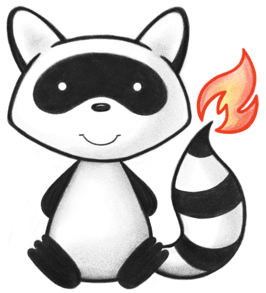
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Raw data describing a biological sequence. 049 */ 050@ResourceDef(name="Sequence", profile="http://hl7.org/fhir/Profile/Sequence") 051public class Sequence extends DomainResource { 052 053 public enum SequenceType { 054 /** 055 * Amino acid sequence 056 */ 057 AA, 058 /** 059 * DNA Sequence 060 */ 061 DNA, 062 /** 063 * RNA Sequence 064 */ 065 RNA, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static SequenceType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("aa".equals(codeString)) 074 return AA; 075 if ("dna".equals(codeString)) 076 return DNA; 077 if ("rna".equals(codeString)) 078 return RNA; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case AA: return "aa"; 087 case DNA: return "dna"; 088 case RNA: return "rna"; 089 case NULL: return null; 090 default: return "?"; 091 } 092 } 093 public String getSystem() { 094 switch (this) { 095 case AA: return "http://hl7.org/fhir/sequence-type"; 096 case DNA: return "http://hl7.org/fhir/sequence-type"; 097 case RNA: return "http://hl7.org/fhir/sequence-type"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getDefinition() { 103 switch (this) { 104 case AA: return "Amino acid sequence"; 105 case DNA: return "DNA Sequence"; 106 case RNA: return "RNA Sequence"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDisplay() { 112 switch (this) { 113 case AA: return "AA Sequence"; 114 case DNA: return "DNA Sequence"; 115 case RNA: return "RNA Sequence"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 } 121 122 public static class SequenceTypeEnumFactory implements EnumFactory<SequenceType> { 123 public SequenceType fromCode(String codeString) throws IllegalArgumentException { 124 if (codeString == null || "".equals(codeString)) 125 if (codeString == null || "".equals(codeString)) 126 return null; 127 if ("aa".equals(codeString)) 128 return SequenceType.AA; 129 if ("dna".equals(codeString)) 130 return SequenceType.DNA; 131 if ("rna".equals(codeString)) 132 return SequenceType.RNA; 133 throw new IllegalArgumentException("Unknown SequenceType code '"+codeString+"'"); 134 } 135 public Enumeration<SequenceType> fromType(PrimitiveType<?> code) throws FHIRException { 136 if (code == null) 137 return null; 138 if (code.isEmpty()) 139 return new Enumeration<SequenceType>(this); 140 String codeString = code.asStringValue(); 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("aa".equals(codeString)) 144 return new Enumeration<SequenceType>(this, SequenceType.AA); 145 if ("dna".equals(codeString)) 146 return new Enumeration<SequenceType>(this, SequenceType.DNA); 147 if ("rna".equals(codeString)) 148 return new Enumeration<SequenceType>(this, SequenceType.RNA); 149 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 150 } 151 public String toCode(SequenceType code) { 152 if (code == SequenceType.AA) 153 return "aa"; 154 if (code == SequenceType.DNA) 155 return "dna"; 156 if (code == SequenceType.RNA) 157 return "rna"; 158 return "?"; 159 } 160 public String toSystem(SequenceType code) { 161 return code.getSystem(); 162 } 163 } 164 165 public enum QualityType { 166 /** 167 * INDEL Comparison 168 */ 169 INDEL, 170 /** 171 * SNP Comparison 172 */ 173 SNP, 174 /** 175 * UNKNOWN Comparison 176 */ 177 UNKNOWN, 178 /** 179 * added to help the parsers with the generic types 180 */ 181 NULL; 182 public static QualityType fromCode(String codeString) throws FHIRException { 183 if (codeString == null || "".equals(codeString)) 184 return null; 185 if ("indel".equals(codeString)) 186 return INDEL; 187 if ("snp".equals(codeString)) 188 return SNP; 189 if ("unknown".equals(codeString)) 190 return UNKNOWN; 191 if (Configuration.isAcceptInvalidEnums()) 192 return null; 193 else 194 throw new FHIRException("Unknown QualityType code '"+codeString+"'"); 195 } 196 public String toCode() { 197 switch (this) { 198 case INDEL: return "indel"; 199 case SNP: return "snp"; 200 case UNKNOWN: return "unknown"; 201 case NULL: return null; 202 default: return "?"; 203 } 204 } 205 public String getSystem() { 206 switch (this) { 207 case INDEL: return "http://hl7.org/fhir/quality-type"; 208 case SNP: return "http://hl7.org/fhir/quality-type"; 209 case UNKNOWN: return "http://hl7.org/fhir/quality-type"; 210 case NULL: return null; 211 default: return "?"; 212 } 213 } 214 public String getDefinition() { 215 switch (this) { 216 case INDEL: return "INDEL Comparison"; 217 case SNP: return "SNP Comparison"; 218 case UNKNOWN: return "UNKNOWN Comparison"; 219 case NULL: return null; 220 default: return "?"; 221 } 222 } 223 public String getDisplay() { 224 switch (this) { 225 case INDEL: return "INDEL Comparison"; 226 case SNP: return "SNP Comparison"; 227 case UNKNOWN: return "UNKNOWN Comparison"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 } 233 234 public static class QualityTypeEnumFactory implements EnumFactory<QualityType> { 235 public QualityType fromCode(String codeString) throws IllegalArgumentException { 236 if (codeString == null || "".equals(codeString)) 237 if (codeString == null || "".equals(codeString)) 238 return null; 239 if ("indel".equals(codeString)) 240 return QualityType.INDEL; 241 if ("snp".equals(codeString)) 242 return QualityType.SNP; 243 if ("unknown".equals(codeString)) 244 return QualityType.UNKNOWN; 245 throw new IllegalArgumentException("Unknown QualityType code '"+codeString+"'"); 246 } 247 public Enumeration<QualityType> fromType(PrimitiveType<?> code) throws FHIRException { 248 if (code == null) 249 return null; 250 if (code.isEmpty()) 251 return new Enumeration<QualityType>(this); 252 String codeString = code.asStringValue(); 253 if (codeString == null || "".equals(codeString)) 254 return null; 255 if ("indel".equals(codeString)) 256 return new Enumeration<QualityType>(this, QualityType.INDEL); 257 if ("snp".equals(codeString)) 258 return new Enumeration<QualityType>(this, QualityType.SNP); 259 if ("unknown".equals(codeString)) 260 return new Enumeration<QualityType>(this, QualityType.UNKNOWN); 261 throw new FHIRException("Unknown QualityType code '"+codeString+"'"); 262 } 263 public String toCode(QualityType code) { 264 if (code == QualityType.INDEL) 265 return "indel"; 266 if (code == QualityType.SNP) 267 return "snp"; 268 if (code == QualityType.UNKNOWN) 269 return "unknown"; 270 return "?"; 271 } 272 public String toSystem(QualityType code) { 273 return code.getSystem(); 274 } 275 } 276 277 public enum RepositoryType { 278 /** 279 * When URL is clicked, the resource can be seen directly (by webpage or by download link format) 280 */ 281 DIRECTLINK, 282 /** 283 * When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format) 284 */ 285 OPENAPI, 286 /** 287 * When logged into the website, the resource can be seen. 288 */ 289 LOGIN, 290 /** 291 * When logged in and follow the API in the website related with URL, the resource can be seen. 292 */ 293 OAUTH, 294 /** 295 * Some other complicated or particular way to get resource from URL. 296 */ 297 OTHER, 298 /** 299 * added to help the parsers with the generic types 300 */ 301 NULL; 302 public static RepositoryType fromCode(String codeString) throws FHIRException { 303 if (codeString == null || "".equals(codeString)) 304 return null; 305 if ("directlink".equals(codeString)) 306 return DIRECTLINK; 307 if ("openapi".equals(codeString)) 308 return OPENAPI; 309 if ("login".equals(codeString)) 310 return LOGIN; 311 if ("oauth".equals(codeString)) 312 return OAUTH; 313 if ("other".equals(codeString)) 314 return OTHER; 315 if (Configuration.isAcceptInvalidEnums()) 316 return null; 317 else 318 throw new FHIRException("Unknown RepositoryType code '"+codeString+"'"); 319 } 320 public String toCode() { 321 switch (this) { 322 case DIRECTLINK: return "directlink"; 323 case OPENAPI: return "openapi"; 324 case LOGIN: return "login"; 325 case OAUTH: return "oauth"; 326 case OTHER: return "other"; 327 case NULL: return null; 328 default: return "?"; 329 } 330 } 331 public String getSystem() { 332 switch (this) { 333 case DIRECTLINK: return "http://hl7.org/fhir/repository-type"; 334 case OPENAPI: return "http://hl7.org/fhir/repository-type"; 335 case LOGIN: return "http://hl7.org/fhir/repository-type"; 336 case OAUTH: return "http://hl7.org/fhir/repository-type"; 337 case OTHER: return "http://hl7.org/fhir/repository-type"; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 public String getDefinition() { 343 switch (this) { 344 case DIRECTLINK: return "When URL is clicked, the resource can be seen directly (by webpage or by download link format)"; 345 case OPENAPI: return "When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format)"; 346 case LOGIN: return "When logged into the website, the resource can be seen."; 347 case OAUTH: return "When logged in and follow the API in the website related with URL, the resource can be seen."; 348 case OTHER: return "Some other complicated or particular way to get resource from URL."; 349 case NULL: return null; 350 default: return "?"; 351 } 352 } 353 public String getDisplay() { 354 switch (this) { 355 case DIRECTLINK: return "Click and see"; 356 case OPENAPI: return "The URL is the RESTful or other kind of API that can access to the result."; 357 case LOGIN: return "Result cannot be access unless an account is logged in"; 358 case OAUTH: return "Result need to be fetched with API and need LOGIN( or cookies are required when visiting the link of resource)"; 359 case OTHER: return "Some other complicated or particular way to get resource from URL."; 360 case NULL: return null; 361 default: return "?"; 362 } 363 } 364 } 365 366 public static class RepositoryTypeEnumFactory implements EnumFactory<RepositoryType> { 367 public RepositoryType fromCode(String codeString) throws IllegalArgumentException { 368 if (codeString == null || "".equals(codeString)) 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("directlink".equals(codeString)) 372 return RepositoryType.DIRECTLINK; 373 if ("openapi".equals(codeString)) 374 return RepositoryType.OPENAPI; 375 if ("login".equals(codeString)) 376 return RepositoryType.LOGIN; 377 if ("oauth".equals(codeString)) 378 return RepositoryType.OAUTH; 379 if ("other".equals(codeString)) 380 return RepositoryType.OTHER; 381 throw new IllegalArgumentException("Unknown RepositoryType code '"+codeString+"'"); 382 } 383 public Enumeration<RepositoryType> fromType(PrimitiveType<?> code) throws FHIRException { 384 if (code == null) 385 return null; 386 if (code.isEmpty()) 387 return new Enumeration<RepositoryType>(this); 388 String codeString = code.asStringValue(); 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("directlink".equals(codeString)) 392 return new Enumeration<RepositoryType>(this, RepositoryType.DIRECTLINK); 393 if ("openapi".equals(codeString)) 394 return new Enumeration<RepositoryType>(this, RepositoryType.OPENAPI); 395 if ("login".equals(codeString)) 396 return new Enumeration<RepositoryType>(this, RepositoryType.LOGIN); 397 if ("oauth".equals(codeString)) 398 return new Enumeration<RepositoryType>(this, RepositoryType.OAUTH); 399 if ("other".equals(codeString)) 400 return new Enumeration<RepositoryType>(this, RepositoryType.OTHER); 401 throw new FHIRException("Unknown RepositoryType code '"+codeString+"'"); 402 } 403 public String toCode(RepositoryType code) { 404 if (code == RepositoryType.DIRECTLINK) 405 return "directlink"; 406 if (code == RepositoryType.OPENAPI) 407 return "openapi"; 408 if (code == RepositoryType.LOGIN) 409 return "login"; 410 if (code == RepositoryType.OAUTH) 411 return "oauth"; 412 if (code == RepositoryType.OTHER) 413 return "other"; 414 return "?"; 415 } 416 public String toSystem(RepositoryType code) { 417 return code.getSystem(); 418 } 419 } 420 421 @Block() 422 public static class SequenceReferenceSeqComponent extends BackboneElement implements IBaseBackboneElement { 423 /** 424 * Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)). 425 */ 426 @Child(name = "chromosome", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 427 @Description(shortDefinition="Chromosome containing genetic finding", formalDefinition="Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340))." ) 428 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chromosome-human") 429 protected CodeableConcept chromosome; 430 431 /** 432 * The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 433 */ 434 @Child(name = "genomeBuild", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 435 @Description(shortDefinition="The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'", formalDefinition="The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used." ) 436 protected StringType genomeBuild; 437 438 /** 439 * Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences. 440 */ 441 @Child(name = "referenceSeqId", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 442 @Description(shortDefinition="Reference identifier", formalDefinition="Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences." ) 443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-referenceSeq") 444 protected CodeableConcept referenceSeqId; 445 446 /** 447 * A Pointer to another Sequence entity as reference sequence. 448 */ 449 @Child(name = "referenceSeqPointer", type = {Sequence.class}, order=4, min=0, max=1, modifier=false, summary=true) 450 @Description(shortDefinition="A Pointer to another Sequence entity as reference sequence", formalDefinition="A Pointer to another Sequence entity as reference sequence." ) 451 protected Reference referenceSeqPointer; 452 453 /** 454 * The actual object that is the target of the reference (A Pointer to another Sequence entity as reference sequence.) 455 */ 456 protected Sequence referenceSeqPointerTarget; 457 458 /** 459 * A string like "ACGT". 460 */ 461 @Child(name = "referenceSeqString", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 462 @Description(shortDefinition="A string to represent reference sequence", formalDefinition="A string like \"ACGT\"." ) 463 protected StringType referenceSeqString; 464 465 /** 466 * Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 467 */ 468 @Child(name = "strand", type = {IntegerType.class}, order=6, min=0, max=1, modifier=false, summary=true) 469 @Description(shortDefinition="Directionality of DNA ( +1/-1)", formalDefinition="Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative." ) 470 protected IntegerType strand; 471 472 /** 473 * Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 474 */ 475 @Child(name = "windowStart", type = {IntegerType.class}, order=7, min=1, max=1, modifier=false, summary=true) 476 @Description(shortDefinition="Start position of the window on the reference sequence", formalDefinition="Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 477 protected IntegerType windowStart; 478 479 /** 480 * End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 481 */ 482 @Child(name = "windowEnd", type = {IntegerType.class}, order=8, min=1, max=1, modifier=false, summary=true) 483 @Description(shortDefinition="End position of the window on the reference sequence", formalDefinition="End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 484 protected IntegerType windowEnd; 485 486 private static final long serialVersionUID = -1675617731L; 487 488 /** 489 * Constructor 490 */ 491 public SequenceReferenceSeqComponent() { 492 super(); 493 } 494 495 /** 496 * Constructor 497 */ 498 public SequenceReferenceSeqComponent(IntegerType windowStart, IntegerType windowEnd) { 499 super(); 500 this.windowStart = windowStart; 501 this.windowEnd = windowEnd; 502 } 503 504 /** 505 * @return {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 506 */ 507 public CodeableConcept getChromosome() { 508 if (this.chromosome == null) 509 if (Configuration.errorOnAutoCreate()) 510 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.chromosome"); 511 else if (Configuration.doAutoCreate()) 512 this.chromosome = new CodeableConcept(); // cc 513 return this.chromosome; 514 } 515 516 public boolean hasChromosome() { 517 return this.chromosome != null && !this.chromosome.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 522 */ 523 public SequenceReferenceSeqComponent setChromosome(CodeableConcept value) { 524 this.chromosome = value; 525 return this; 526 } 527 528 /** 529 * @return {@link #genomeBuild} (The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.). This is the underlying object with id, value and extensions. The accessor "getGenomeBuild" gives direct access to the value 530 */ 531 public StringType getGenomeBuildElement() { 532 if (this.genomeBuild == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.genomeBuild"); 535 else if (Configuration.doAutoCreate()) 536 this.genomeBuild = new StringType(); // bb 537 return this.genomeBuild; 538 } 539 540 public boolean hasGenomeBuildElement() { 541 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 542 } 543 544 public boolean hasGenomeBuild() { 545 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #genomeBuild} (The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.). This is the underlying object with id, value and extensions. The accessor "getGenomeBuild" gives direct access to the value 550 */ 551 public SequenceReferenceSeqComponent setGenomeBuildElement(StringType value) { 552 this.genomeBuild = value; 553 return this; 554 } 555 556 /** 557 * @return The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 558 */ 559 public String getGenomeBuild() { 560 return this.genomeBuild == null ? null : this.genomeBuild.getValue(); 561 } 562 563 /** 564 * @param value The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 565 */ 566 public SequenceReferenceSeqComponent setGenomeBuild(String value) { 567 if (Utilities.noString(value)) 568 this.genomeBuild = null; 569 else { 570 if (this.genomeBuild == null) 571 this.genomeBuild = new StringType(); 572 this.genomeBuild.setValue(value); 573 } 574 return this; 575 } 576 577 /** 578 * @return {@link #referenceSeqId} (Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.) 579 */ 580 public CodeableConcept getReferenceSeqId() { 581 if (this.referenceSeqId == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqId"); 584 else if (Configuration.doAutoCreate()) 585 this.referenceSeqId = new CodeableConcept(); // cc 586 return this.referenceSeqId; 587 } 588 589 public boolean hasReferenceSeqId() { 590 return this.referenceSeqId != null && !this.referenceSeqId.isEmpty(); 591 } 592 593 /** 594 * @param value {@link #referenceSeqId} (Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.) 595 */ 596 public SequenceReferenceSeqComponent setReferenceSeqId(CodeableConcept value) { 597 this.referenceSeqId = value; 598 return this; 599 } 600 601 /** 602 * @return {@link #referenceSeqPointer} (A Pointer to another Sequence entity as reference sequence.) 603 */ 604 public Reference getReferenceSeqPointer() { 605 if (this.referenceSeqPointer == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqPointer"); 608 else if (Configuration.doAutoCreate()) 609 this.referenceSeqPointer = new Reference(); // cc 610 return this.referenceSeqPointer; 611 } 612 613 public boolean hasReferenceSeqPointer() { 614 return this.referenceSeqPointer != null && !this.referenceSeqPointer.isEmpty(); 615 } 616 617 /** 618 * @param value {@link #referenceSeqPointer} (A Pointer to another Sequence entity as reference sequence.) 619 */ 620 public SequenceReferenceSeqComponent setReferenceSeqPointer(Reference value) { 621 this.referenceSeqPointer = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #referenceSeqPointer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A Pointer to another Sequence entity as reference sequence.) 627 */ 628 public Sequence getReferenceSeqPointerTarget() { 629 if (this.referenceSeqPointerTarget == null) 630 if (Configuration.errorOnAutoCreate()) 631 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqPointer"); 632 else if (Configuration.doAutoCreate()) 633 this.referenceSeqPointerTarget = new Sequence(); // aa 634 return this.referenceSeqPointerTarget; 635 } 636 637 /** 638 * @param value {@link #referenceSeqPointer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A Pointer to another Sequence entity as reference sequence.) 639 */ 640 public SequenceReferenceSeqComponent setReferenceSeqPointerTarget(Sequence value) { 641 this.referenceSeqPointerTarget = value; 642 return this; 643 } 644 645 /** 646 * @return {@link #referenceSeqString} (A string like "ACGT".). This is the underlying object with id, value and extensions. The accessor "getReferenceSeqString" gives direct access to the value 647 */ 648 public StringType getReferenceSeqStringElement() { 649 if (this.referenceSeqString == null) 650 if (Configuration.errorOnAutoCreate()) 651 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqString"); 652 else if (Configuration.doAutoCreate()) 653 this.referenceSeqString = new StringType(); // bb 654 return this.referenceSeqString; 655 } 656 657 public boolean hasReferenceSeqStringElement() { 658 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 659 } 660 661 public boolean hasReferenceSeqString() { 662 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 663 } 664 665 /** 666 * @param value {@link #referenceSeqString} (A string like "ACGT".). This is the underlying object with id, value and extensions. The accessor "getReferenceSeqString" gives direct access to the value 667 */ 668 public SequenceReferenceSeqComponent setReferenceSeqStringElement(StringType value) { 669 this.referenceSeqString = value; 670 return this; 671 } 672 673 /** 674 * @return A string like "ACGT". 675 */ 676 public String getReferenceSeqString() { 677 return this.referenceSeqString == null ? null : this.referenceSeqString.getValue(); 678 } 679 680 /** 681 * @param value A string like "ACGT". 682 */ 683 public SequenceReferenceSeqComponent setReferenceSeqString(String value) { 684 if (Utilities.noString(value)) 685 this.referenceSeqString = null; 686 else { 687 if (this.referenceSeqString == null) 688 this.referenceSeqString = new StringType(); 689 this.referenceSeqString.setValue(value); 690 } 691 return this; 692 } 693 694 /** 695 * @return {@link #strand} (Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 696 */ 697 public IntegerType getStrandElement() { 698 if (this.strand == null) 699 if (Configuration.errorOnAutoCreate()) 700 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.strand"); 701 else if (Configuration.doAutoCreate()) 702 this.strand = new IntegerType(); // bb 703 return this.strand; 704 } 705 706 public boolean hasStrandElement() { 707 return this.strand != null && !this.strand.isEmpty(); 708 } 709 710 public boolean hasStrand() { 711 return this.strand != null && !this.strand.isEmpty(); 712 } 713 714 /** 715 * @param value {@link #strand} (Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 716 */ 717 public SequenceReferenceSeqComponent setStrandElement(IntegerType value) { 718 this.strand = value; 719 return this; 720 } 721 722 /** 723 * @return Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 724 */ 725 public int getStrand() { 726 return this.strand == null || this.strand.isEmpty() ? 0 : this.strand.getValue(); 727 } 728 729 /** 730 * @param value Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 731 */ 732 public SequenceReferenceSeqComponent setStrand(int value) { 733 if (this.strand == null) 734 this.strand = new IntegerType(); 735 this.strand.setValue(value); 736 return this; 737 } 738 739 /** 740 * @return {@link #windowStart} (Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 741 */ 742 public IntegerType getWindowStartElement() { 743 if (this.windowStart == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.windowStart"); 746 else if (Configuration.doAutoCreate()) 747 this.windowStart = new IntegerType(); // bb 748 return this.windowStart; 749 } 750 751 public boolean hasWindowStartElement() { 752 return this.windowStart != null && !this.windowStart.isEmpty(); 753 } 754 755 public boolean hasWindowStart() { 756 return this.windowStart != null && !this.windowStart.isEmpty(); 757 } 758 759 /** 760 * @param value {@link #windowStart} (Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 761 */ 762 public SequenceReferenceSeqComponent setWindowStartElement(IntegerType value) { 763 this.windowStart = value; 764 return this; 765 } 766 767 /** 768 * @return Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 769 */ 770 public int getWindowStart() { 771 return this.windowStart == null || this.windowStart.isEmpty() ? 0 : this.windowStart.getValue(); 772 } 773 774 /** 775 * @param value Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 776 */ 777 public SequenceReferenceSeqComponent setWindowStart(int value) { 778 if (this.windowStart == null) 779 this.windowStart = new IntegerType(); 780 this.windowStart.setValue(value); 781 return this; 782 } 783 784 /** 785 * @return {@link #windowEnd} (End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 786 */ 787 public IntegerType getWindowEndElement() { 788 if (this.windowEnd == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.windowEnd"); 791 else if (Configuration.doAutoCreate()) 792 this.windowEnd = new IntegerType(); // bb 793 return this.windowEnd; 794 } 795 796 public boolean hasWindowEndElement() { 797 return this.windowEnd != null && !this.windowEnd.isEmpty(); 798 } 799 800 public boolean hasWindowEnd() { 801 return this.windowEnd != null && !this.windowEnd.isEmpty(); 802 } 803 804 /** 805 * @param value {@link #windowEnd} (End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 806 */ 807 public SequenceReferenceSeqComponent setWindowEndElement(IntegerType value) { 808 this.windowEnd = value; 809 return this; 810 } 811 812 /** 813 * @return End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 814 */ 815 public int getWindowEnd() { 816 return this.windowEnd == null || this.windowEnd.isEmpty() ? 0 : this.windowEnd.getValue(); 817 } 818 819 /** 820 * @param value End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 821 */ 822 public SequenceReferenceSeqComponent setWindowEnd(int value) { 823 if (this.windowEnd == null) 824 this.windowEnd = new IntegerType(); 825 this.windowEnd.setValue(value); 826 return this; 827 } 828 829 protected void listChildren(List<Property> children) { 830 super.listChildren(children); 831 children.add(new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome)); 832 children.add(new Property("genomeBuild", "string", "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 0, 1, genomeBuild)); 833 children.add(new Property("referenceSeqId", "CodeableConcept", "Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 0, 1, referenceSeqId)); 834 children.add(new Property("referenceSeqPointer", "Reference(Sequence)", "A Pointer to another Sequence entity as reference sequence.", 0, 1, referenceSeqPointer)); 835 children.add(new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString)); 836 children.add(new Property("strand", "integer", "Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative.", 0, 1, strand)); 837 children.add(new Property("windowStart", "integer", "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, windowStart)); 838 children.add(new Property("windowEnd", "integer", "End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, windowEnd)); 839 } 840 841 @Override 842 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 843 switch (_hash) { 844 case -1499470472: /*chromosome*/ return new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome); 845 case 1061239735: /*genomeBuild*/ return new Property("genomeBuild", "string", "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 0, 1, genomeBuild); 846 case -1911500465: /*referenceSeqId*/ return new Property("referenceSeqId", "CodeableConcept", "Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 0, 1, referenceSeqId); 847 case 1923414665: /*referenceSeqPointer*/ return new Property("referenceSeqPointer", "Reference(Sequence)", "A Pointer to another Sequence entity as reference sequence.", 0, 1, referenceSeqPointer); 848 case -1648301499: /*referenceSeqString*/ return new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString); 849 case -891993594: /*strand*/ return new Property("strand", "integer", "Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative.", 0, 1, strand); 850 case 1903685202: /*windowStart*/ return new Property("windowStart", "integer", "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, windowStart); 851 case -217026869: /*windowEnd*/ return new Property("windowEnd", "integer", "End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, windowEnd); 852 default: return super.getNamedProperty(_hash, _name, _checkValid); 853 } 854 855 } 856 857 @Override 858 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 859 switch (hash) { 860 case -1499470472: /*chromosome*/ return this.chromosome == null ? new Base[0] : new Base[] {this.chromosome}; // CodeableConcept 861 case 1061239735: /*genomeBuild*/ return this.genomeBuild == null ? new Base[0] : new Base[] {this.genomeBuild}; // StringType 862 case -1911500465: /*referenceSeqId*/ return this.referenceSeqId == null ? new Base[0] : new Base[] {this.referenceSeqId}; // CodeableConcept 863 case 1923414665: /*referenceSeqPointer*/ return this.referenceSeqPointer == null ? new Base[0] : new Base[] {this.referenceSeqPointer}; // Reference 864 case -1648301499: /*referenceSeqString*/ return this.referenceSeqString == null ? new Base[0] : new Base[] {this.referenceSeqString}; // StringType 865 case -891993594: /*strand*/ return this.strand == null ? new Base[0] : new Base[] {this.strand}; // IntegerType 866 case 1903685202: /*windowStart*/ return this.windowStart == null ? new Base[0] : new Base[] {this.windowStart}; // IntegerType 867 case -217026869: /*windowEnd*/ return this.windowEnd == null ? new Base[0] : new Base[] {this.windowEnd}; // IntegerType 868 default: return super.getProperty(hash, name, checkValid); 869 } 870 871 } 872 873 @Override 874 public Base setProperty(int hash, String name, Base value) throws FHIRException { 875 switch (hash) { 876 case -1499470472: // chromosome 877 this.chromosome = castToCodeableConcept(value); // CodeableConcept 878 return value; 879 case 1061239735: // genomeBuild 880 this.genomeBuild = castToString(value); // StringType 881 return value; 882 case -1911500465: // referenceSeqId 883 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 884 return value; 885 case 1923414665: // referenceSeqPointer 886 this.referenceSeqPointer = castToReference(value); // Reference 887 return value; 888 case -1648301499: // referenceSeqString 889 this.referenceSeqString = castToString(value); // StringType 890 return value; 891 case -891993594: // strand 892 this.strand = castToInteger(value); // IntegerType 893 return value; 894 case 1903685202: // windowStart 895 this.windowStart = castToInteger(value); // IntegerType 896 return value; 897 case -217026869: // windowEnd 898 this.windowEnd = castToInteger(value); // IntegerType 899 return value; 900 default: return super.setProperty(hash, name, value); 901 } 902 903 } 904 905 @Override 906 public Base setProperty(String name, Base value) throws FHIRException { 907 if (name.equals("chromosome")) { 908 this.chromosome = castToCodeableConcept(value); // CodeableConcept 909 } else if (name.equals("genomeBuild")) { 910 this.genomeBuild = castToString(value); // StringType 911 } else if (name.equals("referenceSeqId")) { 912 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 913 } else if (name.equals("referenceSeqPointer")) { 914 this.referenceSeqPointer = castToReference(value); // Reference 915 } else if (name.equals("referenceSeqString")) { 916 this.referenceSeqString = castToString(value); // StringType 917 } else if (name.equals("strand")) { 918 this.strand = castToInteger(value); // IntegerType 919 } else if (name.equals("windowStart")) { 920 this.windowStart = castToInteger(value); // IntegerType 921 } else if (name.equals("windowEnd")) { 922 this.windowEnd = castToInteger(value); // IntegerType 923 } else 924 return super.setProperty(name, value); 925 return value; 926 } 927 928 @Override 929 public Base makeProperty(int hash, String name) throws FHIRException { 930 switch (hash) { 931 case -1499470472: return getChromosome(); 932 case 1061239735: return getGenomeBuildElement(); 933 case -1911500465: return getReferenceSeqId(); 934 case 1923414665: return getReferenceSeqPointer(); 935 case -1648301499: return getReferenceSeqStringElement(); 936 case -891993594: return getStrandElement(); 937 case 1903685202: return getWindowStartElement(); 938 case -217026869: return getWindowEndElement(); 939 default: return super.makeProperty(hash, name); 940 } 941 942 } 943 944 @Override 945 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 946 switch (hash) { 947 case -1499470472: /*chromosome*/ return new String[] {"CodeableConcept"}; 948 case 1061239735: /*genomeBuild*/ return new String[] {"string"}; 949 case -1911500465: /*referenceSeqId*/ return new String[] {"CodeableConcept"}; 950 case 1923414665: /*referenceSeqPointer*/ return new String[] {"Reference"}; 951 case -1648301499: /*referenceSeqString*/ return new String[] {"string"}; 952 case -891993594: /*strand*/ return new String[] {"integer"}; 953 case 1903685202: /*windowStart*/ return new String[] {"integer"}; 954 case -217026869: /*windowEnd*/ return new String[] {"integer"}; 955 default: return super.getTypesForProperty(hash, name); 956 } 957 958 } 959 960 @Override 961 public Base addChild(String name) throws FHIRException { 962 if (name.equals("chromosome")) { 963 this.chromosome = new CodeableConcept(); 964 return this.chromosome; 965 } 966 else if (name.equals("genomeBuild")) { 967 throw new FHIRException("Cannot call addChild on a singleton property Sequence.genomeBuild"); 968 } 969 else if (name.equals("referenceSeqId")) { 970 this.referenceSeqId = new CodeableConcept(); 971 return this.referenceSeqId; 972 } 973 else if (name.equals("referenceSeqPointer")) { 974 this.referenceSeqPointer = new Reference(); 975 return this.referenceSeqPointer; 976 } 977 else if (name.equals("referenceSeqString")) { 978 throw new FHIRException("Cannot call addChild on a singleton property Sequence.referenceSeqString"); 979 } 980 else if (name.equals("strand")) { 981 throw new FHIRException("Cannot call addChild on a singleton property Sequence.strand"); 982 } 983 else if (name.equals("windowStart")) { 984 throw new FHIRException("Cannot call addChild on a singleton property Sequence.windowStart"); 985 } 986 else if (name.equals("windowEnd")) { 987 throw new FHIRException("Cannot call addChild on a singleton property Sequence.windowEnd"); 988 } 989 else 990 return super.addChild(name); 991 } 992 993 public SequenceReferenceSeqComponent copy() { 994 SequenceReferenceSeqComponent dst = new SequenceReferenceSeqComponent(); 995 copyValues(dst); 996 dst.chromosome = chromosome == null ? null : chromosome.copy(); 997 dst.genomeBuild = genomeBuild == null ? null : genomeBuild.copy(); 998 dst.referenceSeqId = referenceSeqId == null ? null : referenceSeqId.copy(); 999 dst.referenceSeqPointer = referenceSeqPointer == null ? null : referenceSeqPointer.copy(); 1000 dst.referenceSeqString = referenceSeqString == null ? null : referenceSeqString.copy(); 1001 dst.strand = strand == null ? null : strand.copy(); 1002 dst.windowStart = windowStart == null ? null : windowStart.copy(); 1003 dst.windowEnd = windowEnd == null ? null : windowEnd.copy(); 1004 return dst; 1005 } 1006 1007 @Override 1008 public boolean equalsDeep(Base other_) { 1009 if (!super.equalsDeep(other_)) 1010 return false; 1011 if (!(other_ instanceof SequenceReferenceSeqComponent)) 1012 return false; 1013 SequenceReferenceSeqComponent o = (SequenceReferenceSeqComponent) other_; 1014 return compareDeep(chromosome, o.chromosome, true) && compareDeep(genomeBuild, o.genomeBuild, true) 1015 && compareDeep(referenceSeqId, o.referenceSeqId, true) && compareDeep(referenceSeqPointer, o.referenceSeqPointer, true) 1016 && compareDeep(referenceSeqString, o.referenceSeqString, true) && compareDeep(strand, o.strand, true) 1017 && compareDeep(windowStart, o.windowStart, true) && compareDeep(windowEnd, o.windowEnd, true); 1018 } 1019 1020 @Override 1021 public boolean equalsShallow(Base other_) { 1022 if (!super.equalsShallow(other_)) 1023 return false; 1024 if (!(other_ instanceof SequenceReferenceSeqComponent)) 1025 return false; 1026 SequenceReferenceSeqComponent o = (SequenceReferenceSeqComponent) other_; 1027 return compareValues(genomeBuild, o.genomeBuild, true) && compareValues(referenceSeqString, o.referenceSeqString, true) 1028 && compareValues(strand, o.strand, true) && compareValues(windowStart, o.windowStart, true) && compareValues(windowEnd, o.windowEnd, true) 1029 ; 1030 } 1031 1032 public boolean isEmpty() { 1033 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(chromosome, genomeBuild, referenceSeqId 1034 , referenceSeqPointer, referenceSeqString, strand, windowStart, windowEnd); 1035 } 1036 1037 public String fhirType() { 1038 return "Sequence.referenceSeq"; 1039 1040 } 1041 1042 } 1043 1044 @Block() 1045 public static class SequenceVariantComponent extends BackboneElement implements IBaseBackboneElement { 1046 /** 1047 * Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1048 */ 1049 @Child(name = "start", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1050 @Description(shortDefinition="Start position of the variant on the reference sequence", formalDefinition="Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 1051 protected IntegerType start; 1052 1053 /** 1054 * End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1055 */ 1056 @Child(name = "end", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1057 @Description(shortDefinition="End position of the variant on the reference sequence", formalDefinition="End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 1058 protected IntegerType end; 1059 1060 /** 1061 * An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1062 */ 1063 @Child(name = "observedAllele", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1064 @Description(shortDefinition="Allele that was observed", formalDefinition="An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1065 protected StringType observedAllele; 1066 1067 /** 1068 * An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1069 */ 1070 @Child(name = "referenceAllele", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1071 @Description(shortDefinition="Allele in the reference sequence", formalDefinition="An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1072 protected StringType referenceAllele; 1073 1074 /** 1075 * Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1076 */ 1077 @Child(name = "cigar", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1078 @Description(shortDefinition="Extended CIGAR string for aligning the sequence with reference bases", formalDefinition="Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm)." ) 1079 protected StringType cigar; 1080 1081 /** 1082 * A pointer to an Observation containing variant information. 1083 */ 1084 @Child(name = "variantPointer", type = {Observation.class}, order=6, min=0, max=1, modifier=false, summary=true) 1085 @Description(shortDefinition="Pointer to observed variant information", formalDefinition="A pointer to an Observation containing variant information." ) 1086 protected Reference variantPointer; 1087 1088 /** 1089 * The actual object that is the target of the reference (A pointer to an Observation containing variant information.) 1090 */ 1091 protected Observation variantPointerTarget; 1092 1093 private static final long serialVersionUID = 105611837L; 1094 1095 /** 1096 * Constructor 1097 */ 1098 public SequenceVariantComponent() { 1099 super(); 1100 } 1101 1102 /** 1103 * @return {@link #start} (Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1104 */ 1105 public IntegerType getStartElement() { 1106 if (this.start == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create SequenceVariantComponent.start"); 1109 else if (Configuration.doAutoCreate()) 1110 this.start = new IntegerType(); // bb 1111 return this.start; 1112 } 1113 1114 public boolean hasStartElement() { 1115 return this.start != null && !this.start.isEmpty(); 1116 } 1117 1118 public boolean hasStart() { 1119 return this.start != null && !this.start.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #start} (Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1124 */ 1125 public SequenceVariantComponent setStartElement(IntegerType value) { 1126 this.start = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1132 */ 1133 public int getStart() { 1134 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1135 } 1136 1137 /** 1138 * @param value Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1139 */ 1140 public SequenceVariantComponent setStart(int value) { 1141 if (this.start == null) 1142 this.start = new IntegerType(); 1143 this.start.setValue(value); 1144 return this; 1145 } 1146 1147 /** 1148 * @return {@link #end} (End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1149 */ 1150 public IntegerType getEndElement() { 1151 if (this.end == null) 1152 if (Configuration.errorOnAutoCreate()) 1153 throw new Error("Attempt to auto-create SequenceVariantComponent.end"); 1154 else if (Configuration.doAutoCreate()) 1155 this.end = new IntegerType(); // bb 1156 return this.end; 1157 } 1158 1159 public boolean hasEndElement() { 1160 return this.end != null && !this.end.isEmpty(); 1161 } 1162 1163 public boolean hasEnd() { 1164 return this.end != null && !this.end.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #end} (End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1169 */ 1170 public SequenceVariantComponent setEndElement(IntegerType value) { 1171 this.end = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1177 */ 1178 public int getEnd() { 1179 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1180 } 1181 1182 /** 1183 * @param value End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1184 */ 1185 public SequenceVariantComponent setEnd(int value) { 1186 if (this.end == null) 1187 this.end = new IntegerType(); 1188 this.end.setValue(value); 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #observedAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getObservedAllele" gives direct access to the value 1194 */ 1195 public StringType getObservedAlleleElement() { 1196 if (this.observedAllele == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create SequenceVariantComponent.observedAllele"); 1199 else if (Configuration.doAutoCreate()) 1200 this.observedAllele = new StringType(); // bb 1201 return this.observedAllele; 1202 } 1203 1204 public boolean hasObservedAlleleElement() { 1205 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1206 } 1207 1208 public boolean hasObservedAllele() { 1209 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #observedAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getObservedAllele" gives direct access to the value 1214 */ 1215 public SequenceVariantComponent setObservedAlleleElement(StringType value) { 1216 this.observedAllele = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1222 */ 1223 public String getObservedAllele() { 1224 return this.observedAllele == null ? null : this.observedAllele.getValue(); 1225 } 1226 1227 /** 1228 * @param value An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1229 */ 1230 public SequenceVariantComponent setObservedAllele(String value) { 1231 if (Utilities.noString(value)) 1232 this.observedAllele = null; 1233 else { 1234 if (this.observedAllele == null) 1235 this.observedAllele = new StringType(); 1236 this.observedAllele.setValue(value); 1237 } 1238 return this; 1239 } 1240 1241 /** 1242 * @return {@link #referenceAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReferenceAllele" gives direct access to the value 1243 */ 1244 public StringType getReferenceAlleleElement() { 1245 if (this.referenceAllele == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create SequenceVariantComponent.referenceAllele"); 1248 else if (Configuration.doAutoCreate()) 1249 this.referenceAllele = new StringType(); // bb 1250 return this.referenceAllele; 1251 } 1252 1253 public boolean hasReferenceAlleleElement() { 1254 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1255 } 1256 1257 public boolean hasReferenceAllele() { 1258 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #referenceAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReferenceAllele" gives direct access to the value 1263 */ 1264 public SequenceVariantComponent setReferenceAlleleElement(StringType value) { 1265 this.referenceAllele = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1271 */ 1272 public String getReferenceAllele() { 1273 return this.referenceAllele == null ? null : this.referenceAllele.getValue(); 1274 } 1275 1276 /** 1277 * @param value An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1278 */ 1279 public SequenceVariantComponent setReferenceAllele(String value) { 1280 if (Utilities.noString(value)) 1281 this.referenceAllele = null; 1282 else { 1283 if (this.referenceAllele == null) 1284 this.referenceAllele = new StringType(); 1285 this.referenceAllele.setValue(value); 1286 } 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #cigar} (Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). This is the underlying object with id, value and extensions. The accessor "getCigar" gives direct access to the value 1292 */ 1293 public StringType getCigarElement() { 1294 if (this.cigar == null) 1295 if (Configuration.errorOnAutoCreate()) 1296 throw new Error("Attempt to auto-create SequenceVariantComponent.cigar"); 1297 else if (Configuration.doAutoCreate()) 1298 this.cigar = new StringType(); // bb 1299 return this.cigar; 1300 } 1301 1302 public boolean hasCigarElement() { 1303 return this.cigar != null && !this.cigar.isEmpty(); 1304 } 1305 1306 public boolean hasCigar() { 1307 return this.cigar != null && !this.cigar.isEmpty(); 1308 } 1309 1310 /** 1311 * @param value {@link #cigar} (Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). This is the underlying object with id, value and extensions. The accessor "getCigar" gives direct access to the value 1312 */ 1313 public SequenceVariantComponent setCigarElement(StringType value) { 1314 this.cigar = value; 1315 return this; 1316 } 1317 1318 /** 1319 * @return Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1320 */ 1321 public String getCigar() { 1322 return this.cigar == null ? null : this.cigar.getValue(); 1323 } 1324 1325 /** 1326 * @param value Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1327 */ 1328 public SequenceVariantComponent setCigar(String value) { 1329 if (Utilities.noString(value)) 1330 this.cigar = null; 1331 else { 1332 if (this.cigar == null) 1333 this.cigar = new StringType(); 1334 this.cigar.setValue(value); 1335 } 1336 return this; 1337 } 1338 1339 /** 1340 * @return {@link #variantPointer} (A pointer to an Observation containing variant information.) 1341 */ 1342 public Reference getVariantPointer() { 1343 if (this.variantPointer == null) 1344 if (Configuration.errorOnAutoCreate()) 1345 throw new Error("Attempt to auto-create SequenceVariantComponent.variantPointer"); 1346 else if (Configuration.doAutoCreate()) 1347 this.variantPointer = new Reference(); // cc 1348 return this.variantPointer; 1349 } 1350 1351 public boolean hasVariantPointer() { 1352 return this.variantPointer != null && !this.variantPointer.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #variantPointer} (A pointer to an Observation containing variant information.) 1357 */ 1358 public SequenceVariantComponent setVariantPointer(Reference value) { 1359 this.variantPointer = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return {@link #variantPointer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A pointer to an Observation containing variant information.) 1365 */ 1366 public Observation getVariantPointerTarget() { 1367 if (this.variantPointerTarget == null) 1368 if (Configuration.errorOnAutoCreate()) 1369 throw new Error("Attempt to auto-create SequenceVariantComponent.variantPointer"); 1370 else if (Configuration.doAutoCreate()) 1371 this.variantPointerTarget = new Observation(); // aa 1372 return this.variantPointerTarget; 1373 } 1374 1375 /** 1376 * @param value {@link #variantPointer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A pointer to an Observation containing variant information.) 1377 */ 1378 public SequenceVariantComponent setVariantPointerTarget(Observation value) { 1379 this.variantPointerTarget = value; 1380 return this; 1381 } 1382 1383 protected void listChildren(List<Property> children) { 1384 super.listChildren(children); 1385 children.add(new Property("start", "integer", "Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start)); 1386 children.add(new Property("end", "integer", "End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end)); 1387 children.add(new Property("observedAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, observedAllele)); 1388 children.add(new Property("referenceAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, referenceAllele)); 1389 children.add(new Property("cigar", "string", "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 0, 1, cigar)); 1390 children.add(new Property("variantPointer", "Reference(Observation)", "A pointer to an Observation containing variant information.", 0, 1, variantPointer)); 1391 } 1392 1393 @Override 1394 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1395 switch (_hash) { 1396 case 109757538: /*start*/ return new Property("start", "integer", "Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start); 1397 case 100571: /*end*/ return new Property("end", "integer", "End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end); 1398 case -1418745787: /*observedAllele*/ return new Property("observedAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, observedAllele); 1399 case 364045960: /*referenceAllele*/ return new Property("referenceAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, referenceAllele); 1400 case 94658738: /*cigar*/ return new Property("cigar", "string", "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 0, 1, cigar); 1401 case -1654319624: /*variantPointer*/ return new Property("variantPointer", "Reference(Observation)", "A pointer to an Observation containing variant information.", 0, 1, variantPointer); 1402 default: return super.getNamedProperty(_hash, _name, _checkValid); 1403 } 1404 1405 } 1406 1407 @Override 1408 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1409 switch (hash) { 1410 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IntegerType 1411 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // IntegerType 1412 case -1418745787: /*observedAllele*/ return this.observedAllele == null ? new Base[0] : new Base[] {this.observedAllele}; // StringType 1413 case 364045960: /*referenceAllele*/ return this.referenceAllele == null ? new Base[0] : new Base[] {this.referenceAllele}; // StringType 1414 case 94658738: /*cigar*/ return this.cigar == null ? new Base[0] : new Base[] {this.cigar}; // StringType 1415 case -1654319624: /*variantPointer*/ return this.variantPointer == null ? new Base[0] : new Base[] {this.variantPointer}; // Reference 1416 default: return super.getProperty(hash, name, checkValid); 1417 } 1418 1419 } 1420 1421 @Override 1422 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1423 switch (hash) { 1424 case 109757538: // start 1425 this.start = castToInteger(value); // IntegerType 1426 return value; 1427 case 100571: // end 1428 this.end = castToInteger(value); // IntegerType 1429 return value; 1430 case -1418745787: // observedAllele 1431 this.observedAllele = castToString(value); // StringType 1432 return value; 1433 case 364045960: // referenceAllele 1434 this.referenceAllele = castToString(value); // StringType 1435 return value; 1436 case 94658738: // cigar 1437 this.cigar = castToString(value); // StringType 1438 return value; 1439 case -1654319624: // variantPointer 1440 this.variantPointer = castToReference(value); // Reference 1441 return value; 1442 default: return super.setProperty(hash, name, value); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base setProperty(String name, Base value) throws FHIRException { 1449 if (name.equals("start")) { 1450 this.start = castToInteger(value); // IntegerType 1451 } else if (name.equals("end")) { 1452 this.end = castToInteger(value); // IntegerType 1453 } else if (name.equals("observedAllele")) { 1454 this.observedAllele = castToString(value); // StringType 1455 } else if (name.equals("referenceAllele")) { 1456 this.referenceAllele = castToString(value); // StringType 1457 } else if (name.equals("cigar")) { 1458 this.cigar = castToString(value); // StringType 1459 } else if (name.equals("variantPointer")) { 1460 this.variantPointer = castToReference(value); // Reference 1461 } else 1462 return super.setProperty(name, value); 1463 return value; 1464 } 1465 1466 @Override 1467 public Base makeProperty(int hash, String name) throws FHIRException { 1468 switch (hash) { 1469 case 109757538: return getStartElement(); 1470 case 100571: return getEndElement(); 1471 case -1418745787: return getObservedAlleleElement(); 1472 case 364045960: return getReferenceAlleleElement(); 1473 case 94658738: return getCigarElement(); 1474 case -1654319624: return getVariantPointer(); 1475 default: return super.makeProperty(hash, name); 1476 } 1477 1478 } 1479 1480 @Override 1481 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case 109757538: /*start*/ return new String[] {"integer"}; 1484 case 100571: /*end*/ return new String[] {"integer"}; 1485 case -1418745787: /*observedAllele*/ return new String[] {"string"}; 1486 case 364045960: /*referenceAllele*/ return new String[] {"string"}; 1487 case 94658738: /*cigar*/ return new String[] {"string"}; 1488 case -1654319624: /*variantPointer*/ return new String[] {"Reference"}; 1489 default: return super.getTypesForProperty(hash, name); 1490 } 1491 1492 } 1493 1494 @Override 1495 public Base addChild(String name) throws FHIRException { 1496 if (name.equals("start")) { 1497 throw new FHIRException("Cannot call addChild on a singleton property Sequence.start"); 1498 } 1499 else if (name.equals("end")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property Sequence.end"); 1501 } 1502 else if (name.equals("observedAllele")) { 1503 throw new FHIRException("Cannot call addChild on a singleton property Sequence.observedAllele"); 1504 } 1505 else if (name.equals("referenceAllele")) { 1506 throw new FHIRException("Cannot call addChild on a singleton property Sequence.referenceAllele"); 1507 } 1508 else if (name.equals("cigar")) { 1509 throw new FHIRException("Cannot call addChild on a singleton property Sequence.cigar"); 1510 } 1511 else if (name.equals("variantPointer")) { 1512 this.variantPointer = new Reference(); 1513 return this.variantPointer; 1514 } 1515 else 1516 return super.addChild(name); 1517 } 1518 1519 public SequenceVariantComponent copy() { 1520 SequenceVariantComponent dst = new SequenceVariantComponent(); 1521 copyValues(dst); 1522 dst.start = start == null ? null : start.copy(); 1523 dst.end = end == null ? null : end.copy(); 1524 dst.observedAllele = observedAllele == null ? null : observedAllele.copy(); 1525 dst.referenceAllele = referenceAllele == null ? null : referenceAllele.copy(); 1526 dst.cigar = cigar == null ? null : cigar.copy(); 1527 dst.variantPointer = variantPointer == null ? null : variantPointer.copy(); 1528 return dst; 1529 } 1530 1531 @Override 1532 public boolean equalsDeep(Base other_) { 1533 if (!super.equalsDeep(other_)) 1534 return false; 1535 if (!(other_ instanceof SequenceVariantComponent)) 1536 return false; 1537 SequenceVariantComponent o = (SequenceVariantComponent) other_; 1538 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(observedAllele, o.observedAllele, true) 1539 && compareDeep(referenceAllele, o.referenceAllele, true) && compareDeep(cigar, o.cigar, true) && compareDeep(variantPointer, o.variantPointer, true) 1540 ; 1541 } 1542 1543 @Override 1544 public boolean equalsShallow(Base other_) { 1545 if (!super.equalsShallow(other_)) 1546 return false; 1547 if (!(other_ instanceof SequenceVariantComponent)) 1548 return false; 1549 SequenceVariantComponent o = (SequenceVariantComponent) other_; 1550 return compareValues(start, o.start, true) && compareValues(end, o.end, true) && compareValues(observedAllele, o.observedAllele, true) 1551 && compareValues(referenceAllele, o.referenceAllele, true) && compareValues(cigar, o.cigar, true); 1552 } 1553 1554 public boolean isEmpty() { 1555 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end, observedAllele 1556 , referenceAllele, cigar, variantPointer); 1557 } 1558 1559 public String fhirType() { 1560 return "Sequence.variant"; 1561 1562 } 1563 1564 } 1565 1566 @Block() 1567 public static class SequenceQualityComponent extends BackboneElement implements IBaseBackboneElement { 1568 /** 1569 * INDEL / SNP / Undefined variant. 1570 */ 1571 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1572 @Description(shortDefinition="indel | snp | unknown", formalDefinition="INDEL / SNP / Undefined variant." ) 1573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/quality-type") 1574 protected Enumeration<QualityType> type; 1575 1576 /** 1577 * Gold standard sequence used for comparing against. 1578 */ 1579 @Child(name = "standardSequence", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1580 @Description(shortDefinition="Standard sequence for comparison", formalDefinition="Gold standard sequence used for comparing against." ) 1581 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-quality-standardSequence") 1582 protected CodeableConcept standardSequence; 1583 1584 /** 1585 * Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1586 */ 1587 @Child(name = "start", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1588 @Description(shortDefinition="Start position of the sequence", formalDefinition="Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 1589 protected IntegerType start; 1590 1591 /** 1592 * End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1593 */ 1594 @Child(name = "end", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1595 @Description(shortDefinition="End position of the sequence", formalDefinition="End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 1596 protected IntegerType end; 1597 1598 /** 1599 * The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)). 1600 */ 1601 @Child(name = "score", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=true) 1602 @Description(shortDefinition="Quality score for the comparison", formalDefinition="The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685))." ) 1603 protected Quantity score; 1604 1605 /** 1606 * Which method is used to get sequence quality. 1607 */ 1608 @Child(name = "method", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1609 @Description(shortDefinition="Method to get quality", formalDefinition="Which method is used to get sequence quality." ) 1610 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-quality-method") 1611 protected CodeableConcept method; 1612 1613 /** 1614 * True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1615 */ 1616 @Child(name = "truthTP", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1617 @Description(shortDefinition="True positives from the perspective of the truth data", formalDefinition="True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event." ) 1618 protected DecimalType truthTP; 1619 1620 /** 1621 * True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1622 */ 1623 @Child(name = "queryTP", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1624 @Description(shortDefinition="True positives from the perspective of the query data", formalDefinition="True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event." ) 1625 protected DecimalType queryTP; 1626 1627 /** 1628 * False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 1629 */ 1630 @Child(name = "truthFN", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1631 @Description(shortDefinition="False negatives", formalDefinition="False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here." ) 1632 protected DecimalType truthFN; 1633 1634 /** 1635 * False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 1636 */ 1637 @Child(name = "queryFP", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1638 @Description(shortDefinition="False positives", formalDefinition="False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here." ) 1639 protected DecimalType queryFP; 1640 1641 /** 1642 * The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 1643 */ 1644 @Child(name = "gtFP", type = {DecimalType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1645 @Description(shortDefinition="False positives where the non-REF alleles in the Truth and Query Call Sets match", formalDefinition="The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar)." ) 1646 protected DecimalType gtFP; 1647 1648 /** 1649 * QUERY.TP / (QUERY.TP + QUERY.FP). 1650 */ 1651 @Child(name = "precision", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1652 @Description(shortDefinition="Precision of comparison", formalDefinition="QUERY.TP / (QUERY.TP + QUERY.FP)." ) 1653 protected DecimalType precision; 1654 1655 /** 1656 * TRUTH.TP / (TRUTH.TP + TRUTH.FN). 1657 */ 1658 @Child(name = "recall", type = {DecimalType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1659 @Description(shortDefinition="Recall of comparison", formalDefinition="TRUTH.TP / (TRUTH.TP + TRUTH.FN)." ) 1660 protected DecimalType recall; 1661 1662 /** 1663 * Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 1664 */ 1665 @Child(name = "fScore", type = {DecimalType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1666 @Description(shortDefinition="F-score", formalDefinition="Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall)." ) 1667 protected DecimalType fScore; 1668 1669 private static final long serialVersionUID = -383644463L; 1670 1671 /** 1672 * Constructor 1673 */ 1674 public SequenceQualityComponent() { 1675 super(); 1676 } 1677 1678 /** 1679 * Constructor 1680 */ 1681 public SequenceQualityComponent(Enumeration<QualityType> type) { 1682 super(); 1683 this.type = type; 1684 } 1685 1686 /** 1687 * @return {@link #type} (INDEL / SNP / Undefined variant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1688 */ 1689 public Enumeration<QualityType> getTypeElement() { 1690 if (this.type == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create SequenceQualityComponent.type"); 1693 else if (Configuration.doAutoCreate()) 1694 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); // bb 1695 return this.type; 1696 } 1697 1698 public boolean hasTypeElement() { 1699 return this.type != null && !this.type.isEmpty(); 1700 } 1701 1702 public boolean hasType() { 1703 return this.type != null && !this.type.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #type} (INDEL / SNP / Undefined variant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1708 */ 1709 public SequenceQualityComponent setTypeElement(Enumeration<QualityType> value) { 1710 this.type = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return INDEL / SNP / Undefined variant. 1716 */ 1717 public QualityType getType() { 1718 return this.type == null ? null : this.type.getValue(); 1719 } 1720 1721 /** 1722 * @param value INDEL / SNP / Undefined variant. 1723 */ 1724 public SequenceQualityComponent setType(QualityType value) { 1725 if (this.type == null) 1726 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); 1727 this.type.setValue(value); 1728 return this; 1729 } 1730 1731 /** 1732 * @return {@link #standardSequence} (Gold standard sequence used for comparing against.) 1733 */ 1734 public CodeableConcept getStandardSequence() { 1735 if (this.standardSequence == null) 1736 if (Configuration.errorOnAutoCreate()) 1737 throw new Error("Attempt to auto-create SequenceQualityComponent.standardSequence"); 1738 else if (Configuration.doAutoCreate()) 1739 this.standardSequence = new CodeableConcept(); // cc 1740 return this.standardSequence; 1741 } 1742 1743 public boolean hasStandardSequence() { 1744 return this.standardSequence != null && !this.standardSequence.isEmpty(); 1745 } 1746 1747 /** 1748 * @param value {@link #standardSequence} (Gold standard sequence used for comparing against.) 1749 */ 1750 public SequenceQualityComponent setStandardSequence(CodeableConcept value) { 1751 this.standardSequence = value; 1752 return this; 1753 } 1754 1755 /** 1756 * @return {@link #start} (Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1757 */ 1758 public IntegerType getStartElement() { 1759 if (this.start == null) 1760 if (Configuration.errorOnAutoCreate()) 1761 throw new Error("Attempt to auto-create SequenceQualityComponent.start"); 1762 else if (Configuration.doAutoCreate()) 1763 this.start = new IntegerType(); // bb 1764 return this.start; 1765 } 1766 1767 public boolean hasStartElement() { 1768 return this.start != null && !this.start.isEmpty(); 1769 } 1770 1771 public boolean hasStart() { 1772 return this.start != null && !this.start.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #start} (Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1777 */ 1778 public SequenceQualityComponent setStartElement(IntegerType value) { 1779 this.start = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1785 */ 1786 public int getStart() { 1787 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1788 } 1789 1790 /** 1791 * @param value Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1792 */ 1793 public SequenceQualityComponent setStart(int value) { 1794 if (this.start == null) 1795 this.start = new IntegerType(); 1796 this.start.setValue(value); 1797 return this; 1798 } 1799 1800 /** 1801 * @return {@link #end} (End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1802 */ 1803 public IntegerType getEndElement() { 1804 if (this.end == null) 1805 if (Configuration.errorOnAutoCreate()) 1806 throw new Error("Attempt to auto-create SequenceQualityComponent.end"); 1807 else if (Configuration.doAutoCreate()) 1808 this.end = new IntegerType(); // bb 1809 return this.end; 1810 } 1811 1812 public boolean hasEndElement() { 1813 return this.end != null && !this.end.isEmpty(); 1814 } 1815 1816 public boolean hasEnd() { 1817 return this.end != null && !this.end.isEmpty(); 1818 } 1819 1820 /** 1821 * @param value {@link #end} (End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1822 */ 1823 public SequenceQualityComponent setEndElement(IntegerType value) { 1824 this.end = value; 1825 return this; 1826 } 1827 1828 /** 1829 * @return End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1830 */ 1831 public int getEnd() { 1832 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1833 } 1834 1835 /** 1836 * @param value End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1837 */ 1838 public SequenceQualityComponent setEnd(int value) { 1839 if (this.end == null) 1840 this.end = new IntegerType(); 1841 this.end.setValue(value); 1842 return this; 1843 } 1844 1845 /** 1846 * @return {@link #score} (The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 1847 */ 1848 public Quantity getScore() { 1849 if (this.score == null) 1850 if (Configuration.errorOnAutoCreate()) 1851 throw new Error("Attempt to auto-create SequenceQualityComponent.score"); 1852 else if (Configuration.doAutoCreate()) 1853 this.score = new Quantity(); // cc 1854 return this.score; 1855 } 1856 1857 public boolean hasScore() { 1858 return this.score != null && !this.score.isEmpty(); 1859 } 1860 1861 /** 1862 * @param value {@link #score} (The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 1863 */ 1864 public SequenceQualityComponent setScore(Quantity value) { 1865 this.score = value; 1866 return this; 1867 } 1868 1869 /** 1870 * @return {@link #method} (Which method is used to get sequence quality.) 1871 */ 1872 public CodeableConcept getMethod() { 1873 if (this.method == null) 1874 if (Configuration.errorOnAutoCreate()) 1875 throw new Error("Attempt to auto-create SequenceQualityComponent.method"); 1876 else if (Configuration.doAutoCreate()) 1877 this.method = new CodeableConcept(); // cc 1878 return this.method; 1879 } 1880 1881 public boolean hasMethod() { 1882 return this.method != null && !this.method.isEmpty(); 1883 } 1884 1885 /** 1886 * @param value {@link #method} (Which method is used to get sequence quality.) 1887 */ 1888 public SequenceQualityComponent setMethod(CodeableConcept value) { 1889 this.method = value; 1890 return this; 1891 } 1892 1893 /** 1894 * @return {@link #truthTP} (True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getTruthTP" gives direct access to the value 1895 */ 1896 public DecimalType getTruthTPElement() { 1897 if (this.truthTP == null) 1898 if (Configuration.errorOnAutoCreate()) 1899 throw new Error("Attempt to auto-create SequenceQualityComponent.truthTP"); 1900 else if (Configuration.doAutoCreate()) 1901 this.truthTP = new DecimalType(); // bb 1902 return this.truthTP; 1903 } 1904 1905 public boolean hasTruthTPElement() { 1906 return this.truthTP != null && !this.truthTP.isEmpty(); 1907 } 1908 1909 public boolean hasTruthTP() { 1910 return this.truthTP != null && !this.truthTP.isEmpty(); 1911 } 1912 1913 /** 1914 * @param value {@link #truthTP} (True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getTruthTP" gives direct access to the value 1915 */ 1916 public SequenceQualityComponent setTruthTPElement(DecimalType value) { 1917 this.truthTP = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1923 */ 1924 public BigDecimal getTruthTP() { 1925 return this.truthTP == null ? null : this.truthTP.getValue(); 1926 } 1927 1928 /** 1929 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1930 */ 1931 public SequenceQualityComponent setTruthTP(BigDecimal value) { 1932 if (value == null) 1933 this.truthTP = null; 1934 else { 1935 if (this.truthTP == null) 1936 this.truthTP = new DecimalType(); 1937 this.truthTP.setValue(value); 1938 } 1939 return this; 1940 } 1941 1942 /** 1943 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1944 */ 1945 public SequenceQualityComponent setTruthTP(long value) { 1946 this.truthTP = new DecimalType(); 1947 this.truthTP.setValue(value); 1948 return this; 1949 } 1950 1951 /** 1952 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1953 */ 1954 public SequenceQualityComponent setTruthTP(double value) { 1955 this.truthTP = new DecimalType(); 1956 this.truthTP.setValue(value); 1957 return this; 1958 } 1959 1960 /** 1961 * @return {@link #queryTP} (True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getQueryTP" gives direct access to the value 1962 */ 1963 public DecimalType getQueryTPElement() { 1964 if (this.queryTP == null) 1965 if (Configuration.errorOnAutoCreate()) 1966 throw new Error("Attempt to auto-create SequenceQualityComponent.queryTP"); 1967 else if (Configuration.doAutoCreate()) 1968 this.queryTP = new DecimalType(); // bb 1969 return this.queryTP; 1970 } 1971 1972 public boolean hasQueryTPElement() { 1973 return this.queryTP != null && !this.queryTP.isEmpty(); 1974 } 1975 1976 public boolean hasQueryTP() { 1977 return this.queryTP != null && !this.queryTP.isEmpty(); 1978 } 1979 1980 /** 1981 * @param value {@link #queryTP} (True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getQueryTP" gives direct access to the value 1982 */ 1983 public SequenceQualityComponent setQueryTPElement(DecimalType value) { 1984 this.queryTP = value; 1985 return this; 1986 } 1987 1988 /** 1989 * @return True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1990 */ 1991 public BigDecimal getQueryTP() { 1992 return this.queryTP == null ? null : this.queryTP.getValue(); 1993 } 1994 1995 /** 1996 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1997 */ 1998 public SequenceQualityComponent setQueryTP(BigDecimal value) { 1999 if (value == null) 2000 this.queryTP = null; 2001 else { 2002 if (this.queryTP == null) 2003 this.queryTP = new DecimalType(); 2004 this.queryTP.setValue(value); 2005 } 2006 return this; 2007 } 2008 2009 /** 2010 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 2011 */ 2012 public SequenceQualityComponent setQueryTP(long value) { 2013 this.queryTP = new DecimalType(); 2014 this.queryTP.setValue(value); 2015 return this; 2016 } 2017 2018 /** 2019 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 2020 */ 2021 public SequenceQualityComponent setQueryTP(double value) { 2022 this.queryTP = new DecimalType(); 2023 this.queryTP.setValue(value); 2024 return this; 2025 } 2026 2027 /** 2028 * @return {@link #truthFN} (False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getTruthFN" gives direct access to the value 2029 */ 2030 public DecimalType getTruthFNElement() { 2031 if (this.truthFN == null) 2032 if (Configuration.errorOnAutoCreate()) 2033 throw new Error("Attempt to auto-create SequenceQualityComponent.truthFN"); 2034 else if (Configuration.doAutoCreate()) 2035 this.truthFN = new DecimalType(); // bb 2036 return this.truthFN; 2037 } 2038 2039 public boolean hasTruthFNElement() { 2040 return this.truthFN != null && !this.truthFN.isEmpty(); 2041 } 2042 2043 public boolean hasTruthFN() { 2044 return this.truthFN != null && !this.truthFN.isEmpty(); 2045 } 2046 2047 /** 2048 * @param value {@link #truthFN} (False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getTruthFN" gives direct access to the value 2049 */ 2050 public SequenceQualityComponent setTruthFNElement(DecimalType value) { 2051 this.truthFN = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2057 */ 2058 public BigDecimal getTruthFN() { 2059 return this.truthFN == null ? null : this.truthFN.getValue(); 2060 } 2061 2062 /** 2063 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2064 */ 2065 public SequenceQualityComponent setTruthFN(BigDecimal value) { 2066 if (value == null) 2067 this.truthFN = null; 2068 else { 2069 if (this.truthFN == null) 2070 this.truthFN = new DecimalType(); 2071 this.truthFN.setValue(value); 2072 } 2073 return this; 2074 } 2075 2076 /** 2077 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2078 */ 2079 public SequenceQualityComponent setTruthFN(long value) { 2080 this.truthFN = new DecimalType(); 2081 this.truthFN.setValue(value); 2082 return this; 2083 } 2084 2085 /** 2086 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2087 */ 2088 public SequenceQualityComponent setTruthFN(double value) { 2089 this.truthFN = new DecimalType(); 2090 this.truthFN.setValue(value); 2091 return this; 2092 } 2093 2094 /** 2095 * @return {@link #queryFP} (False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getQueryFP" gives direct access to the value 2096 */ 2097 public DecimalType getQueryFPElement() { 2098 if (this.queryFP == null) 2099 if (Configuration.errorOnAutoCreate()) 2100 throw new Error("Attempt to auto-create SequenceQualityComponent.queryFP"); 2101 else if (Configuration.doAutoCreate()) 2102 this.queryFP = new DecimalType(); // bb 2103 return this.queryFP; 2104 } 2105 2106 public boolean hasQueryFPElement() { 2107 return this.queryFP != null && !this.queryFP.isEmpty(); 2108 } 2109 2110 public boolean hasQueryFP() { 2111 return this.queryFP != null && !this.queryFP.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #queryFP} (False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getQueryFP" gives direct access to the value 2116 */ 2117 public SequenceQualityComponent setQueryFPElement(DecimalType value) { 2118 this.queryFP = value; 2119 return this; 2120 } 2121 2122 /** 2123 * @return False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2124 */ 2125 public BigDecimal getQueryFP() { 2126 return this.queryFP == null ? null : this.queryFP.getValue(); 2127 } 2128 2129 /** 2130 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2131 */ 2132 public SequenceQualityComponent setQueryFP(BigDecimal value) { 2133 if (value == null) 2134 this.queryFP = null; 2135 else { 2136 if (this.queryFP == null) 2137 this.queryFP = new DecimalType(); 2138 this.queryFP.setValue(value); 2139 } 2140 return this; 2141 } 2142 2143 /** 2144 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2145 */ 2146 public SequenceQualityComponent setQueryFP(long value) { 2147 this.queryFP = new DecimalType(); 2148 this.queryFP.setValue(value); 2149 return this; 2150 } 2151 2152 /** 2153 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2154 */ 2155 public SequenceQualityComponent setQueryFP(double value) { 2156 this.queryFP = new DecimalType(); 2157 this.queryFP.setValue(value); 2158 return this; 2159 } 2160 2161 /** 2162 * @return {@link #gtFP} (The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).). This is the underlying object with id, value and extensions. The accessor "getGtFP" gives direct access to the value 2163 */ 2164 public DecimalType getGtFPElement() { 2165 if (this.gtFP == null) 2166 if (Configuration.errorOnAutoCreate()) 2167 throw new Error("Attempt to auto-create SequenceQualityComponent.gtFP"); 2168 else if (Configuration.doAutoCreate()) 2169 this.gtFP = new DecimalType(); // bb 2170 return this.gtFP; 2171 } 2172 2173 public boolean hasGtFPElement() { 2174 return this.gtFP != null && !this.gtFP.isEmpty(); 2175 } 2176 2177 public boolean hasGtFP() { 2178 return this.gtFP != null && !this.gtFP.isEmpty(); 2179 } 2180 2181 /** 2182 * @param value {@link #gtFP} (The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).). This is the underlying object with id, value and extensions. The accessor "getGtFP" gives direct access to the value 2183 */ 2184 public SequenceQualityComponent setGtFPElement(DecimalType value) { 2185 this.gtFP = value; 2186 return this; 2187 } 2188 2189 /** 2190 * @return The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2191 */ 2192 public BigDecimal getGtFP() { 2193 return this.gtFP == null ? null : this.gtFP.getValue(); 2194 } 2195 2196 /** 2197 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2198 */ 2199 public SequenceQualityComponent setGtFP(BigDecimal value) { 2200 if (value == null) 2201 this.gtFP = null; 2202 else { 2203 if (this.gtFP == null) 2204 this.gtFP = new DecimalType(); 2205 this.gtFP.setValue(value); 2206 } 2207 return this; 2208 } 2209 2210 /** 2211 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2212 */ 2213 public SequenceQualityComponent setGtFP(long value) { 2214 this.gtFP = new DecimalType(); 2215 this.gtFP.setValue(value); 2216 return this; 2217 } 2218 2219 /** 2220 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2221 */ 2222 public SequenceQualityComponent setGtFP(double value) { 2223 this.gtFP = new DecimalType(); 2224 this.gtFP.setValue(value); 2225 return this; 2226 } 2227 2228 /** 2229 * @return {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the underlying object with id, value and extensions. The accessor "getPrecision" gives direct access to the value 2230 */ 2231 public DecimalType getPrecisionElement() { 2232 if (this.precision == null) 2233 if (Configuration.errorOnAutoCreate()) 2234 throw new Error("Attempt to auto-create SequenceQualityComponent.precision"); 2235 else if (Configuration.doAutoCreate()) 2236 this.precision = new DecimalType(); // bb 2237 return this.precision; 2238 } 2239 2240 public boolean hasPrecisionElement() { 2241 return this.precision != null && !this.precision.isEmpty(); 2242 } 2243 2244 public boolean hasPrecision() { 2245 return this.precision != null && !this.precision.isEmpty(); 2246 } 2247 2248 /** 2249 * @param value {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the underlying object with id, value and extensions. The accessor "getPrecision" gives direct access to the value 2250 */ 2251 public SequenceQualityComponent setPrecisionElement(DecimalType value) { 2252 this.precision = value; 2253 return this; 2254 } 2255 2256 /** 2257 * @return QUERY.TP / (QUERY.TP + QUERY.FP). 2258 */ 2259 public BigDecimal getPrecision() { 2260 return this.precision == null ? null : this.precision.getValue(); 2261 } 2262 2263 /** 2264 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2265 */ 2266 public SequenceQualityComponent setPrecision(BigDecimal value) { 2267 if (value == null) 2268 this.precision = null; 2269 else { 2270 if (this.precision == null) 2271 this.precision = new DecimalType(); 2272 this.precision.setValue(value); 2273 } 2274 return this; 2275 } 2276 2277 /** 2278 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2279 */ 2280 public SequenceQualityComponent setPrecision(long value) { 2281 this.precision = new DecimalType(); 2282 this.precision.setValue(value); 2283 return this; 2284 } 2285 2286 /** 2287 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2288 */ 2289 public SequenceQualityComponent setPrecision(double value) { 2290 this.precision = new DecimalType(); 2291 this.precision.setValue(value); 2292 return this; 2293 } 2294 2295 /** 2296 * @return {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 2297 */ 2298 public DecimalType getRecallElement() { 2299 if (this.recall == null) 2300 if (Configuration.errorOnAutoCreate()) 2301 throw new Error("Attempt to auto-create SequenceQualityComponent.recall"); 2302 else if (Configuration.doAutoCreate()) 2303 this.recall = new DecimalType(); // bb 2304 return this.recall; 2305 } 2306 2307 public boolean hasRecallElement() { 2308 return this.recall != null && !this.recall.isEmpty(); 2309 } 2310 2311 public boolean hasRecall() { 2312 return this.recall != null && !this.recall.isEmpty(); 2313 } 2314 2315 /** 2316 * @param value {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 2317 */ 2318 public SequenceQualityComponent setRecallElement(DecimalType value) { 2319 this.recall = value; 2320 return this; 2321 } 2322 2323 /** 2324 * @return TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2325 */ 2326 public BigDecimal getRecall() { 2327 return this.recall == null ? null : this.recall.getValue(); 2328 } 2329 2330 /** 2331 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2332 */ 2333 public SequenceQualityComponent setRecall(BigDecimal value) { 2334 if (value == null) 2335 this.recall = null; 2336 else { 2337 if (this.recall == null) 2338 this.recall = new DecimalType(); 2339 this.recall.setValue(value); 2340 } 2341 return this; 2342 } 2343 2344 /** 2345 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2346 */ 2347 public SequenceQualityComponent setRecall(long value) { 2348 this.recall = new DecimalType(); 2349 this.recall.setValue(value); 2350 return this; 2351 } 2352 2353 /** 2354 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2355 */ 2356 public SequenceQualityComponent setRecall(double value) { 2357 this.recall = new DecimalType(); 2358 this.recall.setValue(value); 2359 return this; 2360 } 2361 2362 /** 2363 * @return {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).). This is the underlying object with id, value and extensions. The accessor "getFScore" gives direct access to the value 2364 */ 2365 public DecimalType getFScoreElement() { 2366 if (this.fScore == null) 2367 if (Configuration.errorOnAutoCreate()) 2368 throw new Error("Attempt to auto-create SequenceQualityComponent.fScore"); 2369 else if (Configuration.doAutoCreate()) 2370 this.fScore = new DecimalType(); // bb 2371 return this.fScore; 2372 } 2373 2374 public boolean hasFScoreElement() { 2375 return this.fScore != null && !this.fScore.isEmpty(); 2376 } 2377 2378 public boolean hasFScore() { 2379 return this.fScore != null && !this.fScore.isEmpty(); 2380 } 2381 2382 /** 2383 * @param value {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).). This is the underlying object with id, value and extensions. The accessor "getFScore" gives direct access to the value 2384 */ 2385 public SequenceQualityComponent setFScoreElement(DecimalType value) { 2386 this.fScore = value; 2387 return this; 2388 } 2389 2390 /** 2391 * @return Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2392 */ 2393 public BigDecimal getFScore() { 2394 return this.fScore == null ? null : this.fScore.getValue(); 2395 } 2396 2397 /** 2398 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2399 */ 2400 public SequenceQualityComponent setFScore(BigDecimal value) { 2401 if (value == null) 2402 this.fScore = null; 2403 else { 2404 if (this.fScore == null) 2405 this.fScore = new DecimalType(); 2406 this.fScore.setValue(value); 2407 } 2408 return this; 2409 } 2410 2411 /** 2412 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2413 */ 2414 public SequenceQualityComponent setFScore(long value) { 2415 this.fScore = new DecimalType(); 2416 this.fScore.setValue(value); 2417 return this; 2418 } 2419 2420 /** 2421 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2422 */ 2423 public SequenceQualityComponent setFScore(double value) { 2424 this.fScore = new DecimalType(); 2425 this.fScore.setValue(value); 2426 return this; 2427 } 2428 2429 protected void listChildren(List<Property> children) { 2430 super.listChildren(children); 2431 children.add(new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type)); 2432 children.add(new Property("standardSequence", "CodeableConcept", "Gold standard sequence used for comparing against.", 0, 1, standardSequence)); 2433 children.add(new Property("start", "integer", "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start)); 2434 children.add(new Property("end", "integer", "End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end)); 2435 children.add(new Property("score", "Quantity", "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 0, 1, score)); 2436 children.add(new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method)); 2437 children.add(new Property("truthTP", "decimal", "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, truthTP)); 2438 children.add(new Property("queryTP", "decimal", "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, queryTP)); 2439 children.add(new Property("truthFN", "decimal", "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 0, 1, truthFN)); 2440 children.add(new Property("queryFP", "decimal", "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 0, 1, queryFP)); 2441 children.add(new Property("gtFP", "decimal", "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 0, 1, gtFP)); 2442 children.add(new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision)); 2443 children.add(new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall)); 2444 children.add(new Property("fScore", "decimal", "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, fScore)); 2445 } 2446 2447 @Override 2448 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2449 switch (_hash) { 2450 case 3575610: /*type*/ return new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type); 2451 case -1861227106: /*standardSequence*/ return new Property("standardSequence", "CodeableConcept", "Gold standard sequence used for comparing against.", 0, 1, standardSequence); 2452 case 109757538: /*start*/ return new Property("start", "integer", "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start); 2453 case 100571: /*end*/ return new Property("end", "integer", "End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end); 2454 case 109264530: /*score*/ return new Property("score", "Quantity", "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 0, 1, score); 2455 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method); 2456 case -1048421849: /*truthTP*/ return new Property("truthTP", "decimal", "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, truthTP); 2457 case 655102276: /*queryTP*/ return new Property("queryTP", "decimal", "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, queryTP); 2458 case -1048422285: /*truthFN*/ return new Property("truthFN", "decimal", "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 0, 1, truthFN); 2459 case 655101842: /*queryFP*/ return new Property("queryFP", "decimal", "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 0, 1, queryFP); 2460 case 3182199: /*gtFP*/ return new Property("gtFP", "decimal", "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 0, 1, gtFP); 2461 case -1376177026: /*precision*/ return new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision); 2462 case -934922479: /*recall*/ return new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall); 2463 case -1295082036: /*fScore*/ return new Property("fScore", "decimal", "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, fScore); 2464 default: return super.getNamedProperty(_hash, _name, _checkValid); 2465 } 2466 2467 } 2468 2469 @Override 2470 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2471 switch (hash) { 2472 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<QualityType> 2473 case -1861227106: /*standardSequence*/ return this.standardSequence == null ? new Base[0] : new Base[] {this.standardSequence}; // CodeableConcept 2474 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IntegerType 2475 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // IntegerType 2476 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // Quantity 2477 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 2478 case -1048421849: /*truthTP*/ return this.truthTP == null ? new Base[0] : new Base[] {this.truthTP}; // DecimalType 2479 case 655102276: /*queryTP*/ return this.queryTP == null ? new Base[0] : new Base[] {this.queryTP}; // DecimalType 2480 case -1048422285: /*truthFN*/ return this.truthFN == null ? new Base[0] : new Base[] {this.truthFN}; // DecimalType 2481 case 655101842: /*queryFP*/ return this.queryFP == null ? new Base[0] : new Base[] {this.queryFP}; // DecimalType 2482 case 3182199: /*gtFP*/ return this.gtFP == null ? new Base[0] : new Base[] {this.gtFP}; // DecimalType 2483 case -1376177026: /*precision*/ return this.precision == null ? new Base[0] : new Base[] {this.precision}; // DecimalType 2484 case -934922479: /*recall*/ return this.recall == null ? new Base[0] : new Base[] {this.recall}; // DecimalType 2485 case -1295082036: /*fScore*/ return this.fScore == null ? new Base[0] : new Base[] {this.fScore}; // DecimalType 2486 default: return super.getProperty(hash, name, checkValid); 2487 } 2488 2489 } 2490 2491 @Override 2492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2493 switch (hash) { 2494 case 3575610: // type 2495 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 2496 this.type = (Enumeration) value; // Enumeration<QualityType> 2497 return value; 2498 case -1861227106: // standardSequence 2499 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 2500 return value; 2501 case 109757538: // start 2502 this.start = castToInteger(value); // IntegerType 2503 return value; 2504 case 100571: // end 2505 this.end = castToInteger(value); // IntegerType 2506 return value; 2507 case 109264530: // score 2508 this.score = castToQuantity(value); // Quantity 2509 return value; 2510 case -1077554975: // method 2511 this.method = castToCodeableConcept(value); // CodeableConcept 2512 return value; 2513 case -1048421849: // truthTP 2514 this.truthTP = castToDecimal(value); // DecimalType 2515 return value; 2516 case 655102276: // queryTP 2517 this.queryTP = castToDecimal(value); // DecimalType 2518 return value; 2519 case -1048422285: // truthFN 2520 this.truthFN = castToDecimal(value); // DecimalType 2521 return value; 2522 case 655101842: // queryFP 2523 this.queryFP = castToDecimal(value); // DecimalType 2524 return value; 2525 case 3182199: // gtFP 2526 this.gtFP = castToDecimal(value); // DecimalType 2527 return value; 2528 case -1376177026: // precision 2529 this.precision = castToDecimal(value); // DecimalType 2530 return value; 2531 case -934922479: // recall 2532 this.recall = castToDecimal(value); // DecimalType 2533 return value; 2534 case -1295082036: // fScore 2535 this.fScore = castToDecimal(value); // DecimalType 2536 return value; 2537 default: return super.setProperty(hash, name, value); 2538 } 2539 2540 } 2541 2542 @Override 2543 public Base setProperty(String name, Base value) throws FHIRException { 2544 if (name.equals("type")) { 2545 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 2546 this.type = (Enumeration) value; // Enumeration<QualityType> 2547 } else if (name.equals("standardSequence")) { 2548 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 2549 } else if (name.equals("start")) { 2550 this.start = castToInteger(value); // IntegerType 2551 } else if (name.equals("end")) { 2552 this.end = castToInteger(value); // IntegerType 2553 } else if (name.equals("score")) { 2554 this.score = castToQuantity(value); // Quantity 2555 } else if (name.equals("method")) { 2556 this.method = castToCodeableConcept(value); // CodeableConcept 2557 } else if (name.equals("truthTP")) { 2558 this.truthTP = castToDecimal(value); // DecimalType 2559 } else if (name.equals("queryTP")) { 2560 this.queryTP = castToDecimal(value); // DecimalType 2561 } else if (name.equals("truthFN")) { 2562 this.truthFN = castToDecimal(value); // DecimalType 2563 } else if (name.equals("queryFP")) { 2564 this.queryFP = castToDecimal(value); // DecimalType 2565 } else if (name.equals("gtFP")) { 2566 this.gtFP = castToDecimal(value); // DecimalType 2567 } else if (name.equals("precision")) { 2568 this.precision = castToDecimal(value); // DecimalType 2569 } else if (name.equals("recall")) { 2570 this.recall = castToDecimal(value); // DecimalType 2571 } else if (name.equals("fScore")) { 2572 this.fScore = castToDecimal(value); // DecimalType 2573 } else 2574 return super.setProperty(name, value); 2575 return value; 2576 } 2577 2578 @Override 2579 public Base makeProperty(int hash, String name) throws FHIRException { 2580 switch (hash) { 2581 case 3575610: return getTypeElement(); 2582 case -1861227106: return getStandardSequence(); 2583 case 109757538: return getStartElement(); 2584 case 100571: return getEndElement(); 2585 case 109264530: return getScore(); 2586 case -1077554975: return getMethod(); 2587 case -1048421849: return getTruthTPElement(); 2588 case 655102276: return getQueryTPElement(); 2589 case -1048422285: return getTruthFNElement(); 2590 case 655101842: return getQueryFPElement(); 2591 case 3182199: return getGtFPElement(); 2592 case -1376177026: return getPrecisionElement(); 2593 case -934922479: return getRecallElement(); 2594 case -1295082036: return getFScoreElement(); 2595 default: return super.makeProperty(hash, name); 2596 } 2597 2598 } 2599 2600 @Override 2601 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2602 switch (hash) { 2603 case 3575610: /*type*/ return new String[] {"code"}; 2604 case -1861227106: /*standardSequence*/ return new String[] {"CodeableConcept"}; 2605 case 109757538: /*start*/ return new String[] {"integer"}; 2606 case 100571: /*end*/ return new String[] {"integer"}; 2607 case 109264530: /*score*/ return new String[] {"Quantity"}; 2608 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 2609 case -1048421849: /*truthTP*/ return new String[] {"decimal"}; 2610 case 655102276: /*queryTP*/ return new String[] {"decimal"}; 2611 case -1048422285: /*truthFN*/ return new String[] {"decimal"}; 2612 case 655101842: /*queryFP*/ return new String[] {"decimal"}; 2613 case 3182199: /*gtFP*/ return new String[] {"decimal"}; 2614 case -1376177026: /*precision*/ return new String[] {"decimal"}; 2615 case -934922479: /*recall*/ return new String[] {"decimal"}; 2616 case -1295082036: /*fScore*/ return new String[] {"decimal"}; 2617 default: return super.getTypesForProperty(hash, name); 2618 } 2619 2620 } 2621 2622 @Override 2623 public Base addChild(String name) throws FHIRException { 2624 if (name.equals("type")) { 2625 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 2626 } 2627 else if (name.equals("standardSequence")) { 2628 this.standardSequence = new CodeableConcept(); 2629 return this.standardSequence; 2630 } 2631 else if (name.equals("start")) { 2632 throw new FHIRException("Cannot call addChild on a singleton property Sequence.start"); 2633 } 2634 else if (name.equals("end")) { 2635 throw new FHIRException("Cannot call addChild on a singleton property Sequence.end"); 2636 } 2637 else if (name.equals("score")) { 2638 this.score = new Quantity(); 2639 return this.score; 2640 } 2641 else if (name.equals("method")) { 2642 this.method = new CodeableConcept(); 2643 return this.method; 2644 } 2645 else if (name.equals("truthTP")) { 2646 throw new FHIRException("Cannot call addChild on a singleton property Sequence.truthTP"); 2647 } 2648 else if (name.equals("queryTP")) { 2649 throw new FHIRException("Cannot call addChild on a singleton property Sequence.queryTP"); 2650 } 2651 else if (name.equals("truthFN")) { 2652 throw new FHIRException("Cannot call addChild on a singleton property Sequence.truthFN"); 2653 } 2654 else if (name.equals("queryFP")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property Sequence.queryFP"); 2656 } 2657 else if (name.equals("gtFP")) { 2658 throw new FHIRException("Cannot call addChild on a singleton property Sequence.gtFP"); 2659 } 2660 else if (name.equals("precision")) { 2661 throw new FHIRException("Cannot call addChild on a singleton property Sequence.precision"); 2662 } 2663 else if (name.equals("recall")) { 2664 throw new FHIRException("Cannot call addChild on a singleton property Sequence.recall"); 2665 } 2666 else if (name.equals("fScore")) { 2667 throw new FHIRException("Cannot call addChild on a singleton property Sequence.fScore"); 2668 } 2669 else 2670 return super.addChild(name); 2671 } 2672 2673 public SequenceQualityComponent copy() { 2674 SequenceQualityComponent dst = new SequenceQualityComponent(); 2675 copyValues(dst); 2676 dst.type = type == null ? null : type.copy(); 2677 dst.standardSequence = standardSequence == null ? null : standardSequence.copy(); 2678 dst.start = start == null ? null : start.copy(); 2679 dst.end = end == null ? null : end.copy(); 2680 dst.score = score == null ? null : score.copy(); 2681 dst.method = method == null ? null : method.copy(); 2682 dst.truthTP = truthTP == null ? null : truthTP.copy(); 2683 dst.queryTP = queryTP == null ? null : queryTP.copy(); 2684 dst.truthFN = truthFN == null ? null : truthFN.copy(); 2685 dst.queryFP = queryFP == null ? null : queryFP.copy(); 2686 dst.gtFP = gtFP == null ? null : gtFP.copy(); 2687 dst.precision = precision == null ? null : precision.copy(); 2688 dst.recall = recall == null ? null : recall.copy(); 2689 dst.fScore = fScore == null ? null : fScore.copy(); 2690 return dst; 2691 } 2692 2693 @Override 2694 public boolean equalsDeep(Base other_) { 2695 if (!super.equalsDeep(other_)) 2696 return false; 2697 if (!(other_ instanceof SequenceQualityComponent)) 2698 return false; 2699 SequenceQualityComponent o = (SequenceQualityComponent) other_; 2700 return compareDeep(type, o.type, true) && compareDeep(standardSequence, o.standardSequence, true) 2701 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(score, o.score, true) 2702 && compareDeep(method, o.method, true) && compareDeep(truthTP, o.truthTP, true) && compareDeep(queryTP, o.queryTP, true) 2703 && compareDeep(truthFN, o.truthFN, true) && compareDeep(queryFP, o.queryFP, true) && compareDeep(gtFP, o.gtFP, true) 2704 && compareDeep(precision, o.precision, true) && compareDeep(recall, o.recall, true) && compareDeep(fScore, o.fScore, true) 2705 ; 2706 } 2707 2708 @Override 2709 public boolean equalsShallow(Base other_) { 2710 if (!super.equalsShallow(other_)) 2711 return false; 2712 if (!(other_ instanceof SequenceQualityComponent)) 2713 return false; 2714 SequenceQualityComponent o = (SequenceQualityComponent) other_; 2715 return compareValues(type, o.type, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 2716 && compareValues(truthTP, o.truthTP, true) && compareValues(queryTP, o.queryTP, true) && compareValues(truthFN, o.truthFN, true) 2717 && compareValues(queryFP, o.queryFP, true) && compareValues(gtFP, o.gtFP, true) && compareValues(precision, o.precision, true) 2718 && compareValues(recall, o.recall, true) && compareValues(fScore, o.fScore, true); 2719 } 2720 2721 public boolean isEmpty() { 2722 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, standardSequence, start 2723 , end, score, method, truthTP, queryTP, truthFN, queryFP, gtFP, precision 2724 , recall, fScore); 2725 } 2726 2727 public String fhirType() { 2728 return "Sequence.quality"; 2729 2730 } 2731 2732 } 2733 2734 @Block() 2735 public static class SequenceRepositoryComponent extends BackboneElement implements IBaseBackboneElement { 2736 /** 2737 * Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2738 */ 2739 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2740 @Description(shortDefinition="directlink | openapi | login | oauth | other", formalDefinition="Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource." ) 2741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/repository-type") 2742 protected Enumeration<RepositoryType> type; 2743 2744 /** 2745 * URI of an external repository which contains further details about the genetics data. 2746 */ 2747 @Child(name = "url", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2748 @Description(shortDefinition="URI of the repository", formalDefinition="URI of an external repository which contains further details about the genetics data." ) 2749 protected UriType url; 2750 2751 /** 2752 * URI of an external repository which contains further details about the genetics data. 2753 */ 2754 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2755 @Description(shortDefinition="Repository's name", formalDefinition="URI of an external repository which contains further details about the genetics data." ) 2756 protected StringType name; 2757 2758 /** 2759 * Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2760 */ 2761 @Child(name = "datasetId", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2762 @Description(shortDefinition="Id of the dataset that used to call for dataset in repository", formalDefinition="Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository." ) 2763 protected StringType datasetId; 2764 2765 /** 2766 * Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 2767 */ 2768 @Child(name = "variantsetId", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2769 @Description(shortDefinition="Id of the variantset that used to call for variantset in repository", formalDefinition="Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository." ) 2770 protected StringType variantsetId; 2771 2772 /** 2773 * Id of the read in this external repository. 2774 */ 2775 @Child(name = "readsetId", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2776 @Description(shortDefinition="Id of the read", formalDefinition="Id of the read in this external repository." ) 2777 protected StringType readsetId; 2778 2779 private static final long serialVersionUID = -899243265L; 2780 2781 /** 2782 * Constructor 2783 */ 2784 public SequenceRepositoryComponent() { 2785 super(); 2786 } 2787 2788 /** 2789 * Constructor 2790 */ 2791 public SequenceRepositoryComponent(Enumeration<RepositoryType> type) { 2792 super(); 2793 this.type = type; 2794 } 2795 2796 /** 2797 * @return {@link #type} (Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2798 */ 2799 public Enumeration<RepositoryType> getTypeElement() { 2800 if (this.type == null) 2801 if (Configuration.errorOnAutoCreate()) 2802 throw new Error("Attempt to auto-create SequenceRepositoryComponent.type"); 2803 else if (Configuration.doAutoCreate()) 2804 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); // bb 2805 return this.type; 2806 } 2807 2808 public boolean hasTypeElement() { 2809 return this.type != null && !this.type.isEmpty(); 2810 } 2811 2812 public boolean hasType() { 2813 return this.type != null && !this.type.isEmpty(); 2814 } 2815 2816 /** 2817 * @param value {@link #type} (Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2818 */ 2819 public SequenceRepositoryComponent setTypeElement(Enumeration<RepositoryType> value) { 2820 this.type = value; 2821 return this; 2822 } 2823 2824 /** 2825 * @return Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2826 */ 2827 public RepositoryType getType() { 2828 return this.type == null ? null : this.type.getValue(); 2829 } 2830 2831 /** 2832 * @param value Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2833 */ 2834 public SequenceRepositoryComponent setType(RepositoryType value) { 2835 if (this.type == null) 2836 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); 2837 this.type.setValue(value); 2838 return this; 2839 } 2840 2841 /** 2842 * @return {@link #url} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2843 */ 2844 public UriType getUrlElement() { 2845 if (this.url == null) 2846 if (Configuration.errorOnAutoCreate()) 2847 throw new Error("Attempt to auto-create SequenceRepositoryComponent.url"); 2848 else if (Configuration.doAutoCreate()) 2849 this.url = new UriType(); // bb 2850 return this.url; 2851 } 2852 2853 public boolean hasUrlElement() { 2854 return this.url != null && !this.url.isEmpty(); 2855 } 2856 2857 public boolean hasUrl() { 2858 return this.url != null && !this.url.isEmpty(); 2859 } 2860 2861 /** 2862 * @param value {@link #url} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2863 */ 2864 public SequenceRepositoryComponent setUrlElement(UriType value) { 2865 this.url = value; 2866 return this; 2867 } 2868 2869 /** 2870 * @return URI of an external repository which contains further details about the genetics data. 2871 */ 2872 public String getUrl() { 2873 return this.url == null ? null : this.url.getValue(); 2874 } 2875 2876 /** 2877 * @param value URI of an external repository which contains further details about the genetics data. 2878 */ 2879 public SequenceRepositoryComponent setUrl(String value) { 2880 if (Utilities.noString(value)) 2881 this.url = null; 2882 else { 2883 if (this.url == null) 2884 this.url = new UriType(); 2885 this.url.setValue(value); 2886 } 2887 return this; 2888 } 2889 2890 /** 2891 * @return {@link #name} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2892 */ 2893 public StringType getNameElement() { 2894 if (this.name == null) 2895 if (Configuration.errorOnAutoCreate()) 2896 throw new Error("Attempt to auto-create SequenceRepositoryComponent.name"); 2897 else if (Configuration.doAutoCreate()) 2898 this.name = new StringType(); // bb 2899 return this.name; 2900 } 2901 2902 public boolean hasNameElement() { 2903 return this.name != null && !this.name.isEmpty(); 2904 } 2905 2906 public boolean hasName() { 2907 return this.name != null && !this.name.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #name} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2912 */ 2913 public SequenceRepositoryComponent setNameElement(StringType value) { 2914 this.name = value; 2915 return this; 2916 } 2917 2918 /** 2919 * @return URI of an external repository which contains further details about the genetics data. 2920 */ 2921 public String getName() { 2922 return this.name == null ? null : this.name.getValue(); 2923 } 2924 2925 /** 2926 * @param value URI of an external repository which contains further details about the genetics data. 2927 */ 2928 public SequenceRepositoryComponent setName(String value) { 2929 if (Utilities.noString(value)) 2930 this.name = null; 2931 else { 2932 if (this.name == null) 2933 this.name = new StringType(); 2934 this.name.setValue(value); 2935 } 2936 return this; 2937 } 2938 2939 /** 2940 * @return {@link #datasetId} (Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.). This is the underlying object with id, value and extensions. The accessor "getDatasetId" gives direct access to the value 2941 */ 2942 public StringType getDatasetIdElement() { 2943 if (this.datasetId == null) 2944 if (Configuration.errorOnAutoCreate()) 2945 throw new Error("Attempt to auto-create SequenceRepositoryComponent.datasetId"); 2946 else if (Configuration.doAutoCreate()) 2947 this.datasetId = new StringType(); // bb 2948 return this.datasetId; 2949 } 2950 2951 public boolean hasDatasetIdElement() { 2952 return this.datasetId != null && !this.datasetId.isEmpty(); 2953 } 2954 2955 public boolean hasDatasetId() { 2956 return this.datasetId != null && !this.datasetId.isEmpty(); 2957 } 2958 2959 /** 2960 * @param value {@link #datasetId} (Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.). This is the underlying object with id, value and extensions. The accessor "getDatasetId" gives direct access to the value 2961 */ 2962 public SequenceRepositoryComponent setDatasetIdElement(StringType value) { 2963 this.datasetId = value; 2964 return this; 2965 } 2966 2967 /** 2968 * @return Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2969 */ 2970 public String getDatasetId() { 2971 return this.datasetId == null ? null : this.datasetId.getValue(); 2972 } 2973 2974 /** 2975 * @param value Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2976 */ 2977 public SequenceRepositoryComponent setDatasetId(String value) { 2978 if (Utilities.noString(value)) 2979 this.datasetId = null; 2980 else { 2981 if (this.datasetId == null) 2982 this.datasetId = new StringType(); 2983 this.datasetId.setValue(value); 2984 } 2985 return this; 2986 } 2987 2988 /** 2989 * @return {@link #variantsetId} (Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.). This is the underlying object with id, value and extensions. The accessor "getVariantsetId" gives direct access to the value 2990 */ 2991 public StringType getVariantsetIdElement() { 2992 if (this.variantsetId == null) 2993 if (Configuration.errorOnAutoCreate()) 2994 throw new Error("Attempt to auto-create SequenceRepositoryComponent.variantsetId"); 2995 else if (Configuration.doAutoCreate()) 2996 this.variantsetId = new StringType(); // bb 2997 return this.variantsetId; 2998 } 2999 3000 public boolean hasVariantsetIdElement() { 3001 return this.variantsetId != null && !this.variantsetId.isEmpty(); 3002 } 3003 3004 public boolean hasVariantsetId() { 3005 return this.variantsetId != null && !this.variantsetId.isEmpty(); 3006 } 3007 3008 /** 3009 * @param value {@link #variantsetId} (Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.). This is the underlying object with id, value and extensions. The accessor "getVariantsetId" gives direct access to the value 3010 */ 3011 public SequenceRepositoryComponent setVariantsetIdElement(StringType value) { 3012 this.variantsetId = value; 3013 return this; 3014 } 3015 3016 /** 3017 * @return Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 3018 */ 3019 public String getVariantsetId() { 3020 return this.variantsetId == null ? null : this.variantsetId.getValue(); 3021 } 3022 3023 /** 3024 * @param value Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 3025 */ 3026 public SequenceRepositoryComponent setVariantsetId(String value) { 3027 if (Utilities.noString(value)) 3028 this.variantsetId = null; 3029 else { 3030 if (this.variantsetId == null) 3031 this.variantsetId = new StringType(); 3032 this.variantsetId.setValue(value); 3033 } 3034 return this; 3035 } 3036 3037 /** 3038 * @return {@link #readsetId} (Id of the read in this external repository.). This is the underlying object with id, value and extensions. The accessor "getReadsetId" gives direct access to the value 3039 */ 3040 public StringType getReadsetIdElement() { 3041 if (this.readsetId == null) 3042 if (Configuration.errorOnAutoCreate()) 3043 throw new Error("Attempt to auto-create SequenceRepositoryComponent.readsetId"); 3044 else if (Configuration.doAutoCreate()) 3045 this.readsetId = new StringType(); // bb 3046 return this.readsetId; 3047 } 3048 3049 public boolean hasReadsetIdElement() { 3050 return this.readsetId != null && !this.readsetId.isEmpty(); 3051 } 3052 3053 public boolean hasReadsetId() { 3054 return this.readsetId != null && !this.readsetId.isEmpty(); 3055 } 3056 3057 /** 3058 * @param value {@link #readsetId} (Id of the read in this external repository.). This is the underlying object with id, value and extensions. The accessor "getReadsetId" gives direct access to the value 3059 */ 3060 public SequenceRepositoryComponent setReadsetIdElement(StringType value) { 3061 this.readsetId = value; 3062 return this; 3063 } 3064 3065 /** 3066 * @return Id of the read in this external repository. 3067 */ 3068 public String getReadsetId() { 3069 return this.readsetId == null ? null : this.readsetId.getValue(); 3070 } 3071 3072 /** 3073 * @param value Id of the read in this external repository. 3074 */ 3075 public SequenceRepositoryComponent setReadsetId(String value) { 3076 if (Utilities.noString(value)) 3077 this.readsetId = null; 3078 else { 3079 if (this.readsetId == null) 3080 this.readsetId = new StringType(); 3081 this.readsetId.setValue(value); 3082 } 3083 return this; 3084 } 3085 3086 protected void listChildren(List<Property> children) { 3087 super.listChildren(children); 3088 children.add(new Property("type", "code", "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 0, 1, type)); 3089 children.add(new Property("url", "uri", "URI of an external repository which contains further details about the genetics data.", 0, 1, url)); 3090 children.add(new Property("name", "string", "URI of an external repository which contains further details about the genetics data.", 0, 1, name)); 3091 children.add(new Property("datasetId", "string", "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 0, 1, datasetId)); 3092 children.add(new Property("variantsetId", "string", "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 0, 1, variantsetId)); 3093 children.add(new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId)); 3094 } 3095 3096 @Override 3097 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3098 switch (_hash) { 3099 case 3575610: /*type*/ return new Property("type", "code", "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 0, 1, type); 3100 case 116079: /*url*/ return new Property("url", "uri", "URI of an external repository which contains further details about the genetics data.", 0, 1, url); 3101 case 3373707: /*name*/ return new Property("name", "string", "URI of an external repository which contains further details about the genetics data.", 0, 1, name); 3102 case -345342029: /*datasetId*/ return new Property("datasetId", "string", "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 0, 1, datasetId); 3103 case 1929752504: /*variantsetId*/ return new Property("variantsetId", "string", "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 0, 1, variantsetId); 3104 case -1095407289: /*readsetId*/ return new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId); 3105 default: return super.getNamedProperty(_hash, _name, _checkValid); 3106 } 3107 3108 } 3109 3110 @Override 3111 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3112 switch (hash) { 3113 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<RepositoryType> 3114 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3115 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3116 case -345342029: /*datasetId*/ return this.datasetId == null ? new Base[0] : new Base[] {this.datasetId}; // StringType 3117 case 1929752504: /*variantsetId*/ return this.variantsetId == null ? new Base[0] : new Base[] {this.variantsetId}; // StringType 3118 case -1095407289: /*readsetId*/ return this.readsetId == null ? new Base[0] : new Base[] {this.readsetId}; // StringType 3119 default: return super.getProperty(hash, name, checkValid); 3120 } 3121 3122 } 3123 3124 @Override 3125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3126 switch (hash) { 3127 case 3575610: // type 3128 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 3129 this.type = (Enumeration) value; // Enumeration<RepositoryType> 3130 return value; 3131 case 116079: // url 3132 this.url = castToUri(value); // UriType 3133 return value; 3134 case 3373707: // name 3135 this.name = castToString(value); // StringType 3136 return value; 3137 case -345342029: // datasetId 3138 this.datasetId = castToString(value); // StringType 3139 return value; 3140 case 1929752504: // variantsetId 3141 this.variantsetId = castToString(value); // StringType 3142 return value; 3143 case -1095407289: // readsetId 3144 this.readsetId = castToString(value); // StringType 3145 return value; 3146 default: return super.setProperty(hash, name, value); 3147 } 3148 3149 } 3150 3151 @Override 3152 public Base setProperty(String name, Base value) throws FHIRException { 3153 if (name.equals("type")) { 3154 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 3155 this.type = (Enumeration) value; // Enumeration<RepositoryType> 3156 } else if (name.equals("url")) { 3157 this.url = castToUri(value); // UriType 3158 } else if (name.equals("name")) { 3159 this.name = castToString(value); // StringType 3160 } else if (name.equals("datasetId")) { 3161 this.datasetId = castToString(value); // StringType 3162 } else if (name.equals("variantsetId")) { 3163 this.variantsetId = castToString(value); // StringType 3164 } else if (name.equals("readsetId")) { 3165 this.readsetId = castToString(value); // StringType 3166 } else 3167 return super.setProperty(name, value); 3168 return value; 3169 } 3170 3171 @Override 3172 public Base makeProperty(int hash, String name) throws FHIRException { 3173 switch (hash) { 3174 case 3575610: return getTypeElement(); 3175 case 116079: return getUrlElement(); 3176 case 3373707: return getNameElement(); 3177 case -345342029: return getDatasetIdElement(); 3178 case 1929752504: return getVariantsetIdElement(); 3179 case -1095407289: return getReadsetIdElement(); 3180 default: return super.makeProperty(hash, name); 3181 } 3182 3183 } 3184 3185 @Override 3186 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3187 switch (hash) { 3188 case 3575610: /*type*/ return new String[] {"code"}; 3189 case 116079: /*url*/ return new String[] {"uri"}; 3190 case 3373707: /*name*/ return new String[] {"string"}; 3191 case -345342029: /*datasetId*/ return new String[] {"string"}; 3192 case 1929752504: /*variantsetId*/ return new String[] {"string"}; 3193 case -1095407289: /*readsetId*/ return new String[] {"string"}; 3194 default: return super.getTypesForProperty(hash, name); 3195 } 3196 3197 } 3198 3199 @Override 3200 public Base addChild(String name) throws FHIRException { 3201 if (name.equals("type")) { 3202 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 3203 } 3204 else if (name.equals("url")) { 3205 throw new FHIRException("Cannot call addChild on a singleton property Sequence.url"); 3206 } 3207 else if (name.equals("name")) { 3208 throw new FHIRException("Cannot call addChild on a singleton property Sequence.name"); 3209 } 3210 else if (name.equals("datasetId")) { 3211 throw new FHIRException("Cannot call addChild on a singleton property Sequence.datasetId"); 3212 } 3213 else if (name.equals("variantsetId")) { 3214 throw new FHIRException("Cannot call addChild on a singleton property Sequence.variantsetId"); 3215 } 3216 else if (name.equals("readsetId")) { 3217 throw new FHIRException("Cannot call addChild on a singleton property Sequence.readsetId"); 3218 } 3219 else 3220 return super.addChild(name); 3221 } 3222 3223 public SequenceRepositoryComponent copy() { 3224 SequenceRepositoryComponent dst = new SequenceRepositoryComponent(); 3225 copyValues(dst); 3226 dst.type = type == null ? null : type.copy(); 3227 dst.url = url == null ? null : url.copy(); 3228 dst.name = name == null ? null : name.copy(); 3229 dst.datasetId = datasetId == null ? null : datasetId.copy(); 3230 dst.variantsetId = variantsetId == null ? null : variantsetId.copy(); 3231 dst.readsetId = readsetId == null ? null : readsetId.copy(); 3232 return dst; 3233 } 3234 3235 @Override 3236 public boolean equalsDeep(Base other_) { 3237 if (!super.equalsDeep(other_)) 3238 return false; 3239 if (!(other_ instanceof SequenceRepositoryComponent)) 3240 return false; 3241 SequenceRepositoryComponent o = (SequenceRepositoryComponent) other_; 3242 return compareDeep(type, o.type, true) && compareDeep(url, o.url, true) && compareDeep(name, o.name, true) 3243 && compareDeep(datasetId, o.datasetId, true) && compareDeep(variantsetId, o.variantsetId, true) 3244 && compareDeep(readsetId, o.readsetId, true); 3245 } 3246 3247 @Override 3248 public boolean equalsShallow(Base other_) { 3249 if (!super.equalsShallow(other_)) 3250 return false; 3251 if (!(other_ instanceof SequenceRepositoryComponent)) 3252 return false; 3253 SequenceRepositoryComponent o = (SequenceRepositoryComponent) other_; 3254 return compareValues(type, o.type, true) && compareValues(url, o.url, true) && compareValues(name, o.name, true) 3255 && compareValues(datasetId, o.datasetId, true) && compareValues(variantsetId, o.variantsetId, true) 3256 && compareValues(readsetId, o.readsetId, true); 3257 } 3258 3259 public boolean isEmpty() { 3260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, url, name, datasetId 3261 , variantsetId, readsetId); 3262 } 3263 3264 public String fhirType() { 3265 return "Sequence.repository"; 3266 3267 } 3268 3269 } 3270 3271 /** 3272 * A unique identifier for this particular sequence instance. This is a FHIR-defined id. 3273 */ 3274 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3275 @Description(shortDefinition="Unique ID for this particular sequence. This is a FHIR-defined id", formalDefinition="A unique identifier for this particular sequence instance. This is a FHIR-defined id." ) 3276 protected List<Identifier> identifier; 3277 3278 /** 3279 * Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3280 */ 3281 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3282 @Description(shortDefinition="aa | dna | rna", formalDefinition="Amino Acid Sequence/ DNA Sequence / RNA Sequence." ) 3283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-type") 3284 protected Enumeration<SequenceType> type; 3285 3286 /** 3287 * Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3288 */ 3289 @Child(name = "coordinateSystem", type = {IntegerType.class}, order=2, min=1, max=1, modifier=false, summary=true) 3290 @Description(shortDefinition="Base number of coordinate system (0 for 0-based numbering or coordinates, inclusive start, exclusive end, 1 for 1-based numbering, inclusive start, inclusive end)", formalDefinition="Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end)." ) 3291 protected IntegerType coordinateSystem; 3292 3293 /** 3294 * The patient whose sequencing results are described by this resource. 3295 */ 3296 @Child(name = "patient", type = {Patient.class}, order=3, min=0, max=1, modifier=false, summary=true) 3297 @Description(shortDefinition="Who and/or what this is about", formalDefinition="The patient whose sequencing results are described by this resource." ) 3298 protected Reference patient; 3299 3300 /** 3301 * The actual object that is the target of the reference (The patient whose sequencing results are described by this resource.) 3302 */ 3303 protected Patient patientTarget; 3304 3305 /** 3306 * Specimen used for sequencing. 3307 */ 3308 @Child(name = "specimen", type = {Specimen.class}, order=4, min=0, max=1, modifier=false, summary=true) 3309 @Description(shortDefinition="Specimen used for sequencing", formalDefinition="Specimen used for sequencing." ) 3310 protected Reference specimen; 3311 3312 /** 3313 * The actual object that is the target of the reference (Specimen used for sequencing.) 3314 */ 3315 protected Specimen specimenTarget; 3316 3317 /** 3318 * The method for sequencing, for example, chip information. 3319 */ 3320 @Child(name = "device", type = {Device.class}, order=5, min=0, max=1, modifier=false, summary=true) 3321 @Description(shortDefinition="The method for sequencing", formalDefinition="The method for sequencing, for example, chip information." ) 3322 protected Reference device; 3323 3324 /** 3325 * The actual object that is the target of the reference (The method for sequencing, for example, chip information.) 3326 */ 3327 protected Device deviceTarget; 3328 3329 /** 3330 * The organization or lab that should be responsible for this result. 3331 */ 3332 @Child(name = "performer", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 3333 @Description(shortDefinition="Who should be responsible for test result", formalDefinition="The organization or lab that should be responsible for this result." ) 3334 protected Reference performer; 3335 3336 /** 3337 * The actual object that is the target of the reference (The organization or lab that should be responsible for this result.) 3338 */ 3339 protected Organization performerTarget; 3340 3341 /** 3342 * The number of copies of the seqeunce of interest. (RNASeq). 3343 */ 3344 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=true) 3345 @Description(shortDefinition="The number of copies of the seqeunce of interest. (RNASeq)", formalDefinition="The number of copies of the seqeunce of interest. (RNASeq)." ) 3346 protected Quantity quantity; 3347 3348 /** 3349 * A sequence that is used as a reference to describe variants that are present in a sequence analyzed. 3350 */ 3351 @Child(name = "referenceSeq", type = {}, order=8, min=0, max=1, modifier=false, summary=true) 3352 @Description(shortDefinition="A sequence used as reference", formalDefinition="A sequence that is used as a reference to describe variants that are present in a sequence analyzed." ) 3353 protected SequenceReferenceSeqComponent referenceSeq; 3354 3355 /** 3356 * The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string. 3357 */ 3358 @Child(name = "variant", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3359 @Description(shortDefinition="Variant in sequence", formalDefinition="The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string." ) 3360 protected List<SequenceVariantComponent> variant; 3361 3362 /** 3363 * Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3364 */ 3365 @Child(name = "observedSeq", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3366 @Description(shortDefinition="Sequence that was observed", formalDefinition="Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd." ) 3367 protected StringType observedSeq; 3368 3369 /** 3370 * An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)). 3371 */ 3372 @Child(name = "quality", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3373 @Description(shortDefinition="An set of value as quality of sequence", formalDefinition="An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686))." ) 3374 protected List<SequenceQualityComponent> quality; 3375 3376 /** 3377 * Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3378 */ 3379 @Child(name = "readCoverage", type = {IntegerType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3380 @Description(shortDefinition="Average number of reads representing a given nucleotide in the reconstructed sequence", formalDefinition="Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence." ) 3381 protected IntegerType readCoverage; 3382 3383 /** 3384 * Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq. 3385 */ 3386 @Child(name = "repository", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3387 @Description(shortDefinition="External repository which contains detailed report related with observedSeq in this resource", formalDefinition="Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq." ) 3388 protected List<SequenceRepositoryComponent> repository; 3389 3390 /** 3391 * Pointer to next atomic sequence which at most contains one variant. 3392 */ 3393 @Child(name = "pointer", type = {Sequence.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3394 @Description(shortDefinition="Pointer to next atomic sequence", formalDefinition="Pointer to next atomic sequence which at most contains one variant." ) 3395 protected List<Reference> pointer; 3396 /** 3397 * The actual objects that are the target of the reference (Pointer to next atomic sequence which at most contains one variant.) 3398 */ 3399 protected List<Sequence> pointerTarget; 3400 3401 3402 private static final long serialVersionUID = -2101352712L; 3403 3404 /** 3405 * Constructor 3406 */ 3407 public Sequence() { 3408 super(); 3409 } 3410 3411 /** 3412 * Constructor 3413 */ 3414 public Sequence(IntegerType coordinateSystem) { 3415 super(); 3416 this.coordinateSystem = coordinateSystem; 3417 } 3418 3419 /** 3420 * @return {@link #identifier} (A unique identifier for this particular sequence instance. This is a FHIR-defined id.) 3421 */ 3422 public List<Identifier> getIdentifier() { 3423 if (this.identifier == null) 3424 this.identifier = new ArrayList<Identifier>(); 3425 return this.identifier; 3426 } 3427 3428 /** 3429 * @return Returns a reference to <code>this</code> for easy method chaining 3430 */ 3431 public Sequence setIdentifier(List<Identifier> theIdentifier) { 3432 this.identifier = theIdentifier; 3433 return this; 3434 } 3435 3436 public boolean hasIdentifier() { 3437 if (this.identifier == null) 3438 return false; 3439 for (Identifier item : this.identifier) 3440 if (!item.isEmpty()) 3441 return true; 3442 return false; 3443 } 3444 3445 public Identifier addIdentifier() { //3 3446 Identifier t = new Identifier(); 3447 if (this.identifier == null) 3448 this.identifier = new ArrayList<Identifier>(); 3449 this.identifier.add(t); 3450 return t; 3451 } 3452 3453 public Sequence addIdentifier(Identifier t) { //3 3454 if (t == null) 3455 return this; 3456 if (this.identifier == null) 3457 this.identifier = new ArrayList<Identifier>(); 3458 this.identifier.add(t); 3459 return this; 3460 } 3461 3462 /** 3463 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3464 */ 3465 public Identifier getIdentifierFirstRep() { 3466 if (getIdentifier().isEmpty()) { 3467 addIdentifier(); 3468 } 3469 return getIdentifier().get(0); 3470 } 3471 3472 /** 3473 * @return {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3474 */ 3475 public Enumeration<SequenceType> getTypeElement() { 3476 if (this.type == null) 3477 if (Configuration.errorOnAutoCreate()) 3478 throw new Error("Attempt to auto-create Sequence.type"); 3479 else if (Configuration.doAutoCreate()) 3480 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); // bb 3481 return this.type; 3482 } 3483 3484 public boolean hasTypeElement() { 3485 return this.type != null && !this.type.isEmpty(); 3486 } 3487 3488 public boolean hasType() { 3489 return this.type != null && !this.type.isEmpty(); 3490 } 3491 3492 /** 3493 * @param value {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3494 */ 3495 public Sequence setTypeElement(Enumeration<SequenceType> value) { 3496 this.type = value; 3497 return this; 3498 } 3499 3500 /** 3501 * @return Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3502 */ 3503 public SequenceType getType() { 3504 return this.type == null ? null : this.type.getValue(); 3505 } 3506 3507 /** 3508 * @param value Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3509 */ 3510 public Sequence setType(SequenceType value) { 3511 if (value == null) 3512 this.type = null; 3513 else { 3514 if (this.type == null) 3515 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); 3516 this.type.setValue(value); 3517 } 3518 return this; 3519 } 3520 3521 /** 3522 * @return {@link #coordinateSystem} (Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).). This is the underlying object with id, value and extensions. The accessor "getCoordinateSystem" gives direct access to the value 3523 */ 3524 public IntegerType getCoordinateSystemElement() { 3525 if (this.coordinateSystem == null) 3526 if (Configuration.errorOnAutoCreate()) 3527 throw new Error("Attempt to auto-create Sequence.coordinateSystem"); 3528 else if (Configuration.doAutoCreate()) 3529 this.coordinateSystem = new IntegerType(); // bb 3530 return this.coordinateSystem; 3531 } 3532 3533 public boolean hasCoordinateSystemElement() { 3534 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 3535 } 3536 3537 public boolean hasCoordinateSystem() { 3538 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 3539 } 3540 3541 /** 3542 * @param value {@link #coordinateSystem} (Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).). This is the underlying object with id, value and extensions. The accessor "getCoordinateSystem" gives direct access to the value 3543 */ 3544 public Sequence setCoordinateSystemElement(IntegerType value) { 3545 this.coordinateSystem = value; 3546 return this; 3547 } 3548 3549 /** 3550 * @return Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3551 */ 3552 public int getCoordinateSystem() { 3553 return this.coordinateSystem == null || this.coordinateSystem.isEmpty() ? 0 : this.coordinateSystem.getValue(); 3554 } 3555 3556 /** 3557 * @param value Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3558 */ 3559 public Sequence setCoordinateSystem(int value) { 3560 if (this.coordinateSystem == null) 3561 this.coordinateSystem = new IntegerType(); 3562 this.coordinateSystem.setValue(value); 3563 return this; 3564 } 3565 3566 /** 3567 * @return {@link #patient} (The patient whose sequencing results are described by this resource.) 3568 */ 3569 public Reference getPatient() { 3570 if (this.patient == null) 3571 if (Configuration.errorOnAutoCreate()) 3572 throw new Error("Attempt to auto-create Sequence.patient"); 3573 else if (Configuration.doAutoCreate()) 3574 this.patient = new Reference(); // cc 3575 return this.patient; 3576 } 3577 3578 public boolean hasPatient() { 3579 return this.patient != null && !this.patient.isEmpty(); 3580 } 3581 3582 /** 3583 * @param value {@link #patient} (The patient whose sequencing results are described by this resource.) 3584 */ 3585 public Sequence setPatient(Reference value) { 3586 this.patient = value; 3587 return this; 3588 } 3589 3590 /** 3591 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient whose sequencing results are described by this resource.) 3592 */ 3593 public Patient getPatientTarget() { 3594 if (this.patientTarget == null) 3595 if (Configuration.errorOnAutoCreate()) 3596 throw new Error("Attempt to auto-create Sequence.patient"); 3597 else if (Configuration.doAutoCreate()) 3598 this.patientTarget = new Patient(); // aa 3599 return this.patientTarget; 3600 } 3601 3602 /** 3603 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient whose sequencing results are described by this resource.) 3604 */ 3605 public Sequence setPatientTarget(Patient value) { 3606 this.patientTarget = value; 3607 return this; 3608 } 3609 3610 /** 3611 * @return {@link #specimen} (Specimen used for sequencing.) 3612 */ 3613 public Reference getSpecimen() { 3614 if (this.specimen == null) 3615 if (Configuration.errorOnAutoCreate()) 3616 throw new Error("Attempt to auto-create Sequence.specimen"); 3617 else if (Configuration.doAutoCreate()) 3618 this.specimen = new Reference(); // cc 3619 return this.specimen; 3620 } 3621 3622 public boolean hasSpecimen() { 3623 return this.specimen != null && !this.specimen.isEmpty(); 3624 } 3625 3626 /** 3627 * @param value {@link #specimen} (Specimen used for sequencing.) 3628 */ 3629 public Sequence setSpecimen(Reference value) { 3630 this.specimen = value; 3631 return this; 3632 } 3633 3634 /** 3635 * @return {@link #specimen} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Specimen used for sequencing.) 3636 */ 3637 public Specimen getSpecimenTarget() { 3638 if (this.specimenTarget == null) 3639 if (Configuration.errorOnAutoCreate()) 3640 throw new Error("Attempt to auto-create Sequence.specimen"); 3641 else if (Configuration.doAutoCreate()) 3642 this.specimenTarget = new Specimen(); // aa 3643 return this.specimenTarget; 3644 } 3645 3646 /** 3647 * @param value {@link #specimen} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Specimen used for sequencing.) 3648 */ 3649 public Sequence setSpecimenTarget(Specimen value) { 3650 this.specimenTarget = value; 3651 return this; 3652 } 3653 3654 /** 3655 * @return {@link #device} (The method for sequencing, for example, chip information.) 3656 */ 3657 public Reference getDevice() { 3658 if (this.device == null) 3659 if (Configuration.errorOnAutoCreate()) 3660 throw new Error("Attempt to auto-create Sequence.device"); 3661 else if (Configuration.doAutoCreate()) 3662 this.device = new Reference(); // cc 3663 return this.device; 3664 } 3665 3666 public boolean hasDevice() { 3667 return this.device != null && !this.device.isEmpty(); 3668 } 3669 3670 /** 3671 * @param value {@link #device} (The method for sequencing, for example, chip information.) 3672 */ 3673 public Sequence setDevice(Reference value) { 3674 this.device = value; 3675 return this; 3676 } 3677 3678 /** 3679 * @return {@link #device} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The method for sequencing, for example, chip information.) 3680 */ 3681 public Device getDeviceTarget() { 3682 if (this.deviceTarget == null) 3683 if (Configuration.errorOnAutoCreate()) 3684 throw new Error("Attempt to auto-create Sequence.device"); 3685 else if (Configuration.doAutoCreate()) 3686 this.deviceTarget = new Device(); // aa 3687 return this.deviceTarget; 3688 } 3689 3690 /** 3691 * @param value {@link #device} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The method for sequencing, for example, chip information.) 3692 */ 3693 public Sequence setDeviceTarget(Device value) { 3694 this.deviceTarget = value; 3695 return this; 3696 } 3697 3698 /** 3699 * @return {@link #performer} (The organization or lab that should be responsible for this result.) 3700 */ 3701 public Reference getPerformer() { 3702 if (this.performer == null) 3703 if (Configuration.errorOnAutoCreate()) 3704 throw new Error("Attempt to auto-create Sequence.performer"); 3705 else if (Configuration.doAutoCreate()) 3706 this.performer = new Reference(); // cc 3707 return this.performer; 3708 } 3709 3710 public boolean hasPerformer() { 3711 return this.performer != null && !this.performer.isEmpty(); 3712 } 3713 3714 /** 3715 * @param value {@link #performer} (The organization or lab that should be responsible for this result.) 3716 */ 3717 public Sequence setPerformer(Reference value) { 3718 this.performer = value; 3719 return this; 3720 } 3721 3722 /** 3723 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization or lab that should be responsible for this result.) 3724 */ 3725 public Organization getPerformerTarget() { 3726 if (this.performerTarget == null) 3727 if (Configuration.errorOnAutoCreate()) 3728 throw new Error("Attempt to auto-create Sequence.performer"); 3729 else if (Configuration.doAutoCreate()) 3730 this.performerTarget = new Organization(); // aa 3731 return this.performerTarget; 3732 } 3733 3734 /** 3735 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization or lab that should be responsible for this result.) 3736 */ 3737 public Sequence setPerformerTarget(Organization value) { 3738 this.performerTarget = value; 3739 return this; 3740 } 3741 3742 /** 3743 * @return {@link #quantity} (The number of copies of the seqeunce of interest. (RNASeq).) 3744 */ 3745 public Quantity getQuantity() { 3746 if (this.quantity == null) 3747 if (Configuration.errorOnAutoCreate()) 3748 throw new Error("Attempt to auto-create Sequence.quantity"); 3749 else if (Configuration.doAutoCreate()) 3750 this.quantity = new Quantity(); // cc 3751 return this.quantity; 3752 } 3753 3754 public boolean hasQuantity() { 3755 return this.quantity != null && !this.quantity.isEmpty(); 3756 } 3757 3758 /** 3759 * @param value {@link #quantity} (The number of copies of the seqeunce of interest. (RNASeq).) 3760 */ 3761 public Sequence setQuantity(Quantity value) { 3762 this.quantity = value; 3763 return this; 3764 } 3765 3766 /** 3767 * @return {@link #referenceSeq} (A sequence that is used as a reference to describe variants that are present in a sequence analyzed.) 3768 */ 3769 public SequenceReferenceSeqComponent getReferenceSeq() { 3770 if (this.referenceSeq == null) 3771 if (Configuration.errorOnAutoCreate()) 3772 throw new Error("Attempt to auto-create Sequence.referenceSeq"); 3773 else if (Configuration.doAutoCreate()) 3774 this.referenceSeq = new SequenceReferenceSeqComponent(); // cc 3775 return this.referenceSeq; 3776 } 3777 3778 public boolean hasReferenceSeq() { 3779 return this.referenceSeq != null && !this.referenceSeq.isEmpty(); 3780 } 3781 3782 /** 3783 * @param value {@link #referenceSeq} (A sequence that is used as a reference to describe variants that are present in a sequence analyzed.) 3784 */ 3785 public Sequence setReferenceSeq(SequenceReferenceSeqComponent value) { 3786 this.referenceSeq = value; 3787 return this; 3788 } 3789 3790 /** 3791 * @return {@link #variant} (The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.) 3792 */ 3793 public List<SequenceVariantComponent> getVariant() { 3794 if (this.variant == null) 3795 this.variant = new ArrayList<SequenceVariantComponent>(); 3796 return this.variant; 3797 } 3798 3799 /** 3800 * @return Returns a reference to <code>this</code> for easy method chaining 3801 */ 3802 public Sequence setVariant(List<SequenceVariantComponent> theVariant) { 3803 this.variant = theVariant; 3804 return this; 3805 } 3806 3807 public boolean hasVariant() { 3808 if (this.variant == null) 3809 return false; 3810 for (SequenceVariantComponent item : this.variant) 3811 if (!item.isEmpty()) 3812 return true; 3813 return false; 3814 } 3815 3816 public SequenceVariantComponent addVariant() { //3 3817 SequenceVariantComponent t = new SequenceVariantComponent(); 3818 if (this.variant == null) 3819 this.variant = new ArrayList<SequenceVariantComponent>(); 3820 this.variant.add(t); 3821 return t; 3822 } 3823 3824 public Sequence addVariant(SequenceVariantComponent t) { //3 3825 if (t == null) 3826 return this; 3827 if (this.variant == null) 3828 this.variant = new ArrayList<SequenceVariantComponent>(); 3829 this.variant.add(t); 3830 return this; 3831 } 3832 3833 /** 3834 * @return The first repetition of repeating field {@link #variant}, creating it if it does not already exist 3835 */ 3836 public SequenceVariantComponent getVariantFirstRep() { 3837 if (getVariant().isEmpty()) { 3838 addVariant(); 3839 } 3840 return getVariant().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #observedSeq} (Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.). This is the underlying object with id, value and extensions. The accessor "getObservedSeq" gives direct access to the value 3845 */ 3846 public StringType getObservedSeqElement() { 3847 if (this.observedSeq == null) 3848 if (Configuration.errorOnAutoCreate()) 3849 throw new Error("Attempt to auto-create Sequence.observedSeq"); 3850 else if (Configuration.doAutoCreate()) 3851 this.observedSeq = new StringType(); // bb 3852 return this.observedSeq; 3853 } 3854 3855 public boolean hasObservedSeqElement() { 3856 return this.observedSeq != null && !this.observedSeq.isEmpty(); 3857 } 3858 3859 public boolean hasObservedSeq() { 3860 return this.observedSeq != null && !this.observedSeq.isEmpty(); 3861 } 3862 3863 /** 3864 * @param value {@link #observedSeq} (Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.). This is the underlying object with id, value and extensions. The accessor "getObservedSeq" gives direct access to the value 3865 */ 3866 public Sequence setObservedSeqElement(StringType value) { 3867 this.observedSeq = value; 3868 return this; 3869 } 3870 3871 /** 3872 * @return Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3873 */ 3874 public String getObservedSeq() { 3875 return this.observedSeq == null ? null : this.observedSeq.getValue(); 3876 } 3877 3878 /** 3879 * @param value Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3880 */ 3881 public Sequence setObservedSeq(String value) { 3882 if (Utilities.noString(value)) 3883 this.observedSeq = null; 3884 else { 3885 if (this.observedSeq == null) 3886 this.observedSeq = new StringType(); 3887 this.observedSeq.setValue(value); 3888 } 3889 return this; 3890 } 3891 3892 /** 3893 * @return {@link #quality} (An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).) 3894 */ 3895 public List<SequenceQualityComponent> getQuality() { 3896 if (this.quality == null) 3897 this.quality = new ArrayList<SequenceQualityComponent>(); 3898 return this.quality; 3899 } 3900 3901 /** 3902 * @return Returns a reference to <code>this</code> for easy method chaining 3903 */ 3904 public Sequence setQuality(List<SequenceQualityComponent> theQuality) { 3905 this.quality = theQuality; 3906 return this; 3907 } 3908 3909 public boolean hasQuality() { 3910 if (this.quality == null) 3911 return false; 3912 for (SequenceQualityComponent item : this.quality) 3913 if (!item.isEmpty()) 3914 return true; 3915 return false; 3916 } 3917 3918 public SequenceQualityComponent addQuality() { //3 3919 SequenceQualityComponent t = new SequenceQualityComponent(); 3920 if (this.quality == null) 3921 this.quality = new ArrayList<SequenceQualityComponent>(); 3922 this.quality.add(t); 3923 return t; 3924 } 3925 3926 public Sequence addQuality(SequenceQualityComponent t) { //3 3927 if (t == null) 3928 return this; 3929 if (this.quality == null) 3930 this.quality = new ArrayList<SequenceQualityComponent>(); 3931 this.quality.add(t); 3932 return this; 3933 } 3934 3935 /** 3936 * @return The first repetition of repeating field {@link #quality}, creating it if it does not already exist 3937 */ 3938 public SequenceQualityComponent getQualityFirstRep() { 3939 if (getQuality().isEmpty()) { 3940 addQuality(); 3941 } 3942 return getQuality().get(0); 3943 } 3944 3945 /** 3946 * @return {@link #readCoverage} (Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.). This is the underlying object with id, value and extensions. The accessor "getReadCoverage" gives direct access to the value 3947 */ 3948 public IntegerType getReadCoverageElement() { 3949 if (this.readCoverage == null) 3950 if (Configuration.errorOnAutoCreate()) 3951 throw new Error("Attempt to auto-create Sequence.readCoverage"); 3952 else if (Configuration.doAutoCreate()) 3953 this.readCoverage = new IntegerType(); // bb 3954 return this.readCoverage; 3955 } 3956 3957 public boolean hasReadCoverageElement() { 3958 return this.readCoverage != null && !this.readCoverage.isEmpty(); 3959 } 3960 3961 public boolean hasReadCoverage() { 3962 return this.readCoverage != null && !this.readCoverage.isEmpty(); 3963 } 3964 3965 /** 3966 * @param value {@link #readCoverage} (Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.). This is the underlying object with id, value and extensions. The accessor "getReadCoverage" gives direct access to the value 3967 */ 3968 public Sequence setReadCoverageElement(IntegerType value) { 3969 this.readCoverage = value; 3970 return this; 3971 } 3972 3973 /** 3974 * @return Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3975 */ 3976 public int getReadCoverage() { 3977 return this.readCoverage == null || this.readCoverage.isEmpty() ? 0 : this.readCoverage.getValue(); 3978 } 3979 3980 /** 3981 * @param value Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3982 */ 3983 public Sequence setReadCoverage(int value) { 3984 if (this.readCoverage == null) 3985 this.readCoverage = new IntegerType(); 3986 this.readCoverage.setValue(value); 3987 return this; 3988 } 3989 3990 /** 3991 * @return {@link #repository} (Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.) 3992 */ 3993 public List<SequenceRepositoryComponent> getRepository() { 3994 if (this.repository == null) 3995 this.repository = new ArrayList<SequenceRepositoryComponent>(); 3996 return this.repository; 3997 } 3998 3999 /** 4000 * @return Returns a reference to <code>this</code> for easy method chaining 4001 */ 4002 public Sequence setRepository(List<SequenceRepositoryComponent> theRepository) { 4003 this.repository = theRepository; 4004 return this; 4005 } 4006 4007 public boolean hasRepository() { 4008 if (this.repository == null) 4009 return false; 4010 for (SequenceRepositoryComponent item : this.repository) 4011 if (!item.isEmpty()) 4012 return true; 4013 return false; 4014 } 4015 4016 public SequenceRepositoryComponent addRepository() { //3 4017 SequenceRepositoryComponent t = new SequenceRepositoryComponent(); 4018 if (this.repository == null) 4019 this.repository = new ArrayList<SequenceRepositoryComponent>(); 4020 this.repository.add(t); 4021 return t; 4022 } 4023 4024 public Sequence addRepository(SequenceRepositoryComponent t) { //3 4025 if (t == null) 4026 return this; 4027 if (this.repository == null) 4028 this.repository = new ArrayList<SequenceRepositoryComponent>(); 4029 this.repository.add(t); 4030 return this; 4031 } 4032 4033 /** 4034 * @return The first repetition of repeating field {@link #repository}, creating it if it does not already exist 4035 */ 4036 public SequenceRepositoryComponent getRepositoryFirstRep() { 4037 if (getRepository().isEmpty()) { 4038 addRepository(); 4039 } 4040 return getRepository().get(0); 4041 } 4042 4043 /** 4044 * @return {@link #pointer} (Pointer to next atomic sequence which at most contains one variant.) 4045 */ 4046 public List<Reference> getPointer() { 4047 if (this.pointer == null) 4048 this.pointer = new ArrayList<Reference>(); 4049 return this.pointer; 4050 } 4051 4052 /** 4053 * @return Returns a reference to <code>this</code> for easy method chaining 4054 */ 4055 public Sequence setPointer(List<Reference> thePointer) { 4056 this.pointer = thePointer; 4057 return this; 4058 } 4059 4060 public boolean hasPointer() { 4061 if (this.pointer == null) 4062 return false; 4063 for (Reference item : this.pointer) 4064 if (!item.isEmpty()) 4065 return true; 4066 return false; 4067 } 4068 4069 public Reference addPointer() { //3 4070 Reference t = new Reference(); 4071 if (this.pointer == null) 4072 this.pointer = new ArrayList<Reference>(); 4073 this.pointer.add(t); 4074 return t; 4075 } 4076 4077 public Sequence addPointer(Reference t) { //3 4078 if (t == null) 4079 return this; 4080 if (this.pointer == null) 4081 this.pointer = new ArrayList<Reference>(); 4082 this.pointer.add(t); 4083 return this; 4084 } 4085 4086 /** 4087 * @return The first repetition of repeating field {@link #pointer}, creating it if it does not already exist 4088 */ 4089 public Reference getPointerFirstRep() { 4090 if (getPointer().isEmpty()) { 4091 addPointer(); 4092 } 4093 return getPointer().get(0); 4094 } 4095 4096 /** 4097 * @deprecated Use Reference#setResource(IBaseResource) instead 4098 */ 4099 @Deprecated 4100 public List<Sequence> getPointerTarget() { 4101 if (this.pointerTarget == null) 4102 this.pointerTarget = new ArrayList<Sequence>(); 4103 return this.pointerTarget; 4104 } 4105 4106 /** 4107 * @deprecated Use Reference#setResource(IBaseResource) instead 4108 */ 4109 @Deprecated 4110 public Sequence addPointerTarget() { 4111 Sequence r = new Sequence(); 4112 if (this.pointerTarget == null) 4113 this.pointerTarget = new ArrayList<Sequence>(); 4114 this.pointerTarget.add(r); 4115 return r; 4116 } 4117 4118 protected void listChildren(List<Property> children) { 4119 super.listChildren(children); 4120 children.add(new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4121 children.add(new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type)); 4122 children.add(new Property("coordinateSystem", "integer", "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 0, 1, coordinateSystem)); 4123 children.add(new Property("patient", "Reference(Patient)", "The patient whose sequencing results are described by this resource.", 0, 1, patient)); 4124 children.add(new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen)); 4125 children.add(new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device)); 4126 children.add(new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer)); 4127 children.add(new Property("quantity", "Quantity", "The number of copies of the seqeunce of interest. (RNASeq).", 0, 1, quantity)); 4128 children.add(new Property("referenceSeq", "", "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, referenceSeq)); 4129 children.add(new Property("variant", "", "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 0, java.lang.Integer.MAX_VALUE, variant)); 4130 children.add(new Property("observedSeq", "string", "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 0, 1, observedSeq)); 4131 children.add(new Property("quality", "", "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 0, java.lang.Integer.MAX_VALUE, quality)); 4132 children.add(new Property("readCoverage", "integer", "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 0, 1, readCoverage)); 4133 children.add(new Property("repository", "", "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 0, java.lang.Integer.MAX_VALUE, repository)); 4134 children.add(new Property("pointer", "Reference(Sequence)", "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, pointer)); 4135 } 4136 4137 @Override 4138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4139 switch (_hash) { 4140 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, java.lang.Integer.MAX_VALUE, identifier); 4141 case 3575610: /*type*/ return new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type); 4142 case 354212295: /*coordinateSystem*/ return new Property("coordinateSystem", "integer", "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 0, 1, coordinateSystem); 4143 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient whose sequencing results are described by this resource.", 0, 1, patient); 4144 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen); 4145 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device); 4146 case 481140686: /*performer*/ return new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer); 4147 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of copies of the seqeunce of interest. (RNASeq).", 0, 1, quantity); 4148 case -502547180: /*referenceSeq*/ return new Property("referenceSeq", "", "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, referenceSeq); 4149 case 236785797: /*variant*/ return new Property("variant", "", "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 0, java.lang.Integer.MAX_VALUE, variant); 4150 case 125541495: /*observedSeq*/ return new Property("observedSeq", "string", "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 0, 1, observedSeq); 4151 case 651215103: /*quality*/ return new Property("quality", "", "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 0, java.lang.Integer.MAX_VALUE, quality); 4152 case -1798816354: /*readCoverage*/ return new Property("readCoverage", "integer", "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 0, 1, readCoverage); 4153 case 1950800714: /*repository*/ return new Property("repository", "", "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 0, java.lang.Integer.MAX_VALUE, repository); 4154 case -400605635: /*pointer*/ return new Property("pointer", "Reference(Sequence)", "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, pointer); 4155 default: return super.getNamedProperty(_hash, _name, _checkValid); 4156 } 4157 4158 } 4159 4160 @Override 4161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4162 switch (hash) { 4163 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4164 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SequenceType> 4165 case 354212295: /*coordinateSystem*/ return this.coordinateSystem == null ? new Base[0] : new Base[] {this.coordinateSystem}; // IntegerType 4166 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 4167 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 4168 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 4169 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 4170 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4171 case -502547180: /*referenceSeq*/ return this.referenceSeq == null ? new Base[0] : new Base[] {this.referenceSeq}; // SequenceReferenceSeqComponent 4172 case 236785797: /*variant*/ return this.variant == null ? new Base[0] : this.variant.toArray(new Base[this.variant.size()]); // SequenceVariantComponent 4173 case 125541495: /*observedSeq*/ return this.observedSeq == null ? new Base[0] : new Base[] {this.observedSeq}; // StringType 4174 case 651215103: /*quality*/ return this.quality == null ? new Base[0] : this.quality.toArray(new Base[this.quality.size()]); // SequenceQualityComponent 4175 case -1798816354: /*readCoverage*/ return this.readCoverage == null ? new Base[0] : new Base[] {this.readCoverage}; // IntegerType 4176 case 1950800714: /*repository*/ return this.repository == null ? new Base[0] : this.repository.toArray(new Base[this.repository.size()]); // SequenceRepositoryComponent 4177 case -400605635: /*pointer*/ return this.pointer == null ? new Base[0] : this.pointer.toArray(new Base[this.pointer.size()]); // Reference 4178 default: return super.getProperty(hash, name, checkValid); 4179 } 4180 4181 } 4182 4183 @Override 4184 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4185 switch (hash) { 4186 case -1618432855: // identifier 4187 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4188 return value; 4189 case 3575610: // type 4190 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 4191 this.type = (Enumeration) value; // Enumeration<SequenceType> 4192 return value; 4193 case 354212295: // coordinateSystem 4194 this.coordinateSystem = castToInteger(value); // IntegerType 4195 return value; 4196 case -791418107: // patient 4197 this.patient = castToReference(value); // Reference 4198 return value; 4199 case -2132868344: // specimen 4200 this.specimen = castToReference(value); // Reference 4201 return value; 4202 case -1335157162: // device 4203 this.device = castToReference(value); // Reference 4204 return value; 4205 case 481140686: // performer 4206 this.performer = castToReference(value); // Reference 4207 return value; 4208 case -1285004149: // quantity 4209 this.quantity = castToQuantity(value); // Quantity 4210 return value; 4211 case -502547180: // referenceSeq 4212 this.referenceSeq = (SequenceReferenceSeqComponent) value; // SequenceReferenceSeqComponent 4213 return value; 4214 case 236785797: // variant 4215 this.getVariant().add((SequenceVariantComponent) value); // SequenceVariantComponent 4216 return value; 4217 case 125541495: // observedSeq 4218 this.observedSeq = castToString(value); // StringType 4219 return value; 4220 case 651215103: // quality 4221 this.getQuality().add((SequenceQualityComponent) value); // SequenceQualityComponent 4222 return value; 4223 case -1798816354: // readCoverage 4224 this.readCoverage = castToInteger(value); // IntegerType 4225 return value; 4226 case 1950800714: // repository 4227 this.getRepository().add((SequenceRepositoryComponent) value); // SequenceRepositoryComponent 4228 return value; 4229 case -400605635: // pointer 4230 this.getPointer().add(castToReference(value)); // Reference 4231 return value; 4232 default: return super.setProperty(hash, name, value); 4233 } 4234 4235 } 4236 4237 @Override 4238 public Base setProperty(String name, Base value) throws FHIRException { 4239 if (name.equals("identifier")) { 4240 this.getIdentifier().add(castToIdentifier(value)); 4241 } else if (name.equals("type")) { 4242 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 4243 this.type = (Enumeration) value; // Enumeration<SequenceType> 4244 } else if (name.equals("coordinateSystem")) { 4245 this.coordinateSystem = castToInteger(value); // IntegerType 4246 } else if (name.equals("patient")) { 4247 this.patient = castToReference(value); // Reference 4248 } else if (name.equals("specimen")) { 4249 this.specimen = castToReference(value); // Reference 4250 } else if (name.equals("device")) { 4251 this.device = castToReference(value); // Reference 4252 } else if (name.equals("performer")) { 4253 this.performer = castToReference(value); // Reference 4254 } else if (name.equals("quantity")) { 4255 this.quantity = castToQuantity(value); // Quantity 4256 } else if (name.equals("referenceSeq")) { 4257 this.referenceSeq = (SequenceReferenceSeqComponent) value; // SequenceReferenceSeqComponent 4258 } else if (name.equals("variant")) { 4259 this.getVariant().add((SequenceVariantComponent) value); 4260 } else if (name.equals("observedSeq")) { 4261 this.observedSeq = castToString(value); // StringType 4262 } else if (name.equals("quality")) { 4263 this.getQuality().add((SequenceQualityComponent) value); 4264 } else if (name.equals("readCoverage")) { 4265 this.readCoverage = castToInteger(value); // IntegerType 4266 } else if (name.equals("repository")) { 4267 this.getRepository().add((SequenceRepositoryComponent) value); 4268 } else if (name.equals("pointer")) { 4269 this.getPointer().add(castToReference(value)); 4270 } else 4271 return super.setProperty(name, value); 4272 return value; 4273 } 4274 4275 @Override 4276 public Base makeProperty(int hash, String name) throws FHIRException { 4277 switch (hash) { 4278 case -1618432855: return addIdentifier(); 4279 case 3575610: return getTypeElement(); 4280 case 354212295: return getCoordinateSystemElement(); 4281 case -791418107: return getPatient(); 4282 case -2132868344: return getSpecimen(); 4283 case -1335157162: return getDevice(); 4284 case 481140686: return getPerformer(); 4285 case -1285004149: return getQuantity(); 4286 case -502547180: return getReferenceSeq(); 4287 case 236785797: return addVariant(); 4288 case 125541495: return getObservedSeqElement(); 4289 case 651215103: return addQuality(); 4290 case -1798816354: return getReadCoverageElement(); 4291 case 1950800714: return addRepository(); 4292 case -400605635: return addPointer(); 4293 default: return super.makeProperty(hash, name); 4294 } 4295 4296 } 4297 4298 @Override 4299 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4300 switch (hash) { 4301 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4302 case 3575610: /*type*/ return new String[] {"code"}; 4303 case 354212295: /*coordinateSystem*/ return new String[] {"integer"}; 4304 case -791418107: /*patient*/ return new String[] {"Reference"}; 4305 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 4306 case -1335157162: /*device*/ return new String[] {"Reference"}; 4307 case 481140686: /*performer*/ return new String[] {"Reference"}; 4308 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4309 case -502547180: /*referenceSeq*/ return new String[] {}; 4310 case 236785797: /*variant*/ return new String[] {}; 4311 case 125541495: /*observedSeq*/ return new String[] {"string"}; 4312 case 651215103: /*quality*/ return new String[] {}; 4313 case -1798816354: /*readCoverage*/ return new String[] {"integer"}; 4314 case 1950800714: /*repository*/ return new String[] {}; 4315 case -400605635: /*pointer*/ return new String[] {"Reference"}; 4316 default: return super.getTypesForProperty(hash, name); 4317 } 4318 4319 } 4320 4321 @Override 4322 public Base addChild(String name) throws FHIRException { 4323 if (name.equals("identifier")) { 4324 return addIdentifier(); 4325 } 4326 else if (name.equals("type")) { 4327 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 4328 } 4329 else if (name.equals("coordinateSystem")) { 4330 throw new FHIRException("Cannot call addChild on a singleton property Sequence.coordinateSystem"); 4331 } 4332 else if (name.equals("patient")) { 4333 this.patient = new Reference(); 4334 return this.patient; 4335 } 4336 else if (name.equals("specimen")) { 4337 this.specimen = new Reference(); 4338 return this.specimen; 4339 } 4340 else if (name.equals("device")) { 4341 this.device = new Reference(); 4342 return this.device; 4343 } 4344 else if (name.equals("performer")) { 4345 this.performer = new Reference(); 4346 return this.performer; 4347 } 4348 else if (name.equals("quantity")) { 4349 this.quantity = new Quantity(); 4350 return this.quantity; 4351 } 4352 else if (name.equals("referenceSeq")) { 4353 this.referenceSeq = new SequenceReferenceSeqComponent(); 4354 return this.referenceSeq; 4355 } 4356 else if (name.equals("variant")) { 4357 return addVariant(); 4358 } 4359 else if (name.equals("observedSeq")) { 4360 throw new FHIRException("Cannot call addChild on a singleton property Sequence.observedSeq"); 4361 } 4362 else if (name.equals("quality")) { 4363 return addQuality(); 4364 } 4365 else if (name.equals("readCoverage")) { 4366 throw new FHIRException("Cannot call addChild on a singleton property Sequence.readCoverage"); 4367 } 4368 else if (name.equals("repository")) { 4369 return addRepository(); 4370 } 4371 else if (name.equals("pointer")) { 4372 return addPointer(); 4373 } 4374 else 4375 return super.addChild(name); 4376 } 4377 4378 public String fhirType() { 4379 return "Sequence"; 4380 4381 } 4382 4383 public Sequence copy() { 4384 Sequence dst = new Sequence(); 4385 copyValues(dst); 4386 if (identifier != null) { 4387 dst.identifier = new ArrayList<Identifier>(); 4388 for (Identifier i : identifier) 4389 dst.identifier.add(i.copy()); 4390 }; 4391 dst.type = type == null ? null : type.copy(); 4392 dst.coordinateSystem = coordinateSystem == null ? null : coordinateSystem.copy(); 4393 dst.patient = patient == null ? null : patient.copy(); 4394 dst.specimen = specimen == null ? null : specimen.copy(); 4395 dst.device = device == null ? null : device.copy(); 4396 dst.performer = performer == null ? null : performer.copy(); 4397 dst.quantity = quantity == null ? null : quantity.copy(); 4398 dst.referenceSeq = referenceSeq == null ? null : referenceSeq.copy(); 4399 if (variant != null) { 4400 dst.variant = new ArrayList<SequenceVariantComponent>(); 4401 for (SequenceVariantComponent i : variant) 4402 dst.variant.add(i.copy()); 4403 }; 4404 dst.observedSeq = observedSeq == null ? null : observedSeq.copy(); 4405 if (quality != null) { 4406 dst.quality = new ArrayList<SequenceQualityComponent>(); 4407 for (SequenceQualityComponent i : quality) 4408 dst.quality.add(i.copy()); 4409 }; 4410 dst.readCoverage = readCoverage == null ? null : readCoverage.copy(); 4411 if (repository != null) { 4412 dst.repository = new ArrayList<SequenceRepositoryComponent>(); 4413 for (SequenceRepositoryComponent i : repository) 4414 dst.repository.add(i.copy()); 4415 }; 4416 if (pointer != null) { 4417 dst.pointer = new ArrayList<Reference>(); 4418 for (Reference i : pointer) 4419 dst.pointer.add(i.copy()); 4420 }; 4421 return dst; 4422 } 4423 4424 protected Sequence typedCopy() { 4425 return copy(); 4426 } 4427 4428 @Override 4429 public boolean equalsDeep(Base other_) { 4430 if (!super.equalsDeep(other_)) 4431 return false; 4432 if (!(other_ instanceof Sequence)) 4433 return false; 4434 Sequence o = (Sequence) other_; 4435 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(coordinateSystem, o.coordinateSystem, true) 4436 && compareDeep(patient, o.patient, true) && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4437 && compareDeep(performer, o.performer, true) && compareDeep(quantity, o.quantity, true) && compareDeep(referenceSeq, o.referenceSeq, true) 4438 && compareDeep(variant, o.variant, true) && compareDeep(observedSeq, o.observedSeq, true) && compareDeep(quality, o.quality, true) 4439 && compareDeep(readCoverage, o.readCoverage, true) && compareDeep(repository, o.repository, true) 4440 && compareDeep(pointer, o.pointer, true); 4441 } 4442 4443 @Override 4444 public boolean equalsShallow(Base other_) { 4445 if (!super.equalsShallow(other_)) 4446 return false; 4447 if (!(other_ instanceof Sequence)) 4448 return false; 4449 Sequence o = (Sequence) other_; 4450 return compareValues(type, o.type, true) && compareValues(coordinateSystem, o.coordinateSystem, true) 4451 && compareValues(observedSeq, o.observedSeq, true) && compareValues(readCoverage, o.readCoverage, true) 4452 ; 4453 } 4454 4455 public boolean isEmpty() { 4456 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coordinateSystem 4457 , patient, specimen, device, performer, quantity, referenceSeq, variant, observedSeq 4458 , quality, readCoverage, repository, pointer); 4459 } 4460 4461 @Override 4462 public ResourceType getResourceType() { 4463 return ResourceType.Sequence; 4464 } 4465 4466 /** 4467 * Search parameter: <b>identifier</b> 4468 * <p> 4469 * Description: <b>The unique identity for a particular sequence</b><br> 4470 * Type: <b>token</b><br> 4471 * Path: <b>Sequence.identifier</b><br> 4472 * </p> 4473 */ 4474 @SearchParamDefinition(name="identifier", path="Sequence.identifier", description="The unique identity for a particular sequence", type="token" ) 4475 public static final String SP_IDENTIFIER = "identifier"; 4476 /** 4477 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4478 * <p> 4479 * Description: <b>The unique identity for a particular sequence</b><br> 4480 * Type: <b>token</b><br> 4481 * Path: <b>Sequence.identifier</b><br> 4482 * </p> 4483 */ 4484 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4485 4486 /** 4487 * Search parameter: <b>coordinate</b> 4488 * <p> 4489 * Description: <b>Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.</b><br> 4490 * Type: <b>composite</b><br> 4491 * Path: <b></b><br> 4492 * </p> 4493 */ 4494 @SearchParamDefinition(name="coordinate", path="Sequence.variant", description="Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type="composite", compositeOf={"chromosome", "start"} ) 4495 public static final String SP_COORDINATE = "coordinate"; 4496 /** 4497 * <b>Fluent Client</b> search parameter constant for <b>coordinate</b> 4498 * <p> 4499 * Description: <b>Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.</b><br> 4500 * Type: <b>composite</b><br> 4501 * Path: <b></b><br> 4502 * </p> 4503 */ 4504 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>(SP_COORDINATE); 4505 4506 /** 4507 * Search parameter: <b>patient</b> 4508 * <p> 4509 * Description: <b>The subject that the observation is about</b><br> 4510 * Type: <b>reference</b><br> 4511 * Path: <b>Sequence.patient</b><br> 4512 * </p> 4513 */ 4514 @SearchParamDefinition(name="patient", path="Sequence.patient", description="The subject that the observation is about", type="reference", target={Patient.class } ) 4515 public static final String SP_PATIENT = "patient"; 4516 /** 4517 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4518 * <p> 4519 * Description: <b>The subject that the observation is about</b><br> 4520 * Type: <b>reference</b><br> 4521 * Path: <b>Sequence.patient</b><br> 4522 * </p> 4523 */ 4524 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4525 4526/** 4527 * Constant for fluent queries to be used to add include statements. Specifies 4528 * the path value of "<b>Sequence:patient</b>". 4529 */ 4530 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Sequence:patient").toLocked(); 4531 4532 /** 4533 * Search parameter: <b>chromosome</b> 4534 * <p> 4535 * Description: <b>Chromosome number of the reference sequence</b><br> 4536 * Type: <b>token</b><br> 4537 * Path: <b>Sequence.referenceSeq.chromosome</b><br> 4538 * </p> 4539 */ 4540 @SearchParamDefinition(name="chromosome", path="Sequence.referenceSeq.chromosome", description="Chromosome number of the reference sequence", type="token" ) 4541 public static final String SP_CHROMOSOME = "chromosome"; 4542 /** 4543 * <b>Fluent Client</b> search parameter constant for <b>chromosome</b> 4544 * <p> 4545 * Description: <b>Chromosome number of the reference sequence</b><br> 4546 * Type: <b>token</b><br> 4547 * Path: <b>Sequence.referenceSeq.chromosome</b><br> 4548 * </p> 4549 */ 4550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHROMOSOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHROMOSOME); 4551 4552 /** 4553 * Search parameter: <b>start</b> 4554 * <p> 4555 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.</b><br> 4556 * Type: <b>number</b><br> 4557 * Path: <b>Sequence.referenceSeq.windowStart</b><br> 4558 * </p> 4559 */ 4560 @SearchParamDefinition(name="start", path="Sequence.referenceSeq.windowStart", description="Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.", type="number" ) 4561 public static final String SP_START = "start"; 4562 /** 4563 * <b>Fluent Client</b> search parameter constant for <b>start</b> 4564 * <p> 4565 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.</b><br> 4566 * Type: <b>number</b><br> 4567 * Path: <b>Sequence.referenceSeq.windowStart</b><br> 4568 * </p> 4569 */ 4570 public static final ca.uhn.fhir.rest.gclient.NumberClientParam START = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_START); 4571 4572 /** 4573 * Search parameter: <b>end</b> 4574 * <p> 4575 * Description: <b>End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.</b><br> 4576 * Type: <b>number</b><br> 4577 * Path: <b>Sequence.referenceSeq.windowEnd</b><br> 4578 * </p> 4579 */ 4580 @SearchParamDefinition(name="end", path="Sequence.referenceSeq.windowEnd", description="End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.", type="number" ) 4581 public static final String SP_END = "end"; 4582 /** 4583 * <b>Fluent Client</b> search parameter constant for <b>end</b> 4584 * <p> 4585 * Description: <b>End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.</b><br> 4586 * Type: <b>number</b><br> 4587 * Path: <b>Sequence.referenceSeq.windowEnd</b><br> 4588 * </p> 4589 */ 4590 public static final ca.uhn.fhir.rest.gclient.NumberClientParam END = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_END); 4591 4592 /** 4593 * Search parameter: <b>type</b> 4594 * <p> 4595 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 4596 * Type: <b>token</b><br> 4597 * Path: <b>Sequence.type</b><br> 4598 * </p> 4599 */ 4600 @SearchParamDefinition(name="type", path="Sequence.type", description="Amino Acid Sequence/ DNA Sequence / RNA Sequence", type="token" ) 4601 public static final String SP_TYPE = "type"; 4602 /** 4603 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4604 * <p> 4605 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 4606 * Type: <b>token</b><br> 4607 * Path: <b>Sequence.type</b><br> 4608 * </p> 4609 */ 4610 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4611 4612 4613}