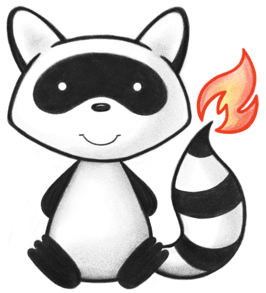
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 051 */ 052@ResourceDef(name="ServiceDefinition", profile="http://hl7.org/fhir/Profile/ServiceDefinition") 053@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "description", "purpose", "usage", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "topic", "contributor", "contact", "copyright", "relatedArtifact", "trigger", "dataRequirement", "operationDefinition"}) 054public class ServiceDefinition extends MetadataResource { 055 056 /** 057 * A formal identifier that is used to identify this service definition when it is represented in other formats, or referenced in a specification, model, design or an instance. This is used for CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts. 058 */ 059 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 060 @Description(shortDefinition="Additional identifier for the service definition", formalDefinition="A formal identifier that is used to identify this service definition when it is represented in other formats, or referenced in a specification, model, design or an instance. This is used for CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts." ) 061 protected List<Identifier> identifier; 062 063 /** 064 * Explaination of why this service definition is needed and why it has been designed as it has. 065 */ 066 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 067 @Description(shortDefinition="Why this service definition is defined", formalDefinition="Explaination of why this service definition is needed and why it has been designed as it has." ) 068 protected MarkdownType purpose; 069 070 /** 071 * A detailed description of how the module is used from a clinical perspective. 072 */ 073 @Child(name = "usage", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 074 @Description(shortDefinition="Describes the clinical usage of the module", formalDefinition="A detailed description of how the module is used from a clinical perspective." ) 075 protected StringType usage; 076 077 /** 078 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 079 */ 080 @Child(name = "approvalDate", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=false) 081 @Description(shortDefinition="When the service definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 082 protected DateType approvalDate; 083 084 /** 085 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 086 */ 087 @Child(name = "lastReviewDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 088 @Description(shortDefinition="When the service definition was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 089 protected DateType lastReviewDate; 090 091 /** 092 * The period during which the service definition content was or is planned to be in active use. 093 */ 094 @Child(name = "effectivePeriod", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 095 @Description(shortDefinition="When the service definition is expected to be used", formalDefinition="The period during which the service definition content was or is planned to be in active use." ) 096 protected Period effectivePeriod; 097 098 /** 099 * Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching. 100 */ 101 @Child(name = "topic", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 102 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching." ) 103 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 104 protected List<CodeableConcept> topic; 105 106 /** 107 * A contributor to the content of the module, including authors, editors, reviewers, and endorsers. 108 */ 109 @Child(name = "contributor", type = {Contributor.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 110 @Description(shortDefinition="A content contributor", formalDefinition="A contributor to the content of the module, including authors, editors, reviewers, and endorsers." ) 111 protected List<Contributor> contributor; 112 113 /** 114 * A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition. 115 */ 116 @Child(name = "copyright", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 117 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition." ) 118 protected MarkdownType copyright; 119 120 /** 121 * Related resources such as additional documentation, justification, or bibliographic references. 122 */ 123 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 124 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related resources such as additional documentation, justification, or bibliographic references." ) 125 protected List<RelatedArtifact> relatedArtifact; 126 127 /** 128 * The trigger element defines when the rule should be invoked. This information is used by consumers of the rule to determine how to integrate the rule into a specific workflow. 129 */ 130 @Child(name = "trigger", type = {TriggerDefinition.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 131 @Description(shortDefinition="\"when\" the module should be invoked", formalDefinition="The trigger element defines when the rule should be invoked. This information is used by consumers of the rule to determine how to integrate the rule into a specific workflow." ) 132 protected List<TriggerDefinition> trigger; 133 134 /** 135 * Data requirements are a machine processable description of the data required by the module in order to perform a successful evaluation. 136 */ 137 @Child(name = "dataRequirement", type = {DataRequirement.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 138 @Description(shortDefinition="What data is used by the module", formalDefinition="Data requirements are a machine processable description of the data required by the module in order to perform a successful evaluation." ) 139 protected List<DataRequirement> dataRequirement; 140 141 /** 142 * A reference to the operation that is used to invoke this service. 143 */ 144 @Child(name = "operationDefinition", type = {OperationDefinition.class}, order=12, min=0, max=1, modifier=false, summary=false) 145 @Description(shortDefinition="Operation to invoke", formalDefinition="A reference to the operation that is used to invoke this service." ) 146 protected Reference operationDefinition; 147 148 /** 149 * The actual object that is the target of the reference (A reference to the operation that is used to invoke this service.) 150 */ 151 protected OperationDefinition operationDefinitionTarget; 152 153 private static final long serialVersionUID = -1517933892L; 154 155 /** 156 * Constructor 157 */ 158 public ServiceDefinition() { 159 super(); 160 } 161 162 /** 163 * Constructor 164 */ 165 public ServiceDefinition(Enumeration<PublicationStatus> status) { 166 super(); 167 this.status = status; 168 } 169 170 /** 171 * @return {@link #url} (An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 172 */ 173 public UriType getUrlElement() { 174 if (this.url == null) 175 if (Configuration.errorOnAutoCreate()) 176 throw new Error("Attempt to auto-create ServiceDefinition.url"); 177 else if (Configuration.doAutoCreate()) 178 this.url = new UriType(); // bb 179 return this.url; 180 } 181 182 public boolean hasUrlElement() { 183 return this.url != null && !this.url.isEmpty(); 184 } 185 186 public boolean hasUrl() { 187 return this.url != null && !this.url.isEmpty(); 188 } 189 190 /** 191 * @param value {@link #url} (An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 192 */ 193 public ServiceDefinition setUrlElement(UriType value) { 194 this.url = value; 195 return this; 196 } 197 198 /** 199 * @return An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions). 200 */ 201 public String getUrl() { 202 return this.url == null ? null : this.url.getValue(); 203 } 204 205 /** 206 * @param value An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions). 207 */ 208 public ServiceDefinition setUrl(String value) { 209 if (Utilities.noString(value)) 210 this.url = null; 211 else { 212 if (this.url == null) 213 this.url = new UriType(); 214 this.url.setValue(value); 215 } 216 return this; 217 } 218 219 /** 220 * @return {@link #identifier} (A formal identifier that is used to identify this service definition when it is represented in other formats, or referenced in a specification, model, design or an instance. This is used for CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.) 221 */ 222 public List<Identifier> getIdentifier() { 223 if (this.identifier == null) 224 this.identifier = new ArrayList<Identifier>(); 225 return this.identifier; 226 } 227 228 /** 229 * @return Returns a reference to <code>this</code> for easy method chaining 230 */ 231 public ServiceDefinition setIdentifier(List<Identifier> theIdentifier) { 232 this.identifier = theIdentifier; 233 return this; 234 } 235 236 public boolean hasIdentifier() { 237 if (this.identifier == null) 238 return false; 239 for (Identifier item : this.identifier) 240 if (!item.isEmpty()) 241 return true; 242 return false; 243 } 244 245 public Identifier addIdentifier() { //3 246 Identifier t = new Identifier(); 247 if (this.identifier == null) 248 this.identifier = new ArrayList<Identifier>(); 249 this.identifier.add(t); 250 return t; 251 } 252 253 public ServiceDefinition addIdentifier(Identifier t) { //3 254 if (t == null) 255 return this; 256 if (this.identifier == null) 257 this.identifier = new ArrayList<Identifier>(); 258 this.identifier.add(t); 259 return this; 260 } 261 262 /** 263 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 264 */ 265 public Identifier getIdentifierFirstRep() { 266 if (getIdentifier().isEmpty()) { 267 addIdentifier(); 268 } 269 return getIdentifier().get(0); 270 } 271 272 /** 273 * @return {@link #version} (The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 274 */ 275 public StringType getVersionElement() { 276 if (this.version == null) 277 if (Configuration.errorOnAutoCreate()) 278 throw new Error("Attempt to auto-create ServiceDefinition.version"); 279 else if (Configuration.doAutoCreate()) 280 this.version = new StringType(); // bb 281 return this.version; 282 } 283 284 public boolean hasVersionElement() { 285 return this.version != null && !this.version.isEmpty(); 286 } 287 288 public boolean hasVersion() { 289 return this.version != null && !this.version.isEmpty(); 290 } 291 292 /** 293 * @param value {@link #version} (The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 294 */ 295 public ServiceDefinition setVersionElement(StringType value) { 296 this.version = value; 297 return this; 298 } 299 300 /** 301 * @return The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 302 */ 303 public String getVersion() { 304 return this.version == null ? null : this.version.getValue(); 305 } 306 307 /** 308 * @param value The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 309 */ 310 public ServiceDefinition setVersion(String value) { 311 if (Utilities.noString(value)) 312 this.version = null; 313 else { 314 if (this.version == null) 315 this.version = new StringType(); 316 this.version.setValue(value); 317 } 318 return this; 319 } 320 321 /** 322 * @return {@link #name} (A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 323 */ 324 public StringType getNameElement() { 325 if (this.name == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create ServiceDefinition.name"); 328 else if (Configuration.doAutoCreate()) 329 this.name = new StringType(); // bb 330 return this.name; 331 } 332 333 public boolean hasNameElement() { 334 return this.name != null && !this.name.isEmpty(); 335 } 336 337 public boolean hasName() { 338 return this.name != null && !this.name.isEmpty(); 339 } 340 341 /** 342 * @param value {@link #name} (A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 343 */ 344 public ServiceDefinition setNameElement(StringType value) { 345 this.name = value; 346 return this; 347 } 348 349 /** 350 * @return A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 351 */ 352 public String getName() { 353 return this.name == null ? null : this.name.getValue(); 354 } 355 356 /** 357 * @param value A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 358 */ 359 public ServiceDefinition setName(String value) { 360 if (Utilities.noString(value)) 361 this.name = null; 362 else { 363 if (this.name == null) 364 this.name = new StringType(); 365 this.name.setValue(value); 366 } 367 return this; 368 } 369 370 /** 371 * @return {@link #title} (A short, descriptive, user-friendly title for the service definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 372 */ 373 public StringType getTitleElement() { 374 if (this.title == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create ServiceDefinition.title"); 377 else if (Configuration.doAutoCreate()) 378 this.title = new StringType(); // bb 379 return this.title; 380 } 381 382 public boolean hasTitleElement() { 383 return this.title != null && !this.title.isEmpty(); 384 } 385 386 public boolean hasTitle() { 387 return this.title != null && !this.title.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #title} (A short, descriptive, user-friendly title for the service definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 392 */ 393 public ServiceDefinition setTitleElement(StringType value) { 394 this.title = value; 395 return this; 396 } 397 398 /** 399 * @return A short, descriptive, user-friendly title for the service definition. 400 */ 401 public String getTitle() { 402 return this.title == null ? null : this.title.getValue(); 403 } 404 405 /** 406 * @param value A short, descriptive, user-friendly title for the service definition. 407 */ 408 public ServiceDefinition setTitle(String value) { 409 if (Utilities.noString(value)) 410 this.title = null; 411 else { 412 if (this.title == null) 413 this.title = new StringType(); 414 this.title.setValue(value); 415 } 416 return this; 417 } 418 419 /** 420 * @return {@link #status} (The status of this service definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 421 */ 422 public Enumeration<PublicationStatus> getStatusElement() { 423 if (this.status == null) 424 if (Configuration.errorOnAutoCreate()) 425 throw new Error("Attempt to auto-create ServiceDefinition.status"); 426 else if (Configuration.doAutoCreate()) 427 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 428 return this.status; 429 } 430 431 public boolean hasStatusElement() { 432 return this.status != null && !this.status.isEmpty(); 433 } 434 435 public boolean hasStatus() { 436 return this.status != null && !this.status.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #status} (The status of this service definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 441 */ 442 public ServiceDefinition setStatusElement(Enumeration<PublicationStatus> value) { 443 this.status = value; 444 return this; 445 } 446 447 /** 448 * @return The status of this service definition. Enables tracking the life-cycle of the content. 449 */ 450 public PublicationStatus getStatus() { 451 return this.status == null ? null : this.status.getValue(); 452 } 453 454 /** 455 * @param value The status of this service definition. Enables tracking the life-cycle of the content. 456 */ 457 public ServiceDefinition setStatus(PublicationStatus value) { 458 if (this.status == null) 459 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 460 this.status.setValue(value); 461 return this; 462 } 463 464 /** 465 * @return {@link #experimental} (A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 466 */ 467 public BooleanType getExperimentalElement() { 468 if (this.experimental == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create ServiceDefinition.experimental"); 471 else if (Configuration.doAutoCreate()) 472 this.experimental = new BooleanType(); // bb 473 return this.experimental; 474 } 475 476 public boolean hasExperimentalElement() { 477 return this.experimental != null && !this.experimental.isEmpty(); 478 } 479 480 public boolean hasExperimental() { 481 return this.experimental != null && !this.experimental.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #experimental} (A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 486 */ 487 public ServiceDefinition setExperimentalElement(BooleanType value) { 488 this.experimental = value; 489 return this; 490 } 491 492 /** 493 * @return A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 494 */ 495 public boolean getExperimental() { 496 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 497 } 498 499 /** 500 * @param value A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 501 */ 502 public ServiceDefinition setExperimental(boolean value) { 503 if (this.experimental == null) 504 this.experimental = new BooleanType(); 505 this.experimental.setValue(value); 506 return this; 507 } 508 509 /** 510 * @return {@link #date} (The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 511 */ 512 public DateTimeType getDateElement() { 513 if (this.date == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create ServiceDefinition.date"); 516 else if (Configuration.doAutoCreate()) 517 this.date = new DateTimeType(); // bb 518 return this.date; 519 } 520 521 public boolean hasDateElement() { 522 return this.date != null && !this.date.isEmpty(); 523 } 524 525 public boolean hasDate() { 526 return this.date != null && !this.date.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #date} (The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 531 */ 532 public ServiceDefinition setDateElement(DateTimeType value) { 533 this.date = value; 534 return this; 535 } 536 537 /** 538 * @return The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes. 539 */ 540 public Date getDate() { 541 return this.date == null ? null : this.date.getValue(); 542 } 543 544 /** 545 * @param value The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes. 546 */ 547 public ServiceDefinition setDate(Date value) { 548 if (value == null) 549 this.date = null; 550 else { 551 if (this.date == null) 552 this.date = new DateTimeType(); 553 this.date.setValue(value); 554 } 555 return this; 556 } 557 558 /** 559 * @return {@link #publisher} (The name of the individual or organization that published the service definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 560 */ 561 public StringType getPublisherElement() { 562 if (this.publisher == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create ServiceDefinition.publisher"); 565 else if (Configuration.doAutoCreate()) 566 this.publisher = new StringType(); // bb 567 return this.publisher; 568 } 569 570 public boolean hasPublisherElement() { 571 return this.publisher != null && !this.publisher.isEmpty(); 572 } 573 574 public boolean hasPublisher() { 575 return this.publisher != null && !this.publisher.isEmpty(); 576 } 577 578 /** 579 * @param value {@link #publisher} (The name of the individual or organization that published the service definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 580 */ 581 public ServiceDefinition setPublisherElement(StringType value) { 582 this.publisher = value; 583 return this; 584 } 585 586 /** 587 * @return The name of the individual or organization that published the service definition. 588 */ 589 public String getPublisher() { 590 return this.publisher == null ? null : this.publisher.getValue(); 591 } 592 593 /** 594 * @param value The name of the individual or organization that published the service definition. 595 */ 596 public ServiceDefinition setPublisher(String value) { 597 if (Utilities.noString(value)) 598 this.publisher = null; 599 else { 600 if (this.publisher == null) 601 this.publisher = new StringType(); 602 this.publisher.setValue(value); 603 } 604 return this; 605 } 606 607 /** 608 * @return {@link #description} (A free text natural language description of the service definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 609 */ 610 public MarkdownType getDescriptionElement() { 611 if (this.description == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create ServiceDefinition.description"); 614 else if (Configuration.doAutoCreate()) 615 this.description = new MarkdownType(); // bb 616 return this.description; 617 } 618 619 public boolean hasDescriptionElement() { 620 return this.description != null && !this.description.isEmpty(); 621 } 622 623 public boolean hasDescription() { 624 return this.description != null && !this.description.isEmpty(); 625 } 626 627 /** 628 * @param value {@link #description} (A free text natural language description of the service definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 629 */ 630 public ServiceDefinition setDescriptionElement(MarkdownType value) { 631 this.description = value; 632 return this; 633 } 634 635 /** 636 * @return A free text natural language description of the service definition from a consumer's perspective. 637 */ 638 public String getDescription() { 639 return this.description == null ? null : this.description.getValue(); 640 } 641 642 /** 643 * @param value A free text natural language description of the service definition from a consumer's perspective. 644 */ 645 public ServiceDefinition setDescription(String value) { 646 if (value == null) 647 this.description = null; 648 else { 649 if (this.description == null) 650 this.description = new MarkdownType(); 651 this.description.setValue(value); 652 } 653 return this; 654 } 655 656 /** 657 * @return {@link #purpose} (Explaination of why this service definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 658 */ 659 public MarkdownType getPurposeElement() { 660 if (this.purpose == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create ServiceDefinition.purpose"); 663 else if (Configuration.doAutoCreate()) 664 this.purpose = new MarkdownType(); // bb 665 return this.purpose; 666 } 667 668 public boolean hasPurposeElement() { 669 return this.purpose != null && !this.purpose.isEmpty(); 670 } 671 672 public boolean hasPurpose() { 673 return this.purpose != null && !this.purpose.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #purpose} (Explaination of why this service definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 678 */ 679 public ServiceDefinition setPurposeElement(MarkdownType value) { 680 this.purpose = value; 681 return this; 682 } 683 684 /** 685 * @return Explaination of why this service definition is needed and why it has been designed as it has. 686 */ 687 public String getPurpose() { 688 return this.purpose == null ? null : this.purpose.getValue(); 689 } 690 691 /** 692 * @param value Explaination of why this service definition is needed and why it has been designed as it has. 693 */ 694 public ServiceDefinition setPurpose(String value) { 695 if (value == null) 696 this.purpose = null; 697 else { 698 if (this.purpose == null) 699 this.purpose = new MarkdownType(); 700 this.purpose.setValue(value); 701 } 702 return this; 703 } 704 705 /** 706 * @return {@link #usage} (A detailed description of how the module is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 707 */ 708 public StringType getUsageElement() { 709 if (this.usage == null) 710 if (Configuration.errorOnAutoCreate()) 711 throw new Error("Attempt to auto-create ServiceDefinition.usage"); 712 else if (Configuration.doAutoCreate()) 713 this.usage = new StringType(); // bb 714 return this.usage; 715 } 716 717 public boolean hasUsageElement() { 718 return this.usage != null && !this.usage.isEmpty(); 719 } 720 721 public boolean hasUsage() { 722 return this.usage != null && !this.usage.isEmpty(); 723 } 724 725 /** 726 * @param value {@link #usage} (A detailed description of how the module is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 727 */ 728 public ServiceDefinition setUsageElement(StringType value) { 729 this.usage = value; 730 return this; 731 } 732 733 /** 734 * @return A detailed description of how the module is used from a clinical perspective. 735 */ 736 public String getUsage() { 737 return this.usage == null ? null : this.usage.getValue(); 738 } 739 740 /** 741 * @param value A detailed description of how the module is used from a clinical perspective. 742 */ 743 public ServiceDefinition setUsage(String value) { 744 if (Utilities.noString(value)) 745 this.usage = null; 746 else { 747 if (this.usage == null) 748 this.usage = new StringType(); 749 this.usage.setValue(value); 750 } 751 return this; 752 } 753 754 /** 755 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 756 */ 757 public DateType getApprovalDateElement() { 758 if (this.approvalDate == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create ServiceDefinition.approvalDate"); 761 else if (Configuration.doAutoCreate()) 762 this.approvalDate = new DateType(); // bb 763 return this.approvalDate; 764 } 765 766 public boolean hasApprovalDateElement() { 767 return this.approvalDate != null && !this.approvalDate.isEmpty(); 768 } 769 770 public boolean hasApprovalDate() { 771 return this.approvalDate != null && !this.approvalDate.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 776 */ 777 public ServiceDefinition setApprovalDateElement(DateType value) { 778 this.approvalDate = value; 779 return this; 780 } 781 782 /** 783 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 784 */ 785 public Date getApprovalDate() { 786 return this.approvalDate == null ? null : this.approvalDate.getValue(); 787 } 788 789 /** 790 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 791 */ 792 public ServiceDefinition setApprovalDate(Date value) { 793 if (value == null) 794 this.approvalDate = null; 795 else { 796 if (this.approvalDate == null) 797 this.approvalDate = new DateType(); 798 this.approvalDate.setValue(value); 799 } 800 return this; 801 } 802 803 /** 804 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 805 */ 806 public DateType getLastReviewDateElement() { 807 if (this.lastReviewDate == null) 808 if (Configuration.errorOnAutoCreate()) 809 throw new Error("Attempt to auto-create ServiceDefinition.lastReviewDate"); 810 else if (Configuration.doAutoCreate()) 811 this.lastReviewDate = new DateType(); // bb 812 return this.lastReviewDate; 813 } 814 815 public boolean hasLastReviewDateElement() { 816 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 817 } 818 819 public boolean hasLastReviewDate() { 820 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 821 } 822 823 /** 824 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 825 */ 826 public ServiceDefinition setLastReviewDateElement(DateType value) { 827 this.lastReviewDate = value; 828 return this; 829 } 830 831 /** 832 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 833 */ 834 public Date getLastReviewDate() { 835 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 836 } 837 838 /** 839 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 840 */ 841 public ServiceDefinition setLastReviewDate(Date value) { 842 if (value == null) 843 this.lastReviewDate = null; 844 else { 845 if (this.lastReviewDate == null) 846 this.lastReviewDate = new DateType(); 847 this.lastReviewDate.setValue(value); 848 } 849 return this; 850 } 851 852 /** 853 * @return {@link #effectivePeriod} (The period during which the service definition content was or is planned to be in active use.) 854 */ 855 public Period getEffectivePeriod() { 856 if (this.effectivePeriod == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create ServiceDefinition.effectivePeriod"); 859 else if (Configuration.doAutoCreate()) 860 this.effectivePeriod = new Period(); // cc 861 return this.effectivePeriod; 862 } 863 864 public boolean hasEffectivePeriod() { 865 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #effectivePeriod} (The period during which the service definition content was or is planned to be in active use.) 870 */ 871 public ServiceDefinition setEffectivePeriod(Period value) { 872 this.effectivePeriod = value; 873 return this; 874 } 875 876 /** 877 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate service definition instances.) 878 */ 879 public List<UsageContext> getUseContext() { 880 if (this.useContext == null) 881 this.useContext = new ArrayList<UsageContext>(); 882 return this.useContext; 883 } 884 885 /** 886 * @return Returns a reference to <code>this</code> for easy method chaining 887 */ 888 public ServiceDefinition setUseContext(List<UsageContext> theUseContext) { 889 this.useContext = theUseContext; 890 return this; 891 } 892 893 public boolean hasUseContext() { 894 if (this.useContext == null) 895 return false; 896 for (UsageContext item : this.useContext) 897 if (!item.isEmpty()) 898 return true; 899 return false; 900 } 901 902 public UsageContext addUseContext() { //3 903 UsageContext t = new UsageContext(); 904 if (this.useContext == null) 905 this.useContext = new ArrayList<UsageContext>(); 906 this.useContext.add(t); 907 return t; 908 } 909 910 public ServiceDefinition addUseContext(UsageContext t) { //3 911 if (t == null) 912 return this; 913 if (this.useContext == null) 914 this.useContext = new ArrayList<UsageContext>(); 915 this.useContext.add(t); 916 return this; 917 } 918 919 /** 920 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 921 */ 922 public UsageContext getUseContextFirstRep() { 923 if (getUseContext().isEmpty()) { 924 addUseContext(); 925 } 926 return getUseContext().get(0); 927 } 928 929 /** 930 * @return {@link #jurisdiction} (A legal or geographic region in which the service definition is intended to be used.) 931 */ 932 public List<CodeableConcept> getJurisdiction() { 933 if (this.jurisdiction == null) 934 this.jurisdiction = new ArrayList<CodeableConcept>(); 935 return this.jurisdiction; 936 } 937 938 /** 939 * @return Returns a reference to <code>this</code> for easy method chaining 940 */ 941 public ServiceDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 942 this.jurisdiction = theJurisdiction; 943 return this; 944 } 945 946 public boolean hasJurisdiction() { 947 if (this.jurisdiction == null) 948 return false; 949 for (CodeableConcept item : this.jurisdiction) 950 if (!item.isEmpty()) 951 return true; 952 return false; 953 } 954 955 public CodeableConcept addJurisdiction() { //3 956 CodeableConcept t = new CodeableConcept(); 957 if (this.jurisdiction == null) 958 this.jurisdiction = new ArrayList<CodeableConcept>(); 959 this.jurisdiction.add(t); 960 return t; 961 } 962 963 public ServiceDefinition addJurisdiction(CodeableConcept t) { //3 964 if (t == null) 965 return this; 966 if (this.jurisdiction == null) 967 this.jurisdiction = new ArrayList<CodeableConcept>(); 968 this.jurisdiction.add(t); 969 return this; 970 } 971 972 /** 973 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 974 */ 975 public CodeableConcept getJurisdictionFirstRep() { 976 if (getJurisdiction().isEmpty()) { 977 addJurisdiction(); 978 } 979 return getJurisdiction().get(0); 980 } 981 982 /** 983 * @return {@link #topic} (Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.) 984 */ 985 public List<CodeableConcept> getTopic() { 986 if (this.topic == null) 987 this.topic = new ArrayList<CodeableConcept>(); 988 return this.topic; 989 } 990 991 /** 992 * @return Returns a reference to <code>this</code> for easy method chaining 993 */ 994 public ServiceDefinition setTopic(List<CodeableConcept> theTopic) { 995 this.topic = theTopic; 996 return this; 997 } 998 999 public boolean hasTopic() { 1000 if (this.topic == null) 1001 return false; 1002 for (CodeableConcept item : this.topic) 1003 if (!item.isEmpty()) 1004 return true; 1005 return false; 1006 } 1007 1008 public CodeableConcept addTopic() { //3 1009 CodeableConcept t = new CodeableConcept(); 1010 if (this.topic == null) 1011 this.topic = new ArrayList<CodeableConcept>(); 1012 this.topic.add(t); 1013 return t; 1014 } 1015 1016 public ServiceDefinition addTopic(CodeableConcept t) { //3 1017 if (t == null) 1018 return this; 1019 if (this.topic == null) 1020 this.topic = new ArrayList<CodeableConcept>(); 1021 this.topic.add(t); 1022 return this; 1023 } 1024 1025 /** 1026 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1027 */ 1028 public CodeableConcept getTopicFirstRep() { 1029 if (getTopic().isEmpty()) { 1030 addTopic(); 1031 } 1032 return getTopic().get(0); 1033 } 1034 1035 /** 1036 * @return {@link #contributor} (A contributor to the content of the module, including authors, editors, reviewers, and endorsers.) 1037 */ 1038 public List<Contributor> getContributor() { 1039 if (this.contributor == null) 1040 this.contributor = new ArrayList<Contributor>(); 1041 return this.contributor; 1042 } 1043 1044 /** 1045 * @return Returns a reference to <code>this</code> for easy method chaining 1046 */ 1047 public ServiceDefinition setContributor(List<Contributor> theContributor) { 1048 this.contributor = theContributor; 1049 return this; 1050 } 1051 1052 public boolean hasContributor() { 1053 if (this.contributor == null) 1054 return false; 1055 for (Contributor item : this.contributor) 1056 if (!item.isEmpty()) 1057 return true; 1058 return false; 1059 } 1060 1061 public Contributor addContributor() { //3 1062 Contributor t = new Contributor(); 1063 if (this.contributor == null) 1064 this.contributor = new ArrayList<Contributor>(); 1065 this.contributor.add(t); 1066 return t; 1067 } 1068 1069 public ServiceDefinition addContributor(Contributor t) { //3 1070 if (t == null) 1071 return this; 1072 if (this.contributor == null) 1073 this.contributor = new ArrayList<Contributor>(); 1074 this.contributor.add(t); 1075 return this; 1076 } 1077 1078 /** 1079 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist 1080 */ 1081 public Contributor getContributorFirstRep() { 1082 if (getContributor().isEmpty()) { 1083 addContributor(); 1084 } 1085 return getContributor().get(0); 1086 } 1087 1088 /** 1089 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1090 */ 1091 public List<ContactDetail> getContact() { 1092 if (this.contact == null) 1093 this.contact = new ArrayList<ContactDetail>(); 1094 return this.contact; 1095 } 1096 1097 /** 1098 * @return Returns a reference to <code>this</code> for easy method chaining 1099 */ 1100 public ServiceDefinition setContact(List<ContactDetail> theContact) { 1101 this.contact = theContact; 1102 return this; 1103 } 1104 1105 public boolean hasContact() { 1106 if (this.contact == null) 1107 return false; 1108 for (ContactDetail item : this.contact) 1109 if (!item.isEmpty()) 1110 return true; 1111 return false; 1112 } 1113 1114 public ContactDetail addContact() { //3 1115 ContactDetail t = new ContactDetail(); 1116 if (this.contact == null) 1117 this.contact = new ArrayList<ContactDetail>(); 1118 this.contact.add(t); 1119 return t; 1120 } 1121 1122 public ServiceDefinition addContact(ContactDetail t) { //3 1123 if (t == null) 1124 return this; 1125 if (this.contact == null) 1126 this.contact = new ArrayList<ContactDetail>(); 1127 this.contact.add(t); 1128 return this; 1129 } 1130 1131 /** 1132 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1133 */ 1134 public ContactDetail getContactFirstRep() { 1135 if (getContact().isEmpty()) { 1136 addContact(); 1137 } 1138 return getContact().get(0); 1139 } 1140 1141 /** 1142 * @return {@link #copyright} (A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1143 */ 1144 public MarkdownType getCopyrightElement() { 1145 if (this.copyright == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create ServiceDefinition.copyright"); 1148 else if (Configuration.doAutoCreate()) 1149 this.copyright = new MarkdownType(); // bb 1150 return this.copyright; 1151 } 1152 1153 public boolean hasCopyrightElement() { 1154 return this.copyright != null && !this.copyright.isEmpty(); 1155 } 1156 1157 public boolean hasCopyright() { 1158 return this.copyright != null && !this.copyright.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #copyright} (A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1163 */ 1164 public ServiceDefinition setCopyrightElement(MarkdownType value) { 1165 this.copyright = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition. 1171 */ 1172 public String getCopyright() { 1173 return this.copyright == null ? null : this.copyright.getValue(); 1174 } 1175 1176 /** 1177 * @param value A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition. 1178 */ 1179 public ServiceDefinition setCopyright(String value) { 1180 if (value == null) 1181 this.copyright = null; 1182 else { 1183 if (this.copyright == null) 1184 this.copyright = new MarkdownType(); 1185 this.copyright.setValue(value); 1186 } 1187 return this; 1188 } 1189 1190 /** 1191 * @return {@link #relatedArtifact} (Related resources such as additional documentation, justification, or bibliographic references.) 1192 */ 1193 public List<RelatedArtifact> getRelatedArtifact() { 1194 if (this.relatedArtifact == null) 1195 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1196 return this.relatedArtifact; 1197 } 1198 1199 /** 1200 * @return Returns a reference to <code>this</code> for easy method chaining 1201 */ 1202 public ServiceDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1203 this.relatedArtifact = theRelatedArtifact; 1204 return this; 1205 } 1206 1207 public boolean hasRelatedArtifact() { 1208 if (this.relatedArtifact == null) 1209 return false; 1210 for (RelatedArtifact item : this.relatedArtifact) 1211 if (!item.isEmpty()) 1212 return true; 1213 return false; 1214 } 1215 1216 public RelatedArtifact addRelatedArtifact() { //3 1217 RelatedArtifact t = new RelatedArtifact(); 1218 if (this.relatedArtifact == null) 1219 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1220 this.relatedArtifact.add(t); 1221 return t; 1222 } 1223 1224 public ServiceDefinition addRelatedArtifact(RelatedArtifact t) { //3 1225 if (t == null) 1226 return this; 1227 if (this.relatedArtifact == null) 1228 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1229 this.relatedArtifact.add(t); 1230 return this; 1231 } 1232 1233 /** 1234 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 1235 */ 1236 public RelatedArtifact getRelatedArtifactFirstRep() { 1237 if (getRelatedArtifact().isEmpty()) { 1238 addRelatedArtifact(); 1239 } 1240 return getRelatedArtifact().get(0); 1241 } 1242 1243 /** 1244 * @return {@link #trigger} (The trigger element defines when the rule should be invoked. This information is used by consumers of the rule to determine how to integrate the rule into a specific workflow.) 1245 */ 1246 public List<TriggerDefinition> getTrigger() { 1247 if (this.trigger == null) 1248 this.trigger = new ArrayList<TriggerDefinition>(); 1249 return this.trigger; 1250 } 1251 1252 /** 1253 * @return Returns a reference to <code>this</code> for easy method chaining 1254 */ 1255 public ServiceDefinition setTrigger(List<TriggerDefinition> theTrigger) { 1256 this.trigger = theTrigger; 1257 return this; 1258 } 1259 1260 public boolean hasTrigger() { 1261 if (this.trigger == null) 1262 return false; 1263 for (TriggerDefinition item : this.trigger) 1264 if (!item.isEmpty()) 1265 return true; 1266 return false; 1267 } 1268 1269 public TriggerDefinition addTrigger() { //3 1270 TriggerDefinition t = new TriggerDefinition(); 1271 if (this.trigger == null) 1272 this.trigger = new ArrayList<TriggerDefinition>(); 1273 this.trigger.add(t); 1274 return t; 1275 } 1276 1277 public ServiceDefinition addTrigger(TriggerDefinition t) { //3 1278 if (t == null) 1279 return this; 1280 if (this.trigger == null) 1281 this.trigger = new ArrayList<TriggerDefinition>(); 1282 this.trigger.add(t); 1283 return this; 1284 } 1285 1286 /** 1287 * @return The first repetition of repeating field {@link #trigger}, creating it if it does not already exist 1288 */ 1289 public TriggerDefinition getTriggerFirstRep() { 1290 if (getTrigger().isEmpty()) { 1291 addTrigger(); 1292 } 1293 return getTrigger().get(0); 1294 } 1295 1296 /** 1297 * @return {@link #dataRequirement} (Data requirements are a machine processable description of the data required by the module in order to perform a successful evaluation.) 1298 */ 1299 public List<DataRequirement> getDataRequirement() { 1300 if (this.dataRequirement == null) 1301 this.dataRequirement = new ArrayList<DataRequirement>(); 1302 return this.dataRequirement; 1303 } 1304 1305 /** 1306 * @return Returns a reference to <code>this</code> for easy method chaining 1307 */ 1308 public ServiceDefinition setDataRequirement(List<DataRequirement> theDataRequirement) { 1309 this.dataRequirement = theDataRequirement; 1310 return this; 1311 } 1312 1313 public boolean hasDataRequirement() { 1314 if (this.dataRequirement == null) 1315 return false; 1316 for (DataRequirement item : this.dataRequirement) 1317 if (!item.isEmpty()) 1318 return true; 1319 return false; 1320 } 1321 1322 public DataRequirement addDataRequirement() { //3 1323 DataRequirement t = new DataRequirement(); 1324 if (this.dataRequirement == null) 1325 this.dataRequirement = new ArrayList<DataRequirement>(); 1326 this.dataRequirement.add(t); 1327 return t; 1328 } 1329 1330 public ServiceDefinition addDataRequirement(DataRequirement t) { //3 1331 if (t == null) 1332 return this; 1333 if (this.dataRequirement == null) 1334 this.dataRequirement = new ArrayList<DataRequirement>(); 1335 this.dataRequirement.add(t); 1336 return this; 1337 } 1338 1339 /** 1340 * @return The first repetition of repeating field {@link #dataRequirement}, creating it if it does not already exist 1341 */ 1342 public DataRequirement getDataRequirementFirstRep() { 1343 if (getDataRequirement().isEmpty()) { 1344 addDataRequirement(); 1345 } 1346 return getDataRequirement().get(0); 1347 } 1348 1349 /** 1350 * @return {@link #operationDefinition} (A reference to the operation that is used to invoke this service.) 1351 */ 1352 public Reference getOperationDefinition() { 1353 if (this.operationDefinition == null) 1354 if (Configuration.errorOnAutoCreate()) 1355 throw new Error("Attempt to auto-create ServiceDefinition.operationDefinition"); 1356 else if (Configuration.doAutoCreate()) 1357 this.operationDefinition = new Reference(); // cc 1358 return this.operationDefinition; 1359 } 1360 1361 public boolean hasOperationDefinition() { 1362 return this.operationDefinition != null && !this.operationDefinition.isEmpty(); 1363 } 1364 1365 /** 1366 * @param value {@link #operationDefinition} (A reference to the operation that is used to invoke this service.) 1367 */ 1368 public ServiceDefinition setOperationDefinition(Reference value) { 1369 this.operationDefinition = value; 1370 return this; 1371 } 1372 1373 /** 1374 * @return {@link #operationDefinition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the operation that is used to invoke this service.) 1375 */ 1376 public OperationDefinition getOperationDefinitionTarget() { 1377 if (this.operationDefinitionTarget == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create ServiceDefinition.operationDefinition"); 1380 else if (Configuration.doAutoCreate()) 1381 this.operationDefinitionTarget = new OperationDefinition(); // aa 1382 return this.operationDefinitionTarget; 1383 } 1384 1385 /** 1386 * @param value {@link #operationDefinition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the operation that is used to invoke this service.) 1387 */ 1388 public ServiceDefinition setOperationDefinitionTarget(OperationDefinition value) { 1389 this.operationDefinitionTarget = value; 1390 return this; 1391 } 1392 1393 protected void listChildren(List<Property> children) { 1394 super.listChildren(children); 1395 children.add(new Property("url", "uri", "An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 1396 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this service definition when it is represented in other formats, or referenced in a specification, model, design or an instance. This is used for CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1397 children.add(new Property("version", "string", "The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 1398 children.add(new Property("name", "string", "A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1399 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the service definition.", 0, 1, title)); 1400 children.add(new Property("status", "code", "The status of this service definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1401 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 1402 children.add(new Property("date", "dateTime", "The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes.", 0, 1, date)); 1403 children.add(new Property("publisher", "string", "The name of the individual or organization that published the service definition.", 0, 1, publisher)); 1404 children.add(new Property("description", "markdown", "A free text natural language description of the service definition from a consumer's perspective.", 0, 1, description)); 1405 children.add(new Property("purpose", "markdown", "Explaination of why this service definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1406 children.add(new Property("usage", "string", "A detailed description of how the module is used from a clinical perspective.", 0, 1, usage)); 1407 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 1408 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 1409 children.add(new Property("effectivePeriod", "Period", "The period during which the service definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 1410 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate service definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1411 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the service definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1412 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 1413 children.add(new Property("contributor", "Contributor", "A contributor to the content of the module, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor)); 1414 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1415 children.add(new Property("copyright", "markdown", "A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition.", 0, 1, copyright)); 1416 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related resources such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1417 children.add(new Property("trigger", "TriggerDefinition", "The trigger element defines when the rule should be invoked. This information is used by consumers of the rule to determine how to integrate the rule into a specific workflow.", 0, java.lang.Integer.MAX_VALUE, trigger)); 1418 children.add(new Property("dataRequirement", "DataRequirement", "Data requirements are a machine processable description of the data required by the module in order to perform a successful evaluation.", 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 1419 children.add(new Property("operationDefinition", "Reference(OperationDefinition)", "A reference to the operation that is used to invoke this service.", 0, 1, operationDefinition)); 1420 } 1421 1422 @Override 1423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1424 switch (_hash) { 1425 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this service definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this service definition is (or will be) published. The URL SHOULD include the major version of the service definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 1426 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this service definition when it is represented in other formats, or referenced in a specification, model, design or an instance. This is used for CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier); 1427 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the service definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the service definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 1428 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the service definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1429 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the service definition.", 0, 1, title); 1430 case -892481550: /*status*/ return new Property("status", "code", "The status of this service definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1431 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this service definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 1432 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the service definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the service definition changes.", 0, 1, date); 1433 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the service definition.", 0, 1, publisher); 1434 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the service definition from a consumer's perspective.", 0, 1, description); 1435 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this service definition is needed and why it has been designed as it has.", 0, 1, purpose); 1436 case 111574433: /*usage*/ return new Property("usage", "string", "A detailed description of how the module is used from a clinical perspective.", 0, 1, usage); 1437 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 1438 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 1439 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the service definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 1440 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate service definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1441 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the service definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1442 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 1443 case -1895276325: /*contributor*/ return new Property("contributor", "Contributor", "A contributor to the content of the module, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor); 1444 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1445 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the service definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the service definition.", 0, 1, copyright); 1446 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related resources such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 1447 case -1059891784: /*trigger*/ return new Property("trigger", "TriggerDefinition", "The trigger element defines when the rule should be invoked. This information is used by consumers of the rule to determine how to integrate the rule into a specific workflow.", 0, java.lang.Integer.MAX_VALUE, trigger); 1448 case 629147193: /*dataRequirement*/ return new Property("dataRequirement", "DataRequirement", "Data requirements are a machine processable description of the data required by the module in order to perform a successful evaluation.", 0, java.lang.Integer.MAX_VALUE, dataRequirement); 1449 case 900960794: /*operationDefinition*/ return new Property("operationDefinition", "Reference(OperationDefinition)", "A reference to the operation that is used to invoke this service.", 0, 1, operationDefinition); 1450 default: return super.getNamedProperty(_hash, _name, _checkValid); 1451 } 1452 1453 } 1454 1455 @Override 1456 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1457 switch (hash) { 1458 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1459 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1460 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1461 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1462 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1463 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1464 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1465 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1466 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1467 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1468 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1469 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 1470 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 1471 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 1472 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 1473 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1474 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1475 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 1476 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Contributor 1477 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1478 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 1479 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 1480 case -1059891784: /*trigger*/ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 1481 case 629147193: /*dataRequirement*/ return this.dataRequirement == null ? new Base[0] : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 1482 case 900960794: /*operationDefinition*/ return this.operationDefinition == null ? new Base[0] : new Base[] {this.operationDefinition}; // Reference 1483 default: return super.getProperty(hash, name, checkValid); 1484 } 1485 1486 } 1487 1488 @Override 1489 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1490 switch (hash) { 1491 case 116079: // url 1492 this.url = castToUri(value); // UriType 1493 return value; 1494 case -1618432855: // identifier 1495 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1496 return value; 1497 case 351608024: // version 1498 this.version = castToString(value); // StringType 1499 return value; 1500 case 3373707: // name 1501 this.name = castToString(value); // StringType 1502 return value; 1503 case 110371416: // title 1504 this.title = castToString(value); // StringType 1505 return value; 1506 case -892481550: // status 1507 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1508 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1509 return value; 1510 case -404562712: // experimental 1511 this.experimental = castToBoolean(value); // BooleanType 1512 return value; 1513 case 3076014: // date 1514 this.date = castToDateTime(value); // DateTimeType 1515 return value; 1516 case 1447404028: // publisher 1517 this.publisher = castToString(value); // StringType 1518 return value; 1519 case -1724546052: // description 1520 this.description = castToMarkdown(value); // MarkdownType 1521 return value; 1522 case -220463842: // purpose 1523 this.purpose = castToMarkdown(value); // MarkdownType 1524 return value; 1525 case 111574433: // usage 1526 this.usage = castToString(value); // StringType 1527 return value; 1528 case 223539345: // approvalDate 1529 this.approvalDate = castToDate(value); // DateType 1530 return value; 1531 case -1687512484: // lastReviewDate 1532 this.lastReviewDate = castToDate(value); // DateType 1533 return value; 1534 case -403934648: // effectivePeriod 1535 this.effectivePeriod = castToPeriod(value); // Period 1536 return value; 1537 case -669707736: // useContext 1538 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1539 return value; 1540 case -507075711: // jurisdiction 1541 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1542 return value; 1543 case 110546223: // topic 1544 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 1545 return value; 1546 case -1895276325: // contributor 1547 this.getContributor().add(castToContributor(value)); // Contributor 1548 return value; 1549 case 951526432: // contact 1550 this.getContact().add(castToContactDetail(value)); // ContactDetail 1551 return value; 1552 case 1522889671: // copyright 1553 this.copyright = castToMarkdown(value); // MarkdownType 1554 return value; 1555 case 666807069: // relatedArtifact 1556 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 1557 return value; 1558 case -1059891784: // trigger 1559 this.getTrigger().add(castToTriggerDefinition(value)); // TriggerDefinition 1560 return value; 1561 case 629147193: // dataRequirement 1562 this.getDataRequirement().add(castToDataRequirement(value)); // DataRequirement 1563 return value; 1564 case 900960794: // operationDefinition 1565 this.operationDefinition = castToReference(value); // Reference 1566 return value; 1567 default: return super.setProperty(hash, name, value); 1568 } 1569 1570 } 1571 1572 @Override 1573 public Base setProperty(String name, Base value) throws FHIRException { 1574 if (name.equals("url")) { 1575 this.url = castToUri(value); // UriType 1576 } else if (name.equals("identifier")) { 1577 this.getIdentifier().add(castToIdentifier(value)); 1578 } else if (name.equals("version")) { 1579 this.version = castToString(value); // StringType 1580 } else if (name.equals("name")) { 1581 this.name = castToString(value); // StringType 1582 } else if (name.equals("title")) { 1583 this.title = castToString(value); // StringType 1584 } else if (name.equals("status")) { 1585 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1586 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1587 } else if (name.equals("experimental")) { 1588 this.experimental = castToBoolean(value); // BooleanType 1589 } else if (name.equals("date")) { 1590 this.date = castToDateTime(value); // DateTimeType 1591 } else if (name.equals("publisher")) { 1592 this.publisher = castToString(value); // StringType 1593 } else if (name.equals("description")) { 1594 this.description = castToMarkdown(value); // MarkdownType 1595 } else if (name.equals("purpose")) { 1596 this.purpose = castToMarkdown(value); // MarkdownType 1597 } else if (name.equals("usage")) { 1598 this.usage = castToString(value); // StringType 1599 } else if (name.equals("approvalDate")) { 1600 this.approvalDate = castToDate(value); // DateType 1601 } else if (name.equals("lastReviewDate")) { 1602 this.lastReviewDate = castToDate(value); // DateType 1603 } else if (name.equals("effectivePeriod")) { 1604 this.effectivePeriod = castToPeriod(value); // Period 1605 } else if (name.equals("useContext")) { 1606 this.getUseContext().add(castToUsageContext(value)); 1607 } else if (name.equals("jurisdiction")) { 1608 this.getJurisdiction().add(castToCodeableConcept(value)); 1609 } else if (name.equals("topic")) { 1610 this.getTopic().add(castToCodeableConcept(value)); 1611 } else if (name.equals("contributor")) { 1612 this.getContributor().add(castToContributor(value)); 1613 } else if (name.equals("contact")) { 1614 this.getContact().add(castToContactDetail(value)); 1615 } else if (name.equals("copyright")) { 1616 this.copyright = castToMarkdown(value); // MarkdownType 1617 } else if (name.equals("relatedArtifact")) { 1618 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 1619 } else if (name.equals("trigger")) { 1620 this.getTrigger().add(castToTriggerDefinition(value)); 1621 } else if (name.equals("dataRequirement")) { 1622 this.getDataRequirement().add(castToDataRequirement(value)); 1623 } else if (name.equals("operationDefinition")) { 1624 this.operationDefinition = castToReference(value); // Reference 1625 } else 1626 return super.setProperty(name, value); 1627 return value; 1628 } 1629 1630 @Override 1631 public Base makeProperty(int hash, String name) throws FHIRException { 1632 switch (hash) { 1633 case 116079: return getUrlElement(); 1634 case -1618432855: return addIdentifier(); 1635 case 351608024: return getVersionElement(); 1636 case 3373707: return getNameElement(); 1637 case 110371416: return getTitleElement(); 1638 case -892481550: return getStatusElement(); 1639 case -404562712: return getExperimentalElement(); 1640 case 3076014: return getDateElement(); 1641 case 1447404028: return getPublisherElement(); 1642 case -1724546052: return getDescriptionElement(); 1643 case -220463842: return getPurposeElement(); 1644 case 111574433: return getUsageElement(); 1645 case 223539345: return getApprovalDateElement(); 1646 case -1687512484: return getLastReviewDateElement(); 1647 case -403934648: return getEffectivePeriod(); 1648 case -669707736: return addUseContext(); 1649 case -507075711: return addJurisdiction(); 1650 case 110546223: return addTopic(); 1651 case -1895276325: return addContributor(); 1652 case 951526432: return addContact(); 1653 case 1522889671: return getCopyrightElement(); 1654 case 666807069: return addRelatedArtifact(); 1655 case -1059891784: return addTrigger(); 1656 case 629147193: return addDataRequirement(); 1657 case 900960794: return getOperationDefinition(); 1658 default: return super.makeProperty(hash, name); 1659 } 1660 1661 } 1662 1663 @Override 1664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1665 switch (hash) { 1666 case 116079: /*url*/ return new String[] {"uri"}; 1667 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1668 case 351608024: /*version*/ return new String[] {"string"}; 1669 case 3373707: /*name*/ return new String[] {"string"}; 1670 case 110371416: /*title*/ return new String[] {"string"}; 1671 case -892481550: /*status*/ return new String[] {"code"}; 1672 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1673 case 3076014: /*date*/ return new String[] {"dateTime"}; 1674 case 1447404028: /*publisher*/ return new String[] {"string"}; 1675 case -1724546052: /*description*/ return new String[] {"markdown"}; 1676 case -220463842: /*purpose*/ return new String[] {"markdown"}; 1677 case 111574433: /*usage*/ return new String[] {"string"}; 1678 case 223539345: /*approvalDate*/ return new String[] {"date"}; 1679 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 1680 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 1681 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1682 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1683 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 1684 case -1895276325: /*contributor*/ return new String[] {"Contributor"}; 1685 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1686 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 1687 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 1688 case -1059891784: /*trigger*/ return new String[] {"TriggerDefinition"}; 1689 case 629147193: /*dataRequirement*/ return new String[] {"DataRequirement"}; 1690 case 900960794: /*operationDefinition*/ return new String[] {"Reference"}; 1691 default: return super.getTypesForProperty(hash, name); 1692 } 1693 1694 } 1695 1696 @Override 1697 public Base addChild(String name) throws FHIRException { 1698 if (name.equals("url")) { 1699 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.url"); 1700 } 1701 else if (name.equals("identifier")) { 1702 return addIdentifier(); 1703 } 1704 else if (name.equals("version")) { 1705 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.version"); 1706 } 1707 else if (name.equals("name")) { 1708 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.name"); 1709 } 1710 else if (name.equals("title")) { 1711 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.title"); 1712 } 1713 else if (name.equals("status")) { 1714 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.status"); 1715 } 1716 else if (name.equals("experimental")) { 1717 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.experimental"); 1718 } 1719 else if (name.equals("date")) { 1720 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.date"); 1721 } 1722 else if (name.equals("publisher")) { 1723 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.publisher"); 1724 } 1725 else if (name.equals("description")) { 1726 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.description"); 1727 } 1728 else if (name.equals("purpose")) { 1729 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.purpose"); 1730 } 1731 else if (name.equals("usage")) { 1732 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.usage"); 1733 } 1734 else if (name.equals("approvalDate")) { 1735 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.approvalDate"); 1736 } 1737 else if (name.equals("lastReviewDate")) { 1738 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.lastReviewDate"); 1739 } 1740 else if (name.equals("effectivePeriod")) { 1741 this.effectivePeriod = new Period(); 1742 return this.effectivePeriod; 1743 } 1744 else if (name.equals("useContext")) { 1745 return addUseContext(); 1746 } 1747 else if (name.equals("jurisdiction")) { 1748 return addJurisdiction(); 1749 } 1750 else if (name.equals("topic")) { 1751 return addTopic(); 1752 } 1753 else if (name.equals("contributor")) { 1754 return addContributor(); 1755 } 1756 else if (name.equals("contact")) { 1757 return addContact(); 1758 } 1759 else if (name.equals("copyright")) { 1760 throw new FHIRException("Cannot call addChild on a singleton property ServiceDefinition.copyright"); 1761 } 1762 else if (name.equals("relatedArtifact")) { 1763 return addRelatedArtifact(); 1764 } 1765 else if (name.equals("trigger")) { 1766 return addTrigger(); 1767 } 1768 else if (name.equals("dataRequirement")) { 1769 return addDataRequirement(); 1770 } 1771 else if (name.equals("operationDefinition")) { 1772 this.operationDefinition = new Reference(); 1773 return this.operationDefinition; 1774 } 1775 else 1776 return super.addChild(name); 1777 } 1778 1779 public String fhirType() { 1780 return "ServiceDefinition"; 1781 1782 } 1783 1784 public ServiceDefinition copy() { 1785 ServiceDefinition dst = new ServiceDefinition(); 1786 copyValues(dst); 1787 dst.url = url == null ? null : url.copy(); 1788 if (identifier != null) { 1789 dst.identifier = new ArrayList<Identifier>(); 1790 for (Identifier i : identifier) 1791 dst.identifier.add(i.copy()); 1792 }; 1793 dst.version = version == null ? null : version.copy(); 1794 dst.name = name == null ? null : name.copy(); 1795 dst.title = title == null ? null : title.copy(); 1796 dst.status = status == null ? null : status.copy(); 1797 dst.experimental = experimental == null ? null : experimental.copy(); 1798 dst.date = date == null ? null : date.copy(); 1799 dst.publisher = publisher == null ? null : publisher.copy(); 1800 dst.description = description == null ? null : description.copy(); 1801 dst.purpose = purpose == null ? null : purpose.copy(); 1802 dst.usage = usage == null ? null : usage.copy(); 1803 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 1804 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 1805 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 1806 if (useContext != null) { 1807 dst.useContext = new ArrayList<UsageContext>(); 1808 for (UsageContext i : useContext) 1809 dst.useContext.add(i.copy()); 1810 }; 1811 if (jurisdiction != null) { 1812 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1813 for (CodeableConcept i : jurisdiction) 1814 dst.jurisdiction.add(i.copy()); 1815 }; 1816 if (topic != null) { 1817 dst.topic = new ArrayList<CodeableConcept>(); 1818 for (CodeableConcept i : topic) 1819 dst.topic.add(i.copy()); 1820 }; 1821 if (contributor != null) { 1822 dst.contributor = new ArrayList<Contributor>(); 1823 for (Contributor i : contributor) 1824 dst.contributor.add(i.copy()); 1825 }; 1826 if (contact != null) { 1827 dst.contact = new ArrayList<ContactDetail>(); 1828 for (ContactDetail i : contact) 1829 dst.contact.add(i.copy()); 1830 }; 1831 dst.copyright = copyright == null ? null : copyright.copy(); 1832 if (relatedArtifact != null) { 1833 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 1834 for (RelatedArtifact i : relatedArtifact) 1835 dst.relatedArtifact.add(i.copy()); 1836 }; 1837 if (trigger != null) { 1838 dst.trigger = new ArrayList<TriggerDefinition>(); 1839 for (TriggerDefinition i : trigger) 1840 dst.trigger.add(i.copy()); 1841 }; 1842 if (dataRequirement != null) { 1843 dst.dataRequirement = new ArrayList<DataRequirement>(); 1844 for (DataRequirement i : dataRequirement) 1845 dst.dataRequirement.add(i.copy()); 1846 }; 1847 dst.operationDefinition = operationDefinition == null ? null : operationDefinition.copy(); 1848 return dst; 1849 } 1850 1851 protected ServiceDefinition typedCopy() { 1852 return copy(); 1853 } 1854 1855 @Override 1856 public boolean equalsDeep(Base other_) { 1857 if (!super.equalsDeep(other_)) 1858 return false; 1859 if (!(other_ instanceof ServiceDefinition)) 1860 return false; 1861 ServiceDefinition o = (ServiceDefinition) other_; 1862 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 1863 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 1864 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(contributor, o.contributor, true) 1865 && compareDeep(copyright, o.copyright, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 1866 && compareDeep(trigger, o.trigger, true) && compareDeep(dataRequirement, o.dataRequirement, true) 1867 && compareDeep(operationDefinition, o.operationDefinition, true); 1868 } 1869 1870 @Override 1871 public boolean equalsShallow(Base other_) { 1872 if (!super.equalsShallow(other_)) 1873 return false; 1874 if (!(other_ instanceof ServiceDefinition)) 1875 return false; 1876 ServiceDefinition o = (ServiceDefinition) other_; 1877 return compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) && compareValues(approvalDate, o.approvalDate, true) 1878 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 1879 ; 1880 } 1881 1882 public boolean isEmpty() { 1883 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, usage 1884 , approvalDate, lastReviewDate, effectivePeriod, topic, contributor, copyright, relatedArtifact 1885 , trigger, dataRequirement, operationDefinition); 1886 } 1887 1888 @Override 1889 public ResourceType getResourceType() { 1890 return ResourceType.ServiceDefinition; 1891 } 1892 1893 /** 1894 * Search parameter: <b>date</b> 1895 * <p> 1896 * Description: <b>The service definition publication date</b><br> 1897 * Type: <b>date</b><br> 1898 * Path: <b>ServiceDefinition.date</b><br> 1899 * </p> 1900 */ 1901 @SearchParamDefinition(name="date", path="ServiceDefinition.date", description="The service definition publication date", type="date" ) 1902 public static final String SP_DATE = "date"; 1903 /** 1904 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1905 * <p> 1906 * Description: <b>The service definition publication date</b><br> 1907 * Type: <b>date</b><br> 1908 * Path: <b>ServiceDefinition.date</b><br> 1909 * </p> 1910 */ 1911 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1912 1913 /** 1914 * Search parameter: <b>identifier</b> 1915 * <p> 1916 * Description: <b>External identifier for the service definition</b><br> 1917 * Type: <b>token</b><br> 1918 * Path: <b>ServiceDefinition.identifier</b><br> 1919 * </p> 1920 */ 1921 @SearchParamDefinition(name="identifier", path="ServiceDefinition.identifier", description="External identifier for the service definition", type="token" ) 1922 public static final String SP_IDENTIFIER = "identifier"; 1923 /** 1924 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1925 * <p> 1926 * Description: <b>External identifier for the service definition</b><br> 1927 * Type: <b>token</b><br> 1928 * Path: <b>ServiceDefinition.identifier</b><br> 1929 * </p> 1930 */ 1931 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1932 1933 /** 1934 * Search parameter: <b>successor</b> 1935 * <p> 1936 * Description: <b>What resource is being referenced</b><br> 1937 * Type: <b>reference</b><br> 1938 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 1939 * </p> 1940 */ 1941 @SearchParamDefinition(name="successor", path="ServiceDefinition.relatedArtifact.where(type='successor').resource", description="What resource is being referenced", type="reference" ) 1942 public static final String SP_SUCCESSOR = "successor"; 1943 /** 1944 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 1945 * <p> 1946 * Description: <b>What resource is being referenced</b><br> 1947 * Type: <b>reference</b><br> 1948 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 1949 * </p> 1950 */ 1951 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 1952 1953/** 1954 * Constant for fluent queries to be used to add include statements. Specifies 1955 * the path value of "<b>ServiceDefinition:successor</b>". 1956 */ 1957 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("ServiceDefinition:successor").toLocked(); 1958 1959 /** 1960 * Search parameter: <b>jurisdiction</b> 1961 * <p> 1962 * Description: <b>Intended jurisdiction for the service definition</b><br> 1963 * Type: <b>token</b><br> 1964 * Path: <b>ServiceDefinition.jurisdiction</b><br> 1965 * </p> 1966 */ 1967 @SearchParamDefinition(name="jurisdiction", path="ServiceDefinition.jurisdiction", description="Intended jurisdiction for the service definition", type="token" ) 1968 public static final String SP_JURISDICTION = "jurisdiction"; 1969 /** 1970 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 1971 * <p> 1972 * Description: <b>Intended jurisdiction for the service definition</b><br> 1973 * Type: <b>token</b><br> 1974 * Path: <b>ServiceDefinition.jurisdiction</b><br> 1975 * </p> 1976 */ 1977 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 1978 1979 /** 1980 * Search parameter: <b>description</b> 1981 * <p> 1982 * Description: <b>The description of the service definition</b><br> 1983 * Type: <b>string</b><br> 1984 * Path: <b>ServiceDefinition.description</b><br> 1985 * </p> 1986 */ 1987 @SearchParamDefinition(name="description", path="ServiceDefinition.description", description="The description of the service definition", type="string" ) 1988 public static final String SP_DESCRIPTION = "description"; 1989 /** 1990 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1991 * <p> 1992 * Description: <b>The description of the service definition</b><br> 1993 * Type: <b>string</b><br> 1994 * Path: <b>ServiceDefinition.description</b><br> 1995 * </p> 1996 */ 1997 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 1998 1999 /** 2000 * Search parameter: <b>derived-from</b> 2001 * <p> 2002 * Description: <b>What resource is being referenced</b><br> 2003 * Type: <b>reference</b><br> 2004 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2005 * </p> 2006 */ 2007 @SearchParamDefinition(name="derived-from", path="ServiceDefinition.relatedArtifact.where(type='derived-from').resource", description="What resource is being referenced", type="reference" ) 2008 public static final String SP_DERIVED_FROM = "derived-from"; 2009 /** 2010 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2011 * <p> 2012 * Description: <b>What resource is being referenced</b><br> 2013 * Type: <b>reference</b><br> 2014 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2015 * </p> 2016 */ 2017 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 2018 2019/** 2020 * Constant for fluent queries to be used to add include statements. Specifies 2021 * the path value of "<b>ServiceDefinition:derived-from</b>". 2022 */ 2023 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ServiceDefinition:derived-from").toLocked(); 2024 2025 /** 2026 * Search parameter: <b>predecessor</b> 2027 * <p> 2028 * Description: <b>What resource is being referenced</b><br> 2029 * Type: <b>reference</b><br> 2030 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2031 * </p> 2032 */ 2033 @SearchParamDefinition(name="predecessor", path="ServiceDefinition.relatedArtifact.where(type='predecessor').resource", description="What resource is being referenced", type="reference" ) 2034 public static final String SP_PREDECESSOR = "predecessor"; 2035 /** 2036 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 2037 * <p> 2038 * Description: <b>What resource is being referenced</b><br> 2039 * Type: <b>reference</b><br> 2040 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2041 * </p> 2042 */ 2043 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 2044 2045/** 2046 * Constant for fluent queries to be used to add include statements. Specifies 2047 * the path value of "<b>ServiceDefinition:predecessor</b>". 2048 */ 2049 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("ServiceDefinition:predecessor").toLocked(); 2050 2051 /** 2052 * Search parameter: <b>title</b> 2053 * <p> 2054 * Description: <b>The human-friendly name of the service definition</b><br> 2055 * Type: <b>string</b><br> 2056 * Path: <b>ServiceDefinition.title</b><br> 2057 * </p> 2058 */ 2059 @SearchParamDefinition(name="title", path="ServiceDefinition.title", description="The human-friendly name of the service definition", type="string" ) 2060 public static final String SP_TITLE = "title"; 2061 /** 2062 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2063 * <p> 2064 * Description: <b>The human-friendly name of the service definition</b><br> 2065 * Type: <b>string</b><br> 2066 * Path: <b>ServiceDefinition.title</b><br> 2067 * </p> 2068 */ 2069 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2070 2071 /** 2072 * Search parameter: <b>composed-of</b> 2073 * <p> 2074 * Description: <b>What resource is being referenced</b><br> 2075 * Type: <b>reference</b><br> 2076 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2077 * </p> 2078 */ 2079 @SearchParamDefinition(name="composed-of", path="ServiceDefinition.relatedArtifact.where(type='composed-of').resource", description="What resource is being referenced", type="reference" ) 2080 public static final String SP_COMPOSED_OF = "composed-of"; 2081 /** 2082 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 2083 * <p> 2084 * Description: <b>What resource is being referenced</b><br> 2085 * Type: <b>reference</b><br> 2086 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2087 * </p> 2088 */ 2089 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 2090 2091/** 2092 * Constant for fluent queries to be used to add include statements. Specifies 2093 * the path value of "<b>ServiceDefinition:composed-of</b>". 2094 */ 2095 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("ServiceDefinition:composed-of").toLocked(); 2096 2097 /** 2098 * Search parameter: <b>version</b> 2099 * <p> 2100 * Description: <b>The business version of the service definition</b><br> 2101 * Type: <b>token</b><br> 2102 * Path: <b>ServiceDefinition.version</b><br> 2103 * </p> 2104 */ 2105 @SearchParamDefinition(name="version", path="ServiceDefinition.version", description="The business version of the service definition", type="token" ) 2106 public static final String SP_VERSION = "version"; 2107 /** 2108 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2109 * <p> 2110 * Description: <b>The business version of the service definition</b><br> 2111 * Type: <b>token</b><br> 2112 * Path: <b>ServiceDefinition.version</b><br> 2113 * </p> 2114 */ 2115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2116 2117 /** 2118 * Search parameter: <b>url</b> 2119 * <p> 2120 * Description: <b>The uri that identifies the service definition</b><br> 2121 * Type: <b>uri</b><br> 2122 * Path: <b>ServiceDefinition.url</b><br> 2123 * </p> 2124 */ 2125 @SearchParamDefinition(name="url", path="ServiceDefinition.url", description="The uri that identifies the service definition", type="uri" ) 2126 public static final String SP_URL = "url"; 2127 /** 2128 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2129 * <p> 2130 * Description: <b>The uri that identifies the service definition</b><br> 2131 * Type: <b>uri</b><br> 2132 * Path: <b>ServiceDefinition.url</b><br> 2133 * </p> 2134 */ 2135 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2136 2137 /** 2138 * Search parameter: <b>effective</b> 2139 * <p> 2140 * Description: <b>The time during which the service definition is intended to be in use</b><br> 2141 * Type: <b>date</b><br> 2142 * Path: <b>ServiceDefinition.effectivePeriod</b><br> 2143 * </p> 2144 */ 2145 @SearchParamDefinition(name="effective", path="ServiceDefinition.effectivePeriod", description="The time during which the service definition is intended to be in use", type="date" ) 2146 public static final String SP_EFFECTIVE = "effective"; 2147 /** 2148 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 2149 * <p> 2150 * Description: <b>The time during which the service definition is intended to be in use</b><br> 2151 * Type: <b>date</b><br> 2152 * Path: <b>ServiceDefinition.effectivePeriod</b><br> 2153 * </p> 2154 */ 2155 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 2156 2157 /** 2158 * Search parameter: <b>depends-on</b> 2159 * <p> 2160 * Description: <b>What resource is being referenced</b><br> 2161 * Type: <b>reference</b><br> 2162 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2163 * </p> 2164 */ 2165 @SearchParamDefinition(name="depends-on", path="ServiceDefinition.relatedArtifact.where(type='depends-on').resource", description="What resource is being referenced", type="reference" ) 2166 public static final String SP_DEPENDS_ON = "depends-on"; 2167 /** 2168 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 2169 * <p> 2170 * Description: <b>What resource is being referenced</b><br> 2171 * Type: <b>reference</b><br> 2172 * Path: <b>ServiceDefinition.relatedArtifact.resource</b><br> 2173 * </p> 2174 */ 2175 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 2176 2177/** 2178 * Constant for fluent queries to be used to add include statements. Specifies 2179 * the path value of "<b>ServiceDefinition:depends-on</b>". 2180 */ 2181 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("ServiceDefinition:depends-on").toLocked(); 2182 2183 /** 2184 * Search parameter: <b>name</b> 2185 * <p> 2186 * Description: <b>Computationally friendly name of the service definition</b><br> 2187 * Type: <b>string</b><br> 2188 * Path: <b>ServiceDefinition.name</b><br> 2189 * </p> 2190 */ 2191 @SearchParamDefinition(name="name", path="ServiceDefinition.name", description="Computationally friendly name of the service definition", type="string" ) 2192 public static final String SP_NAME = "name"; 2193 /** 2194 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2195 * <p> 2196 * Description: <b>Computationally friendly name of the service definition</b><br> 2197 * Type: <b>string</b><br> 2198 * Path: <b>ServiceDefinition.name</b><br> 2199 * </p> 2200 */ 2201 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2202 2203 /** 2204 * Search parameter: <b>publisher</b> 2205 * <p> 2206 * Description: <b>Name of the publisher of the service definition</b><br> 2207 * Type: <b>string</b><br> 2208 * Path: <b>ServiceDefinition.publisher</b><br> 2209 * </p> 2210 */ 2211 @SearchParamDefinition(name="publisher", path="ServiceDefinition.publisher", description="Name of the publisher of the service definition", type="string" ) 2212 public static final String SP_PUBLISHER = "publisher"; 2213 /** 2214 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2215 * <p> 2216 * Description: <b>Name of the publisher of the service definition</b><br> 2217 * Type: <b>string</b><br> 2218 * Path: <b>ServiceDefinition.publisher</b><br> 2219 * </p> 2220 */ 2221 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2222 2223 /** 2224 * Search parameter: <b>topic</b> 2225 * <p> 2226 * Description: <b>Topics associated with the module</b><br> 2227 * Type: <b>token</b><br> 2228 * Path: <b>ServiceDefinition.topic</b><br> 2229 * </p> 2230 */ 2231 @SearchParamDefinition(name="topic", path="ServiceDefinition.topic", description="Topics associated with the module", type="token" ) 2232 public static final String SP_TOPIC = "topic"; 2233 /** 2234 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 2235 * <p> 2236 * Description: <b>Topics associated with the module</b><br> 2237 * Type: <b>token</b><br> 2238 * Path: <b>ServiceDefinition.topic</b><br> 2239 * </p> 2240 */ 2241 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 2242 2243 /** 2244 * Search parameter: <b>status</b> 2245 * <p> 2246 * Description: <b>The current status of the service definition</b><br> 2247 * Type: <b>token</b><br> 2248 * Path: <b>ServiceDefinition.status</b><br> 2249 * </p> 2250 */ 2251 @SearchParamDefinition(name="status", path="ServiceDefinition.status", description="The current status of the service definition", type="token" ) 2252 public static final String SP_STATUS = "status"; 2253 /** 2254 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2255 * <p> 2256 * Description: <b>The current status of the service definition</b><br> 2257 * Type: <b>token</b><br> 2258 * Path: <b>ServiceDefinition.status</b><br> 2259 * </p> 2260 */ 2261 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2262 2263 2264}