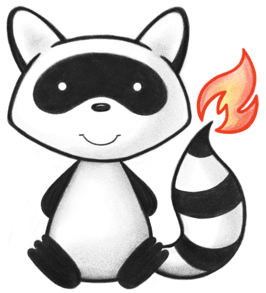
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Raw data describing a biological sequence. 049 */ 050@ResourceDef(name="Sequence", profile="http://hl7.org/fhir/Profile/Sequence") 051public class Sequence extends DomainResource { 052 053 public enum SequenceType { 054 /** 055 * Amino acid sequence 056 */ 057 AA, 058 /** 059 * DNA Sequence 060 */ 061 DNA, 062 /** 063 * RNA Sequence 064 */ 065 RNA, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static SequenceType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("aa".equals(codeString)) 074 return AA; 075 if ("dna".equals(codeString)) 076 return DNA; 077 if ("rna".equals(codeString)) 078 return RNA; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case AA: return "aa"; 087 case DNA: return "dna"; 088 case RNA: return "rna"; 089 case NULL: return null; 090 default: return "?"; 091 } 092 } 093 public String getSystem() { 094 switch (this) { 095 case AA: return "http://hl7.org/fhir/sequence-type"; 096 case DNA: return "http://hl7.org/fhir/sequence-type"; 097 case RNA: return "http://hl7.org/fhir/sequence-type"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getDefinition() { 103 switch (this) { 104 case AA: return "Amino acid sequence"; 105 case DNA: return "DNA Sequence"; 106 case RNA: return "RNA Sequence"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDisplay() { 112 switch (this) { 113 case AA: return "AA Sequence"; 114 case DNA: return "DNA Sequence"; 115 case RNA: return "RNA Sequence"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 } 121 122 public static class SequenceTypeEnumFactory implements EnumFactory<SequenceType> { 123 public SequenceType fromCode(String codeString) throws IllegalArgumentException { 124 if (codeString == null || "".equals(codeString)) 125 if (codeString == null || "".equals(codeString)) 126 return null; 127 if ("aa".equals(codeString)) 128 return SequenceType.AA; 129 if ("dna".equals(codeString)) 130 return SequenceType.DNA; 131 if ("rna".equals(codeString)) 132 return SequenceType.RNA; 133 throw new IllegalArgumentException("Unknown SequenceType code '"+codeString+"'"); 134 } 135 public Enumeration<SequenceType> fromType(PrimitiveType<?> code) throws FHIRException { 136 if (code == null) 137 return null; 138 if (code.isEmpty()) 139 return new Enumeration<SequenceType>(this); 140 String codeString = code.asStringValue(); 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("aa".equals(codeString)) 144 return new Enumeration<SequenceType>(this, SequenceType.AA); 145 if ("dna".equals(codeString)) 146 return new Enumeration<SequenceType>(this, SequenceType.DNA); 147 if ("rna".equals(codeString)) 148 return new Enumeration<SequenceType>(this, SequenceType.RNA); 149 throw new FHIRException("Unknown SequenceType code '"+codeString+"'"); 150 } 151 public String toCode(SequenceType code) { 152 if (code == SequenceType.NULL) 153 return null; 154 if (code == SequenceType.AA) 155 return "aa"; 156 if (code == SequenceType.DNA) 157 return "dna"; 158 if (code == SequenceType.RNA) 159 return "rna"; 160 return "?"; 161 } 162 public String toSystem(SequenceType code) { 163 return code.getSystem(); 164 } 165 } 166 167 public enum QualityType { 168 /** 169 * INDEL Comparison 170 */ 171 INDEL, 172 /** 173 * SNP Comparison 174 */ 175 SNP, 176 /** 177 * UNKNOWN Comparison 178 */ 179 UNKNOWN, 180 /** 181 * added to help the parsers with the generic types 182 */ 183 NULL; 184 public static QualityType fromCode(String codeString) throws FHIRException { 185 if (codeString == null || "".equals(codeString)) 186 return null; 187 if ("indel".equals(codeString)) 188 return INDEL; 189 if ("snp".equals(codeString)) 190 return SNP; 191 if ("unknown".equals(codeString)) 192 return UNKNOWN; 193 if (Configuration.isAcceptInvalidEnums()) 194 return null; 195 else 196 throw new FHIRException("Unknown QualityType code '"+codeString+"'"); 197 } 198 public String toCode() { 199 switch (this) { 200 case INDEL: return "indel"; 201 case SNP: return "snp"; 202 case UNKNOWN: return "unknown"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 public String getSystem() { 208 switch (this) { 209 case INDEL: return "http://hl7.org/fhir/quality-type"; 210 case SNP: return "http://hl7.org/fhir/quality-type"; 211 case UNKNOWN: return "http://hl7.org/fhir/quality-type"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 public String getDefinition() { 217 switch (this) { 218 case INDEL: return "INDEL Comparison"; 219 case SNP: return "SNP Comparison"; 220 case UNKNOWN: return "UNKNOWN Comparison"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 public String getDisplay() { 226 switch (this) { 227 case INDEL: return "INDEL Comparison"; 228 case SNP: return "SNP Comparison"; 229 case UNKNOWN: return "UNKNOWN Comparison"; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 } 235 236 public static class QualityTypeEnumFactory implements EnumFactory<QualityType> { 237 public QualityType fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("indel".equals(codeString)) 242 return QualityType.INDEL; 243 if ("snp".equals(codeString)) 244 return QualityType.SNP; 245 if ("unknown".equals(codeString)) 246 return QualityType.UNKNOWN; 247 throw new IllegalArgumentException("Unknown QualityType code '"+codeString+"'"); 248 } 249 public Enumeration<QualityType> fromType(PrimitiveType<?> code) throws FHIRException { 250 if (code == null) 251 return null; 252 if (code.isEmpty()) 253 return new Enumeration<QualityType>(this); 254 String codeString = code.asStringValue(); 255 if (codeString == null || "".equals(codeString)) 256 return null; 257 if ("indel".equals(codeString)) 258 return new Enumeration<QualityType>(this, QualityType.INDEL); 259 if ("snp".equals(codeString)) 260 return new Enumeration<QualityType>(this, QualityType.SNP); 261 if ("unknown".equals(codeString)) 262 return new Enumeration<QualityType>(this, QualityType.UNKNOWN); 263 throw new FHIRException("Unknown QualityType code '"+codeString+"'"); 264 } 265 public String toCode(QualityType code) { 266 if (code == QualityType.NULL) 267 return null; 268 if (code == QualityType.INDEL) 269 return "indel"; 270 if (code == QualityType.SNP) 271 return "snp"; 272 if (code == QualityType.UNKNOWN) 273 return "unknown"; 274 return "?"; 275 } 276 public String toSystem(QualityType code) { 277 return code.getSystem(); 278 } 279 } 280 281 public enum RepositoryType { 282 /** 283 * When URL is clicked, the resource can be seen directly (by webpage or by download link format) 284 */ 285 DIRECTLINK, 286 /** 287 * When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format) 288 */ 289 OPENAPI, 290 /** 291 * When logged into the website, the resource can be seen. 292 */ 293 LOGIN, 294 /** 295 * When logged in and follow the API in the website related with URL, the resource can be seen. 296 */ 297 OAUTH, 298 /** 299 * Some other complicated or particular way to get resource from URL. 300 */ 301 OTHER, 302 /** 303 * added to help the parsers with the generic types 304 */ 305 NULL; 306 public static RepositoryType fromCode(String codeString) throws FHIRException { 307 if (codeString == null || "".equals(codeString)) 308 return null; 309 if ("directlink".equals(codeString)) 310 return DIRECTLINK; 311 if ("openapi".equals(codeString)) 312 return OPENAPI; 313 if ("login".equals(codeString)) 314 return LOGIN; 315 if ("oauth".equals(codeString)) 316 return OAUTH; 317 if ("other".equals(codeString)) 318 return OTHER; 319 if (Configuration.isAcceptInvalidEnums()) 320 return null; 321 else 322 throw new FHIRException("Unknown RepositoryType code '"+codeString+"'"); 323 } 324 public String toCode() { 325 switch (this) { 326 case DIRECTLINK: return "directlink"; 327 case OPENAPI: return "openapi"; 328 case LOGIN: return "login"; 329 case OAUTH: return "oauth"; 330 case OTHER: return "other"; 331 case NULL: return null; 332 default: return "?"; 333 } 334 } 335 public String getSystem() { 336 switch (this) { 337 case DIRECTLINK: return "http://hl7.org/fhir/repository-type"; 338 case OPENAPI: return "http://hl7.org/fhir/repository-type"; 339 case LOGIN: return "http://hl7.org/fhir/repository-type"; 340 case OAUTH: return "http://hl7.org/fhir/repository-type"; 341 case OTHER: return "http://hl7.org/fhir/repository-type"; 342 case NULL: return null; 343 default: return "?"; 344 } 345 } 346 public String getDefinition() { 347 switch (this) { 348 case DIRECTLINK: return "When URL is clicked, the resource can be seen directly (by webpage or by download link format)"; 349 case OPENAPI: return "When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format)"; 350 case LOGIN: return "When logged into the website, the resource can be seen."; 351 case OAUTH: return "When logged in and follow the API in the website related with URL, the resource can be seen."; 352 case OTHER: return "Some other complicated or particular way to get resource from URL."; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 public String getDisplay() { 358 switch (this) { 359 case DIRECTLINK: return "Click and see"; 360 case OPENAPI: return "The URL is the RESTful or other kind of API that can access to the result."; 361 case LOGIN: return "Result cannot be access unless an account is logged in"; 362 case OAUTH: return "Result need to be fetched with API and need LOGIN( or cookies are required when visiting the link of resource)"; 363 case OTHER: return "Some other complicated or particular way to get resource from URL."; 364 case NULL: return null; 365 default: return "?"; 366 } 367 } 368 } 369 370 public static class RepositoryTypeEnumFactory implements EnumFactory<RepositoryType> { 371 public RepositoryType fromCode(String codeString) throws IllegalArgumentException { 372 if (codeString == null || "".equals(codeString)) 373 if (codeString == null || "".equals(codeString)) 374 return null; 375 if ("directlink".equals(codeString)) 376 return RepositoryType.DIRECTLINK; 377 if ("openapi".equals(codeString)) 378 return RepositoryType.OPENAPI; 379 if ("login".equals(codeString)) 380 return RepositoryType.LOGIN; 381 if ("oauth".equals(codeString)) 382 return RepositoryType.OAUTH; 383 if ("other".equals(codeString)) 384 return RepositoryType.OTHER; 385 throw new IllegalArgumentException("Unknown RepositoryType code '"+codeString+"'"); 386 } 387 public Enumeration<RepositoryType> fromType(PrimitiveType<?> code) throws FHIRException { 388 if (code == null) 389 return null; 390 if (code.isEmpty()) 391 return new Enumeration<RepositoryType>(this); 392 String codeString = code.asStringValue(); 393 if (codeString == null || "".equals(codeString)) 394 return null; 395 if ("directlink".equals(codeString)) 396 return new Enumeration<RepositoryType>(this, RepositoryType.DIRECTLINK); 397 if ("openapi".equals(codeString)) 398 return new Enumeration<RepositoryType>(this, RepositoryType.OPENAPI); 399 if ("login".equals(codeString)) 400 return new Enumeration<RepositoryType>(this, RepositoryType.LOGIN); 401 if ("oauth".equals(codeString)) 402 return new Enumeration<RepositoryType>(this, RepositoryType.OAUTH); 403 if ("other".equals(codeString)) 404 return new Enumeration<RepositoryType>(this, RepositoryType.OTHER); 405 throw new FHIRException("Unknown RepositoryType code '"+codeString+"'"); 406 } 407 public String toCode(RepositoryType code) { 408 if (code == RepositoryType.NULL) 409 return null; 410 if (code == RepositoryType.DIRECTLINK) 411 return "directlink"; 412 if (code == RepositoryType.OPENAPI) 413 return "openapi"; 414 if (code == RepositoryType.LOGIN) 415 return "login"; 416 if (code == RepositoryType.OAUTH) 417 return "oauth"; 418 if (code == RepositoryType.OTHER) 419 return "other"; 420 return "?"; 421 } 422 public String toSystem(RepositoryType code) { 423 return code.getSystem(); 424 } 425 } 426 427 @Block() 428 public static class SequenceReferenceSeqComponent extends BackboneElement implements IBaseBackboneElement { 429 /** 430 * Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)). 431 */ 432 @Child(name = "chromosome", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 433 @Description(shortDefinition="Chromosome containing genetic finding", formalDefinition="Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340))." ) 434 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chromosome-human") 435 protected CodeableConcept chromosome; 436 437 /** 438 * The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 439 */ 440 @Child(name = "genomeBuild", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 441 @Description(shortDefinition="The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'", formalDefinition="The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used." ) 442 protected StringType genomeBuild; 443 444 /** 445 * Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences. 446 */ 447 @Child(name = "referenceSeqId", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 448 @Description(shortDefinition="Reference identifier", formalDefinition="Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences." ) 449 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-referenceSeq") 450 protected CodeableConcept referenceSeqId; 451 452 /** 453 * A Pointer to another Sequence entity as reference sequence. 454 */ 455 @Child(name = "referenceSeqPointer", type = {Sequence.class}, order=4, min=0, max=1, modifier=false, summary=true) 456 @Description(shortDefinition="A Pointer to another Sequence entity as reference sequence", formalDefinition="A Pointer to another Sequence entity as reference sequence." ) 457 protected Reference referenceSeqPointer; 458 459 /** 460 * The actual object that is the target of the reference (A Pointer to another Sequence entity as reference sequence.) 461 */ 462 protected Sequence referenceSeqPointerTarget; 463 464 /** 465 * A string like "ACGT". 466 */ 467 @Child(name = "referenceSeqString", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 468 @Description(shortDefinition="A string to represent reference sequence", formalDefinition="A string like \"ACGT\"." ) 469 protected StringType referenceSeqString; 470 471 /** 472 * Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 473 */ 474 @Child(name = "strand", type = {IntegerType.class}, order=6, min=0, max=1, modifier=false, summary=true) 475 @Description(shortDefinition="Directionality of DNA ( +1/-1)", formalDefinition="Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative." ) 476 protected IntegerType strand; 477 478 /** 479 * Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 480 */ 481 @Child(name = "windowStart", type = {IntegerType.class}, order=7, min=1, max=1, modifier=false, summary=true) 482 @Description(shortDefinition="Start position of the window on the reference sequence", formalDefinition="Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 483 protected IntegerType windowStart; 484 485 /** 486 * End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 487 */ 488 @Child(name = "windowEnd", type = {IntegerType.class}, order=8, min=1, max=1, modifier=false, summary=true) 489 @Description(shortDefinition="End position of the window on the reference sequence", formalDefinition="End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 490 protected IntegerType windowEnd; 491 492 private static final long serialVersionUID = -1675617731L; 493 494 /** 495 * Constructor 496 */ 497 public SequenceReferenceSeqComponent() { 498 super(); 499 } 500 501 /** 502 * Constructor 503 */ 504 public SequenceReferenceSeqComponent(IntegerType windowStart, IntegerType windowEnd) { 505 super(); 506 this.windowStart = windowStart; 507 this.windowEnd = windowEnd; 508 } 509 510 /** 511 * @return {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 512 */ 513 public CodeableConcept getChromosome() { 514 if (this.chromosome == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.chromosome"); 517 else if (Configuration.doAutoCreate()) 518 this.chromosome = new CodeableConcept(); // cc 519 return this.chromosome; 520 } 521 522 public boolean hasChromosome() { 523 return this.chromosome != null && !this.chromosome.isEmpty(); 524 } 525 526 /** 527 * @param value {@link #chromosome} (Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 528 */ 529 public SequenceReferenceSeqComponent setChromosome(CodeableConcept value) { 530 this.chromosome = value; 531 return this; 532 } 533 534 /** 535 * @return {@link #genomeBuild} (The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.). This is the underlying object with id, value and extensions. The accessor "getGenomeBuild" gives direct access to the value 536 */ 537 public StringType getGenomeBuildElement() { 538 if (this.genomeBuild == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.genomeBuild"); 541 else if (Configuration.doAutoCreate()) 542 this.genomeBuild = new StringType(); // bb 543 return this.genomeBuild; 544 } 545 546 public boolean hasGenomeBuildElement() { 547 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 548 } 549 550 public boolean hasGenomeBuild() { 551 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 552 } 553 554 /** 555 * @param value {@link #genomeBuild} (The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.). This is the underlying object with id, value and extensions. The accessor "getGenomeBuild" gives direct access to the value 556 */ 557 public SequenceReferenceSeqComponent setGenomeBuildElement(StringType value) { 558 this.genomeBuild = value; 559 return this; 560 } 561 562 /** 563 * @return The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 564 */ 565 public String getGenomeBuild() { 566 return this.genomeBuild == null ? null : this.genomeBuild.getValue(); 567 } 568 569 /** 570 * @param value The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used. 571 */ 572 public SequenceReferenceSeqComponent setGenomeBuild(String value) { 573 if (Utilities.noString(value)) 574 this.genomeBuild = null; 575 else { 576 if (this.genomeBuild == null) 577 this.genomeBuild = new StringType(); 578 this.genomeBuild.setValue(value); 579 } 580 return this; 581 } 582 583 /** 584 * @return {@link #referenceSeqId} (Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.) 585 */ 586 public CodeableConcept getReferenceSeqId() { 587 if (this.referenceSeqId == null) 588 if (Configuration.errorOnAutoCreate()) 589 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqId"); 590 else if (Configuration.doAutoCreate()) 591 this.referenceSeqId = new CodeableConcept(); // cc 592 return this.referenceSeqId; 593 } 594 595 public boolean hasReferenceSeqId() { 596 return this.referenceSeqId != null && !this.referenceSeqId.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #referenceSeqId} (Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.) 601 */ 602 public SequenceReferenceSeqComponent setReferenceSeqId(CodeableConcept value) { 603 this.referenceSeqId = value; 604 return this; 605 } 606 607 /** 608 * @return {@link #referenceSeqPointer} (A Pointer to another Sequence entity as reference sequence.) 609 */ 610 public Reference getReferenceSeqPointer() { 611 if (this.referenceSeqPointer == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqPointer"); 614 else if (Configuration.doAutoCreate()) 615 this.referenceSeqPointer = new Reference(); // cc 616 return this.referenceSeqPointer; 617 } 618 619 public boolean hasReferenceSeqPointer() { 620 return this.referenceSeqPointer != null && !this.referenceSeqPointer.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #referenceSeqPointer} (A Pointer to another Sequence entity as reference sequence.) 625 */ 626 public SequenceReferenceSeqComponent setReferenceSeqPointer(Reference value) { 627 this.referenceSeqPointer = value; 628 return this; 629 } 630 631 /** 632 * @return {@link #referenceSeqPointer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A Pointer to another Sequence entity as reference sequence.) 633 */ 634 public Sequence getReferenceSeqPointerTarget() { 635 if (this.referenceSeqPointerTarget == null) 636 if (Configuration.errorOnAutoCreate()) 637 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqPointer"); 638 else if (Configuration.doAutoCreate()) 639 this.referenceSeqPointerTarget = new Sequence(); // aa 640 return this.referenceSeqPointerTarget; 641 } 642 643 /** 644 * @param value {@link #referenceSeqPointer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A Pointer to another Sequence entity as reference sequence.) 645 */ 646 public SequenceReferenceSeqComponent setReferenceSeqPointerTarget(Sequence value) { 647 this.referenceSeqPointerTarget = value; 648 return this; 649 } 650 651 /** 652 * @return {@link #referenceSeqString} (A string like "ACGT".). This is the underlying object with id, value and extensions. The accessor "getReferenceSeqString" gives direct access to the value 653 */ 654 public StringType getReferenceSeqStringElement() { 655 if (this.referenceSeqString == null) 656 if (Configuration.errorOnAutoCreate()) 657 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.referenceSeqString"); 658 else if (Configuration.doAutoCreate()) 659 this.referenceSeqString = new StringType(); // bb 660 return this.referenceSeqString; 661 } 662 663 public boolean hasReferenceSeqStringElement() { 664 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 665 } 666 667 public boolean hasReferenceSeqString() { 668 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 669 } 670 671 /** 672 * @param value {@link #referenceSeqString} (A string like "ACGT".). This is the underlying object with id, value and extensions. The accessor "getReferenceSeqString" gives direct access to the value 673 */ 674 public SequenceReferenceSeqComponent setReferenceSeqStringElement(StringType value) { 675 this.referenceSeqString = value; 676 return this; 677 } 678 679 /** 680 * @return A string like "ACGT". 681 */ 682 public String getReferenceSeqString() { 683 return this.referenceSeqString == null ? null : this.referenceSeqString.getValue(); 684 } 685 686 /** 687 * @param value A string like "ACGT". 688 */ 689 public SequenceReferenceSeqComponent setReferenceSeqString(String value) { 690 if (Utilities.noString(value)) 691 this.referenceSeqString = null; 692 else { 693 if (this.referenceSeqString == null) 694 this.referenceSeqString = new StringType(); 695 this.referenceSeqString.setValue(value); 696 } 697 return this; 698 } 699 700 /** 701 * @return {@link #strand} (Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 702 */ 703 public IntegerType getStrandElement() { 704 if (this.strand == null) 705 if (Configuration.errorOnAutoCreate()) 706 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.strand"); 707 else if (Configuration.doAutoCreate()) 708 this.strand = new IntegerType(); // bb 709 return this.strand; 710 } 711 712 public boolean hasStrandElement() { 713 return this.strand != null && !this.strand.isEmpty(); 714 } 715 716 public boolean hasStrand() { 717 return this.strand != null && !this.strand.isEmpty(); 718 } 719 720 /** 721 * @param value {@link #strand} (Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative.). This is the underlying object with id, value and extensions. The accessor "getStrand" gives direct access to the value 722 */ 723 public SequenceReferenceSeqComponent setStrandElement(IntegerType value) { 724 this.strand = value; 725 return this; 726 } 727 728 /** 729 * @return Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 730 */ 731 public int getStrand() { 732 return this.strand == null || this.strand.isEmpty() ? 0 : this.strand.getValue(); 733 } 734 735 /** 736 * @param value Directionality of DNA sequence. Available values are "1" for the plus strand (5' to 3')/Watson/Sense/positive and "-1" for the minus strand(3' to 5')/Crick/Antisense/negative. 737 */ 738 public SequenceReferenceSeqComponent setStrand(int value) { 739 if (this.strand == null) 740 this.strand = new IntegerType(); 741 this.strand.setValue(value); 742 return this; 743 } 744 745 /** 746 * @return {@link #windowStart} (Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 747 */ 748 public IntegerType getWindowStartElement() { 749 if (this.windowStart == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.windowStart"); 752 else if (Configuration.doAutoCreate()) 753 this.windowStart = new IntegerType(); // bb 754 return this.windowStart; 755 } 756 757 public boolean hasWindowStartElement() { 758 return this.windowStart != null && !this.windowStart.isEmpty(); 759 } 760 761 public boolean hasWindowStart() { 762 return this.windowStart != null && !this.windowStart.isEmpty(); 763 } 764 765 /** 766 * @param value {@link #windowStart} (Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getWindowStart" gives direct access to the value 767 */ 768 public SequenceReferenceSeqComponent setWindowStartElement(IntegerType value) { 769 this.windowStart = value; 770 return this; 771 } 772 773 /** 774 * @return Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 775 */ 776 public int getWindowStart() { 777 return this.windowStart == null || this.windowStart.isEmpty() ? 0 : this.windowStart.getValue(); 778 } 779 780 /** 781 * @param value Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 782 */ 783 public SequenceReferenceSeqComponent setWindowStart(int value) { 784 if (this.windowStart == null) 785 this.windowStart = new IntegerType(); 786 this.windowStart.setValue(value); 787 return this; 788 } 789 790 /** 791 * @return {@link #windowEnd} (End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 792 */ 793 public IntegerType getWindowEndElement() { 794 if (this.windowEnd == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create SequenceReferenceSeqComponent.windowEnd"); 797 else if (Configuration.doAutoCreate()) 798 this.windowEnd = new IntegerType(); // bb 799 return this.windowEnd; 800 } 801 802 public boolean hasWindowEndElement() { 803 return this.windowEnd != null && !this.windowEnd.isEmpty(); 804 } 805 806 public boolean hasWindowEnd() { 807 return this.windowEnd != null && !this.windowEnd.isEmpty(); 808 } 809 810 /** 811 * @param value {@link #windowEnd} (End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getWindowEnd" gives direct access to the value 812 */ 813 public SequenceReferenceSeqComponent setWindowEndElement(IntegerType value) { 814 this.windowEnd = value; 815 return this; 816 } 817 818 /** 819 * @return End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 820 */ 821 public int getWindowEnd() { 822 return this.windowEnd == null || this.windowEnd.isEmpty() ? 0 : this.windowEnd.getValue(); 823 } 824 825 /** 826 * @param value End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 827 */ 828 public SequenceReferenceSeqComponent setWindowEnd(int value) { 829 if (this.windowEnd == null) 830 this.windowEnd = new IntegerType(); 831 this.windowEnd.setValue(value); 832 return this; 833 } 834 835 protected void listChildren(List<Property> children) { 836 super.listChildren(children); 837 children.add(new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome)); 838 children.add(new Property("genomeBuild", "string", "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 0, 1, genomeBuild)); 839 children.add(new Property("referenceSeqId", "CodeableConcept", "Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 0, 1, referenceSeqId)); 840 children.add(new Property("referenceSeqPointer", "Reference(Sequence)", "A Pointer to another Sequence entity as reference sequence.", 0, 1, referenceSeqPointer)); 841 children.add(new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString)); 842 children.add(new Property("strand", "integer", "Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative.", 0, 1, strand)); 843 children.add(new Property("windowStart", "integer", "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, windowStart)); 844 children.add(new Property("windowEnd", "integer", "End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, windowEnd)); 845 } 846 847 @Override 848 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 849 switch (_hash) { 850 case -1499470472: /*chromosome*/ return new Property("chromosome", "CodeableConcept", "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 0, 1, chromosome); 851 case 1061239735: /*genomeBuild*/ return new Property("genomeBuild", "string", "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 0, 1, genomeBuild); 852 case -1911500465: /*referenceSeqId*/ return new Property("referenceSeqId", "CodeableConcept", "Reference identifier of reference sequence submitted to NCBI. It must match the type in the Sequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 0, 1, referenceSeqId); 853 case 1923414665: /*referenceSeqPointer*/ return new Property("referenceSeqPointer", "Reference(Sequence)", "A Pointer to another Sequence entity as reference sequence.", 0, 1, referenceSeqPointer); 854 case -1648301499: /*referenceSeqString*/ return new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString); 855 case -891993594: /*strand*/ return new Property("strand", "integer", "Directionality of DNA sequence. Available values are \"1\" for the plus strand (5' to 3')/Watson/Sense/positive and \"-1\" for the minus strand(3' to 5')/Crick/Antisense/negative.", 0, 1, strand); 856 case 1903685202: /*windowStart*/ return new Property("windowStart", "integer", "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, windowStart); 857 case -217026869: /*windowEnd*/ return new Property("windowEnd", "integer", "End position of the window on the reference sequence. If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, windowEnd); 858 default: return super.getNamedProperty(_hash, _name, _checkValid); 859 } 860 861 } 862 863 @Override 864 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 865 switch (hash) { 866 case -1499470472: /*chromosome*/ return this.chromosome == null ? new Base[0] : new Base[] {this.chromosome}; // CodeableConcept 867 case 1061239735: /*genomeBuild*/ return this.genomeBuild == null ? new Base[0] : new Base[] {this.genomeBuild}; // StringType 868 case -1911500465: /*referenceSeqId*/ return this.referenceSeqId == null ? new Base[0] : new Base[] {this.referenceSeqId}; // CodeableConcept 869 case 1923414665: /*referenceSeqPointer*/ return this.referenceSeqPointer == null ? new Base[0] : new Base[] {this.referenceSeqPointer}; // Reference 870 case -1648301499: /*referenceSeqString*/ return this.referenceSeqString == null ? new Base[0] : new Base[] {this.referenceSeqString}; // StringType 871 case -891993594: /*strand*/ return this.strand == null ? new Base[0] : new Base[] {this.strand}; // IntegerType 872 case 1903685202: /*windowStart*/ return this.windowStart == null ? new Base[0] : new Base[] {this.windowStart}; // IntegerType 873 case -217026869: /*windowEnd*/ return this.windowEnd == null ? new Base[0] : new Base[] {this.windowEnd}; // IntegerType 874 default: return super.getProperty(hash, name, checkValid); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 881 switch (hash) { 882 case -1499470472: // chromosome 883 this.chromosome = castToCodeableConcept(value); // CodeableConcept 884 return value; 885 case 1061239735: // genomeBuild 886 this.genomeBuild = castToString(value); // StringType 887 return value; 888 case -1911500465: // referenceSeqId 889 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 890 return value; 891 case 1923414665: // referenceSeqPointer 892 this.referenceSeqPointer = castToReference(value); // Reference 893 return value; 894 case -1648301499: // referenceSeqString 895 this.referenceSeqString = castToString(value); // StringType 896 return value; 897 case -891993594: // strand 898 this.strand = castToInteger(value); // IntegerType 899 return value; 900 case 1903685202: // windowStart 901 this.windowStart = castToInteger(value); // IntegerType 902 return value; 903 case -217026869: // windowEnd 904 this.windowEnd = castToInteger(value); // IntegerType 905 return value; 906 default: return super.setProperty(hash, name, value); 907 } 908 909 } 910 911 @Override 912 public Base setProperty(String name, Base value) throws FHIRException { 913 if (name.equals("chromosome")) { 914 this.chromosome = castToCodeableConcept(value); // CodeableConcept 915 } else if (name.equals("genomeBuild")) { 916 this.genomeBuild = castToString(value); // StringType 917 } else if (name.equals("referenceSeqId")) { 918 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 919 } else if (name.equals("referenceSeqPointer")) { 920 this.referenceSeqPointer = castToReference(value); // Reference 921 } else if (name.equals("referenceSeqString")) { 922 this.referenceSeqString = castToString(value); // StringType 923 } else if (name.equals("strand")) { 924 this.strand = castToInteger(value); // IntegerType 925 } else if (name.equals("windowStart")) { 926 this.windowStart = castToInteger(value); // IntegerType 927 } else if (name.equals("windowEnd")) { 928 this.windowEnd = castToInteger(value); // IntegerType 929 } else 930 return super.setProperty(name, value); 931 return value; 932 } 933 934 @Override 935 public Base makeProperty(int hash, String name) throws FHIRException { 936 switch (hash) { 937 case -1499470472: return getChromosome(); 938 case 1061239735: return getGenomeBuildElement(); 939 case -1911500465: return getReferenceSeqId(); 940 case 1923414665: return getReferenceSeqPointer(); 941 case -1648301499: return getReferenceSeqStringElement(); 942 case -891993594: return getStrandElement(); 943 case 1903685202: return getWindowStartElement(); 944 case -217026869: return getWindowEndElement(); 945 default: return super.makeProperty(hash, name); 946 } 947 948 } 949 950 @Override 951 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 952 switch (hash) { 953 case -1499470472: /*chromosome*/ return new String[] {"CodeableConcept"}; 954 case 1061239735: /*genomeBuild*/ return new String[] {"string"}; 955 case -1911500465: /*referenceSeqId*/ return new String[] {"CodeableConcept"}; 956 case 1923414665: /*referenceSeqPointer*/ return new String[] {"Reference"}; 957 case -1648301499: /*referenceSeqString*/ return new String[] {"string"}; 958 case -891993594: /*strand*/ return new String[] {"integer"}; 959 case 1903685202: /*windowStart*/ return new String[] {"integer"}; 960 case -217026869: /*windowEnd*/ return new String[] {"integer"}; 961 default: return super.getTypesForProperty(hash, name); 962 } 963 964 } 965 966 @Override 967 public Base addChild(String name) throws FHIRException { 968 if (name.equals("chromosome")) { 969 this.chromosome = new CodeableConcept(); 970 return this.chromosome; 971 } 972 else if (name.equals("genomeBuild")) { 973 throw new FHIRException("Cannot call addChild on a singleton property Sequence.genomeBuild"); 974 } 975 else if (name.equals("referenceSeqId")) { 976 this.referenceSeqId = new CodeableConcept(); 977 return this.referenceSeqId; 978 } 979 else if (name.equals("referenceSeqPointer")) { 980 this.referenceSeqPointer = new Reference(); 981 return this.referenceSeqPointer; 982 } 983 else if (name.equals("referenceSeqString")) { 984 throw new FHIRException("Cannot call addChild on a singleton property Sequence.referenceSeqString"); 985 } 986 else if (name.equals("strand")) { 987 throw new FHIRException("Cannot call addChild on a singleton property Sequence.strand"); 988 } 989 else if (name.equals("windowStart")) { 990 throw new FHIRException("Cannot call addChild on a singleton property Sequence.windowStart"); 991 } 992 else if (name.equals("windowEnd")) { 993 throw new FHIRException("Cannot call addChild on a singleton property Sequence.windowEnd"); 994 } 995 else 996 return super.addChild(name); 997 } 998 999 public SequenceReferenceSeqComponent copy() { 1000 SequenceReferenceSeqComponent dst = new SequenceReferenceSeqComponent(); 1001 copyValues(dst); 1002 dst.chromosome = chromosome == null ? null : chromosome.copy(); 1003 dst.genomeBuild = genomeBuild == null ? null : genomeBuild.copy(); 1004 dst.referenceSeqId = referenceSeqId == null ? null : referenceSeqId.copy(); 1005 dst.referenceSeqPointer = referenceSeqPointer == null ? null : referenceSeqPointer.copy(); 1006 dst.referenceSeqString = referenceSeqString == null ? null : referenceSeqString.copy(); 1007 dst.strand = strand == null ? null : strand.copy(); 1008 dst.windowStart = windowStart == null ? null : windowStart.copy(); 1009 dst.windowEnd = windowEnd == null ? null : windowEnd.copy(); 1010 return dst; 1011 } 1012 1013 @Override 1014 public boolean equalsDeep(Base other_) { 1015 if (!super.equalsDeep(other_)) 1016 return false; 1017 if (!(other_ instanceof SequenceReferenceSeqComponent)) 1018 return false; 1019 SequenceReferenceSeqComponent o = (SequenceReferenceSeqComponent) other_; 1020 return compareDeep(chromosome, o.chromosome, true) && compareDeep(genomeBuild, o.genomeBuild, true) 1021 && compareDeep(referenceSeqId, o.referenceSeqId, true) && compareDeep(referenceSeqPointer, o.referenceSeqPointer, true) 1022 && compareDeep(referenceSeqString, o.referenceSeqString, true) && compareDeep(strand, o.strand, true) 1023 && compareDeep(windowStart, o.windowStart, true) && compareDeep(windowEnd, o.windowEnd, true); 1024 } 1025 1026 @Override 1027 public boolean equalsShallow(Base other_) { 1028 if (!super.equalsShallow(other_)) 1029 return false; 1030 if (!(other_ instanceof SequenceReferenceSeqComponent)) 1031 return false; 1032 SequenceReferenceSeqComponent o = (SequenceReferenceSeqComponent) other_; 1033 return compareValues(genomeBuild, o.genomeBuild, true) && compareValues(referenceSeqString, o.referenceSeqString, true) 1034 && compareValues(strand, o.strand, true) && compareValues(windowStart, o.windowStart, true) && compareValues(windowEnd, o.windowEnd, true) 1035 ; 1036 } 1037 1038 public boolean isEmpty() { 1039 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(chromosome, genomeBuild, referenceSeqId 1040 , referenceSeqPointer, referenceSeqString, strand, windowStart, windowEnd); 1041 } 1042 1043 public String fhirType() { 1044 return "Sequence.referenceSeq"; 1045 1046 } 1047 1048 } 1049 1050 @Block() 1051 public static class SequenceVariantComponent extends BackboneElement implements IBaseBackboneElement { 1052 /** 1053 * Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1054 */ 1055 @Child(name = "start", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1056 @Description(shortDefinition="Start position of the variant on the reference sequence", formalDefinition="Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 1057 protected IntegerType start; 1058 1059 /** 1060 * End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1061 */ 1062 @Child(name = "end", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1063 @Description(shortDefinition="End position of the variant on the reference sequence", formalDefinition="End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 1064 protected IntegerType end; 1065 1066 /** 1067 * An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1068 */ 1069 @Child(name = "observedAllele", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1070 @Description(shortDefinition="Allele that was observed", formalDefinition="An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1071 protected StringType observedAllele; 1072 1073 /** 1074 * An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1075 */ 1076 @Child(name = "referenceAllele", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1077 @Description(shortDefinition="Allele in the reference sequence", formalDefinition="An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end." ) 1078 protected StringType referenceAllele; 1079 1080 /** 1081 * Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1082 */ 1083 @Child(name = "cigar", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1084 @Description(shortDefinition="Extended CIGAR string for aligning the sequence with reference bases", formalDefinition="Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm)." ) 1085 protected StringType cigar; 1086 1087 /** 1088 * A pointer to an Observation containing variant information. 1089 */ 1090 @Child(name = "variantPointer", type = {Observation.class}, order=6, min=0, max=1, modifier=false, summary=true) 1091 @Description(shortDefinition="Pointer to observed variant information", formalDefinition="A pointer to an Observation containing variant information." ) 1092 protected Reference variantPointer; 1093 1094 /** 1095 * The actual object that is the target of the reference (A pointer to an Observation containing variant information.) 1096 */ 1097 protected Observation variantPointerTarget; 1098 1099 private static final long serialVersionUID = 105611837L; 1100 1101 /** 1102 * Constructor 1103 */ 1104 public SequenceVariantComponent() { 1105 super(); 1106 } 1107 1108 /** 1109 * @return {@link #start} (Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1110 */ 1111 public IntegerType getStartElement() { 1112 if (this.start == null) 1113 if (Configuration.errorOnAutoCreate()) 1114 throw new Error("Attempt to auto-create SequenceVariantComponent.start"); 1115 else if (Configuration.doAutoCreate()) 1116 this.start = new IntegerType(); // bb 1117 return this.start; 1118 } 1119 1120 public boolean hasStartElement() { 1121 return this.start != null && !this.start.isEmpty(); 1122 } 1123 1124 public boolean hasStart() { 1125 return this.start != null && !this.start.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #start} (Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1130 */ 1131 public SequenceVariantComponent setStartElement(IntegerType value) { 1132 this.start = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1138 */ 1139 public int getStart() { 1140 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1141 } 1142 1143 /** 1144 * @param value Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1145 */ 1146 public SequenceVariantComponent setStart(int value) { 1147 if (this.start == null) 1148 this.start = new IntegerType(); 1149 this.start.setValue(value); 1150 return this; 1151 } 1152 1153 /** 1154 * @return {@link #end} (End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1155 */ 1156 public IntegerType getEndElement() { 1157 if (this.end == null) 1158 if (Configuration.errorOnAutoCreate()) 1159 throw new Error("Attempt to auto-create SequenceVariantComponent.end"); 1160 else if (Configuration.doAutoCreate()) 1161 this.end = new IntegerType(); // bb 1162 return this.end; 1163 } 1164 1165 public boolean hasEndElement() { 1166 return this.end != null && !this.end.isEmpty(); 1167 } 1168 1169 public boolean hasEnd() { 1170 return this.end != null && !this.end.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #end} (End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1175 */ 1176 public SequenceVariantComponent setEndElement(IntegerType value) { 1177 this.end = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1183 */ 1184 public int getEnd() { 1185 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1186 } 1187 1188 /** 1189 * @param value End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1190 */ 1191 public SequenceVariantComponent setEnd(int value) { 1192 if (this.end == null) 1193 this.end = new IntegerType(); 1194 this.end.setValue(value); 1195 return this; 1196 } 1197 1198 /** 1199 * @return {@link #observedAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getObservedAllele" gives direct access to the value 1200 */ 1201 public StringType getObservedAlleleElement() { 1202 if (this.observedAllele == null) 1203 if (Configuration.errorOnAutoCreate()) 1204 throw new Error("Attempt to auto-create SequenceVariantComponent.observedAllele"); 1205 else if (Configuration.doAutoCreate()) 1206 this.observedAllele = new StringType(); // bb 1207 return this.observedAllele; 1208 } 1209 1210 public boolean hasObservedAlleleElement() { 1211 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1212 } 1213 1214 public boolean hasObservedAllele() { 1215 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1216 } 1217 1218 /** 1219 * @param value {@link #observedAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getObservedAllele" gives direct access to the value 1220 */ 1221 public SequenceVariantComponent setObservedAlleleElement(StringType value) { 1222 this.observedAllele = value; 1223 return this; 1224 } 1225 1226 /** 1227 * @return An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1228 */ 1229 public String getObservedAllele() { 1230 return this.observedAllele == null ? null : this.observedAllele.getValue(); 1231 } 1232 1233 /** 1234 * @param value An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1235 */ 1236 public SequenceVariantComponent setObservedAllele(String value) { 1237 if (Utilities.noString(value)) 1238 this.observedAllele = null; 1239 else { 1240 if (this.observedAllele == null) 1241 this.observedAllele = new StringType(); 1242 this.observedAllele.setValue(value); 1243 } 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #referenceAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReferenceAllele" gives direct access to the value 1249 */ 1250 public StringType getReferenceAlleleElement() { 1251 if (this.referenceAllele == null) 1252 if (Configuration.errorOnAutoCreate()) 1253 throw new Error("Attempt to auto-create SequenceVariantComponent.referenceAllele"); 1254 else if (Configuration.doAutoCreate()) 1255 this.referenceAllele = new StringType(); // bb 1256 return this.referenceAllele; 1257 } 1258 1259 public boolean hasReferenceAlleleElement() { 1260 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1261 } 1262 1263 public boolean hasReferenceAllele() { 1264 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1265 } 1266 1267 /** 1268 * @param value {@link #referenceAllele} (An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.). This is the underlying object with id, value and extensions. The accessor "getReferenceAllele" gives direct access to the value 1269 */ 1270 public SequenceVariantComponent setReferenceAlleleElement(StringType value) { 1271 this.referenceAllele = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1277 */ 1278 public String getReferenceAllele() { 1279 return this.referenceAllele == null ? null : this.referenceAllele.getValue(); 1280 } 1281 1282 /** 1283 * @param value An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end. 1284 */ 1285 public SequenceVariantComponent setReferenceAllele(String value) { 1286 if (Utilities.noString(value)) 1287 this.referenceAllele = null; 1288 else { 1289 if (this.referenceAllele == null) 1290 this.referenceAllele = new StringType(); 1291 this.referenceAllele.setValue(value); 1292 } 1293 return this; 1294 } 1295 1296 /** 1297 * @return {@link #cigar} (Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). This is the underlying object with id, value and extensions. The accessor "getCigar" gives direct access to the value 1298 */ 1299 public StringType getCigarElement() { 1300 if (this.cigar == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create SequenceVariantComponent.cigar"); 1303 else if (Configuration.doAutoCreate()) 1304 this.cigar = new StringType(); // bb 1305 return this.cigar; 1306 } 1307 1308 public boolean hasCigarElement() { 1309 return this.cigar != null && !this.cigar.isEmpty(); 1310 } 1311 1312 public boolean hasCigar() { 1313 return this.cigar != null && !this.cigar.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #cigar} (Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). This is the underlying object with id, value and extensions. The accessor "getCigar" gives direct access to the value 1318 */ 1319 public SequenceVariantComponent setCigarElement(StringType value) { 1320 this.cigar = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1326 */ 1327 public String getCigar() { 1328 return this.cigar == null ? null : this.cigar.getValue(); 1329 } 1330 1331 /** 1332 * @param value Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1333 */ 1334 public SequenceVariantComponent setCigar(String value) { 1335 if (Utilities.noString(value)) 1336 this.cigar = null; 1337 else { 1338 if (this.cigar == null) 1339 this.cigar = new StringType(); 1340 this.cigar.setValue(value); 1341 } 1342 return this; 1343 } 1344 1345 /** 1346 * @return {@link #variantPointer} (A pointer to an Observation containing variant information.) 1347 */ 1348 public Reference getVariantPointer() { 1349 if (this.variantPointer == null) 1350 if (Configuration.errorOnAutoCreate()) 1351 throw new Error("Attempt to auto-create SequenceVariantComponent.variantPointer"); 1352 else if (Configuration.doAutoCreate()) 1353 this.variantPointer = new Reference(); // cc 1354 return this.variantPointer; 1355 } 1356 1357 public boolean hasVariantPointer() { 1358 return this.variantPointer != null && !this.variantPointer.isEmpty(); 1359 } 1360 1361 /** 1362 * @param value {@link #variantPointer} (A pointer to an Observation containing variant information.) 1363 */ 1364 public SequenceVariantComponent setVariantPointer(Reference value) { 1365 this.variantPointer = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return {@link #variantPointer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A pointer to an Observation containing variant information.) 1371 */ 1372 public Observation getVariantPointerTarget() { 1373 if (this.variantPointerTarget == null) 1374 if (Configuration.errorOnAutoCreate()) 1375 throw new Error("Attempt to auto-create SequenceVariantComponent.variantPointer"); 1376 else if (Configuration.doAutoCreate()) 1377 this.variantPointerTarget = new Observation(); // aa 1378 return this.variantPointerTarget; 1379 } 1380 1381 /** 1382 * @param value {@link #variantPointer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A pointer to an Observation containing variant information.) 1383 */ 1384 public SequenceVariantComponent setVariantPointerTarget(Observation value) { 1385 this.variantPointerTarget = value; 1386 return this; 1387 } 1388 1389 protected void listChildren(List<Property> children) { 1390 super.listChildren(children); 1391 children.add(new Property("start", "integer", "Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start)); 1392 children.add(new Property("end", "integer", "End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end)); 1393 children.add(new Property("observedAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, observedAllele)); 1394 children.add(new Property("referenceAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, referenceAllele)); 1395 children.add(new Property("cigar", "string", "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 0, 1, cigar)); 1396 children.add(new Property("variantPointer", "Reference(Observation)", "A pointer to an Observation containing variant information.", 0, 1, variantPointer)); 1397 } 1398 1399 @Override 1400 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1401 switch (_hash) { 1402 case 109757538: /*start*/ return new Property("start", "integer", "Start position of the variant on the reference sequence.If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start); 1403 case 100571: /*end*/ return new Property("end", "integer", "End position of the variant on the reference sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end); 1404 case -1418745787: /*observedAllele*/ return new Property("observedAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, observedAllele); 1405 case 364045960: /*referenceAllele*/ return new Property("referenceAllele", "string", "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 0, 1, referenceAllele); 1406 case 94658738: /*cigar*/ return new Property("cigar", "string", "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 0, 1, cigar); 1407 case -1654319624: /*variantPointer*/ return new Property("variantPointer", "Reference(Observation)", "A pointer to an Observation containing variant information.", 0, 1, variantPointer); 1408 default: return super.getNamedProperty(_hash, _name, _checkValid); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1415 switch (hash) { 1416 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IntegerType 1417 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // IntegerType 1418 case -1418745787: /*observedAllele*/ return this.observedAllele == null ? new Base[0] : new Base[] {this.observedAllele}; // StringType 1419 case 364045960: /*referenceAllele*/ return this.referenceAllele == null ? new Base[0] : new Base[] {this.referenceAllele}; // StringType 1420 case 94658738: /*cigar*/ return this.cigar == null ? new Base[0] : new Base[] {this.cigar}; // StringType 1421 case -1654319624: /*variantPointer*/ return this.variantPointer == null ? new Base[0] : new Base[] {this.variantPointer}; // Reference 1422 default: return super.getProperty(hash, name, checkValid); 1423 } 1424 1425 } 1426 1427 @Override 1428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1429 switch (hash) { 1430 case 109757538: // start 1431 this.start = castToInteger(value); // IntegerType 1432 return value; 1433 case 100571: // end 1434 this.end = castToInteger(value); // IntegerType 1435 return value; 1436 case -1418745787: // observedAllele 1437 this.observedAllele = castToString(value); // StringType 1438 return value; 1439 case 364045960: // referenceAllele 1440 this.referenceAllele = castToString(value); // StringType 1441 return value; 1442 case 94658738: // cigar 1443 this.cigar = castToString(value); // StringType 1444 return value; 1445 case -1654319624: // variantPointer 1446 this.variantPointer = castToReference(value); // Reference 1447 return value; 1448 default: return super.setProperty(hash, name, value); 1449 } 1450 1451 } 1452 1453 @Override 1454 public Base setProperty(String name, Base value) throws FHIRException { 1455 if (name.equals("start")) { 1456 this.start = castToInteger(value); // IntegerType 1457 } else if (name.equals("end")) { 1458 this.end = castToInteger(value); // IntegerType 1459 } else if (name.equals("observedAllele")) { 1460 this.observedAllele = castToString(value); // StringType 1461 } else if (name.equals("referenceAllele")) { 1462 this.referenceAllele = castToString(value); // StringType 1463 } else if (name.equals("cigar")) { 1464 this.cigar = castToString(value); // StringType 1465 } else if (name.equals("variantPointer")) { 1466 this.variantPointer = castToReference(value); // Reference 1467 } else 1468 return super.setProperty(name, value); 1469 return value; 1470 } 1471 1472 @Override 1473 public Base makeProperty(int hash, String name) throws FHIRException { 1474 switch (hash) { 1475 case 109757538: return getStartElement(); 1476 case 100571: return getEndElement(); 1477 case -1418745787: return getObservedAlleleElement(); 1478 case 364045960: return getReferenceAlleleElement(); 1479 case 94658738: return getCigarElement(); 1480 case -1654319624: return getVariantPointer(); 1481 default: return super.makeProperty(hash, name); 1482 } 1483 1484 } 1485 1486 @Override 1487 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1488 switch (hash) { 1489 case 109757538: /*start*/ return new String[] {"integer"}; 1490 case 100571: /*end*/ return new String[] {"integer"}; 1491 case -1418745787: /*observedAllele*/ return new String[] {"string"}; 1492 case 364045960: /*referenceAllele*/ return new String[] {"string"}; 1493 case 94658738: /*cigar*/ return new String[] {"string"}; 1494 case -1654319624: /*variantPointer*/ return new String[] {"Reference"}; 1495 default: return super.getTypesForProperty(hash, name); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base addChild(String name) throws FHIRException { 1502 if (name.equals("start")) { 1503 throw new FHIRException("Cannot call addChild on a singleton property Sequence.start"); 1504 } 1505 else if (name.equals("end")) { 1506 throw new FHIRException("Cannot call addChild on a singleton property Sequence.end"); 1507 } 1508 else if (name.equals("observedAllele")) { 1509 throw new FHIRException("Cannot call addChild on a singleton property Sequence.observedAllele"); 1510 } 1511 else if (name.equals("referenceAllele")) { 1512 throw new FHIRException("Cannot call addChild on a singleton property Sequence.referenceAllele"); 1513 } 1514 else if (name.equals("cigar")) { 1515 throw new FHIRException("Cannot call addChild on a singleton property Sequence.cigar"); 1516 } 1517 else if (name.equals("variantPointer")) { 1518 this.variantPointer = new Reference(); 1519 return this.variantPointer; 1520 } 1521 else 1522 return super.addChild(name); 1523 } 1524 1525 public SequenceVariantComponent copy() { 1526 SequenceVariantComponent dst = new SequenceVariantComponent(); 1527 copyValues(dst); 1528 dst.start = start == null ? null : start.copy(); 1529 dst.end = end == null ? null : end.copy(); 1530 dst.observedAllele = observedAllele == null ? null : observedAllele.copy(); 1531 dst.referenceAllele = referenceAllele == null ? null : referenceAllele.copy(); 1532 dst.cigar = cigar == null ? null : cigar.copy(); 1533 dst.variantPointer = variantPointer == null ? null : variantPointer.copy(); 1534 return dst; 1535 } 1536 1537 @Override 1538 public boolean equalsDeep(Base other_) { 1539 if (!super.equalsDeep(other_)) 1540 return false; 1541 if (!(other_ instanceof SequenceVariantComponent)) 1542 return false; 1543 SequenceVariantComponent o = (SequenceVariantComponent) other_; 1544 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(observedAllele, o.observedAllele, true) 1545 && compareDeep(referenceAllele, o.referenceAllele, true) && compareDeep(cigar, o.cigar, true) && compareDeep(variantPointer, o.variantPointer, true) 1546 ; 1547 } 1548 1549 @Override 1550 public boolean equalsShallow(Base other_) { 1551 if (!super.equalsShallow(other_)) 1552 return false; 1553 if (!(other_ instanceof SequenceVariantComponent)) 1554 return false; 1555 SequenceVariantComponent o = (SequenceVariantComponent) other_; 1556 return compareValues(start, o.start, true) && compareValues(end, o.end, true) && compareValues(observedAllele, o.observedAllele, true) 1557 && compareValues(referenceAllele, o.referenceAllele, true) && compareValues(cigar, o.cigar, true); 1558 } 1559 1560 public boolean isEmpty() { 1561 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end, observedAllele 1562 , referenceAllele, cigar, variantPointer); 1563 } 1564 1565 public String fhirType() { 1566 return "Sequence.variant"; 1567 1568 } 1569 1570 } 1571 1572 @Block() 1573 public static class SequenceQualityComponent extends BackboneElement implements IBaseBackboneElement { 1574 /** 1575 * INDEL / SNP / Undefined variant. 1576 */ 1577 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1578 @Description(shortDefinition="indel | snp | unknown", formalDefinition="INDEL / SNP / Undefined variant." ) 1579 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/quality-type") 1580 protected Enumeration<QualityType> type; 1581 1582 /** 1583 * Gold standard sequence used for comparing against. 1584 */ 1585 @Child(name = "standardSequence", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1586 @Description(shortDefinition="Standard sequence for comparison", formalDefinition="Gold standard sequence used for comparing against." ) 1587 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-quality-standardSequence") 1588 protected CodeableConcept standardSequence; 1589 1590 /** 1591 * Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1592 */ 1593 @Child(name = "start", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1594 @Description(shortDefinition="Start position of the sequence", formalDefinition="Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive." ) 1595 protected IntegerType start; 1596 1597 /** 1598 * End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1599 */ 1600 @Child(name = "end", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1601 @Description(shortDefinition="End position of the sequence", formalDefinition="End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position." ) 1602 protected IntegerType end; 1603 1604 /** 1605 * The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)). 1606 */ 1607 @Child(name = "score", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=true) 1608 @Description(shortDefinition="Quality score for the comparison", formalDefinition="The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685))." ) 1609 protected Quantity score; 1610 1611 /** 1612 * Which method is used to get sequence quality. 1613 */ 1614 @Child(name = "method", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1615 @Description(shortDefinition="Method to get quality", formalDefinition="Which method is used to get sequence quality." ) 1616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-quality-method") 1617 protected CodeableConcept method; 1618 1619 /** 1620 * True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1621 */ 1622 @Child(name = "truthTP", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1623 @Description(shortDefinition="True positives from the perspective of the truth data", formalDefinition="True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event." ) 1624 protected DecimalType truthTP; 1625 1626 /** 1627 * True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1628 */ 1629 @Child(name = "queryTP", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1630 @Description(shortDefinition="True positives from the perspective of the query data", formalDefinition="True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event." ) 1631 protected DecimalType queryTP; 1632 1633 /** 1634 * False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 1635 */ 1636 @Child(name = "truthFN", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1637 @Description(shortDefinition="False negatives", formalDefinition="False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here." ) 1638 protected DecimalType truthFN; 1639 1640 /** 1641 * False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 1642 */ 1643 @Child(name = "queryFP", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1644 @Description(shortDefinition="False positives", formalDefinition="False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here." ) 1645 protected DecimalType queryFP; 1646 1647 /** 1648 * The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 1649 */ 1650 @Child(name = "gtFP", type = {DecimalType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1651 @Description(shortDefinition="False positives where the non-REF alleles in the Truth and Query Call Sets match", formalDefinition="The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar)." ) 1652 protected DecimalType gtFP; 1653 1654 /** 1655 * QUERY.TP / (QUERY.TP + QUERY.FP). 1656 */ 1657 @Child(name = "precision", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1658 @Description(shortDefinition="Precision of comparison", formalDefinition="QUERY.TP / (QUERY.TP + QUERY.FP)." ) 1659 protected DecimalType precision; 1660 1661 /** 1662 * TRUTH.TP / (TRUTH.TP + TRUTH.FN). 1663 */ 1664 @Child(name = "recall", type = {DecimalType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1665 @Description(shortDefinition="Recall of comparison", formalDefinition="TRUTH.TP / (TRUTH.TP + TRUTH.FN)." ) 1666 protected DecimalType recall; 1667 1668 /** 1669 * Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 1670 */ 1671 @Child(name = "fScore", type = {DecimalType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1672 @Description(shortDefinition="F-score", formalDefinition="Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall)." ) 1673 protected DecimalType fScore; 1674 1675 private static final long serialVersionUID = -383644463L; 1676 1677 /** 1678 * Constructor 1679 */ 1680 public SequenceQualityComponent() { 1681 super(); 1682 } 1683 1684 /** 1685 * Constructor 1686 */ 1687 public SequenceQualityComponent(Enumeration<QualityType> type) { 1688 super(); 1689 this.type = type; 1690 } 1691 1692 /** 1693 * @return {@link #type} (INDEL / SNP / Undefined variant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1694 */ 1695 public Enumeration<QualityType> getTypeElement() { 1696 if (this.type == null) 1697 if (Configuration.errorOnAutoCreate()) 1698 throw new Error("Attempt to auto-create SequenceQualityComponent.type"); 1699 else if (Configuration.doAutoCreate()) 1700 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); // bb 1701 return this.type; 1702 } 1703 1704 public boolean hasTypeElement() { 1705 return this.type != null && !this.type.isEmpty(); 1706 } 1707 1708 public boolean hasType() { 1709 return this.type != null && !this.type.isEmpty(); 1710 } 1711 1712 /** 1713 * @param value {@link #type} (INDEL / SNP / Undefined variant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1714 */ 1715 public SequenceQualityComponent setTypeElement(Enumeration<QualityType> value) { 1716 this.type = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return INDEL / SNP / Undefined variant. 1722 */ 1723 public QualityType getType() { 1724 return this.type == null ? null : this.type.getValue(); 1725 } 1726 1727 /** 1728 * @param value INDEL / SNP / Undefined variant. 1729 */ 1730 public SequenceQualityComponent setType(QualityType value) { 1731 if (this.type == null) 1732 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); 1733 this.type.setValue(value); 1734 return this; 1735 } 1736 1737 /** 1738 * @return {@link #standardSequence} (Gold standard sequence used for comparing against.) 1739 */ 1740 public CodeableConcept getStandardSequence() { 1741 if (this.standardSequence == null) 1742 if (Configuration.errorOnAutoCreate()) 1743 throw new Error("Attempt to auto-create SequenceQualityComponent.standardSequence"); 1744 else if (Configuration.doAutoCreate()) 1745 this.standardSequence = new CodeableConcept(); // cc 1746 return this.standardSequence; 1747 } 1748 1749 public boolean hasStandardSequence() { 1750 return this.standardSequence != null && !this.standardSequence.isEmpty(); 1751 } 1752 1753 /** 1754 * @param value {@link #standardSequence} (Gold standard sequence used for comparing against.) 1755 */ 1756 public SequenceQualityComponent setStandardSequence(CodeableConcept value) { 1757 this.standardSequence = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #start} (Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1763 */ 1764 public IntegerType getStartElement() { 1765 if (this.start == null) 1766 if (Configuration.errorOnAutoCreate()) 1767 throw new Error("Attempt to auto-create SequenceQualityComponent.start"); 1768 else if (Configuration.doAutoCreate()) 1769 this.start = new IntegerType(); // bb 1770 return this.start; 1771 } 1772 1773 public boolean hasStartElement() { 1774 return this.start != null && !this.start.isEmpty(); 1775 } 1776 1777 public boolean hasStart() { 1778 return this.start != null && !this.start.isEmpty(); 1779 } 1780 1781 /** 1782 * @param value {@link #start} (Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 1783 */ 1784 public SequenceQualityComponent setStartElement(IntegerType value) { 1785 this.start = value; 1786 return this; 1787 } 1788 1789 /** 1790 * @return Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1791 */ 1792 public int getStart() { 1793 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1794 } 1795 1796 /** 1797 * @param value Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive. 1798 */ 1799 public SequenceQualityComponent setStart(int value) { 1800 if (this.start == null) 1801 this.start = new IntegerType(); 1802 this.start.setValue(value); 1803 return this; 1804 } 1805 1806 /** 1807 * @return {@link #end} (End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1808 */ 1809 public IntegerType getEndElement() { 1810 if (this.end == null) 1811 if (Configuration.errorOnAutoCreate()) 1812 throw new Error("Attempt to auto-create SequenceQualityComponent.end"); 1813 else if (Configuration.doAutoCreate()) 1814 this.end = new IntegerType(); // bb 1815 return this.end; 1816 } 1817 1818 public boolean hasEndElement() { 1819 return this.end != null && !this.end.isEmpty(); 1820 } 1821 1822 public boolean hasEnd() { 1823 return this.end != null && !this.end.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #end} (End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1828 */ 1829 public SequenceQualityComponent setEndElement(IntegerType value) { 1830 this.end = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1836 */ 1837 public int getEnd() { 1838 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1839 } 1840 1841 /** 1842 * @param value End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position. 1843 */ 1844 public SequenceQualityComponent setEnd(int value) { 1845 if (this.end == null) 1846 this.end = new IntegerType(); 1847 this.end.setValue(value); 1848 return this; 1849 } 1850 1851 /** 1852 * @return {@link #score} (The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 1853 */ 1854 public Quantity getScore() { 1855 if (this.score == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create SequenceQualityComponent.score"); 1858 else if (Configuration.doAutoCreate()) 1859 this.score = new Quantity(); // cc 1860 return this.score; 1861 } 1862 1863 public boolean hasScore() { 1864 return this.score != null && !this.score.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #score} (The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 1869 */ 1870 public SequenceQualityComponent setScore(Quantity value) { 1871 this.score = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return {@link #method} (Which method is used to get sequence quality.) 1877 */ 1878 public CodeableConcept getMethod() { 1879 if (this.method == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create SequenceQualityComponent.method"); 1882 else if (Configuration.doAutoCreate()) 1883 this.method = new CodeableConcept(); // cc 1884 return this.method; 1885 } 1886 1887 public boolean hasMethod() { 1888 return this.method != null && !this.method.isEmpty(); 1889 } 1890 1891 /** 1892 * @param value {@link #method} (Which method is used to get sequence quality.) 1893 */ 1894 public SequenceQualityComponent setMethod(CodeableConcept value) { 1895 this.method = value; 1896 return this; 1897 } 1898 1899 /** 1900 * @return {@link #truthTP} (True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getTruthTP" gives direct access to the value 1901 */ 1902 public DecimalType getTruthTPElement() { 1903 if (this.truthTP == null) 1904 if (Configuration.errorOnAutoCreate()) 1905 throw new Error("Attempt to auto-create SequenceQualityComponent.truthTP"); 1906 else if (Configuration.doAutoCreate()) 1907 this.truthTP = new DecimalType(); // bb 1908 return this.truthTP; 1909 } 1910 1911 public boolean hasTruthTPElement() { 1912 return this.truthTP != null && !this.truthTP.isEmpty(); 1913 } 1914 1915 public boolean hasTruthTP() { 1916 return this.truthTP != null && !this.truthTP.isEmpty(); 1917 } 1918 1919 /** 1920 * @param value {@link #truthTP} (True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getTruthTP" gives direct access to the value 1921 */ 1922 public SequenceQualityComponent setTruthTPElement(DecimalType value) { 1923 this.truthTP = value; 1924 return this; 1925 } 1926 1927 /** 1928 * @return True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1929 */ 1930 public BigDecimal getTruthTP() { 1931 return this.truthTP == null ? null : this.truthTP.getValue(); 1932 } 1933 1934 /** 1935 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1936 */ 1937 public SequenceQualityComponent setTruthTP(BigDecimal value) { 1938 if (value == null) 1939 this.truthTP = null; 1940 else { 1941 if (this.truthTP == null) 1942 this.truthTP = new DecimalType(); 1943 this.truthTP.setValue(value); 1944 } 1945 return this; 1946 } 1947 1948 /** 1949 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1950 */ 1951 public SequenceQualityComponent setTruthTP(long value) { 1952 this.truthTP = new DecimalType(); 1953 this.truthTP.setValue(value); 1954 return this; 1955 } 1956 1957 /** 1958 * @param value True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1959 */ 1960 public SequenceQualityComponent setTruthTP(double value) { 1961 this.truthTP = new DecimalType(); 1962 this.truthTP.setValue(value); 1963 return this; 1964 } 1965 1966 /** 1967 * @return {@link #queryTP} (True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getQueryTP" gives direct access to the value 1968 */ 1969 public DecimalType getQueryTPElement() { 1970 if (this.queryTP == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create SequenceQualityComponent.queryTP"); 1973 else if (Configuration.doAutoCreate()) 1974 this.queryTP = new DecimalType(); // bb 1975 return this.queryTP; 1976 } 1977 1978 public boolean hasQueryTPElement() { 1979 return this.queryTP != null && !this.queryTP.isEmpty(); 1980 } 1981 1982 public boolean hasQueryTP() { 1983 return this.queryTP != null && !this.queryTP.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #queryTP} (True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.). This is the underlying object with id, value and extensions. The accessor "getQueryTP" gives direct access to the value 1988 */ 1989 public SequenceQualityComponent setQueryTPElement(DecimalType value) { 1990 this.queryTP = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 1996 */ 1997 public BigDecimal getQueryTP() { 1998 return this.queryTP == null ? null : this.queryTP.getValue(); 1999 } 2000 2001 /** 2002 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 2003 */ 2004 public SequenceQualityComponent setQueryTP(BigDecimal value) { 2005 if (value == null) 2006 this.queryTP = null; 2007 else { 2008 if (this.queryTP == null) 2009 this.queryTP = new DecimalType(); 2010 this.queryTP.setValue(value); 2011 } 2012 return this; 2013 } 2014 2015 /** 2016 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 2017 */ 2018 public SequenceQualityComponent setQueryTP(long value) { 2019 this.queryTP = new DecimalType(); 2020 this.queryTP.setValue(value); 2021 return this; 2022 } 2023 2024 /** 2025 * @param value True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event. 2026 */ 2027 public SequenceQualityComponent setQueryTP(double value) { 2028 this.queryTP = new DecimalType(); 2029 this.queryTP.setValue(value); 2030 return this; 2031 } 2032 2033 /** 2034 * @return {@link #truthFN} (False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getTruthFN" gives direct access to the value 2035 */ 2036 public DecimalType getTruthFNElement() { 2037 if (this.truthFN == null) 2038 if (Configuration.errorOnAutoCreate()) 2039 throw new Error("Attempt to auto-create SequenceQualityComponent.truthFN"); 2040 else if (Configuration.doAutoCreate()) 2041 this.truthFN = new DecimalType(); // bb 2042 return this.truthFN; 2043 } 2044 2045 public boolean hasTruthFNElement() { 2046 return this.truthFN != null && !this.truthFN.isEmpty(); 2047 } 2048 2049 public boolean hasTruthFN() { 2050 return this.truthFN != null && !this.truthFN.isEmpty(); 2051 } 2052 2053 /** 2054 * @param value {@link #truthFN} (False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getTruthFN" gives direct access to the value 2055 */ 2056 public SequenceQualityComponent setTruthFNElement(DecimalType value) { 2057 this.truthFN = value; 2058 return this; 2059 } 2060 2061 /** 2062 * @return False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2063 */ 2064 public BigDecimal getTruthFN() { 2065 return this.truthFN == null ? null : this.truthFN.getValue(); 2066 } 2067 2068 /** 2069 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2070 */ 2071 public SequenceQualityComponent setTruthFN(BigDecimal value) { 2072 if (value == null) 2073 this.truthFN = null; 2074 else { 2075 if (this.truthFN == null) 2076 this.truthFN = new DecimalType(); 2077 this.truthFN.setValue(value); 2078 } 2079 return this; 2080 } 2081 2082 /** 2083 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2084 */ 2085 public SequenceQualityComponent setTruthFN(long value) { 2086 this.truthFN = new DecimalType(); 2087 this.truthFN.setValue(value); 2088 return this; 2089 } 2090 2091 /** 2092 * @param value False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here. 2093 */ 2094 public SequenceQualityComponent setTruthFN(double value) { 2095 this.truthFN = new DecimalType(); 2096 this.truthFN.setValue(value); 2097 return this; 2098 } 2099 2100 /** 2101 * @return {@link #queryFP} (False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getQueryFP" gives direct access to the value 2102 */ 2103 public DecimalType getQueryFPElement() { 2104 if (this.queryFP == null) 2105 if (Configuration.errorOnAutoCreate()) 2106 throw new Error("Attempt to auto-create SequenceQualityComponent.queryFP"); 2107 else if (Configuration.doAutoCreate()) 2108 this.queryFP = new DecimalType(); // bb 2109 return this.queryFP; 2110 } 2111 2112 public boolean hasQueryFPElement() { 2113 return this.queryFP != null && !this.queryFP.isEmpty(); 2114 } 2115 2116 public boolean hasQueryFP() { 2117 return this.queryFP != null && !this.queryFP.isEmpty(); 2118 } 2119 2120 /** 2121 * @param value {@link #queryFP} (False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.). This is the underlying object with id, value and extensions. The accessor "getQueryFP" gives direct access to the value 2122 */ 2123 public SequenceQualityComponent setQueryFPElement(DecimalType value) { 2124 this.queryFP = value; 2125 return this; 2126 } 2127 2128 /** 2129 * @return False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2130 */ 2131 public BigDecimal getQueryFP() { 2132 return this.queryFP == null ? null : this.queryFP.getValue(); 2133 } 2134 2135 /** 2136 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2137 */ 2138 public SequenceQualityComponent setQueryFP(BigDecimal value) { 2139 if (value == null) 2140 this.queryFP = null; 2141 else { 2142 if (this.queryFP == null) 2143 this.queryFP = new DecimalType(); 2144 this.queryFP.setValue(value); 2145 } 2146 return this; 2147 } 2148 2149 /** 2150 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2151 */ 2152 public SequenceQualityComponent setQueryFP(long value) { 2153 this.queryFP = new DecimalType(); 2154 this.queryFP.setValue(value); 2155 return this; 2156 } 2157 2158 /** 2159 * @param value False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here. 2160 */ 2161 public SequenceQualityComponent setQueryFP(double value) { 2162 this.queryFP = new DecimalType(); 2163 this.queryFP.setValue(value); 2164 return this; 2165 } 2166 2167 /** 2168 * @return {@link #gtFP} (The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).). This is the underlying object with id, value and extensions. The accessor "getGtFP" gives direct access to the value 2169 */ 2170 public DecimalType getGtFPElement() { 2171 if (this.gtFP == null) 2172 if (Configuration.errorOnAutoCreate()) 2173 throw new Error("Attempt to auto-create SequenceQualityComponent.gtFP"); 2174 else if (Configuration.doAutoCreate()) 2175 this.gtFP = new DecimalType(); // bb 2176 return this.gtFP; 2177 } 2178 2179 public boolean hasGtFPElement() { 2180 return this.gtFP != null && !this.gtFP.isEmpty(); 2181 } 2182 2183 public boolean hasGtFP() { 2184 return this.gtFP != null && !this.gtFP.isEmpty(); 2185 } 2186 2187 /** 2188 * @param value {@link #gtFP} (The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).). This is the underlying object with id, value and extensions. The accessor "getGtFP" gives direct access to the value 2189 */ 2190 public SequenceQualityComponent setGtFPElement(DecimalType value) { 2191 this.gtFP = value; 2192 return this; 2193 } 2194 2195 /** 2196 * @return The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2197 */ 2198 public BigDecimal getGtFP() { 2199 return this.gtFP == null ? null : this.gtFP.getValue(); 2200 } 2201 2202 /** 2203 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2204 */ 2205 public SequenceQualityComponent setGtFP(BigDecimal value) { 2206 if (value == null) 2207 this.gtFP = null; 2208 else { 2209 if (this.gtFP == null) 2210 this.gtFP = new DecimalType(); 2211 this.gtFP.setValue(value); 2212 } 2213 return this; 2214 } 2215 2216 /** 2217 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2218 */ 2219 public SequenceQualityComponent setGtFP(long value) { 2220 this.gtFP = new DecimalType(); 2221 this.gtFP.setValue(value); 2222 return this; 2223 } 2224 2225 /** 2226 * @param value The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar). 2227 */ 2228 public SequenceQualityComponent setGtFP(double value) { 2229 this.gtFP = new DecimalType(); 2230 this.gtFP.setValue(value); 2231 return this; 2232 } 2233 2234 /** 2235 * @return {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the underlying object with id, value and extensions. The accessor "getPrecision" gives direct access to the value 2236 */ 2237 public DecimalType getPrecisionElement() { 2238 if (this.precision == null) 2239 if (Configuration.errorOnAutoCreate()) 2240 throw new Error("Attempt to auto-create SequenceQualityComponent.precision"); 2241 else if (Configuration.doAutoCreate()) 2242 this.precision = new DecimalType(); // bb 2243 return this.precision; 2244 } 2245 2246 public boolean hasPrecisionElement() { 2247 return this.precision != null && !this.precision.isEmpty(); 2248 } 2249 2250 public boolean hasPrecision() { 2251 return this.precision != null && !this.precision.isEmpty(); 2252 } 2253 2254 /** 2255 * @param value {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the underlying object with id, value and extensions. The accessor "getPrecision" gives direct access to the value 2256 */ 2257 public SequenceQualityComponent setPrecisionElement(DecimalType value) { 2258 this.precision = value; 2259 return this; 2260 } 2261 2262 /** 2263 * @return QUERY.TP / (QUERY.TP + QUERY.FP). 2264 */ 2265 public BigDecimal getPrecision() { 2266 return this.precision == null ? null : this.precision.getValue(); 2267 } 2268 2269 /** 2270 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2271 */ 2272 public SequenceQualityComponent setPrecision(BigDecimal value) { 2273 if (value == null) 2274 this.precision = null; 2275 else { 2276 if (this.precision == null) 2277 this.precision = new DecimalType(); 2278 this.precision.setValue(value); 2279 } 2280 return this; 2281 } 2282 2283 /** 2284 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2285 */ 2286 public SequenceQualityComponent setPrecision(long value) { 2287 this.precision = new DecimalType(); 2288 this.precision.setValue(value); 2289 return this; 2290 } 2291 2292 /** 2293 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 2294 */ 2295 public SequenceQualityComponent setPrecision(double value) { 2296 this.precision = new DecimalType(); 2297 this.precision.setValue(value); 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 2303 */ 2304 public DecimalType getRecallElement() { 2305 if (this.recall == null) 2306 if (Configuration.errorOnAutoCreate()) 2307 throw new Error("Attempt to auto-create SequenceQualityComponent.recall"); 2308 else if (Configuration.doAutoCreate()) 2309 this.recall = new DecimalType(); // bb 2310 return this.recall; 2311 } 2312 2313 public boolean hasRecallElement() { 2314 return this.recall != null && !this.recall.isEmpty(); 2315 } 2316 2317 public boolean hasRecall() { 2318 return this.recall != null && !this.recall.isEmpty(); 2319 } 2320 2321 /** 2322 * @param value {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 2323 */ 2324 public SequenceQualityComponent setRecallElement(DecimalType value) { 2325 this.recall = value; 2326 return this; 2327 } 2328 2329 /** 2330 * @return TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2331 */ 2332 public BigDecimal getRecall() { 2333 return this.recall == null ? null : this.recall.getValue(); 2334 } 2335 2336 /** 2337 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2338 */ 2339 public SequenceQualityComponent setRecall(BigDecimal value) { 2340 if (value == null) 2341 this.recall = null; 2342 else { 2343 if (this.recall == null) 2344 this.recall = new DecimalType(); 2345 this.recall.setValue(value); 2346 } 2347 return this; 2348 } 2349 2350 /** 2351 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2352 */ 2353 public SequenceQualityComponent setRecall(long value) { 2354 this.recall = new DecimalType(); 2355 this.recall.setValue(value); 2356 return this; 2357 } 2358 2359 /** 2360 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2361 */ 2362 public SequenceQualityComponent setRecall(double value) { 2363 this.recall = new DecimalType(); 2364 this.recall.setValue(value); 2365 return this; 2366 } 2367 2368 /** 2369 * @return {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).). This is the underlying object with id, value and extensions. The accessor "getFScore" gives direct access to the value 2370 */ 2371 public DecimalType getFScoreElement() { 2372 if (this.fScore == null) 2373 if (Configuration.errorOnAutoCreate()) 2374 throw new Error("Attempt to auto-create SequenceQualityComponent.fScore"); 2375 else if (Configuration.doAutoCreate()) 2376 this.fScore = new DecimalType(); // bb 2377 return this.fScore; 2378 } 2379 2380 public boolean hasFScoreElement() { 2381 return this.fScore != null && !this.fScore.isEmpty(); 2382 } 2383 2384 public boolean hasFScore() { 2385 return this.fScore != null && !this.fScore.isEmpty(); 2386 } 2387 2388 /** 2389 * @param value {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).). This is the underlying object with id, value and extensions. The accessor "getFScore" gives direct access to the value 2390 */ 2391 public SequenceQualityComponent setFScoreElement(DecimalType value) { 2392 this.fScore = value; 2393 return this; 2394 } 2395 2396 /** 2397 * @return Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2398 */ 2399 public BigDecimal getFScore() { 2400 return this.fScore == null ? null : this.fScore.getValue(); 2401 } 2402 2403 /** 2404 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2405 */ 2406 public SequenceQualityComponent setFScore(BigDecimal value) { 2407 if (value == null) 2408 this.fScore = null; 2409 else { 2410 if (this.fScore == null) 2411 this.fScore = new DecimalType(); 2412 this.fScore.setValue(value); 2413 } 2414 return this; 2415 } 2416 2417 /** 2418 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2419 */ 2420 public SequenceQualityComponent setFScore(long value) { 2421 this.fScore = new DecimalType(); 2422 this.fScore.setValue(value); 2423 return this; 2424 } 2425 2426 /** 2427 * @param value Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall). 2428 */ 2429 public SequenceQualityComponent setFScore(double value) { 2430 this.fScore = new DecimalType(); 2431 this.fScore.setValue(value); 2432 return this; 2433 } 2434 2435 protected void listChildren(List<Property> children) { 2436 super.listChildren(children); 2437 children.add(new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type)); 2438 children.add(new Property("standardSequence", "CodeableConcept", "Gold standard sequence used for comparing against.", 0, 1, standardSequence)); 2439 children.add(new Property("start", "integer", "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start)); 2440 children.add(new Property("end", "integer", "End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end)); 2441 children.add(new Property("score", "Quantity", "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 0, 1, score)); 2442 children.add(new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method)); 2443 children.add(new Property("truthTP", "decimal", "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, truthTP)); 2444 children.add(new Property("queryTP", "decimal", "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, queryTP)); 2445 children.add(new Property("truthFN", "decimal", "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 0, 1, truthFN)); 2446 children.add(new Property("queryFP", "decimal", "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 0, 1, queryFP)); 2447 children.add(new Property("gtFP", "decimal", "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 0, 1, gtFP)); 2448 children.add(new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision)); 2449 children.add(new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall)); 2450 children.add(new Property("fScore", "decimal", "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, fScore)); 2451 } 2452 2453 @Override 2454 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2455 switch (_hash) { 2456 case 3575610: /*type*/ return new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type); 2457 case -1861227106: /*standardSequence*/ return new Property("standardSequence", "CodeableConcept", "Gold standard sequence used for comparing against.", 0, 1, standardSequence); 2458 case 109757538: /*start*/ return new Property("start", "integer", "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 0, 1, start); 2459 case 100571: /*end*/ return new Property("end", "integer", "End position of the sequence.If the coordinate system is 0-based then end is is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 0, 1, end); 2460 case 109264530: /*score*/ return new Property("score", "Quantity", "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 0, 1, score); 2461 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method); 2462 case -1048421849: /*truthTP*/ return new Property("truthTP", "decimal", "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, truthTP); 2463 case 655102276: /*queryTP*/ return new Property("queryTP", "decimal", "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 0, 1, queryTP); 2464 case -1048422285: /*truthFN*/ return new Property("truthFN", "decimal", "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 0, 1, truthFN); 2465 case 655101842: /*queryFP*/ return new Property("queryFP", "decimal", "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 0, 1, queryFP); 2466 case 3182199: /*gtFP*/ return new Property("gtFP", "decimal", "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 0, 1, gtFP); 2467 case -1376177026: /*precision*/ return new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision); 2468 case -934922479: /*recall*/ return new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall); 2469 case -1295082036: /*fScore*/ return new Property("fScore", "decimal", "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, fScore); 2470 default: return super.getNamedProperty(_hash, _name, _checkValid); 2471 } 2472 2473 } 2474 2475 @Override 2476 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2477 switch (hash) { 2478 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<QualityType> 2479 case -1861227106: /*standardSequence*/ return this.standardSequence == null ? new Base[0] : new Base[] {this.standardSequence}; // CodeableConcept 2480 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IntegerType 2481 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // IntegerType 2482 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // Quantity 2483 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 2484 case -1048421849: /*truthTP*/ return this.truthTP == null ? new Base[0] : new Base[] {this.truthTP}; // DecimalType 2485 case 655102276: /*queryTP*/ return this.queryTP == null ? new Base[0] : new Base[] {this.queryTP}; // DecimalType 2486 case -1048422285: /*truthFN*/ return this.truthFN == null ? new Base[0] : new Base[] {this.truthFN}; // DecimalType 2487 case 655101842: /*queryFP*/ return this.queryFP == null ? new Base[0] : new Base[] {this.queryFP}; // DecimalType 2488 case 3182199: /*gtFP*/ return this.gtFP == null ? new Base[0] : new Base[] {this.gtFP}; // DecimalType 2489 case -1376177026: /*precision*/ return this.precision == null ? new Base[0] : new Base[] {this.precision}; // DecimalType 2490 case -934922479: /*recall*/ return this.recall == null ? new Base[0] : new Base[] {this.recall}; // DecimalType 2491 case -1295082036: /*fScore*/ return this.fScore == null ? new Base[0] : new Base[] {this.fScore}; // DecimalType 2492 default: return super.getProperty(hash, name, checkValid); 2493 } 2494 2495 } 2496 2497 @Override 2498 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2499 switch (hash) { 2500 case 3575610: // type 2501 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 2502 this.type = (Enumeration) value; // Enumeration<QualityType> 2503 return value; 2504 case -1861227106: // standardSequence 2505 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 2506 return value; 2507 case 109757538: // start 2508 this.start = castToInteger(value); // IntegerType 2509 return value; 2510 case 100571: // end 2511 this.end = castToInteger(value); // IntegerType 2512 return value; 2513 case 109264530: // score 2514 this.score = castToQuantity(value); // Quantity 2515 return value; 2516 case -1077554975: // method 2517 this.method = castToCodeableConcept(value); // CodeableConcept 2518 return value; 2519 case -1048421849: // truthTP 2520 this.truthTP = castToDecimal(value); // DecimalType 2521 return value; 2522 case 655102276: // queryTP 2523 this.queryTP = castToDecimal(value); // DecimalType 2524 return value; 2525 case -1048422285: // truthFN 2526 this.truthFN = castToDecimal(value); // DecimalType 2527 return value; 2528 case 655101842: // queryFP 2529 this.queryFP = castToDecimal(value); // DecimalType 2530 return value; 2531 case 3182199: // gtFP 2532 this.gtFP = castToDecimal(value); // DecimalType 2533 return value; 2534 case -1376177026: // precision 2535 this.precision = castToDecimal(value); // DecimalType 2536 return value; 2537 case -934922479: // recall 2538 this.recall = castToDecimal(value); // DecimalType 2539 return value; 2540 case -1295082036: // fScore 2541 this.fScore = castToDecimal(value); // DecimalType 2542 return value; 2543 default: return super.setProperty(hash, name, value); 2544 } 2545 2546 } 2547 2548 @Override 2549 public Base setProperty(String name, Base value) throws FHIRException { 2550 if (name.equals("type")) { 2551 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 2552 this.type = (Enumeration) value; // Enumeration<QualityType> 2553 } else if (name.equals("standardSequence")) { 2554 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 2555 } else if (name.equals("start")) { 2556 this.start = castToInteger(value); // IntegerType 2557 } else if (name.equals("end")) { 2558 this.end = castToInteger(value); // IntegerType 2559 } else if (name.equals("score")) { 2560 this.score = castToQuantity(value); // Quantity 2561 } else if (name.equals("method")) { 2562 this.method = castToCodeableConcept(value); // CodeableConcept 2563 } else if (name.equals("truthTP")) { 2564 this.truthTP = castToDecimal(value); // DecimalType 2565 } else if (name.equals("queryTP")) { 2566 this.queryTP = castToDecimal(value); // DecimalType 2567 } else if (name.equals("truthFN")) { 2568 this.truthFN = castToDecimal(value); // DecimalType 2569 } else if (name.equals("queryFP")) { 2570 this.queryFP = castToDecimal(value); // DecimalType 2571 } else if (name.equals("gtFP")) { 2572 this.gtFP = castToDecimal(value); // DecimalType 2573 } else if (name.equals("precision")) { 2574 this.precision = castToDecimal(value); // DecimalType 2575 } else if (name.equals("recall")) { 2576 this.recall = castToDecimal(value); // DecimalType 2577 } else if (name.equals("fScore")) { 2578 this.fScore = castToDecimal(value); // DecimalType 2579 } else 2580 return super.setProperty(name, value); 2581 return value; 2582 } 2583 2584 @Override 2585 public Base makeProperty(int hash, String name) throws FHIRException { 2586 switch (hash) { 2587 case 3575610: return getTypeElement(); 2588 case -1861227106: return getStandardSequence(); 2589 case 109757538: return getStartElement(); 2590 case 100571: return getEndElement(); 2591 case 109264530: return getScore(); 2592 case -1077554975: return getMethod(); 2593 case -1048421849: return getTruthTPElement(); 2594 case 655102276: return getQueryTPElement(); 2595 case -1048422285: return getTruthFNElement(); 2596 case 655101842: return getQueryFPElement(); 2597 case 3182199: return getGtFPElement(); 2598 case -1376177026: return getPrecisionElement(); 2599 case -934922479: return getRecallElement(); 2600 case -1295082036: return getFScoreElement(); 2601 default: return super.makeProperty(hash, name); 2602 } 2603 2604 } 2605 2606 @Override 2607 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2608 switch (hash) { 2609 case 3575610: /*type*/ return new String[] {"code"}; 2610 case -1861227106: /*standardSequence*/ return new String[] {"CodeableConcept"}; 2611 case 109757538: /*start*/ return new String[] {"integer"}; 2612 case 100571: /*end*/ return new String[] {"integer"}; 2613 case 109264530: /*score*/ return new String[] {"Quantity"}; 2614 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 2615 case -1048421849: /*truthTP*/ return new String[] {"decimal"}; 2616 case 655102276: /*queryTP*/ return new String[] {"decimal"}; 2617 case -1048422285: /*truthFN*/ return new String[] {"decimal"}; 2618 case 655101842: /*queryFP*/ return new String[] {"decimal"}; 2619 case 3182199: /*gtFP*/ return new String[] {"decimal"}; 2620 case -1376177026: /*precision*/ return new String[] {"decimal"}; 2621 case -934922479: /*recall*/ return new String[] {"decimal"}; 2622 case -1295082036: /*fScore*/ return new String[] {"decimal"}; 2623 default: return super.getTypesForProperty(hash, name); 2624 } 2625 2626 } 2627 2628 @Override 2629 public Base addChild(String name) throws FHIRException { 2630 if (name.equals("type")) { 2631 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 2632 } 2633 else if (name.equals("standardSequence")) { 2634 this.standardSequence = new CodeableConcept(); 2635 return this.standardSequence; 2636 } 2637 else if (name.equals("start")) { 2638 throw new FHIRException("Cannot call addChild on a singleton property Sequence.start"); 2639 } 2640 else if (name.equals("end")) { 2641 throw new FHIRException("Cannot call addChild on a singleton property Sequence.end"); 2642 } 2643 else if (name.equals("score")) { 2644 this.score = new Quantity(); 2645 return this.score; 2646 } 2647 else if (name.equals("method")) { 2648 this.method = new CodeableConcept(); 2649 return this.method; 2650 } 2651 else if (name.equals("truthTP")) { 2652 throw new FHIRException("Cannot call addChild on a singleton property Sequence.truthTP"); 2653 } 2654 else if (name.equals("queryTP")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property Sequence.queryTP"); 2656 } 2657 else if (name.equals("truthFN")) { 2658 throw new FHIRException("Cannot call addChild on a singleton property Sequence.truthFN"); 2659 } 2660 else if (name.equals("queryFP")) { 2661 throw new FHIRException("Cannot call addChild on a singleton property Sequence.queryFP"); 2662 } 2663 else if (name.equals("gtFP")) { 2664 throw new FHIRException("Cannot call addChild on a singleton property Sequence.gtFP"); 2665 } 2666 else if (name.equals("precision")) { 2667 throw new FHIRException("Cannot call addChild on a singleton property Sequence.precision"); 2668 } 2669 else if (name.equals("recall")) { 2670 throw new FHIRException("Cannot call addChild on a singleton property Sequence.recall"); 2671 } 2672 else if (name.equals("fScore")) { 2673 throw new FHIRException("Cannot call addChild on a singleton property Sequence.fScore"); 2674 } 2675 else 2676 return super.addChild(name); 2677 } 2678 2679 public SequenceQualityComponent copy() { 2680 SequenceQualityComponent dst = new SequenceQualityComponent(); 2681 copyValues(dst); 2682 dst.type = type == null ? null : type.copy(); 2683 dst.standardSequence = standardSequence == null ? null : standardSequence.copy(); 2684 dst.start = start == null ? null : start.copy(); 2685 dst.end = end == null ? null : end.copy(); 2686 dst.score = score == null ? null : score.copy(); 2687 dst.method = method == null ? null : method.copy(); 2688 dst.truthTP = truthTP == null ? null : truthTP.copy(); 2689 dst.queryTP = queryTP == null ? null : queryTP.copy(); 2690 dst.truthFN = truthFN == null ? null : truthFN.copy(); 2691 dst.queryFP = queryFP == null ? null : queryFP.copy(); 2692 dst.gtFP = gtFP == null ? null : gtFP.copy(); 2693 dst.precision = precision == null ? null : precision.copy(); 2694 dst.recall = recall == null ? null : recall.copy(); 2695 dst.fScore = fScore == null ? null : fScore.copy(); 2696 return dst; 2697 } 2698 2699 @Override 2700 public boolean equalsDeep(Base other_) { 2701 if (!super.equalsDeep(other_)) 2702 return false; 2703 if (!(other_ instanceof SequenceQualityComponent)) 2704 return false; 2705 SequenceQualityComponent o = (SequenceQualityComponent) other_; 2706 return compareDeep(type, o.type, true) && compareDeep(standardSequence, o.standardSequence, true) 2707 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(score, o.score, true) 2708 && compareDeep(method, o.method, true) && compareDeep(truthTP, o.truthTP, true) && compareDeep(queryTP, o.queryTP, true) 2709 && compareDeep(truthFN, o.truthFN, true) && compareDeep(queryFP, o.queryFP, true) && compareDeep(gtFP, o.gtFP, true) 2710 && compareDeep(precision, o.precision, true) && compareDeep(recall, o.recall, true) && compareDeep(fScore, o.fScore, true) 2711 ; 2712 } 2713 2714 @Override 2715 public boolean equalsShallow(Base other_) { 2716 if (!super.equalsShallow(other_)) 2717 return false; 2718 if (!(other_ instanceof SequenceQualityComponent)) 2719 return false; 2720 SequenceQualityComponent o = (SequenceQualityComponent) other_; 2721 return compareValues(type, o.type, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 2722 && compareValues(truthTP, o.truthTP, true) && compareValues(queryTP, o.queryTP, true) && compareValues(truthFN, o.truthFN, true) 2723 && compareValues(queryFP, o.queryFP, true) && compareValues(gtFP, o.gtFP, true) && compareValues(precision, o.precision, true) 2724 && compareValues(recall, o.recall, true) && compareValues(fScore, o.fScore, true); 2725 } 2726 2727 public boolean isEmpty() { 2728 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, standardSequence, start 2729 , end, score, method, truthTP, queryTP, truthFN, queryFP, gtFP, precision 2730 , recall, fScore); 2731 } 2732 2733 public String fhirType() { 2734 return "Sequence.quality"; 2735 2736 } 2737 2738 } 2739 2740 @Block() 2741 public static class SequenceRepositoryComponent extends BackboneElement implements IBaseBackboneElement { 2742 /** 2743 * Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2744 */ 2745 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2746 @Description(shortDefinition="directlink | openapi | login | oauth | other", formalDefinition="Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource." ) 2747 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/repository-type") 2748 protected Enumeration<RepositoryType> type; 2749 2750 /** 2751 * URI of an external repository which contains further details about the genetics data. 2752 */ 2753 @Child(name = "url", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2754 @Description(shortDefinition="URI of the repository", formalDefinition="URI of an external repository which contains further details about the genetics data." ) 2755 protected UriType url; 2756 2757 /** 2758 * URI of an external repository which contains further details about the genetics data. 2759 */ 2760 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2761 @Description(shortDefinition="Repository's name", formalDefinition="URI of an external repository which contains further details about the genetics data." ) 2762 protected StringType name; 2763 2764 /** 2765 * Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2766 */ 2767 @Child(name = "datasetId", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2768 @Description(shortDefinition="Id of the dataset that used to call for dataset in repository", formalDefinition="Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository." ) 2769 protected StringType datasetId; 2770 2771 /** 2772 * Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 2773 */ 2774 @Child(name = "variantsetId", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2775 @Description(shortDefinition="Id of the variantset that used to call for variantset in repository", formalDefinition="Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository." ) 2776 protected StringType variantsetId; 2777 2778 /** 2779 * Id of the read in this external repository. 2780 */ 2781 @Child(name = "readsetId", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2782 @Description(shortDefinition="Id of the read", formalDefinition="Id of the read in this external repository." ) 2783 protected StringType readsetId; 2784 2785 private static final long serialVersionUID = -899243265L; 2786 2787 /** 2788 * Constructor 2789 */ 2790 public SequenceRepositoryComponent() { 2791 super(); 2792 } 2793 2794 /** 2795 * Constructor 2796 */ 2797 public SequenceRepositoryComponent(Enumeration<RepositoryType> type) { 2798 super(); 2799 this.type = type; 2800 } 2801 2802 /** 2803 * @return {@link #type} (Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2804 */ 2805 public Enumeration<RepositoryType> getTypeElement() { 2806 if (this.type == null) 2807 if (Configuration.errorOnAutoCreate()) 2808 throw new Error("Attempt to auto-create SequenceRepositoryComponent.type"); 2809 else if (Configuration.doAutoCreate()) 2810 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); // bb 2811 return this.type; 2812 } 2813 2814 public boolean hasTypeElement() { 2815 return this.type != null && !this.type.isEmpty(); 2816 } 2817 2818 public boolean hasType() { 2819 return this.type != null && !this.type.isEmpty(); 2820 } 2821 2822 /** 2823 * @param value {@link #type} (Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2824 */ 2825 public SequenceRepositoryComponent setTypeElement(Enumeration<RepositoryType> value) { 2826 this.type = value; 2827 return this; 2828 } 2829 2830 /** 2831 * @return Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2832 */ 2833 public RepositoryType getType() { 2834 return this.type == null ? null : this.type.getValue(); 2835 } 2836 2837 /** 2838 * @param value Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource. 2839 */ 2840 public SequenceRepositoryComponent setType(RepositoryType value) { 2841 if (this.type == null) 2842 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); 2843 this.type.setValue(value); 2844 return this; 2845 } 2846 2847 /** 2848 * @return {@link #url} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2849 */ 2850 public UriType getUrlElement() { 2851 if (this.url == null) 2852 if (Configuration.errorOnAutoCreate()) 2853 throw new Error("Attempt to auto-create SequenceRepositoryComponent.url"); 2854 else if (Configuration.doAutoCreate()) 2855 this.url = new UriType(); // bb 2856 return this.url; 2857 } 2858 2859 public boolean hasUrlElement() { 2860 return this.url != null && !this.url.isEmpty(); 2861 } 2862 2863 public boolean hasUrl() { 2864 return this.url != null && !this.url.isEmpty(); 2865 } 2866 2867 /** 2868 * @param value {@link #url} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2869 */ 2870 public SequenceRepositoryComponent setUrlElement(UriType value) { 2871 this.url = value; 2872 return this; 2873 } 2874 2875 /** 2876 * @return URI of an external repository which contains further details about the genetics data. 2877 */ 2878 public String getUrl() { 2879 return this.url == null ? null : this.url.getValue(); 2880 } 2881 2882 /** 2883 * @param value URI of an external repository which contains further details about the genetics data. 2884 */ 2885 public SequenceRepositoryComponent setUrl(String value) { 2886 if (Utilities.noString(value)) 2887 this.url = null; 2888 else { 2889 if (this.url == null) 2890 this.url = new UriType(); 2891 this.url.setValue(value); 2892 } 2893 return this; 2894 } 2895 2896 /** 2897 * @return {@link #name} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2898 */ 2899 public StringType getNameElement() { 2900 if (this.name == null) 2901 if (Configuration.errorOnAutoCreate()) 2902 throw new Error("Attempt to auto-create SequenceRepositoryComponent.name"); 2903 else if (Configuration.doAutoCreate()) 2904 this.name = new StringType(); // bb 2905 return this.name; 2906 } 2907 2908 public boolean hasNameElement() { 2909 return this.name != null && !this.name.isEmpty(); 2910 } 2911 2912 public boolean hasName() { 2913 return this.name != null && !this.name.isEmpty(); 2914 } 2915 2916 /** 2917 * @param value {@link #name} (URI of an external repository which contains further details about the genetics data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2918 */ 2919 public SequenceRepositoryComponent setNameElement(StringType value) { 2920 this.name = value; 2921 return this; 2922 } 2923 2924 /** 2925 * @return URI of an external repository which contains further details about the genetics data. 2926 */ 2927 public String getName() { 2928 return this.name == null ? null : this.name.getValue(); 2929 } 2930 2931 /** 2932 * @param value URI of an external repository which contains further details about the genetics data. 2933 */ 2934 public SequenceRepositoryComponent setName(String value) { 2935 if (Utilities.noString(value)) 2936 this.name = null; 2937 else { 2938 if (this.name == null) 2939 this.name = new StringType(); 2940 this.name.setValue(value); 2941 } 2942 return this; 2943 } 2944 2945 /** 2946 * @return {@link #datasetId} (Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.). This is the underlying object with id, value and extensions. The accessor "getDatasetId" gives direct access to the value 2947 */ 2948 public StringType getDatasetIdElement() { 2949 if (this.datasetId == null) 2950 if (Configuration.errorOnAutoCreate()) 2951 throw new Error("Attempt to auto-create SequenceRepositoryComponent.datasetId"); 2952 else if (Configuration.doAutoCreate()) 2953 this.datasetId = new StringType(); // bb 2954 return this.datasetId; 2955 } 2956 2957 public boolean hasDatasetIdElement() { 2958 return this.datasetId != null && !this.datasetId.isEmpty(); 2959 } 2960 2961 public boolean hasDatasetId() { 2962 return this.datasetId != null && !this.datasetId.isEmpty(); 2963 } 2964 2965 /** 2966 * @param value {@link #datasetId} (Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.). This is the underlying object with id, value and extensions. The accessor "getDatasetId" gives direct access to the value 2967 */ 2968 public SequenceRepositoryComponent setDatasetIdElement(StringType value) { 2969 this.datasetId = value; 2970 return this; 2971 } 2972 2973 /** 2974 * @return Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2975 */ 2976 public String getDatasetId() { 2977 return this.datasetId == null ? null : this.datasetId.getValue(); 2978 } 2979 2980 /** 2981 * @param value Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository. 2982 */ 2983 public SequenceRepositoryComponent setDatasetId(String value) { 2984 if (Utilities.noString(value)) 2985 this.datasetId = null; 2986 else { 2987 if (this.datasetId == null) 2988 this.datasetId = new StringType(); 2989 this.datasetId.setValue(value); 2990 } 2991 return this; 2992 } 2993 2994 /** 2995 * @return {@link #variantsetId} (Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.). This is the underlying object with id, value and extensions. The accessor "getVariantsetId" gives direct access to the value 2996 */ 2997 public StringType getVariantsetIdElement() { 2998 if (this.variantsetId == null) 2999 if (Configuration.errorOnAutoCreate()) 3000 throw new Error("Attempt to auto-create SequenceRepositoryComponent.variantsetId"); 3001 else if (Configuration.doAutoCreate()) 3002 this.variantsetId = new StringType(); // bb 3003 return this.variantsetId; 3004 } 3005 3006 public boolean hasVariantsetIdElement() { 3007 return this.variantsetId != null && !this.variantsetId.isEmpty(); 3008 } 3009 3010 public boolean hasVariantsetId() { 3011 return this.variantsetId != null && !this.variantsetId.isEmpty(); 3012 } 3013 3014 /** 3015 * @param value {@link #variantsetId} (Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.). This is the underlying object with id, value and extensions. The accessor "getVariantsetId" gives direct access to the value 3016 */ 3017 public SequenceRepositoryComponent setVariantsetIdElement(StringType value) { 3018 this.variantsetId = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 3024 */ 3025 public String getVariantsetId() { 3026 return this.variantsetId == null ? null : this.variantsetId.getValue(); 3027 } 3028 3029 /** 3030 * @param value Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository. 3031 */ 3032 public SequenceRepositoryComponent setVariantsetId(String value) { 3033 if (Utilities.noString(value)) 3034 this.variantsetId = null; 3035 else { 3036 if (this.variantsetId == null) 3037 this.variantsetId = new StringType(); 3038 this.variantsetId.setValue(value); 3039 } 3040 return this; 3041 } 3042 3043 /** 3044 * @return {@link #readsetId} (Id of the read in this external repository.). This is the underlying object with id, value and extensions. The accessor "getReadsetId" gives direct access to the value 3045 */ 3046 public StringType getReadsetIdElement() { 3047 if (this.readsetId == null) 3048 if (Configuration.errorOnAutoCreate()) 3049 throw new Error("Attempt to auto-create SequenceRepositoryComponent.readsetId"); 3050 else if (Configuration.doAutoCreate()) 3051 this.readsetId = new StringType(); // bb 3052 return this.readsetId; 3053 } 3054 3055 public boolean hasReadsetIdElement() { 3056 return this.readsetId != null && !this.readsetId.isEmpty(); 3057 } 3058 3059 public boolean hasReadsetId() { 3060 return this.readsetId != null && !this.readsetId.isEmpty(); 3061 } 3062 3063 /** 3064 * @param value {@link #readsetId} (Id of the read in this external repository.). This is the underlying object with id, value and extensions. The accessor "getReadsetId" gives direct access to the value 3065 */ 3066 public SequenceRepositoryComponent setReadsetIdElement(StringType value) { 3067 this.readsetId = value; 3068 return this; 3069 } 3070 3071 /** 3072 * @return Id of the read in this external repository. 3073 */ 3074 public String getReadsetId() { 3075 return this.readsetId == null ? null : this.readsetId.getValue(); 3076 } 3077 3078 /** 3079 * @param value Id of the read in this external repository. 3080 */ 3081 public SequenceRepositoryComponent setReadsetId(String value) { 3082 if (Utilities.noString(value)) 3083 this.readsetId = null; 3084 else { 3085 if (this.readsetId == null) 3086 this.readsetId = new StringType(); 3087 this.readsetId.setValue(value); 3088 } 3089 return this; 3090 } 3091 3092 protected void listChildren(List<Property> children) { 3093 super.listChildren(children); 3094 children.add(new Property("type", "code", "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 0, 1, type)); 3095 children.add(new Property("url", "uri", "URI of an external repository which contains further details about the genetics data.", 0, 1, url)); 3096 children.add(new Property("name", "string", "URI of an external repository which contains further details about the genetics data.", 0, 1, name)); 3097 children.add(new Property("datasetId", "string", "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 0, 1, datasetId)); 3098 children.add(new Property("variantsetId", "string", "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 0, 1, variantsetId)); 3099 children.add(new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId)); 3100 } 3101 3102 @Override 3103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3104 switch (_hash) { 3105 case 3575610: /*type*/ return new Property("type", "code", "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 0, 1, type); 3106 case 116079: /*url*/ return new Property("url", "uri", "URI of an external repository which contains further details about the genetics data.", 0, 1, url); 3107 case 3373707: /*name*/ return new Property("name", "string", "URI of an external repository which contains further details about the genetics data.", 0, 1, name); 3108 case -345342029: /*datasetId*/ return new Property("datasetId", "string", "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 0, 1, datasetId); 3109 case 1929752504: /*variantsetId*/ return new Property("variantsetId", "string", "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 0, 1, variantsetId); 3110 case -1095407289: /*readsetId*/ return new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId); 3111 default: return super.getNamedProperty(_hash, _name, _checkValid); 3112 } 3113 3114 } 3115 3116 @Override 3117 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3118 switch (hash) { 3119 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<RepositoryType> 3120 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3121 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3122 case -345342029: /*datasetId*/ return this.datasetId == null ? new Base[0] : new Base[] {this.datasetId}; // StringType 3123 case 1929752504: /*variantsetId*/ return this.variantsetId == null ? new Base[0] : new Base[] {this.variantsetId}; // StringType 3124 case -1095407289: /*readsetId*/ return this.readsetId == null ? new Base[0] : new Base[] {this.readsetId}; // StringType 3125 default: return super.getProperty(hash, name, checkValid); 3126 } 3127 3128 } 3129 3130 @Override 3131 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3132 switch (hash) { 3133 case 3575610: // type 3134 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 3135 this.type = (Enumeration) value; // Enumeration<RepositoryType> 3136 return value; 3137 case 116079: // url 3138 this.url = castToUri(value); // UriType 3139 return value; 3140 case 3373707: // name 3141 this.name = castToString(value); // StringType 3142 return value; 3143 case -345342029: // datasetId 3144 this.datasetId = castToString(value); // StringType 3145 return value; 3146 case 1929752504: // variantsetId 3147 this.variantsetId = castToString(value); // StringType 3148 return value; 3149 case -1095407289: // readsetId 3150 this.readsetId = castToString(value); // StringType 3151 return value; 3152 default: return super.setProperty(hash, name, value); 3153 } 3154 3155 } 3156 3157 @Override 3158 public Base setProperty(String name, Base value) throws FHIRException { 3159 if (name.equals("type")) { 3160 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 3161 this.type = (Enumeration) value; // Enumeration<RepositoryType> 3162 } else if (name.equals("url")) { 3163 this.url = castToUri(value); // UriType 3164 } else if (name.equals("name")) { 3165 this.name = castToString(value); // StringType 3166 } else if (name.equals("datasetId")) { 3167 this.datasetId = castToString(value); // StringType 3168 } else if (name.equals("variantsetId")) { 3169 this.variantsetId = castToString(value); // StringType 3170 } else if (name.equals("readsetId")) { 3171 this.readsetId = castToString(value); // StringType 3172 } else 3173 return super.setProperty(name, value); 3174 return value; 3175 } 3176 3177 @Override 3178 public Base makeProperty(int hash, String name) throws FHIRException { 3179 switch (hash) { 3180 case 3575610: return getTypeElement(); 3181 case 116079: return getUrlElement(); 3182 case 3373707: return getNameElement(); 3183 case -345342029: return getDatasetIdElement(); 3184 case 1929752504: return getVariantsetIdElement(); 3185 case -1095407289: return getReadsetIdElement(); 3186 default: return super.makeProperty(hash, name); 3187 } 3188 3189 } 3190 3191 @Override 3192 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3193 switch (hash) { 3194 case 3575610: /*type*/ return new String[] {"code"}; 3195 case 116079: /*url*/ return new String[] {"uri"}; 3196 case 3373707: /*name*/ return new String[] {"string"}; 3197 case -345342029: /*datasetId*/ return new String[] {"string"}; 3198 case 1929752504: /*variantsetId*/ return new String[] {"string"}; 3199 case -1095407289: /*readsetId*/ return new String[] {"string"}; 3200 default: return super.getTypesForProperty(hash, name); 3201 } 3202 3203 } 3204 3205 @Override 3206 public Base addChild(String name) throws FHIRException { 3207 if (name.equals("type")) { 3208 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 3209 } 3210 else if (name.equals("url")) { 3211 throw new FHIRException("Cannot call addChild on a singleton property Sequence.url"); 3212 } 3213 else if (name.equals("name")) { 3214 throw new FHIRException("Cannot call addChild on a singleton property Sequence.name"); 3215 } 3216 else if (name.equals("datasetId")) { 3217 throw new FHIRException("Cannot call addChild on a singleton property Sequence.datasetId"); 3218 } 3219 else if (name.equals("variantsetId")) { 3220 throw new FHIRException("Cannot call addChild on a singleton property Sequence.variantsetId"); 3221 } 3222 else if (name.equals("readsetId")) { 3223 throw new FHIRException("Cannot call addChild on a singleton property Sequence.readsetId"); 3224 } 3225 else 3226 return super.addChild(name); 3227 } 3228 3229 public SequenceRepositoryComponent copy() { 3230 SequenceRepositoryComponent dst = new SequenceRepositoryComponent(); 3231 copyValues(dst); 3232 dst.type = type == null ? null : type.copy(); 3233 dst.url = url == null ? null : url.copy(); 3234 dst.name = name == null ? null : name.copy(); 3235 dst.datasetId = datasetId == null ? null : datasetId.copy(); 3236 dst.variantsetId = variantsetId == null ? null : variantsetId.copy(); 3237 dst.readsetId = readsetId == null ? null : readsetId.copy(); 3238 return dst; 3239 } 3240 3241 @Override 3242 public boolean equalsDeep(Base other_) { 3243 if (!super.equalsDeep(other_)) 3244 return false; 3245 if (!(other_ instanceof SequenceRepositoryComponent)) 3246 return false; 3247 SequenceRepositoryComponent o = (SequenceRepositoryComponent) other_; 3248 return compareDeep(type, o.type, true) && compareDeep(url, o.url, true) && compareDeep(name, o.name, true) 3249 && compareDeep(datasetId, o.datasetId, true) && compareDeep(variantsetId, o.variantsetId, true) 3250 && compareDeep(readsetId, o.readsetId, true); 3251 } 3252 3253 @Override 3254 public boolean equalsShallow(Base other_) { 3255 if (!super.equalsShallow(other_)) 3256 return false; 3257 if (!(other_ instanceof SequenceRepositoryComponent)) 3258 return false; 3259 SequenceRepositoryComponent o = (SequenceRepositoryComponent) other_; 3260 return compareValues(type, o.type, true) && compareValues(url, o.url, true) && compareValues(name, o.name, true) 3261 && compareValues(datasetId, o.datasetId, true) && compareValues(variantsetId, o.variantsetId, true) 3262 && compareValues(readsetId, o.readsetId, true); 3263 } 3264 3265 public boolean isEmpty() { 3266 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, url, name, datasetId 3267 , variantsetId, readsetId); 3268 } 3269 3270 public String fhirType() { 3271 return "Sequence.repository"; 3272 3273 } 3274 3275 } 3276 3277 /** 3278 * A unique identifier for this particular sequence instance. This is a FHIR-defined id. 3279 */ 3280 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3281 @Description(shortDefinition="Unique ID for this particular sequence. This is a FHIR-defined id", formalDefinition="A unique identifier for this particular sequence instance. This is a FHIR-defined id." ) 3282 protected List<Identifier> identifier; 3283 3284 /** 3285 * Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3286 */ 3287 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3288 @Description(shortDefinition="aa | dna | rna", formalDefinition="Amino Acid Sequence/ DNA Sequence / RNA Sequence." ) 3289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sequence-type") 3290 protected Enumeration<SequenceType> type; 3291 3292 /** 3293 * Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3294 */ 3295 @Child(name = "coordinateSystem", type = {IntegerType.class}, order=2, min=1, max=1, modifier=false, summary=true) 3296 @Description(shortDefinition="Base number of coordinate system (0 for 0-based numbering or coordinates, inclusive start, exclusive end, 1 for 1-based numbering, inclusive start, inclusive end)", formalDefinition="Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end)." ) 3297 protected IntegerType coordinateSystem; 3298 3299 /** 3300 * The patient whose sequencing results are described by this resource. 3301 */ 3302 @Child(name = "patient", type = {Patient.class}, order=3, min=0, max=1, modifier=false, summary=true) 3303 @Description(shortDefinition="Who and/or what this is about", formalDefinition="The patient whose sequencing results are described by this resource." ) 3304 protected Reference patient; 3305 3306 /** 3307 * The actual object that is the target of the reference (The patient whose sequencing results are described by this resource.) 3308 */ 3309 protected Patient patientTarget; 3310 3311 /** 3312 * Specimen used for sequencing. 3313 */ 3314 @Child(name = "specimen", type = {Specimen.class}, order=4, min=0, max=1, modifier=false, summary=true) 3315 @Description(shortDefinition="Specimen used for sequencing", formalDefinition="Specimen used for sequencing." ) 3316 protected Reference specimen; 3317 3318 /** 3319 * The actual object that is the target of the reference (Specimen used for sequencing.) 3320 */ 3321 protected Specimen specimenTarget; 3322 3323 /** 3324 * The method for sequencing, for example, chip information. 3325 */ 3326 @Child(name = "device", type = {Device.class}, order=5, min=0, max=1, modifier=false, summary=true) 3327 @Description(shortDefinition="The method for sequencing", formalDefinition="The method for sequencing, for example, chip information." ) 3328 protected Reference device; 3329 3330 /** 3331 * The actual object that is the target of the reference (The method for sequencing, for example, chip information.) 3332 */ 3333 protected Device deviceTarget; 3334 3335 /** 3336 * The organization or lab that should be responsible for this result. 3337 */ 3338 @Child(name = "performer", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 3339 @Description(shortDefinition="Who should be responsible for test result", formalDefinition="The organization or lab that should be responsible for this result." ) 3340 protected Reference performer; 3341 3342 /** 3343 * The actual object that is the target of the reference (The organization or lab that should be responsible for this result.) 3344 */ 3345 protected Organization performerTarget; 3346 3347 /** 3348 * The number of copies of the seqeunce of interest. (RNASeq). 3349 */ 3350 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=true) 3351 @Description(shortDefinition="The number of copies of the seqeunce of interest. (RNASeq)", formalDefinition="The number of copies of the seqeunce of interest. (RNASeq)." ) 3352 protected Quantity quantity; 3353 3354 /** 3355 * A sequence that is used as a reference to describe variants that are present in a sequence analyzed. 3356 */ 3357 @Child(name = "referenceSeq", type = {}, order=8, min=0, max=1, modifier=false, summary=true) 3358 @Description(shortDefinition="A sequence used as reference", formalDefinition="A sequence that is used as a reference to describe variants that are present in a sequence analyzed." ) 3359 protected SequenceReferenceSeqComponent referenceSeq; 3360 3361 /** 3362 * The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string. 3363 */ 3364 @Child(name = "variant", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3365 @Description(shortDefinition="Variant in sequence", formalDefinition="The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string." ) 3366 protected List<SequenceVariantComponent> variant; 3367 3368 /** 3369 * Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3370 */ 3371 @Child(name = "observedSeq", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3372 @Description(shortDefinition="Sequence that was observed", formalDefinition="Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd." ) 3373 protected StringType observedSeq; 3374 3375 /** 3376 * An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)). 3377 */ 3378 @Child(name = "quality", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3379 @Description(shortDefinition="An set of value as quality of sequence", formalDefinition="An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686))." ) 3380 protected List<SequenceQualityComponent> quality; 3381 3382 /** 3383 * Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3384 */ 3385 @Child(name = "readCoverage", type = {IntegerType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3386 @Description(shortDefinition="Average number of reads representing a given nucleotide in the reconstructed sequence", formalDefinition="Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence." ) 3387 protected IntegerType readCoverage; 3388 3389 /** 3390 * Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq. 3391 */ 3392 @Child(name = "repository", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3393 @Description(shortDefinition="External repository which contains detailed report related with observedSeq in this resource", formalDefinition="Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq." ) 3394 protected List<SequenceRepositoryComponent> repository; 3395 3396 /** 3397 * Pointer to next atomic sequence which at most contains one variant. 3398 */ 3399 @Child(name = "pointer", type = {Sequence.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3400 @Description(shortDefinition="Pointer to next atomic sequence", formalDefinition="Pointer to next atomic sequence which at most contains one variant." ) 3401 protected List<Reference> pointer; 3402 /** 3403 * The actual objects that are the target of the reference (Pointer to next atomic sequence which at most contains one variant.) 3404 */ 3405 protected List<Sequence> pointerTarget; 3406 3407 3408 private static final long serialVersionUID = -2101352712L; 3409 3410 /** 3411 * Constructor 3412 */ 3413 public Sequence() { 3414 super(); 3415 } 3416 3417 /** 3418 * Constructor 3419 */ 3420 public Sequence(IntegerType coordinateSystem) { 3421 super(); 3422 this.coordinateSystem = coordinateSystem; 3423 } 3424 3425 /** 3426 * @return {@link #identifier} (A unique identifier for this particular sequence instance. This is a FHIR-defined id.) 3427 */ 3428 public List<Identifier> getIdentifier() { 3429 if (this.identifier == null) 3430 this.identifier = new ArrayList<Identifier>(); 3431 return this.identifier; 3432 } 3433 3434 /** 3435 * @return Returns a reference to <code>this</code> for easy method chaining 3436 */ 3437 public Sequence setIdentifier(List<Identifier> theIdentifier) { 3438 this.identifier = theIdentifier; 3439 return this; 3440 } 3441 3442 public boolean hasIdentifier() { 3443 if (this.identifier == null) 3444 return false; 3445 for (Identifier item : this.identifier) 3446 if (!item.isEmpty()) 3447 return true; 3448 return false; 3449 } 3450 3451 public Identifier addIdentifier() { //3 3452 Identifier t = new Identifier(); 3453 if (this.identifier == null) 3454 this.identifier = new ArrayList<Identifier>(); 3455 this.identifier.add(t); 3456 return t; 3457 } 3458 3459 public Sequence addIdentifier(Identifier t) { //3 3460 if (t == null) 3461 return this; 3462 if (this.identifier == null) 3463 this.identifier = new ArrayList<Identifier>(); 3464 this.identifier.add(t); 3465 return this; 3466 } 3467 3468 /** 3469 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3470 */ 3471 public Identifier getIdentifierFirstRep() { 3472 if (getIdentifier().isEmpty()) { 3473 addIdentifier(); 3474 } 3475 return getIdentifier().get(0); 3476 } 3477 3478 /** 3479 * @return {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3480 */ 3481 public Enumeration<SequenceType> getTypeElement() { 3482 if (this.type == null) 3483 if (Configuration.errorOnAutoCreate()) 3484 throw new Error("Attempt to auto-create Sequence.type"); 3485 else if (Configuration.doAutoCreate()) 3486 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); // bb 3487 return this.type; 3488 } 3489 3490 public boolean hasTypeElement() { 3491 return this.type != null && !this.type.isEmpty(); 3492 } 3493 3494 public boolean hasType() { 3495 return this.type != null && !this.type.isEmpty(); 3496 } 3497 3498 /** 3499 * @param value {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3500 */ 3501 public Sequence setTypeElement(Enumeration<SequenceType> value) { 3502 this.type = value; 3503 return this; 3504 } 3505 3506 /** 3507 * @return Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3508 */ 3509 public SequenceType getType() { 3510 return this.type == null ? null : this.type.getValue(); 3511 } 3512 3513 /** 3514 * @param value Amino Acid Sequence/ DNA Sequence / RNA Sequence. 3515 */ 3516 public Sequence setType(SequenceType value) { 3517 if (value == null) 3518 this.type = null; 3519 else { 3520 if (this.type == null) 3521 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); 3522 this.type.setValue(value); 3523 } 3524 return this; 3525 } 3526 3527 /** 3528 * @return {@link #coordinateSystem} (Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).). This is the underlying object with id, value and extensions. The accessor "getCoordinateSystem" gives direct access to the value 3529 */ 3530 public IntegerType getCoordinateSystemElement() { 3531 if (this.coordinateSystem == null) 3532 if (Configuration.errorOnAutoCreate()) 3533 throw new Error("Attempt to auto-create Sequence.coordinateSystem"); 3534 else if (Configuration.doAutoCreate()) 3535 this.coordinateSystem = new IntegerType(); // bb 3536 return this.coordinateSystem; 3537 } 3538 3539 public boolean hasCoordinateSystemElement() { 3540 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 3541 } 3542 3543 public boolean hasCoordinateSystem() { 3544 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 3545 } 3546 3547 /** 3548 * @param value {@link #coordinateSystem} (Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).). This is the underlying object with id, value and extensions. The accessor "getCoordinateSystem" gives direct access to the value 3549 */ 3550 public Sequence setCoordinateSystemElement(IntegerType value) { 3551 this.coordinateSystem = value; 3552 return this; 3553 } 3554 3555 /** 3556 * @return Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3557 */ 3558 public int getCoordinateSystem() { 3559 return this.coordinateSystem == null || this.coordinateSystem.isEmpty() ? 0 : this.coordinateSystem.getValue(); 3560 } 3561 3562 /** 3563 * @param value Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end). 3564 */ 3565 public Sequence setCoordinateSystem(int value) { 3566 if (this.coordinateSystem == null) 3567 this.coordinateSystem = new IntegerType(); 3568 this.coordinateSystem.setValue(value); 3569 return this; 3570 } 3571 3572 /** 3573 * @return {@link #patient} (The patient whose sequencing results are described by this resource.) 3574 */ 3575 public Reference getPatient() { 3576 if (this.patient == null) 3577 if (Configuration.errorOnAutoCreate()) 3578 throw new Error("Attempt to auto-create Sequence.patient"); 3579 else if (Configuration.doAutoCreate()) 3580 this.patient = new Reference(); // cc 3581 return this.patient; 3582 } 3583 3584 public boolean hasPatient() { 3585 return this.patient != null && !this.patient.isEmpty(); 3586 } 3587 3588 /** 3589 * @param value {@link #patient} (The patient whose sequencing results are described by this resource.) 3590 */ 3591 public Sequence setPatient(Reference value) { 3592 this.patient = value; 3593 return this; 3594 } 3595 3596 /** 3597 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient whose sequencing results are described by this resource.) 3598 */ 3599 public Patient getPatientTarget() { 3600 if (this.patientTarget == null) 3601 if (Configuration.errorOnAutoCreate()) 3602 throw new Error("Attempt to auto-create Sequence.patient"); 3603 else if (Configuration.doAutoCreate()) 3604 this.patientTarget = new Patient(); // aa 3605 return this.patientTarget; 3606 } 3607 3608 /** 3609 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient whose sequencing results are described by this resource.) 3610 */ 3611 public Sequence setPatientTarget(Patient value) { 3612 this.patientTarget = value; 3613 return this; 3614 } 3615 3616 /** 3617 * @return {@link #specimen} (Specimen used for sequencing.) 3618 */ 3619 public Reference getSpecimen() { 3620 if (this.specimen == null) 3621 if (Configuration.errorOnAutoCreate()) 3622 throw new Error("Attempt to auto-create Sequence.specimen"); 3623 else if (Configuration.doAutoCreate()) 3624 this.specimen = new Reference(); // cc 3625 return this.specimen; 3626 } 3627 3628 public boolean hasSpecimen() { 3629 return this.specimen != null && !this.specimen.isEmpty(); 3630 } 3631 3632 /** 3633 * @param value {@link #specimen} (Specimen used for sequencing.) 3634 */ 3635 public Sequence setSpecimen(Reference value) { 3636 this.specimen = value; 3637 return this; 3638 } 3639 3640 /** 3641 * @return {@link #specimen} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Specimen used for sequencing.) 3642 */ 3643 public Specimen getSpecimenTarget() { 3644 if (this.specimenTarget == null) 3645 if (Configuration.errorOnAutoCreate()) 3646 throw new Error("Attempt to auto-create Sequence.specimen"); 3647 else if (Configuration.doAutoCreate()) 3648 this.specimenTarget = new Specimen(); // aa 3649 return this.specimenTarget; 3650 } 3651 3652 /** 3653 * @param value {@link #specimen} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Specimen used for sequencing.) 3654 */ 3655 public Sequence setSpecimenTarget(Specimen value) { 3656 this.specimenTarget = value; 3657 return this; 3658 } 3659 3660 /** 3661 * @return {@link #device} (The method for sequencing, for example, chip information.) 3662 */ 3663 public Reference getDevice() { 3664 if (this.device == null) 3665 if (Configuration.errorOnAutoCreate()) 3666 throw new Error("Attempt to auto-create Sequence.device"); 3667 else if (Configuration.doAutoCreate()) 3668 this.device = new Reference(); // cc 3669 return this.device; 3670 } 3671 3672 public boolean hasDevice() { 3673 return this.device != null && !this.device.isEmpty(); 3674 } 3675 3676 /** 3677 * @param value {@link #device} (The method for sequencing, for example, chip information.) 3678 */ 3679 public Sequence setDevice(Reference value) { 3680 this.device = value; 3681 return this; 3682 } 3683 3684 /** 3685 * @return {@link #device} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The method for sequencing, for example, chip information.) 3686 */ 3687 public Device getDeviceTarget() { 3688 if (this.deviceTarget == null) 3689 if (Configuration.errorOnAutoCreate()) 3690 throw new Error("Attempt to auto-create Sequence.device"); 3691 else if (Configuration.doAutoCreate()) 3692 this.deviceTarget = new Device(); // aa 3693 return this.deviceTarget; 3694 } 3695 3696 /** 3697 * @param value {@link #device} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The method for sequencing, for example, chip information.) 3698 */ 3699 public Sequence setDeviceTarget(Device value) { 3700 this.deviceTarget = value; 3701 return this; 3702 } 3703 3704 /** 3705 * @return {@link #performer} (The organization or lab that should be responsible for this result.) 3706 */ 3707 public Reference getPerformer() { 3708 if (this.performer == null) 3709 if (Configuration.errorOnAutoCreate()) 3710 throw new Error("Attempt to auto-create Sequence.performer"); 3711 else if (Configuration.doAutoCreate()) 3712 this.performer = new Reference(); // cc 3713 return this.performer; 3714 } 3715 3716 public boolean hasPerformer() { 3717 return this.performer != null && !this.performer.isEmpty(); 3718 } 3719 3720 /** 3721 * @param value {@link #performer} (The organization or lab that should be responsible for this result.) 3722 */ 3723 public Sequence setPerformer(Reference value) { 3724 this.performer = value; 3725 return this; 3726 } 3727 3728 /** 3729 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization or lab that should be responsible for this result.) 3730 */ 3731 public Organization getPerformerTarget() { 3732 if (this.performerTarget == null) 3733 if (Configuration.errorOnAutoCreate()) 3734 throw new Error("Attempt to auto-create Sequence.performer"); 3735 else if (Configuration.doAutoCreate()) 3736 this.performerTarget = new Organization(); // aa 3737 return this.performerTarget; 3738 } 3739 3740 /** 3741 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization or lab that should be responsible for this result.) 3742 */ 3743 public Sequence setPerformerTarget(Organization value) { 3744 this.performerTarget = value; 3745 return this; 3746 } 3747 3748 /** 3749 * @return {@link #quantity} (The number of copies of the seqeunce of interest. (RNASeq).) 3750 */ 3751 public Quantity getQuantity() { 3752 if (this.quantity == null) 3753 if (Configuration.errorOnAutoCreate()) 3754 throw new Error("Attempt to auto-create Sequence.quantity"); 3755 else if (Configuration.doAutoCreate()) 3756 this.quantity = new Quantity(); // cc 3757 return this.quantity; 3758 } 3759 3760 public boolean hasQuantity() { 3761 return this.quantity != null && !this.quantity.isEmpty(); 3762 } 3763 3764 /** 3765 * @param value {@link #quantity} (The number of copies of the seqeunce of interest. (RNASeq).) 3766 */ 3767 public Sequence setQuantity(Quantity value) { 3768 this.quantity = value; 3769 return this; 3770 } 3771 3772 /** 3773 * @return {@link #referenceSeq} (A sequence that is used as a reference to describe variants that are present in a sequence analyzed.) 3774 */ 3775 public SequenceReferenceSeqComponent getReferenceSeq() { 3776 if (this.referenceSeq == null) 3777 if (Configuration.errorOnAutoCreate()) 3778 throw new Error("Attempt to auto-create Sequence.referenceSeq"); 3779 else if (Configuration.doAutoCreate()) 3780 this.referenceSeq = new SequenceReferenceSeqComponent(); // cc 3781 return this.referenceSeq; 3782 } 3783 3784 public boolean hasReferenceSeq() { 3785 return this.referenceSeq != null && !this.referenceSeq.isEmpty(); 3786 } 3787 3788 /** 3789 * @param value {@link #referenceSeq} (A sequence that is used as a reference to describe variants that are present in a sequence analyzed.) 3790 */ 3791 public Sequence setReferenceSeq(SequenceReferenceSeqComponent value) { 3792 this.referenceSeq = value; 3793 return this; 3794 } 3795 3796 /** 3797 * @return {@link #variant} (The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.) 3798 */ 3799 public List<SequenceVariantComponent> getVariant() { 3800 if (this.variant == null) 3801 this.variant = new ArrayList<SequenceVariantComponent>(); 3802 return this.variant; 3803 } 3804 3805 /** 3806 * @return Returns a reference to <code>this</code> for easy method chaining 3807 */ 3808 public Sequence setVariant(List<SequenceVariantComponent> theVariant) { 3809 this.variant = theVariant; 3810 return this; 3811 } 3812 3813 public boolean hasVariant() { 3814 if (this.variant == null) 3815 return false; 3816 for (SequenceVariantComponent item : this.variant) 3817 if (!item.isEmpty()) 3818 return true; 3819 return false; 3820 } 3821 3822 public SequenceVariantComponent addVariant() { //3 3823 SequenceVariantComponent t = new SequenceVariantComponent(); 3824 if (this.variant == null) 3825 this.variant = new ArrayList<SequenceVariantComponent>(); 3826 this.variant.add(t); 3827 return t; 3828 } 3829 3830 public Sequence addVariant(SequenceVariantComponent t) { //3 3831 if (t == null) 3832 return this; 3833 if (this.variant == null) 3834 this.variant = new ArrayList<SequenceVariantComponent>(); 3835 this.variant.add(t); 3836 return this; 3837 } 3838 3839 /** 3840 * @return The first repetition of repeating field {@link #variant}, creating it if it does not already exist 3841 */ 3842 public SequenceVariantComponent getVariantFirstRep() { 3843 if (getVariant().isEmpty()) { 3844 addVariant(); 3845 } 3846 return getVariant().get(0); 3847 } 3848 3849 /** 3850 * @return {@link #observedSeq} (Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.). This is the underlying object with id, value and extensions. The accessor "getObservedSeq" gives direct access to the value 3851 */ 3852 public StringType getObservedSeqElement() { 3853 if (this.observedSeq == null) 3854 if (Configuration.errorOnAutoCreate()) 3855 throw new Error("Attempt to auto-create Sequence.observedSeq"); 3856 else if (Configuration.doAutoCreate()) 3857 this.observedSeq = new StringType(); // bb 3858 return this.observedSeq; 3859 } 3860 3861 public boolean hasObservedSeqElement() { 3862 return this.observedSeq != null && !this.observedSeq.isEmpty(); 3863 } 3864 3865 public boolean hasObservedSeq() { 3866 return this.observedSeq != null && !this.observedSeq.isEmpty(); 3867 } 3868 3869 /** 3870 * @param value {@link #observedSeq} (Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.). This is the underlying object with id, value and extensions. The accessor "getObservedSeq" gives direct access to the value 3871 */ 3872 public Sequence setObservedSeqElement(StringType value) { 3873 this.observedSeq = value; 3874 return this; 3875 } 3876 3877 /** 3878 * @return Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3879 */ 3880 public String getObservedSeq() { 3881 return this.observedSeq == null ? null : this.observedSeq.getValue(); 3882 } 3883 3884 /** 3885 * @param value Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd. 3886 */ 3887 public Sequence setObservedSeq(String value) { 3888 if (Utilities.noString(value)) 3889 this.observedSeq = null; 3890 else { 3891 if (this.observedSeq == null) 3892 this.observedSeq = new StringType(); 3893 this.observedSeq.setValue(value); 3894 } 3895 return this; 3896 } 3897 3898 /** 3899 * @return {@link #quality} (An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).) 3900 */ 3901 public List<SequenceQualityComponent> getQuality() { 3902 if (this.quality == null) 3903 this.quality = new ArrayList<SequenceQualityComponent>(); 3904 return this.quality; 3905 } 3906 3907 /** 3908 * @return Returns a reference to <code>this</code> for easy method chaining 3909 */ 3910 public Sequence setQuality(List<SequenceQualityComponent> theQuality) { 3911 this.quality = theQuality; 3912 return this; 3913 } 3914 3915 public boolean hasQuality() { 3916 if (this.quality == null) 3917 return false; 3918 for (SequenceQualityComponent item : this.quality) 3919 if (!item.isEmpty()) 3920 return true; 3921 return false; 3922 } 3923 3924 public SequenceQualityComponent addQuality() { //3 3925 SequenceQualityComponent t = new SequenceQualityComponent(); 3926 if (this.quality == null) 3927 this.quality = new ArrayList<SequenceQualityComponent>(); 3928 this.quality.add(t); 3929 return t; 3930 } 3931 3932 public Sequence addQuality(SequenceQualityComponent t) { //3 3933 if (t == null) 3934 return this; 3935 if (this.quality == null) 3936 this.quality = new ArrayList<SequenceQualityComponent>(); 3937 this.quality.add(t); 3938 return this; 3939 } 3940 3941 /** 3942 * @return The first repetition of repeating field {@link #quality}, creating it if it does not already exist 3943 */ 3944 public SequenceQualityComponent getQualityFirstRep() { 3945 if (getQuality().isEmpty()) { 3946 addQuality(); 3947 } 3948 return getQuality().get(0); 3949 } 3950 3951 /** 3952 * @return {@link #readCoverage} (Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.). This is the underlying object with id, value and extensions. The accessor "getReadCoverage" gives direct access to the value 3953 */ 3954 public IntegerType getReadCoverageElement() { 3955 if (this.readCoverage == null) 3956 if (Configuration.errorOnAutoCreate()) 3957 throw new Error("Attempt to auto-create Sequence.readCoverage"); 3958 else if (Configuration.doAutoCreate()) 3959 this.readCoverage = new IntegerType(); // bb 3960 return this.readCoverage; 3961 } 3962 3963 public boolean hasReadCoverageElement() { 3964 return this.readCoverage != null && !this.readCoverage.isEmpty(); 3965 } 3966 3967 public boolean hasReadCoverage() { 3968 return this.readCoverage != null && !this.readCoverage.isEmpty(); 3969 } 3970 3971 /** 3972 * @param value {@link #readCoverage} (Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.). This is the underlying object with id, value and extensions. The accessor "getReadCoverage" gives direct access to the value 3973 */ 3974 public Sequence setReadCoverageElement(IntegerType value) { 3975 this.readCoverage = value; 3976 return this; 3977 } 3978 3979 /** 3980 * @return Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3981 */ 3982 public int getReadCoverage() { 3983 return this.readCoverage == null || this.readCoverage.isEmpty() ? 0 : this.readCoverage.getValue(); 3984 } 3985 3986 /** 3987 * @param value Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence. 3988 */ 3989 public Sequence setReadCoverage(int value) { 3990 if (this.readCoverage == null) 3991 this.readCoverage = new IntegerType(); 3992 this.readCoverage.setValue(value); 3993 return this; 3994 } 3995 3996 /** 3997 * @return {@link #repository} (Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.) 3998 */ 3999 public List<SequenceRepositoryComponent> getRepository() { 4000 if (this.repository == null) 4001 this.repository = new ArrayList<SequenceRepositoryComponent>(); 4002 return this.repository; 4003 } 4004 4005 /** 4006 * @return Returns a reference to <code>this</code> for easy method chaining 4007 */ 4008 public Sequence setRepository(List<SequenceRepositoryComponent> theRepository) { 4009 this.repository = theRepository; 4010 return this; 4011 } 4012 4013 public boolean hasRepository() { 4014 if (this.repository == null) 4015 return false; 4016 for (SequenceRepositoryComponent item : this.repository) 4017 if (!item.isEmpty()) 4018 return true; 4019 return false; 4020 } 4021 4022 public SequenceRepositoryComponent addRepository() { //3 4023 SequenceRepositoryComponent t = new SequenceRepositoryComponent(); 4024 if (this.repository == null) 4025 this.repository = new ArrayList<SequenceRepositoryComponent>(); 4026 this.repository.add(t); 4027 return t; 4028 } 4029 4030 public Sequence addRepository(SequenceRepositoryComponent t) { //3 4031 if (t == null) 4032 return this; 4033 if (this.repository == null) 4034 this.repository = new ArrayList<SequenceRepositoryComponent>(); 4035 this.repository.add(t); 4036 return this; 4037 } 4038 4039 /** 4040 * @return The first repetition of repeating field {@link #repository}, creating it if it does not already exist 4041 */ 4042 public SequenceRepositoryComponent getRepositoryFirstRep() { 4043 if (getRepository().isEmpty()) { 4044 addRepository(); 4045 } 4046 return getRepository().get(0); 4047 } 4048 4049 /** 4050 * @return {@link #pointer} (Pointer to next atomic sequence which at most contains one variant.) 4051 */ 4052 public List<Reference> getPointer() { 4053 if (this.pointer == null) 4054 this.pointer = new ArrayList<Reference>(); 4055 return this.pointer; 4056 } 4057 4058 /** 4059 * @return Returns a reference to <code>this</code> for easy method chaining 4060 */ 4061 public Sequence setPointer(List<Reference> thePointer) { 4062 this.pointer = thePointer; 4063 return this; 4064 } 4065 4066 public boolean hasPointer() { 4067 if (this.pointer == null) 4068 return false; 4069 for (Reference item : this.pointer) 4070 if (!item.isEmpty()) 4071 return true; 4072 return false; 4073 } 4074 4075 public Reference addPointer() { //3 4076 Reference t = new Reference(); 4077 if (this.pointer == null) 4078 this.pointer = new ArrayList<Reference>(); 4079 this.pointer.add(t); 4080 return t; 4081 } 4082 4083 public Sequence addPointer(Reference t) { //3 4084 if (t == null) 4085 return this; 4086 if (this.pointer == null) 4087 this.pointer = new ArrayList<Reference>(); 4088 this.pointer.add(t); 4089 return this; 4090 } 4091 4092 /** 4093 * @return The first repetition of repeating field {@link #pointer}, creating it if it does not already exist 4094 */ 4095 public Reference getPointerFirstRep() { 4096 if (getPointer().isEmpty()) { 4097 addPointer(); 4098 } 4099 return getPointer().get(0); 4100 } 4101 4102 /** 4103 * @deprecated Use Reference#setResource(IBaseResource) instead 4104 */ 4105 @Deprecated 4106 public List<Sequence> getPointerTarget() { 4107 if (this.pointerTarget == null) 4108 this.pointerTarget = new ArrayList<Sequence>(); 4109 return this.pointerTarget; 4110 } 4111 4112 /** 4113 * @deprecated Use Reference#setResource(IBaseResource) instead 4114 */ 4115 @Deprecated 4116 public Sequence addPointerTarget() { 4117 Sequence r = new Sequence(); 4118 if (this.pointerTarget == null) 4119 this.pointerTarget = new ArrayList<Sequence>(); 4120 this.pointerTarget.add(r); 4121 return r; 4122 } 4123 4124 protected void listChildren(List<Property> children) { 4125 super.listChildren(children); 4126 children.add(new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4127 children.add(new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type)); 4128 children.add(new Property("coordinateSystem", "integer", "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 0, 1, coordinateSystem)); 4129 children.add(new Property("patient", "Reference(Patient)", "The patient whose sequencing results are described by this resource.", 0, 1, patient)); 4130 children.add(new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen)); 4131 children.add(new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device)); 4132 children.add(new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer)); 4133 children.add(new Property("quantity", "Quantity", "The number of copies of the seqeunce of interest. (RNASeq).", 0, 1, quantity)); 4134 children.add(new Property("referenceSeq", "", "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, referenceSeq)); 4135 children.add(new Property("variant", "", "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 0, java.lang.Integer.MAX_VALUE, variant)); 4136 children.add(new Property("observedSeq", "string", "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 0, 1, observedSeq)); 4137 children.add(new Property("quality", "", "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 0, java.lang.Integer.MAX_VALUE, quality)); 4138 children.add(new Property("readCoverage", "integer", "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 0, 1, readCoverage)); 4139 children.add(new Property("repository", "", "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 0, java.lang.Integer.MAX_VALUE, repository)); 4140 children.add(new Property("pointer", "Reference(Sequence)", "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, pointer)); 4141 } 4142 4143 @Override 4144 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4145 switch (_hash) { 4146 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, java.lang.Integer.MAX_VALUE, identifier); 4147 case 3575610: /*type*/ return new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type); 4148 case 354212295: /*coordinateSystem*/ return new Property("coordinateSystem", "integer", "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 0, 1, coordinateSystem); 4149 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient whose sequencing results are described by this resource.", 0, 1, patient); 4150 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen); 4151 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The method for sequencing, for example, chip information.", 0, 1, device); 4152 case 481140686: /*performer*/ return new Property("performer", "Reference(Organization)", "The organization or lab that should be responsible for this result.", 0, 1, performer); 4153 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of copies of the seqeunce of interest. (RNASeq).", 0, 1, quantity); 4154 case -502547180: /*referenceSeq*/ return new Property("referenceSeq", "", "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, referenceSeq); 4155 case 236785797: /*variant*/ return new Property("variant", "", "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 0, java.lang.Integer.MAX_VALUE, variant); 4156 case 125541495: /*observedSeq*/ return new Property("observedSeq", "string", "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall starts from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 0, 1, observedSeq); 4157 case 651215103: /*quality*/ return new Property("quality", "", "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 0, java.lang.Integer.MAX_VALUE, quality); 4158 case -1798816354: /*readCoverage*/ return new Property("readCoverage", "integer", "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 0, 1, readCoverage); 4159 case 1950800714: /*repository*/ return new Property("repository", "", "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 0, java.lang.Integer.MAX_VALUE, repository); 4160 case -400605635: /*pointer*/ return new Property("pointer", "Reference(Sequence)", "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, pointer); 4161 default: return super.getNamedProperty(_hash, _name, _checkValid); 4162 } 4163 4164 } 4165 4166 @Override 4167 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4168 switch (hash) { 4169 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4170 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SequenceType> 4171 case 354212295: /*coordinateSystem*/ return this.coordinateSystem == null ? new Base[0] : new Base[] {this.coordinateSystem}; // IntegerType 4172 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 4173 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : new Base[] {this.specimen}; // Reference 4174 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 4175 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 4176 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4177 case -502547180: /*referenceSeq*/ return this.referenceSeq == null ? new Base[0] : new Base[] {this.referenceSeq}; // SequenceReferenceSeqComponent 4178 case 236785797: /*variant*/ return this.variant == null ? new Base[0] : this.variant.toArray(new Base[this.variant.size()]); // SequenceVariantComponent 4179 case 125541495: /*observedSeq*/ return this.observedSeq == null ? new Base[0] : new Base[] {this.observedSeq}; // StringType 4180 case 651215103: /*quality*/ return this.quality == null ? new Base[0] : this.quality.toArray(new Base[this.quality.size()]); // SequenceQualityComponent 4181 case -1798816354: /*readCoverage*/ return this.readCoverage == null ? new Base[0] : new Base[] {this.readCoverage}; // IntegerType 4182 case 1950800714: /*repository*/ return this.repository == null ? new Base[0] : this.repository.toArray(new Base[this.repository.size()]); // SequenceRepositoryComponent 4183 case -400605635: /*pointer*/ return this.pointer == null ? new Base[0] : this.pointer.toArray(new Base[this.pointer.size()]); // Reference 4184 default: return super.getProperty(hash, name, checkValid); 4185 } 4186 4187 } 4188 4189 @Override 4190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4191 switch (hash) { 4192 case -1618432855: // identifier 4193 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4194 return value; 4195 case 3575610: // type 4196 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 4197 this.type = (Enumeration) value; // Enumeration<SequenceType> 4198 return value; 4199 case 354212295: // coordinateSystem 4200 this.coordinateSystem = castToInteger(value); // IntegerType 4201 return value; 4202 case -791418107: // patient 4203 this.patient = castToReference(value); // Reference 4204 return value; 4205 case -2132868344: // specimen 4206 this.specimen = castToReference(value); // Reference 4207 return value; 4208 case -1335157162: // device 4209 this.device = castToReference(value); // Reference 4210 return value; 4211 case 481140686: // performer 4212 this.performer = castToReference(value); // Reference 4213 return value; 4214 case -1285004149: // quantity 4215 this.quantity = castToQuantity(value); // Quantity 4216 return value; 4217 case -502547180: // referenceSeq 4218 this.referenceSeq = (SequenceReferenceSeqComponent) value; // SequenceReferenceSeqComponent 4219 return value; 4220 case 236785797: // variant 4221 this.getVariant().add((SequenceVariantComponent) value); // SequenceVariantComponent 4222 return value; 4223 case 125541495: // observedSeq 4224 this.observedSeq = castToString(value); // StringType 4225 return value; 4226 case 651215103: // quality 4227 this.getQuality().add((SequenceQualityComponent) value); // SequenceQualityComponent 4228 return value; 4229 case -1798816354: // readCoverage 4230 this.readCoverage = castToInteger(value); // IntegerType 4231 return value; 4232 case 1950800714: // repository 4233 this.getRepository().add((SequenceRepositoryComponent) value); // SequenceRepositoryComponent 4234 return value; 4235 case -400605635: // pointer 4236 this.getPointer().add(castToReference(value)); // Reference 4237 return value; 4238 default: return super.setProperty(hash, name, value); 4239 } 4240 4241 } 4242 4243 @Override 4244 public Base setProperty(String name, Base value) throws FHIRException { 4245 if (name.equals("identifier")) { 4246 this.getIdentifier().add(castToIdentifier(value)); 4247 } else if (name.equals("type")) { 4248 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 4249 this.type = (Enumeration) value; // Enumeration<SequenceType> 4250 } else if (name.equals("coordinateSystem")) { 4251 this.coordinateSystem = castToInteger(value); // IntegerType 4252 } else if (name.equals("patient")) { 4253 this.patient = castToReference(value); // Reference 4254 } else if (name.equals("specimen")) { 4255 this.specimen = castToReference(value); // Reference 4256 } else if (name.equals("device")) { 4257 this.device = castToReference(value); // Reference 4258 } else if (name.equals("performer")) { 4259 this.performer = castToReference(value); // Reference 4260 } else if (name.equals("quantity")) { 4261 this.quantity = castToQuantity(value); // Quantity 4262 } else if (name.equals("referenceSeq")) { 4263 this.referenceSeq = (SequenceReferenceSeqComponent) value; // SequenceReferenceSeqComponent 4264 } else if (name.equals("variant")) { 4265 this.getVariant().add((SequenceVariantComponent) value); 4266 } else if (name.equals("observedSeq")) { 4267 this.observedSeq = castToString(value); // StringType 4268 } else if (name.equals("quality")) { 4269 this.getQuality().add((SequenceQualityComponent) value); 4270 } else if (name.equals("readCoverage")) { 4271 this.readCoverage = castToInteger(value); // IntegerType 4272 } else if (name.equals("repository")) { 4273 this.getRepository().add((SequenceRepositoryComponent) value); 4274 } else if (name.equals("pointer")) { 4275 this.getPointer().add(castToReference(value)); 4276 } else 4277 return super.setProperty(name, value); 4278 return value; 4279 } 4280 4281 @Override 4282 public Base makeProperty(int hash, String name) throws FHIRException { 4283 switch (hash) { 4284 case -1618432855: return addIdentifier(); 4285 case 3575610: return getTypeElement(); 4286 case 354212295: return getCoordinateSystemElement(); 4287 case -791418107: return getPatient(); 4288 case -2132868344: return getSpecimen(); 4289 case -1335157162: return getDevice(); 4290 case 481140686: return getPerformer(); 4291 case -1285004149: return getQuantity(); 4292 case -502547180: return getReferenceSeq(); 4293 case 236785797: return addVariant(); 4294 case 125541495: return getObservedSeqElement(); 4295 case 651215103: return addQuality(); 4296 case -1798816354: return getReadCoverageElement(); 4297 case 1950800714: return addRepository(); 4298 case -400605635: return addPointer(); 4299 default: return super.makeProperty(hash, name); 4300 } 4301 4302 } 4303 4304 @Override 4305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4306 switch (hash) { 4307 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4308 case 3575610: /*type*/ return new String[] {"code"}; 4309 case 354212295: /*coordinateSystem*/ return new String[] {"integer"}; 4310 case -791418107: /*patient*/ return new String[] {"Reference"}; 4311 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 4312 case -1335157162: /*device*/ return new String[] {"Reference"}; 4313 case 481140686: /*performer*/ return new String[] {"Reference"}; 4314 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4315 case -502547180: /*referenceSeq*/ return new String[] {}; 4316 case 236785797: /*variant*/ return new String[] {}; 4317 case 125541495: /*observedSeq*/ return new String[] {"string"}; 4318 case 651215103: /*quality*/ return new String[] {}; 4319 case -1798816354: /*readCoverage*/ return new String[] {"integer"}; 4320 case 1950800714: /*repository*/ return new String[] {}; 4321 case -400605635: /*pointer*/ return new String[] {"Reference"}; 4322 default: return super.getTypesForProperty(hash, name); 4323 } 4324 4325 } 4326 4327 @Override 4328 public Base addChild(String name) throws FHIRException { 4329 if (name.equals("identifier")) { 4330 return addIdentifier(); 4331 } 4332 else if (name.equals("type")) { 4333 throw new FHIRException("Cannot call addChild on a singleton property Sequence.type"); 4334 } 4335 else if (name.equals("coordinateSystem")) { 4336 throw new FHIRException("Cannot call addChild on a singleton property Sequence.coordinateSystem"); 4337 } 4338 else if (name.equals("patient")) { 4339 this.patient = new Reference(); 4340 return this.patient; 4341 } 4342 else if (name.equals("specimen")) { 4343 this.specimen = new Reference(); 4344 return this.specimen; 4345 } 4346 else if (name.equals("device")) { 4347 this.device = new Reference(); 4348 return this.device; 4349 } 4350 else if (name.equals("performer")) { 4351 this.performer = new Reference(); 4352 return this.performer; 4353 } 4354 else if (name.equals("quantity")) { 4355 this.quantity = new Quantity(); 4356 return this.quantity; 4357 } 4358 else if (name.equals("referenceSeq")) { 4359 this.referenceSeq = new SequenceReferenceSeqComponent(); 4360 return this.referenceSeq; 4361 } 4362 else if (name.equals("variant")) { 4363 return addVariant(); 4364 } 4365 else if (name.equals("observedSeq")) { 4366 throw new FHIRException("Cannot call addChild on a singleton property Sequence.observedSeq"); 4367 } 4368 else if (name.equals("quality")) { 4369 return addQuality(); 4370 } 4371 else if (name.equals("readCoverage")) { 4372 throw new FHIRException("Cannot call addChild on a singleton property Sequence.readCoverage"); 4373 } 4374 else if (name.equals("repository")) { 4375 return addRepository(); 4376 } 4377 else if (name.equals("pointer")) { 4378 return addPointer(); 4379 } 4380 else 4381 return super.addChild(name); 4382 } 4383 4384 public String fhirType() { 4385 return "Sequence"; 4386 4387 } 4388 4389 public Sequence copy() { 4390 Sequence dst = new Sequence(); 4391 copyValues(dst); 4392 if (identifier != null) { 4393 dst.identifier = new ArrayList<Identifier>(); 4394 for (Identifier i : identifier) 4395 dst.identifier.add(i.copy()); 4396 }; 4397 dst.type = type == null ? null : type.copy(); 4398 dst.coordinateSystem = coordinateSystem == null ? null : coordinateSystem.copy(); 4399 dst.patient = patient == null ? null : patient.copy(); 4400 dst.specimen = specimen == null ? null : specimen.copy(); 4401 dst.device = device == null ? null : device.copy(); 4402 dst.performer = performer == null ? null : performer.copy(); 4403 dst.quantity = quantity == null ? null : quantity.copy(); 4404 dst.referenceSeq = referenceSeq == null ? null : referenceSeq.copy(); 4405 if (variant != null) { 4406 dst.variant = new ArrayList<SequenceVariantComponent>(); 4407 for (SequenceVariantComponent i : variant) 4408 dst.variant.add(i.copy()); 4409 }; 4410 dst.observedSeq = observedSeq == null ? null : observedSeq.copy(); 4411 if (quality != null) { 4412 dst.quality = new ArrayList<SequenceQualityComponent>(); 4413 for (SequenceQualityComponent i : quality) 4414 dst.quality.add(i.copy()); 4415 }; 4416 dst.readCoverage = readCoverage == null ? null : readCoverage.copy(); 4417 if (repository != null) { 4418 dst.repository = new ArrayList<SequenceRepositoryComponent>(); 4419 for (SequenceRepositoryComponent i : repository) 4420 dst.repository.add(i.copy()); 4421 }; 4422 if (pointer != null) { 4423 dst.pointer = new ArrayList<Reference>(); 4424 for (Reference i : pointer) 4425 dst.pointer.add(i.copy()); 4426 }; 4427 return dst; 4428 } 4429 4430 protected Sequence typedCopy() { 4431 return copy(); 4432 } 4433 4434 @Override 4435 public boolean equalsDeep(Base other_) { 4436 if (!super.equalsDeep(other_)) 4437 return false; 4438 if (!(other_ instanceof Sequence)) 4439 return false; 4440 Sequence o = (Sequence) other_; 4441 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(coordinateSystem, o.coordinateSystem, true) 4442 && compareDeep(patient, o.patient, true) && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4443 && compareDeep(performer, o.performer, true) && compareDeep(quantity, o.quantity, true) && compareDeep(referenceSeq, o.referenceSeq, true) 4444 && compareDeep(variant, o.variant, true) && compareDeep(observedSeq, o.observedSeq, true) && compareDeep(quality, o.quality, true) 4445 && compareDeep(readCoverage, o.readCoverage, true) && compareDeep(repository, o.repository, true) 4446 && compareDeep(pointer, o.pointer, true); 4447 } 4448 4449 @Override 4450 public boolean equalsShallow(Base other_) { 4451 if (!super.equalsShallow(other_)) 4452 return false; 4453 if (!(other_ instanceof Sequence)) 4454 return false; 4455 Sequence o = (Sequence) other_; 4456 return compareValues(type, o.type, true) && compareValues(coordinateSystem, o.coordinateSystem, true) 4457 && compareValues(observedSeq, o.observedSeq, true) && compareValues(readCoverage, o.readCoverage, true) 4458 ; 4459 } 4460 4461 public boolean isEmpty() { 4462 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coordinateSystem 4463 , patient, specimen, device, performer, quantity, referenceSeq, variant, observedSeq 4464 , quality, readCoverage, repository, pointer); 4465 } 4466 4467 @Override 4468 public ResourceType getResourceType() { 4469 return ResourceType.Sequence; 4470 } 4471 4472 /** 4473 * Search parameter: <b>identifier</b> 4474 * <p> 4475 * Description: <b>The unique identity for a particular sequence</b><br> 4476 * Type: <b>token</b><br> 4477 * Path: <b>Sequence.identifier</b><br> 4478 * </p> 4479 */ 4480 @SearchParamDefinition(name="identifier", path="Sequence.identifier", description="The unique identity for a particular sequence", type="token" ) 4481 public static final String SP_IDENTIFIER = "identifier"; 4482 /** 4483 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4484 * <p> 4485 * Description: <b>The unique identity for a particular sequence</b><br> 4486 * Type: <b>token</b><br> 4487 * Path: <b>Sequence.identifier</b><br> 4488 * </p> 4489 */ 4490 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4491 4492 /** 4493 * Search parameter: <b>coordinate</b> 4494 * <p> 4495 * Description: <b>Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.</b><br> 4496 * Type: <b>composite</b><br> 4497 * Path: <b></b><br> 4498 * </p> 4499 */ 4500 @SearchParamDefinition(name="coordinate", path="Sequence.variant", description="Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type="composite", compositeOf={"chromosome", "start"} ) 4501 public static final String SP_COORDINATE = "coordinate"; 4502 /** 4503 * <b>Fluent Client</b> search parameter constant for <b>coordinate</b> 4504 * <p> 4505 * Description: <b>Search parameter for region of the reference DNA sequence string. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `coordinate=1$lt345$gt123`, this means it will search for the Sequence resource on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.</b><br> 4506 * Type: <b>composite</b><br> 4507 * Path: <b></b><br> 4508 * </p> 4509 */ 4510 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>(SP_COORDINATE); 4511 4512 /** 4513 * Search parameter: <b>patient</b> 4514 * <p> 4515 * Description: <b>The subject that the observation is about</b><br> 4516 * Type: <b>reference</b><br> 4517 * Path: <b>Sequence.patient</b><br> 4518 * </p> 4519 */ 4520 @SearchParamDefinition(name="patient", path="Sequence.patient", description="The subject that the observation is about", type="reference", target={Patient.class } ) 4521 public static final String SP_PATIENT = "patient"; 4522 /** 4523 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4524 * <p> 4525 * Description: <b>The subject that the observation is about</b><br> 4526 * Type: <b>reference</b><br> 4527 * Path: <b>Sequence.patient</b><br> 4528 * </p> 4529 */ 4530 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4531 4532/** 4533 * Constant for fluent queries to be used to add include statements. Specifies 4534 * the path value of "<b>Sequence:patient</b>". 4535 */ 4536 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Sequence:patient").toLocked(); 4537 4538 /** 4539 * Search parameter: <b>chromosome</b> 4540 * <p> 4541 * Description: <b>Chromosome number of the reference sequence</b><br> 4542 * Type: <b>token</b><br> 4543 * Path: <b>Sequence.referenceSeq.chromosome</b><br> 4544 * </p> 4545 */ 4546 @SearchParamDefinition(name="chromosome", path="Sequence.referenceSeq.chromosome", description="Chromosome number of the reference sequence", type="token" ) 4547 public static final String SP_CHROMOSOME = "chromosome"; 4548 /** 4549 * <b>Fluent Client</b> search parameter constant for <b>chromosome</b> 4550 * <p> 4551 * Description: <b>Chromosome number of the reference sequence</b><br> 4552 * Type: <b>token</b><br> 4553 * Path: <b>Sequence.referenceSeq.chromosome</b><br> 4554 * </p> 4555 */ 4556 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHROMOSOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHROMOSOME); 4557 4558 /** 4559 * Search parameter: <b>start</b> 4560 * <p> 4561 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.</b><br> 4562 * Type: <b>number</b><br> 4563 * Path: <b>Sequence.referenceSeq.windowStart</b><br> 4564 * </p> 4565 */ 4566 @SearchParamDefinition(name="start", path="Sequence.referenceSeq.windowStart", description="Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.", type="number" ) 4567 public static final String SP_START = "start"; 4568 /** 4569 * <b>Fluent Client</b> search parameter constant for <b>start</b> 4570 * <p> 4571 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.</b><br> 4572 * Type: <b>number</b><br> 4573 * Path: <b>Sequence.referenceSeq.windowStart</b><br> 4574 * </p> 4575 */ 4576 public static final ca.uhn.fhir.rest.gclient.NumberClientParam START = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_START); 4577 4578 /** 4579 * Search parameter: <b>end</b> 4580 * <p> 4581 * Description: <b>End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.</b><br> 4582 * Type: <b>number</b><br> 4583 * Path: <b>Sequence.referenceSeq.windowEnd</b><br> 4584 * </p> 4585 */ 4586 @SearchParamDefinition(name="end", path="Sequence.referenceSeq.windowEnd", description="End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.", type="number" ) 4587 public static final String SP_END = "end"; 4588 /** 4589 * <b>Fluent Client</b> search parameter constant for <b>end</b> 4590 * <p> 4591 * Description: <b>End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.</b><br> 4592 * Type: <b>number</b><br> 4593 * Path: <b>Sequence.referenceSeq.windowEnd</b><br> 4594 * </p> 4595 */ 4596 public static final ca.uhn.fhir.rest.gclient.NumberClientParam END = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_END); 4597 4598 /** 4599 * Search parameter: <b>type</b> 4600 * <p> 4601 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 4602 * Type: <b>token</b><br> 4603 * Path: <b>Sequence.type</b><br> 4604 * </p> 4605 */ 4606 @SearchParamDefinition(name="type", path="Sequence.type", description="Amino Acid Sequence/ DNA Sequence / RNA Sequence", type="token" ) 4607 public static final String SP_TYPE = "type"; 4608 /** 4609 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4610 * <p> 4611 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 4612 * Type: <b>token</b><br> 4613 * Path: <b>Sequence.type</b><br> 4614 * </p> 4615 */ 4616 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4617 4618 4619}