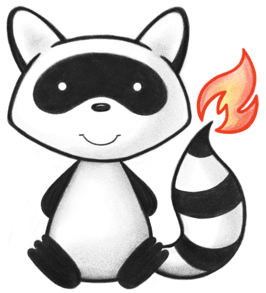
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * A container for slots of time that may be available for booking appointments. 047 */ 048@ResourceDef(name="Schedule", profile="http://hl7.org/fhir/Profile/Schedule") 049public class Schedule extends DomainResource { 050 051 /** 052 * External Ids for this item. 053 */ 054 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 055 @Description(shortDefinition="External Ids for this item", formalDefinition="External Ids for this item." ) 056 protected List<Identifier> identifier; 057 058 /** 059 * Whether this schedule record is in active use, or should not be used (such as was entered in error). 060 */ 061 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 062 @Description(shortDefinition="Whether this schedule is in active use", formalDefinition="Whether this schedule record is in active use, or should not be used (such as was entered in error)." ) 063 protected BooleanType active; 064 065 /** 066 * A broad categorisation of the service that is to be performed during this appointment. 067 */ 068 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="A broad categorisation of the service that is to be performed during this appointment", formalDefinition="A broad categorisation of the service that is to be performed during this appointment." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 071 protected CodeableConcept serviceCategory; 072 073 /** 074 * The specific service that is to be performed during this appointment. 075 */ 076 @Child(name = "serviceType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 077 @Description(shortDefinition="The specific service that is to be performed during this appointment", formalDefinition="The specific service that is to be performed during this appointment." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 079 protected List<CodeableConcept> serviceType; 080 081 /** 082 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 083 */ 084 @Child(name = "specialty", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 085 @Description(shortDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 086 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 087 protected List<CodeableConcept> specialty; 088 089 /** 090 * The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson. 091 */ 092 @Child(name = "actor", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 093 @Description(shortDefinition="The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson", formalDefinition="The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson." ) 094 protected List<Reference> actor; 095 /** 096 * The actual objects that are the target of the reference (The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson.) 097 */ 098 protected List<Resource> actorTarget; 099 100 101 /** 102 * The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates. 103 */ 104 @Child(name = "planningHorizon", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 105 @Description(shortDefinition="The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates", formalDefinition="The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates." ) 106 protected Period planningHorizon; 107 108 /** 109 * Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 110 */ 111 @Child(name = "comment", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 112 @Description(shortDefinition="Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated", formalDefinition="Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated." ) 113 protected StringType comment; 114 115 private static final long serialVersionUID = -266680210L; 116 117 /** 118 * Constructor 119 */ 120 public Schedule() { 121 super(); 122 } 123 124 /** 125 * @return {@link #identifier} (External Ids for this item.) 126 */ 127 public List<Identifier> getIdentifier() { 128 if (this.identifier == null) 129 this.identifier = new ArrayList<Identifier>(); 130 return this.identifier; 131 } 132 133 /** 134 * @return Returns a reference to <code>this</code> for easy method chaining 135 */ 136 public Schedule setIdentifier(List<Identifier> theIdentifier) { 137 this.identifier = theIdentifier; 138 return this; 139 } 140 141 public boolean hasIdentifier() { 142 if (this.identifier == null) 143 return false; 144 for (Identifier item : this.identifier) 145 if (!item.isEmpty()) 146 return true; 147 return false; 148 } 149 150 public Identifier addIdentifier() { //3 151 Identifier t = new Identifier(); 152 if (this.identifier == null) 153 this.identifier = new ArrayList<Identifier>(); 154 this.identifier.add(t); 155 return t; 156 } 157 158 public Schedule addIdentifier(Identifier t) { //3 159 if (t == null) 160 return this; 161 if (this.identifier == null) 162 this.identifier = new ArrayList<Identifier>(); 163 this.identifier.add(t); 164 return this; 165 } 166 167 /** 168 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 169 */ 170 public Identifier getIdentifierFirstRep() { 171 if (getIdentifier().isEmpty()) { 172 addIdentifier(); 173 } 174 return getIdentifier().get(0); 175 } 176 177 /** 178 * @return {@link #active} (Whether this schedule record is in active use, or should not be used (such as was entered in error).). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 179 */ 180 public BooleanType getActiveElement() { 181 if (this.active == null) 182 if (Configuration.errorOnAutoCreate()) 183 throw new Error("Attempt to auto-create Schedule.active"); 184 else if (Configuration.doAutoCreate()) 185 this.active = new BooleanType(); // bb 186 return this.active; 187 } 188 189 public boolean hasActiveElement() { 190 return this.active != null && !this.active.isEmpty(); 191 } 192 193 public boolean hasActive() { 194 return this.active != null && !this.active.isEmpty(); 195 } 196 197 /** 198 * @param value {@link #active} (Whether this schedule record is in active use, or should not be used (such as was entered in error).). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 199 */ 200 public Schedule setActiveElement(BooleanType value) { 201 this.active = value; 202 return this; 203 } 204 205 /** 206 * @return Whether this schedule record is in active use, or should not be used (such as was entered in error). 207 */ 208 public boolean getActive() { 209 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 210 } 211 212 /** 213 * @param value Whether this schedule record is in active use, or should not be used (such as was entered in error). 214 */ 215 public Schedule setActive(boolean value) { 216 if (this.active == null) 217 this.active = new BooleanType(); 218 this.active.setValue(value); 219 return this; 220 } 221 222 /** 223 * @return {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 224 */ 225 public CodeableConcept getServiceCategory() { 226 if (this.serviceCategory == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create Schedule.serviceCategory"); 229 else if (Configuration.doAutoCreate()) 230 this.serviceCategory = new CodeableConcept(); // cc 231 return this.serviceCategory; 232 } 233 234 public boolean hasServiceCategory() { 235 return this.serviceCategory != null && !this.serviceCategory.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 240 */ 241 public Schedule setServiceCategory(CodeableConcept value) { 242 this.serviceCategory = value; 243 return this; 244 } 245 246 /** 247 * @return {@link #serviceType} (The specific service that is to be performed during this appointment.) 248 */ 249 public List<CodeableConcept> getServiceType() { 250 if (this.serviceType == null) 251 this.serviceType = new ArrayList<CodeableConcept>(); 252 return this.serviceType; 253 } 254 255 /** 256 * @return Returns a reference to <code>this</code> for easy method chaining 257 */ 258 public Schedule setServiceType(List<CodeableConcept> theServiceType) { 259 this.serviceType = theServiceType; 260 return this; 261 } 262 263 public boolean hasServiceType() { 264 if (this.serviceType == null) 265 return false; 266 for (CodeableConcept item : this.serviceType) 267 if (!item.isEmpty()) 268 return true; 269 return false; 270 } 271 272 public CodeableConcept addServiceType() { //3 273 CodeableConcept t = new CodeableConcept(); 274 if (this.serviceType == null) 275 this.serviceType = new ArrayList<CodeableConcept>(); 276 this.serviceType.add(t); 277 return t; 278 } 279 280 public Schedule addServiceType(CodeableConcept t) { //3 281 if (t == null) 282 return this; 283 if (this.serviceType == null) 284 this.serviceType = new ArrayList<CodeableConcept>(); 285 this.serviceType.add(t); 286 return this; 287 } 288 289 /** 290 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist 291 */ 292 public CodeableConcept getServiceTypeFirstRep() { 293 if (getServiceType().isEmpty()) { 294 addServiceType(); 295 } 296 return getServiceType().get(0); 297 } 298 299 /** 300 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 301 */ 302 public List<CodeableConcept> getSpecialty() { 303 if (this.specialty == null) 304 this.specialty = new ArrayList<CodeableConcept>(); 305 return this.specialty; 306 } 307 308 /** 309 * @return Returns a reference to <code>this</code> for easy method chaining 310 */ 311 public Schedule setSpecialty(List<CodeableConcept> theSpecialty) { 312 this.specialty = theSpecialty; 313 return this; 314 } 315 316 public boolean hasSpecialty() { 317 if (this.specialty == null) 318 return false; 319 for (CodeableConcept item : this.specialty) 320 if (!item.isEmpty()) 321 return true; 322 return false; 323 } 324 325 public CodeableConcept addSpecialty() { //3 326 CodeableConcept t = new CodeableConcept(); 327 if (this.specialty == null) 328 this.specialty = new ArrayList<CodeableConcept>(); 329 this.specialty.add(t); 330 return t; 331 } 332 333 public Schedule addSpecialty(CodeableConcept t) { //3 334 if (t == null) 335 return this; 336 if (this.specialty == null) 337 this.specialty = new ArrayList<CodeableConcept>(); 338 this.specialty.add(t); 339 return this; 340 } 341 342 /** 343 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 344 */ 345 public CodeableConcept getSpecialtyFirstRep() { 346 if (getSpecialty().isEmpty()) { 347 addSpecialty(); 348 } 349 return getSpecialty().get(0); 350 } 351 352 /** 353 * @return {@link #actor} (The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson.) 354 */ 355 public List<Reference> getActor() { 356 if (this.actor == null) 357 this.actor = new ArrayList<Reference>(); 358 return this.actor; 359 } 360 361 /** 362 * @return Returns a reference to <code>this</code> for easy method chaining 363 */ 364 public Schedule setActor(List<Reference> theActor) { 365 this.actor = theActor; 366 return this; 367 } 368 369 public boolean hasActor() { 370 if (this.actor == null) 371 return false; 372 for (Reference item : this.actor) 373 if (!item.isEmpty()) 374 return true; 375 return false; 376 } 377 378 public Reference addActor() { //3 379 Reference t = new Reference(); 380 if (this.actor == null) 381 this.actor = new ArrayList<Reference>(); 382 this.actor.add(t); 383 return t; 384 } 385 386 public Schedule addActor(Reference t) { //3 387 if (t == null) 388 return this; 389 if (this.actor == null) 390 this.actor = new ArrayList<Reference>(); 391 this.actor.add(t); 392 return this; 393 } 394 395 /** 396 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 397 */ 398 public Reference getActorFirstRep() { 399 if (getActor().isEmpty()) { 400 addActor(); 401 } 402 return getActor().get(0); 403 } 404 405 /** 406 * @deprecated Use Reference#setResource(IBaseResource) instead 407 */ 408 @Deprecated 409 public List<Resource> getActorTarget() { 410 if (this.actorTarget == null) 411 this.actorTarget = new ArrayList<Resource>(); 412 return this.actorTarget; 413 } 414 415 /** 416 * @return {@link #planningHorizon} (The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates.) 417 */ 418 public Period getPlanningHorizon() { 419 if (this.planningHorizon == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create Schedule.planningHorizon"); 422 else if (Configuration.doAutoCreate()) 423 this.planningHorizon = new Period(); // cc 424 return this.planningHorizon; 425 } 426 427 public boolean hasPlanningHorizon() { 428 return this.planningHorizon != null && !this.planningHorizon.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #planningHorizon} (The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates.) 433 */ 434 public Schedule setPlanningHorizon(Period value) { 435 this.planningHorizon = value; 436 return this; 437 } 438 439 /** 440 * @return {@link #comment} (Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 441 */ 442 public StringType getCommentElement() { 443 if (this.comment == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create Schedule.comment"); 446 else if (Configuration.doAutoCreate()) 447 this.comment = new StringType(); // bb 448 return this.comment; 449 } 450 451 public boolean hasCommentElement() { 452 return this.comment != null && !this.comment.isEmpty(); 453 } 454 455 public boolean hasComment() { 456 return this.comment != null && !this.comment.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #comment} (Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 461 */ 462 public Schedule setCommentElement(StringType value) { 463 this.comment = value; 464 return this; 465 } 466 467 /** 468 * @return Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 469 */ 470 public String getComment() { 471 return this.comment == null ? null : this.comment.getValue(); 472 } 473 474 /** 475 * @param value Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 476 */ 477 public Schedule setComment(String value) { 478 if (Utilities.noString(value)) 479 this.comment = null; 480 else { 481 if (this.comment == null) 482 this.comment = new StringType(); 483 this.comment.setValue(value); 484 } 485 return this; 486 } 487 488 protected void listChildren(List<Property> children) { 489 super.listChildren(children); 490 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 491 children.add(new Property("active", "boolean", "Whether this schedule record is in active use, or should not be used (such as was entered in error).", 0, 1, active)); 492 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory)); 493 children.add(new Property("serviceType", "CodeableConcept", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 494 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 495 children.add(new Property("actor", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", "The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson.", 0, java.lang.Integer.MAX_VALUE, actor)); 496 children.add(new Property("planningHorizon", "Period", "The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 0, 1, planningHorizon)); 497 children.add(new Property("comment", "string", "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 0, 1, comment)); 498 } 499 500 @Override 501 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 502 switch (_hash) { 503 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 504 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this schedule record is in active use, or should not be used (such as was entered in error).", 0, 1, active); 505 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory); 506 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableConcept", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType); 507 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 508 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", "The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, PractitionerRole, Device, Patient or RelatedPerson.", 0, java.lang.Integer.MAX_VALUE, actor); 509 case -1718507650: /*planningHorizon*/ return new Property("planningHorizon", "Period", "The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 0, 1, planningHorizon); 510 case 950398559: /*comment*/ return new Property("comment", "string", "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 0, 1, comment); 511 default: return super.getNamedProperty(_hash, _name, _checkValid); 512 } 513 514 } 515 516 @Override 517 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 518 switch (hash) { 519 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 520 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 521 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : new Base[] {this.serviceCategory}; // CodeableConcept 522 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 523 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 524 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // Reference 525 case -1718507650: /*planningHorizon*/ return this.planningHorizon == null ? new Base[0] : new Base[] {this.planningHorizon}; // Period 526 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 527 default: return super.getProperty(hash, name, checkValid); 528 } 529 530 } 531 532 @Override 533 public Base setProperty(int hash, String name, Base value) throws FHIRException { 534 switch (hash) { 535 case -1618432855: // identifier 536 this.getIdentifier().add(castToIdentifier(value)); // Identifier 537 return value; 538 case -1422950650: // active 539 this.active = castToBoolean(value); // BooleanType 540 return value; 541 case 1281188563: // serviceCategory 542 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 543 return value; 544 case -1928370289: // serviceType 545 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 546 return value; 547 case -1694759682: // specialty 548 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 549 return value; 550 case 92645877: // actor 551 this.getActor().add(castToReference(value)); // Reference 552 return value; 553 case -1718507650: // planningHorizon 554 this.planningHorizon = castToPeriod(value); // Period 555 return value; 556 case 950398559: // comment 557 this.comment = castToString(value); // StringType 558 return value; 559 default: return super.setProperty(hash, name, value); 560 } 561 562 } 563 564 @Override 565 public Base setProperty(String name, Base value) throws FHIRException { 566 if (name.equals("identifier")) { 567 this.getIdentifier().add(castToIdentifier(value)); 568 } else if (name.equals("active")) { 569 this.active = castToBoolean(value); // BooleanType 570 } else if (name.equals("serviceCategory")) { 571 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 572 } else if (name.equals("serviceType")) { 573 this.getServiceType().add(castToCodeableConcept(value)); 574 } else if (name.equals("specialty")) { 575 this.getSpecialty().add(castToCodeableConcept(value)); 576 } else if (name.equals("actor")) { 577 this.getActor().add(castToReference(value)); 578 } else if (name.equals("planningHorizon")) { 579 this.planningHorizon = castToPeriod(value); // Period 580 } else if (name.equals("comment")) { 581 this.comment = castToString(value); // StringType 582 } else 583 return super.setProperty(name, value); 584 return value; 585 } 586 587 @Override 588 public Base makeProperty(int hash, String name) throws FHIRException { 589 switch (hash) { 590 case -1618432855: return addIdentifier(); 591 case -1422950650: return getActiveElement(); 592 case 1281188563: return getServiceCategory(); 593 case -1928370289: return addServiceType(); 594 case -1694759682: return addSpecialty(); 595 case 92645877: return addActor(); 596 case -1718507650: return getPlanningHorizon(); 597 case 950398559: return getCommentElement(); 598 default: return super.makeProperty(hash, name); 599 } 600 601 } 602 603 @Override 604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 605 switch (hash) { 606 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 607 case -1422950650: /*active*/ return new String[] {"boolean"}; 608 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 609 case -1928370289: /*serviceType*/ return new String[] {"CodeableConcept"}; 610 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 611 case 92645877: /*actor*/ return new String[] {"Reference"}; 612 case -1718507650: /*planningHorizon*/ return new String[] {"Period"}; 613 case 950398559: /*comment*/ return new String[] {"string"}; 614 default: return super.getTypesForProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("identifier")) { 622 return addIdentifier(); 623 } 624 else if (name.equals("active")) { 625 throw new FHIRException("Cannot call addChild on a singleton property Schedule.active"); 626 } 627 else if (name.equals("serviceCategory")) { 628 this.serviceCategory = new CodeableConcept(); 629 return this.serviceCategory; 630 } 631 else if (name.equals("serviceType")) { 632 return addServiceType(); 633 } 634 else if (name.equals("specialty")) { 635 return addSpecialty(); 636 } 637 else if (name.equals("actor")) { 638 return addActor(); 639 } 640 else if (name.equals("planningHorizon")) { 641 this.planningHorizon = new Period(); 642 return this.planningHorizon; 643 } 644 else if (name.equals("comment")) { 645 throw new FHIRException("Cannot call addChild on a singleton property Schedule.comment"); 646 } 647 else 648 return super.addChild(name); 649 } 650 651 public String fhirType() { 652 return "Schedule"; 653 654 } 655 656 public Schedule copy() { 657 Schedule dst = new Schedule(); 658 copyValues(dst); 659 if (identifier != null) { 660 dst.identifier = new ArrayList<Identifier>(); 661 for (Identifier i : identifier) 662 dst.identifier.add(i.copy()); 663 }; 664 dst.active = active == null ? null : active.copy(); 665 dst.serviceCategory = serviceCategory == null ? null : serviceCategory.copy(); 666 if (serviceType != null) { 667 dst.serviceType = new ArrayList<CodeableConcept>(); 668 for (CodeableConcept i : serviceType) 669 dst.serviceType.add(i.copy()); 670 }; 671 if (specialty != null) { 672 dst.specialty = new ArrayList<CodeableConcept>(); 673 for (CodeableConcept i : specialty) 674 dst.specialty.add(i.copy()); 675 }; 676 if (actor != null) { 677 dst.actor = new ArrayList<Reference>(); 678 for (Reference i : actor) 679 dst.actor.add(i.copy()); 680 }; 681 dst.planningHorizon = planningHorizon == null ? null : planningHorizon.copy(); 682 dst.comment = comment == null ? null : comment.copy(); 683 return dst; 684 } 685 686 protected Schedule typedCopy() { 687 return copy(); 688 } 689 690 @Override 691 public boolean equalsDeep(Base other_) { 692 if (!super.equalsDeep(other_)) 693 return false; 694 if (!(other_ instanceof Schedule)) 695 return false; 696 Schedule o = (Schedule) other_; 697 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(serviceCategory, o.serviceCategory, true) 698 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(actor, o.actor, true) 699 && compareDeep(planningHorizon, o.planningHorizon, true) && compareDeep(comment, o.comment, true) 700 ; 701 } 702 703 @Override 704 public boolean equalsShallow(Base other_) { 705 if (!super.equalsShallow(other_)) 706 return false; 707 if (!(other_ instanceof Schedule)) 708 return false; 709 Schedule o = (Schedule) other_; 710 return compareValues(active, o.active, true) && compareValues(comment, o.comment, true); 711 } 712 713 public boolean isEmpty() { 714 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, serviceCategory 715 , serviceType, specialty, actor, planningHorizon, comment); 716 } 717 718 @Override 719 public ResourceType getResourceType() { 720 return ResourceType.Schedule; 721 } 722 723 /** 724 * Search parameter: <b>actor</b> 725 * <p> 726 * Description: <b>The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for</b><br> 727 * Type: <b>reference</b><br> 728 * Path: <b>Schedule.actor</b><br> 729 * </p> 730 */ 731 @SearchParamDefinition(name="actor", path="Schedule.actor", description="The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 732 public static final String SP_ACTOR = "actor"; 733 /** 734 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 735 * <p> 736 * Description: <b>The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for</b><br> 737 * Type: <b>reference</b><br> 738 * Path: <b>Schedule.actor</b><br> 739 * </p> 740 */ 741 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 742 743/** 744 * Constant for fluent queries to be used to add include statements. Specifies 745 * the path value of "<b>Schedule:actor</b>". 746 */ 747 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Schedule:actor").toLocked(); 748 749 /** 750 * Search parameter: <b>date</b> 751 * <p> 752 * Description: <b>Search for Schedule resources that have a period that contains this date specified</b><br> 753 * Type: <b>date</b><br> 754 * Path: <b>Schedule.planningHorizon</b><br> 755 * </p> 756 */ 757 @SearchParamDefinition(name="date", path="Schedule.planningHorizon", description="Search for Schedule resources that have a period that contains this date specified", type="date" ) 758 public static final String SP_DATE = "date"; 759 /** 760 * <b>Fluent Client</b> search parameter constant for <b>date</b> 761 * <p> 762 * Description: <b>Search for Schedule resources that have a period that contains this date specified</b><br> 763 * Type: <b>date</b><br> 764 * Path: <b>Schedule.planningHorizon</b><br> 765 * </p> 766 */ 767 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 768 769 /** 770 * Search parameter: <b>identifier</b> 771 * <p> 772 * Description: <b>A Schedule Identifier</b><br> 773 * Type: <b>token</b><br> 774 * Path: <b>Schedule.identifier</b><br> 775 * </p> 776 */ 777 @SearchParamDefinition(name="identifier", path="Schedule.identifier", description="A Schedule Identifier", type="token" ) 778 public static final String SP_IDENTIFIER = "identifier"; 779 /** 780 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 781 * <p> 782 * Description: <b>A Schedule Identifier</b><br> 783 * Type: <b>token</b><br> 784 * Path: <b>Schedule.identifier</b><br> 785 * </p> 786 */ 787 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 788 789 /** 790 * Search parameter: <b>active</b> 791 * <p> 792 * Description: <b>Is the schedule in active use</b><br> 793 * Type: <b>token</b><br> 794 * Path: <b>Schedule.active</b><br> 795 * </p> 796 */ 797 @SearchParamDefinition(name="active", path="Schedule.active", description="Is the schedule in active use", type="token" ) 798 public static final String SP_ACTIVE = "active"; 799 /** 800 * <b>Fluent Client</b> search parameter constant for <b>active</b> 801 * <p> 802 * Description: <b>Is the schedule in active use</b><br> 803 * Type: <b>token</b><br> 804 * Path: <b>Schedule.active</b><br> 805 * </p> 806 */ 807 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 808 809 /** 810 * Search parameter: <b>type</b> 811 * <p> 812 * Description: <b>The type of appointments that can be booked into associated slot(s)</b><br> 813 * Type: <b>token</b><br> 814 * Path: <b>Schedule.serviceType</b><br> 815 * </p> 816 */ 817 @SearchParamDefinition(name="type", path="Schedule.serviceType", description="The type of appointments that can be booked into associated slot(s)", type="token" ) 818 public static final String SP_TYPE = "type"; 819 /** 820 * <b>Fluent Client</b> search parameter constant for <b>type</b> 821 * <p> 822 * Description: <b>The type of appointments that can be booked into associated slot(s)</b><br> 823 * Type: <b>token</b><br> 824 * Path: <b>Schedule.serviceType</b><br> 825 * </p> 826 */ 827 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 828 829 830}