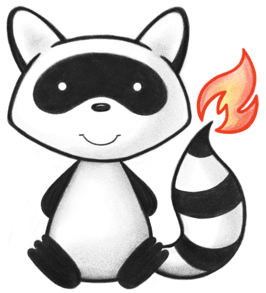
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 049 */ 050@ResourceDef(name="ResearchStudy", profile="http://hl7.org/fhir/Profile/ResearchStudy") 051public class ResearchStudy extends DomainResource { 052 053 public enum ResearchStudyStatus { 054 /** 055 * The study is undergoing design but the process of selecting study subjects and capturing data has not yet begun. 056 */ 057 DRAFT, 058 /** 059 * The study is currently being executed 060 */ 061 INPROGRESS, 062 /** 063 * Execution of the study has been temporarily paused 064 */ 065 SUSPENDED, 066 /** 067 * The study was terminated prior to the final determination of results 068 */ 069 STOPPED, 070 /** 071 * The information sought by the study has been gathered and compiled and no further work is being performed 072 */ 073 COMPLETED, 074 /** 075 * This study never actually existed. The record is retained for tracking purposes in the event decisions may have been made based on this erroneous information. 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static ResearchStudyStatus fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("in-progress".equals(codeString)) 088 return INPROGRESS; 089 if ("suspended".equals(codeString)) 090 return SUSPENDED; 091 if ("stopped".equals(codeString)) 092 return STOPPED; 093 if ("completed".equals(codeString)) 094 return COMPLETED; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if (Configuration.isAcceptInvalidEnums()) 098 return null; 099 else 100 throw new FHIRException("Unknown ResearchStudyStatus code '"+codeString+"'"); 101 } 102 public String toCode() { 103 switch (this) { 104 case DRAFT: return "draft"; 105 case INPROGRESS: return "in-progress"; 106 case SUSPENDED: return "suspended"; 107 case STOPPED: return "stopped"; 108 case COMPLETED: return "completed"; 109 case ENTEREDINERROR: return "entered-in-error"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getSystem() { 115 switch (this) { 116 case DRAFT: return "http://hl7.org/fhir/research-study-status"; 117 case INPROGRESS: return "http://hl7.org/fhir/research-study-status"; 118 case SUSPENDED: return "http://hl7.org/fhir/research-study-status"; 119 case STOPPED: return "http://hl7.org/fhir/research-study-status"; 120 case COMPLETED: return "http://hl7.org/fhir/research-study-status"; 121 case ENTEREDINERROR: return "http://hl7.org/fhir/research-study-status"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 public String getDefinition() { 127 switch (this) { 128 case DRAFT: return "The study is undergoing design but the process of selecting study subjects and capturing data has not yet begun."; 129 case INPROGRESS: return "The study is currently being executed"; 130 case SUSPENDED: return "Execution of the study has been temporarily paused"; 131 case STOPPED: return "The study was terminated prior to the final determination of results"; 132 case COMPLETED: return "The information sought by the study has been gathered and compiled and no further work is being performed"; 133 case ENTEREDINERROR: return "This study never actually existed. The record is retained for tracking purposes in the event decisions may have been made based on this erroneous information."; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case DRAFT: return "Draft"; 141 case INPROGRESS: return "In-progress"; 142 case SUSPENDED: return "Suspended"; 143 case STOPPED: return "Stopped"; 144 case COMPLETED: return "Completed"; 145 case ENTEREDINERROR: return "Entered in error"; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 } 151 152 public static class ResearchStudyStatusEnumFactory implements EnumFactory<ResearchStudyStatus> { 153 public ResearchStudyStatus fromCode(String codeString) throws IllegalArgumentException { 154 if (codeString == null || "".equals(codeString)) 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("draft".equals(codeString)) 158 return ResearchStudyStatus.DRAFT; 159 if ("in-progress".equals(codeString)) 160 return ResearchStudyStatus.INPROGRESS; 161 if ("suspended".equals(codeString)) 162 return ResearchStudyStatus.SUSPENDED; 163 if ("stopped".equals(codeString)) 164 return ResearchStudyStatus.STOPPED; 165 if ("completed".equals(codeString)) 166 return ResearchStudyStatus.COMPLETED; 167 if ("entered-in-error".equals(codeString)) 168 return ResearchStudyStatus.ENTEREDINERROR; 169 throw new IllegalArgumentException("Unknown ResearchStudyStatus code '"+codeString+"'"); 170 } 171 public Enumeration<ResearchStudyStatus> fromType(PrimitiveType<?> code) throws FHIRException { 172 if (code == null) 173 return null; 174 if (code.isEmpty()) 175 return new Enumeration<ResearchStudyStatus>(this); 176 String codeString = code.asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("draft".equals(codeString)) 180 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.DRAFT); 181 if ("in-progress".equals(codeString)) 182 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.INPROGRESS); 183 if ("suspended".equals(codeString)) 184 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.SUSPENDED); 185 if ("stopped".equals(codeString)) 186 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.STOPPED); 187 if ("completed".equals(codeString)) 188 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.COMPLETED); 189 if ("entered-in-error".equals(codeString)) 190 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.ENTEREDINERROR); 191 throw new FHIRException("Unknown ResearchStudyStatus code '"+codeString+"'"); 192 } 193 public String toCode(ResearchStudyStatus code) { 194 if (code == ResearchStudyStatus.NULL) 195 return null; 196 if (code == ResearchStudyStatus.DRAFT) 197 return "draft"; 198 if (code == ResearchStudyStatus.INPROGRESS) 199 return "in-progress"; 200 if (code == ResearchStudyStatus.SUSPENDED) 201 return "suspended"; 202 if (code == ResearchStudyStatus.STOPPED) 203 return "stopped"; 204 if (code == ResearchStudyStatus.COMPLETED) 205 return "completed"; 206 if (code == ResearchStudyStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 return "?"; 209 } 210 public String toSystem(ResearchStudyStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 @Block() 216 public static class ResearchStudyArmComponent extends BackboneElement implements IBaseBackboneElement { 217 /** 218 * Unique, human-readable label for this arm of the study. 219 */ 220 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 221 @Description(shortDefinition="Label for study arm", formalDefinition="Unique, human-readable label for this arm of the study." ) 222 protected StringType name; 223 224 /** 225 * Categorization of study arm, e.g. experimental, active comparator, placebo comparater. 226 */ 227 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 228 @Description(shortDefinition="Categorization of study arm", formalDefinition="Categorization of study arm, e.g. experimental, active comparator, placebo comparater." ) 229 protected CodeableConcept code; 230 231 /** 232 * A succinct description of the path through the study that would be followed by a subject adhering to this arm. 233 */ 234 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="Short explanation of study path", formalDefinition="A succinct description of the path through the study that would be followed by a subject adhering to this arm." ) 236 protected StringType description; 237 238 private static final long serialVersionUID = 1433183343L; 239 240 /** 241 * Constructor 242 */ 243 public ResearchStudyArmComponent() { 244 super(); 245 } 246 247 /** 248 * Constructor 249 */ 250 public ResearchStudyArmComponent(StringType name) { 251 super(); 252 this.name = name; 253 } 254 255 /** 256 * @return {@link #name} (Unique, human-readable label for this arm of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 257 */ 258 public StringType getNameElement() { 259 if (this.name == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create ResearchStudyArmComponent.name"); 262 else if (Configuration.doAutoCreate()) 263 this.name = new StringType(); // bb 264 return this.name; 265 } 266 267 public boolean hasNameElement() { 268 return this.name != null && !this.name.isEmpty(); 269 } 270 271 public boolean hasName() { 272 return this.name != null && !this.name.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #name} (Unique, human-readable label for this arm of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 277 */ 278 public ResearchStudyArmComponent setNameElement(StringType value) { 279 this.name = value; 280 return this; 281 } 282 283 /** 284 * @return Unique, human-readable label for this arm of the study. 285 */ 286 public String getName() { 287 return this.name == null ? null : this.name.getValue(); 288 } 289 290 /** 291 * @param value Unique, human-readable label for this arm of the study. 292 */ 293 public ResearchStudyArmComponent setName(String value) { 294 if (this.name == null) 295 this.name = new StringType(); 296 this.name.setValue(value); 297 return this; 298 } 299 300 /** 301 * @return {@link #code} (Categorization of study arm, e.g. experimental, active comparator, placebo comparater.) 302 */ 303 public CodeableConcept getCode() { 304 if (this.code == null) 305 if (Configuration.errorOnAutoCreate()) 306 throw new Error("Attempt to auto-create ResearchStudyArmComponent.code"); 307 else if (Configuration.doAutoCreate()) 308 this.code = new CodeableConcept(); // cc 309 return this.code; 310 } 311 312 public boolean hasCode() { 313 return this.code != null && !this.code.isEmpty(); 314 } 315 316 /** 317 * @param value {@link #code} (Categorization of study arm, e.g. experimental, active comparator, placebo comparater.) 318 */ 319 public ResearchStudyArmComponent setCode(CodeableConcept value) { 320 this.code = value; 321 return this; 322 } 323 324 /** 325 * @return {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this arm.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 326 */ 327 public StringType getDescriptionElement() { 328 if (this.description == null) 329 if (Configuration.errorOnAutoCreate()) 330 throw new Error("Attempt to auto-create ResearchStudyArmComponent.description"); 331 else if (Configuration.doAutoCreate()) 332 this.description = new StringType(); // bb 333 return this.description; 334 } 335 336 public boolean hasDescriptionElement() { 337 return this.description != null && !this.description.isEmpty(); 338 } 339 340 public boolean hasDescription() { 341 return this.description != null && !this.description.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this arm.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 346 */ 347 public ResearchStudyArmComponent setDescriptionElement(StringType value) { 348 this.description = value; 349 return this; 350 } 351 352 /** 353 * @return A succinct description of the path through the study that would be followed by a subject adhering to this arm. 354 */ 355 public String getDescription() { 356 return this.description == null ? null : this.description.getValue(); 357 } 358 359 /** 360 * @param value A succinct description of the path through the study that would be followed by a subject adhering to this arm. 361 */ 362 public ResearchStudyArmComponent setDescription(String value) { 363 if (Utilities.noString(value)) 364 this.description = null; 365 else { 366 if (this.description == null) 367 this.description = new StringType(); 368 this.description.setValue(value); 369 } 370 return this; 371 } 372 373 protected void listChildren(List<Property> children) { 374 super.listChildren(children); 375 children.add(new Property("name", "string", "Unique, human-readable label for this arm of the study.", 0, 1, name)); 376 children.add(new Property("code", "CodeableConcept", "Categorization of study arm, e.g. experimental, active comparator, placebo comparater.", 0, 1, code)); 377 children.add(new Property("description", "string", "A succinct description of the path through the study that would be followed by a subject adhering to this arm.", 0, 1, description)); 378 } 379 380 @Override 381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 382 switch (_hash) { 383 case 3373707: /*name*/ return new Property("name", "string", "Unique, human-readable label for this arm of the study.", 0, 1, name); 384 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Categorization of study arm, e.g. experimental, active comparator, placebo comparater.", 0, 1, code); 385 case -1724546052: /*description*/ return new Property("description", "string", "A succinct description of the path through the study that would be followed by a subject adhering to this arm.", 0, 1, description); 386 default: return super.getNamedProperty(_hash, _name, _checkValid); 387 } 388 389 } 390 391 @Override 392 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 393 switch (hash) { 394 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 395 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 396 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 397 default: return super.getProperty(hash, name, checkValid); 398 } 399 400 } 401 402 @Override 403 public Base setProperty(int hash, String name, Base value) throws FHIRException { 404 switch (hash) { 405 case 3373707: // name 406 this.name = castToString(value); // StringType 407 return value; 408 case 3059181: // code 409 this.code = castToCodeableConcept(value); // CodeableConcept 410 return value; 411 case -1724546052: // description 412 this.description = castToString(value); // StringType 413 return value; 414 default: return super.setProperty(hash, name, value); 415 } 416 417 } 418 419 @Override 420 public Base setProperty(String name, Base value) throws FHIRException { 421 if (name.equals("name")) { 422 this.name = castToString(value); // StringType 423 } else if (name.equals("code")) { 424 this.code = castToCodeableConcept(value); // CodeableConcept 425 } else if (name.equals("description")) { 426 this.description = castToString(value); // StringType 427 } else 428 return super.setProperty(name, value); 429 return value; 430 } 431 432 @Override 433 public Base makeProperty(int hash, String name) throws FHIRException { 434 switch (hash) { 435 case 3373707: return getNameElement(); 436 case 3059181: return getCode(); 437 case -1724546052: return getDescriptionElement(); 438 default: return super.makeProperty(hash, name); 439 } 440 441 } 442 443 @Override 444 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 445 switch (hash) { 446 case 3373707: /*name*/ return new String[] {"string"}; 447 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 448 case -1724546052: /*description*/ return new String[] {"string"}; 449 default: return super.getTypesForProperty(hash, name); 450 } 451 452 } 453 454 @Override 455 public Base addChild(String name) throws FHIRException { 456 if (name.equals("name")) { 457 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.name"); 458 } 459 else if (name.equals("code")) { 460 this.code = new CodeableConcept(); 461 return this.code; 462 } 463 else if (name.equals("description")) { 464 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 465 } 466 else 467 return super.addChild(name); 468 } 469 470 public ResearchStudyArmComponent copy() { 471 ResearchStudyArmComponent dst = new ResearchStudyArmComponent(); 472 copyValues(dst); 473 dst.name = name == null ? null : name.copy(); 474 dst.code = code == null ? null : code.copy(); 475 dst.description = description == null ? null : description.copy(); 476 return dst; 477 } 478 479 @Override 480 public boolean equalsDeep(Base other_) { 481 if (!super.equalsDeep(other_)) 482 return false; 483 if (!(other_ instanceof ResearchStudyArmComponent)) 484 return false; 485 ResearchStudyArmComponent o = (ResearchStudyArmComponent) other_; 486 return compareDeep(name, o.name, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 487 ; 488 } 489 490 @Override 491 public boolean equalsShallow(Base other_) { 492 if (!super.equalsShallow(other_)) 493 return false; 494 if (!(other_ instanceof ResearchStudyArmComponent)) 495 return false; 496 ResearchStudyArmComponent o = (ResearchStudyArmComponent) other_; 497 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 498 } 499 500 public boolean isEmpty() { 501 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, code, description 502 ); 503 } 504 505 public String fhirType() { 506 return "ResearchStudy.arm"; 507 508 } 509 510 } 511 512 /** 513 * Identifiers assigned to this research study by the sponsor or other systems. 514 */ 515 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 516 @Description(shortDefinition="Business Identifier for study", formalDefinition="Identifiers assigned to this research study by the sponsor or other systems." ) 517 protected List<Identifier> identifier; 518 519 /** 520 * A short, descriptive user-friendly label for the study. 521 */ 522 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 523 @Description(shortDefinition="Name for this study", formalDefinition="A short, descriptive user-friendly label for the study." ) 524 protected StringType title; 525 526 /** 527 * The set of steps expected to be performed as part of the execution of the study. 528 */ 529 @Child(name = "protocol", type = {PlanDefinition.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 530 @Description(shortDefinition="Steps followed in executing study", formalDefinition="The set of steps expected to be performed as part of the execution of the study." ) 531 protected List<Reference> protocol; 532 /** 533 * The actual objects that are the target of the reference (The set of steps expected to be performed as part of the execution of the study.) 534 */ 535 protected List<PlanDefinition> protocolTarget; 536 537 538 /** 539 * A larger research study of which this particular study is a component or step. 540 */ 541 @Child(name = "partOf", type = {ResearchStudy.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 542 @Description(shortDefinition="Part of larger study", formalDefinition="A larger research study of which this particular study is a component or step." ) 543 protected List<Reference> partOf; 544 /** 545 * The actual objects that are the target of the reference (A larger research study of which this particular study is a component or step.) 546 */ 547 protected List<ResearchStudy> partOfTarget; 548 549 550 /** 551 * The current state of the study. 552 */ 553 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 554 @Description(shortDefinition="draft | in-progress | suspended | stopped | completed | entered-in-error", formalDefinition="The current state of the study." ) 555 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-status") 556 protected Enumeration<ResearchStudyStatus> status; 557 558 /** 559 * Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc. 560 */ 561 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 562 @Description(shortDefinition="Classifications for the study", formalDefinition="Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc." ) 563 protected List<CodeableConcept> category; 564 565 /** 566 * The condition(s), medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about. 567 */ 568 @Child(name = "focus", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 569 @Description(shortDefinition="Drugs, devices, conditions, etc. under study", formalDefinition="The condition(s), medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about." ) 570 protected List<CodeableConcept> focus; 571 572 /** 573 * Contact details to assist a user in learning more about or engaging with the study. 574 */ 575 @Child(name = "contact", type = {ContactDetail.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 576 @Description(shortDefinition="Contact details for the study", formalDefinition="Contact details to assist a user in learning more about or engaging with the study." ) 577 protected List<ContactDetail> contact; 578 579 /** 580 * Citations, references and other related documents. 581 */ 582 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 583 @Description(shortDefinition="References and dependencies", formalDefinition="Citations, references and other related documents." ) 584 protected List<RelatedArtifact> relatedArtifact; 585 586 /** 587 * Key terms to aid in searching for or filtering the study. 588 */ 589 @Child(name = "keyword", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 590 @Description(shortDefinition="Used to search for the study", formalDefinition="Key terms to aid in searching for or filtering the study." ) 591 protected List<CodeableConcept> keyword; 592 593 /** 594 * Indicates a country, state or other region where the study is taking place. 595 */ 596 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 597 @Description(shortDefinition="Geographic region(s) for study", formalDefinition="Indicates a country, state or other region where the study is taking place." ) 598 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 599 protected List<CodeableConcept> jurisdiction; 600 601 /** 602 * A full description of how the study is being conducted. 603 */ 604 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 605 @Description(shortDefinition="What this is study doing", formalDefinition="A full description of how the study is being conducted." ) 606 protected MarkdownType description; 607 608 /** 609 * Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. " 200 female Europeans between the ages of 20 and 45 with early onset diabetes". 610 */ 611 @Child(name = "enrollment", type = {Group.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 612 @Description(shortDefinition="Inclusion & exclusion criteria", formalDefinition="Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\"." ) 613 protected List<Reference> enrollment; 614 /** 615 * The actual objects that are the target of the reference (Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. " 200 female Europeans between the ages of 20 and 45 with early onset diabetes".) 616 */ 617 protected List<Group> enrollmentTarget; 618 619 620 /** 621 * Identifies the start date and the expected (or actual, depending on status) end date for the study. 622 */ 623 @Child(name = "period", type = {Period.class}, order=13, min=0, max=1, modifier=false, summary=true) 624 @Description(shortDefinition="When the study began and ended", formalDefinition="Identifies the start date and the expected (or actual, depending on status) end date for the study." ) 625 protected Period period; 626 627 /** 628 * The organization responsible for the execution of the study. 629 */ 630 @Child(name = "sponsor", type = {Organization.class}, order=14, min=0, max=1, modifier=false, summary=true) 631 @Description(shortDefinition="Organization responsible for the study", formalDefinition="The organization responsible for the execution of the study." ) 632 protected Reference sponsor; 633 634 /** 635 * The actual object that is the target of the reference (The organization responsible for the execution of the study.) 636 */ 637 protected Organization sponsorTarget; 638 639 /** 640 * Indicates the individual who has primary oversite of the execution of the study. 641 */ 642 @Child(name = "principalInvestigator", type = {Practitioner.class}, order=15, min=0, max=1, modifier=false, summary=true) 643 @Description(shortDefinition="The individual responsible for the study", formalDefinition="Indicates the individual who has primary oversite of the execution of the study." ) 644 protected Reference principalInvestigator; 645 646 /** 647 * The actual object that is the target of the reference (Indicates the individual who has primary oversite of the execution of the study.) 648 */ 649 protected Practitioner principalInvestigatorTarget; 650 651 /** 652 * Clinic, hospital or other healthcare location that is participating in the study. 653 */ 654 @Child(name = "site", type = {Location.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 655 @Description(shortDefinition="Location involved in study execution", formalDefinition="Clinic, hospital or other healthcare location that is participating in the study." ) 656 protected List<Reference> site; 657 /** 658 * The actual objects that are the target of the reference (Clinic, hospital or other healthcare location that is participating in the study.) 659 */ 660 protected List<Location> siteTarget; 661 662 663 /** 664 * A description and/or code explaining the premature termination of the study. 665 */ 666 @Child(name = "reasonStopped", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 667 @Description(shortDefinition="Reason for terminating study early", formalDefinition="A description and/or code explaining the premature termination of the study." ) 668 protected CodeableConcept reasonStopped; 669 670 /** 671 * Comments made about the event by the performer, subject or other participants. 672 */ 673 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 674 @Description(shortDefinition="Comments made about the event", formalDefinition="Comments made about the event by the performer, subject or other participants." ) 675 protected List<Annotation> note; 676 677 /** 678 * Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. 679 */ 680 @Child(name = "arm", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 681 @Description(shortDefinition="Defined path through the study for a subject", formalDefinition="Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up." ) 682 protected List<ResearchStudyArmComponent> arm; 683 684 private static final long serialVersionUID = -1804662501L; 685 686 /** 687 * Constructor 688 */ 689 public ResearchStudy() { 690 super(); 691 } 692 693 /** 694 * Constructor 695 */ 696 public ResearchStudy(Enumeration<ResearchStudyStatus> status) { 697 super(); 698 this.status = status; 699 } 700 701 /** 702 * @return {@link #identifier} (Identifiers assigned to this research study by the sponsor or other systems.) 703 */ 704 public List<Identifier> getIdentifier() { 705 if (this.identifier == null) 706 this.identifier = new ArrayList<Identifier>(); 707 return this.identifier; 708 } 709 710 /** 711 * @return Returns a reference to <code>this</code> for easy method chaining 712 */ 713 public ResearchStudy setIdentifier(List<Identifier> theIdentifier) { 714 this.identifier = theIdentifier; 715 return this; 716 } 717 718 public boolean hasIdentifier() { 719 if (this.identifier == null) 720 return false; 721 for (Identifier item : this.identifier) 722 if (!item.isEmpty()) 723 return true; 724 return false; 725 } 726 727 public Identifier addIdentifier() { //3 728 Identifier t = new Identifier(); 729 if (this.identifier == null) 730 this.identifier = new ArrayList<Identifier>(); 731 this.identifier.add(t); 732 return t; 733 } 734 735 public ResearchStudy addIdentifier(Identifier t) { //3 736 if (t == null) 737 return this; 738 if (this.identifier == null) 739 this.identifier = new ArrayList<Identifier>(); 740 this.identifier.add(t); 741 return this; 742 } 743 744 /** 745 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 746 */ 747 public Identifier getIdentifierFirstRep() { 748 if (getIdentifier().isEmpty()) { 749 addIdentifier(); 750 } 751 return getIdentifier().get(0); 752 } 753 754 /** 755 * @return {@link #title} (A short, descriptive user-friendly label for the study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 756 */ 757 public StringType getTitleElement() { 758 if (this.title == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create ResearchStudy.title"); 761 else if (Configuration.doAutoCreate()) 762 this.title = new StringType(); // bb 763 return this.title; 764 } 765 766 public boolean hasTitleElement() { 767 return this.title != null && !this.title.isEmpty(); 768 } 769 770 public boolean hasTitle() { 771 return this.title != null && !this.title.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #title} (A short, descriptive user-friendly label for the study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 776 */ 777 public ResearchStudy setTitleElement(StringType value) { 778 this.title = value; 779 return this; 780 } 781 782 /** 783 * @return A short, descriptive user-friendly label for the study. 784 */ 785 public String getTitle() { 786 return this.title == null ? null : this.title.getValue(); 787 } 788 789 /** 790 * @param value A short, descriptive user-friendly label for the study. 791 */ 792 public ResearchStudy setTitle(String value) { 793 if (Utilities.noString(value)) 794 this.title = null; 795 else { 796 if (this.title == null) 797 this.title = new StringType(); 798 this.title.setValue(value); 799 } 800 return this; 801 } 802 803 /** 804 * @return {@link #protocol} (The set of steps expected to be performed as part of the execution of the study.) 805 */ 806 public List<Reference> getProtocol() { 807 if (this.protocol == null) 808 this.protocol = new ArrayList<Reference>(); 809 return this.protocol; 810 } 811 812 /** 813 * @return Returns a reference to <code>this</code> for easy method chaining 814 */ 815 public ResearchStudy setProtocol(List<Reference> theProtocol) { 816 this.protocol = theProtocol; 817 return this; 818 } 819 820 public boolean hasProtocol() { 821 if (this.protocol == null) 822 return false; 823 for (Reference item : this.protocol) 824 if (!item.isEmpty()) 825 return true; 826 return false; 827 } 828 829 public Reference addProtocol() { //3 830 Reference t = new Reference(); 831 if (this.protocol == null) 832 this.protocol = new ArrayList<Reference>(); 833 this.protocol.add(t); 834 return t; 835 } 836 837 public ResearchStudy addProtocol(Reference t) { //3 838 if (t == null) 839 return this; 840 if (this.protocol == null) 841 this.protocol = new ArrayList<Reference>(); 842 this.protocol.add(t); 843 return this; 844 } 845 846 /** 847 * @return The first repetition of repeating field {@link #protocol}, creating it if it does not already exist 848 */ 849 public Reference getProtocolFirstRep() { 850 if (getProtocol().isEmpty()) { 851 addProtocol(); 852 } 853 return getProtocol().get(0); 854 } 855 856 /** 857 * @deprecated Use Reference#setResource(IBaseResource) instead 858 */ 859 @Deprecated 860 public List<PlanDefinition> getProtocolTarget() { 861 if (this.protocolTarget == null) 862 this.protocolTarget = new ArrayList<PlanDefinition>(); 863 return this.protocolTarget; 864 } 865 866 /** 867 * @deprecated Use Reference#setResource(IBaseResource) instead 868 */ 869 @Deprecated 870 public PlanDefinition addProtocolTarget() { 871 PlanDefinition r = new PlanDefinition(); 872 if (this.protocolTarget == null) 873 this.protocolTarget = new ArrayList<PlanDefinition>(); 874 this.protocolTarget.add(r); 875 return r; 876 } 877 878 /** 879 * @return {@link #partOf} (A larger research study of which this particular study is a component or step.) 880 */ 881 public List<Reference> getPartOf() { 882 if (this.partOf == null) 883 this.partOf = new ArrayList<Reference>(); 884 return this.partOf; 885 } 886 887 /** 888 * @return Returns a reference to <code>this</code> for easy method chaining 889 */ 890 public ResearchStudy setPartOf(List<Reference> thePartOf) { 891 this.partOf = thePartOf; 892 return this; 893 } 894 895 public boolean hasPartOf() { 896 if (this.partOf == null) 897 return false; 898 for (Reference item : this.partOf) 899 if (!item.isEmpty()) 900 return true; 901 return false; 902 } 903 904 public Reference addPartOf() { //3 905 Reference t = new Reference(); 906 if (this.partOf == null) 907 this.partOf = new ArrayList<Reference>(); 908 this.partOf.add(t); 909 return t; 910 } 911 912 public ResearchStudy addPartOf(Reference t) { //3 913 if (t == null) 914 return this; 915 if (this.partOf == null) 916 this.partOf = new ArrayList<Reference>(); 917 this.partOf.add(t); 918 return this; 919 } 920 921 /** 922 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 923 */ 924 public Reference getPartOfFirstRep() { 925 if (getPartOf().isEmpty()) { 926 addPartOf(); 927 } 928 return getPartOf().get(0); 929 } 930 931 /** 932 * @deprecated Use Reference#setResource(IBaseResource) instead 933 */ 934 @Deprecated 935 public List<ResearchStudy> getPartOfTarget() { 936 if (this.partOfTarget == null) 937 this.partOfTarget = new ArrayList<ResearchStudy>(); 938 return this.partOfTarget; 939 } 940 941 /** 942 * @deprecated Use Reference#setResource(IBaseResource) instead 943 */ 944 @Deprecated 945 public ResearchStudy addPartOfTarget() { 946 ResearchStudy r = new ResearchStudy(); 947 if (this.partOfTarget == null) 948 this.partOfTarget = new ArrayList<ResearchStudy>(); 949 this.partOfTarget.add(r); 950 return r; 951 } 952 953 /** 954 * @return {@link #status} (The current state of the study.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 955 */ 956 public Enumeration<ResearchStudyStatus> getStatusElement() { 957 if (this.status == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create ResearchStudy.status"); 960 else if (Configuration.doAutoCreate()) 961 this.status = new Enumeration<ResearchStudyStatus>(new ResearchStudyStatusEnumFactory()); // bb 962 return this.status; 963 } 964 965 public boolean hasStatusElement() { 966 return this.status != null && !this.status.isEmpty(); 967 } 968 969 public boolean hasStatus() { 970 return this.status != null && !this.status.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #status} (The current state of the study.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 975 */ 976 public ResearchStudy setStatusElement(Enumeration<ResearchStudyStatus> value) { 977 this.status = value; 978 return this; 979 } 980 981 /** 982 * @return The current state of the study. 983 */ 984 public ResearchStudyStatus getStatus() { 985 return this.status == null ? null : this.status.getValue(); 986 } 987 988 /** 989 * @param value The current state of the study. 990 */ 991 public ResearchStudy setStatus(ResearchStudyStatus value) { 992 if (this.status == null) 993 this.status = new Enumeration<ResearchStudyStatus>(new ResearchStudyStatusEnumFactory()); 994 this.status.setValue(value); 995 return this; 996 } 997 998 /** 999 * @return {@link #category} (Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.) 1000 */ 1001 public List<CodeableConcept> getCategory() { 1002 if (this.category == null) 1003 this.category = new ArrayList<CodeableConcept>(); 1004 return this.category; 1005 } 1006 1007 /** 1008 * @return Returns a reference to <code>this</code> for easy method chaining 1009 */ 1010 public ResearchStudy setCategory(List<CodeableConcept> theCategory) { 1011 this.category = theCategory; 1012 return this; 1013 } 1014 1015 public boolean hasCategory() { 1016 if (this.category == null) 1017 return false; 1018 for (CodeableConcept item : this.category) 1019 if (!item.isEmpty()) 1020 return true; 1021 return false; 1022 } 1023 1024 public CodeableConcept addCategory() { //3 1025 CodeableConcept t = new CodeableConcept(); 1026 if (this.category == null) 1027 this.category = new ArrayList<CodeableConcept>(); 1028 this.category.add(t); 1029 return t; 1030 } 1031 1032 public ResearchStudy addCategory(CodeableConcept t) { //3 1033 if (t == null) 1034 return this; 1035 if (this.category == null) 1036 this.category = new ArrayList<CodeableConcept>(); 1037 this.category.add(t); 1038 return this; 1039 } 1040 1041 /** 1042 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 1043 */ 1044 public CodeableConcept getCategoryFirstRep() { 1045 if (getCategory().isEmpty()) { 1046 addCategory(); 1047 } 1048 return getCategory().get(0); 1049 } 1050 1051 /** 1052 * @return {@link #focus} (The condition(s), medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.) 1053 */ 1054 public List<CodeableConcept> getFocus() { 1055 if (this.focus == null) 1056 this.focus = new ArrayList<CodeableConcept>(); 1057 return this.focus; 1058 } 1059 1060 /** 1061 * @return Returns a reference to <code>this</code> for easy method chaining 1062 */ 1063 public ResearchStudy setFocus(List<CodeableConcept> theFocus) { 1064 this.focus = theFocus; 1065 return this; 1066 } 1067 1068 public boolean hasFocus() { 1069 if (this.focus == null) 1070 return false; 1071 for (CodeableConcept item : this.focus) 1072 if (!item.isEmpty()) 1073 return true; 1074 return false; 1075 } 1076 1077 public CodeableConcept addFocus() { //3 1078 CodeableConcept t = new CodeableConcept(); 1079 if (this.focus == null) 1080 this.focus = new ArrayList<CodeableConcept>(); 1081 this.focus.add(t); 1082 return t; 1083 } 1084 1085 public ResearchStudy addFocus(CodeableConcept t) { //3 1086 if (t == null) 1087 return this; 1088 if (this.focus == null) 1089 this.focus = new ArrayList<CodeableConcept>(); 1090 this.focus.add(t); 1091 return this; 1092 } 1093 1094 /** 1095 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist 1096 */ 1097 public CodeableConcept getFocusFirstRep() { 1098 if (getFocus().isEmpty()) { 1099 addFocus(); 1100 } 1101 return getFocus().get(0); 1102 } 1103 1104 /** 1105 * @return {@link #contact} (Contact details to assist a user in learning more about or engaging with the study.) 1106 */ 1107 public List<ContactDetail> getContact() { 1108 if (this.contact == null) 1109 this.contact = new ArrayList<ContactDetail>(); 1110 return this.contact; 1111 } 1112 1113 /** 1114 * @return Returns a reference to <code>this</code> for easy method chaining 1115 */ 1116 public ResearchStudy setContact(List<ContactDetail> theContact) { 1117 this.contact = theContact; 1118 return this; 1119 } 1120 1121 public boolean hasContact() { 1122 if (this.contact == null) 1123 return false; 1124 for (ContactDetail item : this.contact) 1125 if (!item.isEmpty()) 1126 return true; 1127 return false; 1128 } 1129 1130 public ContactDetail addContact() { //3 1131 ContactDetail t = new ContactDetail(); 1132 if (this.contact == null) 1133 this.contact = new ArrayList<ContactDetail>(); 1134 this.contact.add(t); 1135 return t; 1136 } 1137 1138 public ResearchStudy addContact(ContactDetail t) { //3 1139 if (t == null) 1140 return this; 1141 if (this.contact == null) 1142 this.contact = new ArrayList<ContactDetail>(); 1143 this.contact.add(t); 1144 return this; 1145 } 1146 1147 /** 1148 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1149 */ 1150 public ContactDetail getContactFirstRep() { 1151 if (getContact().isEmpty()) { 1152 addContact(); 1153 } 1154 return getContact().get(0); 1155 } 1156 1157 /** 1158 * @return {@link #relatedArtifact} (Citations, references and other related documents.) 1159 */ 1160 public List<RelatedArtifact> getRelatedArtifact() { 1161 if (this.relatedArtifact == null) 1162 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1163 return this.relatedArtifact; 1164 } 1165 1166 /** 1167 * @return Returns a reference to <code>this</code> for easy method chaining 1168 */ 1169 public ResearchStudy setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1170 this.relatedArtifact = theRelatedArtifact; 1171 return this; 1172 } 1173 1174 public boolean hasRelatedArtifact() { 1175 if (this.relatedArtifact == null) 1176 return false; 1177 for (RelatedArtifact item : this.relatedArtifact) 1178 if (!item.isEmpty()) 1179 return true; 1180 return false; 1181 } 1182 1183 public RelatedArtifact addRelatedArtifact() { //3 1184 RelatedArtifact t = new RelatedArtifact(); 1185 if (this.relatedArtifact == null) 1186 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1187 this.relatedArtifact.add(t); 1188 return t; 1189 } 1190 1191 public ResearchStudy addRelatedArtifact(RelatedArtifact t) { //3 1192 if (t == null) 1193 return this; 1194 if (this.relatedArtifact == null) 1195 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1196 this.relatedArtifact.add(t); 1197 return this; 1198 } 1199 1200 /** 1201 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 1202 */ 1203 public RelatedArtifact getRelatedArtifactFirstRep() { 1204 if (getRelatedArtifact().isEmpty()) { 1205 addRelatedArtifact(); 1206 } 1207 return getRelatedArtifact().get(0); 1208 } 1209 1210 /** 1211 * @return {@link #keyword} (Key terms to aid in searching for or filtering the study.) 1212 */ 1213 public List<CodeableConcept> getKeyword() { 1214 if (this.keyword == null) 1215 this.keyword = new ArrayList<CodeableConcept>(); 1216 return this.keyword; 1217 } 1218 1219 /** 1220 * @return Returns a reference to <code>this</code> for easy method chaining 1221 */ 1222 public ResearchStudy setKeyword(List<CodeableConcept> theKeyword) { 1223 this.keyword = theKeyword; 1224 return this; 1225 } 1226 1227 public boolean hasKeyword() { 1228 if (this.keyword == null) 1229 return false; 1230 for (CodeableConcept item : this.keyword) 1231 if (!item.isEmpty()) 1232 return true; 1233 return false; 1234 } 1235 1236 public CodeableConcept addKeyword() { //3 1237 CodeableConcept t = new CodeableConcept(); 1238 if (this.keyword == null) 1239 this.keyword = new ArrayList<CodeableConcept>(); 1240 this.keyword.add(t); 1241 return t; 1242 } 1243 1244 public ResearchStudy addKeyword(CodeableConcept t) { //3 1245 if (t == null) 1246 return this; 1247 if (this.keyword == null) 1248 this.keyword = new ArrayList<CodeableConcept>(); 1249 this.keyword.add(t); 1250 return this; 1251 } 1252 1253 /** 1254 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist 1255 */ 1256 public CodeableConcept getKeywordFirstRep() { 1257 if (getKeyword().isEmpty()) { 1258 addKeyword(); 1259 } 1260 return getKeyword().get(0); 1261 } 1262 1263 /** 1264 * @return {@link #jurisdiction} (Indicates a country, state or other region where the study is taking place.) 1265 */ 1266 public List<CodeableConcept> getJurisdiction() { 1267 if (this.jurisdiction == null) 1268 this.jurisdiction = new ArrayList<CodeableConcept>(); 1269 return this.jurisdiction; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public ResearchStudy setJurisdiction(List<CodeableConcept> theJurisdiction) { 1276 this.jurisdiction = theJurisdiction; 1277 return this; 1278 } 1279 1280 public boolean hasJurisdiction() { 1281 if (this.jurisdiction == null) 1282 return false; 1283 for (CodeableConcept item : this.jurisdiction) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public CodeableConcept addJurisdiction() { //3 1290 CodeableConcept t = new CodeableConcept(); 1291 if (this.jurisdiction == null) 1292 this.jurisdiction = new ArrayList<CodeableConcept>(); 1293 this.jurisdiction.add(t); 1294 return t; 1295 } 1296 1297 public ResearchStudy addJurisdiction(CodeableConcept t) { //3 1298 if (t == null) 1299 return this; 1300 if (this.jurisdiction == null) 1301 this.jurisdiction = new ArrayList<CodeableConcept>(); 1302 this.jurisdiction.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1308 */ 1309 public CodeableConcept getJurisdictionFirstRep() { 1310 if (getJurisdiction().isEmpty()) { 1311 addJurisdiction(); 1312 } 1313 return getJurisdiction().get(0); 1314 } 1315 1316 /** 1317 * @return {@link #description} (A full description of how the study is being conducted.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1318 */ 1319 public MarkdownType getDescriptionElement() { 1320 if (this.description == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create ResearchStudy.description"); 1323 else if (Configuration.doAutoCreate()) 1324 this.description = new MarkdownType(); // bb 1325 return this.description; 1326 } 1327 1328 public boolean hasDescriptionElement() { 1329 return this.description != null && !this.description.isEmpty(); 1330 } 1331 1332 public boolean hasDescription() { 1333 return this.description != null && !this.description.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #description} (A full description of how the study is being conducted.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1338 */ 1339 public ResearchStudy setDescriptionElement(MarkdownType value) { 1340 this.description = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return A full description of how the study is being conducted. 1346 */ 1347 public String getDescription() { 1348 return this.description == null ? null : this.description.getValue(); 1349 } 1350 1351 /** 1352 * @param value A full description of how the study is being conducted. 1353 */ 1354 public ResearchStudy setDescription(String value) { 1355 if (value == null) 1356 this.description = null; 1357 else { 1358 if (this.description == null) 1359 this.description = new MarkdownType(); 1360 this.description.setValue(value); 1361 } 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #enrollment} (Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. " 200 female Europeans between the ages of 20 and 45 with early onset diabetes".) 1367 */ 1368 public List<Reference> getEnrollment() { 1369 if (this.enrollment == null) 1370 this.enrollment = new ArrayList<Reference>(); 1371 return this.enrollment; 1372 } 1373 1374 /** 1375 * @return Returns a reference to <code>this</code> for easy method chaining 1376 */ 1377 public ResearchStudy setEnrollment(List<Reference> theEnrollment) { 1378 this.enrollment = theEnrollment; 1379 return this; 1380 } 1381 1382 public boolean hasEnrollment() { 1383 if (this.enrollment == null) 1384 return false; 1385 for (Reference item : this.enrollment) 1386 if (!item.isEmpty()) 1387 return true; 1388 return false; 1389 } 1390 1391 public Reference addEnrollment() { //3 1392 Reference t = new Reference(); 1393 if (this.enrollment == null) 1394 this.enrollment = new ArrayList<Reference>(); 1395 this.enrollment.add(t); 1396 return t; 1397 } 1398 1399 public ResearchStudy addEnrollment(Reference t) { //3 1400 if (t == null) 1401 return this; 1402 if (this.enrollment == null) 1403 this.enrollment = new ArrayList<Reference>(); 1404 this.enrollment.add(t); 1405 return this; 1406 } 1407 1408 /** 1409 * @return The first repetition of repeating field {@link #enrollment}, creating it if it does not already exist 1410 */ 1411 public Reference getEnrollmentFirstRep() { 1412 if (getEnrollment().isEmpty()) { 1413 addEnrollment(); 1414 } 1415 return getEnrollment().get(0); 1416 } 1417 1418 /** 1419 * @deprecated Use Reference#setResource(IBaseResource) instead 1420 */ 1421 @Deprecated 1422 public List<Group> getEnrollmentTarget() { 1423 if (this.enrollmentTarget == null) 1424 this.enrollmentTarget = new ArrayList<Group>(); 1425 return this.enrollmentTarget; 1426 } 1427 1428 /** 1429 * @deprecated Use Reference#setResource(IBaseResource) instead 1430 */ 1431 @Deprecated 1432 public Group addEnrollmentTarget() { 1433 Group r = new Group(); 1434 if (this.enrollmentTarget == null) 1435 this.enrollmentTarget = new ArrayList<Group>(); 1436 this.enrollmentTarget.add(r); 1437 return r; 1438 } 1439 1440 /** 1441 * @return {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 1442 */ 1443 public Period getPeriod() { 1444 if (this.period == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create ResearchStudy.period"); 1447 else if (Configuration.doAutoCreate()) 1448 this.period = new Period(); // cc 1449 return this.period; 1450 } 1451 1452 public boolean hasPeriod() { 1453 return this.period != null && !this.period.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 1458 */ 1459 public ResearchStudy setPeriod(Period value) { 1460 this.period = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #sponsor} (The organization responsible for the execution of the study.) 1466 */ 1467 public Reference getSponsor() { 1468 if (this.sponsor == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create ResearchStudy.sponsor"); 1471 else if (Configuration.doAutoCreate()) 1472 this.sponsor = new Reference(); // cc 1473 return this.sponsor; 1474 } 1475 1476 public boolean hasSponsor() { 1477 return this.sponsor != null && !this.sponsor.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #sponsor} (The organization responsible for the execution of the study.) 1482 */ 1483 public ResearchStudy setSponsor(Reference value) { 1484 this.sponsor = value; 1485 return this; 1486 } 1487 1488 /** 1489 * @return {@link #sponsor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization responsible for the execution of the study.) 1490 */ 1491 public Organization getSponsorTarget() { 1492 if (this.sponsorTarget == null) 1493 if (Configuration.errorOnAutoCreate()) 1494 throw new Error("Attempt to auto-create ResearchStudy.sponsor"); 1495 else if (Configuration.doAutoCreate()) 1496 this.sponsorTarget = new Organization(); // aa 1497 return this.sponsorTarget; 1498 } 1499 1500 /** 1501 * @param value {@link #sponsor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization responsible for the execution of the study.) 1502 */ 1503 public ResearchStudy setSponsorTarget(Organization value) { 1504 this.sponsorTarget = value; 1505 return this; 1506 } 1507 1508 /** 1509 * @return {@link #principalInvestigator} (Indicates the individual who has primary oversite of the execution of the study.) 1510 */ 1511 public Reference getPrincipalInvestigator() { 1512 if (this.principalInvestigator == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create ResearchStudy.principalInvestigator"); 1515 else if (Configuration.doAutoCreate()) 1516 this.principalInvestigator = new Reference(); // cc 1517 return this.principalInvestigator; 1518 } 1519 1520 public boolean hasPrincipalInvestigator() { 1521 return this.principalInvestigator != null && !this.principalInvestigator.isEmpty(); 1522 } 1523 1524 /** 1525 * @param value {@link #principalInvestigator} (Indicates the individual who has primary oversite of the execution of the study.) 1526 */ 1527 public ResearchStudy setPrincipalInvestigator(Reference value) { 1528 this.principalInvestigator = value; 1529 return this; 1530 } 1531 1532 /** 1533 * @return {@link #principalInvestigator} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the individual who has primary oversite of the execution of the study.) 1534 */ 1535 public Practitioner getPrincipalInvestigatorTarget() { 1536 if (this.principalInvestigatorTarget == null) 1537 if (Configuration.errorOnAutoCreate()) 1538 throw new Error("Attempt to auto-create ResearchStudy.principalInvestigator"); 1539 else if (Configuration.doAutoCreate()) 1540 this.principalInvestigatorTarget = new Practitioner(); // aa 1541 return this.principalInvestigatorTarget; 1542 } 1543 1544 /** 1545 * @param value {@link #principalInvestigator} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the individual who has primary oversite of the execution of the study.) 1546 */ 1547 public ResearchStudy setPrincipalInvestigatorTarget(Practitioner value) { 1548 this.principalInvestigatorTarget = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return {@link #site} (Clinic, hospital or other healthcare location that is participating in the study.) 1554 */ 1555 public List<Reference> getSite() { 1556 if (this.site == null) 1557 this.site = new ArrayList<Reference>(); 1558 return this.site; 1559 } 1560 1561 /** 1562 * @return Returns a reference to <code>this</code> for easy method chaining 1563 */ 1564 public ResearchStudy setSite(List<Reference> theSite) { 1565 this.site = theSite; 1566 return this; 1567 } 1568 1569 public boolean hasSite() { 1570 if (this.site == null) 1571 return false; 1572 for (Reference item : this.site) 1573 if (!item.isEmpty()) 1574 return true; 1575 return false; 1576 } 1577 1578 public Reference addSite() { //3 1579 Reference t = new Reference(); 1580 if (this.site == null) 1581 this.site = new ArrayList<Reference>(); 1582 this.site.add(t); 1583 return t; 1584 } 1585 1586 public ResearchStudy addSite(Reference t) { //3 1587 if (t == null) 1588 return this; 1589 if (this.site == null) 1590 this.site = new ArrayList<Reference>(); 1591 this.site.add(t); 1592 return this; 1593 } 1594 1595 /** 1596 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist 1597 */ 1598 public Reference getSiteFirstRep() { 1599 if (getSite().isEmpty()) { 1600 addSite(); 1601 } 1602 return getSite().get(0); 1603 } 1604 1605 /** 1606 * @deprecated Use Reference#setResource(IBaseResource) instead 1607 */ 1608 @Deprecated 1609 public List<Location> getSiteTarget() { 1610 if (this.siteTarget == null) 1611 this.siteTarget = new ArrayList<Location>(); 1612 return this.siteTarget; 1613 } 1614 1615 /** 1616 * @deprecated Use Reference#setResource(IBaseResource) instead 1617 */ 1618 @Deprecated 1619 public Location addSiteTarget() { 1620 Location r = new Location(); 1621 if (this.siteTarget == null) 1622 this.siteTarget = new ArrayList<Location>(); 1623 this.siteTarget.add(r); 1624 return r; 1625 } 1626 1627 /** 1628 * @return {@link #reasonStopped} (A description and/or code explaining the premature termination of the study.) 1629 */ 1630 public CodeableConcept getReasonStopped() { 1631 if (this.reasonStopped == null) 1632 if (Configuration.errorOnAutoCreate()) 1633 throw new Error("Attempt to auto-create ResearchStudy.reasonStopped"); 1634 else if (Configuration.doAutoCreate()) 1635 this.reasonStopped = new CodeableConcept(); // cc 1636 return this.reasonStopped; 1637 } 1638 1639 public boolean hasReasonStopped() { 1640 return this.reasonStopped != null && !this.reasonStopped.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #reasonStopped} (A description and/or code explaining the premature termination of the study.) 1645 */ 1646 public ResearchStudy setReasonStopped(CodeableConcept value) { 1647 this.reasonStopped = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #note} (Comments made about the event by the performer, subject or other participants.) 1653 */ 1654 public List<Annotation> getNote() { 1655 if (this.note == null) 1656 this.note = new ArrayList<Annotation>(); 1657 return this.note; 1658 } 1659 1660 /** 1661 * @return Returns a reference to <code>this</code> for easy method chaining 1662 */ 1663 public ResearchStudy setNote(List<Annotation> theNote) { 1664 this.note = theNote; 1665 return this; 1666 } 1667 1668 public boolean hasNote() { 1669 if (this.note == null) 1670 return false; 1671 for (Annotation item : this.note) 1672 if (!item.isEmpty()) 1673 return true; 1674 return false; 1675 } 1676 1677 public Annotation addNote() { //3 1678 Annotation t = new Annotation(); 1679 if (this.note == null) 1680 this.note = new ArrayList<Annotation>(); 1681 this.note.add(t); 1682 return t; 1683 } 1684 1685 public ResearchStudy addNote(Annotation t) { //3 1686 if (t == null) 1687 return this; 1688 if (this.note == null) 1689 this.note = new ArrayList<Annotation>(); 1690 this.note.add(t); 1691 return this; 1692 } 1693 1694 /** 1695 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1696 */ 1697 public Annotation getNoteFirstRep() { 1698 if (getNote().isEmpty()) { 1699 addNote(); 1700 } 1701 return getNote().get(0); 1702 } 1703 1704 /** 1705 * @return {@link #arm} (Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.) 1706 */ 1707 public List<ResearchStudyArmComponent> getArm() { 1708 if (this.arm == null) 1709 this.arm = new ArrayList<ResearchStudyArmComponent>(); 1710 return this.arm; 1711 } 1712 1713 /** 1714 * @return Returns a reference to <code>this</code> for easy method chaining 1715 */ 1716 public ResearchStudy setArm(List<ResearchStudyArmComponent> theArm) { 1717 this.arm = theArm; 1718 return this; 1719 } 1720 1721 public boolean hasArm() { 1722 if (this.arm == null) 1723 return false; 1724 for (ResearchStudyArmComponent item : this.arm) 1725 if (!item.isEmpty()) 1726 return true; 1727 return false; 1728 } 1729 1730 public ResearchStudyArmComponent addArm() { //3 1731 ResearchStudyArmComponent t = new ResearchStudyArmComponent(); 1732 if (this.arm == null) 1733 this.arm = new ArrayList<ResearchStudyArmComponent>(); 1734 this.arm.add(t); 1735 return t; 1736 } 1737 1738 public ResearchStudy addArm(ResearchStudyArmComponent t) { //3 1739 if (t == null) 1740 return this; 1741 if (this.arm == null) 1742 this.arm = new ArrayList<ResearchStudyArmComponent>(); 1743 this.arm.add(t); 1744 return this; 1745 } 1746 1747 /** 1748 * @return The first repetition of repeating field {@link #arm}, creating it if it does not already exist 1749 */ 1750 public ResearchStudyArmComponent getArmFirstRep() { 1751 if (getArm().isEmpty()) { 1752 addArm(); 1753 } 1754 return getArm().get(0); 1755 } 1756 1757 protected void listChildren(List<Property> children) { 1758 super.listChildren(children); 1759 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1760 children.add(new Property("title", "string", "A short, descriptive user-friendly label for the study.", 0, 1, title)); 1761 children.add(new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol)); 1762 children.add(new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1763 children.add(new Property("status", "code", "The current state of the study.", 0, 1, status)); 1764 children.add(new Property("category", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1765 children.add(new Property("focus", "CodeableConcept", "The condition(s), medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus)); 1766 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in learning more about or engaging with the study.", 0, java.lang.Integer.MAX_VALUE, contact)); 1767 children.add(new Property("relatedArtifact", "RelatedArtifact", "Citations, references and other related documents.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1768 children.add(new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword)); 1769 children.add(new Property("jurisdiction", "CodeableConcept", "Indicates a country, state or other region where the study is taking place.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1770 children.add(new Property("description", "markdown", "A full description of how the study is being conducted.", 0, 1, description)); 1771 children.add(new Property("enrollment", "Reference(Group)", "Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\".", 0, java.lang.Integer.MAX_VALUE, enrollment)); 1772 children.add(new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period)); 1773 children.add(new Property("sponsor", "Reference(Organization)", "The organization responsible for the execution of the study.", 0, 1, sponsor)); 1774 children.add(new Property("principalInvestigator", "Reference(Practitioner)", "Indicates the individual who has primary oversite of the execution of the study.", 0, 1, principalInvestigator)); 1775 children.add(new Property("site", "Reference(Location)", "Clinic, hospital or other healthcare location that is participating in the study.", 0, java.lang.Integer.MAX_VALUE, site)); 1776 children.add(new Property("reasonStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, reasonStopped)); 1777 children.add(new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1778 children.add(new Property("arm", "", "Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.", 0, java.lang.Integer.MAX_VALUE, arm)); 1779 } 1780 1781 @Override 1782 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1783 switch (_hash) { 1784 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1785 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive user-friendly label for the study.", 0, 1, title); 1786 case -989163880: /*protocol*/ return new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol); 1787 case -995410646: /*partOf*/ return new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1788 case -892481550: /*status*/ return new Property("status", "code", "The current state of the study.", 0, 1, status); 1789 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, category); 1790 case 97604824: /*focus*/ return new Property("focus", "CodeableConcept", "The condition(s), medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus); 1791 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in learning more about or engaging with the study.", 0, java.lang.Integer.MAX_VALUE, contact); 1792 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Citations, references and other related documents.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 1793 case -814408215: /*keyword*/ return new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword); 1794 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "Indicates a country, state or other region where the study is taking place.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1795 case -1724546052: /*description*/ return new Property("description", "markdown", "A full description of how the study is being conducted.", 0, 1, description); 1796 case 116089604: /*enrollment*/ return new Property("enrollment", "Reference(Group)", "Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\".", 0, java.lang.Integer.MAX_VALUE, enrollment); 1797 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period); 1798 case -1998892262: /*sponsor*/ return new Property("sponsor", "Reference(Organization)", "The organization responsible for the execution of the study.", 0, 1, sponsor); 1799 case 1437117175: /*principalInvestigator*/ return new Property("principalInvestigator", "Reference(Practitioner)", "Indicates the individual who has primary oversite of the execution of the study.", 0, 1, principalInvestigator); 1800 case 3530567: /*site*/ return new Property("site", "Reference(Location)", "Clinic, hospital or other healthcare location that is participating in the study.", 0, java.lang.Integer.MAX_VALUE, site); 1801 case 1181369065: /*reasonStopped*/ return new Property("reasonStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, reasonStopped); 1802 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1803 case 96860: /*arm*/ return new Property("arm", "", "Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.", 0, java.lang.Integer.MAX_VALUE, arm); 1804 default: return super.getNamedProperty(_hash, _name, _checkValid); 1805 } 1806 1807 } 1808 1809 @Override 1810 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1811 switch (hash) { 1812 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1813 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1814 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // Reference 1815 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1816 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ResearchStudyStatus> 1817 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1818 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // CodeableConcept 1819 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1820 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 1821 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // CodeableConcept 1822 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1823 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1824 case 116089604: /*enrollment*/ return this.enrollment == null ? new Base[0] : this.enrollment.toArray(new Base[this.enrollment.size()]); // Reference 1825 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1826 case -1998892262: /*sponsor*/ return this.sponsor == null ? new Base[0] : new Base[] {this.sponsor}; // Reference 1827 case 1437117175: /*principalInvestigator*/ return this.principalInvestigator == null ? new Base[0] : new Base[] {this.principalInvestigator}; // Reference 1828 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 1829 case 1181369065: /*reasonStopped*/ return this.reasonStopped == null ? new Base[0] : new Base[] {this.reasonStopped}; // CodeableConcept 1830 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1831 case 96860: /*arm*/ return this.arm == null ? new Base[0] : this.arm.toArray(new Base[this.arm.size()]); // ResearchStudyArmComponent 1832 default: return super.getProperty(hash, name, checkValid); 1833 } 1834 1835 } 1836 1837 @Override 1838 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1839 switch (hash) { 1840 case -1618432855: // identifier 1841 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1842 return value; 1843 case 110371416: // title 1844 this.title = castToString(value); // StringType 1845 return value; 1846 case -989163880: // protocol 1847 this.getProtocol().add(castToReference(value)); // Reference 1848 return value; 1849 case -995410646: // partOf 1850 this.getPartOf().add(castToReference(value)); // Reference 1851 return value; 1852 case -892481550: // status 1853 value = new ResearchStudyStatusEnumFactory().fromType(castToCode(value)); 1854 this.status = (Enumeration) value; // Enumeration<ResearchStudyStatus> 1855 return value; 1856 case 50511102: // category 1857 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1858 return value; 1859 case 97604824: // focus 1860 this.getFocus().add(castToCodeableConcept(value)); // CodeableConcept 1861 return value; 1862 case 951526432: // contact 1863 this.getContact().add(castToContactDetail(value)); // ContactDetail 1864 return value; 1865 case 666807069: // relatedArtifact 1866 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 1867 return value; 1868 case -814408215: // keyword 1869 this.getKeyword().add(castToCodeableConcept(value)); // CodeableConcept 1870 return value; 1871 case -507075711: // jurisdiction 1872 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1873 return value; 1874 case -1724546052: // description 1875 this.description = castToMarkdown(value); // MarkdownType 1876 return value; 1877 case 116089604: // enrollment 1878 this.getEnrollment().add(castToReference(value)); // Reference 1879 return value; 1880 case -991726143: // period 1881 this.period = castToPeriod(value); // Period 1882 return value; 1883 case -1998892262: // sponsor 1884 this.sponsor = castToReference(value); // Reference 1885 return value; 1886 case 1437117175: // principalInvestigator 1887 this.principalInvestigator = castToReference(value); // Reference 1888 return value; 1889 case 3530567: // site 1890 this.getSite().add(castToReference(value)); // Reference 1891 return value; 1892 case 1181369065: // reasonStopped 1893 this.reasonStopped = castToCodeableConcept(value); // CodeableConcept 1894 return value; 1895 case 3387378: // note 1896 this.getNote().add(castToAnnotation(value)); // Annotation 1897 return value; 1898 case 96860: // arm 1899 this.getArm().add((ResearchStudyArmComponent) value); // ResearchStudyArmComponent 1900 return value; 1901 default: return super.setProperty(hash, name, value); 1902 } 1903 1904 } 1905 1906 @Override 1907 public Base setProperty(String name, Base value) throws FHIRException { 1908 if (name.equals("identifier")) { 1909 this.getIdentifier().add(castToIdentifier(value)); 1910 } else if (name.equals("title")) { 1911 this.title = castToString(value); // StringType 1912 } else if (name.equals("protocol")) { 1913 this.getProtocol().add(castToReference(value)); 1914 } else if (name.equals("partOf")) { 1915 this.getPartOf().add(castToReference(value)); 1916 } else if (name.equals("status")) { 1917 value = new ResearchStudyStatusEnumFactory().fromType(castToCode(value)); 1918 this.status = (Enumeration) value; // Enumeration<ResearchStudyStatus> 1919 } else if (name.equals("category")) { 1920 this.getCategory().add(castToCodeableConcept(value)); 1921 } else if (name.equals("focus")) { 1922 this.getFocus().add(castToCodeableConcept(value)); 1923 } else if (name.equals("contact")) { 1924 this.getContact().add(castToContactDetail(value)); 1925 } else if (name.equals("relatedArtifact")) { 1926 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 1927 } else if (name.equals("keyword")) { 1928 this.getKeyword().add(castToCodeableConcept(value)); 1929 } else if (name.equals("jurisdiction")) { 1930 this.getJurisdiction().add(castToCodeableConcept(value)); 1931 } else if (name.equals("description")) { 1932 this.description = castToMarkdown(value); // MarkdownType 1933 } else if (name.equals("enrollment")) { 1934 this.getEnrollment().add(castToReference(value)); 1935 } else if (name.equals("period")) { 1936 this.period = castToPeriod(value); // Period 1937 } else if (name.equals("sponsor")) { 1938 this.sponsor = castToReference(value); // Reference 1939 } else if (name.equals("principalInvestigator")) { 1940 this.principalInvestigator = castToReference(value); // Reference 1941 } else if (name.equals("site")) { 1942 this.getSite().add(castToReference(value)); 1943 } else if (name.equals("reasonStopped")) { 1944 this.reasonStopped = castToCodeableConcept(value); // CodeableConcept 1945 } else if (name.equals("note")) { 1946 this.getNote().add(castToAnnotation(value)); 1947 } else if (name.equals("arm")) { 1948 this.getArm().add((ResearchStudyArmComponent) value); 1949 } else 1950 return super.setProperty(name, value); 1951 return value; 1952 } 1953 1954 @Override 1955 public Base makeProperty(int hash, String name) throws FHIRException { 1956 switch (hash) { 1957 case -1618432855: return addIdentifier(); 1958 case 110371416: return getTitleElement(); 1959 case -989163880: return addProtocol(); 1960 case -995410646: return addPartOf(); 1961 case -892481550: return getStatusElement(); 1962 case 50511102: return addCategory(); 1963 case 97604824: return addFocus(); 1964 case 951526432: return addContact(); 1965 case 666807069: return addRelatedArtifact(); 1966 case -814408215: return addKeyword(); 1967 case -507075711: return addJurisdiction(); 1968 case -1724546052: return getDescriptionElement(); 1969 case 116089604: return addEnrollment(); 1970 case -991726143: return getPeriod(); 1971 case -1998892262: return getSponsor(); 1972 case 1437117175: return getPrincipalInvestigator(); 1973 case 3530567: return addSite(); 1974 case 1181369065: return getReasonStopped(); 1975 case 3387378: return addNote(); 1976 case 96860: return addArm(); 1977 default: return super.makeProperty(hash, name); 1978 } 1979 1980 } 1981 1982 @Override 1983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1984 switch (hash) { 1985 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1986 case 110371416: /*title*/ return new String[] {"string"}; 1987 case -989163880: /*protocol*/ return new String[] {"Reference"}; 1988 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1989 case -892481550: /*status*/ return new String[] {"code"}; 1990 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1991 case 97604824: /*focus*/ return new String[] {"CodeableConcept"}; 1992 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1993 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 1994 case -814408215: /*keyword*/ return new String[] {"CodeableConcept"}; 1995 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1996 case -1724546052: /*description*/ return new String[] {"markdown"}; 1997 case 116089604: /*enrollment*/ return new String[] {"Reference"}; 1998 case -991726143: /*period*/ return new String[] {"Period"}; 1999 case -1998892262: /*sponsor*/ return new String[] {"Reference"}; 2000 case 1437117175: /*principalInvestigator*/ return new String[] {"Reference"}; 2001 case 3530567: /*site*/ return new String[] {"Reference"}; 2002 case 1181369065: /*reasonStopped*/ return new String[] {"CodeableConcept"}; 2003 case 3387378: /*note*/ return new String[] {"Annotation"}; 2004 case 96860: /*arm*/ return new String[] {}; 2005 default: return super.getTypesForProperty(hash, name); 2006 } 2007 2008 } 2009 2010 @Override 2011 public Base addChild(String name) throws FHIRException { 2012 if (name.equals("identifier")) { 2013 return addIdentifier(); 2014 } 2015 else if (name.equals("title")) { 2016 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.title"); 2017 } 2018 else if (name.equals("protocol")) { 2019 return addProtocol(); 2020 } 2021 else if (name.equals("partOf")) { 2022 return addPartOf(); 2023 } 2024 else if (name.equals("status")) { 2025 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.status"); 2026 } 2027 else if (name.equals("category")) { 2028 return addCategory(); 2029 } 2030 else if (name.equals("focus")) { 2031 return addFocus(); 2032 } 2033 else if (name.equals("contact")) { 2034 return addContact(); 2035 } 2036 else if (name.equals("relatedArtifact")) { 2037 return addRelatedArtifact(); 2038 } 2039 else if (name.equals("keyword")) { 2040 return addKeyword(); 2041 } 2042 else if (name.equals("jurisdiction")) { 2043 return addJurisdiction(); 2044 } 2045 else if (name.equals("description")) { 2046 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 2047 } 2048 else if (name.equals("enrollment")) { 2049 return addEnrollment(); 2050 } 2051 else if (name.equals("period")) { 2052 this.period = new Period(); 2053 return this.period; 2054 } 2055 else if (name.equals("sponsor")) { 2056 this.sponsor = new Reference(); 2057 return this.sponsor; 2058 } 2059 else if (name.equals("principalInvestigator")) { 2060 this.principalInvestigator = new Reference(); 2061 return this.principalInvestigator; 2062 } 2063 else if (name.equals("site")) { 2064 return addSite(); 2065 } 2066 else if (name.equals("reasonStopped")) { 2067 this.reasonStopped = new CodeableConcept(); 2068 return this.reasonStopped; 2069 } 2070 else if (name.equals("note")) { 2071 return addNote(); 2072 } 2073 else if (name.equals("arm")) { 2074 return addArm(); 2075 } 2076 else 2077 return super.addChild(name); 2078 } 2079 2080 public String fhirType() { 2081 return "ResearchStudy"; 2082 2083 } 2084 2085 public ResearchStudy copy() { 2086 ResearchStudy dst = new ResearchStudy(); 2087 copyValues(dst); 2088 if (identifier != null) { 2089 dst.identifier = new ArrayList<Identifier>(); 2090 for (Identifier i : identifier) 2091 dst.identifier.add(i.copy()); 2092 }; 2093 dst.title = title == null ? null : title.copy(); 2094 if (protocol != null) { 2095 dst.protocol = new ArrayList<Reference>(); 2096 for (Reference i : protocol) 2097 dst.protocol.add(i.copy()); 2098 }; 2099 if (partOf != null) { 2100 dst.partOf = new ArrayList<Reference>(); 2101 for (Reference i : partOf) 2102 dst.partOf.add(i.copy()); 2103 }; 2104 dst.status = status == null ? null : status.copy(); 2105 if (category != null) { 2106 dst.category = new ArrayList<CodeableConcept>(); 2107 for (CodeableConcept i : category) 2108 dst.category.add(i.copy()); 2109 }; 2110 if (focus != null) { 2111 dst.focus = new ArrayList<CodeableConcept>(); 2112 for (CodeableConcept i : focus) 2113 dst.focus.add(i.copy()); 2114 }; 2115 if (contact != null) { 2116 dst.contact = new ArrayList<ContactDetail>(); 2117 for (ContactDetail i : contact) 2118 dst.contact.add(i.copy()); 2119 }; 2120 if (relatedArtifact != null) { 2121 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2122 for (RelatedArtifact i : relatedArtifact) 2123 dst.relatedArtifact.add(i.copy()); 2124 }; 2125 if (keyword != null) { 2126 dst.keyword = new ArrayList<CodeableConcept>(); 2127 for (CodeableConcept i : keyword) 2128 dst.keyword.add(i.copy()); 2129 }; 2130 if (jurisdiction != null) { 2131 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2132 for (CodeableConcept i : jurisdiction) 2133 dst.jurisdiction.add(i.copy()); 2134 }; 2135 dst.description = description == null ? null : description.copy(); 2136 if (enrollment != null) { 2137 dst.enrollment = new ArrayList<Reference>(); 2138 for (Reference i : enrollment) 2139 dst.enrollment.add(i.copy()); 2140 }; 2141 dst.period = period == null ? null : period.copy(); 2142 dst.sponsor = sponsor == null ? null : sponsor.copy(); 2143 dst.principalInvestigator = principalInvestigator == null ? null : principalInvestigator.copy(); 2144 if (site != null) { 2145 dst.site = new ArrayList<Reference>(); 2146 for (Reference i : site) 2147 dst.site.add(i.copy()); 2148 }; 2149 dst.reasonStopped = reasonStopped == null ? null : reasonStopped.copy(); 2150 if (note != null) { 2151 dst.note = new ArrayList<Annotation>(); 2152 for (Annotation i : note) 2153 dst.note.add(i.copy()); 2154 }; 2155 if (arm != null) { 2156 dst.arm = new ArrayList<ResearchStudyArmComponent>(); 2157 for (ResearchStudyArmComponent i : arm) 2158 dst.arm.add(i.copy()); 2159 }; 2160 return dst; 2161 } 2162 2163 protected ResearchStudy typedCopy() { 2164 return copy(); 2165 } 2166 2167 @Override 2168 public boolean equalsDeep(Base other_) { 2169 if (!super.equalsDeep(other_)) 2170 return false; 2171 if (!(other_ instanceof ResearchStudy)) 2172 return false; 2173 ResearchStudy o = (ResearchStudy) other_; 2174 return compareDeep(identifier, o.identifier, true) && compareDeep(title, o.title, true) && compareDeep(protocol, o.protocol, true) 2175 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2176 && compareDeep(focus, o.focus, true) && compareDeep(contact, o.contact, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 2177 && compareDeep(keyword, o.keyword, true) && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(description, o.description, true) 2178 && compareDeep(enrollment, o.enrollment, true) && compareDeep(period, o.period, true) && compareDeep(sponsor, o.sponsor, true) 2179 && compareDeep(principalInvestigator, o.principalInvestigator, true) && compareDeep(site, o.site, true) 2180 && compareDeep(reasonStopped, o.reasonStopped, true) && compareDeep(note, o.note, true) && compareDeep(arm, o.arm, true) 2181 ; 2182 } 2183 2184 @Override 2185 public boolean equalsShallow(Base other_) { 2186 if (!super.equalsShallow(other_)) 2187 return false; 2188 if (!(other_ instanceof ResearchStudy)) 2189 return false; 2190 ResearchStudy o = (ResearchStudy) other_; 2191 return compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(description, o.description, true) 2192 ; 2193 } 2194 2195 public boolean isEmpty() { 2196 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, title, protocol 2197 , partOf, status, category, focus, contact, relatedArtifact, keyword, jurisdiction 2198 , description, enrollment, period, sponsor, principalInvestigator, site, reasonStopped 2199 , note, arm); 2200 } 2201 2202 @Override 2203 public ResourceType getResourceType() { 2204 return ResourceType.ResearchStudy; 2205 } 2206 2207 /** 2208 * Search parameter: <b>date</b> 2209 * <p> 2210 * Description: <b>When the study began and ended</b><br> 2211 * Type: <b>date</b><br> 2212 * Path: <b>ResearchStudy.period</b><br> 2213 * </p> 2214 */ 2215 @SearchParamDefinition(name="date", path="ResearchStudy.period", description="When the study began and ended", type="date" ) 2216 public static final String SP_DATE = "date"; 2217 /** 2218 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2219 * <p> 2220 * Description: <b>When the study began and ended</b><br> 2221 * Type: <b>date</b><br> 2222 * Path: <b>ResearchStudy.period</b><br> 2223 * </p> 2224 */ 2225 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2226 2227 /** 2228 * Search parameter: <b>identifier</b> 2229 * <p> 2230 * Description: <b>Business Identifier for study</b><br> 2231 * Type: <b>token</b><br> 2232 * Path: <b>ResearchStudy.identifier</b><br> 2233 * </p> 2234 */ 2235 @SearchParamDefinition(name="identifier", path="ResearchStudy.identifier", description="Business Identifier for study", type="token" ) 2236 public static final String SP_IDENTIFIER = "identifier"; 2237 /** 2238 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2239 * <p> 2240 * Description: <b>Business Identifier for study</b><br> 2241 * Type: <b>token</b><br> 2242 * Path: <b>ResearchStudy.identifier</b><br> 2243 * </p> 2244 */ 2245 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2246 2247 /** 2248 * Search parameter: <b>partof</b> 2249 * <p> 2250 * Description: <b>Part of larger study</b><br> 2251 * Type: <b>reference</b><br> 2252 * Path: <b>ResearchStudy.partOf</b><br> 2253 * </p> 2254 */ 2255 @SearchParamDefinition(name="partof", path="ResearchStudy.partOf", description="Part of larger study", type="reference", target={ResearchStudy.class } ) 2256 public static final String SP_PARTOF = "partof"; 2257 /** 2258 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 2259 * <p> 2260 * Description: <b>Part of larger study</b><br> 2261 * Type: <b>reference</b><br> 2262 * Path: <b>ResearchStudy.partOf</b><br> 2263 * </p> 2264 */ 2265 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTOF); 2266 2267/** 2268 * Constant for fluent queries to be used to add include statements. Specifies 2269 * the path value of "<b>ResearchStudy:partof</b>". 2270 */ 2271 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include("ResearchStudy:partof").toLocked(); 2272 2273 /** 2274 * Search parameter: <b>sponsor</b> 2275 * <p> 2276 * Description: <b>Organization responsible for the study</b><br> 2277 * Type: <b>reference</b><br> 2278 * Path: <b>ResearchStudy.sponsor</b><br> 2279 * </p> 2280 */ 2281 @SearchParamDefinition(name="sponsor", path="ResearchStudy.sponsor", description="Organization responsible for the study", type="reference", target={Organization.class } ) 2282 public static final String SP_SPONSOR = "sponsor"; 2283 /** 2284 * <b>Fluent Client</b> search parameter constant for <b>sponsor</b> 2285 * <p> 2286 * Description: <b>Organization responsible for the study</b><br> 2287 * Type: <b>reference</b><br> 2288 * Path: <b>ResearchStudy.sponsor</b><br> 2289 * </p> 2290 */ 2291 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPONSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPONSOR); 2292 2293/** 2294 * Constant for fluent queries to be used to add include statements. Specifies 2295 * the path value of "<b>ResearchStudy:sponsor</b>". 2296 */ 2297 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPONSOR = new ca.uhn.fhir.model.api.Include("ResearchStudy:sponsor").toLocked(); 2298 2299 /** 2300 * Search parameter: <b>jurisdiction</b> 2301 * <p> 2302 * Description: <b>Geographic region(s) for study</b><br> 2303 * Type: <b>token</b><br> 2304 * Path: <b>ResearchStudy.jurisdiction</b><br> 2305 * </p> 2306 */ 2307 @SearchParamDefinition(name="jurisdiction", path="ResearchStudy.jurisdiction", description="Geographic region(s) for study", type="token" ) 2308 public static final String SP_JURISDICTION = "jurisdiction"; 2309 /** 2310 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2311 * <p> 2312 * Description: <b>Geographic region(s) for study</b><br> 2313 * Type: <b>token</b><br> 2314 * Path: <b>ResearchStudy.jurisdiction</b><br> 2315 * </p> 2316 */ 2317 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2318 2319 /** 2320 * Search parameter: <b>focus</b> 2321 * <p> 2322 * Description: <b>Drugs, devices, conditions, etc. under study</b><br> 2323 * Type: <b>token</b><br> 2324 * Path: <b>ResearchStudy.focus</b><br> 2325 * </p> 2326 */ 2327 @SearchParamDefinition(name="focus", path="ResearchStudy.focus", description="Drugs, devices, conditions, etc. under study", type="token" ) 2328 public static final String SP_FOCUS = "focus"; 2329 /** 2330 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2331 * <p> 2332 * Description: <b>Drugs, devices, conditions, etc. under study</b><br> 2333 * Type: <b>token</b><br> 2334 * Path: <b>ResearchStudy.focus</b><br> 2335 * </p> 2336 */ 2337 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS); 2338 2339 /** 2340 * Search parameter: <b>principalinvestigator</b> 2341 * <p> 2342 * Description: <b>The individual responsible for the study</b><br> 2343 * Type: <b>reference</b><br> 2344 * Path: <b>ResearchStudy.principalInvestigator</b><br> 2345 * </p> 2346 */ 2347 @SearchParamDefinition(name="principalinvestigator", path="ResearchStudy.principalInvestigator", description="The individual responsible for the study", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2348 public static final String SP_PRINCIPALINVESTIGATOR = "principalinvestigator"; 2349 /** 2350 * <b>Fluent Client</b> search parameter constant for <b>principalinvestigator</b> 2351 * <p> 2352 * Description: <b>The individual responsible for the study</b><br> 2353 * Type: <b>reference</b><br> 2354 * Path: <b>ResearchStudy.principalInvestigator</b><br> 2355 * </p> 2356 */ 2357 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRINCIPALINVESTIGATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRINCIPALINVESTIGATOR); 2358 2359/** 2360 * Constant for fluent queries to be used to add include statements. Specifies 2361 * the path value of "<b>ResearchStudy:principalinvestigator</b>". 2362 */ 2363 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRINCIPALINVESTIGATOR = new ca.uhn.fhir.model.api.Include("ResearchStudy:principalinvestigator").toLocked(); 2364 2365 /** 2366 * Search parameter: <b>title</b> 2367 * <p> 2368 * Description: <b>Name for this study</b><br> 2369 * Type: <b>string</b><br> 2370 * Path: <b>ResearchStudy.title</b><br> 2371 * </p> 2372 */ 2373 @SearchParamDefinition(name="title", path="ResearchStudy.title", description="Name for this study", type="string" ) 2374 public static final String SP_TITLE = "title"; 2375 /** 2376 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2377 * <p> 2378 * Description: <b>Name for this study</b><br> 2379 * Type: <b>string</b><br> 2380 * Path: <b>ResearchStudy.title</b><br> 2381 * </p> 2382 */ 2383 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2384 2385 /** 2386 * Search parameter: <b>protocol</b> 2387 * <p> 2388 * Description: <b>Steps followed in executing study</b><br> 2389 * Type: <b>reference</b><br> 2390 * Path: <b>ResearchStudy.protocol</b><br> 2391 * </p> 2392 */ 2393 @SearchParamDefinition(name="protocol", path="ResearchStudy.protocol", description="Steps followed in executing study", type="reference", target={PlanDefinition.class } ) 2394 public static final String SP_PROTOCOL = "protocol"; 2395 /** 2396 * <b>Fluent Client</b> search parameter constant for <b>protocol</b> 2397 * <p> 2398 * Description: <b>Steps followed in executing study</b><br> 2399 * Type: <b>reference</b><br> 2400 * Path: <b>ResearchStudy.protocol</b><br> 2401 * </p> 2402 */ 2403 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROTOCOL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROTOCOL); 2404 2405/** 2406 * Constant for fluent queries to be used to add include statements. Specifies 2407 * the path value of "<b>ResearchStudy:protocol</b>". 2408 */ 2409 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROTOCOL = new ca.uhn.fhir.model.api.Include("ResearchStudy:protocol").toLocked(); 2410 2411 /** 2412 * Search parameter: <b>site</b> 2413 * <p> 2414 * Description: <b>Location involved in study execution</b><br> 2415 * Type: <b>reference</b><br> 2416 * Path: <b>ResearchStudy.site</b><br> 2417 * </p> 2418 */ 2419 @SearchParamDefinition(name="site", path="ResearchStudy.site", description="Location involved in study execution", type="reference", target={Location.class } ) 2420 public static final String SP_SITE = "site"; 2421 /** 2422 * <b>Fluent Client</b> search parameter constant for <b>site</b> 2423 * <p> 2424 * Description: <b>Location involved in study execution</b><br> 2425 * Type: <b>reference</b><br> 2426 * Path: <b>ResearchStudy.site</b><br> 2427 * </p> 2428 */ 2429 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SITE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SITE); 2430 2431/** 2432 * Constant for fluent queries to be used to add include statements. Specifies 2433 * the path value of "<b>ResearchStudy:site</b>". 2434 */ 2435 public static final ca.uhn.fhir.model.api.Include INCLUDE_SITE = new ca.uhn.fhir.model.api.Include("ResearchStudy:site").toLocked(); 2436 2437 /** 2438 * Search parameter: <b>category</b> 2439 * <p> 2440 * Description: <b>Classifications for the study</b><br> 2441 * Type: <b>token</b><br> 2442 * Path: <b>ResearchStudy.category</b><br> 2443 * </p> 2444 */ 2445 @SearchParamDefinition(name="category", path="ResearchStudy.category", description="Classifications for the study", type="token" ) 2446 public static final String SP_CATEGORY = "category"; 2447 /** 2448 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2449 * <p> 2450 * Description: <b>Classifications for the study</b><br> 2451 * Type: <b>token</b><br> 2452 * Path: <b>ResearchStudy.category</b><br> 2453 * </p> 2454 */ 2455 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2456 2457 /** 2458 * Search parameter: <b>keyword</b> 2459 * <p> 2460 * Description: <b>Used to search for the study</b><br> 2461 * Type: <b>token</b><br> 2462 * Path: <b>ResearchStudy.keyword</b><br> 2463 * </p> 2464 */ 2465 @SearchParamDefinition(name="keyword", path="ResearchStudy.keyword", description="Used to search for the study", type="token" ) 2466 public static final String SP_KEYWORD = "keyword"; 2467 /** 2468 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 2469 * <p> 2470 * Description: <b>Used to search for the study</b><br> 2471 * Type: <b>token</b><br> 2472 * Path: <b>ResearchStudy.keyword</b><br> 2473 * </p> 2474 */ 2475 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 2476 2477 /** 2478 * Search parameter: <b>status</b> 2479 * <p> 2480 * Description: <b>draft | in-progress | suspended | stopped | completed | entered-in-error</b><br> 2481 * Type: <b>token</b><br> 2482 * Path: <b>ResearchStudy.status</b><br> 2483 * </p> 2484 */ 2485 @SearchParamDefinition(name="status", path="ResearchStudy.status", description="draft | in-progress | suspended | stopped | completed | entered-in-error", type="token" ) 2486 public static final String SP_STATUS = "status"; 2487 /** 2488 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2489 * <p> 2490 * Description: <b>draft | in-progress | suspended | stopped | completed | entered-in-error</b><br> 2491 * Type: <b>token</b><br> 2492 * Path: <b>ResearchStudy.status</b><br> 2493 * </p> 2494 */ 2495 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2496 2497 2498}