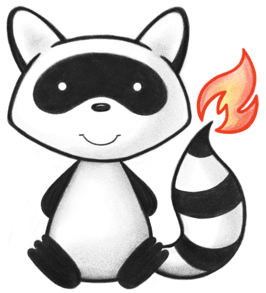
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.dstu3.model.Enumerations.SearchParamType; 042import org.hl7.fhir.dstu3.model.Enumerations.SearchParamTypeEnumFactory; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053/** 054 * A search parameter that defines a named search item that can be used to search/filter on a resource. 055 */ 056@ResourceDef(name="SearchParameter", profile="http://hl7.org/fhir/Profile/SearchParameter") 057@ChildOrder(names={"url", "version", "name", "status", "experimental", "date", "publisher", "contact", "useContext", "jurisdiction", "purpose", "code", "base", "type", "derivedFrom", "description", "expression", "xpath", "xpathUsage", "target", "comparator", "modifier", "chain", "component"}) 058public class SearchParameter extends MetadataResource { 059 060 public enum XPathUsageType { 061 /** 062 * The search parameter is derived directly from the selected nodes based on the type definitions. 063 */ 064 NORMAL, 065 /** 066 * The search parameter is derived by a phonetic transform from the selected nodes. 067 */ 068 PHONETIC, 069 /** 070 * The search parameter is based on a spatial transform of the selected nodes. 071 */ 072 NEARBY, 073 /** 074 * The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle. 075 */ 076 DISTANCE, 077 /** 078 * The interpretation of the xpath statement is unknown (and can't be automated). 079 */ 080 OTHER, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static XPathUsageType fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("normal".equals(codeString)) 089 return NORMAL; 090 if ("phonetic".equals(codeString)) 091 return PHONETIC; 092 if ("nearby".equals(codeString)) 093 return NEARBY; 094 if ("distance".equals(codeString)) 095 return DISTANCE; 096 if ("other".equals(codeString)) 097 return OTHER; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown XPathUsageType code '"+codeString+"'"); 102 } 103 public String toCode() { 104 switch (this) { 105 case NORMAL: return "normal"; 106 case PHONETIC: return "phonetic"; 107 case NEARBY: return "nearby"; 108 case DISTANCE: return "distance"; 109 case OTHER: return "other"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getSystem() { 115 switch (this) { 116 case NORMAL: return "http://hl7.org/fhir/search-xpath-usage"; 117 case PHONETIC: return "http://hl7.org/fhir/search-xpath-usage"; 118 case NEARBY: return "http://hl7.org/fhir/search-xpath-usage"; 119 case DISTANCE: return "http://hl7.org/fhir/search-xpath-usage"; 120 case OTHER: return "http://hl7.org/fhir/search-xpath-usage"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getDefinition() { 126 switch (this) { 127 case NORMAL: return "The search parameter is derived directly from the selected nodes based on the type definitions."; 128 case PHONETIC: return "The search parameter is derived by a phonetic transform from the selected nodes."; 129 case NEARBY: return "The search parameter is based on a spatial transform of the selected nodes."; 130 case DISTANCE: return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 131 case OTHER: return "The interpretation of the xpath statement is unknown (and can't be automated)."; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 public String getDisplay() { 137 switch (this) { 138 case NORMAL: return "Normal"; 139 case PHONETIC: return "Phonetic"; 140 case NEARBY: return "Nearby"; 141 case DISTANCE: return "Distance"; 142 case OTHER: return "Other"; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 } 148 149 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 150 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("normal".equals(codeString)) 155 return XPathUsageType.NORMAL; 156 if ("phonetic".equals(codeString)) 157 return XPathUsageType.PHONETIC; 158 if ("nearby".equals(codeString)) 159 return XPathUsageType.NEARBY; 160 if ("distance".equals(codeString)) 161 return XPathUsageType.DISTANCE; 162 if ("other".equals(codeString)) 163 return XPathUsageType.OTHER; 164 throw new IllegalArgumentException("Unknown XPathUsageType code '"+codeString+"'"); 165 } 166 public Enumeration<XPathUsageType> fromType(PrimitiveType<?> code) throws FHIRException { 167 if (code == null) 168 return null; 169 if (code.isEmpty()) 170 return new Enumeration<XPathUsageType>(this); 171 String codeString = code.asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("normal".equals(codeString)) 175 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL); 176 if ("phonetic".equals(codeString)) 177 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC); 178 if ("nearby".equals(codeString)) 179 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY); 180 if ("distance".equals(codeString)) 181 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE); 182 if ("other".equals(codeString)) 183 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER); 184 throw new FHIRException("Unknown XPathUsageType code '"+codeString+"'"); 185 } 186 public String toCode(XPathUsageType code) { 187 if (code == XPathUsageType.NULL) 188 return null; 189 if (code == XPathUsageType.NORMAL) 190 return "normal"; 191 if (code == XPathUsageType.PHONETIC) 192 return "phonetic"; 193 if (code == XPathUsageType.NEARBY) 194 return "nearby"; 195 if (code == XPathUsageType.DISTANCE) 196 return "distance"; 197 if (code == XPathUsageType.OTHER) 198 return "other"; 199 return "?"; 200 } 201 public String toSystem(XPathUsageType code) { 202 return code.getSystem(); 203 } 204 } 205 206 public enum SearchComparator { 207 /** 208 * the value for the parameter in the resource is equal to the provided value 209 */ 210 EQ, 211 /** 212 * the value for the parameter in the resource is not equal to the provided value 213 */ 214 NE, 215 /** 216 * the value for the parameter in the resource is greater than the provided value 217 */ 218 GT, 219 /** 220 * the value for the parameter in the resource is less than the provided value 221 */ 222 LT, 223 /** 224 * the value for the parameter in the resource is greater or equal to the provided value 225 */ 226 GE, 227 /** 228 * the value for the parameter in the resource is less or equal to the provided value 229 */ 230 LE, 231 /** 232 * the value for the parameter in the resource starts after the provided value 233 */ 234 SA, 235 /** 236 * the value for the parameter in the resource ends before the provided value 237 */ 238 EB, 239 /** 240 * the value for the parameter in the resource is approximately the same to the provided value. 241 */ 242 AP, 243 /** 244 * added to help the parsers with the generic types 245 */ 246 NULL; 247 public static SearchComparator fromCode(String codeString) throws FHIRException { 248 if (codeString == null || "".equals(codeString)) 249 return null; 250 if ("eq".equals(codeString)) 251 return EQ; 252 if ("ne".equals(codeString)) 253 return NE; 254 if ("gt".equals(codeString)) 255 return GT; 256 if ("lt".equals(codeString)) 257 return LT; 258 if ("ge".equals(codeString)) 259 return GE; 260 if ("le".equals(codeString)) 261 return LE; 262 if ("sa".equals(codeString)) 263 return SA; 264 if ("eb".equals(codeString)) 265 return EB; 266 if ("ap".equals(codeString)) 267 return AP; 268 if (Configuration.isAcceptInvalidEnums()) 269 return null; 270 else 271 throw new FHIRException("Unknown SearchComparator code '"+codeString+"'"); 272 } 273 public String toCode() { 274 switch (this) { 275 case EQ: return "eq"; 276 case NE: return "ne"; 277 case GT: return "gt"; 278 case LT: return "lt"; 279 case GE: return "ge"; 280 case LE: return "le"; 281 case SA: return "sa"; 282 case EB: return "eb"; 283 case AP: return "ap"; 284 case NULL: return null; 285 default: return "?"; 286 } 287 } 288 public String getSystem() { 289 switch (this) { 290 case EQ: return "http://hl7.org/fhir/search-comparator"; 291 case NE: return "http://hl7.org/fhir/search-comparator"; 292 case GT: return "http://hl7.org/fhir/search-comparator"; 293 case LT: return "http://hl7.org/fhir/search-comparator"; 294 case GE: return "http://hl7.org/fhir/search-comparator"; 295 case LE: return "http://hl7.org/fhir/search-comparator"; 296 case SA: return "http://hl7.org/fhir/search-comparator"; 297 case EB: return "http://hl7.org/fhir/search-comparator"; 298 case AP: return "http://hl7.org/fhir/search-comparator"; 299 case NULL: return null; 300 default: return "?"; 301 } 302 } 303 public String getDefinition() { 304 switch (this) { 305 case EQ: return "the value for the parameter in the resource is equal to the provided value"; 306 case NE: return "the value for the parameter in the resource is not equal to the provided value"; 307 case GT: return "the value for the parameter in the resource is greater than the provided value"; 308 case LT: return "the value for the parameter in the resource is less than the provided value"; 309 case GE: return "the value for the parameter in the resource is greater or equal to the provided value"; 310 case LE: return "the value for the parameter in the resource is less or equal to the provided value"; 311 case SA: return "the value for the parameter in the resource starts after the provided value"; 312 case EB: return "the value for the parameter in the resource ends before the provided value"; 313 case AP: return "the value for the parameter in the resource is approximately the same to the provided value."; 314 case NULL: return null; 315 default: return "?"; 316 } 317 } 318 public String getDisplay() { 319 switch (this) { 320 case EQ: return "Equals"; 321 case NE: return "Not Equals"; 322 case GT: return "Greater Than"; 323 case LT: return "Less Then"; 324 case GE: return "Greater or Equals"; 325 case LE: return "Less of Equal"; 326 case SA: return "Starts After"; 327 case EB: return "Ends Before"; 328 case AP: return "Approximately"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 } 334 335 public static class SearchComparatorEnumFactory implements EnumFactory<SearchComparator> { 336 public SearchComparator fromCode(String codeString) throws IllegalArgumentException { 337 if (codeString == null || "".equals(codeString)) 338 if (codeString == null || "".equals(codeString)) 339 return null; 340 if ("eq".equals(codeString)) 341 return SearchComparator.EQ; 342 if ("ne".equals(codeString)) 343 return SearchComparator.NE; 344 if ("gt".equals(codeString)) 345 return SearchComparator.GT; 346 if ("lt".equals(codeString)) 347 return SearchComparator.LT; 348 if ("ge".equals(codeString)) 349 return SearchComparator.GE; 350 if ("le".equals(codeString)) 351 return SearchComparator.LE; 352 if ("sa".equals(codeString)) 353 return SearchComparator.SA; 354 if ("eb".equals(codeString)) 355 return SearchComparator.EB; 356 if ("ap".equals(codeString)) 357 return SearchComparator.AP; 358 throw new IllegalArgumentException("Unknown SearchComparator code '"+codeString+"'"); 359 } 360 public Enumeration<SearchComparator> fromType(PrimitiveType<?> code) throws FHIRException { 361 if (code == null) 362 return null; 363 if (code.isEmpty()) 364 return new Enumeration<SearchComparator>(this); 365 String codeString = code.asStringValue(); 366 if (codeString == null || "".equals(codeString)) 367 return null; 368 if ("eq".equals(codeString)) 369 return new Enumeration<SearchComparator>(this, SearchComparator.EQ); 370 if ("ne".equals(codeString)) 371 return new Enumeration<SearchComparator>(this, SearchComparator.NE); 372 if ("gt".equals(codeString)) 373 return new Enumeration<SearchComparator>(this, SearchComparator.GT); 374 if ("lt".equals(codeString)) 375 return new Enumeration<SearchComparator>(this, SearchComparator.LT); 376 if ("ge".equals(codeString)) 377 return new Enumeration<SearchComparator>(this, SearchComparator.GE); 378 if ("le".equals(codeString)) 379 return new Enumeration<SearchComparator>(this, SearchComparator.LE); 380 if ("sa".equals(codeString)) 381 return new Enumeration<SearchComparator>(this, SearchComparator.SA); 382 if ("eb".equals(codeString)) 383 return new Enumeration<SearchComparator>(this, SearchComparator.EB); 384 if ("ap".equals(codeString)) 385 return new Enumeration<SearchComparator>(this, SearchComparator.AP); 386 throw new FHIRException("Unknown SearchComparator code '"+codeString+"'"); 387 } 388 public String toCode(SearchComparator code) { 389 if (code == SearchComparator.NULL) 390 return null; 391 if (code == SearchComparator.EQ) 392 return "eq"; 393 if (code == SearchComparator.NE) 394 return "ne"; 395 if (code == SearchComparator.GT) 396 return "gt"; 397 if (code == SearchComparator.LT) 398 return "lt"; 399 if (code == SearchComparator.GE) 400 return "ge"; 401 if (code == SearchComparator.LE) 402 return "le"; 403 if (code == SearchComparator.SA) 404 return "sa"; 405 if (code == SearchComparator.EB) 406 return "eb"; 407 if (code == SearchComparator.AP) 408 return "ap"; 409 return "?"; 410 } 411 public String toSystem(SearchComparator code) { 412 return code.getSystem(); 413 } 414 } 415 416 public enum SearchModifierCode { 417 /** 418 * The search parameter returns resources that have a value or not. 419 */ 420 MISSING, 421 /** 422 * The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents). 423 */ 424 EXACT, 425 /** 426 * The search parameter returns resources that include the supplied parameter value anywhere within the field being searched. 427 */ 428 CONTAINS, 429 /** 430 * The search parameter returns resources that do not contain a match. 431 */ 432 NOT, 433 /** 434 * The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text. 435 */ 436 TEXT, 437 /** 438 * The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set. 439 */ 440 IN, 441 /** 442 * The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set. 443 */ 444 NOTIN, 445 /** 446 * The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships). 447 */ 448 BELOW, 449 /** 450 * The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships). 451 */ 452 ABOVE, 453 /** 454 * The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.). 455 */ 456 TYPE, 457 /** 458 * added to help the parsers with the generic types 459 */ 460 NULL; 461 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 462 if (codeString == null || "".equals(codeString)) 463 return null; 464 if ("missing".equals(codeString)) 465 return MISSING; 466 if ("exact".equals(codeString)) 467 return EXACT; 468 if ("contains".equals(codeString)) 469 return CONTAINS; 470 if ("not".equals(codeString)) 471 return NOT; 472 if ("text".equals(codeString)) 473 return TEXT; 474 if ("in".equals(codeString)) 475 return IN; 476 if ("not-in".equals(codeString)) 477 return NOTIN; 478 if ("below".equals(codeString)) 479 return BELOW; 480 if ("above".equals(codeString)) 481 return ABOVE; 482 if ("type".equals(codeString)) 483 return TYPE; 484 if (Configuration.isAcceptInvalidEnums()) 485 return null; 486 else 487 throw new FHIRException("Unknown SearchModifierCode code '"+codeString+"'"); 488 } 489 public String toCode() { 490 switch (this) { 491 case MISSING: return "missing"; 492 case EXACT: return "exact"; 493 case CONTAINS: return "contains"; 494 case NOT: return "not"; 495 case TEXT: return "text"; 496 case IN: return "in"; 497 case NOTIN: return "not-in"; 498 case BELOW: return "below"; 499 case ABOVE: return "above"; 500 case TYPE: return "type"; 501 case NULL: return null; 502 default: return "?"; 503 } 504 } 505 public String getSystem() { 506 switch (this) { 507 case MISSING: return "http://hl7.org/fhir/search-modifier-code"; 508 case EXACT: return "http://hl7.org/fhir/search-modifier-code"; 509 case CONTAINS: return "http://hl7.org/fhir/search-modifier-code"; 510 case NOT: return "http://hl7.org/fhir/search-modifier-code"; 511 case TEXT: return "http://hl7.org/fhir/search-modifier-code"; 512 case IN: return "http://hl7.org/fhir/search-modifier-code"; 513 case NOTIN: return "http://hl7.org/fhir/search-modifier-code"; 514 case BELOW: return "http://hl7.org/fhir/search-modifier-code"; 515 case ABOVE: return "http://hl7.org/fhir/search-modifier-code"; 516 case TYPE: return "http://hl7.org/fhir/search-modifier-code"; 517 case NULL: return null; 518 default: return "?"; 519 } 520 } 521 public String getDefinition() { 522 switch (this) { 523 case MISSING: return "The search parameter returns resources that have a value or not."; 524 case EXACT: return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 525 case CONTAINS: return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 526 case NOT: return "The search parameter returns resources that do not contain a match."; 527 case TEXT: return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 528 case IN: return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 529 case NOTIN: return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 530 case BELOW: return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 531 case ABOVE: return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 532 case TYPE: return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 533 case NULL: return null; 534 default: return "?"; 535 } 536 } 537 public String getDisplay() { 538 switch (this) { 539 case MISSING: return "Missing"; 540 case EXACT: return "Exact"; 541 case CONTAINS: return "Contains"; 542 case NOT: return "Not"; 543 case TEXT: return "Text"; 544 case IN: return "In"; 545 case NOTIN: return "Not In"; 546 case BELOW: return "Below"; 547 case ABOVE: return "Above"; 548 case TYPE: return "Type"; 549 case NULL: return null; 550 default: return "?"; 551 } 552 } 553 } 554 555 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 556 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 557 if (codeString == null || "".equals(codeString)) 558 if (codeString == null || "".equals(codeString)) 559 return null; 560 if ("missing".equals(codeString)) 561 return SearchModifierCode.MISSING; 562 if ("exact".equals(codeString)) 563 return SearchModifierCode.EXACT; 564 if ("contains".equals(codeString)) 565 return SearchModifierCode.CONTAINS; 566 if ("not".equals(codeString)) 567 return SearchModifierCode.NOT; 568 if ("text".equals(codeString)) 569 return SearchModifierCode.TEXT; 570 if ("in".equals(codeString)) 571 return SearchModifierCode.IN; 572 if ("not-in".equals(codeString)) 573 return SearchModifierCode.NOTIN; 574 if ("below".equals(codeString)) 575 return SearchModifierCode.BELOW; 576 if ("above".equals(codeString)) 577 return SearchModifierCode.ABOVE; 578 if ("type".equals(codeString)) 579 return SearchModifierCode.TYPE; 580 throw new IllegalArgumentException("Unknown SearchModifierCode code '"+codeString+"'"); 581 } 582 public Enumeration<SearchModifierCode> fromType(PrimitiveType<?> code) throws FHIRException { 583 if (code == null) 584 return null; 585 if (code.isEmpty()) 586 return new Enumeration<SearchModifierCode>(this); 587 String codeString = code.asStringValue(); 588 if (codeString == null || "".equals(codeString)) 589 return null; 590 if ("missing".equals(codeString)) 591 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING); 592 if ("exact".equals(codeString)) 593 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT); 594 if ("contains".equals(codeString)) 595 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS); 596 if ("not".equals(codeString)) 597 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT); 598 if ("text".equals(codeString)) 599 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT); 600 if ("in".equals(codeString)) 601 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN); 602 if ("not-in".equals(codeString)) 603 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN); 604 if ("below".equals(codeString)) 605 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW); 606 if ("above".equals(codeString)) 607 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE); 608 if ("type".equals(codeString)) 609 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE); 610 throw new FHIRException("Unknown SearchModifierCode code '"+codeString+"'"); 611 } 612 public String toCode(SearchModifierCode code) { 613 if (code == SearchModifierCode.NULL) 614 return null; 615 if (code == SearchModifierCode.MISSING) 616 return "missing"; 617 if (code == SearchModifierCode.EXACT) 618 return "exact"; 619 if (code == SearchModifierCode.CONTAINS) 620 return "contains"; 621 if (code == SearchModifierCode.NOT) 622 return "not"; 623 if (code == SearchModifierCode.TEXT) 624 return "text"; 625 if (code == SearchModifierCode.IN) 626 return "in"; 627 if (code == SearchModifierCode.NOTIN) 628 return "not-in"; 629 if (code == SearchModifierCode.BELOW) 630 return "below"; 631 if (code == SearchModifierCode.ABOVE) 632 return "above"; 633 if (code == SearchModifierCode.TYPE) 634 return "type"; 635 return "?"; 636 } 637 public String toSystem(SearchModifierCode code) { 638 return code.getSystem(); 639 } 640 } 641 642 @Block() 643 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 644 /** 645 * The definition of the search parameter that describes this part. 646 */ 647 @Child(name = "definition", type = {SearchParameter.class}, order=1, min=1, max=1, modifier=false, summary=false) 648 @Description(shortDefinition="Defines how the part works", formalDefinition="The definition of the search parameter that describes this part." ) 649 protected Reference definition; 650 651 /** 652 * The actual object that is the target of the reference (The definition of the search parameter that describes this part.) 653 */ 654 protected SearchParameter definitionTarget; 655 656 /** 657 * A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 658 */ 659 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 660 @Description(shortDefinition="Subexpression relative to main expression", formalDefinition="A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression." ) 661 protected StringType expression; 662 663 private static final long serialVersionUID = -1105563614L; 664 665 /** 666 * Constructor 667 */ 668 public SearchParameterComponentComponent() { 669 super(); 670 } 671 672 /** 673 * Constructor 674 */ 675 public SearchParameterComponentComponent(Reference definition, StringType expression) { 676 super(); 677 this.definition = definition; 678 this.expression = expression; 679 } 680 681 /** 682 * @return {@link #definition} (The definition of the search parameter that describes this part.) 683 */ 684 public Reference getDefinition() { 685 if (this.definition == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 688 else if (Configuration.doAutoCreate()) 689 this.definition = new Reference(); // cc 690 return this.definition; 691 } 692 693 public boolean hasDefinition() { 694 return this.definition != null && !this.definition.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #definition} (The definition of the search parameter that describes this part.) 699 */ 700 public SearchParameterComponentComponent setDefinition(Reference value) { 701 this.definition = value; 702 return this; 703 } 704 705 /** 706 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The definition of the search parameter that describes this part.) 707 */ 708 public SearchParameter getDefinitionTarget() { 709 if (this.definitionTarget == null) 710 if (Configuration.errorOnAutoCreate()) 711 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 712 else if (Configuration.doAutoCreate()) 713 this.definitionTarget = new SearchParameter(); // aa 714 return this.definitionTarget; 715 } 716 717 /** 718 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The definition of the search parameter that describes this part.) 719 */ 720 public SearchParameterComponentComponent setDefinitionTarget(SearchParameter value) { 721 this.definitionTarget = value; 722 return this; 723 } 724 725 /** 726 * @return {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 727 */ 728 public StringType getExpressionElement() { 729 if (this.expression == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 732 else if (Configuration.doAutoCreate()) 733 this.expression = new StringType(); // bb 734 return this.expression; 735 } 736 737 public boolean hasExpressionElement() { 738 return this.expression != null && !this.expression.isEmpty(); 739 } 740 741 public boolean hasExpression() { 742 return this.expression != null && !this.expression.isEmpty(); 743 } 744 745 /** 746 * @param value {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 747 */ 748 public SearchParameterComponentComponent setExpressionElement(StringType value) { 749 this.expression = value; 750 return this; 751 } 752 753 /** 754 * @return A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 755 */ 756 public String getExpression() { 757 return this.expression == null ? null : this.expression.getValue(); 758 } 759 760 /** 761 * @param value A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 762 */ 763 public SearchParameterComponentComponent setExpression(String value) { 764 if (this.expression == null) 765 this.expression = new StringType(); 766 this.expression.setValue(value); 767 return this; 768 } 769 770 protected void listChildren(List<Property> children) { 771 super.listChildren(children); 772 children.add(new Property("definition", "Reference(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition)); 773 children.add(new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression)); 774 } 775 776 @Override 777 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 778 switch (_hash) { 779 case -1014418093: /*definition*/ return new Property("definition", "Reference(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition); 780 case -1795452264: /*expression*/ return new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression); 781 default: return super.getNamedProperty(_hash, _name, _checkValid); 782 } 783 784 } 785 786 @Override 787 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 788 switch (hash) { 789 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 790 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 791 default: return super.getProperty(hash, name, checkValid); 792 } 793 794 } 795 796 @Override 797 public Base setProperty(int hash, String name, Base value) throws FHIRException { 798 switch (hash) { 799 case -1014418093: // definition 800 this.definition = castToReference(value); // Reference 801 return value; 802 case -1795452264: // expression 803 this.expression = castToString(value); // StringType 804 return value; 805 default: return super.setProperty(hash, name, value); 806 } 807 808 } 809 810 @Override 811 public Base setProperty(String name, Base value) throws FHIRException { 812 if (name.equals("definition")) { 813 this.definition = castToReference(value); // Reference 814 } else if (name.equals("expression")) { 815 this.expression = castToString(value); // StringType 816 } else 817 return super.setProperty(name, value); 818 return value; 819 } 820 821 @Override 822 public Base makeProperty(int hash, String name) throws FHIRException { 823 switch (hash) { 824 case -1014418093: return getDefinition(); 825 case -1795452264: return getExpressionElement(); 826 default: return super.makeProperty(hash, name); 827 } 828 829 } 830 831 @Override 832 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 833 switch (hash) { 834 case -1014418093: /*definition*/ return new String[] {"Reference"}; 835 case -1795452264: /*expression*/ return new String[] {"string"}; 836 default: return super.getTypesForProperty(hash, name); 837 } 838 839 } 840 841 @Override 842 public Base addChild(String name) throws FHIRException { 843 if (name.equals("definition")) { 844 this.definition = new Reference(); 845 return this.definition; 846 } 847 else if (name.equals("expression")) { 848 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 849 } 850 else 851 return super.addChild(name); 852 } 853 854 public SearchParameterComponentComponent copy() { 855 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 856 copyValues(dst); 857 dst.definition = definition == null ? null : definition.copy(); 858 dst.expression = expression == null ? null : expression.copy(); 859 return dst; 860 } 861 862 @Override 863 public boolean equalsDeep(Base other_) { 864 if (!super.equalsDeep(other_)) 865 return false; 866 if (!(other_ instanceof SearchParameterComponentComponent)) 867 return false; 868 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 869 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true) 870 ; 871 } 872 873 @Override 874 public boolean equalsShallow(Base other_) { 875 if (!super.equalsShallow(other_)) 876 return false; 877 if (!(other_ instanceof SearchParameterComponentComponent)) 878 return false; 879 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 880 return compareValues(expression, o.expression, true); 881 } 882 883 public boolean isEmpty() { 884 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 885 } 886 887 public String fhirType() { 888 return "SearchParameter.component"; 889 890 } 891 892 } 893 894 /** 895 * Explaination of why this search parameter is needed and why it has been designed as it has. 896 */ 897 @Child(name = "purpose", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 898 @Description(shortDefinition="Why this search parameter is defined", formalDefinition="Explaination of why this search parameter is needed and why it has been designed as it has." ) 899 protected MarkdownType purpose; 900 901 /** 902 * The code used in the URL or the parameter name in a parameters resource for this search parameter. 903 */ 904 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 905 @Description(shortDefinition="Code used in URL", formalDefinition="The code used in the URL or the parameter name in a parameters resource for this search parameter." ) 906 protected CodeType code; 907 908 /** 909 * The base resource type(s) that this search parameter can be used against. 910 */ 911 @Child(name = "base", type = {CodeType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 912 @Description(shortDefinition="The resource type(s) this search parameter applies to", formalDefinition="The base resource type(s) that this search parameter can be used against." ) 913 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 914 protected List<CodeType> base; 915 916 /** 917 * The type of value a search parameter refers to, and how the content is interpreted. 918 */ 919 @Child(name = "type", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 920 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri", formalDefinition="The type of value a search parameter refers to, and how the content is interpreted." ) 921 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 922 protected Enumeration<SearchParamType> type; 923 924 /** 925 * Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 926 */ 927 @Child(name = "derivedFrom", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=false) 928 @Description(shortDefinition="Original Definition for the search parameter", formalDefinition="Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter." ) 929 protected UriType derivedFrom; 930 931 /** 932 * A FHIRPath expression that returns a set of elements for the search parameter. 933 */ 934 @Child(name = "expression", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 935 @Description(shortDefinition="FHIRPath expression that extracts the values", formalDefinition="A FHIRPath expression that returns a set of elements for the search parameter." ) 936 protected StringType expression; 937 938 /** 939 * An XPath expression that returns a set of elements for the search parameter. 940 */ 941 @Child(name = "xpath", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 942 @Description(shortDefinition="XPath that extracts the values", formalDefinition="An XPath expression that returns a set of elements for the search parameter." ) 943 protected StringType xpath; 944 945 /** 946 * How the search parameter relates to the set of elements returned by evaluating the xpath query. 947 */ 948 @Child(name = "xpathUsage", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="normal | phonetic | nearby | distance | other", formalDefinition="How the search parameter relates to the set of elements returned by evaluating the xpath query." ) 950 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-xpath-usage") 951 protected Enumeration<XPathUsageType> xpathUsage; 952 953 /** 954 * Types of resource (if a resource is referenced). 955 */ 956 @Child(name = "target", type = {CodeType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 957 @Description(shortDefinition="Types of resource (if a resource reference)", formalDefinition="Types of resource (if a resource is referenced)." ) 958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 959 protected List<CodeType> target; 960 961 /** 962 * Comparators supported for the search parameter. 963 */ 964 @Child(name = "comparator", type = {CodeType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 965 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators supported for the search parameter." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 967 protected List<Enumeration<SearchComparator>> comparator; 968 969 /** 970 * A modifier supported for the search parameter. 971 */ 972 @Child(name = "modifier", type = {CodeType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 973 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type", formalDefinition="A modifier supported for the search parameter." ) 974 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 975 protected List<Enumeration<SearchModifierCode>> modifier; 976 977 /** 978 * Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type. 979 */ 980 @Child(name = "chain", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 981 @Description(shortDefinition="Chained names supported", formalDefinition="Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type." ) 982 protected List<StringType> chain; 983 984 /** 985 * Used to define the parts of a composite search parameter. 986 */ 987 @Child(name = "component", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 988 @Description(shortDefinition="For Composite resources to define the parts", formalDefinition="Used to define the parts of a composite search parameter." ) 989 protected List<SearchParameterComponentComponent> component; 990 991 private static final long serialVersionUID = -769368159L; 992 993 /** 994 * Constructor 995 */ 996 public SearchParameter() { 997 super(); 998 } 999 1000 /** 1001 * Constructor 1002 */ 1003 public SearchParameter(UriType url, StringType name, Enumeration<PublicationStatus> status, CodeType code, Enumeration<SearchParamType> type, MarkdownType description) { 1004 super(); 1005 this.url = url; 1006 this.name = name; 1007 this.status = status; 1008 this.code = code; 1009 this.type = type; 1010 this.description = description; 1011 } 1012 1013 /** 1014 * @return {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1015 */ 1016 public UriType getUrlElement() { 1017 if (this.url == null) 1018 if (Configuration.errorOnAutoCreate()) 1019 throw new Error("Attempt to auto-create SearchParameter.url"); 1020 else if (Configuration.doAutoCreate()) 1021 this.url = new UriType(); // bb 1022 return this.url; 1023 } 1024 1025 public boolean hasUrlElement() { 1026 return this.url != null && !this.url.isEmpty(); 1027 } 1028 1029 public boolean hasUrl() { 1030 return this.url != null && !this.url.isEmpty(); 1031 } 1032 1033 /** 1034 * @param value {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1035 */ 1036 public SearchParameter setUrlElement(UriType value) { 1037 this.url = value; 1038 return this; 1039 } 1040 1041 /** 1042 * @return An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions). 1043 */ 1044 public String getUrl() { 1045 return this.url == null ? null : this.url.getValue(); 1046 } 1047 1048 /** 1049 * @param value An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions). 1050 */ 1051 public SearchParameter setUrl(String value) { 1052 if (this.url == null) 1053 this.url = new UriType(); 1054 this.url.setValue(value); 1055 return this; 1056 } 1057 1058 /** 1059 * @return {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1060 */ 1061 public StringType getVersionElement() { 1062 if (this.version == null) 1063 if (Configuration.errorOnAutoCreate()) 1064 throw new Error("Attempt to auto-create SearchParameter.version"); 1065 else if (Configuration.doAutoCreate()) 1066 this.version = new StringType(); // bb 1067 return this.version; 1068 } 1069 1070 public boolean hasVersionElement() { 1071 return this.version != null && !this.version.isEmpty(); 1072 } 1073 1074 public boolean hasVersion() { 1075 return this.version != null && !this.version.isEmpty(); 1076 } 1077 1078 /** 1079 * @param value {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1080 */ 1081 public SearchParameter setVersionElement(StringType value) { 1082 this.version = value; 1083 return this; 1084 } 1085 1086 /** 1087 * @return The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1088 */ 1089 public String getVersion() { 1090 return this.version == null ? null : this.version.getValue(); 1091 } 1092 1093 /** 1094 * @param value The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1095 */ 1096 public SearchParameter setVersion(String value) { 1097 if (Utilities.noString(value)) 1098 this.version = null; 1099 else { 1100 if (this.version == null) 1101 this.version = new StringType(); 1102 this.version.setValue(value); 1103 } 1104 return this; 1105 } 1106 1107 /** 1108 * @return {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1109 */ 1110 public StringType getNameElement() { 1111 if (this.name == null) 1112 if (Configuration.errorOnAutoCreate()) 1113 throw new Error("Attempt to auto-create SearchParameter.name"); 1114 else if (Configuration.doAutoCreate()) 1115 this.name = new StringType(); // bb 1116 return this.name; 1117 } 1118 1119 public boolean hasNameElement() { 1120 return this.name != null && !this.name.isEmpty(); 1121 } 1122 1123 public boolean hasName() { 1124 return this.name != null && !this.name.isEmpty(); 1125 } 1126 1127 /** 1128 * @param value {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1129 */ 1130 public SearchParameter setNameElement(StringType value) { 1131 this.name = value; 1132 return this; 1133 } 1134 1135 /** 1136 * @return A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1137 */ 1138 public String getName() { 1139 return this.name == null ? null : this.name.getValue(); 1140 } 1141 1142 /** 1143 * @param value A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1144 */ 1145 public SearchParameter setName(String value) { 1146 if (this.name == null) 1147 this.name = new StringType(); 1148 this.name.setValue(value); 1149 return this; 1150 } 1151 1152 /** 1153 * @return {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1154 */ 1155 public Enumeration<PublicationStatus> getStatusElement() { 1156 if (this.status == null) 1157 if (Configuration.errorOnAutoCreate()) 1158 throw new Error("Attempt to auto-create SearchParameter.status"); 1159 else if (Configuration.doAutoCreate()) 1160 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1161 return this.status; 1162 } 1163 1164 public boolean hasStatusElement() { 1165 return this.status != null && !this.status.isEmpty(); 1166 } 1167 1168 public boolean hasStatus() { 1169 return this.status != null && !this.status.isEmpty(); 1170 } 1171 1172 /** 1173 * @param value {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1174 */ 1175 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1176 this.status = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return The status of this search parameter. Enables tracking the life-cycle of the content. 1182 */ 1183 public PublicationStatus getStatus() { 1184 return this.status == null ? null : this.status.getValue(); 1185 } 1186 1187 /** 1188 * @param value The status of this search parameter. Enables tracking the life-cycle of the content. 1189 */ 1190 public SearchParameter setStatus(PublicationStatus value) { 1191 if (this.status == null) 1192 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1193 this.status.setValue(value); 1194 return this; 1195 } 1196 1197 /** 1198 * @return {@link #experimental} (A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1199 */ 1200 public BooleanType getExperimentalElement() { 1201 if (this.experimental == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1204 else if (Configuration.doAutoCreate()) 1205 this.experimental = new BooleanType(); // bb 1206 return this.experimental; 1207 } 1208 1209 public boolean hasExperimentalElement() { 1210 return this.experimental != null && !this.experimental.isEmpty(); 1211 } 1212 1213 public boolean hasExperimental() { 1214 return this.experimental != null && !this.experimental.isEmpty(); 1215 } 1216 1217 /** 1218 * @param value {@link #experimental} (A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1219 */ 1220 public SearchParameter setExperimentalElement(BooleanType value) { 1221 this.experimental = value; 1222 return this; 1223 } 1224 1225 /** 1226 * @return A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1227 */ 1228 public boolean getExperimental() { 1229 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1230 } 1231 1232 /** 1233 * @param value A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1234 */ 1235 public SearchParameter setExperimental(boolean value) { 1236 if (this.experimental == null) 1237 this.experimental = new BooleanType(); 1238 this.experimental.setValue(value); 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #date} (The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1244 */ 1245 public DateTimeType getDateElement() { 1246 if (this.date == null) 1247 if (Configuration.errorOnAutoCreate()) 1248 throw new Error("Attempt to auto-create SearchParameter.date"); 1249 else if (Configuration.doAutoCreate()) 1250 this.date = new DateTimeType(); // bb 1251 return this.date; 1252 } 1253 1254 public boolean hasDateElement() { 1255 return this.date != null && !this.date.isEmpty(); 1256 } 1257 1258 public boolean hasDate() { 1259 return this.date != null && !this.date.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #date} (The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1264 */ 1265 public SearchParameter setDateElement(DateTimeType value) { 1266 this.date = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1272 */ 1273 public Date getDate() { 1274 return this.date == null ? null : this.date.getValue(); 1275 } 1276 1277 /** 1278 * @param value The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1279 */ 1280 public SearchParameter setDate(Date value) { 1281 if (value == null) 1282 this.date = null; 1283 else { 1284 if (this.date == null) 1285 this.date = new DateTimeType(); 1286 this.date.setValue(value); 1287 } 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #publisher} (The name of the individual or organization that published the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1293 */ 1294 public StringType getPublisherElement() { 1295 if (this.publisher == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1298 else if (Configuration.doAutoCreate()) 1299 this.publisher = new StringType(); // bb 1300 return this.publisher; 1301 } 1302 1303 public boolean hasPublisherElement() { 1304 return this.publisher != null && !this.publisher.isEmpty(); 1305 } 1306 1307 public boolean hasPublisher() { 1308 return this.publisher != null && !this.publisher.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #publisher} (The name of the individual or organization that published the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1313 */ 1314 public SearchParameter setPublisherElement(StringType value) { 1315 this.publisher = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return The name of the individual or organization that published the search parameter. 1321 */ 1322 public String getPublisher() { 1323 return this.publisher == null ? null : this.publisher.getValue(); 1324 } 1325 1326 /** 1327 * @param value The name of the individual or organization that published the search parameter. 1328 */ 1329 public SearchParameter setPublisher(String value) { 1330 if (Utilities.noString(value)) 1331 this.publisher = null; 1332 else { 1333 if (this.publisher == null) 1334 this.publisher = new StringType(); 1335 this.publisher.setValue(value); 1336 } 1337 return this; 1338 } 1339 1340 /** 1341 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1342 */ 1343 public List<ContactDetail> getContact() { 1344 if (this.contact == null) 1345 this.contact = new ArrayList<ContactDetail>(); 1346 return this.contact; 1347 } 1348 1349 /** 1350 * @return Returns a reference to <code>this</code> for easy method chaining 1351 */ 1352 public SearchParameter setContact(List<ContactDetail> theContact) { 1353 this.contact = theContact; 1354 return this; 1355 } 1356 1357 public boolean hasContact() { 1358 if (this.contact == null) 1359 return false; 1360 for (ContactDetail item : this.contact) 1361 if (!item.isEmpty()) 1362 return true; 1363 return false; 1364 } 1365 1366 public ContactDetail addContact() { //3 1367 ContactDetail t = new ContactDetail(); 1368 if (this.contact == null) 1369 this.contact = new ArrayList<ContactDetail>(); 1370 this.contact.add(t); 1371 return t; 1372 } 1373 1374 public SearchParameter addContact(ContactDetail t) { //3 1375 if (t == null) 1376 return this; 1377 if (this.contact == null) 1378 this.contact = new ArrayList<ContactDetail>(); 1379 this.contact.add(t); 1380 return this; 1381 } 1382 1383 /** 1384 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1385 */ 1386 public ContactDetail getContactFirstRep() { 1387 if (getContact().isEmpty()) { 1388 addContact(); 1389 } 1390 return getContact().get(0); 1391 } 1392 1393 /** 1394 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.) 1395 */ 1396 public List<UsageContext> getUseContext() { 1397 if (this.useContext == null) 1398 this.useContext = new ArrayList<UsageContext>(); 1399 return this.useContext; 1400 } 1401 1402 /** 1403 * @return Returns a reference to <code>this</code> for easy method chaining 1404 */ 1405 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1406 this.useContext = theUseContext; 1407 return this; 1408 } 1409 1410 public boolean hasUseContext() { 1411 if (this.useContext == null) 1412 return false; 1413 for (UsageContext item : this.useContext) 1414 if (!item.isEmpty()) 1415 return true; 1416 return false; 1417 } 1418 1419 public UsageContext addUseContext() { //3 1420 UsageContext t = new UsageContext(); 1421 if (this.useContext == null) 1422 this.useContext = new ArrayList<UsageContext>(); 1423 this.useContext.add(t); 1424 return t; 1425 } 1426 1427 public SearchParameter addUseContext(UsageContext t) { //3 1428 if (t == null) 1429 return this; 1430 if (this.useContext == null) 1431 this.useContext = new ArrayList<UsageContext>(); 1432 this.useContext.add(t); 1433 return this; 1434 } 1435 1436 /** 1437 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1438 */ 1439 public UsageContext getUseContextFirstRep() { 1440 if (getUseContext().isEmpty()) { 1441 addUseContext(); 1442 } 1443 return getUseContext().get(0); 1444 } 1445 1446 /** 1447 * @return {@link #jurisdiction} (A legal or geographic region in which the search parameter is intended to be used.) 1448 */ 1449 public List<CodeableConcept> getJurisdiction() { 1450 if (this.jurisdiction == null) 1451 this.jurisdiction = new ArrayList<CodeableConcept>(); 1452 return this.jurisdiction; 1453 } 1454 1455 /** 1456 * @return Returns a reference to <code>this</code> for easy method chaining 1457 */ 1458 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 1459 this.jurisdiction = theJurisdiction; 1460 return this; 1461 } 1462 1463 public boolean hasJurisdiction() { 1464 if (this.jurisdiction == null) 1465 return false; 1466 for (CodeableConcept item : this.jurisdiction) 1467 if (!item.isEmpty()) 1468 return true; 1469 return false; 1470 } 1471 1472 public CodeableConcept addJurisdiction() { //3 1473 CodeableConcept t = new CodeableConcept(); 1474 if (this.jurisdiction == null) 1475 this.jurisdiction = new ArrayList<CodeableConcept>(); 1476 this.jurisdiction.add(t); 1477 return t; 1478 } 1479 1480 public SearchParameter addJurisdiction(CodeableConcept t) { //3 1481 if (t == null) 1482 return this; 1483 if (this.jurisdiction == null) 1484 this.jurisdiction = new ArrayList<CodeableConcept>(); 1485 this.jurisdiction.add(t); 1486 return this; 1487 } 1488 1489 /** 1490 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1491 */ 1492 public CodeableConcept getJurisdictionFirstRep() { 1493 if (getJurisdiction().isEmpty()) { 1494 addJurisdiction(); 1495 } 1496 return getJurisdiction().get(0); 1497 } 1498 1499 /** 1500 * @return {@link #purpose} (Explaination of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1501 */ 1502 public MarkdownType getPurposeElement() { 1503 if (this.purpose == null) 1504 if (Configuration.errorOnAutoCreate()) 1505 throw new Error("Attempt to auto-create SearchParameter.purpose"); 1506 else if (Configuration.doAutoCreate()) 1507 this.purpose = new MarkdownType(); // bb 1508 return this.purpose; 1509 } 1510 1511 public boolean hasPurposeElement() { 1512 return this.purpose != null && !this.purpose.isEmpty(); 1513 } 1514 1515 public boolean hasPurpose() { 1516 return this.purpose != null && !this.purpose.isEmpty(); 1517 } 1518 1519 /** 1520 * @param value {@link #purpose} (Explaination of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1521 */ 1522 public SearchParameter setPurposeElement(MarkdownType value) { 1523 this.purpose = value; 1524 return this; 1525 } 1526 1527 /** 1528 * @return Explaination of why this search parameter is needed and why it has been designed as it has. 1529 */ 1530 public String getPurpose() { 1531 return this.purpose == null ? null : this.purpose.getValue(); 1532 } 1533 1534 /** 1535 * @param value Explaination of why this search parameter is needed and why it has been designed as it has. 1536 */ 1537 public SearchParameter setPurpose(String value) { 1538 if (value == null) 1539 this.purpose = null; 1540 else { 1541 if (this.purpose == null) 1542 this.purpose = new MarkdownType(); 1543 this.purpose.setValue(value); 1544 } 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #code} (The code used in the URL or the parameter name in a parameters resource for this search parameter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1550 */ 1551 public CodeType getCodeElement() { 1552 if (this.code == null) 1553 if (Configuration.errorOnAutoCreate()) 1554 throw new Error("Attempt to auto-create SearchParameter.code"); 1555 else if (Configuration.doAutoCreate()) 1556 this.code = new CodeType(); // bb 1557 return this.code; 1558 } 1559 1560 public boolean hasCodeElement() { 1561 return this.code != null && !this.code.isEmpty(); 1562 } 1563 1564 public boolean hasCode() { 1565 return this.code != null && !this.code.isEmpty(); 1566 } 1567 1568 /** 1569 * @param value {@link #code} (The code used in the URL or the parameter name in a parameters resource for this search parameter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1570 */ 1571 public SearchParameter setCodeElement(CodeType value) { 1572 this.code = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return The code used in the URL or the parameter name in a parameters resource for this search parameter. 1578 */ 1579 public String getCode() { 1580 return this.code == null ? null : this.code.getValue(); 1581 } 1582 1583 /** 1584 * @param value The code used in the URL or the parameter name in a parameters resource for this search parameter. 1585 */ 1586 public SearchParameter setCode(String value) { 1587 if (this.code == null) 1588 this.code = new CodeType(); 1589 this.code.setValue(value); 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1595 */ 1596 public List<CodeType> getBase() { 1597 if (this.base == null) 1598 this.base = new ArrayList<CodeType>(); 1599 return this.base; 1600 } 1601 1602 /** 1603 * @return Returns a reference to <code>this</code> for easy method chaining 1604 */ 1605 public SearchParameter setBase(List<CodeType> theBase) { 1606 this.base = theBase; 1607 return this; 1608 } 1609 1610 public boolean hasBase() { 1611 if (this.base == null) 1612 return false; 1613 for (CodeType item : this.base) 1614 if (!item.isEmpty()) 1615 return true; 1616 return false; 1617 } 1618 1619 /** 1620 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1621 */ 1622 public CodeType addBaseElement() {//2 1623 CodeType t = new CodeType(); 1624 if (this.base == null) 1625 this.base = new ArrayList<CodeType>(); 1626 this.base.add(t); 1627 return t; 1628 } 1629 1630 /** 1631 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1632 */ 1633 public SearchParameter addBase(String value) { //1 1634 CodeType t = new CodeType(); 1635 t.setValue(value); 1636 if (this.base == null) 1637 this.base = new ArrayList<CodeType>(); 1638 this.base.add(t); 1639 return this; 1640 } 1641 1642 /** 1643 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1644 */ 1645 public boolean hasBase(String value) { 1646 if (this.base == null) 1647 return false; 1648 for (CodeType v : this.base) 1649 if (v.getValue().equals(value)) // code 1650 return true; 1651 return false; 1652 } 1653 1654 /** 1655 * @return {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1656 */ 1657 public Enumeration<SearchParamType> getTypeElement() { 1658 if (this.type == null) 1659 if (Configuration.errorOnAutoCreate()) 1660 throw new Error("Attempt to auto-create SearchParameter.type"); 1661 else if (Configuration.doAutoCreate()) 1662 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1663 return this.type; 1664 } 1665 1666 public boolean hasTypeElement() { 1667 return this.type != null && !this.type.isEmpty(); 1668 } 1669 1670 public boolean hasType() { 1671 return this.type != null && !this.type.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1676 */ 1677 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1678 this.type = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return The type of value a search parameter refers to, and how the content is interpreted. 1684 */ 1685 public SearchParamType getType() { 1686 return this.type == null ? null : this.type.getValue(); 1687 } 1688 1689 /** 1690 * @param value The type of value a search parameter refers to, and how the content is interpreted. 1691 */ 1692 public SearchParameter setType(SearchParamType value) { 1693 if (this.type == null) 1694 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1695 this.type.setValue(value); 1696 return this; 1697 } 1698 1699 /** 1700 * @return {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 1701 */ 1702 public UriType getDerivedFromElement() { 1703 if (this.derivedFrom == null) 1704 if (Configuration.errorOnAutoCreate()) 1705 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 1706 else if (Configuration.doAutoCreate()) 1707 this.derivedFrom = new UriType(); // bb 1708 return this.derivedFrom; 1709 } 1710 1711 public boolean hasDerivedFromElement() { 1712 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1713 } 1714 1715 public boolean hasDerivedFrom() { 1716 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1717 } 1718 1719 /** 1720 * @param value {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 1721 */ 1722 public SearchParameter setDerivedFromElement(UriType value) { 1723 this.derivedFrom = value; 1724 return this; 1725 } 1726 1727 /** 1728 * @return Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1729 */ 1730 public String getDerivedFrom() { 1731 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 1732 } 1733 1734 /** 1735 * @param value Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1736 */ 1737 public SearchParameter setDerivedFrom(String value) { 1738 if (Utilities.noString(value)) 1739 this.derivedFrom = null; 1740 else { 1741 if (this.derivedFrom == null) 1742 this.derivedFrom = new UriType(); 1743 this.derivedFrom.setValue(value); 1744 } 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #description} (A free text natural language description of the search parameter from a consumer's perspective. and how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1750 */ 1751 public MarkdownType getDescriptionElement() { 1752 if (this.description == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create SearchParameter.description"); 1755 else if (Configuration.doAutoCreate()) 1756 this.description = new MarkdownType(); // bb 1757 return this.description; 1758 } 1759 1760 public boolean hasDescriptionElement() { 1761 return this.description != null && !this.description.isEmpty(); 1762 } 1763 1764 public boolean hasDescription() { 1765 return this.description != null && !this.description.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #description} (A free text natural language description of the search parameter from a consumer's perspective. and how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1770 */ 1771 public SearchParameter setDescriptionElement(MarkdownType value) { 1772 this.description = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return A free text natural language description of the search parameter from a consumer's perspective. and how it used. 1778 */ 1779 public String getDescription() { 1780 return this.description == null ? null : this.description.getValue(); 1781 } 1782 1783 /** 1784 * @param value A free text natural language description of the search parameter from a consumer's perspective. and how it used. 1785 */ 1786 public SearchParameter setDescription(String value) { 1787 if (this.description == null) 1788 this.description = new MarkdownType(); 1789 this.description.setValue(value); 1790 return this; 1791 } 1792 1793 /** 1794 * @return {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1795 */ 1796 public StringType getExpressionElement() { 1797 if (this.expression == null) 1798 if (Configuration.errorOnAutoCreate()) 1799 throw new Error("Attempt to auto-create SearchParameter.expression"); 1800 else if (Configuration.doAutoCreate()) 1801 this.expression = new StringType(); // bb 1802 return this.expression; 1803 } 1804 1805 public boolean hasExpressionElement() { 1806 return this.expression != null && !this.expression.isEmpty(); 1807 } 1808 1809 public boolean hasExpression() { 1810 return this.expression != null && !this.expression.isEmpty(); 1811 } 1812 1813 /** 1814 * @param value {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1815 */ 1816 public SearchParameter setExpressionElement(StringType value) { 1817 this.expression = value; 1818 return this; 1819 } 1820 1821 /** 1822 * @return A FHIRPath expression that returns a set of elements for the search parameter. 1823 */ 1824 public String getExpression() { 1825 return this.expression == null ? null : this.expression.getValue(); 1826 } 1827 1828 /** 1829 * @param value A FHIRPath expression that returns a set of elements for the search parameter. 1830 */ 1831 public SearchParameter setExpression(String value) { 1832 if (Utilities.noString(value)) 1833 this.expression = null; 1834 else { 1835 if (this.expression == null) 1836 this.expression = new StringType(); 1837 this.expression.setValue(value); 1838 } 1839 return this; 1840 } 1841 1842 /** 1843 * @return {@link #xpath} (An XPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 1844 */ 1845 public StringType getXpathElement() { 1846 if (this.xpath == null) 1847 if (Configuration.errorOnAutoCreate()) 1848 throw new Error("Attempt to auto-create SearchParameter.xpath"); 1849 else if (Configuration.doAutoCreate()) 1850 this.xpath = new StringType(); // bb 1851 return this.xpath; 1852 } 1853 1854 public boolean hasXpathElement() { 1855 return this.xpath != null && !this.xpath.isEmpty(); 1856 } 1857 1858 public boolean hasXpath() { 1859 return this.xpath != null && !this.xpath.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #xpath} (An XPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 1864 */ 1865 public SearchParameter setXpathElement(StringType value) { 1866 this.xpath = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return An XPath expression that returns a set of elements for the search parameter. 1872 */ 1873 public String getXpath() { 1874 return this.xpath == null ? null : this.xpath.getValue(); 1875 } 1876 1877 /** 1878 * @param value An XPath expression that returns a set of elements for the search parameter. 1879 */ 1880 public SearchParameter setXpath(String value) { 1881 if (Utilities.noString(value)) 1882 this.xpath = null; 1883 else { 1884 if (this.xpath == null) 1885 this.xpath = new StringType(); 1886 this.xpath.setValue(value); 1887 } 1888 return this; 1889 } 1890 1891 /** 1892 * @return {@link #xpathUsage} (How the search parameter relates to the set of elements returned by evaluating the xpath query.). This is the underlying object with id, value and extensions. The accessor "getXpathUsage" gives direct access to the value 1893 */ 1894 public Enumeration<XPathUsageType> getXpathUsageElement() { 1895 if (this.xpathUsage == null) 1896 if (Configuration.errorOnAutoCreate()) 1897 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 1898 else if (Configuration.doAutoCreate()) 1899 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 1900 return this.xpathUsage; 1901 } 1902 1903 public boolean hasXpathUsageElement() { 1904 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1905 } 1906 1907 public boolean hasXpathUsage() { 1908 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1909 } 1910 1911 /** 1912 * @param value {@link #xpathUsage} (How the search parameter relates to the set of elements returned by evaluating the xpath query.). This is the underlying object with id, value and extensions. The accessor "getXpathUsage" gives direct access to the value 1913 */ 1914 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 1915 this.xpathUsage = value; 1916 return this; 1917 } 1918 1919 /** 1920 * @return How the search parameter relates to the set of elements returned by evaluating the xpath query. 1921 */ 1922 public XPathUsageType getXpathUsage() { 1923 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 1924 } 1925 1926 /** 1927 * @param value How the search parameter relates to the set of elements returned by evaluating the xpath query. 1928 */ 1929 public SearchParameter setXpathUsage(XPathUsageType value) { 1930 if (value == null) 1931 this.xpathUsage = null; 1932 else { 1933 if (this.xpathUsage == null) 1934 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 1935 this.xpathUsage.setValue(value); 1936 } 1937 return this; 1938 } 1939 1940 /** 1941 * @return {@link #target} (Types of resource (if a resource is referenced).) 1942 */ 1943 public List<CodeType> getTarget() { 1944 if (this.target == null) 1945 this.target = new ArrayList<CodeType>(); 1946 return this.target; 1947 } 1948 1949 /** 1950 * @return Returns a reference to <code>this</code> for easy method chaining 1951 */ 1952 public SearchParameter setTarget(List<CodeType> theTarget) { 1953 this.target = theTarget; 1954 return this; 1955 } 1956 1957 public boolean hasTarget() { 1958 if (this.target == null) 1959 return false; 1960 for (CodeType item : this.target) 1961 if (!item.isEmpty()) 1962 return true; 1963 return false; 1964 } 1965 1966 /** 1967 * @return {@link #target} (Types of resource (if a resource is referenced).) 1968 */ 1969 public CodeType addTargetElement() {//2 1970 CodeType t = new CodeType(); 1971 if (this.target == null) 1972 this.target = new ArrayList<CodeType>(); 1973 this.target.add(t); 1974 return t; 1975 } 1976 1977 /** 1978 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1979 */ 1980 public SearchParameter addTarget(String value) { //1 1981 CodeType t = new CodeType(); 1982 t.setValue(value); 1983 if (this.target == null) 1984 this.target = new ArrayList<CodeType>(); 1985 this.target.add(t); 1986 return this; 1987 } 1988 1989 /** 1990 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1991 */ 1992 public boolean hasTarget(String value) { 1993 if (this.target == null) 1994 return false; 1995 for (CodeType v : this.target) 1996 if (v.getValue().equals(value)) // code 1997 return true; 1998 return false; 1999 } 2000 2001 /** 2002 * @return {@link #comparator} (Comparators supported for the search parameter.) 2003 */ 2004 public List<Enumeration<SearchComparator>> getComparator() { 2005 if (this.comparator == null) 2006 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2007 return this.comparator; 2008 } 2009 2010 /** 2011 * @return Returns a reference to <code>this</code> for easy method chaining 2012 */ 2013 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2014 this.comparator = theComparator; 2015 return this; 2016 } 2017 2018 public boolean hasComparator() { 2019 if (this.comparator == null) 2020 return false; 2021 for (Enumeration<SearchComparator> item : this.comparator) 2022 if (!item.isEmpty()) 2023 return true; 2024 return false; 2025 } 2026 2027 /** 2028 * @return {@link #comparator} (Comparators supported for the search parameter.) 2029 */ 2030 public Enumeration<SearchComparator> addComparatorElement() {//2 2031 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2032 if (this.comparator == null) 2033 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2034 this.comparator.add(t); 2035 return t; 2036 } 2037 2038 /** 2039 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2040 */ 2041 public SearchParameter addComparator(SearchComparator value) { //1 2042 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2043 t.setValue(value); 2044 if (this.comparator == null) 2045 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2046 this.comparator.add(t); 2047 return this; 2048 } 2049 2050 /** 2051 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2052 */ 2053 public boolean hasComparator(SearchComparator value) { 2054 if (this.comparator == null) 2055 return false; 2056 for (Enumeration<SearchComparator> v : this.comparator) 2057 if (v.getValue().equals(value)) // code 2058 return true; 2059 return false; 2060 } 2061 2062 /** 2063 * @return {@link #modifier} (A modifier supported for the search parameter.) 2064 */ 2065 public List<Enumeration<SearchModifierCode>> getModifier() { 2066 if (this.modifier == null) 2067 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2068 return this.modifier; 2069 } 2070 2071 /** 2072 * @return Returns a reference to <code>this</code> for easy method chaining 2073 */ 2074 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2075 this.modifier = theModifier; 2076 return this; 2077 } 2078 2079 public boolean hasModifier() { 2080 if (this.modifier == null) 2081 return false; 2082 for (Enumeration<SearchModifierCode> item : this.modifier) 2083 if (!item.isEmpty()) 2084 return true; 2085 return false; 2086 } 2087 2088 /** 2089 * @return {@link #modifier} (A modifier supported for the search parameter.) 2090 */ 2091 public Enumeration<SearchModifierCode> addModifierElement() {//2 2092 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2093 if (this.modifier == null) 2094 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2095 this.modifier.add(t); 2096 return t; 2097 } 2098 2099 /** 2100 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2101 */ 2102 public SearchParameter addModifier(SearchModifierCode value) { //1 2103 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2104 t.setValue(value); 2105 if (this.modifier == null) 2106 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2107 this.modifier.add(t); 2108 return this; 2109 } 2110 2111 /** 2112 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2113 */ 2114 public boolean hasModifier(SearchModifierCode value) { 2115 if (this.modifier == null) 2116 return false; 2117 for (Enumeration<SearchModifierCode> v : this.modifier) 2118 if (v.getValue().equals(value)) // code 2119 return true; 2120 return false; 2121 } 2122 2123 /** 2124 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2125 */ 2126 public List<StringType> getChain() { 2127 if (this.chain == null) 2128 this.chain = new ArrayList<StringType>(); 2129 return this.chain; 2130 } 2131 2132 /** 2133 * @return Returns a reference to <code>this</code> for easy method chaining 2134 */ 2135 public SearchParameter setChain(List<StringType> theChain) { 2136 this.chain = theChain; 2137 return this; 2138 } 2139 2140 public boolean hasChain() { 2141 if (this.chain == null) 2142 return false; 2143 for (StringType item : this.chain) 2144 if (!item.isEmpty()) 2145 return true; 2146 return false; 2147 } 2148 2149 /** 2150 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2151 */ 2152 public StringType addChainElement() {//2 2153 StringType t = new StringType(); 2154 if (this.chain == null) 2155 this.chain = new ArrayList<StringType>(); 2156 this.chain.add(t); 2157 return t; 2158 } 2159 2160 /** 2161 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2162 */ 2163 public SearchParameter addChain(String value) { //1 2164 StringType t = new StringType(); 2165 t.setValue(value); 2166 if (this.chain == null) 2167 this.chain = new ArrayList<StringType>(); 2168 this.chain.add(t); 2169 return this; 2170 } 2171 2172 /** 2173 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2174 */ 2175 public boolean hasChain(String value) { 2176 if (this.chain == null) 2177 return false; 2178 for (StringType v : this.chain) 2179 if (v.getValue().equals(value)) // string 2180 return true; 2181 return false; 2182 } 2183 2184 /** 2185 * @return {@link #component} (Used to define the parts of a composite search parameter.) 2186 */ 2187 public List<SearchParameterComponentComponent> getComponent() { 2188 if (this.component == null) 2189 this.component = new ArrayList<SearchParameterComponentComponent>(); 2190 return this.component; 2191 } 2192 2193 /** 2194 * @return Returns a reference to <code>this</code> for easy method chaining 2195 */ 2196 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2197 this.component = theComponent; 2198 return this; 2199 } 2200 2201 public boolean hasComponent() { 2202 if (this.component == null) 2203 return false; 2204 for (SearchParameterComponentComponent item : this.component) 2205 if (!item.isEmpty()) 2206 return true; 2207 return false; 2208 } 2209 2210 public SearchParameterComponentComponent addComponent() { //3 2211 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2212 if (this.component == null) 2213 this.component = new ArrayList<SearchParameterComponentComponent>(); 2214 this.component.add(t); 2215 return t; 2216 } 2217 2218 public SearchParameter addComponent(SearchParameterComponentComponent t) { //3 2219 if (t == null) 2220 return this; 2221 if (this.component == null) 2222 this.component = new ArrayList<SearchParameterComponentComponent>(); 2223 this.component.add(t); 2224 return this; 2225 } 2226 2227 /** 2228 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist 2229 */ 2230 public SearchParameterComponentComponent getComponentFirstRep() { 2231 if (getComponent().isEmpty()) { 2232 addComponent(); 2233 } 2234 return getComponent().get(0); 2235 } 2236 2237 protected void listChildren(List<Property> children) { 2238 super.listChildren(children); 2239 children.add(new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2240 children.add(new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2241 children.add(new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2242 children.add(new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2243 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2244 children.add(new Property("date", "dateTime", "The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date)); 2245 children.add(new Property("publisher", "string", "The name of the individual or organization that published the search parameter.", 0, 1, publisher)); 2246 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2247 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2248 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2249 children.add(new Property("purpose", "markdown", "Explaination of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2250 children.add(new Property("code", "code", "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, code)); 2251 children.add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base)); 2252 children.add(new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 2253 children.add(new Property("derivedFrom", "uri", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom)); 2254 children.add(new Property("description", "markdown", "A free text natural language description of the search parameter from a consumer's perspective. and how it used.", 0, 1, description)); 2255 children.add(new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2256 children.add(new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath)); 2257 children.add(new Property("xpathUsage", "code", "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, xpathUsage)); 2258 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target)); 2259 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 2260 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2261 children.add(new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain)); 2262 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component)); 2263 } 2264 2265 @Override 2266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2267 switch (_hash) { 2268 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2269 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2270 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2271 case -892481550: /*status*/ return new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2272 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2273 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date); 2274 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the search parameter.", 0, 1, publisher); 2275 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2276 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2277 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2278 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 2279 case 3059181: /*code*/ return new Property("code", "code", "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, code); 2280 case 3016401: /*base*/ return new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base); 2281 case 3575610: /*type*/ return new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 2282 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "uri", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom); 2283 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the search parameter from a consumer's perspective. and how it used.", 0, 1, description); 2284 case -1795452264: /*expression*/ return new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 2285 case 114256029: /*xpath*/ return new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath); 2286 case 1801322244: /*xpathUsage*/ return new Property("xpathUsage", "code", "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, xpathUsage); 2287 case -880905839: /*target*/ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target); 2288 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 2289 case -615513385: /*modifier*/ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 2290 case 94623425: /*chain*/ return new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain); 2291 case -1399907075: /*component*/ return new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component); 2292 default: return super.getNamedProperty(_hash, _name, _checkValid); 2293 } 2294 2295 } 2296 2297 @Override 2298 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2299 switch (hash) { 2300 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2301 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2302 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2303 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2304 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2305 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2306 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2307 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2308 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2309 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2310 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2311 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2312 case 3016401: /*base*/ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // CodeType 2313 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 2314 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // UriType 2315 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2316 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 2317 case 114256029: /*xpath*/ return this.xpath == null ? new Base[0] : new Base[] {this.xpath}; // StringType 2318 case 1801322244: /*xpathUsage*/ return this.xpathUsage == null ? new Base[0] : new Base[] {this.xpathUsage}; // Enumeration<XPathUsageType> 2319 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // CodeType 2320 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 2321 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 2322 case 94623425: /*chain*/ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 2323 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 2324 default: return super.getProperty(hash, name, checkValid); 2325 } 2326 2327 } 2328 2329 @Override 2330 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2331 switch (hash) { 2332 case 116079: // url 2333 this.url = castToUri(value); // UriType 2334 return value; 2335 case 351608024: // version 2336 this.version = castToString(value); // StringType 2337 return value; 2338 case 3373707: // name 2339 this.name = castToString(value); // StringType 2340 return value; 2341 case -892481550: // status 2342 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2343 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2344 return value; 2345 case -404562712: // experimental 2346 this.experimental = castToBoolean(value); // BooleanType 2347 return value; 2348 case 3076014: // date 2349 this.date = castToDateTime(value); // DateTimeType 2350 return value; 2351 case 1447404028: // publisher 2352 this.publisher = castToString(value); // StringType 2353 return value; 2354 case 951526432: // contact 2355 this.getContact().add(castToContactDetail(value)); // ContactDetail 2356 return value; 2357 case -669707736: // useContext 2358 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2359 return value; 2360 case -507075711: // jurisdiction 2361 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2362 return value; 2363 case -220463842: // purpose 2364 this.purpose = castToMarkdown(value); // MarkdownType 2365 return value; 2366 case 3059181: // code 2367 this.code = castToCode(value); // CodeType 2368 return value; 2369 case 3016401: // base 2370 this.getBase().add(castToCode(value)); // CodeType 2371 return value; 2372 case 3575610: // type 2373 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 2374 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2375 return value; 2376 case 1077922663: // derivedFrom 2377 this.derivedFrom = castToUri(value); // UriType 2378 return value; 2379 case -1724546052: // description 2380 this.description = castToMarkdown(value); // MarkdownType 2381 return value; 2382 case -1795452264: // expression 2383 this.expression = castToString(value); // StringType 2384 return value; 2385 case 114256029: // xpath 2386 this.xpath = castToString(value); // StringType 2387 return value; 2388 case 1801322244: // xpathUsage 2389 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 2390 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 2391 return value; 2392 case -880905839: // target 2393 this.getTarget().add(castToCode(value)); // CodeType 2394 return value; 2395 case -844673834: // comparator 2396 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 2397 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 2398 return value; 2399 case -615513385: // modifier 2400 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 2401 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 2402 return value; 2403 case 94623425: // chain 2404 this.getChain().add(castToString(value)); // StringType 2405 return value; 2406 case -1399907075: // component 2407 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 2408 return value; 2409 default: return super.setProperty(hash, name, value); 2410 } 2411 2412 } 2413 2414 @Override 2415 public Base setProperty(String name, Base value) throws FHIRException { 2416 if (name.equals("url")) { 2417 this.url = castToUri(value); // UriType 2418 } else if (name.equals("version")) { 2419 this.version = castToString(value); // StringType 2420 } else if (name.equals("name")) { 2421 this.name = castToString(value); // StringType 2422 } else if (name.equals("status")) { 2423 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2424 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2425 } else if (name.equals("experimental")) { 2426 this.experimental = castToBoolean(value); // BooleanType 2427 } else if (name.equals("date")) { 2428 this.date = castToDateTime(value); // DateTimeType 2429 } else if (name.equals("publisher")) { 2430 this.publisher = castToString(value); // StringType 2431 } else if (name.equals("contact")) { 2432 this.getContact().add(castToContactDetail(value)); 2433 } else if (name.equals("useContext")) { 2434 this.getUseContext().add(castToUsageContext(value)); 2435 } else if (name.equals("jurisdiction")) { 2436 this.getJurisdiction().add(castToCodeableConcept(value)); 2437 } else if (name.equals("purpose")) { 2438 this.purpose = castToMarkdown(value); // MarkdownType 2439 } else if (name.equals("code")) { 2440 this.code = castToCode(value); // CodeType 2441 } else if (name.equals("base")) { 2442 this.getBase().add(castToCode(value)); 2443 } else if (name.equals("type")) { 2444 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 2445 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2446 } else if (name.equals("derivedFrom")) { 2447 this.derivedFrom = castToUri(value); // UriType 2448 } else if (name.equals("description")) { 2449 this.description = castToMarkdown(value); // MarkdownType 2450 } else if (name.equals("expression")) { 2451 this.expression = castToString(value); // StringType 2452 } else if (name.equals("xpath")) { 2453 this.xpath = castToString(value); // StringType 2454 } else if (name.equals("xpathUsage")) { 2455 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 2456 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 2457 } else if (name.equals("target")) { 2458 this.getTarget().add(castToCode(value)); 2459 } else if (name.equals("comparator")) { 2460 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 2461 this.getComparator().add((Enumeration) value); 2462 } else if (name.equals("modifier")) { 2463 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 2464 this.getModifier().add((Enumeration) value); 2465 } else if (name.equals("chain")) { 2466 this.getChain().add(castToString(value)); 2467 } else if (name.equals("component")) { 2468 this.getComponent().add((SearchParameterComponentComponent) value); 2469 } else 2470 return super.setProperty(name, value); 2471 return value; 2472 } 2473 2474 @Override 2475 public Base makeProperty(int hash, String name) throws FHIRException { 2476 switch (hash) { 2477 case 116079: return getUrlElement(); 2478 case 351608024: return getVersionElement(); 2479 case 3373707: return getNameElement(); 2480 case -892481550: return getStatusElement(); 2481 case -404562712: return getExperimentalElement(); 2482 case 3076014: return getDateElement(); 2483 case 1447404028: return getPublisherElement(); 2484 case 951526432: return addContact(); 2485 case -669707736: return addUseContext(); 2486 case -507075711: return addJurisdiction(); 2487 case -220463842: return getPurposeElement(); 2488 case 3059181: return getCodeElement(); 2489 case 3016401: return addBaseElement(); 2490 case 3575610: return getTypeElement(); 2491 case 1077922663: return getDerivedFromElement(); 2492 case -1724546052: return getDescriptionElement(); 2493 case -1795452264: return getExpressionElement(); 2494 case 114256029: return getXpathElement(); 2495 case 1801322244: return getXpathUsageElement(); 2496 case -880905839: return addTargetElement(); 2497 case -844673834: return addComparatorElement(); 2498 case -615513385: return addModifierElement(); 2499 case 94623425: return addChainElement(); 2500 case -1399907075: return addComponent(); 2501 default: return super.makeProperty(hash, name); 2502 } 2503 2504 } 2505 2506 @Override 2507 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2508 switch (hash) { 2509 case 116079: /*url*/ return new String[] {"uri"}; 2510 case 351608024: /*version*/ return new String[] {"string"}; 2511 case 3373707: /*name*/ return new String[] {"string"}; 2512 case -892481550: /*status*/ return new String[] {"code"}; 2513 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2514 case 3076014: /*date*/ return new String[] {"dateTime"}; 2515 case 1447404028: /*publisher*/ return new String[] {"string"}; 2516 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2517 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2518 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2519 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2520 case 3059181: /*code*/ return new String[] {"code"}; 2521 case 3016401: /*base*/ return new String[] {"code"}; 2522 case 3575610: /*type*/ return new String[] {"code"}; 2523 case 1077922663: /*derivedFrom*/ return new String[] {"uri"}; 2524 case -1724546052: /*description*/ return new String[] {"markdown"}; 2525 case -1795452264: /*expression*/ return new String[] {"string"}; 2526 case 114256029: /*xpath*/ return new String[] {"string"}; 2527 case 1801322244: /*xpathUsage*/ return new String[] {"code"}; 2528 case -880905839: /*target*/ return new String[] {"code"}; 2529 case -844673834: /*comparator*/ return new String[] {"code"}; 2530 case -615513385: /*modifier*/ return new String[] {"code"}; 2531 case 94623425: /*chain*/ return new String[] {"string"}; 2532 case -1399907075: /*component*/ return new String[] {}; 2533 default: return super.getTypesForProperty(hash, name); 2534 } 2535 2536 } 2537 2538 @Override 2539 public Base addChild(String name) throws FHIRException { 2540 if (name.equals("url")) { 2541 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 2542 } 2543 else if (name.equals("version")) { 2544 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 2545 } 2546 else if (name.equals("name")) { 2547 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 2548 } 2549 else if (name.equals("status")) { 2550 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 2551 } 2552 else if (name.equals("experimental")) { 2553 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 2554 } 2555 else if (name.equals("date")) { 2556 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 2557 } 2558 else if (name.equals("publisher")) { 2559 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 2560 } 2561 else if (name.equals("contact")) { 2562 return addContact(); 2563 } 2564 else if (name.equals("useContext")) { 2565 return addUseContext(); 2566 } 2567 else if (name.equals("jurisdiction")) { 2568 return addJurisdiction(); 2569 } 2570 else if (name.equals("purpose")) { 2571 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 2572 } 2573 else if (name.equals("code")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 2575 } 2576 else if (name.equals("base")) { 2577 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 2578 } 2579 else if (name.equals("type")) { 2580 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 2581 } 2582 else if (name.equals("derivedFrom")) { 2583 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 2584 } 2585 else if (name.equals("description")) { 2586 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 2587 } 2588 else if (name.equals("expression")) { 2589 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 2590 } 2591 else if (name.equals("xpath")) { 2592 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 2593 } 2594 else if (name.equals("xpathUsage")) { 2595 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 2596 } 2597 else if (name.equals("target")) { 2598 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 2599 } 2600 else if (name.equals("comparator")) { 2601 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 2602 } 2603 else if (name.equals("modifier")) { 2604 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 2605 } 2606 else if (name.equals("chain")) { 2607 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 2608 } 2609 else if (name.equals("component")) { 2610 return addComponent(); 2611 } 2612 else 2613 return super.addChild(name); 2614 } 2615 2616 public String fhirType() { 2617 return "SearchParameter"; 2618 2619 } 2620 2621 public SearchParameter copy() { 2622 SearchParameter dst = new SearchParameter(); 2623 copyValues(dst); 2624 dst.url = url == null ? null : url.copy(); 2625 dst.version = version == null ? null : version.copy(); 2626 dst.name = name == null ? null : name.copy(); 2627 dst.status = status == null ? null : status.copy(); 2628 dst.experimental = experimental == null ? null : experimental.copy(); 2629 dst.date = date == null ? null : date.copy(); 2630 dst.publisher = publisher == null ? null : publisher.copy(); 2631 if (contact != null) { 2632 dst.contact = new ArrayList<ContactDetail>(); 2633 for (ContactDetail i : contact) 2634 dst.contact.add(i.copy()); 2635 }; 2636 if (useContext != null) { 2637 dst.useContext = new ArrayList<UsageContext>(); 2638 for (UsageContext i : useContext) 2639 dst.useContext.add(i.copy()); 2640 }; 2641 if (jurisdiction != null) { 2642 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2643 for (CodeableConcept i : jurisdiction) 2644 dst.jurisdiction.add(i.copy()); 2645 }; 2646 dst.purpose = purpose == null ? null : purpose.copy(); 2647 dst.code = code == null ? null : code.copy(); 2648 if (base != null) { 2649 dst.base = new ArrayList<CodeType>(); 2650 for (CodeType i : base) 2651 dst.base.add(i.copy()); 2652 }; 2653 dst.type = type == null ? null : type.copy(); 2654 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 2655 dst.description = description == null ? null : description.copy(); 2656 dst.expression = expression == null ? null : expression.copy(); 2657 dst.xpath = xpath == null ? null : xpath.copy(); 2658 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 2659 if (target != null) { 2660 dst.target = new ArrayList<CodeType>(); 2661 for (CodeType i : target) 2662 dst.target.add(i.copy()); 2663 }; 2664 if (comparator != null) { 2665 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2666 for (Enumeration<SearchComparator> i : comparator) 2667 dst.comparator.add(i.copy()); 2668 }; 2669 if (modifier != null) { 2670 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2671 for (Enumeration<SearchModifierCode> i : modifier) 2672 dst.modifier.add(i.copy()); 2673 }; 2674 if (chain != null) { 2675 dst.chain = new ArrayList<StringType>(); 2676 for (StringType i : chain) 2677 dst.chain.add(i.copy()); 2678 }; 2679 if (component != null) { 2680 dst.component = new ArrayList<SearchParameterComponentComponent>(); 2681 for (SearchParameterComponentComponent i : component) 2682 dst.component.add(i.copy()); 2683 }; 2684 return dst; 2685 } 2686 2687 protected SearchParameter typedCopy() { 2688 return copy(); 2689 } 2690 2691 @Override 2692 public boolean equalsDeep(Base other_) { 2693 if (!super.equalsDeep(other_)) 2694 return false; 2695 if (!(other_ instanceof SearchParameter)) 2696 return false; 2697 SearchParameter o = (SearchParameter) other_; 2698 return compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) 2699 && compareDeep(type, o.type, true) && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(expression, o.expression, true) 2700 && compareDeep(xpath, o.xpath, true) && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true) 2701 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true) && compareDeep(chain, o.chain, true) 2702 && compareDeep(component, o.component, true); 2703 } 2704 2705 @Override 2706 public boolean equalsShallow(Base other_) { 2707 if (!super.equalsShallow(other_)) 2708 return false; 2709 if (!(other_ instanceof SearchParameter)) 2710 return false; 2711 SearchParameter o = (SearchParameter) other_; 2712 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) && compareValues(base, o.base, true) 2713 && compareValues(type, o.type, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(expression, o.expression, true) 2714 && compareValues(xpath, o.xpath, true) && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true) 2715 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true) && compareValues(chain, o.chain, true) 2716 ; 2717 } 2718 2719 public boolean isEmpty() { 2720 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, code, base, type 2721 , derivedFrom, expression, xpath, xpathUsage, target, comparator, modifier, chain 2722 , component); 2723 } 2724 2725 @Override 2726 public ResourceType getResourceType() { 2727 return ResourceType.SearchParameter; 2728 } 2729 2730 /** 2731 * Search parameter: <b>date</b> 2732 * <p> 2733 * Description: <b>The search parameter publication date</b><br> 2734 * Type: <b>date</b><br> 2735 * Path: <b>SearchParameter.date</b><br> 2736 * </p> 2737 */ 2738 @SearchParamDefinition(name="date", path="SearchParameter.date", description="The search parameter publication date", type="date" ) 2739 public static final String SP_DATE = "date"; 2740 /** 2741 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2742 * <p> 2743 * Description: <b>The search parameter publication date</b><br> 2744 * Type: <b>date</b><br> 2745 * Path: <b>SearchParameter.date</b><br> 2746 * </p> 2747 */ 2748 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2749 2750 /** 2751 * Search parameter: <b>code</b> 2752 * <p> 2753 * Description: <b>Code used in URL</b><br> 2754 * Type: <b>token</b><br> 2755 * Path: <b>SearchParameter.code</b><br> 2756 * </p> 2757 */ 2758 @SearchParamDefinition(name="code", path="SearchParameter.code", description="Code used in URL", type="token" ) 2759 public static final String SP_CODE = "code"; 2760 /** 2761 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2762 * <p> 2763 * Description: <b>Code used in URL</b><br> 2764 * Type: <b>token</b><br> 2765 * Path: <b>SearchParameter.code</b><br> 2766 * </p> 2767 */ 2768 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2769 2770 /** 2771 * Search parameter: <b>jurisdiction</b> 2772 * <p> 2773 * Description: <b>Intended jurisdiction for the search parameter</b><br> 2774 * Type: <b>token</b><br> 2775 * Path: <b>SearchParameter.jurisdiction</b><br> 2776 * </p> 2777 */ 2778 @SearchParamDefinition(name="jurisdiction", path="SearchParameter.jurisdiction", description="Intended jurisdiction for the search parameter", type="token" ) 2779 public static final String SP_JURISDICTION = "jurisdiction"; 2780 /** 2781 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2782 * <p> 2783 * Description: <b>Intended jurisdiction for the search parameter</b><br> 2784 * Type: <b>token</b><br> 2785 * Path: <b>SearchParameter.jurisdiction</b><br> 2786 * </p> 2787 */ 2788 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2789 2790 /** 2791 * Search parameter: <b>description</b> 2792 * <p> 2793 * Description: <b>The description of the search parameter</b><br> 2794 * Type: <b>string</b><br> 2795 * Path: <b>SearchParameter.description</b><br> 2796 * </p> 2797 */ 2798 @SearchParamDefinition(name="description", path="SearchParameter.description", description="The description of the search parameter", type="string" ) 2799 public static final String SP_DESCRIPTION = "description"; 2800 /** 2801 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2802 * <p> 2803 * Description: <b>The description of the search parameter</b><br> 2804 * Type: <b>string</b><br> 2805 * Path: <b>SearchParameter.description</b><br> 2806 * </p> 2807 */ 2808 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2809 2810 /** 2811 * Search parameter: <b>derived-from</b> 2812 * <p> 2813 * Description: <b>Original Definition for the search parameter</b><br> 2814 * Type: <b>uri</b><br> 2815 * Path: <b>SearchParameter.derivedFrom</b><br> 2816 * </p> 2817 */ 2818 @SearchParamDefinition(name="derived-from", path="SearchParameter.derivedFrom", description="Original Definition for the search parameter", type="uri" ) 2819 public static final String SP_DERIVED_FROM = "derived-from"; 2820 /** 2821 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2822 * <p> 2823 * Description: <b>Original Definition for the search parameter</b><br> 2824 * Type: <b>uri</b><br> 2825 * Path: <b>SearchParameter.derivedFrom</b><br> 2826 * </p> 2827 */ 2828 public static final ca.uhn.fhir.rest.gclient.UriClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DERIVED_FROM); 2829 2830 /** 2831 * Search parameter: <b>type</b> 2832 * <p> 2833 * Description: <b>number | date | string | token | reference | composite | quantity | uri</b><br> 2834 * Type: <b>token</b><br> 2835 * Path: <b>SearchParameter.type</b><br> 2836 * </p> 2837 */ 2838 @SearchParamDefinition(name="type", path="SearchParameter.type", description="number | date | string | token | reference | composite | quantity | uri", type="token" ) 2839 public static final String SP_TYPE = "type"; 2840 /** 2841 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2842 * <p> 2843 * Description: <b>number | date | string | token | reference | composite | quantity | uri</b><br> 2844 * Type: <b>token</b><br> 2845 * Path: <b>SearchParameter.type</b><br> 2846 * </p> 2847 */ 2848 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2849 2850 /** 2851 * Search parameter: <b>version</b> 2852 * <p> 2853 * Description: <b>The business version of the search parameter</b><br> 2854 * Type: <b>token</b><br> 2855 * Path: <b>SearchParameter.version</b><br> 2856 * </p> 2857 */ 2858 @SearchParamDefinition(name="version", path="SearchParameter.version", description="The business version of the search parameter", type="token" ) 2859 public static final String SP_VERSION = "version"; 2860 /** 2861 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2862 * <p> 2863 * Description: <b>The business version of the search parameter</b><br> 2864 * Type: <b>token</b><br> 2865 * Path: <b>SearchParameter.version</b><br> 2866 * </p> 2867 */ 2868 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2869 2870 /** 2871 * Search parameter: <b>url</b> 2872 * <p> 2873 * Description: <b>The uri that identifies the search parameter</b><br> 2874 * Type: <b>uri</b><br> 2875 * Path: <b>SearchParameter.url</b><br> 2876 * </p> 2877 */ 2878 @SearchParamDefinition(name="url", path="SearchParameter.url", description="The uri that identifies the search parameter", type="uri" ) 2879 public static final String SP_URL = "url"; 2880 /** 2881 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2882 * <p> 2883 * Description: <b>The uri that identifies the search parameter</b><br> 2884 * Type: <b>uri</b><br> 2885 * Path: <b>SearchParameter.url</b><br> 2886 * </p> 2887 */ 2888 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2889 2890 /** 2891 * Search parameter: <b>target</b> 2892 * <p> 2893 * Description: <b>Types of resource (if a resource reference)</b><br> 2894 * Type: <b>token</b><br> 2895 * Path: <b>SearchParameter.target</b><br> 2896 * </p> 2897 */ 2898 @SearchParamDefinition(name="target", path="SearchParameter.target", description="Types of resource (if a resource reference)", type="token" ) 2899 public static final String SP_TARGET = "target"; 2900 /** 2901 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2902 * <p> 2903 * Description: <b>Types of resource (if a resource reference)</b><br> 2904 * Type: <b>token</b><br> 2905 * Path: <b>SearchParameter.target</b><br> 2906 * </p> 2907 */ 2908 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET); 2909 2910 /** 2911 * Search parameter: <b>component</b> 2912 * <p> 2913 * Description: <b>Defines how the part works</b><br> 2914 * Type: <b>reference</b><br> 2915 * Path: <b>SearchParameter.component.definition</b><br> 2916 * </p> 2917 */ 2918 @SearchParamDefinition(name="component", path="SearchParameter.component.definition", description="Defines how the part works", type="reference", target={SearchParameter.class } ) 2919 public static final String SP_COMPONENT = "component"; 2920 /** 2921 * <b>Fluent Client</b> search parameter constant for <b>component</b> 2922 * <p> 2923 * Description: <b>Defines how the part works</b><br> 2924 * Type: <b>reference</b><br> 2925 * Path: <b>SearchParameter.component.definition</b><br> 2926 * </p> 2927 */ 2928 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT); 2929 2930/** 2931 * Constant for fluent queries to be used to add include statements. Specifies 2932 * the path value of "<b>SearchParameter:component</b>". 2933 */ 2934 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include("SearchParameter:component").toLocked(); 2935 2936 /** 2937 * Search parameter: <b>name</b> 2938 * <p> 2939 * Description: <b>Computationally friendly name of the search parameter</b><br> 2940 * Type: <b>string</b><br> 2941 * Path: <b>SearchParameter.name</b><br> 2942 * </p> 2943 */ 2944 @SearchParamDefinition(name="name", path="SearchParameter.name", description="Computationally friendly name of the search parameter", type="string" ) 2945 public static final String SP_NAME = "name"; 2946 /** 2947 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2948 * <p> 2949 * Description: <b>Computationally friendly name of the search parameter</b><br> 2950 * Type: <b>string</b><br> 2951 * Path: <b>SearchParameter.name</b><br> 2952 * </p> 2953 */ 2954 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2955 2956 /** 2957 * Search parameter: <b>publisher</b> 2958 * <p> 2959 * Description: <b>Name of the publisher of the search parameter</b><br> 2960 * Type: <b>string</b><br> 2961 * Path: <b>SearchParameter.publisher</b><br> 2962 * </p> 2963 */ 2964 @SearchParamDefinition(name="publisher", path="SearchParameter.publisher", description="Name of the publisher of the search parameter", type="string" ) 2965 public static final String SP_PUBLISHER = "publisher"; 2966 /** 2967 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2968 * <p> 2969 * Description: <b>Name of the publisher of the search parameter</b><br> 2970 * Type: <b>string</b><br> 2971 * Path: <b>SearchParameter.publisher</b><br> 2972 * </p> 2973 */ 2974 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2975 2976 /** 2977 * Search parameter: <b>status</b> 2978 * <p> 2979 * Description: <b>The current status of the search parameter</b><br> 2980 * Type: <b>token</b><br> 2981 * Path: <b>SearchParameter.status</b><br> 2982 * </p> 2983 */ 2984 @SearchParamDefinition(name="status", path="SearchParameter.status", description="The current status of the search parameter", type="token" ) 2985 public static final String SP_STATUS = "status"; 2986 /** 2987 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2988 * <p> 2989 * Description: <b>The current status of the search parameter</b><br> 2990 * Type: <b>token</b><br> 2991 * Path: <b>SearchParameter.status</b><br> 2992 * </p> 2993 */ 2994 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2995 2996 /** 2997 * Search parameter: <b>base</b> 2998 * <p> 2999 * Description: <b>The resource type(s) this search parameter applies to</b><br> 3000 * Type: <b>token</b><br> 3001 * Path: <b>SearchParameter.base</b><br> 3002 * </p> 3003 */ 3004 @SearchParamDefinition(name="base", path="SearchParameter.base", description="The resource type(s) this search parameter applies to", type="token" ) 3005 public static final String SP_BASE = "base"; 3006 /** 3007 * <b>Fluent Client</b> search parameter constant for <b>base</b> 3008 * <p> 3009 * Description: <b>The resource type(s) this search parameter applies to</b><br> 3010 * Type: <b>token</b><br> 3011 * Path: <b>SearchParameter.base</b><br> 3012 * </p> 3013 */ 3014 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE); 3015 3016 3017}