalCode, and/or text</b><br> 973 * Type: <b>string</b><br> 974 * Path: <b>RelatedPerson.address</b><br> 975 * </p> 976 */ 977 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 978 979 /** 980 * Search parameter: <b>birthdate</b> 981 * <p> 982 * Description: <b>The Related Person's date of birth</b><br> 983 * Type: <b>date</b><br> 984 * Path: <b>RelatedPerson.birthDate</b><br> 985 * </p> 986 */ 987 @SearchParamDefinition(name="birthdate", path="RelatedPerson.birthDate", description="The Related Person's date of birth", type="date" ) 988 public static final String SP_BIRTHDATE = "birthdate"; 989 /** 990 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 991 * <p> 992 * Description: <b>The Related Person's date of birth</b><br> 993 * Type: <b>date</b><br> 994 * Path: <b>RelatedPerson.birthDate</b><br> 995 * </p> 996 */ 997 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 998 999 /** 1000 * Search parameter: <b>address-state</b> 1001 * <p> 1002 * Description: <b>A state specified in an address</b><br> 1003 * Type: <b>string</b><br> 1004 * Path: <b>RelatedPerson.address.state</b><br> 1005 * </p> 1006 */ 1007 @SearchParamDefinition(name="address-state", path="RelatedPerson.address.state", description="A state specified in an address", type="string" ) 1008 public static final String SP_ADDRESS_STATE = "address-state"; 1009 /** 1010 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1011 * <p> 1012 * Description: <b>A state specified in an address</b><br> 1013 * Type: <b>string</b><br> 1014 * Path: <b>RelatedPerson.address.state</b><br> 1015 * </p> 1016 */ 1017 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1018 1019 /** 1020 * Search parameter: <b>gender</b> 1021 * <p> 1022 * Description: <b>Gender of the related person</b><br> 1023 * Type: <b>token</b><br> 1024 * Path: <b>RelatedPerson.gender</b><br> 1025 * </p> 1026 */ 1027 @SearchParamDefinition(name="gender", path="RelatedPerson.gender", description="Gender of the related person", type="token" ) 1028 public static final String SP_GENDER = "gender"; 1029 /** 1030 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1031 * <p> 1032 * Description: <b>Gender of the related person</b><br> 1033 * Type: <b>token</b><br> 1034 * Path: <b>RelatedPerson.gender</b><br> 1035 * </p> 1036 */ 1037 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 1038 1039 /** 1040 * Search parameter: <b>active</b> 1041 * <p> 1042 * Description: <b>Indicates if the related person record is active</b><br> 1043 * Type: <b>token</b><br> 1044 * Path: <b>RelatedPerson.active</b><br> 1045 * </p> 1046 */ 1047 @SearchParamDefinition(name="active", path="RelatedPerson.active", description="Indicates if the related person record is active", type="token" ) 1048 public static final String SP_ACTIVE = "active"; 1049 /** 1050 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1051 * <p> 1052 * Description: <b>Indicates if the related person record is active</b><br> 1053 * Type: <b>token</b><br> 1054 * Path: <b>RelatedPerson.active</b><br> 1055 * </p> 1056 */ 1057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1058 1059 /** 1060 * Search parameter: <b>address-postalcode</b> 1061 * <p> 1062 * Description: <b>A postal code specified in an address</b><br> 1063 * Type: <b>string</b><br> 1064 * Path: <b>RelatedPerson.address.postalCode</b><br> 1065 * </p> 1066 */ 1067 @SearchParamDefinition(name="address-postalcode", path="RelatedPerson.address.postalCode", description="A postal code specified in an address", type="string" ) 1068 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1069 /** 1070 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1071 * <p> 1072 * Description: <b>A postal code specified in an address</b><br> 1073 * Type: <b>string</b><br> 1074 * Path: <b>RelatedPerson.address.postalCode</b><br> 1075 * </p> 1076 */ 1077 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1078 1079 /** 1080 * Search parameter: <b>address-country</b> 1081 * <p> 1082 * Description: <b>A country specified in an address</b><br> 1083 * Type: <b>string</b><br> 1084 * Path: <b>RelatedPerson.address.country</b><br> 1085 * </p> 1086 */ 1087 @SearchParamDefinition(name="address-country", path="RelatedPerson.address.country", description="A country specified in an address", type="string" ) 1088 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1089 /** 1090 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1091 * <p> 1092 * Description: <b>A country specified in an address</b><br> 1093 * Type: <b>string</b><br> 1094 * Path: <b>RelatedPerson.address.country</b><br> 1095 * </p> 1096 */ 1097 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1098 1099 /** 1100 * Search parameter: <b>phonetic</b> 1101 * <p> 1102 * Description: <b>A portion of name using some kind of phonetic matching algorithm</b><br> 1103 * Type: <b>string</b><br> 1104 * Path: <b>RelatedPerson.name</b><br> 1105 * </p> 1106 */ 1107 @SearchParamDefinition(name="phonetic", path="RelatedPerson.name", description="A portion of name using some kind of phonetic matching algorithm", type="string" ) 1108 public static final String SP_PHONETIC = "phonetic"; 1109 /** 1110 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1111 * <p> 1112 * Description: <b>A portion of name using some kind of phonetic matching algorithm</b><br> 1113 * Type: <b>string</b><br> 1114 * Path: <b>RelatedPerson.name</b><br> 1115 * </p> 1116 */ 1117 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 1118 1119 /** 1120 * Search parameter: <b>phone</b> 1121 * <p> 1122 * Description: <b>A value in a phone contact</b><br> 1123 * Type: <b>token</b><br> 1124 * Path: <b>RelatedPerson.telecom(system=phone)</b><br> 1125 * </p> 1126 */ 1127 @SearchParamDefinition(name="phone", path="RelatedPerson.telecom.where(system='phone')", description="A value in a phone contact", type="token" ) 1128 public static final String SP_PHONE = "phone"; 1129 /** 1130 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1131 * <p> 1132 * Description: <b>A value in a phone contact</b><br> 1133 * Type: <b>token</b><br> 1134 * Path: <b>RelatedPerson.telecom(system=phone)</b><br> 1135 * </p> 1136 */ 1137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 1138 1139 /** 1140 * Search parameter: <b>patient</b> 1141 * <p> 1142 * Description: <b>The patient this related person is related to</b><br> 1143 * Type: <b>reference</b><br> 1144 * Path: <b>RelatedPerson.patient</b><br> 1145 * </p> 1146 */ 1147 @SearchParamDefinition(name="patient", path="RelatedPerson.patient", description="The patient this related person is related to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1148 public static final String SP_PATIENT = "patient"; 1149 /** 1150 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1151 * <p> 1152 * Description: <b>The patient this related person is related to</b><br> 1153 * Type: <b>reference</b><br> 1154 * Path: <b>RelatedPerson.patient</b><br> 1155 * </p> 1156 */ 1157 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1158 1159/** 1160 * Constant for fluent queries to be used to add include statements. Specifies 1161 * the path value of "<b>RelatedPerson:patient</b>". 1162 */ 1163 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RelatedPerson:patient").toLocked(); 1164 1165 /** 1166 * Search parameter: <b>name</b> 1167 * <p> 1168 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1169 * Type: <b>string</b><br> 1170 * Path: <b>RelatedPerson.name</b><br> 1171 * </p> 1172 */ 1173 @SearchParamDefinition(name="name", path="RelatedPerson.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1174 public static final String SP_NAME = "name"; 1175 /** 1176 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1177 * <p> 1178 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1179 * Type: <b>string</b><br> 1180 * Path: <b>RelatedPerson.name</b><br> 1181 * </p> 1182 */ 1183 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1184 1185 /** 1186 * Search parameter: <b>address-use</b> 1187 * <p> 1188 * Description: <b>A use code specified in an address</b><br> 1189 * Type: <b>token</b><br> 1190 * Path: <b>RelatedPerson.address.use</b><br> 1191 * </p> 1192 */ 1193 @SearchParamDefinition(name="address-use", path="RelatedPerson.address.use", description="A use code specified in an address", type="token" ) 1194 public static final String SP_ADDRESS_USE = "address-use"; 1195 /** 1196 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1197 * <p> 1198 * Description: <b>A use code specified in an address</b><br> 1199 * Type: <b>token</b><br> 1200 * Path: <b>RelatedPerson.address.use</b><br> 1201 * </p> 1202 */ 1203 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1204 1205 /** 1206 * Search parameter: <b>telecom</b> 1207 * <p> 1208 * Description: <b>The value in any kind of contact</b><br> 1209 * Type: <b>token</b><br> 1210 * Path: <b>RelatedPerson.telecom</b><br> 1211 * </p> 1212 */ 1213 @SearchParamDefinition(name="telecom", path="RelatedPerson.telecom", description="The value in any kind of contact", type="token" ) 1214 public static final String SP_TELECOM = "telecom"; 1215 /** 1216 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1217 * <p> 1218 * Description: <b>The value in any kind of contact</b><br> 1219 * Type: <b>token</b><br> 1220 * Path: <b>RelatedPerson.telecom</b><br> 1221 * </p> 1222 */ 1223 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 1224 1225 /** 1226 * Search parameter: <b>address-city</b> 1227 * <p> 1228 * Description: <b>A city specified in an address</b><br> 1229 * Type: <b>string</b><br> 1230 * Path: <b>RelatedPerson.address.city</b><br> 1231 * </p> 1232 */ 1233 @SearchParamDefinition(name="address-city", path="RelatedPerson.address.city", description="A city specified in an address", type="string" ) 1234 public static final String SP_ADDRESS_CITY = "address-city"; 1235 /** 1236 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1237 * <p> 1238 * Description: <b>A city specified in an address</b><br> 1239 * Type: <b>string</b><br> 1240 * Path: <b>RelatedPerson.address.city</b><br> 1241 * </p> 1242 */ 1243 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1244 1245 /** 1246 * Search parameter: <b>email</b> 1247 * <p> 1248 * Description: <b>A value in an email contact</b><br> 1249 * Type: <b>token</b><br> 1250 * Path: <b>RelatedPerson.telecom(system=email)</b><br> 1251 * </p> 1252 */ 1253 @SearchParamDefinition(name="email", path="RelatedPerson.telecom.where(system='email')", description="A value in an email contact", type="token" ) 1254 public static final String SP_EMAIL = "email"; 1255 /** 1256 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1257 * <p> 1258 * Description: <b>A value in an email contact</b><br> 1259 * Type: <b>token</b><br> 1260 * Path: <b>RelatedPerson.telecom(system=email)</b><br> 1261 * </p> 1262 */ 1263 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1264 1265 1266}