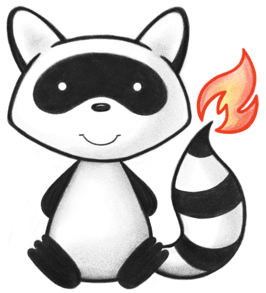
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IAnyResource; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043/** 044 * This is the base resource type for everything. 045 */ 046public abstract class Resource extends BaseResource implements IAnyResource { 047 048 /** 049 * The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes. 050 */ 051 @Child(name = "id", type = {IdType.class}, order=0, min=0, max=1, modifier=false, summary=true) 052 @Description(shortDefinition="Logical id of this artifact", formalDefinition="The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes." ) 053 protected IdType id; 054 055 /** 056 * The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 057 */ 058 @Child(name = "meta", type = {Meta.class}, order=1, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Metadata about the resource", formalDefinition="The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource." ) 060 protected Meta meta; 061 062 /** 063 * A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. 064 */ 065 @Child(name = "implicitRules", type = {UriType.class}, order=2, min=0, max=1, modifier=true, summary=true) 066 @Description(shortDefinition="A set of rules under which this content was created", formalDefinition="A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content." ) 067 protected UriType implicitRules; 068 069 /** 070 * The base language in which the resource is written. 071 */ 072 @Child(name = "language", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 073 @Description(shortDefinition="Language of the resource content", formalDefinition="The base language in which the resource is written." ) 074 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 075 protected CodeType language; 076 077 private static final long serialVersionUID = -559462759L; 078 079 /** 080 * Constructor 081 */ 082 public Resource() { 083 super(); 084 } 085 086 /** 087 * @return {@link #id} (The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 088 */ 089 public IdType getIdElement() { 090 if (this.id == null) 091 if (Configuration.errorOnAutoCreate()) 092 throw new Error("Attempt to auto-create Resource.id"); 093 else if (Configuration.doAutoCreate()) 094 this.id = new IdType(); // bb 095 return this.id; 096 } 097 098 public boolean hasIdElement() { 099 return this.id != null && !this.id.isEmpty(); 100 } 101 102 public boolean hasId() { 103 return this.id != null && !this.id.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #id} (The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 108 */ 109 public Resource setIdElement(IdType value) { 110 this.id = value; 111 return this; 112 } 113 114 /** 115 * @return The id value of the resource. Once assigned, this value never 116 * changes. 117 * 118 * @see IdType 119 * @see IdType#getValue() 120 */ 121 public String getId() { 122 return this.id == null ? null : this.id.getValue(); 123 } 124 125 /** 126 * @return The most complete id value of the resource, containing all 127 * available context and history. Once assigned this value never changes. 128 * NOTE: this value is NOT limited to just the logical id property of a 129 * resource id. 130 * @see IdType 131 * @see IdType#getValue() 132 */ 133 public Resource setId(String value) { 134 if (Utilities.noString(value)) 135 this.id = null; 136 else { 137 if (this.id == null) 138 this.id = new IdType(); 139 this.id.setValue(value); 140 } 141 return this; 142 } 143 144 /** 145 * @return the logical ID part of this resource's id 146 * @see IdType#getIdPart() 147 */ 148 public String getIdPart() { 149 return getIdElement().getIdPart(); 150 } 151 152 /** 153 * @return {@link #meta} (The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.) 154 */ 155 public Meta getMeta() { 156 if (this.meta == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create Resource.meta"); 159 else if (Configuration.doAutoCreate()) 160 this.meta = new Meta(); // cc 161 return this.meta; 162 } 163 164 public boolean hasMeta() { 165 return this.meta != null && !this.meta.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #meta} (The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.) 170 */ 171 public Resource setMeta(Meta value) { 172 this.meta = value; 173 return this; 174 } 175 176 /** 177 * @return {@link #implicitRules} (A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.). This is the underlying object with id, value and extensions. The accessor "getImplicitRules" gives direct access to the value 178 */ 179 public UriType getImplicitRulesElement() { 180 if (this.implicitRules == null) 181 if (Configuration.errorOnAutoCreate()) 182 throw new Error("Attempt to auto-create Resource.implicitRules"); 183 else if (Configuration.doAutoCreate()) 184 this.implicitRules = new UriType(); // bb 185 return this.implicitRules; 186 } 187 188 public boolean hasImplicitRulesElement() { 189 return this.implicitRules != null && !this.implicitRules.isEmpty(); 190 } 191 192 public boolean hasImplicitRules() { 193 return this.implicitRules != null && !this.implicitRules.isEmpty(); 194 } 195 196 /** 197 * @param value {@link #implicitRules} (A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.). This is the underlying object with id, value and extensions. The accessor "getImplicitRules" gives direct access to the value 198 */ 199 public Resource setImplicitRulesElement(UriType value) { 200 this.implicitRules = value; 201 return this; 202 } 203 204 /** 205 * @return A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. 206 */ 207 public String getImplicitRules() { 208 return this.implicitRules == null ? null : this.implicitRules.getValue(); 209 } 210 211 /** 212 * @param value A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. 213 */ 214 public Resource setImplicitRules(String value) { 215 if (Utilities.noString(value)) 216 this.implicitRules = null; 217 else { 218 if (this.implicitRules == null) 219 this.implicitRules = new UriType(); 220 this.implicitRules.setValue(value); 221 } 222 return this; 223 } 224 225 /** 226 * @return {@link #language} (The base language in which the resource is written.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 227 */ 228 public CodeType getLanguageElement() { 229 if (this.language == null) 230 if (Configuration.errorOnAutoCreate()) 231 throw new Error("Attempt to auto-create Resource.language"); 232 else if (Configuration.doAutoCreate()) 233 this.language = new CodeType(); // bb 234 return this.language; 235 } 236 237 public boolean hasLanguageElement() { 238 return this.language != null && !this.language.isEmpty(); 239 } 240 241 public boolean hasLanguage() { 242 return this.language != null && !this.language.isEmpty(); 243 } 244 245 /** 246 * @param value {@link #language} (The base language in which the resource is written.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 247 */ 248 public Resource setLanguageElement(CodeType value) { 249 this.language = value; 250 return this; 251 } 252 253 /** 254 * @return The base language in which the resource is written. 255 */ 256 public String getLanguage() { 257 return this.language == null ? null : this.language.getValue(); 258 } 259 260 /** 261 * @param value The base language in which the resource is written. 262 */ 263 public Resource setLanguage(String value) { 264 if (Utilities.noString(value)) 265 this.language = null; 266 else { 267 if (this.language == null) 268 this.language = new CodeType(); 269 this.language.setValue(value); 270 } 271 return this; 272 } 273 274 protected void listChildren(List<Property> children) { 275 children.add(new Property("id", "id", "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 0, 1, id)); 276 children.add(new Property("meta", "Meta", "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.", 0, 1, meta)); 277 children.add(new Property("implicitRules", "uri", "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.", 0, 1, implicitRules)); 278 children.add(new Property("language", "code", "The base language in which the resource is written.", 0, 1, language)); 279 } 280 281 @Override 282 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 283 switch (_hash) { 284 case 3355: /*id*/ return new Property("id", "id", "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 0, 1, id); 285 case 3347973: /*meta*/ return new Property("meta", "Meta", "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.", 0, 1, meta); 286 case -961826286: /*implicitRules*/ return new Property("implicitRules", "uri", "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.", 0, 1, implicitRules); 287 case -1613589672: /*language*/ return new Property("language", "code", "The base language in which the resource is written.", 0, 1, language); 288 default: return super.getNamedProperty(_hash, _name, _checkValid); 289 } 290 291 } 292 293 @Override 294 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 295 switch (hash) { 296 case 3355: /*id*/ return this.id == null ? new Base[0] : new Base[] {this.id}; // IdType 297 case 3347973: /*meta*/ return this.meta == null ? new Base[0] : new Base[] {this.meta}; // Meta 298 case -961826286: /*implicitRules*/ return this.implicitRules == null ? new Base[0] : new Base[] {this.implicitRules}; // UriType 299 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 300 default: return super.getProperty(hash, name, checkValid); 301 } 302 303 } 304 305 @Override 306 public Base setProperty(int hash, String name, Base value) throws FHIRException { 307 switch (hash) { 308 case 3355: // id 309 this.id = castToId(value); // IdType 310 return value; 311 case 3347973: // meta 312 this.meta = castToMeta(value); // Meta 313 return value; 314 case -961826286: // implicitRules 315 this.implicitRules = castToUri(value); // UriType 316 return value; 317 case -1613589672: // language 318 this.language = castToCode(value); // CodeType 319 return value; 320 default: return super.setProperty(hash, name, value); 321 } 322 323 } 324 325 @Override 326 public Base setProperty(String name, Base value) throws FHIRException { 327 if (name.equals("id")) { 328 this.id = castToId(value); // IdType 329 } else if (name.equals("meta")) { 330 this.meta = castToMeta(value); // Meta 331 } else if (name.equals("implicitRules")) { 332 this.implicitRules = castToUri(value); // UriType 333 } else if (name.equals("language")) { 334 this.language = castToCode(value); // CodeType 335 } else 336 return super.setProperty(name, value); 337 return value; 338 } 339 340 @Override 341 public Base makeProperty(int hash, String name) throws FHIRException { 342 switch (hash) { 343 case 3355: return getIdElement(); 344 case 3347973: return getMeta(); 345 case -961826286: return getImplicitRulesElement(); 346 case -1613589672: return getLanguageElement(); 347 default: return super.makeProperty(hash, name); 348 } 349 350 } 351 352 @Override 353 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 354 switch (hash) { 355 case 3355: /*id*/ return new String[] {"id"}; 356 case 3347973: /*meta*/ return new String[] {"Meta"}; 357 case -961826286: /*implicitRules*/ return new String[] {"uri"}; 358 case -1613589672: /*language*/ return new String[] {"code"}; 359 default: return super.getTypesForProperty(hash, name); 360 } 361 362 } 363 364 @Override 365 public Base addChild(String name) throws FHIRException { 366 if (name.equals("id")) { 367 throw new FHIRException("Cannot call addChild on a singleton property Resource.id"); 368 } 369 else if (name.equals("meta")) { 370 this.meta = new Meta(); 371 return this.meta; 372 } 373 else if (name.equals("implicitRules")) { 374 throw new FHIRException("Cannot call addChild on a singleton property Resource.implicitRules"); 375 } 376 else if (name.equals("language")) { 377 throw new FHIRException("Cannot call addChild on a singleton property Resource.language"); 378 } 379 else 380 return super.addChild(name); 381 } 382 383 public String fhirType() { 384 return "Resource"; 385 386 } 387 388 public abstract Resource copy(); 389 390 public void copyValues(Resource dst) { 391 dst.id = id == null ? null : id.copy(); 392 dst.meta = meta == null ? null : meta.copy(); 393 dst.implicitRules = implicitRules == null ? null : implicitRules.copy(); 394 dst.language = language == null ? null : language.copy(); 395 } 396 397 @Override 398 public boolean equalsDeep(Base other_) { 399 if (!super.equalsDeep(other_)) 400 return false; 401 if (!(other_ instanceof Resource)) 402 return false; 403 Resource o = (Resource) other_; 404 return compareDeep(id, o.id, true) && compareDeep(meta, o.meta, true) && compareDeep(implicitRules, o.implicitRules, true) 405 && compareDeep(language, o.language, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other_) { 410 if (!super.equalsShallow(other_)) 411 return false; 412 if (!(other_ instanceof Resource)) 413 return false; 414 Resource o = (Resource) other_; 415 return compareValues(id, o.id, true) && compareValues(implicitRules, o.implicitRules, true) && compareValues(language, o.language, true) 416 ; 417 } 418 419 public boolean isEmpty() { 420 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, meta, implicitRules 421 , language); 422 } 423 424 425 @Override 426 public String getIdBase() { 427 return getId(); 428 } 429 430 @Override 431 public void setIdBase(String value) { 432 setId(value); 433 } 434 public abstract ResourceType getResourceType(); 435 436}