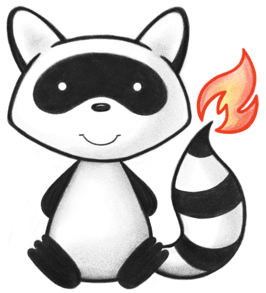
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 052 */ 053@ResourceDef(name="RequestGroup", profile="http://hl7.org/fhir/Profile/RequestGroup") 054public class RequestGroup extends DomainResource { 055 056 public enum RequestStatus { 057 /** 058 * The request has been created but is not yet complete or ready for action 059 */ 060 DRAFT, 061 /** 062 * The request is ready to be acted upon 063 */ 064 ACTIVE, 065 /** 066 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 067 */ 068 SUSPENDED, 069 /** 070 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 071 */ 072 CANCELLED, 073 /** 074 * Activity against the request has been sufficiently completed to the satisfaction of the requester 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 079 */ 080 ENTEREDINERROR, 081 /** 082 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static RequestStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("draft".equals(codeString)) 093 return DRAFT; 094 if ("active".equals(codeString)) 095 return ACTIVE; 096 if ("suspended".equals(codeString)) 097 return SUSPENDED; 098 if ("cancelled".equals(codeString)) 099 return CANCELLED; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case DRAFT: return "draft"; 114 case ACTIVE: return "active"; 115 case SUSPENDED: return "suspended"; 116 case CANCELLED: return "cancelled"; 117 case COMPLETED: return "completed"; 118 case ENTEREDINERROR: return "entered-in-error"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case DRAFT: return "http://hl7.org/fhir/request-status"; 127 case ACTIVE: return "http://hl7.org/fhir/request-status"; 128 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 129 case CANCELLED: return "http://hl7.org/fhir/request-status"; 130 case COMPLETED: return "http://hl7.org/fhir/request-status"; 131 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 140 case ACTIVE: return "The request is ready to be acted upon"; 141 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 142 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 143 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 144 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 145 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case DRAFT: return "Draft"; 153 case ACTIVE: return "Active"; 154 case SUSPENDED: return "Suspended"; 155 case CANCELLED: return "Cancelled"; 156 case COMPLETED: return "Completed"; 157 case ENTEREDINERROR: return "Entered in Error"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 166 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("draft".equals(codeString)) 171 return RequestStatus.DRAFT; 172 if ("active".equals(codeString)) 173 return RequestStatus.ACTIVE; 174 if ("suspended".equals(codeString)) 175 return RequestStatus.SUSPENDED; 176 if ("cancelled".equals(codeString)) 177 return RequestStatus.CANCELLED; 178 if ("completed".equals(codeString)) 179 return RequestStatus.COMPLETED; 180 if ("entered-in-error".equals(codeString)) 181 return RequestStatus.ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return RequestStatus.UNKNOWN; 184 throw new IllegalArgumentException("Unknown RequestStatus code '"+codeString+"'"); 185 } 186 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<RequestStatus>(this); 191 String codeString = code.asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("draft".equals(codeString)) 195 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT); 196 if ("active".equals(codeString)) 197 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE); 198 if ("suspended".equals(codeString)) 199 return new Enumeration<RequestStatus>(this, RequestStatus.SUSPENDED); 200 if ("cancelled".equals(codeString)) 201 return new Enumeration<RequestStatus>(this, RequestStatus.CANCELLED); 202 if ("completed".equals(codeString)) 203 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN); 208 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 209 } 210 public String toCode(RequestStatus code) { 211 if (code == RequestStatus.NULL) 212 return null; 213 if (code == RequestStatus.DRAFT) 214 return "draft"; 215 if (code == RequestStatus.ACTIVE) 216 return "active"; 217 if (code == RequestStatus.SUSPENDED) 218 return "suspended"; 219 if (code == RequestStatus.CANCELLED) 220 return "cancelled"; 221 if (code == RequestStatus.COMPLETED) 222 return "completed"; 223 if (code == RequestStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == RequestStatus.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(RequestStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 public enum RequestIntent { 235 /** 236 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 237 */ 238 PROPOSAL, 239 /** 240 * The request represents an intension to ensure something occurs without providing an authorization for others to act 241 */ 242 PLAN, 243 /** 244 * The request represents a request/demand and authorization for action 245 */ 246 ORDER, 247 /** 248 * The request represents an original authorization for action 249 */ 250 ORIGINALORDER, 251 /** 252 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 253 */ 254 REFLEXORDER, 255 /** 256 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 257 */ 258 FILLERORDER, 259 /** 260 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 261 */ 262 INSTANCEORDER, 263 /** 264 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 265 266Refer to [[[RequestGroup]]] for additional information on how this status is used 267 */ 268 OPTION, 269 /** 270 * added to help the parsers with the generic types 271 */ 272 NULL; 273 public static RequestIntent fromCode(String codeString) throws FHIRException { 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("proposal".equals(codeString)) 277 return PROPOSAL; 278 if ("plan".equals(codeString)) 279 return PLAN; 280 if ("order".equals(codeString)) 281 return ORDER; 282 if ("original-order".equals(codeString)) 283 return ORIGINALORDER; 284 if ("reflex-order".equals(codeString)) 285 return REFLEXORDER; 286 if ("filler-order".equals(codeString)) 287 return FILLERORDER; 288 if ("instance-order".equals(codeString)) 289 return INSTANCEORDER; 290 if ("option".equals(codeString)) 291 return OPTION; 292 if (Configuration.isAcceptInvalidEnums()) 293 return null; 294 else 295 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 296 } 297 public String toCode() { 298 switch (this) { 299 case PROPOSAL: return "proposal"; 300 case PLAN: return "plan"; 301 case ORDER: return "order"; 302 case ORIGINALORDER: return "original-order"; 303 case REFLEXORDER: return "reflex-order"; 304 case FILLERORDER: return "filler-order"; 305 case INSTANCEORDER: return "instance-order"; 306 case OPTION: return "option"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 public String getSystem() { 312 switch (this) { 313 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 314 case PLAN: return "http://hl7.org/fhir/request-intent"; 315 case ORDER: return "http://hl7.org/fhir/request-intent"; 316 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 317 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 318 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 319 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 320 case OPTION: return "http://hl7.org/fhir/request-intent"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 public String getDefinition() { 326 switch (this) { 327 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 328 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 329 case ORDER: return "The request represents a request/demand and authorization for action"; 330 case ORIGINALORDER: return "The request represents an original authorization for action"; 331 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 332 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 333 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 334 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getDisplay() { 340 switch (this) { 341 case PROPOSAL: return "Proposal"; 342 case PLAN: return "Plan"; 343 case ORDER: return "Order"; 344 case ORIGINALORDER: return "Original Order"; 345 case REFLEXORDER: return "Reflex Order"; 346 case FILLERORDER: return "Filler Order"; 347 case INSTANCEORDER: return "Instance Order"; 348 case OPTION: return "Option"; 349 case NULL: return null; 350 default: return "?"; 351 } 352 } 353 } 354 355 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 356 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 357 if (codeString == null || "".equals(codeString)) 358 if (codeString == null || "".equals(codeString)) 359 return null; 360 if ("proposal".equals(codeString)) 361 return RequestIntent.PROPOSAL; 362 if ("plan".equals(codeString)) 363 return RequestIntent.PLAN; 364 if ("order".equals(codeString)) 365 return RequestIntent.ORDER; 366 if ("original-order".equals(codeString)) 367 return RequestIntent.ORIGINALORDER; 368 if ("reflex-order".equals(codeString)) 369 return RequestIntent.REFLEXORDER; 370 if ("filler-order".equals(codeString)) 371 return RequestIntent.FILLERORDER; 372 if ("instance-order".equals(codeString)) 373 return RequestIntent.INSTANCEORDER; 374 if ("option".equals(codeString)) 375 return RequestIntent.OPTION; 376 throw new IllegalArgumentException("Unknown RequestIntent code '"+codeString+"'"); 377 } 378 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 379 if (code == null) 380 return null; 381 if (code.isEmpty()) 382 return new Enumeration<RequestIntent>(this); 383 String codeString = code.asStringValue(); 384 if (codeString == null || "".equals(codeString)) 385 return null; 386 if ("proposal".equals(codeString)) 387 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL); 388 if ("plan".equals(codeString)) 389 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN); 390 if ("order".equals(codeString)) 391 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER); 392 if ("original-order".equals(codeString)) 393 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER); 394 if ("reflex-order".equals(codeString)) 395 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER); 396 if ("filler-order".equals(codeString)) 397 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER); 398 if ("instance-order".equals(codeString)) 399 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER); 400 if ("option".equals(codeString)) 401 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION); 402 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 403 } 404 public String toCode(RequestIntent code) { 405 if (code == RequestIntent.NULL) 406 return null; 407 if (code == RequestIntent.PROPOSAL) 408 return "proposal"; 409 if (code == RequestIntent.PLAN) 410 return "plan"; 411 if (code == RequestIntent.ORDER) 412 return "order"; 413 if (code == RequestIntent.ORIGINALORDER) 414 return "original-order"; 415 if (code == RequestIntent.REFLEXORDER) 416 return "reflex-order"; 417 if (code == RequestIntent.FILLERORDER) 418 return "filler-order"; 419 if (code == RequestIntent.INSTANCEORDER) 420 return "instance-order"; 421 if (code == RequestIntent.OPTION) 422 return "option"; 423 return "?"; 424 } 425 public String toSystem(RequestIntent code) { 426 return code.getSystem(); 427 } 428 } 429 430 public enum RequestPriority { 431 /** 432 * The request has normal priority 433 */ 434 ROUTINE, 435 /** 436 * The request should be actioned promptly - higher priority than routine 437 */ 438 URGENT, 439 /** 440 * The request should be actioned as soon as possible - higher priority than urgent 441 */ 442 ASAP, 443 /** 444 * The request should be actioned immediately - highest possible priority. E.g. an emergency 445 */ 446 STAT, 447 /** 448 * added to help the parsers with the generic types 449 */ 450 NULL; 451 public static RequestPriority fromCode(String codeString) throws FHIRException { 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("routine".equals(codeString)) 455 return ROUTINE; 456 if ("urgent".equals(codeString)) 457 return URGENT; 458 if ("asap".equals(codeString)) 459 return ASAP; 460 if ("stat".equals(codeString)) 461 return STAT; 462 if (Configuration.isAcceptInvalidEnums()) 463 return null; 464 else 465 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 466 } 467 public String toCode() { 468 switch (this) { 469 case ROUTINE: return "routine"; 470 case URGENT: return "urgent"; 471 case ASAP: return "asap"; 472 case STAT: return "stat"; 473 case NULL: return null; 474 default: return "?"; 475 } 476 } 477 public String getSystem() { 478 switch (this) { 479 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 480 case URGENT: return "http://hl7.org/fhir/request-priority"; 481 case ASAP: return "http://hl7.org/fhir/request-priority"; 482 case STAT: return "http://hl7.org/fhir/request-priority"; 483 case NULL: return null; 484 default: return "?"; 485 } 486 } 487 public String getDefinition() { 488 switch (this) { 489 case ROUTINE: return "The request has normal priority"; 490 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 491 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 492 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 493 case NULL: return null; 494 default: return "?"; 495 } 496 } 497 public String getDisplay() { 498 switch (this) { 499 case ROUTINE: return "Routine"; 500 case URGENT: return "Urgent"; 501 case ASAP: return "ASAP"; 502 case STAT: return "STAT"; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 } 508 509 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 510 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 511 if (codeString == null || "".equals(codeString)) 512 if (codeString == null || "".equals(codeString)) 513 return null; 514 if ("routine".equals(codeString)) 515 return RequestPriority.ROUTINE; 516 if ("urgent".equals(codeString)) 517 return RequestPriority.URGENT; 518 if ("asap".equals(codeString)) 519 return RequestPriority.ASAP; 520 if ("stat".equals(codeString)) 521 return RequestPriority.STAT; 522 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 523 } 524 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 525 if (code == null) 526 return null; 527 if (code.isEmpty()) 528 return new Enumeration<RequestPriority>(this); 529 String codeString = code.asStringValue(); 530 if (codeString == null || "".equals(codeString)) 531 return null; 532 if ("routine".equals(codeString)) 533 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE); 534 if ("urgent".equals(codeString)) 535 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT); 536 if ("asap".equals(codeString)) 537 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP); 538 if ("stat".equals(codeString)) 539 return new Enumeration<RequestPriority>(this, RequestPriority.STAT); 540 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 541 } 542 public String toCode(RequestPriority code) { 543 if (code == RequestPriority.NULL) 544 return null; 545 if (code == RequestPriority.ROUTINE) 546 return "routine"; 547 if (code == RequestPriority.URGENT) 548 return "urgent"; 549 if (code == RequestPriority.ASAP) 550 return "asap"; 551 if (code == RequestPriority.STAT) 552 return "stat"; 553 return "?"; 554 } 555 public String toSystem(RequestPriority code) { 556 return code.getSystem(); 557 } 558 } 559 560 public enum ActionConditionKind { 561 /** 562 * The condition describes whether or not a given action is applicable 563 */ 564 APPLICABILITY, 565 /** 566 * The condition is a starting condition for the action 567 */ 568 START, 569 /** 570 * The condition is a stop, or exit condition for the action 571 */ 572 STOP, 573 /** 574 * added to help the parsers with the generic types 575 */ 576 NULL; 577 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 578 if (codeString == null || "".equals(codeString)) 579 return null; 580 if ("applicability".equals(codeString)) 581 return APPLICABILITY; 582 if ("start".equals(codeString)) 583 return START; 584 if ("stop".equals(codeString)) 585 return STOP; 586 if (Configuration.isAcceptInvalidEnums()) 587 return null; 588 else 589 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 590 } 591 public String toCode() { 592 switch (this) { 593 case APPLICABILITY: return "applicability"; 594 case START: return "start"; 595 case STOP: return "stop"; 596 case NULL: return null; 597 default: return "?"; 598 } 599 } 600 public String getSystem() { 601 switch (this) { 602 case APPLICABILITY: return "http://hl7.org/fhir/action-condition-kind"; 603 case START: return "http://hl7.org/fhir/action-condition-kind"; 604 case STOP: return "http://hl7.org/fhir/action-condition-kind"; 605 case NULL: return null; 606 default: return "?"; 607 } 608 } 609 public String getDefinition() { 610 switch (this) { 611 case APPLICABILITY: return "The condition describes whether or not a given action is applicable"; 612 case START: return "The condition is a starting condition for the action"; 613 case STOP: return "The condition is a stop, or exit condition for the action"; 614 case NULL: return null; 615 default: return "?"; 616 } 617 } 618 public String getDisplay() { 619 switch (this) { 620 case APPLICABILITY: return "Applicability"; 621 case START: return "Start"; 622 case STOP: return "Stop"; 623 case NULL: return null; 624 default: return "?"; 625 } 626 } 627 } 628 629 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 630 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 631 if (codeString == null || "".equals(codeString)) 632 if (codeString == null || "".equals(codeString)) 633 return null; 634 if ("applicability".equals(codeString)) 635 return ActionConditionKind.APPLICABILITY; 636 if ("start".equals(codeString)) 637 return ActionConditionKind.START; 638 if ("stop".equals(codeString)) 639 return ActionConditionKind.STOP; 640 throw new IllegalArgumentException("Unknown ActionConditionKind code '"+codeString+"'"); 641 } 642 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 643 if (code == null) 644 return null; 645 if (code.isEmpty()) 646 return new Enumeration<ActionConditionKind>(this); 647 String codeString = code.asStringValue(); 648 if (codeString == null || "".equals(codeString)) 649 return null; 650 if ("applicability".equals(codeString)) 651 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY); 652 if ("start".equals(codeString)) 653 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START); 654 if ("stop".equals(codeString)) 655 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP); 656 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 657 } 658 public String toCode(ActionConditionKind code) { 659 if (code == ActionConditionKind.NULL) 660 return null; 661 if (code == ActionConditionKind.APPLICABILITY) 662 return "applicability"; 663 if (code == ActionConditionKind.START) 664 return "start"; 665 if (code == ActionConditionKind.STOP) 666 return "stop"; 667 return "?"; 668 } 669 public String toSystem(ActionConditionKind code) { 670 return code.getSystem(); 671 } 672 } 673 674 public enum ActionRelationshipType { 675 /** 676 * The action must be performed before the start of the related action 677 */ 678 BEFORESTART, 679 /** 680 * The action must be performed before the related action 681 */ 682 BEFORE, 683 /** 684 * The action must be performed before the end of the related action 685 */ 686 BEFOREEND, 687 /** 688 * The action must be performed concurrent with the start of the related action 689 */ 690 CONCURRENTWITHSTART, 691 /** 692 * The action must be performed concurrent with the related action 693 */ 694 CONCURRENT, 695 /** 696 * The action must be performed concurrent with the end of the related action 697 */ 698 CONCURRENTWITHEND, 699 /** 700 * The action must be performed after the start of the related action 701 */ 702 AFTERSTART, 703 /** 704 * The action must be performed after the related action 705 */ 706 AFTER, 707 /** 708 * The action must be performed after the end of the related action 709 */ 710 AFTEREND, 711 /** 712 * added to help the parsers with the generic types 713 */ 714 NULL; 715 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 716 if (codeString == null || "".equals(codeString)) 717 return null; 718 if ("before-start".equals(codeString)) 719 return BEFORESTART; 720 if ("before".equals(codeString)) 721 return BEFORE; 722 if ("before-end".equals(codeString)) 723 return BEFOREEND; 724 if ("concurrent-with-start".equals(codeString)) 725 return CONCURRENTWITHSTART; 726 if ("concurrent".equals(codeString)) 727 return CONCURRENT; 728 if ("concurrent-with-end".equals(codeString)) 729 return CONCURRENTWITHEND; 730 if ("after-start".equals(codeString)) 731 return AFTERSTART; 732 if ("after".equals(codeString)) 733 return AFTER; 734 if ("after-end".equals(codeString)) 735 return AFTEREND; 736 if (Configuration.isAcceptInvalidEnums()) 737 return null; 738 else 739 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 740 } 741 public String toCode() { 742 switch (this) { 743 case BEFORESTART: return "before-start"; 744 case BEFORE: return "before"; 745 case BEFOREEND: return "before-end"; 746 case CONCURRENTWITHSTART: return "concurrent-with-start"; 747 case CONCURRENT: return "concurrent"; 748 case CONCURRENTWITHEND: return "concurrent-with-end"; 749 case AFTERSTART: return "after-start"; 750 case AFTER: return "after"; 751 case AFTEREND: return "after-end"; 752 case NULL: return null; 753 default: return "?"; 754 } 755 } 756 public String getSystem() { 757 switch (this) { 758 case BEFORESTART: return "http://hl7.org/fhir/action-relationship-type"; 759 case BEFORE: return "http://hl7.org/fhir/action-relationship-type"; 760 case BEFOREEND: return "http://hl7.org/fhir/action-relationship-type"; 761 case CONCURRENTWITHSTART: return "http://hl7.org/fhir/action-relationship-type"; 762 case CONCURRENT: return "http://hl7.org/fhir/action-relationship-type"; 763 case CONCURRENTWITHEND: return "http://hl7.org/fhir/action-relationship-type"; 764 case AFTERSTART: return "http://hl7.org/fhir/action-relationship-type"; 765 case AFTER: return "http://hl7.org/fhir/action-relationship-type"; 766 case AFTEREND: return "http://hl7.org/fhir/action-relationship-type"; 767 case NULL: return null; 768 default: return "?"; 769 } 770 } 771 public String getDefinition() { 772 switch (this) { 773 case BEFORESTART: return "The action must be performed before the start of the related action"; 774 case BEFORE: return "The action must be performed before the related action"; 775 case BEFOREEND: return "The action must be performed before the end of the related action"; 776 case CONCURRENTWITHSTART: return "The action must be performed concurrent with the start of the related action"; 777 case CONCURRENT: return "The action must be performed concurrent with the related action"; 778 case CONCURRENTWITHEND: return "The action must be performed concurrent with the end of the related action"; 779 case AFTERSTART: return "The action must be performed after the start of the related action"; 780 case AFTER: return "The action must be performed after the related action"; 781 case AFTEREND: return "The action must be performed after the end of the related action"; 782 case NULL: return null; 783 default: return "?"; 784 } 785 } 786 public String getDisplay() { 787 switch (this) { 788 case BEFORESTART: return "Before Start"; 789 case BEFORE: return "Before"; 790 case BEFOREEND: return "Before End"; 791 case CONCURRENTWITHSTART: return "Concurrent With Start"; 792 case CONCURRENT: return "Concurrent"; 793 case CONCURRENTWITHEND: return "Concurrent With End"; 794 case AFTERSTART: return "After Start"; 795 case AFTER: return "After"; 796 case AFTEREND: return "After End"; 797 case NULL: return null; 798 default: return "?"; 799 } 800 } 801 } 802 803 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 804 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 805 if (codeString == null || "".equals(codeString)) 806 if (codeString == null || "".equals(codeString)) 807 return null; 808 if ("before-start".equals(codeString)) 809 return ActionRelationshipType.BEFORESTART; 810 if ("before".equals(codeString)) 811 return ActionRelationshipType.BEFORE; 812 if ("before-end".equals(codeString)) 813 return ActionRelationshipType.BEFOREEND; 814 if ("concurrent-with-start".equals(codeString)) 815 return ActionRelationshipType.CONCURRENTWITHSTART; 816 if ("concurrent".equals(codeString)) 817 return ActionRelationshipType.CONCURRENT; 818 if ("concurrent-with-end".equals(codeString)) 819 return ActionRelationshipType.CONCURRENTWITHEND; 820 if ("after-start".equals(codeString)) 821 return ActionRelationshipType.AFTERSTART; 822 if ("after".equals(codeString)) 823 return ActionRelationshipType.AFTER; 824 if ("after-end".equals(codeString)) 825 return ActionRelationshipType.AFTEREND; 826 throw new IllegalArgumentException("Unknown ActionRelationshipType code '"+codeString+"'"); 827 } 828 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 829 if (code == null) 830 return null; 831 if (code.isEmpty()) 832 return new Enumeration<ActionRelationshipType>(this); 833 String codeString = code.asStringValue(); 834 if (codeString == null || "".equals(codeString)) 835 return null; 836 if ("before-start".equals(codeString)) 837 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART); 838 if ("before".equals(codeString)) 839 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE); 840 if ("before-end".equals(codeString)) 841 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND); 842 if ("concurrent-with-start".equals(codeString)) 843 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART); 844 if ("concurrent".equals(codeString)) 845 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT); 846 if ("concurrent-with-end".equals(codeString)) 847 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND); 848 if ("after-start".equals(codeString)) 849 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART); 850 if ("after".equals(codeString)) 851 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER); 852 if ("after-end".equals(codeString)) 853 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND); 854 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 855 } 856 public String toCode(ActionRelationshipType code) { 857 if (code == ActionRelationshipType.NULL) 858 return null; 859 if (code == ActionRelationshipType.BEFORESTART) 860 return "before-start"; 861 if (code == ActionRelationshipType.BEFORE) 862 return "before"; 863 if (code == ActionRelationshipType.BEFOREEND) 864 return "before-end"; 865 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 866 return "concurrent-with-start"; 867 if (code == ActionRelationshipType.CONCURRENT) 868 return "concurrent"; 869 if (code == ActionRelationshipType.CONCURRENTWITHEND) 870 return "concurrent-with-end"; 871 if (code == ActionRelationshipType.AFTERSTART) 872 return "after-start"; 873 if (code == ActionRelationshipType.AFTER) 874 return "after"; 875 if (code == ActionRelationshipType.AFTEREND) 876 return "after-end"; 877 return "?"; 878 } 879 public String toSystem(ActionRelationshipType code) { 880 return code.getSystem(); 881 } 882 } 883 884 public enum ActionGroupingBehavior { 885 /** 886 * Any group marked with this behavior should be displayed as a visual group to the end user 887 */ 888 VISUALGROUP, 889 /** 890 * A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so 891 */ 892 LOGICALGROUP, 893 /** 894 * A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of "AtMostOne", unless it's a required action, in which case, it would be "ExactlyOne" 895 */ 896 SENTENCEGROUP, 897 /** 898 * added to help the parsers with the generic types 899 */ 900 NULL; 901 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 902 if (codeString == null || "".equals(codeString)) 903 return null; 904 if ("visual-group".equals(codeString)) 905 return VISUALGROUP; 906 if ("logical-group".equals(codeString)) 907 return LOGICALGROUP; 908 if ("sentence-group".equals(codeString)) 909 return SENTENCEGROUP; 910 if (Configuration.isAcceptInvalidEnums()) 911 return null; 912 else 913 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 914 } 915 public String toCode() { 916 switch (this) { 917 case VISUALGROUP: return "visual-group"; 918 case LOGICALGROUP: return "logical-group"; 919 case SENTENCEGROUP: return "sentence-group"; 920 case NULL: return null; 921 default: return "?"; 922 } 923 } 924 public String getSystem() { 925 switch (this) { 926 case VISUALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 927 case LOGICALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 928 case SENTENCEGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 929 case NULL: return null; 930 default: return "?"; 931 } 932 } 933 public String getDefinition() { 934 switch (this) { 935 case VISUALGROUP: return "Any group marked with this behavior should be displayed as a visual group to the end user"; 936 case LOGICALGROUP: return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so"; 937 case SENTENCEGROUP: return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\""; 938 case NULL: return null; 939 default: return "?"; 940 } 941 } 942 public String getDisplay() { 943 switch (this) { 944 case VISUALGROUP: return "Visual Group"; 945 case LOGICALGROUP: return "Logical Group"; 946 case SENTENCEGROUP: return "Sentence Group"; 947 case NULL: return null; 948 default: return "?"; 949 } 950 } 951 } 952 953 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 954 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 955 if (codeString == null || "".equals(codeString)) 956 if (codeString == null || "".equals(codeString)) 957 return null; 958 if ("visual-group".equals(codeString)) 959 return ActionGroupingBehavior.VISUALGROUP; 960 if ("logical-group".equals(codeString)) 961 return ActionGroupingBehavior.LOGICALGROUP; 962 if ("sentence-group".equals(codeString)) 963 return ActionGroupingBehavior.SENTENCEGROUP; 964 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 965 } 966 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 967 if (code == null) 968 return null; 969 if (code.isEmpty()) 970 return new Enumeration<ActionGroupingBehavior>(this); 971 String codeString = code.asStringValue(); 972 if (codeString == null || "".equals(codeString)) 973 return null; 974 if ("visual-group".equals(codeString)) 975 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP); 976 if ("logical-group".equals(codeString)) 977 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP); 978 if ("sentence-group".equals(codeString)) 979 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP); 980 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 981 } 982 public String toCode(ActionGroupingBehavior code) { 983 if (code == ActionGroupingBehavior.NULL) 984 return null; 985 if (code == ActionGroupingBehavior.VISUALGROUP) 986 return "visual-group"; 987 if (code == ActionGroupingBehavior.LOGICALGROUP) 988 return "logical-group"; 989 if (code == ActionGroupingBehavior.SENTENCEGROUP) 990 return "sentence-group"; 991 return "?"; 992 } 993 public String toSystem(ActionGroupingBehavior code) { 994 return code.getSystem(); 995 } 996 } 997 998 public enum ActionSelectionBehavior { 999 /** 1000 * Any number of the actions in the group may be chosen, from zero to all 1001 */ 1002 ANY, 1003 /** 1004 * All the actions in the group must be selected as a single unit 1005 */ 1006 ALL, 1007 /** 1008 * All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected 1009 */ 1010 ALLORNONE, 1011 /** 1012 * The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group 1013 */ 1014 EXACTLYONE, 1015 /** 1016 * The end user may choose zero or at most one of the actions in the group 1017 */ 1018 ATMOSTONE, 1019 /** 1020 * The end user must choose a minimum of one, and as many additional as desired 1021 */ 1022 ONEORMORE, 1023 /** 1024 * added to help the parsers with the generic types 1025 */ 1026 NULL; 1027 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 1028 if (codeString == null || "".equals(codeString)) 1029 return null; 1030 if ("any".equals(codeString)) 1031 return ANY; 1032 if ("all".equals(codeString)) 1033 return ALL; 1034 if ("all-or-none".equals(codeString)) 1035 return ALLORNONE; 1036 if ("exactly-one".equals(codeString)) 1037 return EXACTLYONE; 1038 if ("at-most-one".equals(codeString)) 1039 return ATMOSTONE; 1040 if ("one-or-more".equals(codeString)) 1041 return ONEORMORE; 1042 if (Configuration.isAcceptInvalidEnums()) 1043 return null; 1044 else 1045 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1046 } 1047 public String toCode() { 1048 switch (this) { 1049 case ANY: return "any"; 1050 case ALL: return "all"; 1051 case ALLORNONE: return "all-or-none"; 1052 case EXACTLYONE: return "exactly-one"; 1053 case ATMOSTONE: return "at-most-one"; 1054 case ONEORMORE: return "one-or-more"; 1055 case NULL: return null; 1056 default: return "?"; 1057 } 1058 } 1059 public String getSystem() { 1060 switch (this) { 1061 case ANY: return "http://hl7.org/fhir/action-selection-behavior"; 1062 case ALL: return "http://hl7.org/fhir/action-selection-behavior"; 1063 case ALLORNONE: return "http://hl7.org/fhir/action-selection-behavior"; 1064 case EXACTLYONE: return "http://hl7.org/fhir/action-selection-behavior"; 1065 case ATMOSTONE: return "http://hl7.org/fhir/action-selection-behavior"; 1066 case ONEORMORE: return "http://hl7.org/fhir/action-selection-behavior"; 1067 case NULL: return null; 1068 default: return "?"; 1069 } 1070 } 1071 public String getDefinition() { 1072 switch (this) { 1073 case ANY: return "Any number of the actions in the group may be chosen, from zero to all"; 1074 case ALL: return "All the actions in the group must be selected as a single unit"; 1075 case ALLORNONE: return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected"; 1076 case EXACTLYONE: return "The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group"; 1077 case ATMOSTONE: return "The end user may choose zero or at most one of the actions in the group"; 1078 case ONEORMORE: return "The end user must choose a minimum of one, and as many additional as desired"; 1079 case NULL: return null; 1080 default: return "?"; 1081 } 1082 } 1083 public String getDisplay() { 1084 switch (this) { 1085 case ANY: return "Any"; 1086 case ALL: return "All"; 1087 case ALLORNONE: return "All Or None"; 1088 case EXACTLYONE: return "Exactly One"; 1089 case ATMOSTONE: return "At Most One"; 1090 case ONEORMORE: return "One Or More"; 1091 case NULL: return null; 1092 default: return "?"; 1093 } 1094 } 1095 } 1096 1097 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1098 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1099 if (codeString == null || "".equals(codeString)) 1100 if (codeString == null || "".equals(codeString)) 1101 return null; 1102 if ("any".equals(codeString)) 1103 return ActionSelectionBehavior.ANY; 1104 if ("all".equals(codeString)) 1105 return ActionSelectionBehavior.ALL; 1106 if ("all-or-none".equals(codeString)) 1107 return ActionSelectionBehavior.ALLORNONE; 1108 if ("exactly-one".equals(codeString)) 1109 return ActionSelectionBehavior.EXACTLYONE; 1110 if ("at-most-one".equals(codeString)) 1111 return ActionSelectionBehavior.ATMOSTONE; 1112 if ("one-or-more".equals(codeString)) 1113 return ActionSelectionBehavior.ONEORMORE; 1114 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1115 } 1116 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1117 if (code == null) 1118 return null; 1119 if (code.isEmpty()) 1120 return new Enumeration<ActionSelectionBehavior>(this); 1121 String codeString = code.asStringValue(); 1122 if (codeString == null || "".equals(codeString)) 1123 return null; 1124 if ("any".equals(codeString)) 1125 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY); 1126 if ("all".equals(codeString)) 1127 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL); 1128 if ("all-or-none".equals(codeString)) 1129 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE); 1130 if ("exactly-one".equals(codeString)) 1131 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE); 1132 if ("at-most-one".equals(codeString)) 1133 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE); 1134 if ("one-or-more".equals(codeString)) 1135 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE); 1136 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1137 } 1138 public String toCode(ActionSelectionBehavior code) { 1139 if (code == ActionSelectionBehavior.NULL) 1140 return null; 1141 if (code == ActionSelectionBehavior.ANY) 1142 return "any"; 1143 if (code == ActionSelectionBehavior.ALL) 1144 return "all"; 1145 if (code == ActionSelectionBehavior.ALLORNONE) 1146 return "all-or-none"; 1147 if (code == ActionSelectionBehavior.EXACTLYONE) 1148 return "exactly-one"; 1149 if (code == ActionSelectionBehavior.ATMOSTONE) 1150 return "at-most-one"; 1151 if (code == ActionSelectionBehavior.ONEORMORE) 1152 return "one-or-more"; 1153 return "?"; 1154 } 1155 public String toSystem(ActionSelectionBehavior code) { 1156 return code.getSystem(); 1157 } 1158 } 1159 1160 public enum ActionRequiredBehavior { 1161 /** 1162 * An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action 1163 */ 1164 MUST, 1165 /** 1166 * An action with this behavior may be included in the set of actions processed by the end user 1167 */ 1168 COULD, 1169 /** 1170 * An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included 1171 */ 1172 MUSTUNLESSDOCUMENTED, 1173 /** 1174 * added to help the parsers with the generic types 1175 */ 1176 NULL; 1177 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1178 if (codeString == null || "".equals(codeString)) 1179 return null; 1180 if ("must".equals(codeString)) 1181 return MUST; 1182 if ("could".equals(codeString)) 1183 return COULD; 1184 if ("must-unless-documented".equals(codeString)) 1185 return MUSTUNLESSDOCUMENTED; 1186 if (Configuration.isAcceptInvalidEnums()) 1187 return null; 1188 else 1189 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1190 } 1191 public String toCode() { 1192 switch (this) { 1193 case MUST: return "must"; 1194 case COULD: return "could"; 1195 case MUSTUNLESSDOCUMENTED: return "must-unless-documented"; 1196 case NULL: return null; 1197 default: return "?"; 1198 } 1199 } 1200 public String getSystem() { 1201 switch (this) { 1202 case MUST: return "http://hl7.org/fhir/action-required-behavior"; 1203 case COULD: return "http://hl7.org/fhir/action-required-behavior"; 1204 case MUSTUNLESSDOCUMENTED: return "http://hl7.org/fhir/action-required-behavior"; 1205 case NULL: return null; 1206 default: return "?"; 1207 } 1208 } 1209 public String getDefinition() { 1210 switch (this) { 1211 case MUST: return "An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action"; 1212 case COULD: return "An action with this behavior may be included in the set of actions processed by the end user"; 1213 case MUSTUNLESSDOCUMENTED: return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included"; 1214 case NULL: return null; 1215 default: return "?"; 1216 } 1217 } 1218 public String getDisplay() { 1219 switch (this) { 1220 case MUST: return "Must"; 1221 case COULD: return "Could"; 1222 case MUSTUNLESSDOCUMENTED: return "Must Unless Documented"; 1223 case NULL: return null; 1224 default: return "?"; 1225 } 1226 } 1227 } 1228 1229 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1230 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1231 if (codeString == null || "".equals(codeString)) 1232 if (codeString == null || "".equals(codeString)) 1233 return null; 1234 if ("must".equals(codeString)) 1235 return ActionRequiredBehavior.MUST; 1236 if ("could".equals(codeString)) 1237 return ActionRequiredBehavior.COULD; 1238 if ("must-unless-documented".equals(codeString)) 1239 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1240 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1241 } 1242 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1243 if (code == null) 1244 return null; 1245 if (code.isEmpty()) 1246 return new Enumeration<ActionRequiredBehavior>(this); 1247 String codeString = code.asStringValue(); 1248 if (codeString == null || "".equals(codeString)) 1249 return null; 1250 if ("must".equals(codeString)) 1251 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST); 1252 if ("could".equals(codeString)) 1253 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD); 1254 if ("must-unless-documented".equals(codeString)) 1255 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED); 1256 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1257 } 1258 public String toCode(ActionRequiredBehavior code) { 1259 if (code == ActionRequiredBehavior.NULL) 1260 return null; 1261 if (code == ActionRequiredBehavior.MUST) 1262 return "must"; 1263 if (code == ActionRequiredBehavior.COULD) 1264 return "could"; 1265 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1266 return "must-unless-documented"; 1267 return "?"; 1268 } 1269 public String toSystem(ActionRequiredBehavior code) { 1270 return code.getSystem(); 1271 } 1272 } 1273 1274 public enum ActionPrecheckBehavior { 1275 /** 1276 * An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider "pre-checking" such an action as a convenience for the user 1277 */ 1278 YES, 1279 /** 1280 * An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not "pre-check" such an action 1281 */ 1282 NO, 1283 /** 1284 * added to help the parsers with the generic types 1285 */ 1286 NULL; 1287 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1288 if (codeString == null || "".equals(codeString)) 1289 return null; 1290 if ("yes".equals(codeString)) 1291 return YES; 1292 if ("no".equals(codeString)) 1293 return NO; 1294 if (Configuration.isAcceptInvalidEnums()) 1295 return null; 1296 else 1297 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1298 } 1299 public String toCode() { 1300 switch (this) { 1301 case YES: return "yes"; 1302 case NO: return "no"; 1303 case NULL: return null; 1304 default: return "?"; 1305 } 1306 } 1307 public String getSystem() { 1308 switch (this) { 1309 case YES: return "http://hl7.org/fhir/action-precheck-behavior"; 1310 case NO: return "http://hl7.org/fhir/action-precheck-behavior"; 1311 case NULL: return null; 1312 default: return "?"; 1313 } 1314 } 1315 public String getDefinition() { 1316 switch (this) { 1317 case YES: return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user"; 1318 case NO: return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action"; 1319 case NULL: return null; 1320 default: return "?"; 1321 } 1322 } 1323 public String getDisplay() { 1324 switch (this) { 1325 case YES: return "Yes"; 1326 case NO: return "No"; 1327 case NULL: return null; 1328 default: return "?"; 1329 } 1330 } 1331 } 1332 1333 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1334 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1335 if (codeString == null || "".equals(codeString)) 1336 if (codeString == null || "".equals(codeString)) 1337 return null; 1338 if ("yes".equals(codeString)) 1339 return ActionPrecheckBehavior.YES; 1340 if ("no".equals(codeString)) 1341 return ActionPrecheckBehavior.NO; 1342 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1343 } 1344 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1345 if (code == null) 1346 return null; 1347 if (code.isEmpty()) 1348 return new Enumeration<ActionPrecheckBehavior>(this); 1349 String codeString = code.asStringValue(); 1350 if (codeString == null || "".equals(codeString)) 1351 return null; 1352 if ("yes".equals(codeString)) 1353 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES); 1354 if ("no".equals(codeString)) 1355 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO); 1356 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1357 } 1358 public String toCode(ActionPrecheckBehavior code) { 1359 if (code == ActionPrecheckBehavior.NULL) 1360 return null; 1361 if (code == ActionPrecheckBehavior.YES) 1362 return "yes"; 1363 if (code == ActionPrecheckBehavior.NO) 1364 return "no"; 1365 return "?"; 1366 } 1367 public String toSystem(ActionPrecheckBehavior code) { 1368 return code.getSystem(); 1369 } 1370 } 1371 1372 public enum ActionCardinalityBehavior { 1373 /** 1374 * The action may only be selected one time 1375 */ 1376 SINGLE, 1377 /** 1378 * The action may be selected multiple times 1379 */ 1380 MULTIPLE, 1381 /** 1382 * added to help the parsers with the generic types 1383 */ 1384 NULL; 1385 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1386 if (codeString == null || "".equals(codeString)) 1387 return null; 1388 if ("single".equals(codeString)) 1389 return SINGLE; 1390 if ("multiple".equals(codeString)) 1391 return MULTIPLE; 1392 if (Configuration.isAcceptInvalidEnums()) 1393 return null; 1394 else 1395 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1396 } 1397 public String toCode() { 1398 switch (this) { 1399 case SINGLE: return "single"; 1400 case MULTIPLE: return "multiple"; 1401 case NULL: return null; 1402 default: return "?"; 1403 } 1404 } 1405 public String getSystem() { 1406 switch (this) { 1407 case SINGLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1408 case MULTIPLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1409 case NULL: return null; 1410 default: return "?"; 1411 } 1412 } 1413 public String getDefinition() { 1414 switch (this) { 1415 case SINGLE: return "The action may only be selected one time"; 1416 case MULTIPLE: return "The action may be selected multiple times"; 1417 case NULL: return null; 1418 default: return "?"; 1419 } 1420 } 1421 public String getDisplay() { 1422 switch (this) { 1423 case SINGLE: return "Single"; 1424 case MULTIPLE: return "Multiple"; 1425 case NULL: return null; 1426 default: return "?"; 1427 } 1428 } 1429 } 1430 1431 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1432 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1433 if (codeString == null || "".equals(codeString)) 1434 if (codeString == null || "".equals(codeString)) 1435 return null; 1436 if ("single".equals(codeString)) 1437 return ActionCardinalityBehavior.SINGLE; 1438 if ("multiple".equals(codeString)) 1439 return ActionCardinalityBehavior.MULTIPLE; 1440 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1441 } 1442 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1443 if (code == null) 1444 return null; 1445 if (code.isEmpty()) 1446 return new Enumeration<ActionCardinalityBehavior>(this); 1447 String codeString = code.asStringValue(); 1448 if (codeString == null || "".equals(codeString)) 1449 return null; 1450 if ("single".equals(codeString)) 1451 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE); 1452 if ("multiple".equals(codeString)) 1453 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE); 1454 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1455 } 1456 public String toCode(ActionCardinalityBehavior code) { 1457 if (code == ActionCardinalityBehavior.NULL) 1458 return null; 1459 if (code == ActionCardinalityBehavior.SINGLE) 1460 return "single"; 1461 if (code == ActionCardinalityBehavior.MULTIPLE) 1462 return "multiple"; 1463 return "?"; 1464 } 1465 public String toSystem(ActionCardinalityBehavior code) { 1466 return code.getSystem(); 1467 } 1468 } 1469 1470 @Block() 1471 public static class RequestGroupActionComponent extends BackboneElement implements IBaseBackboneElement { 1472 /** 1473 * A user-visible label for the action. 1474 */ 1475 @Child(name = "label", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1476 @Description(shortDefinition="User-visible label for the action (e.g. 1. or A.)", formalDefinition="A user-visible label for the action." ) 1477 protected StringType label; 1478 1479 /** 1480 * The title of the action displayed to a user. 1481 */ 1482 @Child(name = "title", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1483 @Description(shortDefinition="User-visible title", formalDefinition="The title of the action displayed to a user." ) 1484 protected StringType title; 1485 1486 /** 1487 * A short description of the action used to provide a summary to display to the user. 1488 */ 1489 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1490 @Description(shortDefinition="Short description of the action", formalDefinition="A short description of the action used to provide a summary to display to the user." ) 1491 protected StringType description; 1492 1493 /** 1494 * A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1495 */ 1496 @Child(name = "textEquivalent", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1497 @Description(shortDefinition="Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition="A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically." ) 1498 protected StringType textEquivalent; 1499 1500 /** 1501 * A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template. 1502 */ 1503 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1504 @Description(shortDefinition="Code representing the meaning of the action or sub-actions", formalDefinition="A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template." ) 1505 protected List<CodeableConcept> code; 1506 1507 /** 1508 * Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources. 1509 */ 1510 @Child(name = "documentation", type = {RelatedArtifact.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1511 @Description(shortDefinition="Supporting documentation for the intended performer of the action", formalDefinition="Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources." ) 1512 protected List<RelatedArtifact> documentation; 1513 1514 /** 1515 * An expression that describes applicability criteria, or start/stop conditions for the action. 1516 */ 1517 @Child(name = "condition", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1518 @Description(shortDefinition="Whether or not the action is applicable", formalDefinition="An expression that describes applicability criteria, or start/stop conditions for the action." ) 1519 protected List<RequestGroupActionConditionComponent> condition; 1520 1521 /** 1522 * A relationship to another action such as "before" or "30-60 minutes after start of". 1523 */ 1524 @Child(name = "relatedAction", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1525 @Description(shortDefinition="Relationship to another action", formalDefinition="A relationship to another action such as \"before\" or \"30-60 minutes after start of\"." ) 1526 protected List<RequestGroupActionRelatedActionComponent> relatedAction; 1527 1528 /** 1529 * An optional value describing when the action should be performed. 1530 */ 1531 @Child(name = "timing", type = {DateTimeType.class, Period.class, Duration.class, Range.class, Timing.class}, order=9, min=0, max=1, modifier=false, summary=false) 1532 @Description(shortDefinition="When the action should take place", formalDefinition="An optional value describing when the action should be performed." ) 1533 protected Type timing; 1534 1535 /** 1536 * The participant that should perform or be responsible for this action. 1537 */ 1538 @Child(name = "participant", type = {Patient.class, Person.class, Practitioner.class, RelatedPerson.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1539 @Description(shortDefinition="Who should perform the action", formalDefinition="The participant that should perform or be responsible for this action." ) 1540 protected List<Reference> participant; 1541 /** 1542 * The actual objects that are the target of the reference (The participant that should perform or be responsible for this action.) 1543 */ 1544 protected List<Resource> participantTarget; 1545 1546 1547 /** 1548 * The type of action to perform (create, update, remove). 1549 */ 1550 @Child(name = "type", type = {Coding.class}, order=11, min=0, max=1, modifier=false, summary=false) 1551 @Description(shortDefinition="create | update | remove | fire-event", formalDefinition="The type of action to perform (create, update, remove)." ) 1552 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-type") 1553 protected Coding type; 1554 1555 /** 1556 * Defines the grouping behavior for the action and its children. 1557 */ 1558 @Child(name = "groupingBehavior", type = {CodeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1559 @Description(shortDefinition="visual-group | logical-group | sentence-group", formalDefinition="Defines the grouping behavior for the action and its children." ) 1560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-grouping-behavior") 1561 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 1562 1563 /** 1564 * Defines the selection behavior for the action and its children. 1565 */ 1566 @Child(name = "selectionBehavior", type = {CodeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 1567 @Description(shortDefinition="any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition="Defines the selection behavior for the action and its children." ) 1568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-selection-behavior") 1569 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 1570 1571 /** 1572 * Defines the requiredness behavior for the action. 1573 */ 1574 @Child(name = "requiredBehavior", type = {CodeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1575 @Description(shortDefinition="must | could | must-unless-documented", formalDefinition="Defines the requiredness behavior for the action." ) 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-required-behavior") 1577 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 1578 1579 /** 1580 * Defines whether the action should usually be preselected. 1581 */ 1582 @Child(name = "precheckBehavior", type = {CodeType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1583 @Description(shortDefinition="yes | no", formalDefinition="Defines whether the action should usually be preselected." ) 1584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-precheck-behavior") 1585 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 1586 1587 /** 1588 * Defines whether the action can be selected multiple times. 1589 */ 1590 @Child(name = "cardinalityBehavior", type = {CodeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1591 @Description(shortDefinition="single | multiple", formalDefinition="Defines whether the action can be selected multiple times." ) 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 1593 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 1594 1595 /** 1596 * The resource that is the target of the action (e.g. CommunicationRequest). 1597 */ 1598 @Child(name = "resource", type = {Reference.class}, order=17, min=0, max=1, modifier=false, summary=false) 1599 @Description(shortDefinition="The target of the action", formalDefinition="The resource that is the target of the action (e.g. CommunicationRequest)." ) 1600 protected Reference resource; 1601 1602 /** 1603 * The actual object that is the target of the reference (The resource that is the target of the action (e.g. CommunicationRequest).) 1604 */ 1605 protected Resource resourceTarget; 1606 1607 /** 1608 * Sub actions. 1609 */ 1610 @Child(name = "action", type = {RequestGroupActionComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1611 @Description(shortDefinition="Sub action", formalDefinition="Sub actions." ) 1612 protected List<RequestGroupActionComponent> action; 1613 1614 private static final long serialVersionUID = 362859874L; 1615 1616 /** 1617 * Constructor 1618 */ 1619 public RequestGroupActionComponent() { 1620 super(); 1621 } 1622 1623 /** 1624 * @return {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1625 */ 1626 public StringType getLabelElement() { 1627 if (this.label == null) 1628 if (Configuration.errorOnAutoCreate()) 1629 throw new Error("Attempt to auto-create RequestGroupActionComponent.label"); 1630 else if (Configuration.doAutoCreate()) 1631 this.label = new StringType(); // bb 1632 return this.label; 1633 } 1634 1635 public boolean hasLabelElement() { 1636 return this.label != null && !this.label.isEmpty(); 1637 } 1638 1639 public boolean hasLabel() { 1640 return this.label != null && !this.label.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1645 */ 1646 public RequestGroupActionComponent setLabelElement(StringType value) { 1647 this.label = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return A user-visible label for the action. 1653 */ 1654 public String getLabel() { 1655 return this.label == null ? null : this.label.getValue(); 1656 } 1657 1658 /** 1659 * @param value A user-visible label for the action. 1660 */ 1661 public RequestGroupActionComponent setLabel(String value) { 1662 if (Utilities.noString(value)) 1663 this.label = null; 1664 else { 1665 if (this.label == null) 1666 this.label = new StringType(); 1667 this.label.setValue(value); 1668 } 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1674 */ 1675 public StringType getTitleElement() { 1676 if (this.title == null) 1677 if (Configuration.errorOnAutoCreate()) 1678 throw new Error("Attempt to auto-create RequestGroupActionComponent.title"); 1679 else if (Configuration.doAutoCreate()) 1680 this.title = new StringType(); // bb 1681 return this.title; 1682 } 1683 1684 public boolean hasTitleElement() { 1685 return this.title != null && !this.title.isEmpty(); 1686 } 1687 1688 public boolean hasTitle() { 1689 return this.title != null && !this.title.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1694 */ 1695 public RequestGroupActionComponent setTitleElement(StringType value) { 1696 this.title = value; 1697 return this; 1698 } 1699 1700 /** 1701 * @return The title of the action displayed to a user. 1702 */ 1703 public String getTitle() { 1704 return this.title == null ? null : this.title.getValue(); 1705 } 1706 1707 /** 1708 * @param value The title of the action displayed to a user. 1709 */ 1710 public RequestGroupActionComponent setTitle(String value) { 1711 if (Utilities.noString(value)) 1712 this.title = null; 1713 else { 1714 if (this.title == null) 1715 this.title = new StringType(); 1716 this.title.setValue(value); 1717 } 1718 return this; 1719 } 1720 1721 /** 1722 * @return {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1723 */ 1724 public StringType getDescriptionElement() { 1725 if (this.description == null) 1726 if (Configuration.errorOnAutoCreate()) 1727 throw new Error("Attempt to auto-create RequestGroupActionComponent.description"); 1728 else if (Configuration.doAutoCreate()) 1729 this.description = new StringType(); // bb 1730 return this.description; 1731 } 1732 1733 public boolean hasDescriptionElement() { 1734 return this.description != null && !this.description.isEmpty(); 1735 } 1736 1737 public boolean hasDescription() { 1738 return this.description != null && !this.description.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1743 */ 1744 public RequestGroupActionComponent setDescriptionElement(StringType value) { 1745 this.description = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return A short description of the action used to provide a summary to display to the user. 1751 */ 1752 public String getDescription() { 1753 return this.description == null ? null : this.description.getValue(); 1754 } 1755 1756 /** 1757 * @param value A short description of the action used to provide a summary to display to the user. 1758 */ 1759 public RequestGroupActionComponent setDescription(String value) { 1760 if (Utilities.noString(value)) 1761 this.description = null; 1762 else { 1763 if (this.description == null) 1764 this.description = new StringType(); 1765 this.description.setValue(value); 1766 } 1767 return this; 1768 } 1769 1770 /** 1771 * @return {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 1772 */ 1773 public StringType getTextEquivalentElement() { 1774 if (this.textEquivalent == null) 1775 if (Configuration.errorOnAutoCreate()) 1776 throw new Error("Attempt to auto-create RequestGroupActionComponent.textEquivalent"); 1777 else if (Configuration.doAutoCreate()) 1778 this.textEquivalent = new StringType(); // bb 1779 return this.textEquivalent; 1780 } 1781 1782 public boolean hasTextEquivalentElement() { 1783 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 1784 } 1785 1786 public boolean hasTextEquivalent() { 1787 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 1788 } 1789 1790 /** 1791 * @param value {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 1792 */ 1793 public RequestGroupActionComponent setTextEquivalentElement(StringType value) { 1794 this.textEquivalent = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1800 */ 1801 public String getTextEquivalent() { 1802 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 1803 } 1804 1805 /** 1806 * @param value A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1807 */ 1808 public RequestGroupActionComponent setTextEquivalent(String value) { 1809 if (Utilities.noString(value)) 1810 this.textEquivalent = null; 1811 else { 1812 if (this.textEquivalent == null) 1813 this.textEquivalent = new StringType(); 1814 this.textEquivalent.setValue(value); 1815 } 1816 return this; 1817 } 1818 1819 /** 1820 * @return {@link #code} (A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.) 1821 */ 1822 public List<CodeableConcept> getCode() { 1823 if (this.code == null) 1824 this.code = new ArrayList<CodeableConcept>(); 1825 return this.code; 1826 } 1827 1828 /** 1829 * @return Returns a reference to <code>this</code> for easy method chaining 1830 */ 1831 public RequestGroupActionComponent setCode(List<CodeableConcept> theCode) { 1832 this.code = theCode; 1833 return this; 1834 } 1835 1836 public boolean hasCode() { 1837 if (this.code == null) 1838 return false; 1839 for (CodeableConcept item : this.code) 1840 if (!item.isEmpty()) 1841 return true; 1842 return false; 1843 } 1844 1845 public CodeableConcept addCode() { //3 1846 CodeableConcept t = new CodeableConcept(); 1847 if (this.code == null) 1848 this.code = new ArrayList<CodeableConcept>(); 1849 this.code.add(t); 1850 return t; 1851 } 1852 1853 public RequestGroupActionComponent addCode(CodeableConcept t) { //3 1854 if (t == null) 1855 return this; 1856 if (this.code == null) 1857 this.code = new ArrayList<CodeableConcept>(); 1858 this.code.add(t); 1859 return this; 1860 } 1861 1862 /** 1863 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1864 */ 1865 public CodeableConcept getCodeFirstRep() { 1866 if (getCode().isEmpty()) { 1867 addCode(); 1868 } 1869 return getCode().get(0); 1870 } 1871 1872 /** 1873 * @return {@link #documentation} (Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.) 1874 */ 1875 public List<RelatedArtifact> getDocumentation() { 1876 if (this.documentation == null) 1877 this.documentation = new ArrayList<RelatedArtifact>(); 1878 return this.documentation; 1879 } 1880 1881 /** 1882 * @return Returns a reference to <code>this</code> for easy method chaining 1883 */ 1884 public RequestGroupActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 1885 this.documentation = theDocumentation; 1886 return this; 1887 } 1888 1889 public boolean hasDocumentation() { 1890 if (this.documentation == null) 1891 return false; 1892 for (RelatedArtifact item : this.documentation) 1893 if (!item.isEmpty()) 1894 return true; 1895 return false; 1896 } 1897 1898 public RelatedArtifact addDocumentation() { //3 1899 RelatedArtifact t = new RelatedArtifact(); 1900 if (this.documentation == null) 1901 this.documentation = new ArrayList<RelatedArtifact>(); 1902 this.documentation.add(t); 1903 return t; 1904 } 1905 1906 public RequestGroupActionComponent addDocumentation(RelatedArtifact t) { //3 1907 if (t == null) 1908 return this; 1909 if (this.documentation == null) 1910 this.documentation = new ArrayList<RelatedArtifact>(); 1911 this.documentation.add(t); 1912 return this; 1913 } 1914 1915 /** 1916 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist 1917 */ 1918 public RelatedArtifact getDocumentationFirstRep() { 1919 if (getDocumentation().isEmpty()) { 1920 addDocumentation(); 1921 } 1922 return getDocumentation().get(0); 1923 } 1924 1925 /** 1926 * @return {@link #condition} (An expression that describes applicability criteria, or start/stop conditions for the action.) 1927 */ 1928 public List<RequestGroupActionConditionComponent> getCondition() { 1929 if (this.condition == null) 1930 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1931 return this.condition; 1932 } 1933 1934 /** 1935 * @return Returns a reference to <code>this</code> for easy method chaining 1936 */ 1937 public RequestGroupActionComponent setCondition(List<RequestGroupActionConditionComponent> theCondition) { 1938 this.condition = theCondition; 1939 return this; 1940 } 1941 1942 public boolean hasCondition() { 1943 if (this.condition == null) 1944 return false; 1945 for (RequestGroupActionConditionComponent item : this.condition) 1946 if (!item.isEmpty()) 1947 return true; 1948 return false; 1949 } 1950 1951 public RequestGroupActionConditionComponent addCondition() { //3 1952 RequestGroupActionConditionComponent t = new RequestGroupActionConditionComponent(); 1953 if (this.condition == null) 1954 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1955 this.condition.add(t); 1956 return t; 1957 } 1958 1959 public RequestGroupActionComponent addCondition(RequestGroupActionConditionComponent t) { //3 1960 if (t == null) 1961 return this; 1962 if (this.condition == null) 1963 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1964 this.condition.add(t); 1965 return this; 1966 } 1967 1968 /** 1969 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist 1970 */ 1971 public RequestGroupActionConditionComponent getConditionFirstRep() { 1972 if (getCondition().isEmpty()) { 1973 addCondition(); 1974 } 1975 return getCondition().get(0); 1976 } 1977 1978 /** 1979 * @return {@link #relatedAction} (A relationship to another action such as "before" or "30-60 minutes after start of".) 1980 */ 1981 public List<RequestGroupActionRelatedActionComponent> getRelatedAction() { 1982 if (this.relatedAction == null) 1983 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 1984 return this.relatedAction; 1985 } 1986 1987 /** 1988 * @return Returns a reference to <code>this</code> for easy method chaining 1989 */ 1990 public RequestGroupActionComponent setRelatedAction(List<RequestGroupActionRelatedActionComponent> theRelatedAction) { 1991 this.relatedAction = theRelatedAction; 1992 return this; 1993 } 1994 1995 public boolean hasRelatedAction() { 1996 if (this.relatedAction == null) 1997 return false; 1998 for (RequestGroupActionRelatedActionComponent item : this.relatedAction) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public RequestGroupActionRelatedActionComponent addRelatedAction() { //3 2005 RequestGroupActionRelatedActionComponent t = new RequestGroupActionRelatedActionComponent(); 2006 if (this.relatedAction == null) 2007 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2008 this.relatedAction.add(t); 2009 return t; 2010 } 2011 2012 public RequestGroupActionComponent addRelatedAction(RequestGroupActionRelatedActionComponent t) { //3 2013 if (t == null) 2014 return this; 2015 if (this.relatedAction == null) 2016 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2017 this.relatedAction.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field {@link #relatedAction}, creating it if it does not already exist 2023 */ 2024 public RequestGroupActionRelatedActionComponent getRelatedActionFirstRep() { 2025 if (getRelatedAction().isEmpty()) { 2026 addRelatedAction(); 2027 } 2028 return getRelatedAction().get(0); 2029 } 2030 2031 /** 2032 * @return {@link #timing} (An optional value describing when the action should be performed.) 2033 */ 2034 public Type getTiming() { 2035 return this.timing; 2036 } 2037 2038 /** 2039 * @return {@link #timing} (An optional value describing when the action should be performed.) 2040 */ 2041 public DateTimeType getTimingDateTimeType() throws FHIRException { 2042 if (this.timing == null) 2043 return null; 2044 if (!(this.timing instanceof DateTimeType)) 2045 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 2046 return (DateTimeType) this.timing; 2047 } 2048 2049 public boolean hasTimingDateTimeType() { 2050 return this.timing instanceof DateTimeType; 2051 } 2052 2053 /** 2054 * @return {@link #timing} (An optional value describing when the action should be performed.) 2055 */ 2056 public Period getTimingPeriod() throws FHIRException { 2057 if (this.timing == null) 2058 return null; 2059 if (!(this.timing instanceof Period)) 2060 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 2061 return (Period) this.timing; 2062 } 2063 2064 public boolean hasTimingPeriod() { 2065 return this.timing instanceof Period; 2066 } 2067 2068 /** 2069 * @return {@link #timing} (An optional value describing when the action should be performed.) 2070 */ 2071 public Duration getTimingDuration() throws FHIRException { 2072 if (this.timing == null) 2073 return null; 2074 if (!(this.timing instanceof Duration)) 2075 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 2076 return (Duration) this.timing; 2077 } 2078 2079 public boolean hasTimingDuration() { 2080 return this.timing instanceof Duration; 2081 } 2082 2083 /** 2084 * @return {@link #timing} (An optional value describing when the action should be performed.) 2085 */ 2086 public Range getTimingRange() throws FHIRException { 2087 if (this.timing == null) 2088 return null; 2089 if (!(this.timing instanceof Range)) 2090 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 2091 return (Range) this.timing; 2092 } 2093 2094 public boolean hasTimingRange() { 2095 return this.timing instanceof Range; 2096 } 2097 2098 /** 2099 * @return {@link #timing} (An optional value describing when the action should be performed.) 2100 */ 2101 public Timing getTimingTiming() throws FHIRException { 2102 if (this.timing == null) 2103 return null; 2104 if (!(this.timing instanceof Timing)) 2105 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 2106 return (Timing) this.timing; 2107 } 2108 2109 public boolean hasTimingTiming() { 2110 return this.timing instanceof Timing; 2111 } 2112 2113 public boolean hasTiming() { 2114 return this.timing != null && !this.timing.isEmpty(); 2115 } 2116 2117 /** 2118 * @param value {@link #timing} (An optional value describing when the action should be performed.) 2119 */ 2120 public RequestGroupActionComponent setTiming(Type value) throws FHIRFormatError { 2121 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2122 throw new FHIRFormatError("Not the right type for RequestGroup.action.timing[x]: "+value.fhirType()); 2123 this.timing = value; 2124 return this; 2125 } 2126 2127 /** 2128 * @return {@link #participant} (The participant that should perform or be responsible for this action.) 2129 */ 2130 public List<Reference> getParticipant() { 2131 if (this.participant == null) 2132 this.participant = new ArrayList<Reference>(); 2133 return this.participant; 2134 } 2135 2136 /** 2137 * @return Returns a reference to <code>this</code> for easy method chaining 2138 */ 2139 public RequestGroupActionComponent setParticipant(List<Reference> theParticipant) { 2140 this.participant = theParticipant; 2141 return this; 2142 } 2143 2144 public boolean hasParticipant() { 2145 if (this.participant == null) 2146 return false; 2147 for (Reference item : this.participant) 2148 if (!item.isEmpty()) 2149 return true; 2150 return false; 2151 } 2152 2153 public Reference addParticipant() { //3 2154 Reference t = new Reference(); 2155 if (this.participant == null) 2156 this.participant = new ArrayList<Reference>(); 2157 this.participant.add(t); 2158 return t; 2159 } 2160 2161 public RequestGroupActionComponent addParticipant(Reference t) { //3 2162 if (t == null) 2163 return this; 2164 if (this.participant == null) 2165 this.participant = new ArrayList<Reference>(); 2166 this.participant.add(t); 2167 return this; 2168 } 2169 2170 /** 2171 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 2172 */ 2173 public Reference getParticipantFirstRep() { 2174 if (getParticipant().isEmpty()) { 2175 addParticipant(); 2176 } 2177 return getParticipant().get(0); 2178 } 2179 2180 /** 2181 * @return {@link #type} (The type of action to perform (create, update, remove).) 2182 */ 2183 public Coding getType() { 2184 if (this.type == null) 2185 if (Configuration.errorOnAutoCreate()) 2186 throw new Error("Attempt to auto-create RequestGroupActionComponent.type"); 2187 else if (Configuration.doAutoCreate()) 2188 this.type = new Coding(); // cc 2189 return this.type; 2190 } 2191 2192 public boolean hasType() { 2193 return this.type != null && !this.type.isEmpty(); 2194 } 2195 2196 /** 2197 * @param value {@link #type} (The type of action to perform (create, update, remove).) 2198 */ 2199 public RequestGroupActionComponent setType(Coding value) { 2200 this.type = value; 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2206 */ 2207 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2208 if (this.groupingBehavior == null) 2209 if (Configuration.errorOnAutoCreate()) 2210 throw new Error("Attempt to auto-create RequestGroupActionComponent.groupingBehavior"); 2211 else if (Configuration.doAutoCreate()) 2212 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2213 return this.groupingBehavior; 2214 } 2215 2216 public boolean hasGroupingBehaviorElement() { 2217 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2218 } 2219 2220 public boolean hasGroupingBehavior() { 2221 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2222 } 2223 2224 /** 2225 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2226 */ 2227 public RequestGroupActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 2228 this.groupingBehavior = value; 2229 return this; 2230 } 2231 2232 /** 2233 * @return Defines the grouping behavior for the action and its children. 2234 */ 2235 public ActionGroupingBehavior getGroupingBehavior() { 2236 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 2237 } 2238 2239 /** 2240 * @param value Defines the grouping behavior for the action and its children. 2241 */ 2242 public RequestGroupActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 2243 if (value == null) 2244 this.groupingBehavior = null; 2245 else { 2246 if (this.groupingBehavior == null) 2247 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 2248 this.groupingBehavior.setValue(value); 2249 } 2250 return this; 2251 } 2252 2253 /** 2254 * @return {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 2255 */ 2256 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 2257 if (this.selectionBehavior == null) 2258 if (Configuration.errorOnAutoCreate()) 2259 throw new Error("Attempt to auto-create RequestGroupActionComponent.selectionBehavior"); 2260 else if (Configuration.doAutoCreate()) 2261 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 2262 return this.selectionBehavior; 2263 } 2264 2265 public boolean hasSelectionBehaviorElement() { 2266 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2267 } 2268 2269 public boolean hasSelectionBehavior() { 2270 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2271 } 2272 2273 /** 2274 * @param value {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 2275 */ 2276 public RequestGroupActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 2277 this.selectionBehavior = value; 2278 return this; 2279 } 2280 2281 /** 2282 * @return Defines the selection behavior for the action and its children. 2283 */ 2284 public ActionSelectionBehavior getSelectionBehavior() { 2285 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 2286 } 2287 2288 /** 2289 * @param value Defines the selection behavior for the action and its children. 2290 */ 2291 public RequestGroupActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 2292 if (value == null) 2293 this.selectionBehavior = null; 2294 else { 2295 if (this.selectionBehavior == null) 2296 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 2297 this.selectionBehavior.setValue(value); 2298 } 2299 return this; 2300 } 2301 2302 /** 2303 * @return {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 2304 */ 2305 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 2306 if (this.requiredBehavior == null) 2307 if (Configuration.errorOnAutoCreate()) 2308 throw new Error("Attempt to auto-create RequestGroupActionComponent.requiredBehavior"); 2309 else if (Configuration.doAutoCreate()) 2310 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 2311 return this.requiredBehavior; 2312 } 2313 2314 public boolean hasRequiredBehaviorElement() { 2315 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2316 } 2317 2318 public boolean hasRequiredBehavior() { 2319 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2320 } 2321 2322 /** 2323 * @param value {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 2324 */ 2325 public RequestGroupActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 2326 this.requiredBehavior = value; 2327 return this; 2328 } 2329 2330 /** 2331 * @return Defines the requiredness behavior for the action. 2332 */ 2333 public ActionRequiredBehavior getRequiredBehavior() { 2334 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 2335 } 2336 2337 /** 2338 * @param value Defines the requiredness behavior for the action. 2339 */ 2340 public RequestGroupActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 2341 if (value == null) 2342 this.requiredBehavior = null; 2343 else { 2344 if (this.requiredBehavior == null) 2345 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 2346 this.requiredBehavior.setValue(value); 2347 } 2348 return this; 2349 } 2350 2351 /** 2352 * @return {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 2353 */ 2354 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 2355 if (this.precheckBehavior == null) 2356 if (Configuration.errorOnAutoCreate()) 2357 throw new Error("Attempt to auto-create RequestGroupActionComponent.precheckBehavior"); 2358 else if (Configuration.doAutoCreate()) 2359 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 2360 return this.precheckBehavior; 2361 } 2362 2363 public boolean hasPrecheckBehaviorElement() { 2364 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2365 } 2366 2367 public boolean hasPrecheckBehavior() { 2368 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2369 } 2370 2371 /** 2372 * @param value {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 2373 */ 2374 public RequestGroupActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 2375 this.precheckBehavior = value; 2376 return this; 2377 } 2378 2379 /** 2380 * @return Defines whether the action should usually be preselected. 2381 */ 2382 public ActionPrecheckBehavior getPrecheckBehavior() { 2383 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 2384 } 2385 2386 /** 2387 * @param value Defines whether the action should usually be preselected. 2388 */ 2389 public RequestGroupActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 2390 if (value == null) 2391 this.precheckBehavior = null; 2392 else { 2393 if (this.precheckBehavior == null) 2394 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 2395 this.precheckBehavior.setValue(value); 2396 } 2397 return this; 2398 } 2399 2400 /** 2401 * @return {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 2402 */ 2403 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 2404 if (this.cardinalityBehavior == null) 2405 if (Configuration.errorOnAutoCreate()) 2406 throw new Error("Attempt to auto-create RequestGroupActionComponent.cardinalityBehavior"); 2407 else if (Configuration.doAutoCreate()) 2408 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); // bb 2409 return this.cardinalityBehavior; 2410 } 2411 2412 public boolean hasCardinalityBehaviorElement() { 2413 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 2414 } 2415 2416 public boolean hasCardinalityBehavior() { 2417 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 2418 } 2419 2420 /** 2421 * @param value {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 2422 */ 2423 public RequestGroupActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 2424 this.cardinalityBehavior = value; 2425 return this; 2426 } 2427 2428 /** 2429 * @return Defines whether the action can be selected multiple times. 2430 */ 2431 public ActionCardinalityBehavior getCardinalityBehavior() { 2432 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 2433 } 2434 2435 /** 2436 * @param value Defines whether the action can be selected multiple times. 2437 */ 2438 public RequestGroupActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 2439 if (value == null) 2440 this.cardinalityBehavior = null; 2441 else { 2442 if (this.cardinalityBehavior == null) 2443 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); 2444 this.cardinalityBehavior.setValue(value); 2445 } 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 2451 */ 2452 public Reference getResource() { 2453 if (this.resource == null) 2454 if (Configuration.errorOnAutoCreate()) 2455 throw new Error("Attempt to auto-create RequestGroupActionComponent.resource"); 2456 else if (Configuration.doAutoCreate()) 2457 this.resource = new Reference(); // cc 2458 return this.resource; 2459 } 2460 2461 public boolean hasResource() { 2462 return this.resource != null && !this.resource.isEmpty(); 2463 } 2464 2465 /** 2466 * @param value {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 2467 */ 2468 public RequestGroupActionComponent setResource(Reference value) { 2469 this.resource = value; 2470 return this; 2471 } 2472 2473 /** 2474 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that is the target of the action (e.g. CommunicationRequest).) 2475 */ 2476 public Resource getResourceTarget() { 2477 return this.resourceTarget; 2478 } 2479 2480 /** 2481 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that is the target of the action (e.g. CommunicationRequest).) 2482 */ 2483 public RequestGroupActionComponent setResourceTarget(Resource value) { 2484 this.resourceTarget = value; 2485 return this; 2486 } 2487 2488 /** 2489 * @return {@link #action} (Sub actions.) 2490 */ 2491 public List<RequestGroupActionComponent> getAction() { 2492 if (this.action == null) 2493 this.action = new ArrayList<RequestGroupActionComponent>(); 2494 return this.action; 2495 } 2496 2497 /** 2498 * @return Returns a reference to <code>this</code> for easy method chaining 2499 */ 2500 public RequestGroupActionComponent setAction(List<RequestGroupActionComponent> theAction) { 2501 this.action = theAction; 2502 return this; 2503 } 2504 2505 public boolean hasAction() { 2506 if (this.action == null) 2507 return false; 2508 for (RequestGroupActionComponent item : this.action) 2509 if (!item.isEmpty()) 2510 return true; 2511 return false; 2512 } 2513 2514 public RequestGroupActionComponent addAction() { //3 2515 RequestGroupActionComponent t = new RequestGroupActionComponent(); 2516 if (this.action == null) 2517 this.action = new ArrayList<RequestGroupActionComponent>(); 2518 this.action.add(t); 2519 return t; 2520 } 2521 2522 public RequestGroupActionComponent addAction(RequestGroupActionComponent t) { //3 2523 if (t == null) 2524 return this; 2525 if (this.action == null) 2526 this.action = new ArrayList<RequestGroupActionComponent>(); 2527 this.action.add(t); 2528 return this; 2529 } 2530 2531 /** 2532 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 2533 */ 2534 public RequestGroupActionComponent getActionFirstRep() { 2535 if (getAction().isEmpty()) { 2536 addAction(); 2537 } 2538 return getAction().get(0); 2539 } 2540 2541 protected void listChildren(List<Property> children) { 2542 super.listChildren(children); 2543 children.add(new Property("label", "string", "A user-visible label for the action.", 0, 1, label)); 2544 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 2545 children.add(new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 2546 children.add(new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent)); 2547 children.add(new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code)); 2548 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 2549 children.add(new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition)); 2550 children.add(new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction)); 2551 children.add(new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing)); 2552 children.add(new Property("participant", "Reference(Patient|Person|Practitioner|RelatedPerson)", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant)); 2553 children.add(new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type)); 2554 children.add(new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 2555 children.add(new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 2556 children.add(new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior)); 2557 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior)); 2558 children.add(new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 2559 children.add(new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource)); 2560 children.add(new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action)); 2561 } 2562 2563 @Override 2564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2565 switch (_hash) { 2566 case 102727412: /*label*/ return new Property("label", "string", "A user-visible label for the action.", 0, 1, label); 2567 case 110371416: /*title*/ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 2568 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 2569 case -900391049: /*textEquivalent*/ return new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent); 2570 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code); 2571 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 2572 case -861311717: /*condition*/ return new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition); 2573 case -384107967: /*relatedAction*/ return new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction); 2574 case 164632566: /*timing[x]*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2575 case -873664438: /*timing*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2576 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2577 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2578 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2579 case -710871277: /*timingRange*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2580 case -497554124: /*timingTiming*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2581 case 767422259: /*participant*/ return new Property("participant", "Reference(Patient|Person|Practitioner|RelatedPerson)", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant); 2582 case 3575610: /*type*/ return new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type); 2583 case 586678389: /*groupingBehavior*/ return new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 2584 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 2585 case -1163906287: /*requiredBehavior*/ return new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior); 2586 case -1174249033: /*precheckBehavior*/ return new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 2587 case -922577408: /*cardinalityBehavior*/ return new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 2588 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource); 2589 case -1422950858: /*action*/ return new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action); 2590 default: return super.getNamedProperty(_hash, _name, _checkValid); 2591 } 2592 2593 } 2594 2595 @Override 2596 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2597 switch (hash) { 2598 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 2599 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2600 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2601 case -900391049: /*textEquivalent*/ return this.textEquivalent == null ? new Base[0] : new Base[] {this.textEquivalent}; // StringType 2602 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2603 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 2604 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // RequestGroupActionConditionComponent 2605 case -384107967: /*relatedAction*/ return this.relatedAction == null ? new Base[0] : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // RequestGroupActionRelatedActionComponent 2606 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 2607 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // Reference 2608 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 2609 case 586678389: /*groupingBehavior*/ return this.groupingBehavior == null ? new Base[0] : new Base[] {this.groupingBehavior}; // Enumeration<ActionGroupingBehavior> 2610 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<ActionSelectionBehavior> 2611 case -1163906287: /*requiredBehavior*/ return this.requiredBehavior == null ? new Base[0] : new Base[] {this.requiredBehavior}; // Enumeration<ActionRequiredBehavior> 2612 case -1174249033: /*precheckBehavior*/ return this.precheckBehavior == null ? new Base[0] : new Base[] {this.precheckBehavior}; // Enumeration<ActionPrecheckBehavior> 2613 case -922577408: /*cardinalityBehavior*/ return this.cardinalityBehavior == null ? new Base[0] : new Base[] {this.cardinalityBehavior}; // Enumeration<ActionCardinalityBehavior> 2614 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 2615 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 2616 default: return super.getProperty(hash, name, checkValid); 2617 } 2618 2619 } 2620 2621 @Override 2622 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2623 switch (hash) { 2624 case 102727412: // label 2625 this.label = castToString(value); // StringType 2626 return value; 2627 case 110371416: // title 2628 this.title = castToString(value); // StringType 2629 return value; 2630 case -1724546052: // description 2631 this.description = castToString(value); // StringType 2632 return value; 2633 case -900391049: // textEquivalent 2634 this.textEquivalent = castToString(value); // StringType 2635 return value; 2636 case 3059181: // code 2637 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 2638 return value; 2639 case 1587405498: // documentation 2640 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 2641 return value; 2642 case -861311717: // condition 2643 this.getCondition().add((RequestGroupActionConditionComponent) value); // RequestGroupActionConditionComponent 2644 return value; 2645 case -384107967: // relatedAction 2646 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); // RequestGroupActionRelatedActionComponent 2647 return value; 2648 case -873664438: // timing 2649 this.timing = castToType(value); // Type 2650 return value; 2651 case 767422259: // participant 2652 this.getParticipant().add(castToReference(value)); // Reference 2653 return value; 2654 case 3575610: // type 2655 this.type = castToCoding(value); // Coding 2656 return value; 2657 case 586678389: // groupingBehavior 2658 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 2659 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 2660 return value; 2661 case 168639486: // selectionBehavior 2662 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 2663 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 2664 return value; 2665 case -1163906287: // requiredBehavior 2666 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 2667 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 2668 return value; 2669 case -1174249033: // precheckBehavior 2670 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 2671 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 2672 return value; 2673 case -922577408: // cardinalityBehavior 2674 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 2675 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 2676 return value; 2677 case -341064690: // resource 2678 this.resource = castToReference(value); // Reference 2679 return value; 2680 case -1422950858: // action 2681 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 2682 return value; 2683 default: return super.setProperty(hash, name, value); 2684 } 2685 2686 } 2687 2688 @Override 2689 public Base setProperty(String name, Base value) throws FHIRException { 2690 if (name.equals("label")) { 2691 this.label = castToString(value); // StringType 2692 } else if (name.equals("title")) { 2693 this.title = castToString(value); // StringType 2694 } else if (name.equals("description")) { 2695 this.description = castToString(value); // StringType 2696 } else if (name.equals("textEquivalent")) { 2697 this.textEquivalent = castToString(value); // StringType 2698 } else if (name.equals("code")) { 2699 this.getCode().add(castToCodeableConcept(value)); 2700 } else if (name.equals("documentation")) { 2701 this.getDocumentation().add(castToRelatedArtifact(value)); 2702 } else if (name.equals("condition")) { 2703 this.getCondition().add((RequestGroupActionConditionComponent) value); 2704 } else if (name.equals("relatedAction")) { 2705 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); 2706 } else if (name.equals("timing[x]")) { 2707 this.timing = castToType(value); // Type 2708 } else if (name.equals("participant")) { 2709 this.getParticipant().add(castToReference(value)); 2710 } else if (name.equals("type")) { 2711 this.type = castToCoding(value); // Coding 2712 } else if (name.equals("groupingBehavior")) { 2713 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 2714 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 2715 } else if (name.equals("selectionBehavior")) { 2716 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 2717 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 2718 } else if (name.equals("requiredBehavior")) { 2719 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 2720 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 2721 } else if (name.equals("precheckBehavior")) { 2722 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 2723 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 2724 } else if (name.equals("cardinalityBehavior")) { 2725 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 2726 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 2727 } else if (name.equals("resource")) { 2728 this.resource = castToReference(value); // Reference 2729 } else if (name.equals("action")) { 2730 this.getAction().add((RequestGroupActionComponent) value); 2731 } else 2732 return super.setProperty(name, value); 2733 return value; 2734 } 2735 2736 @Override 2737 public Base makeProperty(int hash, String name) throws FHIRException { 2738 switch (hash) { 2739 case 102727412: return getLabelElement(); 2740 case 110371416: return getTitleElement(); 2741 case -1724546052: return getDescriptionElement(); 2742 case -900391049: return getTextEquivalentElement(); 2743 case 3059181: return addCode(); 2744 case 1587405498: return addDocumentation(); 2745 case -861311717: return addCondition(); 2746 case -384107967: return addRelatedAction(); 2747 case 164632566: return getTiming(); 2748 case -873664438: return getTiming(); 2749 case 767422259: return addParticipant(); 2750 case 3575610: return getType(); 2751 case 586678389: return getGroupingBehaviorElement(); 2752 case 168639486: return getSelectionBehaviorElement(); 2753 case -1163906287: return getRequiredBehaviorElement(); 2754 case -1174249033: return getPrecheckBehaviorElement(); 2755 case -922577408: return getCardinalityBehaviorElement(); 2756 case -341064690: return getResource(); 2757 case -1422950858: return addAction(); 2758 default: return super.makeProperty(hash, name); 2759 } 2760 2761 } 2762 2763 @Override 2764 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2765 switch (hash) { 2766 case 102727412: /*label*/ return new String[] {"string"}; 2767 case 110371416: /*title*/ return new String[] {"string"}; 2768 case -1724546052: /*description*/ return new String[] {"string"}; 2769 case -900391049: /*textEquivalent*/ return new String[] {"string"}; 2770 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2771 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 2772 case -861311717: /*condition*/ return new String[] {}; 2773 case -384107967: /*relatedAction*/ return new String[] {}; 2774 case -873664438: /*timing*/ return new String[] {"dateTime", "Period", "Duration", "Range", "Timing"}; 2775 case 767422259: /*participant*/ return new String[] {"Reference"}; 2776 case 3575610: /*type*/ return new String[] {"Coding"}; 2777 case 586678389: /*groupingBehavior*/ return new String[] {"code"}; 2778 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 2779 case -1163906287: /*requiredBehavior*/ return new String[] {"code"}; 2780 case -1174249033: /*precheckBehavior*/ return new String[] {"code"}; 2781 case -922577408: /*cardinalityBehavior*/ return new String[] {"code"}; 2782 case -341064690: /*resource*/ return new String[] {"Reference"}; 2783 case -1422950858: /*action*/ return new String[] {"@RequestGroup.action"}; 2784 default: return super.getTypesForProperty(hash, name); 2785 } 2786 2787 } 2788 2789 @Override 2790 public Base addChild(String name) throws FHIRException { 2791 if (name.equals("label")) { 2792 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.label"); 2793 } 2794 else if (name.equals("title")) { 2795 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.title"); 2796 } 2797 else if (name.equals("description")) { 2798 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 2799 } 2800 else if (name.equals("textEquivalent")) { 2801 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.textEquivalent"); 2802 } 2803 else if (name.equals("code")) { 2804 return addCode(); 2805 } 2806 else if (name.equals("documentation")) { 2807 return addDocumentation(); 2808 } 2809 else if (name.equals("condition")) { 2810 return addCondition(); 2811 } 2812 else if (name.equals("relatedAction")) { 2813 return addRelatedAction(); 2814 } 2815 else if (name.equals("timingDateTime")) { 2816 this.timing = new DateTimeType(); 2817 return this.timing; 2818 } 2819 else if (name.equals("timingPeriod")) { 2820 this.timing = new Period(); 2821 return this.timing; 2822 } 2823 else if (name.equals("timingDuration")) { 2824 this.timing = new Duration(); 2825 return this.timing; 2826 } 2827 else if (name.equals("timingRange")) { 2828 this.timing = new Range(); 2829 return this.timing; 2830 } 2831 else if (name.equals("timingTiming")) { 2832 this.timing = new Timing(); 2833 return this.timing; 2834 } 2835 else if (name.equals("participant")) { 2836 return addParticipant(); 2837 } 2838 else if (name.equals("type")) { 2839 this.type = new Coding(); 2840 return this.type; 2841 } 2842 else if (name.equals("groupingBehavior")) { 2843 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.groupingBehavior"); 2844 } 2845 else if (name.equals("selectionBehavior")) { 2846 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.selectionBehavior"); 2847 } 2848 else if (name.equals("requiredBehavior")) { 2849 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.requiredBehavior"); 2850 } 2851 else if (name.equals("precheckBehavior")) { 2852 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.precheckBehavior"); 2853 } 2854 else if (name.equals("cardinalityBehavior")) { 2855 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.cardinalityBehavior"); 2856 } 2857 else if (name.equals("resource")) { 2858 this.resource = new Reference(); 2859 return this.resource; 2860 } 2861 else if (name.equals("action")) { 2862 return addAction(); 2863 } 2864 else 2865 return super.addChild(name); 2866 } 2867 2868 public RequestGroupActionComponent copy() { 2869 RequestGroupActionComponent dst = new RequestGroupActionComponent(); 2870 copyValues(dst); 2871 dst.label = label == null ? null : label.copy(); 2872 dst.title = title == null ? null : title.copy(); 2873 dst.description = description == null ? null : description.copy(); 2874 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 2875 if (code != null) { 2876 dst.code = new ArrayList<CodeableConcept>(); 2877 for (CodeableConcept i : code) 2878 dst.code.add(i.copy()); 2879 }; 2880 if (documentation != null) { 2881 dst.documentation = new ArrayList<RelatedArtifact>(); 2882 for (RelatedArtifact i : documentation) 2883 dst.documentation.add(i.copy()); 2884 }; 2885 if (condition != null) { 2886 dst.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2887 for (RequestGroupActionConditionComponent i : condition) 2888 dst.condition.add(i.copy()); 2889 }; 2890 if (relatedAction != null) { 2891 dst.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2892 for (RequestGroupActionRelatedActionComponent i : relatedAction) 2893 dst.relatedAction.add(i.copy()); 2894 }; 2895 dst.timing = timing == null ? null : timing.copy(); 2896 if (participant != null) { 2897 dst.participant = new ArrayList<Reference>(); 2898 for (Reference i : participant) 2899 dst.participant.add(i.copy()); 2900 }; 2901 dst.type = type == null ? null : type.copy(); 2902 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 2903 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 2904 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 2905 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 2906 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 2907 dst.resource = resource == null ? null : resource.copy(); 2908 if (action != null) { 2909 dst.action = new ArrayList<RequestGroupActionComponent>(); 2910 for (RequestGroupActionComponent i : action) 2911 dst.action.add(i.copy()); 2912 }; 2913 return dst; 2914 } 2915 2916 @Override 2917 public boolean equalsDeep(Base other_) { 2918 if (!super.equalsDeep(other_)) 2919 return false; 2920 if (!(other_ instanceof RequestGroupActionComponent)) 2921 return false; 2922 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 2923 return compareDeep(label, o.label, true) && compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 2924 && compareDeep(textEquivalent, o.textEquivalent, true) && compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true) 2925 && compareDeep(condition, o.condition, true) && compareDeep(relatedAction, o.relatedAction, true) 2926 && compareDeep(timing, o.timing, true) && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 2927 && compareDeep(groupingBehavior, o.groupingBehavior, true) && compareDeep(selectionBehavior, o.selectionBehavior, true) 2928 && compareDeep(requiredBehavior, o.requiredBehavior, true) && compareDeep(precheckBehavior, o.precheckBehavior, true) 2929 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(resource, o.resource, true) 2930 && compareDeep(action, o.action, true); 2931 } 2932 2933 @Override 2934 public boolean equalsShallow(Base other_) { 2935 if (!super.equalsShallow(other_)) 2936 return false; 2937 if (!(other_ instanceof RequestGroupActionComponent)) 2938 return false; 2939 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 2940 return compareValues(label, o.label, true) && compareValues(title, o.title, true) && compareValues(description, o.description, true) 2941 && compareValues(textEquivalent, o.textEquivalent, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 2942 && compareValues(selectionBehavior, o.selectionBehavior, true) && compareValues(requiredBehavior, o.requiredBehavior, true) 2943 && compareValues(precheckBehavior, o.precheckBehavior, true) && compareValues(cardinalityBehavior, o.cardinalityBehavior, true) 2944 ; 2945 } 2946 2947 public boolean isEmpty() { 2948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, title, description 2949 , textEquivalent, code, documentation, condition, relatedAction, timing, participant 2950 , type, groupingBehavior, selectionBehavior, requiredBehavior, precheckBehavior, cardinalityBehavior 2951 , resource, action); 2952 } 2953 2954 public String fhirType() { 2955 return "RequestGroup.action"; 2956 2957 } 2958 2959 } 2960 2961 @Block() 2962 public static class RequestGroupActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 2963 /** 2964 * The kind of condition. 2965 */ 2966 @Child(name = "kind", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2967 @Description(shortDefinition="applicability | start | stop", formalDefinition="The kind of condition." ) 2968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-condition-kind") 2969 protected Enumeration<ActionConditionKind> kind; 2970 2971 /** 2972 * A brief, natural language description of the condition that effectively communicates the intended semantics. 2973 */ 2974 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2975 @Description(shortDefinition="Natural language description of the condition", formalDefinition="A brief, natural language description of the condition that effectively communicates the intended semantics." ) 2976 protected StringType description; 2977 2978 /** 2979 * The media type of the language for the expression. 2980 */ 2981 @Child(name = "language", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2982 @Description(shortDefinition="Language of the expression", formalDefinition="The media type of the language for the expression." ) 2983 protected StringType language; 2984 2985 /** 2986 * An expression that returns true or false, indicating whether or not the condition is satisfied. 2987 */ 2988 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2989 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether or not the condition is satisfied." ) 2990 protected StringType expression; 2991 2992 private static final long serialVersionUID = 944300105L; 2993 2994 /** 2995 * Constructor 2996 */ 2997 public RequestGroupActionConditionComponent() { 2998 super(); 2999 } 3000 3001 /** 3002 * Constructor 3003 */ 3004 public RequestGroupActionConditionComponent(Enumeration<ActionConditionKind> kind) { 3005 super(); 3006 this.kind = kind; 3007 } 3008 3009 /** 3010 * @return {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 3011 */ 3012 public Enumeration<ActionConditionKind> getKindElement() { 3013 if (this.kind == null) 3014 if (Configuration.errorOnAutoCreate()) 3015 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.kind"); 3016 else if (Configuration.doAutoCreate()) 3017 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 3018 return this.kind; 3019 } 3020 3021 public boolean hasKindElement() { 3022 return this.kind != null && !this.kind.isEmpty(); 3023 } 3024 3025 public boolean hasKind() { 3026 return this.kind != null && !this.kind.isEmpty(); 3027 } 3028 3029 /** 3030 * @param value {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 3031 */ 3032 public RequestGroupActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 3033 this.kind = value; 3034 return this; 3035 } 3036 3037 /** 3038 * @return The kind of condition. 3039 */ 3040 public ActionConditionKind getKind() { 3041 return this.kind == null ? null : this.kind.getValue(); 3042 } 3043 3044 /** 3045 * @param value The kind of condition. 3046 */ 3047 public RequestGroupActionConditionComponent setKind(ActionConditionKind value) { 3048 if (this.kind == null) 3049 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 3050 this.kind.setValue(value); 3051 return this; 3052 } 3053 3054 /** 3055 * @return {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3056 */ 3057 public StringType getDescriptionElement() { 3058 if (this.description == null) 3059 if (Configuration.errorOnAutoCreate()) 3060 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.description"); 3061 else if (Configuration.doAutoCreate()) 3062 this.description = new StringType(); // bb 3063 return this.description; 3064 } 3065 3066 public boolean hasDescriptionElement() { 3067 return this.description != null && !this.description.isEmpty(); 3068 } 3069 3070 public boolean hasDescription() { 3071 return this.description != null && !this.description.isEmpty(); 3072 } 3073 3074 /** 3075 * @param value {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3076 */ 3077 public RequestGroupActionConditionComponent setDescriptionElement(StringType value) { 3078 this.description = value; 3079 return this; 3080 } 3081 3082 /** 3083 * @return A brief, natural language description of the condition that effectively communicates the intended semantics. 3084 */ 3085 public String getDescription() { 3086 return this.description == null ? null : this.description.getValue(); 3087 } 3088 3089 /** 3090 * @param value A brief, natural language description of the condition that effectively communicates the intended semantics. 3091 */ 3092 public RequestGroupActionConditionComponent setDescription(String value) { 3093 if (Utilities.noString(value)) 3094 this.description = null; 3095 else { 3096 if (this.description == null) 3097 this.description = new StringType(); 3098 this.description.setValue(value); 3099 } 3100 return this; 3101 } 3102 3103 /** 3104 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3105 */ 3106 public StringType getLanguageElement() { 3107 if (this.language == null) 3108 if (Configuration.errorOnAutoCreate()) 3109 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.language"); 3110 else if (Configuration.doAutoCreate()) 3111 this.language = new StringType(); // bb 3112 return this.language; 3113 } 3114 3115 public boolean hasLanguageElement() { 3116 return this.language != null && !this.language.isEmpty(); 3117 } 3118 3119 public boolean hasLanguage() { 3120 return this.language != null && !this.language.isEmpty(); 3121 } 3122 3123 /** 3124 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3125 */ 3126 public RequestGroupActionConditionComponent setLanguageElement(StringType value) { 3127 this.language = value; 3128 return this; 3129 } 3130 3131 /** 3132 * @return The media type of the language for the expression. 3133 */ 3134 public String getLanguage() { 3135 return this.language == null ? null : this.language.getValue(); 3136 } 3137 3138 /** 3139 * @param value The media type of the language for the expression. 3140 */ 3141 public RequestGroupActionConditionComponent setLanguage(String value) { 3142 if (Utilities.noString(value)) 3143 this.language = null; 3144 else { 3145 if (this.language == null) 3146 this.language = new StringType(); 3147 this.language.setValue(value); 3148 } 3149 return this; 3150 } 3151 3152 /** 3153 * @return {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3154 */ 3155 public StringType getExpressionElement() { 3156 if (this.expression == null) 3157 if (Configuration.errorOnAutoCreate()) 3158 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.expression"); 3159 else if (Configuration.doAutoCreate()) 3160 this.expression = new StringType(); // bb 3161 return this.expression; 3162 } 3163 3164 public boolean hasExpressionElement() { 3165 return this.expression != null && !this.expression.isEmpty(); 3166 } 3167 3168 public boolean hasExpression() { 3169 return this.expression != null && !this.expression.isEmpty(); 3170 } 3171 3172 /** 3173 * @param value {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3174 */ 3175 public RequestGroupActionConditionComponent setExpressionElement(StringType value) { 3176 this.expression = value; 3177 return this; 3178 } 3179 3180 /** 3181 * @return An expression that returns true or false, indicating whether or not the condition is satisfied. 3182 */ 3183 public String getExpression() { 3184 return this.expression == null ? null : this.expression.getValue(); 3185 } 3186 3187 /** 3188 * @param value An expression that returns true or false, indicating whether or not the condition is satisfied. 3189 */ 3190 public RequestGroupActionConditionComponent setExpression(String value) { 3191 if (Utilities.noString(value)) 3192 this.expression = null; 3193 else { 3194 if (this.expression == null) 3195 this.expression = new StringType(); 3196 this.expression.setValue(value); 3197 } 3198 return this; 3199 } 3200 3201 protected void listChildren(List<Property> children) { 3202 super.listChildren(children); 3203 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 3204 children.add(new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description)); 3205 children.add(new Property("language", "string", "The media type of the language for the expression.", 0, 1, language)); 3206 children.add(new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression)); 3207 } 3208 3209 @Override 3210 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3211 switch (_hash) { 3212 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 3213 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description); 3214 case -1613589672: /*language*/ return new Property("language", "string", "The media type of the language for the expression.", 0, 1, language); 3215 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression); 3216 default: return super.getNamedProperty(_hash, _name, _checkValid); 3217 } 3218 3219 } 3220 3221 @Override 3222 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3223 switch (hash) { 3224 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActionConditionKind> 3225 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3226 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // StringType 3227 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 3228 default: return super.getProperty(hash, name, checkValid); 3229 } 3230 3231 } 3232 3233 @Override 3234 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3235 switch (hash) { 3236 case 3292052: // kind 3237 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3238 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3239 return value; 3240 case -1724546052: // description 3241 this.description = castToString(value); // StringType 3242 return value; 3243 case -1613589672: // language 3244 this.language = castToString(value); // StringType 3245 return value; 3246 case -1795452264: // expression 3247 this.expression = castToString(value); // StringType 3248 return value; 3249 default: return super.setProperty(hash, name, value); 3250 } 3251 3252 } 3253 3254 @Override 3255 public Base setProperty(String name, Base value) throws FHIRException { 3256 if (name.equals("kind")) { 3257 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3258 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3259 } else if (name.equals("description")) { 3260 this.description = castToString(value); // StringType 3261 } else if (name.equals("language")) { 3262 this.language = castToString(value); // StringType 3263 } else if (name.equals("expression")) { 3264 this.expression = castToString(value); // StringType 3265 } else 3266 return super.setProperty(name, value); 3267 return value; 3268 } 3269 3270 @Override 3271 public Base makeProperty(int hash, String name) throws FHIRException { 3272 switch (hash) { 3273 case 3292052: return getKindElement(); 3274 case -1724546052: return getDescriptionElement(); 3275 case -1613589672: return getLanguageElement(); 3276 case -1795452264: return getExpressionElement(); 3277 default: return super.makeProperty(hash, name); 3278 } 3279 3280 } 3281 3282 @Override 3283 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3284 switch (hash) { 3285 case 3292052: /*kind*/ return new String[] {"code"}; 3286 case -1724546052: /*description*/ return new String[] {"string"}; 3287 case -1613589672: /*language*/ return new String[] {"string"}; 3288 case -1795452264: /*expression*/ return new String[] {"string"}; 3289 default: return super.getTypesForProperty(hash, name); 3290 } 3291 3292 } 3293 3294 @Override 3295 public Base addChild(String name) throws FHIRException { 3296 if (name.equals("kind")) { 3297 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.kind"); 3298 } 3299 else if (name.equals("description")) { 3300 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 3301 } 3302 else if (name.equals("language")) { 3303 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.language"); 3304 } 3305 else if (name.equals("expression")) { 3306 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.expression"); 3307 } 3308 else 3309 return super.addChild(name); 3310 } 3311 3312 public RequestGroupActionConditionComponent copy() { 3313 RequestGroupActionConditionComponent dst = new RequestGroupActionConditionComponent(); 3314 copyValues(dst); 3315 dst.kind = kind == null ? null : kind.copy(); 3316 dst.description = description == null ? null : description.copy(); 3317 dst.language = language == null ? null : language.copy(); 3318 dst.expression = expression == null ? null : expression.copy(); 3319 return dst; 3320 } 3321 3322 @Override 3323 public boolean equalsDeep(Base other_) { 3324 if (!super.equalsDeep(other_)) 3325 return false; 3326 if (!(other_ instanceof RequestGroupActionConditionComponent)) 3327 return false; 3328 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 3329 return compareDeep(kind, o.kind, true) && compareDeep(description, o.description, true) && compareDeep(language, o.language, true) 3330 && compareDeep(expression, o.expression, true); 3331 } 3332 3333 @Override 3334 public boolean equalsShallow(Base other_) { 3335 if (!super.equalsShallow(other_)) 3336 return false; 3337 if (!(other_ instanceof RequestGroupActionConditionComponent)) 3338 return false; 3339 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 3340 return compareValues(kind, o.kind, true) && compareValues(description, o.description, true) && compareValues(language, o.language, true) 3341 && compareValues(expression, o.expression, true); 3342 } 3343 3344 public boolean isEmpty() { 3345 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, description, language 3346 , expression); 3347 } 3348 3349 public String fhirType() { 3350 return "RequestGroup.action.condition"; 3351 3352 } 3353 3354 } 3355 3356 @Block() 3357 public static class RequestGroupActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 3358 /** 3359 * The element id of the action this is related to. 3360 */ 3361 @Child(name = "actionId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3362 @Description(shortDefinition="What action this is related to", formalDefinition="The element id of the action this is related to." ) 3363 protected IdType actionId; 3364 3365 /** 3366 * The relationship of this action to the related action. 3367 */ 3368 @Child(name = "relationship", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 3369 @Description(shortDefinition="before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition="The relationship of this action to the related action." ) 3370 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 3371 protected Enumeration<ActionRelationshipType> relationship; 3372 3373 /** 3374 * A duration or range of durations to apply to the relationship. For example, 30-60 minutes before. 3375 */ 3376 @Child(name = "offset", type = {Duration.class, Range.class}, order=3, min=0, max=1, modifier=false, summary=false) 3377 @Description(shortDefinition="Time offset for the relationship", formalDefinition="A duration or range of durations to apply to the relationship. For example, 30-60 minutes before." ) 3378 protected Type offset; 3379 3380 private static final long serialVersionUID = 1063306770L; 3381 3382 /** 3383 * Constructor 3384 */ 3385 public RequestGroupActionRelatedActionComponent() { 3386 super(); 3387 } 3388 3389 /** 3390 * Constructor 3391 */ 3392 public RequestGroupActionRelatedActionComponent(IdType actionId, Enumeration<ActionRelationshipType> relationship) { 3393 super(); 3394 this.actionId = actionId; 3395 this.relationship = relationship; 3396 } 3397 3398 /** 3399 * @return {@link #actionId} (The element id of the action this is related to.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 3400 */ 3401 public IdType getActionIdElement() { 3402 if (this.actionId == null) 3403 if (Configuration.errorOnAutoCreate()) 3404 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.actionId"); 3405 else if (Configuration.doAutoCreate()) 3406 this.actionId = new IdType(); // bb 3407 return this.actionId; 3408 } 3409 3410 public boolean hasActionIdElement() { 3411 return this.actionId != null && !this.actionId.isEmpty(); 3412 } 3413 3414 public boolean hasActionId() { 3415 return this.actionId != null && !this.actionId.isEmpty(); 3416 } 3417 3418 /** 3419 * @param value {@link #actionId} (The element id of the action this is related to.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 3420 */ 3421 public RequestGroupActionRelatedActionComponent setActionIdElement(IdType value) { 3422 this.actionId = value; 3423 return this; 3424 } 3425 3426 /** 3427 * @return The element id of the action this is related to. 3428 */ 3429 public String getActionId() { 3430 return this.actionId == null ? null : this.actionId.getValue(); 3431 } 3432 3433 /** 3434 * @param value The element id of the action this is related to. 3435 */ 3436 public RequestGroupActionRelatedActionComponent setActionId(String value) { 3437 if (this.actionId == null) 3438 this.actionId = new IdType(); 3439 this.actionId.setValue(value); 3440 return this; 3441 } 3442 3443 /** 3444 * @return {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3445 */ 3446 public Enumeration<ActionRelationshipType> getRelationshipElement() { 3447 if (this.relationship == null) 3448 if (Configuration.errorOnAutoCreate()) 3449 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.relationship"); 3450 else if (Configuration.doAutoCreate()) 3451 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 3452 return this.relationship; 3453 } 3454 3455 public boolean hasRelationshipElement() { 3456 return this.relationship != null && !this.relationship.isEmpty(); 3457 } 3458 3459 public boolean hasRelationship() { 3460 return this.relationship != null && !this.relationship.isEmpty(); 3461 } 3462 3463 /** 3464 * @param value {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3465 */ 3466 public RequestGroupActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 3467 this.relationship = value; 3468 return this; 3469 } 3470 3471 /** 3472 * @return The relationship of this action to the related action. 3473 */ 3474 public ActionRelationshipType getRelationship() { 3475 return this.relationship == null ? null : this.relationship.getValue(); 3476 } 3477 3478 /** 3479 * @param value The relationship of this action to the related action. 3480 */ 3481 public RequestGroupActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 3482 if (this.relationship == null) 3483 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 3484 this.relationship.setValue(value); 3485 return this; 3486 } 3487 3488 /** 3489 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3490 */ 3491 public Type getOffset() { 3492 return this.offset; 3493 } 3494 3495 /** 3496 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3497 */ 3498 public Duration getOffsetDuration() throws FHIRException { 3499 if (this.offset == null) 3500 return null; 3501 if (!(this.offset instanceof Duration)) 3502 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.offset.getClass().getName()+" was encountered"); 3503 return (Duration) this.offset; 3504 } 3505 3506 public boolean hasOffsetDuration() { 3507 return this.offset instanceof Duration; 3508 } 3509 3510 /** 3511 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3512 */ 3513 public Range getOffsetRange() throws FHIRException { 3514 if (this.offset == null) 3515 return null; 3516 if (!(this.offset instanceof Range)) 3517 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.offset.getClass().getName()+" was encountered"); 3518 return (Range) this.offset; 3519 } 3520 3521 public boolean hasOffsetRange() { 3522 return this.offset instanceof Range; 3523 } 3524 3525 public boolean hasOffset() { 3526 return this.offset != null && !this.offset.isEmpty(); 3527 } 3528 3529 /** 3530 * @param value {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3531 */ 3532 public RequestGroupActionRelatedActionComponent setOffset(Type value) throws FHIRFormatError { 3533 if (value != null && !(value instanceof Duration || value instanceof Range)) 3534 throw new FHIRFormatError("Not the right type for RequestGroup.action.relatedAction.offset[x]: "+value.fhirType()); 3535 this.offset = value; 3536 return this; 3537 } 3538 3539 protected void listChildren(List<Property> children) { 3540 super.listChildren(children); 3541 children.add(new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId)); 3542 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship)); 3543 children.add(new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset)); 3544 } 3545 3546 @Override 3547 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3548 switch (_hash) { 3549 case -1656172047: /*actionId*/ return new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId); 3550 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship); 3551 case -1960684787: /*offset[x]*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3552 case -1019779949: /*offset*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3553 case 134075207: /*offsetDuration*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3554 case 1263585386: /*offsetRange*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3555 default: return super.getNamedProperty(_hash, _name, _checkValid); 3556 } 3557 3558 } 3559 3560 @Override 3561 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3562 switch (hash) { 3563 case -1656172047: /*actionId*/ return this.actionId == null ? new Base[0] : new Base[] {this.actionId}; // IdType 3564 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ActionRelationshipType> 3565 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // Type 3566 default: return super.getProperty(hash, name, checkValid); 3567 } 3568 3569 } 3570 3571 @Override 3572 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3573 switch (hash) { 3574 case -1656172047: // actionId 3575 this.actionId = castToId(value); // IdType 3576 return value; 3577 case -261851592: // relationship 3578 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 3579 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3580 return value; 3581 case -1019779949: // offset 3582 this.offset = castToType(value); // Type 3583 return value; 3584 default: return super.setProperty(hash, name, value); 3585 } 3586 3587 } 3588 3589 @Override 3590 public Base setProperty(String name, Base value) throws FHIRException { 3591 if (name.equals("actionId")) { 3592 this.actionId = castToId(value); // IdType 3593 } else if (name.equals("relationship")) { 3594 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 3595 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3596 } else if (name.equals("offset[x]")) { 3597 this.offset = castToType(value); // Type 3598 } else 3599 return super.setProperty(name, value); 3600 return value; 3601 } 3602 3603 @Override 3604 public Base makeProperty(int hash, String name) throws FHIRException { 3605 switch (hash) { 3606 case -1656172047: return getActionIdElement(); 3607 case -261851592: return getRelationshipElement(); 3608 case -1960684787: return getOffset(); 3609 case -1019779949: return getOffset(); 3610 default: return super.makeProperty(hash, name); 3611 } 3612 3613 } 3614 3615 @Override 3616 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3617 switch (hash) { 3618 case -1656172047: /*actionId*/ return new String[] {"id"}; 3619 case -261851592: /*relationship*/ return new String[] {"code"}; 3620 case -1019779949: /*offset*/ return new String[] {"Duration", "Range"}; 3621 default: return super.getTypesForProperty(hash, name); 3622 } 3623 3624 } 3625 3626 @Override 3627 public Base addChild(String name) throws FHIRException { 3628 if (name.equals("actionId")) { 3629 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.actionId"); 3630 } 3631 else if (name.equals("relationship")) { 3632 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.relationship"); 3633 } 3634 else if (name.equals("offsetDuration")) { 3635 this.offset = new Duration(); 3636 return this.offset; 3637 } 3638 else if (name.equals("offsetRange")) { 3639 this.offset = new Range(); 3640 return this.offset; 3641 } 3642 else 3643 return super.addChild(name); 3644 } 3645 3646 public RequestGroupActionRelatedActionComponent copy() { 3647 RequestGroupActionRelatedActionComponent dst = new RequestGroupActionRelatedActionComponent(); 3648 copyValues(dst); 3649 dst.actionId = actionId == null ? null : actionId.copy(); 3650 dst.relationship = relationship == null ? null : relationship.copy(); 3651 dst.offset = offset == null ? null : offset.copy(); 3652 return dst; 3653 } 3654 3655 @Override 3656 public boolean equalsDeep(Base other_) { 3657 if (!super.equalsDeep(other_)) 3658 return false; 3659 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 3660 return false; 3661 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 3662 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 3663 && compareDeep(offset, o.offset, true); 3664 } 3665 3666 @Override 3667 public boolean equalsShallow(Base other_) { 3668 if (!super.equalsShallow(other_)) 3669 return false; 3670 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 3671 return false; 3672 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 3673 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true) 3674 ; 3675 } 3676 3677 public boolean isEmpty() { 3678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset 3679 ); 3680 } 3681 3682 public String fhirType() { 3683 return "RequestGroup.action.relatedAction"; 3684 3685 } 3686 3687 } 3688 3689 /** 3690 * Allows a service to provide a unique, business identifier for the request. 3691 */ 3692 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3693 @Description(shortDefinition="Business identifier", formalDefinition="Allows a service to provide a unique, business identifier for the request." ) 3694 protected List<Identifier> identifier; 3695 3696 /** 3697 * A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request. 3698 */ 3699 @Child(name = "definition", type = {Reference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3700 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request." ) 3701 protected List<Reference> definition; 3702 /** 3703 * The actual objects that are the target of the reference (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 3704 */ 3705 protected List<Resource> definitionTarget; 3706 3707 3708 /** 3709 * A plan, proposal or order that is fulfilled in whole or in part by this request. 3710 */ 3711 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3712 @Description(shortDefinition="Fulfills plan, proposal, or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this request." ) 3713 protected List<Reference> basedOn; 3714 /** 3715 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this request.) 3716 */ 3717 protected List<Resource> basedOnTarget; 3718 3719 3720 /** 3721 * Completed or terminated request(s) whose function is taken by this new request. 3722 */ 3723 @Child(name = "replaces", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3724 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 3725 protected List<Reference> replaces; 3726 /** 3727 * The actual objects that are the target of the reference (Completed or terminated request(s) whose function is taken by this new request.) 3728 */ 3729 protected List<Resource> replacesTarget; 3730 3731 3732 /** 3733 * A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form. 3734 */ 3735 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 3736 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form." ) 3737 protected Identifier groupIdentifier; 3738 3739 /** 3740 * The current state of the request. For request groups, the status reflects the status of all the requests in the group. 3741 */ 3742 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 3743 @Description(shortDefinition="draft | active | suspended | cancelled | completed | entered-in-error | unknown", formalDefinition="The current state of the request. For request groups, the status reflects the status of all the requests in the group." ) 3744 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 3745 protected Enumeration<RequestStatus> status; 3746 3747 /** 3748 * Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 3749 */ 3750 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3751 @Description(shortDefinition="proposal | plan | order", formalDefinition="Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain." ) 3752 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 3753 protected Enumeration<RequestIntent> intent; 3754 3755 /** 3756 * Indicates how quickly the request should be addressed with respect to other requests. 3757 */ 3758 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3759 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the request should be addressed with respect to other requests." ) 3760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 3761 protected Enumeration<RequestPriority> priority; 3762 3763 /** 3764 * The subject for which the request group was created. 3765 */ 3766 @Child(name = "subject", type = {Patient.class, Group.class}, order=8, min=0, max=1, modifier=false, summary=false) 3767 @Description(shortDefinition="Who the request group is about", formalDefinition="The subject for which the request group was created." ) 3768 protected Reference subject; 3769 3770 /** 3771 * The actual object that is the target of the reference (The subject for which the request group was created.) 3772 */ 3773 protected Resource subjectTarget; 3774 3775 /** 3776 * Describes the context of the request group, if any. 3777 */ 3778 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=9, min=0, max=1, modifier=false, summary=false) 3779 @Description(shortDefinition="Encounter or Episode for the request group", formalDefinition="Describes the context of the request group, if any." ) 3780 protected Reference context; 3781 3782 /** 3783 * The actual object that is the target of the reference (Describes the context of the request group, if any.) 3784 */ 3785 protected Resource contextTarget; 3786 3787 /** 3788 * Indicates when the request group was created. 3789 */ 3790 @Child(name = "authoredOn", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 3791 @Description(shortDefinition="When the request group was authored", formalDefinition="Indicates when the request group was created." ) 3792 protected DateTimeType authoredOn; 3793 3794 /** 3795 * Provides a reference to the author of the request group. 3796 */ 3797 @Child(name = "author", type = {Device.class, Practitioner.class}, order=11, min=0, max=1, modifier=false, summary=false) 3798 @Description(shortDefinition="Device or practitioner that authored the request group", formalDefinition="Provides a reference to the author of the request group." ) 3799 protected Reference author; 3800 3801 /** 3802 * The actual object that is the target of the reference (Provides a reference to the author of the request group.) 3803 */ 3804 protected Resource authorTarget; 3805 3806 /** 3807 * Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response. 3808 */ 3809 @Child(name = "reason", type = {CodeableConcept.class, Reference.class}, order=12, min=0, max=1, modifier=false, summary=false) 3810 @Description(shortDefinition="Reason for the request group", formalDefinition="Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response." ) 3811 protected Type reason; 3812 3813 /** 3814 * Provides a mechanism to communicate additional information about the response. 3815 */ 3816 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3817 @Description(shortDefinition="Additional notes about the response", formalDefinition="Provides a mechanism to communicate additional information about the response." ) 3818 protected List<Annotation> note; 3819 3820 /** 3821 * The actions, if any, produced by the evaluation of the artifact. 3822 */ 3823 @Child(name = "action", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3824 @Description(shortDefinition="Proposed actions, if any", formalDefinition="The actions, if any, produced by the evaluation of the artifact." ) 3825 protected List<RequestGroupActionComponent> action; 3826 3827 private static final long serialVersionUID = -1812083587L; 3828 3829 /** 3830 * Constructor 3831 */ 3832 public RequestGroup() { 3833 super(); 3834 } 3835 3836 /** 3837 * Constructor 3838 */ 3839 public RequestGroup(Enumeration<RequestStatus> status, Enumeration<RequestIntent> intent) { 3840 super(); 3841 this.status = status; 3842 this.intent = intent; 3843 } 3844 3845 /** 3846 * @return {@link #identifier} (Allows a service to provide a unique, business identifier for the request.) 3847 */ 3848 public List<Identifier> getIdentifier() { 3849 if (this.identifier == null) 3850 this.identifier = new ArrayList<Identifier>(); 3851 return this.identifier; 3852 } 3853 3854 /** 3855 * @return Returns a reference to <code>this</code> for easy method chaining 3856 */ 3857 public RequestGroup setIdentifier(List<Identifier> theIdentifier) { 3858 this.identifier = theIdentifier; 3859 return this; 3860 } 3861 3862 public boolean hasIdentifier() { 3863 if (this.identifier == null) 3864 return false; 3865 for (Identifier item : this.identifier) 3866 if (!item.isEmpty()) 3867 return true; 3868 return false; 3869 } 3870 3871 public Identifier addIdentifier() { //3 3872 Identifier t = new Identifier(); 3873 if (this.identifier == null) 3874 this.identifier = new ArrayList<Identifier>(); 3875 this.identifier.add(t); 3876 return t; 3877 } 3878 3879 public RequestGroup addIdentifier(Identifier t) { //3 3880 if (t == null) 3881 return this; 3882 if (this.identifier == null) 3883 this.identifier = new ArrayList<Identifier>(); 3884 this.identifier.add(t); 3885 return this; 3886 } 3887 3888 /** 3889 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3890 */ 3891 public Identifier getIdentifierFirstRep() { 3892 if (getIdentifier().isEmpty()) { 3893 addIdentifier(); 3894 } 3895 return getIdentifier().get(0); 3896 } 3897 3898 /** 3899 * @return {@link #definition} (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 3900 */ 3901 public List<Reference> getDefinition() { 3902 if (this.definition == null) 3903 this.definition = new ArrayList<Reference>(); 3904 return this.definition; 3905 } 3906 3907 /** 3908 * @return Returns a reference to <code>this</code> for easy method chaining 3909 */ 3910 public RequestGroup setDefinition(List<Reference> theDefinition) { 3911 this.definition = theDefinition; 3912 return this; 3913 } 3914 3915 public boolean hasDefinition() { 3916 if (this.definition == null) 3917 return false; 3918 for (Reference item : this.definition) 3919 if (!item.isEmpty()) 3920 return true; 3921 return false; 3922 } 3923 3924 public Reference addDefinition() { //3 3925 Reference t = new Reference(); 3926 if (this.definition == null) 3927 this.definition = new ArrayList<Reference>(); 3928 this.definition.add(t); 3929 return t; 3930 } 3931 3932 public RequestGroup addDefinition(Reference t) { //3 3933 if (t == null) 3934 return this; 3935 if (this.definition == null) 3936 this.definition = new ArrayList<Reference>(); 3937 this.definition.add(t); 3938 return this; 3939 } 3940 3941 /** 3942 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 3943 */ 3944 public Reference getDefinitionFirstRep() { 3945 if (getDefinition().isEmpty()) { 3946 addDefinition(); 3947 } 3948 return getDefinition().get(0); 3949 } 3950 3951 /** 3952 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this request.) 3953 */ 3954 public List<Reference> getBasedOn() { 3955 if (this.basedOn == null) 3956 this.basedOn = new ArrayList<Reference>(); 3957 return this.basedOn; 3958 } 3959 3960 /** 3961 * @return Returns a reference to <code>this</code> for easy method chaining 3962 */ 3963 public RequestGroup setBasedOn(List<Reference> theBasedOn) { 3964 this.basedOn = theBasedOn; 3965 return this; 3966 } 3967 3968 public boolean hasBasedOn() { 3969 if (this.basedOn == null) 3970 return false; 3971 for (Reference item : this.basedOn) 3972 if (!item.isEmpty()) 3973 return true; 3974 return false; 3975 } 3976 3977 public Reference addBasedOn() { //3 3978 Reference t = new Reference(); 3979 if (this.basedOn == null) 3980 this.basedOn = new ArrayList<Reference>(); 3981 this.basedOn.add(t); 3982 return t; 3983 } 3984 3985 public RequestGroup addBasedOn(Reference t) { //3 3986 if (t == null) 3987 return this; 3988 if (this.basedOn == null) 3989 this.basedOn = new ArrayList<Reference>(); 3990 this.basedOn.add(t); 3991 return this; 3992 } 3993 3994 /** 3995 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 3996 */ 3997 public Reference getBasedOnFirstRep() { 3998 if (getBasedOn().isEmpty()) { 3999 addBasedOn(); 4000 } 4001 return getBasedOn().get(0); 4002 } 4003 4004 /** 4005 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 4006 */ 4007 public List<Reference> getReplaces() { 4008 if (this.replaces == null) 4009 this.replaces = new ArrayList<Reference>(); 4010 return this.replaces; 4011 } 4012 4013 /** 4014 * @return Returns a reference to <code>this</code> for easy method chaining 4015 */ 4016 public RequestGroup setReplaces(List<Reference> theReplaces) { 4017 this.replaces = theReplaces; 4018 return this; 4019 } 4020 4021 public boolean hasReplaces() { 4022 if (this.replaces == null) 4023 return false; 4024 for (Reference item : this.replaces) 4025 if (!item.isEmpty()) 4026 return true; 4027 return false; 4028 } 4029 4030 public Reference addReplaces() { //3 4031 Reference t = new Reference(); 4032 if (this.replaces == null) 4033 this.replaces = new ArrayList<Reference>(); 4034 this.replaces.add(t); 4035 return t; 4036 } 4037 4038 public RequestGroup addReplaces(Reference t) { //3 4039 if (t == null) 4040 return this; 4041 if (this.replaces == null) 4042 this.replaces = new ArrayList<Reference>(); 4043 this.replaces.add(t); 4044 return this; 4045 } 4046 4047 /** 4048 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 4049 */ 4050 public Reference getReplacesFirstRep() { 4051 if (getReplaces().isEmpty()) { 4052 addReplaces(); 4053 } 4054 return getReplaces().get(0); 4055 } 4056 4057 /** 4058 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 4059 */ 4060 public Identifier getGroupIdentifier() { 4061 if (this.groupIdentifier == null) 4062 if (Configuration.errorOnAutoCreate()) 4063 throw new Error("Attempt to auto-create RequestGroup.groupIdentifier"); 4064 else if (Configuration.doAutoCreate()) 4065 this.groupIdentifier = new Identifier(); // cc 4066 return this.groupIdentifier; 4067 } 4068 4069 public boolean hasGroupIdentifier() { 4070 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4071 } 4072 4073 /** 4074 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 4075 */ 4076 public RequestGroup setGroupIdentifier(Identifier value) { 4077 this.groupIdentifier = value; 4078 return this; 4079 } 4080 4081 /** 4082 * @return {@link #status} (The current state of the request. For request groups, the status reflects the status of all the requests in the group.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4083 */ 4084 public Enumeration<RequestStatus> getStatusElement() { 4085 if (this.status == null) 4086 if (Configuration.errorOnAutoCreate()) 4087 throw new Error("Attempt to auto-create RequestGroup.status"); 4088 else if (Configuration.doAutoCreate()) 4089 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 4090 return this.status; 4091 } 4092 4093 public boolean hasStatusElement() { 4094 return this.status != null && !this.status.isEmpty(); 4095 } 4096 4097 public boolean hasStatus() { 4098 return this.status != null && !this.status.isEmpty(); 4099 } 4100 4101 /** 4102 * @param value {@link #status} (The current state of the request. For request groups, the status reflects the status of all the requests in the group.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4103 */ 4104 public RequestGroup setStatusElement(Enumeration<RequestStatus> value) { 4105 this.status = value; 4106 return this; 4107 } 4108 4109 /** 4110 * @return The current state of the request. For request groups, the status reflects the status of all the requests in the group. 4111 */ 4112 public RequestStatus getStatus() { 4113 return this.status == null ? null : this.status.getValue(); 4114 } 4115 4116 /** 4117 * @param value The current state of the request. For request groups, the status reflects the status of all the requests in the group. 4118 */ 4119 public RequestGroup setStatus(RequestStatus value) { 4120 if (this.status == null) 4121 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 4122 this.status.setValue(value); 4123 return this; 4124 } 4125 4126 /** 4127 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4128 */ 4129 public Enumeration<RequestIntent> getIntentElement() { 4130 if (this.intent == null) 4131 if (Configuration.errorOnAutoCreate()) 4132 throw new Error("Attempt to auto-create RequestGroup.intent"); 4133 else if (Configuration.doAutoCreate()) 4134 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 4135 return this.intent; 4136 } 4137 4138 public boolean hasIntentElement() { 4139 return this.intent != null && !this.intent.isEmpty(); 4140 } 4141 4142 public boolean hasIntent() { 4143 return this.intent != null && !this.intent.isEmpty(); 4144 } 4145 4146 /** 4147 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4148 */ 4149 public RequestGroup setIntentElement(Enumeration<RequestIntent> value) { 4150 this.intent = value; 4151 return this; 4152 } 4153 4154 /** 4155 * @return Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4156 */ 4157 public RequestIntent getIntent() { 4158 return this.intent == null ? null : this.intent.getValue(); 4159 } 4160 4161 /** 4162 * @param value Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4163 */ 4164 public RequestGroup setIntent(RequestIntent value) { 4165 if (this.intent == null) 4166 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 4167 this.intent.setValue(value); 4168 return this; 4169 } 4170 4171 /** 4172 * @return {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4173 */ 4174 public Enumeration<RequestPriority> getPriorityElement() { 4175 if (this.priority == null) 4176 if (Configuration.errorOnAutoCreate()) 4177 throw new Error("Attempt to auto-create RequestGroup.priority"); 4178 else if (Configuration.doAutoCreate()) 4179 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 4180 return this.priority; 4181 } 4182 4183 public boolean hasPriorityElement() { 4184 return this.priority != null && !this.priority.isEmpty(); 4185 } 4186 4187 public boolean hasPriority() { 4188 return this.priority != null && !this.priority.isEmpty(); 4189 } 4190 4191 /** 4192 * @param value {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4193 */ 4194 public RequestGroup setPriorityElement(Enumeration<RequestPriority> value) { 4195 this.priority = value; 4196 return this; 4197 } 4198 4199 /** 4200 * @return Indicates how quickly the request should be addressed with respect to other requests. 4201 */ 4202 public RequestPriority getPriority() { 4203 return this.priority == null ? null : this.priority.getValue(); 4204 } 4205 4206 /** 4207 * @param value Indicates how quickly the request should be addressed with respect to other requests. 4208 */ 4209 public RequestGroup setPriority(RequestPriority value) { 4210 if (value == null) 4211 this.priority = null; 4212 else { 4213 if (this.priority == null) 4214 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4215 this.priority.setValue(value); 4216 } 4217 return this; 4218 } 4219 4220 /** 4221 * @return {@link #subject} (The subject for which the request group was created.) 4222 */ 4223 public Reference getSubject() { 4224 if (this.subject == null) 4225 if (Configuration.errorOnAutoCreate()) 4226 throw new Error("Attempt to auto-create RequestGroup.subject"); 4227 else if (Configuration.doAutoCreate()) 4228 this.subject = new Reference(); // cc 4229 return this.subject; 4230 } 4231 4232 public boolean hasSubject() { 4233 return this.subject != null && !this.subject.isEmpty(); 4234 } 4235 4236 /** 4237 * @param value {@link #subject} (The subject for which the request group was created.) 4238 */ 4239 public RequestGroup setSubject(Reference value) { 4240 this.subject = value; 4241 return this; 4242 } 4243 4244 /** 4245 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The subject for which the request group was created.) 4246 */ 4247 public Resource getSubjectTarget() { 4248 return this.subjectTarget; 4249 } 4250 4251 /** 4252 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The subject for which the request group was created.) 4253 */ 4254 public RequestGroup setSubjectTarget(Resource value) { 4255 this.subjectTarget = value; 4256 return this; 4257 } 4258 4259 /** 4260 * @return {@link #context} (Describes the context of the request group, if any.) 4261 */ 4262 public Reference getContext() { 4263 if (this.context == null) 4264 if (Configuration.errorOnAutoCreate()) 4265 throw new Error("Attempt to auto-create RequestGroup.context"); 4266 else if (Configuration.doAutoCreate()) 4267 this.context = new Reference(); // cc 4268 return this.context; 4269 } 4270 4271 public boolean hasContext() { 4272 return this.context != null && !this.context.isEmpty(); 4273 } 4274 4275 /** 4276 * @param value {@link #context} (Describes the context of the request group, if any.) 4277 */ 4278 public RequestGroup setContext(Reference value) { 4279 this.context = value; 4280 return this; 4281 } 4282 4283 /** 4284 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the context of the request group, if any.) 4285 */ 4286 public Resource getContextTarget() { 4287 return this.contextTarget; 4288 } 4289 4290 /** 4291 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the context of the request group, if any.) 4292 */ 4293 public RequestGroup setContextTarget(Resource value) { 4294 this.contextTarget = value; 4295 return this; 4296 } 4297 4298 /** 4299 * @return {@link #authoredOn} (Indicates when the request group was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4300 */ 4301 public DateTimeType getAuthoredOnElement() { 4302 if (this.authoredOn == null) 4303 if (Configuration.errorOnAutoCreate()) 4304 throw new Error("Attempt to auto-create RequestGroup.authoredOn"); 4305 else if (Configuration.doAutoCreate()) 4306 this.authoredOn = new DateTimeType(); // bb 4307 return this.authoredOn; 4308 } 4309 4310 public boolean hasAuthoredOnElement() { 4311 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4312 } 4313 4314 public boolean hasAuthoredOn() { 4315 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4316 } 4317 4318 /** 4319 * @param value {@link #authoredOn} (Indicates when the request group was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4320 */ 4321 public RequestGroup setAuthoredOnElement(DateTimeType value) { 4322 this.authoredOn = value; 4323 return this; 4324 } 4325 4326 /** 4327 * @return Indicates when the request group was created. 4328 */ 4329 public Date getAuthoredOn() { 4330 return this.authoredOn == null ? null : this.authoredOn.getValue(); 4331 } 4332 4333 /** 4334 * @param value Indicates when the request group was created. 4335 */ 4336 public RequestGroup setAuthoredOn(Date value) { 4337 if (value == null) 4338 this.authoredOn = null; 4339 else { 4340 if (this.authoredOn == null) 4341 this.authoredOn = new DateTimeType(); 4342 this.authoredOn.setValue(value); 4343 } 4344 return this; 4345 } 4346 4347 /** 4348 * @return {@link #author} (Provides a reference to the author of the request group.) 4349 */ 4350 public Reference getAuthor() { 4351 if (this.author == null) 4352 if (Configuration.errorOnAutoCreate()) 4353 throw new Error("Attempt to auto-create RequestGroup.author"); 4354 else if (Configuration.doAutoCreate()) 4355 this.author = new Reference(); // cc 4356 return this.author; 4357 } 4358 4359 public boolean hasAuthor() { 4360 return this.author != null && !this.author.isEmpty(); 4361 } 4362 4363 /** 4364 * @param value {@link #author} (Provides a reference to the author of the request group.) 4365 */ 4366 public RequestGroup setAuthor(Reference value) { 4367 this.author = value; 4368 return this; 4369 } 4370 4371 /** 4372 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Provides a reference to the author of the request group.) 4373 */ 4374 public Resource getAuthorTarget() { 4375 return this.authorTarget; 4376 } 4377 4378 /** 4379 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Provides a reference to the author of the request group.) 4380 */ 4381 public RequestGroup setAuthorTarget(Resource value) { 4382 this.authorTarget = value; 4383 return this; 4384 } 4385 4386 /** 4387 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4388 */ 4389 public Type getReason() { 4390 return this.reason; 4391 } 4392 4393 /** 4394 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4395 */ 4396 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 4397 if (this.reason == null) 4398 return null; 4399 if (!(this.reason instanceof CodeableConcept)) 4400 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 4401 return (CodeableConcept) this.reason; 4402 } 4403 4404 public boolean hasReasonCodeableConcept() { 4405 return this.reason instanceof CodeableConcept; 4406 } 4407 4408 /** 4409 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4410 */ 4411 public Reference getReasonReference() throws FHIRException { 4412 if (this.reason == null) 4413 return null; 4414 if (!(this.reason instanceof Reference)) 4415 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 4416 return (Reference) this.reason; 4417 } 4418 4419 public boolean hasReasonReference() { 4420 return this.reason instanceof Reference; 4421 } 4422 4423 public boolean hasReason() { 4424 return this.reason != null && !this.reason.isEmpty(); 4425 } 4426 4427 /** 4428 * @param value {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4429 */ 4430 public RequestGroup setReason(Type value) throws FHIRFormatError { 4431 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 4432 throw new FHIRFormatError("Not the right type for RequestGroup.reason[x]: "+value.fhirType()); 4433 this.reason = value; 4434 return this; 4435 } 4436 4437 /** 4438 * @return {@link #note} (Provides a mechanism to communicate additional information about the response.) 4439 */ 4440 public List<Annotation> getNote() { 4441 if (this.note == null) 4442 this.note = new ArrayList<Annotation>(); 4443 return this.note; 4444 } 4445 4446 /** 4447 * @return Returns a reference to <code>this</code> for easy method chaining 4448 */ 4449 public RequestGroup setNote(List<Annotation> theNote) { 4450 this.note = theNote; 4451 return this; 4452 } 4453 4454 public boolean hasNote() { 4455 if (this.note == null) 4456 return false; 4457 for (Annotation item : this.note) 4458 if (!item.isEmpty()) 4459 return true; 4460 return false; 4461 } 4462 4463 public Annotation addNote() { //3 4464 Annotation t = new Annotation(); 4465 if (this.note == null) 4466 this.note = new ArrayList<Annotation>(); 4467 this.note.add(t); 4468 return t; 4469 } 4470 4471 public RequestGroup addNote(Annotation t) { //3 4472 if (t == null) 4473 return this; 4474 if (this.note == null) 4475 this.note = new ArrayList<Annotation>(); 4476 this.note.add(t); 4477 return this; 4478 } 4479 4480 /** 4481 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 4482 */ 4483 public Annotation getNoteFirstRep() { 4484 if (getNote().isEmpty()) { 4485 addNote(); 4486 } 4487 return getNote().get(0); 4488 } 4489 4490 /** 4491 * @return {@link #action} (The actions, if any, produced by the evaluation of the artifact.) 4492 */ 4493 public List<RequestGroupActionComponent> getAction() { 4494 if (this.action == null) 4495 this.action = new ArrayList<RequestGroupActionComponent>(); 4496 return this.action; 4497 } 4498 4499 /** 4500 * @return Returns a reference to <code>this</code> for easy method chaining 4501 */ 4502 public RequestGroup setAction(List<RequestGroupActionComponent> theAction) { 4503 this.action = theAction; 4504 return this; 4505 } 4506 4507 public boolean hasAction() { 4508 if (this.action == null) 4509 return false; 4510 for (RequestGroupActionComponent item : this.action) 4511 if (!item.isEmpty()) 4512 return true; 4513 return false; 4514 } 4515 4516 public RequestGroupActionComponent addAction() { //3 4517 RequestGroupActionComponent t = new RequestGroupActionComponent(); 4518 if (this.action == null) 4519 this.action = new ArrayList<RequestGroupActionComponent>(); 4520 this.action.add(t); 4521 return t; 4522 } 4523 4524 public RequestGroup addAction(RequestGroupActionComponent t) { //3 4525 if (t == null) 4526 return this; 4527 if (this.action == null) 4528 this.action = new ArrayList<RequestGroupActionComponent>(); 4529 this.action.add(t); 4530 return this; 4531 } 4532 4533 /** 4534 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 4535 */ 4536 public RequestGroupActionComponent getActionFirstRep() { 4537 if (getAction().isEmpty()) { 4538 addAction(); 4539 } 4540 return getAction().get(0); 4541 } 4542 4543 protected void listChildren(List<Property> children) { 4544 super.listChildren(children); 4545 children.add(new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4546 children.add(new Property("definition", "Reference(Any)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 4547 children.add(new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 4548 children.add(new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 4549 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier)); 4550 children.add(new Property("status", "code", "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 0, 1, status)); 4551 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent)); 4552 children.add(new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 4553 children.add(new Property("subject", "Reference(Patient|Group)", "The subject for which the request group was created.", 0, 1, subject)); 4554 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Describes the context of the request group, if any.", 0, 1, context)); 4555 children.add(new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn)); 4556 children.add(new Property("author", "Reference(Device|Practitioner)", "Provides a reference to the author of the request group.", 0, 1, author)); 4557 children.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason)); 4558 children.add(new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note)); 4559 children.add(new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action)); 4560 } 4561 4562 @Override 4563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4564 switch (_hash) { 4565 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier); 4566 case -1014418093: /*definition*/ return new Property("definition", "Reference(Any)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 4567 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 4568 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 4569 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier); 4570 case -892481550: /*status*/ return new Property("status", "code", "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 0, 1, status); 4571 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent); 4572 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 4573 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The subject for which the request group was created.", 0, 1, subject); 4574 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Describes the context of the request group, if any.", 0, 1, context); 4575 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn); 4576 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner)", "Provides a reference to the author of the request group.", 0, 1, author); 4577 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4578 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4579 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4580 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4581 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note); 4582 case -1422950858: /*action*/ return new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action); 4583 default: return super.getNamedProperty(_hash, _name, _checkValid); 4584 } 4585 4586 } 4587 4588 @Override 4589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4590 switch (hash) { 4591 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4592 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 4593 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4594 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 4595 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 4596 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 4597 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 4598 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 4599 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 4600 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 4601 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 4602 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 4603 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 4604 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4605 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 4606 default: return super.getProperty(hash, name, checkValid); 4607 } 4608 4609 } 4610 4611 @Override 4612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4613 switch (hash) { 4614 case -1618432855: // identifier 4615 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4616 return value; 4617 case -1014418093: // definition 4618 this.getDefinition().add(castToReference(value)); // Reference 4619 return value; 4620 case -332612366: // basedOn 4621 this.getBasedOn().add(castToReference(value)); // Reference 4622 return value; 4623 case -430332865: // replaces 4624 this.getReplaces().add(castToReference(value)); // Reference 4625 return value; 4626 case -445338488: // groupIdentifier 4627 this.groupIdentifier = castToIdentifier(value); // Identifier 4628 return value; 4629 case -892481550: // status 4630 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 4631 this.status = (Enumeration) value; // Enumeration<RequestStatus> 4632 return value; 4633 case -1183762788: // intent 4634 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 4635 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4636 return value; 4637 case -1165461084: // priority 4638 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4639 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4640 return value; 4641 case -1867885268: // subject 4642 this.subject = castToReference(value); // Reference 4643 return value; 4644 case 951530927: // context 4645 this.context = castToReference(value); // Reference 4646 return value; 4647 case -1500852503: // authoredOn 4648 this.authoredOn = castToDateTime(value); // DateTimeType 4649 return value; 4650 case -1406328437: // author 4651 this.author = castToReference(value); // Reference 4652 return value; 4653 case -934964668: // reason 4654 this.reason = castToType(value); // Type 4655 return value; 4656 case 3387378: // note 4657 this.getNote().add(castToAnnotation(value)); // Annotation 4658 return value; 4659 case -1422950858: // action 4660 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 4661 return value; 4662 default: return super.setProperty(hash, name, value); 4663 } 4664 4665 } 4666 4667 @Override 4668 public Base setProperty(String name, Base value) throws FHIRException { 4669 if (name.equals("identifier")) { 4670 this.getIdentifier().add(castToIdentifier(value)); 4671 } else if (name.equals("definition")) { 4672 this.getDefinition().add(castToReference(value)); 4673 } else if (name.equals("basedOn")) { 4674 this.getBasedOn().add(castToReference(value)); 4675 } else if (name.equals("replaces")) { 4676 this.getReplaces().add(castToReference(value)); 4677 } else if (name.equals("groupIdentifier")) { 4678 this.groupIdentifier = castToIdentifier(value); // Identifier 4679 } else if (name.equals("status")) { 4680 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 4681 this.status = (Enumeration) value; // Enumeration<RequestStatus> 4682 } else if (name.equals("intent")) { 4683 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 4684 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4685 } else if (name.equals("priority")) { 4686 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4687 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4688 } else if (name.equals("subject")) { 4689 this.subject = castToReference(value); // Reference 4690 } else if (name.equals("context")) { 4691 this.context = castToReference(value); // Reference 4692 } else if (name.equals("authoredOn")) { 4693 this.authoredOn = castToDateTime(value); // DateTimeType 4694 } else if (name.equals("author")) { 4695 this.author = castToReference(value); // Reference 4696 } else if (name.equals("reason[x]")) { 4697 this.reason = castToType(value); // Type 4698 } else if (name.equals("note")) { 4699 this.getNote().add(castToAnnotation(value)); 4700 } else if (name.equals("action")) { 4701 this.getAction().add((RequestGroupActionComponent) value); 4702 } else 4703 return super.setProperty(name, value); 4704 return value; 4705 } 4706 4707 @Override 4708 public Base makeProperty(int hash, String name) throws FHIRException { 4709 switch (hash) { 4710 case -1618432855: return addIdentifier(); 4711 case -1014418093: return addDefinition(); 4712 case -332612366: return addBasedOn(); 4713 case -430332865: return addReplaces(); 4714 case -445338488: return getGroupIdentifier(); 4715 case -892481550: return getStatusElement(); 4716 case -1183762788: return getIntentElement(); 4717 case -1165461084: return getPriorityElement(); 4718 case -1867885268: return getSubject(); 4719 case 951530927: return getContext(); 4720 case -1500852503: return getAuthoredOnElement(); 4721 case -1406328437: return getAuthor(); 4722 case -669418564: return getReason(); 4723 case -934964668: return getReason(); 4724 case 3387378: return addNote(); 4725 case -1422950858: return addAction(); 4726 default: return super.makeProperty(hash, name); 4727 } 4728 4729 } 4730 4731 @Override 4732 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4733 switch (hash) { 4734 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4735 case -1014418093: /*definition*/ return new String[] {"Reference"}; 4736 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 4737 case -430332865: /*replaces*/ return new String[] {"Reference"}; 4738 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 4739 case -892481550: /*status*/ return new String[] {"code"}; 4740 case -1183762788: /*intent*/ return new String[] {"code"}; 4741 case -1165461084: /*priority*/ return new String[] {"code"}; 4742 case -1867885268: /*subject*/ return new String[] {"Reference"}; 4743 case 951530927: /*context*/ return new String[] {"Reference"}; 4744 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 4745 case -1406328437: /*author*/ return new String[] {"Reference"}; 4746 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 4747 case 3387378: /*note*/ return new String[] {"Annotation"}; 4748 case -1422950858: /*action*/ return new String[] {}; 4749 default: return super.getTypesForProperty(hash, name); 4750 } 4751 4752 } 4753 4754 @Override 4755 public Base addChild(String name) throws FHIRException { 4756 if (name.equals("identifier")) { 4757 return addIdentifier(); 4758 } 4759 else if (name.equals("definition")) { 4760 return addDefinition(); 4761 } 4762 else if (name.equals("basedOn")) { 4763 return addBasedOn(); 4764 } 4765 else if (name.equals("replaces")) { 4766 return addReplaces(); 4767 } 4768 else if (name.equals("groupIdentifier")) { 4769 this.groupIdentifier = new Identifier(); 4770 return this.groupIdentifier; 4771 } 4772 else if (name.equals("status")) { 4773 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.status"); 4774 } 4775 else if (name.equals("intent")) { 4776 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.intent"); 4777 } 4778 else if (name.equals("priority")) { 4779 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 4780 } 4781 else if (name.equals("subject")) { 4782 this.subject = new Reference(); 4783 return this.subject; 4784 } 4785 else if (name.equals("context")) { 4786 this.context = new Reference(); 4787 return this.context; 4788 } 4789 else if (name.equals("authoredOn")) { 4790 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.authoredOn"); 4791 } 4792 else if (name.equals("author")) { 4793 this.author = new Reference(); 4794 return this.author; 4795 } 4796 else if (name.equals("reasonCodeableConcept")) { 4797 this.reason = new CodeableConcept(); 4798 return this.reason; 4799 } 4800 else if (name.equals("reasonReference")) { 4801 this.reason = new Reference(); 4802 return this.reason; 4803 } 4804 else if (name.equals("note")) { 4805 return addNote(); 4806 } 4807 else if (name.equals("action")) { 4808 return addAction(); 4809 } 4810 else 4811 return super.addChild(name); 4812 } 4813 4814 public String fhirType() { 4815 return "RequestGroup"; 4816 4817 } 4818 4819 public RequestGroup copy() { 4820 RequestGroup dst = new RequestGroup(); 4821 copyValues(dst); 4822 if (identifier != null) { 4823 dst.identifier = new ArrayList<Identifier>(); 4824 for (Identifier i : identifier) 4825 dst.identifier.add(i.copy()); 4826 }; 4827 if (definition != null) { 4828 dst.definition = new ArrayList<Reference>(); 4829 for (Reference i : definition) 4830 dst.definition.add(i.copy()); 4831 }; 4832 if (basedOn != null) { 4833 dst.basedOn = new ArrayList<Reference>(); 4834 for (Reference i : basedOn) 4835 dst.basedOn.add(i.copy()); 4836 }; 4837 if (replaces != null) { 4838 dst.replaces = new ArrayList<Reference>(); 4839 for (Reference i : replaces) 4840 dst.replaces.add(i.copy()); 4841 }; 4842 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 4843 dst.status = status == null ? null : status.copy(); 4844 dst.intent = intent == null ? null : intent.copy(); 4845 dst.priority = priority == null ? null : priority.copy(); 4846 dst.subject = subject == null ? null : subject.copy(); 4847 dst.context = context == null ? null : context.copy(); 4848 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4849 dst.author = author == null ? null : author.copy(); 4850 dst.reason = reason == null ? null : reason.copy(); 4851 if (note != null) { 4852 dst.note = new ArrayList<Annotation>(); 4853 for (Annotation i : note) 4854 dst.note.add(i.copy()); 4855 }; 4856 if (action != null) { 4857 dst.action = new ArrayList<RequestGroupActionComponent>(); 4858 for (RequestGroupActionComponent i : action) 4859 dst.action.add(i.copy()); 4860 }; 4861 return dst; 4862 } 4863 4864 protected RequestGroup typedCopy() { 4865 return copy(); 4866 } 4867 4868 @Override 4869 public boolean equalsDeep(Base other_) { 4870 if (!super.equalsDeep(other_)) 4871 return false; 4872 if (!(other_ instanceof RequestGroup)) 4873 return false; 4874 RequestGroup o = (RequestGroup) other_; 4875 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 4876 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 4877 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 4878 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(authoredOn, o.authoredOn, true) 4879 && compareDeep(author, o.author, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 4880 && compareDeep(action, o.action, true); 4881 } 4882 4883 @Override 4884 public boolean equalsShallow(Base other_) { 4885 if (!super.equalsShallow(other_)) 4886 return false; 4887 if (!(other_ instanceof RequestGroup)) 4888 return false; 4889 RequestGroup o = (RequestGroup) other_; 4890 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 4891 && compareValues(authoredOn, o.authoredOn, true); 4892 } 4893 4894 public boolean isEmpty() { 4895 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 4896 , replaces, groupIdentifier, status, intent, priority, subject, context, authoredOn 4897 , author, reason, note, action); 4898 } 4899 4900 @Override 4901 public ResourceType getResourceType() { 4902 return ResourceType.RequestGroup; 4903 } 4904 4905 /** 4906 * Search parameter: <b>authored</b> 4907 * <p> 4908 * Description: <b>The date the request group was authored</b><br> 4909 * Type: <b>date</b><br> 4910 * Path: <b>RequestGroup.authoredOn</b><br> 4911 * </p> 4912 */ 4913 @SearchParamDefinition(name="authored", path="RequestGroup.authoredOn", description="The date the request group was authored", type="date" ) 4914 public static final String SP_AUTHORED = "authored"; 4915 /** 4916 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 4917 * <p> 4918 * Description: <b>The date the request group was authored</b><br> 4919 * Type: <b>date</b><br> 4920 * Path: <b>RequestGroup.authoredOn</b><br> 4921 * </p> 4922 */ 4923 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 4924 4925 /** 4926 * Search parameter: <b>identifier</b> 4927 * <p> 4928 * Description: <b>External identifiers for the request group</b><br> 4929 * Type: <b>token</b><br> 4930 * Path: <b>RequestGroup.identifier</b><br> 4931 * </p> 4932 */ 4933 @SearchParamDefinition(name="identifier", path="RequestGroup.identifier", description="External identifiers for the request group", type="token" ) 4934 public static final String SP_IDENTIFIER = "identifier"; 4935 /** 4936 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4937 * <p> 4938 * Description: <b>External identifiers for the request group</b><br> 4939 * Type: <b>token</b><br> 4940 * Path: <b>RequestGroup.identifier</b><br> 4941 * </p> 4942 */ 4943 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4944 4945 /** 4946 * Search parameter: <b>subject</b> 4947 * <p> 4948 * Description: <b>The subject that the request group is about</b><br> 4949 * Type: <b>reference</b><br> 4950 * Path: <b>RequestGroup.subject</b><br> 4951 * </p> 4952 */ 4953 @SearchParamDefinition(name="subject", path="RequestGroup.subject", description="The subject that the request group is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 4954 public static final String SP_SUBJECT = "subject"; 4955 /** 4956 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4957 * <p> 4958 * Description: <b>The subject that the request group is about</b><br> 4959 * Type: <b>reference</b><br> 4960 * Path: <b>RequestGroup.subject</b><br> 4961 * </p> 4962 */ 4963 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4964 4965/** 4966 * Constant for fluent queries to be used to add include statements. Specifies 4967 * the path value of "<b>RequestGroup:subject</b>". 4968 */ 4969 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RequestGroup:subject").toLocked(); 4970 4971 /** 4972 * Search parameter: <b>author</b> 4973 * <p> 4974 * Description: <b>The author of the request group</b><br> 4975 * Type: <b>reference</b><br> 4976 * Path: <b>RequestGroup.author</b><br> 4977 * </p> 4978 */ 4979 @SearchParamDefinition(name="author", path="RequestGroup.author", description="The author of the request group", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Practitioner.class } ) 4980 public static final String SP_AUTHOR = "author"; 4981 /** 4982 * <b>Fluent Client</b> search parameter constant for <b>author</b> 4983 * <p> 4984 * Description: <b>The author of the request group</b><br> 4985 * Type: <b>reference</b><br> 4986 * Path: <b>RequestGroup.author</b><br> 4987 * </p> 4988 */ 4989 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 4990 4991/** 4992 * Constant for fluent queries to be used to add include statements. Specifies 4993 * the path value of "<b>RequestGroup:author</b>". 4994 */ 4995 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("RequestGroup:author").toLocked(); 4996 4997 /** 4998 * Search parameter: <b>encounter</b> 4999 * <p> 5000 * Description: <b>The encounter the request group applies to</b><br> 5001 * Type: <b>reference</b><br> 5002 * Path: <b>RequestGroup.context</b><br> 5003 * </p> 5004 */ 5005 @SearchParamDefinition(name="encounter", path="RequestGroup.context", description="The encounter the request group applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 5006 public static final String SP_ENCOUNTER = "encounter"; 5007 /** 5008 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5009 * <p> 5010 * Description: <b>The encounter the request group applies to</b><br> 5011 * Type: <b>reference</b><br> 5012 * Path: <b>RequestGroup.context</b><br> 5013 * </p> 5014 */ 5015 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 5016 5017/** 5018 * Constant for fluent queries to be used to add include statements. Specifies 5019 * the path value of "<b>RequestGroup:encounter</b>". 5020 */ 5021 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("RequestGroup:encounter").toLocked(); 5022 5023 /** 5024 * Search parameter: <b>priority</b> 5025 * <p> 5026 * Description: <b>The priority of the request group</b><br> 5027 * Type: <b>token</b><br> 5028 * Path: <b>RequestGroup.priority</b><br> 5029 * </p> 5030 */ 5031 @SearchParamDefinition(name="priority", path="RequestGroup.priority", description="The priority of the request group", type="token" ) 5032 public static final String SP_PRIORITY = "priority"; 5033 /** 5034 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5035 * <p> 5036 * Description: <b>The priority of the request group</b><br> 5037 * Type: <b>token</b><br> 5038 * Path: <b>RequestGroup.priority</b><br> 5039 * </p> 5040 */ 5041 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 5042 5043 /** 5044 * Search parameter: <b>intent</b> 5045 * <p> 5046 * Description: <b>The intent of the request group</b><br> 5047 * Type: <b>token</b><br> 5048 * Path: <b>RequestGroup.intent</b><br> 5049 * </p> 5050 */ 5051 @SearchParamDefinition(name="intent", path="RequestGroup.intent", description="The intent of the request group", type="token" ) 5052 public static final String SP_INTENT = "intent"; 5053 /** 5054 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5055 * <p> 5056 * Description: <b>The intent of the request group</b><br> 5057 * Type: <b>token</b><br> 5058 * Path: <b>RequestGroup.intent</b><br> 5059 * </p> 5060 */ 5061 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 5062 5063 /** 5064 * Search parameter: <b>participant</b> 5065 * <p> 5066 * Description: <b>The participant in the requests in the group</b><br> 5067 * Type: <b>reference</b><br> 5068 * Path: <b>RequestGroup.action.participant</b><br> 5069 * </p> 5070 */ 5071 @SearchParamDefinition(name="participant", path="RequestGroup.action.participant", description="The participant in the requests in the group", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Person.class, Practitioner.class, RelatedPerson.class } ) 5072 public static final String SP_PARTICIPANT = "participant"; 5073 /** 5074 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 5075 * <p> 5076 * Description: <b>The participant in the requests in the group</b><br> 5077 * Type: <b>reference</b><br> 5078 * Path: <b>RequestGroup.action.participant</b><br> 5079 * </p> 5080 */ 5081 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 5082 5083/** 5084 * Constant for fluent queries to be used to add include statements. Specifies 5085 * the path value of "<b>RequestGroup:participant</b>". 5086 */ 5087 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("RequestGroup:participant").toLocked(); 5088 5089 /** 5090 * Search parameter: <b>group-identifier</b> 5091 * <p> 5092 * Description: <b>The group identifier for the request group</b><br> 5093 * Type: <b>token</b><br> 5094 * Path: <b>RequestGroup.groupIdentifier</b><br> 5095 * </p> 5096 */ 5097 @SearchParamDefinition(name="group-identifier", path="RequestGroup.groupIdentifier", description="The group identifier for the request group", type="token" ) 5098 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5099 /** 5100 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5101 * <p> 5102 * Description: <b>The group identifier for the request group</b><br> 5103 * Type: <b>token</b><br> 5104 * Path: <b>RequestGroup.groupIdentifier</b><br> 5105 * </p> 5106 */ 5107 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 5108 5109 /** 5110 * Search parameter: <b>patient</b> 5111 * <p> 5112 * Description: <b>The identity of a patient to search for request groups</b><br> 5113 * Type: <b>reference</b><br> 5114 * Path: <b>RequestGroup.subject</b><br> 5115 * </p> 5116 */ 5117 @SearchParamDefinition(name="patient", path="RequestGroup.subject", description="The identity of a patient to search for request groups", type="reference", target={Patient.class } ) 5118 public static final String SP_PATIENT = "patient"; 5119 /** 5120 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5121 * <p> 5122 * Description: <b>The identity of a patient to search for request groups</b><br> 5123 * Type: <b>reference</b><br> 5124 * Path: <b>RequestGroup.subject</b><br> 5125 * </p> 5126 */ 5127 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5128 5129/** 5130 * Constant for fluent queries to be used to add include statements. Specifies 5131 * the path value of "<b>RequestGroup:patient</b>". 5132 */ 5133 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RequestGroup:patient").toLocked(); 5134 5135 /** 5136 * Search parameter: <b>context</b> 5137 * <p> 5138 * Description: <b>The context the request group applies to</b><br> 5139 * Type: <b>reference</b><br> 5140 * Path: <b>RequestGroup.context</b><br> 5141 * </p> 5142 */ 5143 @SearchParamDefinition(name="context", path="RequestGroup.context", description="The context the request group applies to", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 5144 public static final String SP_CONTEXT = "context"; 5145 /** 5146 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5147 * <p> 5148 * Description: <b>The context the request group applies to</b><br> 5149 * Type: <b>reference</b><br> 5150 * Path: <b>RequestGroup.context</b><br> 5151 * </p> 5152 */ 5153 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 5154 5155/** 5156 * Constant for fluent queries to be used to add include statements. Specifies 5157 * the path value of "<b>RequestGroup:context</b>". 5158 */ 5159 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("RequestGroup:context").toLocked(); 5160 5161 /** 5162 * Search parameter: <b>definition</b> 5163 * <p> 5164 * Description: <b>The definition from which the request group is realized</b><br> 5165 * Type: <b>reference</b><br> 5166 * Path: <b>RequestGroup.definition</b><br> 5167 * </p> 5168 */ 5169 @SearchParamDefinition(name="definition", path="RequestGroup.definition", description="The definition from which the request group is realized", type="reference" ) 5170 public static final String SP_DEFINITION = "definition"; 5171 /** 5172 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 5173 * <p> 5174 * Description: <b>The definition from which the request group is realized</b><br> 5175 * Type: <b>reference</b><br> 5176 * Path: <b>RequestGroup.definition</b><br> 5177 * </p> 5178 */ 5179 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 5180 5181/** 5182 * Constant for fluent queries to be used to add include statements. Specifies 5183 * the path value of "<b>RequestGroup:definition</b>". 5184 */ 5185 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("RequestGroup:definition").toLocked(); 5186 5187 /** 5188 * Search parameter: <b>status</b> 5189 * <p> 5190 * Description: <b>The status of the request group</b><br> 5191 * Type: <b>token</b><br> 5192 * Path: <b>RequestGroup.status</b><br> 5193 * </p> 5194 */ 5195 @SearchParamDefinition(name="status", path="RequestGroup.status", description="The status of the request group", type="token" ) 5196 public static final String SP_STATUS = "status"; 5197 /** 5198 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5199 * <p> 5200 * Description: <b>The status of the request group</b><br> 5201 * Type: <b>token</b><br> 5202 * Path: <b>RequestGroup.status</b><br> 5203 * </p> 5204 */ 5205 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5206 5207 5208}