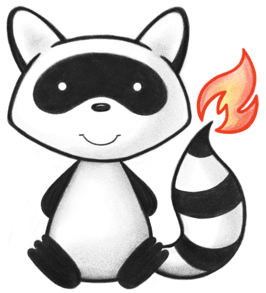
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.List; 040 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 053 */ 054@ResourceDef(name="RiskAssessment", profile="http://hl7.org/fhir/Profile/RiskAssessment") 055public class RiskAssessment extends DomainResource { 056 057 public enum RiskAssessmentStatus { 058 /** 059 * The existence of the observation is registered, but there is no result yet available. 060 */ 061 REGISTERED, 062 /** 063 * This is an initial or interim observation: data may be incomplete or unverified. 064 */ 065 PRELIMINARY, 066 /** 067 * The observation is complete. 068 */ 069 FINAL, 070 /** 071 * Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections. 072 */ 073 AMENDED, 074 /** 075 * Subsequent to being Final, the observation has been modified to correct an error in the test result. 076 */ 077 CORRECTED, 078 /** 079 * The observation is unavailable because the measurement was not started or not completed (also sometimes called "aborted"). 080 */ 081 CANCELLED, 082 /** 083 * The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, but the authoring system does not know which. 088 */ 089 UNKNOWN, 090 /** 091 * added to help the parsers with the generic types 092 */ 093 NULL; 094 public static RiskAssessmentStatus fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("registered".equals(codeString)) 098 return REGISTERED; 099 if ("preliminary".equals(codeString)) 100 return PRELIMINARY; 101 if ("final".equals(codeString)) 102 return FINAL; 103 if ("amended".equals(codeString)) 104 return AMENDED; 105 if ("corrected".equals(codeString)) 106 return CORRECTED; 107 if ("cancelled".equals(codeString)) 108 return CANCELLED; 109 if ("entered-in-error".equals(codeString)) 110 return ENTEREDINERROR; 111 if ("unknown".equals(codeString)) 112 return UNKNOWN; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown RiskAssessmentStatus code '"+codeString+"'"); 117 } 118 public String toCode() { 119 switch (this) { 120 case REGISTERED: return "registered"; 121 case PRELIMINARY: return "preliminary"; 122 case FINAL: return "final"; 123 case AMENDED: return "amended"; 124 case CORRECTED: return "corrected"; 125 case CANCELLED: return "cancelled"; 126 case ENTEREDINERROR: return "entered-in-error"; 127 case UNKNOWN: return "unknown"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getSystem() { 133 switch (this) { 134 case REGISTERED: return "http://hl7.org/fhir/observation-status"; 135 case PRELIMINARY: return "http://hl7.org/fhir/observation-status"; 136 case FINAL: return "http://hl7.org/fhir/observation-status"; 137 case AMENDED: return "http://hl7.org/fhir/observation-status"; 138 case CORRECTED: return "http://hl7.org/fhir/observation-status"; 139 case CANCELLED: return "http://hl7.org/fhir/observation-status"; 140 case ENTEREDINERROR: return "http://hl7.org/fhir/observation-status"; 141 case UNKNOWN: return "http://hl7.org/fhir/observation-status"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDefinition() { 147 switch (this) { 148 case REGISTERED: return "The existence of the observation is registered, but there is no result yet available."; 149 case PRELIMINARY: return "This is an initial or interim observation: data may be incomplete or unverified."; 150 case FINAL: return "The observation is complete."; 151 case AMENDED: return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 152 case CORRECTED: return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 153 case CANCELLED: return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 154 case ENTEREDINERROR: return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 155 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring system does not know which."; 156 case NULL: return null; 157 default: return "?"; 158 } 159 } 160 public String getDisplay() { 161 switch (this) { 162 case REGISTERED: return "Registered"; 163 case PRELIMINARY: return "Preliminary"; 164 case FINAL: return "Final"; 165 case AMENDED: return "Amended"; 166 case CORRECTED: return "Corrected"; 167 case CANCELLED: return "Cancelled"; 168 case ENTEREDINERROR: return "Entered in Error"; 169 case UNKNOWN: return "Unknown"; 170 case NULL: return null; 171 default: return "?"; 172 } 173 } 174 } 175 176 public static class RiskAssessmentStatusEnumFactory implements EnumFactory<RiskAssessmentStatus> { 177 public RiskAssessmentStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("registered".equals(codeString)) 182 return RiskAssessmentStatus.REGISTERED; 183 if ("preliminary".equals(codeString)) 184 return RiskAssessmentStatus.PRELIMINARY; 185 if ("final".equals(codeString)) 186 return RiskAssessmentStatus.FINAL; 187 if ("amended".equals(codeString)) 188 return RiskAssessmentStatus.AMENDED; 189 if ("corrected".equals(codeString)) 190 return RiskAssessmentStatus.CORRECTED; 191 if ("cancelled".equals(codeString)) 192 return RiskAssessmentStatus.CANCELLED; 193 if ("entered-in-error".equals(codeString)) 194 return RiskAssessmentStatus.ENTEREDINERROR; 195 if ("unknown".equals(codeString)) 196 return RiskAssessmentStatus.UNKNOWN; 197 throw new IllegalArgumentException("Unknown RiskAssessmentStatus code '"+codeString+"'"); 198 } 199 public Enumeration<RiskAssessmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 200 if (code == null) 201 return null; 202 if (code.isEmpty()) 203 return new Enumeration<RiskAssessmentStatus>(this); 204 String codeString = code.asStringValue(); 205 if (codeString == null || "".equals(codeString)) 206 return null; 207 if ("registered".equals(codeString)) 208 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.REGISTERED); 209 if ("preliminary".equals(codeString)) 210 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.PRELIMINARY); 211 if ("final".equals(codeString)) 212 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.FINAL); 213 if ("amended".equals(codeString)) 214 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.AMENDED); 215 if ("corrected".equals(codeString)) 216 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CORRECTED); 217 if ("cancelled".equals(codeString)) 218 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CANCELLED); 219 if ("entered-in-error".equals(codeString)) 220 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.ENTEREDINERROR); 221 if ("unknown".equals(codeString)) 222 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.UNKNOWN); 223 throw new FHIRException("Unknown RiskAssessmentStatus code '"+codeString+"'"); 224 } 225 public String toCode(RiskAssessmentStatus code) { 226 if (code == RiskAssessmentStatus.NULL) 227 return null; 228 if (code == RiskAssessmentStatus.REGISTERED) 229 return "registered"; 230 if (code == RiskAssessmentStatus.PRELIMINARY) 231 return "preliminary"; 232 if (code == RiskAssessmentStatus.FINAL) 233 return "final"; 234 if (code == RiskAssessmentStatus.AMENDED) 235 return "amended"; 236 if (code == RiskAssessmentStatus.CORRECTED) 237 return "corrected"; 238 if (code == RiskAssessmentStatus.CANCELLED) 239 return "cancelled"; 240 if (code == RiskAssessmentStatus.ENTEREDINERROR) 241 return "entered-in-error"; 242 if (code == RiskAssessmentStatus.UNKNOWN) 243 return "unknown"; 244 return "?"; 245 } 246 public String toSystem(RiskAssessmentStatus code) { 247 return code.getSystem(); 248 } 249 } 250 251 @Block() 252 public static class RiskAssessmentPredictionComponent extends BackboneElement implements IBaseBackboneElement { 253 /** 254 * One of the potential outcomes for the patient (e.g. remission, death, a particular condition). 255 */ 256 @Child(name = "outcome", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 257 @Description(shortDefinition="Possible outcome for the subject", formalDefinition="One of the potential outcomes for the patient (e.g. remission, death, a particular condition)." ) 258 protected CodeableConcept outcome; 259 260 /** 261 * How likely is the outcome (in the specified timeframe). 262 */ 263 @Child(name = "probability", type = {DecimalType.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 264 @Description(shortDefinition="Likelihood of specified outcome", formalDefinition="How likely is the outcome (in the specified timeframe)." ) 265 protected Type probability; 266 267 /** 268 * How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high). 269 */ 270 @Child(name = "qualitativeRisk", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 271 @Description(shortDefinition="Likelihood of specified outcome as a qualitative value", formalDefinition="How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high)." ) 272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/risk-probability") 273 protected CodeableConcept qualitativeRisk; 274 275 /** 276 * Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 277 */ 278 @Child(name = "relativeRisk", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 279 @Description(shortDefinition="Relative likelihood", formalDefinition="Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.)." ) 280 protected DecimalType relativeRisk; 281 282 /** 283 * Indicates the period of time or age range of the subject to which the specified probability applies. 284 */ 285 @Child(name = "when", type = {Period.class, Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 286 @Description(shortDefinition="Timeframe or age range", formalDefinition="Indicates the period of time or age range of the subject to which the specified probability applies." ) 287 protected Type when; 288 289 /** 290 * Additional information explaining the basis for the prediction. 291 */ 292 @Child(name = "rationale", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 293 @Description(shortDefinition="Explanation of prediction", formalDefinition="Additional information explaining the basis for the prediction." ) 294 protected StringType rationale; 295 296 private static final long serialVersionUID = 1283401747L; 297 298 /** 299 * Constructor 300 */ 301 public RiskAssessmentPredictionComponent() { 302 super(); 303 } 304 305 /** 306 * Constructor 307 */ 308 public RiskAssessmentPredictionComponent(CodeableConcept outcome) { 309 super(); 310 this.outcome = outcome; 311 } 312 313 /** 314 * @return {@link #outcome} (One of the potential outcomes for the patient (e.g. remission, death, a particular condition).) 315 */ 316 public CodeableConcept getOutcome() { 317 if (this.outcome == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.outcome"); 320 else if (Configuration.doAutoCreate()) 321 this.outcome = new CodeableConcept(); // cc 322 return this.outcome; 323 } 324 325 public boolean hasOutcome() { 326 return this.outcome != null && !this.outcome.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #outcome} (One of the potential outcomes for the patient (e.g. remission, death, a particular condition).) 331 */ 332 public RiskAssessmentPredictionComponent setOutcome(CodeableConcept value) { 333 this.outcome = value; 334 return this; 335 } 336 337 /** 338 * @return {@link #probability} (How likely is the outcome (in the specified timeframe).) 339 */ 340 public Type getProbability() { 341 return this.probability; 342 } 343 344 /** 345 * @return {@link #probability} (How likely is the outcome (in the specified timeframe).) 346 */ 347 public DecimalType getProbabilityDecimalType() throws FHIRException { 348 if (this.probability == null) 349 return null; 350 if (!(this.probability instanceof DecimalType)) 351 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.probability.getClass().getName()+" was encountered"); 352 return (DecimalType) this.probability; 353 } 354 355 public boolean hasProbabilityDecimalType() { 356 return this != null && this.probability instanceof DecimalType; 357 } 358 359 /** 360 * @return {@link #probability} (How likely is the outcome (in the specified timeframe).) 361 */ 362 public Range getProbabilityRange() throws FHIRException { 363 if (this.probability == null) 364 return null; 365 if (!(this.probability instanceof Range)) 366 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.probability.getClass().getName()+" was encountered"); 367 return (Range) this.probability; 368 } 369 370 public boolean hasProbabilityRange() { 371 return this != null && this.probability instanceof Range; 372 } 373 374 public boolean hasProbability() { 375 return this.probability != null && !this.probability.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #probability} (How likely is the outcome (in the specified timeframe).) 380 */ 381 public RiskAssessmentPredictionComponent setProbability(Type value) throws FHIRFormatError { 382 if (value != null && !(value instanceof DecimalType || value instanceof Range)) 383 throw new FHIRFormatError("Not the right type for RiskAssessment.prediction.probability[x]: "+value.fhirType()); 384 this.probability = value; 385 return this; 386 } 387 388 /** 389 * @return {@link #qualitativeRisk} (How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high).) 390 */ 391 public CodeableConcept getQualitativeRisk() { 392 if (this.qualitativeRisk == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.qualitativeRisk"); 395 else if (Configuration.doAutoCreate()) 396 this.qualitativeRisk = new CodeableConcept(); // cc 397 return this.qualitativeRisk; 398 } 399 400 public boolean hasQualitativeRisk() { 401 return this.qualitativeRisk != null && !this.qualitativeRisk.isEmpty(); 402 } 403 404 /** 405 * @param value {@link #qualitativeRisk} (How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high).) 406 */ 407 public RiskAssessmentPredictionComponent setQualitativeRisk(CodeableConcept value) { 408 this.qualitativeRisk = value; 409 return this; 410 } 411 412 /** 413 * @return {@link #relativeRisk} (Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).). This is the underlying object with id, value and extensions. The accessor "getRelativeRisk" gives direct access to the value 414 */ 415 public DecimalType getRelativeRiskElement() { 416 if (this.relativeRisk == null) 417 if (Configuration.errorOnAutoCreate()) 418 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.relativeRisk"); 419 else if (Configuration.doAutoCreate()) 420 this.relativeRisk = new DecimalType(); // bb 421 return this.relativeRisk; 422 } 423 424 public boolean hasRelativeRiskElement() { 425 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 426 } 427 428 public boolean hasRelativeRisk() { 429 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 430 } 431 432 /** 433 * @param value {@link #relativeRisk} (Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).). This is the underlying object with id, value and extensions. The accessor "getRelativeRisk" gives direct access to the value 434 */ 435 public RiskAssessmentPredictionComponent setRelativeRiskElement(DecimalType value) { 436 this.relativeRisk = value; 437 return this; 438 } 439 440 /** 441 * @return Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 442 */ 443 public BigDecimal getRelativeRisk() { 444 return this.relativeRisk == null ? null : this.relativeRisk.getValue(); 445 } 446 447 /** 448 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 449 */ 450 public RiskAssessmentPredictionComponent setRelativeRisk(BigDecimal value) { 451 if (value == null) 452 this.relativeRisk = null; 453 else { 454 if (this.relativeRisk == null) 455 this.relativeRisk = new DecimalType(); 456 this.relativeRisk.setValue(value); 457 } 458 return this; 459 } 460 461 /** 462 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 463 */ 464 public RiskAssessmentPredictionComponent setRelativeRisk(long value) { 465 this.relativeRisk = new DecimalType(); 466 this.relativeRisk.setValue(value); 467 return this; 468 } 469 470 /** 471 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 472 */ 473 public RiskAssessmentPredictionComponent setRelativeRisk(double value) { 474 this.relativeRisk = new DecimalType(); 475 this.relativeRisk.setValue(value); 476 return this; 477 } 478 479 /** 480 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 481 */ 482 public Type getWhen() { 483 return this.when; 484 } 485 486 /** 487 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 488 */ 489 public Period getWhenPeriod() throws FHIRException { 490 if (this.when == null) 491 return null; 492 if (!(this.when instanceof Period)) 493 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 494 return (Period) this.when; 495 } 496 497 public boolean hasWhenPeriod() { 498 return this != null && this.when instanceof Period; 499 } 500 501 /** 502 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 503 */ 504 public Range getWhenRange() throws FHIRException { 505 if (this.when == null) 506 return null; 507 if (!(this.when instanceof Range)) 508 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.when.getClass().getName()+" was encountered"); 509 return (Range) this.when; 510 } 511 512 public boolean hasWhenRange() { 513 return this != null && this.when instanceof Range; 514 } 515 516 public boolean hasWhen() { 517 return this.when != null && !this.when.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 522 */ 523 public RiskAssessmentPredictionComponent setWhen(Type value) throws FHIRFormatError { 524 if (value != null && !(value instanceof Period || value instanceof Range)) 525 throw new FHIRFormatError("Not the right type for RiskAssessment.prediction.when[x]: "+value.fhirType()); 526 this.when = value; 527 return this; 528 } 529 530 /** 531 * @return {@link #rationale} (Additional information explaining the basis for the prediction.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 532 */ 533 public StringType getRationaleElement() { 534 if (this.rationale == null) 535 if (Configuration.errorOnAutoCreate()) 536 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.rationale"); 537 else if (Configuration.doAutoCreate()) 538 this.rationale = new StringType(); // bb 539 return this.rationale; 540 } 541 542 public boolean hasRationaleElement() { 543 return this.rationale != null && !this.rationale.isEmpty(); 544 } 545 546 public boolean hasRationale() { 547 return this.rationale != null && !this.rationale.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #rationale} (Additional information explaining the basis for the prediction.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 552 */ 553 public RiskAssessmentPredictionComponent setRationaleElement(StringType value) { 554 this.rationale = value; 555 return this; 556 } 557 558 /** 559 * @return Additional information explaining the basis for the prediction. 560 */ 561 public String getRationale() { 562 return this.rationale == null ? null : this.rationale.getValue(); 563 } 564 565 /** 566 * @param value Additional information explaining the basis for the prediction. 567 */ 568 public RiskAssessmentPredictionComponent setRationale(String value) { 569 if (Utilities.noString(value)) 570 this.rationale = null; 571 else { 572 if (this.rationale == null) 573 this.rationale = new StringType(); 574 this.rationale.setValue(value); 575 } 576 return this; 577 } 578 579 protected void listChildren(List<Property> children) { 580 super.listChildren(children); 581 children.add(new Property("outcome", "CodeableConcept", "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, outcome)); 582 children.add(new Property("probability[x]", "decimal|Range", "How likely is the outcome (in the specified timeframe).", 0, 1, probability)); 583 children.add(new Property("qualitativeRisk", "CodeableConcept", "How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high).", 0, 1, qualitativeRisk)); 584 children.add(new Property("relativeRisk", "decimal", "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 0, 1, relativeRisk)); 585 children.add(new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when)); 586 children.add(new Property("rationale", "string", "Additional information explaining the basis for the prediction.", 0, 1, rationale)); 587 } 588 589 @Override 590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 591 switch (_hash) { 592 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, outcome); 593 case 1430185003: /*probability[x]*/ return new Property("probability[x]", "decimal|Range", "How likely is the outcome (in the specified timeframe).", 0, 1, probability); 594 case -1290561483: /*probability*/ return new Property("probability[x]", "decimal|Range", "How likely is the outcome (in the specified timeframe).", 0, 1, probability); 595 case 888495452: /*probabilityDecimal*/ return new Property("probability[x]", "decimal|Range", "How likely is the outcome (in the specified timeframe).", 0, 1, probability); 596 case 9275912: /*probabilityRange*/ return new Property("probability[x]", "decimal|Range", "How likely is the outcome (in the specified timeframe).", 0, 1, probability); 597 case 123308730: /*qualitativeRisk*/ return new Property("qualitativeRisk", "CodeableConcept", "How likely is the outcome (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, high).", 0, 1, qualitativeRisk); 598 case -70741061: /*relativeRisk*/ return new Property("relativeRisk", "decimal", "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 0, 1, relativeRisk); 599 case 1312831238: /*when[x]*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 600 case 3648314: /*when*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 601 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 602 case -1098542557: /*whenRange*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 603 case 345689335: /*rationale*/ return new Property("rationale", "string", "Additional information explaining the basis for the prediction.", 0, 1, rationale); 604 default: return super.getNamedProperty(_hash, _name, _checkValid); 605 } 606 607 } 608 609 @Override 610 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 611 switch (hash) { 612 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 613 case -1290561483: /*probability*/ return this.probability == null ? new Base[0] : new Base[] {this.probability}; // Type 614 case 123308730: /*qualitativeRisk*/ return this.qualitativeRisk == null ? new Base[0] : new Base[] {this.qualitativeRisk}; // CodeableConcept 615 case -70741061: /*relativeRisk*/ return this.relativeRisk == null ? new Base[0] : new Base[] {this.relativeRisk}; // DecimalType 616 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // Type 617 case 345689335: /*rationale*/ return this.rationale == null ? new Base[0] : new Base[] {this.rationale}; // StringType 618 default: return super.getProperty(hash, name, checkValid); 619 } 620 621 } 622 623 @Override 624 public Base setProperty(int hash, String name, Base value) throws FHIRException { 625 switch (hash) { 626 case -1106507950: // outcome 627 this.outcome = castToCodeableConcept(value); // CodeableConcept 628 return value; 629 case -1290561483: // probability 630 this.probability = castToType(value); // Type 631 return value; 632 case 123308730: // qualitativeRisk 633 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 634 return value; 635 case -70741061: // relativeRisk 636 this.relativeRisk = castToDecimal(value); // DecimalType 637 return value; 638 case 3648314: // when 639 this.when = castToType(value); // Type 640 return value; 641 case 345689335: // rationale 642 this.rationale = castToString(value); // StringType 643 return value; 644 default: return super.setProperty(hash, name, value); 645 } 646 647 } 648 649 @Override 650 public Base setProperty(String name, Base value) throws FHIRException { 651 if (name.equals("outcome")) { 652 this.outcome = castToCodeableConcept(value); // CodeableConcept 653 } else if (name.equals("probability[x]")) { 654 this.probability = castToType(value); // Type 655 } else if (name.equals("qualitativeRisk")) { 656 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 657 } else if (name.equals("relativeRisk")) { 658 this.relativeRisk = castToDecimal(value); // DecimalType 659 } else if (name.equals("when[x]")) { 660 this.when = castToType(value); // Type 661 } else if (name.equals("rationale")) { 662 this.rationale = castToString(value); // StringType 663 } else 664 return super.setProperty(name, value); 665 return value; 666 } 667 668 @Override 669 public Base makeProperty(int hash, String name) throws FHIRException { 670 switch (hash) { 671 case -1106507950: return getOutcome(); 672 case 1430185003: return getProbability(); 673 case -1290561483: return getProbability(); 674 case 123308730: return getQualitativeRisk(); 675 case -70741061: return getRelativeRiskElement(); 676 case 1312831238: return getWhen(); 677 case 3648314: return getWhen(); 678 case 345689335: return getRationaleElement(); 679 default: return super.makeProperty(hash, name); 680 } 681 682 } 683 684 @Override 685 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 686 switch (hash) { 687 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 688 case -1290561483: /*probability*/ return new String[] {"decimal", "Range"}; 689 case 123308730: /*qualitativeRisk*/ return new String[] {"CodeableConcept"}; 690 case -70741061: /*relativeRisk*/ return new String[] {"decimal"}; 691 case 3648314: /*when*/ return new String[] {"Period", "Range"}; 692 case 345689335: /*rationale*/ return new String[] {"string"}; 693 default: return super.getTypesForProperty(hash, name); 694 } 695 696 } 697 698 @Override 699 public Base addChild(String name) throws FHIRException { 700 if (name.equals("outcome")) { 701 this.outcome = new CodeableConcept(); 702 return this.outcome; 703 } 704 else if (name.equals("probabilityDecimal")) { 705 this.probability = new DecimalType(); 706 return this.probability; 707 } 708 else if (name.equals("probabilityRange")) { 709 this.probability = new Range(); 710 return this.probability; 711 } 712 else if (name.equals("qualitativeRisk")) { 713 this.qualitativeRisk = new CodeableConcept(); 714 return this.qualitativeRisk; 715 } 716 else if (name.equals("relativeRisk")) { 717 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.relativeRisk"); 718 } 719 else if (name.equals("whenPeriod")) { 720 this.when = new Period(); 721 return this.when; 722 } 723 else if (name.equals("whenRange")) { 724 this.when = new Range(); 725 return this.when; 726 } 727 else if (name.equals("rationale")) { 728 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.rationale"); 729 } 730 else 731 return super.addChild(name); 732 } 733 734 public RiskAssessmentPredictionComponent copy() { 735 RiskAssessmentPredictionComponent dst = new RiskAssessmentPredictionComponent(); 736 copyValues(dst); 737 dst.outcome = outcome == null ? null : outcome.copy(); 738 dst.probability = probability == null ? null : probability.copy(); 739 dst.qualitativeRisk = qualitativeRisk == null ? null : qualitativeRisk.copy(); 740 dst.relativeRisk = relativeRisk == null ? null : relativeRisk.copy(); 741 dst.when = when == null ? null : when.copy(); 742 dst.rationale = rationale == null ? null : rationale.copy(); 743 return dst; 744 } 745 746 @Override 747 public boolean equalsDeep(Base other_) { 748 if (!super.equalsDeep(other_)) 749 return false; 750 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 751 return false; 752 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 753 return compareDeep(outcome, o.outcome, true) && compareDeep(probability, o.probability, true) && compareDeep(qualitativeRisk, o.qualitativeRisk, true) 754 && compareDeep(relativeRisk, o.relativeRisk, true) && compareDeep(when, o.when, true) && compareDeep(rationale, o.rationale, true) 755 ; 756 } 757 758 @Override 759 public boolean equalsShallow(Base other_) { 760 if (!super.equalsShallow(other_)) 761 return false; 762 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 763 return false; 764 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 765 return compareValues(relativeRisk, o.relativeRisk, true) && compareValues(rationale, o.rationale, true) 766 ; 767 } 768 769 public boolean isEmpty() { 770 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outcome, probability, qualitativeRisk 771 , relativeRisk, when, rationale); 772 } 773 774 public String fhirType() { 775 return "RiskAssessment.prediction"; 776 777 } 778 779 } 780 781 /** 782 * Business identifier assigned to the risk assessment. 783 */ 784 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 785 @Description(shortDefinition="Unique identifier for the assessment", formalDefinition="Business identifier assigned to the risk assessment." ) 786 protected Identifier identifier; 787 788 /** 789 * A reference to the request that is fulfilled by this risk assessment. 790 */ 791 @Child(name = "basedOn", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 792 @Description(shortDefinition="Request fulfilled by this assessment", formalDefinition="A reference to the request that is fulfilled by this risk assessment." ) 793 protected Reference basedOn; 794 795 /** 796 * The actual object that is the target of the reference (A reference to the request that is fulfilled by this risk assessment.) 797 */ 798 protected Resource basedOnTarget; 799 800 /** 801 * A reference to a resource that this risk assessment is part of, such as a Procedure. 802 */ 803 @Child(name = "parent", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 804 @Description(shortDefinition="Part of this occurrence", formalDefinition="A reference to a resource that this risk assessment is part of, such as a Procedure." ) 805 protected Reference parent; 806 807 /** 808 * The actual object that is the target of the reference (A reference to a resource that this risk assessment is part of, such as a Procedure.) 809 */ 810 protected Resource parentTarget; 811 812 /** 813 * The status of the RiskAssessment, using the same statuses as an Observation. 814 */ 815 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 816 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="The status of the RiskAssessment, using the same statuses as an Observation." ) 817 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 818 protected Enumeration<RiskAssessmentStatus> status; 819 820 /** 821 * The algorithm, process or mechanism used to evaluate the risk. 822 */ 823 @Child(name = "method", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 824 @Description(shortDefinition="Evaluation mechanism", formalDefinition="The algorithm, process or mechanism used to evaluate the risk." ) 825 protected CodeableConcept method; 826 827 /** 828 * The type of the risk assessment performed. 829 */ 830 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 831 @Description(shortDefinition="Type of assessment", formalDefinition="The type of the risk assessment performed." ) 832 protected CodeableConcept code; 833 834 /** 835 * The patient or group the risk assessment applies to. 836 */ 837 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=0, max=1, modifier=false, summary=true) 838 @Description(shortDefinition="Who/what does assessment apply to?", formalDefinition="The patient or group the risk assessment applies to." ) 839 protected Reference subject; 840 841 /** 842 * The actual object that is the target of the reference (The patient or group the risk assessment applies to.) 843 */ 844 protected Resource subjectTarget; 845 846 /** 847 * The encounter where the assessment was performed. 848 */ 849 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=7, min=0, max=1, modifier=false, summary=true) 850 @Description(shortDefinition="Where was assessment performed?", formalDefinition="The encounter where the assessment was performed." ) 851 protected Reference context; 852 853 /** 854 * The actual object that is the target of the reference (The encounter where the assessment was performed.) 855 */ 856 protected Resource contextTarget; 857 858 /** 859 * The date (and possibly time) the risk assessment was performed. 860 */ 861 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 862 @Description(shortDefinition="When was assessment made?", formalDefinition="The date (and possibly time) the risk assessment was performed." ) 863 protected Type occurrence; 864 865 /** 866 * For assessments or prognosis specific to a particular condition, indicates the condition being assessed. 867 */ 868 @Child(name = "condition", type = {Condition.class}, order=9, min=0, max=1, modifier=false, summary=true) 869 @Description(shortDefinition="Condition assessed", formalDefinition="For assessments or prognosis specific to a particular condition, indicates the condition being assessed." ) 870 protected Reference condition; 871 872 /** 873 * The actual object that is the target of the reference (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 874 */ 875 protected Condition conditionTarget; 876 877 /** 878 * The provider or software application that performed the assessment. 879 */ 880 @Child(name = "performer", type = {Practitioner.class, Device.class}, order=10, min=0, max=1, modifier=false, summary=true) 881 @Description(shortDefinition="Who did assessment?", formalDefinition="The provider or software application that performed the assessment." ) 882 protected Reference performer; 883 884 /** 885 * The actual object that is the target of the reference (The provider or software application that performed the assessment.) 886 */ 887 protected Resource performerTarget; 888 889 /** 890 * The reason the risk assessment was performed. 891 */ 892 @Child(name = "reason", type = {CodeableConcept.class, Reference.class}, order=11, min=0, max=1, modifier=false, summary=false) 893 @Description(shortDefinition="Why the assessment was necessary?", formalDefinition="The reason the risk assessment was performed." ) 894 protected Type reason; 895 896 /** 897 * Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.). 898 */ 899 @Child(name = "basis", type = {Reference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 900 @Description(shortDefinition="Information used in assessment", formalDefinition="Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.)." ) 901 protected List<Reference> basis; 902 /** 903 * The actual objects that are the target of the reference (Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).) 904 */ 905 protected List<Resource> basisTarget; 906 907 908 /** 909 * Describes the expected outcome for the subject. 910 */ 911 @Child(name = "prediction", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 912 @Description(shortDefinition="Outcome predicted", formalDefinition="Describes the expected outcome for the subject." ) 913 protected List<RiskAssessmentPredictionComponent> prediction; 914 915 /** 916 * A description of the steps that might be taken to reduce the identified risk(s). 917 */ 918 @Child(name = "mitigation", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 919 @Description(shortDefinition="How to reduce risk", formalDefinition="A description of the steps that might be taken to reduce the identified risk(s)." ) 920 protected StringType mitigation; 921 922 /** 923 * Additional comments about the risk assessment. 924 */ 925 @Child(name = "comment", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=false) 926 @Description(shortDefinition="Comments on the risk assessment", formalDefinition="Additional comments about the risk assessment." ) 927 protected StringType comment; 928 929 private static final long serialVersionUID = -715866284L; 930 931 /** 932 * Constructor 933 */ 934 public RiskAssessment() { 935 super(); 936 } 937 938 /** 939 * Constructor 940 */ 941 public RiskAssessment(Enumeration<RiskAssessmentStatus> status) { 942 super(); 943 this.status = status; 944 } 945 946 /** 947 * @return {@link #identifier} (Business identifier assigned to the risk assessment.) 948 */ 949 public Identifier getIdentifier() { 950 if (this.identifier == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create RiskAssessment.identifier"); 953 else if (Configuration.doAutoCreate()) 954 this.identifier = new Identifier(); // cc 955 return this.identifier; 956 } 957 958 public boolean hasIdentifier() { 959 return this.identifier != null && !this.identifier.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #identifier} (Business identifier assigned to the risk assessment.) 964 */ 965 public RiskAssessment setIdentifier(Identifier value) { 966 this.identifier = value; 967 return this; 968 } 969 970 /** 971 * @return {@link #basedOn} (A reference to the request that is fulfilled by this risk assessment.) 972 */ 973 public Reference getBasedOn() { 974 if (this.basedOn == null) 975 if (Configuration.errorOnAutoCreate()) 976 throw new Error("Attempt to auto-create RiskAssessment.basedOn"); 977 else if (Configuration.doAutoCreate()) 978 this.basedOn = new Reference(); // cc 979 return this.basedOn; 980 } 981 982 public boolean hasBasedOn() { 983 return this.basedOn != null && !this.basedOn.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #basedOn} (A reference to the request that is fulfilled by this risk assessment.) 988 */ 989 public RiskAssessment setBasedOn(Reference value) { 990 this.basedOn = value; 991 return this; 992 } 993 994 /** 995 * @return {@link #basedOn} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the request that is fulfilled by this risk assessment.) 996 */ 997 public Resource getBasedOnTarget() { 998 return this.basedOnTarget; 999 } 1000 1001 /** 1002 * @param value {@link #basedOn} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the request that is fulfilled by this risk assessment.) 1003 */ 1004 public RiskAssessment setBasedOnTarget(Resource value) { 1005 this.basedOnTarget = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #parent} (A reference to a resource that this risk assessment is part of, such as a Procedure.) 1011 */ 1012 public Reference getParent() { 1013 if (this.parent == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create RiskAssessment.parent"); 1016 else if (Configuration.doAutoCreate()) 1017 this.parent = new Reference(); // cc 1018 return this.parent; 1019 } 1020 1021 public boolean hasParent() { 1022 return this.parent != null && !this.parent.isEmpty(); 1023 } 1024 1025 /** 1026 * @param value {@link #parent} (A reference to a resource that this risk assessment is part of, such as a Procedure.) 1027 */ 1028 public RiskAssessment setParent(Reference value) { 1029 this.parent = value; 1030 return this; 1031 } 1032 1033 /** 1034 * @return {@link #parent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a resource that this risk assessment is part of, such as a Procedure.) 1035 */ 1036 public Resource getParentTarget() { 1037 return this.parentTarget; 1038 } 1039 1040 /** 1041 * @param value {@link #parent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a resource that this risk assessment is part of, such as a Procedure.) 1042 */ 1043 public RiskAssessment setParentTarget(Resource value) { 1044 this.parentTarget = value; 1045 return this; 1046 } 1047 1048 /** 1049 * @return {@link #status} (The status of the RiskAssessment, using the same statuses as an Observation.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1050 */ 1051 public Enumeration<RiskAssessmentStatus> getStatusElement() { 1052 if (this.status == null) 1053 if (Configuration.errorOnAutoCreate()) 1054 throw new Error("Attempt to auto-create RiskAssessment.status"); 1055 else if (Configuration.doAutoCreate()) 1056 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); // bb 1057 return this.status; 1058 } 1059 1060 public boolean hasStatusElement() { 1061 return this.status != null && !this.status.isEmpty(); 1062 } 1063 1064 public boolean hasStatus() { 1065 return this.status != null && !this.status.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #status} (The status of the RiskAssessment, using the same statuses as an Observation.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1070 */ 1071 public RiskAssessment setStatusElement(Enumeration<RiskAssessmentStatus> value) { 1072 this.status = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return The status of the RiskAssessment, using the same statuses as an Observation. 1078 */ 1079 public RiskAssessmentStatus getStatus() { 1080 return this.status == null ? null : this.status.getValue(); 1081 } 1082 1083 /** 1084 * @param value The status of the RiskAssessment, using the same statuses as an Observation. 1085 */ 1086 public RiskAssessment setStatus(RiskAssessmentStatus value) { 1087 if (this.status == null) 1088 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); 1089 this.status.setValue(value); 1090 return this; 1091 } 1092 1093 /** 1094 * @return {@link #method} (The algorithm, process or mechanism used to evaluate the risk.) 1095 */ 1096 public CodeableConcept getMethod() { 1097 if (this.method == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create RiskAssessment.method"); 1100 else if (Configuration.doAutoCreate()) 1101 this.method = new CodeableConcept(); // cc 1102 return this.method; 1103 } 1104 1105 public boolean hasMethod() { 1106 return this.method != null && !this.method.isEmpty(); 1107 } 1108 1109 /** 1110 * @param value {@link #method} (The algorithm, process or mechanism used to evaluate the risk.) 1111 */ 1112 public RiskAssessment setMethod(CodeableConcept value) { 1113 this.method = value; 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #code} (The type of the risk assessment performed.) 1119 */ 1120 public CodeableConcept getCode() { 1121 if (this.code == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create RiskAssessment.code"); 1124 else if (Configuration.doAutoCreate()) 1125 this.code = new CodeableConcept(); // cc 1126 return this.code; 1127 } 1128 1129 public boolean hasCode() { 1130 return this.code != null && !this.code.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #code} (The type of the risk assessment performed.) 1135 */ 1136 public RiskAssessment setCode(CodeableConcept value) { 1137 this.code = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return {@link #subject} (The patient or group the risk assessment applies to.) 1143 */ 1144 public Reference getSubject() { 1145 if (this.subject == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create RiskAssessment.subject"); 1148 else if (Configuration.doAutoCreate()) 1149 this.subject = new Reference(); // cc 1150 return this.subject; 1151 } 1152 1153 public boolean hasSubject() { 1154 return this.subject != null && !this.subject.isEmpty(); 1155 } 1156 1157 /** 1158 * @param value {@link #subject} (The patient or group the risk assessment applies to.) 1159 */ 1160 public RiskAssessment setSubject(Reference value) { 1161 this.subject = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient or group the risk assessment applies to.) 1167 */ 1168 public Resource getSubjectTarget() { 1169 return this.subjectTarget; 1170 } 1171 1172 /** 1173 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient or group the risk assessment applies to.) 1174 */ 1175 public RiskAssessment setSubjectTarget(Resource value) { 1176 this.subjectTarget = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #context} (The encounter where the assessment was performed.) 1182 */ 1183 public Reference getContext() { 1184 if (this.context == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create RiskAssessment.context"); 1187 else if (Configuration.doAutoCreate()) 1188 this.context = new Reference(); // cc 1189 return this.context; 1190 } 1191 1192 public boolean hasContext() { 1193 return this.context != null && !this.context.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #context} (The encounter where the assessment was performed.) 1198 */ 1199 public RiskAssessment setContext(Reference value) { 1200 this.context = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter where the assessment was performed.) 1206 */ 1207 public Resource getContextTarget() { 1208 return this.contextTarget; 1209 } 1210 1211 /** 1212 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter where the assessment was performed.) 1213 */ 1214 public RiskAssessment setContextTarget(Resource value) { 1215 this.contextTarget = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 1221 */ 1222 public Type getOccurrence() { 1223 return this.occurrence; 1224 } 1225 1226 /** 1227 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 1228 */ 1229 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1230 if (this.occurrence == null) 1231 return null; 1232 if (!(this.occurrence instanceof DateTimeType)) 1233 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1234 return (DateTimeType) this.occurrence; 1235 } 1236 1237 public boolean hasOccurrenceDateTimeType() { 1238 return this != null && this.occurrence instanceof DateTimeType; 1239 } 1240 1241 /** 1242 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 1243 */ 1244 public Period getOccurrencePeriod() throws FHIRException { 1245 if (this.occurrence == null) 1246 return null; 1247 if (!(this.occurrence instanceof Period)) 1248 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1249 return (Period) this.occurrence; 1250 } 1251 1252 public boolean hasOccurrencePeriod() { 1253 return this != null && this.occurrence instanceof Period; 1254 } 1255 1256 public boolean hasOccurrence() { 1257 return this.occurrence != null && !this.occurrence.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 1262 */ 1263 public RiskAssessment setOccurrence(Type value) throws FHIRFormatError { 1264 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1265 throw new FHIRFormatError("Not the right type for RiskAssessment.occurrence[x]: "+value.fhirType()); 1266 this.occurrence = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return {@link #condition} (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1272 */ 1273 public Reference getCondition() { 1274 if (this.condition == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1277 else if (Configuration.doAutoCreate()) 1278 this.condition = new Reference(); // cc 1279 return this.condition; 1280 } 1281 1282 public boolean hasCondition() { 1283 return this.condition != null && !this.condition.isEmpty(); 1284 } 1285 1286 /** 1287 * @param value {@link #condition} (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1288 */ 1289 public RiskAssessment setCondition(Reference value) { 1290 this.condition = value; 1291 return this; 1292 } 1293 1294 /** 1295 * @return {@link #condition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1296 */ 1297 public Condition getConditionTarget() { 1298 if (this.conditionTarget == null) 1299 if (Configuration.errorOnAutoCreate()) 1300 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1301 else if (Configuration.doAutoCreate()) 1302 this.conditionTarget = new Condition(); // aa 1303 return this.conditionTarget; 1304 } 1305 1306 /** 1307 * @param value {@link #condition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1308 */ 1309 public RiskAssessment setConditionTarget(Condition value) { 1310 this.conditionTarget = value; 1311 return this; 1312 } 1313 1314 /** 1315 * @return {@link #performer} (The provider or software application that performed the assessment.) 1316 */ 1317 public Reference getPerformer() { 1318 if (this.performer == null) 1319 if (Configuration.errorOnAutoCreate()) 1320 throw new Error("Attempt to auto-create RiskAssessment.performer"); 1321 else if (Configuration.doAutoCreate()) 1322 this.performer = new Reference(); // cc 1323 return this.performer; 1324 } 1325 1326 public boolean hasPerformer() { 1327 return this.performer != null && !this.performer.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #performer} (The provider or software application that performed the assessment.) 1332 */ 1333 public RiskAssessment setPerformer(Reference value) { 1334 this.performer = value; 1335 return this; 1336 } 1337 1338 /** 1339 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider or software application that performed the assessment.) 1340 */ 1341 public Resource getPerformerTarget() { 1342 return this.performerTarget; 1343 } 1344 1345 /** 1346 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider or software application that performed the assessment.) 1347 */ 1348 public RiskAssessment setPerformerTarget(Resource value) { 1349 this.performerTarget = value; 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #reason} (The reason the risk assessment was performed.) 1355 */ 1356 public Type getReason() { 1357 return this.reason; 1358 } 1359 1360 /** 1361 * @return {@link #reason} (The reason the risk assessment was performed.) 1362 */ 1363 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 1364 if (this.reason == null) 1365 return null; 1366 if (!(this.reason instanceof CodeableConcept)) 1367 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 1368 return (CodeableConcept) this.reason; 1369 } 1370 1371 public boolean hasReasonCodeableConcept() { 1372 return this != null && this.reason instanceof CodeableConcept; 1373 } 1374 1375 /** 1376 * @return {@link #reason} (The reason the risk assessment was performed.) 1377 */ 1378 public Reference getReasonReference() throws FHIRException { 1379 if (this.reason == null) 1380 return null; 1381 if (!(this.reason instanceof Reference)) 1382 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 1383 return (Reference) this.reason; 1384 } 1385 1386 public boolean hasReasonReference() { 1387 return this != null && this.reason instanceof Reference; 1388 } 1389 1390 public boolean hasReason() { 1391 return this.reason != null && !this.reason.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #reason} (The reason the risk assessment was performed.) 1396 */ 1397 public RiskAssessment setReason(Type value) throws FHIRFormatError { 1398 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1399 throw new FHIRFormatError("Not the right type for RiskAssessment.reason[x]: "+value.fhirType()); 1400 this.reason = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #basis} (Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).) 1406 */ 1407 public List<Reference> getBasis() { 1408 if (this.basis == null) 1409 this.basis = new ArrayList<Reference>(); 1410 return this.basis; 1411 } 1412 1413 /** 1414 * @return Returns a reference to <code>this</code> for easy method chaining 1415 */ 1416 public RiskAssessment setBasis(List<Reference> theBasis) { 1417 this.basis = theBasis; 1418 return this; 1419 } 1420 1421 public boolean hasBasis() { 1422 if (this.basis == null) 1423 return false; 1424 for (Reference item : this.basis) 1425 if (!item.isEmpty()) 1426 return true; 1427 return false; 1428 } 1429 1430 public Reference addBasis() { //3 1431 Reference t = new Reference(); 1432 if (this.basis == null) 1433 this.basis = new ArrayList<Reference>(); 1434 this.basis.add(t); 1435 return t; 1436 } 1437 1438 public RiskAssessment addBasis(Reference t) { //3 1439 if (t == null) 1440 return this; 1441 if (this.basis == null) 1442 this.basis = new ArrayList<Reference>(); 1443 this.basis.add(t); 1444 return this; 1445 } 1446 1447 /** 1448 * @return The first repetition of repeating field {@link #basis}, creating it if it does not already exist 1449 */ 1450 public Reference getBasisFirstRep() { 1451 if (getBasis().isEmpty()) { 1452 addBasis(); 1453 } 1454 return getBasis().get(0); 1455 } 1456 1457 /** 1458 * @deprecated Use Reference#setResource(IBaseResource) instead 1459 */ 1460 @Deprecated 1461 public List<Resource> getBasisTarget() { 1462 if (this.basisTarget == null) 1463 this.basisTarget = new ArrayList<Resource>(); 1464 return this.basisTarget; 1465 } 1466 1467 /** 1468 * @return {@link #prediction} (Describes the expected outcome for the subject.) 1469 */ 1470 public List<RiskAssessmentPredictionComponent> getPrediction() { 1471 if (this.prediction == null) 1472 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1473 return this.prediction; 1474 } 1475 1476 /** 1477 * @return Returns a reference to <code>this</code> for easy method chaining 1478 */ 1479 public RiskAssessment setPrediction(List<RiskAssessmentPredictionComponent> thePrediction) { 1480 this.prediction = thePrediction; 1481 return this; 1482 } 1483 1484 public boolean hasPrediction() { 1485 if (this.prediction == null) 1486 return false; 1487 for (RiskAssessmentPredictionComponent item : this.prediction) 1488 if (!item.isEmpty()) 1489 return true; 1490 return false; 1491 } 1492 1493 public RiskAssessmentPredictionComponent addPrediction() { //3 1494 RiskAssessmentPredictionComponent t = new RiskAssessmentPredictionComponent(); 1495 if (this.prediction == null) 1496 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1497 this.prediction.add(t); 1498 return t; 1499 } 1500 1501 public RiskAssessment addPrediction(RiskAssessmentPredictionComponent t) { //3 1502 if (t == null) 1503 return this; 1504 if (this.prediction == null) 1505 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1506 this.prediction.add(t); 1507 return this; 1508 } 1509 1510 /** 1511 * @return The first repetition of repeating field {@link #prediction}, creating it if it does not already exist 1512 */ 1513 public RiskAssessmentPredictionComponent getPredictionFirstRep() { 1514 if (getPrediction().isEmpty()) { 1515 addPrediction(); 1516 } 1517 return getPrediction().get(0); 1518 } 1519 1520 /** 1521 * @return {@link #mitigation} (A description of the steps that might be taken to reduce the identified risk(s).). This is the underlying object with id, value and extensions. The accessor "getMitigation" gives direct access to the value 1522 */ 1523 public StringType getMitigationElement() { 1524 if (this.mitigation == null) 1525 if (Configuration.errorOnAutoCreate()) 1526 throw new Error("Attempt to auto-create RiskAssessment.mitigation"); 1527 else if (Configuration.doAutoCreate()) 1528 this.mitigation = new StringType(); // bb 1529 return this.mitigation; 1530 } 1531 1532 public boolean hasMitigationElement() { 1533 return this.mitigation != null && !this.mitigation.isEmpty(); 1534 } 1535 1536 public boolean hasMitigation() { 1537 return this.mitigation != null && !this.mitigation.isEmpty(); 1538 } 1539 1540 /** 1541 * @param value {@link #mitigation} (A description of the steps that might be taken to reduce the identified risk(s).). This is the underlying object with id, value and extensions. The accessor "getMitigation" gives direct access to the value 1542 */ 1543 public RiskAssessment setMitigationElement(StringType value) { 1544 this.mitigation = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return A description of the steps that might be taken to reduce the identified risk(s). 1550 */ 1551 public String getMitigation() { 1552 return this.mitigation == null ? null : this.mitigation.getValue(); 1553 } 1554 1555 /** 1556 * @param value A description of the steps that might be taken to reduce the identified risk(s). 1557 */ 1558 public RiskAssessment setMitigation(String value) { 1559 if (Utilities.noString(value)) 1560 this.mitigation = null; 1561 else { 1562 if (this.mitigation == null) 1563 this.mitigation = new StringType(); 1564 this.mitigation.setValue(value); 1565 } 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #comment} (Additional comments about the risk assessment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1571 */ 1572 public StringType getCommentElement() { 1573 if (this.comment == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create RiskAssessment.comment"); 1576 else if (Configuration.doAutoCreate()) 1577 this.comment = new StringType(); // bb 1578 return this.comment; 1579 } 1580 1581 public boolean hasCommentElement() { 1582 return this.comment != null && !this.comment.isEmpty(); 1583 } 1584 1585 public boolean hasComment() { 1586 return this.comment != null && !this.comment.isEmpty(); 1587 } 1588 1589 /** 1590 * @param value {@link #comment} (Additional comments about the risk assessment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1591 */ 1592 public RiskAssessment setCommentElement(StringType value) { 1593 this.comment = value; 1594 return this; 1595 } 1596 1597 /** 1598 * @return Additional comments about the risk assessment. 1599 */ 1600 public String getComment() { 1601 return this.comment == null ? null : this.comment.getValue(); 1602 } 1603 1604 /** 1605 * @param value Additional comments about the risk assessment. 1606 */ 1607 public RiskAssessment setComment(String value) { 1608 if (Utilities.noString(value)) 1609 this.comment = null; 1610 else { 1611 if (this.comment == null) 1612 this.comment = new StringType(); 1613 this.comment.setValue(value); 1614 } 1615 return this; 1616 } 1617 1618 protected void listChildren(List<Property> children) { 1619 super.listChildren(children); 1620 children.add(new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, 1, identifier)); 1621 children.add(new Property("basedOn", "Reference(Any)", "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn)); 1622 children.add(new Property("parent", "Reference(Any)", "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent)); 1623 children.add(new Property("status", "code", "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status)); 1624 children.add(new Property("method", "CodeableConcept", "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method)); 1625 children.add(new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code)); 1626 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group the risk assessment applies to.", 0, 1, subject)); 1627 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter where the assessment was performed.", 0, 1, context)); 1628 children.add(new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence)); 1629 children.add(new Property("condition", "Reference(Condition)", "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 1, condition)); 1630 children.add(new Property("performer", "Reference(Practitioner|Device)", "The provider or software application that performed the assessment.", 0, 1, performer)); 1631 children.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "The reason the risk assessment was performed.", 0, 1, reason)); 1632 children.add(new Property("basis", "Reference(Any)", "Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).", 0, java.lang.Integer.MAX_VALUE, basis)); 1633 children.add(new Property("prediction", "", "Describes the expected outcome for the subject.", 0, java.lang.Integer.MAX_VALUE, prediction)); 1634 children.add(new Property("mitigation", "string", "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation)); 1635 children.add(new Property("comment", "string", "Additional comments about the risk assessment.", 0, 1, comment)); 1636 } 1637 1638 @Override 1639 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1640 switch (_hash) { 1641 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, 1, identifier); 1642 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn); 1643 case -995424086: /*parent*/ return new Property("parent", "Reference(Any)", "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent); 1644 case -892481550: /*status*/ return new Property("status", "code", "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status); 1645 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method); 1646 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code); 1647 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group the risk assessment applies to.", 0, 1, subject); 1648 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter where the assessment was performed.", 0, 1, context); 1649 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1650 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1651 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1652 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1653 case -861311717: /*condition*/ return new Property("condition", "Reference(Condition)", "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 1, condition); 1654 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|Device)", "The provider or software application that performed the assessment.", 0, 1, performer); 1655 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "The reason the risk assessment was performed.", 0, 1, reason); 1656 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "The reason the risk assessment was performed.", 0, 1, reason); 1657 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "The reason the risk assessment was performed.", 0, 1, reason); 1658 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "The reason the risk assessment was performed.", 0, 1, reason); 1659 case 93508670: /*basis*/ return new Property("basis", "Reference(Any)", "Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).", 0, java.lang.Integer.MAX_VALUE, basis); 1660 case 1161234575: /*prediction*/ return new Property("prediction", "", "Describes the expected outcome for the subject.", 0, java.lang.Integer.MAX_VALUE, prediction); 1661 case 1293793087: /*mitigation*/ return new Property("mitigation", "string", "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation); 1662 case 950398559: /*comment*/ return new Property("comment", "string", "Additional comments about the risk assessment.", 0, 1, comment); 1663 default: return super.getNamedProperty(_hash, _name, _checkValid); 1664 } 1665 1666 } 1667 1668 @Override 1669 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1670 switch (hash) { 1671 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1672 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : new Base[] {this.basedOn}; // Reference 1673 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 1674 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RiskAssessmentStatus> 1675 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 1676 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1677 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1678 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1679 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1680 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Reference 1681 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1682 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 1683 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // Reference 1684 case 1161234575: /*prediction*/ return this.prediction == null ? new Base[0] : this.prediction.toArray(new Base[this.prediction.size()]); // RiskAssessmentPredictionComponent 1685 case 1293793087: /*mitigation*/ return this.mitigation == null ? new Base[0] : new Base[] {this.mitigation}; // StringType 1686 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 1687 default: return super.getProperty(hash, name, checkValid); 1688 } 1689 1690 } 1691 1692 @Override 1693 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1694 switch (hash) { 1695 case -1618432855: // identifier 1696 this.identifier = castToIdentifier(value); // Identifier 1697 return value; 1698 case -332612366: // basedOn 1699 this.basedOn = castToReference(value); // Reference 1700 return value; 1701 case -995424086: // parent 1702 this.parent = castToReference(value); // Reference 1703 return value; 1704 case -892481550: // status 1705 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 1706 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 1707 return value; 1708 case -1077554975: // method 1709 this.method = castToCodeableConcept(value); // CodeableConcept 1710 return value; 1711 case 3059181: // code 1712 this.code = castToCodeableConcept(value); // CodeableConcept 1713 return value; 1714 case -1867885268: // subject 1715 this.subject = castToReference(value); // Reference 1716 return value; 1717 case 951530927: // context 1718 this.context = castToReference(value); // Reference 1719 return value; 1720 case 1687874001: // occurrence 1721 this.occurrence = castToType(value); // Type 1722 return value; 1723 case -861311717: // condition 1724 this.condition = castToReference(value); // Reference 1725 return value; 1726 case 481140686: // performer 1727 this.performer = castToReference(value); // Reference 1728 return value; 1729 case -934964668: // reason 1730 this.reason = castToType(value); // Type 1731 return value; 1732 case 93508670: // basis 1733 this.getBasis().add(castToReference(value)); // Reference 1734 return value; 1735 case 1161234575: // prediction 1736 this.getPrediction().add((RiskAssessmentPredictionComponent) value); // RiskAssessmentPredictionComponent 1737 return value; 1738 case 1293793087: // mitigation 1739 this.mitigation = castToString(value); // StringType 1740 return value; 1741 case 950398559: // comment 1742 this.comment = castToString(value); // StringType 1743 return value; 1744 default: return super.setProperty(hash, name, value); 1745 } 1746 1747 } 1748 1749 @Override 1750 public Base setProperty(String name, Base value) throws FHIRException { 1751 if (name.equals("identifier")) { 1752 this.identifier = castToIdentifier(value); // Identifier 1753 } else if (name.equals("basedOn")) { 1754 this.basedOn = castToReference(value); // Reference 1755 } else if (name.equals("parent")) { 1756 this.parent = castToReference(value); // Reference 1757 } else if (name.equals("status")) { 1758 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 1759 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 1760 } else if (name.equals("method")) { 1761 this.method = castToCodeableConcept(value); // CodeableConcept 1762 } else if (name.equals("code")) { 1763 this.code = castToCodeableConcept(value); // CodeableConcept 1764 } else if (name.equals("subject")) { 1765 this.subject = castToReference(value); // Reference 1766 } else if (name.equals("context")) { 1767 this.context = castToReference(value); // Reference 1768 } else if (name.equals("occurrence[x]")) { 1769 this.occurrence = castToType(value); // Type 1770 } else if (name.equals("condition")) { 1771 this.condition = castToReference(value); // Reference 1772 } else if (name.equals("performer")) { 1773 this.performer = castToReference(value); // Reference 1774 } else if (name.equals("reason[x]")) { 1775 this.reason = castToType(value); // Type 1776 } else if (name.equals("basis")) { 1777 this.getBasis().add(castToReference(value)); 1778 } else if (name.equals("prediction")) { 1779 this.getPrediction().add((RiskAssessmentPredictionComponent) value); 1780 } else if (name.equals("mitigation")) { 1781 this.mitigation = castToString(value); // StringType 1782 } else if (name.equals("comment")) { 1783 this.comment = castToString(value); // StringType 1784 } else 1785 return super.setProperty(name, value); 1786 return value; 1787 } 1788 1789 @Override 1790 public Base makeProperty(int hash, String name) throws FHIRException { 1791 switch (hash) { 1792 case -1618432855: return getIdentifier(); 1793 case -332612366: return getBasedOn(); 1794 case -995424086: return getParent(); 1795 case -892481550: return getStatusElement(); 1796 case -1077554975: return getMethod(); 1797 case 3059181: return getCode(); 1798 case -1867885268: return getSubject(); 1799 case 951530927: return getContext(); 1800 case -2022646513: return getOccurrence(); 1801 case 1687874001: return getOccurrence(); 1802 case -861311717: return getCondition(); 1803 case 481140686: return getPerformer(); 1804 case -669418564: return getReason(); 1805 case -934964668: return getReason(); 1806 case 93508670: return addBasis(); 1807 case 1161234575: return addPrediction(); 1808 case 1293793087: return getMitigationElement(); 1809 case 950398559: return getCommentElement(); 1810 default: return super.makeProperty(hash, name); 1811 } 1812 1813 } 1814 1815 @Override 1816 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1817 switch (hash) { 1818 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1819 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1820 case -995424086: /*parent*/ return new String[] {"Reference"}; 1821 case -892481550: /*status*/ return new String[] {"code"}; 1822 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1823 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1824 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1825 case 951530927: /*context*/ return new String[] {"Reference"}; 1826 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 1827 case -861311717: /*condition*/ return new String[] {"Reference"}; 1828 case 481140686: /*performer*/ return new String[] {"Reference"}; 1829 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 1830 case 93508670: /*basis*/ return new String[] {"Reference"}; 1831 case 1161234575: /*prediction*/ return new String[] {}; 1832 case 1293793087: /*mitigation*/ return new String[] {"string"}; 1833 case 950398559: /*comment*/ return new String[] {"string"}; 1834 default: return super.getTypesForProperty(hash, name); 1835 } 1836 1837 } 1838 1839 @Override 1840 public Base addChild(String name) throws FHIRException { 1841 if (name.equals("identifier")) { 1842 this.identifier = new Identifier(); 1843 return this.identifier; 1844 } 1845 else if (name.equals("basedOn")) { 1846 this.basedOn = new Reference(); 1847 return this.basedOn; 1848 } 1849 else if (name.equals("parent")) { 1850 this.parent = new Reference(); 1851 return this.parent; 1852 } 1853 else if (name.equals("status")) { 1854 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.status"); 1855 } 1856 else if (name.equals("method")) { 1857 this.method = new CodeableConcept(); 1858 return this.method; 1859 } 1860 else if (name.equals("code")) { 1861 this.code = new CodeableConcept(); 1862 return this.code; 1863 } 1864 else if (name.equals("subject")) { 1865 this.subject = new Reference(); 1866 return this.subject; 1867 } 1868 else if (name.equals("context")) { 1869 this.context = new Reference(); 1870 return this.context; 1871 } 1872 else if (name.equals("occurrenceDateTime")) { 1873 this.occurrence = new DateTimeType(); 1874 return this.occurrence; 1875 } 1876 else if (name.equals("occurrencePeriod")) { 1877 this.occurrence = new Period(); 1878 return this.occurrence; 1879 } 1880 else if (name.equals("condition")) { 1881 this.condition = new Reference(); 1882 return this.condition; 1883 } 1884 else if (name.equals("performer")) { 1885 this.performer = new Reference(); 1886 return this.performer; 1887 } 1888 else if (name.equals("reasonCodeableConcept")) { 1889 this.reason = new CodeableConcept(); 1890 return this.reason; 1891 } 1892 else if (name.equals("reasonReference")) { 1893 this.reason = new Reference(); 1894 return this.reason; 1895 } 1896 else if (name.equals("basis")) { 1897 return addBasis(); 1898 } 1899 else if (name.equals("prediction")) { 1900 return addPrediction(); 1901 } 1902 else if (name.equals("mitigation")) { 1903 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.mitigation"); 1904 } 1905 else if (name.equals("comment")) { 1906 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.comment"); 1907 } 1908 else 1909 return super.addChild(name); 1910 } 1911 1912 public String fhirType() { 1913 return "RiskAssessment"; 1914 1915 } 1916 1917 public RiskAssessment copy() { 1918 RiskAssessment dst = new RiskAssessment(); 1919 copyValues(dst); 1920 dst.identifier = identifier == null ? null : identifier.copy(); 1921 dst.basedOn = basedOn == null ? null : basedOn.copy(); 1922 dst.parent = parent == null ? null : parent.copy(); 1923 dst.status = status == null ? null : status.copy(); 1924 dst.method = method == null ? null : method.copy(); 1925 dst.code = code == null ? null : code.copy(); 1926 dst.subject = subject == null ? null : subject.copy(); 1927 dst.context = context == null ? null : context.copy(); 1928 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1929 dst.condition = condition == null ? null : condition.copy(); 1930 dst.performer = performer == null ? null : performer.copy(); 1931 dst.reason = reason == null ? null : reason.copy(); 1932 if (basis != null) { 1933 dst.basis = new ArrayList<Reference>(); 1934 for (Reference i : basis) 1935 dst.basis.add(i.copy()); 1936 }; 1937 if (prediction != null) { 1938 dst.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1939 for (RiskAssessmentPredictionComponent i : prediction) 1940 dst.prediction.add(i.copy()); 1941 }; 1942 dst.mitigation = mitigation == null ? null : mitigation.copy(); 1943 dst.comment = comment == null ? null : comment.copy(); 1944 return dst; 1945 } 1946 1947 protected RiskAssessment typedCopy() { 1948 return copy(); 1949 } 1950 1951 @Override 1952 public boolean equalsDeep(Base other_) { 1953 if (!super.equalsDeep(other_)) 1954 return false; 1955 if (!(other_ instanceof RiskAssessment)) 1956 return false; 1957 RiskAssessment o = (RiskAssessment) other_; 1958 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(parent, o.parent, true) 1959 && compareDeep(status, o.status, true) && compareDeep(method, o.method, true) && compareDeep(code, o.code, true) 1960 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) 1961 && compareDeep(condition, o.condition, true) && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) 1962 && compareDeep(basis, o.basis, true) && compareDeep(prediction, o.prediction, true) && compareDeep(mitigation, o.mitigation, true) 1963 && compareDeep(comment, o.comment, true); 1964 } 1965 1966 @Override 1967 public boolean equalsShallow(Base other_) { 1968 if (!super.equalsShallow(other_)) 1969 return false; 1970 if (!(other_ instanceof RiskAssessment)) 1971 return false; 1972 RiskAssessment o = (RiskAssessment) other_; 1973 return compareValues(status, o.status, true) && compareValues(mitigation, o.mitigation, true) && compareValues(comment, o.comment, true) 1974 ; 1975 } 1976 1977 public boolean isEmpty() { 1978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, parent 1979 , status, method, code, subject, context, occurrence, condition, performer, reason 1980 , basis, prediction, mitigation, comment); 1981 } 1982 1983 @Override 1984 public ResourceType getResourceType() { 1985 return ResourceType.RiskAssessment; 1986 } 1987 1988 /** 1989 * Search parameter: <b>date</b> 1990 * <p> 1991 * Description: <b>When was assessment made?</b><br> 1992 * Type: <b>date</b><br> 1993 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 1994 * </p> 1995 */ 1996 @SearchParamDefinition(name="date", path="RiskAssessment.occurrence.as(DateTime)", description="When was assessment made?", type="date" ) 1997 public static final String SP_DATE = "date"; 1998 /** 1999 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2000 * <p> 2001 * Description: <b>When was assessment made?</b><br> 2002 * Type: <b>date</b><br> 2003 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 2004 * </p> 2005 */ 2006 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2007 2008 /** 2009 * Search parameter: <b>identifier</b> 2010 * <p> 2011 * Description: <b>Unique identifier for the assessment</b><br> 2012 * Type: <b>token</b><br> 2013 * Path: <b>RiskAssessment.identifier</b><br> 2014 * </p> 2015 */ 2016 @SearchParamDefinition(name="identifier", path="RiskAssessment.identifier", description="Unique identifier for the assessment", type="token" ) 2017 public static final String SP_IDENTIFIER = "identifier"; 2018 /** 2019 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2020 * <p> 2021 * Description: <b>Unique identifier for the assessment</b><br> 2022 * Type: <b>token</b><br> 2023 * Path: <b>RiskAssessment.identifier</b><br> 2024 * </p> 2025 */ 2026 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2027 2028 /** 2029 * Search parameter: <b>condition</b> 2030 * <p> 2031 * Description: <b>Condition assessed</b><br> 2032 * Type: <b>reference</b><br> 2033 * Path: <b>RiskAssessment.condition</b><br> 2034 * </p> 2035 */ 2036 @SearchParamDefinition(name="condition", path="RiskAssessment.condition", description="Condition assessed", type="reference", target={Condition.class } ) 2037 public static final String SP_CONDITION = "condition"; 2038 /** 2039 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2040 * <p> 2041 * Description: <b>Condition assessed</b><br> 2042 * Type: <b>reference</b><br> 2043 * Path: <b>RiskAssessment.condition</b><br> 2044 * </p> 2045 */ 2046 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONDITION); 2047 2048/** 2049 * Constant for fluent queries to be used to add include statements. Specifies 2050 * the path value of "<b>RiskAssessment:condition</b>". 2051 */ 2052 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include("RiskAssessment:condition").toLocked(); 2053 2054 /** 2055 * Search parameter: <b>performer</b> 2056 * <p> 2057 * Description: <b>Who did assessment?</b><br> 2058 * Type: <b>reference</b><br> 2059 * Path: <b>RiskAssessment.performer</b><br> 2060 * </p> 2061 */ 2062 @SearchParamDefinition(name="performer", path="RiskAssessment.performer", description="Who did assessment?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Practitioner.class } ) 2063 public static final String SP_PERFORMER = "performer"; 2064 /** 2065 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2066 * <p> 2067 * Description: <b>Who did assessment?</b><br> 2068 * Type: <b>reference</b><br> 2069 * Path: <b>RiskAssessment.performer</b><br> 2070 * </p> 2071 */ 2072 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2073 2074/** 2075 * Constant for fluent queries to be used to add include statements. Specifies 2076 * the path value of "<b>RiskAssessment:performer</b>". 2077 */ 2078 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("RiskAssessment:performer").toLocked(); 2079 2080 /** 2081 * Search parameter: <b>method</b> 2082 * <p> 2083 * Description: <b>Evaluation mechanism</b><br> 2084 * Type: <b>token</b><br> 2085 * Path: <b>RiskAssessment.method</b><br> 2086 * </p> 2087 */ 2088 @SearchParamDefinition(name="method", path="RiskAssessment.method", description="Evaluation mechanism", type="token" ) 2089 public static final String SP_METHOD = "method"; 2090 /** 2091 * <b>Fluent Client</b> search parameter constant for <b>method</b> 2092 * <p> 2093 * Description: <b>Evaluation mechanism</b><br> 2094 * Type: <b>token</b><br> 2095 * Path: <b>RiskAssessment.method</b><br> 2096 * </p> 2097 */ 2098 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 2099 2100 /** 2101 * Search parameter: <b>subject</b> 2102 * <p> 2103 * Description: <b>Who/what does assessment apply to?</b><br> 2104 * Type: <b>reference</b><br> 2105 * Path: <b>RiskAssessment.subject</b><br> 2106 * </p> 2107 */ 2108 @SearchParamDefinition(name="subject", path="RiskAssessment.subject", description="Who/what does assessment apply to?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2109 public static final String SP_SUBJECT = "subject"; 2110 /** 2111 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2112 * <p> 2113 * Description: <b>Who/what does assessment apply to?</b><br> 2114 * Type: <b>reference</b><br> 2115 * Path: <b>RiskAssessment.subject</b><br> 2116 * </p> 2117 */ 2118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2119 2120/** 2121 * Constant for fluent queries to be used to add include statements. Specifies 2122 * the path value of "<b>RiskAssessment:subject</b>". 2123 */ 2124 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RiskAssessment:subject").toLocked(); 2125 2126 /** 2127 * Search parameter: <b>patient</b> 2128 * <p> 2129 * Description: <b>Who/what does assessment apply to?</b><br> 2130 * Type: <b>reference</b><br> 2131 * Path: <b>RiskAssessment.subject</b><br> 2132 * </p> 2133 */ 2134 @SearchParamDefinition(name="patient", path="RiskAssessment.subject", description="Who/what does assessment apply to?", type="reference", target={Patient.class } ) 2135 public static final String SP_PATIENT = "patient"; 2136 /** 2137 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2138 * <p> 2139 * Description: <b>Who/what does assessment apply to?</b><br> 2140 * Type: <b>reference</b><br> 2141 * Path: <b>RiskAssessment.subject</b><br> 2142 * </p> 2143 */ 2144 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2145 2146/** 2147 * Constant for fluent queries to be used to add include statements. Specifies 2148 * the path value of "<b>RiskAssessment:patient</b>". 2149 */ 2150 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RiskAssessment:patient").toLocked(); 2151 2152 /** 2153 * Search parameter: <b>probability</b> 2154 * <p> 2155 * Description: <b>Likelihood of specified outcome</b><br> 2156 * Type: <b>number</b><br> 2157 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2158 * </p> 2159 */ 2160 @SearchParamDefinition(name="probability", path="RiskAssessment.prediction.probability", description="Likelihood of specified outcome", type="number" ) 2161 public static final String SP_PROBABILITY = "probability"; 2162 /** 2163 * <b>Fluent Client</b> search parameter constant for <b>probability</b> 2164 * <p> 2165 * Description: <b>Likelihood of specified outcome</b><br> 2166 * Type: <b>number</b><br> 2167 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2168 * </p> 2169 */ 2170 public static final ca.uhn.fhir.rest.gclient.NumberClientParam PROBABILITY = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_PROBABILITY); 2171 2172 /** 2173 * Search parameter: <b>risk</b> 2174 * <p> 2175 * Description: <b>Likelihood of specified outcome as a qualitative value</b><br> 2176 * Type: <b>token</b><br> 2177 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2178 * </p> 2179 */ 2180 @SearchParamDefinition(name="risk", path="RiskAssessment.prediction.qualitativeRisk", description="Likelihood of specified outcome as a qualitative value", type="token" ) 2181 public static final String SP_RISK = "risk"; 2182 /** 2183 * <b>Fluent Client</b> search parameter constant for <b>risk</b> 2184 * <p> 2185 * Description: <b>Likelihood of specified outcome as a qualitative value</b><br> 2186 * Type: <b>token</b><br> 2187 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2188 * </p> 2189 */ 2190 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RISK = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RISK); 2191 2192 /** 2193 * Search parameter: <b>encounter</b> 2194 * <p> 2195 * Description: <b>Where was assessment performed?</b><br> 2196 * Type: <b>reference</b><br> 2197 * Path: <b>RiskAssessment.context</b><br> 2198 * </p> 2199 */ 2200 @SearchParamDefinition(name="encounter", path="RiskAssessment.context", description="Where was assessment performed?", type="reference", target={Encounter.class } ) 2201 public static final String SP_ENCOUNTER = "encounter"; 2202 /** 2203 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2204 * <p> 2205 * Description: <b>Where was assessment performed?</b><br> 2206 * Type: <b>reference</b><br> 2207 * Path: <b>RiskAssessment.context</b><br> 2208 * </p> 2209 */ 2210 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2211 2212/** 2213 * Constant for fluent queries to be used to add include statements. Specifies 2214 * the path value of "<b>RiskAssessment:encounter</b>". 2215 */ 2216 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("RiskAssessment:encounter").toLocked(); 2217 2218 2219}