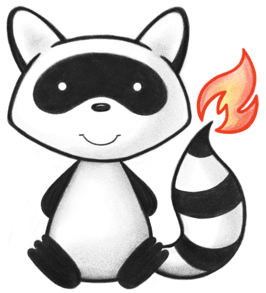
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043/** 044 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 045 */ 046@DatatypeDef(name="SampledData") 047public class SampledData extends Type implements ICompositeType { 048 049 /** 050 * The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series. 051 */ 052 @Child(name = "origin", type = {SimpleQuantity.class}, order=0, min=1, max=1, modifier=false, summary=true) 053 @Description(shortDefinition="Zero value and units", formalDefinition="The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series." ) 054 protected SimpleQuantity origin; 055 056 /** 057 * The length of time between sampling times, measured in milliseconds. 058 */ 059 @Child(name = "period", type = {DecimalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Number of milliseconds between samples", formalDefinition="The length of time between sampling times, measured in milliseconds." ) 061 protected DecimalType period; 062 063 /** 064 * A correction factor that is applied to the sampled data points before they are added to the origin. 065 */ 066 @Child(name = "factor", type = {DecimalType.class}, order=2, min=0, max=1, modifier=false, summary=true) 067 @Description(shortDefinition="Multiply data by this before adding to origin", formalDefinition="A correction factor that is applied to the sampled data points before they are added to the origin." ) 068 protected DecimalType factor; 069 070 /** 071 * The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 072 */ 073 @Child(name = "lowerLimit", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Lower limit of detection", formalDefinition="The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit)." ) 075 protected DecimalType lowerLimit; 076 077 /** 078 * The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 079 */ 080 @Child(name = "upperLimit", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="Upper limit of detection", formalDefinition="The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit)." ) 082 protected DecimalType upperLimit; 083 084 /** 085 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 086 */ 087 @Child(name = "dimensions", type = {PositiveIntType.class}, order=5, min=1, max=1, modifier=false, summary=true) 088 @Description(shortDefinition="Number of sample points at each time point", formalDefinition="The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once." ) 089 protected PositiveIntType dimensions; 090 091 /** 092 * A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value. 093 */ 094 @Child(name = "data", type = {StringType.class}, order=6, min=1, max=1, modifier=false, summary=false) 095 @Description(shortDefinition="Decimal values with spaces, or \"E\" | \"U\" | \"L\"", formalDefinition="A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value." ) 096 protected StringType data; 097 098 private static final long serialVersionUID = -1763278368L; 099 100 /** 101 * Constructor 102 */ 103 public SampledData() { 104 super(); 105 } 106 107 /** 108 * Constructor 109 */ 110 public SampledData(SimpleQuantity origin, DecimalType period, PositiveIntType dimensions, StringType data) { 111 super(); 112 this.origin = origin; 113 this.period = period; 114 this.dimensions = dimensions; 115 this.data = data; 116 } 117 118 /** 119 * @return {@link #origin} (The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.) 120 */ 121 public SimpleQuantity getOrigin() { 122 if (this.origin == null) 123 if (Configuration.errorOnAutoCreate()) 124 throw new Error("Attempt to auto-create SampledData.origin"); 125 else if (Configuration.doAutoCreate()) 126 this.origin = new SimpleQuantity(); // cc 127 return this.origin; 128 } 129 130 public boolean hasOrigin() { 131 return this.origin != null && !this.origin.isEmpty(); 132 } 133 134 /** 135 * @param value {@link #origin} (The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.) 136 */ 137 public SampledData setOrigin(SimpleQuantity value) { 138 this.origin = value; 139 return this; 140 } 141 142 /** 143 * @return {@link #period} (The length of time between sampling times, measured in milliseconds.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 144 */ 145 public DecimalType getPeriodElement() { 146 if (this.period == null) 147 if (Configuration.errorOnAutoCreate()) 148 throw new Error("Attempt to auto-create SampledData.period"); 149 else if (Configuration.doAutoCreate()) 150 this.period = new DecimalType(); // bb 151 return this.period; 152 } 153 154 public boolean hasPeriodElement() { 155 return this.period != null && !this.period.isEmpty(); 156 } 157 158 public boolean hasPeriod() { 159 return this.period != null && !this.period.isEmpty(); 160 } 161 162 /** 163 * @param value {@link #period} (The length of time between sampling times, measured in milliseconds.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 164 */ 165 public SampledData setPeriodElement(DecimalType value) { 166 this.period = value; 167 return this; 168 } 169 170 /** 171 * @return The length of time between sampling times, measured in milliseconds. 172 */ 173 public BigDecimal getPeriod() { 174 return this.period == null ? null : this.period.getValue(); 175 } 176 177 /** 178 * @param value The length of time between sampling times, measured in milliseconds. 179 */ 180 public SampledData setPeriod(BigDecimal value) { 181 if (this.period == null) 182 this.period = new DecimalType(); 183 this.period.setValue(value); 184 return this; 185 } 186 187 /** 188 * @param value The length of time between sampling times, measured in milliseconds. 189 */ 190 public SampledData setPeriod(long value) { 191 this.period = new DecimalType(); 192 this.period.setValue(value); 193 return this; 194 } 195 196 /** 197 * @param value The length of time between sampling times, measured in milliseconds. 198 */ 199 public SampledData setPeriod(double value) { 200 this.period = new DecimalType(); 201 this.period.setValue(value); 202 return this; 203 } 204 205 /** 206 * @return {@link #factor} (A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 207 */ 208 public DecimalType getFactorElement() { 209 if (this.factor == null) 210 if (Configuration.errorOnAutoCreate()) 211 throw new Error("Attempt to auto-create SampledData.factor"); 212 else if (Configuration.doAutoCreate()) 213 this.factor = new DecimalType(); // bb 214 return this.factor; 215 } 216 217 public boolean hasFactorElement() { 218 return this.factor != null && !this.factor.isEmpty(); 219 } 220 221 public boolean hasFactor() { 222 return this.factor != null && !this.factor.isEmpty(); 223 } 224 225 /** 226 * @param value {@link #factor} (A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 227 */ 228 public SampledData setFactorElement(DecimalType value) { 229 this.factor = value; 230 return this; 231 } 232 233 /** 234 * @return A correction factor that is applied to the sampled data points before they are added to the origin. 235 */ 236 public BigDecimal getFactor() { 237 return this.factor == null ? null : this.factor.getValue(); 238 } 239 240 /** 241 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 242 */ 243 public SampledData setFactor(BigDecimal value) { 244 if (value == null) 245 this.factor = null; 246 else { 247 if (this.factor == null) 248 this.factor = new DecimalType(); 249 this.factor.setValue(value); 250 } 251 return this; 252 } 253 254 /** 255 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 256 */ 257 public SampledData setFactor(long value) { 258 this.factor = new DecimalType(); 259 this.factor.setValue(value); 260 return this; 261 } 262 263 /** 264 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 265 */ 266 public SampledData setFactor(double value) { 267 this.factor = new DecimalType(); 268 this.factor.setValue(value); 269 return this; 270 } 271 272 /** 273 * @return {@link #lowerLimit} (The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value 274 */ 275 public DecimalType getLowerLimitElement() { 276 if (this.lowerLimit == null) 277 if (Configuration.errorOnAutoCreate()) 278 throw new Error("Attempt to auto-create SampledData.lowerLimit"); 279 else if (Configuration.doAutoCreate()) 280 this.lowerLimit = new DecimalType(); // bb 281 return this.lowerLimit; 282 } 283 284 public boolean hasLowerLimitElement() { 285 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 286 } 287 288 public boolean hasLowerLimit() { 289 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 290 } 291 292 /** 293 * @param value {@link #lowerLimit} (The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value 294 */ 295 public SampledData setLowerLimitElement(DecimalType value) { 296 this.lowerLimit = value; 297 return this; 298 } 299 300 /** 301 * @return The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 302 */ 303 public BigDecimal getLowerLimit() { 304 return this.lowerLimit == null ? null : this.lowerLimit.getValue(); 305 } 306 307 /** 308 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 309 */ 310 public SampledData setLowerLimit(BigDecimal value) { 311 if (value == null) 312 this.lowerLimit = null; 313 else { 314 if (this.lowerLimit == null) 315 this.lowerLimit = new DecimalType(); 316 this.lowerLimit.setValue(value); 317 } 318 return this; 319 } 320 321 /** 322 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 323 */ 324 public SampledData setLowerLimit(long value) { 325 this.lowerLimit = new DecimalType(); 326 this.lowerLimit.setValue(value); 327 return this; 328 } 329 330 /** 331 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 332 */ 333 public SampledData setLowerLimit(double value) { 334 this.lowerLimit = new DecimalType(); 335 this.lowerLimit.setValue(value); 336 return this; 337 } 338 339 /** 340 * @return {@link #upperLimit} (The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value 341 */ 342 public DecimalType getUpperLimitElement() { 343 if (this.upperLimit == null) 344 if (Configuration.errorOnAutoCreate()) 345 throw new Error("Attempt to auto-create SampledData.upperLimit"); 346 else if (Configuration.doAutoCreate()) 347 this.upperLimit = new DecimalType(); // bb 348 return this.upperLimit; 349 } 350 351 public boolean hasUpperLimitElement() { 352 return this.upperLimit != null && !this.upperLimit.isEmpty(); 353 } 354 355 public boolean hasUpperLimit() { 356 return this.upperLimit != null && !this.upperLimit.isEmpty(); 357 } 358 359 /** 360 * @param value {@link #upperLimit} (The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value 361 */ 362 public SampledData setUpperLimitElement(DecimalType value) { 363 this.upperLimit = value; 364 return this; 365 } 366 367 /** 368 * @return The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 369 */ 370 public BigDecimal getUpperLimit() { 371 return this.upperLimit == null ? null : this.upperLimit.getValue(); 372 } 373 374 /** 375 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 376 */ 377 public SampledData setUpperLimit(BigDecimal value) { 378 if (value == null) 379 this.upperLimit = null; 380 else { 381 if (this.upperLimit == null) 382 this.upperLimit = new DecimalType(); 383 this.upperLimit.setValue(value); 384 } 385 return this; 386 } 387 388 /** 389 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 390 */ 391 public SampledData setUpperLimit(long value) { 392 this.upperLimit = new DecimalType(); 393 this.upperLimit.setValue(value); 394 return this; 395 } 396 397 /** 398 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 399 */ 400 public SampledData setUpperLimit(double value) { 401 this.upperLimit = new DecimalType(); 402 this.upperLimit.setValue(value); 403 return this; 404 } 405 406 /** 407 * @return {@link #dimensions} (The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value 408 */ 409 public PositiveIntType getDimensionsElement() { 410 if (this.dimensions == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create SampledData.dimensions"); 413 else if (Configuration.doAutoCreate()) 414 this.dimensions = new PositiveIntType(); // bb 415 return this.dimensions; 416 } 417 418 public boolean hasDimensionsElement() { 419 return this.dimensions != null && !this.dimensions.isEmpty(); 420 } 421 422 public boolean hasDimensions() { 423 return this.dimensions != null && !this.dimensions.isEmpty(); 424 } 425 426 /** 427 * @param value {@link #dimensions} (The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value 428 */ 429 public SampledData setDimensionsElement(PositiveIntType value) { 430 this.dimensions = value; 431 return this; 432 } 433 434 /** 435 * @return The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 436 */ 437 public int getDimensions() { 438 return this.dimensions == null || this.dimensions.isEmpty() ? 0 : this.dimensions.getValue(); 439 } 440 441 /** 442 * @param value The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 443 */ 444 public SampledData setDimensions(int value) { 445 if (this.dimensions == null) 446 this.dimensions = new PositiveIntType(); 447 this.dimensions.setValue(value); 448 return this; 449 } 450 451 /** 452 * @return {@link #data} (A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 453 */ 454 public StringType getDataElement() { 455 if (this.data == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create SampledData.data"); 458 else if (Configuration.doAutoCreate()) 459 this.data = new StringType(); // bb 460 return this.data; 461 } 462 463 public boolean hasDataElement() { 464 return this.data != null && !this.data.isEmpty(); 465 } 466 467 public boolean hasData() { 468 return this.data != null && !this.data.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #data} (A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 473 */ 474 public SampledData setDataElement(StringType value) { 475 this.data = value; 476 return this; 477 } 478 479 /** 480 * @return A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value. 481 */ 482 public String getData() { 483 return this.data == null ? null : this.data.getValue(); 484 } 485 486 /** 487 * @param value A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value. 488 */ 489 public SampledData setData(String value) { 490 if (this.data == null) 491 this.data = new StringType(); 492 this.data.setValue(value); 493 return this; 494 } 495 496 protected void listChildren(List<Property> children) { 497 super.listChildren(children); 498 children.add(new Property("origin", "SimpleQuantity", "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 0, 1, origin)); 499 children.add(new Property("period", "decimal", "The length of time between sampling times, measured in milliseconds.", 0, 1, period)); 500 children.add(new Property("factor", "decimal", "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, factor)); 501 children.add(new Property("lowerLimit", "decimal", "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 0, 1, lowerLimit)); 502 children.add(new Property("upperLimit", "decimal", "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 0, 1, upperLimit)); 503 children.add(new Property("dimensions", "positiveInt", "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 0, 1, dimensions)); 504 children.add(new Property("data", "string", "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.", 0, 1, data)); 505 } 506 507 @Override 508 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 509 switch (_hash) { 510 case -1008619738: /*origin*/ return new Property("origin", "SimpleQuantity", "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 0, 1, origin); 511 case -991726143: /*period*/ return new Property("period", "decimal", "The length of time between sampling times, measured in milliseconds.", 0, 1, period); 512 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, factor); 513 case 1209133370: /*lowerLimit*/ return new Property("lowerLimit", "decimal", "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 0, 1, lowerLimit); 514 case -1681713095: /*upperLimit*/ return new Property("upperLimit", "decimal", "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 0, 1, upperLimit); 515 case 414334925: /*dimensions*/ return new Property("dimensions", "positiveInt", "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 0, 1, dimensions); 516 case 3076010: /*data*/ return new Property("data", "string", "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.", 0, 1, data); 517 default: return super.getNamedProperty(_hash, _name, _checkValid); 518 } 519 520 } 521 522 @Override 523 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 524 switch (hash) { 525 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // SimpleQuantity 526 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DecimalType 527 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 528 case 1209133370: /*lowerLimit*/ return this.lowerLimit == null ? new Base[0] : new Base[] {this.lowerLimit}; // DecimalType 529 case -1681713095: /*upperLimit*/ return this.upperLimit == null ? new Base[0] : new Base[] {this.upperLimit}; // DecimalType 530 case 414334925: /*dimensions*/ return this.dimensions == null ? new Base[0] : new Base[] {this.dimensions}; // PositiveIntType 531 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // StringType 532 default: return super.getProperty(hash, name, checkValid); 533 } 534 535 } 536 537 @Override 538 public Base setProperty(int hash, String name, Base value) throws FHIRException { 539 switch (hash) { 540 case -1008619738: // origin 541 this.origin = castToSimpleQuantity(value); // SimpleQuantity 542 return value; 543 case -991726143: // period 544 this.period = castToDecimal(value); // DecimalType 545 return value; 546 case -1282148017: // factor 547 this.factor = castToDecimal(value); // DecimalType 548 return value; 549 case 1209133370: // lowerLimit 550 this.lowerLimit = castToDecimal(value); // DecimalType 551 return value; 552 case -1681713095: // upperLimit 553 this.upperLimit = castToDecimal(value); // DecimalType 554 return value; 555 case 414334925: // dimensions 556 this.dimensions = castToPositiveInt(value); // PositiveIntType 557 return value; 558 case 3076010: // data 559 this.data = castToString(value); // StringType 560 return value; 561 default: return super.setProperty(hash, name, value); 562 } 563 564 } 565 566 @Override 567 public Base setProperty(String name, Base value) throws FHIRException { 568 if (name.equals("origin")) { 569 this.origin = castToSimpleQuantity(value); // SimpleQuantity 570 } else if (name.equals("period")) { 571 this.period = castToDecimal(value); // DecimalType 572 } else if (name.equals("factor")) { 573 this.factor = castToDecimal(value); // DecimalType 574 } else if (name.equals("lowerLimit")) { 575 this.lowerLimit = castToDecimal(value); // DecimalType 576 } else if (name.equals("upperLimit")) { 577 this.upperLimit = castToDecimal(value); // DecimalType 578 } else if (name.equals("dimensions")) { 579 this.dimensions = castToPositiveInt(value); // PositiveIntType 580 } else if (name.equals("data")) { 581 this.data = castToString(value); // StringType 582 } else 583 return super.setProperty(name, value); 584 return value; 585 } 586 587 @Override 588 public Base makeProperty(int hash, String name) throws FHIRException { 589 switch (hash) { 590 case -1008619738: return getOrigin(); 591 case -991726143: return getPeriodElement(); 592 case -1282148017: return getFactorElement(); 593 case 1209133370: return getLowerLimitElement(); 594 case -1681713095: return getUpperLimitElement(); 595 case 414334925: return getDimensionsElement(); 596 case 3076010: return getDataElement(); 597 default: return super.makeProperty(hash, name); 598 } 599 600 } 601 602 @Override 603 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 604 switch (hash) { 605 case -1008619738: /*origin*/ return new String[] {"SimpleQuantity"}; 606 case -991726143: /*period*/ return new String[] {"decimal"}; 607 case -1282148017: /*factor*/ return new String[] {"decimal"}; 608 case 1209133370: /*lowerLimit*/ return new String[] {"decimal"}; 609 case -1681713095: /*upperLimit*/ return new String[] {"decimal"}; 610 case 414334925: /*dimensions*/ return new String[] {"positiveInt"}; 611 case 3076010: /*data*/ return new String[] {"string"}; 612 default: return super.getTypesForProperty(hash, name); 613 } 614 615 } 616 617 @Override 618 public Base addChild(String name) throws FHIRException { 619 if (name.equals("origin")) { 620 this.origin = new SimpleQuantity(); 621 return this.origin; 622 } 623 else if (name.equals("period")) { 624 throw new FHIRException("Cannot call addChild on a singleton property SampledData.period"); 625 } 626 else if (name.equals("factor")) { 627 throw new FHIRException("Cannot call addChild on a singleton property SampledData.factor"); 628 } 629 else if (name.equals("lowerLimit")) { 630 throw new FHIRException("Cannot call addChild on a singleton property SampledData.lowerLimit"); 631 } 632 else if (name.equals("upperLimit")) { 633 throw new FHIRException("Cannot call addChild on a singleton property SampledData.upperLimit"); 634 } 635 else if (name.equals("dimensions")) { 636 throw new FHIRException("Cannot call addChild on a singleton property SampledData.dimensions"); 637 } 638 else if (name.equals("data")) { 639 throw new FHIRException("Cannot call addChild on a singleton property SampledData.data"); 640 } 641 else 642 return super.addChild(name); 643 } 644 645 public String fhirType() { 646 return "SampledData"; 647 648 } 649 650 public SampledData copy() { 651 SampledData dst = new SampledData(); 652 copyValues(dst); 653 dst.origin = origin == null ? null : origin.copy(); 654 dst.period = period == null ? null : period.copy(); 655 dst.factor = factor == null ? null : factor.copy(); 656 dst.lowerLimit = lowerLimit == null ? null : lowerLimit.copy(); 657 dst.upperLimit = upperLimit == null ? null : upperLimit.copy(); 658 dst.dimensions = dimensions == null ? null : dimensions.copy(); 659 dst.data = data == null ? null : data.copy(); 660 return dst; 661 } 662 663 protected SampledData typedCopy() { 664 return copy(); 665 } 666 667 @Override 668 public boolean equalsDeep(Base other_) { 669 if (!super.equalsDeep(other_)) 670 return false; 671 if (!(other_ instanceof SampledData)) 672 return false; 673 SampledData o = (SampledData) other_; 674 return compareDeep(origin, o.origin, true) && compareDeep(period, o.period, true) && compareDeep(factor, o.factor, true) 675 && compareDeep(lowerLimit, o.lowerLimit, true) && compareDeep(upperLimit, o.upperLimit, true) && compareDeep(dimensions, o.dimensions, true) 676 && compareDeep(data, o.data, true); 677 } 678 679 @Override 680 public boolean equalsShallow(Base other_) { 681 if (!super.equalsShallow(other_)) 682 return false; 683 if (!(other_ instanceof SampledData)) 684 return false; 685 SampledData o = (SampledData) other_; 686 return compareValues(period, o.period, true) && compareValues(factor, o.factor, true) && compareValues(lowerLimit, o.lowerLimit, true) 687 && compareValues(upperLimit, o.upperLimit, true) && compareValues(dimensions, o.dimensions, true) && compareValues(data, o.data, true) 688 ; 689 } 690 691 public boolean isEmpty() { 692 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(origin, period, factor, lowerLimit 693 , upperLimit, dimensions, data); 694 } 695 696 697}