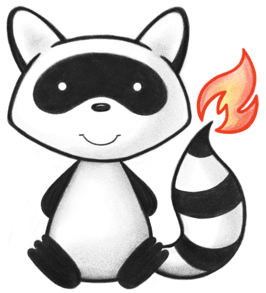
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 052 */ 053@ResourceDef(name="RequestGroup", profile="http://hl7.org/fhir/Profile/RequestGroup") 054public class RequestGroup extends DomainResource { 055 056 public enum RequestStatus { 057 /** 058 * The request has been created but is not yet complete or ready for action 059 */ 060 DRAFT, 061 /** 062 * The request is ready to be acted upon 063 */ 064 ACTIVE, 065 /** 066 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 067 */ 068 SUSPENDED, 069 /** 070 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 071 */ 072 CANCELLED, 073 /** 074 * Activity against the request has been sufficiently completed to the satisfaction of the requester 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 079 */ 080 ENTEREDINERROR, 081 /** 082 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static RequestStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("draft".equals(codeString)) 093 return DRAFT; 094 if ("active".equals(codeString)) 095 return ACTIVE; 096 if ("suspended".equals(codeString)) 097 return SUSPENDED; 098 if ("cancelled".equals(codeString)) 099 return CANCELLED; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case DRAFT: return "draft"; 114 case ACTIVE: return "active"; 115 case SUSPENDED: return "suspended"; 116 case CANCELLED: return "cancelled"; 117 case COMPLETED: return "completed"; 118 case ENTEREDINERROR: return "entered-in-error"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case DRAFT: return "http://hl7.org/fhir/request-status"; 127 case ACTIVE: return "http://hl7.org/fhir/request-status"; 128 case SUSPENDED: return "http://hl7.org/fhir/request-status"; 129 case CANCELLED: return "http://hl7.org/fhir/request-status"; 130 case COMPLETED: return "http://hl7.org/fhir/request-status"; 131 case ENTEREDINERROR: return "http://hl7.org/fhir/request-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/request-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 140 case ACTIVE: return "The request is ready to be acted upon"; 141 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 142 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 143 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 144 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 145 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" . One of the listed statuses is presumed to apply, but the system creating the request doesn't know."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case DRAFT: return "Draft"; 153 case ACTIVE: return "Active"; 154 case SUSPENDED: return "Suspended"; 155 case CANCELLED: return "Cancelled"; 156 case COMPLETED: return "Completed"; 157 case ENTEREDINERROR: return "Entered in Error"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 166 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("draft".equals(codeString)) 171 return RequestStatus.DRAFT; 172 if ("active".equals(codeString)) 173 return RequestStatus.ACTIVE; 174 if ("suspended".equals(codeString)) 175 return RequestStatus.SUSPENDED; 176 if ("cancelled".equals(codeString)) 177 return RequestStatus.CANCELLED; 178 if ("completed".equals(codeString)) 179 return RequestStatus.COMPLETED; 180 if ("entered-in-error".equals(codeString)) 181 return RequestStatus.ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return RequestStatus.UNKNOWN; 184 throw new IllegalArgumentException("Unknown RequestStatus code '"+codeString+"'"); 185 } 186 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<RequestStatus>(this); 191 String codeString = code.asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("draft".equals(codeString)) 195 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT); 196 if ("active".equals(codeString)) 197 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE); 198 if ("suspended".equals(codeString)) 199 return new Enumeration<RequestStatus>(this, RequestStatus.SUSPENDED); 200 if ("cancelled".equals(codeString)) 201 return new Enumeration<RequestStatus>(this, RequestStatus.CANCELLED); 202 if ("completed".equals(codeString)) 203 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN); 208 throw new FHIRException("Unknown RequestStatus code '"+codeString+"'"); 209 } 210 public String toCode(RequestStatus code) { 211 if (code == RequestStatus.NULL) 212 return null; 213 if (code == RequestStatus.DRAFT) 214 return "draft"; 215 if (code == RequestStatus.ACTIVE) 216 return "active"; 217 if (code == RequestStatus.SUSPENDED) 218 return "suspended"; 219 if (code == RequestStatus.CANCELLED) 220 return "cancelled"; 221 if (code == RequestStatus.COMPLETED) 222 return "completed"; 223 if (code == RequestStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == RequestStatus.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(RequestStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 public enum RequestIntent { 235 /** 236 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 237 */ 238 PROPOSAL, 239 /** 240 * The request represents an intension to ensure something occurs without providing an authorization for others to act 241 */ 242 PLAN, 243 /** 244 * The request represents a request/demand and authorization for action 245 */ 246 ORDER, 247 /** 248 * The request represents an original authorization for action 249 */ 250 ORIGINALORDER, 251 /** 252 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 253 */ 254 REFLEXORDER, 255 /** 256 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 257 */ 258 FILLERORDER, 259 /** 260 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 261 */ 262 INSTANCEORDER, 263 /** 264 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 265 266Refer to [[[RequestGroup]]] for additional information on how this status is used 267 */ 268 OPTION, 269 /** 270 * added to help the parsers with the generic types 271 */ 272 NULL; 273 public static RequestIntent fromCode(String codeString) throws FHIRException { 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("proposal".equals(codeString)) 277 return PROPOSAL; 278 if ("plan".equals(codeString)) 279 return PLAN; 280 if ("order".equals(codeString)) 281 return ORDER; 282 if ("original-order".equals(codeString)) 283 return ORIGINALORDER; 284 if ("reflex-order".equals(codeString)) 285 return REFLEXORDER; 286 if ("filler-order".equals(codeString)) 287 return FILLERORDER; 288 if ("instance-order".equals(codeString)) 289 return INSTANCEORDER; 290 if ("option".equals(codeString)) 291 return OPTION; 292 if (Configuration.isAcceptInvalidEnums()) 293 return null; 294 else 295 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 296 } 297 public String toCode() { 298 switch (this) { 299 case PROPOSAL: return "proposal"; 300 case PLAN: return "plan"; 301 case ORDER: return "order"; 302 case ORIGINALORDER: return "original-order"; 303 case REFLEXORDER: return "reflex-order"; 304 case FILLERORDER: return "filler-order"; 305 case INSTANCEORDER: return "instance-order"; 306 case OPTION: return "option"; 307 case NULL: return null; 308 default: return "?"; 309 } 310 } 311 public String getSystem() { 312 switch (this) { 313 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 314 case PLAN: return "http://hl7.org/fhir/request-intent"; 315 case ORDER: return "http://hl7.org/fhir/request-intent"; 316 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 317 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 318 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 319 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 320 case OPTION: return "http://hl7.org/fhir/request-intent"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 public String getDefinition() { 326 switch (this) { 327 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 328 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 329 case ORDER: return "The request represents a request/demand and authorization for action"; 330 case ORIGINALORDER: return "The request represents an original authorization for action"; 331 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 332 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 333 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 334 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getDisplay() { 340 switch (this) { 341 case PROPOSAL: return "Proposal"; 342 case PLAN: return "Plan"; 343 case ORDER: return "Order"; 344 case ORIGINALORDER: return "Original Order"; 345 case REFLEXORDER: return "Reflex Order"; 346 case FILLERORDER: return "Filler Order"; 347 case INSTANCEORDER: return "Instance Order"; 348 case OPTION: return "Option"; 349 case NULL: return null; 350 default: return "?"; 351 } 352 } 353 } 354 355 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 356 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 357 if (codeString == null || "".equals(codeString)) 358 if (codeString == null || "".equals(codeString)) 359 return null; 360 if ("proposal".equals(codeString)) 361 return RequestIntent.PROPOSAL; 362 if ("plan".equals(codeString)) 363 return RequestIntent.PLAN; 364 if ("order".equals(codeString)) 365 return RequestIntent.ORDER; 366 if ("original-order".equals(codeString)) 367 return RequestIntent.ORIGINALORDER; 368 if ("reflex-order".equals(codeString)) 369 return RequestIntent.REFLEXORDER; 370 if ("filler-order".equals(codeString)) 371 return RequestIntent.FILLERORDER; 372 if ("instance-order".equals(codeString)) 373 return RequestIntent.INSTANCEORDER; 374 if ("option".equals(codeString)) 375 return RequestIntent.OPTION; 376 throw new IllegalArgumentException("Unknown RequestIntent code '"+codeString+"'"); 377 } 378 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 379 if (code == null) 380 return null; 381 if (code.isEmpty()) 382 return new Enumeration<RequestIntent>(this); 383 String codeString = code.asStringValue(); 384 if (codeString == null || "".equals(codeString)) 385 return null; 386 if ("proposal".equals(codeString)) 387 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL); 388 if ("plan".equals(codeString)) 389 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN); 390 if ("order".equals(codeString)) 391 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER); 392 if ("original-order".equals(codeString)) 393 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER); 394 if ("reflex-order".equals(codeString)) 395 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER); 396 if ("filler-order".equals(codeString)) 397 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER); 398 if ("instance-order".equals(codeString)) 399 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER); 400 if ("option".equals(codeString)) 401 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION); 402 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 403 } 404 public String toCode(RequestIntent code) { 405 if (code == RequestIntent.NULL) 406 return null; 407 if (code == RequestIntent.PROPOSAL) 408 return "proposal"; 409 if (code == RequestIntent.PLAN) 410 return "plan"; 411 if (code == RequestIntent.ORDER) 412 return "order"; 413 if (code == RequestIntent.ORIGINALORDER) 414 return "original-order"; 415 if (code == RequestIntent.REFLEXORDER) 416 return "reflex-order"; 417 if (code == RequestIntent.FILLERORDER) 418 return "filler-order"; 419 if (code == RequestIntent.INSTANCEORDER) 420 return "instance-order"; 421 if (code == RequestIntent.OPTION) 422 return "option"; 423 return "?"; 424 } 425 public String toSystem(RequestIntent code) { 426 return code.getSystem(); 427 } 428 } 429 430 public enum RequestPriority { 431 /** 432 * The request has normal priority 433 */ 434 ROUTINE, 435 /** 436 * The request should be actioned promptly - higher priority than routine 437 */ 438 URGENT, 439 /** 440 * The request should be actioned as soon as possible - higher priority than urgent 441 */ 442 ASAP, 443 /** 444 * The request should be actioned immediately - highest possible priority. E.g. an emergency 445 */ 446 STAT, 447 /** 448 * added to help the parsers with the generic types 449 */ 450 NULL; 451 public static RequestPriority fromCode(String codeString) throws FHIRException { 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("routine".equals(codeString)) 455 return ROUTINE; 456 if ("urgent".equals(codeString)) 457 return URGENT; 458 if ("asap".equals(codeString)) 459 return ASAP; 460 if ("stat".equals(codeString)) 461 return STAT; 462 if (Configuration.isAcceptInvalidEnums()) 463 return null; 464 else 465 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 466 } 467 public String toCode() { 468 switch (this) { 469 case ROUTINE: return "routine"; 470 case URGENT: return "urgent"; 471 case ASAP: return "asap"; 472 case STAT: return "stat"; 473 case NULL: return null; 474 default: return "?"; 475 } 476 } 477 public String getSystem() { 478 switch (this) { 479 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 480 case URGENT: return "http://hl7.org/fhir/request-priority"; 481 case ASAP: return "http://hl7.org/fhir/request-priority"; 482 case STAT: return "http://hl7.org/fhir/request-priority"; 483 case NULL: return null; 484 default: return "?"; 485 } 486 } 487 public String getDefinition() { 488 switch (this) { 489 case ROUTINE: return "The request has normal priority"; 490 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 491 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 492 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 493 case NULL: return null; 494 default: return "?"; 495 } 496 } 497 public String getDisplay() { 498 switch (this) { 499 case ROUTINE: return "Routine"; 500 case URGENT: return "Urgent"; 501 case ASAP: return "ASAP"; 502 case STAT: return "STAT"; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 } 508 509 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 510 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 511 if (codeString == null || "".equals(codeString)) 512 if (codeString == null || "".equals(codeString)) 513 return null; 514 if ("routine".equals(codeString)) 515 return RequestPriority.ROUTINE; 516 if ("urgent".equals(codeString)) 517 return RequestPriority.URGENT; 518 if ("asap".equals(codeString)) 519 return RequestPriority.ASAP; 520 if ("stat".equals(codeString)) 521 return RequestPriority.STAT; 522 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 523 } 524 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 525 if (code == null) 526 return null; 527 if (code.isEmpty()) 528 return new Enumeration<RequestPriority>(this); 529 String codeString = code.asStringValue(); 530 if (codeString == null || "".equals(codeString)) 531 return null; 532 if ("routine".equals(codeString)) 533 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE); 534 if ("urgent".equals(codeString)) 535 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT); 536 if ("asap".equals(codeString)) 537 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP); 538 if ("stat".equals(codeString)) 539 return new Enumeration<RequestPriority>(this, RequestPriority.STAT); 540 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 541 } 542 public String toCode(RequestPriority code) { 543 if (code == RequestPriority.NULL) 544 return null; 545 if (code == RequestPriority.ROUTINE) 546 return "routine"; 547 if (code == RequestPriority.URGENT) 548 return "urgent"; 549 if (code == RequestPriority.ASAP) 550 return "asap"; 551 if (code == RequestPriority.STAT) 552 return "stat"; 553 return "?"; 554 } 555 public String toSystem(RequestPriority code) { 556 return code.getSystem(); 557 } 558 } 559 560 public enum ActionConditionKind { 561 /** 562 * The condition describes whether or not a given action is applicable 563 */ 564 APPLICABILITY, 565 /** 566 * The condition is a starting condition for the action 567 */ 568 START, 569 /** 570 * The condition is a stop, or exit condition for the action 571 */ 572 STOP, 573 /** 574 * added to help the parsers with the generic types 575 */ 576 NULL; 577 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 578 if (codeString == null || "".equals(codeString)) 579 return null; 580 if ("applicability".equals(codeString)) 581 return APPLICABILITY; 582 if ("start".equals(codeString)) 583 return START; 584 if ("stop".equals(codeString)) 585 return STOP; 586 if (Configuration.isAcceptInvalidEnums()) 587 return null; 588 else 589 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 590 } 591 public String toCode() { 592 switch (this) { 593 case APPLICABILITY: return "applicability"; 594 case START: return "start"; 595 case STOP: return "stop"; 596 case NULL: return null; 597 default: return "?"; 598 } 599 } 600 public String getSystem() { 601 switch (this) { 602 case APPLICABILITY: return "http://hl7.org/fhir/action-condition-kind"; 603 case START: return "http://hl7.org/fhir/action-condition-kind"; 604 case STOP: return "http://hl7.org/fhir/action-condition-kind"; 605 case NULL: return null; 606 default: return "?"; 607 } 608 } 609 public String getDefinition() { 610 switch (this) { 611 case APPLICABILITY: return "The condition describes whether or not a given action is applicable"; 612 case START: return "The condition is a starting condition for the action"; 613 case STOP: return "The condition is a stop, or exit condition for the action"; 614 case NULL: return null; 615 default: return "?"; 616 } 617 } 618 public String getDisplay() { 619 switch (this) { 620 case APPLICABILITY: return "Applicability"; 621 case START: return "Start"; 622 case STOP: return "Stop"; 623 case NULL: return null; 624 default: return "?"; 625 } 626 } 627 } 628 629 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 630 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 631 if (codeString == null || "".equals(codeString)) 632 if (codeString == null || "".equals(codeString)) 633 return null; 634 if ("applicability".equals(codeString)) 635 return ActionConditionKind.APPLICABILITY; 636 if ("start".equals(codeString)) 637 return ActionConditionKind.START; 638 if ("stop".equals(codeString)) 639 return ActionConditionKind.STOP; 640 throw new IllegalArgumentException("Unknown ActionConditionKind code '"+codeString+"'"); 641 } 642 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 643 if (code == null) 644 return null; 645 if (code.isEmpty()) 646 return new Enumeration<ActionConditionKind>(this); 647 String codeString = code.asStringValue(); 648 if (codeString == null || "".equals(codeString)) 649 return null; 650 if ("applicability".equals(codeString)) 651 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY); 652 if ("start".equals(codeString)) 653 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START); 654 if ("stop".equals(codeString)) 655 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP); 656 throw new FHIRException("Unknown ActionConditionKind code '"+codeString+"'"); 657 } 658 public String toCode(ActionConditionKind code) { 659 if (code == ActionConditionKind.NULL) 660 return null; 661 if (code == ActionConditionKind.APPLICABILITY) 662 return "applicability"; 663 if (code == ActionConditionKind.START) 664 return "start"; 665 if (code == ActionConditionKind.STOP) 666 return "stop"; 667 return "?"; 668 } 669 public String toSystem(ActionConditionKind code) { 670 return code.getSystem(); 671 } 672 } 673 674 public enum ActionRelationshipType { 675 /** 676 * The action must be performed before the start of the related action 677 */ 678 BEFORESTART, 679 /** 680 * The action must be performed before the related action 681 */ 682 BEFORE, 683 /** 684 * The action must be performed before the end of the related action 685 */ 686 BEFOREEND, 687 /** 688 * The action must be performed concurrent with the start of the related action 689 */ 690 CONCURRENTWITHSTART, 691 /** 692 * The action must be performed concurrent with the related action 693 */ 694 CONCURRENT, 695 /** 696 * The action must be performed concurrent with the end of the related action 697 */ 698 CONCURRENTWITHEND, 699 /** 700 * The action must be performed after the start of the related action 701 */ 702 AFTERSTART, 703 /** 704 * The action must be performed after the related action 705 */ 706 AFTER, 707 /** 708 * The action must be performed after the end of the related action 709 */ 710 AFTEREND, 711 /** 712 * added to help the parsers with the generic types 713 */ 714 NULL; 715 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 716 if (codeString == null || "".equals(codeString)) 717 return null; 718 if ("before-start".equals(codeString)) 719 return BEFORESTART; 720 if ("before".equals(codeString)) 721 return BEFORE; 722 if ("before-end".equals(codeString)) 723 return BEFOREEND; 724 if ("concurrent-with-start".equals(codeString)) 725 return CONCURRENTWITHSTART; 726 if ("concurrent".equals(codeString)) 727 return CONCURRENT; 728 if ("concurrent-with-end".equals(codeString)) 729 return CONCURRENTWITHEND; 730 if ("after-start".equals(codeString)) 731 return AFTERSTART; 732 if ("after".equals(codeString)) 733 return AFTER; 734 if ("after-end".equals(codeString)) 735 return AFTEREND; 736 if (Configuration.isAcceptInvalidEnums()) 737 return null; 738 else 739 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 740 } 741 public String toCode() { 742 switch (this) { 743 case BEFORESTART: return "before-start"; 744 case BEFORE: return "before"; 745 case BEFOREEND: return "before-end"; 746 case CONCURRENTWITHSTART: return "concurrent-with-start"; 747 case CONCURRENT: return "concurrent"; 748 case CONCURRENTWITHEND: return "concurrent-with-end"; 749 case AFTERSTART: return "after-start"; 750 case AFTER: return "after"; 751 case AFTEREND: return "after-end"; 752 case NULL: return null; 753 default: return "?"; 754 } 755 } 756 public String getSystem() { 757 switch (this) { 758 case BEFORESTART: return "http://hl7.org/fhir/action-relationship-type"; 759 case BEFORE: return "http://hl7.org/fhir/action-relationship-type"; 760 case BEFOREEND: return "http://hl7.org/fhir/action-relationship-type"; 761 case CONCURRENTWITHSTART: return "http://hl7.org/fhir/action-relationship-type"; 762 case CONCURRENT: return "http://hl7.org/fhir/action-relationship-type"; 763 case CONCURRENTWITHEND: return "http://hl7.org/fhir/action-relationship-type"; 764 case AFTERSTART: return "http://hl7.org/fhir/action-relationship-type"; 765 case AFTER: return "http://hl7.org/fhir/action-relationship-type"; 766 case AFTEREND: return "http://hl7.org/fhir/action-relationship-type"; 767 case NULL: return null; 768 default: return "?"; 769 } 770 } 771 public String getDefinition() { 772 switch (this) { 773 case BEFORESTART: return "The action must be performed before the start of the related action"; 774 case BEFORE: return "The action must be performed before the related action"; 775 case BEFOREEND: return "The action must be performed before the end of the related action"; 776 case CONCURRENTWITHSTART: return "The action must be performed concurrent with the start of the related action"; 777 case CONCURRENT: return "The action must be performed concurrent with the related action"; 778 case CONCURRENTWITHEND: return "The action must be performed concurrent with the end of the related action"; 779 case AFTERSTART: return "The action must be performed after the start of the related action"; 780 case AFTER: return "The action must be performed after the related action"; 781 case AFTEREND: return "The action must be performed after the end of the related action"; 782 case NULL: return null; 783 default: return "?"; 784 } 785 } 786 public String getDisplay() { 787 switch (this) { 788 case BEFORESTART: return "Before Start"; 789 case BEFORE: return "Before"; 790 case BEFOREEND: return "Before End"; 791 case CONCURRENTWITHSTART: return "Concurrent With Start"; 792 case CONCURRENT: return "Concurrent"; 793 case CONCURRENTWITHEND: return "Concurrent With End"; 794 case AFTERSTART: return "After Start"; 795 case AFTER: return "After"; 796 case AFTEREND: return "After End"; 797 case NULL: return null; 798 default: return "?"; 799 } 800 } 801 } 802 803 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 804 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 805 if (codeString == null || "".equals(codeString)) 806 if (codeString == null || "".equals(codeString)) 807 return null; 808 if ("before-start".equals(codeString)) 809 return ActionRelationshipType.BEFORESTART; 810 if ("before".equals(codeString)) 811 return ActionRelationshipType.BEFORE; 812 if ("before-end".equals(codeString)) 813 return ActionRelationshipType.BEFOREEND; 814 if ("concurrent-with-start".equals(codeString)) 815 return ActionRelationshipType.CONCURRENTWITHSTART; 816 if ("concurrent".equals(codeString)) 817 return ActionRelationshipType.CONCURRENT; 818 if ("concurrent-with-end".equals(codeString)) 819 return ActionRelationshipType.CONCURRENTWITHEND; 820 if ("after-start".equals(codeString)) 821 return ActionRelationshipType.AFTERSTART; 822 if ("after".equals(codeString)) 823 return ActionRelationshipType.AFTER; 824 if ("after-end".equals(codeString)) 825 return ActionRelationshipType.AFTEREND; 826 throw new IllegalArgumentException("Unknown ActionRelationshipType code '"+codeString+"'"); 827 } 828 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 829 if (code == null) 830 return null; 831 if (code.isEmpty()) 832 return new Enumeration<ActionRelationshipType>(this); 833 String codeString = code.asStringValue(); 834 if (codeString == null || "".equals(codeString)) 835 return null; 836 if ("before-start".equals(codeString)) 837 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART); 838 if ("before".equals(codeString)) 839 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE); 840 if ("before-end".equals(codeString)) 841 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND); 842 if ("concurrent-with-start".equals(codeString)) 843 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART); 844 if ("concurrent".equals(codeString)) 845 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT); 846 if ("concurrent-with-end".equals(codeString)) 847 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND); 848 if ("after-start".equals(codeString)) 849 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART); 850 if ("after".equals(codeString)) 851 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER); 852 if ("after-end".equals(codeString)) 853 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND); 854 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 855 } 856 public String toCode(ActionRelationshipType code) { 857 if (code == ActionRelationshipType.NULL) 858 return null; 859 if (code == ActionRelationshipType.BEFORESTART) 860 return "before-start"; 861 if (code == ActionRelationshipType.BEFORE) 862 return "before"; 863 if (code == ActionRelationshipType.BEFOREEND) 864 return "before-end"; 865 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 866 return "concurrent-with-start"; 867 if (code == ActionRelationshipType.CONCURRENT) 868 return "concurrent"; 869 if (code == ActionRelationshipType.CONCURRENTWITHEND) 870 return "concurrent-with-end"; 871 if (code == ActionRelationshipType.AFTERSTART) 872 return "after-start"; 873 if (code == ActionRelationshipType.AFTER) 874 return "after"; 875 if (code == ActionRelationshipType.AFTEREND) 876 return "after-end"; 877 return "?"; 878 } 879 public String toSystem(ActionRelationshipType code) { 880 return code.getSystem(); 881 } 882 } 883 884 public enum ActionGroupingBehavior { 885 /** 886 * Any group marked with this behavior should be displayed as a visual group to the end user 887 */ 888 VISUALGROUP, 889 /** 890 * A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so 891 */ 892 LOGICALGROUP, 893 /** 894 * A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of "AtMostOne", unless it's a required action, in which case, it would be "ExactlyOne" 895 */ 896 SENTENCEGROUP, 897 /** 898 * added to help the parsers with the generic types 899 */ 900 NULL; 901 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 902 if (codeString == null || "".equals(codeString)) 903 return null; 904 if ("visual-group".equals(codeString)) 905 return VISUALGROUP; 906 if ("logical-group".equals(codeString)) 907 return LOGICALGROUP; 908 if ("sentence-group".equals(codeString)) 909 return SENTENCEGROUP; 910 if (Configuration.isAcceptInvalidEnums()) 911 return null; 912 else 913 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 914 } 915 public String toCode() { 916 switch (this) { 917 case VISUALGROUP: return "visual-group"; 918 case LOGICALGROUP: return "logical-group"; 919 case SENTENCEGROUP: return "sentence-group"; 920 case NULL: return null; 921 default: return "?"; 922 } 923 } 924 public String getSystem() { 925 switch (this) { 926 case VISUALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 927 case LOGICALGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 928 case SENTENCEGROUP: return "http://hl7.org/fhir/action-grouping-behavior"; 929 case NULL: return null; 930 default: return "?"; 931 } 932 } 933 public String getDefinition() { 934 switch (this) { 935 case VISUALGROUP: return "Any group marked with this behavior should be displayed as a visual group to the end user"; 936 case LOGICALGROUP: return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so"; 937 case SENTENCEGROUP: return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\""; 938 case NULL: return null; 939 default: return "?"; 940 } 941 } 942 public String getDisplay() { 943 switch (this) { 944 case VISUALGROUP: return "Visual Group"; 945 case LOGICALGROUP: return "Logical Group"; 946 case SENTENCEGROUP: return "Sentence Group"; 947 case NULL: return null; 948 default: return "?"; 949 } 950 } 951 } 952 953 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 954 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 955 if (codeString == null || "".equals(codeString)) 956 if (codeString == null || "".equals(codeString)) 957 return null; 958 if ("visual-group".equals(codeString)) 959 return ActionGroupingBehavior.VISUALGROUP; 960 if ("logical-group".equals(codeString)) 961 return ActionGroupingBehavior.LOGICALGROUP; 962 if ("sentence-group".equals(codeString)) 963 return ActionGroupingBehavior.SENTENCEGROUP; 964 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 965 } 966 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 967 if (code == null) 968 return null; 969 if (code.isEmpty()) 970 return new Enumeration<ActionGroupingBehavior>(this); 971 String codeString = code.asStringValue(); 972 if (codeString == null || "".equals(codeString)) 973 return null; 974 if ("visual-group".equals(codeString)) 975 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP); 976 if ("logical-group".equals(codeString)) 977 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP); 978 if ("sentence-group".equals(codeString)) 979 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP); 980 throw new FHIRException("Unknown ActionGroupingBehavior code '"+codeString+"'"); 981 } 982 public String toCode(ActionGroupingBehavior code) { 983 if (code == ActionGroupingBehavior.NULL) 984 return null; 985 if (code == ActionGroupingBehavior.VISUALGROUP) 986 return "visual-group"; 987 if (code == ActionGroupingBehavior.LOGICALGROUP) 988 return "logical-group"; 989 if (code == ActionGroupingBehavior.SENTENCEGROUP) 990 return "sentence-group"; 991 return "?"; 992 } 993 public String toSystem(ActionGroupingBehavior code) { 994 return code.getSystem(); 995 } 996 } 997 998 public enum ActionSelectionBehavior { 999 /** 1000 * Any number of the actions in the group may be chosen, from zero to all 1001 */ 1002 ANY, 1003 /** 1004 * All the actions in the group must be selected as a single unit 1005 */ 1006 ALL, 1007 /** 1008 * All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected 1009 */ 1010 ALLORNONE, 1011 /** 1012 * The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group 1013 */ 1014 EXACTLYONE, 1015 /** 1016 * The end user may choose zero or at most one of the actions in the group 1017 */ 1018 ATMOSTONE, 1019 /** 1020 * The end user must choose a minimum of one, and as many additional as desired 1021 */ 1022 ONEORMORE, 1023 /** 1024 * added to help the parsers with the generic types 1025 */ 1026 NULL; 1027 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 1028 if (codeString == null || "".equals(codeString)) 1029 return null; 1030 if ("any".equals(codeString)) 1031 return ANY; 1032 if ("all".equals(codeString)) 1033 return ALL; 1034 if ("all-or-none".equals(codeString)) 1035 return ALLORNONE; 1036 if ("exactly-one".equals(codeString)) 1037 return EXACTLYONE; 1038 if ("at-most-one".equals(codeString)) 1039 return ATMOSTONE; 1040 if ("one-or-more".equals(codeString)) 1041 return ONEORMORE; 1042 if (Configuration.isAcceptInvalidEnums()) 1043 return null; 1044 else 1045 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1046 } 1047 public String toCode() { 1048 switch (this) { 1049 case ANY: return "any"; 1050 case ALL: return "all"; 1051 case ALLORNONE: return "all-or-none"; 1052 case EXACTLYONE: return "exactly-one"; 1053 case ATMOSTONE: return "at-most-one"; 1054 case ONEORMORE: return "one-or-more"; 1055 case NULL: return null; 1056 default: return "?"; 1057 } 1058 } 1059 public String getSystem() { 1060 switch (this) { 1061 case ANY: return "http://hl7.org/fhir/action-selection-behavior"; 1062 case ALL: return "http://hl7.org/fhir/action-selection-behavior"; 1063 case ALLORNONE: return "http://hl7.org/fhir/action-selection-behavior"; 1064 case EXACTLYONE: return "http://hl7.org/fhir/action-selection-behavior"; 1065 case ATMOSTONE: return "http://hl7.org/fhir/action-selection-behavior"; 1066 case ONEORMORE: return "http://hl7.org/fhir/action-selection-behavior"; 1067 case NULL: return null; 1068 default: return "?"; 1069 } 1070 } 1071 public String getDefinition() { 1072 switch (this) { 1073 case ANY: return "Any number of the actions in the group may be chosen, from zero to all"; 1074 case ALL: return "All the actions in the group must be selected as a single unit"; 1075 case ALLORNONE: return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected"; 1076 case EXACTLYONE: return "The end user must choose one and only one of the selectable actions in the group. The user may not choose none of the actions in the group"; 1077 case ATMOSTONE: return "The end user may choose zero or at most one of the actions in the group"; 1078 case ONEORMORE: return "The end user must choose a minimum of one, and as many additional as desired"; 1079 case NULL: return null; 1080 default: return "?"; 1081 } 1082 } 1083 public String getDisplay() { 1084 switch (this) { 1085 case ANY: return "Any"; 1086 case ALL: return "All"; 1087 case ALLORNONE: return "All Or None"; 1088 case EXACTLYONE: return "Exactly One"; 1089 case ATMOSTONE: return "At Most One"; 1090 case ONEORMORE: return "One Or More"; 1091 case NULL: return null; 1092 default: return "?"; 1093 } 1094 } 1095 } 1096 1097 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1098 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1099 if (codeString == null || "".equals(codeString)) 1100 if (codeString == null || "".equals(codeString)) 1101 return null; 1102 if ("any".equals(codeString)) 1103 return ActionSelectionBehavior.ANY; 1104 if ("all".equals(codeString)) 1105 return ActionSelectionBehavior.ALL; 1106 if ("all-or-none".equals(codeString)) 1107 return ActionSelectionBehavior.ALLORNONE; 1108 if ("exactly-one".equals(codeString)) 1109 return ActionSelectionBehavior.EXACTLYONE; 1110 if ("at-most-one".equals(codeString)) 1111 return ActionSelectionBehavior.ATMOSTONE; 1112 if ("one-or-more".equals(codeString)) 1113 return ActionSelectionBehavior.ONEORMORE; 1114 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1115 } 1116 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1117 if (code == null) 1118 return null; 1119 if (code.isEmpty()) 1120 return new Enumeration<ActionSelectionBehavior>(this); 1121 String codeString = code.asStringValue(); 1122 if (codeString == null || "".equals(codeString)) 1123 return null; 1124 if ("any".equals(codeString)) 1125 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY); 1126 if ("all".equals(codeString)) 1127 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL); 1128 if ("all-or-none".equals(codeString)) 1129 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE); 1130 if ("exactly-one".equals(codeString)) 1131 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE); 1132 if ("at-most-one".equals(codeString)) 1133 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE); 1134 if ("one-or-more".equals(codeString)) 1135 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE); 1136 throw new FHIRException("Unknown ActionSelectionBehavior code '"+codeString+"'"); 1137 } 1138 public String toCode(ActionSelectionBehavior code) { 1139 if (code == ActionSelectionBehavior.NULL) 1140 return null; 1141 if (code == ActionSelectionBehavior.ANY) 1142 return "any"; 1143 if (code == ActionSelectionBehavior.ALL) 1144 return "all"; 1145 if (code == ActionSelectionBehavior.ALLORNONE) 1146 return "all-or-none"; 1147 if (code == ActionSelectionBehavior.EXACTLYONE) 1148 return "exactly-one"; 1149 if (code == ActionSelectionBehavior.ATMOSTONE) 1150 return "at-most-one"; 1151 if (code == ActionSelectionBehavior.ONEORMORE) 1152 return "one-or-more"; 1153 return "?"; 1154 } 1155 public String toSystem(ActionSelectionBehavior code) { 1156 return code.getSystem(); 1157 } 1158 } 1159 1160 public enum ActionRequiredBehavior { 1161 /** 1162 * An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action 1163 */ 1164 MUST, 1165 /** 1166 * An action with this behavior may be included in the set of actions processed by the end user 1167 */ 1168 COULD, 1169 /** 1170 * An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included 1171 */ 1172 MUSTUNLESSDOCUMENTED, 1173 /** 1174 * added to help the parsers with the generic types 1175 */ 1176 NULL; 1177 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1178 if (codeString == null || "".equals(codeString)) 1179 return null; 1180 if ("must".equals(codeString)) 1181 return MUST; 1182 if ("could".equals(codeString)) 1183 return COULD; 1184 if ("must-unless-documented".equals(codeString)) 1185 return MUSTUNLESSDOCUMENTED; 1186 if (Configuration.isAcceptInvalidEnums()) 1187 return null; 1188 else 1189 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1190 } 1191 public String toCode() { 1192 switch (this) { 1193 case MUST: return "must"; 1194 case COULD: return "could"; 1195 case MUSTUNLESSDOCUMENTED: return "must-unless-documented"; 1196 case NULL: return null; 1197 default: return "?"; 1198 } 1199 } 1200 public String getSystem() { 1201 switch (this) { 1202 case MUST: return "http://hl7.org/fhir/action-required-behavior"; 1203 case COULD: return "http://hl7.org/fhir/action-required-behavior"; 1204 case MUSTUNLESSDOCUMENTED: return "http://hl7.org/fhir/action-required-behavior"; 1205 case NULL: return null; 1206 default: return "?"; 1207 } 1208 } 1209 public String getDefinition() { 1210 switch (this) { 1211 case MUST: return "An action with this behavior must be included in the actions processed by the end user; the end user may not choose not to include this action"; 1212 case COULD: return "An action with this behavior may be included in the set of actions processed by the end user"; 1213 case MUSTUNLESSDOCUMENTED: return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included"; 1214 case NULL: return null; 1215 default: return "?"; 1216 } 1217 } 1218 public String getDisplay() { 1219 switch (this) { 1220 case MUST: return "Must"; 1221 case COULD: return "Could"; 1222 case MUSTUNLESSDOCUMENTED: return "Must Unless Documented"; 1223 case NULL: return null; 1224 default: return "?"; 1225 } 1226 } 1227 } 1228 1229 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1230 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1231 if (codeString == null || "".equals(codeString)) 1232 if (codeString == null || "".equals(codeString)) 1233 return null; 1234 if ("must".equals(codeString)) 1235 return ActionRequiredBehavior.MUST; 1236 if ("could".equals(codeString)) 1237 return ActionRequiredBehavior.COULD; 1238 if ("must-unless-documented".equals(codeString)) 1239 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1240 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1241 } 1242 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1243 if (code == null) 1244 return null; 1245 if (code.isEmpty()) 1246 return new Enumeration<ActionRequiredBehavior>(this); 1247 String codeString = code.asStringValue(); 1248 if (codeString == null || "".equals(codeString)) 1249 return null; 1250 if ("must".equals(codeString)) 1251 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST); 1252 if ("could".equals(codeString)) 1253 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD); 1254 if ("must-unless-documented".equals(codeString)) 1255 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED); 1256 throw new FHIRException("Unknown ActionRequiredBehavior code '"+codeString+"'"); 1257 } 1258 public String toCode(ActionRequiredBehavior code) { 1259 if (code == ActionRequiredBehavior.NULL) 1260 return null; 1261 if (code == ActionRequiredBehavior.MUST) 1262 return "must"; 1263 if (code == ActionRequiredBehavior.COULD) 1264 return "could"; 1265 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1266 return "must-unless-documented"; 1267 return "?"; 1268 } 1269 public String toSystem(ActionRequiredBehavior code) { 1270 return code.getSystem(); 1271 } 1272 } 1273 1274 public enum ActionPrecheckBehavior { 1275 /** 1276 * An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider "pre-checking" such an action as a convenience for the user 1277 */ 1278 YES, 1279 /** 1280 * An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not "pre-check" such an action 1281 */ 1282 NO, 1283 /** 1284 * added to help the parsers with the generic types 1285 */ 1286 NULL; 1287 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1288 if (codeString == null || "".equals(codeString)) 1289 return null; 1290 if ("yes".equals(codeString)) 1291 return YES; 1292 if ("no".equals(codeString)) 1293 return NO; 1294 if (Configuration.isAcceptInvalidEnums()) 1295 return null; 1296 else 1297 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1298 } 1299 public String toCode() { 1300 switch (this) { 1301 case YES: return "yes"; 1302 case NO: return "no"; 1303 case NULL: return null; 1304 default: return "?"; 1305 } 1306 } 1307 public String getSystem() { 1308 switch (this) { 1309 case YES: return "http://hl7.org/fhir/action-precheck-behavior"; 1310 case NO: return "http://hl7.org/fhir/action-precheck-behavior"; 1311 case NULL: return null; 1312 default: return "?"; 1313 } 1314 } 1315 public String getDefinition() { 1316 switch (this) { 1317 case YES: return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user"; 1318 case NO: return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action"; 1319 case NULL: return null; 1320 default: return "?"; 1321 } 1322 } 1323 public String getDisplay() { 1324 switch (this) { 1325 case YES: return "Yes"; 1326 case NO: return "No"; 1327 case NULL: return null; 1328 default: return "?"; 1329 } 1330 } 1331 } 1332 1333 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1334 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1335 if (codeString == null || "".equals(codeString)) 1336 if (codeString == null || "".equals(codeString)) 1337 return null; 1338 if ("yes".equals(codeString)) 1339 return ActionPrecheckBehavior.YES; 1340 if ("no".equals(codeString)) 1341 return ActionPrecheckBehavior.NO; 1342 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1343 } 1344 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1345 if (code == null) 1346 return null; 1347 if (code.isEmpty()) 1348 return new Enumeration<ActionPrecheckBehavior>(this); 1349 String codeString = code.asStringValue(); 1350 if (codeString == null || "".equals(codeString)) 1351 return null; 1352 if ("yes".equals(codeString)) 1353 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES); 1354 if ("no".equals(codeString)) 1355 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO); 1356 throw new FHIRException("Unknown ActionPrecheckBehavior code '"+codeString+"'"); 1357 } 1358 public String toCode(ActionPrecheckBehavior code) { 1359 if (code == ActionPrecheckBehavior.NULL) 1360 return null; 1361 if (code == ActionPrecheckBehavior.YES) 1362 return "yes"; 1363 if (code == ActionPrecheckBehavior.NO) 1364 return "no"; 1365 return "?"; 1366 } 1367 public String toSystem(ActionPrecheckBehavior code) { 1368 return code.getSystem(); 1369 } 1370 } 1371 1372 public enum ActionCardinalityBehavior { 1373 /** 1374 * The action may only be selected one time 1375 */ 1376 SINGLE, 1377 /** 1378 * The action may be selected multiple times 1379 */ 1380 MULTIPLE, 1381 /** 1382 * added to help the parsers with the generic types 1383 */ 1384 NULL; 1385 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1386 if (codeString == null || "".equals(codeString)) 1387 return null; 1388 if ("single".equals(codeString)) 1389 return SINGLE; 1390 if ("multiple".equals(codeString)) 1391 return MULTIPLE; 1392 if (Configuration.isAcceptInvalidEnums()) 1393 return null; 1394 else 1395 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1396 } 1397 public String toCode() { 1398 switch (this) { 1399 case SINGLE: return "single"; 1400 case MULTIPLE: return "multiple"; 1401 case NULL: return null; 1402 default: return "?"; 1403 } 1404 } 1405 public String getSystem() { 1406 switch (this) { 1407 case SINGLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1408 case MULTIPLE: return "http://hl7.org/fhir/action-cardinality-behavior"; 1409 case NULL: return null; 1410 default: return "?"; 1411 } 1412 } 1413 public String getDefinition() { 1414 switch (this) { 1415 case SINGLE: return "The action may only be selected one time"; 1416 case MULTIPLE: return "The action may be selected multiple times"; 1417 case NULL: return null; 1418 default: return "?"; 1419 } 1420 } 1421 public String getDisplay() { 1422 switch (this) { 1423 case SINGLE: return "Single"; 1424 case MULTIPLE: return "Multiple"; 1425 case NULL: return null; 1426 default: return "?"; 1427 } 1428 } 1429 } 1430 1431 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1432 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1433 if (codeString == null || "".equals(codeString)) 1434 if (codeString == null || "".equals(codeString)) 1435 return null; 1436 if ("single".equals(codeString)) 1437 return ActionCardinalityBehavior.SINGLE; 1438 if ("multiple".equals(codeString)) 1439 return ActionCardinalityBehavior.MULTIPLE; 1440 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1441 } 1442 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1443 if (code == null) 1444 return null; 1445 if (code.isEmpty()) 1446 return new Enumeration<ActionCardinalityBehavior>(this); 1447 String codeString = code.asStringValue(); 1448 if (codeString == null || "".equals(codeString)) 1449 return null; 1450 if ("single".equals(codeString)) 1451 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE); 1452 if ("multiple".equals(codeString)) 1453 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE); 1454 throw new FHIRException("Unknown ActionCardinalityBehavior code '"+codeString+"'"); 1455 } 1456 public String toCode(ActionCardinalityBehavior code) { 1457 if (code == ActionCardinalityBehavior.NULL) 1458 return null; 1459 if (code == ActionCardinalityBehavior.SINGLE) 1460 return "single"; 1461 if (code == ActionCardinalityBehavior.MULTIPLE) 1462 return "multiple"; 1463 return "?"; 1464 } 1465 public String toSystem(ActionCardinalityBehavior code) { 1466 return code.getSystem(); 1467 } 1468 } 1469 1470 @Block() 1471 public static class RequestGroupActionComponent extends BackboneElement implements IBaseBackboneElement { 1472 /** 1473 * A user-visible label for the action. 1474 */ 1475 @Child(name = "label", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1476 @Description(shortDefinition="User-visible label for the action (e.g. 1. or A.)", formalDefinition="A user-visible label for the action." ) 1477 protected StringType label; 1478 1479 /** 1480 * The title of the action displayed to a user. 1481 */ 1482 @Child(name = "title", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1483 @Description(shortDefinition="User-visible title", formalDefinition="The title of the action displayed to a user." ) 1484 protected StringType title; 1485 1486 /** 1487 * A short description of the action used to provide a summary to display to the user. 1488 */ 1489 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1490 @Description(shortDefinition="Short description of the action", formalDefinition="A short description of the action used to provide a summary to display to the user." ) 1491 protected StringType description; 1492 1493 /** 1494 * A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1495 */ 1496 @Child(name = "textEquivalent", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1497 @Description(shortDefinition="Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition="A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically." ) 1498 protected StringType textEquivalent; 1499 1500 /** 1501 * A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template. 1502 */ 1503 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1504 @Description(shortDefinition="Code representing the meaning of the action or sub-actions", formalDefinition="A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template." ) 1505 protected List<CodeableConcept> code; 1506 1507 /** 1508 * Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources. 1509 */ 1510 @Child(name = "documentation", type = {RelatedArtifact.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1511 @Description(shortDefinition="Supporting documentation for the intended performer of the action", formalDefinition="Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources." ) 1512 protected List<RelatedArtifact> documentation; 1513 1514 /** 1515 * An expression that describes applicability criteria, or start/stop conditions for the action. 1516 */ 1517 @Child(name = "condition", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1518 @Description(shortDefinition="Whether or not the action is applicable", formalDefinition="An expression that describes applicability criteria, or start/stop conditions for the action." ) 1519 protected List<RequestGroupActionConditionComponent> condition; 1520 1521 /** 1522 * A relationship to another action such as "before" or "30-60 minutes after start of". 1523 */ 1524 @Child(name = "relatedAction", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1525 @Description(shortDefinition="Relationship to another action", formalDefinition="A relationship to another action such as \"before\" or \"30-60 minutes after start of\"." ) 1526 protected List<RequestGroupActionRelatedActionComponent> relatedAction; 1527 1528 /** 1529 * An optional value describing when the action should be performed. 1530 */ 1531 @Child(name = "timing", type = {DateTimeType.class, Period.class, Duration.class, Range.class, Timing.class}, order=9, min=0, max=1, modifier=false, summary=false) 1532 @Description(shortDefinition="When the action should take place", formalDefinition="An optional value describing when the action should be performed." ) 1533 protected Type timing; 1534 1535 /** 1536 * The participant that should perform or be responsible for this action. 1537 */ 1538 @Child(name = "participant", type = {Patient.class, Person.class, Practitioner.class, RelatedPerson.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1539 @Description(shortDefinition="Who should perform the action", formalDefinition="The participant that should perform or be responsible for this action." ) 1540 protected List<Reference> participant; 1541 /** 1542 * The actual objects that are the target of the reference (The participant that should perform or be responsible for this action.) 1543 */ 1544 protected List<Resource> participantTarget; 1545 1546 1547 /** 1548 * The type of action to perform (create, update, remove). 1549 */ 1550 @Child(name = "type", type = {Coding.class}, order=11, min=0, max=1, modifier=false, summary=false) 1551 @Description(shortDefinition="create | update | remove | fire-event", formalDefinition="The type of action to perform (create, update, remove)." ) 1552 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-type") 1553 protected Coding type; 1554 1555 /** 1556 * Defines the grouping behavior for the action and its children. 1557 */ 1558 @Child(name = "groupingBehavior", type = {CodeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1559 @Description(shortDefinition="visual-group | logical-group | sentence-group", formalDefinition="Defines the grouping behavior for the action and its children." ) 1560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-grouping-behavior") 1561 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 1562 1563 /** 1564 * Defines the selection behavior for the action and its children. 1565 */ 1566 @Child(name = "selectionBehavior", type = {CodeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 1567 @Description(shortDefinition="any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition="Defines the selection behavior for the action and its children." ) 1568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-selection-behavior") 1569 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 1570 1571 /** 1572 * Defines the requiredness behavior for the action. 1573 */ 1574 @Child(name = "requiredBehavior", type = {CodeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1575 @Description(shortDefinition="must | could | must-unless-documented", formalDefinition="Defines the requiredness behavior for the action." ) 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-required-behavior") 1577 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 1578 1579 /** 1580 * Defines whether the action should usually be preselected. 1581 */ 1582 @Child(name = "precheckBehavior", type = {CodeType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1583 @Description(shortDefinition="yes | no", formalDefinition="Defines whether the action should usually be preselected." ) 1584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-precheck-behavior") 1585 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 1586 1587 /** 1588 * Defines whether the action can be selected multiple times. 1589 */ 1590 @Child(name = "cardinalityBehavior", type = {CodeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1591 @Description(shortDefinition="single | multiple", formalDefinition="Defines whether the action can be selected multiple times." ) 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 1593 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 1594 1595 /** 1596 * The resource that is the target of the action (e.g. CommunicationRequest). 1597 */ 1598 @Child(name = "resource", type = {Reference.class}, order=17, min=0, max=1, modifier=false, summary=false) 1599 @Description(shortDefinition="The target of the action", formalDefinition="The resource that is the target of the action (e.g. CommunicationRequest)." ) 1600 protected Reference resource; 1601 1602 /** 1603 * The actual object that is the target of the reference (The resource that is the target of the action (e.g. CommunicationRequest).) 1604 */ 1605 protected Resource resourceTarget; 1606 1607 /** 1608 * Sub actions. 1609 */ 1610 @Child(name = "action", type = {RequestGroupActionComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1611 @Description(shortDefinition="Sub action", formalDefinition="Sub actions." ) 1612 protected List<RequestGroupActionComponent> action; 1613 1614 private static final long serialVersionUID = 362859874L; 1615 1616 /** 1617 * Constructor 1618 */ 1619 public RequestGroupActionComponent() { 1620 super(); 1621 } 1622 1623 /** 1624 * @return {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1625 */ 1626 public StringType getLabelElement() { 1627 if (this.label == null) 1628 if (Configuration.errorOnAutoCreate()) 1629 throw new Error("Attempt to auto-create RequestGroupActionComponent.label"); 1630 else if (Configuration.doAutoCreate()) 1631 this.label = new StringType(); // bb 1632 return this.label; 1633 } 1634 1635 public boolean hasLabelElement() { 1636 return this.label != null && !this.label.isEmpty(); 1637 } 1638 1639 public boolean hasLabel() { 1640 return this.label != null && !this.label.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #label} (A user-visible label for the action.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 1645 */ 1646 public RequestGroupActionComponent setLabelElement(StringType value) { 1647 this.label = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return A user-visible label for the action. 1653 */ 1654 public String getLabel() { 1655 return this.label == null ? null : this.label.getValue(); 1656 } 1657 1658 /** 1659 * @param value A user-visible label for the action. 1660 */ 1661 public RequestGroupActionComponent setLabel(String value) { 1662 if (Utilities.noString(value)) 1663 this.label = null; 1664 else { 1665 if (this.label == null) 1666 this.label = new StringType(); 1667 this.label.setValue(value); 1668 } 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1674 */ 1675 public StringType getTitleElement() { 1676 if (this.title == null) 1677 if (Configuration.errorOnAutoCreate()) 1678 throw new Error("Attempt to auto-create RequestGroupActionComponent.title"); 1679 else if (Configuration.doAutoCreate()) 1680 this.title = new StringType(); // bb 1681 return this.title; 1682 } 1683 1684 public boolean hasTitleElement() { 1685 return this.title != null && !this.title.isEmpty(); 1686 } 1687 1688 public boolean hasTitle() { 1689 return this.title != null && !this.title.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1694 */ 1695 public RequestGroupActionComponent setTitleElement(StringType value) { 1696 this.title = value; 1697 return this; 1698 } 1699 1700 /** 1701 * @return The title of the action displayed to a user. 1702 */ 1703 public String getTitle() { 1704 return this.title == null ? null : this.title.getValue(); 1705 } 1706 1707 /** 1708 * @param value The title of the action displayed to a user. 1709 */ 1710 public RequestGroupActionComponent setTitle(String value) { 1711 if (Utilities.noString(value)) 1712 this.title = null; 1713 else { 1714 if (this.title == null) 1715 this.title = new StringType(); 1716 this.title.setValue(value); 1717 } 1718 return this; 1719 } 1720 1721 /** 1722 * @return {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1723 */ 1724 public StringType getDescriptionElement() { 1725 if (this.description == null) 1726 if (Configuration.errorOnAutoCreate()) 1727 throw new Error("Attempt to auto-create RequestGroupActionComponent.description"); 1728 else if (Configuration.doAutoCreate()) 1729 this.description = new StringType(); // bb 1730 return this.description; 1731 } 1732 1733 public boolean hasDescriptionElement() { 1734 return this.description != null && !this.description.isEmpty(); 1735 } 1736 1737 public boolean hasDescription() { 1738 return this.description != null && !this.description.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1743 */ 1744 public RequestGroupActionComponent setDescriptionElement(StringType value) { 1745 this.description = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return A short description of the action used to provide a summary to display to the user. 1751 */ 1752 public String getDescription() { 1753 return this.description == null ? null : this.description.getValue(); 1754 } 1755 1756 /** 1757 * @param value A short description of the action used to provide a summary to display to the user. 1758 */ 1759 public RequestGroupActionComponent setDescription(String value) { 1760 if (Utilities.noString(value)) 1761 this.description = null; 1762 else { 1763 if (this.description == null) 1764 this.description = new StringType(); 1765 this.description.setValue(value); 1766 } 1767 return this; 1768 } 1769 1770 /** 1771 * @return {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 1772 */ 1773 public StringType getTextEquivalentElement() { 1774 if (this.textEquivalent == null) 1775 if (Configuration.errorOnAutoCreate()) 1776 throw new Error("Attempt to auto-create RequestGroupActionComponent.textEquivalent"); 1777 else if (Configuration.doAutoCreate()) 1778 this.textEquivalent = new StringType(); // bb 1779 return this.textEquivalent; 1780 } 1781 1782 public boolean hasTextEquivalentElement() { 1783 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 1784 } 1785 1786 public boolean hasTextEquivalent() { 1787 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 1788 } 1789 1790 /** 1791 * @param value {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 1792 */ 1793 public RequestGroupActionComponent setTextEquivalentElement(StringType value) { 1794 this.textEquivalent = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1800 */ 1801 public String getTextEquivalent() { 1802 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 1803 } 1804 1805 /** 1806 * @param value A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically. 1807 */ 1808 public RequestGroupActionComponent setTextEquivalent(String value) { 1809 if (Utilities.noString(value)) 1810 this.textEquivalent = null; 1811 else { 1812 if (this.textEquivalent == null) 1813 this.textEquivalent = new StringType(); 1814 this.textEquivalent.setValue(value); 1815 } 1816 return this; 1817 } 1818 1819 /** 1820 * @return {@link #code} (A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.) 1821 */ 1822 public List<CodeableConcept> getCode() { 1823 if (this.code == null) 1824 this.code = new ArrayList<CodeableConcept>(); 1825 return this.code; 1826 } 1827 1828 /** 1829 * @return Returns a reference to <code>this</code> for easy method chaining 1830 */ 1831 public RequestGroupActionComponent setCode(List<CodeableConcept> theCode) { 1832 this.code = theCode; 1833 return this; 1834 } 1835 1836 public boolean hasCode() { 1837 if (this.code == null) 1838 return false; 1839 for (CodeableConcept item : this.code) 1840 if (!item.isEmpty()) 1841 return true; 1842 return false; 1843 } 1844 1845 public CodeableConcept addCode() { //3 1846 CodeableConcept t = new CodeableConcept(); 1847 if (this.code == null) 1848 this.code = new ArrayList<CodeableConcept>(); 1849 this.code.add(t); 1850 return t; 1851 } 1852 1853 public RequestGroupActionComponent addCode(CodeableConcept t) { //3 1854 if (t == null) 1855 return this; 1856 if (this.code == null) 1857 this.code = new ArrayList<CodeableConcept>(); 1858 this.code.add(t); 1859 return this; 1860 } 1861 1862 /** 1863 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 1864 */ 1865 public CodeableConcept getCodeFirstRep() { 1866 if (getCode().isEmpty()) { 1867 addCode(); 1868 } 1869 return getCode().get(0); 1870 } 1871 1872 /** 1873 * @return {@link #documentation} (Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.) 1874 */ 1875 public List<RelatedArtifact> getDocumentation() { 1876 if (this.documentation == null) 1877 this.documentation = new ArrayList<RelatedArtifact>(); 1878 return this.documentation; 1879 } 1880 1881 /** 1882 * @return Returns a reference to <code>this</code> for easy method chaining 1883 */ 1884 public RequestGroupActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 1885 this.documentation = theDocumentation; 1886 return this; 1887 } 1888 1889 public boolean hasDocumentation() { 1890 if (this.documentation == null) 1891 return false; 1892 for (RelatedArtifact item : this.documentation) 1893 if (!item.isEmpty()) 1894 return true; 1895 return false; 1896 } 1897 1898 public RelatedArtifact addDocumentation() { //3 1899 RelatedArtifact t = new RelatedArtifact(); 1900 if (this.documentation == null) 1901 this.documentation = new ArrayList<RelatedArtifact>(); 1902 this.documentation.add(t); 1903 return t; 1904 } 1905 1906 public RequestGroupActionComponent addDocumentation(RelatedArtifact t) { //3 1907 if (t == null) 1908 return this; 1909 if (this.documentation == null) 1910 this.documentation = new ArrayList<RelatedArtifact>(); 1911 this.documentation.add(t); 1912 return this; 1913 } 1914 1915 /** 1916 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist 1917 */ 1918 public RelatedArtifact getDocumentationFirstRep() { 1919 if (getDocumentation().isEmpty()) { 1920 addDocumentation(); 1921 } 1922 return getDocumentation().get(0); 1923 } 1924 1925 /** 1926 * @return {@link #condition} (An expression that describes applicability criteria, or start/stop conditions for the action.) 1927 */ 1928 public List<RequestGroupActionConditionComponent> getCondition() { 1929 if (this.condition == null) 1930 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1931 return this.condition; 1932 } 1933 1934 /** 1935 * @return Returns a reference to <code>this</code> for easy method chaining 1936 */ 1937 public RequestGroupActionComponent setCondition(List<RequestGroupActionConditionComponent> theCondition) { 1938 this.condition = theCondition; 1939 return this; 1940 } 1941 1942 public boolean hasCondition() { 1943 if (this.condition == null) 1944 return false; 1945 for (RequestGroupActionConditionComponent item : this.condition) 1946 if (!item.isEmpty()) 1947 return true; 1948 return false; 1949 } 1950 1951 public RequestGroupActionConditionComponent addCondition() { //3 1952 RequestGroupActionConditionComponent t = new RequestGroupActionConditionComponent(); 1953 if (this.condition == null) 1954 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1955 this.condition.add(t); 1956 return t; 1957 } 1958 1959 public RequestGroupActionComponent addCondition(RequestGroupActionConditionComponent t) { //3 1960 if (t == null) 1961 return this; 1962 if (this.condition == null) 1963 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 1964 this.condition.add(t); 1965 return this; 1966 } 1967 1968 /** 1969 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist 1970 */ 1971 public RequestGroupActionConditionComponent getConditionFirstRep() { 1972 if (getCondition().isEmpty()) { 1973 addCondition(); 1974 } 1975 return getCondition().get(0); 1976 } 1977 1978 /** 1979 * @return {@link #relatedAction} (A relationship to another action such as "before" or "30-60 minutes after start of".) 1980 */ 1981 public List<RequestGroupActionRelatedActionComponent> getRelatedAction() { 1982 if (this.relatedAction == null) 1983 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 1984 return this.relatedAction; 1985 } 1986 1987 /** 1988 * @return Returns a reference to <code>this</code> for easy method chaining 1989 */ 1990 public RequestGroupActionComponent setRelatedAction(List<RequestGroupActionRelatedActionComponent> theRelatedAction) { 1991 this.relatedAction = theRelatedAction; 1992 return this; 1993 } 1994 1995 public boolean hasRelatedAction() { 1996 if (this.relatedAction == null) 1997 return false; 1998 for (RequestGroupActionRelatedActionComponent item : this.relatedAction) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public RequestGroupActionRelatedActionComponent addRelatedAction() { //3 2005 RequestGroupActionRelatedActionComponent t = new RequestGroupActionRelatedActionComponent(); 2006 if (this.relatedAction == null) 2007 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2008 this.relatedAction.add(t); 2009 return t; 2010 } 2011 2012 public RequestGroupActionComponent addRelatedAction(RequestGroupActionRelatedActionComponent t) { //3 2013 if (t == null) 2014 return this; 2015 if (this.relatedAction == null) 2016 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2017 this.relatedAction.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field {@link #relatedAction}, creating it if it does not already exist 2023 */ 2024 public RequestGroupActionRelatedActionComponent getRelatedActionFirstRep() { 2025 if (getRelatedAction().isEmpty()) { 2026 addRelatedAction(); 2027 } 2028 return getRelatedAction().get(0); 2029 } 2030 2031 /** 2032 * @return {@link #timing} (An optional value describing when the action should be performed.) 2033 */ 2034 public Type getTiming() { 2035 return this.timing; 2036 } 2037 2038 /** 2039 * @return {@link #timing} (An optional value describing when the action should be performed.) 2040 */ 2041 public DateTimeType getTimingDateTimeType() throws FHIRException { 2042 if (this.timing == null) 2043 return null; 2044 if (!(this.timing instanceof DateTimeType)) 2045 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 2046 return (DateTimeType) this.timing; 2047 } 2048 2049 public boolean hasTimingDateTimeType() { 2050 return this != null && this.timing instanceof DateTimeType; 2051 } 2052 2053 /** 2054 * @return {@link #timing} (An optional value describing when the action should be performed.) 2055 */ 2056 public Period getTimingPeriod() throws FHIRException { 2057 if (this.timing == null) 2058 return null; 2059 if (!(this.timing instanceof Period)) 2060 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 2061 return (Period) this.timing; 2062 } 2063 2064 public boolean hasTimingPeriod() { 2065 return this != null && this.timing instanceof Period; 2066 } 2067 2068 /** 2069 * @return {@link #timing} (An optional value describing when the action should be performed.) 2070 */ 2071 public Duration getTimingDuration() throws FHIRException { 2072 if (this.timing == null) 2073 return null; 2074 if (!(this.timing instanceof Duration)) 2075 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 2076 return (Duration) this.timing; 2077 } 2078 2079 public boolean hasTimingDuration() { 2080 return this != null && this.timing instanceof Duration; 2081 } 2082 2083 /** 2084 * @return {@link #timing} (An optional value describing when the action should be performed.) 2085 */ 2086 public Range getTimingRange() throws FHIRException { 2087 if (this.timing == null) 2088 return null; 2089 if (!(this.timing instanceof Range)) 2090 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 2091 return (Range) this.timing; 2092 } 2093 2094 public boolean hasTimingRange() { 2095 return this != null && this.timing instanceof Range; 2096 } 2097 2098 /** 2099 * @return {@link #timing} (An optional value describing when the action should be performed.) 2100 */ 2101 public Timing getTimingTiming() throws FHIRException { 2102 if (this.timing == null) 2103 return null; 2104 if (!(this.timing instanceof Timing)) 2105 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 2106 return (Timing) this.timing; 2107 } 2108 2109 public boolean hasTimingTiming() { 2110 return this != null && this.timing instanceof Timing; 2111 } 2112 2113 public boolean hasTiming() { 2114 return this.timing != null && !this.timing.isEmpty(); 2115 } 2116 2117 /** 2118 * @param value {@link #timing} (An optional value describing when the action should be performed.) 2119 */ 2120 public RequestGroupActionComponent setTiming(Type value) throws FHIRFormatError { 2121 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2122 throw new FHIRFormatError("Not the right type for RequestGroup.action.timing[x]: "+value.fhirType()); 2123 this.timing = value; 2124 return this; 2125 } 2126 2127 /** 2128 * @return {@link #participant} (The participant that should perform or be responsible for this action.) 2129 */ 2130 public List<Reference> getParticipant() { 2131 if (this.participant == null) 2132 this.participant = new ArrayList<Reference>(); 2133 return this.participant; 2134 } 2135 2136 /** 2137 * @return Returns a reference to <code>this</code> for easy method chaining 2138 */ 2139 public RequestGroupActionComponent setParticipant(List<Reference> theParticipant) { 2140 this.participant = theParticipant; 2141 return this; 2142 } 2143 2144 public boolean hasParticipant() { 2145 if (this.participant == null) 2146 return false; 2147 for (Reference item : this.participant) 2148 if (!item.isEmpty()) 2149 return true; 2150 return false; 2151 } 2152 2153 public Reference addParticipant() { //3 2154 Reference t = new Reference(); 2155 if (this.participant == null) 2156 this.participant = new ArrayList<Reference>(); 2157 this.participant.add(t); 2158 return t; 2159 } 2160 2161 public RequestGroupActionComponent addParticipant(Reference t) { //3 2162 if (t == null) 2163 return this; 2164 if (this.participant == null) 2165 this.participant = new ArrayList<Reference>(); 2166 this.participant.add(t); 2167 return this; 2168 } 2169 2170 /** 2171 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 2172 */ 2173 public Reference getParticipantFirstRep() { 2174 if (getParticipant().isEmpty()) { 2175 addParticipant(); 2176 } 2177 return getParticipant().get(0); 2178 } 2179 2180 /** 2181 * @deprecated Use Reference#setResource(IBaseResource) instead 2182 */ 2183 @Deprecated 2184 public List<Resource> getParticipantTarget() { 2185 if (this.participantTarget == null) 2186 this.participantTarget = new ArrayList<Resource>(); 2187 return this.participantTarget; 2188 } 2189 2190 /** 2191 * @return {@link #type} (The type of action to perform (create, update, remove).) 2192 */ 2193 public Coding getType() { 2194 if (this.type == null) 2195 if (Configuration.errorOnAutoCreate()) 2196 throw new Error("Attempt to auto-create RequestGroupActionComponent.type"); 2197 else if (Configuration.doAutoCreate()) 2198 this.type = new Coding(); // cc 2199 return this.type; 2200 } 2201 2202 public boolean hasType() { 2203 return this.type != null && !this.type.isEmpty(); 2204 } 2205 2206 /** 2207 * @param value {@link #type} (The type of action to perform (create, update, remove).) 2208 */ 2209 public RequestGroupActionComponent setType(Coding value) { 2210 this.type = value; 2211 return this; 2212 } 2213 2214 /** 2215 * @return {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2216 */ 2217 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2218 if (this.groupingBehavior == null) 2219 if (Configuration.errorOnAutoCreate()) 2220 throw new Error("Attempt to auto-create RequestGroupActionComponent.groupingBehavior"); 2221 else if (Configuration.doAutoCreate()) 2222 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2223 return this.groupingBehavior; 2224 } 2225 2226 public boolean hasGroupingBehaviorElement() { 2227 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2228 } 2229 2230 public boolean hasGroupingBehavior() { 2231 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2232 } 2233 2234 /** 2235 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 2236 */ 2237 public RequestGroupActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 2238 this.groupingBehavior = value; 2239 return this; 2240 } 2241 2242 /** 2243 * @return Defines the grouping behavior for the action and its children. 2244 */ 2245 public ActionGroupingBehavior getGroupingBehavior() { 2246 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 2247 } 2248 2249 /** 2250 * @param value Defines the grouping behavior for the action and its children. 2251 */ 2252 public RequestGroupActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 2253 if (value == null) 2254 this.groupingBehavior = null; 2255 else { 2256 if (this.groupingBehavior == null) 2257 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 2258 this.groupingBehavior.setValue(value); 2259 } 2260 return this; 2261 } 2262 2263 /** 2264 * @return {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 2265 */ 2266 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 2267 if (this.selectionBehavior == null) 2268 if (Configuration.errorOnAutoCreate()) 2269 throw new Error("Attempt to auto-create RequestGroupActionComponent.selectionBehavior"); 2270 else if (Configuration.doAutoCreate()) 2271 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 2272 return this.selectionBehavior; 2273 } 2274 2275 public boolean hasSelectionBehaviorElement() { 2276 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2277 } 2278 2279 public boolean hasSelectionBehavior() { 2280 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2281 } 2282 2283 /** 2284 * @param value {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 2285 */ 2286 public RequestGroupActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 2287 this.selectionBehavior = value; 2288 return this; 2289 } 2290 2291 /** 2292 * @return Defines the selection behavior for the action and its children. 2293 */ 2294 public ActionSelectionBehavior getSelectionBehavior() { 2295 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 2296 } 2297 2298 /** 2299 * @param value Defines the selection behavior for the action and its children. 2300 */ 2301 public RequestGroupActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 2302 if (value == null) 2303 this.selectionBehavior = null; 2304 else { 2305 if (this.selectionBehavior == null) 2306 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 2307 this.selectionBehavior.setValue(value); 2308 } 2309 return this; 2310 } 2311 2312 /** 2313 * @return {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 2314 */ 2315 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 2316 if (this.requiredBehavior == null) 2317 if (Configuration.errorOnAutoCreate()) 2318 throw new Error("Attempt to auto-create RequestGroupActionComponent.requiredBehavior"); 2319 else if (Configuration.doAutoCreate()) 2320 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 2321 return this.requiredBehavior; 2322 } 2323 2324 public boolean hasRequiredBehaviorElement() { 2325 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2326 } 2327 2328 public boolean hasRequiredBehavior() { 2329 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2330 } 2331 2332 /** 2333 * @param value {@link #requiredBehavior} (Defines the requiredness behavior for the action.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 2334 */ 2335 public RequestGroupActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 2336 this.requiredBehavior = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return Defines the requiredness behavior for the action. 2342 */ 2343 public ActionRequiredBehavior getRequiredBehavior() { 2344 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 2345 } 2346 2347 /** 2348 * @param value Defines the requiredness behavior for the action. 2349 */ 2350 public RequestGroupActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 2351 if (value == null) 2352 this.requiredBehavior = null; 2353 else { 2354 if (this.requiredBehavior == null) 2355 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 2356 this.requiredBehavior.setValue(value); 2357 } 2358 return this; 2359 } 2360 2361 /** 2362 * @return {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 2363 */ 2364 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 2365 if (this.precheckBehavior == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create RequestGroupActionComponent.precheckBehavior"); 2368 else if (Configuration.doAutoCreate()) 2369 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 2370 return this.precheckBehavior; 2371 } 2372 2373 public boolean hasPrecheckBehaviorElement() { 2374 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2375 } 2376 2377 public boolean hasPrecheckBehavior() { 2378 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2379 } 2380 2381 /** 2382 * @param value {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 2383 */ 2384 public RequestGroupActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 2385 this.precheckBehavior = value; 2386 return this; 2387 } 2388 2389 /** 2390 * @return Defines whether the action should usually be preselected. 2391 */ 2392 public ActionPrecheckBehavior getPrecheckBehavior() { 2393 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 2394 } 2395 2396 /** 2397 * @param value Defines whether the action should usually be preselected. 2398 */ 2399 public RequestGroupActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 2400 if (value == null) 2401 this.precheckBehavior = null; 2402 else { 2403 if (this.precheckBehavior == null) 2404 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 2405 this.precheckBehavior.setValue(value); 2406 } 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 2412 */ 2413 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 2414 if (this.cardinalityBehavior == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create RequestGroupActionComponent.cardinalityBehavior"); 2417 else if (Configuration.doAutoCreate()) 2418 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); // bb 2419 return this.cardinalityBehavior; 2420 } 2421 2422 public boolean hasCardinalityBehaviorElement() { 2423 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 2424 } 2425 2426 public boolean hasCardinalityBehavior() { 2427 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 2428 } 2429 2430 /** 2431 * @param value {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 2432 */ 2433 public RequestGroupActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 2434 this.cardinalityBehavior = value; 2435 return this; 2436 } 2437 2438 /** 2439 * @return Defines whether the action can be selected multiple times. 2440 */ 2441 public ActionCardinalityBehavior getCardinalityBehavior() { 2442 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 2443 } 2444 2445 /** 2446 * @param value Defines whether the action can be selected multiple times. 2447 */ 2448 public RequestGroupActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 2449 if (value == null) 2450 this.cardinalityBehavior = null; 2451 else { 2452 if (this.cardinalityBehavior == null) 2453 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); 2454 this.cardinalityBehavior.setValue(value); 2455 } 2456 return this; 2457 } 2458 2459 /** 2460 * @return {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 2461 */ 2462 public Reference getResource() { 2463 if (this.resource == null) 2464 if (Configuration.errorOnAutoCreate()) 2465 throw new Error("Attempt to auto-create RequestGroupActionComponent.resource"); 2466 else if (Configuration.doAutoCreate()) 2467 this.resource = new Reference(); // cc 2468 return this.resource; 2469 } 2470 2471 public boolean hasResource() { 2472 return this.resource != null && !this.resource.isEmpty(); 2473 } 2474 2475 /** 2476 * @param value {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 2477 */ 2478 public RequestGroupActionComponent setResource(Reference value) { 2479 this.resource = value; 2480 return this; 2481 } 2482 2483 /** 2484 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The resource that is the target of the action (e.g. CommunicationRequest).) 2485 */ 2486 public Resource getResourceTarget() { 2487 return this.resourceTarget; 2488 } 2489 2490 /** 2491 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The resource that is the target of the action (e.g. CommunicationRequest).) 2492 */ 2493 public RequestGroupActionComponent setResourceTarget(Resource value) { 2494 this.resourceTarget = value; 2495 return this; 2496 } 2497 2498 /** 2499 * @return {@link #action} (Sub actions.) 2500 */ 2501 public List<RequestGroupActionComponent> getAction() { 2502 if (this.action == null) 2503 this.action = new ArrayList<RequestGroupActionComponent>(); 2504 return this.action; 2505 } 2506 2507 /** 2508 * @return Returns a reference to <code>this</code> for easy method chaining 2509 */ 2510 public RequestGroupActionComponent setAction(List<RequestGroupActionComponent> theAction) { 2511 this.action = theAction; 2512 return this; 2513 } 2514 2515 public boolean hasAction() { 2516 if (this.action == null) 2517 return false; 2518 for (RequestGroupActionComponent item : this.action) 2519 if (!item.isEmpty()) 2520 return true; 2521 return false; 2522 } 2523 2524 public RequestGroupActionComponent addAction() { //3 2525 RequestGroupActionComponent t = new RequestGroupActionComponent(); 2526 if (this.action == null) 2527 this.action = new ArrayList<RequestGroupActionComponent>(); 2528 this.action.add(t); 2529 return t; 2530 } 2531 2532 public RequestGroupActionComponent addAction(RequestGroupActionComponent t) { //3 2533 if (t == null) 2534 return this; 2535 if (this.action == null) 2536 this.action = new ArrayList<RequestGroupActionComponent>(); 2537 this.action.add(t); 2538 return this; 2539 } 2540 2541 /** 2542 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 2543 */ 2544 public RequestGroupActionComponent getActionFirstRep() { 2545 if (getAction().isEmpty()) { 2546 addAction(); 2547 } 2548 return getAction().get(0); 2549 } 2550 2551 protected void listChildren(List<Property> children) { 2552 super.listChildren(children); 2553 children.add(new Property("label", "string", "A user-visible label for the action.", 0, 1, label)); 2554 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 2555 children.add(new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 2556 children.add(new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent)); 2557 children.add(new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code)); 2558 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 2559 children.add(new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition)); 2560 children.add(new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction)); 2561 children.add(new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing)); 2562 children.add(new Property("participant", "Reference(Patient|Person|Practitioner|RelatedPerson)", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant)); 2563 children.add(new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type)); 2564 children.add(new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 2565 children.add(new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 2566 children.add(new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior)); 2567 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior)); 2568 children.add(new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 2569 children.add(new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource)); 2570 children.add(new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action)); 2571 } 2572 2573 @Override 2574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2575 switch (_hash) { 2576 case 102727412: /*label*/ return new Property("label", "string", "A user-visible label for the action.", 0, 1, label); 2577 case 110371416: /*title*/ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 2578 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 2579 case -900391049: /*textEquivalent*/ return new Property("textEquivalent", "string", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that may not be capable of interpreting it dynamically.", 0, 1, textEquivalent); 2580 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a the section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code); 2581 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 2582 case -861311717: /*condition*/ return new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition); 2583 case -384107967: /*relatedAction*/ return new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction); 2584 case 164632566: /*timing[x]*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2585 case -873664438: /*timing*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2586 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2587 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2588 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2589 case -710871277: /*timingRange*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2590 case -497554124: /*timingTiming*/ return new Property("timing[x]", "dateTime|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 2591 case 767422259: /*participant*/ return new Property("participant", "Reference(Patient|Person|Practitioner|RelatedPerson)", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant); 2592 case 3575610: /*type*/ return new Property("type", "Coding", "The type of action to perform (create, update, remove).", 0, 1, type); 2593 case 586678389: /*groupingBehavior*/ return new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 2594 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 2595 case -1163906287: /*requiredBehavior*/ return new Property("requiredBehavior", "code", "Defines the requiredness behavior for the action.", 0, 1, requiredBehavior); 2596 case -1174249033: /*precheckBehavior*/ return new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 2597 case -922577408: /*cardinalityBehavior*/ return new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 2598 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource); 2599 case -1422950858: /*action*/ return new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action); 2600 default: return super.getNamedProperty(_hash, _name, _checkValid); 2601 } 2602 2603 } 2604 2605 @Override 2606 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2607 switch (hash) { 2608 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 2609 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2610 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2611 case -900391049: /*textEquivalent*/ return this.textEquivalent == null ? new Base[0] : new Base[] {this.textEquivalent}; // StringType 2612 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2613 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 2614 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // RequestGroupActionConditionComponent 2615 case -384107967: /*relatedAction*/ return this.relatedAction == null ? new Base[0] : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // RequestGroupActionRelatedActionComponent 2616 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 2617 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // Reference 2618 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 2619 case 586678389: /*groupingBehavior*/ return this.groupingBehavior == null ? new Base[0] : new Base[] {this.groupingBehavior}; // Enumeration<ActionGroupingBehavior> 2620 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<ActionSelectionBehavior> 2621 case -1163906287: /*requiredBehavior*/ return this.requiredBehavior == null ? new Base[0] : new Base[] {this.requiredBehavior}; // Enumeration<ActionRequiredBehavior> 2622 case -1174249033: /*precheckBehavior*/ return this.precheckBehavior == null ? new Base[0] : new Base[] {this.precheckBehavior}; // Enumeration<ActionPrecheckBehavior> 2623 case -922577408: /*cardinalityBehavior*/ return this.cardinalityBehavior == null ? new Base[0] : new Base[] {this.cardinalityBehavior}; // Enumeration<ActionCardinalityBehavior> 2624 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 2625 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 2626 default: return super.getProperty(hash, name, checkValid); 2627 } 2628 2629 } 2630 2631 @Override 2632 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2633 switch (hash) { 2634 case 102727412: // label 2635 this.label = castToString(value); // StringType 2636 return value; 2637 case 110371416: // title 2638 this.title = castToString(value); // StringType 2639 return value; 2640 case -1724546052: // description 2641 this.description = castToString(value); // StringType 2642 return value; 2643 case -900391049: // textEquivalent 2644 this.textEquivalent = castToString(value); // StringType 2645 return value; 2646 case 3059181: // code 2647 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 2648 return value; 2649 case 1587405498: // documentation 2650 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 2651 return value; 2652 case -861311717: // condition 2653 this.getCondition().add((RequestGroupActionConditionComponent) value); // RequestGroupActionConditionComponent 2654 return value; 2655 case -384107967: // relatedAction 2656 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); // RequestGroupActionRelatedActionComponent 2657 return value; 2658 case -873664438: // timing 2659 this.timing = castToType(value); // Type 2660 return value; 2661 case 767422259: // participant 2662 this.getParticipant().add(castToReference(value)); // Reference 2663 return value; 2664 case 3575610: // type 2665 this.type = castToCoding(value); // Coding 2666 return value; 2667 case 586678389: // groupingBehavior 2668 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 2669 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 2670 return value; 2671 case 168639486: // selectionBehavior 2672 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 2673 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 2674 return value; 2675 case -1163906287: // requiredBehavior 2676 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 2677 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 2678 return value; 2679 case -1174249033: // precheckBehavior 2680 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 2681 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 2682 return value; 2683 case -922577408: // cardinalityBehavior 2684 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 2685 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 2686 return value; 2687 case -341064690: // resource 2688 this.resource = castToReference(value); // Reference 2689 return value; 2690 case -1422950858: // action 2691 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 2692 return value; 2693 default: return super.setProperty(hash, name, value); 2694 } 2695 2696 } 2697 2698 @Override 2699 public Base setProperty(String name, Base value) throws FHIRException { 2700 if (name.equals("label")) { 2701 this.label = castToString(value); // StringType 2702 } else if (name.equals("title")) { 2703 this.title = castToString(value); // StringType 2704 } else if (name.equals("description")) { 2705 this.description = castToString(value); // StringType 2706 } else if (name.equals("textEquivalent")) { 2707 this.textEquivalent = castToString(value); // StringType 2708 } else if (name.equals("code")) { 2709 this.getCode().add(castToCodeableConcept(value)); 2710 } else if (name.equals("documentation")) { 2711 this.getDocumentation().add(castToRelatedArtifact(value)); 2712 } else if (name.equals("condition")) { 2713 this.getCondition().add((RequestGroupActionConditionComponent) value); 2714 } else if (name.equals("relatedAction")) { 2715 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); 2716 } else if (name.equals("timing[x]")) { 2717 this.timing = castToType(value); // Type 2718 } else if (name.equals("participant")) { 2719 this.getParticipant().add(castToReference(value)); 2720 } else if (name.equals("type")) { 2721 this.type = castToCoding(value); // Coding 2722 } else if (name.equals("groupingBehavior")) { 2723 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 2724 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 2725 } else if (name.equals("selectionBehavior")) { 2726 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 2727 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 2728 } else if (name.equals("requiredBehavior")) { 2729 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 2730 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 2731 } else if (name.equals("precheckBehavior")) { 2732 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 2733 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 2734 } else if (name.equals("cardinalityBehavior")) { 2735 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 2736 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 2737 } else if (name.equals("resource")) { 2738 this.resource = castToReference(value); // Reference 2739 } else if (name.equals("action")) { 2740 this.getAction().add((RequestGroupActionComponent) value); 2741 } else 2742 return super.setProperty(name, value); 2743 return value; 2744 } 2745 2746 @Override 2747 public Base makeProperty(int hash, String name) throws FHIRException { 2748 switch (hash) { 2749 case 102727412: return getLabelElement(); 2750 case 110371416: return getTitleElement(); 2751 case -1724546052: return getDescriptionElement(); 2752 case -900391049: return getTextEquivalentElement(); 2753 case 3059181: return addCode(); 2754 case 1587405498: return addDocumentation(); 2755 case -861311717: return addCondition(); 2756 case -384107967: return addRelatedAction(); 2757 case 164632566: return getTiming(); 2758 case -873664438: return getTiming(); 2759 case 767422259: return addParticipant(); 2760 case 3575610: return getType(); 2761 case 586678389: return getGroupingBehaviorElement(); 2762 case 168639486: return getSelectionBehaviorElement(); 2763 case -1163906287: return getRequiredBehaviorElement(); 2764 case -1174249033: return getPrecheckBehaviorElement(); 2765 case -922577408: return getCardinalityBehaviorElement(); 2766 case -341064690: return getResource(); 2767 case -1422950858: return addAction(); 2768 default: return super.makeProperty(hash, name); 2769 } 2770 2771 } 2772 2773 @Override 2774 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2775 switch (hash) { 2776 case 102727412: /*label*/ return new String[] {"string"}; 2777 case 110371416: /*title*/ return new String[] {"string"}; 2778 case -1724546052: /*description*/ return new String[] {"string"}; 2779 case -900391049: /*textEquivalent*/ return new String[] {"string"}; 2780 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2781 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 2782 case -861311717: /*condition*/ return new String[] {}; 2783 case -384107967: /*relatedAction*/ return new String[] {}; 2784 case -873664438: /*timing*/ return new String[] {"dateTime", "Period", "Duration", "Range", "Timing"}; 2785 case 767422259: /*participant*/ return new String[] {"Reference"}; 2786 case 3575610: /*type*/ return new String[] {"Coding"}; 2787 case 586678389: /*groupingBehavior*/ return new String[] {"code"}; 2788 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 2789 case -1163906287: /*requiredBehavior*/ return new String[] {"code"}; 2790 case -1174249033: /*precheckBehavior*/ return new String[] {"code"}; 2791 case -922577408: /*cardinalityBehavior*/ return new String[] {"code"}; 2792 case -341064690: /*resource*/ return new String[] {"Reference"}; 2793 case -1422950858: /*action*/ return new String[] {"@RequestGroup.action"}; 2794 default: return super.getTypesForProperty(hash, name); 2795 } 2796 2797 } 2798 2799 @Override 2800 public Base addChild(String name) throws FHIRException { 2801 if (name.equals("label")) { 2802 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.label"); 2803 } 2804 else if (name.equals("title")) { 2805 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.title"); 2806 } 2807 else if (name.equals("description")) { 2808 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 2809 } 2810 else if (name.equals("textEquivalent")) { 2811 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.textEquivalent"); 2812 } 2813 else if (name.equals("code")) { 2814 return addCode(); 2815 } 2816 else if (name.equals("documentation")) { 2817 return addDocumentation(); 2818 } 2819 else if (name.equals("condition")) { 2820 return addCondition(); 2821 } 2822 else if (name.equals("relatedAction")) { 2823 return addRelatedAction(); 2824 } 2825 else if (name.equals("timingDateTime")) { 2826 this.timing = new DateTimeType(); 2827 return this.timing; 2828 } 2829 else if (name.equals("timingPeriod")) { 2830 this.timing = new Period(); 2831 return this.timing; 2832 } 2833 else if (name.equals("timingDuration")) { 2834 this.timing = new Duration(); 2835 return this.timing; 2836 } 2837 else if (name.equals("timingRange")) { 2838 this.timing = new Range(); 2839 return this.timing; 2840 } 2841 else if (name.equals("timingTiming")) { 2842 this.timing = new Timing(); 2843 return this.timing; 2844 } 2845 else if (name.equals("participant")) { 2846 return addParticipant(); 2847 } 2848 else if (name.equals("type")) { 2849 this.type = new Coding(); 2850 return this.type; 2851 } 2852 else if (name.equals("groupingBehavior")) { 2853 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.groupingBehavior"); 2854 } 2855 else if (name.equals("selectionBehavior")) { 2856 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.selectionBehavior"); 2857 } 2858 else if (name.equals("requiredBehavior")) { 2859 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.requiredBehavior"); 2860 } 2861 else if (name.equals("precheckBehavior")) { 2862 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.precheckBehavior"); 2863 } 2864 else if (name.equals("cardinalityBehavior")) { 2865 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.cardinalityBehavior"); 2866 } 2867 else if (name.equals("resource")) { 2868 this.resource = new Reference(); 2869 return this.resource; 2870 } 2871 else if (name.equals("action")) { 2872 return addAction(); 2873 } 2874 else 2875 return super.addChild(name); 2876 } 2877 2878 public RequestGroupActionComponent copy() { 2879 RequestGroupActionComponent dst = new RequestGroupActionComponent(); 2880 copyValues(dst); 2881 dst.label = label == null ? null : label.copy(); 2882 dst.title = title == null ? null : title.copy(); 2883 dst.description = description == null ? null : description.copy(); 2884 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 2885 if (code != null) { 2886 dst.code = new ArrayList<CodeableConcept>(); 2887 for (CodeableConcept i : code) 2888 dst.code.add(i.copy()); 2889 }; 2890 if (documentation != null) { 2891 dst.documentation = new ArrayList<RelatedArtifact>(); 2892 for (RelatedArtifact i : documentation) 2893 dst.documentation.add(i.copy()); 2894 }; 2895 if (condition != null) { 2896 dst.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2897 for (RequestGroupActionConditionComponent i : condition) 2898 dst.condition.add(i.copy()); 2899 }; 2900 if (relatedAction != null) { 2901 dst.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2902 for (RequestGroupActionRelatedActionComponent i : relatedAction) 2903 dst.relatedAction.add(i.copy()); 2904 }; 2905 dst.timing = timing == null ? null : timing.copy(); 2906 if (participant != null) { 2907 dst.participant = new ArrayList<Reference>(); 2908 for (Reference i : participant) 2909 dst.participant.add(i.copy()); 2910 }; 2911 dst.type = type == null ? null : type.copy(); 2912 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 2913 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 2914 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 2915 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 2916 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 2917 dst.resource = resource == null ? null : resource.copy(); 2918 if (action != null) { 2919 dst.action = new ArrayList<RequestGroupActionComponent>(); 2920 for (RequestGroupActionComponent i : action) 2921 dst.action.add(i.copy()); 2922 }; 2923 return dst; 2924 } 2925 2926 @Override 2927 public boolean equalsDeep(Base other_) { 2928 if (!super.equalsDeep(other_)) 2929 return false; 2930 if (!(other_ instanceof RequestGroupActionComponent)) 2931 return false; 2932 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 2933 return compareDeep(label, o.label, true) && compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 2934 && compareDeep(textEquivalent, o.textEquivalent, true) && compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true) 2935 && compareDeep(condition, o.condition, true) && compareDeep(relatedAction, o.relatedAction, true) 2936 && compareDeep(timing, o.timing, true) && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 2937 && compareDeep(groupingBehavior, o.groupingBehavior, true) && compareDeep(selectionBehavior, o.selectionBehavior, true) 2938 && compareDeep(requiredBehavior, o.requiredBehavior, true) && compareDeep(precheckBehavior, o.precheckBehavior, true) 2939 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(resource, o.resource, true) 2940 && compareDeep(action, o.action, true); 2941 } 2942 2943 @Override 2944 public boolean equalsShallow(Base other_) { 2945 if (!super.equalsShallow(other_)) 2946 return false; 2947 if (!(other_ instanceof RequestGroupActionComponent)) 2948 return false; 2949 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 2950 return compareValues(label, o.label, true) && compareValues(title, o.title, true) && compareValues(description, o.description, true) 2951 && compareValues(textEquivalent, o.textEquivalent, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 2952 && compareValues(selectionBehavior, o.selectionBehavior, true) && compareValues(requiredBehavior, o.requiredBehavior, true) 2953 && compareValues(precheckBehavior, o.precheckBehavior, true) && compareValues(cardinalityBehavior, o.cardinalityBehavior, true) 2954 ; 2955 } 2956 2957 public boolean isEmpty() { 2958 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, title, description 2959 , textEquivalent, code, documentation, condition, relatedAction, timing, participant 2960 , type, groupingBehavior, selectionBehavior, requiredBehavior, precheckBehavior, cardinalityBehavior 2961 , resource, action); 2962 } 2963 2964 public String fhirType() { 2965 return "RequestGroup.action"; 2966 2967 } 2968 2969 } 2970 2971 @Block() 2972 public static class RequestGroupActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 2973 /** 2974 * The kind of condition. 2975 */ 2976 @Child(name = "kind", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2977 @Description(shortDefinition="applicability | start | stop", formalDefinition="The kind of condition." ) 2978 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-condition-kind") 2979 protected Enumeration<ActionConditionKind> kind; 2980 2981 /** 2982 * A brief, natural language description of the condition that effectively communicates the intended semantics. 2983 */ 2984 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2985 @Description(shortDefinition="Natural language description of the condition", formalDefinition="A brief, natural language description of the condition that effectively communicates the intended semantics." ) 2986 protected StringType description; 2987 2988 /** 2989 * The media type of the language for the expression. 2990 */ 2991 @Child(name = "language", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2992 @Description(shortDefinition="Language of the expression", formalDefinition="The media type of the language for the expression." ) 2993 protected StringType language; 2994 2995 /** 2996 * An expression that returns true or false, indicating whether or not the condition is satisfied. 2997 */ 2998 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2999 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether or not the condition is satisfied." ) 3000 protected StringType expression; 3001 3002 private static final long serialVersionUID = 944300105L; 3003 3004 /** 3005 * Constructor 3006 */ 3007 public RequestGroupActionConditionComponent() { 3008 super(); 3009 } 3010 3011 /** 3012 * Constructor 3013 */ 3014 public RequestGroupActionConditionComponent(Enumeration<ActionConditionKind> kind) { 3015 super(); 3016 this.kind = kind; 3017 } 3018 3019 /** 3020 * @return {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 3021 */ 3022 public Enumeration<ActionConditionKind> getKindElement() { 3023 if (this.kind == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.kind"); 3026 else if (Configuration.doAutoCreate()) 3027 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 3028 return this.kind; 3029 } 3030 3031 public boolean hasKindElement() { 3032 return this.kind != null && !this.kind.isEmpty(); 3033 } 3034 3035 public boolean hasKind() { 3036 return this.kind != null && !this.kind.isEmpty(); 3037 } 3038 3039 /** 3040 * @param value {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 3041 */ 3042 public RequestGroupActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 3043 this.kind = value; 3044 return this; 3045 } 3046 3047 /** 3048 * @return The kind of condition. 3049 */ 3050 public ActionConditionKind getKind() { 3051 return this.kind == null ? null : this.kind.getValue(); 3052 } 3053 3054 /** 3055 * @param value The kind of condition. 3056 */ 3057 public RequestGroupActionConditionComponent setKind(ActionConditionKind value) { 3058 if (this.kind == null) 3059 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 3060 this.kind.setValue(value); 3061 return this; 3062 } 3063 3064 /** 3065 * @return {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3066 */ 3067 public StringType getDescriptionElement() { 3068 if (this.description == null) 3069 if (Configuration.errorOnAutoCreate()) 3070 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.description"); 3071 else if (Configuration.doAutoCreate()) 3072 this.description = new StringType(); // bb 3073 return this.description; 3074 } 3075 3076 public boolean hasDescriptionElement() { 3077 return this.description != null && !this.description.isEmpty(); 3078 } 3079 3080 public boolean hasDescription() { 3081 return this.description != null && !this.description.isEmpty(); 3082 } 3083 3084 /** 3085 * @param value {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3086 */ 3087 public RequestGroupActionConditionComponent setDescriptionElement(StringType value) { 3088 this.description = value; 3089 return this; 3090 } 3091 3092 /** 3093 * @return A brief, natural language description of the condition that effectively communicates the intended semantics. 3094 */ 3095 public String getDescription() { 3096 return this.description == null ? null : this.description.getValue(); 3097 } 3098 3099 /** 3100 * @param value A brief, natural language description of the condition that effectively communicates the intended semantics. 3101 */ 3102 public RequestGroupActionConditionComponent setDescription(String value) { 3103 if (Utilities.noString(value)) 3104 this.description = null; 3105 else { 3106 if (this.description == null) 3107 this.description = new StringType(); 3108 this.description.setValue(value); 3109 } 3110 return this; 3111 } 3112 3113 /** 3114 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3115 */ 3116 public StringType getLanguageElement() { 3117 if (this.language == null) 3118 if (Configuration.errorOnAutoCreate()) 3119 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.language"); 3120 else if (Configuration.doAutoCreate()) 3121 this.language = new StringType(); // bb 3122 return this.language; 3123 } 3124 3125 public boolean hasLanguageElement() { 3126 return this.language != null && !this.language.isEmpty(); 3127 } 3128 3129 public boolean hasLanguage() { 3130 return this.language != null && !this.language.isEmpty(); 3131 } 3132 3133 /** 3134 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3135 */ 3136 public RequestGroupActionConditionComponent setLanguageElement(StringType value) { 3137 this.language = value; 3138 return this; 3139 } 3140 3141 /** 3142 * @return The media type of the language for the expression. 3143 */ 3144 public String getLanguage() { 3145 return this.language == null ? null : this.language.getValue(); 3146 } 3147 3148 /** 3149 * @param value The media type of the language for the expression. 3150 */ 3151 public RequestGroupActionConditionComponent setLanguage(String value) { 3152 if (Utilities.noString(value)) 3153 this.language = null; 3154 else { 3155 if (this.language == null) 3156 this.language = new StringType(); 3157 this.language.setValue(value); 3158 } 3159 return this; 3160 } 3161 3162 /** 3163 * @return {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3164 */ 3165 public StringType getExpressionElement() { 3166 if (this.expression == null) 3167 if (Configuration.errorOnAutoCreate()) 3168 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.expression"); 3169 else if (Configuration.doAutoCreate()) 3170 this.expression = new StringType(); // bb 3171 return this.expression; 3172 } 3173 3174 public boolean hasExpressionElement() { 3175 return this.expression != null && !this.expression.isEmpty(); 3176 } 3177 3178 public boolean hasExpression() { 3179 return this.expression != null && !this.expression.isEmpty(); 3180 } 3181 3182 /** 3183 * @param value {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3184 */ 3185 public RequestGroupActionConditionComponent setExpressionElement(StringType value) { 3186 this.expression = value; 3187 return this; 3188 } 3189 3190 /** 3191 * @return An expression that returns true or false, indicating whether or not the condition is satisfied. 3192 */ 3193 public String getExpression() { 3194 return this.expression == null ? null : this.expression.getValue(); 3195 } 3196 3197 /** 3198 * @param value An expression that returns true or false, indicating whether or not the condition is satisfied. 3199 */ 3200 public RequestGroupActionConditionComponent setExpression(String value) { 3201 if (Utilities.noString(value)) 3202 this.expression = null; 3203 else { 3204 if (this.expression == null) 3205 this.expression = new StringType(); 3206 this.expression.setValue(value); 3207 } 3208 return this; 3209 } 3210 3211 protected void listChildren(List<Property> children) { 3212 super.listChildren(children); 3213 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 3214 children.add(new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description)); 3215 children.add(new Property("language", "string", "The media type of the language for the expression.", 0, 1, language)); 3216 children.add(new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression)); 3217 } 3218 3219 @Override 3220 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3221 switch (_hash) { 3222 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 3223 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description); 3224 case -1613589672: /*language*/ return new Property("language", "string", "The media type of the language for the expression.", 0, 1, language); 3225 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression); 3226 default: return super.getNamedProperty(_hash, _name, _checkValid); 3227 } 3228 3229 } 3230 3231 @Override 3232 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3233 switch (hash) { 3234 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActionConditionKind> 3235 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3236 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // StringType 3237 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 3238 default: return super.getProperty(hash, name, checkValid); 3239 } 3240 3241 } 3242 3243 @Override 3244 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3245 switch (hash) { 3246 case 3292052: // kind 3247 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3248 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3249 return value; 3250 case -1724546052: // description 3251 this.description = castToString(value); // StringType 3252 return value; 3253 case -1613589672: // language 3254 this.language = castToString(value); // StringType 3255 return value; 3256 case -1795452264: // expression 3257 this.expression = castToString(value); // StringType 3258 return value; 3259 default: return super.setProperty(hash, name, value); 3260 } 3261 3262 } 3263 3264 @Override 3265 public Base setProperty(String name, Base value) throws FHIRException { 3266 if (name.equals("kind")) { 3267 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3268 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3269 } else if (name.equals("description")) { 3270 this.description = castToString(value); // StringType 3271 } else if (name.equals("language")) { 3272 this.language = castToString(value); // StringType 3273 } else if (name.equals("expression")) { 3274 this.expression = castToString(value); // StringType 3275 } else 3276 return super.setProperty(name, value); 3277 return value; 3278 } 3279 3280 @Override 3281 public Base makeProperty(int hash, String name) throws FHIRException { 3282 switch (hash) { 3283 case 3292052: return getKindElement(); 3284 case -1724546052: return getDescriptionElement(); 3285 case -1613589672: return getLanguageElement(); 3286 case -1795452264: return getExpressionElement(); 3287 default: return super.makeProperty(hash, name); 3288 } 3289 3290 } 3291 3292 @Override 3293 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3294 switch (hash) { 3295 case 3292052: /*kind*/ return new String[] {"code"}; 3296 case -1724546052: /*description*/ return new String[] {"string"}; 3297 case -1613589672: /*language*/ return new String[] {"string"}; 3298 case -1795452264: /*expression*/ return new String[] {"string"}; 3299 default: return super.getTypesForProperty(hash, name); 3300 } 3301 3302 } 3303 3304 @Override 3305 public Base addChild(String name) throws FHIRException { 3306 if (name.equals("kind")) { 3307 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.kind"); 3308 } 3309 else if (name.equals("description")) { 3310 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 3311 } 3312 else if (name.equals("language")) { 3313 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.language"); 3314 } 3315 else if (name.equals("expression")) { 3316 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.expression"); 3317 } 3318 else 3319 return super.addChild(name); 3320 } 3321 3322 public RequestGroupActionConditionComponent copy() { 3323 RequestGroupActionConditionComponent dst = new RequestGroupActionConditionComponent(); 3324 copyValues(dst); 3325 dst.kind = kind == null ? null : kind.copy(); 3326 dst.description = description == null ? null : description.copy(); 3327 dst.language = language == null ? null : language.copy(); 3328 dst.expression = expression == null ? null : expression.copy(); 3329 return dst; 3330 } 3331 3332 @Override 3333 public boolean equalsDeep(Base other_) { 3334 if (!super.equalsDeep(other_)) 3335 return false; 3336 if (!(other_ instanceof RequestGroupActionConditionComponent)) 3337 return false; 3338 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 3339 return compareDeep(kind, o.kind, true) && compareDeep(description, o.description, true) && compareDeep(language, o.language, true) 3340 && compareDeep(expression, o.expression, true); 3341 } 3342 3343 @Override 3344 public boolean equalsShallow(Base other_) { 3345 if (!super.equalsShallow(other_)) 3346 return false; 3347 if (!(other_ instanceof RequestGroupActionConditionComponent)) 3348 return false; 3349 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 3350 return compareValues(kind, o.kind, true) && compareValues(description, o.description, true) && compareValues(language, o.language, true) 3351 && compareValues(expression, o.expression, true); 3352 } 3353 3354 public boolean isEmpty() { 3355 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, description, language 3356 , expression); 3357 } 3358 3359 public String fhirType() { 3360 return "RequestGroup.action.condition"; 3361 3362 } 3363 3364 } 3365 3366 @Block() 3367 public static class RequestGroupActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 3368 /** 3369 * The element id of the action this is related to. 3370 */ 3371 @Child(name = "actionId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3372 @Description(shortDefinition="What action this is related to", formalDefinition="The element id of the action this is related to." ) 3373 protected IdType actionId; 3374 3375 /** 3376 * The relationship of this action to the related action. 3377 */ 3378 @Child(name = "relationship", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 3379 @Description(shortDefinition="before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition="The relationship of this action to the related action." ) 3380 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 3381 protected Enumeration<ActionRelationshipType> relationship; 3382 3383 /** 3384 * A duration or range of durations to apply to the relationship. For example, 30-60 minutes before. 3385 */ 3386 @Child(name = "offset", type = {Duration.class, Range.class}, order=3, min=0, max=1, modifier=false, summary=false) 3387 @Description(shortDefinition="Time offset for the relationship", formalDefinition="A duration or range of durations to apply to the relationship. For example, 30-60 minutes before." ) 3388 protected Type offset; 3389 3390 private static final long serialVersionUID = 1063306770L; 3391 3392 /** 3393 * Constructor 3394 */ 3395 public RequestGroupActionRelatedActionComponent() { 3396 super(); 3397 } 3398 3399 /** 3400 * Constructor 3401 */ 3402 public RequestGroupActionRelatedActionComponent(IdType actionId, Enumeration<ActionRelationshipType> relationship) { 3403 super(); 3404 this.actionId = actionId; 3405 this.relationship = relationship; 3406 } 3407 3408 /** 3409 * @return {@link #actionId} (The element id of the action this is related to.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 3410 */ 3411 public IdType getActionIdElement() { 3412 if (this.actionId == null) 3413 if (Configuration.errorOnAutoCreate()) 3414 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.actionId"); 3415 else if (Configuration.doAutoCreate()) 3416 this.actionId = new IdType(); // bb 3417 return this.actionId; 3418 } 3419 3420 public boolean hasActionIdElement() { 3421 return this.actionId != null && !this.actionId.isEmpty(); 3422 } 3423 3424 public boolean hasActionId() { 3425 return this.actionId != null && !this.actionId.isEmpty(); 3426 } 3427 3428 /** 3429 * @param value {@link #actionId} (The element id of the action this is related to.). This is the underlying object with id, value and extensions. The accessor "getActionId" gives direct access to the value 3430 */ 3431 public RequestGroupActionRelatedActionComponent setActionIdElement(IdType value) { 3432 this.actionId = value; 3433 return this; 3434 } 3435 3436 /** 3437 * @return The element id of the action this is related to. 3438 */ 3439 public String getActionId() { 3440 return this.actionId == null ? null : this.actionId.getValue(); 3441 } 3442 3443 /** 3444 * @param value The element id of the action this is related to. 3445 */ 3446 public RequestGroupActionRelatedActionComponent setActionId(String value) { 3447 if (this.actionId == null) 3448 this.actionId = new IdType(); 3449 this.actionId.setValue(value); 3450 return this; 3451 } 3452 3453 /** 3454 * @return {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3455 */ 3456 public Enumeration<ActionRelationshipType> getRelationshipElement() { 3457 if (this.relationship == null) 3458 if (Configuration.errorOnAutoCreate()) 3459 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.relationship"); 3460 else if (Configuration.doAutoCreate()) 3461 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 3462 return this.relationship; 3463 } 3464 3465 public boolean hasRelationshipElement() { 3466 return this.relationship != null && !this.relationship.isEmpty(); 3467 } 3468 3469 public boolean hasRelationship() { 3470 return this.relationship != null && !this.relationship.isEmpty(); 3471 } 3472 3473 /** 3474 * @param value {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3475 */ 3476 public RequestGroupActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 3477 this.relationship = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return The relationship of this action to the related action. 3483 */ 3484 public ActionRelationshipType getRelationship() { 3485 return this.relationship == null ? null : this.relationship.getValue(); 3486 } 3487 3488 /** 3489 * @param value The relationship of this action to the related action. 3490 */ 3491 public RequestGroupActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 3492 if (this.relationship == null) 3493 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 3494 this.relationship.setValue(value); 3495 return this; 3496 } 3497 3498 /** 3499 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3500 */ 3501 public Type getOffset() { 3502 return this.offset; 3503 } 3504 3505 /** 3506 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3507 */ 3508 public Duration getOffsetDuration() throws FHIRException { 3509 if (this.offset == null) 3510 return null; 3511 if (!(this.offset instanceof Duration)) 3512 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.offset.getClass().getName()+" was encountered"); 3513 return (Duration) this.offset; 3514 } 3515 3516 public boolean hasOffsetDuration() { 3517 return this != null && this.offset instanceof Duration; 3518 } 3519 3520 /** 3521 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3522 */ 3523 public Range getOffsetRange() throws FHIRException { 3524 if (this.offset == null) 3525 return null; 3526 if (!(this.offset instanceof Range)) 3527 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.offset.getClass().getName()+" was encountered"); 3528 return (Range) this.offset; 3529 } 3530 3531 public boolean hasOffsetRange() { 3532 return this != null && this.offset instanceof Range; 3533 } 3534 3535 public boolean hasOffset() { 3536 return this.offset != null && !this.offset.isEmpty(); 3537 } 3538 3539 /** 3540 * @param value {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3541 */ 3542 public RequestGroupActionRelatedActionComponent setOffset(Type value) throws FHIRFormatError { 3543 if (value != null && !(value instanceof Duration || value instanceof Range)) 3544 throw new FHIRFormatError("Not the right type for RequestGroup.action.relatedAction.offset[x]: "+value.fhirType()); 3545 this.offset = value; 3546 return this; 3547 } 3548 3549 protected void listChildren(List<Property> children) { 3550 super.listChildren(children); 3551 children.add(new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId)); 3552 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship)); 3553 children.add(new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset)); 3554 } 3555 3556 @Override 3557 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3558 switch (_hash) { 3559 case -1656172047: /*actionId*/ return new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId); 3560 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship); 3561 case -1960684787: /*offset[x]*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3562 case -1019779949: /*offset*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3563 case 134075207: /*offsetDuration*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3564 case 1263585386: /*offsetRange*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3565 default: return super.getNamedProperty(_hash, _name, _checkValid); 3566 } 3567 3568 } 3569 3570 @Override 3571 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3572 switch (hash) { 3573 case -1656172047: /*actionId*/ return this.actionId == null ? new Base[0] : new Base[] {this.actionId}; // IdType 3574 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ActionRelationshipType> 3575 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // Type 3576 default: return super.getProperty(hash, name, checkValid); 3577 } 3578 3579 } 3580 3581 @Override 3582 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3583 switch (hash) { 3584 case -1656172047: // actionId 3585 this.actionId = castToId(value); // IdType 3586 return value; 3587 case -261851592: // relationship 3588 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 3589 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3590 return value; 3591 case -1019779949: // offset 3592 this.offset = castToType(value); // Type 3593 return value; 3594 default: return super.setProperty(hash, name, value); 3595 } 3596 3597 } 3598 3599 @Override 3600 public Base setProperty(String name, Base value) throws FHIRException { 3601 if (name.equals("actionId")) { 3602 this.actionId = castToId(value); // IdType 3603 } else if (name.equals("relationship")) { 3604 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 3605 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3606 } else if (name.equals("offset[x]")) { 3607 this.offset = castToType(value); // Type 3608 } else 3609 return super.setProperty(name, value); 3610 return value; 3611 } 3612 3613 @Override 3614 public Base makeProperty(int hash, String name) throws FHIRException { 3615 switch (hash) { 3616 case -1656172047: return getActionIdElement(); 3617 case -261851592: return getRelationshipElement(); 3618 case -1960684787: return getOffset(); 3619 case -1019779949: return getOffset(); 3620 default: return super.makeProperty(hash, name); 3621 } 3622 3623 } 3624 3625 @Override 3626 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3627 switch (hash) { 3628 case -1656172047: /*actionId*/ return new String[] {"id"}; 3629 case -261851592: /*relationship*/ return new String[] {"code"}; 3630 case -1019779949: /*offset*/ return new String[] {"Duration", "Range"}; 3631 default: return super.getTypesForProperty(hash, name); 3632 } 3633 3634 } 3635 3636 @Override 3637 public Base addChild(String name) throws FHIRException { 3638 if (name.equals("actionId")) { 3639 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.actionId"); 3640 } 3641 else if (name.equals("relationship")) { 3642 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.relationship"); 3643 } 3644 else if (name.equals("offsetDuration")) { 3645 this.offset = new Duration(); 3646 return this.offset; 3647 } 3648 else if (name.equals("offsetRange")) { 3649 this.offset = new Range(); 3650 return this.offset; 3651 } 3652 else 3653 return super.addChild(name); 3654 } 3655 3656 public RequestGroupActionRelatedActionComponent copy() { 3657 RequestGroupActionRelatedActionComponent dst = new RequestGroupActionRelatedActionComponent(); 3658 copyValues(dst); 3659 dst.actionId = actionId == null ? null : actionId.copy(); 3660 dst.relationship = relationship == null ? null : relationship.copy(); 3661 dst.offset = offset == null ? null : offset.copy(); 3662 return dst; 3663 } 3664 3665 @Override 3666 public boolean equalsDeep(Base other_) { 3667 if (!super.equalsDeep(other_)) 3668 return false; 3669 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 3670 return false; 3671 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 3672 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 3673 && compareDeep(offset, o.offset, true); 3674 } 3675 3676 @Override 3677 public boolean equalsShallow(Base other_) { 3678 if (!super.equalsShallow(other_)) 3679 return false; 3680 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 3681 return false; 3682 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 3683 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true) 3684 ; 3685 } 3686 3687 public boolean isEmpty() { 3688 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset 3689 ); 3690 } 3691 3692 public String fhirType() { 3693 return "RequestGroup.action.relatedAction"; 3694 3695 } 3696 3697 } 3698 3699 /** 3700 * Allows a service to provide a unique, business identifier for the request. 3701 */ 3702 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3703 @Description(shortDefinition="Business identifier", formalDefinition="Allows a service to provide a unique, business identifier for the request." ) 3704 protected List<Identifier> identifier; 3705 3706 /** 3707 * A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request. 3708 */ 3709 @Child(name = "definition", type = {Reference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3710 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request." ) 3711 protected List<Reference> definition; 3712 /** 3713 * The actual objects that are the target of the reference (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 3714 */ 3715 protected List<Resource> definitionTarget; 3716 3717 3718 /** 3719 * A plan, proposal or order that is fulfilled in whole or in part by this request. 3720 */ 3721 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3722 @Description(shortDefinition="Fulfills plan, proposal, or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this request." ) 3723 protected List<Reference> basedOn; 3724 /** 3725 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this request.) 3726 */ 3727 protected List<Resource> basedOnTarget; 3728 3729 3730 /** 3731 * Completed or terminated request(s) whose function is taken by this new request. 3732 */ 3733 @Child(name = "replaces", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3734 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 3735 protected List<Reference> replaces; 3736 /** 3737 * The actual objects that are the target of the reference (Completed or terminated request(s) whose function is taken by this new request.) 3738 */ 3739 protected List<Resource> replacesTarget; 3740 3741 3742 /** 3743 * A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form. 3744 */ 3745 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 3746 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form." ) 3747 protected Identifier groupIdentifier; 3748 3749 /** 3750 * The current state of the request. For request groups, the status reflects the status of all the requests in the group. 3751 */ 3752 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 3753 @Description(shortDefinition="draft | active | suspended | cancelled | completed | entered-in-error | unknown", formalDefinition="The current state of the request. For request groups, the status reflects the status of all the requests in the group." ) 3754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 3755 protected Enumeration<RequestStatus> status; 3756 3757 /** 3758 * Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 3759 */ 3760 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3761 @Description(shortDefinition="proposal | plan | order", formalDefinition="Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain." ) 3762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 3763 protected Enumeration<RequestIntent> intent; 3764 3765 /** 3766 * Indicates how quickly the request should be addressed with respect to other requests. 3767 */ 3768 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3769 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the request should be addressed with respect to other requests." ) 3770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 3771 protected Enumeration<RequestPriority> priority; 3772 3773 /** 3774 * The subject for which the request group was created. 3775 */ 3776 @Child(name = "subject", type = {Patient.class, Group.class}, order=8, min=0, max=1, modifier=false, summary=false) 3777 @Description(shortDefinition="Who the request group is about", formalDefinition="The subject for which the request group was created." ) 3778 protected Reference subject; 3779 3780 /** 3781 * The actual object that is the target of the reference (The subject for which the request group was created.) 3782 */ 3783 protected Resource subjectTarget; 3784 3785 /** 3786 * Describes the context of the request group, if any. 3787 */ 3788 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=9, min=0, max=1, modifier=false, summary=false) 3789 @Description(shortDefinition="Encounter or Episode for the request group", formalDefinition="Describes the context of the request group, if any." ) 3790 protected Reference context; 3791 3792 /** 3793 * The actual object that is the target of the reference (Describes the context of the request group, if any.) 3794 */ 3795 protected Resource contextTarget; 3796 3797 /** 3798 * Indicates when the request group was created. 3799 */ 3800 @Child(name = "authoredOn", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 3801 @Description(shortDefinition="When the request group was authored", formalDefinition="Indicates when the request group was created." ) 3802 protected DateTimeType authoredOn; 3803 3804 /** 3805 * Provides a reference to the author of the request group. 3806 */ 3807 @Child(name = "author", type = {Device.class, Practitioner.class}, order=11, min=0, max=1, modifier=false, summary=false) 3808 @Description(shortDefinition="Device or practitioner that authored the request group", formalDefinition="Provides a reference to the author of the request group." ) 3809 protected Reference author; 3810 3811 /** 3812 * The actual object that is the target of the reference (Provides a reference to the author of the request group.) 3813 */ 3814 protected Resource authorTarget; 3815 3816 /** 3817 * Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response. 3818 */ 3819 @Child(name = "reason", type = {CodeableConcept.class, Reference.class}, order=12, min=0, max=1, modifier=false, summary=false) 3820 @Description(shortDefinition="Reason for the request group", formalDefinition="Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response." ) 3821 protected Type reason; 3822 3823 /** 3824 * Provides a mechanism to communicate additional information about the response. 3825 */ 3826 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3827 @Description(shortDefinition="Additional notes about the response", formalDefinition="Provides a mechanism to communicate additional information about the response." ) 3828 protected List<Annotation> note; 3829 3830 /** 3831 * The actions, if any, produced by the evaluation of the artifact. 3832 */ 3833 @Child(name = "action", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3834 @Description(shortDefinition="Proposed actions, if any", formalDefinition="The actions, if any, produced by the evaluation of the artifact." ) 3835 protected List<RequestGroupActionComponent> action; 3836 3837 private static final long serialVersionUID = -1812083587L; 3838 3839 /** 3840 * Constructor 3841 */ 3842 public RequestGroup() { 3843 super(); 3844 } 3845 3846 /** 3847 * Constructor 3848 */ 3849 public RequestGroup(Enumeration<RequestStatus> status, Enumeration<RequestIntent> intent) { 3850 super(); 3851 this.status = status; 3852 this.intent = intent; 3853 } 3854 3855 /** 3856 * @return {@link #identifier} (Allows a service to provide a unique, business identifier for the request.) 3857 */ 3858 public List<Identifier> getIdentifier() { 3859 if (this.identifier == null) 3860 this.identifier = new ArrayList<Identifier>(); 3861 return this.identifier; 3862 } 3863 3864 /** 3865 * @return Returns a reference to <code>this</code> for easy method chaining 3866 */ 3867 public RequestGroup setIdentifier(List<Identifier> theIdentifier) { 3868 this.identifier = theIdentifier; 3869 return this; 3870 } 3871 3872 public boolean hasIdentifier() { 3873 if (this.identifier == null) 3874 return false; 3875 for (Identifier item : this.identifier) 3876 if (!item.isEmpty()) 3877 return true; 3878 return false; 3879 } 3880 3881 public Identifier addIdentifier() { //3 3882 Identifier t = new Identifier(); 3883 if (this.identifier == null) 3884 this.identifier = new ArrayList<Identifier>(); 3885 this.identifier.add(t); 3886 return t; 3887 } 3888 3889 public RequestGroup addIdentifier(Identifier t) { //3 3890 if (t == null) 3891 return this; 3892 if (this.identifier == null) 3893 this.identifier = new ArrayList<Identifier>(); 3894 this.identifier.add(t); 3895 return this; 3896 } 3897 3898 /** 3899 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3900 */ 3901 public Identifier getIdentifierFirstRep() { 3902 if (getIdentifier().isEmpty()) { 3903 addIdentifier(); 3904 } 3905 return getIdentifier().get(0); 3906 } 3907 3908 /** 3909 * @return {@link #definition} (A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 3910 */ 3911 public List<Reference> getDefinition() { 3912 if (this.definition == null) 3913 this.definition = new ArrayList<Reference>(); 3914 return this.definition; 3915 } 3916 3917 /** 3918 * @return Returns a reference to <code>this</code> for easy method chaining 3919 */ 3920 public RequestGroup setDefinition(List<Reference> theDefinition) { 3921 this.definition = theDefinition; 3922 return this; 3923 } 3924 3925 public boolean hasDefinition() { 3926 if (this.definition == null) 3927 return false; 3928 for (Reference item : this.definition) 3929 if (!item.isEmpty()) 3930 return true; 3931 return false; 3932 } 3933 3934 public Reference addDefinition() { //3 3935 Reference t = new Reference(); 3936 if (this.definition == null) 3937 this.definition = new ArrayList<Reference>(); 3938 this.definition.add(t); 3939 return t; 3940 } 3941 3942 public RequestGroup addDefinition(Reference t) { //3 3943 if (t == null) 3944 return this; 3945 if (this.definition == null) 3946 this.definition = new ArrayList<Reference>(); 3947 this.definition.add(t); 3948 return this; 3949 } 3950 3951 /** 3952 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 3953 */ 3954 public Reference getDefinitionFirstRep() { 3955 if (getDefinition().isEmpty()) { 3956 addDefinition(); 3957 } 3958 return getDefinition().get(0); 3959 } 3960 3961 /** 3962 * @deprecated Use Reference#setResource(IBaseResource) instead 3963 */ 3964 @Deprecated 3965 public List<Resource> getDefinitionTarget() { 3966 if (this.definitionTarget == null) 3967 this.definitionTarget = new ArrayList<Resource>(); 3968 return this.definitionTarget; 3969 } 3970 3971 /** 3972 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this request.) 3973 */ 3974 public List<Reference> getBasedOn() { 3975 if (this.basedOn == null) 3976 this.basedOn = new ArrayList<Reference>(); 3977 return this.basedOn; 3978 } 3979 3980 /** 3981 * @return Returns a reference to <code>this</code> for easy method chaining 3982 */ 3983 public RequestGroup setBasedOn(List<Reference> theBasedOn) { 3984 this.basedOn = theBasedOn; 3985 return this; 3986 } 3987 3988 public boolean hasBasedOn() { 3989 if (this.basedOn == null) 3990 return false; 3991 for (Reference item : this.basedOn) 3992 if (!item.isEmpty()) 3993 return true; 3994 return false; 3995 } 3996 3997 public Reference addBasedOn() { //3 3998 Reference t = new Reference(); 3999 if (this.basedOn == null) 4000 this.basedOn = new ArrayList<Reference>(); 4001 this.basedOn.add(t); 4002 return t; 4003 } 4004 4005 public RequestGroup addBasedOn(Reference t) { //3 4006 if (t == null) 4007 return this; 4008 if (this.basedOn == null) 4009 this.basedOn = new ArrayList<Reference>(); 4010 this.basedOn.add(t); 4011 return this; 4012 } 4013 4014 /** 4015 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 4016 */ 4017 public Reference getBasedOnFirstRep() { 4018 if (getBasedOn().isEmpty()) { 4019 addBasedOn(); 4020 } 4021 return getBasedOn().get(0); 4022 } 4023 4024 /** 4025 * @deprecated Use Reference#setResource(IBaseResource) instead 4026 */ 4027 @Deprecated 4028 public List<Resource> getBasedOnTarget() { 4029 if (this.basedOnTarget == null) 4030 this.basedOnTarget = new ArrayList<Resource>(); 4031 return this.basedOnTarget; 4032 } 4033 4034 /** 4035 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 4036 */ 4037 public List<Reference> getReplaces() { 4038 if (this.replaces == null) 4039 this.replaces = new ArrayList<Reference>(); 4040 return this.replaces; 4041 } 4042 4043 /** 4044 * @return Returns a reference to <code>this</code> for easy method chaining 4045 */ 4046 public RequestGroup setReplaces(List<Reference> theReplaces) { 4047 this.replaces = theReplaces; 4048 return this; 4049 } 4050 4051 public boolean hasReplaces() { 4052 if (this.replaces == null) 4053 return false; 4054 for (Reference item : this.replaces) 4055 if (!item.isEmpty()) 4056 return true; 4057 return false; 4058 } 4059 4060 public Reference addReplaces() { //3 4061 Reference t = new Reference(); 4062 if (this.replaces == null) 4063 this.replaces = new ArrayList<Reference>(); 4064 this.replaces.add(t); 4065 return t; 4066 } 4067 4068 public RequestGroup addReplaces(Reference t) { //3 4069 if (t == null) 4070 return this; 4071 if (this.replaces == null) 4072 this.replaces = new ArrayList<Reference>(); 4073 this.replaces.add(t); 4074 return this; 4075 } 4076 4077 /** 4078 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 4079 */ 4080 public Reference getReplacesFirstRep() { 4081 if (getReplaces().isEmpty()) { 4082 addReplaces(); 4083 } 4084 return getReplaces().get(0); 4085 } 4086 4087 /** 4088 * @deprecated Use Reference#setResource(IBaseResource) instead 4089 */ 4090 @Deprecated 4091 public List<Resource> getReplacesTarget() { 4092 if (this.replacesTarget == null) 4093 this.replacesTarget = new ArrayList<Resource>(); 4094 return this.replacesTarget; 4095 } 4096 4097 /** 4098 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 4099 */ 4100 public Identifier getGroupIdentifier() { 4101 if (this.groupIdentifier == null) 4102 if (Configuration.errorOnAutoCreate()) 4103 throw new Error("Attempt to auto-create RequestGroup.groupIdentifier"); 4104 else if (Configuration.doAutoCreate()) 4105 this.groupIdentifier = new Identifier(); // cc 4106 return this.groupIdentifier; 4107 } 4108 4109 public boolean hasGroupIdentifier() { 4110 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4111 } 4112 4113 /** 4114 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.) 4115 */ 4116 public RequestGroup setGroupIdentifier(Identifier value) { 4117 this.groupIdentifier = value; 4118 return this; 4119 } 4120 4121 /** 4122 * @return {@link #status} (The current state of the request. For request groups, the status reflects the status of all the requests in the group.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4123 */ 4124 public Enumeration<RequestStatus> getStatusElement() { 4125 if (this.status == null) 4126 if (Configuration.errorOnAutoCreate()) 4127 throw new Error("Attempt to auto-create RequestGroup.status"); 4128 else if (Configuration.doAutoCreate()) 4129 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 4130 return this.status; 4131 } 4132 4133 public boolean hasStatusElement() { 4134 return this.status != null && !this.status.isEmpty(); 4135 } 4136 4137 public boolean hasStatus() { 4138 return this.status != null && !this.status.isEmpty(); 4139 } 4140 4141 /** 4142 * @param value {@link #status} (The current state of the request. For request groups, the status reflects the status of all the requests in the group.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4143 */ 4144 public RequestGroup setStatusElement(Enumeration<RequestStatus> value) { 4145 this.status = value; 4146 return this; 4147 } 4148 4149 /** 4150 * @return The current state of the request. For request groups, the status reflects the status of all the requests in the group. 4151 */ 4152 public RequestStatus getStatus() { 4153 return this.status == null ? null : this.status.getValue(); 4154 } 4155 4156 /** 4157 * @param value The current state of the request. For request groups, the status reflects the status of all the requests in the group. 4158 */ 4159 public RequestGroup setStatus(RequestStatus value) { 4160 if (this.status == null) 4161 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 4162 this.status.setValue(value); 4163 return this; 4164 } 4165 4166 /** 4167 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4168 */ 4169 public Enumeration<RequestIntent> getIntentElement() { 4170 if (this.intent == null) 4171 if (Configuration.errorOnAutoCreate()) 4172 throw new Error("Attempt to auto-create RequestGroup.intent"); 4173 else if (Configuration.doAutoCreate()) 4174 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 4175 return this.intent; 4176 } 4177 4178 public boolean hasIntentElement() { 4179 return this.intent != null && !this.intent.isEmpty(); 4180 } 4181 4182 public boolean hasIntent() { 4183 return this.intent != null && !this.intent.isEmpty(); 4184 } 4185 4186 /** 4187 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4188 */ 4189 public RequestGroup setIntentElement(Enumeration<RequestIntent> value) { 4190 this.intent = value; 4191 return this; 4192 } 4193 4194 /** 4195 * @return Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4196 */ 4197 public RequestIntent getIntent() { 4198 return this.intent == null ? null : this.intent.getValue(); 4199 } 4200 4201 /** 4202 * @param value Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4203 */ 4204 public RequestGroup setIntent(RequestIntent value) { 4205 if (this.intent == null) 4206 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 4207 this.intent.setValue(value); 4208 return this; 4209 } 4210 4211 /** 4212 * @return {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4213 */ 4214 public Enumeration<RequestPriority> getPriorityElement() { 4215 if (this.priority == null) 4216 if (Configuration.errorOnAutoCreate()) 4217 throw new Error("Attempt to auto-create RequestGroup.priority"); 4218 else if (Configuration.doAutoCreate()) 4219 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 4220 return this.priority; 4221 } 4222 4223 public boolean hasPriorityElement() { 4224 return this.priority != null && !this.priority.isEmpty(); 4225 } 4226 4227 public boolean hasPriority() { 4228 return this.priority != null && !this.priority.isEmpty(); 4229 } 4230 4231 /** 4232 * @param value {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4233 */ 4234 public RequestGroup setPriorityElement(Enumeration<RequestPriority> value) { 4235 this.priority = value; 4236 return this; 4237 } 4238 4239 /** 4240 * @return Indicates how quickly the request should be addressed with respect to other requests. 4241 */ 4242 public RequestPriority getPriority() { 4243 return this.priority == null ? null : this.priority.getValue(); 4244 } 4245 4246 /** 4247 * @param value Indicates how quickly the request should be addressed with respect to other requests. 4248 */ 4249 public RequestGroup setPriority(RequestPriority value) { 4250 if (value == null) 4251 this.priority = null; 4252 else { 4253 if (this.priority == null) 4254 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4255 this.priority.setValue(value); 4256 } 4257 return this; 4258 } 4259 4260 /** 4261 * @return {@link #subject} (The subject for which the request group was created.) 4262 */ 4263 public Reference getSubject() { 4264 if (this.subject == null) 4265 if (Configuration.errorOnAutoCreate()) 4266 throw new Error("Attempt to auto-create RequestGroup.subject"); 4267 else if (Configuration.doAutoCreate()) 4268 this.subject = new Reference(); // cc 4269 return this.subject; 4270 } 4271 4272 public boolean hasSubject() { 4273 return this.subject != null && !this.subject.isEmpty(); 4274 } 4275 4276 /** 4277 * @param value {@link #subject} (The subject for which the request group was created.) 4278 */ 4279 public RequestGroup setSubject(Reference value) { 4280 this.subject = value; 4281 return this; 4282 } 4283 4284 /** 4285 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The subject for which the request group was created.) 4286 */ 4287 public Resource getSubjectTarget() { 4288 return this.subjectTarget; 4289 } 4290 4291 /** 4292 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The subject for which the request group was created.) 4293 */ 4294 public RequestGroup setSubjectTarget(Resource value) { 4295 this.subjectTarget = value; 4296 return this; 4297 } 4298 4299 /** 4300 * @return {@link #context} (Describes the context of the request group, if any.) 4301 */ 4302 public Reference getContext() { 4303 if (this.context == null) 4304 if (Configuration.errorOnAutoCreate()) 4305 throw new Error("Attempt to auto-create RequestGroup.context"); 4306 else if (Configuration.doAutoCreate()) 4307 this.context = new Reference(); // cc 4308 return this.context; 4309 } 4310 4311 public boolean hasContext() { 4312 return this.context != null && !this.context.isEmpty(); 4313 } 4314 4315 /** 4316 * @param value {@link #context} (Describes the context of the request group, if any.) 4317 */ 4318 public RequestGroup setContext(Reference value) { 4319 this.context = value; 4320 return this; 4321 } 4322 4323 /** 4324 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the context of the request group, if any.) 4325 */ 4326 public Resource getContextTarget() { 4327 return this.contextTarget; 4328 } 4329 4330 /** 4331 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the context of the request group, if any.) 4332 */ 4333 public RequestGroup setContextTarget(Resource value) { 4334 this.contextTarget = value; 4335 return this; 4336 } 4337 4338 /** 4339 * @return {@link #authoredOn} (Indicates when the request group was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4340 */ 4341 public DateTimeType getAuthoredOnElement() { 4342 if (this.authoredOn == null) 4343 if (Configuration.errorOnAutoCreate()) 4344 throw new Error("Attempt to auto-create RequestGroup.authoredOn"); 4345 else if (Configuration.doAutoCreate()) 4346 this.authoredOn = new DateTimeType(); // bb 4347 return this.authoredOn; 4348 } 4349 4350 public boolean hasAuthoredOnElement() { 4351 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4352 } 4353 4354 public boolean hasAuthoredOn() { 4355 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4356 } 4357 4358 /** 4359 * @param value {@link #authoredOn} (Indicates when the request group was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4360 */ 4361 public RequestGroup setAuthoredOnElement(DateTimeType value) { 4362 this.authoredOn = value; 4363 return this; 4364 } 4365 4366 /** 4367 * @return Indicates when the request group was created. 4368 */ 4369 public Date getAuthoredOn() { 4370 return this.authoredOn == null ? null : this.authoredOn.getValue(); 4371 } 4372 4373 /** 4374 * @param value Indicates when the request group was created. 4375 */ 4376 public RequestGroup setAuthoredOn(Date value) { 4377 if (value == null) 4378 this.authoredOn = null; 4379 else { 4380 if (this.authoredOn == null) 4381 this.authoredOn = new DateTimeType(); 4382 this.authoredOn.setValue(value); 4383 } 4384 return this; 4385 } 4386 4387 /** 4388 * @return {@link #author} (Provides a reference to the author of the request group.) 4389 */ 4390 public Reference getAuthor() { 4391 if (this.author == null) 4392 if (Configuration.errorOnAutoCreate()) 4393 throw new Error("Attempt to auto-create RequestGroup.author"); 4394 else if (Configuration.doAutoCreate()) 4395 this.author = new Reference(); // cc 4396 return this.author; 4397 } 4398 4399 public boolean hasAuthor() { 4400 return this.author != null && !this.author.isEmpty(); 4401 } 4402 4403 /** 4404 * @param value {@link #author} (Provides a reference to the author of the request group.) 4405 */ 4406 public RequestGroup setAuthor(Reference value) { 4407 this.author = value; 4408 return this; 4409 } 4410 4411 /** 4412 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Provides a reference to the author of the request group.) 4413 */ 4414 public Resource getAuthorTarget() { 4415 return this.authorTarget; 4416 } 4417 4418 /** 4419 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Provides a reference to the author of the request group.) 4420 */ 4421 public RequestGroup setAuthorTarget(Resource value) { 4422 this.authorTarget = value; 4423 return this; 4424 } 4425 4426 /** 4427 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4428 */ 4429 public Type getReason() { 4430 return this.reason; 4431 } 4432 4433 /** 4434 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4435 */ 4436 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 4437 if (this.reason == null) 4438 return null; 4439 if (!(this.reason instanceof CodeableConcept)) 4440 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 4441 return (CodeableConcept) this.reason; 4442 } 4443 4444 public boolean hasReasonCodeableConcept() { 4445 return this != null && this.reason instanceof CodeableConcept; 4446 } 4447 4448 /** 4449 * @return {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4450 */ 4451 public Reference getReasonReference() throws FHIRException { 4452 if (this.reason == null) 4453 return null; 4454 if (!(this.reason instanceof Reference)) 4455 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 4456 return (Reference) this.reason; 4457 } 4458 4459 public boolean hasReasonReference() { 4460 return this != null && this.reason instanceof Reference; 4461 } 4462 4463 public boolean hasReason() { 4464 return this.reason != null && !this.reason.isEmpty(); 4465 } 4466 4467 /** 4468 * @param value {@link #reason} (Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 4469 */ 4470 public RequestGroup setReason(Type value) throws FHIRFormatError { 4471 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 4472 throw new FHIRFormatError("Not the right type for RequestGroup.reason[x]: "+value.fhirType()); 4473 this.reason = value; 4474 return this; 4475 } 4476 4477 /** 4478 * @return {@link #note} (Provides a mechanism to communicate additional information about the response.) 4479 */ 4480 public List<Annotation> getNote() { 4481 if (this.note == null) 4482 this.note = new ArrayList<Annotation>(); 4483 return this.note; 4484 } 4485 4486 /** 4487 * @return Returns a reference to <code>this</code> for easy method chaining 4488 */ 4489 public RequestGroup setNote(List<Annotation> theNote) { 4490 this.note = theNote; 4491 return this; 4492 } 4493 4494 public boolean hasNote() { 4495 if (this.note == null) 4496 return false; 4497 for (Annotation item : this.note) 4498 if (!item.isEmpty()) 4499 return true; 4500 return false; 4501 } 4502 4503 public Annotation addNote() { //3 4504 Annotation t = new Annotation(); 4505 if (this.note == null) 4506 this.note = new ArrayList<Annotation>(); 4507 this.note.add(t); 4508 return t; 4509 } 4510 4511 public RequestGroup addNote(Annotation t) { //3 4512 if (t == null) 4513 return this; 4514 if (this.note == null) 4515 this.note = new ArrayList<Annotation>(); 4516 this.note.add(t); 4517 return this; 4518 } 4519 4520 /** 4521 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 4522 */ 4523 public Annotation getNoteFirstRep() { 4524 if (getNote().isEmpty()) { 4525 addNote(); 4526 } 4527 return getNote().get(0); 4528 } 4529 4530 /** 4531 * @return {@link #action} (The actions, if any, produced by the evaluation of the artifact.) 4532 */ 4533 public List<RequestGroupActionComponent> getAction() { 4534 if (this.action == null) 4535 this.action = new ArrayList<RequestGroupActionComponent>(); 4536 return this.action; 4537 } 4538 4539 /** 4540 * @return Returns a reference to <code>this</code> for easy method chaining 4541 */ 4542 public RequestGroup setAction(List<RequestGroupActionComponent> theAction) { 4543 this.action = theAction; 4544 return this; 4545 } 4546 4547 public boolean hasAction() { 4548 if (this.action == null) 4549 return false; 4550 for (RequestGroupActionComponent item : this.action) 4551 if (!item.isEmpty()) 4552 return true; 4553 return false; 4554 } 4555 4556 public RequestGroupActionComponent addAction() { //3 4557 RequestGroupActionComponent t = new RequestGroupActionComponent(); 4558 if (this.action == null) 4559 this.action = new ArrayList<RequestGroupActionComponent>(); 4560 this.action.add(t); 4561 return t; 4562 } 4563 4564 public RequestGroup addAction(RequestGroupActionComponent t) { //3 4565 if (t == null) 4566 return this; 4567 if (this.action == null) 4568 this.action = new ArrayList<RequestGroupActionComponent>(); 4569 this.action.add(t); 4570 return this; 4571 } 4572 4573 /** 4574 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 4575 */ 4576 public RequestGroupActionComponent getActionFirstRep() { 4577 if (getAction().isEmpty()) { 4578 addAction(); 4579 } 4580 return getAction().get(0); 4581 } 4582 4583 protected void listChildren(List<Property> children) { 4584 super.listChildren(children); 4585 children.add(new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4586 children.add(new Property("definition", "Reference(Any)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 4587 children.add(new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 4588 children.add(new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 4589 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier)); 4590 children.add(new Property("status", "code", "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 0, 1, status)); 4591 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent)); 4592 children.add(new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 4593 children.add(new Property("subject", "Reference(Patient|Group)", "The subject for which the request group was created.", 0, 1, subject)); 4594 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Describes the context of the request group, if any.", 0, 1, context)); 4595 children.add(new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn)); 4596 children.add(new Property("author", "Reference(Device|Practitioner)", "Provides a reference to the author of the request group.", 0, 1, author)); 4597 children.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason)); 4598 children.add(new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note)); 4599 children.add(new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action)); 4600 } 4601 4602 @Override 4603 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4604 switch (_hash) { 4605 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier); 4606 case -1014418093: /*definition*/ return new Property("definition", "Reference(Any)", "A protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 4607 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 4608 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 4609 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 0, 1, groupIdentifier); 4610 case -892481550: /*status*/ return new Property("status", "code", "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 0, 1, status); 4611 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent); 4612 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 4613 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The subject for which the request group was created.", 0, 1, subject); 4614 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Describes the context of the request group, if any.", 0, 1, context); 4615 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn); 4616 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner)", "Provides a reference to the author of the request group.", 0, 1, author); 4617 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4618 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4619 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4620 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request group was created. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 4621 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note); 4622 case -1422950858: /*action*/ return new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action); 4623 default: return super.getNamedProperty(_hash, _name, _checkValid); 4624 } 4625 4626 } 4627 4628 @Override 4629 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4630 switch (hash) { 4631 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4632 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 4633 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4634 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 4635 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 4636 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 4637 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 4638 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 4639 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 4640 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 4641 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 4642 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 4643 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 4644 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4645 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 4646 default: return super.getProperty(hash, name, checkValid); 4647 } 4648 4649 } 4650 4651 @Override 4652 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4653 switch (hash) { 4654 case -1618432855: // identifier 4655 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4656 return value; 4657 case -1014418093: // definition 4658 this.getDefinition().add(castToReference(value)); // Reference 4659 return value; 4660 case -332612366: // basedOn 4661 this.getBasedOn().add(castToReference(value)); // Reference 4662 return value; 4663 case -430332865: // replaces 4664 this.getReplaces().add(castToReference(value)); // Reference 4665 return value; 4666 case -445338488: // groupIdentifier 4667 this.groupIdentifier = castToIdentifier(value); // Identifier 4668 return value; 4669 case -892481550: // status 4670 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 4671 this.status = (Enumeration) value; // Enumeration<RequestStatus> 4672 return value; 4673 case -1183762788: // intent 4674 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 4675 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4676 return value; 4677 case -1165461084: // priority 4678 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4679 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4680 return value; 4681 case -1867885268: // subject 4682 this.subject = castToReference(value); // Reference 4683 return value; 4684 case 951530927: // context 4685 this.context = castToReference(value); // Reference 4686 return value; 4687 case -1500852503: // authoredOn 4688 this.authoredOn = castToDateTime(value); // DateTimeType 4689 return value; 4690 case -1406328437: // author 4691 this.author = castToReference(value); // Reference 4692 return value; 4693 case -934964668: // reason 4694 this.reason = castToType(value); // Type 4695 return value; 4696 case 3387378: // note 4697 this.getNote().add(castToAnnotation(value)); // Annotation 4698 return value; 4699 case -1422950858: // action 4700 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 4701 return value; 4702 default: return super.setProperty(hash, name, value); 4703 } 4704 4705 } 4706 4707 @Override 4708 public Base setProperty(String name, Base value) throws FHIRException { 4709 if (name.equals("identifier")) { 4710 this.getIdentifier().add(castToIdentifier(value)); 4711 } else if (name.equals("definition")) { 4712 this.getDefinition().add(castToReference(value)); 4713 } else if (name.equals("basedOn")) { 4714 this.getBasedOn().add(castToReference(value)); 4715 } else if (name.equals("replaces")) { 4716 this.getReplaces().add(castToReference(value)); 4717 } else if (name.equals("groupIdentifier")) { 4718 this.groupIdentifier = castToIdentifier(value); // Identifier 4719 } else if (name.equals("status")) { 4720 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 4721 this.status = (Enumeration) value; // Enumeration<RequestStatus> 4722 } else if (name.equals("intent")) { 4723 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 4724 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4725 } else if (name.equals("priority")) { 4726 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4727 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4728 } else if (name.equals("subject")) { 4729 this.subject = castToReference(value); // Reference 4730 } else if (name.equals("context")) { 4731 this.context = castToReference(value); // Reference 4732 } else if (name.equals("authoredOn")) { 4733 this.authoredOn = castToDateTime(value); // DateTimeType 4734 } else if (name.equals("author")) { 4735 this.author = castToReference(value); // Reference 4736 } else if (name.equals("reason[x]")) { 4737 this.reason = castToType(value); // Type 4738 } else if (name.equals("note")) { 4739 this.getNote().add(castToAnnotation(value)); 4740 } else if (name.equals("action")) { 4741 this.getAction().add((RequestGroupActionComponent) value); 4742 } else 4743 return super.setProperty(name, value); 4744 return value; 4745 } 4746 4747 @Override 4748 public Base makeProperty(int hash, String name) throws FHIRException { 4749 switch (hash) { 4750 case -1618432855: return addIdentifier(); 4751 case -1014418093: return addDefinition(); 4752 case -332612366: return addBasedOn(); 4753 case -430332865: return addReplaces(); 4754 case -445338488: return getGroupIdentifier(); 4755 case -892481550: return getStatusElement(); 4756 case -1183762788: return getIntentElement(); 4757 case -1165461084: return getPriorityElement(); 4758 case -1867885268: return getSubject(); 4759 case 951530927: return getContext(); 4760 case -1500852503: return getAuthoredOnElement(); 4761 case -1406328437: return getAuthor(); 4762 case -669418564: return getReason(); 4763 case -934964668: return getReason(); 4764 case 3387378: return addNote(); 4765 case -1422950858: return addAction(); 4766 default: return super.makeProperty(hash, name); 4767 } 4768 4769 } 4770 4771 @Override 4772 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4773 switch (hash) { 4774 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4775 case -1014418093: /*definition*/ return new String[] {"Reference"}; 4776 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 4777 case -430332865: /*replaces*/ return new String[] {"Reference"}; 4778 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 4779 case -892481550: /*status*/ return new String[] {"code"}; 4780 case -1183762788: /*intent*/ return new String[] {"code"}; 4781 case -1165461084: /*priority*/ return new String[] {"code"}; 4782 case -1867885268: /*subject*/ return new String[] {"Reference"}; 4783 case 951530927: /*context*/ return new String[] {"Reference"}; 4784 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 4785 case -1406328437: /*author*/ return new String[] {"Reference"}; 4786 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 4787 case 3387378: /*note*/ return new String[] {"Annotation"}; 4788 case -1422950858: /*action*/ return new String[] {}; 4789 default: return super.getTypesForProperty(hash, name); 4790 } 4791 4792 } 4793 4794 @Override 4795 public Base addChild(String name) throws FHIRException { 4796 if (name.equals("identifier")) { 4797 return addIdentifier(); 4798 } 4799 else if (name.equals("definition")) { 4800 return addDefinition(); 4801 } 4802 else if (name.equals("basedOn")) { 4803 return addBasedOn(); 4804 } 4805 else if (name.equals("replaces")) { 4806 return addReplaces(); 4807 } 4808 else if (name.equals("groupIdentifier")) { 4809 this.groupIdentifier = new Identifier(); 4810 return this.groupIdentifier; 4811 } 4812 else if (name.equals("status")) { 4813 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.status"); 4814 } 4815 else if (name.equals("intent")) { 4816 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.intent"); 4817 } 4818 else if (name.equals("priority")) { 4819 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 4820 } 4821 else if (name.equals("subject")) { 4822 this.subject = new Reference(); 4823 return this.subject; 4824 } 4825 else if (name.equals("context")) { 4826 this.context = new Reference(); 4827 return this.context; 4828 } 4829 else if (name.equals("authoredOn")) { 4830 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.authoredOn"); 4831 } 4832 else if (name.equals("author")) { 4833 this.author = new Reference(); 4834 return this.author; 4835 } 4836 else if (name.equals("reasonCodeableConcept")) { 4837 this.reason = new CodeableConcept(); 4838 return this.reason; 4839 } 4840 else if (name.equals("reasonReference")) { 4841 this.reason = new Reference(); 4842 return this.reason; 4843 } 4844 else if (name.equals("note")) { 4845 return addNote(); 4846 } 4847 else if (name.equals("action")) { 4848 return addAction(); 4849 } 4850 else 4851 return super.addChild(name); 4852 } 4853 4854 public String fhirType() { 4855 return "RequestGroup"; 4856 4857 } 4858 4859 public RequestGroup copy() { 4860 RequestGroup dst = new RequestGroup(); 4861 copyValues(dst); 4862 if (identifier != null) { 4863 dst.identifier = new ArrayList<Identifier>(); 4864 for (Identifier i : identifier) 4865 dst.identifier.add(i.copy()); 4866 }; 4867 if (definition != null) { 4868 dst.definition = new ArrayList<Reference>(); 4869 for (Reference i : definition) 4870 dst.definition.add(i.copy()); 4871 }; 4872 if (basedOn != null) { 4873 dst.basedOn = new ArrayList<Reference>(); 4874 for (Reference i : basedOn) 4875 dst.basedOn.add(i.copy()); 4876 }; 4877 if (replaces != null) { 4878 dst.replaces = new ArrayList<Reference>(); 4879 for (Reference i : replaces) 4880 dst.replaces.add(i.copy()); 4881 }; 4882 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 4883 dst.status = status == null ? null : status.copy(); 4884 dst.intent = intent == null ? null : intent.copy(); 4885 dst.priority = priority == null ? null : priority.copy(); 4886 dst.subject = subject == null ? null : subject.copy(); 4887 dst.context = context == null ? null : context.copy(); 4888 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4889 dst.author = author == null ? null : author.copy(); 4890 dst.reason = reason == null ? null : reason.copy(); 4891 if (note != null) { 4892 dst.note = new ArrayList<Annotation>(); 4893 for (Annotation i : note) 4894 dst.note.add(i.copy()); 4895 }; 4896 if (action != null) { 4897 dst.action = new ArrayList<RequestGroupActionComponent>(); 4898 for (RequestGroupActionComponent i : action) 4899 dst.action.add(i.copy()); 4900 }; 4901 return dst; 4902 } 4903 4904 protected RequestGroup typedCopy() { 4905 return copy(); 4906 } 4907 4908 @Override 4909 public boolean equalsDeep(Base other_) { 4910 if (!super.equalsDeep(other_)) 4911 return false; 4912 if (!(other_ instanceof RequestGroup)) 4913 return false; 4914 RequestGroup o = (RequestGroup) other_; 4915 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 4916 && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 4917 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 4918 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(authoredOn, o.authoredOn, true) 4919 && compareDeep(author, o.author, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 4920 && compareDeep(action, o.action, true); 4921 } 4922 4923 @Override 4924 public boolean equalsShallow(Base other_) { 4925 if (!super.equalsShallow(other_)) 4926 return false; 4927 if (!(other_ instanceof RequestGroup)) 4928 return false; 4929 RequestGroup o = (RequestGroup) other_; 4930 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 4931 && compareValues(authoredOn, o.authoredOn, true); 4932 } 4933 4934 public boolean isEmpty() { 4935 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 4936 , replaces, groupIdentifier, status, intent, priority, subject, context, authoredOn 4937 , author, reason, note, action); 4938 } 4939 4940 @Override 4941 public ResourceType getResourceType() { 4942 return ResourceType.RequestGroup; 4943 } 4944 4945 /** 4946 * Search parameter: <b>authored</b> 4947 * <p> 4948 * Description: <b>The date the request group was authored</b><br> 4949 * Type: <b>date</b><br> 4950 * Path: <b>RequestGroup.authoredOn</b><br> 4951 * </p> 4952 */ 4953 @SearchParamDefinition(name="authored", path="RequestGroup.authoredOn", description="The date the request group was authored", type="date" ) 4954 public static final String SP_AUTHORED = "authored"; 4955 /** 4956 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 4957 * <p> 4958 * Description: <b>The date the request group was authored</b><br> 4959 * Type: <b>date</b><br> 4960 * Path: <b>RequestGroup.authoredOn</b><br> 4961 * </p> 4962 */ 4963 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 4964 4965 /** 4966 * Search parameter: <b>identifier</b> 4967 * <p> 4968 * Description: <b>External identifiers for the request group</b><br> 4969 * Type: <b>token</b><br> 4970 * Path: <b>RequestGroup.identifier</b><br> 4971 * </p> 4972 */ 4973 @SearchParamDefinition(name="identifier", path="RequestGroup.identifier", description="External identifiers for the request group", type="token" ) 4974 public static final String SP_IDENTIFIER = "identifier"; 4975 /** 4976 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4977 * <p> 4978 * Description: <b>External identifiers for the request group</b><br> 4979 * Type: <b>token</b><br> 4980 * Path: <b>RequestGroup.identifier</b><br> 4981 * </p> 4982 */ 4983 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4984 4985 /** 4986 * Search parameter: <b>subject</b> 4987 * <p> 4988 * Description: <b>The subject that the request group is about</b><br> 4989 * Type: <b>reference</b><br> 4990 * Path: <b>RequestGroup.subject</b><br> 4991 * </p> 4992 */ 4993 @SearchParamDefinition(name="subject", path="RequestGroup.subject", description="The subject that the request group is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 4994 public static final String SP_SUBJECT = "subject"; 4995 /** 4996 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4997 * <p> 4998 * Description: <b>The subject that the request group is about</b><br> 4999 * Type: <b>reference</b><br> 5000 * Path: <b>RequestGroup.subject</b><br> 5001 * </p> 5002 */ 5003 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 5004 5005/** 5006 * Constant for fluent queries to be used to add include statements. Specifies 5007 * the path value of "<b>RequestGroup:subject</b>". 5008 */ 5009 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RequestGroup:subject").toLocked(); 5010 5011 /** 5012 * Search parameter: <b>author</b> 5013 * <p> 5014 * Description: <b>The author of the request group</b><br> 5015 * Type: <b>reference</b><br> 5016 * Path: <b>RequestGroup.author</b><br> 5017 * </p> 5018 */ 5019 @SearchParamDefinition(name="author", path="RequestGroup.author", description="The author of the request group", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Practitioner.class } ) 5020 public static final String SP_AUTHOR = "author"; 5021 /** 5022 * <b>Fluent Client</b> search parameter constant for <b>author</b> 5023 * <p> 5024 * Description: <b>The author of the request group</b><br> 5025 * Type: <b>reference</b><br> 5026 * Path: <b>RequestGroup.author</b><br> 5027 * </p> 5028 */ 5029 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 5030 5031/** 5032 * Constant for fluent queries to be used to add include statements. Specifies 5033 * the path value of "<b>RequestGroup:author</b>". 5034 */ 5035 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("RequestGroup:author").toLocked(); 5036 5037 /** 5038 * Search parameter: <b>encounter</b> 5039 * <p> 5040 * Description: <b>The encounter the request group applies to</b><br> 5041 * Type: <b>reference</b><br> 5042 * Path: <b>RequestGroup.context</b><br> 5043 * </p> 5044 */ 5045 @SearchParamDefinition(name="encounter", path="RequestGroup.context", description="The encounter the request group applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 5046 public static final String SP_ENCOUNTER = "encounter"; 5047 /** 5048 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5049 * <p> 5050 * Description: <b>The encounter the request group applies to</b><br> 5051 * Type: <b>reference</b><br> 5052 * Path: <b>RequestGroup.context</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 5056 5057/** 5058 * Constant for fluent queries to be used to add include statements. Specifies 5059 * the path value of "<b>RequestGroup:encounter</b>". 5060 */ 5061 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("RequestGroup:encounter").toLocked(); 5062 5063 /** 5064 * Search parameter: <b>priority</b> 5065 * <p> 5066 * Description: <b>The priority of the request group</b><br> 5067 * Type: <b>token</b><br> 5068 * Path: <b>RequestGroup.priority</b><br> 5069 * </p> 5070 */ 5071 @SearchParamDefinition(name="priority", path="RequestGroup.priority", description="The priority of the request group", type="token" ) 5072 public static final String SP_PRIORITY = "priority"; 5073 /** 5074 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5075 * <p> 5076 * Description: <b>The priority of the request group</b><br> 5077 * Type: <b>token</b><br> 5078 * Path: <b>RequestGroup.priority</b><br> 5079 * </p> 5080 */ 5081 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 5082 5083 /** 5084 * Search parameter: <b>intent</b> 5085 * <p> 5086 * Description: <b>The intent of the request group</b><br> 5087 * Type: <b>token</b><br> 5088 * Path: <b>RequestGroup.intent</b><br> 5089 * </p> 5090 */ 5091 @SearchParamDefinition(name="intent", path="RequestGroup.intent", description="The intent of the request group", type="token" ) 5092 public static final String SP_INTENT = "intent"; 5093 /** 5094 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5095 * <p> 5096 * Description: <b>The intent of the request group</b><br> 5097 * Type: <b>token</b><br> 5098 * Path: <b>RequestGroup.intent</b><br> 5099 * </p> 5100 */ 5101 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 5102 5103 /** 5104 * Search parameter: <b>participant</b> 5105 * <p> 5106 * Description: <b>The participant in the requests in the group</b><br> 5107 * Type: <b>reference</b><br> 5108 * Path: <b>RequestGroup.action.participant</b><br> 5109 * </p> 5110 */ 5111 @SearchParamDefinition(name="participant", path="RequestGroup.action.participant", description="The participant in the requests in the group", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Patient.class, Person.class, Practitioner.class, RelatedPerson.class } ) 5112 public static final String SP_PARTICIPANT = "participant"; 5113 /** 5114 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 5115 * <p> 5116 * Description: <b>The participant in the requests in the group</b><br> 5117 * Type: <b>reference</b><br> 5118 * Path: <b>RequestGroup.action.participant</b><br> 5119 * </p> 5120 */ 5121 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 5122 5123/** 5124 * Constant for fluent queries to be used to add include statements. Specifies 5125 * the path value of "<b>RequestGroup:participant</b>". 5126 */ 5127 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("RequestGroup:participant").toLocked(); 5128 5129 /** 5130 * Search parameter: <b>group-identifier</b> 5131 * <p> 5132 * Description: <b>The group identifier for the request group</b><br> 5133 * Type: <b>token</b><br> 5134 * Path: <b>RequestGroup.groupIdentifier</b><br> 5135 * </p> 5136 */ 5137 @SearchParamDefinition(name="group-identifier", path="RequestGroup.groupIdentifier", description="The group identifier for the request group", type="token" ) 5138 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5139 /** 5140 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5141 * <p> 5142 * Description: <b>The group identifier for the request group</b><br> 5143 * Type: <b>token</b><br> 5144 * Path: <b>RequestGroup.groupIdentifier</b><br> 5145 * </p> 5146 */ 5147 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 5148 5149 /** 5150 * Search parameter: <b>patient</b> 5151 * <p> 5152 * Description: <b>The identity of a patient to search for request groups</b><br> 5153 * Type: <b>reference</b><br> 5154 * Path: <b>RequestGroup.subject</b><br> 5155 * </p> 5156 */ 5157 @SearchParamDefinition(name="patient", path="RequestGroup.subject", description="The identity of a patient to search for request groups", type="reference", target={Patient.class } ) 5158 public static final String SP_PATIENT = "patient"; 5159 /** 5160 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5161 * <p> 5162 * Description: <b>The identity of a patient to search for request groups</b><br> 5163 * Type: <b>reference</b><br> 5164 * Path: <b>RequestGroup.subject</b><br> 5165 * </p> 5166 */ 5167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5168 5169/** 5170 * Constant for fluent queries to be used to add include statements. Specifies 5171 * the path value of "<b>RequestGroup:patient</b>". 5172 */ 5173 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RequestGroup:patient").toLocked(); 5174 5175 /** 5176 * Search parameter: <b>context</b> 5177 * <p> 5178 * Description: <b>The context the request group applies to</b><br> 5179 * Type: <b>reference</b><br> 5180 * Path: <b>RequestGroup.context</b><br> 5181 * </p> 5182 */ 5183 @SearchParamDefinition(name="context", path="RequestGroup.context", description="The context the request group applies to", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 5184 public static final String SP_CONTEXT = "context"; 5185 /** 5186 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5187 * <p> 5188 * Description: <b>The context the request group applies to</b><br> 5189 * Type: <b>reference</b><br> 5190 * Path: <b>RequestGroup.context</b><br> 5191 * </p> 5192 */ 5193 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 5194 5195/** 5196 * Constant for fluent queries to be used to add include statements. Specifies 5197 * the path value of "<b>RequestGroup:context</b>". 5198 */ 5199 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("RequestGroup:context").toLocked(); 5200 5201 /** 5202 * Search parameter: <b>definition</b> 5203 * <p> 5204 * Description: <b>The definition from which the request group is realized</b><br> 5205 * Type: <b>reference</b><br> 5206 * Path: <b>RequestGroup.definition</b><br> 5207 * </p> 5208 */ 5209 @SearchParamDefinition(name="definition", path="RequestGroup.definition", description="The definition from which the request group is realized", type="reference" ) 5210 public static final String SP_DEFINITION = "definition"; 5211 /** 5212 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 5213 * <p> 5214 * Description: <b>The definition from which the request group is realized</b><br> 5215 * Type: <b>reference</b><br> 5216 * Path: <b>RequestGroup.definition</b><br> 5217 * </p> 5218 */ 5219 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 5220 5221/** 5222 * Constant for fluent queries to be used to add include statements. Specifies 5223 * the path value of "<b>RequestGroup:definition</b>". 5224 */ 5225 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("RequestGroup:definition").toLocked(); 5226 5227 /** 5228 * Search parameter: <b>status</b> 5229 * <p> 5230 * Description: <b>The status of the request group</b><br> 5231 * Type: <b>token</b><br> 5232 * Path: <b>RequestGroup.status</b><br> 5233 * </p> 5234 */ 5235 @SearchParamDefinition(name="status", path="RequestGroup.status", description="The status of the request group", type="token" ) 5236 public static final String SP_STATUS = "status"; 5237 /** 5238 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5239 * <p> 5240 * Description: <b>The status of the request group</b><br> 5241 * Type: <b>token</b><br> 5242 * Path: <b>RequestGroup.status</b><br> 5243 * </p> 5244 */ 5245 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5246 5247 5248}