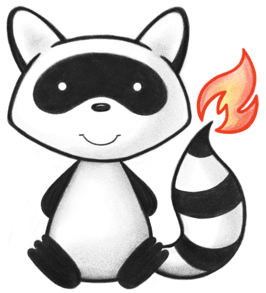
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044/** 045 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 046 */ 047@ResourceDef(name="ResearchSubject", profile="http://hl7.org/fhir/Profile/ResearchSubject") 048public class ResearchSubject extends DomainResource { 049 050 public enum ResearchSubjectStatus { 051 /** 052 * The subject has been identified as a potential participant in the study but has not yet agreed to participate 053 */ 054 CANDIDATE, 055 /** 056 * The subject has agreed to participate in the study but has not yet begun performing any action within the study 057 */ 058 ENROLLED, 059 /** 060 * The subject is currently being monitored and/or subject to treatment as part of the study 061 */ 062 ACTIVE, 063 /** 064 * The subject has temporarily discontinued monitoring/treatment as part of the study 065 */ 066 SUSPENDED, 067 /** 068 * The subject has permanently ended participation in the study prior to completion of the intended monitoring/treatment 069 */ 070 WITHDRAWN, 071 /** 072 * All intended monitoring/treatment of the subject has been completed and their engagement with the study is now ended 073 */ 074 COMPLETED, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static ResearchSubjectStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("candidate".equals(codeString)) 083 return CANDIDATE; 084 if ("enrolled".equals(codeString)) 085 return ENROLLED; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("suspended".equals(codeString)) 089 return SUSPENDED; 090 if ("withdrawn".equals(codeString)) 091 return WITHDRAWN; 092 if ("completed".equals(codeString)) 093 return COMPLETED; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ResearchSubjectStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case CANDIDATE: return "candidate"; 102 case ENROLLED: return "enrolled"; 103 case ACTIVE: return "active"; 104 case SUSPENDED: return "suspended"; 105 case WITHDRAWN: return "withdrawn"; 106 case COMPLETED: return "completed"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case CANDIDATE: return "http://hl7.org/fhir/research-subject-status"; 114 case ENROLLED: return "http://hl7.org/fhir/research-subject-status"; 115 case ACTIVE: return "http://hl7.org/fhir/research-subject-status"; 116 case SUSPENDED: return "http://hl7.org/fhir/research-subject-status"; 117 case WITHDRAWN: return "http://hl7.org/fhir/research-subject-status"; 118 case COMPLETED: return "http://hl7.org/fhir/research-subject-status"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDefinition() { 124 switch (this) { 125 case CANDIDATE: return "The subject has been identified as a potential participant in the study but has not yet agreed to participate"; 126 case ENROLLED: return "The subject has agreed to participate in the study but has not yet begun performing any action within the study"; 127 case ACTIVE: return "The subject is currently being monitored and/or subject to treatment as part of the study"; 128 case SUSPENDED: return "The subject has temporarily discontinued monitoring/treatment as part of the study"; 129 case WITHDRAWN: return "The subject has permanently ended participation in the study prior to completion of the intended monitoring/treatment"; 130 case COMPLETED: return "All intended monitoring/treatment of the subject has been completed and their engagement with the study is now ended"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDisplay() { 136 switch (this) { 137 case CANDIDATE: return "Candidate"; 138 case ENROLLED: return "Enrolled"; 139 case ACTIVE: return "Active"; 140 case SUSPENDED: return "Suspended"; 141 case WITHDRAWN: return "Withdrawn"; 142 case COMPLETED: return "Completed"; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 } 148 149 public static class ResearchSubjectStatusEnumFactory implements EnumFactory<ResearchSubjectStatus> { 150 public ResearchSubjectStatus fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("candidate".equals(codeString)) 155 return ResearchSubjectStatus.CANDIDATE; 156 if ("enrolled".equals(codeString)) 157 return ResearchSubjectStatus.ENROLLED; 158 if ("active".equals(codeString)) 159 return ResearchSubjectStatus.ACTIVE; 160 if ("suspended".equals(codeString)) 161 return ResearchSubjectStatus.SUSPENDED; 162 if ("withdrawn".equals(codeString)) 163 return ResearchSubjectStatus.WITHDRAWN; 164 if ("completed".equals(codeString)) 165 return ResearchSubjectStatus.COMPLETED; 166 throw new IllegalArgumentException("Unknown ResearchSubjectStatus code '"+codeString+"'"); 167 } 168 public Enumeration<ResearchSubjectStatus> fromType(PrimitiveType<?> code) throws FHIRException { 169 if (code == null) 170 return null; 171 if (code.isEmpty()) 172 return new Enumeration<ResearchSubjectStatus>(this); 173 String codeString = code.asStringValue(); 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("candidate".equals(codeString)) 177 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.CANDIDATE); 178 if ("enrolled".equals(codeString)) 179 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ENROLLED); 180 if ("active".equals(codeString)) 181 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ACTIVE); 182 if ("suspended".equals(codeString)) 183 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.SUSPENDED); 184 if ("withdrawn".equals(codeString)) 185 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.WITHDRAWN); 186 if ("completed".equals(codeString)) 187 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.COMPLETED); 188 throw new FHIRException("Unknown ResearchSubjectStatus code '"+codeString+"'"); 189 } 190 public String toCode(ResearchSubjectStatus code) { 191 if (code == ResearchSubjectStatus.NULL) 192 return null; 193 if (code == ResearchSubjectStatus.CANDIDATE) 194 return "candidate"; 195 if (code == ResearchSubjectStatus.ENROLLED) 196 return "enrolled"; 197 if (code == ResearchSubjectStatus.ACTIVE) 198 return "active"; 199 if (code == ResearchSubjectStatus.SUSPENDED) 200 return "suspended"; 201 if (code == ResearchSubjectStatus.WITHDRAWN) 202 return "withdrawn"; 203 if (code == ResearchSubjectStatus.COMPLETED) 204 return "completed"; 205 return "?"; 206 } 207 public String toSystem(ResearchSubjectStatus code) { 208 return code.getSystem(); 209 } 210 } 211 212 /** 213 * Identifiers assigned to this research study by the sponsor or other systems. 214 */ 215 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 216 @Description(shortDefinition="Business Identifier for research subject", formalDefinition="Identifiers assigned to this research study by the sponsor or other systems." ) 217 protected Identifier identifier; 218 219 /** 220 * The current state of the subject. 221 */ 222 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 223 @Description(shortDefinition="candidate | enrolled | active | suspended | withdrawn | completed", formalDefinition="The current state of the subject." ) 224 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-subject-status") 225 protected Enumeration<ResearchSubjectStatus> status; 226 227 /** 228 * The dates the subject began and ended their participation in the study. 229 */ 230 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 231 @Description(shortDefinition="Start and end of participation", formalDefinition="The dates the subject began and ended their participation in the study." ) 232 protected Period period; 233 234 /** 235 * Reference to the study the subject is participating in. 236 */ 237 @Child(name = "study", type = {ResearchStudy.class}, order=3, min=1, max=1, modifier=false, summary=true) 238 @Description(shortDefinition="Study subject is part of", formalDefinition="Reference to the study the subject is participating in." ) 239 protected Reference study; 240 241 /** 242 * The actual object that is the target of the reference (Reference to the study the subject is participating in.) 243 */ 244 protected ResearchStudy studyTarget; 245 246 /** 247 * The record of the person or animal who is involved in the study. 248 */ 249 @Child(name = "individual", type = {Patient.class}, order=4, min=1, max=1, modifier=false, summary=true) 250 @Description(shortDefinition="Who is part of study", formalDefinition="The record of the person or animal who is involved in the study." ) 251 protected Reference individual; 252 253 /** 254 * The actual object that is the target of the reference (The record of the person or animal who is involved in the study.) 255 */ 256 protected Patient individualTarget; 257 258 /** 259 * The name of the arm in the study the subject is expected to follow as part of this study. 260 */ 261 @Child(name = "assignedArm", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 262 @Description(shortDefinition="What path should be followed", formalDefinition="The name of the arm in the study the subject is expected to follow as part of this study." ) 263 protected StringType assignedArm; 264 265 /** 266 * The name of the arm in the study the subject actually followed as part of this study. 267 */ 268 @Child(name = "actualArm", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 269 @Description(shortDefinition="What path was followed", formalDefinition="The name of the arm in the study the subject actually followed as part of this study." ) 270 protected StringType actualArm; 271 272 /** 273 * A record of the patient's informed agreement to participate in the study. 274 */ 275 @Child(name = "consent", type = {Consent.class}, order=7, min=0, max=1, modifier=false, summary=false) 276 @Description(shortDefinition="Agreement to participate in study", formalDefinition="A record of the patient's informed agreement to participate in the study." ) 277 protected Reference consent; 278 279 /** 280 * The actual object that is the target of the reference (A record of the patient's informed agreement to participate in the study.) 281 */ 282 protected Consent consentTarget; 283 284 private static final long serialVersionUID = -1730128953L; 285 286 /** 287 * Constructor 288 */ 289 public ResearchSubject() { 290 super(); 291 } 292 293 /** 294 * Constructor 295 */ 296 public ResearchSubject(Enumeration<ResearchSubjectStatus> status, Reference study, Reference individual) { 297 super(); 298 this.status = status; 299 this.study = study; 300 this.individual = individual; 301 } 302 303 /** 304 * @return {@link #identifier} (Identifiers assigned to this research study by the sponsor or other systems.) 305 */ 306 public Identifier getIdentifier() { 307 if (this.identifier == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create ResearchSubject.identifier"); 310 else if (Configuration.doAutoCreate()) 311 this.identifier = new Identifier(); // cc 312 return this.identifier; 313 } 314 315 public boolean hasIdentifier() { 316 return this.identifier != null && !this.identifier.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #identifier} (Identifiers assigned to this research study by the sponsor or other systems.) 321 */ 322 public ResearchSubject setIdentifier(Identifier value) { 323 this.identifier = value; 324 return this; 325 } 326 327 /** 328 * @return {@link #status} (The current state of the subject.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 329 */ 330 public Enumeration<ResearchSubjectStatus> getStatusElement() { 331 if (this.status == null) 332 if (Configuration.errorOnAutoCreate()) 333 throw new Error("Attempt to auto-create ResearchSubject.status"); 334 else if (Configuration.doAutoCreate()) 335 this.status = new Enumeration<ResearchSubjectStatus>(new ResearchSubjectStatusEnumFactory()); // bb 336 return this.status; 337 } 338 339 public boolean hasStatusElement() { 340 return this.status != null && !this.status.isEmpty(); 341 } 342 343 public boolean hasStatus() { 344 return this.status != null && !this.status.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #status} (The current state of the subject.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 349 */ 350 public ResearchSubject setStatusElement(Enumeration<ResearchSubjectStatus> value) { 351 this.status = value; 352 return this; 353 } 354 355 /** 356 * @return The current state of the subject. 357 */ 358 public ResearchSubjectStatus getStatus() { 359 return this.status == null ? null : this.status.getValue(); 360 } 361 362 /** 363 * @param value The current state of the subject. 364 */ 365 public ResearchSubject setStatus(ResearchSubjectStatus value) { 366 if (this.status == null) 367 this.status = new Enumeration<ResearchSubjectStatus>(new ResearchSubjectStatusEnumFactory()); 368 this.status.setValue(value); 369 return this; 370 } 371 372 /** 373 * @return {@link #period} (The dates the subject began and ended their participation in the study.) 374 */ 375 public Period getPeriod() { 376 if (this.period == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create ResearchSubject.period"); 379 else if (Configuration.doAutoCreate()) 380 this.period = new Period(); // cc 381 return this.period; 382 } 383 384 public boolean hasPeriod() { 385 return this.period != null && !this.period.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #period} (The dates the subject began and ended their participation in the study.) 390 */ 391 public ResearchSubject setPeriod(Period value) { 392 this.period = value; 393 return this; 394 } 395 396 /** 397 * @return {@link #study} (Reference to the study the subject is participating in.) 398 */ 399 public Reference getStudy() { 400 if (this.study == null) 401 if (Configuration.errorOnAutoCreate()) 402 throw new Error("Attempt to auto-create ResearchSubject.study"); 403 else if (Configuration.doAutoCreate()) 404 this.study = new Reference(); // cc 405 return this.study; 406 } 407 408 public boolean hasStudy() { 409 return this.study != null && !this.study.isEmpty(); 410 } 411 412 /** 413 * @param value {@link #study} (Reference to the study the subject is participating in.) 414 */ 415 public ResearchSubject setStudy(Reference value) { 416 this.study = value; 417 return this; 418 } 419 420 /** 421 * @return {@link #study} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the study the subject is participating in.) 422 */ 423 public ResearchStudy getStudyTarget() { 424 if (this.studyTarget == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create ResearchSubject.study"); 427 else if (Configuration.doAutoCreate()) 428 this.studyTarget = new ResearchStudy(); // aa 429 return this.studyTarget; 430 } 431 432 /** 433 * @param value {@link #study} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the study the subject is participating in.) 434 */ 435 public ResearchSubject setStudyTarget(ResearchStudy value) { 436 this.studyTarget = value; 437 return this; 438 } 439 440 /** 441 * @return {@link #individual} (The record of the person or animal who is involved in the study.) 442 */ 443 public Reference getIndividual() { 444 if (this.individual == null) 445 if (Configuration.errorOnAutoCreate()) 446 throw new Error("Attempt to auto-create ResearchSubject.individual"); 447 else if (Configuration.doAutoCreate()) 448 this.individual = new Reference(); // cc 449 return this.individual; 450 } 451 452 public boolean hasIndividual() { 453 return this.individual != null && !this.individual.isEmpty(); 454 } 455 456 /** 457 * @param value {@link #individual} (The record of the person or animal who is involved in the study.) 458 */ 459 public ResearchSubject setIndividual(Reference value) { 460 this.individual = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #individual} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The record of the person or animal who is involved in the study.) 466 */ 467 public Patient getIndividualTarget() { 468 if (this.individualTarget == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create ResearchSubject.individual"); 471 else if (Configuration.doAutoCreate()) 472 this.individualTarget = new Patient(); // aa 473 return this.individualTarget; 474 } 475 476 /** 477 * @param value {@link #individual} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The record of the person or animal who is involved in the study.) 478 */ 479 public ResearchSubject setIndividualTarget(Patient value) { 480 this.individualTarget = value; 481 return this; 482 } 483 484 /** 485 * @return {@link #assignedArm} (The name of the arm in the study the subject is expected to follow as part of this study.). This is the underlying object with id, value and extensions. The accessor "getAssignedArm" gives direct access to the value 486 */ 487 public StringType getAssignedArmElement() { 488 if (this.assignedArm == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create ResearchSubject.assignedArm"); 491 else if (Configuration.doAutoCreate()) 492 this.assignedArm = new StringType(); // bb 493 return this.assignedArm; 494 } 495 496 public boolean hasAssignedArmElement() { 497 return this.assignedArm != null && !this.assignedArm.isEmpty(); 498 } 499 500 public boolean hasAssignedArm() { 501 return this.assignedArm != null && !this.assignedArm.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #assignedArm} (The name of the arm in the study the subject is expected to follow as part of this study.). This is the underlying object with id, value and extensions. The accessor "getAssignedArm" gives direct access to the value 506 */ 507 public ResearchSubject setAssignedArmElement(StringType value) { 508 this.assignedArm = value; 509 return this; 510 } 511 512 /** 513 * @return The name of the arm in the study the subject is expected to follow as part of this study. 514 */ 515 public String getAssignedArm() { 516 return this.assignedArm == null ? null : this.assignedArm.getValue(); 517 } 518 519 /** 520 * @param value The name of the arm in the study the subject is expected to follow as part of this study. 521 */ 522 public ResearchSubject setAssignedArm(String value) { 523 if (Utilities.noString(value)) 524 this.assignedArm = null; 525 else { 526 if (this.assignedArm == null) 527 this.assignedArm = new StringType(); 528 this.assignedArm.setValue(value); 529 } 530 return this; 531 } 532 533 /** 534 * @return {@link #actualArm} (The name of the arm in the study the subject actually followed as part of this study.). This is the underlying object with id, value and extensions. The accessor "getActualArm" gives direct access to the value 535 */ 536 public StringType getActualArmElement() { 537 if (this.actualArm == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create ResearchSubject.actualArm"); 540 else if (Configuration.doAutoCreate()) 541 this.actualArm = new StringType(); // bb 542 return this.actualArm; 543 } 544 545 public boolean hasActualArmElement() { 546 return this.actualArm != null && !this.actualArm.isEmpty(); 547 } 548 549 public boolean hasActualArm() { 550 return this.actualArm != null && !this.actualArm.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #actualArm} (The name of the arm in the study the subject actually followed as part of this study.). This is the underlying object with id, value and extensions. The accessor "getActualArm" gives direct access to the value 555 */ 556 public ResearchSubject setActualArmElement(StringType value) { 557 this.actualArm = value; 558 return this; 559 } 560 561 /** 562 * @return The name of the arm in the study the subject actually followed as part of this study. 563 */ 564 public String getActualArm() { 565 return this.actualArm == null ? null : this.actualArm.getValue(); 566 } 567 568 /** 569 * @param value The name of the arm in the study the subject actually followed as part of this study. 570 */ 571 public ResearchSubject setActualArm(String value) { 572 if (Utilities.noString(value)) 573 this.actualArm = null; 574 else { 575 if (this.actualArm == null) 576 this.actualArm = new StringType(); 577 this.actualArm.setValue(value); 578 } 579 return this; 580 } 581 582 /** 583 * @return {@link #consent} (A record of the patient's informed agreement to participate in the study.) 584 */ 585 public Reference getConsent() { 586 if (this.consent == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create ResearchSubject.consent"); 589 else if (Configuration.doAutoCreate()) 590 this.consent = new Reference(); // cc 591 return this.consent; 592 } 593 594 public boolean hasConsent() { 595 return this.consent != null && !this.consent.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #consent} (A record of the patient's informed agreement to participate in the study.) 600 */ 601 public ResearchSubject setConsent(Reference value) { 602 this.consent = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #consent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A record of the patient's informed agreement to participate in the study.) 608 */ 609 public Consent getConsentTarget() { 610 if (this.consentTarget == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create ResearchSubject.consent"); 613 else if (Configuration.doAutoCreate()) 614 this.consentTarget = new Consent(); // aa 615 return this.consentTarget; 616 } 617 618 /** 619 * @param value {@link #consent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A record of the patient's informed agreement to participate in the study.) 620 */ 621 public ResearchSubject setConsentTarget(Consent value) { 622 this.consentTarget = value; 623 return this; 624 } 625 626 protected void listChildren(List<Property> children) { 627 super.listChildren(children); 628 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, 1, identifier)); 629 children.add(new Property("status", "code", "The current state of the subject.", 0, 1, status)); 630 children.add(new Property("period", "Period", "The dates the subject began and ended their participation in the study.", 0, 1, period)); 631 children.add(new Property("study", "Reference(ResearchStudy)", "Reference to the study the subject is participating in.", 0, 1, study)); 632 children.add(new Property("individual", "Reference(Patient)", "The record of the person or animal who is involved in the study.", 0, 1, individual)); 633 children.add(new Property("assignedArm", "string", "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, assignedArm)); 634 children.add(new Property("actualArm", "string", "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualArm)); 635 children.add(new Property("consent", "Reference(Consent)", "A record of the patient's informed agreement to participate in the study.", 0, 1, consent)); 636 } 637 638 @Override 639 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 640 switch (_hash) { 641 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, 1, identifier); 642 case -892481550: /*status*/ return new Property("status", "code", "The current state of the subject.", 0, 1, status); 643 case -991726143: /*period*/ return new Property("period", "Period", "The dates the subject began and ended their participation in the study.", 0, 1, period); 644 case 109776329: /*study*/ return new Property("study", "Reference(ResearchStudy)", "Reference to the study the subject is participating in.", 0, 1, study); 645 case -46292327: /*individual*/ return new Property("individual", "Reference(Patient)", "The record of the person or animal who is involved in the study.", 0, 1, individual); 646 case 1741912494: /*assignedArm*/ return new Property("assignedArm", "string", "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, assignedArm); 647 case 528827886: /*actualArm*/ return new Property("actualArm", "string", "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualArm); 648 case 951500826: /*consent*/ return new Property("consent", "Reference(Consent)", "A record of the patient's informed agreement to participate in the study.", 0, 1, consent); 649 default: return super.getNamedProperty(_hash, _name, _checkValid); 650 } 651 652 } 653 654 @Override 655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 656 switch (hash) { 657 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 658 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ResearchSubjectStatus> 659 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 660 case 109776329: /*study*/ return this.study == null ? new Base[0] : new Base[] {this.study}; // Reference 661 case -46292327: /*individual*/ return this.individual == null ? new Base[0] : new Base[] {this.individual}; // Reference 662 case 1741912494: /*assignedArm*/ return this.assignedArm == null ? new Base[0] : new Base[] {this.assignedArm}; // StringType 663 case 528827886: /*actualArm*/ return this.actualArm == null ? new Base[0] : new Base[] {this.actualArm}; // StringType 664 case 951500826: /*consent*/ return this.consent == null ? new Base[0] : new Base[] {this.consent}; // Reference 665 default: return super.getProperty(hash, name, checkValid); 666 } 667 668 } 669 670 @Override 671 public Base setProperty(int hash, String name, Base value) throws FHIRException { 672 switch (hash) { 673 case -1618432855: // identifier 674 this.identifier = castToIdentifier(value); // Identifier 675 return value; 676 case -892481550: // status 677 value = new ResearchSubjectStatusEnumFactory().fromType(castToCode(value)); 678 this.status = (Enumeration) value; // Enumeration<ResearchSubjectStatus> 679 return value; 680 case -991726143: // period 681 this.period = castToPeriod(value); // Period 682 return value; 683 case 109776329: // study 684 this.study = castToReference(value); // Reference 685 return value; 686 case -46292327: // individual 687 this.individual = castToReference(value); // Reference 688 return value; 689 case 1741912494: // assignedArm 690 this.assignedArm = castToString(value); // StringType 691 return value; 692 case 528827886: // actualArm 693 this.actualArm = castToString(value); // StringType 694 return value; 695 case 951500826: // consent 696 this.consent = castToReference(value); // Reference 697 return value; 698 default: return super.setProperty(hash, name, value); 699 } 700 701 } 702 703 @Override 704 public Base setProperty(String name, Base value) throws FHIRException { 705 if (name.equals("identifier")) { 706 this.identifier = castToIdentifier(value); // Identifier 707 } else if (name.equals("status")) { 708 value = new ResearchSubjectStatusEnumFactory().fromType(castToCode(value)); 709 this.status = (Enumeration) value; // Enumeration<ResearchSubjectStatus> 710 } else if (name.equals("period")) { 711 this.period = castToPeriod(value); // Period 712 } else if (name.equals("study")) { 713 this.study = castToReference(value); // Reference 714 } else if (name.equals("individual")) { 715 this.individual = castToReference(value); // Reference 716 } else if (name.equals("assignedArm")) { 717 this.assignedArm = castToString(value); // StringType 718 } else if (name.equals("actualArm")) { 719 this.actualArm = castToString(value); // StringType 720 } else if (name.equals("consent")) { 721 this.consent = castToReference(value); // Reference 722 } else 723 return super.setProperty(name, value); 724 return value; 725 } 726 727 @Override 728 public Base makeProperty(int hash, String name) throws FHIRException { 729 switch (hash) { 730 case -1618432855: return getIdentifier(); 731 case -892481550: return getStatusElement(); 732 case -991726143: return getPeriod(); 733 case 109776329: return getStudy(); 734 case -46292327: return getIndividual(); 735 case 1741912494: return getAssignedArmElement(); 736 case 528827886: return getActualArmElement(); 737 case 951500826: return getConsent(); 738 default: return super.makeProperty(hash, name); 739 } 740 741 } 742 743 @Override 744 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 745 switch (hash) { 746 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 747 case -892481550: /*status*/ return new String[] {"code"}; 748 case -991726143: /*period*/ return new String[] {"Period"}; 749 case 109776329: /*study*/ return new String[] {"Reference"}; 750 case -46292327: /*individual*/ return new String[] {"Reference"}; 751 case 1741912494: /*assignedArm*/ return new String[] {"string"}; 752 case 528827886: /*actualArm*/ return new String[] {"string"}; 753 case 951500826: /*consent*/ return new String[] {"Reference"}; 754 default: return super.getTypesForProperty(hash, name); 755 } 756 757 } 758 759 @Override 760 public Base addChild(String name) throws FHIRException { 761 if (name.equals("identifier")) { 762 this.identifier = new Identifier(); 763 return this.identifier; 764 } 765 else if (name.equals("status")) { 766 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.status"); 767 } 768 else if (name.equals("period")) { 769 this.period = new Period(); 770 return this.period; 771 } 772 else if (name.equals("study")) { 773 this.study = new Reference(); 774 return this.study; 775 } 776 else if (name.equals("individual")) { 777 this.individual = new Reference(); 778 return this.individual; 779 } 780 else if (name.equals("assignedArm")) { 781 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.assignedArm"); 782 } 783 else if (name.equals("actualArm")) { 784 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.actualArm"); 785 } 786 else if (name.equals("consent")) { 787 this.consent = new Reference(); 788 return this.consent; 789 } 790 else 791 return super.addChild(name); 792 } 793 794 public String fhirType() { 795 return "ResearchSubject"; 796 797 } 798 799 public ResearchSubject copy() { 800 ResearchSubject dst = new ResearchSubject(); 801 copyValues(dst); 802 dst.identifier = identifier == null ? null : identifier.copy(); 803 dst.status = status == null ? null : status.copy(); 804 dst.period = period == null ? null : period.copy(); 805 dst.study = study == null ? null : study.copy(); 806 dst.individual = individual == null ? null : individual.copy(); 807 dst.assignedArm = assignedArm == null ? null : assignedArm.copy(); 808 dst.actualArm = actualArm == null ? null : actualArm.copy(); 809 dst.consent = consent == null ? null : consent.copy(); 810 return dst; 811 } 812 813 protected ResearchSubject typedCopy() { 814 return copy(); 815 } 816 817 @Override 818 public boolean equalsDeep(Base other_) { 819 if (!super.equalsDeep(other_)) 820 return false; 821 if (!(other_ instanceof ResearchSubject)) 822 return false; 823 ResearchSubject o = (ResearchSubject) other_; 824 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(period, o.period, true) 825 && compareDeep(study, o.study, true) && compareDeep(individual, o.individual, true) && compareDeep(assignedArm, o.assignedArm, true) 826 && compareDeep(actualArm, o.actualArm, true) && compareDeep(consent, o.consent, true); 827 } 828 829 @Override 830 public boolean equalsShallow(Base other_) { 831 if (!super.equalsShallow(other_)) 832 return false; 833 if (!(other_ instanceof ResearchSubject)) 834 return false; 835 ResearchSubject o = (ResearchSubject) other_; 836 return compareValues(status, o.status, true) && compareValues(assignedArm, o.assignedArm, true) && compareValues(actualArm, o.actualArm, true) 837 ; 838 } 839 840 public boolean isEmpty() { 841 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, period 842 , study, individual, assignedArm, actualArm, consent); 843 } 844 845 @Override 846 public ResourceType getResourceType() { 847 return ResourceType.ResearchSubject; 848 } 849 850 /** 851 * Search parameter: <b>date</b> 852 * <p> 853 * Description: <b>Start and end of participation</b><br> 854 * Type: <b>date</b><br> 855 * Path: <b>ResearchSubject.period</b><br> 856 * </p> 857 */ 858 @SearchParamDefinition(name="date", path="ResearchSubject.period", description="Start and end of participation", type="date" ) 859 public static final String SP_DATE = "date"; 860 /** 861 * <b>Fluent Client</b> search parameter constant for <b>date</b> 862 * <p> 863 * Description: <b>Start and end of participation</b><br> 864 * Type: <b>date</b><br> 865 * Path: <b>ResearchSubject.period</b><br> 866 * </p> 867 */ 868 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 869 870 /** 871 * Search parameter: <b>identifier</b> 872 * <p> 873 * Description: <b>Business Identifier for research subject</b><br> 874 * Type: <b>token</b><br> 875 * Path: <b>ResearchSubject.identifier</b><br> 876 * </p> 877 */ 878 @SearchParamDefinition(name="identifier", path="ResearchSubject.identifier", description="Business Identifier for research subject", type="token" ) 879 public static final String SP_IDENTIFIER = "identifier"; 880 /** 881 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 882 * <p> 883 * Description: <b>Business Identifier for research subject</b><br> 884 * Type: <b>token</b><br> 885 * Path: <b>ResearchSubject.identifier</b><br> 886 * </p> 887 */ 888 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 889 890 /** 891 * Search parameter: <b>individual</b> 892 * <p> 893 * Description: <b>Who is part of study</b><br> 894 * Type: <b>reference</b><br> 895 * Path: <b>ResearchSubject.individual</b><br> 896 * </p> 897 */ 898 @SearchParamDefinition(name="individual", path="ResearchSubject.individual", description="Who is part of study", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 899 public static final String SP_INDIVIDUAL = "individual"; 900 /** 901 * <b>Fluent Client</b> search parameter constant for <b>individual</b> 902 * <p> 903 * Description: <b>Who is part of study</b><br> 904 * Type: <b>reference</b><br> 905 * Path: <b>ResearchSubject.individual</b><br> 906 * </p> 907 */ 908 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INDIVIDUAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INDIVIDUAL); 909 910/** 911 * Constant for fluent queries to be used to add include statements. Specifies 912 * the path value of "<b>ResearchSubject:individual</b>". 913 */ 914 public static final ca.uhn.fhir.model.api.Include INCLUDE_INDIVIDUAL = new ca.uhn.fhir.model.api.Include("ResearchSubject:individual").toLocked(); 915 916 /** 917 * Search parameter: <b>patient</b> 918 * <p> 919 * Description: <b>Who is part of study</b><br> 920 * Type: <b>reference</b><br> 921 * Path: <b>ResearchSubject.individual</b><br> 922 * </p> 923 */ 924 @SearchParamDefinition(name="patient", path="ResearchSubject.individual", description="Who is part of study", type="reference", target={Patient.class } ) 925 public static final String SP_PATIENT = "patient"; 926 /** 927 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 928 * <p> 929 * Description: <b>Who is part of study</b><br> 930 * Type: <b>reference</b><br> 931 * Path: <b>ResearchSubject.individual</b><br> 932 * </p> 933 */ 934 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 935 936/** 937 * Constant for fluent queries to be used to add include statements. Specifies 938 * the path value of "<b>ResearchSubject:patient</b>". 939 */ 940 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ResearchSubject:patient").toLocked(); 941 942 /** 943 * Search parameter: <b>status</b> 944 * <p> 945 * Description: <b>candidate | enrolled | active | suspended | withdrawn | completed</b><br> 946 * Type: <b>token</b><br> 947 * Path: <b>ResearchSubject.status</b><br> 948 * </p> 949 */ 950 @SearchParamDefinition(name="status", path="ResearchSubject.status", description="candidate | enrolled | active | suspended | withdrawn | completed", type="token" ) 951 public static final String SP_STATUS = "status"; 952 /** 953 * <b>Fluent Client</b> search parameter constant for <b>status</b> 954 * <p> 955 * Description: <b>candidate | enrolled | active | suspended | withdrawn | completed</b><br> 956 * Type: <b>token</b><br> 957 * Path: <b>ResearchSubject.status</b><br> 958 * </p> 959 */ 960 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 961 962 963}