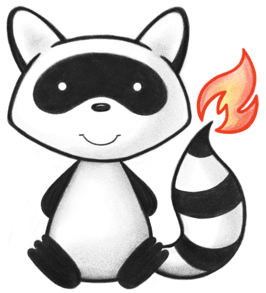
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.dstu3.model.Enumerations.SearchParamType; 042import org.hl7.fhir.dstu3.model.Enumerations.SearchParamTypeEnumFactory; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053/** 054 * A search parameter that defines a named search item that can be used to search/filter on a resource. 055 */ 056@ResourceDef(name="SearchParameter", profile="http://hl7.org/fhir/Profile/SearchParameter") 057@ChildOrder(names={"url", "version", "name", "status", "experimental", "date", "publisher", "contact", "useContext", "jurisdiction", "purpose", "code", "base", "type", "derivedFrom", "description", "expression", "xpath", "xpathUsage", "target", "comparator", "modifier", "chain", "component"}) 058public class SearchParameter extends MetadataResource { 059 060 public enum XPathUsageType { 061 /** 062 * The search parameter is derived directly from the selected nodes based on the type definitions. 063 */ 064 NORMAL, 065 /** 066 * The search parameter is derived by a phonetic transform from the selected nodes. 067 */ 068 PHONETIC, 069 /** 070 * The search parameter is based on a spatial transform of the selected nodes. 071 */ 072 NEARBY, 073 /** 074 * The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle. 075 */ 076 DISTANCE, 077 /** 078 * The interpretation of the xpath statement is unknown (and can't be automated). 079 */ 080 OTHER, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static XPathUsageType fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("normal".equals(codeString)) 089 return NORMAL; 090 if ("phonetic".equals(codeString)) 091 return PHONETIC; 092 if ("nearby".equals(codeString)) 093 return NEARBY; 094 if ("distance".equals(codeString)) 095 return DISTANCE; 096 if ("other".equals(codeString)) 097 return OTHER; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown XPathUsageType code '"+codeString+"'"); 102 } 103 public String toCode() { 104 switch (this) { 105 case NORMAL: return "normal"; 106 case PHONETIC: return "phonetic"; 107 case NEARBY: return "nearby"; 108 case DISTANCE: return "distance"; 109 case OTHER: return "other"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getSystem() { 115 switch (this) { 116 case NORMAL: return "http://hl7.org/fhir/search-xpath-usage"; 117 case PHONETIC: return "http://hl7.org/fhir/search-xpath-usage"; 118 case NEARBY: return "http://hl7.org/fhir/search-xpath-usage"; 119 case DISTANCE: return "http://hl7.org/fhir/search-xpath-usage"; 120 case OTHER: return "http://hl7.org/fhir/search-xpath-usage"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getDefinition() { 126 switch (this) { 127 case NORMAL: return "The search parameter is derived directly from the selected nodes based on the type definitions."; 128 case PHONETIC: return "The search parameter is derived by a phonetic transform from the selected nodes."; 129 case NEARBY: return "The search parameter is based on a spatial transform of the selected nodes."; 130 case DISTANCE: return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 131 case OTHER: return "The interpretation of the xpath statement is unknown (and can't be automated)."; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 public String getDisplay() { 137 switch (this) { 138 case NORMAL: return "Normal"; 139 case PHONETIC: return "Phonetic"; 140 case NEARBY: return "Nearby"; 141 case DISTANCE: return "Distance"; 142 case OTHER: return "Other"; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 } 148 149 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 150 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("normal".equals(codeString)) 155 return XPathUsageType.NORMAL; 156 if ("phonetic".equals(codeString)) 157 return XPathUsageType.PHONETIC; 158 if ("nearby".equals(codeString)) 159 return XPathUsageType.NEARBY; 160 if ("distance".equals(codeString)) 161 return XPathUsageType.DISTANCE; 162 if ("other".equals(codeString)) 163 return XPathUsageType.OTHER; 164 throw new IllegalArgumentException("Unknown XPathUsageType code '"+codeString+"'"); 165 } 166 public Enumeration<XPathUsageType> fromType(PrimitiveType<?> code) throws FHIRException { 167 if (code == null) 168 return null; 169 if (code.isEmpty()) 170 return new Enumeration<XPathUsageType>(this); 171 String codeString = code.asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("normal".equals(codeString)) 175 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL); 176 if ("phonetic".equals(codeString)) 177 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC); 178 if ("nearby".equals(codeString)) 179 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY); 180 if ("distance".equals(codeString)) 181 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE); 182 if ("other".equals(codeString)) 183 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER); 184 throw new FHIRException("Unknown XPathUsageType code '"+codeString+"'"); 185 } 186 public String toCode(XPathUsageType code) { 187 if (code == XPathUsageType.NORMAL) 188 return "normal"; 189 if (code == XPathUsageType.PHONETIC) 190 return "phonetic"; 191 if (code == XPathUsageType.NEARBY) 192 return "nearby"; 193 if (code == XPathUsageType.DISTANCE) 194 return "distance"; 195 if (code == XPathUsageType.OTHER) 196 return "other"; 197 return "?"; 198 } 199 public String toSystem(XPathUsageType code) { 200 return code.getSystem(); 201 } 202 } 203 204 public enum SearchComparator { 205 /** 206 * the value for the parameter in the resource is equal to the provided value 207 */ 208 EQ, 209 /** 210 * the value for the parameter in the resource is not equal to the provided value 211 */ 212 NE, 213 /** 214 * the value for the parameter in the resource is greater than the provided value 215 */ 216 GT, 217 /** 218 * the value for the parameter in the resource is less than the provided value 219 */ 220 LT, 221 /** 222 * the value for the parameter in the resource is greater or equal to the provided value 223 */ 224 GE, 225 /** 226 * the value for the parameter in the resource is less or equal to the provided value 227 */ 228 LE, 229 /** 230 * the value for the parameter in the resource starts after the provided value 231 */ 232 SA, 233 /** 234 * the value for the parameter in the resource ends before the provided value 235 */ 236 EB, 237 /** 238 * the value for the parameter in the resource is approximately the same to the provided value. 239 */ 240 AP, 241 /** 242 * added to help the parsers with the generic types 243 */ 244 NULL; 245 public static SearchComparator fromCode(String codeString) throws FHIRException { 246 if (codeString == null || "".equals(codeString)) 247 return null; 248 if ("eq".equals(codeString)) 249 return EQ; 250 if ("ne".equals(codeString)) 251 return NE; 252 if ("gt".equals(codeString)) 253 return GT; 254 if ("lt".equals(codeString)) 255 return LT; 256 if ("ge".equals(codeString)) 257 return GE; 258 if ("le".equals(codeString)) 259 return LE; 260 if ("sa".equals(codeString)) 261 return SA; 262 if ("eb".equals(codeString)) 263 return EB; 264 if ("ap".equals(codeString)) 265 return AP; 266 if (Configuration.isAcceptInvalidEnums()) 267 return null; 268 else 269 throw new FHIRException("Unknown SearchComparator code '"+codeString+"'"); 270 } 271 public String toCode() { 272 switch (this) { 273 case EQ: return "eq"; 274 case NE: return "ne"; 275 case GT: return "gt"; 276 case LT: return "lt"; 277 case GE: return "ge"; 278 case LE: return "le"; 279 case SA: return "sa"; 280 case EB: return "eb"; 281 case AP: return "ap"; 282 case NULL: return null; 283 default: return "?"; 284 } 285 } 286 public String getSystem() { 287 switch (this) { 288 case EQ: return "http://hl7.org/fhir/search-comparator"; 289 case NE: return "http://hl7.org/fhir/search-comparator"; 290 case GT: return "http://hl7.org/fhir/search-comparator"; 291 case LT: return "http://hl7.org/fhir/search-comparator"; 292 case GE: return "http://hl7.org/fhir/search-comparator"; 293 case LE: return "http://hl7.org/fhir/search-comparator"; 294 case SA: return "http://hl7.org/fhir/search-comparator"; 295 case EB: return "http://hl7.org/fhir/search-comparator"; 296 case AP: return "http://hl7.org/fhir/search-comparator"; 297 case NULL: return null; 298 default: return "?"; 299 } 300 } 301 public String getDefinition() { 302 switch (this) { 303 case EQ: return "the value for the parameter in the resource is equal to the provided value"; 304 case NE: return "the value for the parameter in the resource is not equal to the provided value"; 305 case GT: return "the value for the parameter in the resource is greater than the provided value"; 306 case LT: return "the value for the parameter in the resource is less than the provided value"; 307 case GE: return "the value for the parameter in the resource is greater or equal to the provided value"; 308 case LE: return "the value for the parameter in the resource is less or equal to the provided value"; 309 case SA: return "the value for the parameter in the resource starts after the provided value"; 310 case EB: return "the value for the parameter in the resource ends before the provided value"; 311 case AP: return "the value for the parameter in the resource is approximately the same to the provided value."; 312 case NULL: return null; 313 default: return "?"; 314 } 315 } 316 public String getDisplay() { 317 switch (this) { 318 case EQ: return "Equals"; 319 case NE: return "Not Equals"; 320 case GT: return "Greater Than"; 321 case LT: return "Less Then"; 322 case GE: return "Greater or Equals"; 323 case LE: return "Less of Equal"; 324 case SA: return "Starts After"; 325 case EB: return "Ends Before"; 326 case AP: return "Approximately"; 327 case NULL: return null; 328 default: return "?"; 329 } 330 } 331 } 332 333 public static class SearchComparatorEnumFactory implements EnumFactory<SearchComparator> { 334 public SearchComparator fromCode(String codeString) throws IllegalArgumentException { 335 if (codeString == null || "".equals(codeString)) 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("eq".equals(codeString)) 339 return SearchComparator.EQ; 340 if ("ne".equals(codeString)) 341 return SearchComparator.NE; 342 if ("gt".equals(codeString)) 343 return SearchComparator.GT; 344 if ("lt".equals(codeString)) 345 return SearchComparator.LT; 346 if ("ge".equals(codeString)) 347 return SearchComparator.GE; 348 if ("le".equals(codeString)) 349 return SearchComparator.LE; 350 if ("sa".equals(codeString)) 351 return SearchComparator.SA; 352 if ("eb".equals(codeString)) 353 return SearchComparator.EB; 354 if ("ap".equals(codeString)) 355 return SearchComparator.AP; 356 throw new IllegalArgumentException("Unknown SearchComparator code '"+codeString+"'"); 357 } 358 public Enumeration<SearchComparator> fromType(PrimitiveType<?> code) throws FHIRException { 359 if (code == null) 360 return null; 361 if (code.isEmpty()) 362 return new Enumeration<SearchComparator>(this); 363 String codeString = code.asStringValue(); 364 if (codeString == null || "".equals(codeString)) 365 return null; 366 if ("eq".equals(codeString)) 367 return new Enumeration<SearchComparator>(this, SearchComparator.EQ); 368 if ("ne".equals(codeString)) 369 return new Enumeration<SearchComparator>(this, SearchComparator.NE); 370 if ("gt".equals(codeString)) 371 return new Enumeration<SearchComparator>(this, SearchComparator.GT); 372 if ("lt".equals(codeString)) 373 return new Enumeration<SearchComparator>(this, SearchComparator.LT); 374 if ("ge".equals(codeString)) 375 return new Enumeration<SearchComparator>(this, SearchComparator.GE); 376 if ("le".equals(codeString)) 377 return new Enumeration<SearchComparator>(this, SearchComparator.LE); 378 if ("sa".equals(codeString)) 379 return new Enumeration<SearchComparator>(this, SearchComparator.SA); 380 if ("eb".equals(codeString)) 381 return new Enumeration<SearchComparator>(this, SearchComparator.EB); 382 if ("ap".equals(codeString)) 383 return new Enumeration<SearchComparator>(this, SearchComparator.AP); 384 throw new FHIRException("Unknown SearchComparator code '"+codeString+"'"); 385 } 386 public String toCode(SearchComparator code) { 387 if (code == SearchComparator.EQ) 388 return "eq"; 389 if (code == SearchComparator.NE) 390 return "ne"; 391 if (code == SearchComparator.GT) 392 return "gt"; 393 if (code == SearchComparator.LT) 394 return "lt"; 395 if (code == SearchComparator.GE) 396 return "ge"; 397 if (code == SearchComparator.LE) 398 return "le"; 399 if (code == SearchComparator.SA) 400 return "sa"; 401 if (code == SearchComparator.EB) 402 return "eb"; 403 if (code == SearchComparator.AP) 404 return "ap"; 405 return "?"; 406 } 407 public String toSystem(SearchComparator code) { 408 return code.getSystem(); 409 } 410 } 411 412 public enum SearchModifierCode { 413 /** 414 * The search parameter returns resources that have a value or not. 415 */ 416 MISSING, 417 /** 418 * The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents). 419 */ 420 EXACT, 421 /** 422 * The search parameter returns resources that include the supplied parameter value anywhere within the field being searched. 423 */ 424 CONTAINS, 425 /** 426 * The search parameter returns resources that do not contain a match. 427 */ 428 NOT, 429 /** 430 * The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text. 431 */ 432 TEXT, 433 /** 434 * The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set. 435 */ 436 IN, 437 /** 438 * The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set. 439 */ 440 NOTIN, 441 /** 442 * The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships). 443 */ 444 BELOW, 445 /** 446 * The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships). 447 */ 448 ABOVE, 449 /** 450 * The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.). 451 */ 452 TYPE, 453 /** 454 * added to help the parsers with the generic types 455 */ 456 NULL; 457 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 458 if (codeString == null || "".equals(codeString)) 459 return null; 460 if ("missing".equals(codeString)) 461 return MISSING; 462 if ("exact".equals(codeString)) 463 return EXACT; 464 if ("contains".equals(codeString)) 465 return CONTAINS; 466 if ("not".equals(codeString)) 467 return NOT; 468 if ("text".equals(codeString)) 469 return TEXT; 470 if ("in".equals(codeString)) 471 return IN; 472 if ("not-in".equals(codeString)) 473 return NOTIN; 474 if ("below".equals(codeString)) 475 return BELOW; 476 if ("above".equals(codeString)) 477 return ABOVE; 478 if ("type".equals(codeString)) 479 return TYPE; 480 if (Configuration.isAcceptInvalidEnums()) 481 return null; 482 else 483 throw new FHIRException("Unknown SearchModifierCode code '"+codeString+"'"); 484 } 485 public String toCode() { 486 switch (this) { 487 case MISSING: return "missing"; 488 case EXACT: return "exact"; 489 case CONTAINS: return "contains"; 490 case NOT: return "not"; 491 case TEXT: return "text"; 492 case IN: return "in"; 493 case NOTIN: return "not-in"; 494 case BELOW: return "below"; 495 case ABOVE: return "above"; 496 case TYPE: return "type"; 497 case NULL: return null; 498 default: return "?"; 499 } 500 } 501 public String getSystem() { 502 switch (this) { 503 case MISSING: return "http://hl7.org/fhir/search-modifier-code"; 504 case EXACT: return "http://hl7.org/fhir/search-modifier-code"; 505 case CONTAINS: return "http://hl7.org/fhir/search-modifier-code"; 506 case NOT: return "http://hl7.org/fhir/search-modifier-code"; 507 case TEXT: return "http://hl7.org/fhir/search-modifier-code"; 508 case IN: return "http://hl7.org/fhir/search-modifier-code"; 509 case NOTIN: return "http://hl7.org/fhir/search-modifier-code"; 510 case BELOW: return "http://hl7.org/fhir/search-modifier-code"; 511 case ABOVE: return "http://hl7.org/fhir/search-modifier-code"; 512 case TYPE: return "http://hl7.org/fhir/search-modifier-code"; 513 case NULL: return null; 514 default: return "?"; 515 } 516 } 517 public String getDefinition() { 518 switch (this) { 519 case MISSING: return "The search parameter returns resources that have a value or not."; 520 case EXACT: return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 521 case CONTAINS: return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 522 case NOT: return "The search parameter returns resources that do not contain a match."; 523 case TEXT: return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 524 case IN: return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 525 case NOTIN: return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 526 case BELOW: return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 527 case ABOVE: return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 528 case TYPE: return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 529 case NULL: return null; 530 default: return "?"; 531 } 532 } 533 public String getDisplay() { 534 switch (this) { 535 case MISSING: return "Missing"; 536 case EXACT: return "Exact"; 537 case CONTAINS: return "Contains"; 538 case NOT: return "Not"; 539 case TEXT: return "Text"; 540 case IN: return "In"; 541 case NOTIN: return "Not In"; 542 case BELOW: return "Below"; 543 case ABOVE: return "Above"; 544 case TYPE: return "Type"; 545 case NULL: return null; 546 default: return "?"; 547 } 548 } 549 } 550 551 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 552 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 553 if (codeString == null || "".equals(codeString)) 554 if (codeString == null || "".equals(codeString)) 555 return null; 556 if ("missing".equals(codeString)) 557 return SearchModifierCode.MISSING; 558 if ("exact".equals(codeString)) 559 return SearchModifierCode.EXACT; 560 if ("contains".equals(codeString)) 561 return SearchModifierCode.CONTAINS; 562 if ("not".equals(codeString)) 563 return SearchModifierCode.NOT; 564 if ("text".equals(codeString)) 565 return SearchModifierCode.TEXT; 566 if ("in".equals(codeString)) 567 return SearchModifierCode.IN; 568 if ("not-in".equals(codeString)) 569 return SearchModifierCode.NOTIN; 570 if ("below".equals(codeString)) 571 return SearchModifierCode.BELOW; 572 if ("above".equals(codeString)) 573 return SearchModifierCode.ABOVE; 574 if ("type".equals(codeString)) 575 return SearchModifierCode.TYPE; 576 throw new IllegalArgumentException("Unknown SearchModifierCode code '"+codeString+"'"); 577 } 578 public Enumeration<SearchModifierCode> fromType(PrimitiveType<?> code) throws FHIRException { 579 if (code == null) 580 return null; 581 if (code.isEmpty()) 582 return new Enumeration<SearchModifierCode>(this); 583 String codeString = code.asStringValue(); 584 if (codeString == null || "".equals(codeString)) 585 return null; 586 if ("missing".equals(codeString)) 587 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING); 588 if ("exact".equals(codeString)) 589 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT); 590 if ("contains".equals(codeString)) 591 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS); 592 if ("not".equals(codeString)) 593 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT); 594 if ("text".equals(codeString)) 595 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT); 596 if ("in".equals(codeString)) 597 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN); 598 if ("not-in".equals(codeString)) 599 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN); 600 if ("below".equals(codeString)) 601 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW); 602 if ("above".equals(codeString)) 603 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE); 604 if ("type".equals(codeString)) 605 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE); 606 throw new FHIRException("Unknown SearchModifierCode code '"+codeString+"'"); 607 } 608 public String toCode(SearchModifierCode code) { 609 if (code == SearchModifierCode.MISSING) 610 return "missing"; 611 if (code == SearchModifierCode.EXACT) 612 return "exact"; 613 if (code == SearchModifierCode.CONTAINS) 614 return "contains"; 615 if (code == SearchModifierCode.NOT) 616 return "not"; 617 if (code == SearchModifierCode.TEXT) 618 return "text"; 619 if (code == SearchModifierCode.IN) 620 return "in"; 621 if (code == SearchModifierCode.NOTIN) 622 return "not-in"; 623 if (code == SearchModifierCode.BELOW) 624 return "below"; 625 if (code == SearchModifierCode.ABOVE) 626 return "above"; 627 if (code == SearchModifierCode.TYPE) 628 return "type"; 629 return "?"; 630 } 631 public String toSystem(SearchModifierCode code) { 632 return code.getSystem(); 633 } 634 } 635 636 @Block() 637 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 638 /** 639 * The definition of the search parameter that describes this part. 640 */ 641 @Child(name = "definition", type = {SearchParameter.class}, order=1, min=1, max=1, modifier=false, summary=false) 642 @Description(shortDefinition="Defines how the part works", formalDefinition="The definition of the search parameter that describes this part." ) 643 protected Reference definition; 644 645 /** 646 * The actual object that is the target of the reference (The definition of the search parameter that describes this part.) 647 */ 648 protected SearchParameter definitionTarget; 649 650 /** 651 * A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 652 */ 653 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 654 @Description(shortDefinition="Subexpression relative to main expression", formalDefinition="A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression." ) 655 protected StringType expression; 656 657 private static final long serialVersionUID = -1105563614L; 658 659 /** 660 * Constructor 661 */ 662 public SearchParameterComponentComponent() { 663 super(); 664 } 665 666 /** 667 * Constructor 668 */ 669 public SearchParameterComponentComponent(Reference definition, StringType expression) { 670 super(); 671 this.definition = definition; 672 this.expression = expression; 673 } 674 675 /** 676 * @return {@link #definition} (The definition of the search parameter that describes this part.) 677 */ 678 public Reference getDefinition() { 679 if (this.definition == null) 680 if (Configuration.errorOnAutoCreate()) 681 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 682 else if (Configuration.doAutoCreate()) 683 this.definition = new Reference(); // cc 684 return this.definition; 685 } 686 687 public boolean hasDefinition() { 688 return this.definition != null && !this.definition.isEmpty(); 689 } 690 691 /** 692 * @param value {@link #definition} (The definition of the search parameter that describes this part.) 693 */ 694 public SearchParameterComponentComponent setDefinition(Reference value) { 695 this.definition = value; 696 return this; 697 } 698 699 /** 700 * @return {@link #definition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The definition of the search parameter that describes this part.) 701 */ 702 public SearchParameter getDefinitionTarget() { 703 if (this.definitionTarget == null) 704 if (Configuration.errorOnAutoCreate()) 705 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 706 else if (Configuration.doAutoCreate()) 707 this.definitionTarget = new SearchParameter(); // aa 708 return this.definitionTarget; 709 } 710 711 /** 712 * @param value {@link #definition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The definition of the search parameter that describes this part.) 713 */ 714 public SearchParameterComponentComponent setDefinitionTarget(SearchParameter value) { 715 this.definitionTarget = value; 716 return this; 717 } 718 719 /** 720 * @return {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 721 */ 722 public StringType getExpressionElement() { 723 if (this.expression == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 726 else if (Configuration.doAutoCreate()) 727 this.expression = new StringType(); // bb 728 return this.expression; 729 } 730 731 public boolean hasExpressionElement() { 732 return this.expression != null && !this.expression.isEmpty(); 733 } 734 735 public boolean hasExpression() { 736 return this.expression != null && !this.expression.isEmpty(); 737 } 738 739 /** 740 * @param value {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 741 */ 742 public SearchParameterComponentComponent setExpressionElement(StringType value) { 743 this.expression = value; 744 return this; 745 } 746 747 /** 748 * @return A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 749 */ 750 public String getExpression() { 751 return this.expression == null ? null : this.expression.getValue(); 752 } 753 754 /** 755 * @param value A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 756 */ 757 public SearchParameterComponentComponent setExpression(String value) { 758 if (this.expression == null) 759 this.expression = new StringType(); 760 this.expression.setValue(value); 761 return this; 762 } 763 764 protected void listChildren(List<Property> children) { 765 super.listChildren(children); 766 children.add(new Property("definition", "Reference(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition)); 767 children.add(new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression)); 768 } 769 770 @Override 771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 772 switch (_hash) { 773 case -1014418093: /*definition*/ return new Property("definition", "Reference(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition); 774 case -1795452264: /*expression*/ return new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression); 775 default: return super.getNamedProperty(_hash, _name, _checkValid); 776 } 777 778 } 779 780 @Override 781 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 782 switch (hash) { 783 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 784 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 785 default: return super.getProperty(hash, name, checkValid); 786 } 787 788 } 789 790 @Override 791 public Base setProperty(int hash, String name, Base value) throws FHIRException { 792 switch (hash) { 793 case -1014418093: // definition 794 this.definition = castToReference(value); // Reference 795 return value; 796 case -1795452264: // expression 797 this.expression = castToString(value); // StringType 798 return value; 799 default: return super.setProperty(hash, name, value); 800 } 801 802 } 803 804 @Override 805 public Base setProperty(String name, Base value) throws FHIRException { 806 if (name.equals("definition")) { 807 this.definition = castToReference(value); // Reference 808 } else if (name.equals("expression")) { 809 this.expression = castToString(value); // StringType 810 } else 811 return super.setProperty(name, value); 812 return value; 813 } 814 815 @Override 816 public Base makeProperty(int hash, String name) throws FHIRException { 817 switch (hash) { 818 case -1014418093: return getDefinition(); 819 case -1795452264: return getExpressionElement(); 820 default: return super.makeProperty(hash, name); 821 } 822 823 } 824 825 @Override 826 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case -1014418093: /*definition*/ return new String[] {"Reference"}; 829 case -1795452264: /*expression*/ return new String[] {"string"}; 830 default: return super.getTypesForProperty(hash, name); 831 } 832 833 } 834 835 @Override 836 public Base addChild(String name) throws FHIRException { 837 if (name.equals("definition")) { 838 this.definition = new Reference(); 839 return this.definition; 840 } 841 else if (name.equals("expression")) { 842 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 843 } 844 else 845 return super.addChild(name); 846 } 847 848 public SearchParameterComponentComponent copy() { 849 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 850 copyValues(dst); 851 dst.definition = definition == null ? null : definition.copy(); 852 dst.expression = expression == null ? null : expression.copy(); 853 return dst; 854 } 855 856 @Override 857 public boolean equalsDeep(Base other_) { 858 if (!super.equalsDeep(other_)) 859 return false; 860 if (!(other_ instanceof SearchParameterComponentComponent)) 861 return false; 862 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 863 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true) 864 ; 865 } 866 867 @Override 868 public boolean equalsShallow(Base other_) { 869 if (!super.equalsShallow(other_)) 870 return false; 871 if (!(other_ instanceof SearchParameterComponentComponent)) 872 return false; 873 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 874 return compareValues(expression, o.expression, true); 875 } 876 877 public boolean isEmpty() { 878 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 879 } 880 881 public String fhirType() { 882 return "SearchParameter.component"; 883 884 } 885 886 } 887 888 /** 889 * Explaination of why this search parameter is needed and why it has been designed as it has. 890 */ 891 @Child(name = "purpose", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 892 @Description(shortDefinition="Why this search parameter is defined", formalDefinition="Explaination of why this search parameter is needed and why it has been designed as it has." ) 893 protected MarkdownType purpose; 894 895 /** 896 * The code used in the URL or the parameter name in a parameters resource for this search parameter. 897 */ 898 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 899 @Description(shortDefinition="Code used in URL", formalDefinition="The code used in the URL or the parameter name in a parameters resource for this search parameter." ) 900 protected CodeType code; 901 902 /** 903 * The base resource type(s) that this search parameter can be used against. 904 */ 905 @Child(name = "base", type = {CodeType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 906 @Description(shortDefinition="The resource type(s) this search parameter applies to", formalDefinition="The base resource type(s) that this search parameter can be used against." ) 907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 908 protected List<CodeType> base; 909 910 /** 911 * The type of value a search parameter refers to, and how the content is interpreted. 912 */ 913 @Child(name = "type", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 914 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri", formalDefinition="The type of value a search parameter refers to, and how the content is interpreted." ) 915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 916 protected Enumeration<SearchParamType> type; 917 918 /** 919 * Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 920 */ 921 @Child(name = "derivedFrom", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=false) 922 @Description(shortDefinition="Original Definition for the search parameter", formalDefinition="Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter." ) 923 protected UriType derivedFrom; 924 925 /** 926 * A FHIRPath expression that returns a set of elements for the search parameter. 927 */ 928 @Child(name = "expression", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 929 @Description(shortDefinition="FHIRPath expression that extracts the values", formalDefinition="A FHIRPath expression that returns a set of elements for the search parameter." ) 930 protected StringType expression; 931 932 /** 933 * An XPath expression that returns a set of elements for the search parameter. 934 */ 935 @Child(name = "xpath", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 936 @Description(shortDefinition="XPath that extracts the values", formalDefinition="An XPath expression that returns a set of elements for the search parameter." ) 937 protected StringType xpath; 938 939 /** 940 * How the search parameter relates to the set of elements returned by evaluating the xpath query. 941 */ 942 @Child(name = "xpathUsage", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 943 @Description(shortDefinition="normal | phonetic | nearby | distance | other", formalDefinition="How the search parameter relates to the set of elements returned by evaluating the xpath query." ) 944 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-xpath-usage") 945 protected Enumeration<XPathUsageType> xpathUsage; 946 947 /** 948 * Types of resource (if a resource is referenced). 949 */ 950 @Child(name = "target", type = {CodeType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 951 @Description(shortDefinition="Types of resource (if a resource reference)", formalDefinition="Types of resource (if a resource is referenced)." ) 952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 953 protected List<CodeType> target; 954 955 /** 956 * Comparators supported for the search parameter. 957 */ 958 @Child(name = "comparator", type = {CodeType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 959 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators supported for the search parameter." ) 960 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 961 protected List<Enumeration<SearchComparator>> comparator; 962 963 /** 964 * A modifier supported for the search parameter. 965 */ 966 @Child(name = "modifier", type = {CodeType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 967 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type", formalDefinition="A modifier supported for the search parameter." ) 968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 969 protected List<Enumeration<SearchModifierCode>> modifier; 970 971 /** 972 * Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type. 973 */ 974 @Child(name = "chain", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 975 @Description(shortDefinition="Chained names supported", formalDefinition="Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type." ) 976 protected List<StringType> chain; 977 978 /** 979 * Used to define the parts of a composite search parameter. 980 */ 981 @Child(name = "component", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 982 @Description(shortDefinition="For Composite resources to define the parts", formalDefinition="Used to define the parts of a composite search parameter." ) 983 protected List<SearchParameterComponentComponent> component; 984 985 private static final long serialVersionUID = -769368159L; 986 987 /** 988 * Constructor 989 */ 990 public SearchParameter() { 991 super(); 992 } 993 994 /** 995 * Constructor 996 */ 997 public SearchParameter(UriType url, StringType name, Enumeration<PublicationStatus> status, CodeType code, Enumeration<SearchParamType> type, MarkdownType description) { 998 super(); 999 this.url = url; 1000 this.name = name; 1001 this.status = status; 1002 this.code = code; 1003 this.type = type; 1004 this.description = description; 1005 } 1006 1007 /** 1008 * @return {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1009 */ 1010 public UriType getUrlElement() { 1011 if (this.url == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create SearchParameter.url"); 1014 else if (Configuration.doAutoCreate()) 1015 this.url = new UriType(); // bb 1016 return this.url; 1017 } 1018 1019 public boolean hasUrlElement() { 1020 return this.url != null && !this.url.isEmpty(); 1021 } 1022 1023 public boolean hasUrl() { 1024 return this.url != null && !this.url.isEmpty(); 1025 } 1026 1027 /** 1028 * @param value {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1029 */ 1030 public SearchParameter setUrlElement(UriType value) { 1031 this.url = value; 1032 return this; 1033 } 1034 1035 /** 1036 * @return An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions). 1037 */ 1038 public String getUrl() { 1039 return this.url == null ? null : this.url.getValue(); 1040 } 1041 1042 /** 1043 * @param value An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions). 1044 */ 1045 public SearchParameter setUrl(String value) { 1046 if (this.url == null) 1047 this.url = new UriType(); 1048 this.url.setValue(value); 1049 return this; 1050 } 1051 1052 /** 1053 * @return {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1054 */ 1055 public StringType getVersionElement() { 1056 if (this.version == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create SearchParameter.version"); 1059 else if (Configuration.doAutoCreate()) 1060 this.version = new StringType(); // bb 1061 return this.version; 1062 } 1063 1064 public boolean hasVersionElement() { 1065 return this.version != null && !this.version.isEmpty(); 1066 } 1067 1068 public boolean hasVersion() { 1069 return this.version != null && !this.version.isEmpty(); 1070 } 1071 1072 /** 1073 * @param value {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1074 */ 1075 public SearchParameter setVersionElement(StringType value) { 1076 this.version = value; 1077 return this; 1078 } 1079 1080 /** 1081 * @return The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1082 */ 1083 public String getVersion() { 1084 return this.version == null ? null : this.version.getValue(); 1085 } 1086 1087 /** 1088 * @param value The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1089 */ 1090 public SearchParameter setVersion(String value) { 1091 if (Utilities.noString(value)) 1092 this.version = null; 1093 else { 1094 if (this.version == null) 1095 this.version = new StringType(); 1096 this.version.setValue(value); 1097 } 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1103 */ 1104 public StringType getNameElement() { 1105 if (this.name == null) 1106 if (Configuration.errorOnAutoCreate()) 1107 throw new Error("Attempt to auto-create SearchParameter.name"); 1108 else if (Configuration.doAutoCreate()) 1109 this.name = new StringType(); // bb 1110 return this.name; 1111 } 1112 1113 public boolean hasNameElement() { 1114 return this.name != null && !this.name.isEmpty(); 1115 } 1116 1117 public boolean hasName() { 1118 return this.name != null && !this.name.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1123 */ 1124 public SearchParameter setNameElement(StringType value) { 1125 this.name = value; 1126 return this; 1127 } 1128 1129 /** 1130 * @return A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1131 */ 1132 public String getName() { 1133 return this.name == null ? null : this.name.getValue(); 1134 } 1135 1136 /** 1137 * @param value A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1138 */ 1139 public SearchParameter setName(String value) { 1140 if (this.name == null) 1141 this.name = new StringType(); 1142 this.name.setValue(value); 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1148 */ 1149 public Enumeration<PublicationStatus> getStatusElement() { 1150 if (this.status == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create SearchParameter.status"); 1153 else if (Configuration.doAutoCreate()) 1154 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1155 return this.status; 1156 } 1157 1158 public boolean hasStatusElement() { 1159 return this.status != null && !this.status.isEmpty(); 1160 } 1161 1162 public boolean hasStatus() { 1163 return this.status != null && !this.status.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1168 */ 1169 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1170 this.status = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return The status of this search parameter. Enables tracking the life-cycle of the content. 1176 */ 1177 public PublicationStatus getStatus() { 1178 return this.status == null ? null : this.status.getValue(); 1179 } 1180 1181 /** 1182 * @param value The status of this search parameter. Enables tracking the life-cycle of the content. 1183 */ 1184 public SearchParameter setStatus(PublicationStatus value) { 1185 if (this.status == null) 1186 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1187 this.status.setValue(value); 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #experimental} (A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1193 */ 1194 public BooleanType getExperimentalElement() { 1195 if (this.experimental == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1198 else if (Configuration.doAutoCreate()) 1199 this.experimental = new BooleanType(); // bb 1200 return this.experimental; 1201 } 1202 1203 public boolean hasExperimentalElement() { 1204 return this.experimental != null && !this.experimental.isEmpty(); 1205 } 1206 1207 public boolean hasExperimental() { 1208 return this.experimental != null && !this.experimental.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #experimental} (A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1213 */ 1214 public SearchParameter setExperimentalElement(BooleanType value) { 1215 this.experimental = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1221 */ 1222 public boolean getExperimental() { 1223 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1224 } 1225 1226 /** 1227 * @param value A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1228 */ 1229 public SearchParameter setExperimental(boolean value) { 1230 if (this.experimental == null) 1231 this.experimental = new BooleanType(); 1232 this.experimental.setValue(value); 1233 return this; 1234 } 1235 1236 /** 1237 * @return {@link #date} (The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1238 */ 1239 public DateTimeType getDateElement() { 1240 if (this.date == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create SearchParameter.date"); 1243 else if (Configuration.doAutoCreate()) 1244 this.date = new DateTimeType(); // bb 1245 return this.date; 1246 } 1247 1248 public boolean hasDateElement() { 1249 return this.date != null && !this.date.isEmpty(); 1250 } 1251 1252 public boolean hasDate() { 1253 return this.date != null && !this.date.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #date} (The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1258 */ 1259 public SearchParameter setDateElement(DateTimeType value) { 1260 this.date = value; 1261 return this; 1262 } 1263 1264 /** 1265 * @return The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1266 */ 1267 public Date getDate() { 1268 return this.date == null ? null : this.date.getValue(); 1269 } 1270 1271 /** 1272 * @param value The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1273 */ 1274 public SearchParameter setDate(Date value) { 1275 if (value == null) 1276 this.date = null; 1277 else { 1278 if (this.date == null) 1279 this.date = new DateTimeType(); 1280 this.date.setValue(value); 1281 } 1282 return this; 1283 } 1284 1285 /** 1286 * @return {@link #publisher} (The name of the individual or organization that published the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1287 */ 1288 public StringType getPublisherElement() { 1289 if (this.publisher == null) 1290 if (Configuration.errorOnAutoCreate()) 1291 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1292 else if (Configuration.doAutoCreate()) 1293 this.publisher = new StringType(); // bb 1294 return this.publisher; 1295 } 1296 1297 public boolean hasPublisherElement() { 1298 return this.publisher != null && !this.publisher.isEmpty(); 1299 } 1300 1301 public boolean hasPublisher() { 1302 return this.publisher != null && !this.publisher.isEmpty(); 1303 } 1304 1305 /** 1306 * @param value {@link #publisher} (The name of the individual or organization that published the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1307 */ 1308 public SearchParameter setPublisherElement(StringType value) { 1309 this.publisher = value; 1310 return this; 1311 } 1312 1313 /** 1314 * @return The name of the individual or organization that published the search parameter. 1315 */ 1316 public String getPublisher() { 1317 return this.publisher == null ? null : this.publisher.getValue(); 1318 } 1319 1320 /** 1321 * @param value The name of the individual or organization that published the search parameter. 1322 */ 1323 public SearchParameter setPublisher(String value) { 1324 if (Utilities.noString(value)) 1325 this.publisher = null; 1326 else { 1327 if (this.publisher == null) 1328 this.publisher = new StringType(); 1329 this.publisher.setValue(value); 1330 } 1331 return this; 1332 } 1333 1334 /** 1335 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1336 */ 1337 public List<ContactDetail> getContact() { 1338 if (this.contact == null) 1339 this.contact = new ArrayList<ContactDetail>(); 1340 return this.contact; 1341 } 1342 1343 /** 1344 * @return Returns a reference to <code>this</code> for easy method chaining 1345 */ 1346 public SearchParameter setContact(List<ContactDetail> theContact) { 1347 this.contact = theContact; 1348 return this; 1349 } 1350 1351 public boolean hasContact() { 1352 if (this.contact == null) 1353 return false; 1354 for (ContactDetail item : this.contact) 1355 if (!item.isEmpty()) 1356 return true; 1357 return false; 1358 } 1359 1360 public ContactDetail addContact() { //3 1361 ContactDetail t = new ContactDetail(); 1362 if (this.contact == null) 1363 this.contact = new ArrayList<ContactDetail>(); 1364 this.contact.add(t); 1365 return t; 1366 } 1367 1368 public SearchParameter addContact(ContactDetail t) { //3 1369 if (t == null) 1370 return this; 1371 if (this.contact == null) 1372 this.contact = new ArrayList<ContactDetail>(); 1373 this.contact.add(t); 1374 return this; 1375 } 1376 1377 /** 1378 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1379 */ 1380 public ContactDetail getContactFirstRep() { 1381 if (getContact().isEmpty()) { 1382 addContact(); 1383 } 1384 return getContact().get(0); 1385 } 1386 1387 /** 1388 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.) 1389 */ 1390 public List<UsageContext> getUseContext() { 1391 if (this.useContext == null) 1392 this.useContext = new ArrayList<UsageContext>(); 1393 return this.useContext; 1394 } 1395 1396 /** 1397 * @return Returns a reference to <code>this</code> for easy method chaining 1398 */ 1399 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1400 this.useContext = theUseContext; 1401 return this; 1402 } 1403 1404 public boolean hasUseContext() { 1405 if (this.useContext == null) 1406 return false; 1407 for (UsageContext item : this.useContext) 1408 if (!item.isEmpty()) 1409 return true; 1410 return false; 1411 } 1412 1413 public UsageContext addUseContext() { //3 1414 UsageContext t = new UsageContext(); 1415 if (this.useContext == null) 1416 this.useContext = new ArrayList<UsageContext>(); 1417 this.useContext.add(t); 1418 return t; 1419 } 1420 1421 public SearchParameter addUseContext(UsageContext t) { //3 1422 if (t == null) 1423 return this; 1424 if (this.useContext == null) 1425 this.useContext = new ArrayList<UsageContext>(); 1426 this.useContext.add(t); 1427 return this; 1428 } 1429 1430 /** 1431 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1432 */ 1433 public UsageContext getUseContextFirstRep() { 1434 if (getUseContext().isEmpty()) { 1435 addUseContext(); 1436 } 1437 return getUseContext().get(0); 1438 } 1439 1440 /** 1441 * @return {@link #jurisdiction} (A legal or geographic region in which the search parameter is intended to be used.) 1442 */ 1443 public List<CodeableConcept> getJurisdiction() { 1444 if (this.jurisdiction == null) 1445 this.jurisdiction = new ArrayList<CodeableConcept>(); 1446 return this.jurisdiction; 1447 } 1448 1449 /** 1450 * @return Returns a reference to <code>this</code> for easy method chaining 1451 */ 1452 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 1453 this.jurisdiction = theJurisdiction; 1454 return this; 1455 } 1456 1457 public boolean hasJurisdiction() { 1458 if (this.jurisdiction == null) 1459 return false; 1460 for (CodeableConcept item : this.jurisdiction) 1461 if (!item.isEmpty()) 1462 return true; 1463 return false; 1464 } 1465 1466 public CodeableConcept addJurisdiction() { //3 1467 CodeableConcept t = new CodeableConcept(); 1468 if (this.jurisdiction == null) 1469 this.jurisdiction = new ArrayList<CodeableConcept>(); 1470 this.jurisdiction.add(t); 1471 return t; 1472 } 1473 1474 public SearchParameter addJurisdiction(CodeableConcept t) { //3 1475 if (t == null) 1476 return this; 1477 if (this.jurisdiction == null) 1478 this.jurisdiction = new ArrayList<CodeableConcept>(); 1479 this.jurisdiction.add(t); 1480 return this; 1481 } 1482 1483 /** 1484 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1485 */ 1486 public CodeableConcept getJurisdictionFirstRep() { 1487 if (getJurisdiction().isEmpty()) { 1488 addJurisdiction(); 1489 } 1490 return getJurisdiction().get(0); 1491 } 1492 1493 /** 1494 * @return {@link #purpose} (Explaination of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1495 */ 1496 public MarkdownType getPurposeElement() { 1497 if (this.purpose == null) 1498 if (Configuration.errorOnAutoCreate()) 1499 throw new Error("Attempt to auto-create SearchParameter.purpose"); 1500 else if (Configuration.doAutoCreate()) 1501 this.purpose = new MarkdownType(); // bb 1502 return this.purpose; 1503 } 1504 1505 public boolean hasPurposeElement() { 1506 return this.purpose != null && !this.purpose.isEmpty(); 1507 } 1508 1509 public boolean hasPurpose() { 1510 return this.purpose != null && !this.purpose.isEmpty(); 1511 } 1512 1513 /** 1514 * @param value {@link #purpose} (Explaination of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1515 */ 1516 public SearchParameter setPurposeElement(MarkdownType value) { 1517 this.purpose = value; 1518 return this; 1519 } 1520 1521 /** 1522 * @return Explaination of why this search parameter is needed and why it has been designed as it has. 1523 */ 1524 public String getPurpose() { 1525 return this.purpose == null ? null : this.purpose.getValue(); 1526 } 1527 1528 /** 1529 * @param value Explaination of why this search parameter is needed and why it has been designed as it has. 1530 */ 1531 public SearchParameter setPurpose(String value) { 1532 if (value == null) 1533 this.purpose = null; 1534 else { 1535 if (this.purpose == null) 1536 this.purpose = new MarkdownType(); 1537 this.purpose.setValue(value); 1538 } 1539 return this; 1540 } 1541 1542 /** 1543 * @return {@link #code} (The code used in the URL or the parameter name in a parameters resource for this search parameter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1544 */ 1545 public CodeType getCodeElement() { 1546 if (this.code == null) 1547 if (Configuration.errorOnAutoCreate()) 1548 throw new Error("Attempt to auto-create SearchParameter.code"); 1549 else if (Configuration.doAutoCreate()) 1550 this.code = new CodeType(); // bb 1551 return this.code; 1552 } 1553 1554 public boolean hasCodeElement() { 1555 return this.code != null && !this.code.isEmpty(); 1556 } 1557 1558 public boolean hasCode() { 1559 return this.code != null && !this.code.isEmpty(); 1560 } 1561 1562 /** 1563 * @param value {@link #code} (The code used in the URL or the parameter name in a parameters resource for this search parameter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1564 */ 1565 public SearchParameter setCodeElement(CodeType value) { 1566 this.code = value; 1567 return this; 1568 } 1569 1570 /** 1571 * @return The code used in the URL or the parameter name in a parameters resource for this search parameter. 1572 */ 1573 public String getCode() { 1574 return this.code == null ? null : this.code.getValue(); 1575 } 1576 1577 /** 1578 * @param value The code used in the URL or the parameter name in a parameters resource for this search parameter. 1579 */ 1580 public SearchParameter setCode(String value) { 1581 if (this.code == null) 1582 this.code = new CodeType(); 1583 this.code.setValue(value); 1584 return this; 1585 } 1586 1587 /** 1588 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1589 */ 1590 public List<CodeType> getBase() { 1591 if (this.base == null) 1592 this.base = new ArrayList<CodeType>(); 1593 return this.base; 1594 } 1595 1596 /** 1597 * @return Returns a reference to <code>this</code> for easy method chaining 1598 */ 1599 public SearchParameter setBase(List<CodeType> theBase) { 1600 this.base = theBase; 1601 return this; 1602 } 1603 1604 public boolean hasBase() { 1605 if (this.base == null) 1606 return false; 1607 for (CodeType item : this.base) 1608 if (!item.isEmpty()) 1609 return true; 1610 return false; 1611 } 1612 1613 /** 1614 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1615 */ 1616 public CodeType addBaseElement() {//2 1617 CodeType t = new CodeType(); 1618 if (this.base == null) 1619 this.base = new ArrayList<CodeType>(); 1620 this.base.add(t); 1621 return t; 1622 } 1623 1624 /** 1625 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1626 */ 1627 public SearchParameter addBase(String value) { //1 1628 CodeType t = new CodeType(); 1629 t.setValue(value); 1630 if (this.base == null) 1631 this.base = new ArrayList<CodeType>(); 1632 this.base.add(t); 1633 return this; 1634 } 1635 1636 /** 1637 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1638 */ 1639 public boolean hasBase(String value) { 1640 if (this.base == null) 1641 return false; 1642 for (CodeType v : this.base) 1643 if (v.getValue().equals(value)) // code 1644 return true; 1645 return false; 1646 } 1647 1648 /** 1649 * @return {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1650 */ 1651 public Enumeration<SearchParamType> getTypeElement() { 1652 if (this.type == null) 1653 if (Configuration.errorOnAutoCreate()) 1654 throw new Error("Attempt to auto-create SearchParameter.type"); 1655 else if (Configuration.doAutoCreate()) 1656 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1657 return this.type; 1658 } 1659 1660 public boolean hasTypeElement() { 1661 return this.type != null && !this.type.isEmpty(); 1662 } 1663 1664 public boolean hasType() { 1665 return this.type != null && !this.type.isEmpty(); 1666 } 1667 1668 /** 1669 * @param value {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1670 */ 1671 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1672 this.type = value; 1673 return this; 1674 } 1675 1676 /** 1677 * @return The type of value a search parameter refers to, and how the content is interpreted. 1678 */ 1679 public SearchParamType getType() { 1680 return this.type == null ? null : this.type.getValue(); 1681 } 1682 1683 /** 1684 * @param value The type of value a search parameter refers to, and how the content is interpreted. 1685 */ 1686 public SearchParameter setType(SearchParamType value) { 1687 if (this.type == null) 1688 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1689 this.type.setValue(value); 1690 return this; 1691 } 1692 1693 /** 1694 * @return {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 1695 */ 1696 public UriType getDerivedFromElement() { 1697 if (this.derivedFrom == null) 1698 if (Configuration.errorOnAutoCreate()) 1699 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 1700 else if (Configuration.doAutoCreate()) 1701 this.derivedFrom = new UriType(); // bb 1702 return this.derivedFrom; 1703 } 1704 1705 public boolean hasDerivedFromElement() { 1706 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1707 } 1708 1709 public boolean hasDerivedFrom() { 1710 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1711 } 1712 1713 /** 1714 * @param value {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 1715 */ 1716 public SearchParameter setDerivedFromElement(UriType value) { 1717 this.derivedFrom = value; 1718 return this; 1719 } 1720 1721 /** 1722 * @return Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1723 */ 1724 public String getDerivedFrom() { 1725 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 1726 } 1727 1728 /** 1729 * @param value Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1730 */ 1731 public SearchParameter setDerivedFrom(String value) { 1732 if (Utilities.noString(value)) 1733 this.derivedFrom = null; 1734 else { 1735 if (this.derivedFrom == null) 1736 this.derivedFrom = new UriType(); 1737 this.derivedFrom.setValue(value); 1738 } 1739 return this; 1740 } 1741 1742 /** 1743 * @return {@link #description} (A free text natural language description of the search parameter from a consumer's perspective. and how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1744 */ 1745 public MarkdownType getDescriptionElement() { 1746 if (this.description == null) 1747 if (Configuration.errorOnAutoCreate()) 1748 throw new Error("Attempt to auto-create SearchParameter.description"); 1749 else if (Configuration.doAutoCreate()) 1750 this.description = new MarkdownType(); // bb 1751 return this.description; 1752 } 1753 1754 public boolean hasDescriptionElement() { 1755 return this.description != null && !this.description.isEmpty(); 1756 } 1757 1758 public boolean hasDescription() { 1759 return this.description != null && !this.description.isEmpty(); 1760 } 1761 1762 /** 1763 * @param value {@link #description} (A free text natural language description of the search parameter from a consumer's perspective. and how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1764 */ 1765 public SearchParameter setDescriptionElement(MarkdownType value) { 1766 this.description = value; 1767 return this; 1768 } 1769 1770 /** 1771 * @return A free text natural language description of the search parameter from a consumer's perspective. and how it used. 1772 */ 1773 public String getDescription() { 1774 return this.description == null ? null : this.description.getValue(); 1775 } 1776 1777 /** 1778 * @param value A free text natural language description of the search parameter from a consumer's perspective. and how it used. 1779 */ 1780 public SearchParameter setDescription(String value) { 1781 if (this.description == null) 1782 this.description = new MarkdownType(); 1783 this.description.setValue(value); 1784 return this; 1785 } 1786 1787 /** 1788 * @return {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1789 */ 1790 public StringType getExpressionElement() { 1791 if (this.expression == null) 1792 if (Configuration.errorOnAutoCreate()) 1793 throw new Error("Attempt to auto-create SearchParameter.expression"); 1794 else if (Configuration.doAutoCreate()) 1795 this.expression = new StringType(); // bb 1796 return this.expression; 1797 } 1798 1799 public boolean hasExpressionElement() { 1800 return this.expression != null && !this.expression.isEmpty(); 1801 } 1802 1803 public boolean hasExpression() { 1804 return this.expression != null && !this.expression.isEmpty(); 1805 } 1806 1807 /** 1808 * @param value {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1809 */ 1810 public SearchParameter setExpressionElement(StringType value) { 1811 this.expression = value; 1812 return this; 1813 } 1814 1815 /** 1816 * @return A FHIRPath expression that returns a set of elements for the search parameter. 1817 */ 1818 public String getExpression() { 1819 return this.expression == null ? null : this.expression.getValue(); 1820 } 1821 1822 /** 1823 * @param value A FHIRPath expression that returns a set of elements for the search parameter. 1824 */ 1825 public SearchParameter setExpression(String value) { 1826 if (Utilities.noString(value)) 1827 this.expression = null; 1828 else { 1829 if (this.expression == null) 1830 this.expression = new StringType(); 1831 this.expression.setValue(value); 1832 } 1833 return this; 1834 } 1835 1836 /** 1837 * @return {@link #xpath} (An XPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 1838 */ 1839 public StringType getXpathElement() { 1840 if (this.xpath == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create SearchParameter.xpath"); 1843 else if (Configuration.doAutoCreate()) 1844 this.xpath = new StringType(); // bb 1845 return this.xpath; 1846 } 1847 1848 public boolean hasXpathElement() { 1849 return this.xpath != null && !this.xpath.isEmpty(); 1850 } 1851 1852 public boolean hasXpath() { 1853 return this.xpath != null && !this.xpath.isEmpty(); 1854 } 1855 1856 /** 1857 * @param value {@link #xpath} (An XPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 1858 */ 1859 public SearchParameter setXpathElement(StringType value) { 1860 this.xpath = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return An XPath expression that returns a set of elements for the search parameter. 1866 */ 1867 public String getXpath() { 1868 return this.xpath == null ? null : this.xpath.getValue(); 1869 } 1870 1871 /** 1872 * @param value An XPath expression that returns a set of elements for the search parameter. 1873 */ 1874 public SearchParameter setXpath(String value) { 1875 if (Utilities.noString(value)) 1876 this.xpath = null; 1877 else { 1878 if (this.xpath == null) 1879 this.xpath = new StringType(); 1880 this.xpath.setValue(value); 1881 } 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #xpathUsage} (How the search parameter relates to the set of elements returned by evaluating the xpath query.). This is the underlying object with id, value and extensions. The accessor "getXpathUsage" gives direct access to the value 1887 */ 1888 public Enumeration<XPathUsageType> getXpathUsageElement() { 1889 if (this.xpathUsage == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 1892 else if (Configuration.doAutoCreate()) 1893 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 1894 return this.xpathUsage; 1895 } 1896 1897 public boolean hasXpathUsageElement() { 1898 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1899 } 1900 1901 public boolean hasXpathUsage() { 1902 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1903 } 1904 1905 /** 1906 * @param value {@link #xpathUsage} (How the search parameter relates to the set of elements returned by evaluating the xpath query.). This is the underlying object with id, value and extensions. The accessor "getXpathUsage" gives direct access to the value 1907 */ 1908 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 1909 this.xpathUsage = value; 1910 return this; 1911 } 1912 1913 /** 1914 * @return How the search parameter relates to the set of elements returned by evaluating the xpath query. 1915 */ 1916 public XPathUsageType getXpathUsage() { 1917 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 1918 } 1919 1920 /** 1921 * @param value How the search parameter relates to the set of elements returned by evaluating the xpath query. 1922 */ 1923 public SearchParameter setXpathUsage(XPathUsageType value) { 1924 if (value == null) 1925 this.xpathUsage = null; 1926 else { 1927 if (this.xpathUsage == null) 1928 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 1929 this.xpathUsage.setValue(value); 1930 } 1931 return this; 1932 } 1933 1934 /** 1935 * @return {@link #target} (Types of resource (if a resource is referenced).) 1936 */ 1937 public List<CodeType> getTarget() { 1938 if (this.target == null) 1939 this.target = new ArrayList<CodeType>(); 1940 return this.target; 1941 } 1942 1943 /** 1944 * @return Returns a reference to <code>this</code> for easy method chaining 1945 */ 1946 public SearchParameter setTarget(List<CodeType> theTarget) { 1947 this.target = theTarget; 1948 return this; 1949 } 1950 1951 public boolean hasTarget() { 1952 if (this.target == null) 1953 return false; 1954 for (CodeType item : this.target) 1955 if (!item.isEmpty()) 1956 return true; 1957 return false; 1958 } 1959 1960 /** 1961 * @return {@link #target} (Types of resource (if a resource is referenced).) 1962 */ 1963 public CodeType addTargetElement() {//2 1964 CodeType t = new CodeType(); 1965 if (this.target == null) 1966 this.target = new ArrayList<CodeType>(); 1967 this.target.add(t); 1968 return t; 1969 } 1970 1971 /** 1972 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1973 */ 1974 public SearchParameter addTarget(String value) { //1 1975 CodeType t = new CodeType(); 1976 t.setValue(value); 1977 if (this.target == null) 1978 this.target = new ArrayList<CodeType>(); 1979 this.target.add(t); 1980 return this; 1981 } 1982 1983 /** 1984 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1985 */ 1986 public boolean hasTarget(String value) { 1987 if (this.target == null) 1988 return false; 1989 for (CodeType v : this.target) 1990 if (v.getValue().equals(value)) // code 1991 return true; 1992 return false; 1993 } 1994 1995 /** 1996 * @return {@link #comparator} (Comparators supported for the search parameter.) 1997 */ 1998 public List<Enumeration<SearchComparator>> getComparator() { 1999 if (this.comparator == null) 2000 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2001 return this.comparator; 2002 } 2003 2004 /** 2005 * @return Returns a reference to <code>this</code> for easy method chaining 2006 */ 2007 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2008 this.comparator = theComparator; 2009 return this; 2010 } 2011 2012 public boolean hasComparator() { 2013 if (this.comparator == null) 2014 return false; 2015 for (Enumeration<SearchComparator> item : this.comparator) 2016 if (!item.isEmpty()) 2017 return true; 2018 return false; 2019 } 2020 2021 /** 2022 * @return {@link #comparator} (Comparators supported for the search parameter.) 2023 */ 2024 public Enumeration<SearchComparator> addComparatorElement() {//2 2025 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2026 if (this.comparator == null) 2027 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2028 this.comparator.add(t); 2029 return t; 2030 } 2031 2032 /** 2033 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2034 */ 2035 public SearchParameter addComparator(SearchComparator value) { //1 2036 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2037 t.setValue(value); 2038 if (this.comparator == null) 2039 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2040 this.comparator.add(t); 2041 return this; 2042 } 2043 2044 /** 2045 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2046 */ 2047 public boolean hasComparator(SearchComparator value) { 2048 if (this.comparator == null) 2049 return false; 2050 for (Enumeration<SearchComparator> v : this.comparator) 2051 if (v.getValue().equals(value)) // code 2052 return true; 2053 return false; 2054 } 2055 2056 /** 2057 * @return {@link #modifier} (A modifier supported for the search parameter.) 2058 */ 2059 public List<Enumeration<SearchModifierCode>> getModifier() { 2060 if (this.modifier == null) 2061 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2062 return this.modifier; 2063 } 2064 2065 /** 2066 * @return Returns a reference to <code>this</code> for easy method chaining 2067 */ 2068 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2069 this.modifier = theModifier; 2070 return this; 2071 } 2072 2073 public boolean hasModifier() { 2074 if (this.modifier == null) 2075 return false; 2076 for (Enumeration<SearchModifierCode> item : this.modifier) 2077 if (!item.isEmpty()) 2078 return true; 2079 return false; 2080 } 2081 2082 /** 2083 * @return {@link #modifier} (A modifier supported for the search parameter.) 2084 */ 2085 public Enumeration<SearchModifierCode> addModifierElement() {//2 2086 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2087 if (this.modifier == null) 2088 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2089 this.modifier.add(t); 2090 return t; 2091 } 2092 2093 /** 2094 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2095 */ 2096 public SearchParameter addModifier(SearchModifierCode value) { //1 2097 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2098 t.setValue(value); 2099 if (this.modifier == null) 2100 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2101 this.modifier.add(t); 2102 return this; 2103 } 2104 2105 /** 2106 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2107 */ 2108 public boolean hasModifier(SearchModifierCode value) { 2109 if (this.modifier == null) 2110 return false; 2111 for (Enumeration<SearchModifierCode> v : this.modifier) 2112 if (v.getValue().equals(value)) // code 2113 return true; 2114 return false; 2115 } 2116 2117 /** 2118 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2119 */ 2120 public List<StringType> getChain() { 2121 if (this.chain == null) 2122 this.chain = new ArrayList<StringType>(); 2123 return this.chain; 2124 } 2125 2126 /** 2127 * @return Returns a reference to <code>this</code> for easy method chaining 2128 */ 2129 public SearchParameter setChain(List<StringType> theChain) { 2130 this.chain = theChain; 2131 return this; 2132 } 2133 2134 public boolean hasChain() { 2135 if (this.chain == null) 2136 return false; 2137 for (StringType item : this.chain) 2138 if (!item.isEmpty()) 2139 return true; 2140 return false; 2141 } 2142 2143 /** 2144 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2145 */ 2146 public StringType addChainElement() {//2 2147 StringType t = new StringType(); 2148 if (this.chain == null) 2149 this.chain = new ArrayList<StringType>(); 2150 this.chain.add(t); 2151 return t; 2152 } 2153 2154 /** 2155 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2156 */ 2157 public SearchParameter addChain(String value) { //1 2158 StringType t = new StringType(); 2159 t.setValue(value); 2160 if (this.chain == null) 2161 this.chain = new ArrayList<StringType>(); 2162 this.chain.add(t); 2163 return this; 2164 } 2165 2166 /** 2167 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2168 */ 2169 public boolean hasChain(String value) { 2170 if (this.chain == null) 2171 return false; 2172 for (StringType v : this.chain) 2173 if (v.getValue().equals(value)) // string 2174 return true; 2175 return false; 2176 } 2177 2178 /** 2179 * @return {@link #component} (Used to define the parts of a composite search parameter.) 2180 */ 2181 public List<SearchParameterComponentComponent> getComponent() { 2182 if (this.component == null) 2183 this.component = new ArrayList<SearchParameterComponentComponent>(); 2184 return this.component; 2185 } 2186 2187 /** 2188 * @return Returns a reference to <code>this</code> for easy method chaining 2189 */ 2190 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2191 this.component = theComponent; 2192 return this; 2193 } 2194 2195 public boolean hasComponent() { 2196 if (this.component == null) 2197 return false; 2198 for (SearchParameterComponentComponent item : this.component) 2199 if (!item.isEmpty()) 2200 return true; 2201 return false; 2202 } 2203 2204 public SearchParameterComponentComponent addComponent() { //3 2205 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2206 if (this.component == null) 2207 this.component = new ArrayList<SearchParameterComponentComponent>(); 2208 this.component.add(t); 2209 return t; 2210 } 2211 2212 public SearchParameter addComponent(SearchParameterComponentComponent t) { //3 2213 if (t == null) 2214 return this; 2215 if (this.component == null) 2216 this.component = new ArrayList<SearchParameterComponentComponent>(); 2217 this.component.add(t); 2218 return this; 2219 } 2220 2221 /** 2222 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist 2223 */ 2224 public SearchParameterComponentComponent getComponentFirstRep() { 2225 if (getComponent().isEmpty()) { 2226 addComponent(); 2227 } 2228 return getComponent().get(0); 2229 } 2230 2231 protected void listChildren(List<Property> children) { 2232 super.listChildren(children); 2233 children.add(new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2234 children.add(new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2235 children.add(new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2236 children.add(new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2237 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2238 children.add(new Property("date", "dateTime", "The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date)); 2239 children.add(new Property("publisher", "string", "The name of the individual or organization that published the search parameter.", 0, 1, publisher)); 2240 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2241 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2242 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2243 children.add(new Property("purpose", "markdown", "Explaination of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2244 children.add(new Property("code", "code", "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, code)); 2245 children.add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base)); 2246 children.add(new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 2247 children.add(new Property("derivedFrom", "uri", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom)); 2248 children.add(new Property("description", "markdown", "A free text natural language description of the search parameter from a consumer's perspective. and how it used.", 0, 1, description)); 2249 children.add(new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2250 children.add(new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath)); 2251 children.add(new Property("xpathUsage", "code", "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, xpathUsage)); 2252 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target)); 2253 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 2254 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2255 children.add(new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain)); 2256 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component)); 2257 } 2258 2259 @Override 2260 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2261 switch (_hash) { 2262 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published. The URL SHOULD include the major version of the search parameter. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2263 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2264 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2265 case -892481550: /*status*/ return new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2266 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2267 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the search parameter was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date); 2268 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the search parameter.", 0, 1, publisher); 2269 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2270 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2271 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2272 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 2273 case 3059181: /*code*/ return new Property("code", "code", "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, code); 2274 case 3016401: /*base*/ return new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base); 2275 case 3575610: /*type*/ return new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 2276 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "uri", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. I.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom); 2277 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the search parameter from a consumer's perspective. and how it used.", 0, 1, description); 2278 case -1795452264: /*expression*/ return new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 2279 case 114256029: /*xpath*/ return new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath); 2280 case 1801322244: /*xpathUsage*/ return new Property("xpathUsage", "code", "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, xpathUsage); 2281 case -880905839: /*target*/ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target); 2282 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 2283 case -615513385: /*modifier*/ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 2284 case 94623425: /*chain*/ return new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain); 2285 case -1399907075: /*component*/ return new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component); 2286 default: return super.getNamedProperty(_hash, _name, _checkValid); 2287 } 2288 2289 } 2290 2291 @Override 2292 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2293 switch (hash) { 2294 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2295 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2296 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2297 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2298 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2299 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2300 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2301 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2302 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2303 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2304 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2305 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2306 case 3016401: /*base*/ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // CodeType 2307 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 2308 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // UriType 2309 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2310 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 2311 case 114256029: /*xpath*/ return this.xpath == null ? new Base[0] : new Base[] {this.xpath}; // StringType 2312 case 1801322244: /*xpathUsage*/ return this.xpathUsage == null ? new Base[0] : new Base[] {this.xpathUsage}; // Enumeration<XPathUsageType> 2313 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // CodeType 2314 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 2315 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 2316 case 94623425: /*chain*/ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 2317 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 2318 default: return super.getProperty(hash, name, checkValid); 2319 } 2320 2321 } 2322 2323 @Override 2324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2325 switch (hash) { 2326 case 116079: // url 2327 this.url = castToUri(value); // UriType 2328 return value; 2329 case 351608024: // version 2330 this.version = castToString(value); // StringType 2331 return value; 2332 case 3373707: // name 2333 this.name = castToString(value); // StringType 2334 return value; 2335 case -892481550: // status 2336 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2337 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2338 return value; 2339 case -404562712: // experimental 2340 this.experimental = castToBoolean(value); // BooleanType 2341 return value; 2342 case 3076014: // date 2343 this.date = castToDateTime(value); // DateTimeType 2344 return value; 2345 case 1447404028: // publisher 2346 this.publisher = castToString(value); // StringType 2347 return value; 2348 case 951526432: // contact 2349 this.getContact().add(castToContactDetail(value)); // ContactDetail 2350 return value; 2351 case -669707736: // useContext 2352 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2353 return value; 2354 case -507075711: // jurisdiction 2355 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2356 return value; 2357 case -220463842: // purpose 2358 this.purpose = castToMarkdown(value); // MarkdownType 2359 return value; 2360 case 3059181: // code 2361 this.code = castToCode(value); // CodeType 2362 return value; 2363 case 3016401: // base 2364 this.getBase().add(castToCode(value)); // CodeType 2365 return value; 2366 case 3575610: // type 2367 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 2368 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2369 return value; 2370 case 1077922663: // derivedFrom 2371 this.derivedFrom = castToUri(value); // UriType 2372 return value; 2373 case -1724546052: // description 2374 this.description = castToMarkdown(value); // MarkdownType 2375 return value; 2376 case -1795452264: // expression 2377 this.expression = castToString(value); // StringType 2378 return value; 2379 case 114256029: // xpath 2380 this.xpath = castToString(value); // StringType 2381 return value; 2382 case 1801322244: // xpathUsage 2383 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 2384 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 2385 return value; 2386 case -880905839: // target 2387 this.getTarget().add(castToCode(value)); // CodeType 2388 return value; 2389 case -844673834: // comparator 2390 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 2391 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 2392 return value; 2393 case -615513385: // modifier 2394 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 2395 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 2396 return value; 2397 case 94623425: // chain 2398 this.getChain().add(castToString(value)); // StringType 2399 return value; 2400 case -1399907075: // component 2401 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 2402 return value; 2403 default: return super.setProperty(hash, name, value); 2404 } 2405 2406 } 2407 2408 @Override 2409 public Base setProperty(String name, Base value) throws FHIRException { 2410 if (name.equals("url")) { 2411 this.url = castToUri(value); // UriType 2412 } else if (name.equals("version")) { 2413 this.version = castToString(value); // StringType 2414 } else if (name.equals("name")) { 2415 this.name = castToString(value); // StringType 2416 } else if (name.equals("status")) { 2417 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2418 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2419 } else if (name.equals("experimental")) { 2420 this.experimental = castToBoolean(value); // BooleanType 2421 } else if (name.equals("date")) { 2422 this.date = castToDateTime(value); // DateTimeType 2423 } else if (name.equals("publisher")) { 2424 this.publisher = castToString(value); // StringType 2425 } else if (name.equals("contact")) { 2426 this.getContact().add(castToContactDetail(value)); 2427 } else if (name.equals("useContext")) { 2428 this.getUseContext().add(castToUsageContext(value)); 2429 } else if (name.equals("jurisdiction")) { 2430 this.getJurisdiction().add(castToCodeableConcept(value)); 2431 } else if (name.equals("purpose")) { 2432 this.purpose = castToMarkdown(value); // MarkdownType 2433 } else if (name.equals("code")) { 2434 this.code = castToCode(value); // CodeType 2435 } else if (name.equals("base")) { 2436 this.getBase().add(castToCode(value)); 2437 } else if (name.equals("type")) { 2438 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 2439 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2440 } else if (name.equals("derivedFrom")) { 2441 this.derivedFrom = castToUri(value); // UriType 2442 } else if (name.equals("description")) { 2443 this.description = castToMarkdown(value); // MarkdownType 2444 } else if (name.equals("expression")) { 2445 this.expression = castToString(value); // StringType 2446 } else if (name.equals("xpath")) { 2447 this.xpath = castToString(value); // StringType 2448 } else if (name.equals("xpathUsage")) { 2449 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 2450 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 2451 } else if (name.equals("target")) { 2452 this.getTarget().add(castToCode(value)); 2453 } else if (name.equals("comparator")) { 2454 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 2455 this.getComparator().add((Enumeration) value); 2456 } else if (name.equals("modifier")) { 2457 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 2458 this.getModifier().add((Enumeration) value); 2459 } else if (name.equals("chain")) { 2460 this.getChain().add(castToString(value)); 2461 } else if (name.equals("component")) { 2462 this.getComponent().add((SearchParameterComponentComponent) value); 2463 } else 2464 return super.setProperty(name, value); 2465 return value; 2466 } 2467 2468 @Override 2469 public Base makeProperty(int hash, String name) throws FHIRException { 2470 switch (hash) { 2471 case 116079: return getUrlElement(); 2472 case 351608024: return getVersionElement(); 2473 case 3373707: return getNameElement(); 2474 case -892481550: return getStatusElement(); 2475 case -404562712: return getExperimentalElement(); 2476 case 3076014: return getDateElement(); 2477 case 1447404028: return getPublisherElement(); 2478 case 951526432: return addContact(); 2479 case -669707736: return addUseContext(); 2480 case -507075711: return addJurisdiction(); 2481 case -220463842: return getPurposeElement(); 2482 case 3059181: return getCodeElement(); 2483 case 3016401: return addBaseElement(); 2484 case 3575610: return getTypeElement(); 2485 case 1077922663: return getDerivedFromElement(); 2486 case -1724546052: return getDescriptionElement(); 2487 case -1795452264: return getExpressionElement(); 2488 case 114256029: return getXpathElement(); 2489 case 1801322244: return getXpathUsageElement(); 2490 case -880905839: return addTargetElement(); 2491 case -844673834: return addComparatorElement(); 2492 case -615513385: return addModifierElement(); 2493 case 94623425: return addChainElement(); 2494 case -1399907075: return addComponent(); 2495 default: return super.makeProperty(hash, name); 2496 } 2497 2498 } 2499 2500 @Override 2501 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2502 switch (hash) { 2503 case 116079: /*url*/ return new String[] {"uri"}; 2504 case 351608024: /*version*/ return new String[] {"string"}; 2505 case 3373707: /*name*/ return new String[] {"string"}; 2506 case -892481550: /*status*/ return new String[] {"code"}; 2507 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2508 case 3076014: /*date*/ return new String[] {"dateTime"}; 2509 case 1447404028: /*publisher*/ return new String[] {"string"}; 2510 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2511 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2512 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2513 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2514 case 3059181: /*code*/ return new String[] {"code"}; 2515 case 3016401: /*base*/ return new String[] {"code"}; 2516 case 3575610: /*type*/ return new String[] {"code"}; 2517 case 1077922663: /*derivedFrom*/ return new String[] {"uri"}; 2518 case -1724546052: /*description*/ return new String[] {"markdown"}; 2519 case -1795452264: /*expression*/ return new String[] {"string"}; 2520 case 114256029: /*xpath*/ return new String[] {"string"}; 2521 case 1801322244: /*xpathUsage*/ return new String[] {"code"}; 2522 case -880905839: /*target*/ return new String[] {"code"}; 2523 case -844673834: /*comparator*/ return new String[] {"code"}; 2524 case -615513385: /*modifier*/ return new String[] {"code"}; 2525 case 94623425: /*chain*/ return new String[] {"string"}; 2526 case -1399907075: /*component*/ return new String[] {}; 2527 default: return super.getTypesForProperty(hash, name); 2528 } 2529 2530 } 2531 2532 @Override 2533 public Base addChild(String name) throws FHIRException { 2534 if (name.equals("url")) { 2535 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 2536 } 2537 else if (name.equals("version")) { 2538 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 2539 } 2540 else if (name.equals("name")) { 2541 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 2542 } 2543 else if (name.equals("status")) { 2544 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 2545 } 2546 else if (name.equals("experimental")) { 2547 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 2548 } 2549 else if (name.equals("date")) { 2550 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 2551 } 2552 else if (name.equals("publisher")) { 2553 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 2554 } 2555 else if (name.equals("contact")) { 2556 return addContact(); 2557 } 2558 else if (name.equals("useContext")) { 2559 return addUseContext(); 2560 } 2561 else if (name.equals("jurisdiction")) { 2562 return addJurisdiction(); 2563 } 2564 else if (name.equals("purpose")) { 2565 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 2566 } 2567 else if (name.equals("code")) { 2568 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 2569 } 2570 else if (name.equals("base")) { 2571 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 2572 } 2573 else if (name.equals("type")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 2575 } 2576 else if (name.equals("derivedFrom")) { 2577 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 2578 } 2579 else if (name.equals("description")) { 2580 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 2581 } 2582 else if (name.equals("expression")) { 2583 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 2584 } 2585 else if (name.equals("xpath")) { 2586 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 2587 } 2588 else if (name.equals("xpathUsage")) { 2589 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 2590 } 2591 else if (name.equals("target")) { 2592 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 2593 } 2594 else if (name.equals("comparator")) { 2595 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 2596 } 2597 else if (name.equals("modifier")) { 2598 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 2599 } 2600 else if (name.equals("chain")) { 2601 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 2602 } 2603 else if (name.equals("component")) { 2604 return addComponent(); 2605 } 2606 else 2607 return super.addChild(name); 2608 } 2609 2610 public String fhirType() { 2611 return "SearchParameter"; 2612 2613 } 2614 2615 public SearchParameter copy() { 2616 SearchParameter dst = new SearchParameter(); 2617 copyValues(dst); 2618 dst.url = url == null ? null : url.copy(); 2619 dst.version = version == null ? null : version.copy(); 2620 dst.name = name == null ? null : name.copy(); 2621 dst.status = status == null ? null : status.copy(); 2622 dst.experimental = experimental == null ? null : experimental.copy(); 2623 dst.date = date == null ? null : date.copy(); 2624 dst.publisher = publisher == null ? null : publisher.copy(); 2625 if (contact != null) { 2626 dst.contact = new ArrayList<ContactDetail>(); 2627 for (ContactDetail i : contact) 2628 dst.contact.add(i.copy()); 2629 }; 2630 if (useContext != null) { 2631 dst.useContext = new ArrayList<UsageContext>(); 2632 for (UsageContext i : useContext) 2633 dst.useContext.add(i.copy()); 2634 }; 2635 if (jurisdiction != null) { 2636 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2637 for (CodeableConcept i : jurisdiction) 2638 dst.jurisdiction.add(i.copy()); 2639 }; 2640 dst.purpose = purpose == null ? null : purpose.copy(); 2641 dst.code = code == null ? null : code.copy(); 2642 if (base != null) { 2643 dst.base = new ArrayList<CodeType>(); 2644 for (CodeType i : base) 2645 dst.base.add(i.copy()); 2646 }; 2647 dst.type = type == null ? null : type.copy(); 2648 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 2649 dst.description = description == null ? null : description.copy(); 2650 dst.expression = expression == null ? null : expression.copy(); 2651 dst.xpath = xpath == null ? null : xpath.copy(); 2652 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 2653 if (target != null) { 2654 dst.target = new ArrayList<CodeType>(); 2655 for (CodeType i : target) 2656 dst.target.add(i.copy()); 2657 }; 2658 if (comparator != null) { 2659 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2660 for (Enumeration<SearchComparator> i : comparator) 2661 dst.comparator.add(i.copy()); 2662 }; 2663 if (modifier != null) { 2664 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2665 for (Enumeration<SearchModifierCode> i : modifier) 2666 dst.modifier.add(i.copy()); 2667 }; 2668 if (chain != null) { 2669 dst.chain = new ArrayList<StringType>(); 2670 for (StringType i : chain) 2671 dst.chain.add(i.copy()); 2672 }; 2673 if (component != null) { 2674 dst.component = new ArrayList<SearchParameterComponentComponent>(); 2675 for (SearchParameterComponentComponent i : component) 2676 dst.component.add(i.copy()); 2677 }; 2678 return dst; 2679 } 2680 2681 protected SearchParameter typedCopy() { 2682 return copy(); 2683 } 2684 2685 @Override 2686 public boolean equalsDeep(Base other_) { 2687 if (!super.equalsDeep(other_)) 2688 return false; 2689 if (!(other_ instanceof SearchParameter)) 2690 return false; 2691 SearchParameter o = (SearchParameter) other_; 2692 return compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) 2693 && compareDeep(type, o.type, true) && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(expression, o.expression, true) 2694 && compareDeep(xpath, o.xpath, true) && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true) 2695 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true) && compareDeep(chain, o.chain, true) 2696 && compareDeep(component, o.component, true); 2697 } 2698 2699 @Override 2700 public boolean equalsShallow(Base other_) { 2701 if (!super.equalsShallow(other_)) 2702 return false; 2703 if (!(other_ instanceof SearchParameter)) 2704 return false; 2705 SearchParameter o = (SearchParameter) other_; 2706 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) && compareValues(base, o.base, true) 2707 && compareValues(type, o.type, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(expression, o.expression, true) 2708 && compareValues(xpath, o.xpath, true) && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true) 2709 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true) && compareValues(chain, o.chain, true) 2710 ; 2711 } 2712 2713 public boolean isEmpty() { 2714 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, code, base, type 2715 , derivedFrom, expression, xpath, xpathUsage, target, comparator, modifier, chain 2716 , component); 2717 } 2718 2719 @Override 2720 public ResourceType getResourceType() { 2721 return ResourceType.SearchParameter; 2722 } 2723 2724 /** 2725 * Search parameter: <b>date</b> 2726 * <p> 2727 * Description: <b>The search parameter publication date</b><br> 2728 * Type: <b>date</b><br> 2729 * Path: <b>SearchParameter.date</b><br> 2730 * </p> 2731 */ 2732 @SearchParamDefinition(name="date", path="SearchParameter.date", description="The search parameter publication date", type="date" ) 2733 public static final String SP_DATE = "date"; 2734 /** 2735 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2736 * <p> 2737 * Description: <b>The search parameter publication date</b><br> 2738 * Type: <b>date</b><br> 2739 * Path: <b>SearchParameter.date</b><br> 2740 * </p> 2741 */ 2742 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2743 2744 /** 2745 * Search parameter: <b>code</b> 2746 * <p> 2747 * Description: <b>Code used in URL</b><br> 2748 * Type: <b>token</b><br> 2749 * Path: <b>SearchParameter.code</b><br> 2750 * </p> 2751 */ 2752 @SearchParamDefinition(name="code", path="SearchParameter.code", description="Code used in URL", type="token" ) 2753 public static final String SP_CODE = "code"; 2754 /** 2755 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2756 * <p> 2757 * Description: <b>Code used in URL</b><br> 2758 * Type: <b>token</b><br> 2759 * Path: <b>SearchParameter.code</b><br> 2760 * </p> 2761 */ 2762 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2763 2764 /** 2765 * Search parameter: <b>jurisdiction</b> 2766 * <p> 2767 * Description: <b>Intended jurisdiction for the search parameter</b><br> 2768 * Type: <b>token</b><br> 2769 * Path: <b>SearchParameter.jurisdiction</b><br> 2770 * </p> 2771 */ 2772 @SearchParamDefinition(name="jurisdiction", path="SearchParameter.jurisdiction", description="Intended jurisdiction for the search parameter", type="token" ) 2773 public static final String SP_JURISDICTION = "jurisdiction"; 2774 /** 2775 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2776 * <p> 2777 * Description: <b>Intended jurisdiction for the search parameter</b><br> 2778 * Type: <b>token</b><br> 2779 * Path: <b>SearchParameter.jurisdiction</b><br> 2780 * </p> 2781 */ 2782 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2783 2784 /** 2785 * Search parameter: <b>description</b> 2786 * <p> 2787 * Description: <b>The description of the search parameter</b><br> 2788 * Type: <b>string</b><br> 2789 * Path: <b>SearchParameter.description</b><br> 2790 * </p> 2791 */ 2792 @SearchParamDefinition(name="description", path="SearchParameter.description", description="The description of the search parameter", type="string" ) 2793 public static final String SP_DESCRIPTION = "description"; 2794 /** 2795 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2796 * <p> 2797 * Description: <b>The description of the search parameter</b><br> 2798 * Type: <b>string</b><br> 2799 * Path: <b>SearchParameter.description</b><br> 2800 * </p> 2801 */ 2802 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2803 2804 /** 2805 * Search parameter: <b>derived-from</b> 2806 * <p> 2807 * Description: <b>Original Definition for the search parameter</b><br> 2808 * Type: <b>uri</b><br> 2809 * Path: <b>SearchParameter.derivedFrom</b><br> 2810 * </p> 2811 */ 2812 @SearchParamDefinition(name="derived-from", path="SearchParameter.derivedFrom", description="Original Definition for the search parameter", type="uri" ) 2813 public static final String SP_DERIVED_FROM = "derived-from"; 2814 /** 2815 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2816 * <p> 2817 * Description: <b>Original Definition for the search parameter</b><br> 2818 * Type: <b>uri</b><br> 2819 * Path: <b>SearchParameter.derivedFrom</b><br> 2820 * </p> 2821 */ 2822 public static final ca.uhn.fhir.rest.gclient.UriClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DERIVED_FROM); 2823 2824 /** 2825 * Search parameter: <b>type</b> 2826 * <p> 2827 * Description: <b>number | date | string | token | reference | composite | quantity | uri</b><br> 2828 * Type: <b>token</b><br> 2829 * Path: <b>SearchParameter.type</b><br> 2830 * </p> 2831 */ 2832 @SearchParamDefinition(name="type", path="SearchParameter.type", description="number | date | string | token | reference | composite | quantity | uri", type="token" ) 2833 public static final String SP_TYPE = "type"; 2834 /** 2835 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2836 * <p> 2837 * Description: <b>number | date | string | token | reference | composite | quantity | uri</b><br> 2838 * Type: <b>token</b><br> 2839 * Path: <b>SearchParameter.type</b><br> 2840 * </p> 2841 */ 2842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2843 2844 /** 2845 * Search parameter: <b>version</b> 2846 * <p> 2847 * Description: <b>The business version of the search parameter</b><br> 2848 * Type: <b>token</b><br> 2849 * Path: <b>SearchParameter.version</b><br> 2850 * </p> 2851 */ 2852 @SearchParamDefinition(name="version", path="SearchParameter.version", description="The business version of the search parameter", type="token" ) 2853 public static final String SP_VERSION = "version"; 2854 /** 2855 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2856 * <p> 2857 * Description: <b>The business version of the search parameter</b><br> 2858 * Type: <b>token</b><br> 2859 * Path: <b>SearchParameter.version</b><br> 2860 * </p> 2861 */ 2862 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2863 2864 /** 2865 * Search parameter: <b>url</b> 2866 * <p> 2867 * Description: <b>The uri that identifies the search parameter</b><br> 2868 * Type: <b>uri</b><br> 2869 * Path: <b>SearchParameter.url</b><br> 2870 * </p> 2871 */ 2872 @SearchParamDefinition(name="url", path="SearchParameter.url", description="The uri that identifies the search parameter", type="uri" ) 2873 public static final String SP_URL = "url"; 2874 /** 2875 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2876 * <p> 2877 * Description: <b>The uri that identifies the search parameter</b><br> 2878 * Type: <b>uri</b><br> 2879 * Path: <b>SearchParameter.url</b><br> 2880 * </p> 2881 */ 2882 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2883 2884 /** 2885 * Search parameter: <b>target</b> 2886 * <p> 2887 * Description: <b>Types of resource (if a resource reference)</b><br> 2888 * Type: <b>token</b><br> 2889 * Path: <b>SearchParameter.target</b><br> 2890 * </p> 2891 */ 2892 @SearchParamDefinition(name="target", path="SearchParameter.target", description="Types of resource (if a resource reference)", type="token" ) 2893 public static final String SP_TARGET = "target"; 2894 /** 2895 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2896 * <p> 2897 * Description: <b>Types of resource (if a resource reference)</b><br> 2898 * Type: <b>token</b><br> 2899 * Path: <b>SearchParameter.target</b><br> 2900 * </p> 2901 */ 2902 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET); 2903 2904 /** 2905 * Search parameter: <b>component</b> 2906 * <p> 2907 * Description: <b>Defines how the part works</b><br> 2908 * Type: <b>reference</b><br> 2909 * Path: <b>SearchParameter.component.definition</b><br> 2910 * </p> 2911 */ 2912 @SearchParamDefinition(name="component", path="SearchParameter.component.definition", description="Defines how the part works", type="reference", target={SearchParameter.class } ) 2913 public static final String SP_COMPONENT = "component"; 2914 /** 2915 * <b>Fluent Client</b> search parameter constant for <b>component</b> 2916 * <p> 2917 * Description: <b>Defines how the part works</b><br> 2918 * Type: <b>reference</b><br> 2919 * Path: <b>SearchParameter.component.definition</b><br> 2920 * </p> 2921 */ 2922 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT); 2923 2924/** 2925 * Constant for fluent queries to be used to add include statements. Specifies 2926 * the path value of "<b>SearchParameter:component</b>". 2927 */ 2928 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include("SearchParameter:component").toLocked(); 2929 2930 /** 2931 * Search parameter: <b>name</b> 2932 * <p> 2933 * Description: <b>Computationally friendly name of the search parameter</b><br> 2934 * Type: <b>string</b><br> 2935 * Path: <b>SearchParameter.name</b><br> 2936 * </p> 2937 */ 2938 @SearchParamDefinition(name="name", path="SearchParameter.name", description="Computationally friendly name of the search parameter", type="string" ) 2939 public static final String SP_NAME = "name"; 2940 /** 2941 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2942 * <p> 2943 * Description: <b>Computationally friendly name of the search parameter</b><br> 2944 * Type: <b>string</b><br> 2945 * Path: <b>SearchParameter.name</b><br> 2946 * </p> 2947 */ 2948 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2949 2950 /** 2951 * Search parameter: <b>publisher</b> 2952 * <p> 2953 * Description: <b>Name of the publisher of the search parameter</b><br> 2954 * Type: <b>string</b><br> 2955 * Path: <b>SearchParameter.publisher</b><br> 2956 * </p> 2957 */ 2958 @SearchParamDefinition(name="publisher", path="SearchParameter.publisher", description="Name of the publisher of the search parameter", type="string" ) 2959 public static final String SP_PUBLISHER = "publisher"; 2960 /** 2961 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2962 * <p> 2963 * Description: <b>Name of the publisher of the search parameter</b><br> 2964 * Type: <b>string</b><br> 2965 * Path: <b>SearchParameter.publisher</b><br> 2966 * </p> 2967 */ 2968 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2969 2970 /** 2971 * Search parameter: <b>status</b> 2972 * <p> 2973 * Description: <b>The current status of the search parameter</b><br> 2974 * Type: <b>token</b><br> 2975 * Path: <b>SearchParameter.status</b><br> 2976 * </p> 2977 */ 2978 @SearchParamDefinition(name="status", path="SearchParameter.status", description="The current status of the search parameter", type="token" ) 2979 public static final String SP_STATUS = "status"; 2980 /** 2981 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2982 * <p> 2983 * Description: <b>The current status of the search parameter</b><br> 2984 * Type: <b>token</b><br> 2985 * Path: <b>SearchParameter.status</b><br> 2986 * </p> 2987 */ 2988 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2989 2990 /** 2991 * Search parameter: <b>base</b> 2992 * <p> 2993 * Description: <b>The resource type(s) this search parameter applies to</b><br> 2994 * Type: <b>token</b><br> 2995 * Path: <b>SearchParameter.base</b><br> 2996 * </p> 2997 */ 2998 @SearchParamDefinition(name="base", path="SearchParameter.base", description="The resource type(s) this search parameter applies to", type="token" ) 2999 public static final String SP_BASE = "base"; 3000 /** 3001 * <b>Fluent Client</b> search parameter constant for <b>base</b> 3002 * <p> 3003 * Description: <b>The resource type(s) this search parameter applies to</b><br> 3004 * Type: <b>token</b><br> 3005 * Path: <b>SearchParameter.base</b><br> 3006 * </p> 3007 */ 3008 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE); 3009 3010 3011}